diff --git a/app.py b/app.py new file mode 100644 index 0000000000000000000000000000000000000000..e8b5d032a2e162c8b0ce26b6be973fd08f6740b3 --- /dev/null +++ b/app.py @@ -0,0 +1,194 @@ +from vits.models import SynthesizerInfer +from omegaconf import OmegaConf +import torchcrepe +import torch +import io +import os +import gradio as gr +import librosa +import numpy as np +import soundfile + +import logging + +logging.getLogger('numba').setLevel(logging.WARNING) +logging.getLogger('markdown_it').setLevel(logging.WARNING) +logging.getLogger('urllib3').setLevel(logging.WARNING) +logging.getLogger('matplotlib').setLevel(logging.WARNING) + + +def load_svc_model(checkpoint_path, model): + assert os.path.isfile(checkpoint_path) + checkpoint_dict = torch.load(checkpoint_path, map_location="cpu") + saved_state_dict = checkpoint_dict["model_g"] + state_dict = model.state_dict() + new_state_dict = {} + for k, v in state_dict.items(): + new_state_dict[k] = saved_state_dict[k] + model.load_state_dict(new_state_dict) + return model + + +def compute_f0_nn(filename, device): + audio, sr = librosa.load(filename, sr=16000) + assert sr == 16000 + # Load audio + audio = torch.tensor(np.copy(audio))[None] + # Here we'll use a 20 millisecond hop length + hop_length = 320 + # Provide a sensible frequency range for your domain (upper limit is 2006 Hz) + # This would be a reasonable range for speech + fmin = 50 + fmax = 1000 + # Select a model capacity--one of "tiny" or "full" + model = "full" + # Pick a batch size that doesn't cause memory errors on your gpu + batch_size = 512 + # Compute pitch using first gpu + pitch, periodicity = torchcrepe.predict( + audio, + sr, + hop_length, + fmin, + fmax, + model, + batch_size=batch_size, + device=device, + return_periodicity=True, + ) + pitch = np.repeat(pitch, 2, -1) # 320 -> 160 * 2 + periodicity = np.repeat(periodicity, 2, -1) # 320 -> 160 * 2 + # CREPE was not trained on silent audio. some error on silent need filter. + periodicity = torchcrepe.filter.median(periodicity, 9) + pitch = torchcrepe.filter.mean(pitch, 9) + pitch[periodicity < 0.1] = 0 + pitch = pitch.squeeze(0) + return pitch + + +device = torch.device("cuda" if torch.cuda.is_available() else "cpu") +hp = OmegaConf.load("configs/base.yaml") +model = SynthesizerInfer( + hp.data.filter_length // 2 + 1, + hp.data.segment_size // hp.data.hop_length, + hp) +load_svc_model("vits_pretrain/sovits5.0-48k-debug.pth", model) +model.eval() +model.to(device) + + +def svc_change(argswave, argsspk): + + argsppg = "svc_tmp.ppg.npy" + os.system(f"python whisper/inference.py -w {argswave} -p {argsppg}") + + spk = np.load(argsspk) + spk = torch.FloatTensor(spk) + + ppg = np.load(argsppg) + ppg = np.repeat(ppg, 2, 0) # 320 PPG -> 160 * 2 + ppg = torch.FloatTensor(ppg) + + pit = compute_f0_nn(argswave, device) + pit = torch.FloatTensor(pit) + + len_pit = pit.size()[0] + len_ppg = ppg.size()[0] + len_min = min(len_pit, len_ppg) + pit = pit[:len_min] + ppg = ppg[:len_min, :] + + with torch.no_grad(): + + spk = spk.unsqueeze(0).to(device) + source = pit.unsqueeze(0).to(device) + source = model.pitch2source(source) + + hop_size = hp.data.hop_length + all_frame = len_min + hop_frame = 10 + out_chunk = 2500 # 25 S + out_index = 0 + out_audio = [] + + while (out_index + out_chunk < all_frame): + if (out_index == 0): # start frame + cut_s = out_index + cut_s_48k = 0 + else: + cut_s = out_index - hop_frame + cut_s_48k = hop_frame * hop_size + + if (out_index + out_chunk + hop_frame > all_frame): # end frame + cut_e = out_index + out_chunk + cut_e_48k = 0 + else: + cut_e = out_index + out_chunk + hop_frame + cut_e_48k = -1 * hop_frame * hop_size + + sub_ppg = ppg[cut_s:cut_e, :].unsqueeze(0).to(device) + sub_pit = pit[cut_s:cut_e].unsqueeze(0).to(device) + sub_len = torch.LongTensor([cut_e - cut_s]).to(device) + sub_har = source[:, :, cut_s * + hop_size:cut_e * hop_size].to(device) + sub_out = model.inference(sub_ppg, sub_pit, spk, sub_len, sub_har) + sub_out = sub_out[0, 0].data.cpu().detach().numpy() + + sub_out = sub_out[cut_s_48k:cut_e_48k] + out_audio.extend(sub_out) + out_index = out_index + out_chunk + + if (out_index < all_frame): + cut_s = out_index - hop_frame + cut_s_48k = hop_frame * hop_size + sub_ppg = ppg[cut_s:, :].unsqueeze(0).to(device) + sub_pit = pit[cut_s:].unsqueeze(0).to(device) + sub_len = torch.LongTensor([all_frame - cut_s]).to(device) + sub_har = source[:, :, cut_s * hop_size:].to(device) + sub_out = model.inference(sub_ppg, sub_pit, spk, sub_len, sub_har) + sub_out = sub_out[0, 0].data.cpu().detach().numpy() + + sub_out = sub_out[cut_s_48k:] + out_audio.extend(sub_out) + out_audio = np.asarray(out_audio) + + return out_audio + + +def svc_main(sid, input_audio): + if input_audio is None: + return "You need to upload an audio", None + sampling_rate, audio = input_audio + audio = (audio / np.iinfo(audio.dtype).max).astype(np.float32) + if len(audio.shape) > 1: + audio = librosa.to_mono(audio.transpose(1, 0)) + if sampling_rate != 16000: + audio = librosa.resample(audio, orig_sr=sampling_rate, target_sr=16000) + if (len(audio) > 16000*100): + audio = audio[:16000*100] + wav_path = "temp.wav" + soundfile.write(wav_path, audio, 16000, format="wav") + out_audio = svc_change(wav_path, f"configs/singers/singer00{sid}.npy") + return "Success", (48000, out_audio) + + +app = gr.Blocks() +with app: + with gr.Tabs(): + with gr.TabItem("sovits 5.0"): + gr.Markdown(value=""" + 基于开源数据:Multi-Singer + + https://github.com/Multi-Singer/Multi-Singer.github.io + + [轻度伴奏可以无需去伴奏]就能直接进行歌声转换的SVC库 + """) + sid = gr.Dropdown(label="音色", choices=[ + "22", "33", "47", "51"], value="47") + vc_input3 = gr.Audio(label="上传音频") + vc_submit = gr.Button("转换", variant="primary") + vc_output1 = gr.Textbox(label="Output Message") + vc_output2 = gr.Audio(label="准换后音频") + vc_submit.click(svc_main, [sid, vc_input3], [vc_output1, vc_output2]) + + app.launch() diff --git a/configs/base.yaml b/configs/base.yaml new file mode 100644 index 0000000000000000000000000000000000000000..0c57681508bbd98d5df6c6d866d2825018fb7280 --- /dev/null +++ b/configs/base.yaml @@ -0,0 +1,69 @@ +train: + model: "sovits" + seed: 1234 + epochs: 10000 + learning_rate: 2e-4 + betas: [0.8, 0.99] + lr_decay: 0.999875 + eps: 1e-9 + batch_size: 8 + c_stft: 5 + c_mel: 2.5 + c_kl: 1.0 + port: 8001 + pretrain: "" +############################# +data: + training_files: "files/train.txt" + validation_files: "files/valid.txt" + segment_size: 12000 # WARNING: base on hop_length + max_wav_value: 32768.0 + sampling_rate: 48000 + filter_length: 2048 + hop_length: 480 + win_length: 2048 + mel_channels: 80 + mel_fmin: 0.0 + mel_fmax: 24000.0 +############################# +vits: + ppg_dim: 1024 + spk_dim: 256 + gin_channels: 256 + inter_channels: 192 + hidden_channels: 192 + filter_channels: 512 +############################# +gen: + upsample_input: 192 + upsample_rates: [6,5,4,2,2] + upsample_kernel_sizes: [20,15,8,4,4] + upsample_initial_channel: 256 + resblock_kernel_sizes: [3,7,11] + resblock_dilation_sizes: [[1,3,5], [1,3,5], [1,3,5]] +############################# +mpd: + periods: [2,3,5,7,11] + kernel_size: 5 + stride: 3 + use_spectral_norm: False + lReLU_slope: 0.2 +############################# +mrd: + resolutions: "[(1024, 120, 600), (2048, 240, 1200), (512, 50, 240)]" # (filter_length, hop_length, win_length) + use_spectral_norm: False + lReLU_slope: 0.2 +############################# +log: + info_interval: 100 + eval_interval: 5 + save_interval: 5 + num_audio: 6 + pth_dir: 'chkpt' + log_dir: 'logs' +############################# +dist_config: + dist_backend: "nccl" + dist_url: "tcp://localhost:54321" + world_size: 1 + diff --git a/configs/singers/singer0001.npy b/configs/singers/singer0001.npy new file mode 100644 index 0000000000000000000000000000000000000000..9352a330a6ac78e14129c5062d2235c05b15668c --- /dev/null +++ b/configs/singers/singer0001.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:e2879921d43bdbf11fc5d6ac91f434f905a2c5e59d75368bfbf3c6bdbddcb3cf +size 1152 diff --git a/configs/singers/singer0002.npy b/configs/singers/singer0002.npy new file mode 100644 index 0000000000000000000000000000000000000000..b8ccb3f218758254f2971a3dbeaa5340e7377c7f --- /dev/null +++ b/configs/singers/singer0002.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:fbe5c7925c2fdb514e2c5b450de1d2737ec7f86f1c65eeb488c1888c0b9a7069 +size 1152 diff --git a/configs/singers/singer0003.npy b/configs/singers/singer0003.npy new file mode 100644 index 0000000000000000000000000000000000000000..3a92f50cbd7336703910831f03ac7d1cb029a90e --- /dev/null +++ b/configs/singers/singer0003.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:5665126aeb6c6fab89c79b90debf2ce2e64b321076dcb414089eff8848eac8b4 +size 1152 diff --git a/configs/singers/singer0004.npy b/configs/singers/singer0004.npy new file mode 100644 index 0000000000000000000000000000000000000000..6ef48a0a0cb042ff8c419f747261c579aa66520e --- /dev/null +++ b/configs/singers/singer0004.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:79f0fe5993e9adcaeae25b0fa68265d40c9c1b5539ca12d6e438477de2177819 +size 1152 diff --git a/configs/singers/singer0005.npy b/configs/singers/singer0005.npy new file mode 100644 index 0000000000000000000000000000000000000000..ebe4251d2aef83c2c9db470bec9cdff8cf97e769 --- /dev/null +++ b/configs/singers/singer0005.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:1158fb447929cf9400a31675cf9992fd3ed7558e061562189d9e6bf56d83fb2a +size 1152 diff --git a/configs/singers/singer0006.npy b/configs/singers/singer0006.npy new file mode 100644 index 0000000000000000000000000000000000000000..6336c044c3b98765c5bc1f9121ef36465bcaf79e --- /dev/null +++ b/configs/singers/singer0006.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:06c1fd3a9afaa7944e4b81b7ca787e667b0dae8c7e90c6d24177245449f4e940 +size 1152 diff --git a/configs/singers/singer0007.npy b/configs/singers/singer0007.npy new file mode 100644 index 0000000000000000000000000000000000000000..b401dcf6e4f774798010ccbf27cd8622943e7174 --- /dev/null +++ b/configs/singers/singer0007.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:36611b9e57545332b9fb97fd35a356fbe8d60258f2f5e2232168481bb6dfab5b +size 1152 diff --git a/configs/singers/singer0008.npy b/configs/singers/singer0008.npy new file mode 100644 index 0000000000000000000000000000000000000000..f28df113963e42afba4d76af39ec150a66de406c --- /dev/null +++ b/configs/singers/singer0008.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:8584ad6f3569a1307082cd410085d9a562807e962274b89b72487c7bc79124d4 +size 1152 diff --git a/configs/singers/singer0009.npy b/configs/singers/singer0009.npy new file mode 100644 index 0000000000000000000000000000000000000000..15d125808f4475d4b9e9a2714839b61f36c2ae1c --- /dev/null +++ b/configs/singers/singer0009.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:b069db4e3e5ca389ffba974c74eab46caf4c60545773e5f7e5e253310619073e +size 1152 diff --git a/configs/singers/singer0010.npy b/configs/singers/singer0010.npy new file mode 100644 index 0000000000000000000000000000000000000000..bda76fe1913f4310bb2dab2a3eb411454aac8d12 --- /dev/null +++ b/configs/singers/singer0010.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:7d4d92735e4bac1618e89198d113013db09061b6c1f74ba0c500b70b097cd407 +size 1152 diff --git a/configs/singers/singer0011.npy b/configs/singers/singer0011.npy new file mode 100644 index 0000000000000000000000000000000000000000..0fd56c80b7fc42933a3c1b2a0c37e2e7291e44f8 --- /dev/null +++ b/configs/singers/singer0011.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:942388b4276dc06ee365f59c324ce1642e4bf810dcc99992739787e3b9ad135d +size 1152 diff --git a/configs/singers/singer0012.npy b/configs/singers/singer0012.npy new file mode 100644 index 0000000000000000000000000000000000000000..54261d088430996e5a0abf019c47632031bb8886 --- /dev/null +++ b/configs/singers/singer0012.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:3411efcf4ee4f534cea2b742c2eca166ae971efbceab21fb41b77b8923a1ba3a +size 1152 diff --git a/configs/singers/singer0013.npy b/configs/singers/singer0013.npy new file mode 100644 index 0000000000000000000000000000000000000000..3eedaf46d7a1fe7865d7c2783375e0f4a010f154 --- /dev/null +++ b/configs/singers/singer0013.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:6e8e30cd1bce61405db194278dd7bf207d16abf656dd22f9a20f29e3657674f3 +size 1152 diff --git a/configs/singers/singer0014.npy b/configs/singers/singer0014.npy new file mode 100644 index 0000000000000000000000000000000000000000..602e8f6203eb05962b3102f319e0ec99db9c097b --- /dev/null +++ b/configs/singers/singer0014.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:f9cc8200753b4ba7605c9a13bf454b100025965135c5d816f7440ec53a2e6dd4 +size 1152 diff --git a/configs/singers/singer0015.npy b/configs/singers/singer0015.npy new file mode 100644 index 0000000000000000000000000000000000000000..dfe824316e9fa153390c87f5d1fd1d4b34caab3b --- /dev/null +++ b/configs/singers/singer0015.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:dcb58688e51dbdeb22e5dd85d27ff3904c4594c78420b8e9c9ab481adbecc5fe +size 1152 diff --git a/configs/singers/singer0016.npy b/configs/singers/singer0016.npy new file mode 100644 index 0000000000000000000000000000000000000000..5ce37e18e3b70a9c149e29743f227b5fd4cfdffd --- /dev/null +++ b/configs/singers/singer0016.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:66a3c6162b8c937e9e8bbdc806b873866afce4b110664831642f7b41922bbf39 +size 1152 diff --git a/configs/singers/singer0017.npy b/configs/singers/singer0017.npy new file mode 100644 index 0000000000000000000000000000000000000000..4104cb371c4e57ca071ec299e39e7320cc3e4569 --- /dev/null +++ b/configs/singers/singer0017.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:84782c98c930bd980f350837f4b3e8e193c49ef46aef9f92471c6136659975a9 +size 1152 diff --git a/configs/singers/singer0018.npy b/configs/singers/singer0018.npy new file mode 100644 index 0000000000000000000000000000000000000000..fc43cc1750632008cc8057ce24b39e759bb3a047 --- /dev/null +++ b/configs/singers/singer0018.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:731ebafda06aecedfd79941978149a0f87595f04e24eab7ed5300defe9070fc0 +size 1152 diff --git a/configs/singers/singer0019.npy b/configs/singers/singer0019.npy new file mode 100644 index 0000000000000000000000000000000000000000..5e32ca3dca1e975ba5bae37b730fb3a764fc8595 --- /dev/null +++ b/configs/singers/singer0019.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:3d88e620994e4413c4c58ffb9239ef46ded60ff3eab0715c7af96cbe4092198f +size 1152 diff --git a/configs/singers/singer0020.npy b/configs/singers/singer0020.npy new file mode 100644 index 0000000000000000000000000000000000000000..88a0e64f47ac03688db8b45329f4de9554f86835 --- /dev/null +++ b/configs/singers/singer0020.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:3e5abaabe5457a20161351dcf5f8737d63a2a92fb1de1842ea9e92e47b9ca6fe +size 1152 diff --git a/configs/singers/singer0021.npy b/configs/singers/singer0021.npy new file mode 100644 index 0000000000000000000000000000000000000000..d80f97eac1be1779490a37a503aaac6ac1f5d130 --- /dev/null +++ b/configs/singers/singer0021.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:1d7f99c92c89a44c1f2dd0688f033f0593c8c88b0537b092928bfbaa63a8d3e9 +size 1152 diff --git a/configs/singers/singer0022.npy b/configs/singers/singer0022.npy new file mode 100644 index 0000000000000000000000000000000000000000..64dbba2610e35274f13da90702f608f283ddb0f4 --- /dev/null +++ b/configs/singers/singer0022.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:33becb1da48b12ba4957a0ef0b25bbd51e100d5762ebc4c7d381f6b957e682a2 +size 1152 diff --git a/configs/singers/singer0023.npy b/configs/singers/singer0023.npy new file mode 100644 index 0000000000000000000000000000000000000000..6cb1c218971618be8de47f9c8daec658d4578531 --- /dev/null +++ b/configs/singers/singer0023.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:7f49cbaf3f7653f48f80854a513a334f31dca719a09cca66e257995ce4a741a9 +size 1152 diff --git a/configs/singers/singer0024.npy b/configs/singers/singer0024.npy new file mode 100644 index 0000000000000000000000000000000000000000..0ca92912b8959fc8743b9628f08c7595b6eb94f9 --- /dev/null +++ b/configs/singers/singer0024.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:92ed584994d56473c8bab0799d213e927c5a2928facef2b93a2f95f764d868b4 +size 1152 diff --git a/configs/singers/singer0025.npy b/configs/singers/singer0025.npy new file mode 100644 index 0000000000000000000000000000000000000000..05bd93acfad034e7e83e7965c4ce4557a4b7a277 --- /dev/null +++ b/configs/singers/singer0025.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:14b7e1f55393d5beaa2f3bbd0ef7f2be7e108993c680acb265ff24df19f7062b +size 1152 diff --git a/configs/singers/singer0026.npy b/configs/singers/singer0026.npy new file mode 100644 index 0000000000000000000000000000000000000000..cddbd2fddc6a51caf1a2f111f3bd6b1d3dbe2c18 --- /dev/null +++ b/configs/singers/singer0026.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:92ecc9aa68f136960c00e98aaca16e92c38960bc7eb9687aee90190972974726 +size 1152 diff --git a/configs/singers/singer0027.npy b/configs/singers/singer0027.npy new file mode 100644 index 0000000000000000000000000000000000000000..aedcbf0c48465cb3273ba46d180711901ca911ea --- /dev/null +++ b/configs/singers/singer0027.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:f5a8a1c2a445179d38664fb55c84ee9a36350beee50efa9f850d29b394447bfa +size 1152 diff --git a/configs/singers/singer0028.npy b/configs/singers/singer0028.npy new file mode 100644 index 0000000000000000000000000000000000000000..788e6fdeb897960c0b1e203f9750cd2ae3969975 --- /dev/null +++ b/configs/singers/singer0028.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:b79b8266c8d368dc99f49a347b2631e1e5cfb44056b5a9ab4470b42f9851ee35 +size 1152 diff --git a/configs/singers/singer0029.npy b/configs/singers/singer0029.npy new file mode 100644 index 0000000000000000000000000000000000000000..0340a7adc7bde1ada08e8e1962ce94c6f72a9d2f --- /dev/null +++ b/configs/singers/singer0029.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:60fa5fd9e8ba14d7f6d67304842f16382f7d2e739969bde9551222ff8c282775 +size 1152 diff --git a/configs/singers/singer0030.npy b/configs/singers/singer0030.npy new file mode 100644 index 0000000000000000000000000000000000000000..3597a4cf4e540f6aaae2f5f0ecd6f21d52a15658 --- /dev/null +++ b/configs/singers/singer0030.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:2f5070e4196c91fa713aed20aedb2a570a7b2ad8301ee61f59821dafaea3c6a7 +size 1152 diff --git a/configs/singers/singer0031.npy b/configs/singers/singer0031.npy new file mode 100644 index 0000000000000000000000000000000000000000..73be80545df0ca0a007ce914569e088082db62d8 --- /dev/null +++ b/configs/singers/singer0031.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:47f4f8c065be1c5448c1b80e5c99087e7357cf1f8a8a55f2d844ccf1ca4931e6 +size 1152 diff --git a/configs/singers/singer0032.npy b/configs/singers/singer0032.npy new file mode 100644 index 0000000000000000000000000000000000000000..09d9d322a3232c0ab60cd05ea0257534b05e3c35 --- /dev/null +++ b/configs/singers/singer0032.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:019f40cf49cb7ccb44fb9c6a9f6345e84f837185a1642623144b4e2969c8738b +size 1152 diff --git a/configs/singers/singer0033.npy b/configs/singers/singer0033.npy new file mode 100644 index 0000000000000000000000000000000000000000..a6efd8966cb9e1f2ed87cbc103cdb70ceb279213 --- /dev/null +++ b/configs/singers/singer0033.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:2e05e212c93fc9e7b13174dd76721ee891bb4ea8bb1638a4c43523ed65d30f67 +size 1152 diff --git a/configs/singers/singer0034.npy b/configs/singers/singer0034.npy new file mode 100644 index 0000000000000000000000000000000000000000..1c23504832579cc0c9c3a7b505a7d6b8cc1efd81 --- /dev/null +++ b/configs/singers/singer0034.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:715a089dd9b3e5cbf021b0f41055f59208911e49cccf375ecf8b82544f325c3d +size 1152 diff --git a/configs/singers/singer0035.npy b/configs/singers/singer0035.npy new file mode 100644 index 0000000000000000000000000000000000000000..894595cd6678a1befdfb843a02ee09ad7badc03c --- /dev/null +++ b/configs/singers/singer0035.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:9af8cd05182ec53ff573bce53dad049759bea1de5656915f414910eaf47f61ed +size 1152 diff --git a/configs/singers/singer0036.npy b/configs/singers/singer0036.npy new file mode 100644 index 0000000000000000000000000000000000000000..de86320c15d7e9e3162edc9eadb783fb306b79c3 --- /dev/null +++ b/configs/singers/singer0036.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:3cec474244d86acfd24d6abf7e033b24b40b838cba2fcd3b4d0e5611313d67ef +size 1152 diff --git a/configs/singers/singer0037.npy b/configs/singers/singer0037.npy new file mode 100644 index 0000000000000000000000000000000000000000..36488b5b3c83fca4e3617aaefe5138002c150cd9 --- /dev/null +++ b/configs/singers/singer0037.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:316e3435d373e352fe95fcb2ec0ab1c8afdeb270ce9f13c940ba91187eecdcf3 +size 1152 diff --git a/configs/singers/singer0038.npy b/configs/singers/singer0038.npy new file mode 100644 index 0000000000000000000000000000000000000000..9c234763efb8227d82a4770dfc0b5b885d124f13 --- /dev/null +++ b/configs/singers/singer0038.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:0e6458e251512dab86abce504490de6762f9c2de66ddbc853c24c3d05eb39c96 +size 1152 diff --git a/configs/singers/singer0039.npy b/configs/singers/singer0039.npy new file mode 100644 index 0000000000000000000000000000000000000000..64b2bc8072f901df785c32d47b5926e98179ee50 --- /dev/null +++ b/configs/singers/singer0039.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:c2e484ae33eef7ac92dd784e9e3b9bca7e6c0838d50b43c674da47620f281f20 +size 1152 diff --git a/configs/singers/singer0040.npy b/configs/singers/singer0040.npy new file mode 100644 index 0000000000000000000000000000000000000000..96dd086113c61fac151de03b22a12daf768d7f41 --- /dev/null +++ b/configs/singers/singer0040.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:7b3a104163ad4cf87caff70b845b2c3e70190ce430a8f21247d350ef102071dc +size 1152 diff --git a/configs/singers/singer0041.npy b/configs/singers/singer0041.npy new file mode 100644 index 0000000000000000000000000000000000000000..265f3dc086d595a0675e38fd35f5c433cc3dbcef --- /dev/null +++ b/configs/singers/singer0041.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:962ba35045f952562bbf68239c8abfda4e1888118fae7ef19814282abee2d28e +size 1152 diff --git a/configs/singers/singer0042.npy b/configs/singers/singer0042.npy new file mode 100644 index 0000000000000000000000000000000000000000..7b13c99f9ef87b7e64bcdac1216f772239946b4f --- /dev/null +++ b/configs/singers/singer0042.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:0cf0871ba7e939c90f2f027862f80e11151d8b1a21b6624ee05f184d024b35a3 +size 1152 diff --git a/configs/singers/singer0043.npy b/configs/singers/singer0043.npy new file mode 100644 index 0000000000000000000000000000000000000000..11b3e4a998bb8eb219aa7b2bbfbd36234293e6e4 --- /dev/null +++ b/configs/singers/singer0043.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:9494ed20a9b095d19cce17619a6372ba3371f980c643078ffda8649a30ac2f8b +size 1152 diff --git a/configs/singers/singer0044.npy b/configs/singers/singer0044.npy new file mode 100644 index 0000000000000000000000000000000000000000..a12211417bf2d237b0c164c2d275ed95bd3ff175 --- /dev/null +++ b/configs/singers/singer0044.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:c12949caa6176fbe5f323cf643d29eef14af9a3ee03be27c938d8bb6fc2922f1 +size 1152 diff --git a/configs/singers/singer0045.npy b/configs/singers/singer0045.npy new file mode 100644 index 0000000000000000000000000000000000000000..04962d6066594759e2fd3ec9d69687f6179d8e74 --- /dev/null +++ b/configs/singers/singer0045.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:222adf210792d1b2745ef98b717f57e0d309d8176e9b59ff56063c1e2001728d +size 1152 diff --git a/configs/singers/singer0046.npy b/configs/singers/singer0046.npy new file mode 100644 index 0000000000000000000000000000000000000000..74976cf00a14a550f4189100409ba940e5f81c7c --- /dev/null +++ b/configs/singers/singer0046.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:6070f66f028928114493a363e4117636afefb1a094c54ffc01f89ef261ad1882 +size 1152 diff --git a/configs/singers/singer0047.npy b/configs/singers/singer0047.npy new file mode 100644 index 0000000000000000000000000000000000000000..50304b9bb81a71beb480c2ce786a2c8ff0aa8db5 --- /dev/null +++ b/configs/singers/singer0047.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:7c8bb2fb993a55d13996df74463408c8e7e8d5e24b391887813e2ac1c204c9c4 +size 1152 diff --git a/configs/singers/singer0048.npy b/configs/singers/singer0048.npy new file mode 100644 index 0000000000000000000000000000000000000000..71f0fbde409976dbc079d27b957d269d3dd59129 --- /dev/null +++ b/configs/singers/singer0048.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:5b33ee26125ae840494dc2cb3839f7a2f6b48571c15ebc7f0aa9f2b0fef5022e +size 1152 diff --git a/configs/singers/singer0049.npy b/configs/singers/singer0049.npy new file mode 100644 index 0000000000000000000000000000000000000000..00eb5f5b705f1236aebc44ccc40d87fe071e12b0 --- /dev/null +++ b/configs/singers/singer0049.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:e9a8d97dd4d320e4049c39e112587416d06aa70ec52c05417519bac70fe76556 +size 1152 diff --git a/configs/singers/singer0050.npy b/configs/singers/singer0050.npy new file mode 100644 index 0000000000000000000000000000000000000000..23f9815b6ed783ba964a8bed34e1a7353fd3873c --- /dev/null +++ b/configs/singers/singer0050.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:8fc8cf73923c6a567a134bffa037b3c9d1dcfde75d5a976238df222d91517d9f +size 1152 diff --git a/configs/singers/singer0051.npy b/configs/singers/singer0051.npy new file mode 100644 index 0000000000000000000000000000000000000000..1ffac1a5057ba9bd5b8dc77ab4d8ddb1f02c8333 --- /dev/null +++ b/configs/singers/singer0051.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:a142fdcdb2fa1e69d09df6de3f96bf863038da4e27f51320adb5483cb4f5d306 +size 1152 diff --git a/configs/singers/singer0052.npy b/configs/singers/singer0052.npy new file mode 100644 index 0000000000000000000000000000000000000000..ce1c360dd3c195b30157c33bb49138d55b72c586 --- /dev/null +++ b/configs/singers/singer0052.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:a2fb5dcbe59a636f84c9522a3278b601358584fdc340dff8db06eaa544fddd4b +size 1152 diff --git a/configs/singers/singer0053.npy b/configs/singers/singer0053.npy new file mode 100644 index 0000000000000000000000000000000000000000..e2328ccf8b490bf37b622bf663292cd0c32f92b8 --- /dev/null +++ b/configs/singers/singer0053.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:893f50a74fdf3e7f27debc52fd739cd96a147ae2dcbeb34e2fb8fd328fa698a5 +size 1152 diff --git a/configs/singers/singer0054.npy b/configs/singers/singer0054.npy new file mode 100644 index 0000000000000000000000000000000000000000..aa47e0a5d03bc259e93ce2fe0cb8cef9ddc01a2b --- /dev/null +++ b/configs/singers/singer0054.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:151b0206dd960b1304f8d752e0502e9c7b0260326f7b932b278773aa0c5bb3ef +size 1152 diff --git a/configs/singers/singer0055.npy b/configs/singers/singer0055.npy new file mode 100644 index 0000000000000000000000000000000000000000..944ff6e36130ab3aaa2aea7f89edc4a50fff960d --- /dev/null +++ b/configs/singers/singer0055.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:aa92ab16df7f82f5bde66501629310a5a250ff61d5f467a7ff2b0c97fe6d8066 +size 1152 diff --git a/configs/singers/singer0056.npy b/configs/singers/singer0056.npy new file mode 100644 index 0000000000000000000000000000000000000000..79f339eb5ef8319b5410749e19a5c647d7c2e81f --- /dev/null +++ b/configs/singers/singer0056.npy @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:07022464dcb678dddd3957ea431e53b4c79fd1904927d2622faef5c521da1b5e +size 1152 diff --git a/requirements.txt b/requirements.txt new file mode 100644 index 0000000000000000000000000000000000000000..992de39d92daf3b117a270ccb512161a5aa77a01 --- /dev/null +++ b/requirements.txt @@ -0,0 +1,15 @@ +ffmpeg +ffmpeg-python +omegaconf +praat-parselmouth +pyffmpeg +scikit-learn +scipy +soundfile +toolz +torch +torchaudio +torchcrepe +transformers +tqdm +librosa diff --git a/vits/LICENSE b/vits/LICENSE new file mode 100644 index 0000000000000000000000000000000000000000..6a6c3181fcdc4e20901a6ecbee5a406b78a5b560 --- /dev/null +++ b/vits/LICENSE @@ -0,0 +1,21 @@ +MIT License + +Copyright (c) 2021 Jaehyeon Kim + +Permission is hereby granted, free of charge, to any person obtaining a copy +of this software and associated documentation files (the "Software"), to deal +in the Software without restriction, including without limitation the rights +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell +copies of the Software, and to permit persons to whom the Software is +furnished to do so, subject to the following conditions: + +The above copyright notice and this permission notice shall be included in all +copies or substantial portions of the Software. + +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE +SOFTWARE. diff --git a/vits/__init__.py b/vits/__init__.py new file mode 100644 index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391 diff --git a/vits/attentions.py b/vits/attentions.py new file mode 100644 index 0000000000000000000000000000000000000000..26624519b01497cc402dea5f860cf5022d1e7c89 --- /dev/null +++ b/vits/attentions.py @@ -0,0 +1,416 @@ +import copy +import math +import numpy as np +import torch +from torch import nn +from torch.nn import functional as F + +from vits import commons +from vits.modules import LayerNorm + + +class Encoder(nn.Module): + def __init__( + self, + hidden_channels, + filter_channels, + n_heads, + n_layers, + kernel_size=1, + p_dropout=0.0, + window_size=4, + **kwargs + ): + super().