Spaces:
Sleeping
Sleeping
import streamlit as st | |
import requests | |
from langchain_groq import ChatGroq | |
from streamlit_chat import message | |
import plotly.express as px | |
import pandas as pd | |
import sqlite3 | |
from datetime import datetime, timedelta | |
import re | |
import os | |
from streamlit_option_menu import option_menu | |
import fitz # PyMuPDF | |
from bs4 import BeautifulSoup | |
GROQ_API_KEY = st.secrets["GROQ_API_KEY"] | |
RAPIDAPI_KEY = st.secrets["RAPIDAPI_KEY"] | |
YOUTUBE_API_KEY = st.secrets["YOUTUBE_API_KEY"] | |
THE_MUSE_API_KEY = st.secrets.get("THE_MUSE_API_KEY", "") | |
BLS_API_KEY = st.secrets.get("BLS_API_KEY", "") | |
llm = ChatGroq( | |
temperature=0, | |
groq_api_key=GROQ_API_KEY, | |
model_name="llama-3.1-70b-versatile" | |
) | |
def extract_text_from_pdf(pdf_file): | |
""" | |
Extracts text from an uploaded PDF file. | |
""" | |
text = "" | |
try: | |
with fitz.open(stream=pdf_file.read(), filetype="pdf") as doc: | |
for page in doc: | |
text += page.get_text() | |
return text | |
except Exception as e: | |
st.error(f"Error extracting text from PDF: {e}") | |
return "" | |
def extract_job_description(job_link): | |
""" | |
Fetches and extracts job description text from a given URL. | |
""" | |
try: | |
headers = { | |
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64)" | |
} | |
response = requests.get(job_link, headers=headers) | |
response.raise_for_status() | |
soup = BeautifulSoup(response.text, 'html.parser') | |
# You might need to adjust the selectors based on the website's structure | |
job_description = soup.get_text(separator='\n') | |
return job_description.strip() | |
except Exception as e: | |
st.error(f"Error fetching job description: {e}") | |
return "" | |
def extract_requirements(job_description): | |
""" | |
Uses Groq to extract job requirements from the job description. | |
""" | |
prompt = f""" | |
The following is a job description: | |
{job_description} | |
Extract the list of job requirements, qualifications, and skills from the job description. Provide them as a numbered list. | |
Requirements: | |
""" | |
try: | |
response = llm.invoke(prompt) | |
requirements = response.content.strip() | |
return requirements | |
except Exception as e: | |
st.error(f"Error extracting requirements: {e}") | |
return "" | |
def generate_email(job_description, requirements, resume_text): | |
""" | |
Generates a personalized cold email using Groq based on the job description, requirements, and resume. | |
""" | |
prompt = f""" | |
You are Adithya S Nair, a recent Computer Science graduate specializing in Artificial Intelligence and Machine Learning. Craft a concise and professional cold email to a potential employer based on the following information: | |
**Job Description:** | |
{job_description} | |
**Extracted Requirements:** | |
{requirements} | |
**Your Resume:** | |
{resume_text} | |
**Email Requirements:** | |
- **Introduction:** Briefly introduce yourself and mention the specific job you are applying for. | |
- **Body:** Highlight your relevant skills, projects, internships, and leadership experiences that align with the job requirements. | |
- **Value Proposition:** Explain how your fresh perspective and recent academic knowledge can add value to the company. | |
- **Closing:** Express enthusiasm for the opportunity, mention your willingness for an interview, and thank the recipient for their time. | |
""" | |
try: | |
response = llm.invoke(prompt) | |
email_text = response.content.strip() | |
return email_text | |
except Exception as e: | |
st.error(f"Error generating email: {e}") | |
return "" | |
def generate_cover_letter(job_description, requirements, resume_text): | |
""" | |
Generates a personalized cover letter using Groq based on the job description, requirements, and resume. | |
""" | |
prompt = f""" | |
You are Adithya S Nair, a recent Computer Science graduate specializing in Artificial Intelligence and Machine Learning. Compose a personalized and professional cover letter based on the following information: | |
**Job Description:** | |
{job_description} | |
**Extracted Requirements:** | |
{requirements} | |
**Your Resume:** | |
{resume_text} | |
**Cover Letter Requirements:** | |
1. **Greeting:** Address the hiring manager by name if available; otherwise, use a generic greeting such as "Dear Hiring Manager." | |
2. **Introduction:** Begin with an engaging opening that mentions the specific position you are applying for and conveys your enthusiasm. | |
3. **Body:** | |
- **Skills and Experiences:** Highlight relevant technical skills, projects, internships, and leadership roles that align with the job requirements. | |
- **Alignment:** Demonstrate how your academic background and hands-on experiences make you a suitable candidate for the role. | |
4. **Value Proposition:** Explain how your fresh perspective, recent academic knowledge, and eagerness to learn can contribute to the company's success. | |
5. **Conclusion:** End with a strong closing statement expressing your interest in an interview, your availability, and gratitude for the hiring manager’s time and consideration. | |
6. **Professional Tone:** Maintain a respectful and professional tone throughout the letter. | |
""" | |
try: | |
response = llm.invoke(prompt) | |
cover_letter = response.content.strip() | |
return cover_letter | |
except Exception as e: | |
st.error(f"Error generating cover letter: {e}") | |
return "" | |
def extract_skills(text): | |
""" | |
Extracts a list of skills from the resume text using Groq. | |
""" | |
prompt = f""" | |
Extract a comprehensive list of technical and soft skills from the following resume text. Provide the skills as a comma-separated list. | |
Resume Text: | |
{text} | |
Skills: | |
""" | |
try: | |
response = llm.invoke(prompt) | |
skills = response.content.strip() | |
# Clean and split the skills | |
skills_list = [skill.strip() for skill in re.split(',|\n', skills) if skill.strip()] | |
return skills_list | |
except Exception as e: | |
st.error(f"Error extracting skills: {e}") | |
return [] | |
def suggest_keywords(resume_text, job_description=None): | |
""" | |
Suggests additional relevant keywords to enhance resume compatibility with ATS. | |
""" | |
prompt = f""" | |
Analyze the following resume text and suggest additional relevant keywords that can enhance its compatibility with Applicant Tracking Systems (ATS). If a job description is provided, tailor the keywords to align with the job requirements. | |
Resume Text: | |
{resume_text} | |
Job Description: | |
{job_description if job_description else "N/A"} | |
Suggested Keywords: | |
""" | |
try: | |
response = llm.invoke(prompt) | |
keywords = response.content.strip() | |
keywords_list = [keyword.strip() for keyword in re.split(',|\n', keywords) if keyword.strip()] | |
return keywords_list | |
except Exception as e: | |
st.error(f"Error suggesting keywords: {e}") | |
return [] | |
def create_skill_distribution_chart(skills): | |
""" | |
Creates a bar chart showing the distribution of skills. | |
""" | |
skill_counts = {} | |
for skill in skills: | |