__init__() + self.hidden_channels = hidden_channels + self.filter_channels = filter_channels + self.n_heads = n_heads + self.n_layers = n_layers + self.kernel_size = kernel_size + self.p_dropout = p_dropout + self.window_size = window_size + + self.drop = nn.Dropout(p_dropout) + self.attn_layers = nn.ModuleList() + self.norm_layers_1 = nn.ModuleList() + self.ffn_layers = nn.ModuleList() + self.norm_layers_2 = nn.ModuleList() + for i in range(self.n_layers): + self.attn_layers.append( + MultiHeadAttention( + hidden_channels, + hidden_channels, + n_heads, + p_dropout=p_dropout, + window_size=window_size, + ) + ) + self.norm_layers_1.append(LayerNorm(hidden_channels)) + self.ffn_layers.append( + FFN( + hidden_channels, + hidden_channels, + filter_channels, + kernel_size, + p_dropout=p_dropout, + ) + ) + self.norm_layers_2.append(LayerNorm(hidden_channels)) + + def forward(self, x, x_mask): + attn_mask = x_mask.unsqueeze(2) * x_mask.unsqueeze(-1) + x = x * x_mask + for i in range(self.n_layers): + y = self.attn_layers[i](x, x, attn_mask) + y = self.drop(y) + x = self.norm_layers_1[i](x + y) + + y = self.ffn_layers[i](x, x_mask) + y = self.drop(y) + x = self.norm_layers_2[i](x + y) + x = x * x_mask + return x + + +class Decoder(nn.Module): + def __init__( + self, + hidden_channels, + filter_channels, + n_heads, + n_layers, + kernel_size=1, + p_dropout=0.0, + proximal_bias=False, + proximal_init=True, + **kwargs + ): + super().__init__() + self.hidden_channels = hidden_channels + self.filter_channels = filter_channels + self.n_heads = n_heads + self.n_layers = n_layers + self.kernel_size = kernel_size + self.p_dropout = p_dropout + self.proximal_bias = proximal_bias + self.proximal_init = proximal_init + + self.drop = nn.Dropout(p_dropout) + self.self_attn_layers = nn.ModuleList() + self.norm_layers_0 = nn.ModuleList() + self.encdec_attn_layers = nn.ModuleList() + self.norm_layers_1 = nn.ModuleList() + self.ffn_layers = nn.ModuleList() + self.norm_layers_2 = nn.ModuleList() + for i in range(self.n_layers): + self.self_attn_layers.append( + MultiHeadAttention( + hidden_channels, + hidden_channels, + n_heads, + p_dropout=p_dropout, + proximal_bias=proximal_bias, + proximal_init=proximal_init, + ) + ) + self.norm_layers_0.append(LayerNorm(hidden_channels)) + self.encdec_attn_layers.append( + MultiHeadAttention( + hidden_channels, hidden_channels, n_heads, p_dropout=p_dropout + ) + ) + self.norm_layers_1.append(LayerNorm(hidden_channels)) + self.ffn_layers.append( + FFN( + hidden_channels, + hidden_channels, + filter_channels, + kernel_size, + p_dropout=p_dropout, + causal=True, + ) + ) + self.norm_layers_2.append(LayerNorm(hidden_channels)) + + def forward(self, x, x_mask, h, h_mask): + """ + x: decoder input + h: encoder output + """ + self_attn_mask = commons.subsequent_mask(x_mask.size(2)).to( + device=x.device, dtype=x.dtype + ) + encdec_attn_mask = h_mask.unsqueeze(2) * x_mask.unsqueeze(-1) + x = x * x_mask + for i in range(self.n_layers): + y = self.self_attn_layers[i](x, x, self_attn_mask) + y = self.drop(y) + x = self.norm_layers_0[i](x + y) + + y = self.encdec_attn_layers[i](x, h, encdec_attn_mask) + y = self.drop(y) + x = self.norm_layers_1[i](x + y) + + y = self.ffn_layers[i](x, x_mask) + y = self.drop(y) + x = self.norm_layers_2[i](x + y) + x = x * x_mask + return x + + +class MultiHeadAttention(nn.Module): + def __init__( + self, + channels, + out_channels, + n_heads, + p_dropout=0.0, + window_size=None, + heads_share=True, + block_length=None, + proximal_bias=False, + proximal_init=False, + ): + super().__init__() + assert channels % n_heads == 0 + + self.channels = channels + self.out_channels = out_channels + self.n_heads = n_heads + self.p_dropout = p_dropout + self.window_size = window_size + self.heads_share = heads_share + self.block_length = block_length + self.proximal_bias = proximal_bias + self.proximal_init = proximal_init + self.attn = None + + self.k_channels = channels // n_heads + self.conv_q = nn.Conv1d(channels, channels, 1) + self.conv_k = nn.Conv1d(channels, channels, 1) + self.conv_v = nn.Conv1d(channels, channels, 1) + self.conv_o = nn.Conv1d(channels, out_channels, 1) + self.drop = nn.Dropout(p_dropout) + + if window_size is not None: + n_heads_rel = 1 if heads_share else n_heads + rel_stddev = self.k_channels**-0.5 + self.emb_rel_k = nn.Parameter( + torch.randn(n_heads_rel, window_size * 2 + 1, self.k_channels) + * rel_stddev + ) + self.emb_rel_v = nn.Parameter( + torch.randn(n_heads_rel, window_size * 2 + 1, self.k_channels) + * rel_stddev + ) + + nn.init.xavier_uniform_(self.conv_q.weight) + nn.init.xavier_uniform_(self.conv_k.weight) + nn.init.xavier_uniform_(self.conv_v.weight) + if proximal_init: + with torch.no_grad(): + self.conv_k.weight.copy_(self.conv_q.weight) + self.conv_k.bias.copy_(self.conv_q.bias) + + def forward(self, x, c, attn_mask=None): + q = self.conv_q(x) + k = self.conv_k(c) + v = self.conv_v(c) + + x, self.attn = self.attention(q, k, v, mask=attn_mask) + + x = self.conv_o(x) + return x + + def attention(self, query, key, value, mask=None): + # reshape [b, d, t] -> [b, n_h, t, d_k] + b, d, t_s, t_t = (*key.size(), query.size(2)) + query = query.view(b, self.n_heads, self.k_channels, t_t).transpose(2, 3) + key = key.view(b, self.n_heads, self.k_channels, t_s).transpose(2, 3) + value = value.view(b, self.n_heads, self.k_channels, t_s).transpose(2, 3) + + scores = torch.matmul(query / math.sqrt(self.k_channels), key.transpose(-2, -1)) + if self.window_size is not None: + assert ( + t_s == t_t + ), "Relative attention is only available for self-attention." + key_relative_embeddings = self._get_relative_embeddings(self.emb_rel_k, t_s) + rel_logits = self._matmul_with_relative_keys( + query / math.sqrt(self.k_channels), key_relative_embeddings + ) + scores_local = self._relative_position_to_absolute_position(rel_logits) + scores = scores + scores_local + if self.proximal_bias: + assert t_s == t_t, "Proximal bias is only available for self-attention." + scores = scores + self._attention_bias_proximal(t_s).to( + device=scores.device, dtype=scores.dtype + ) + if mask is not None: + scores = scores.masked_fill(mask == 0, -1e4) + if self.block_length is not None: + assert ( + t_s == t_t + ), "Local attention is only available for self-attention." + block_mask = ( + torch.ones_like(scores) + .triu(-self.block_length) + .tril(self.block_length) + ) + scores = scores.masked_fill(block_mask == 0, -1e4) + p_attn = F.softmax(scores, dim=-1) # [b, n_h, t_t, t_s] + p_attn = self.drop(p_attn) + output = torch.matmul(p_attn, value) + if self.window_size is not None: + relative_weights = self._absolute_position_to_relative_position(p_attn) + value_relative_embeddings = self._get_relative_embeddings( + self.emb_rel_v, t_s + ) + output = output + self._matmul_with_relative_values( + relative_weights, value_relative_embeddings + ) + output = ( + output.transpose(2, 3).contiguous().view(b, d, t_t) + ) # [b, n_h, t_t, d_k] -> [b, d, t_t] + return output, p_attn + + def _matmul_with_relative_values(self, x, y): + """ + x: [b, h, l, m] + y: [h or 1, m, d] + ret: [b, h, l, d] + """ + ret = torch.matmul(x, y.unsqueeze(0)) + return ret + + def _matmul_with_relative_keys(self, x, y): + """ + x: [b, h, l, d] + y: [h or 1, m, d] + ret: [b, h, l, m] + """ + ret = torch.matmul(x, y.unsqueeze(0).transpose(-2, -1)) + return ret + + def _get_relative_embeddings(self, relative_embeddings, length): + max_relative_position = 2 * self.window_size + 1 + # Pad first before slice to avoid using cond ops. + pad_length = max(length - (self.window_size + 1), 0) + slice_start_position = max((self.window_size + 1) - length, 0) + slice_end_position = slice_start_position + 2 * length - 1 + if pad_length > 0: + padded_relative_embeddings = F.pad( + relative_embeddings, + commons.convert_pad_shape([[0, 0], [pad_length, pad_length], [0, 0]]), + ) + else: + padded_relative_embeddings = relative_embeddings + used_relative_embeddings = padded_relative_embeddings[ + :, slice_start_position:slice_end_position + ] + return used_relative_embeddings + + def _relative_position_to_absolute_position(self, x): + """ + x: [b, h, l, 2*l-1] + ret: [b, h, l, l] + """ + batch, heads, length, _ = x.size() + # Concat columns of pad to shift from relative to absolute indexing. + x = F.pad(x, commons.convert_pad_shape([[0, 0], [0, 0], [0, 0], [0, 1]])) + + # Concat extra elements so to add up to shape (len+1, 2*len-1). + x_flat = x.view([batch, heads, length * 2 * length]) + x_flat = F.pad( + x_flat, commons.convert_pad_shape([[0, 0], [0, 0], [0, length - 1]]) + ) + + # Reshape and slice out the padded elements. + x_final = x_flat.view([batch, heads, length + 1, 2 * length - 1])[ + :, :, :length, length - 1 : + ] + return x_final + + def _absolute_position_to_relative_position(self, x): + """ + x: [b, h, l, l] + ret: [b, h, l, 2*l-1] + """ + batch, heads, length, _ = x.size() + # padd along column + x = F.pad( + x, commons.convert_pad_shape([[0, 0], [0, 0], [0, 0], [0, length - 1]]) + ) + x_flat = x.view([batch, heads, length**2 + length * (length - 1)]) + # add 0's in the beginning that will skew the elements after reshape + x_flat = F.pad(x_flat, commons.convert_pad_shape([[0, 0], [0, 0], [length, 0]])) + x_final = x_flat.view([batch, heads, length, 2 * length])[:, :, :, 1:] + return x_final + + def _attention_bias_proximal(self, length): + """Bias for self-attention to encourage attention to close positions. + Args: + length: an integer scalar. + Returns: + a Tensor with shape [1, 1, length, length] + """ + r = torch.arange(length, dtype=torch.float32) + diff = torch.unsqueeze(r, 0) - torch.unsqueeze(r, 1) + return torch.unsqueeze(torch.unsqueeze(-torch.log1p(torch.abs(diff)), 0), 0) + + +class FFN(nn.Module): + def __init__( + self, + in_channels, + out_channels, + filter_channels, + kernel_size, + p_dropout=0.0, + activation=None, + causal=False, + ): + super().__init__() + self.in_channels = in_channels + self.out_channels = out_channels + self.filter_channels = filter_channels + self.kernel_size = kernel_size + self.p_dropout = p_dropout + self.activation = activation + self.causal = causal + + if causal: + self.padding = self._causal_padding + else: + self.padding = self._same_padding + + self.conv_1 = nn.Conv1d(in_channels, filter_channels, kernel_size) + self.conv_2 = nn.Conv1d(filter_channels, out_channels, kernel_size) + self.drop = nn.Dropout(p_dropout) + + def forward(self, x, x_mask): + x = self.conv_1(self.padding(x * x_mask)) + if self.activation == "gelu": + x = x * torch.sigmoid(1.702 * x) + else: + x = torch.relu(x) + x = self.drop(x) + x = self.conv_2(self.padding(x * x_mask)) + return x * x_mask + + def _causal_padding(self, x): + if self.kernel_size == 1: + return x + pad_l = self.kernel_size - 1 + pad_r = 0 + padding = [[0, 0], [0, 0], [pad_l, pad_r]] + x = F.pad(x, commons.convert_pad_shape(padding)) + return x + + def _same_padding(self, x): + if self.kernel_size == 1: + return x + pad_l = (self.kernel_size - 1) // 2 + pad_r = self.kernel_size // 2 + padding = [[0, 0], [0, 0], [pad_l, pad_r]] + x = F.pad(x, commons.convert_pad_shape(padding)) + return x diff --git a/vits/commons.py b/vits/commons.py new file mode 100644 index 0000000000000000000000000000000000000000..045a538d5a3ef8033eca70639a894346b11d5f61 --- /dev/null +++ b/vits/commons.py @@ -0,0 +1,187 @@ +import math +import numpy as np +import torch +from torch import nn +from torch.nn import functional as F + + +def slice_pitch_segments(x, ids_str, segment_size=4): + ret = torch.zeros_like(x[:, :segment_size]) + for i in range(x.size(0)): + idx_str = ids_str[i] + idx_end = idx_str + segment_size + ret[i] = x[i, idx_str:idx_end] + return ret + + +def rand_slice_segments_with_pitch(x, pitch, x_lengths=None, segment_size=4): + b, d, t = x.size() + if x_lengths is None: + x_lengths = t + ids_str_max = x_lengths - segment_size + 1 + ids_str = (torch.rand([b]).to(device=x.device) * ids_str_max).to(dtype=torch.long) + ret = slice_segments(x, ids_str, segment_size) + ret_pitch = slice_pitch_segments(pitch, ids_str, segment_size) + return ret, ret_pitch, ids_str + + +def rand_spec_segments(x, x_lengths=None, segment_size=4): + b, d, t = x.size() + if x_lengths is None: + x_lengths = t + ids_str_max = x_lengths - segment_size + ids_str = (torch.rand([b]).to(device=x.device) * ids_str_max).to(dtype=torch.long) + ret = slice_segments(x, ids_str, segment_size) + return ret, ids_str + + +def init_weights(m, mean=0.0, std=0.01): + classname = m.__class__.__name__ + if classname.find("Conv") != -1: + m.weight.data.normal_(mean, std) + + +def get_padding(kernel_size, dilation=1): + return int((kernel_size * dilation - dilation) / 2) + + +def convert_pad_shape(pad_shape): + l = pad_shape[::-1] + pad_shape = [item for sublist in l for item in sublist] + return pad_shape + + +def kl_divergence(m_p, logs_p, m_q, logs_q): + """KL(P||Q)""" + kl = (logs_q - logs_p) - 0.5 + kl += ( + 0.5 * (torch.exp(2.0 * logs_p) + ((m_p - m_q) ** 2)) * torch.exp(-2.0 * logs_q) + ) + return kl + + +def rand_gumbel(shape): + """Sample from the Gumbel distribution, protect from overflows.""" + uniform_samples = torch.rand(shape) * 0.99998 + 0.00001 + return -torch.log(-torch.log(uniform_samples)) + + +def rand_gumbel_like(x): + g = rand_gumbel(x.size()).to(dtype=x.dtype, device=x.device) + return g + + +def slice_segments(x, ids_str, segment_size=4): + ret = torch.zeros_like(x[:, :, :segment_size]) + for i in range(x.size(0)): + idx_str = ids_str[i] + idx_end = idx_str + segment_size + ret[i] = x[i, :, idx_str:idx_end] + return ret + + +def rand_slice_segments(x, x_lengths=None, segment_size=4): + b, d, t = x.size() + if x_lengths is None: + x_lengths = t + ids_str_max = x_lengths - segment_size + 1 + ids_str = (torch.rand([b]).to(device=x.device) * ids_str_max).to(dtype=torch.long) + ret = slice_segments(x, ids_str, segment_size) + return ret, ids_str + + +def get_timing_signal_1d(length, channels, min_timescale=1.0, max_timescale=1.0e4): + position = torch.arange(length, dtype=torch.float) + num_timescales = channels // 2 + log_timescale_increment = math.log(float(max_timescale) / float(min_timescale)) / ( + num_timescales - 1 + ) + inv_timescales = min_timescale * torch.exp( + torch.arange(num_timescales, dtype=torch.float) * -log_timescale_increment + ) + scaled_time = position.unsqueeze(0) * inv_timescales.unsqueeze(1) + signal = torch.cat([torch.sin(scaled_time), torch.cos(scaled_time)], 0) + signal = F.pad(signal, [0, 0, 0, channels % 2]) + signal = signal.view(1, channels, length) + return signal + + +def add_timing_signal_1d(x, min_timescale=1.0, max_timescale=1.0e4): + b, channels, length = x.size() + signal = get_timing_signal_1d(length, channels, min_timescale, max_timescale) + return x + signal.to(dtype=x.dtype, device=x.device) + + +def cat_timing_signal_1d(x, min_timescale=1.0, max_timescale=1.0e4, axis=1): + b, channels, length = x.size() + signal = get_timing_signal_1d(length, channels, min_timescale, max_timescale) + return torch.cat([x, signal.to(dtype=x.dtype, device=x.device)], axis) + + +def subsequent_mask(length): + mask = torch.tril(torch.ones(length, length)).unsqueeze(0).unsqueeze(0) + return mask + + +@torch.jit.script +def fused_add_tanh_sigmoid_multiply(input_a, input_b, n_channels): + n_channels_int = n_channels[0] + in_act = input_a + input_b + t_act = torch.tanh(in_act[:, :n_channels_int, :]) + s_act = torch.sigmoid(in_act[:, n_channels_int:, :]) + acts = t_act * s_act + return acts + + +def convert_pad_shape(pad_shape): + l = pad_shape[::-1] + pad_shape = [item for sublist in l for item in sublist] + return pad_shape + + +def shift_1d(x): + x = F.pad(x, convert_pad_shape([[0, 0], [0, 0], [1, 0]]))[:, :, :-1] + return x + + +def sequence_mask(length, max_length=None): + if max_length is None: + max_length = length.max() + x = torch.arange(max_length, dtype=length.dtype, device=length.device) + return x.unsqueeze(0) < length.unsqueeze(1) + + +def generate_path(duration, mask): + """ + duration: [b, 1, t_x] + mask: [b, 1, t_y, t_x] + """ + device = duration.device + + b, _, t_y, t_x = mask.shape + cum_duration = torch.cumsum(duration, -1) + + cum_duration_flat = cum_duration.view(b * t_x) + path = sequence_mask(cum_duration_flat, t_y).to(mask.dtype) + path = path.view(b, t_x, t_y) + path = path - F.pad(path, convert_pad_shape([[0, 0], [1, 0], [0, 0]]))[:, :-1] + path = path.unsqueeze(1).transpose(2, 3) * mask + return path + + +def clip_grad_value_(parameters, clip_value, norm_type=2): + if isinstance(parameters, torch.Tensor): + parameters = [parameters] + parameters = list(filter(lambda p: p.grad is not None, parameters)) + norm_type = float(norm_type) + if clip_value is not None: + clip_value = float(clip_value) + + total_norm = 0 + for p in parameters: + param_norm = p.grad.data.norm(norm_type) + total_norm += param_norm.item() ** norm_type + if clip_value is not None: + p.grad.data.clamp_(min=-clip_value, max=clip_value) + total_norm = total_norm ** (1.0 / norm_type) + return total_norm diff --git a/vits/data_utils.py b/vits/data_utils.py new file mode 100644 index 0000000000000000000000000000000000000000..e324f287603a82db668f679f74caf4ac5df9cf35 --- /dev/null +++ b/vits/data_utils.py @@ -0,0 +1,306 @@ +import os +import numpy as np +import random +import torch +import torch.utils.data + + +from vits.utils import load_wav_to_torch + + +def load_filepaths(filename, split="|"): + with open(filename, encoding='utf-8') as f: + filepaths = [line.strip().split(split) for line in f] + return filepaths + + +class TextAudioSpeakerSet(torch.utils.data.Dataset): + def __init__(self, filename, hparams): + self.items = load_filepaths(filename) + self.max_wav_value = hparams.max_wav_value + self.sampling_rate = hparams.sampling_rate + self.segment_size = hparams.segment_size + self.hop_length = hparams.hop_length + self._filter() + print(f'----------{len(self.items)}----------') + + def _filter(self): + lengths = [] + items_new = [] + items_min = int(self.segment_size / self.hop_length * 2) # 1 S + items_max = int(self.segment_size / self.hop_length * 9) # 4.5 S + for wavpath, spec, pitch, ppg, spk in self.items: + if not os.path.isfile(wavpath): + continue + if not os.path.isfile(spec): + continue + if not os.path.isfile(pitch): + continue + if not os.path.isfile(ppg): + continue + if not os.path.isfile(spk): + continue + temp = np.load(pitch) + usel = int(temp.shape[0] - 1) # useful length + if (usel < items_min): + continue + if (usel >= items_max): + usel = items_max + items_new.append([wavpath, spec, pitch, ppg, spk, usel]) + lengths.append(usel) + self.items = items_new + self.lengths = lengths + + def read_wav(self, filename): + audio, sampling_rate = load_wav_to_torch(filename) + assert sampling_rate == self.sampling_rate, f"error: this sample rate of {filename} is {sampling_rate}" + audio_norm = audio / self.max_wav_value + audio_norm = audio_norm.unsqueeze(0) + return audio_norm + + def __getitem__(self, index): + return self.my_getitem(index) + + def __len__(self): + return len(self.items) + + def my_getitem(self, idx): + item = self.items[idx] + # print(item) + wav = item[0] + spe = item[1] + pit = item[2] + ppg = item[3] + spk = item[4] + use = item[5] + + wav = self.read_wav(wav) + spe = torch.load(spe) + + pit = np.load(pit) + ppg = np.load(ppg) + ppg = np.repeat(ppg, 2, 0) # 320 PPG -> 160 * 2 + spk = np.load(spk) + + pit = torch.FloatTensor(pit) + ppg = torch.FloatTensor(ppg) + spk = torch.FloatTensor(spk) + + len_pit = pit.size()[0] + len_ppg = ppg.size()[0] + len_min = min(len_pit, len_ppg) + len_wav = len_min * self.hop_length + + pit = pit[:len_min] + ppg = ppg[:len_min, :] + spe = spe[:, :len_min] + wav = wav[:, :len_wav] + if len_min > use: + max_frame_start = ppg.size(0) - use - 1 + frame_start = random.randint(0, max_frame_start) + frame_end = frame_start + use + + pit = pit[frame_start:frame_end] + ppg = ppg[frame_start:frame_end, :] + spe = spe[:, frame_start:frame_end] + + wav_start = frame_start * self.hop_length + wav_end = frame_end * self.hop_length + wav = wav[:, wav_start:wav_end] + # print(spe.shape) + # print(wav.shape) + # print(ppg.shape) + # print(pit.shape) + # print(spk.shape) + return spe, wav, ppg, pit, spk + + +class TextAudioSpeakerCollate: + """Zero-pads model inputs and targets""" + + def __call__(self, batch): + # Right zero-pad all one-hot text sequences to max input length + # mel: [freq, length] + # wav: [1, length] + # ppg: [len, 1024] + # pit: [len] + # spk: [256] + _, ids_sorted_decreasing = torch.sort( + torch.LongTensor([x[0].size(1) for x in batch]), dim=0, descending=True + ) + + max_spe_len = max([x[0].size(1) for x in batch]) + max_wav_len = max([x[1].size(1) for x in batch]) + spe_lengths = torch.LongTensor(len(batch)) + wav_lengths = torch.LongTensor(len(batch)) + spe_padded = torch.FloatTensor( + len(batch), batch[0][0].size(0), max_spe_len) + wav_padded = torch.FloatTensor(len(batch), 1, max_wav_len) + spe_padded.zero_() + wav_padded.zero_() + + max_ppg_len = max([x[2].size(0) for x in batch]) + ppg_lengths = torch.FloatTensor(len(batch)) + ppg_padded = torch.FloatTensor( + len(batch), max_ppg_len, batch[0][2].size(1)) + pit_padded = torch.FloatTensor(len(batch), max_ppg_len) + ppg_padded.zero_() + pit_padded.zero_() + spk = torch.FloatTensor(len(batch), batch[0][4].size(0)) + + for i in range(len(ids_sorted_decreasing)): + row = batch[ids_sorted_decreasing[i]] + + spe = row[0] + spe_padded[i, :, : spe.size(1)] = spe + spe_lengths[i] = spe.size(1) + + wav = row[1] + wav_padded[i, :, : wav.size(1)] = wav + wav_lengths[i] = wav.size(1) + + ppg = row[2] + ppg_padded[i, : ppg.size(0), :] = ppg + ppg_lengths[i] = ppg.size(0) + + pit = row[3] + pit_padded[i, : pit.size(0)] = pit + + spk[i] = row[4] + # print(ppg_padded.shape) + # print(ppg_lengths.shape) + # print(pit_padded.shape) + # print(spk.shape) + # print(spe_padded.shape) + # print(spe_lengths.shape) + # print(wav_padded.shape) + # print(wav_lengths.shape) + return ( + ppg_padded, + ppg_lengths, + pit_padded, + spk, + spe_padded, + spe_lengths, + wav_padded, + wav_lengths, + ) + + +class DistributedBucketSampler(torch.utils.data.distributed.DistributedSampler): + """ + Maintain similar input lengths in a batch. + Length groups are specified by boundaries. + Ex) boundaries = [b1, b2, b3] -> any batch is included either {x | b1 < length(x) <=b2} or {x | b2 < length(x) <= b3}. + It removes samples which are not included in the boundaries. + Ex) boundaries = [b1, b2, b3] -> any x s.t. length(x) <= b1 or length(x) > b3 are discarded. + """ + + def __init__( + self, + dataset, + batch_size, + boundaries, + num_replicas=None, + rank=None, + shuffle=True, + ): + super().__init__(dataset, num_replicas=num_replicas, rank=rank, shuffle=shuffle) + self.lengths = dataset.lengths + self.batch_size = batch_size + self.boundaries = boundaries + + self.buckets, self.num_samples_per_bucket = self._create_buckets() + self.total_size = sum(self.num_samples_per_bucket) + self.num_samples = self.total_size // self.num_replicas + + def _create_buckets(self): + buckets = [[] for _ in range(len(self.boundaries) - 1)] + for i in range(len(self.lengths)): + length = self.lengths[i] + idx_bucket = self._bisect(length) + if idx_bucket != -1: + buckets[idx_bucket].append(i) + + for i in range(len(buckets) - 1, 0, -1): + if len(buckets[i]) == 0: + buckets.pop(i) + self.boundaries.pop(i + 1) + + num_samples_per_bucket = [] + for i in range(len(buckets)): + len_bucket = len(buckets[i]) + total_batch_size = self.num_replicas * self.batch_size + rem = ( + total_batch_size - (len_bucket % total_batch_size) + ) % total_batch_size + num_samples_per_bucket.append(len_bucket + rem) + return buckets, num_samples_per_bucket + + def __iter__(self): + # deterministically shuffle based on epoch + g = torch.Generator() + g.manual_seed(self.epoch) + + indices = [] + if self.shuffle: + for bucket in self.buckets: + indices.append(torch.randperm( + len(bucket), generator=g).tolist()) + else: + for bucket in self.buckets: + indices.append(list(range(len(bucket)))) + + batches = [] + for i in range(len(self.buckets)): + bucket = self.buckets[i] + len_bucket = len(bucket) + ids_bucket = indices[i] + num_samples_bucket = self.num_samples_per_bucket[i] + + # add extra samples to make it evenly divisible + rem = num_samples_bucket - len_bucket + ids_bucket = ( + ids_bucket + + ids_bucket * (rem // len_bucket) + + ids_bucket[: (rem % len_bucket)] + ) + + # subsample + ids_bucket = ids_bucket[self.rank:: self.num_replicas] + + # batching + for j in range(len(ids_bucket) // self.batch_size): + batch = [ + bucket[idx] + for idx in ids_bucket[ + j * self.batch_size: (j + 1) * self.batch_size + ] + ] + batches.append(batch) + + if self.shuffle: + batch_ids = torch.randperm(len(batches), generator=g).tolist() + batches = [batches[i] for i in batch_ids] + self.batches = batches + + assert len(self.batches) * self.batch_size == self.num_samples + return iter(self.batches) + + def _bisect(self, x, lo=0, hi=None): + if hi is None: + hi = len(self.boundaries) - 1 + + if hi > lo: + mid = (hi + lo) // 2 + if self.boundaries[mid] < x and x <= self.boundaries[mid + 1]: + return mid + elif x <= self.boundaries[mid]: + return self._bisect(x, lo, mid) + else: + return self._bisect(x, mid + 1, hi) + else: + return -1 + + def __len__(self): + return self.num_samples // self.batch_size diff --git a/vits/losses.py b/vits/losses.py new file mode 100644 index 0000000000000000000000000000000000000000..9244de65482650fedea4de6e3cc26d4700ee76e9 --- /dev/null +++ b/vits/losses.py @@ -0,0 +1,79 @@ +import torch + + +def feature_loss(fmap_r, fmap_g): + loss = 0 + for dr, dg in zip(fmap_r, fmap_g): + for rl, gl in zip(dr, dg): + rl = rl.float().detach() + gl = gl.float() + loss += torch.mean(torch.abs(rl - gl)) + + return loss * 2 + + +def discriminator_loss(disc_real_outputs, disc_generated_outputs): + loss = 0 + r_losses = [] + g_losses = [] + for dr, dg in zip(disc_real_outputs, disc_generated_outputs): + dr = dr.float() + dg = dg.float() + r_loss = torch.mean((1 - dr) ** 2) + g_loss = torch.mean(dg**2) + loss += r_loss + g_loss + r_losses.append(r_loss.item()) + g_losses.append(g_loss.item()) + + return loss, r_losses, g_losses + + +def generator_loss(disc_outputs): + loss = 0 + gen_losses = [] + for dg in disc_outputs: + dg = dg.float() + l = torch.mean((1 - dg) ** 2) + gen_losses.append(l) + loss += l + + return loss, gen_losses + + +def kl_loss(z_p, logs_q, m_p, logs_p, total_logdet, z_mask): + """ + z_p, logs_q: [b, h, t_t] + m_p, logs_p: [b, h, t_t] + total_logdet: [b] - total_logdet summed over each batch + """ + z_p = z_p.float() + logs_q = logs_q.float() + m_p = m_p.float() + logs_p = logs_p.float() + z_mask = z_mask.float() + + kl = logs_p - logs_q - 0.5 + kl += 0.5 * ((z_p - m_p) ** 2) * torch.exp(-2.0 * logs_p) + kl = torch.sum(kl * z_mask) + # add total_logdet (Negative LL) + kl -= torch.sum(total_logdet) + l = kl / torch.sum(z_mask) + return l + + +def kl_loss_back(z_p, logs_q, m_p, logs_p, z_mask): + """ + z_p, logs_q: [b, h, t_t] + m_p, logs_p: [b, h, t_t] + """ + z_p = z_p.float() + logs_q = logs_q.float() + m_p = m_p.float() + logs_p = logs_p.float() + z_mask = z_mask.float() + + kl = logs_p - logs_q - 0.5 + kl += 0.5 * ((z_p - m_p) ** 2) * torch.exp(-2.0 * logs_p) + kl = torch.sum(kl * z_mask) + l = kl / torch.sum(z_mask) + return l diff --git a/vits/models.py b/vits/models.py new file mode 100644 index 0000000000000000000000000000000000000000..9de6f7cce8512aa1bb73c9f78a0a23d219f8e8ec --- /dev/null +++ b/vits/models.py @@ -0,0 +1,240 @@ + +import torch + +from torch import nn +from torch.nn import functional as F +from vits import attentions +from vits import commons +from vits import modules +from vits.utils import f0_to_coarse +from vits_decoder.generator import Generator + + +class TextEncoder(nn.Module): + def __init__(self, + in_channels, + out_channels, + hidden_channels, + filter_channels, + n_heads, + n_layers, + kernel_size, + p_dropout): + super().__init__() + self.out_channels = out_channels + self.pre = nn.Conv1d(in_channels, hidden_channels, kernel_size=5, padding=2) + self.pit = nn.Embedding(256, hidden_channels) + self.enc = attentions.Encoder( + hidden_channels, + filter_channels, + n_heads, + n_layers, + kernel_size, + p_dropout) + self.proj = nn.Conv1d(hidden_channels, out_channels * 2, 1) + + def forward(self, x, x_lengths, f0): + x = torch.transpose(x, 1, -1) # [b, h, t] + x_mask = torch.unsqueeze(commons.sequence_mask(x_lengths, x.size(2)), 1).to( + x.dtype + ) + x = self.pre(x) * x_mask + x = x + self.pit(f0).transpose(1, 2) + x = self.enc(x * x_mask, x_mask) + stats = self.proj(x) * x_mask + m, logs = torch.split(stats, self.out_channels, dim=1) + z = (m + torch.randn_like(m) * torch.exp(logs)) * x_mask + return z, m, logs, x_mask + + +class ResidualCouplingBlock(nn.Module): + def __init__( + self, + channels, + hidden_channels, + kernel_size, + dilation_rate, + n_layers, + n_flows=4, + gin_channels=0, + ): + super().__init__() + self.flows = nn.ModuleList() + for i in range(n_flows): + self.flows.append( + modules.ResidualCouplingLayer( + channels, + hidden_channels, + kernel_size, + dilation_rate, + n_layers, + gin_channels=gin_channels, + mean_only=True, + ) + ) + self.flows.append(modules.Flip()) + + def forward(self, x, x_mask, g=None, reverse=False): + if not reverse: + total_logdet = 0 + for flow in self.flows: + x, log_det = flow(x, x_mask, g=g, reverse=reverse) + total_logdet += log_det + return x, total_logdet + else: + total_logdet = 0 + for flow in reversed(self.flows): + x, log_det = flow(x, x_mask, g=g, reverse=reverse) + total_logdet += log_det + return x, total_logdet + + def remove_weight_norm(self): + for i in range(self.n_flows): + self.flows[i * 2].remove_weight_norm() + + +class PosteriorEncoder(nn.Module): + def __init__( + self, + in_channels, + out_channels, + hidden_channels, + kernel_size, + dilation_rate, + n_layers, + gin_channels=0, + ): + super().__init__() + self.out_channels = out_channels + self.pre = nn.Conv1d(in_channels, hidden_channels, 1) + self.enc = modules.WN( + hidden_channels, + kernel_size, + dilation_rate, + n_layers, + gin_channels=gin_channels, + ) + self.proj = nn.Conv1d(hidden_channels, out_channels * 2, 1) + + def forward(self, x, x_lengths, g=None): + x_mask = torch.unsqueeze(commons.sequence_mask(x_lengths, x.size(2)), 1).to( + x.dtype + ) + x = self.pre(x) * x_mask + x = self.enc(x, x_mask, g=g) + stats = self.proj(x) * x_mask + m, logs = torch.split(stats, self.out_channels, dim=1) + z = (m + torch.randn_like(m) * torch.exp(logs)) * x_mask + return z, m, logs, x_mask + + def remove_weight_norm(self): + self.enc.remove_weight_norm() + + +class SynthesizerTrn(nn.Module): + def __init__( + self, + spec_channels, + segment_size, + hp + ): + super().__init__() + self.segment_size = segment_size + self.emb_g = nn.Linear(hp.vits.spk_dim, hp.vits.gin_channels) + self.enc_p = TextEncoder( + hp.vits.ppg_dim, + hp.vits.inter_channels, + hp.vits.hidden_channels, + hp.vits.filter_channels, + 2, + 6, + 3, + 0.1, + ) + self.enc_q = PosteriorEncoder( + spec_channels, + hp.vits.inter_channels, + hp.vits.hidden_channels, + 5, + 1, + 16, + gin_channels=hp.vits.gin_channels, + ) + self.flow = ResidualCouplingBlock( + hp.vits.inter_channels, + hp.vits.hidden_channels, + 5, + 1, + 4, + gin_channels=hp.vits.spk_dim + ) + self.dec = Generator(hp=hp) + + def forward(self, ppg, pit, spec, spk, ppg_l, spec_l): + g = self.emb_g(F.normalize(spk)).unsqueeze(-1) + z_p, m_p, logs_p, ppg_mask = self.enc_p( + ppg, ppg_l, f0=f0_to_coarse(pit)) + z_q, m_q, logs_q, spec_mask = self.enc_q(spec, spec_l, g=g) + + z_slice, pit_slice, ids_slice = commons.rand_slice_segments_with_pitch( + z_q, pit, spec_l, self.segment_size) + audio = self.dec(spk, z_slice, pit_slice) + + # SNAC to flow + z_f, logdet_f = self.flow(z_q, spec_mask, g=spk) + z_r, logdet_r = self.flow(z_p, spec_mask, g=spk, reverse=True) + return audio, ids_slice, spec_mask, (z_f, z_r, z_p, m_p, logs_p, z_q, m_q, logs_q, logdet_f, logdet_r) + + def infer(self, ppg, pit, spk, ppg_l): + z_p, m_p, logs_p, ppg_mask = self.enc_p( + ppg, ppg_l, f0=f0_to_coarse(pit)) + z, _ = self.flow(z_p, ppg_mask, g=spk, reverse=True) + o = self.dec(spk, z * ppg_mask, f0=pit) + return o + + +class SynthesizerInfer(nn.Module): + def __init__( + self, + spec_channels, + segment_size, + hp + ): + super().__init__() + self.segment_size = segment_size + self.enc_p = TextEncoder( + hp.vits.ppg_dim, + hp.vits.inter_channels, + hp.vits.hidden_channels, + hp.vits.filter_channels, + 2, + 6, + 3, + 0.1, + ) + self.flow = ResidualCouplingBlock( + hp.vits.inter_channels, + hp.vits.hidden_channels, + 5, + 1, + 4, + gin_channels=hp.vits.spk_dim + ) + self.dec = Generator(hp=hp) + + def remove_weight_norm(self): + self.flow.remove_weight_norm() + self.dec.remove_weight_norm() + + def pitch2source(self, f0): + return self.dec.pitch2source(f0) + + def source2wav(self, source): + return self.dec.source2wav(source) + + def inference(self, ppg, pit, spk, ppg_l, source): + z_p, m_p, logs_p, ppg_mask = self.enc_p( + ppg, ppg_l, f0=f0_to_coarse(pit)) + z, _ = self.flow(z_p, ppg_mask, g=spk, reverse=True) + o = self.dec.inference(spk, z * ppg_mask, source) + return o diff --git a/vits/modules.py b/vits/modules.py new file mode 100644 index 0000000000000000000000000000000000000000..4fe730b8bd45de6ae7a25cb8213b50a6f662db8e --- /dev/null +++ b/vits/modules.py @@ -0,0 +1,475 @@ +import copy +import math +import numpy as np +import scipy +import torch +from torch import nn +from torch.nn import functional as F + +from torch.nn import Conv1d, ConvTranspose1d, AvgPool1d, Conv2d +from torch.nn.utils import weight_norm, remove_weight_norm + +from vits import commons +from vits.commons import init_weights, get_padding + + +LRELU_SLOPE = 0.1 + + +class LayerNorm(nn.Module): + def __init__(self, channels, eps=1e-5): + super().__init__() + self.channels = channels + self.eps = eps + + self.gamma = nn.Parameter(torch.ones(channels)) + self.beta = nn.Parameter(torch.zeros(channels)) + + def forward(self, x): + x = x.transpose(1, -1) + x = F.layer_norm(x, (self.channels,), self.gamma, self.beta, self.eps) + return x.transpose(1, -1) + + +class ConvReluNorm(nn.Module): + def __init__( + self, + in_channels, + hidden_channels, + out_channels, + kernel_size, + n_layers, + p_dropout, + ): + super().__init__() + self.in_channels = in_channels + self.hidden_channels = hidden_channels + self.out_channels = out_channels + self.kernel_size = kernel_size + self.n_layers = n_layers + self.p_dropout = p_dropout + assert n_layers > 1, "Number of layers should be larger than 0." + + self.conv_layers = nn.ModuleList() + self.norm_layers = nn.ModuleList() + self.conv_layers.append( + nn.Conv1d( + in_channels, hidden_channels, kernel_size, padding=kernel_size // 2 + ) + ) + self.norm_layers.append(LayerNorm(hidden_channels)) + self.relu_drop = nn.Sequential(nn.ReLU(), nn.Dropout(p_dropout)) + for _ in range(n_layers - 1): + self.conv_layers.append( + nn.Conv1d( + hidden_channels, + hidden_channels, + kernel_size, + padding=kernel_size // 2, + ) + ) + self.norm_layers.append(LayerNorm(hidden_channels)) + self.proj = nn.Conv1d(hidden_channels, out_channels, 1) + self.proj.weight.data.zero_() + self.proj.bias.data.zero_() + + def forward(self, x, x_mask): + x_org = x + for i in range(self.n_layers): + x = self.conv_layers[i](x * x_mask) + x = self.norm_layers[i](x) + x = self.relu_drop(x) + x = x_org + self.proj(x) + return x * x_mask + + +class DDSConv(nn.Module): + """ + Dialted and Depth-Separable Convolution + """ + + def __init__(self, channels, kernel_size, n_layers, p_dropout=0.0): + super().__init__() + self.channels = channels + self.kernel_size = kernel_size + self.n_layers = n_layers + self.p_dropout = p_dropout + + self.drop = nn.Dropout(p_dropout) + self.convs_sep = nn.ModuleList() + self.convs_1x1 = nn.ModuleList() + self.norms_1 = nn.ModuleList() + self.norms_2 = nn.ModuleList() + for i in range(n_layers): + dilation = kernel_size**i + padding = (kernel_size * dilation - dilation) // 2 + self.convs_sep.append( + nn.Conv1d( + channels, + channels, + kernel_size, + groups=channels, + dilation=dilation, + padding=padding, + ) + ) + self.convs_1x1.append(nn.Conv1d(channels, channels, 1)) + self.norms_1.append(LayerNorm(channels)) + self.norms_2.append(LayerNorm(channels)) + + def forward(self, x, x_mask, g=None): + if g is not None: + x = x + g + for i in range(self.n_layers): + y = self.convs_sep[i](x * x_mask) + y = self.norms_1[i](y) + y = F.gelu(y) + y = self.convs_1x1[i](y) + y = self.norms_2[i](y) + y = F.gelu(y) + y = self.drop(y) + x = x + y + return x * x_mask + + +class WN(torch.nn.Module): + def __init__( + self, + hidden_channels, + kernel_size, + dilation_rate, + n_layers, + gin_channels=0, + p_dropout=0, + ): + super(WN, self).__init__() + assert kernel_size % 2 == 1 + self.hidden_channels = hidden_channels + self.kernel_size = (kernel_size,) + self.dilation_rate = dilation_rate + self.n_layers = n_layers + self.gin_channels = gin_channels + self.p_dropout = p_dropout + + self.in_layers = torch.nn.ModuleList() + self.res_skip_layers = torch.nn.ModuleList() + self.drop = nn.Dropout(p_dropout) + + if gin_channels != 0: + cond_layer = torch.nn.Conv1d( + gin_channels, 2 * hidden_channels * n_layers, 1 + ) + self.cond_layer = torch.nn.utils.weight_norm(cond_layer, name="weight") + + for i in range(n_layers): + dilation = dilation_rate**i + padding = int((kernel_size * dilation - dilation) / 2) + in_layer = torch.nn.Conv1d( + hidden_channels, + 2 * hidden_channels, + kernel_size, + dilation=dilation, + padding=padding, + ) + in_layer = torch.nn.utils.weight_norm(in_layer, name="weight") + self.in_layers.append(in_layer) + + # last one is not necessary + if i < n_layers - 1: + res_skip_channels = 2 * hidden_channels + else: + res_skip_channels = hidden_channels + + res_skip_layer = torch.nn.Conv1d(hidden_channels, res_skip_channels, 1) + res_skip_layer = torch.nn.utils.weight_norm(res_skip_layer, name="weight") + self.res_skip_layers.append(res_skip_layer) + + def forward(self, x, x_mask, g=None, **kwargs): + output = torch.zeros_like(x) + n_channels_tensor = torch.IntTensor([self.hidden_channels]) + + if g is not None: + g = self.cond_layer(g) + + for i in range(self.n_layers): + x_in = self.in_layers[i](x) + if g is not None: + cond_offset = i * 2 * self.hidden_channels + g_l = g[:, cond_offset : cond_offset + 2 * self.hidden_channels, :] + else: + g_l = torch.zeros_like(x_in) + + acts = commons.fused_add_tanh_sigmoid_multiply(x_in, g_l, n_channels_tensor) + acts = self.drop(acts) + + res_skip_acts = self.res_skip_layers[i](acts) + if i < self.n_layers - 1: + res_acts = res_skip_acts[:, : self.hidden_channels, :] + x = (x + res_acts) * x_mask + output = output + res_skip_acts[:, self.hidden_channels:, :] + else: + output = output + res_skip_acts + return output * x_mask + + def remove_weight_norm(self): + if self.gin_channels != 0: + torch.nn.utils.remove_weight_norm(self.cond_layer) + for l in self.in_layers: + torch.nn.utils.remove_weight_norm(l) + for l in self.res_skip_layers: + torch.nn.utils.remove_weight_norm(l) + + +class ResBlock1(torch.nn.Module): + def __init__(self, channels, kernel_size=3, dilation=(1, 3, 5)): + super(ResBlock1, self).__init__() + self.convs1 = nn.ModuleList( + [ + weight_norm( + Conv1d( + channels, + channels, + kernel_size, + 1, + dilation=dilation[0], + padding=get_padding(kernel_size, dilation[0]), + ) + ), + weight_norm( + Conv1d( + channels, + channels, + kernel_size, + 1, + dilation=dilation[1], + padding=get_padding(kernel_size, dilation[1]), + ) + ), + weight_norm( + Conv1d( + channels, + channels, + kernel_size, + 1, + dilation=dilation[2], + padding=get_padding(kernel_size, dilation[2]), + ) + ), + ] + ) + self.convs1.apply(init_weights) + + self.convs2 = nn.ModuleList( + [ + weight_norm( + Conv1d( + channels, + channels, + kernel_size, + 1, + dilation=1, + padding=get_padding(kernel_size, 1), + ) + ), + weight_norm( + Conv1d( + channels, + channels, + kernel_size, + 1, + dilation=1, + padding=get_padding(kernel_size, 1), + ) + ), + weight_norm( + Conv1d( + channels, + channels, + kernel_size, + 1, + dilation=1, + padding=get_padding(kernel_size, 1), + ) + ), + ] + ) + self.convs2.apply(init_weights) + + def forward(self, x, x_mask=None): + for c1, c2 in zip(self.convs1, self.convs2): + xt = F.leaky_relu(x, LRELU_SLOPE) + if x_mask is not None: + xt = xt * x_mask + xt = c1(xt) + xt = F.leaky_relu(xt, LRELU_SLOPE) + if x_mask is not None: + xt = xt * x_mask + xt = c2(xt) + x = xt + x + if x_mask is not None: + x = x * x_mask + return x + + def remove_weight_norm(self): + for l in self.convs1: + remove_weight_norm(l) + for l in self.convs2: + remove_weight_norm(l) + + +class ResBlock2(torch.nn.Module): + def __init__(self, channels, kernel_size=3, dilation=(1, 3)): + super(ResBlock2, self).__init__() + self.convs = nn.ModuleList( + [ + weight_norm( + Conv1d( + channels, + channels, + kernel_size, + 1, + dilation=dilation[0], + padding=get_padding(kernel_size, dilation[0]), + ) + ), + weight_norm( + Conv1d( + channels, + channels, + kernel_size, + 1, + dilation=dilation[1], + padding=get_padding(kernel_size, dilation[1]), + ) + ), + ] + ) + self.convs.apply(init_weights) + + def forward(self, x, x_mask=None): + for c in self.convs: + xt = F.leaky_relu(x, LRELU_SLOPE) + if x_mask is not None: + xt = xt * x_mask + xt = c(xt) + x = xt + x + if x_mask is not None: + x = x * x_mask + return x + + def remove_weight_norm(self): + for l in self.convs: + remove_weight_norm(l) + + +class Log(nn.Module): + def forward(self, x, x_mask, reverse=False, **kwargs): + if not reverse: + y = torch.log(torch.clamp_min(x, 1e-5)) * x_mask + logdet = torch.sum(-y, [1, 2]) + return y, logdet + else: + x = torch.exp(x) * x_mask + return x + + +class Flip(nn.Module): + def forward(self, x, *args, reverse=False, **kwargs): + x = torch.flip(x, [1]) + logdet = torch.zeros(x.size(0)).to(dtype=x.dtype, device=x.device) + return x, logdet + + +class ElementwiseAffine(nn.Module): + def __init__(self, channels): + super().__init__() + self.channels = channels + self.m = nn.Parameter(torch.zeros(channels, 1)) + self.logs = nn.Parameter(torch.zeros(channels, 1)) + + def forward(self, x, x_mask, reverse=False, **kwargs): + if not reverse: + y = self.m + torch.exp(self.logs) * x + y = y * x_mask + logdet = torch.sum(self.logs * x_mask, [1, 2]) + return y, logdet + else: + x = (x - self.m) * torch.exp(-self.logs) * x_mask + return x + + +class ResidualCouplingLayer(nn.Module): + def __init__( + self, + channels, + hidden_channels, + kernel_size, + dilation_rate, + n_layers, + p_dropout=0, + gin_channels=0, + mean_only=False, + ): + assert channels % 2 == 0, "channels should be divisible by 2" + super().__init__() + self.channels = channels + self.hidden_channels = hidden_channels + self.kernel_size = kernel_size + self.dilation_rate = dilation_rate + self.n_layers = n_layers + self.half_channels = channels // 2 + self.mean_only = mean_only + + self.pre = nn.Conv1d(self.half_channels, hidden_channels, 1) + # no use gin_channels + self.enc = WN( + hidden_channels, + kernel_size, + dilation_rate, + n_layers, + p_dropout=p_dropout, + ) + self.post = nn.Conv1d( + hidden_channels, self.half_channels * (2 - mean_only), 1) + self.post.weight.data.zero_() + self.post.bias.data.zero_() + # SNAC Speaker-normalized Affine Coupling Layer + self.snac = nn.Conv1d(gin_channels, 2 * self.half_channels, 1) + + def forward(self, x, x_mask, g=None, reverse=False): + speaker = self.snac(g.unsqueeze(-1)) + speaker_m, speaker_v = speaker.chunk(2, dim=1) # (B, half_channels, 1) + x0, x1 = torch.split(x, [self.half_channels] * 2, 1) + # x0 norm + x0_norm = (x0 - speaker_m) * torch.exp(-speaker_v) * x_mask + h = self.pre(x0_norm) * x_mask + # don't use global condition + h = self.enc(h, x_mask) + stats = self.post(h) * x_mask + if not self.mean_only: + m, logs = torch.split(stats, [self.half_channels] * 2, 1) + else: + m = stats + logs = torch.zeros_like(m) + + if not reverse: + # x1 norm before affine xform + x1_norm = (x1 - speaker_m) * torch.exp(-speaker_v) * x_mask + x1 = (m + x1_norm * torch.exp(logs)) * x_mask + x = torch.cat([x0, x1], 1) + # speaker var to logdet + logdet = torch.sum(logs * x_mask, [1, 2]) - torch.sum( + speaker_v.expand(-1, -1, logs.size(-1)) * x_mask, [1, 2]) + return x, logdet + else: + x1 = (x1 - m) * torch.exp(-logs) * x_mask + # x1 denorm before output + x1 = (speaker_m + x1 * torch.exp(speaker_v)) * x_mask + x = torch.cat([x0, x1], 1) + # speaker var to logdet + logdet = torch.sum(logs * x_mask, [1, 2]) + torch.sum( + speaker_v.expand(-1, -1, logs.size(-1)) * x_mask, [1, 2]) + return x, logdet + + def remove_weight_norm(self): + self.enc.remove_weight_norm() diff --git a/vits/spectrogram.py b/vits/spectrogram.py new file mode 100644 index 0000000000000000000000000000000000000000..67b54b1757f977f840ba97e0ad28b241fceeecd7 --- /dev/null +++ b/vits/spectrogram.py @@ -0,0 +1,140 @@ +import torch +import torch.utils.data + +from librosa.filters import mel as librosa_mel_fn + +MAX_WAV_VALUE = 32768.0 + + +def dynamic_range_compression_torch(x, C=1, clip_val=1e-5): + """ + PARAMS + ------ + C: compression factor + """ + return torch.log(torch.clamp(x, min=clip_val) * C) + + +def dynamic_range_decompression_torch(x, C=1): + """ + PARAMS + ------ + C: compression factor used to compress + """ + return torch.exp(x) / C + + +def spectral_normalize_torch(magnitudes): + output = dynamic_range_compression_torch(magnitudes) + return output + + +def spectral_de_normalize_torch(magnitudes): + output = dynamic_range_decompression_torch(magnitudes) + return output + + +mel_basis = {} +hann_window = {} + + +def spectrogram_torch(y, n_fft, sampling_rate, hop_size, win_size, center=False): + if torch.min(y) < -1.0: + print("min value is ", torch.min(y)) + if torch.max(y) > 1.0: + print("max value is ", torch.max(y)) + + global hann_window + dtype_device = str(y.dtype) + "_" + str(y.device) + wnsize_dtype_device = str(win_size) + "_" + dtype_device + if wnsize_dtype_device not in hann_window: + hann_window[wnsize_dtype_device] = torch.hann_window(win_size).to( + dtype=y.dtype, device=y.device + ) + + y = torch.nn.functional.pad( + y.unsqueeze(1), + (int((n_fft - hop_size) / 2), int((n_fft - hop_size) / 2)), + mode="reflect", + ) + y = y.squeeze(1) + + spec = torch.stft( + y, + n_fft, + hop_length=hop_size, + win_length=win_size, + window=hann_window[wnsize_dtype_device], + center=center, + pad_mode="reflect", + normalized=False, + onesided=True, + return_complex=False, + ) + + spec = torch.sqrt(spec.pow(2).sum(-1) + 1e-6) + return spec + + +def spec_to_mel_torch(spec, n_fft, num_mels, sampling_rate, fmin, fmax): + global mel_basis + dtype_device = str(spec.dtype) + "_" + str(spec.device) + fmax_dtype_device = str(fmax) + "_" + dtype_device + if fmax_dtype_device not in mel_basis: + mel = librosa_mel_fn(sr=sampling_rate, n_fft=n_fft, n_mels=num_mels, fmin=fmin, fmax=fmax) + mel_basis[fmax_dtype_device] = torch.from_numpy(mel).to( + dtype=spec.dtype, device=spec.device + ) + spec = torch.matmul(mel_basis[fmax_dtype_device], spec) + spec = spectral_normalize_torch(spec) + return spec + + +def mel_spectrogram_torch( + y, n_fft, num_mels, sampling_rate, hop_size, win_size, fmin, fmax, center=False +): + if torch.min(y) < -1.0: + print("min value is ", torch.min(y)) + if torch.max(y) > 1.0: + print("max value is ", torch.max(y)) + + global mel_basis, hann_window + dtype_device = str(y.dtype) + "_" + str(y.device) + fmax_dtype_device = str(fmax) + "_" + dtype_device + wnsize_dtype_device = str(win_size) + "_" + dtype_device + if fmax_dtype_device not in mel_basis: + mel = librosa_mel_fn(sr=sampling_rate, n_fft=n_fft, n_mels=num_mels, fmin=fmin, fmax=fmax) + mel_basis[fmax_dtype_device] = torch.from_numpy(mel).to( + dtype=y.dtype, device=y.device + ) + if wnsize_dtype_device not in hann_window: + hann_window[wnsize_dtype_device] = torch.hann_window(win_size).to( + dtype=y.dtype, device=y.device + ) + + y = torch.nn.functional.pad( + y.unsqueeze(1), + (int((n_fft - hop_size) / 2), int((n_fft - hop_size) / 2)), + mode="reflect", + ) + y = y.squeeze(1) + + spec = torch.stft( + y, + n_fft, + hop_length=hop_size, + win_length=win_size, + window=hann_window[wnsize_dtype_device], + center=center, + pad_mode="reflect", + normalized=False, + onesided=True, + return_complex=False, + ) + + spec = torch.sqrt(spec.pow(2).sum(-1) + 1e-6) + + spec = torch.matmul(mel_basis[fmax_dtype_device], spec) + spec = spectral_normalize_torch(spec) + + return spec diff --git a/vits/utils.py b/vits/utils.py new file mode 100644 index 0000000000000000000000000000000000000000..dcd2a496090d41d759340cacd3819372867214b9 --- /dev/null +++ b/vits/utils.py @@ -0,0 +1,52 @@ +import os +import argparse +import numpy as np +import torch + +from scipy.io.wavfile import read +from omegaconf import OmegaConf + +MATPLOTLIB_FLAG = False + + +def load_wav_to_torch(full_path): + sampling_rate, data = read(full_path) + return torch.FloatTensor(data.astype(np.float32)), sampling_rate + + +f0_bin = 256 +f0_max = 1100.0 +f0_min = 50.0 +f0_mel_min = 1127 * np.log(1 + f0_min / 700) +f0_mel_max = 1127 * np.log(1 + f0_max / 700) + + +def f0_to_coarse(f0): + is_torch = isinstance(f0, torch.Tensor) + f0_mel = 1127 * (1 + f0 / 700).log() if is_torch else 1127 * \ + np.log(1 + f0 / 700) + f0_mel[f0_mel > 0] = (f0_mel[f0_mel > 0] - f0_mel_min) * \ + (f0_bin - 2) / (f0_mel_max - f0_mel_min) + 1 + + f0_mel[f0_mel <= 1] = 1 + f0_mel[f0_mel > f0_bin - 1] = f0_bin - 1 + f0_coarse = ( + f0_mel + 0.5).long() if is_torch else np.rint(f0_mel).astype(np.int) + assert f0_coarse.max() <= 255 and f0_coarse.min( + ) >= 1, (f0_coarse.max(), f0_coarse.min()) + return f0_coarse + + +def get_hparams(init=True): + parser = argparse.ArgumentParser() + parser.add_argument('-c', '--config', type=str, default="./configs/base.yaml", + help='YAML file for configuration') + args = parser.parse_args() + hparams = OmegaConf.load(args.config) + model_dir = os.path.join("./logs", hparams.train.model) + if not os.path.exists(model_dir): + os.makedirs(model_dir) + config_save_path = os.path.join(model_dir, "config.json") + os.system(f"cp {args.config} {config_save_path}") + hparams.model_dir = model_dir + return hparams diff --git a/vits_decoder/__init__.py b/vits_decoder/__init__.py new file mode 100644 index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391 diff --git a/vits_decoder/alias/__init__.py b/vits_decoder/alias/__init__.py new file mode 100644 index 0000000000000000000000000000000000000000..a2318b63198250856809c0cb46210a4147b829bc --- /dev/null +++ b/vits_decoder/alias/__init__.py @@ -0,0 +1,6 @@ +# Adapted from https://github.com/junjun3518/alias-free-torch under the Apache License 2.0 +# LICENSE is in incl_licenses directory. + +from .filter import * +from .resample import * +from .act import * \ No newline at end of file diff --git a/vits_decoder/alias/act.py b/vits_decoder/alias/act.py new file mode 100644 index 0000000000000000000000000000000000000000..028debd697dd60458aae75010057df038bd3518a --- /dev/null +++ b/vits_decoder/alias/act.py @@ -0,0 +1,28 @@ +# Adapted from https://github.com/junjun3518/alias-free-torch under the Apache License 2.0 +# LICENSE is in incl_licenses directory. + +import torch.nn as nn +from .resample import UpSample1d, DownSample1d + + +class Activation1d(nn.Module): + def __init__(self, + activation, + up_ratio: int = 2, + down_ratio: int = 2, + up_kernel_size: int = 12, + down_kernel_size: int = 12): + super().__init__() + self.up_ratio = up_ratio + self.down_ratio = down_ratio + self.act = activation + self.upsample = UpSample1d(up_ratio, up_kernel_size) + self.downsample = DownSample1d(down_ratio, down_kernel_size) + + # x: [B,C,T] + def forward(self, x): + x = self.upsample(x) + x = self.act(x) + x = self.downsample(x) + + return x \ No newline at end of file diff --git a/vits_decoder/alias/activations.py b/vits_decoder/alias/activations.py new file mode 100644 index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391 diff --git a/vits_decoder/alias/filter.py b/vits_decoder/alias/filter.py new file mode 100644 index 0000000000000000000000000000000000000000..7ad6ea87c1f10ddd94c544037791d7a4634d5ae1 --- /dev/null +++ b/vits_decoder/alias/filter.py @@ -0,0 +1,95 @@ +# Adapted from https://github.com/junjun3518/alias-free-torch under the Apache License 2.0 +# LICENSE is in incl_licenses directory. + +import torch +import torch.nn as nn +import torch.nn.functional as F +import math + +if 'sinc' in dir(torch): + sinc = torch.sinc +else: + # This code is adopted from adefossez's julius.core.sinc under the MIT License + # https://adefossez.github.io/julius/julius/core.html + # LICENSE is in incl_licenses directory. + def sinc(x: torch.Tensor): + """ + Implementation of sinc, i.e. sin(pi * x) / (pi * x) + __Warning__: Different to julius.sinc, the input is multiplied by `pi`! + """ + return torch.where(x == 0, + torch.tensor(1., device=x.device, dtype=x.dtype), + torch.sin(math.pi * x) / math.pi / x) + + +# This code is adopted from adefossez's julius.lowpass.LowPassFilters under the MIT License +# https://adefossez.github.io/julius/julius/lowpass.html +# LICENSE is in incl_licenses directory. +def kaiser_sinc_filter1d(cutoff, half_width, kernel_size): # return filter [1,1,kernel_size] + even = (kernel_size % 2 == 0) + half_size = kernel_size // 2 + + #For kaiser window + delta_f = 4 * half_width + A = 2.285 * (half_size - 1) * math.pi * delta_f + 7.95 + if A > 50.: + beta = 0.1102 * (A - 8.7) + elif A >= 21.: + beta = 0.5842 * (A - 21)**0.4 + 0.07886 * (A - 21.) + else: + beta = 0. + window = torch.kaiser_window(kernel_size, beta=beta, periodic=False) + + # ratio = 0.5/cutoff -> 2 * cutoff = 1 / ratio + if even: + time = (torch.arange(-half_size, half_size) + 0.5) + else: + time = torch.arange(kernel_size) - half_size + if cutoff == 0: + filter_ = torch.zeros_like(time) + else: + filter_ = 2 * cutoff * window * sinc(2 * cutoff * time) + # Normalize filter to have sum = 1, otherwise we will have a small leakage + # of the constant component in the input signal. + filter_ /= filter_.sum() + filter = filter_.view(1, 1, kernel_size) + + return filter + + +class LowPassFilter1d(nn.Module): + def __init__(self, + cutoff=0.5, + half_width=0.6, + stride: int = 1, + padding: bool = True, + padding_mode: str = 'replicate', + kernel_size: int = 12): + # kernel_size should be even number for stylegan3 setup, + # in this implementation, odd number is also possible. + super().__init__() + if cutoff < -0.: + raise ValueError("Minimum cutoff must be larger than zero.") + if cutoff > 0.5: + raise ValueError("A cutoff above 0.5 does not make sense.") + self.kernel_size = kernel_size + self.even = (kernel_size % 2 == 0) + self.pad_left = kernel_size // 2 - int(self.even) + self.pad_right = kernel_size // 2 + self.stride = stride + self.padding = padding + self.padding_mode = padding_mode + filter = kaiser_sinc_filter1d(cutoff, half_width, kernel_size) + self.register_buffer("filter", filter) + + #input [B, C, T] + def forward(self, x): + _, C, _ = x.shape + + if self.padding: + x = F.pad(x, (self.pad_left, self.pad_right), + mode=self.padding_mode) + out = F.conv1d(x, self.filter.expand(C, -1, -1), + stride=self.stride, groups=C) + + return out \ No newline at end of file diff --git a/vits_decoder/alias/resample.py b/vits_decoder/alias/resample.py new file mode 100644 index 0000000000000000000000000000000000000000..750e6c3402cc5ac939c4b9d075246562e0e1d1a7 --- /dev/null +++ b/vits_decoder/alias/resample.py @@ -0,0 +1,49 @@ +# Adapted from https://github.com/junjun3518/alias-free-torch under the Apache License 2.0 +# LICENSE is in incl_licenses directory. + +import torch.nn as nn +from torch.nn import functional as F +from .filter import LowPassFilter1d +from .filter import kaiser_sinc_filter1d + + +class UpSample1d(nn.Module): + def __init__(self, ratio=2, kernel_size=None): + super().