skill_counts[skill] = skill_counts.get(skill, 0) + 1 | |
df = pd.DataFrame(list(skill_counts.items()), columns=['Skill', 'Count']) | |
fig = px.bar(df, x='Skill', y='Count', title='Skill Distribution') | |
return fig | |
def create_experience_timeline(resume_text): | |
""" | |
Creates an experience timeline from the resume text. | |
""" | |
# Extract work experience details using Groq | |
prompt = f""" | |
From the following resume text, extract the job titles, companies, and durations of employment. Provide the information in a table format with columns: Job Title, Company, Duration (in years). | |
Resume Text: | |
{resume_text} | |
Table: | |
""" | |
try: | |
response = llm.invoke(prompt) | |
table_text = response.content.strip() | |
# Parse the table_text to create a DataFrame | |
data = [] | |
for line in table_text.split('\n'): | |
if line.strip() and not line.lower().startswith("job title"): | |
parts = line.split('|') | |
if len(parts) == 3: | |
job_title = parts[0].strip() | |
company = parts[1].strip() | |
duration = parts[2].strip() | |
# Convert duration to a float representing years | |
duration_years = parse_duration(duration) | |
data.append({"Job Title": job_title, "Company": company, "Duration (years)": duration_years}) | |
df = pd.DataFrame(data) | |
if not df.empty: | |
# Create a cumulative duration for timeline | |
df['Start Year'] = df['Duration (years)'].cumsum() - df['Duration (years)'] | |
df['End Year'] = df['Duration (years)'].cumsum() | |
fig = px.timeline(df, x_start="Start Year", x_end="End Year", y="Job Title", color="Company", title="Experience Timeline") | |
fig.update_yaxes(categoryorder="total ascending") | |
return fig | |
else: | |
return None | |
except Exception as e: | |
st.error(f"Error creating experience timeline: {e}") | |
return None | |
def parse_duration(duration_str): | |
""" | |
Parses duration strings like '2 years' or '6 months' into float years. | |
""" | |
try: | |
if 'year' in duration_str.lower(): | |
years = float(re.findall(r'\d+\.?\d*', duration_str)[0]) | |
return years | |
elif 'month' in duration_str.lower(): | |
months = float(re.findall(r'\d+\.?\d*', duration_str)[0]) | |
return months / 12 | |
else: | |
return 0 | |
except: | |
return 0 | |
# ------------------------------- | |
# API Integration Functions | |
# ------------------------------- | |
# Remotive API Integration | |
# Cache results for 1 day | |
def fetch_remotive_jobs_api(job_title, location=None, category=None, remote=True, max_results=50): | |
""" | |
Fetches job listings from Remotive API based on user preferences. | |
Args: | |
job_title (str): The job title to search for. | |
location (str, optional): The job location. Defaults to None. | |
category (str, optional): The job category. Defaults to None. | |
remote (bool, optional): Whether to fetch remote jobs. Defaults to True. | |
max_results (int, optional): Maximum number of jobs to fetch. Defaults to 50. | |
Returns: | |
list: A list of job dictionaries. | |
""" | |
base_url = "https://remotive.com/api/remote-jobs" | |
params = { | |
"search": job_title, | |
"limit": max_results | |
} | |
if category: | |
params["category"] = category | |
try: | |
response = requests.get(base_url, params=params) | |
response.raise_for_status() | |
jobs = response.json().get("jobs", []) | |
if remote: | |
# Filter for remote jobs if not already | |
jobs = [job for job in jobs if job.get("candidate_required_location") == "Worldwide" or job.get("remote") == True] | |
return jobs | |
except requests.exceptions.RequestException as e: | |
st.error(f"Error fetching jobs from Remotive: {e}") | |
return [] | |
# The Muse API Integration | |
# Cache results for 1 day | |
def fetch_muse_jobs_api(job_title, location=None, category=None, max_results=50): | |
""" | |
Fetches job listings from The Muse API based on user preferences. | |
Args: | |
job_title (str): The job title to search for. | |
location (str, optional): The job location. Defaults to None. | |
category (str, optional): The job category. Defaults to None. | |
max_results (int, optional): Maximum number of jobs to fetch. Defaults to 50. | |
Returns: | |
list: A list of job dictionaries. | |
""" | |
base_url = "https://www.themuse.com/api/public/jobs" | |
headers = { | |
"Content-Type": "application/json" | |
} | |
params = { | |
"page": 1, | |
"per_page": max_results, | |
"category": category, | |
"location": location, | |
"company": None # Can be extended based on needs | |
} | |
try: | |
response = requests.get(base_url, params=params, headers=headers) | |
response.raise_for_status() | |
jobs = response.json().get("results", []) | |
# Filter based on job title | |
filtered_jobs = [job for job in jobs if job_title.lower() in job.get("name", "").lower()] | |
return filtered_jobs | |
except requests.exceptions.RequestException as e: | |
st.error(f"Error fetching jobs from The Muse: {e}") | |
return [] | |
# Indeed API Integration using /list and /get | |
# Cache results for 1 day | |
def fetch_indeed_jobs_list_api(job_title, location="United States", distance="1.0", language="en_GB", remoteOnly="false", datePosted="month", employmentTypes="fulltime;parttime;intern;contractor", index=0, page_size=10): | |
""" | |
Fetches a list of job IDs from Indeed API based on user preferences. | |
Args: | |
job_title (str): The job title to search for. | |
location (str, optional): The job location. Defaults to "United States". | |
distance (str, optional): Search radius in miles. Defaults to "1.0". | |
language (str, optional): Language code. Defaults to "en_GB". | |
remoteOnly (str, optional): "true" or "false". Defaults to "false". | |
datePosted (str, optional): e.g., "month". Defaults to "month". | |
employmentTypes (str, optional): e.g., "fulltime;parttime;intern;contractor". Defaults to "fulltime;parttime;intern;contractor". | |
index (int, optional): Pagination index. Defaults to 0. | |
page_size (int, optional): Number of jobs to fetch. Defaults to 10. | |
Returns: | |
list: A list of job IDs. | |
""" | |
url = "https://jobs-api14.p.rapidapi.com/list" | |
querystring = { | |
"query": job_title, | |
"location": location, | |
"distance": distance, | |
"language": language, | |
"remoteOnly": remoteOnly, | |
"datePosted": datePosted, | |
"employmentTypes": employmentTypes, | |
"index": str(index), | |
"page_size": str(page_size) | |
} | |
headers = { | |
"x-rapidapi-key": RAPIDAPI_KEY, | |
"x-rapidapi-host": "jobs-api14.p.rapidapi.com" | |
} | |
try: | |
response = requests.get(url, headers=headers, params=querystring) | |
response.raise_for_status() | |
data = response.json() | |
job_ids = [job["id"] for job in data.get("jobs", [])] | |
return job_ids | |
except requests.exceptions.HTTPError as http_err: | |
if response.status_code == 400: | |
st.error("❌ Bad Request: Please check the parameters you're sending.") | |
elif response.status_code == 403: | |
st.error("❌ Access Forbidden: Check your API key and permissions.") | |
elif response.status_code == 404: | |
st.error("❌ Resource Not Found: Verify the endpoint and parameters.") | |
else: | |
st.error(f"❌ HTTP error occurred: {http_err}") | |