__init__() + self.ratio = ratio + self.kernel_size = int(6 * ratio // 2) * 2 if kernel_size is None else kernel_size + self.stride = ratio + self.pad = self.kernel_size // ratio - 1 + self.pad_left = self.pad * self.stride + (self.kernel_size - self.stride) // 2 + self.pad_right = self.pad * self.stride + (self.kernel_size - self.stride + 1) // 2 + filter = kaiser_sinc_filter1d(cutoff=0.5 / ratio, + half_width=0.6 / ratio, + kernel_size=self.kernel_size) + self.register_buffer("filter", filter) + + # x: [B, C, T] + def forward(self, x): + _, C, _ = x.shape + + x = F.pad(x, (self.pad, self.pad), mode='replicate') + x = self.ratio * F.conv_transpose1d( + x, self.filter.expand(C, -1, -1), stride=self.stride, groups=C) + x = x[..., self.pad_left:-self.pad_right] + + return x + + +class DownSample1d(nn.Module): + def __init__(self, ratio=2, kernel_size=None): + super().__init__() + self.ratio = ratio + self.kernel_size = int(6 * ratio // 2) * 2 if kernel_size is None else kernel_size + self.lowpass = LowPassFilter1d(cutoff=0.5 / ratio, + half_width=0.6 / ratio, + stride=ratio, + kernel_size=self.kernel_size) + + def forward(self, x): + xx = self.lowpass(x) + + return xx \ No newline at end of file diff --git a/vits_decoder/bigv.py b/vits_decoder/bigv.py new file mode 100644 index 0000000000000000000000000000000000000000..f55bb8bdc26cd4249512514c5eec4a05cda88f28 --- /dev/null +++ b/vits_decoder/bigv.py @@ -0,0 +1,131 @@ +import torch +import torch.nn.functional as F +import torch.nn as nn + +from torch import nn, sin, pow +from torch.nn import Parameter +from torch.nn import Conv1d +from torch.nn.utils import weight_norm, remove_weight_norm + + +from .alias import * + + +def init_weights(m, mean=0.0, std=0.01): + classname = m.__class__.__name__ + if classname.find("Conv") != -1: + m.weight.data.normal_(mean, std) + + +def get_padding(kernel_size, dilation=1): + return int((kernel_size*dilation - dilation)/2) + + +class SnakeBeta(nn.Module): + ''' + A modified Snake function which uses separate parameters for the magnitude of the periodic components + Shape: + - Input: (B, C, T) + - Output: (B, C, T), same shape as the input + Parameters: + - alpha - trainable parameter that controls frequency + - beta - trainable parameter that controls magnitude + References: + - This activation function is a modified version based on this paper by Liu Ziyin, Tilman Hartwig, Masahito Ueda: + https://arxiv.org/abs/2006.08195 + Examples: + >>> a1 = snakebeta(256) + >>> x = torch.randn(256) + >>> x = a1(x) + ''' + + def __init__(self, in_features, alpha=1.0, alpha_trainable=True, alpha_logscale=False): + ''' + Initialization. + INPUT: + - in_features: shape of the input + - alpha - trainable parameter that controls frequency + - beta - trainable parameter that controls magnitude + alpha is initialized to 1 by default, higher values = higher-frequency. + beta is initialized to 1 by default, higher values = higher-magnitude. + alpha will be trained along with the rest of your model. + ''' + super(SnakeBeta, self).__init__() + self.in_features = in_features + # initialize alpha + self.alpha_logscale = alpha_logscale + if self.alpha_logscale: # log scale alphas initialized to zeros + self.alpha = Parameter(torch.zeros(in_features) * alpha) + self.beta = Parameter(torch.zeros(in_features) * alpha) + else: # linear scale alphas initialized to ones + self.alpha = Parameter(torch.ones(in_features) * alpha) + self.beta = Parameter(torch.ones(in_features) * alpha) + self.alpha.requires_grad = alpha_trainable + self.beta.requires_grad = alpha_trainable + self.no_div_by_zero = 0.000000001 + + def forward(self, x): + ''' + Forward pass of the function. + Applies the function to the input elementwise. + SnakeBeta ∶= x + 1/b * sin^2 (xa) + ''' + alpha = self.alpha.unsqueeze( + 0).unsqueeze(-1) # line up with x to [B, C, T] + beta = self.beta.unsqueeze(0).unsqueeze(-1) + if self.alpha_logscale: + alpha = torch.exp(alpha) + beta = torch.exp(beta) + x = x + (1.0 / (beta + self.no_div_by_zero)) * pow(sin(x * alpha), 2) + return x + + +class AMPBlock(torch.nn.Module): + def __init__(self, h, channels, kernel_size=3, dilation=(1, 3, 5)): + super(AMPBlock, self).__init__() + self.h = h + self.convs1 = nn.ModuleList([ + weight_norm(Conv1d(channels, channels, kernel_size, 1, dilation=dilation[0], + padding=get_padding(kernel_size, dilation[0]))), + weight_norm(Conv1d(channels, channels, kernel_size, 1, dilation=dilation[1], + padding=get_padding(kernel_size, dilation[1]))), + weight_norm(Conv1d(channels, channels, kernel_size, 1, dilation=dilation[2], + padding=get_padding(kernel_size, dilation[2]))) + ]) + self.convs1.apply(init_weights) + + self.convs2 = nn.ModuleList([ + weight_norm(Conv1d(channels, channels, kernel_size, 1, dilation=1, + padding=get_padding(kernel_size, 1))), + weight_norm(Conv1d(channels, channels, kernel_size, 1, dilation=1, + padding=get_padding(kernel_size, 1))), + weight_norm(Conv1d(channels, channels, kernel_size, 1, dilation=1, + padding=get_padding(kernel_size, 1))) + ]) + self.convs2.apply(init_weights) + + # total number of conv layers + self.num_layers = len(self.convs1) + len(self.convs2) + + # periodic nonlinearity with snakebeta function and anti-aliasing + self.activations = nn.ModuleList([ + Activation1d( + activation=SnakeBeta(channels, alpha_logscale=True)) + for _ in range(self.num_layers) + ]) + + def forward(self, x): + acts1, acts2 = self.activations[::2], self.activations[1::2] + for c1, c2, a1, a2 in zip(self.convs1, self.convs2, acts1, acts2): + xt = a1(x) + xt = c1(xt) + xt = a2(xt) + xt = c2(xt) + x = xt + x + return x + + def remove_weight_norm(self): + for l in self.convs1: + remove_weight_norm(l) + for l in self.convs2: + remove_weight_norm(l) \ No newline at end of file diff --git a/vits_decoder/discriminator.py b/vits_decoder/discriminator.py new file mode 100644 index 0000000000000000000000000000000000000000..576f8d0a3893d5c78b00d514961d6585298d0d86 --- /dev/null +++ b/vits_decoder/discriminator.py @@ -0,0 +1,32 @@ +import torch +import torch.nn as nn + +from .mpd import MultiPeriodDiscriminator +from .mrd import MultiResolutionDiscriminator +from omegaconf import OmegaConf + +class Discriminator(nn.Module): + def __init__(self, hp): + super(Discriminator, self).__init__() + self.MRD = MultiResolutionDiscriminator(hp) + self.MPD = MultiPeriodDiscriminator(hp) + + def forward(self, x): + return self.MRD(x), self.MPD(x) + +if __name__ == '__main__': + hp = OmegaConf.load('../config/default.yaml') + model = Discriminator(hp) + + x = torch.randn(3, 1, 16384) + print(x.shape) + + mrd_output, mpd_output = model(x) + for features, score in mpd_output: + for feat in features: + print(feat.shape) + print(score.shape) + + pytorch_total_params = sum(p.numel() for p in model.parameters() if p.requires_grad) + print(pytorch_total_params) + diff --git a/vits_decoder/generator.py b/vits_decoder/generator.py new file mode 100644 index 0000000000000000000000000000000000000000..e122ce33c0af295ab6a683a10cdf6314ca2702f3 --- /dev/null +++ b/vits_decoder/generator.py @@ -0,0 +1,201 @@ +import torch +import torch.nn as nn +import numpy as np + +from torch.nn import Conv1d +from torch.nn import ConvTranspose1d +from torch.nn.utils import weight_norm +from torch.nn.utils import remove_weight_norm + +from .nsf import SourceModuleHnNSF +from .bigv import init_weights, SnakeBeta, AMPBlock +from .alias import Activation1d + + +class SpeakerAdapter(nn.Module): + + def __init__(self, + speaker_dim, + adapter_dim, + epsilon=1e-5 + ): + super(SpeakerAdapter, self).__init__() + self.speaker_dim = speaker_dim + self.adapter_dim = adapter_dim + self.epsilon = epsilon + self.W_scale = nn.Linear(self.speaker_dim, self.adapter_dim) + self.W_bias = nn.Linear(self.speaker_dim, self.adapter_dim) + self.reset_parameters() + + def reset_parameters(self): + torch.nn.init.constant_(self.W_scale.weight, 0.0) + torch.nn.init.constant_(self.W_scale.bias, 1.0) + torch.nn.init.constant_(self.W_bias.weight, 0.0) + torch.nn.init.constant_(self.W_bias.bias, 0.0) + + def forward(self, x, speaker_embedding): + x = x.transpose(1, -1) + mean = x.mean(dim=-1, keepdim=True) + var = ((x - mean) ** 2).mean(dim=-1, keepdim=True) + std = (var + self.epsilon).sqrt() + y = (x - mean) / std + scale = self.W_scale(speaker_embedding) + bias = self.W_bias(speaker_embedding) + y *= scale.unsqueeze(1) + y += bias.unsqueeze(1) + y = y.transpose(1, -1) + return y + + +class Generator(torch.nn.Module): + # this is our main BigVGAN model. Applies anti-aliased periodic activation for resblocks. + def __init__(self, hp): + super(Generator, self).__init__() + self.hp = hp + self.num_kernels = len(hp.gen.resblock_kernel_sizes) + self.num_upsamples = len(hp.gen.upsample_rates) + # speaker adaper, 256 should change by what speaker encoder you use + self.adapter = SpeakerAdapter(hp.vits.spk_dim, hp.gen.upsample_input) + # pre conv + self.conv_pre = nn.utils.weight_norm( + Conv1d(hp.gen.upsample_input, hp.gen.upsample_initial_channel, 7, 1, padding=3)) + # nsf + self.f0_upsamp = torch.nn.Upsample( + scale_factor=np.prod(hp.gen.upsample_rates)) + self.m_source = SourceModuleHnNSF() + self.noise_convs = nn.ModuleList() + # transposed conv-based upsamplers. does not apply anti-aliasing + self.ups = nn.ModuleList() + for i, (u, k) in enumerate(zip(hp.gen.upsample_rates, hp.gen.upsample_kernel_sizes)): + # print(f'ups: {i} {k}, {u}, {(k - u) // 2}') + # base + self.ups.append(nn.ModuleList([ + weight_norm(ConvTranspose1d(hp.gen.upsample_initial_channel // (2 ** i), + hp.gen.upsample_initial_channel // ( + 2 ** (i + 1)), + k, u, padding=(k - u) // 2)) + ])) + # nsf + if i + 1 < len(hp.gen.upsample_rates): + stride_f0 = np.prod(hp.gen.upsample_rates[i + 1:]) + stride_f0 = int(stride_f0) + self.noise_convs.append( + Conv1d( + 1, + hp.gen.upsample_initial_channel // (2 ** (i + 1)), + kernel_size=stride_f0 * 2, + stride=stride_f0, + padding=stride_f0 // 2, + ) + ) + else: + self.noise_convs.append( + Conv1d(1, hp.gen.upsample_initial_channel // + (2 ** (i + 1)), kernel_size=1) + ) + + # residual blocks using anti-aliased multi-periodicity composition modules (AMP) + self.resblocks = nn.ModuleList() + for i in range(len(self.ups)): + ch = hp.gen.upsample_initial_channel // (2 ** (i + 1)) + for k, d in zip(hp.gen.resblock_kernel_sizes, hp.gen.resblock_dilation_sizes): + self.resblocks.append(AMPBlock(hp, ch, k, d)) + + # post conv + activation_post = SnakeBeta(ch, alpha_logscale=True) + self.activation_post = Activation1d(activation=activation_post) + self.conv_post = weight_norm(Conv1d(ch, 1, 7, 1, padding=3)) + + # weight initialization + for i in range(len(self.ups)): + self.ups[i].apply(init_weights) + self.conv_post.apply(init_weights) + + def forward(self, spk, x, f0): + # adapter + x = self.adapter(x, spk) + # nsf + f0 = f0[:, None] + f0 = self.f0_upsamp(f0).transpose(1, 2) + har_source = self.m_source(f0) + har_source = har_source.transpose(1, 2) + x = self.conv_pre(x) + + for i in range(self.num_upsamples): + # upsampling + for i_up in range(len(self.ups[i])): + x = self.ups[i][i_up](x) + # nsf + x_source = self.noise_convs[i](har_source) + x = x + x_source + # AMP blocks + xs = None + for j in range(self.num_kernels): + if xs is None: + xs = self.resblocks[i * self.num_kernels + j](x) + else: + xs += self.resblocks[i * self.num_kernels + j](x) + x = xs / self.num_kernels + + # post conv + x = self.activation_post(x) + x = self.conv_post(x) + x = torch.tanh(x) + return x + + def remove_weight_norm(self): + for l in self.ups: + for l_i in l: + remove_weight_norm(l_i) + for l in self.resblocks: + l.remove_weight_norm() + remove_weight_norm(self.conv_pre) + remove_weight_norm(self.conv_post) + + def eval(self, inference=False): + super(Generator, self).eval() + # don't remove weight norm while validation in training loop + if inference: + self.remove_weight_norm() + + def pitch2source(self, f0): + f0 = f0[:, None] + f0 = self.f0_upsamp(f0).transpose(1, 2) # [1,len,1] + har_source = self.m_source(f0) + har_source = har_source.transpose(1, 2) # [1,1,len] + return har_source + + def source2wav(self, audio): + MAX_WAV_VALUE = 32768.0 + audio = audio.squeeze() + audio = MAX_WAV_VALUE * audio + audio = audio.clamp(min=-MAX_WAV_VALUE, max=MAX_WAV_VALUE-1) + audio = audio.short() + return audio.cpu().detach().numpy() + + def inference(self, spk, x, har_source): + # adapter + x = self.adapter(x, spk) + x = self.conv_pre(x) + + for i in range(self.num_upsamples): + # upsampling + for i_up in range(len(self.ups[i])): + x = self.ups[i][i_up](x) + # nsf + x_source = self.noise_convs[i](har_source) + x = x + x_source + # AMP blocks + xs = None + for j in range(self.num_kernels): + if xs is None: + xs = self.resblocks[i * self.num_kernels + j](x) + else: + xs += self.resblocks[i * self.num_kernels + j](x) + x = xs / self.num_kernels + + # post conv + x = self.activation_post(x) + x = self.conv_post(x) + x = torch.tanh(x) + return x diff --git a/vits_decoder/mpd.py b/vits_decoder/mpd.py new file mode 100644 index 0000000000000000000000000000000000000000..2dc63e859dd2920f9d02b285ebc4dae8cf318d6a --- /dev/null +++ b/vits_decoder/mpd.py @@ -0,0 +1,61 @@ +import torch +import torch.nn as nn +import torch.nn.functional as F +from torch.nn.utils import weight_norm, spectral_norm + +class DiscriminatorP(nn.Module): + def __init__(self, hp, period): + super(DiscriminatorP, self).__init__() + + self.LRELU_SLOPE = hp.mpd.lReLU_slope + self.period = period + + kernel_size = hp.mpd.kernel_size + stride = hp.mpd.stride + norm_f = weight_norm if hp.mpd.use_spectral_norm == False else spectral_norm + + self.convs = nn.ModuleList([ + norm_f(nn.Conv2d(1, 64, (kernel_size, 1), (stride, 1), padding=(kernel_size // 2, 0))), + norm_f(nn.Conv2d(64, 128, (kernel_size, 1), (stride, 1), padding=(kernel_size // 2, 0))), + norm_f(nn.Conv2d(128, 256, (kernel_size, 1), (stride, 1), padding=(kernel_size // 2, 0))), + norm_f(nn.Conv2d(256, 512, (kernel_size, 1), (stride, 1), padding=(kernel_size // 2, 0))), + norm_f(nn.Conv2d(512, 1024, (kernel_size, 1), 1, padding=(kernel_size // 2, 0))), + ]) + self.conv_post = norm_f(nn.Conv2d(1024, 1, (3, 1), 1, padding=(1, 0))) + + def forward(self, x): + fmap = [] + + # 1d to 2d + b, c, t = x.shape + if t % self.period != 0: # pad first + n_pad = self.period - (t % self.period) + x = F.pad(x, (0, n_pad), "reflect") + t = t + n_pad + x = x.view(b, c, t // self.period, self.period) + + for l in self.convs: + x = l(x) + x = F.leaky_relu(x, self.LRELU_SLOPE) + fmap.append(x) + x = self.conv_post(x) + fmap.append(x) + x = torch.flatten(x, 1, -1) + + return fmap, x + + +class MultiPeriodDiscriminator(nn.Module): + def __init__(self, hp): + super(MultiPeriodDiscriminator, self).__init__() + + self.discriminators = nn.ModuleList( + [DiscriminatorP(hp, period) for period in hp.mpd.periods] + ) + + def forward(self, x): + ret = list() + for disc in self.discriminators: + ret.append(disc(x)) + + return ret # [(feat, score), (feat, score), (feat, score), (feat, score), (feat, score)] diff --git a/vits_decoder/mrd.py b/vits_decoder/mrd.py new file mode 100644 index 0000000000000000000000000000000000000000..da6db1a416366603d2e65b400d66c44262e2baef --- /dev/null +++ b/vits_decoder/mrd.py @@ -0,0 +1,62 @@ +import torch +import torch.nn as nn +import torch.nn.functional as F +from torch.nn.utils import weight_norm, spectral_norm + +class DiscriminatorR(torch.nn.Module): + def __init__(self, hp, resolution): + super(DiscriminatorR, self).__init__() + + self.resolution = resolution + self.LRELU_SLOPE = hp.mpd.lReLU_slope + + norm_f = weight_norm if hp.mrd.use_spectral_norm == False else spectral_norm + + self.convs = nn.ModuleList([ + norm_f(nn.Conv2d(1, 32, (3, 9), padding=(1, 4))), + norm_f(nn.Conv2d(32, 32, (3, 9), stride=(1, 2), padding=(1, 4))), + norm_f(nn.Conv2d(32, 32, (3, 9), stride=(1, 2), padding=(1, 4))), + norm_f(nn.Conv2d(32, 32, (3, 9), stride=(1, 2), padding=(1, 4))), + norm_f(nn.Conv2d(32, 32, (3, 3), padding=(1, 1))), + ]) + self.conv_post = norm_f(nn.Conv2d(32, 1, (3, 3), padding=(1, 1))) + + def forward(self, x): + fmap = [] + + x = self.spectrogram(x) + x = x.unsqueeze(1) + for l in self.convs: + x = l(x) + x = F.leaky_relu(x, self.LRELU_SLOPE) + fmap.append(x) + x = self.conv_post(x) + fmap.append(x) + x = torch.flatten(x, 1, -1) + + return fmap, x + + def spectrogram(self, x): + n_fft, hop_length, win_length = self.resolution + x = F.pad(x, (int((n_fft - hop_length) / 2), int((n_fft - hop_length) / 2)), mode='reflect') + x = x.squeeze(1) + x = torch.stft(x, n_fft=n_fft, hop_length=hop_length, win_length=win_length, center=False, return_complex=False) #[B, F, TT, 2] + mag = torch.norm(x, p=2, dim =-1) #[B, F, TT] + + return mag + + +class MultiResolutionDiscriminator(torch.nn.Module): + def __init__(self, hp): + super(MultiResolutionDiscriminator, self).__init__() + self.resolutions = eval(hp.mrd.resolutions) + self.discriminators = nn.ModuleList( + [DiscriminatorR(hp, resolution) for resolution in self.resolutions] + ) + + def forward(self, x): + ret = list() + for disc in self.discriminators: + ret.append(disc(x)) + + return ret # [(feat, score), (feat, score), (feat, score)] diff --git a/vits_decoder/nsf.py b/vits_decoder/nsf.py new file mode 100644 index 0000000000000000000000000000000000000000..88b9c22f9d296a869ac6e94618910c3264d006fd --- /dev/null +++ b/vits_decoder/nsf.py @@ -0,0 +1,409 @@ +import torch +import numpy as np +import sys +import torch.nn.functional as torch_nn_func + + +class PulseGen(torch.nn.Module): + """Definition of Pulse train generator + + There are many ways to implement pulse generator. + Here, PulseGen is based on SinGen. For a perfect + """ + + def __init__(self, samp_rate, pulse_amp=0.1, noise_std=0.003, voiced_threshold=0): + super(PulseGen, self).__init__() + self.pulse_amp = pulse_amp + self.sampling_rate = samp_rate + self.voiced_threshold = voiced_threshold + self.noise_std = noise_std + self.l_sinegen = SineGen( + self.sampling_rate, + harmonic_num=0, + sine_amp=self.pulse_amp, + noise_std=0, + voiced_threshold=self.voiced_threshold, + flag_for_pulse=True, + ) + + def forward(self, f0): + """Pulse train generator + pulse_train, uv = forward(f0) + input F0: tensor(batchsize=1, length, dim=1) + f0 for unvoiced steps should be 0 + output pulse_train: tensor(batchsize=1, length, dim) + output uv: tensor(batchsize=1, length, 1) + + Note: self.l_sine doesn't make sure that the initial phase of + a voiced segment is np.pi, the first pulse in a voiced segment + may not be at the first time step within a voiced segment + """ + with torch.no_grad(): + sine_wav, uv, noise = self.l_sinegen(f0) + + # sine without additive noise + pure_sine = sine_wav - noise + + # step t corresponds to a pulse if + # sine[t] > sine[t+1] & sine[t] > sine[t-1] + # & sine[t-1], sine[t+1], and sine[t] are voiced + # or + # sine[t] is voiced, sine[t-1] is unvoiced + # we use torch.roll to simulate sine[t+1] and sine[t-1] + sine_1 = torch.roll(pure_sine, shifts=1, dims=1) + uv_1 = torch.roll(uv, shifts=1, dims=1) + uv_1[:, 0, :] = 0 + sine_2 = torch.roll(pure_sine, shifts=-1, dims=1) + uv_2 = torch.roll(uv, shifts=-1, dims=1) + uv_2[:, -1, :] = 0 + + loc = (pure_sine > sine_1) * (pure_sine > sine_2) \ + * (uv_1 > 0) * (uv_2 > 0) * (uv > 0) \ + + (uv_1 < 1) * (uv > 0) + + # pulse train without noise + pulse_train = pure_sine * loc + + # additive noise to pulse train + # note that noise from sinegen is zero in voiced regions + pulse_noise = torch.randn_like(pure_sine) * self.noise_std + + # with additive noise on pulse, and unvoiced regions + pulse_train += pulse_noise * loc + pulse_noise * (1 - uv) + return pulse_train, sine_wav, uv, pulse_noise + + +class SignalsConv1d(torch.nn.Module): + """Filtering input signal with time invariant filter + Note: FIRFilter conducted filtering given fixed FIR weight + SignalsConv1d convolves two signals + Note: this is based on torch.nn.functional.conv1d + + """ + + def __init__(self): + super(SignalsConv1d, self).__init__() + + def forward(self, signal, system_ir): + """output = forward(signal, system_ir) + + signal: (batchsize, length1, dim) + system_ir: (length2, dim) + + output: (batchsize, length1, dim) + """ + if signal.shape[-1] != system_ir.shape[-1]: + print("Error: SignalsConv1d expects shape:") + print("signal (batchsize, length1, dim)") + print("system_id (batchsize, length2, dim)") + print("But received signal: {:s}".format(str(signal.shape))) + print(" system_ir: {:s}".format(str(system_ir.shape))) + sys.exit(1) + padding_length = system_ir.shape[0] - 1 + groups = signal.shape[-1] + + # pad signal on the left + signal_pad = torch_nn_func.pad(signal.permute(0, 2, 1), (padding_length, 0)) + # prepare system impulse response as (dim, 1, length2) + # also flip the impulse response + ir = torch.flip(system_ir.unsqueeze(1).permute(2, 1, 0), dims=[2]) + # convolute + output = torch_nn_func.conv1d(signal_pad, ir, groups=groups) + return output.permute(0, 2, 1) + + +class CyclicNoiseGen_v1(torch.nn.Module): + """CyclicnoiseGen_v1 + Cyclic noise with a single parameter of beta. + Pytorch v1 implementation assumes f_t is also fixed + """ + + def __init__(self, samp_rate, noise_std=0.003, voiced_threshold=0): + super(CyclicNoiseGen_v1, self).__init__() + self.samp_rate = samp_rate + self.noise_std = noise_std + self.voiced_threshold = voiced_threshold + + self.l_pulse = PulseGen( + samp_rate, + pulse_amp=1.0, + noise_std=noise_std, + voiced_threshold=voiced_threshold, + ) + self.l_conv = SignalsConv1d() + + def noise_decay(self, beta, f0mean): + """decayed_noise = noise_decay(beta, f0mean) + decayed_noise = n[t]exp(-t * f_mean / beta / samp_rate) + + beta: (dim=1) or (batchsize=1, 1, dim=1) + f0mean (batchsize=1, 1, dim=1) + + decayed_noise (batchsize=1, length, dim=1) + """ + with torch.no_grad(): + # exp(-1.0 n / T) < 0.01 => n > -log(0.01)*T = 4.60*T + # truncate the noise when decayed by -40 dB + length = 4.6 * self.samp_rate / f0mean + length = length.int() + time_idx = torch.arange(0, length, device=beta.device) + time_idx = time_idx.unsqueeze(0).unsqueeze(2) + time_idx = time_idx.repeat(beta.shape[0], 1, beta.shape[2]) + + noise = torch.randn(time_idx.shape, device=beta.device) + + # due to Pytorch implementation, use f0_mean as the f0 factor + decay = torch.exp(-time_idx * f0mean / beta / self.samp_rate) + return noise * self.noise_std * decay + + def forward(self, f0s, beta): + """Producde cyclic-noise""" + # pulse train + pulse_train, sine_wav, uv, noise = self.l_pulse(f0s) + pure_pulse = pulse_train - noise + + # decayed_noise (length, dim=1) + if (uv < 1).all(): + # all unvoiced + cyc_noise = torch.zeros_like(sine_wav) + else: + f0mean = f0s[uv > 0].mean() + + decayed_noise = self.noise_decay(beta, f0mean)[0, :, :] + # convolute + cyc_noise = self.l_conv(pure_pulse, decayed_noise) + + # add noise in invoiced segments + cyc_noise = cyc_noise + noise * (1.0 - uv) + return cyc_noise, pulse_train, sine_wav, uv, noise + + +class SineGen(torch.nn.Module): + """Definition of sine generator + SineGen(samp_rate, harmonic_num = 0, + sine_amp = 0.1, noise_std = 0.003, + voiced_threshold = 0, + flag_for_pulse=False) + + samp_rate: sampling rate in Hz + harmonic_num: number of harmonic overtones (default 0) + sine_amp: amplitude of sine-wavefrom (default 0.1) + noise_std: std of Gaussian noise (default 0.003) + voiced_thoreshold: F0 threshold for U/V classification (default 0) + flag_for_pulse: this SinGen is used inside PulseGen (default False) + + Note: when flag_for_pulse is True, the first time step of a voiced + segment is always sin(np.pi) or cos(0) + """ + + def __init__( + self, + samp_rate, + harmonic_num=0, + sine_amp=0.1, + noise_std=0.003, + voiced_threshold=0, + flag_for_pulse=False, + ): + super(SineGen, self).__init__() + self.sine_amp = sine_amp + self.noise_std = noise_std + self.harmonic_num = harmonic_num + self.dim = self.harmonic_num + 1 + self.sampling_rate = samp_rate + self.voiced_threshold = voiced_threshold + self.flag_for_pulse = flag_for_pulse + + def _f02uv(self, f0): + # generate uv signal + uv = torch.ones_like(f0) + uv = uv * (f0 > self.voiced_threshold) + return uv + + def _f02sine(self, f0_values): + """f0_values: (batchsize, length, dim) + where dim indicates fundamental tone and overtones + """ + # convert to F0 in rad. The interger part n can be ignored + # because 2 * np.pi * n doesn't affect phase + rad_values = (f0_values / self.sampling_rate) % 1 + + # initial phase noise (no noise for fundamental component) + rand_ini = torch.rand( + f0_values.shape[0], f0_values.shape[2], device=f0_values.device + ) + rand_ini[:, 0] = 0 + rad_values[:, 0, :] = rad_values[:, 0, :] + rand_ini + + # instantanouse phase sine[t] = sin(2*pi \sum_i=1 ^{t} rad) + if not self.flag_for_pulse: + # for normal case + + # To prevent torch.cumsum numerical overflow, + # it is necessary to add -1 whenever \sum_k=1^n rad_value_k > 1. + # Buffer tmp_over_one_idx indicates the time step to add -1. + # This will not change F0 of sine because (x-1) * 2*pi = x * 2*pi + tmp_over_one = torch.cumsum(rad_values, 1) % 1 + tmp_over_one_idx = (tmp_over_one[:, 1:, :] - tmp_over_one[:, :-1, :]) < 0 + cumsum_shift = torch.zeros_like(rad_values) + cumsum_shift[:, 1:, :] = tmp_over_one_idx * -1.0 + + sines = torch.sin( + torch.cumsum(rad_values + cumsum_shift, dim=1) * 2 * np.pi + ) + else: + # If necessary, make sure that the first time step of every + # voiced segments is sin(pi) or cos(0) + # This is used for pulse-train generation + + # identify the last time step in unvoiced segments + uv = self._f02uv(f0_values) + uv_1 = torch.roll(uv, shifts=-1, dims=1) + uv_1[:, -1, :] = 1 + u_loc = (uv < 1) * (uv_1 > 0) + + # get the instantanouse phase + tmp_cumsum = torch.cumsum(rad_values, dim=1) + # different batch needs to be processed differently + for idx in range(f0_values.shape[0]): + temp_sum = tmp_cumsum[idx, u_loc[idx, :, 0], :] + temp_sum[1:, :] = temp_sum[1:, :] - temp_sum[0:-1, :] + # stores the accumulation of i.phase within + # each voiced segments + tmp_cumsum[idx, :, :] = 0 + tmp_cumsum[idx, u_loc[idx, :, 0], :] = temp_sum + + # rad_values - tmp_cumsum: remove the accumulation of i.phase + # within the previous voiced segment. + i_phase = torch.cumsum(rad_values - tmp_cumsum, dim=1) + + # get the sines + sines = torch.cos(i_phase * 2 * np.pi) + return sines + + def forward(self, f0): + """sine_tensor, uv = forward(f0) + input F0: tensor(batchsize=1, length, dim=1) + f0 for unvoiced steps should be 0 + output sine_tensor: tensor(batchsize=1, length, dim) + output uv: tensor(batchsize=1, length, 1) + """ + with torch.no_grad(): + f0_buf = torch.zeros(f0.shape[0], f0.shape[1], self.dim, device=f0.device) + # fundamental component + f0_buf[:, :, 0] = f0[:, :, 0] + for idx in np.arange(self.harmonic_num): + # idx + 2: the (idx+1)-th overtone, (idx+2)-th harmonic + f0_buf[:, :, idx + 1] = f0_buf[:, :, 0] * (idx + 2) + + # generate sine waveforms + sine_waves = self._f02sine(f0_buf) * self.sine_amp + + # generate uv signal + # uv = torch.ones(f0.shape) + # uv = uv * (f0 > self.voiced_threshold) + uv = self._f02uv(f0) + + # noise: for unvoiced should be similar to sine_amp + # std = self.sine_amp/3 -> max value ~ self.sine_amp + # . for voiced regions is self.noise_std + noise_amp = uv * self.noise_std + (1 - uv) * self.sine_amp / 3 + noise = noise_amp * torch.randn_like(sine_waves) + + # first: set the unvoiced part to 0 by uv + # then: additive noise + sine_waves = sine_waves * uv + noise + return sine_waves + + +class SourceModuleCycNoise_v1(torch.nn.Module): + """SourceModuleCycNoise_v1 + SourceModule(sampling_rate, noise_std=0.003, voiced_threshod=0) + sampling_rate: sampling_rate in Hz + + noise_std: std of Gaussian noise (default: 0.003) + voiced_threshold: threshold to set U/V given F0 (default: 0) + + cyc, noise, uv = SourceModuleCycNoise_v1(F0_upsampled, beta) + F0_upsampled (batchsize, length, 1) + beta (1) + cyc (batchsize, length, 1) + noise (batchsize, length, 1) + uv (batchsize, length, 1) + """ + + def __init__(self, sampling_rate, noise_std=0.003, voiced_threshod=0): + super(SourceModuleCycNoise_v1, self).__init__() + self.sampling_rate = sampling_rate + self.noise_std = noise_std + self.l_cyc_gen = CyclicNoiseGen_v1(sampling_rate, noise_std, voiced_threshod) + + def forward(self, f0_upsamped, beta): + """ + cyc, noise, uv = SourceModuleCycNoise_v1(F0, beta) + F0_upsampled (batchsize, length, 1) + beta (1) + cyc (batchsize, length, 1) + noise (batchsize, length, 1) + uv (batchsize, length, 1) + """ + # source for harmonic branch + cyc, pulse, sine, uv, add_noi = self.l_cyc_gen(f0_upsamped, beta) + + # source for noise branch, in the same shape as uv + noise = torch.randn_like(uv) * self.noise_std / 3 + return cyc, noise, uv + + +class SourceModuleHnNSF(torch.nn.Module): + """SourceModule for hn-nsf + SourceModule(sampling_rate, harmonic_num=0, sine_amp=0.1, + add_noise_std=0.003, voiced_threshod=0) + sampling_rate: sampling_rate in Hz + harmonic_num: number of harmonic above F0 (default: 0) + sine_amp: amplitude of sine source signal (default: 0.1) + add_noise_std: std of additive Gaussian noise (default: 0.003) + note that amplitude of noise in unvoiced is decided + by sine_amp + voiced_threshold: threhold to set U/V given F0 (default: 0) + + Sine_source, noise_source = SourceModuleHnNSF(F0_sampled) + F0_sampled (batchsize, length, 1) + Sine_source (batchsize, length, 1) + noise_source (batchsize, length 1) + uv (batchsize, length, 1) + """ + + def __init__( + self, + sampling_rate=48000, + harmonic_num=10, + sine_amp=0.1, + add_noise_std=0.003, + voiced_threshod=0, + ): + super(SourceModuleHnNSF, self).