return [] | |
except requests.exceptions.RequestException as req_err: | |
st.error(f"❌ Request Exception: {req_err}") | |
return [] | |
except Exception as e: | |
st.error(f"❌ An unexpected error occurred: {e}") | |
return [] | |
# Cache results for 1 day | |
def fetch_indeed_job_details_api(job_id, language="en_GB"): | |
""" | |
Fetches job details from Indeed API based on job ID. | |
Args: | |
job_id (str): The job ID. | |
language (str, optional): Language code. Defaults to "en_GB". | |
Returns: | |
dict: Job details. | |
""" | |
url = "https://jobs-api14.p.rapidapi.com/get" | |
querystring = { | |
"id": job_id, | |
"language": language | |
} | |
headers = { | |
"x-rapidapi-key": RAPIDAPI_KEY, | |
"x-rapidapi-host": "jobs-api14.p.rapidapi.com" | |
} | |
try: | |
response = requests.get(url, headers=headers, params=querystring) | |
response.raise_for_status() | |
job_details = response.json() | |
return job_details | |
except requests.exceptions.HTTPError as http_err: | |
if response.status_code == 400: | |
st.error("❌ Bad Request: Please check the job ID and parameters.") | |
elif response.status_code == 403: | |
st.error("❌ Access Forbidden: Check your API key and permissions.") | |
elif response.status_code == 404: | |
st.error("❌ Job Not Found: Verify the job ID.") | |
else: | |
st.error(f"❌ HTTP error occurred: {http_err}") | |
return {} | |
except requests.exceptions.RequestException as req_err: | |
st.error(f"❌ Request Exception: {req_err}") | |
return {} | |
except Exception as e: | |
st.error(f"❌ An unexpected error occurred: {e}") | |
return {} | |
def recommend_indeed_jobs(user_skills, user_preferences): | |
""" | |
Recommends jobs from Indeed API based on user skills and preferences. | |
Args: | |
user_skills (list): List of user's skills. | |
user_preferences (dict): User preferences like job title, location, category. | |
Returns: | |
list: Recommended job listings. | |
""" | |
job_title = user_preferences.get("job_title", "") | |
location = user_preferences.get("location", "United States") | |
category = user_preferences.get("category", "") | |
language = "en_GB" | |
# Fetch job IDs | |
job_ids = fetch_indeed_jobs_list_api(job_title, location=location, category=category, page_size=5) # Limiting to 5 for API call count | |
recommended_jobs = [] | |
api_calls_needed = len(job_ids) # Each /get call counts as one | |
# Check if enough API calls are left | |
if not can_make_api_calls(api_calls_needed): | |
st.error("❌ You have reached your monthly API request limit. Please try again next month.") | |
return [] | |
for job_id in job_ids: | |
job_details = fetch_indeed_job_details_api(job_id, language=language) | |
if job_details and not job_details.get("hasError", True): | |
job_description = job_details.get("description", "").lower() | |
match_score = sum(skill.lower() in job_description for skill in user_skills) | |
if match_score > 0: | |
recommended_jobs.append((match_score, job_details)) | |
decrement_api_calls(1) | |
# Sort jobs based on match_score | |
recommended_jobs.sort(reverse=True, key=lambda x: x[0]) | |
# Return only the job dictionaries | |
return [job for score, job in recommended_jobs[:10]] # Top 10 recommendations | |
def recommend_jobs(user_skills, user_preferences): | |
""" | |
Recommends jobs based on user skills and preferences from Remotive, The Muse, and Indeed APIs. | |
Args: | |
user_skills (list): List of user's skills. | |
user_preferences (dict): User preferences like job title, location, category. | |
Returns: | |
list: Recommended job listings. | |
""" | |
# Fetch from Remotive | |
remotive_jobs = fetch_remotive_jobs_api(user_preferences.get("job_title", ""), user_preferences.get("location"), user_preferences.get("category")) | |
# Fetch from The Muse | |
muse_jobs = fetch_muse_jobs_api(user_preferences.get("job_title", ""), user_preferences.get("location"), user_preferences.get("category")) | |
# Fetch from Indeed | |
indeed_jobs = recommend_indeed_jobs(user_skills, user_preferences) | |
# Combine all job listings | |
combined_jobs = remotive_jobs + muse_jobs + indeed_jobs | |
# Remove duplicates based on job URL | |
unique_jobs = {} | |
for job in combined_jobs: | |
url = job.get("url") or job.get("redirect_url") or job.get("url_standard") | |
if url and url not in unique_jobs: | |
unique_jobs[url] = job | |
return list(unique_jobs.values()) | |
# ------------------------------- | |
# BLS API Integration and Display | |
# ------------------------------- | |
# Cache results for 1 day | |
def fetch_bls_data(series_ids, start_year=2020, end_year=datetime.now().year): | |
""" | |
Fetches labor market data from the BLS API. | |
Args: | |
series_ids (list): List of BLS series IDs. | |
start_year (int, optional): Start year for data. Defaults to 2020. | |
end_year (int, optional): End year for data. Defaults to current year. | |
Returns: | |
dict: BLS data response. | |
""" | |
bls_url = "https://api.bls.gov/publicAPI/v2/timeseries/data/" | |
headers = { | |
"Content-Type": "application/json" | |
} | |
payload = { | |
"seriesid": series_ids, | |
"startyear": str(start_year), | |
"endyear": str(end_year) | |
} | |
try: | |
response = requests.post(bls_url, json=payload, headers=headers) | |
response.raise_for_status() | |
data = response.json() | |
if data.get("status") == "REQUEST_SUCCEEDED": | |
return data.get("Results", {}) | |
else: | |
st.error("BLS API request failed.") | |
return {} | |
except requests.exceptions.RequestException as e: | |
st.error(f"Error fetching data from BLS: {e}") | |
return {} | |
def display_bls_data(series_id, title): | |
""" | |
Processes and displays BLS data with visualizations. | |
Args: | |
series_id (str): BLS series ID. | |
title (str): Title for the visualization. | |
""" | |
data = fetch_bls_data([series_id]) | |
if not data: | |
st.info("No data available.") | |
return | |
series_data = data.get("series", [])[0] | |
series_title = series_data.get("title", title) | |
observations = series_data.get("data", []) | |
# Extract year and value | |
years = [int(obs["year"]) for obs in observations] | |
values = [float(obs["value"].replace(',', '')) for obs in observations] | |
df = pd.DataFrame({ | |
"Year": years, | |
"Value": values | |
}).sort_values("Year") | |
st.markdown(f"### {series_title}") | |
fig = px.line(df, x="Year", y="Value", title=series_title, markers=True) | |
st.plotly_chart(fig, use_container_width=True) | |
# ------------------------------- | |
# API Usage Counter Functions | |
# ------------------------------- | |
def init_api_usage_db(): | |
""" | |
Initializes the SQLite database and creates the api_usage table if it doesn't exist. | |
""" | |
conn = sqlite3.connect('applications.db') | |
c = conn.cursor() | |
c.execute(''' | |
CREATE TABLE IF NOT EXISTS api_usage ( | |
id INTEGER PRIMARY KEY AUTOINCREMENT, | |
count INTEGER, | |
last_reset DATE | |
) | |
''') | |
# Check if a row exists, if not, initialize | |
c.execute('SELECT COUNT(*) FROM api_usage') | |
if c.fetchone()[0] == 0: | |
# Initialize with 25 requests and current date | |
c.execute('INSERT INTO api_usage (count, last_reset) VALUES (?, ?)', (25, datetime.now().date())) | |
conn.commit() | |
conn.close() | |
def get_api_usage(): | |
""" | |
Retrieves the current API usage count and last reset date. | |
Returns: | |
tuple: (count, last_reset_date) | |
""" | |
conn = sqlite3.connect('applications.db') | |
c = conn.cursor() | |
c.execute('SELECT count, last_reset FROM api_usage WHERE id = 1') | |
row = c.fetchone() | |
conn.close() | |
if row: | |
return row[0], datetime.strptime(row[1], "%Y-%m-%d").date() | |
else: | |
return 25, datetime.now().date() | |
def reset_api_usage(): | |
""" | |
Resets the API usage count to 25 and updates the last reset date. | |
""" | |
conn = sqlite3.connect('applications.db') | |
c = conn.cursor() | |
c.execute('UPDATE api_usage SET count = ?, last_reset = ? WHERE id = 1', (25, datetime.now().date())) | |
conn.commit() | |
conn.close() | |
def can_make_api_calls(requests_needed): | |
""" | |
Checks if there are enough API calls remaining. | |
Args: | |
requests_needed (int): Number of API calls required. | |
Returns: | |
bool: True if allowed, False otherwise. | |
""" | |
count, last_reset = get_api_usage() | |
today = datetime.now().date() | |
if today >= last_reset + timedelta(days=30): | |
reset_api_usage() | |
count, last_reset = get_api_usage() | |
if count >= requests_needed: | |
return True | |
else: | |
return False | |
def decrement_api_calls(requests_used): | |
""" | |
Decrements the API usage count by the number of requests used. | |
Args: | |
requests_used (int): Number of API calls used. | |
""" | |
conn = sqlite3.connect('applications.db') | |
c = conn.cursor() | |
c.execute('SELECT count FROM api_usage WHERE id = 1') | |
row = c.fetchone() | |
if row: | |
new_count = row[0] - requests_used | |
if new_count < 0: | |
new_count = 0 | |
c.execute('UPDATE api_usage SET count = ? WHERE id = 1', (new_count,)) | |
conn.commit() | |
conn.close() | |
# ------------------------------- | |
# Application Tracking Database Functions | |
# ------------------------------- | |
def init_db(): | |
""" | |
Initializes the SQLite database and creates the applications table if it doesn't exist. | |
""" | |
conn = sqlite3.connect('applications.db') | |
c = conn.cursor() | |
c.execute(''' | |
CREATE TABLE IF NOT EXISTS applications ( | |
id INTEGER PRIMARY KEY AUTOINCREMENT, | |
job_title TEXT, | |
company TEXT, | |
application_date TEXT, | |
status TEXT, | |
deadline TEXT, | |
notes TEXT, | |
job_description TEXT, | |
resume_text TEXT, | |
skills TEXT | |
) | |
''') | |
conn.commit() | |
conn.close() | |
def add_application(job_title, company, application_date, status, deadline, notes, job_description, resume_text, skills): | |
""" | |
Adds a new job application to the database. | |
""" | |
conn = sqlite3.connect('applications.db') | |
c = conn.cursor() | |
c.execute(''' | |
INSERT INTO applications (job_title, company, application_date, status, deadline, notes, job_description, resume_text, skills) | |
VALUES (?, ?, ?, ?, ?, ?, ?, ?, ?) | |
''', (job_title, company, application_date, status, deadline, notes, job_description, resume_text, ', '.join(skills))) | |
conn.commit() | |
conn.close() | |
def fetch_applications(): | |
""" | |
Fetches all applications from the database. | |
""" | |
conn = sqlite3.connect('applications.db') | |
c = conn.cursor() | |
c.execute('SELECT * FROM applications') | |
data = c.fetchall() | |
conn.close() | |
applications = [] | |
for app in data: | |
applications.append({ | |
"ID": app[0], | |
"Job Title": app[1], | |
"Company": app[2], | |
"Application Date": app[3], | |
"Status": app[4], | |
"Deadline": app[5], | |
"Notes": app[6], | |
"Job Description": app[7], | |
"Resume Text": app[8], | |
"Skills": app[9].split(', ') if app[9] else [] | |
}) | |
return applications | |
def update_application_status(app_id, new_status): | |
""" | |
Updates the status of an application. | |
""" | |
conn = sqlite3.connect('applications.db') | |
c = conn.cursor() | |
c.execute('UPDATE applications SET status = ? WHERE id = ?', (new_status, app_id)) | |
conn.commit() | |
conn.close() | |
def delete_application(app_id): | |
""" | |
Deletes an application from the database. | |
""" | |
conn = sqlite3.connect('applications.db') | |
c = conn.cursor() | |
c.execute('DELETE FROM applications WHERE id = ?', (app_id,)) | |
conn.commit() | |
conn.close() | |
# ------------------------------- | |
# Learning Path Generation Function | |
# ------------------------------- | |
# Cache results for 1 day | |
def generate_learning_path(career_goal, current_skills): | |
""" | |
Generates a personalized learning path using Groq based on career goal and current skills. | |
""" | |
prompt = f""" | |
Based on the following career goal and current skills, create a personalized learning path that includes recommended courses, projects, and milestones to achieve the career goal. | |
**Career Goal:** | |
{career_goal} | |
**Current Skills:** | |
{current_skills} | |
**Learning Path:** | |
""" | |
try: | |
response = llm.invoke(prompt) | |
learning_path = response.content.strip() | |
return learning_path | |
except Exception as e: | |
st.error(f"Error generating learning path: {e}") | |
return "" | |
# ------------------------------- | |
# YouTube Video Search and Embed Functions | |
# ------------------------------- | |
# Cache results for 1 day | |
def search_youtube_videos(query, max_results=2, video_duration="long"): | |
""" | |
Searches YouTube for videos matching the query and returns video URLs. | |
Args: | |
query (str): Search query. | |
max_results (int, optional): Number of videos to return. Defaults to 2. | |
video_duration (str, optional): Duration filter ('any', 'short', 'medium', 'long'). Defaults to "long". | |
Returns: | |
list: List of YouTube video URLs. | |
""" | |
search_url = "https://www.googleapis.com/youtube/v3/search" | |
params = { | |
"part": "snippet", | |
"q": query, | |
"type": "video", | |
"maxResults": max_results, | |
"videoDuration": video_duration, | |
"key": YOUTUBE_API_KEY | |
} | |
try: | |
response = requests.get(search_url, params=params) | |
response.raise_for_status() | |
results = response.json().get("items", []) | |
video_urls = [f"https://www.youtube.com/watch?v={item['id']['videoId']}" for item in results] | |
return video_urls | |
except requests.exceptions.RequestException as e: | |
st.error(f"❌ Error fetching YouTube videos: {e}") | |
return [] | |
def embed_youtube_videos(video_urls, module_name): | |
""" | |
Embeds YouTube videos in the Streamlit app. | |
Args: | |
video_urls (list): List of YouTube video URLs. | |
module_name (str): Name of the module for context. | |
""" | |
for url in video_urls: | |
st.video(url) | |
# ------------------------------- | |
# Job Recommendations and BLS Integration | |
# ------------------------------- | |
def labor_market_insights_module(): | |
st.header("📈 Labor Market Insights") | |
st.write(""" | |
Gain valuable insights into the current labor market trends, employment rates, and industry growth to make informed career decisions. | |
""") | |