__init__() + + self.sine_amp = sine_amp + self.noise_std = add_noise_std + + # to produce sine waveforms + self.l_sin_gen = SineGen( + sampling_rate, harmonic_num, sine_amp, add_noise_std, voiced_threshod + ) + + # to merge source harmonics into a single excitation + self.l_linear = torch.nn.Linear(harmonic_num + 1, 1) + self.l_tanh = torch.nn.Tanh() + + def forward(self, x): + """ + Sine_source, noise_source = SourceModuleHnNSF(F0_sampled) + F0_sampled (batchsize, length, 1) + Sine_source (batchsize, length, 1) + noise_source (batchsize, length 1) + """ + # source for harmonic branch + sine_wavs = self.l_sin_gen(x) + sine_merge = self.l_tanh(self.l_linear(sine_wavs)) + return sine_merge diff --git a/vits_pretrain/README.md b/vits_pretrain/README.md new file mode 100644 index 0000000000000000000000000000000000000000..5e13d08f9a9c3fbc1d21e06e760abb0dce647ef0 --- /dev/null +++ b/vits_pretrain/README.md @@ -0,0 +1,3 @@ +Path for: + + vits_pretrain.pt \ No newline at end of file diff --git a/vits_pretrain/sovits5.0-48k-debug.pth b/vits_pretrain/sovits5.0-48k-debug.pth new file mode 100644 index 0000000000000000000000000000000000000000..b00531e1557b5e6141159b04ef9294ffcf0de56a --- /dev/null +++ b/vits_pretrain/sovits5.0-48k-debug.pth @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:b2c3e49cebb2968266659507c80a007375f49d616ee216cf084cb3f87e93083d +size 67866609 diff --git a/whisper/LICENSE b/whisper/LICENSE new file mode 100644 index 0000000000000000000000000000000000000000..d25552598bb9c5400612159ed4bab92ce12a5ce5 --- /dev/null +++ b/whisper/LICENSE @@ -0,0 +1,21 @@ +MIT License + +Copyright (c) 2022 OpenAI + +Permission is hereby granted, free of charge, to any person obtaining a copy +of this software and associated documentation files (the "Software"), to deal +in the Software without restriction, including without limitation the rights +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell +copies of the Software, and to permit persons to whom the Software is +furnished to do so, subject to the following conditions: + +The above copyright notice and this permission notice shall be included in all +copies or substantial portions of the Software. + +THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE +SOFTWARE. diff --git a/whisper/README.md b/whisper/README.md new file mode 100644 index 0000000000000000000000000000000000000000..9ea3a38e58aa56be82a79e31461849083917babb --- /dev/null +++ b/whisper/README.md @@ -0,0 +1,147 @@ +# Whisper + +[[Blog]](https://openai.com/blog/whisper) +[[Paper]](https://arxiv.org/abs/2212.04356) +[[Model card]](https://github.com/openai/whisper/blob/main/model-card.md) +[[Colab example]](https://colab.research.google.com/github/openai/whisper/blob/master/notebooks/LibriSpeech.ipynb) + +Whisper is a general-purpose speech recognition model. It is trained on a large dataset of diverse audio and is also a multitasking model that can perform multilingual speech recognition, speech translation, and language identification. + + +## Approach + +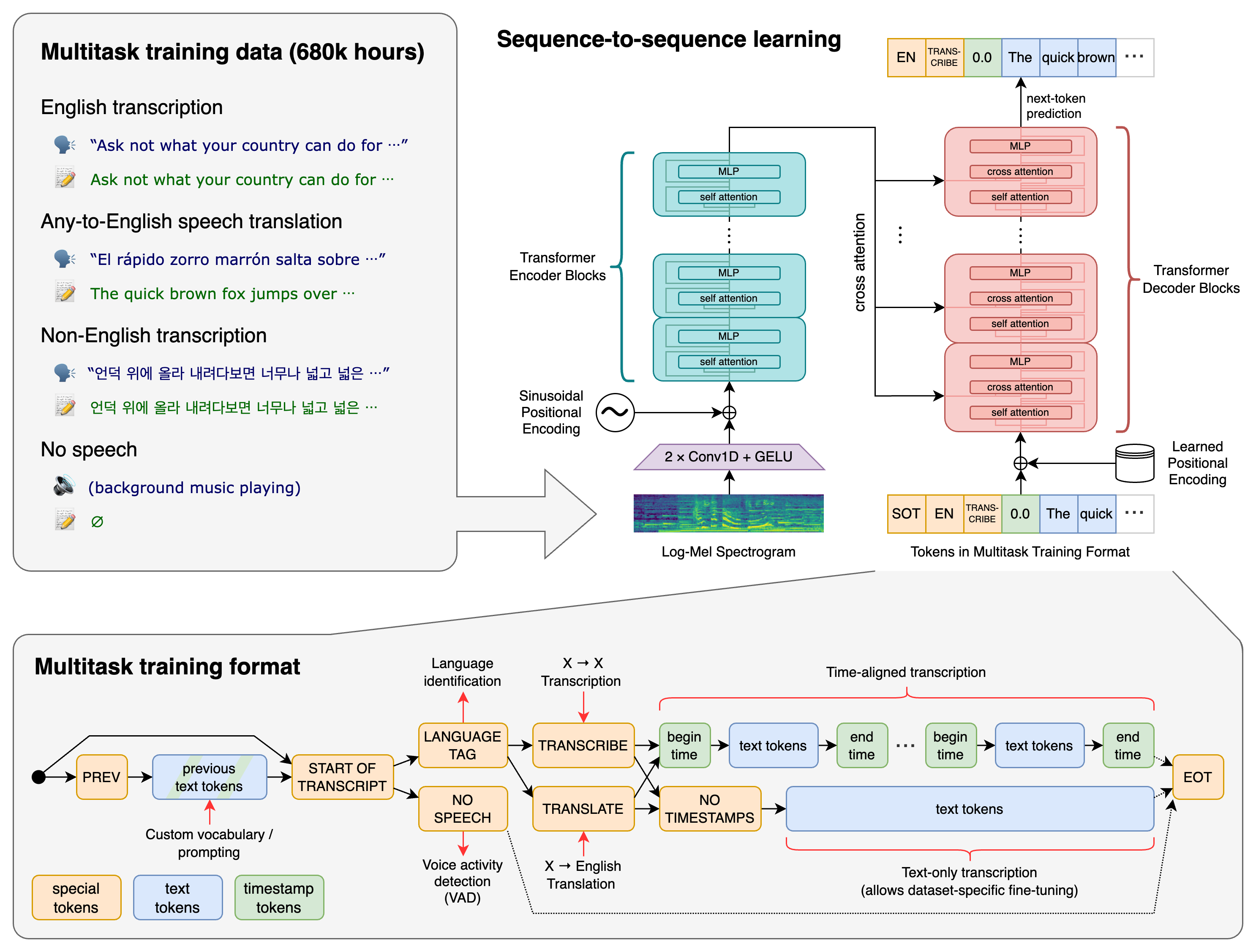 + +A Transformer sequence-to-sequence model is trained on various speech processing tasks, including multilingual speech recognition, speech translation, spoken language identification, and voice activity detection. These tasks are jointly represented as a sequence of tokens to be predicted by the decoder, allowing a single model to replace many stages of a traditional speech-processing pipeline. The multitask training format uses a set of special tokens that serve as task specifiers or classification targets. + + +## Setup + +We used Python 3.9.9 and [PyTorch](https://pytorch.org/) 1.10.1 to train and test our models, but the codebase is expected to be compatible with Python 3.8-3.10 and recent PyTorch versions. The codebase also depends on a few Python packages, most notably [HuggingFace Transformers](https://huggingface.co/docs/transformers/index) for their fast tokenizer implementation and [ffmpeg-python](https://github.com/kkroening/ffmpeg-python) for reading audio files. You can download and install (or update to) the latest release of Whisper with the following command: + + pip install -U openai-whisper + +Alternatively, the following command will pull and install the latest commit from this repository, along with its Python dependencies: + + pip install git+https://github.com/openai/whisper.git + +To update the package to the latest version of this repository, please run: + + pip install --upgrade --no-deps --force-reinstall git+https://github.com/openai/whisper.git + +It also requires the command-line tool [`ffmpeg`](https://ffmpeg.org/) to be installed on your system, which is available from most package managers: + +```bash +# on Ubuntu or Debian +sudo apt update && sudo apt install ffmpeg + +# on Arch Linux +sudo pacman -S ffmpeg + +# on MacOS using Homebrew (https://brew.sh/) +brew install ffmpeg + +# on Windows using Chocolatey (https://chocolatey.org/) +choco install ffmpeg + +# on Windows using Scoop (https://scoop.sh/) +scoop install ffmpeg +``` + +You may need [`rust`](http://rust-lang.org) installed as well, in case [tokenizers](https://pypi.org/project/tokenizers/) does not provide a pre-built wheel for your platform. If you see installation errors during the `pip install` command above, please follow the [Getting started page](https://www.rust-lang.org/learn/get-started) to install Rust development environment. Additionally, you may need to configure the `PATH` environment variable, e.g. `export PATH="$HOME/.cargo/bin:$PATH"`. If the installation fails with `No module named 'setuptools_rust'`, you need to install `setuptools_rust`, e.g. by running: + +```bash +pip install setuptools-rust +``` + + +## Available models and languages + +There are five model sizes, four with English-only versions, offering speed and accuracy tradeoffs. Below are the names of the available models and their approximate memory requirements and relative speed. + + +| Size | Parameters | English-only model | Multilingual model | Required VRAM | Relative speed | +|:------:|:----------:|:------------------:|:------------------:|:-------------:|:--------------:| +| tiny | 39 M | `tiny.en` | `tiny` | ~1 GB | ~32x | +| base | 74 M | `base.en` | `base` | ~1 GB | ~16x | +| small | 244 M | `small.en` | `small` | ~2 GB | ~6x | +| medium | 769 M | `medium.en` | `medium` | ~5 GB | ~2x | +| large | 1550 M | N/A | `large` | ~10 GB | 1x | + +The `.en` models for English-only applications tend to perform better, especially for the `tiny.en` and `base.en` models. We observed that the difference becomes less significant for the `small.en` and `medium.en` models. + +Whisper's performance varies widely depending on the language. The figure below shows a WER (Word Error Rate) breakdown by languages of the Fleurs dataset using the `large-v2` model. More WER and BLEU scores corresponding to the other models and datasets can be found in Appendix D in [the paper](https://arxiv.org/abs/2212.04356). The smaller, the better. + + + + + +## Command-line usage + +The following command will transcribe speech in audio files, using the `medium` model: + + whisper audio.flac audio.mp3 audio.wav --model medium + +The default setting (which selects the `small` model) works well for transcribing English. To transcribe an audio file containing non-English speech, you can specify the language using the `--language` option: + + whisper japanese.wav --language Japanese + +Adding `--task translate` will translate the speech into English: + + whisper japanese.wav --language Japanese --task translate + +Run the following to view all available options: + + whisper --help + +See [tokenizer.py](https://github.com/openai/whisper/blob/main/whisper/tokenizer.py) for the list of all available languages. + + +## Python usage + +Transcription can also be performed within Python: + +```python +import whisper + +model = whisper.load_model("base") +result = model.transcribe("audio.mp3") +print(result["text"]) +``` + +Internally, the `transcribe()` method reads the entire file and processes the audio with a sliding 30-second window, performing autoregressive sequence-to-sequence predictions on each window. + +Below is an example usage of `whisper.detect_language()` and `whisper.decode()` which provide lower-level access to the model. + +```python +import whisper + +model = whisper.load_model("base") + +# load audio and pad/trim it to fit 30 seconds +audio = whisper.load_audio("audio.mp3") +audio = whisper.pad_or_trim(audio) + +# make log-Mel spectrogram and move to the same device as the model +mel = whisper.log_mel_spectrogram(audio).to(model.device) + +# detect the spoken language +_, probs = model.detect_language(mel) +print(f"Detected language: {max(probs, key=probs.get)}") + +# decode the audio +options = whisper.DecodingOptions() +result = whisper.decode(model, mel, options) + +# print the recognized text +print(result.text) +``` + +## More examples + +Please use the [🙌 Show and tell](https://github.com/openai/whisper/discussions/categories/show-and-tell) category in Discussions for sharing more example usages of Whisper and third-party extensions such as web demos, integrations with other tools, ports for different platforms, etc. + + +## License + +Whisper's code and model weights are released under the MIT License. See [LICENSE](https://github.com/openai/whisper/blob/main/LICENSE) for further details. \ No newline at end of file diff --git a/whisper/__init__.py b/whisper/__init__.py new file mode 100644 index 0000000000000000000000000000000000000000..e69de29bb2d1d6434b8b29ae775ad8c2e48c5391 diff --git a/whisper/assets/gpt2/merges.txt b/whisper/assets/gpt2/merges.txt new file mode 100644 index 0000000000000000000000000000000000000000..6636bda4a1fd7a63653dffb22683b8162c8de956 --- /dev/null +++ b/whisper/assets/gpt2/merges.txt @@ -0,0 +1,50001 @@ +#version: 0.2 - Trained by `huggingface/tokenizers` +Ġ t +Ġ a +h e +i n +r e +o n +Ġt he +e r +Ġ s +a t +Ġ w +Ġ o +e n +Ġ c +i t +i s +a n +o r +e s +Ġ b +e d +Ġ f +in g +Ġ p +o u +Ġa n +a l +a r +Ġt o +Ġ m +Ġo f +Ġ in +Ġ d +Ġ h +Ġan d +i c +a s +l e +Ġt h +i on +o m +l l +en t +Ġ n +Ġ l +s t +Ġ re +v e +Ġ e +r o +l y +Ġb e +Ġ g +Ġ T +c t +Ġ S +i d +o t +Ġ I +u t +e t +Ġ A +Ġ is +Ġ on +i m +a m +o w +a y +a d +s e +Ġth at +Ġ C +i g +Ġf or +a c +Ġ y +v er +u r +Ġ u +l d +Ġs t +Ġ M +' s +Ġ he +Ġ it +at ion +it h +i r +c e +Ġy ou +i l +Ġ B +Ġw h +o l +Ġ P +Ġw ith +Ġ 1 +t er +c h +Ġa s +Ġw e +Ġ ( +n d +i ll +Ġ D +i f +Ġ 2 +a g +er s +k e +Ġ " +Ġ H +e m +Ġc on +Ġ W +Ġ R +he r +Ġw as +Ġ r +o d +Ġ F +u l +at e +Ġa t +r i +p p +o re +ĠT he +Ġs e +u s +Ġp ro +Ġh a +u m +Ġa re +Ġd e +a in +an d +Ġo r +ig h +es t +is t +a b +r om +Ġ N +t h +Ġc om +Ġ G +u n +o p +0 0 +Ġ L +Ġn ot +es s +Ġe x +Ġ v +re s +Ġ E +e w +it y +an t +Ġb y +e l +o s +or t +o c +q u +Ġf rom +Ġha ve +Ġs u +i ve +ou ld +Ġs h +Ġth is +n t +r a +p e +igh t +ar t +m ent +Ġa l +u st +en d +- - +al l +Ġ O +ac k +Ġc h +Ġ le +i es +re d +ar d +â Ģ +ou t +Ġ J +Ġa b +e ar +i v +al ly +ou r +o st +g h +p t +Ġp l +as t +Ġc an +a k +om e +u d +T he +Ġh is +Ġd o +Ġg o +Ġh as +g e +' t +Ġ U +r ou +Ġs a +Ġ j +Ġb ut +Ġw or +Ġa ll +e ct +Ġ k +am e +Ġw ill +o k +Ġw he +Ġthe y +id e +0 1 +f f +ic h +p l +t her +Ġt r +. . +Ġin t +i e +u re +ag e +Ġn e +i al +a p +in e +ic e +Ġm e +Ġo ut +an s +on e +on g +ion s +Ġwh o +Ġ K +Ġu p +Ġthe ir +Ġa d +Ġ 3 +Ġu s +at ed +ou s +Ġm ore +u e +o g +ĠS t +in d +i ke +Ġs o +im e +p er +. " +b er +i z +a ct +Ġon e +Ġsa id +Ġ - +a re +Ġyou r +c c +ĠT h +Ġc l +e p +a ke +ab le +i p +Ġcon t +Ġwh ich +i a +Ġ im +Ġab out +Ġwe re +ver y +u b +Ġh ad +Ġ en +Ġcom p +, " +ĠI n +Ġu n +Ġa g +i re +ac e +a u +ar y +Ġw ould +as s +r y +Ġ âĢ +c l +o ok +e re +s o +Ġ V +ig n +i b +Ġof f +Ġt e +v en +Ġ Y +i le +o se +it e +or m +Ġ2 01 +Ġre s +Ġm an +Ġp er +Ġo ther +or d +ul t +Ġbe en +Ġl ike +as e +an ce +k s +ay s +ow n +en ce +Ġd is +ct ion +Ġan y +Ġa pp +Ġs p +in t +res s +ation s +a il +Ġ 4 +ic al +Ġthe m +Ġhe r +ou nt +ĠC h +Ġa r +Ġ if +Ġthe re +Ġp e +Ġy ear +a v +Ġm y +Ġs ome +Ġwhe n +ou gh +ac h +Ġth an +r u +on d +ic k +Ġo ver +ve l +Ġ qu +Ċ Ċ +Ġs c +re at +re e +ĠI t +ou nd +p ort +Ġal so +Ġp art +f ter +Ġk n +Ġbe c +Ġt ime +en s +Ġ 5 +op le +Ġwh at +Ġn o +d u +m er +an g +Ġn ew +-- -- +Ġg et +or y +it ion +ing s +Ġj ust +Ġint o +Ġ 0 +ent s +o ve +t e +Ġpe ople +Ġp re +Ġit s +Ġre c +Ġt w +i an +ir st +ar k +or s +Ġwor k +ad e +o b +Ġs he +Ġo ur +w n +in k +l ic +Ġ1 9 +ĠH e +is h +nd er +au se +Ġh im +on s +Ġ [ +Ġ ro +f orm +i ld +at es +ver s +Ġon ly +o ll +Ġs pe +c k +e ll +am p +Ġa cc +Ġb l +i ous +ur n +f t +o od +Ġh ow +he d +Ġ ' +Ġa fter +a w +Ġat t +o v +n e +Ġpl ay +er v +ic t +Ġc ould +it t +Ġa m +Ġf irst +Ġ 6 +Ġa ct +Ġ $ +e c +h ing +u al +u ll +Ġcom m +o y +o ld +c es +at er +Ġf e +Ġbe t +w e +if f +Ġtw o +oc k +Ġb ack +) . +id ent +Ġu nder +rou gh +se l +x t +Ġm ay +rou nd +Ġp o +p h +is s +Ġd es +Ġm ost +Ġd id +Ġad d +j ect +Ġin c +f ore +Ġp ol +on t +Ġag ain +cl ud +ter n +Ġkn ow +Ġne ed +Ġcon s +Ġc o +Ġ . +Ġw ant +Ġse e +Ġ 7 +n ing +i ew +ĠTh is +c ed +Ġe ven +Ġin d +t y +ĠW e +at h +Ġthe se +Ġp r +Ġu se +Ġbec ause +Ġf l +n g +Ġn ow +ĠâĢ ĵ +c om +is e +Ġm ake +Ġthe n +ow er +Ġe very +ĠU n +Ġse c +os s +u ch +Ġe m +Ġ = +ĠR e +i ed +r it +Ġin v +le ct +Ġsu pp +at ing +Ġl ook +m an +pe ct +Ġ 8 +ro w +Ġb u +Ġwhe re +if ic +Ġyear s +i ly +Ġd iff +Ġsh ould +Ġre m +T h +I n +Ġe v +d ay +' re +ri b +Ġre l +s s +Ġde f +Ġr ight +Ġs y +) , +l es +00 0 +he n +Ġth rough +ĠT r +_ _ +Ġw ay +Ġd on +Ġ , +Ġ1 0 +as ed +Ġas s +ub lic +Ġre g +ĠA nd +i x +Ġ very +Ġin clud +ot her +Ġim p +ot h +Ġsu b +ĠâĢ Ķ +Ġbe ing +ar g +ĠW h += = +ib le +Ġdo es +an ge +r am +Ġ 9 +er t +p s +it ed +ation al +Ġb r +Ġd own +Ġman y +ak ing +Ġc all +ur ing +it ies +Ġp h +ic s +al s +Ġde c +at ive +en er +Ġbe fore +il ity +Ġwe ll +Ġm uch +ers on +Ġth ose +Ġsu ch +Ġ ke +Ġ end +ĠB ut +as on +t ing +Ġl ong +e f +Ġth ink +y s +Ġbe l +Ġs m +it s +a x +Ġo wn +Ġpro v +Ġs et +if e +ment s +b le +w ard +Ġsh ow +Ġp res +m s +om et +Ġo b +Ġs ay +ĠS h +t s +f ul +Ġe ff +Ġg u +Ġin st +u nd +re n +c ess +Ġ ent +ĠY ou +Ġgo od +Ġst art +in ce +Ġm ade +t t +st em +ol og +u p +Ġ | +um p +Ġhe l +ver n +ul ar +u ally +Ġa c +Ġm on +Ġl ast +Ġ2 00 +1 0 +Ġst ud +u res +ĠA r +sel f +ar s +mer ic +u es +c y +Ġm in +oll ow +Ġc ol +i o +Ġm od +Ġc ount +ĠC om +he s +Ġf in +a ir +i er +âĢ Ķ +re ad +an k +at ch +e ver +Ġst r +Ġpo int +or k +ĠN ew +Ġs ur +o ol +al k +em ent +Ġus ed +ra ct +we en +Ġs ame +ou n +ĠA l +c i +Ġdiff ere +Ġwh ile +---- ---- +Ġg ame +ce pt +Ġs im +.. . +Ġin ter +e k +Ġre port +Ġpro du +Ġst ill +l ed +a h +Ġhe re +Ġwor ld +Ġth ough +Ġn um +ar ch +im es +al e +ĠS e +ĠI f +/ / +ĠL e +Ġre t +Ġre f +Ġtr ans +n er +ut ion +ter s +Ġt ake +ĠC l +Ġcon f +w ay +a ve +Ġgo ing +Ġs l +u g +ĠA meric +Ġspe c +Ġh and +Ġbet ween +ist s +ĠD e +o ot +I t +Ġe ar +Ġagain st +Ġh igh +g an +a z +at her +Ġex p +Ġo p +Ġin s +Ġg r +Ġhel p +Ġre qu +et s +in s +ĠP ro +is m +Ġf ound +l and +at a +us s +am es +Ġp erson +Ġg reat +p r +Ġs ign +ĠA n +' ve +Ġs omet +Ġs er +h ip +Ġr un +Ġ : +Ġt er +ire ct +Ġf ollow +Ġd et +ic es +Ġf ind +1 2 +Ġm em +Ġc r +e red +e x +Ġex t +ut h +en se +c o +Ġte am +v ing +ou se +as h +at t +v ed +Ġsy stem +ĠA s +d er +iv es +m in +Ġle ad +ĠB l +c ent +Ġa round +Ġgo vern +Ġc ur +vel op +an y +Ġc our +al th +ag es +iz e +Ġc ar +od e +Ġl aw +Ġre ad +' m +c on +Ġre al +Ġsupp ort +Ġ1 2 +.. .. +Ġre ally +n ess +Ġf act +Ġd ay +Ġb oth +y ing +Ġs erv +ĠF or +Ġth ree +Ġw om +Ġm ed +od y +ĠThe y +5 0 +Ġex per +t on +Ġe ach +ak es +Ġc he +Ġc re +in es +Ġre p +1 9 +g g +ill ion +Ġg rou +ut e +i k +W e +g et +E R +Ġm et +Ġs ays +o x +Ġd uring +er n +iz ed +a red +Ġf am +ic ally +Ġha pp +ĠI s +Ġch ar +m ed +v ent +Ġg ener +i ent +p le +i et +re nt +1 1 +v es +pt ion +Ġ2 0 +form ation +Ġc or +Ġoff ic +ie ld +Ġto o +is ion +Ġin f +Ġ Z +t he +o ad +Ġp ublic +Ġpro g +r ic +* * +Ġw ar +Ġp ower +v iew +Ġf ew +Ġl oc +Ġdiffere nt +Ġst ate +Ġhe ad +' ll +Ġp oss +Ġst at +re t +ant s +Ġv al +Ġis s +Ġc le +i vers +an c +Ġex pl +Ġan other +Ġ Q +Ġa v +th ing +n ce +W h +Ġch ild +Ġs ince +i red +l ess +Ġl ife +Ġde velop +itt le +Ġde p +Ġp ass +ã ĥ +Ġt urn +or n +Th is +b ers +ro ss +ĠA d +Ġf r +Ġres p +Ġsec ond +o h +Ġ / +Ġdis c +Ġ & +Ġsomet hing +Ġcomp le +Ġ ed +Ġf il +Ġmon th +a j +u c +Ġgovern ment +Ġwith out +Ġle g +Ġd ist +Ġp ut +Ġqu est +an n +Ġpro t +2 0 +Ġne ver +i ence +Ġle vel +Ġar t +Ġth ings +Ġm ight +Ġeff ect +Ġcont ro +Ġc ent +Ġ1 8 +Ġall ow +Ġbel ie +ch ool +ot t +Ġinc re +Ġfe el +Ġres ult +Ġl ot +Ġf un +ot e +Ġt y +ere st +Ġcont in +Ġus ing +Ġb ig +2 01 +Ġas k +Ġb est +Ġ ) +I N +Ġo pp +3 0 +Ġnum ber +in ess +S t +le ase +Ġc a +Ġm ust +Ġd irect +Ġg l +Ġ < +Ġop en +Ġp ost +Ġcom e +Ġse em +ord ing +Ġwe ek +ate ly +it al +Ġe l +ri end +Ġf ar +Ġt ra +in al +Ġp ri +ĠU S +Ġpl ace +Ġfor m +Ġto ld +" : +ain s +at ure +ĠTr ump +Ġst and +Ġ # +id er +ĠF r +Ġne xt +Ġs oc +Ġp ur +Ġle t +Ġl ittle +Ġh um +Ġ i +r on +1 5 +Ġ1 5 +Ġcomm un +Ġm ark +ĠThe re +Ġw r +ĠTh at +Ġin formation +w ays +Ġb us +a pp +Ġinv est +m e +Ġh ard +ain ed +e ad +Ġim port +Ġapp ro +Ġt est +Ġt ri +Ġre st +os ed +Ġf ull +Ġc are +ĠS p +Ġc ase +O N +Ġs k +Ġl ess +Ġ + +Ġpart ic +ĠP l +ab ly +u ck +is hed +ch n +b e +Ġl ist +at or +Ġto p +Ġad v +ĠB e +ru ct +Ġd em +r ation +l ing +g y +re en +g er +Ġh ome +Ġle ft +Ġbet ter +Ġd ata +Ġ1 1 +Ġatt ack +Ġpro ble +l ine +ard s +Ġbe h +r al +ĠH ow +ĠS he +ar ge +Ġ -- +: // +Ġb ro +ĠP h +at s +Ġbu ild +w w +id ed +a im +as es +en cy +Ġm ain +in ed +Ġinclud ing +Ġ { +Ġg ot +Ġint erest +Ġke ep +Ġ X +Ġe as +ain ing +Ġcl ass +âĢ ¦ +ĠN o +Ġv ar +Ġsm all +amp le +A T +Ġ ide +ĠS o +Ġre ce +Ġpol it +Ġm ov +Ġpl an +Ġper cent +iv ing +Ġc amp +Ġp ay +1 4 +s c +is ed +Ġu nt +one y +pl oy +== == +Ġdid n +ĠI nd +el s +ert ain +Ġp os +__ __ +i ver +Ġpro cess +Ġprog ram +if ied +ĠR ep +1 6 +u ro +olog y +at ter +in a +Ġn ame +ĠA ll +Ġf our +Ġret urn +v ious +b s +Ġcall ed +Ġm ove +ĠS c +ir d +Ġgrou p +Ġb re +Ġm en +Ġc ap +t en +e e +Ġd ri +le g +he re +uth or +Ġp at +Ġcur rent +id es +Ġp op +t o +ent ion +Ġal ways +Ġm il +Ġwom en +Ġ1 6 +Ġo ld +iv en +ra ph +ĠO r +r or +ent ly +Ġn ear +ĠE x +re am +s h +Ġ1 4 +Ġf ree +iss ion +st and +ĠC on +al ity +us ed +1 3 +Ġdes ign +Ġch ange +Ġch ang +Ġb o +Ġv is +em ber +Ġb ook +read y +Ġk ill +2 5 +pp ed +Ġa way +Ġab le +Ġcount ry +Ġcon st +ar n +Ġor der +A R +i or +i um +or th +1 8 +ail able +Ġs w +Ġm illion +Ġ1 3 +at ic +t ed +ĠG o +Ġo per +en g +Ġth ing +aj or +con om +ĠCom m +Ġwh y +u red +ur al +Ġs chool +b y +ĠM ar +Ġa ff +Ġd ays +Ġan n +us h +an e +I f +e g +Ġpro f +Ġhe alth +ou th +B ut +ion al +. , +Ġs ol +Ġal ready +Ġ3 0 +Ġchar act +H e +Ġf riend +E S +i ans +ic le +' d +ĠO n +Ġle ast +Ġp rom +Ġd r +Ġh ist +it her +Ġ est +i qu +1 7 +s on +Ġte ll +Ġt alk +oh n +o int +le ction +A N +Ġunt il +au gh +Ġl ater +Ġ ve +Ġv iew +end ing +iv ed +Ġwor d +w are +Ġc ost +Ġen ough +Ġg ive +ĠUn ited +Ġte chn +are nt +O R +Ġp ar +ĠD r +Ġ201 6 +r ist +er ing +Ġ  +Ġl arge +s ide +ac y +cc ess +Ġw in +Ġimport ant +Ġ19 9 +Ġdoes n +Ġ1 7 +Ġbus iness +Ġcle ar +Ġre se +" , +ur y +Ġe qu +as ter +al f +ĠAmeric an +n ect +Ġex pect +ivers ity +Ġo cc +ĠF l +Ġk ind +Ġme an +Ġp ast +Ġde v +Ġb as +le t +ra ft +Ġor gan +Ġde l +Ġper form +Ġst ory +Ġse ason +ĠC ol +Ġcl aim +Ġc ame +Ġwith in +Ġl ine +Ġpro ject +ĠA t +Ġcontro l +end ed +ĠS y +Ġa ir +iz ation +Ġ * +le y +Ġm oney +id d +Y ou +f or +Ġfam ily +Ġm aking +Ġb it +Ġpol ice +Ġhapp en +Ġ vers +on y +u ff +ĠW hen +Ġs it +ide o +l f +is on +Ġsu re +g in +Ġapp ear +Ġl ight +Ġ es +o f +Ġw ater +Ġt imes +n ot +Ġg row +Ġcomp any +ĠT e +ow s +Ġm ar +our ce +i ol +ar m +b r +Ġex ample +Ġcon c +Ġf ore +ĠT o +p ro +E N +ri es +Ġ2 5 +ĠC an +ne y +Ġact ually +Ġe ver +ur ity +ak en +ap s +Ġt ax +Ġm ajor +am a +Ġof ten +er al +Ġhum an +Ġj ob +is ter +Ġav ailable +oc r +en n +a id +iv id +Ġrec ord +? " +Ġs ing +ĠA m +id ence +Ġnew s +st er +Ġe conom +Ġfollow ing +ĠB r +is ing +Ġh our +m ost +um ent +Ġse x +Ġdes c +Ġbec ome +ĠE d +Ġto ok +Ġha ving +Ġprodu ct +a ult +A s +ar ing +Ġme ans +Ġh op +un e +Ġch o +Ġc ertain +Ġn on +Ġde al +2 4 +le ment +oc i +en e +Ġs ide +ĠP r +ĠM ay +Ġre ason +u ed +c hed +ul ation +Ġe lect +Ġoffic ial +Ġposs ible +Ġh old +and s +ot s +Ġc ity +or ies +Ġse ver +Ġchild ren +Ġon ce +Ġact iv +l er +Ġn ight +it ions +ĠJ ohn +a pe +pl ay +Ġd one +Ġl im +Ġwork ing +ĠP res +or ld +e b +ĠC o +Ġb ody +ail s +ut es +ĠM r +Ġwhe ther +Ġa uthor +ro p +Ġpro per +Ġse en +) ; +Ġf ac +ĠS u +Ġcon d +it ing +Ġcour se +Ġ } +-------- -------- +a ign +Ġev ent +Ġen g +Ġp ot +Ġin tern +i am +Ġsh ort +em pt +ã Ĥ +ĠG od +il ar +8 0 +Ġor ig +I S +our n +ab ility +it ive +Ġd am +Ġ1 00 +Ġp ress +Ġdo ing +Ġprot ect +r ing +Ġthough t +Ġquest ion +re w +ĠW ar +Ġsever al +ĠSt ate +Ġg iven +Ġf und +ĠT w +Ġw ent +an ces +w ork +p or +m y +4 0 +Ġar g +art ment +ust om +Ġpol ic +Ġme et +Ġc reat +2 2 +ĠSt ates +Ġg ames +ra w +ut ure +Ġunder stand +ur s +ĠO b +l ish +s y +Ġm akes +Ġw on +ag on +Ġh tt +Ġl ove +ent ial +Ġcomple te +p ar +ĠI m +A L +Ġacc ount + ł +ore d +ver t +Ġ ident +Ġ201 5 +Ġother s +ĠM in +i ber +ver age +The re +ition al +d d +Ġpro b +Ġyou ng +Ġal ong +Ġacc ording +Ġy et +Ġmem bers +ĠWh at +o id +ĠM an +A nd +Ġam ong +a i +Ġem ploy +ĠR es +Ġ > +Ġinv ol +Ġl ow +a f +ĠC ar +Ġh ig +ĠO ne +ĠS ec +in ation +Ġlike ly +Ġan t +ag ed +ĠR uss +Ġb en +Ġre le +F or +b ack +ĠN ot +Ġpres ident +b all +Ġacc ess +ivid ual +ĠD em +ĠE uro +6 0 +Ġkn own +ir l +ĠG r +Ġear ly +u se +iet y +âĢ ĵ +Ġf ight +Ġs ent +Ġto day +Ġmark et +" . +Ġb ased +Ġstr ong +ur ther +Ġde b +m ber +Ġproble m +Ġde ath +Ġsoc ial +im ate +A S +ort un +Ġcamp aign +er y +C h +Ġe y +i ally +Ġm us +w h +p os +Ġ er +Ġsa f +Ġmonth s +ir on +Ġv iol +Ġf ive +Ġst re +Ġplay ers +in c +al d +y ear +a un +Ġsu ccess +Ġpres ent +ere nce +Ġ201 4 +Ġsu gg +Ġpartic ular +Ġtr y +Ġsugg est +ĠCh rist +on es +Ġpri v +2 3 +Ġc rit +Ġl and +Ġloc al +if y +2 9 +Ġa ut +E D +ĠG u +Ġm ult +Ġpolit ical +Ġask ed +Ġfor mer +it ter +ri pt +Ġcl ose +Ġp ract +ĠY ork +Ġget ting +Ġac ross +Ġcom b +Ġbelie ve +Ġ z +Ġto get +Ġtoget her +ĠC ent +ir c +Ġind ividual +ĠM c +2 7 +is k +ĠE ng +Ġf ace +Ġ2 4 +Ġval ue +Ġare a +e v +Ġw rit +ĠPres ident +Ġv ot +Ġke y +Ġm om +p ut +Ġany thing +Ġexper ience +att le +Ġm ind +a ff +om m +Ġf uture +g ed +Ġc ut +Ġto t +it ch +Ġv ideo +Ġinvest ig +Ġn et +ĠM y +r ict +i en +. ) +Ġimp ro +th ough +ward s +Ġcon nect +ĠM ed +sel ves +ens ive +m b +o ber +at ors +A n +Ġ5 0 +Ġre du +res ent +Ġab ove +Ġf re +ĠEuro pe +s w +Ġam ount +ĠA pp +Ġe ither +Ġmil it +Ġan al +Ġf ail +ĠE n +al es +Ġspec ial +Ġbl ack +I T +c her +Ġlook ing +Ġf ire +y n +Ġal most +o on +Ġstud y +Ġm iss +c hes +ro wn +Ġt re +Ġcommun ity +Ġmed ia +Ġf ood +Ġcom es +ĠUn iversity +Ġsing le +Wh at +u ly +Ġh alf +ag ue +h od +ĠRep ublic +Ġstart ed +Ġqu ick +ot o +b ook +Ġiss ue +it or +Ġel se +Ġcons ider +2 6 +ro du +Ġt aken +2 8 +9 9 +ĠW ith +Ġtr ue +Ġw a +Ġtr ad +Ġag o +Ġm ess +ie f +Ġadd ed +o ke +Ġb ad +Ġf av +3 3 +Ġsim ilar +as k +ĠD on +Ġcharact er +ort s +ĠH ouse +Ġreport ed +Ġty pe +v al +i od +ĠHow ever +Ġt arg +Ġent ire +pp ing +Ġhist ory +Ġl ive +ff ic +.... .... +ed eral +Ġtr ying +Ġdisc uss +ĠH ar +ac es +l ished +Ġse lf +os p +re st +Ġro om +el t +Ġf all +ol ution +Ġe t +Ġ x +Ġis n +Ġide a +b o +Ġs ound +ĠD ep +Ġsome one +ci ally +ull y +Ġf oc +Ġob ject +if t +ap er +Ġplay er +Ġr ather +Ġserv ice +as hing +ĠD o +ĠP art +ru g +m on +p ly +Ġm or +Ġnot hing +Ġprov ide +I C +un g +Ġpart y +Ġex ist +Ġm ag +7 0 +Ġr ul +Ġh ouse +Ġbeh ind +Ġhow ever +ĠW orld +Ġs um +Ġapp lic +Ġ ; +Ġfun ction +g r +ĠP ol +Ġfr ont +2 00 +Ġser ies +Ġt em +Ġty p +ill s +Ġo pt +Ġpoint s +Ġbel ow +itt ed +Ġspec ific +Ġ201 7 +um b +Ġr a +Ġpre vious +Ġpre t +re me +Ġc ustom +Ġcour t +ĠM e +Ġre pl +Ġwho le +g o +c er +Ġt reat +ĠA ct +Ġprob ably +Ġle arn +end er +ĠA ss +Ġvers ion +n ow +Ġche ck +ĠC al +R E +min ist +O n +our ces +Ġben ef +Ġd oc +Ġdet er +Ġen c +Ġsu per +Ġadd ress +Ġv ict +Ġ201 3 +Ġme as +t r +Ġf ield +W hen +Ġsign ific +u ge +Ġfe at +Ġcomm on +l oad +Ġbe gin +Ġbr ing +Ġa ction +er man +Ġdesc rib +Ġind ust +Ġwant ed +ri ed +m ing +Ġatt empt +4 5 +f er +Ġd ue +ress ion +# # +Ġsh all +Ġs ix +o o +Ġst ep +Ġp ub +Ġhim self +Ġ2 3 +Ġc op +Ġd est +Ġst op +A C +ib ility +Ġl ab +ic ult +Ġhour s +Ġcre ate +Ġf urther +ĠAmeric a +ĠC ity +Ġd ou +he ad +S T +ĠN orth +c ing +Ġn ational +u le +ĠIn st +Ġt aking +ĠQ u +ir t +Ġre d +Ġrese arch +v iron +ĠG e +Ġbre ak +an a +Ġsp ace +ater ial +Ġrec ent +ĠA b +Ġgener al +Ġh it +Ġper iod +Ġevery thing +ive ly +Ġph ys +Ġsay ing +an ks +Ġc ou +Ġc ult +ac ed +e al +u ation +Ġc oun +l u +Ġinclud e +Ġpos ition +ĠA fter +ĠCan ad +ĠE m +Ġim m +ĠR ed +Ġp ick +Ġcom pl +Ġm atter +re g +e xt +ang u +is c +o le +a ut +Ġcomp et +e ed +f ect +Ġ2 1 +ĠS en +ĠThe se +as ing +Ġcan not +Ġin it +Ġrel ations +ac hed +Ġb ar +Ġ4 0 +ĠT H +Ġ201 2 +Ġv ol +Ġg round +Ġsec urity +Ġup d +il t +3 5 +Ġconc ern +ĠJ ust +Ġwh ite +Ġseem s +ĠH er +pe cially +i ents +Ġann oun +Ġf ig +ight s +Ġst ri +l ike +id s +Ġs us +Ġw atch +Ġ â +Ġw ind +ĠC ont +Ġit self +Ġm ass +A l +y le +iqu e +ĠN ational +Ġab s +Ġp ack +Ġout side +Ġan im +Ġp ain +et er +Ġman ag +du ct +og n +Ġ ] +ĠSe pt +se c +o ff +ĠJ an +Ġf oot +ad es +Ġth ird +Ġm ot +Ġev idence +int on +Ġth reat +a pt +pl es +c le +Ġl o +Ġde cl +Ġit em +med i +Ġrep resent +om b +am er +Ġsignific ant +og raph +s u +Ġc al +i res +00 00 +I D +A M +Ġsim ply +Ġlong er +Ġf ile +O T +c he +S o +ate g +or g +ĠH is +Ġen er +Ġd om +Ġup on +il i +": " +Ġthem selves +Ġcom ing +Ġqu ite +Ġdiff icult +ĠB ar +il ities +re l +end s +c ial +6 4 +Ġwom an +ra p +y r +Ġne cess +ip s +Ġte xt +Ġrequ ire +Ġmilit ary +Ġre view +Ġresp ons +7 5 +Ġsub ject +Ġinst ead +Ġiss ues +Ġg en +" ," +Ġmin utes +Ġwe ap +r ay +am ed +t ime +b l +H ow +Ġc ode +ĠS m +Ġhig her +ĠSt e +r is +Ġp age +Ġstud ents +ĠIn tern +Ġmet hod +ĠA ug +ĠP er +ĠA g +Ġpolic y +ĠS w +Ġex ec +Ġac cept +um e +rib ut +Ġword s +Ġfin al +Ġchang es +ĠDem ocr +Ġfriend s +Ġres pect +Ġe p +Ġcomp an +iv il +Ġdam age +** ** +og le +viron ment +Ġne g +ent al +Ġa p +Ġtot al +iv al +! " +l im +Ġneed s +Ġag re +Ġdevelop ment +Ġa ge +ip le +2 1 +Ġresult s +ĠA f +S h +Ġg un +ĠOb ama +ro ll +Ġ @ +Ġright s +ĠB rit +Ġrun ning +Ġwas n +Ġp ort +Ġr ate +Ġpret ty +Ġtarg et +Ġsa w +Ġc irc +Ġwor ks +ic ro +al t +o ver +ww w +Th at +l ier +Ġevery one +ud e +Ġp ie +idd le +ra el +Ġr ad +Ġbl ock +Ġw alk +T o +ã ģ +n es +ĠA ust +a ul +ro te +ĠS outh +ess ion +op h +Ġshow s +Ġs ite +Ġj o +Ġr isk +cl us +l t +Ġin j +id ing +ĠS pe +Ġch all +ir m +Ġ2 2 +itt ing +st r +Ġh y +L E +ke y +Ġbe gan +at ur +ashing ton +l am +ĠD av +b it +Ġs ize +ĠP ar +3 8 +ourn al +f ace +Ġdec ision +Ġl arg +Ġj ud +re ct +Ġcontin ue +ĠO ct +ove red +ĠI nt +==== ==== +Ġp arent +ĠW ill +Ġeas y +Ġd rug +ang er +Ġs ense +Ġd i +id ay +Ġener gy +ist ic +Ġass oci +ar ter +ob al +e ks +ĠE l +ur ch +Ġg irl +o e +it le +Ġ2 8 +ĠC he +Ġrequ est +Ġso on +Ġh ost +k y +Ġst ates +om es +Ġm aterial +le x +Ġmom ent +Ġan sw +on se +Ġes pecially +Ġn orm +Ġserv ices +p ite +r an +Ġro le +4 4 +) : +Ġc red +C l +____ ____ +Ġm at +Ġl og +ĠCl inton +O U +Ġoff ice +Ġ2 6 +Ġch arg +Ġtr ack +m a +Ġhe art +Ġb all +Ġperson al +Ġbuild ing +n a +s et +b ody +ĠBl ack +Ġincre ase +itt en +Ġneed ed +3 6 +3 2 += " +Ġl ost +Ġbec ame +Ġgrou ps +ĠM us +Ġw rote +ĠP e +Ġpro p +j oy +à © +ĠWh ite +Ġde ad +. ' +Ġhtt p +Ġwe bs +O S +Ġins ide +Ġwr ong +Ġstat ement +Ġ ... +y l +Ġfil m +Ġmus ic +Ġsh are +ific ation +Ġre lease +Ġfor ward +Ġst ay +Ġcomp ut +it te +s er +Ġorig inal +Ġc ard +Ġc and +Ġd iv +at ural +Ġfav or +O M +Ġc ases +us es +Ġse ction +Ġle ave +g ing +ov ed +ĠW ashington +3 9 +ĠG l +Ġrequ ired +act ion +ap an +o or +it er +ĠK ing +Ġcount ries +ĠG erman +ll ing +Ġ2 7 +3 4 +Ġquest ions +Ġpr im +Ġc ell +Ġsh oot +Ġany one +ĠW est +Ġaff ect +ep end +Ġon line +ĠIs rael +ĠSept ember +Ġab ility +Ġcont ent +is es +Ġre ve +Ġl aun +Ġind ic +Ġfor ce +c ast +Ġso ld +av ing +f l +Ġso ft +Ġcompan ies +ce ed +Ġart icle +Ġa ud +Ġre v +Ġed uc +Ġplay ing +0 5 +Ġhe ld +ct or +Ġrele ased +Ġf ederal +3 7 +Ġad minist +Ġinter view +Ġinst all +Ġrece ived +Ġs ource +u k +P h +Ġser ious +Ġcre ated +Ġc ause +Ġim medi +Ġdef in +u el +ĠDep artment +ct ions +ĠC our +ĠN ow +z e +it es +it ution +Ġl ate +Ġspe ak +n ers +Ġleg al +ar i +ĠC or +Ġwe eks +Ġmod el +Ġp red +Ġex act +B C +ĠB y +IN G +os ing +Ġt akes +Ġreg ard +Ġopp ortun +Ġpr ice +Ġ19 8 +ĠA pr +f ully +Ġor d +Ġproble ms +ru ction +h am +ĠC ount +le ge +Ġlead ers +E T +le v +Ġde ep +olog ical +es e +h aps +ĠS ome +Ġp ers +Ġcont ract +Ġrelations hip +s p +ou d +Ġb ase +4 8 +m it +A d +anc ial +Ġcons um +Ġpot ential +Ġl angu +re m +et h +Ġrel ig +ress ed +6 6 +Ġl ink +Ġl ower +ay er +ĠJ une +Ġf em +un t +er c +ur d +Ġcont act +Ġ ill +Ġm other +Ġest ab +h tt +ĠM arch +ĠB ro +ĠCh ina +Ġ2 9 +Ġs qu +Ġprov ided +Ġa verage +as ons +Ġ201 1 +Ġex am +l in +5 5 +n ed +Ġper fect +Ġt ou +al se +u x +Ġbu y +Ġsh ot +Ġcol lect +Ġph ot +Ġplay ed +Ġsur pr +Ġofficial s +Ġsim ple +av y +Ġindust ry +Ġhand s +g round +Ġp ull +Ġr ound +Ġus er +Ġr ange +u ary +Ġpriv ate +op s +e es +Ġw ays +ĠM ich +Ġve h +Ġex cept +Ġter ms +im um +pp er +I ON +ore s +ĠDr agon +ou l +Ġd en +Ġperform ance +Ġb ill +c il +4 7 +Ġen vironment +Ġex c +ad d +Ġwor th +Ġp ict +Ġch ance +Ġ201 8 +b or +Ġspe ed +ict ion +Ġal leg +ĠJ apan +at ory +re et +Ġm atch +ĠI I +Ġst ru +ord er +Ġst e +Ġl iving +Ġst ruct +in o +Ġse par +her n +Ġresp onse +Ġen joy +Ġv ia +A D +um ents +ace book +Ġmem ber +ib r +iz ing +Ġto ol +ĠM on +ĠWh ile +h ood +ĠA ng +ĠD ef +Ġoff er +T r +a ur +Ġturn ed +ĠJ uly +d own +an ced +Ġrec ently +ĠE ar +Ġc e +ĠSt ar +ĠC ong +rough t +Ġbl ood +Ġhop e +Ġcom ment +ain t +Ġar ri +il es +Ġpartic ip +ough t +ri ption +0 8 +4 9 +Ġg ave +Ġse lect +Ġkill ed +sy ch +Ġgo es +i j +Ġc oll +Ġimp act +at ives +ĠS er +0 9 +ĠAug ust +Ġb oy +d e +ĠD es +Ġf elt +U S +Ġexpect ed +Ġim age +ĠM ark +cc ording +o ice +E C +ĠM ag +en ed +h old +ĠP ost +Ġpre vent +N o +Ġinvol ved +Ġey es +Ġquick ly +A t +un k +Ġbeh av +Ġ ur +Ġl ed +c ome +e y +Ġcand id +Ġear lier +Ġfoc us +et y +P ro +led ge +ix ed +ill ed +Ġpop ular +A P +Ġset t +l ight +Ġvar ious +in ks +Ġlevel s +Ġro ad +ell ig +ab les +he l +itte e +ĠG ener +y pe +Ġhe ard +ic les +Ġm is +Ġus ers +ĠS an +Ġimpro ve +Ġf ather +Ġse arch +The y +v il +Ġprof ess +Ġkn ew +Ġl oss +Ġev ents +6 5 +Ġb illion +0 7 +0 2 +ĠNew s +ĠA M +Ġco ver +w here +ens ion +Ġb ott +Ġare as +en ces +op e +ĠTw itter +a el +Ġget s +ĠGo ogle +Ġs n +i ant +Ġv ote +Ġnear ly +Ġinclud ed +Ġrec ogn +z z +m m +al ed +Ġhappen ed +0 4 +Ġh ot +Ġwho se +Ġc ivil +Ġsu ff +o es +it iz +ĠSy ri +Ġresp ond +Ġh on +Ġfeat ures +Ġeconom ic +ĠApr il +r im +Ġtechn ology +Ġo ption +ag ing +Ġpur ch +R e +Ġl at +ch ie +is l +Ġrec omm +u f +Ġtr aining +Ġeffect s +Ġf ast +Ġ201 0 +Ġocc ur +Ġwebs ite +Ġem ail +Ġs ens +e ch +Ġo il +Ġinf lu +Ġcurrent ly +ĠS ch +ĠAd d +Ġgo al +Ġsc ient +Ġcon v +1 00 +em y +Ġdec ided +Ġtra vel +Ġm ention +L L +0 3 +Ġe lection +Ġph one +Ġlook s +Ġsit uation +Ġc y +Ġh or +b ed +ĠCour t +a ily +av es +Ġqu ality +ĠCom p +w ise +Ġt able +Ġst aff +ĠW ind +et t +Ġtri ed +ide red +Ġadd ition +Ġb ox +Ġl ack +ar ily +Ġw ide +Ġm id +Ġbo ard +ys is +Ġant i +h a +Ġd ig +en ing +Ġd ro +C on +6 8 +Ġsl ow +b ased +se qu +Ġp ath +E x +ak er +Ġwork ed +Ġp en +Ġeng ine +Ġlook ed +ĠSu per +ĠS erv +Ġvict im +U n +Ġproper ty +Ġint rodu +Ġexec ut +ĠP M +L e +Ġcol or +ĠM ore +Ġ6 0 +Ġnet work +Ġd ate +c ul +id ge +Ġext ra +3 1 +Ġs le +6 7 +Ġw ond +Ġreport s +j ust +ĠAust ral +Ġcap ital +Ġen s +Ġcomm and +Ġallow ed +Ġpre p +Ġca pt +h ib +Ġnum bers +ch an +Ġf air +m p +om s +Ġre ach +W ith +t ain +Ġbro ad +Ġcou ple +ec ause +ly ing +ĠF eb +Ġsc reen +Ġl ives +Ġpri or +ĠCong ress +A r +Ġappro ach +Ġe mer +ar ies +ĠD is +s erv +ĠN e +Ġbu ilt +c ies +Ġre pe +Ġrul es +for ce +ĠP al +Ġfin ancial +Ġcons idered +ĠCh ar +n ces +ĠI S +Ġb rought +Ġb i +i ers +ĠS im +O P +Ġproduct s +Ġvis it +Ġdoc ument +Ġcon duct +Ġcomplete ly +in ing +ĠCal if +ib ly +Ġwr itten +ĠT V +em ents +Ġd raw +O ne +Ġpub lished +Ġsec ret +r ain +he t +ĠF acebook +ond ay +ĠU p +Ġsex ual +Ġth ous +ĠP at +Ġ ess +Ġstand ard +Ġar m +g es +ect ion +Ġf ell +Ġfore ign +an i +ĠFr iday +Ġreg ular +in ary +Ġincre ased +Ġus ually +Ġdem on +Ġd ark +Ġadd itional +ro l +ĠO f +Ġprodu ction +! ! +und red +Ġintern ational +id ents +ĠF ree +rou p +Ġr ace +Ġm ach +Ġh uge +A ll +le ar +ove mber +Ġto wn +Ġatt ention +ĠO ff +y ond +ĠThe n +f ield +Ġter ror +ra z +ĠB o +Ġmeet ing +ĠP ark +Ġar rest +Ġf ear +Ġa w +ĠV al +or ing +' , +Ġext reme +ar r +Ġwork ers +A fter +Ġ3 1 +n et +am ent +Ġdirect ly +Ġpop ulation +ub e +ĠOct ober +ĠI N +ĠJan uary +5 9 +ĠDav id +Ġc ross +ce mber +ĠF irst +Ġmess age +ir it +Ġn ation +Ġp oll +is ions +Ġansw er +n y +is ode +Ġcar ry +ĠRuss ia +Ġhe ar +eng th +ro y +Ġn atural +in ally +Ġdo g +m itted +Ġtr ade +Ġsub st +Ġmult iple +ĠAf ric +Ġf ans +Ġs ort +Ġgl obal +ic ation +ĠW ed +ar a +Ġa chie +Ġlangu age +ve y +Ġt al +Ġnecess ary +Ġdet ails +Ġs en +ĠS und +ĠRe g +ĠR ec +0 6 +Ġs il +ress ive +Ġmed ical +un ch +orn ia +Ġu nd +f ort +oc ks +ĠM onday +ues day +c raft +7 7 +ur t +Ġ ver +ĠH ill +Ġrece ive +Ġmor ning +es tern +Ġb ank +Ġs at +ir th +ĠH igh +Ġdev ice +ĠTH E +ĠCent er +Ġsaf e +Ġp le +ĠCanad a +Ġsystem s +Ġass ist +Ġsur v +Ġb attle +ĠS oc +vert is +S he +Ġp aper +Ġgrow th +Ġc ast +S c +Ġpl ans +ll ed +Ġpart s +Ġw all +Ġmove ment +Ġpract ice +im ately +Ġdis play +Ġsomet imes +om p +ĠP aul +ĠY es +k ing +5 8 +o ly +Ġs on +Ġav oid +ok es +ĠJ ew +Ġto wards +as c +Ġ // +ĠK ore +Ġtalk ing +Ġcor rect +Ġsp ent +ic ks +i able +e ared +Ġter m +Ġwant s +om ing +Ġ ut +Ġdou b +Ġfor ces +Ġp lease +6 9 +ĠN ovember +at form +ond on +Ġon es +Ġimmedi ately +ĠRuss ian +ĠM et +Ġde g +Ġparent s +C H +ĠAmeric ans +al y +ĠM od +Ġsh own +Ġcond itions +Ġst uff +Ġre b +ĠY our +Ġinclud es +n own +ĠS am +Ġexper ien +m ission +ĠE ven +augh t +Ġannoun ced +ĠRepublic an +Ġdeter min +Ġdescrib ed +ĠCount y +( ) +Ġdo or +Ġchang ed +Ġne igh +ĠH ere +Ġcle an +Ġp an +ĠDe cember +ĠEurope an +ir ing +ap ter +Ġcl ub +ĠT uesday +Ġp aid +ĠN et +Ġattack s +Ġcharact ers +Ġal one +Ġdirect or +d om +Ġ3 5 +Ġl oad +Ġr out +ĠCalif ornia +Ġfin ally +Ġr ac +Ġcont r +Ġexact ly +res h +p ri +ĠIs lam +Ġn ature +Ġcare er +Ġlat est +Ġcon vers +ĠS l +p ose +ci ent +ĠIn c +iv ity +8 8 +ĠA tt +ĠM or +nes day +Ġwe ight +k en +Ġnot e +Ġteam s +Ġ \ +air s +ĠG reen +Ġh undred +on ent +Ġstre ng +Ġcons ist +ic ated +Ġreg ul +Ġl ic +ast ic +Ġt en +urs day +ellig ence +ous ly +ĠU K +B I +Ġcost s +Ġind epend +ĠA P +Ġnorm al +Ġh om +Ġob vious +Ġs we +Ġst ar +Ġread y +ac her +Ġimp lement +g est +Ġs ong +ĠG et +ĠL ab +Ġinterest ing +us ing +Ġg iving +ĠSund ay +Ġet c +Ġm iddle +Ġrem ember +r ight +os ition +ut ions +Ġm ax +4 6 +Ġyour self +Ġdem and +Ġtreat ment +Ġd anger +ĠC ons +Ġgu y +ĠBrit ish +Ġphys ical +Ġrel ated +Ġrem ain +Ġcould n +Ġref er +Ġc itiz +b ox +EN T +bo ard +Ġin n +I G +er o +ĠSt reet +osp ital +ren ch +cher s +Ġst ra +O L +ag er +ĠA N +Ġeas ily +I A +en ge +in y +Ġcl os +ock ed +Ġus es +ĠC oun +I m +u ild +? ? +m ore +Ġan g +Ġwr ite +ol ute +5 7 +Ġlead er +Ġread ing +< / +Ġaut om +est s +4 3 +Ġleg isl +ĠG old +Ġdesign ed +ĠS T +ĠLe g +a res +Ġbe aut +ĠT ex +Ġappear s +Ġstru gg +ĠR om +Ġ 00 +Ġcho ice +Ġparticular ly +ĠF rom +op er +ĠL ondon +ann ed +Ġallow s +ob ile +Ġdiffere nce +âĢ ¢ +ĠV iew +ĠWed nesday +Ġal though +Ġrel ative +Ġapplic ation +ate ver +Ġare n +Ġmy self +Ġim ag +Ġdis e +Ġsoc iety +Ġfre qu +ĠEng lish +Ġpo or +ĠD ay +Ġwrit ing +Ġse ven +Ġstart ing +Ġb ud +Ġpr int +ĠTr ans +uf act +ĠSt ud +n ew +Ġcr im +Ġg ives +Ġco ol +a e +i ance +ĠGener al +Ġthink ing +Ġsa ve +Ġlim ited +ĠPart y +Ġmean ing +p en +ow ers +ĠJ ack +E M +Ġn ice +ru pt +Ġg as +Ġe ight +Ġfe et +Ġeff ort +Ġ ign +ic it +B l +co in +Ġop in +Ġbr ain +Wh ile +he st +ĠTh ursday +Ġwould n +augh ter +Ġtou ch +le ments +Ġstud ies +Ġcent er +c ont +or ge +Ġcomput er +Ġinvestig ation +P l +or ks +Ġ200 8 +Ġincre asing +Ġst ore +Ġcom ments +Ġb al +m en +Ġdo ll +Ġl iber +Ġw ife +Ġlaw s +atur day +it ness +Ġmod ern +ĠS k +Ġadminist ration +Ġopportun ity +Ġs al +Ġpower ful +M y +Ġclaim s +ĠEar th +ord s +Ġt itle +Ġes c +n ame +N ot +om en +Ġbe yond +Ġc amer +Ġse ll +it ute +ear ch +Ġapp l +im ent +4 2 +ĠAr t +Ġun f +Ġviol ence +ur g +ĠE ast +Ġcomp ared +Ġopt ions +Ġthrough out +Ġv s +ig r +. [ +ac hes +7 8 +Ġfil es +F L +E L +ar ian +ĠJ ames +ĠA ir +an ch +Ġdet ail +Ġpie ce +P S +Ġn amed +Ġeduc ation +Ġdri ve +Ġitem s +Ġstud ent +ic ed +: : +ic o +Ġth row +Ġsc ene +Ġcomple x +Ġ200 9 +Ġpre c +ĠB re +7 9 +Ġcon cept +Ġstat us +am ing +Ġd ied +Ġknow ledge +Ġbegin ning +O D +ru ary +Ġcertain ly +Ġgu ys +Ġsl ight +in n +ound s +Ġf ine +Ġf at +ic ations +Ġper haps +ĠA nt +Ġinc ome +Ġhtt ps +Ġmajor ity +port s +st on +Ġgreat er +Ġfe ed +ent ially +Ġsaf ety +Ġun ique +and om +Ġg one +Ġshow ed +Ġhist or +Ġcoun ter +i us +id a +Ġlead ing +i pe +Ġs end +ĠDon ald +er ve +Ġdef ense +ines e +Ġy es +ĠF ire +ĠMus lim +ra q +Ġcontin ued +os h +Ġprov ides +Ġpr ison +ĠP re +Ġhapp y +Ġeconom y +Ġtr ust +ag s +ĠG ame +Ġweap ons +um an +ĠC le +it ation +Ġanal ysis +ĠT imes +Ġsc ience +- > +Ġfig ure +Ġdis app +ent y +Ġsoft ware +Ġu lt +Ġoffic ers +N ew +I s +Ġrem ains +ĠInd ia +Ġp sych +ri ef +Ġc at +es c +Ġob serv +Ġst age +ĠD ark +Ġent er +ch ange +Ġpass ed +Ġdes pite +ĠO ut +Ġmov ie +r s +Ġv oice +m ine +ĠPl ay +Ġto ward +ĠT er +Ġreg ion +Ġval ues +or ters +Ġm ount +Ġoffic er +ĠO ther +b an +Ġh ous +w ood +ro om +I V +ĠS un +se e +ĠO ver +ro g +9 0 +Ġl ay +ĠT ur +a wn +Ġpress ure +ĠS ub +Ġbook s +ed om +ĠS and +A A +ag o +Ġre asons +f ord +Ġactiv ity +U T +N ow +ĠSen ate +ce ll +n ight +Ġcall s +in ter +Ġlet ter +ĠR ob +ĠJ e +Ġcho ose +ĠL aw +G et +B e +Ġro b +Ġtyp es +Ġpl atform +Ġqu arter +R A +ĠT ime +Ġmay be +ĠC r +9 5 +p re +Ġmov ing +Ġl if +Ġgo ld +Ġs om +Ġpat ients +Ġtr uth +ĠK e +ur ance +ant ly +m ar +Ġchar ge +ĠG reat +Ġce le +---------------- ---------------- +Ġro ck +ro id +an cy +Ġcred it +a ud +B y +ĠE very +Ġmov ed +ing er +rib ution +Ġn ames +Ġstra ight +ĠHe alth +ĠW ell +Ġfe ature +Ġr ule +Ġsc he +in ated +ĠMich ael +ber g +4 1 +il ed +b and +Ġcl ick +ĠAng el +on ents +Â Ń +ĠI raq +ĠS aturday +Ġa ware +p art +Ġpat tern +O W +ĠL et +Ġgr ad +ign ed +Ġassoci ated +Ġst yle +n o +i ation +a ith +il ies +Ġst ories +ur ation +Ġindividual s +ĠâĢ ¦ +m iss +ĠAss oci +ish ing +ab y +Ġsum mer +ĠB en +Ġ3 2 +Ġar ch +ut y +ĠTex as +h ol +Ġfull y +Ġm ill +Ġfollow ed +ĠB ill +ĠInd ian +ĠSec ret +ĠB el +ĠFeb ruary +Ġjob s +Ġseem ed +ĠGo vern +i pped +Ġreal ity +Ġl ines +Ġp ark +Ġmeas ure +ĠO ur +I M +Ġbro ther +Ġgrow ing +Ġb an +Ġest im +Ġc ry +ĠS chool +Ġme chan +ĠO F +ĠWind ows +Ġr ates +ĠO h +Ġpos itive +Ġcult ure +ist ics +ic a +Ġh ar +y a +ite ly +i pp +Ġm ap +en cies +ĠWill iam +I I +ak ers +5 6 +ĠM art +ĠR em +Ġal tern +it ude +Ġco ach +row d +D on +Ġk ids +Ġj ournal +Ġcor por +Ġf alse +Ġwe b +Ġsle ep +Ġcont ain +Ġst o +Ġb ed +iver se +ĠR ich +ĠCh inese +Ġp un +Ġme ant +k nown +Ġnot ice +Ġfavor ite +a ven +Ġcond ition +Ġpur pose +) ) +Ġorgan ization +Ġchall eng +Ġman ufact +Ġsus p +ĠA c +Ġcrit ic +un es +uc lear +Ġm er +vent ion +Ġ8 0 +Ġm ist +ĠU s +ĠT or +htt p +ol f +Ġlarg er +Ġadv ant +Ġrese ar +Ġact ions +m l +Ġke pt +Ġa im +, ' +c ol +Ġbenef its +if ying +Ġact ual +ĠIntern ational +Ġveh icle +Ġch ief +Ġeff orts +ĠLe ague +ĠM ost +Ġwa it +Ġad ult +Ġover all +Ġspe ech +Ġhigh ly +Ġfem ale +Ġer ror +Ġeffect ive +5 4 +Ġenc our +w ell +Ġfail ed +Ġcons erv +Ġprogram s +Ġt rou +Ġa head +5 00 +vertis ement +I P +ĠF ound +p ir +Ġ % +Ġcr ime +and er +Ġloc ation +ĠI ran +Ġbehav ior +az ing +Ġr are +Ġem b +Ġca used +Ġsh ip +Ġact ive +Ġcont ribut +Ġg reen +Ġac qu +Ġref lect +ven ue +Ġf irm +Ġb irth +] . +Ġclear ly +Ġem ot +Ġag ency +ri age +Ġmem ory +9 8 +S A +ĠSe e +ac ing +C C +Ġbig gest +Ġr ap +Ġbas ic +Ġb and +e at +Ġsus pect +ĠM ac +Ġ9 0 +m ark +ist an +Ġsp read +am s +k i +as y +ra v +ĠR ober +Ġdemon str +r ated +Ġabs olute +Ġpl aces +Ġim pl +ibr ary +Ġc ards +Ġdest roy +Ġv irt +ve re +Ġapp eared +y an +p oint +Ġbe g +Ġtem per +s pe +ant ed +ear s +ĠD irect +Ġl ength +Ġbl og +am b +Ġint eg +Ġres ources +ac c +if ul +Ġsp ot +Ġfor ced +Ġthous ands +ĠMin ister +Ġqu al +ĠF rench +at ically +Ġgener ally +Ġdr ink +Ġth us +I L +od es +Ġappro pri +ĠRe ad +Ġwh om +Ġey e +Ġcol lege +Ġ4 5 +ire ction +Ġens ure +Ġapp arent +id ers +Ġrelig ious +Ġmin or +ol ic +Ġt ro +ĠWh y +rib ute +m et +Ġprim ary +Ġdevelop ed +Ġpe ace +Ġsk in +st e +av a +Ġbl ue +Ġfam ilies +Ġ ir +Ġapp ly +Ġin form +ĠSm ith +C T +i i +Ġlim it +Ġres ist +........ ........ +um n +Ġconf lic +Ġtw e +ud d +ĠT om +Ġl iter +qu e +b on +Ġha ir +Ġevent ually +Ġp us +Ġhelp ed +Ġag g +or ney +ĠApp le +Ġf it +ĠS ur +Ġpre m +Ġs ales +Ġsecond s +Ġstreng th +Ġfeel ing +¿ ½ +Ġt our +Ġknow s +o om +Ġex erc +Ġsom ew +ï ¿½ +> > +Ġsp okes +Ġide as +Ġreg ist +so ft +ĠD el +ĠP C +Ġpro pos +Ġlaun ch +Ġbott om +T H +ĠP lease +v est +it z +ĠIn ter +Ġsc ript +Ġr at +ar ning +Ġ il +ĠJ er +ĠA re +Ġwh atever +ok en +ci ence +Ġmod e +Ġag ree +Ġs ources +Ġinit ial +Ġrest rict +Ġwond er +us ion +## ## +ĠS il +vil le +Ġb urn +t w +as ion +Ġ £ +Ġn or +u ing +Ġre ached +Ġs un +Ġc ateg +ig ration +Ġc ook +Ġprom ot +Ġm ale +Ġcl imate +Ġf ix +Ġalleg ed +U R +all ed +Ġim ages +C ont +ot a +Ġschool s +i os +Ġd rop +Ġst ream +ĠM o +Ġprevious ly +al ing +Ġp et +Ġdou ble +Ġ( @ +ann el +Ġdef ault +t ies +Ġr ank +ĠD ec +ĠCoun cil +Ġweap on +Ġst ock +Ġanal y +ĠSt r +Ġpict ure +ĠPol ice +f erence +Ġcent ury +Ġcitiz ens +Ġon to +Ġexp and +Ġhe ro +ĠS ol +Ġw ild +Ġupd ate +Ġcustom ers +r ont +d ef +Ġl ik +Ġcrim inal +ĠChrist ian +S P +7 6 +Ġle aving +Ġother wise +ĠD ist +Ġbas is +5 2 +5 3 +ic ip +ĠB er +Ġrecomm end +Ġfl oor +Ġc rowd +ol es +Ġ7 0 +Ġcent ral +ĠE v +Ġd ream +Ġdown load +Ġconf ir +ĠTh om +Ġwind ow +Ġhapp ens +Ġun it +Ġt end +Ġs pl +Ġbec omes +Ġfight ing +Ġpred ict +ĠP ress +ĠP ower +Ġhe avy +ak ed +Ġf an +or ter +ate gy +B A +iz es +Ġsp end +H ere +Ġ200 7 +Ġad op +ĠH am +Ġfoot ball +ĠP ort +od ay +5 1 +amp ions +Ġtrans fer +h t +Ġ3 8 +ter m +ac ity +Ġb ur +] , +tern al +r ig +b ut +Ġthere fore +ĠB ecause +res p +re y +Ġm ission +S ome +Ġnot ed +Ġass um +Ġdise ase +Ġed it +Ġprog ress +r d +ĠB rown +oc al +Ġadd ing +Ġra ised +ĠAn y +Ġt ick +Ġsee ing +ĠPe ople +Ġagre ement +Ġser ver +Ġw at +Ġdeb ate +Ġsupp osed +il ing +Ġlarg est +Ġsuccess ful +ĠP ri +ĠDemocr atic +Ġj ump +ĠSyri a +Ġown ers +Ġoff ers +Ġshoot ing +Ġeff ic +se y +Ġha ven +ver se +te red +ĠL ight +im al +ĠB ig +Ġdef end +Ġbe at +Ġrecord s +% ) +Ġsc en +Ġemploy ees +Ġdev ices +he m +Ġcom mer +ĠM ex +Ġbenef it +ĠPro f +Ġil leg +Ġsur face +ĠAl so +Ġh arm +ing ly +w ide +ĠA lex +Ġsh ut +ĠC ur +Ġl ose +p m +Ġchall enge +se mb +Ġst ation +Ġint elligence +Ġacc ur +ĠFl or +Ġrequ ires +ĠM al +b um +Ġh ospital +Ġsp irit +Ġoff ered +Ġprodu ce +ĠComm un +Ġcreat ing +Ġcr is +s pect +Ġend ed +Ġd aily +Ġvot ers +land s +i as +i h +on a +Ġsm art +ĠOff ice +ĠL ord +ri al +ĠIntern et +Ġcirc um +Ġextreme ly +' . +Ġopin ion +ĠM il +Ġg ain +B S +ĠF in +y p +Ġuse ful +Ġbud get +Ġcom fort +is f +Ġback ground +el ine +Ġep isode +Ġen emy +Ġtri al +Ġestab lish +d ate +ĠC ap +Ġcontin ues +Ġshow ing +ĠUn ion +w ith +Ġpost ed +ĠSy stem +Ġe at +ri an +Ġr ise +ĠGerman y +il s +Ġsign ed +Ġv ill +Ġgr and +m or +ĠEng land +Ġproject s +um ber +Ġconf erence +z a +Ġrespons ible +ĠAr ab +Ġlearn ed +âĢĶ âĢĶ +i pping +ĠGe orge +O C +Ġreturn ed +ĠAustral ia +Ġb rief +Q u +Ġbr and +ill ing +ab led +Ġhig hest +Ġtr ain +ĠComm ission +wh ile +Ġn om +cept ion +Ġm ut +ĠBl ue +Ġinc ident +v ant +8 6 +ĠI D +Ġn uclear +7 4 +ĠL ike +ĠR E +ĠM icro +l i +m ail +Ġcharg es +8 9 +Ġad just +ad o +Ġear th +N A +Ġpr ices +P A +Ġd raft +Ġrun s +Ġcandid ate +ens es +Ġmanag ement +ĠPh il +ĠM iss +Ġte ach +g ram +Ġunderstand ing +a it +ic ago +A dd +ĠE p +sec ut +Ġsepar ate +Ġinst ance +Ġe th +Ġun less +**** **** +ĠF ore +in ate +Ġoper ations +S p +Ġf aith +g ar +ĠCh urch +ron ic +Ġconf ig +os ure +Ġactiv ities +Ġtrad itional +Ġ3 6 +Ġd irection +Ġmach ine +Ġsur round +Ġp ush +un ction +ĠE U +Ġeas ier +Ġarg ument +G B +Ġm icro +Ġsp ending +iz ations +Ġthe ory +ad ow +Ġcall ing +ĠL ast +Ġd er +Ġinflu ence +Ġcomm it +Ġph oto +Ġun c +ist ry +g n +ast e +ack s +Ġdis p +ad y +d o +ĠG ood +Ġ ` +Ġw ish +Ġreve aled +Âł Âł +l ig +Ġen force +ĠComm ittee +Ġche m +Ġmil es +Ġinterest ed +Ġsol ution +ic y +in ct +Ġ- > +ĠD et +Ġrem oved +Ġcomp ar +e ah +Ġpl ant +ĠS ince +Ġachie ve +Ġadvant age +Ġslight ly +b ing +Ġpl aced +u nder +201 5 +ĠM ad +Ġt im +os es +Ġc ru +ĠR ock +Ġmost ly +Ġneg ative +Ġset ting +Ġprodu ced +Ġm ur +Ġconnect ion +ĠM er +Ġdri ver +Ġexecut ive +Ġass ault +Ġb orn +ĠV er +t ained +Ġstruct ure +Ġredu ce +Ġdec ades +Ġd ed +u ke +ĠM any +idd en +Ġle ague +S e +Ġjo in +Ġdis co +Ġd ie +c ks +act ions +Ġass ess +ag n +Ġgo als +our s +I R +Ġsen ior +ill er +m od +ip ment +oc ol +u y +ĠQ ue +Ġpart ies +ir gin +Ġle arning +it able +Ġstre et +Ġcamer a +A pp +Ġsk ills +b re +c ious +Ġcele br +ĠFr anc +Ġexist ing +Ġwill ing +l or +Ġ id +ĠSp ace +Ġcrit ical +ĠL a +ortun ately +Ġser ve +Ġc old +Ġspec ies +T S +Ġanim als +ĠB ay +Ġold er +ĠU nder +est ic +ĠT re +Ġte acher +Ġpre fer +v is +Ġth read +ĠM att +Ġmanag er +ãĥ » +Ġprofess ional +ĠV ol +Ġnot es +The se +ul a +Ġf resh +ent ed +u zz +ed y +clus ion +ĠR el +Ġdoub t +E O +Ġopen ed +ĠB it +Ad vertisement +Ġgu ess +ĠU N +Ġse qu +Ġexpl ain +ott en +Ġatt ract +ak s +Ġstr ing +Ġcont ext +oss ible +ĠRepublic ans +Ġsol id +Ġc ities +Ġask ing +Ġr andom +u ps +ur ies +ar ant +dd en +g l +ĠFlor ida +Ġdep end +ĠSc ott +Ġ3 3 +Ġi T +ic on +Ġmention ed +Ġ2 000 +Ġclaim ed +Ġdefin itely +ul f +Ġc ore +Ġopen ing +ĠCon st +wh ich +ĠT ra +A G +7 2 +Ġbelie ved +ad a +Ġ4 8 +ĠSec urity +yr ight +ĠP et +ĠL ou +Ġhold ing +======== ======== +Ġ ice +Ġb row +Ġauthor ities +h ost +w ord +Ġsc ore +ĠD iv +Ġcell s +Ġtrans l +Ġneigh bor +Ġrem ove +u ct +Ġdist rict +ĠA ccording +Ġwor se +Ġconcern s +Ġpresident ial +Ġpolic ies +ĠH all +7 3 +Ġh us +A Y +Ġ200 6 +ĠJ ud +Ġindepend ent +ĠJust ice +ili ar +pr int +igh ter +Ġprotect ion +z en +Ġsu dden +h ouse +ĠJ es +P R +ĠIn f +Ġb ul +Ġ _ +ĠServ ice +ĠP R +Ġstr ategy +ff ect +Ġgirl s +Ġmiss ing +oy al +ĠTe am +ul ated +Ġd at +Ġpolit ics +ab or +A ccording +Ġspe ll +Ġg raph +ort hern +T C +A b +Ġlab or +is her +Ġk ick +ĠiT unes +Ġstep s +pos es +Ġsmall er +E n +ber t +Ġro ll +Ġresear chers +Ġcl osed +Ġtrans port +Ġlaw y +________ ________ +ĠCh icago +Ġas pect +Ġn one +Ġmar riage +9 6 +Ġe lements +ĠF re +ĠS al +Ġd ram +F C +t op +e qu +Ġhe aring +Ġsupport ed +Ġtest ing +co hol +Ġmass ive +Ġst ick +Ġgu ard +is co +ph one +F rom +How ever +Ġb order +Ġcop y +ograph y +l ist +7 1 +Ġown er +cl ass +ru it +r ate +ĠO nce +Ġdig ital +Ġt ask +ER S +Ġinc red +t es ++ + +ĠFr ance +Ġb reat +ow l +Ġiss ued +ĠW estern +Ġdet ect +Ġpart ners +Ġsh ared +ĠC all +Ġcan cer +ac he +rib e +Ġexpl ained +Ġhe at +{ " +Ġinvest ment +ĠB ook +Ġw ood +Ġtool s +ĠAl though +Ġbelie f +Ġcris is +Ġg e +ĠM P +Ġoper ation +ty pe +~ ~ +g a +Ġcont ains +ant a +Ġexp ress +ĠG roup +ĠJ ournal +k a +Ġam b +ĠUS A +Ġfind ing +Ġfund ing +h ow +Ġestab lished +ide os +Ġdeg ree +Ġdanger ous +ang ing +Ġfre edom +pp ort +out hern +Ġch urch +Ġc atch +ĠTw o +Ġpres ence +ĠGu ard +U p +Ġauthor ity +ĠPro ject +Ġbut ton +Ġcon sequ +Ġval id +Ġwe ak +Ġstart s +Ġref erence +ĠM em +" ) +U N +or age +ĠO pen +Ġcol lection +y m +g ency +Ġbeaut iful +ro s +Ġtell s +Ġwa iting +n el +Ġprov iding +ĠDemocr ats +Ġd aughter +Ġm aster +Ġpur poses +ĠJapan ese +Ġequ al +Ġturn s +Ġdoc uments +Ġwatch ing +R es +Ġr an +201 4 +Ġre ject +ĠKore a +Ġvictim s +Le vel +ere nces +Ġw itness +Ġ3 4 +Ġre form +com ing +Ġocc up +Ġc aught +Ġtra ffic +ad ing +Ġmod els +ar io +Ġserv ed +Ġb atter +u ate +ĠSecret ary +Ġagre ed +Ġtr uly +yn am +ĠR et +Ġun its +ĠRes earch +h and +az ine +ĠM ike +Ġvar iety +ot al +Ġam azing +Ġconfir med +Ġentire ly +Ġpurch ase +Ġe lement +Ġc ash +Ġdeter mine +D e +Ġc ars +ĠW all +â ĸ +Ġview s +Ġdrug s +Ġdep artment +ĠSt ep +u it +Ġ3 9 +as ure +ĠCl ass +Ġc overed +ĠB ank +Ġme re +u ana +Ġmult i +Ġm ix +Ġun like +lev ision +Ġsto pped +Ġs em +ĠG al +ul es +Ġwe l +ĠJohn son +l a +Ġsk ill +Ġbec oming +ri e +Ġappropri ate +f e +ell ow +ĠPro t +ul ate +oc ation +Ġweek end +od ies +Ġsit es +Ġanim al +ĠT im +Ġsc ale +Ġcharg ed +Ġinst ruct +ill a +Ġmethod s +Ġc ert +Ġjud ge +ĠH el +Ġdoll ars +Ġstand ing +ĠS qu +Ġdeb t +l iam +Ġdri ving +ĠS um +ĠEd ition +Ġal bum +and on +I F +ĠU k +6 3 +ad er +Ġcommer cial +es h +ĠGovern ment +Ġdisc overed +Ġout put +ĠHill ary +ĠCar ol +Ġ200 5 +Ġab use +anc ing +Ġsw itch +Ġann ual +T w +Ġst ated +ag ement +in ner +Ġdem ocr +Ġres idents +Ġallow ing +Ġfact ors +od d +Ġf uck +em ies +Ġoccur red +ot i +Ġn orth +ĠP ublic +Ġinj ury +Ġins urance +C L +oll y +ã Ģ +Ġrepe ated +Ġar ms +ang ed +Ġconst ruction +Ġf le +P U +ic ians +Ġfor ms +ĠMc C +ant ic +Ġm ental +p ire +Ġequ ipment +Ġf ant +Ġdiscuss ion +Ġregard ing +k in +ar p +Ġch air +og ue +Ġpro ceed +ĠI d +O ur +Ġmur der +M an +Ġ4 9 +as p +Ġsupp ly +Ġin put +Ġwe alth +liam ent +Ġpro ced +or ial +ĠSt at +ĠN FL +hen s +ĠInst itute +Ġput ting +ourn ament +et ic +Ġloc ated +Ġk id +er ia +r un +Ġpr inc +Ġ ! +go ing +ĠB et +Ġcl ot +Ġtell ing +Ġprop osed +i ot +or ry +Ġfund s +g ment +ĠL ife +Ġb aby +ĠB ack +Ġsp oke +Im age +Ġear n +ĠA T +g u +Ġex change +ĠL in +ov ing +Ġp air +M ore +az on +Ġarrest ed +Ġkill ing +c an +ĠC ard +y d +Ġident ified +Ġm obile +Ġthan ks +ony m +ĠF orm +Ġhundred s +ĠCh ris +ĠC at +Ġtre nd +h at +ĠA v +om an +Ġelect ric +ĠW il +S E +O f +Ġrest aur +ot ed +Ġtr ig +Ġn ine +Ġb omb +Wh y + ¯ +Ġco verage +Ġapp eal +ĠRober t +ĠS up +Ġfin ished +Ġfl ow +Ġdel iver +Ġcal cul +Ġphot os +Ġph il +Ġpie ces +Ġapp re +k es +Ġr ough +D o +Ġpart ner +Ġconcern ed +Ġ3 7 +ĠG en +C ol +ct ors +Ġ= > +st ate +Ġsuggest ed +ĠFor ce +C E +Ġher self +ĠPl an +w orks +o oth +ren cy +Ġcor ner +Ġhus band +Ġintern et +ĠA ut +em s +os en +ĠAt l +g en +Ġbal ance +6 2 +Ġsound s +te xt +Ġar r +ov es +Ġmill ions +Ġrad io +Ġsat isf +ĠD am +M r +G o +S pe +Ġcomb at +r ant +ĠG ree +Ġf uel +Ġdist ance +Ġtest s +Ġdec re +ĠE r +Ġman aged +D S +Ġt it +Ġmeas ures +ĠL iber +Ġatt end +as hed +ĠJ ose +ĠN ight +d it +ĠN ov +ĠE nd +out s +Ġgener ation +Ġadv oc +y th +Ġconvers ation +ĠS ky +act ive +ce l +ri er +ĠFr ank +Ġg ender +Ġcon cent +Ġcar ried +and a +ĠV irgin +Ġarri ved +ic ide +ad ed +Ġfail ure +Ġmin imum +le ts +Ġwor st +Ġkeep ing +Ġint ended +Ġilleg al +Ġsub sc +Ġdetermin ed +Ġtri p +Y es +Ġra ise +Ġ ~ +Ġfeel s +Ġpack age +ĠJ o +h i +201 6 +re al +Ġf ra +Ġsy mb +M e +uck y +p ret +ĠK h +ĠEd it +ĠWe b +em ic +ĠCol or +Ġjust ice +I nt +Ġfar m +ck now +" > +el ess +Ġredu ced +Ġ5 00 +x x +ĠR ad +ĠW ood +Ġcl in +Ġhy p +il er +ur a +k ins +8 5 +6 1 +ĠThe ir +ĠM ary +Ġs an +Ġno vel +ĠWh o +Ġcap acity +Ġimp ossible +Ġpl ays +Ġmin ister +ij uana +ic ate +ĠS et +Ġf ram +Ġ ing +Ġcommun ities +ĠF BI +it a +Ġb on +Ġstr ateg +Ġinterest s +l ock +g ers +m as +ĠAN D +Ġconflic t +Ġrequire ments +Ġs ac +Ġoper ating +in i +rel ated +Ġcomm itted +Ġrelative ly +Ġs outh +¯ ¯ +Ġaff ord +Ġident ity +Ġdec isions +Ġacc used +pl ace +Ġvict ory +o ch +i at +N ame +C om +t ion +ed s +Ġsee k +Ġt ight +ĠIm ages +Ġinit i +Ġhum ans +Ġfam iliar +Ġaud ience +Ġintern al +vent ure +Ġs ides +ĠT O +Ġd im +Ġcon clud +Ġapp oint +Ġenforce ment +ĠJ im +ĠAssoci ation +Ġcircum st +ĠCanad ian +Ġjo ined +Ġdiffere nces +ĠL os +Ġprot est +Ġtw ice +w in +Ġgl ass +ars h +ĠAr my +Ġexp ression +Ġdec ide +Ġplan ning +an ia +Ġhand le +ĠMicro soft +ĠN or +Ġmax imum +ĠRe v +Ġse a +Ġev al +Ġhel ps +re f +Ġb ound +Ġm outh +Ġstand ards +Ġcl im +ĠC amp +ĠF ox +cl es +Ġar my +ĠTe chn +ack ing +x y +S S +Ġ4 2 +Ġbu g +ĠUk rain +ĠM ax +ĠJ ones +ĠSh ow +l o +Ġplan et +Ġ7 5 +Ġwin ning +Ġf aster +Ġspe ct +Ġbro ken +T R +Ġdef ined +Ġhealth y +Ġcompet ition +htt ps +ĠIs land +ĠF e +Ġannoun ce +ĠC up +ĠInst ead +Ġcl ient +Ġposs ibly +se ction +ock et +l ook +Ġfin ish +Ġcre w +Ġres erv +Ġed itor +Ġh ate +Ġs ale +Ġcontro vers +Ġp ages +w ing +Ġnum er +Ġopp osition +Ġ200 4 +Ġref uge +Ġfl ight +Ġap art +ĠL at +A meric +ĠAfric a +Ġapplic ations +ĠPal est +ĠB ur +Ġg ar +ĠSoc ial +Ġup gr +Ġsh ape +Ġspe aking +ans ion +a o +ĠS n +Ġwor ry +ĠBrit ain +P lease +rou d +Ġh un +Ġintrodu ced +Ġd iet +I nd +ĠSec ond +Ġfun ctions +ut s +ĠE ach +ĠJe ff +Ġst ress +Ġaccount s +Ġgu arant +ĠAn n +ed ia +Ġhon est +Ġt ree +ĠAfric an +ĠB ush +} , +Ġs ch +ĠOn ly +Ġf if +ig an +Ġexerc ise +ĠEx p +Ġscient ists +Ġlegisl ation +ĠW ork +ĠS pr +à Ĥ +ĠH uman +Ġ è +Ġsur vey +Ġr ich +ri p +Ġmain tain +Ġfl o +Ġleaders hip +st ream +ĠIslam ic +Ġ 01 +ĠCol lege +Ġmag ic +ĠPr ime +Ġfig ures +201 7 +ind er +x ual +ĠDe ad +Ġabsolute ly +Ġfour th +Ġpresent ed +resp ond +rib le +Ġal cohol +at o +ĠD E +por ary +Ġgr ab +Ġvar i +Ġqu ant +ĠPh oto +Ġpl us +r ick +ar ks +Ġaltern ative +Ġp il +Ġappro x +th at +Ġobject s +ĠR o +ĠAnd roid +Ġsignificant ly +ĠR oad +k ay +R ead +av or +Ġa cknow +ĠH D +ĠS ing +O r +ĠM ont +Ġun s +pro f +Ġneg oti +ĠAr ch +ik i +Ġte levision +ĠJew ish +Ġcomm ittee +Ġmot or +Ġappear ance +Ġs itting +Ġstri ke +ĠD own +com p +ĠH ist +Ġf old +ac ement +ĠLou is +Ġbel ong +ĠâĢ ¢ +Ġm ort +Ġprep ared +Ġ6 4 +ĠM aster +Ġind eed +ĠD en +Ġre nt +T A +our ney +ar c +S u +9 7 +Ġadv ice +Ġchang ing +Ġlist ed +Ġlaun ched +is ation +ĠP eter +is hes +Ġl ived +ĠM el +ĠSup reme +ĠF ederal +Ġ) ; +ruct ure +Ġset s +Ġphil os +u ous +Ġ ł +Ġappl ied +ĠN OT +Ġhous ing +ĠM ount +Ġo dd +Ġsu st +D A +ffic ient +Ġ ? +ol ved +Ġp owers +Ġth r +Ġrem aining +ĠW ater +L C +Ġca uses +ãģ ® +Ġman ner +ad s +Ġsuggest s +Ġend s +stand ing +f ig +ĠD un +id th +Ġg ay +Ġter min +ĠAngel es +M S +Ġscient ific +Ġco al +ap ers +b ar +ĠThom as +Ġsy m +ĠR un +th is +P C +igr ants +Ġmin ute +ĠDist rict +cell ent +Ġle aves +Ġcomple ted +am in +Ġfoc used +Ġmon itor +Ġveh icles +M A +ĠM ass +ĠGr and +Ġaffect ed +itution al +Ġconst ruct +Ġfollow s +Ġt on +re ens +Ġh omes +ĠE xt +ĠLe vel +r ast +ĠI r +Ġel im +Ġlarge ly +ĠJ oe +Ġvot es +all s +Ġbusiness es +ĠFound ation +ĠCent ral +Ġy ards +Ġmaterial s +ul ner +Ġgu ide +Ġclos er +um s +Ġsp orts +ed er +J ust +Ġtax es +8 4 +ĠO ld +Ġdec ade +ol a +Ġv ir +Ġdro pped +Ġdel ay +it ect +Ġsec ure +ste in +le vel +Ġtre ated +Ġfil ed +ain e +Ġv an +Ġm ir +Ġcol umn +ict ed +e per +Ġro t +Ġcons ult +Ġent ry +Ġmar ijuana +ĠD ou +Ġapparent ly +ok ing +clus ive +Ġincre ases +an o +Ġspecific ally +Ġte le +ens ions +Ġrelig ion +ab ilities +Ġfr ame +ĠN ote +ĠLe e +Ġhelp ing +Ġed ge +ost on +Ġorgan izations +à ĥ +ĠB oth +hip s +Ġbig ger +Ġbo ost +ĠSt and +Ġro w +ul s +ab ase +Ġr id +L et +are n +ra ve +Ġst ret +P D +Ġv ision +Ġwe aring +Ġappre ci +Ġa ward +ĠU se +Ġfact or +w ar +ul ations +) ( +Ġg od +Ġter rit +Ġpar am +ast s +8 7 +Ġen emies +ĠG ames +F F +Ġacc ident +W ell +ĠMart in +T ER +Ġat h +ĠHe ll +Ġfor g +Ġve ter +ĠMed ic +f ree +Ġst ars +Ġexp ensive +Ġac ad +ra wn +ĠW he +Ġl ock +Ġform at +Ġsold iers +s m +Ġag ent +Ġrespons ibility +or a +ĠS cience +Ġrap id +Ġt ough +ĠJes us +Ġbelie ves +M L +Ġwe ar +le te +Ãĥ ÃĤ +ĠD ri +Ġcomm ission +ĠB ob +O h +ap ed +Ġwar m +ÃĥÃĤ ÃĥÃĤ +Ġ200 3 +ort ion +Ġhas n +ust er +Ġun ivers +ĠI ll +Ġk ing +olog ies +9 4 +ĠT em +ĠM os +Ġpat ient +ĠMex ico +ce an +ĠDe ath +ĠSand ers +y ou +ĠC ast +ĠComp any +pt y +Ġhappen ing +F P +ĠB attle +Ġb ought +A m +M od +U s +ut ers +ĠC re +ĠTh ose +Ġ4 4 +is er +Ġs oul +ĠT op +ĠHar ry +ĠA w +Ġse at +ff ee +Ġrev olution +Ġ( " +ĠD uring +et te +Ġr ing +Ġoff ensive +Ġreturn s +Ġv ideos +Ġdis cl +Ġfam ous +en ced +ĠS ign +ĠR iver +Ġ3 00 +P M +ĠB us +ĠC H +Ġcandid ates +ard en +Ġpercent age +Ġvis ual +Ġthan k +Ġtrou ble +ner gy +Ġ200 1 +Ġpro ve +ash ion +Ġen h +ĠL ong +U M +Ġconnect ed +Ġposs ibility +O ver +Ġexper t +Ġl ibrary +art s +ĠDirect or +Ġfell ow +9 2 +ir ty +Ġd ry +Ġsign s +ĠL ove +Ġqu iet +f oot +Ġp ure +ĠH un +Ġf illed +ph as +ĠE lect +end ment +ĠEx pl +Ġun able +n s +m o +Ġv ast +ob e +Ġident ify +app ing +ĠCarol ina +g ress +Ġpro te +Ġf ish +Ġcircumst ances +raz y +ĠPh ot +Ġb odies +ĠM ur +Ġdevelop ing +ĠA R +Ġexperien ced +Ġsubst ant +ĠBo ard +es ome +Ġdom estic +Ġcomb ined +ĠP ut +Ġchem ical +ĠCh ild +Ġpo ol +ĠC y +Ġe gg +c ons +st ers +Ġh urt +Ġmark ets +Ġconserv ative +Ġsupp orters +Ġag encies +id el +O b +ur b +Ġ4 3 +ĠDef ense +y e +ĠA p +du le +Ġtemper ature +Ġconduct ed +ĠCh ief +Ġpull ed +Ġf ol +L ast +ont o +os is +V ER +D es +ĠP an +F irst +Ġadv ance +Ġlic ense +r ors +ĠJ on +Ġimag ine +Ġhe ll +Ġf ixed +Ġinc or +os ite +ĠL og +ick en +] : +Ġsurpr ise +h ab +Ġc raft +ol t +ĠJ ul +Ġd ial +Ġrele vant +Ġent ered +Ġlead s +ĠA D +ĠCle an +Ġpict ures +ess or +Ġal t +Ġpay ing +P er +ĠMark et +Ġupd ates +am ily +ĠT ype +ĠH ome +Ġ5 5 +semb ly +rom e +8 3 +Ġgreat est +Ġhe ight +Ġhe av +ain ts +Ġlist en +as er +ĠS H +Ġcap able +ac le +Ġpers pect +in ating +Ġoff ering +ry pt +ĠDe velop +ab in +r c +Ġbr ight +al ty +ar row +Ġsupp l +ind ing +ack ed +gy pt +ĠAn other +p g +ĠVirgin ia +ĠL u +Ġpl anned +Ġp it +Ġswe et +T ype +ĠD i +Ġtyp ically +ĠFranc isco +Ġpro spect +ĠD an +Ġte en +re es +Ġsc hed +Ġh ol +Ġsc r +Ġlot s +l ife +Ġnews p +Ġfor get +ĠN one +ĠM iddle +ĠR yan +ed d +Ġse vere +Ġsu it +ll er +9 3 +Ġcor respond +Ġexpl os +u ations +Ġfl ag +g ame +r id +Ġpr