# Define BLS Series IDs based on desired data | |
# Example: Unemployment rate (Series ID: LNS14000000) | |
# Reference: https://www.bls.gov/web/laus/laumstrk.htm | |
unemployment_series_id = "LNS14000000" # Unemployment Rate | |
employment_series_id = "CEU0000000001" # Total Employment | |
# Display Unemployment Rate | |
display_bls_data(unemployment_series_id, "Unemployment Rate (%)") | |
# Display Total Employment | |
display_bls_data(employment_series_id, "Total Employment") | |
# Additional Insights | |
st.subheader("💡 Additional Insights") | |
st.write(""" | |
- **Industry Growth:** Understanding which industries are growing can help you target your job search effectively. | |
- **Salary Trends:** Keeping an eye on salary trends ensures that you negotiate effectively and align your expectations. | |
- **Geographical Demand:** Some regions may have higher demand for certain roles, guiding your location preferences. | |
""") | |
# ------------------------------- | |
# Page Functions | |
# ------------------------------- | |
def email_generator_page(): | |
st.header("📧 Automated Email Generator") | |
st.write(""" | |
Generate personalized cold emails based on job postings and your resume. | |
""") | |
# Create two columns for input fields | |
col1, col2 = st.columns(2) | |
with col1: | |
job_link = st.text_input("🔗 Enter the job link:") | |
with col2: | |
uploaded_file = st.file_uploader("📄 Upload your resume (PDF format):", type="pdf") | |
if st.button("Generate Email"): | |
if not job_link: | |
st.error("Please enter a job link.") | |
return | |
if not uploaded_file: | |
st.error("Please upload your resume.") | |
return | |
with st.spinner("Processing..."): | |
# Extract job description | |
job_description = extract_job_description(job_link) | |
if not job_description: | |
st.error("Failed to extract job description.") | |
return | |
# Extract requirements | |
requirements = extract_requirements(job_description) | |
if not requirements: | |
st.error("Failed to extract requirements.") | |
return | |
# Extract resume text | |
resume_text = extract_text_from_pdf(uploaded_file) | |
if not resume_text: | |
st.error("Failed to extract text from resume.") | |
return | |
# Generate email | |
email_text = generate_email(job_description, requirements, resume_text) | |
if email_text: | |
st.subheader("📨 Generated Email:") | |
st.write(email_text) | |
# Provide download option | |
st.download_button( | |
label="Download Email", | |
data=email_text, | |
file_name="generated_email.txt", | |
mime="text/plain" | |
) | |
else: | |
st.error("Failed to generate email.") | |
def cover_letter_generator_page(): | |
st.header("📝 Automated Cover Letter Generator") | |
st.write(""" | |
Generate personalized cover letters based on job postings and your resume. | |
""") | |
# Create two columns for input fields | |
col1, col2 = st.columns(2) | |
with col1: | |
job_link = st.text_input("🔗 Enter the job link:") | |
with col2: | |
uploaded_file = st.file_uploader("📄 Upload your resume (PDF format):", type="pdf") | |
if st.button("Generate Cover Letter"): | |
if not job_link: | |
st.error("Please enter a job link.") | |
return | |
if not uploaded_file: | |
st.error("Please upload your resume.") | |
return | |
with st.spinner("Processing..."): | |
# Extract job description | |
job_description = extract_job_description(job_link) | |
if not job_description: | |
st.error("Failed to extract job description.") | |
return | |
# Extract requirements | |
requirements = extract_requirements(job_description) | |
if not requirements: | |
st.error("Failed to extract requirements.") | |
return | |
# Extract resume text | |
resume_text = extract_text_from_pdf(uploaded_file) | |
if not resume_text: | |
st.error("Failed to extract text from resume.") | |
return | |
# Generate cover letter | |
cover_letter = generate_cover_letter(job_description, requirements, resume_text) | |
if cover_letter: | |
st.subheader("📝 Generated Cover Letter:") | |
st.write(cover_letter) | |
# Provide download option | |
st.download_button( | |
label="Download Cover Letter", | |
data=cover_letter, | |
file_name="generated_cover_letter.txt", | |
mime="text/plain" | |
) | |
else: | |
st.error("Failed to generate cover letter.") | |
def resume_analysis_page(): | |
st.header("📄 Resume Analysis and Optimization") | |
st.write(""" | |
Enhance your resume's effectiveness with our comprehensive analysis tools. Upload your resume to extract key information, receive optimization suggestions, and visualize your skills and experience. | |
""") | |
uploaded_file = st.file_uploader("📂 Upload your resume (PDF format):", type="pdf") | |
if uploaded_file: | |
resume_text = extract_text_from_pdf(uploaded_file) | |
if resume_text: | |
st.success("✅ Resume uploaded successfully!") | |
# Extracted Information | |
st.subheader("🔍 Extracted Information") | |
# Create tabs for organized sections | |
tabs = st.tabs(["💼 Skills", "🔑 Suggested Keywords"]) | |
with tabs[0]: | |
skills = extract_skills(resume_text) | |
if skills: | |
st.markdown("**Identified Skills:**") | |
# Display skills as bullet points in columns | |
cols = st.columns(4) | |
for idx, skill in enumerate(skills, 1): | |
cols[idx % 4].write(f"- {skill}") | |
else: | |
st.info("No skills extracted.") | |
with tabs[1]: | |
keywords = suggest_keywords(resume_text) | |
if keywords: | |
st.markdown("**Suggested Keywords for ATS Optimization:**") | |
# Display keywords as bullet points in columns | |
cols = st.columns(4) | |
for idx, keyword in enumerate(keywords, 1): | |
cols[idx % 4].write(f"- {keyword}") | |
else: | |
st.info("No keywords suggested.") | |
# Optimization Suggestions | |
st.subheader("🛠️ Optimization Suggestions") | |
if keywords: | |
st.markdown(""" | |
- **Keyword Optimization:** Incorporate the suggested keywords to improve ATS compatibility. | |
- **Enhance Relevant Sections:** Highlight skills and experiences that align closely with job descriptions. | |
""") | |
else: | |
st.markdown("- **Keyword Optimization:** No keywords suggested.") | |
st.markdown(""" | |
- **Formatting:** Ensure consistent formatting for headings, bullet points, and text alignment to enhance readability. | |
- **Quantify Achievements:** Where possible, quantify your accomplishments to demonstrate impact. | |
- **Tailor Your Resume:** Customize your resume for each job application to emphasize relevant experiences. | |
""") | |
# Visual Resume Analytics | |
st.subheader("📊 Visual Resume Analytics") | |
# Create two columns for charts | |
viz_col1, viz_col2 = st.columns(2) | |
with viz_col1: | |
if skills: | |
st.markdown("**Skill Distribution:**") | |
fig_skills = create_skill_distribution_chart(skills) | |
st.plotly_chart(fig_skills, use_container_width=True) | |
else: | |
st.info("No skills to display.") | |
with viz_col2: | |
fig_experience = create_experience_timeline(resume_text) | |
if fig_experience: | |
st.markdown("**Experience Timeline:**") | |
st.plotly_chart(fig_experience, use_container_width=True) | |
else: | |
st.info("Not enough data to generate an experience timeline.") | |
# Save the resume and analysis to the database | |
st.subheader("💾 Save Resume Analysis") | |
if st.button("Save Resume Analysis"): | |
add_application( | |
job_title="N/A", | |
company="N/A", | |
application_date=datetime.now().strftime("%Y-%m-%d"), | |
status="N/A", | |
deadline="N/A", | |
notes="Resume Analysis", | |
job_description="N/A", | |
resume_text=resume_text, | |
skills=skills | |
) | |
st.success("✅ Resume analysis saved successfully!") | |
else: | |
st.error("❌ Failed to extract text from resume.") | |
def application_tracking_dashboard(): | |
st.header("📋 Application Tracking Dashboard") | |
# Initialize database | |
init_db() | |
init_api_usage_db() | |
# Form to add a new application | |
st.subheader("➕ Add New Application") | |
with st.form("add_application"): | |
job_title = st.text_input("🖇️ Job Title") | |
company = st.text_input("🏢 Company") | |
application_date = st.date_input("📅 Application Date", datetime.today()) | |
status = st.selectbox("📈 Status", ["Applied", "Interviewing", "Offered", "Rejected"]) | |
deadline = st.date_input("⏰ Application Deadline", datetime.today() + timedelta(days=30)) | |
notes = st.text_area("📝 Notes") | |
uploaded_file = st.file_uploader("📂 Upload Job Description (PDF)", type="pdf") | |
uploaded_resume = st.file_uploader("📄 Upload Resume (PDF)", type="pdf") | |
submitted = st.form_submit_button("➕ Add Application") | |
if submitted: | |
if uploaded_file: | |
job_description = extract_text_from_pdf(uploaded_file) | |
else: | |
job_description = "" | |
if uploaded_resume: | |
resume_text = extract_text_from_pdf(uploaded_resume) | |
skills = extract_skills(resume_text) | |
else: | |
resume_text = "" | |
skills = [] | |
add_application( | |
job_title=job_title, | |
company=company, | |
application_date=application_date.strftime("%Y-%m-%d"), | |
status=status, | |
deadline=deadline.strftime("%Y-%m-%d"), | |
notes=notes, | |
job_description=job_description, | |
resume_text=resume_text, | |
skills=skills | |
) | |
st.success("✅ Application added successfully!") | |
# Display applications | |
st.subheader("📊 Your Applications") | |
applications = fetch_applications() | |
if applications: | |
df = pd.DataFrame(applications) | |
df = df.drop(columns=["Job Description", "Resume Text", "Skills"]) | |
st.dataframe(df) | |
# Export Button | |
csv = df.to_csv(index=False).encode('utf-8') | |
st.download_button( | |
label="💾 Download Applications as CSV", | |
data=csv, | |
file_name='applications.csv', | |
mime='text/csv', | |
) | |
# Import Button | |
st.subheader("📥 Import Applications") | |
uploaded_csv = st.file_uploader("📁 Upload a CSV file", type="csv") | |
if uploaded_csv: | |
try: | |
imported_df = pd.read_csv(uploaded_csv) | |
# Validate required columns | |
required_columns = {"Job Title", "Company", "Application Date", "Status", "Deadline", "Notes"} | |
if not required_columns.issubset(imported_df.columns): | |
st.error("❌ Uploaded CSV is missing required columns.") | |
else: | |
for index, row in imported_df.iterrows(): | |
job_title = row.get("Job Title", "N/A") | |
company = row.get("Company", "N/A") | |
application_date = row.get("Application Date", datetime.now().strftime("%Y-%m-%d")) | |
status = row.get("Status", "Applied") | |
deadline = row.get("Deadline", "") | |
notes = row.get("Notes", "") | |
job_description = row.get("Job Description", "") | |
resume_text = row.get("Resume Text", "") | |
skills = row.get("Skills", "").split(', ') if row.get("Skills") else [] | |
add_application( | |
job_title=job_title, | |
company=company, | |
application_date=application_date, | |
status=status, | |
deadline=deadline, | |
notes=notes, | |
job_description=job_description, | |
resume_text=resume_text, | |
skills=skills | |
) | |
st.success("✅ Applications imported successfully!") | |
except Exception as e: | |
st.error(f"❌ Error importing applications: {e}") | |
# Actions: Update Status or Delete | |
for app in applications: | |
with st.expander(f"{app['Job Title']} at {app['Company']}"): | |
st.write(f"**📅 Application Date:** {app['Application Date']}") | |
st.write(f"**⏰ Deadline:** {app['Deadline']}") | |
st.write(f"**📈 Status:** {app['Status']}") | |
st.write(f"**📝 Notes:** {app['Notes']}") | |
if app['Job Description']: | |
st.write("**📄 Job Description:**") | |
st.write(app['Job Description'][:500] + "...") | |
if app['Skills']: | |
st.write("**💼 Skills:**", ', '.join(app['Skills'])) | |
# Update status | |
new_status = st.selectbox("🔄 Update Status:", ["Applied", "Interviewing", "Offered", "Rejected"], key=f"status_{app['ID']}") | |
if st.button("🔁 Update Status", key=f"update_{app['ID']}"): | |
update_application_status(app['ID'], new_status) | |
st.success("✅ Status updated successfully!") | |
# Delete application | |
if st.button("🗑️ Delete Application", key=f"delete_{app['ID']}"): | |
delete_application(app['ID']) | |
st.success("✅ Application deleted successfully!") | |
else: | |
st.write("ℹ️ No applications found.") | |
def job_recommendations_module(): | |
st.header("🔍 Job Matching & Recommendations") | |
st.write(""" | |
Discover job opportunities tailored to your skills and preferences. Get personalized recommendations from multiple job platforms. | |
""") | |
# User Preferences Form | |
st.subheader("🎯 Set Your Preferences") | |
with st.form("preferences_form"): | |
job_title = st.text_input("🔍 Desired Job Title", placeholder="e.g., Data Scientist, Backend Developer") | |
location = st.text_input("📍 Preferred Location", placeholder="e.g., New York, NY, USA or Remote") | |
category = st.selectbox("📂 Job Category", ["", "Engineering", "Marketing", "Design", "Sales", "Finance", "Healthcare", "Education", "Other"]) | |
user_skills_input = st.text_input("💡 Your Skills (comma-separated)", placeholder="e.g., Python, Machine Learning, SQL") | |
submitted = st.form_submit_button("🚀 Get Recommendations") | |
if submitted: | |
if not job_title or not user_skills_input: | |
st.error("❌ Please enter both job title and your skills.") | |
return | |
user_skills = [skill.strip() for skill in user_skills_input.split(",") if skill.strip()] | |
user_preferences = { | |
"job_title": job_title, | |
"location": location, | |
"category": category | |
} | |
with st.spinner("🔄 Fetching job recommendations..."): | |
# Fetch recommendations from all APIs (Remotive, The Muse, Indeed) | |
recommended_jobs = recommend_jobs(user_skills, user_preferences) | |
if recommended_jobs: | |
st.subheader("💼 Recommended Jobs:") | |
for idx, job in enumerate(recommended_jobs, 1): | |
# Depending on the API, job data structure might differ | |
job_title_display = job.get("title") or job.get("name") or job.get("jobTitle") | |
company_display = job.get("company", {}).get("name") or job.get("company_name") or job.get("employer", {}).get("name") | |
location_display = job.get("candidate_required_location") or job.get("location") or job.get("country") | |
salary_display = "N/A" # Salary is removed | |
job_url = job.get("url") or job.get("redirect_url") or job.get("url_standard") | |
st.markdown(f"### {idx}. {job_title_display}") | |
st.markdown(f"**🏢 Company:** {company_display}") | |
st.markdown(f"**📍 Location:** {location_display}") | |
st.markdown(f"**🔗 Job URL:** [Apply Here]({job_url})") | |
st.write("---") | |
else: | |
st.info("ℹ️ No job recommendations found based on your criteria.") | |
def interview_preparation_module(): | |
st.header("🎤 Interview Preparation") | |
st.write(""" | |
Prepare for your interviews with tailored mock questions and expert answers. | |
""") | |
# Create two columns for input fields | |
col1, col2 = st.columns(2) | |
with col1: | |
job_title = st.text_input("🔍 Enter the job title you're applying for:") | |
with col2: | |
company = st.text_input("🏢 Enter the company name:") | |
if st.button("🎯 Generate Mock Interview Questions"): | |
if not job_title or not company: | |
st.error("❌ Please enter both job title and company name.") | |
return | |
with st.spinner("⏳ Generating questions..."): | |
# Prompt to generate 50 interview questions with answers | |
prompt = f""" | |
Generate a list of 50 interview questions along with their answers for the position of {job_title} at {company}. Each question should be followed by a concise and professional answer. | |
""" | |
try: | |
# Invoke the LLM to get questions and answers | |
qa_text = llm.invoke(prompt).content.strip() | |
# Split into question-answer pairs | |
qa_pairs = qa_text.split('\n\n') | |
st.subheader("🗣️ Mock Interview Questions and Answers:") | |
for idx, qa in enumerate(qa_pairs, 1): | |
if qa.strip(): | |
parts = qa.split('\n', 1) | |
if len(parts) == 2: | |
question = parts[0].strip() | |
answer = parts[1].strip() | |
st.markdown(f"**Q{idx}: {question}**") | |
st.markdown(f"**A:** {answer}") | |
st.write("---") | |
except Exception as e: | |
st.error(f"❌ Error generating interview questions: {e}") | |
def personalized_learning_paths_module(): | |
st.header("📚 Personalized Learning Paths") | |
st.write(""" | |
Receive tailored learning plans to help you acquire the skills needed for your desired career, complemented with curated video resources. | |
""") | |
# Create two columns for input fields | |
col1, col2 = st.columns(2) | |
with col1: | |
career_goal = st.text_input("🎯 Enter your career goal (e.g., Data Scientist, Machine Learning Engineer):") | |
with col2: | |
current_skills = st.text_input("💡 Enter your current skills (comma-separated):") | |
if st.button("🚀 Generate Learning Path"): | |
if not career_goal or not current_skills: | |
st.error("❌ Please enter both career goal and current skills.") | |
return | |
with st.spinner("🔄 Generating your personalized learning path..."): | |
learning_path = generate_learning_path(career_goal, current_skills) | |
if learning_path: | |
st.subheader("📜 Your Personalized Learning Path:") | |
st.write(learning_path) | |
# Assuming the learning path is divided into modules/subparts separated by newlines or numbering | |
# We'll extract subparts and embed YouTube videos for each | |
# Example format: | |
# 1. Module One | |
# 2. Module Two | |
# etc. | |
# Split learning path into modules | |
modules = re.split(r'\d+\.\s+', learning_path) | |
modules = [module.strip() for module in modules if module.strip()] | |
st.subheader("📹 Recommended YouTube Videos for Each Module:") | |
for module in modules: | |
# Search for long videos related to the module | |
video_urls = search_youtube_videos(query=module, max_results=2, video_duration="long") | |
if video_urls: | |
st.markdown(f"### {module}") | |
embed_youtube_videos(video_urls, module) | |
else: | |
st.write(f"No videos found for **{module}**.") | |
else: | |
st.error("❌ Failed to generate learning path.") | |
def networking_opportunities_module(): | |
st.header("🤝 Networking Opportunities") | |
st.write(""" | |
Expand your professional network by connecting with relevant industry peers and joining professional groups. | |
""") | |
# Create two columns for input fields | |
col1, col2 = st.columns(2) | |
with col1: | |
user_skills = st.text_input("💡 Enter your key skills (comma-separated):") | |
with col2: | |
industry = st.text_input("🏭 Enter your industry (e.g., Technology, Finance):") | |
if st.button("🔍 Find Networking Opportunities"): | |
if not user_skills or not industry: | |
st.error("❌ Please enter both key skills and industry.") | |
return | |
with st.spinner("🔄 Fetching networking opportunities..."): | |
# Suggest LinkedIn groups or connections based on skills and industry | |
prompt = f""" | |
Based on the following skills: {user_skills}, and industry: {industry}, suggest relevant LinkedIn groups, professional organizations, and industry events for networking. | |
""" | |
try: | |
suggestions = llm.invoke(prompt).content.strip() | |
st.subheader("🔗 Recommended Networking Groups and Events:") | |
st.write(suggestions) | |
except Exception as e: | |
st.error(f"❌ Error fetching networking opportunities: {e}") | |
def feedback_and_improvement_module(): | |
st.header("🗣️ Feedback and Continuous Improvement") | |
st.write(""" | |
We value your feedback! Let us know how we can improve your experience. | |
""") | |
with st.form("feedback_form"): | |
name = st.text_input("👤 Your Name") | |
email = st.text_input("📧 Your Email") | |
feedback_type = st.selectbox("📂 Type of Feedback", ["Bug Report", "Feature Request", "General Feedback"]) | |
feedback = st.text_area("📝 Your Feedback") | |
submitted = st.form_submit_button("✅ Submit") | |
if submitted: | |
if not name or not email or not feedback: | |
st.error("❌ Please fill in all the fields.") | |
else: | |
# Here you can implement logic to store feedback, e.g., in a database or send via email | |
# For demonstration, we'll print to the console | |
print(f"Feedback from {name} ({email}): {feedback_type} - {feedback}") | |
st.success("✅ Thank you for your feedback!") | |
def gamification_module(): | |
st.header("🏆 Gamification and Achievements") | |
st.write(""" | |
Stay motivated by earning badges and tracking your progress! | |
""") | |
# Initialize database | |
init_db() | |
# Example achievements | |
applications = fetch_applications() | |
num_apps = len(applications) | |
achievements = { | |
"First Application": num_apps >= 1, | |
"5 Applications": num_apps >= 5, | |
"10 Applications": num_apps >= 10, | |
"Resume Optimized": any(app['Skills'] for app in applications), | |
"Interview Scheduled": any(app['Status'] == 'Interviewing' for app in applications) | |
} | |
for achievement, earned in achievements.items(): | |
if earned: | |
st.success(f"🎉 {achievement}") | |
else: | |
st.info(f"🔜 {achievement}") | |
# Progress Bar | |
progress = min(num_apps / 10, 1.0) # Ensure progress is between 0.0 and 1.0 | |
st.write("**Overall Progress:**") | |
st.progress(progress) | |
st.write(f"{progress * 100:.0f}% complete") | |
def resource_library_page(): | |
st.header("📚 Resource Library") | |
st.write(""" | |
Access a collection of templates and guides to enhance your job search. | |
""") | |
resources = [ | |
{ | |
"title": "Resume Template", | |
"description": "A professional resume template in DOCX format.", | |
"file": "./resume_template.docx" | |
}, | |
{ | |
"title": "Cover Letter Template", | |
"description": "A customizable cover letter template.", | |
"file": "./cover_letter_template.docx" | |
}, | |
{ | |
"title": "Job Application Checklist", | |
"description": "Ensure you have all the necessary steps covered during your job search.", | |
"file": "./application_checklist.pdf" | |
} | |
] | |
for resource in resources: | |
st.markdown(f"### {resource['title']}") | |
st.write(resource['description']) | |
try: | |
with open(resource['file'], "rb") as file: | |
btn = st.download_button( | |
label="⬇️ Download", | |
data=file, | |
file_name=os.path.basename(resource['file']), | |
mime="application/octet-stream" | |
) | |
except FileNotFoundError: | |
st.error(f"❌ File {resource['file']} not found. Please ensure the file is in the correct directory.") | |
st.write("---") | |
def success_stories_page(): | |
st.header("🌟 Success Stories") | |
st.write(""" | |
Hear from our users who have successfully landed their dream jobs with our assistance! | |
""") | |
# Example testimonials | |
testimonials = [ | |
{ | |
"name": "Rahul Sharma", | |
"position": "Data Scientist at TechCorp", | |
"testimonial": "This app transformed my job search process. The resume analysis and personalized emails were game-changers!", | |
"image": "images/user1.jpg" # Replace with actual image paths | |
}, | |
{ | |
"name": "Priya Mehta", | |
"position": "Machine Learning Engineer at InnovateX", | |
"testimonial": "The interview preparation module helped me ace my interviews with confidence. Highly recommended!", | |
"image": "images/user2.jpg" | |
} | |
] | |
for user in testimonials: | |
col1, col2 = st.columns([1, 3]) | |
with col1: | |
try: | |
st.image(user["image"], width=100) | |
except: | |
st.write("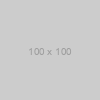") | |
with col2: | |
st.write(f"**{user['name']}**") | |
st.write(f"*{user['position']}*") | |
st.write(f"\"{user['testimonial']}\"") | |
st.write("---") | |
def chatbot_support_page(): | |
st.header("🤖 AI-Powered Chatbot Support") | |
st.write(""" | |
Have questions or need assistance? Chat with our AI-powered assistant! | |
""") | |
# Initialize session state for chatbot | |
if 'chat_history' not in st.session_state: | |
st.session_state['chat_history'] = [] | |
# User input | |
user_input = st.text_input("🗨️ You:", key="user_input") | |
if st.button("Send"): | |
if user_input: | |
# Append user message to chat history | |
st.session_state['chat_history'].append({"message": user_input, "is_user": True}) | |
prompt = f""" | |
You are a helpful assistant for a Job Application Assistant app. Answer the user's query based on the following context: | |
{user_input} | |
""" | |
try: | |
# Invoke the LLM to get a response | |
response = llm.invoke(prompt) | |
assistant_message = response.content.strip() | |
# Append assistant response to chat history | |
st.session_state['chat_history'].append({"message": assistant_message, "is_user": False}) | |
except Exception as e: | |
error_message = "❌ Sorry, I encountered an error while processing your request." | |
st.session_state['chat_history'].append({"message": error_message, "is_user": False}) | |
st.error(f"❌ Error in chatbot: {e}") | |
# Display chat history using streamlit-chat | |
for chat in st.session_state['chat_history']: | |
if chat['is_user']: | |
message(chat['message'], is_user=True, avatar_style="thumbs") | |
else: | |
message(chat['message'], is_user=False, avatar_style="bottts") | |
def help_page(): | |
st.header("❓ Help & FAQ") | |
with st.expander("🛠️ How do I generate a cover letter?"): | |
st.write(""" | |
To generate a cover letter, navigate to the **Cover Letter Generator** section, enter the job link, upload your resume, and click on **Generate Cover Letter**. | |
""") | |
with st.expander("📋 How do I track my applications?"): | |
st.write(""" | |
Use the **Application Tracking Dashboard** to add new applications, update their status, and monitor deadlines. | |
""") | |
with st.expander("📄 How can I optimize my resume?"): | |
st.write(""" | |
Upload your resume in the **Resume Analysis** section to extract skills and receive optimization suggestions. | |
""") | |
with st.expander("📥 How do I import my applications?"): | |
st.write(""" | |
In the **Application Tracking Dashboard**, use the **Import Applications** section to upload a CSV file containing your applications. Ensure the CSV has the required columns. | |
""") | |
with st.expander("🗣️ How do I provide feedback?"): | |
st.write(""" | |
Navigate to the **Feedback and Continuous Improvement** section, fill out the form, and submit your feedback. | |
""") | |
# ------------------------------- | |
# Main App Function | |
# ------------------------------- | |
def main_app(): | |
# Apply a consistent theme or style | |
st.markdown( | |
""" | |
<style> | |
.reportview-container { | |
background-color: #f5f5f5; | |
} | |
.sidebar .sidebar-content { | |
background-image: linear-gradient(#2e7bcf, #2e7bcf); | |
color: white; | |
} | |
</style> | |
""", | |
unsafe_allow_html=True | |
) | |
# Sidebar Navigation using streamlit_option_menu | |
with st.sidebar: | |
selected = option_menu( | |
menu_title="📂 Main Menu", | |
options=["Email Generator", "Cover Letter Generator", "Resume Analysis", "Application Tracking", | |
"Job Recommendations", "Labor Market Insights", "Interview Preparation", "Personalized Learning Paths", | |
"Networking Opportunities", "Feedback", "Gamification", "Resource Library", | |
"Success Stories", "Chatbot Support", "Help"], | |
icons=["envelope", "file-earmark-text", "file-person", "briefcase", | |
"search", "bar-chart-line", "microphone", "book", | |
"people", "chat-left-text", "trophy", "collection", | |
"star", "robot", "question-circle"], | |
menu_icon="cast", | |
default_index=0, | |
styles={ | |
"container": {"padding": "5!important", "background-color": "#2e7bcf"}, | |
"icon": {"color": "white", "font-size": "18px"}, | |
"nav-link": {"font-size": "16px", "text-align": "left", "margin": "0px", "--hover-color": "#6b9eff"}, | |
"nav-link-selected": {"background-color": "#1e5aab"}, | |
} | |
) | |
# Route to the selected page | |
if selected == "Email Generator": | |
email_generator_page() | |
elif selected == "Cover Letter Generator": | |
cover_letter_generator_page() | |
elif selected == "Resume Analysis": | |
resume_analysis_page() | |
elif selected == "Application Tracking": | |
application_tracking_dashboard() | |
elif selected == "Job Recommendations": | |
job_recommendations_module() | |
elif selected == "Labor Market Insights": | |
labor_market_insights_module() | |
elif selected == "Interview Preparation": | |
interview_preparation_module() | |
elif selected == "Personalized Learning Paths": | |
personalized_learning_paths_module() | |
elif selected == "Networking Opportunities": | |
networking_opportunities_module() | |
elif selected == "Feedback": | |
feedback_and_improvement_module() | |
elif selected == "Gamification": | |
gamification_module() | |
elif selected == "Resource Library": | |
resource_library_page() | |
elif selected == "Success Stories": | |
success_stories_page() | |
elif selected == "Chatbot Support": | |
chatbot_support_page() | |
elif selected == "Help": | |
help_page() | |
if __name__ == "__main__": | |
main_app() | |