in +ĠD ata +Ġde ploy +ĠEn ter +su it +gh an +ĠM en +Ġthough ts +Ġmat ters +Ġad apt +ĠA ri +Ġf ill +Ġfor th +Ġs am +Ġ4 1 +Ġpay ment +ĠH or +Ġsp ring +du c +Ġl osing +Ġbring ing +F O +al a +Ġdist ribution +he red +b our +ĠIsrael i +om a +Ġcomb ination +Ġpl enty +V E +C an +ĠH aw +Ġper man +ĠSpe cial +Ġto w +Ġsee king +Ġexam ples +Ġclass es +c r +Ġbe er +Ġmov es +ĠI P +ĠK n +Ġpan el +E ven +Ġproper ly +Ġr is +Ġpl ug +Ġestim ated +E very +Ġdef ensive +ag raph +Ġpre gn +Ġinst it +ĠV ict +Ġvol ume +Ġpos itions +Ġl inks +ĠPro gram +ĠWe ek +ag ues +Ġtrans form +k er +ĠC EO +Ġc as +Ġopp onent +Ġtwe et +ĠC ode +Ġsh op +Ġf ly +Ġtal ks +Ġb ag +Ph one +Ġa id +Ġpl ants +Ġ6 5 +Ġatt orney +ar ters +qu est +ĠMag ic +Ġbeg ins +Ġmy ster +Ġenvironment al +Ġst orage +N N +Ġm arg +Ġs ke +Ġmet al +ell y +Ġord ered +Ġrem ained +Ġl oved +Ġprom pt +Ġupd ated +Ġexper ts +Ġwalk ing +Ġan cient +Ġperform ed +AT E +Ġne ither +i ency +Ġmanufact ure +ĠP ak +Ġselect ed +Ġm ine +Ġult imately +Ġexpl an +Ġlab el +ĠServ ices +ribut ed +Tr ump +Ġsy n +ĠU lt +S C +Ġme at +Ġg iant +ĠW ars +ĠO N +Ġad m +Ġinter pret +Ġeven ing +Ġev il +ĠB oston +ĠW ild +Ġ à +ĠBit coin +ĠAm azon +D r +ĠIn formation +Ġobvious ly +Ġadv anced +Ph oto +ol ar +Ġwe ather +Ġsymb ol +Ġso le +Ġpot entially +ost er +Ġorig inally +m un +3 00 +az e +ess ions +Ġde ck +Ġst ood +Ġyou th +ĠB ern +R ep +ĠT est +Ġbas ically +ot ic +Ġinvol ve +ol it +ly n +S ee +Ġair craft +Ġconf irm +E W +Ġmess ages +ĠRich ard +Ġk it +Ġpro hib +Ġv ulner +is ters +Ġexist ence +Ġturn ing +ĠS P +Ġdes ire +Ġfl at +Ġm ent +se ason +ang es +Ġneighbor hood +ĠL ake +AT ION +Ġpoint ed +b ur +Ġinn ov +uc ks +U L +Ġprofess or +Ġexp ressed +A B +ic ious +Ġ200 2 +ĠDe v +Ġs ession +Ġb are +s en +Ġdis s +ĠC ath +ĠP ass +ĠP oint +Ġdo ctor +or row +ail ed +ĠR ub +ĠD C +ĠChar l +p erson +Ġwrit er +igh ters +ure au +Ġob lig +Ġrecord ed +Ġbro ke +Ġord ers +il ty +Ġmot ion +in ity +l aw +ad ium +Ġimm igration +Ġcontr ast +Ġb att +Ġex cellent +Ġtechn ical +am i +Ġt un +Ġcl oud +ĠY ear +ge on +Ġcre ation +Ġstr ange +Ġa uth +Ġfor t +b orn +Ġext ent +ĠT oday +ĠCl ub +Ġr ain +Ġs ample +Ġaccept ed +Ġt act +Ġf ired +ĠS on +Ġstand s +Ġb oot +Ġ4 7 +Ġstat ements +Ġvers ions +Ġse lling +ound ed +Ġ199 0 +Ġwere n +ĠW atch +Ġexper iment +P ost +Ġret ail +ul ed +In st +un te +ãĥ ¼ +Ġdep art +Ġb ond +i very +om pl +Ġre action +ĠSyri an +ĠP ac +app ed +ani el +D P +Ġres olution +Ġre act +Ġappro ved +on om +m ond +ĠO ffic +-- - +Ġrepl ace +Ġt ack +Ġsp ort +Ġch ain +Ġemer gency +r ad +ĠPalest in +Ġ4 6 +Ġautom atically +Ġrout e +Ġp al +Ġb anks +ĠPar is +ĠMed ia +ro ad +ic ing +i xt +ist ed +Ġg rew +Ġco ord +ĠW here +om in +Ġsub s +� � +Ġ ± +Ġcorpor ate +Ġse lection +n oon +ĠRep ort +c s +clud ing +ord ers +anc he +ĠIt s +Ġslow ly +ĠE gypt +ĠA cc +Ġcol le +iqu es +E X +Ġattempt s +ur l +ĠC ross +Ġfind ings +ĠS C +ĠO R +Ġind ex +ens ity +ĠW ay +ĠL and +Ġsh ock +d is +Ġd ynam +Ġc art +m osp +S ince +i est +ĠB oy +Ġst orm +ĠCont in +201 3 +he w +il it +Ġess ential +iqu id +O ther +ive red +Ġreason able +A ct +Ġsub sequ +ĠP ack +ĠF ort +Ġconsider ing +Ġun iversity +l og +Ġmar ried +Ġill ust +ĠTr ue +£ ı +Ġnumer ous +rast ructure +Ġserious ly +Ġrefer red +u a +Ġconsist ent +on na +ĠRe al +ru ption +ci ples +Ġfact s +9 1 +ot es +er g +The n +Ġacc ompl +N ote +Ġre venue +Ġpass ing +Ġm al +e en +ĠY et +Ġg ather +ter day +ew ork +ĠA uthor +P e +Ġopt im +Ġr ub +Ġè £ı +Ġun known +st one +Ġun ion +ol ve +Ġopportun ities +Ġbrow ser +ĠW al +ĠC ost +Ġreport ing +st s +p et +Ġs and +Ġsudden ly +Ġsurpr ising +ĠV R +Ġsomew hat +ĠB as +ult ure +iz z +ĠC D +Ġchalleng es +Ġsett ings +Ġexperien ces +ĠF ull +Ġcan n +Ġrece iving +ES T +Ġj oint +Ġcult ural +Ġa st +8 2 +as tern +ce ived +ĠC ru +Ġb ull +p ired +am m +Ġfac ing +p ower +Ġb oss +ĠH ol +Ġinst r +Ġincreasing ly +Ġsh ift +Ġstre ets +ĠWilliam s +ab b +Ġl ie +Ġl augh +ĠC a +P L +Ġadult s +Ġcustom er +Ġob tained +Ġsupport ing +ht ml +f ire +Ġdetail ed +Ġpick ed +ĠR ight +ld er +E E +st ood +ĠK im +Ġw ire +Ġs ight +Ġdevelop ers +Ġpers ons +Ġs ad +Ġc up +Ġwar ning +Ġboy s +l ong +Ġb ird +f o +Ġw al +Ġobserv ed +Ġz one +iven ess +Ġch annel +c ript +Ġref used +ĠAg ain +Ġsu c +Ġspokes man +ĠRe f +r ite +ou ston +ãĥ ³ +ĠS her +Ġact s +ĠN ame +Ġstrugg le +ar ry +omet imes +Ġdisc rim +H T +Ġcateg ory +Ġreal ize +Ġemploy ee +ĠAf ghan +en ger +Ġgun s +ĠSte ve +ĠM ot +ĠO l +ok ed +Ġth ick +Ġfair ly +ill y +Ġsur ve +ĠM at +we ight +â Ķ +Ġtro ops +Ġag ents +Ġbatter y +Ġmot iv +à ¡ +S ec +d en +o very +L S +Ġfl u +Ġconf ident +ĠO per +Ġem pty +Ġp hen +Ġse ctor +Ġexc ited +Ġrem ote +ap h +o en +Ġdestroy ed +Ġmor al +ĠH P +ĠR on +Ġd ress +ĠB at +Ġl it +ĠM S +Ġa f +H L +r um +is ms +Ġshould n +Ġsym pt +ĠTor onto +het ic +Ġcar bon +Ġinstall ed +Ġviol ent +Ġsol ar +j a +Ġpract ices +Ġr ide +ĠP enn +Ġimpro ved +Ġaud io +Ġbehav i +ĠP S +Ġe ating +D ata +ĠRe view +p ass +cl aim +u ated +ang ers +c hen +Ġproper ties +Ġany where +An other +Ġbl ow +ĠJack son +Ġp roud +Ġplan e +l ines +Ġsqu are +Ġpro of +ans as +Ġtalk ed +m akers +Ġs ister +Ġhold s +Ġres ident +Ġ= = +Ġresist ance +Ġspl it +Ġpro secut +Ġconf idence +res ents +Ġcut s +Ġexcept ion +Ġz ero +Get ty +Ġcop yright +Ġtot ally +orm al +ific ations +ĠAustral ian +Ġs ick +Ġ1 50 +Ġhouse hold +Ġfe es +Ġdri vers +og en +ĠN Y +Ġnecess arily +Ġregul ations +ear ing +s l +Ġperspect ive +c are +ic ial +H is +Ġesc ape +Ġsurpr ised +ĠV an +ur rent +Ġv ac +8 1 +ĠTh us +Ġem phas +ĠCh ampions +ĠI ce +Ġn arr +Ġhead s +Ġca using +b el +f ortunately +ĠM a +Ġtarg ets +ci pl +Ġafter noon +Ġadd s +ĠMay be +ĠF our +ess ed +ple te +Ġus ual +ch o +ing u +Ġwith d +ĠE nergy +ĠE conom +O O +Ġart icles +Ġinj ured +Ġman age +Ġexpl ains +Ġdi agn +R ec +at ures +Ġlink ed +Ġdiscuss ed +Ġexpl o +Ġocc asion +ath an +Ġopp osite +Ġfac es +Ġden ied +ĠK night +Ġn ut +Ġapprox imately +Ġdisapp oint +onym ous +ĠB est +ĠL o +ĠH y +ĠA ff +Ġvot ing +an while +ĠII I +Ġinstit utions +ag ram +ĠD aily +Ġdr ag +Ġnear by +Ġgu ilty +Ġcon ver +P re +s hip +Ġre ward +Ġphilos oph +ĠS S +u gh +Ġapp s +f riend +Ġu pper +Ġad vert +Ġs now +Ġfr ust +Ġour selves +F r +ĠD ie +amp ion +Ġdis miss +Ġc ere +Ġsign al +f rom +Ġ ). +Ġ5 2 +Ġcr imes +it ors +est ival +use um +Ġcoun cil +ĠS aud +M ay +ĠG un +ic ian +et her +Ġsu fficient +ĠH en +so le +Ġhistor ical +ĠF ar +ĠT urn +Ġp in +Ġsuc ceed +m at +ly mp +Ġtrad ition +ĠO k +Ġc ro +Ġdesc ription +al le +Ġsk y +T e +Ġwide ly +Ġw ave +Ġdefin ition +ĠJew s +Ġcy cle +Ġref ere +Ġbr ings +us al +Ġal ive +Ġfrequ ently +Ġint ention +ĠCont rol +l v +y stem +Ġpriv acy +g ent +ren ce +ĠQu est +ĠChrist mas +Ġr ail +Ġco oper +Ġtest ed +ĠC apt +as ks +Ġcomfort able +Ġdel ivered +sc ape +Ġdep th +ĠG OP +Ġwrit es +Ġass ets +Ġsa v +im ents +Ġtrans ition +Ġart ist +ĠL ook +Ġl ob +Ġcomp onents +ar ity +Ġwalk ed +Ġro ot +Ġparticip ants +Ġnot iced +Ġres c +Ġn av +ĠAd minist +d a +ut ral +pl ate +Ġimport ance +Ġass ert +ious ly +c ription +Ġinj uries +ĠChe ck +Ġregist ered +Ġint ent +Ġmiss ed +ograph ic +Ġsent ence +oun ter +Ġassist ance +ev in +Ġdat abase +Ġbuild ings +Ġclass ic +Ġth inks +ĠOh io +P r +ug g +Ġfe e +p an +Ġeffect ively +Ġfac ility +Ġbe ar +Ġch apter +Ġdog s +ĠCol umb +Ġl atter +it ial +Ġad mitted +T V +ĠGe org +Ġpost s +\ \ +Ġlawy er +Ġequ ival +Ġm and +Ġcontro lled +ĠW alk +ĠAnd rew +Ġmen u +am ental +Ġprotect ed +v a +Ġadminist r +or al +Ġre in +ĠS ar +Ġamount s +Ġn ative +ĠM oon +Ġrep resents +Ġab andon +Ġcarry ing +Ġt ank +m ary +Ġdecl ared +T ube +Ġh at +Ġpun ish +el lect +m es +Ġun iverse +ĠR od +ph y +Ġinf rastructure +Ġ5 1 +Ġopp osed +ow nt +c a +ĠM ake +Ġhard ware +Ġco ffee +R el +b al +w orld +ĠS af +ĠSe a +in als +Ġown ed +Ġh all +ers ion +Ġdescrib e +ĠP ot +Ġport ion +Ġat mosp +Ġgovern ments +Ġdep ending +Ġoff ense +Ġtr ick +aw a +ĠL ine +ĠV is +ĠH ard +ĠOr ig +ĠCl ick +Ġdes k +ĠVal ley +ĠS ov +Ġmov ies +Ġrem ark +Ġm ail +Ġcons cious +Ġrul ing +ĠR ights +Ġmed ic +he nt +ĠW omen +> < +Ġrepl aced +ĠP rem +ĠTh anks +Ġre new +ĠB all +if orm +Ġsh ots +C omm +Ġar med +Ġconst ant +Ġt aste +Ġreal ized +Ġbu ff +Ġm o +Ġeffic ient +M ost +or ation +if ies +Ġcommun ication +Ġfl ood +Ġconsequ ences +Ġany way +ig g +ĠG M +ĠTh ank +Ġ iron +Ġev olution +ĠC op +tw itter +Ġ9 5 +Ġrelationship s +ad el +ĠYou ng +Ġpropos al +ay ers +uild ing +ĠH ot +OR E +c os +Ġcoll abor +P G +ax y +Ġknow ing +Ġsupport s +ow ed +Ġcontrol s +Ġmere ly +um er +Ġath let +Ġf ashion +p ath +Ġg ift +Ġer a +AN D +Ġkind s +ĠKore an +Ġleg it +ul ous +Ġess entially +Ġthe rap +n ic +Ġsuff ered +Ġh ur +Ġprom ise +Ġex cess +Ġover w +Ġpr ime +ĠH ouston +er ry +ĠM s +R S +201 2 +Ġst ores +ĠO lymp +Ġj ourney +Al though +S ub +ĠE duc +ĠCh apter +Ġrequest s +Ġconsum ers +Ġt iny +Ġis ol +ĠF air +b a +ĠY OU +Ġcr ash +ce ler +Ġemot ional +Ġgood s +Ġelect ed +Ġmod er +ĠLin ux +Ġbl ocks +Ġis land +ĠSoc iety +Ġelect ions +Ġbroad cast +Ġche ap +Ġn ations +Ġse asons +4 00 +Ġwas te +ĠS at +Ġfield s +em ploy +Ġprof ile +Ġauth ors +AL L +ĠG ra +w est +ĠT y +Ġdeath s +Ġv acc +Ġfor med +Ġd u +Ġon going +ĠMuslim s +el f +ig ure +Ġass ume +ĠUkrain e +w ater +Ġco ast +Ġvot ed +g or +ĠA S +ĠMich igan +az a +ĠAr m +i ro +Ġf lex +as ters +' ' +Ġwel come +ar l +Ġloc ations +ig ation +ĠF il +Ġbu ying +Ġarch itect +Ġhard er +ĠC ub +Ġinter face +Ġrestaur ant +Ġdisco ver +Ġex ceed +Ġfav our +ger y +Ġd uty +Ġp itch +ad or +ĠM ach +b oy +Ġrespond ed +Ġext ended +her s +M any +ra id +if er +ĠIn s +S er +Ġmed ium +s he +ĠS ports +Ġmag azine +ut ation +Ġlim its +ĠG all +Ġex ternal +raz il +Ġyoung er +t le +Ġrem ind +ĠC ON +Ġimmedi ate +Ġh idden +Ġvol unte +Ġsim pl +od cast +Ġph ase +d r +Ġpl ot +Ġexp osure +R I +og rap +v in +an ish +ĠAc ad +ĠEng ine +Ġexp ansion +ĠP ay +Y our +Ġpus hed +ĠE ll +ĠHe ad +Ġmarket ing +ĠA C +k et +Ġh its +Ġg ro +ĠA ge +ĠSc ot +] [ +Ġst im +Ġi Phone +Ī Ĵ +Ġn arrow +ĠGet ty +ĠTur key +Ġperfect ly +Ġen able +ut ch +Ġprec ise +Ġreg ime +Ġsh if +Ġcomp ens +g un +d iv +Ġch osen +ĠK en +An y +Ġtre es +Ġrecomm ended +ĠR en +u able +ĠH T +F ollow +E G +ĠH and +ĠK enn +Ġarg uments +Ġex ists +Ġb ike +ĠCons erv +Ġbre aking +ĠG ar +Ġc razy +Ġvirt ual +ay lor +ix el +Ġ19 80 +Ġper mission +ĠSer ies +Ġconsum er +Ġclose ly +c alled +Ġ5 4 +Ġhop es +Ġar ray +ĠW in +ĠLab our +Ġsp ons +ĠI re +Ġp ow +Ġread ers +Ġemploy ment +Ġcreat ure +Ġresult ing +Ġaccur ate +Ġmom ents +Ġarg ued +Ġp ed +D uring +Ġ5 3 +ĠT al +Ġs ought +Ġsuff ering +Ġ icon +le e +Ġ( $ +al ian + ° +Ġp ra +Ġbon us +( " +k o +Ġact ing +D E +f all +Ġcompar ison +Ġsm ooth +ĠN AS +u pp +ĠJose ph +ep ing +ĠT ake +ĠM id +Ġs ending +f ast +ĠF all +Ġdeal ing +us er +ĠOr gan +C o +Ġatt ached +Ġse es +% . +Ġtyp ical +AR T +Ġfind s +ĠAs ia +um in +ĠC ore +ĠE nt +in ent +u ce +ĠBl ood +ĠN ever +Ġem ails +Ġhigh light +Ġconf ront +at us +ut ed +Ġun us +Ġtop ic +ĠAd am +Ġb le +at i +Ġunder stood +S et +st ruct +T P +Ġm ob +a a +ĠSt art +pect ed +se ll +Ġded icated +ĠC A +u an +Ġsong s +esc ription +Ġte ch +Ġr ape +Ġas ide +Ġgr ant +Ġ5 6 +s ub +Ġarg ue +Ġcont aining +Ġsche dule +Ġliber al +Ġpublic ly +Ġheav ily +ĠU t +in er +ĠS ection +ĠC are +we et +l s +D is +âĶ Ģ +ĠF ollow +B ack +ĠI T +Ġb es +j i +ĠH it +est ed +Ġevery body +ĠSw ed +Ġfem in +Ġfac ilities +Ġcon ven +C omp +ĠO S +c ore +Ġan x +Ġdiv ision +ĠC am +ĠSt an +m ates +Ġexpl ore +pl om +Ġsh ares +pl oad +an es +Ġide al +et ers +ĠB ase +Ġpl astic +Ġdist inct +ĠNet work +ĠSe attle +Ġtrad ing +ens us +int end +Ġex hib +Ġinit ially +ĠF ood +Ġthous and +ĠBus iness +act er +Ġpar agraph +Ġrough ly +Ġw ww +Ġcreat ive +ĠCon f +Ġconsum ption +Ġfil ms +ag an +Ġob tain +Ġt all +Ġt or +Ġacknow led +Ġg rown +al o +K E +Ġ4 00 +end ers +t aining +U G +Ġsu icide +Ġwat ched +ĠL ist +al i +re hens +Ġsurround ing +Ġp ip +Ġf lying +ĠJ ava +ord an +Ġserv ing +in ations +p ost +Ġsh o +A v +Ġj ail +z y +Ġ199 9 +Ġ< / +Ġliter ally +ĠS ir +Ġexp osed +Ġl ies +st ar +Ġb at +Ġear ned +ĠD ig +Ġspec ified +ĠSe ason +Ġdeg rees +Don ald +Ġcent re +Ġsh aring +Ġwin ter +ĠC O +C he +Ġ Î +M P +Ġun w +Ġfew er +ĠM ir +Ġsomew here +ĠK ey +Ġattack ed +ĠK ir +Ġdom ain +Ġstrong er +Ġ9 9 +Ġpen alty +I d +Sc ript +Ġdecl ined +Ġne ck +Ġfra ud +Ġcur rency +Ġr ising +R C +â̦ â̦ +H z +Ġt ab +Ġtal ent +n am +ĠN BA +Ġvill age +Ġleg s +ĠN ext +E d +Ġac id +Ġhy d +8 00 +Ġinvol ving +ĠIm age +ĠBe fore +F l +Ġyes terday +S ource +Ġterror ist +Ġsu p +Ġsy nt +ĠSaud i +Ġw est +Ġr u +b urg +Ġvis ible +Ġstru ck +r ison +Ġaw esome +Ġd rawn +Ġansw ers +ĠG irl +ĠR am +Ġthreat s +Ġdef eat +os it +Ġv ent +atur ally +Americ an +end a +ĠH oly +Ġr um +% , +c ase +ĠHist ory +ĠYou Tube +Ġsit uations +ĠD NA +S te +Ġsa ved +It em +Ġrec ip +olog ist +Ġfac ed +Ġel ig +O nce +ĠL i +u h +Ġmist ake +ĠDiv ision +ĠB ell +Ġsympt oms + ® +Ġdom in +Ġfall ing +Ġend ing +as hes +Ġmat ches +ĠOn line +Ġexplan ation +D ef +red it +Ġany more +ĠT otal +ĠF OR +us hed +Ġlet ters +Ġris ks +ĠO K +Ġreported ly +: \ +Ġpl ate +Ġsubject s +Ġattempt ed +if ier +ian a +Ġunlike ly +ĠTh ough +um a +ĠIn vest +ĠPr in +ic an +ĠD ar +ĠColor ado +au g +Ġve get +a os +ri a +Ġshe l +Ġmark ed +Ġ( ) +Ġsp r +p o +ĠL ink +Ġdef e +ĠJ r +Ġthem e +Ġpass ion +ĠP en +Ġinf o +iz er +Ġsh it +ĠC ivil +ap se +c re +Ġpo ly +Ġcomp onent +ĠChar les +ĠIre land +ĠPro v +Ġdo ctors +Ġgr anted +Ġpain t +Ġhon or +Ġsm oke +Ġpay ments +Ġprim arily +ĠKing dom +r ich +ate ll +Ġde als +Ġsched uled +Ġfund amental +Ġprote in +Ġnewsp aper +Ġcl ients +yth on +ĠD ate +h us +Ġfeed back +Ġstret ch +Ġc ock +Ġhot el +ĠQue en +Ġsu gar +Ġj u +Ġmil k +Ġappro val +ĠL ive +Ġequival ent +ef ully +Ġins ert +z ona +Ġext ension +d ri +J ohn +Ġacc omp +S m +ĠF und +Ġconst antly +Ġ` ` +Ġgener ated +ĠA ction +ĠP sych +ĠT ri +Ġrecogn ize +Ġv ary +ph a +ĠR a +d f +et ch +ĠSov iet +Tw o +Ġpattern s +Ġprof ession +an ing +T ime +ĠL im +Ġcol ors +ĠA z +ĠT R +Ġinf ect +Ġphen omen +Ġshe ll +Al so +Ġput s +Ġdel ivery +Ġbro wn +Ġprocess ing +Ġlight s +ess age +ĠBro ok +ĠA ud +l ation +Ġindust rial +L ike +ĠB razil +rou s +ES S +ĠL uc +Ġsome how +Ġ8 5 +Ġpro port +Ġpolit icians +Ġindic ate +Ġh ole +Ġtechn iques +Ġcompet itive +Ġph r +Ġv o +ist ent +ĠD ream +Ġcamp us +Ġaspect s +Ġhelp ful +Ġsh ield +or se +Ġtrig ger +m al +Ġ5 8 +Ġt ort +Ġperson ally +Ġt ag +Ġkeep s +ĠV ideo +Ġben ch +Ġg ap +a ire +Ġe ast +Ġrec overy +per ial +Ġprof it +ĠM ic +Ġ5 7 +Ġcol on +Ġstrong ly +st yle +Ġalleg ations +h an +Ġrep orters +j o +r ine +arg et +and al +Ġ0 3 +Ġfl ash +tr ans +Ġstr ict +Ġpark ing +ĠPak istan +Ġl i +Ġwe ird +ĠE ric +Ġreg ions +ĠJ un +Ġint ellect +ĠW H +od ing +rib utes +up id +ĠT it +Ġf inger +or ia +Ġe lev +ĠF ield +Ġcon clusion +; ; +Ġfeel ings +Ġext ensive +Ġm ixed +Ġne uro +v y +Ġhar ass +ĠC irc +ou ch +Ġterrit ory +Ġsuccess fully +M ar +Ġing red +Ġoverw hel +Ġl ayer +V iew +Ġall ies +ill ance +ĠTh ree +Ġb unch +Ġnorm ally +Ġnet works +Ġsac r +ĠC IA +b les +Ġch ose +Ġopp onents +Ġregard less +Ġfr anch +Ġpre f +ĠP o +Ġbr idge +ann a +ĠSil ver +Ġw age +p age +ri or +Ġrad ical +ĠL ittle +Ġman ip +Ġsecret ary +Ġg ang +D R +F A +Ġdec ent +ĠSp irit +Ġun cle +ĠDevelop ment +Ġinvest ors +Ġwall s +Ġpub lish +Ġgener ate +iss ions +c ar +Ġprom ote +Ġcut ting +Ġche st +Ġdrink ing +Ġcollect ed +Ġ7 2 +Ġhop ing +Ġem br +gor ith +Ġwar ned +Ġinstruct ions +O G +ĠD id +ĠAg ency +Ġg ear +Ġcritic ism +ĠF urther +Ġut il +ann y +R ed +Ġcoun sel +ĠAs ian +Ġredu ction +p ool +Ġteach ing +Ġdeep ly +i y +Ġestim ates +Ġcho ices +Ġperman ent +in em +ke l +Ġf asc +p se +f ile +ĠL ow +ĠP erson +Ġt ournament +st al +Ġm el +U ST +ĠR ay +az i +V al +Ġcont ained +ĠH olly +Ġw ake +Ġreve al +Ġprocess es +ĠIS IS +Ġ0 9 +Ġbl ind +Ġste el +ĠB ad +Ġcare fully +app y +ro it +Ġg aming +Ġhous es +ĠC oll +Ġtr uck +er m +Ġsc ored +Ġocc as +ret urn +b ound +v ar +Ġsh arp +Ġaf raid +ĠE X +am ber +c ific +Ġsche me +N C +ĠPol it +Ġdecl ine +Ġ199 8 +Ġpus hing +Ġposs ession +Ġpriv ile +Ġteacher s +Ġy ield +H A +ĠDav is +it led +#### #### +Ġr ig +ĠD aniel +ac on +Ġh ide +ut en +Ġcolle agues +Ġprin ciples +Ġl oud +Ġs in +ĠDem on +Ġst one +Ġ0 2 +Ġt aught +Ġter rible +Ġst uck +ĠPol icy +te en +Ġimplement ation +ĠB BC +ĠAP I +Ġwhe el +all as +Ġch ampions +ol ars +play er +Ġrepeated ly +ĠSt ill +Ġlik es +ast y +es ter +ĠCath olic +R L +Ġb ath +Ġno ise +t itle +Ġn orthern +P art +Ġmag n +Ġf ab +ĠAs h +Ġdis pl +Ġtick et +Ġm urd +Ġalong side +ĠMus ic +Ġr iver +ĠSte el +ĠC L +ĠPl ayer +ĠM ult +ow ing +re p +s ize +Ġt ur +ĠGeorg ia +isc al +ra ction +Ġc able +Ġ5 9 +Ġw ins +Ġup coming +Ġsurv ive +Ġins pired +ĠEduc ation +Ġstat istics +ĠF oot +iam i +Ġy ellow +ĠP age +. - +ĠH as +Ġur ban +Ġa x +es sel +\ " +Ġquarter back +Ġreg ister +ĠLab or +Ġab ilities +ĠF amily +Ġvar iable +ĠPr ice +Ġcont em +Ġth in +ĠE qu +d ata +Ġg otten +Ġconst it +Ġas ks +Ġt ail +Ġexc iting +ĠE ffect +ĠSp anish +Ġencour age +ins on +ĠA h +Ġcommit ment +C S +Ġr ally +Ġ: : +Ġsubs id +Ġsp in +Ġcapt ured +201 8 +Ġinn oc +Ġalleged ly +ĠC ome +Ġart ists +ĠN umber +Ġelect ronic +Ġreg ional +ap es +Ġw ra +Ġmy th +pr ise +ĠM iller +ĠC reat +ĠEp isode +b ell +Ġdirect ed +Ġext ract +Ġs orry +Ġv ice +ag ger +ĠSu pport +Ġ6 6 +ĠI ron +Ġwonder ful +Ġg ra +N et +ion e +E ng +Ġsh ips +ik es +ĠK evin +it ar +Ġactiv ists +tr ue +ĠAri zona +ent h +ĠDes pite +ĠS E +Ġha bit +ern el +Ġin qu +Ġab ortion +Ġv oid +Ġexpl icit +Ġeng aged +Ġang ry +Ġr ating +Ġfr ag +b ro +ick ing +d ev +Ġwor ried +Ġob ser +Ġap artment +ĠG T +Ġest ate +ĠConst itution +em on +ĠS now +Ġcount y +Ġdis ag +ĠStep hen +Ġimm igrants +w ind +ĠN ations +Ġfol ks +O ut +Ġg all +Ġtarget ed +Ġst ead +ĠB on +ĠL ib +Ġinform ed +Ġ12 0 +ch ain +idel ines +or ough +Ġdri ven +Ġregular ly +Ġbas ket +Ġprinc iple +oc ument +Ġst un +ib ilities +ĠRom an +ĠAb out +Ġal ert +Ġdemocr acy +Ġrepresent ed +H S +c ers +p arent +Ar t +p ack +Ġdi plom +re ts +ĠN O +Ġcapt ure +ĠAd v +Ħ ¢ +Ġannounce ment +ĠL ear +Ġh ook +Ġpur s +ĠS uch +ĠC amer +Ġrefuge es +ĠV e +P ol +Ġrecogn ized +l ib +Ġhad n +A ss +Ġpil ot +us hing +Ġreturn ing +Ġtra il +ĠSt one +Ġrout ine +Ġcour ts +Ġdes per +Ġfriend ly +ĠIt aly +Ġpl ed +Ġbreat h +Ġstud io +N S +Ġimp ressive +ĠAfghan istan +Ġf ing +Ġd ownt +ink ing +ĠR og +i ary +col or +se x +ar on +Ġf ault +ĠN ick +D own +ĠR ose +ĠS outhern +X X +is odes +L ist +6 00 +Ġout come +er r +Ġelse where +Ġret ire +Ġp ounds +ĠGl obal +Pe ople +Ġcommun ications +Ġlo an +Ġrat io +ĠEm pire +Ġg onna +Ġinv ent +D F +Ġ19 70 +ĠComm on +p at +Ġprom ised +Ġd inner +ĠH om +Ġcreat es +Ġoper ate +ver ty +ĠJ ordan +et ime +Ġsust ain +R eg +Ġincred ible +im a +Ġwar rant +Ġm m +A tt +Ġlaw suit +Ġreview s +it ure +ĠS ource +l ights +ĠF ord +Ġ6 3 +g roup +st ore +Ġfeat ured +Ġfore ver +Ġpo verty +ĠP op +ĠC NN +az z +ab is +ach ing +Ġl aid +ĠSu pp +Ġfil ter +en a +ĠCommun ity +Ġcreat ures +u ction +ĠR oyal +Ġassoci ation +ĠCon nect +ĠBr ad +âĸ Ī +l ers +the re +ĠG i +Ġval uable +AC K +ĠT aylor +Ġl iquid +ĠAtt orney +ĠCar l +ĠF inal +ag a +ĠWil son +B ecause +ĠProf essor +ak a +Ġincred ibly +r ance +! ) +R ef +s k +Ġsol utions +Ġatmosp here +Ġbl ame +um es +ĠN ob +C A +um ps +r ical +ĠPut in +ĠD est +or ic +ĠP A +Ġrespect ively +w an +Ġfif th +â Ħ¢ +ĠC ry +Ġgovern or +res ident +Ġpurch ased +Ġh ack +Ġint ense +ob s +Ġorig in +Ġdef ine +Ġcare ful +** * +Ġshould er +Cl ick +Ġt ied +Ġdest ruction +ou red +Ġno body +Ġh o +ĠEx per +Ġt ip +" ; +Ġtechn ique +Ġj ur +ĠP ok +b ow +Ġleg end +Ġacc ord +Ġbus y +ĠInt el +Ġh ang +ak i +. ] +âĢĶâĢĶ âĢĶâĢĶ +Ġsur gery +Ġrep rodu +Ġun iform +Ġscen es +c ode +Ġ6 2 +l isher +ĠH ave +ph ia +Ġcry pt +Ġrec on +Ġsc ream +Ġadop ted +Ġsc ores +N e +ĠIt alian +in cluding +B O +Ġindic ated +Ġent ertain +G u +T ext +i el +Ġtw enty +Ġeng age +off s +ĠPac ific +Ġsm ile +Ġperson nel +Ġto ler +Ġdo ors +Ġt one +Ġmach ines +Ġent ering +ten ance +C O +ĠJer sey +Ġfore st +Ġhor se +Ġcompl aint +ĠSpr ing +y o +ĠPl us +ed ing +ĠRet urn +qu arters +ial s +c ow +Ġacad emic +Ġf ruit +Ġ199 6 +og ether +Ġw ine +Ġpur su +ĠSte ven +Ġlic ens +Wh o +Ġclot hes +re ction +Ġsqu ad +Ġst able +Ġr aw +z ens +St ar +ut ies +anc er +Ġke ys +ĠM u +Ġcompl icated +ig er +ĠTe xt +Ġabs or +Ġ6 8 +Ġfun ny +Ġrel ief +ĠL ew +ĠC ook +Ġch art +Ġdraw ing +G E +Ġmod ule +ĠB ull +I LL +Ġs alt +0000 0000 +il le +Ġres ource +aw ay +adel phia +ĠB ru +Ġ6 7 +Ġsome body +Ġparticip ate +Ġro se +we red +Ġmus cle +Ġcons ent +Ġcontin uing +ĠGuard ian +ĠOr der +reg on +Ġre ar +Ġprov ision +Ġlik ed +ri ent +Ġb ra +Tr ans +Ġmeet ings +Ġto x +Ġcon vent +Ġaut o +Ġrec ording +ĠSo ft +00 1 +ĠR oll +Ġprogram ming +Ġp ic +Ġprov ed +Ġst ab +ĠA st +Ġca ption +ul ating +ĠAtt ack +Ġnew ly +Ġ199 7 +f r +Ġdis cipl +ĠGree k +Ġed ition +ĠDo es +ĠB ox +if le +ack et +Ġpass es +Ġgu est +Ġac celer +it als +U D +Ġaut hent +ĠR est +ov al +t a +u ine +Ġarm or +ĠT own +Ġcomp at +Ġinc hes +Des pite +Ġass ign +he rent +Ġprep are +ĠM eg +oc key +Ġdep ends +Ġtrack s +w atch +Ġl ists +ĠN orthern +Ġal ter +re c +ĠE astern +Ġcond em +Ġevery where +? ' +Ġaff ili +Ġf ought +": {" +Ġm ac +it arian +Ġsc ope +ĠA L +aw s +ar ms +Ġqu e +Ġenjoy ed +nes ota +Ġagg ressive +ĠSt ory +ĠI V +Ġrec ipe +Ġrare ly +ĠMed ical +val ue +ang el +ay ing +omet hing +Ġsub section +Ġs outhern +Ġfrequ ency +re te +roll ed +ult s +ĠN ic +Ġbeh alf +Ġsequ ence +ab et +Ġcontrovers ial +Ġcomp rom +Ġwork er +Ġmain ly +Ġal gorith +ĠM ajor +or ce +g ender +Ġorgan ized +Ġf ake +Ġconclud ed +ĠE D +ĠEx ec +r age +Ġch ances +ber ry +ĠTr ad +Ġconfig uration +Ġwithd raw +Ġf ro +ud es +ĠBro ther +ĠB rian +Ġtri es +Ġsam ples +Ġb id +ĠGold en +Ġphot ograph +if est +ĠD O +ĠPar liament +******** ******** +R em +Ġcont est +Ġsign ing +p x +ĠZ eal +âĶĢ âĶĢ +E ar +Ġex it +Be fore +ĠCor por +n ull +mon th +Ġrac ial +ott ed +ĠV eg +ĠRe uters +Ġsw ord +ps on +ĠRom ney +a ed +Ġt rib +Ġin ner +Ġprot ocol +ĠB i +ĠM iami +ever al +p ress +Ġsh ipping +ĠAm endment +ĠHow ard +con nect +ĠD isc +ĠJ ac +iam ond +ĠThere fore +s es +ĠPrin cess +ĠUS B +ĠAn th +Ġsurve illance +Ġap olog +Ġ6 1 +ow a +Ġf ulf +j s +Ġl uck +ust ed +Ġ § +n i +Ġant icip +em an +Ġwin ner +Ġsil ver +ll a +ic ity +Ġunus ual +Ġcr ack +Ġt ies +e z +Ġpract ical +Ġprov ince +ĠPl ace +Ġprior ity +IC E +Ġdescrib es +Ġbr anch +F orm +ask a +miss ions +b i +Ġp orn +ĠTur k +Ġent hus +Ġf ighters +Ġ0 8 +ĠDet roit +Ġfound ation +av id +A re +Ġjud gment +cl ing +Ġsol ve +ĠDes ign +W here +hes is +ĠT ro +a fter +Ġne utral +ĠPalestin ian +ĠHolly wood +Ġadv is +ĠN on +y es +ol is +Ġrep utation +Ġsm ell +Ġb read +ĠB ul +ĠBe ach +Ġclaim ing +Ġgen etic +Ġtechn ologies +Ġupgr ade +row s +Ġdevelop er +ĠJ osh +ĠDis ney +erv ed +ip al +Ġun ex +Ġbare ly +t hen +ĠP ub +Ġill ness +et ary +ĠB al +Ġp atch +Ġbut t +Ġst upid +ĠD og +ĠD allas +f ront +ie ce +Ġprot ests +Ġch at +oen ix +Ġw ing +Ġpar liament +Ġ7 7 +ose xual +Ġre nder +pt ions +ĠCo ast +os a +ĠG reg +h op +ĠMan agement +Ġbit coin +Ġrec over +Ġincor por +or ne +ĠUs ing +Ġpre ced +Ġthreat ened +Ġspirit ual +ĠE vent +ĠF red +Ġadvert ising +Ġimprove ments +ĠC ustom +Ġer rors +Ġsens itive +ĠN avy +Ġcre am +L ook +Ġex clusive +Ġcomp rehens +Ġde leg +Ġcon ce +Ġrem em +Ġstruct ures +Ġst ored +N D +Ġ1 000 +U P +ĠB udd +A F +w oman +ĠAcad emy +ð Ł +se a +Ġtem porary +Ab out +es ters +Ġtick ets +Ġposs ess +in ch +o z +Ġl a +Ġcontract s +Ġun p +Ġc ig +ĠK at +ult ural +as m +Ġmount ain +ĠCapt ain +St ep +m aking +ĠSp ain +Ġequ ally +Ġl ands +at ers +Ġreject ed +er a +im m +ri x +C D +Ġtrans action +g ener +less ly +Ġ| | +Ġc os +ĠHen ry +Ġprov isions +Ġg ained +Ġdirect ory +Ġra ising +ĠS ep +ol en +ond er +Ġcon sole +in st +Ġb om +Ġunc ertain +1 50 +ock ing +Ġmeas ured +Ġpl ain +Ġse ats +Ġd ict +S L +af e +Ġest imate +iz on +at hered +Ġcontribut ed +Ġep isodes +omm od +G r +AN T +Ġ6 9 +G ener +Ġ2 50 +vious ly +rog en +Ġterror ism +Ġmove ments +ent le +oun ce +ĠS oul +Ġpre v +ĠT able +act s +ri ors +t ab +Ġsuff er +Ġn erv +Ġmain stream +ĠW olf +Ġfranch ise +b at +Ġdem ands +Ġag enda +Ġdo zen +Ġclin ical +iz ard +ĠO p +t d +Ġvis ited +ĠPer haps +Ġact or +Ġde lic +Ġcont ribute +Ġin ject +ĠE s +ac co +Ġlist ening +Ġcon gress +epend ent +Ġprem ium +Ġ7 6 +ĠIr ish +Ġass igned +ĠPh ys +Ġworld wide +Ġnarr ative +ot ype +m ont +b ase +ĠB owl +ĠAdminist ration +Ġrel ation +ĠE V +C P +Ġco vers +Ġ7 8 +Ġcert ific +Ġgr ass +Ġ0 4 +pir acy +ir a +Ġengine ering +ĠM ars +Ġun employ +ĠFore ign +st ract +Ġv en +Ġst eal +Ġrepl ied +Ġult imate +Ġtit les +d ated +Ġj oy +a us +Ġhy per +ak u +Ġoffic ially +ĠPro duct +Ġdifficult y +per or +Ġresult ed +rib ed +l ink +wh o +~~ ~~ +ĠSpe ed +ĠV iet +W ind +ĠBar ack +Ġrestrict ions +ĠSh are +Ġ199 5 +ition ally +Ġbeaut y +op t +Ġm aps +ĠC R +ĠN ation +ĠCru z +W ill +Ġelectric ity +Ġor g +Ġb urd +Ġviol ation +Ġus age +Ġper mit +ĠCh ron +ĠF ant +Ġn aturally +Ġ0 7 +Ġth rown +ĠAw oken +Ġal ien +ĠHer o +ĠK ent +ĠR ick +ri ke +Ġp ace +}, {" +G L +Ġpo ison +ĠT ower +Ġform al +al ysis +Ġgen uine +Ġk il +a ver +Ġproced ure +ĠPro p +intend o +ĠM ain +as ant +Ġtr ained +G ame +ĠL oad +ĠM A +Ġcru cial +Ġle ts +ĠF R +Ġch ampion +1 01 +ĠCon ference +Ġwrit ers +Ġconnect ions +Ġo kay +ir ms +ĠR and +Ġenc ounter +ĠB uff +Ġachie ved +Ġche cks +isc ons +Ġassist ant +Ġwhen ever +ĠA ccess +ĠU r +b in +Ġcl ock +is p +op her +Ġb orrow +Ġm ad +Ġperson ality +on ly +IS T +ab ama +Ġg ains +Ġcommon ly +Ġter r +Ġhyp ot +Ġre ly +Ġt iss +iscons in +Ġrid ic +f unction +ĠO regon +Ġun com +r ating +el and +ĠN C +Ġm oon +ann on +Ġvulner able +ut ive +³³ ³³ +ĠRad io +Ġw estern +se ct +ĠT ony +Ġocc urs +ĠO s +ĠH on +Ã Ń +Ġv essel +ĠScot land +Ġdiscrim ination +Ġsubsequ ent +st ring +Ġfant asy +ĠSh adow +Ġtest im +W E +it i +r as +Ġbo at +Ġmar ks +Ġord inary +Ġre n +Ġrepresent ative +Ġpet ition +Ġ7 3 +Ġad venture +Ġign ore +ĠPhil adelphia +ĠS av +V P +Ġfact ory +Ġt asks +Ġdep ression +z ed +................ ................ +ĠSt orm +Ġc ogn +Ġelig ible +Ġredu cing +v ia +Ġ0 5 +Ġstri king +Ġdoll ar +h o +O V +Ġinstr ument +Ġphilosoph y +ĠMo ore +ĠA venue +Ġrul ed +ĠFr ont +IN E +ĠM ah +Ġscen ario +ĠNAS A +Ġen orm +Ġdeb ut +Ġte a +T oday +Ġabs ence +S im +Ġh am +le ep +Ġt ables +ĠHe art +M I +K e +re qu +V D +m ap +Ġchair man +Ġp ump +Ġrapid ly +v i +Ġsubstant ial +E P +d es +ch ant +ili pp +ĠS anta +ri ers +anche ster +L oad +ĠC ase +Ġsa ving +Ġ7 4 +ĠA FP +er ning +oun ced +ĠMin nesota +ĠW as +Ġrec ru +Ġassess ment +ĠB ron +U E +Ġdynam ic +Ġf urn +ul ator +Ġprop ag +h igh +Ġacc ommod +Ġst ack +ĠS us +w rit +Ġre ven +ĠGod d +ĠZeal and +ab s +Ġbr ut +Ġper pet +h ot +Ġhard ly +ĠB urn +ãĤ ¹ +Ġst y +Ġtrans actions +Ġg ate +Ġsc reens +Ġsub mitted +Ġ1 01 +Ġlangu ages +ugh t +em en +Ġfall s +Ġc oc +Ĥ ¬ +Ġstri kes +p a +Ġdel iber +ĠI M +Ġrel ax +ann els +ĠSen ator +Ġext rem +Ġ} , +ĠDe b +Ġbe ll +Ġdis order +c ut +Ġi OS +Ġl ocked +Ġem issions +Ġshort ly +" ] +ĠJud ge +ĠS ometimes +Ġr ival +Ġd ust +Ġreach ing +F ile +¯¯ ¯¯ +ino is +ĠJ ason +Ġs atell +are t +Ġst ations +Ġag ric +ĠTechn ology +com es +ĠUn fortunately +ĠChild ren +Ġappl ies +ast ed +Ġan ger +ail ability +ĠDam age +Ġcomp are +ĠStand ard +Ġaim ed +ĠB a +angu age +Ġreg ulation +Ġj ury +Ġair port +Ġse ctions +ĠPr ince +em ed +Ġmedic ine +Ġh itting +Ġsp ark +ol ves +Ġad s +St ate +Ġfood s +Ġrepl acement +Ġch icken +Ġlow est +Ġmind s +Ġinvol ves +u i +Ġarr ang +Ġproced ures +ĠWh ich +ivers ary +Ġb ills +Ġimprove ment +Ġin ev +Ġexpect ations +Ġintellect ual +Ġsp aces +Ġmechan ism +2 50 +bre ak +ĠZ e +ĠT enn +ĠB alt +Ġbar rel +Ġstat ic +man n +Pol ice +Ġt ips +Ġhand ling +c us +od ed +il ton +ir y +Ġjournal ists +our se +Ġcom ic +Ġnom ine +IT Y +Ġvers us +Ġlo op +Ġsur f +ĠInd ust +ĠHun ter +Ġbelief s +is an +Ġset up +Ġbre w +im age +Ġcomput ers +f ol +} ," +ĠMed al +Ġtax p +Ġdisplay ed +Ġg rav +Ġf iscal +M on +ĠMos cow +ĠK ong +ĠCent re +Ġcamer as +ĠMr s +ĠH ay +Ġa ver +ĠK elly +p y +Ġrequire ment +Ġent itled +omb ie +Ġsh adow +ag ic +ĠA k +Ġel ite +Ġdiv ided +Ġhead ing +Ġcop ies +Ġloss es +Ġv it +k ed +ĠB ry +Ġan s +ĠSte am +Ġrep orter +he im +ĠIt em +Ġsuper ior +d on +ere nt +à ¶ +Ġtherap y +Ġpe ak +ĠMod el +Ġl ying +Ġg am +z er +r itten +Ġrespons es +Ġconsider ation +ĠB ible +Ġl oyal +Ġinst ant +Ġp m +ĠFore st +à ¼ +Ġext end +Ġconv icted +Ġfound er +Ġconv in +ĠO ak +che ck +Ġsch olars +p ed +Ġover se +T op +c ount +ĠAr k + · +Ġ0 6 +ĠL A +m d +ĠLat in +im ental +ĠC PU +Ġsubst ance +Ġminor ity +Ġmanufact uring +E r +ocol ate +Ġatt ended +ĠMan ager +r ations +Ġappreci ate +om y +GB T +id ency +B L +Ġguarant ee +pos ition +Ġo cean +clud e +Ġhead ed +Ġt ape +Ġlo ose +Ġlog ic +Ġpro ven +Ġsp ir +Ġad mit +is a +Ġinvestig ate +Ġ199 4 +sy lv +ĠL ost +c est +Ġ7 1 +Ġrequest ed +Ġwind ows +ĠPok é +ĠWith out +M et +Ġbehavi our +Ġread er +Ġh ung +ĠKe ep +Ġro les +Ġimplement ed +Ġbl ank +Ġserv es +ĠJ ay +Ġc ited +ĠF riend +prof it +ap on +Ġrep air +it em +arr ass +Ġcrit ics +ad i +ĠF ather +Ġsh out +Ġf ool +Ġ8 8 +Ġprodu cing +Ġl ib +Ġround s +Ġcirc le +Ġpre par +Ġsub mit +Ġn ic +mor row +ãĥ « +U nder +Ġv ital +ater n +Ġpass word +Ġpublic ation +Ġprom inent +Ġspeak s +Ġb ars +Ġde eper +ĠM ill +port ed +Ġw id +Ġbut ter +Ġsm oking +Ġindic ates +K ey +rop ri +ĠF ile +all ing +ast ing +ĠR us +Ġad j +Ġ7 9 +av al +Ġpres um +bur gh +on ic +Ġf ur +Ġpoll s +ik a +Ġsecond ary +Ġmon ster +ig s +ĠCur rent +E vent +Ġowners hip +end ar +Ġarri ve +ĠT ax +Ġn ull +ĠPri v +Ġth ro +Ġk iss +c at +Ġup set +ang le +it ches +ect or +olog ists +ĠGal axy +Ġcor ruption +Ġh int +ent er +ĠH ospital +Ġgreat ly +Ġbeg un +es y +Ġso il +ĠAnt on +Ġmain tenance +ãĥ © +Ġdo zens +Ġhuman ity +ĠAl abama +Ġr om +w orth +ap ing +sylv ania +l ah +Ġg athered +G A +Ġattack ing +f ound +ĠSqu are +Ġar bit +ict ions +ĠW isconsin +Ġd ance +ĠS aint +arch y +Ġbase ball +Ġcontribut ions +Ġliter ature +Ġex ha +per ty +t est +Ġb ab +Ġcontain er +let ter +Ġfall en +Ġwebs ites +Ġbott le +ĠS ac +Ġbre ast +ĠP L +Ġveter an +Ġinterview s +ĠA le +Ġb anned +eng ers +ĠRev olution +in th +Ġconc erning +IV E +Ġexp enses +ĠMatt hew +ĠColumb ia +d s +ist ance +Ġent ity +.. ." +Ġrel iable +Ġpar alle +ĠChrist ians +Ġopin ions +Ġin du +l ow +Ġcompet e +Ġth orough +Ġemploy ed +Ġestablish ment +ig en +ĠC ro +Ġlawy ers +ĠSt ation +T E +ĠL ind +ĠP ur +it ary +Ġeffic iency +âĢ IJ +ĠL y +Ġm ask +Ġdis aster +Ġag es +ER E +es is +ĠH old +Ġcas ual +b led +Ġen abled +ĠEn vironment +ĠInt elligence +i per +ĠM ap +ĠB E +Ġemer ged +is dom +Ġc abin +Ġregist ration +Ġfing ers +Ġro ster +Ġfram ework +ĠDo ctor +et ts +Ġtransport ation +Ġaware ness +H er +Ġattempt ing +O ff +ĠSt ore +ÃĥÃĤÃĥÃĤ ÃĥÃĤÃĥÃĤ +ĠK now +Ġdef ence +Ġsc an +ĠT en +ĠCh air +ĠP H +ĠAtl anta +Ġfuck ing +Ġans wered +b n +ĠK ar +Ġcateg ories +Ġr ational +Ġc ust +Ġrob ot +Ġcorrect ly +Ġg if +Ġgraph ics +m ic +Ġground s +ĠO pp +i ate +Ġdist ributed +Ġsan ctions +Ġchalleng ing +ut o +Ġingred ients +Ġinv ited +Ġfound ed +ĠRe qu +d ed +Ġb owl +Ġbrother s +ĠH a +I O +Ġw ages +im ore +oc ial +Ġse ed +ative ly +Ġaddress es +ĠI owa +ab eth +Ġatt itude +is d +ch ild +Ġm ole +Ġdisco very +y ard +B r +Ġ8 2 +Ġsuppl ies +ell ing +Ġdist ingu +C R +Ġre cept +Ġ vert +Ġsw im +b ec +d oor +ĠY eah +Ġg al +Ġinter act +ĠE SP +ĠC S +amp s +Ġconvin ced +Ġobject ive +Ġdis h +ĠPhot os +l ad +Ġdownt own +o il +in ction +Ġto morrow +ĠC OM +Ġsurv ival +sh ot +Ġsett lement +C ons +ĠX box +int erest +ĠS M +arg o +en ess +Ġeth nic +b ered +M in +ĠT ok +Ġinc ent +ĠComm and +Ġmain tained +Ġbreak s +br idge +at ar +ag g +ĠF inally +un icip +ĠO nt +le ft +Ġrecogn ition +Ġ* / +ĠP ers +Ġwe lf +Ġaddress ed +ĠK ansas +Ġvir us +Ġwhere as +Ġp apers +ram s +ĠMin istry +Ġple asure +Ġacqu ired +Ġd uration +j pg +Ġcal m +ĠN HL +Ġburn ing +Ġfold er +ick ed +ĠP y +ĠIll inois +Cl ass +ĠGodd ess +Ġperform ing +Ġwelf are +j ar +In ter +Ġl in +Ġenh ance +Ġnot ion +f are +yp es +ĠAre a +Ġcann abis +ĠDie go +f s +ĠM anchester +com m +in ite +Ġcover ing +ĠS ound +Ġ19 60 +Ġ8 4 +e lect +z ing +Ġcitiz en +Ġph ones +Ġr aid +Ġign ored +ĠOb ject +Ġu pload +c ard +Ġmod ified +Ġroom s +ia h +r ange +he ast +ach us +Ġsuggest ing +âĢ ĭ +gr ade +E l +Ġclot hing +Ġr h +ĠH an +un ity +en cing +ĠAust in +sec ution +t ra +d em +ĠQ ual +Ġhe aven +Ġst ages +Ġw edd +pl us +ific ial +ĠIm m +ĠH o +iet ies +Ġphr ase +Ġbr ill +act ory +Ġprov iders +Ġsil ence +Ġa er +ĠA I +ĠAd venture +Ġplatform s +Ġdemonstr ated +Ġinter f +ing ton +Ġr aces +Ġgr ade +ult ane +ĠTh rough +f alse +Ġb ow +ĠA B +Ġfl avor +Ġhistor ic +g ov +Ġcol our +Ġview ed +ĠEm ail +el come +Ġinter vention +Ġd iversity +Ġperiod s +Ġre verse +ĠV ery +Ġqu ote +ĠLe ft +th rough +Ġsc rew +Ġland ing +Ġp ill +Ġw et +Ġprot esters +Ġrepe at +av ed +er k +Ġsal ary +ĠPenn sylvania +St ill +Ġmay or +Ġkit chen +Ġfeat uring +ĠM useum +ĠT ournament +ĠF al +Ġser vers +U C +Ġany body +im g +ĠTr ade +ixt ure +the less +Ġfin ance +Ġcl osing +ĠPat ri +i ac +ab el +Ġ> > +or ous +Ġf irms +sc reen +un a +Ġemb arrass +ul se +Ġlet ting +Ġth rew +ile y +Ġch annels +l an +ĠVeg as +Ġse ar +Ġfant astic +ar re +uzz le +ĠD er +Th ose +Ġsw ing +Ġshe et +ind ex +co ver +og an +Ġvari ables +ĠTe ch +Ġsp oken +ac hel +ĠD a +ĠMount ain +Ġload ed +Ġfoot age +vers ion +Ġun l +ĠPh oenix +Ġthrow ing +Ġf iring +Ġtrack ing +Ġw idth +Ġstrugg ling +ro oms +ot ion +Ġmonth ly +ĠSer ver +Ġegg s +op en +M C +Ġ199 3 +Ġh ired +Ġstay ed +ĠAll en +Ġst ro +Ġ9 8 +st ep +ĠTurk ish +Ġfab ric +ist ing +ĠD om +Ġd ates +Ġpr on +Ġbasket ball +Ġl ucky +ĠArab ia +Ġassum ed +est y +Ġaff airs +Ġgl ad +ĠInd eed +ĠF A +ĠW ord +Ġjo ining +if ice +p read +ir ts +ĠSe lect +Ġpop ulations +aw are +Ġn ose +Ġcompl aints +st art +Ġsc oring +Th anks +Ġmin ing +Ġvisit ors +S H +Ġdam aged +Ġcharacter istics +ĠP ent +D C +Ġ8 3 +ĠS ix +r ates +Ġfl ags +ĠB rew +d og +M ark +// // +Ġexec ution +Ġj oke +ph ones +Ġtestim ony +Ġob st +Q L +ĠC ut +Ġstud ied +ĠN intendo +ick et +ĠN BC +Ġl ad +ĠB ra +ĠM oh +Ġk ernel +Ġoverwhel ming +Ġag ed +Ġapplic able +ĠC ond +Ġroad s +ĠBl ock +m ade +od ge +Ġcomm ands +Ġoff ices +vel and +Ġt ut +Ġrece iver +ĠF ro +Ġsho pping +Ġi P +ĠSt re +ĠA BC +Ġentertain ment +ĠB ow +ort ed +M c +Ġread s +gr ad +ĠCol lect +Ġâ ĪĴ +ĠCap ital +eder ation +Ġemploy er +Ġinvolve ment +Ġanx iety +al ia +Ġro of +ĠAm ong +ĠDemocr at +Ġstat s +ĠV ill +Ġconst itutional +Ġrefer ring +itt y +Ġtack le +out ube +Ġback ed +ĠH ong +ĠBro ad +Ġe le +ĠO tt +Ġ199 2 +h our +achus etts +C al +Ġdefe ated +Ġ8 1 +es p +Ġseem ingly +w as +ĠJ enn +ĠK urd +Ġg ene +Ġdisc ount +R et +EC T +( ); +Ġclub s +Ġs id +ĠM arsh +Che ck +Ġp p +ĠE ag +ides pread +Ġbe ings +F T +Ġintrodu ction +ĠCh ange +AR D +Ġ1 10 +ad ows +ier ce +Ġme al +a uthor +ĠB ang +lah oma +Ġr anks +201 1 +?? ?? +m ax +Ġcoll apse +Ġop ens +Ġe cho +Ġs oph +Ġrac ist +Ġenorm ous +Ġw aves +Ġt ap +Ġcomprehens ive +. -- +ĠR oy +Ġfarm ers +Rel ated +a ired +ron es +ĠC rim +Ġproport ion +Ġdesign s +Ġnegoti ations +Ġvirt ually +ĠBat man +Ġwar n +Ġlegit imate +m ate +Ġcon vention +, , +net ic +ĠS D +Ġconsist ently +Ġcompens ation +Ġpunish ment +Ġy e +Ġt ie +ĠB ureau +ir lf +ĠB u +ĠA ren +ĠPh ilipp +Ġkn ife +Ġmem ories +ĠR oss +Ġang le +Ġ8 6 +ĠTh under +Ġre nd +ĠT our +Ġcount s +s ung +ĠIm p +Ġeduc ational +Ġaccess ible +C OM +Ġd rew +y er +G l +am ine +OR T +O B +I B +m aster +Ġtri als +og y +h ar +ĠTr ust +Ġprefer red +irlf riend +ĠN ev +Ġb in +Ġc ow +P age +Ġsign ature +ĠB L +7 00 +Ġret ired +Ġby tes +Ġneigh b +ĠLeg end +Ġdev ast +Ġsuspect ed +is ons +ĠPoké mon +sc ale +Ġcap abilities +Ġre vel +Ġche ese +d y +igr ant +Ġfail ing +b its +ĠHer oes +ĠG host +ĠS cient +Ġappoint ed +ur i +Ġinst itution +Ġexpand ed +g reg +Ġmonitor ing +Ġp odcast +Ġcoal ition +Ġ9 6 +J o +Ġst olen +ĠS ab +Ġstop s +Ġhol iday +Ġint r +C ar +Bl ack +ĠL GBT +Ġwar ming +ĠAnd erson +Ġ8 9 +Ġprodu cer +M ed +Ġaccur acy +ĠMar vel +iz abeth +ĠPat rick +m ony +Ġmin i +ac les +Ġover t +the y +Ġmembers hip +ĠV en +Ġex ch +Ġrem oval +ĠD ave +T Y +m ad +ĠF ind +Ġad equ +Ġe c +Ġte eth +Ġemot ion +Ġper m +Ġsole ly +d b +Ġextra ord +IG HT +c al +Ġgu idelines +Ġd ying +Ġsusp ended +ĠPrem ier +ĠAnth ony +el ve +Ġd ad +ĠE th +ĠFoot ball +Ġabandon ed +Ġ< < +Ġm arch +Ġhor ror +â̦ " +Ġchild hood +Ġcampaign s +Ġl unch +ĠAl bert +bl ock +âĸĪ âĸĪ +ound ing +Ġb one +or gan +ad ers +ĠFl ash +ĠDri ve +Ġton ight +Ġw ars +ĠF L +Ġform ation +con st +New s +Ġcom pe +or ious +ĠSt aff +Ġdiscuss ions +ĠProt ection +ĠJ am +Ġcrit eria +Ġinstall ation +Ġaccompl ish +iz za +Ġpub lisher +Ġresc ue +ĠT ry +U LL +ĠS om +ĠH op +ore t +th s +ord on +Ġp ocket +ĠIn v +Down load +ĠCr ime +Ġb ene +ĠGu ide +ĠAs sembly +Ġparam eters +I E +ĠAlex ander +Ġconc ert +ĠSc he +Ġsh oes +Ġvis iting +Ġrec all +Ġb ub +Ġr ural +Ġconc rete +ĠR os +N ext +R uss +Ġlo ans +ĠSh ield +Ġtre m +hem at +k g +ĠHar ris +is ition +ĠM ove +ĠF C +Ġf ate +ĠCh o +Ġt ired +Ġprinc ipal +h ist +ien ces +ath y +Ġse vent +Ġm ood +Ġstrateg ic +Ġdise ases +Ġfor um +Ġtem por +Ġhead quarters +P ar +ig e +fl ix +Ġgu itar +Ġ9 4 +On ly +Ġrele ases +ro ph +================ ================ +Ġ6 00 +ĠContin ue +ig ate +ĠC rit +sy stem +Ġdis abled +Ġunex pected +ith ub +Ġuncle ar +ĠE st +Ġcontr ad +Ġstrateg ies +vent ures +Ġpass age +AM E +Ġimpro ving +Ġreve als +Ġdecre ase +ov a +Ġann oy +ĠSh ort +ĠL ibrary +Ġcy ber +n ell +ĠH ur +ĠC B +Ġphot ograp +U I +Ġs ed +G e +Ġ8 7 +Ġd iverse +Ġencour aged +Ġcons piracy +Ġbird s +Ġoper ator +Ġhand ful +Ġclass ified +? ) +Ġdram atic +Ġinvestig ators +it o +Ġw idespread +ĠR oom +-------------------------------- -------------------------------- +Ġcollect ive +Ġjournal ist +St ring +Ġtemper atures +il a +Ġgu id +Ġins pect +Ġmiss ile +ĠMay or +Ġman ual +Ġsim ultane +Ġrat ings +Ġsu ck +Ġ9 7 +Ġunivers al +Ġph arm +Ġdis rupt +ian o +A V +Ġf t +Ġstat ist +old s +ĠWalk er +ph p +Ġunder t +ĠL as +ish op +nt il +res hold +ĠWhe ther +M s +Ġden y +ĠCl oud +Ġprov ider +Ġsurv iv +ĠUp date +h as +Ġmist akes +ch arge +pl ed +r ity +Ġn ode +ĠMass achusetts +ool s +lic ation +Ġf ails +em ale +or i +back s +Ġsh irt +Ġ' ' +ĠN AT +Ġwat ers +els on +Ġe ase +Ġsc ar +Ġcont ents +m ind +Ġcont ribution +Ġsh r +Ġhand ed +Ġst ability +Ġtra ve +E m +Ġmir ror +12 3 +Ġwe igh +Ġf iction +ou ver +ist ant +r ition +ĠF ed +Ġphys ically +Ġst ake +ĠArt icle +ĠAr c +ĠLew is +ĠM ind +Ġdemonstr ate +Ġprof its +v ision +om ic +ol id +Ġbatt les +Ġdri ves +Ġeas tern +ĠS ony +!! ! +ar ation +v ard +ĠG L +port ation +Ġ9 2 +Ġlaw makers +Ġprotect ing +ĠE PA +Ġy eah +Ġsh ame +ol ph +e ven +x it +Ġatt ach +Ġrepresent ing +Ġob s +ĠUt ah +iff s +ĠFre edom +à ³ +A K +Ġinc idents +it age +Ġview ers +c d +Ġm ouse +Ġcl ar +Ġaccord ance +Ġb ot +c or +ĠSum mer +he ld +Ġinnoc ent +Ġiniti ative +ol s +________________ ________________ +Ġsp ots +p ace +Ġconvent ional +Ġcorpor ations +Ġblock ed +H D +at tered +Ġref ers +Ġbu ck +ĠDig ital +12 0 +Ġtop ics +T F +Ä ģ +br id +re ement +Ġunder lying +ĠM ember +Ġinvestig ating +Ġpregn ancy +Ġtouch down +ĠB and +ĠCall er +Ġinst ances +P P +w a +G ood +Ġ199 1 +ĠC old +Ġfear s +Ġrem arks +Ĩ Ĵ +at al +Ġm it +Ġexper iments +i pt +Col or +ind u +Up date +Ġ9 3 +A g +Ġ å +anc ouver +B oth +Ġjud ges +Ob ject +Ġst ere +umb n +Ġparticip ation +ĠSt ars +ĠJ ere +Ġweek ly +ĠB an +Ġconvers ations +ĠP itt +u z +ĠIndian a +ĠK ick +Ġinf ection +Ġhero es +Ġsett led +Ġstri p +Ġh al +Ġd ump +ĠS ci +Ġl es +Ġref erences +ĠU RL +ĠBr idge +Ġwant ing +For ce +Ġex clus +Me anwhile +m n +Ġg entle +m aker +sen al +ĠG ro +ou ri +ĠR ain +ĠAll iance +Ġl ift +el a +S D +ĠCle veland +Ġrank ed +Ġst adium +Ġdead ly +ä ¸ +Ġr iding +ar ia +ĠAr mor +Ġdocument ation +ĠGree ce +ree k +Ġl ens +ĠS a +Ġg ross +ĠE mer +ag ers +ĠD ub +ĠR h +ĠAM D +Ġarri val +Ġdes ert +Ġsupp lement +ĠRes p +Ġkn ee +Ġmarg in +f ont +og g +201 0 +ĠP ir +ĠP rom +iv als +Ġint ake +Ġdifferent ly +ug s +Ġb its +clud ed +Ġsearch ing +ĠD u +um ble +Ġfunction al +ĠBalt imore +ĠC ould +Ġdes ired +Ġcirc uit +ĠL yn +ĠG O +ĠF alse +re pre +' : +alt ies +Ġmin im +Ġdro ve +ĠSh ould +Ġh ip +Ġpro s +Ġut ility +ĠN ature +ĠM ode +P resident +o pp +r at +form ance +Ġconcent ration +Ġf ont +ĠB ud +Ġam id +Ġre vers +ĠM L +B ar +Ġinter action +Ġjur isd +Ġspell s +d ep +f il +Ġcivil ians +ut ter +ĠCo oper +ĠBel ow +Ġent rance +Ġcon vert +Ġcontrovers y +ow ered +Ġcontr ary +Ġar c +ĠExec utive +ĠOffic er +Ġpack ages +Ġprog ressive +w idth +Ġreserv ed +v ol +ĠSam sung +Ġprint ed +Ġcent ers +Ġintrodu ce +ĠKenn edy +Ġodd s +Ġsure ly +Ġindepend ence +Ġpass engers +repre ne +ĠBe h +Ġl oves +ĠESP N +Ġfac ilit +Ġident ical +Ġdo ct +Ġpartners hip +con f +ĠH ide +Ġconf used +ĠC ow +M en +Ġw rest +ĠIraq i +Ġh oles +ĠStud ies +Ġpregn ant +h ard +Ġsign als +I X +Ġpull ing +Ġgrad uate +Ġnomine e +D ate +Ġper mitted +Ġâ Ĥ¬ +ĠOk lahoma +St art +Ġauthor ized +Ġal arm +ĠC os +v an +Ġgener ations +c ular +Ġdr agon +ĠSoft ware +ĠEd ward +Ġcontro ller +S en +ge red +ĠV ik +Ġappro ached +Th ank +Ġcan ce +Ġform ula +ĠSm all +Ġweak ness +Ġr amp +it udes +j ud +Ġbrill iant +Ġacc us +s ource +Ġ8 00 +ĠE vil +S w +Ġhom eless +we ek +i ens +r ics +ĠTh ird +T O +Ġorgan ic +Ġpresent ation +ag h +ĠDown load +v ation +Ġas sembly +or able +hold ers +ĠBern ie +ĠHel p +Ġt ong +ĠF ight +Ġbe ach +B ook +ĠL ic +Ġr ush +ĠR ound +ou p +ĠMar x +Ġcalcul ated +ĠDe vil +ĠSar ah +Ġoccasion ally +Ġbul let +Av ailable +g ate +Ġ9 1 +Ġh osp +Ġprom ises +ĠH IV +ĠSt adium +ĠSt ock +ĠCorpor ation +g age +N G +ĠC redit +Ġs ne +ib l +Ġacc um +s uch +Ġterror ists +Ġconscious ness +ĠZ h +Ġdram a +ool a +pir ation +Ġlab our +ĠN in +Ġut ter +Ġdemocr atic +Ġass ass +il ation +Ġg est +Ġab road +Ġmet ab +Ġs orts +Ġfl av +U B +Ġm g +ĠNot hing +ĠO d +Ġmus ical +200 9 +Ġdro ps +oc ated +ater al +0000 00 +Ġg re +Ġequ ality +Ġburd en +Ġv ig +ĠLe ader +-------- ---- +Ġcere mony +Ġf ighter +Ġact ors +Ġ æ +am an +F i +Ġal ign +put er +Ġe lder +ĠN SA +Ġrepresent ation +ĠOnt ario +IT H +usal em +Ġharass ment +itz er +Ġsy mp +Ġbox es +ĠD R +Ġman ifest +at re +Ġ ^ +Ġd ies +le ton +Ġmiss ions +et he +Ġres olve +Ġfollow ers +Ġas c +Ġk m +l ord +am med +Ġsil ent +ĠAssoci ated +Ġtim ing +Ġprison ers +ĠK ings +ĠF ive +Ġtow er +Ġappro aches +Ġprecise ly +Ġb ureau +ĠM other +ĠI ss +Ġkey board +it ual +Ġfund ed +Ġstay ing +Ġpsych ological +Ġm ile +ĠLe on +ĠBar b +w ill +Ġw ider +ĠAtl antic +Ġt ill +ĠR ome +ro t +Ġaccomp an +Ġfl our +ac o +W orld +ĠExp ress +ĠY u +C or +Ġple ased +part y +Ġpoint ing +Ġinf lation +Ġro y +Ġ ), +ain er +Ġwedd ing +orm on +Ġrequ iring +Ġqual ified +Ġse gment +EN D +Ġs izes +e als +Ġcor rupt +ass ador +Ġcele b +Ġdream s +ĠM ess +Ġcheck ing +ĠV ersion +Ġprep aring +Ġact ively +ĠD iff +Ġl ux +ĠW inter +act eria +ĠN E +Ġdep uty +Ġtrans gender +Ġsum mary +Ġin her +er ies +ch ar +ĠY an +Ġkn ock +ĠP ath +Ġl ip +roll er +Ġimp ression +Ġcelebr ate +Ġsl ide +Ġgu ests +Ġcl ip +F S +Ġsav ings +Ġcapt ain +Ġleg acy +ĠDen ver +Ġw ounded +tab oola +AC T +Ġpurs ue +Ġo xy +Ġ q +Ġsem i +ĠN eed +ĠAff airs +Ġob sc +Ġcheck ed +Ġd ual +C ode +ĠM D +le m +ult y +Ġ © +ĠEl izabeth +Ġcent uries +ard ed +s rc +Ġev ident +enn is +at in +Ġunemploy ment +ĠMar io +Ġint im +Ch rist +Ġbi ological +Ġsold ier +ĠAdd ed +Ġm ath +ĠG il +Ġbi as +Ġd ating +ĠO cean +Ġm ice +M us +h ire +ĠT es +Ser ver +lim ited +S ize +Ġmet ers +Ġrock et +es see +Ġcertific ate +ĠIran ian +AS S +Ġgr id +D ec +Ġro lling +com mun +ĠSwed en +b ury +Ġtiss ue +Ġrac ism +ĠL ocal +Ġmyster y +Ġexam ine +Ġst em +Ġs its +Ġhop ed +ot ing +Ġdial ogue +Ġpers u +W atch +l ay +M AN +Ġch ronic +ĠPort land +mark et +ĠS EC +Ġparalle l +Ġsc andal +Ġcar ries +Ġphenomen on +h uman +ack er +ĠO x +Ġretire ment +tain ment +ov ie +ĠG ear +Ġd uties +Ġdo se +Ġsc roll +M B +in f +Ġsa uce +Ġland scape +red dit +ĠChampions hip +ĠRed dit +al id +Ġco in +Ġover s +Ġpost ing +ab out +Ġf el +and y +Ġb old +Ġfocus ing +e ffect +G R +Ġde emed +Ġrecommend ations +Ġste pped +Ġvot er +ĠDe ep +ĠInst agram +Ġmoder ate +ĠMary land +Ġrestrict ed +ĠM B +ĠCh all +Ġto b +Ġc ir +ĠO cc +ĠE ver +Ġcoll aps +IN FO += - +ĠP ict +ĠAcc ount +n c +Ġo ught +Ġex port +Ġdr unk +( ' +Ġw ise +ĠM ort +ne cess +Ġan cest +ĠInc re +Ġfrequ ent +m ir +Ġinterpret ation +Ġdepend ent +Ġco ins +ĠB ol +V ideo +ĠJust in +Ġfat al +Ġcook ing +Ġconf usion +ip her +Ġcust ody +ĠMor gan +om ach +ĠGovern or +Ġrestaur ants +el ing +Ġacknowled ged +Ġthe r +Ġgen es +ch ing +He y +Ġtact ics +ĠMex ican +Ġv end +Ġhe s +qu er +Ġnot ing +ĠCamer on +Ġtarget ing +ro ck +Ġcred its +Ġemot ions +Ġrepresent atives +new s +Ġlegisl ative +Ġrem oving +Ġtweet ed +ĠCar ter +ĠF ixed +Ġfor cing +Ġspeak er +Ġm ales +ĠViet nam +l ined +Ġconcept s +Ġvo ices +o ir +ĠT rib +W he +ĠJer usalem +ĠS ant +Ġc ul +Ġl ady +ĠHaw ai +Ġar ts +ĠIn n +ĠMach ine +ĠEm peror +Ġsl ot +g ly +ĠPro cess +II I +Ġathlet es +ĠTem ple +ĠRep resent +Ġpres c +Ġt ons +Ġgold en +Ġp unch +ĠG R +iver pool +Ġen act +Ġlob by +Ġm os +Ġpick ing +Ġlif etime +Ġcogn itive +E ach +z o +Ġd ub +Ġcons ists +ol n +Ġf estival +am ous +Ġint ellig +w ords +ĠSm art +Ġde le +Ġl apt +Ġmag ical +ĠS in +b us +ur ities +igh th +ĠRub y +ĠS ure +ol ving +Ġj un +O ST +Ġimp osed +Ġast ron +Ġcor rel +ĠN S +ĠK it +ĠF uture +b urn +Ġimm une +oc us +Ġcour ses +ĠSt ring +Ġle an +Ġg host +Ġout comes +Ġexp ense +Ġevery day +Ġaccept able +A h +Ġequ ipped +Ġor ange +F R +ĠD utch +Th ough +ĠR ank +Q U +ĠRober ts +wh at +re nd +Ġdisapp ear +Ġsp awn +ĠL am +o is +Ġdes erve +Ġmin imal +Ġnerv ous +ĠW ould +Ġro ok +ĠV ancouver +Ġres ign +sh ire +ĠW orks +ĠB uild +Ġafford able +ĠG ary +ĠAren a +Ġh anging +Ġimpl ications +ĠS ong +Ġmain taining +Ġgu ards +C ON +Ġder ived +Ġexecut ed +Ġthe ories +Ġqu oted +ĠAnd re +og a +sel ess +in fo +ĠBel g +Ġt ears +ĠSur v +Ġbirth day +ig ious +im mer +Ġspect rum +Ġarchitect ure +Ġrec ruit +arm a +T able +Ġmon sters +ĠG ov +Ġdest ination +Ġattract ive +Ġf oss +ĠMore over +Ġpres ents +TH E +Ġrep ly +pt on +Ġc um +Ġdel ight +Ġaffect s +Ġdon ations +ĠT oy +ĠH im +M ENT +Ġover come +it ched +ĠFant asy +ĠH at +ĠBe ast +b ott +Ġinvestig ations +R un +Ġhun ting +d i +f und +Ġs essions +est yle +Ġport ray +oid s +Y eah +Ġcommun icate +Ġcom edy +ĠY ang +Ġbel t +ĠMar ine +Ġpredict ed +Pl ay +Ġimportant ly +Ġremark able +Ġelim inate +D avid +Ġb ind +V ID +Ġadvoc ates +ĠG aza +im p +D B +ĠN a +ĠSim ilar +I ES +Ġchar ity +v as +m ath +Ġâ ĸ +ok er +nd um +Ġcap s +ĠH al +2 000 +e an +Ġfle et +Ġrec re +R ight +Ġsleep ing +ij ing +k ind +Ġdesign ated +à ¤ +Ġanim ation +ke e +ĠInt rodu +Ġ/ > +Ġdelay ed +Ġtrem end +Ġcur ious +U se +Ġle ct +d am +Ġinnov ation +ĠPoint s +Ġload ing +Ġdisp ute +ct ic +ird s +ĠB Y +Ġn urs +ĠVal ue +ION S +ĠH um +Ġtem plate +m ers +Ġappear ances +ĠEnter tainment +Ġtransl ation +Ġsa ke +Ġbene ath +Ġin hib +Ġe uro +abet es +Ġstud ying +ĠM as +Ġper ceived +Ġexam ined +Ġe ager +Ġco aches +Ġim per +ch i +Ġprodu ces +" ). +ĠEvery one +Ġm unicip +Ġg irlfriend +Ġh ire +ĠV ice +Ġsu itable +op y +Ġin equ +ĠD uke +f ish +f irst +ĠO bs +Ġinter ior +ĠBru ce +ĠR y +Ġanal ys +Ġconsider able +Ġfore cast +Ġf ert +ors hip +ĠD rug +ĠA LL +: " +th ur +ĠM ail +Ġball ot +Ġinst antly +ĠCh annel +Ġp icks +Ġ198 9 +Ġt ent +ol i +Ġcivil ian +b ling +ell o +b u +Ġin ch +Ġlog o +Ġcooper ation +Ġwal ks +Ġinvest ments +Ġimp rison +ĠF estival +ĠK y +Ġleg ally +Ġg ri +ch arg +S l +Ġthreat ening +du ction +fl ow +Ġdismiss ed +ibr aries +c ap +e le +ĠMc G +ĠHar vard +ĠConserv ative +ĠC BS +p ng +Ġro ots +ĠH aving +umb led +ĠF un +\ / +ĠS earch +ple x +Ġdiscuss ing +Ġcontin u +ĠT ai +ĠW ik +F ree +f it +Ġref use +Ġmanag ing +Ġsy nd +ip edia +w alk +Ġprofession als +Ġguid ance +Ġunivers ities +Ġas semb +unt u +F inally +AS E +ĠAut o +ĠH ad +Ġann iversary +L D +ĠD ur +ĠUlt imate +ih ad +pro duct +Ġtrans it +Ġrest ore +Ġexpl aining +Ġass et +Ġtransfer red +Ġbur st +ap olis +ĠMag azine +ĠC ra +ĠB R +gg ed +ĠH E +M ich +b et +ĠL ady +yl um +erv es +Ġme ets +wh ite +L og +Ġcorrespond ing +Ġins isted +G G +Ġsurround ed +Ġt ens +Ġl ane +Ġco inc +h ome +Ġexist ed +ect ed +ĠDou ble +lam m +Ġske pt +ex p +Ġper ception +ie v +ĠBe ing +o ft +Ġadop t +. : +] ; +Wind ows +Ġsatell ite +AS H +Ġinf ant +d escription +ĠMe anwhile +c m +oc a +ĠT reat +act or +Ġtob acco +ĠN orm +em ption +Ġfl esh +Ġj e +o op +ĠHe aven +Ġbe ating +an im +Ġgather ing +Ġcult iv +G O +ab e +ĠJon athan +ĠSaf ety +Ġbad ly +pro t +Ġcho osing +Ġcontact ed +Ġqu it +Ġdist ur +Ġst ir +Ġto ken +D et +ĠP a +Ġfunction ality +00 3 +s ome +Ġlimit ations +Ġmet h +b uild +con fig +N T +re ll +ble m +ĠM om +Ġveter ans +ĠH u +Ġtrend s +are r +ĠG iven +ĠCa ption +m ay +AS T +Ġwond ering +ĠCl ark +n ormal +Ġsepar ated +Ġdes p +st ic +b rew +Ġrel ating +ĠN ik +ĠF arm +Ġenthus i +g ood +d eb +Ġactiv ist +Ġm art +Ġexplos ion +ĠEconom ic +L ink +Ġins ight +Ġconven ient +Ġcounter part +su pport +ĠV irt +ag en +ĠTenn essee +ĠSim on +ĠA ward +OC K +ĠF igure +Ġoverse as +Ġpr ide +ĠC as +n ote +m g +C urrent +Ġdispl ays +cont ent +Ġtravel ing +Ġhosp itals +ĠFin ancial +ĠP ast +Ġdefend ant +Ġstream ing +m ble +ĠBer lin +uk i +Ġdist ribut +Ġant ib +Ġch ocolate +ĠCast le +Ġinter rupt +ĠR ow +Ġconvers ion +Ġbug s +ĠR ather +li est +L Y +ĠJe an +com mon +ak h +Ġ1 30 +ot ton +ĠDe an +Ġam endment +Ġgame play +ĠWar ren +od a +Ġhigh lights +Ġir re +ĠNAT O +Ġball s +Ġdemand ing +U RE +ĠL uke +F igure +st op +on ia +z one +iz ers +ĠW R +Ġaward ed +Ġregul atory +ĠH art +ĠS N +pl ing +Ġs our +ĠP ixel +us ive +Ġf et +ĠS ent +Ġautom atic +Ġf er +vern ment +ĠKh an +T ON +f ather +Ġextraord inary +th rop +ĠP ython +ĠG PU +Ġsex ually +Ġdesk top +it ivity +ĠAnton io +Ġo rient +Ġe ars +ob by +ous es +vertis ements +Ġmanufacture rs +ic ient +min ute +Ġconv iction +Ġg arden +p ublic +Ġsatisf ied +f old +O K +Ġin hab +ĠTh ink +Ġprogram me +Ġst omach +Ġcoord in +Ġh oly +Ġth reshold +Ġr het +Ġser ial +Ġemploy ers +ĠEvery thing +ra h +Ġb other +Ġbr ands +Val ue +ĠT ed +ĠPlan et +Ġp ink +ĠFurther more +s a +P E +re ck +ĠUS D +ot te +Ġ& & +Ġland ed +g ets +Ġprodu cers +Ġhealth care +Ġdomin ant +Ġdest ro +Ġam ended +ch ron +Ġf its +ĠSy d +ĠAuthor ity +AT CH +Ġfight s +ĠL LC +Ġ-- - +ĠCor p +Ġtox ic +spe cific +ĠC orn +ĠChe l +Ġtele phone +ĠP ant +Ġmyster ious +aun ch +od ox +med ia +Ġwitness es +ag u +Ġquestion ed +ĠBre xit +ĠRem ember +ene z +Ġend orse +iat ric +ĠId ent +Ġridic ulous +1 10 +Ġpr ayer +Ġscient ist +Ġ19 50 +ĠA qu +Ġunder ground +ĠU FC +m are +ĠL ater +w ich +Ġsubsc rib +Ġhost s +Ġer r +Ġgr ants +ant om +Ġsum mon +ear ly +ĠC lear +ĠPr im +Ġsusp ension +Ġguarant eed +app er +Ġr ice +ĠSe an +ĠSh in +Ġrefere ndum +Ġfl ed +r ust +Ġ3 60 +ter y +Ġsh ocked +B R +ĠO il +ĠAll ah +Ġpart ly +Ġign or +Ġtrans mission +Ġhom osexual +ivers al +Ġhop efully +ãĤ ¤ +Ġless on +L eg +Ġ .. +Y et +t able +app ropri +re tt +Ġbo ards +Ġincor rect +Ġb acteria +ar u +am ac +Ġsn ap +.' " +Ġpar ad +t em +he art +Ġav ailability +Ġw isdom +Ġ( + +Ġpri est +ĠÂł ĠÂł +O pen +Ġsp an +Ġparam eter +Ġconv ince +Ġ( %) +r ac +Ġf o +Ġsafe ly +Ġconver ted +ĠOlymp ic +Ġres erve +Ġhe aling +ĠM ine +M ax +Ġin herent +ĠGra ham +Ġinteg rated +D em +Ġpip eline +Ġapp lying +Ġem bed +ĠCharl ie +Ġc ave +200 8 +Ġcons ensus +Ġre wards +P al +ĠHT ML +Ġpopular ity +look ing +ĠSw ord +ĠAr ts +' ) +Ġelect ron +clus ions +Ġinteg rity +Ġexclus ively +Ġgr ace +Ġtort ure +Ġburn ed +tw o +Ġ18 0 +P rodu +Ġent reprene +raph ics +Ġg ym +ric ane +ĠT am +Ġadministr ative +Ġmanufacture r +Ġ vel +ĠN i +Ġisol ated +ĠMedic ine +Ġback up +Ġpromot ing +Ġcommand er +Ġfle e +ĠRus sell +Ġforg otten +ĠMiss ouri +Ġres idence +m ons +Ġrese mb +Ġw and +Ġmeaning ful +P T +Ġb ol +Ġhe lic +Ġwealth y +Ġr ifle +str ong +row ing +pl an +as ury +â̦ . +Ġexpand ing +ĠHam ilton +Ġrece ives +S I +eat ures +ĠAn im +RE E +P ut +Ġbrief ly +ri ve +Ġstim ul +Ġ`` ( +Ġ __ +Ġch ip +Ġha z +Ġpri ze +ĠTh ings +AC E +ul in +d ict +ok u +Ġassoci ate +ock ets +y outube +St ory +ateg ory +Ġm ild +ail ing +ĠY e +O rig +ĠK a +or ig +Ġpropag anda +Ġan onymous +Ġstrugg led +Ġout rage +AT ED +ĠBe ijing +r ary +Ġle ather +Ġworld s +Ġbroad er +12 5 +id al +ĠBet ter +Ġt ear +E xt +Ġpropos als +Ġit er +ĠSqu ad +Ġvol unt +m i +D id +ĠP u +p in +Ġspeak ers +Ġb orders +Ġfig ured += ' +Ġsimultane ously +aed a +Ġcharg ing +Ġur ged +Ġcon j +25 6 +ĠG ordon +mer ce +Ġdocument ary +Sh are +it ol +ON E +ĠG arden +h att +ĠThom pson +ane ous +ap ore +Ġt anks +Ġless ons +tr ack +Ġout standing +Ġvolunte ers +Ġsp ray +Ġmanag ers +l arge +Ġcamp s +Ġart ificial +ĠR u +Ġb ags +th al +Ġcompat ible +ĠBl ade +Ġf ed +Ġarg ues +F I +Ġunf air +Ġcor n +Ġoff set +Ġdirect ions +Ġdisappoint ed +ĠCon vention +Ġview ing +M E +oc ity +Ġtown s +Ġlay ers +Ġro lled +Ġjump ed +Ġatt ribute +Ġun necess +inc oln +Ġsupp ose +ĠNet her +ch a +Ġbur ied +Ġsix th +B en +ress ing +OU R +Ġw ound +Ġcy cl +Ġmechan isms +Ġcongress ional +ĠE lement +Ġagre ements +Ġdec or +Ġclos est +ĠM it +Go ogle +} } +Ġm ixture +Ġflu id +S ign +ĠSch olar +Ġp ist +ask et +ab ling +Ġrac ing +he ro +ri el +ass y +Ġche aper +b en +Ġvert ical +amac are +ĠRead ing +g ments +Ġhelic op +Ġsacr ifice +ay a +p aren +V A +ĠL es +ĠStud io +Ġviol ations +ĠAn na +ac er +é ¾ +ĠR at +ĠBe ck +ĠD ick +ĠA CT +Ġcomp osition +Ġtext ure +ĠO wn +Ġsmart phone +ĠN A +Ġfor b +im port +Ġdef ending +il st +re r +Ġo h +ĠJere my +Ġbank ing +cept ions +Ġrespect ive +/ . +Ġdr inks +ĠW i +Ġb ands +ĠL iverpool +Ġg rip +ĠB uy +Ġopen ly +Ġreview ed +per t +Ġver ify +ĠCo le +ĠW ales +M O +Ġun pre +Ġshel ter +ĠIm perial +Ġgu i +ĠD ak +Ġsuggest ions +Ġexplicit ly +Ġsl ave +Ġblock chain +Ġcompet ing +Ġprom ising +S ON +Ġsoc cer +Ġconst itution +4 29 +Ġdist ract +ĠU ser +es ides +ĠMet hod +ĠTok yo +Ġaccompan ied +Cl ient +s ur +al og +Ġident ification +Ġinv asion +as ma +Ġindust ries +pp ers +Ġsub tle +ĠUn it +n atural +Ġsurv ived +Ġfl aw +ĺ ħ +ĠH oll +Ġdef icit +Ġtut orial +ĠCh ance +Ġarg uing +Ġcontem porary +Ġinteg ration +for ward +Ġt um +it is +Ġh iding +ĠD omin +ĠT an +ĠB uilding +ĠV in +Ġspokes person +ĠNot es +Ġemer ging +Ġprepar ation +Ġpro st +Ġsuspect s +Ġaut onom +D escription +Ġdeal t +ĠP ear +Ġstead y +Ġdecre ased +Ġso vere +ĠCl in +Ġgrad ually +ors es +ĠW AR +S erv +ãĤ ¢ +h r +Ġd irty +ĠB arn +ĠB C +Ġd il +Ġcal endar +Ġcompl iance +Ġch amber +b b +Ġpass enger +ate ful +ĠT itle +ĠSyd ney +ĠG ot +Ġdark ness +Ġdef ect +Ġpack ed +ass ion +Ġgod s +Ġh arsh +IC K +le ans +Ġalgorith m +Ġoxy gen +Ġvis its +Ġbl ade +Ġkil omet +ĠKent ucky +Ġkill er +P ack +enn y +Ġdiv ine +Ġnom ination +be ing +Ġeng ines +Ġc ats +Ġbuff er +ĠPh ill +Ġtra ff +AG E +Ġtong ue +Ġrad iation +ere r +m em +ĠExpl icit +é¾ į +Ġcou ples +Ġphys ics +ĠMc K +Ġpolit ically +aw ks +ĠBl oom +Ġwor ship +e ger +ut er +ĠF O +Ġmat hemat +Ġsent enced +Ġdis k +ĠM arg +Ġ/ * +P I +Ġoption al +Ġbab ies +Ġse eds +ĠScott ish +Ġth y +] ] +ĠHit ler +P H +ng th +Ġrec overed +ing e +Ġpow der +Ġl ips +Ġdesign er +Ġdis orders +Ġcour age +Ġch aos +" },{" +Ġcar rier +b ably +H igh +ĠR T +es ity +l en +Ġrout es +u ating +F il +N OT +w all +s burgh +Ġeng aging +ĠJava Script +ore r +li hood +Ġun ions +ĠF ederation +ĠTes la +Ġcomple tion +ĠT a +Ġprivile ge +ĠOr ange +Ġne ur +paren cy +Ġb ones +Ġtit led +Ġprosecut ors +ĠM E +Ġengine er +ĠUn iverse +ĠH ig +n ie +o ard +Ġheart s +ĠG re +uss ion +Ġmin istry +Ġpen et +ĠN ut +ĠO w +ĠX P +in stein +Ġbul k +S ystem +ic ism +ĠMarket able +Ġpre val +Ġpost er +Ġatt ending +ur able +Ġlicens ed +ĠG h +et ry +ĠTrad able +Ġbl ast +à ¤ +ĠTit an +ell ed +d ie +H ave +ĠFl ame +Ġprof ound +Ġparticip ating +Ġan ime +ĠE ss +Ġspec ify +Ġregard ed +ĠSpe ll +Ġs ons +own ed +Ġm erc +Ġexper imental +land o +h s +ĠDun geon +in os +Ġcomp ly +ĠSystem s +ar th +Ġse ized +l ocal +ĠGirl s +ud o +on ed +ĠF le +Ġconstruct ed +Ġhost ed +Ġsc ared +act ic +ĠIs lands +ĠM ORE +Ġbl ess +Ġblock ing +Ġch ips +Ġev ac +P s +Ġcorpor ation +Ġo x +Ġlight ing +Ġneighb ors +ĠU b +ar o +Ġbe ef +ĠU ber +F acebook +ar med +it ate +ĠR ating +ĠQu ick +Ġoccup ied +Ġaim s +ĠAdd itionally +ĠInt erest +Ġdram atically +Ġhe al +Ġpain ting +Ġengine ers +M M +ĠM ust +Ġquant ity +P aul +Ġearn ings +ĠPost s +st ra +ãĥ¼ ãĥ +Ġst ance +Ġdro pping +sc ript +Ġd ressed +M ake +Ġjust ify +ĠL td +Ġprompt ed +Ġscr ut +Ġspeed s +ĠGi ants +om er +ĠEd itor +Ġdescrib ing +ĠL ie +ment ed +Ġnow here +oc aly +Ġinst ruction +fort able +Ġent ities +Ġc m +ĠN atural +Ġinqu iry +Ġpress ed +iz ont +for ced +Ġra ises +ĠNet flix +ĠS ide +Ġout er +Ġamong st +im s +ows ki +Ġclim b +ne ver +Ġcomb ine +d ing +Ġcomp r +Ġsignific ance +Ġremem bered +ĠNev ada +ĠT el +ĠSc ar +ĠWar riors +ĠJ ane +Ġcou p +b as +Ġtermin al +, - +O H +Ġt ension +Ġw ings +ĠMy ster +�� �� +ĠUn like +val id +viron ments +ĠAl i +Ġn aked +book s +ĠM un +ĠG ulf +Ġd ensity +Ġdim in +Ġdesper ate +Ġpres idency +Ġ198 6 +h y +IN D +Ġun lock +im ens +Ġhand led +ĠE b +Ġdisapp eared +Ġgen re +Ġ198 8 +Ġdetermin ation +St ream +ik o +ap ters +Ġacknow ledge +J an +Ġcapital ism +P at +Ġ20 20 +Ġpain ful +Ġcur ve +Ġbom bs +st orm +ĠMet al +en cer +ĠF ig +ĠA aron +anc hes +Ġins piration +Ġexha ust +t ains +ash i +Ġdesc ript +Ġr itual +ĠChel sea +Ġpromot ion +ĠH ung +ĠW ard +iv a +ĠE T +Ġto ss +all ow +ĠFranc is +D ep +Ġhapp iness +ĠGl ass +Ġbet a +Ġstreng then +N E +o a +Ġbutt ons +ĠMur ray +Ġkick ed +Qu est +ĠT alk +ĠS everal +ĠZ ero +Ġdr one +ul k +Ġc am +ĠM obile +Ġprevent ing +Ġret ro +ĠA x +Ġcru el +Ġflo at +. ), +Ġfil ing +ĠGr ant +ĠB or +Ġr ib +Ġchampions hip +ĠM erc +Ġsty les +Ġc ake +Ġbuild s +ĠS elf +io x +Ġep ic +oy d +B el +ĠSt ew +. ( +ah u +ĠBe yond +Ġout s +Ġsol o +ĠT ree +Ġpres erve +Ġt ub +AR E +ro c +ĠIm pro +ĠW right +Ġbu nd +Ġtr aged +Ġoccas ional +b ian +Sec ond +r ons +Ġinter actions +form ed +s ing +Ġown s +Ġh ockey +Gener al +Ġlog ical +Ġexp end +Ġesc al +ĠGr iff +ĠC rown +ĠRes erve +Ġsto pping +Ġexc use +sec ond +Ġoper ated +Ġre aches +ĠMal ays +Ġpoll ution +ĠBrook lyn +Ġde lete +Ġhas h +Bl ock +ah a +âĢ ³ +Ġsh orter +p iece +> </ +Ġh orm +ĠW at +ĠBre ak +Ġprohib ited +Ġint ensity +ĠAl an +Ġli ability +? ! +and ed +Ġneigh bour +ĠCol lection +Ġf ires +Ġrevolution ary +f ly +ĠOr leans +Wh ite +ĠW rit +ĠD awn +Ġsett le +Ġexec ute +B M +Ġspokes woman +Ġlif estyle +Ġclick ing +ĠK ill +ĠLiber al +ĠN azi +Ġtra iler +Ġmount ains +Ġdam n +z es +p es +Ġpress ing +Ġb ail +ĠOrgan ization +Ġp ir +Ġth irty +Ġelect rical +Ġ1 15 +ĠP oly +ĠR ap +ĠSt rike +ĠC ann +Ġdemand ed +Ġback ing +def ault +spe ed +ĠLeg isl +Ġmother s +ĠB ody +Ġvar iation +ced ented +p owered +le ading +N ever +Ġg rave +ĠAnt i +A W +Ġinterview ed +ĠG ab +ĠF at +Ġrook ie +u u +Ġdep os +ix on +Ġam pl +ret ion +ĠHe at +Ġpeace ful +S M +ie ve +Ġd iver +ĠVict oria +Ġm ic +p df +Ġst ating +Ġl ung +Ġcritic ized +Ġvacc ine +ĠLoad ing +ur se +T ake +ĠFr an +ĠS old +ĠRob in +Ġdetect ed +ĠSc ript +Ġadjust ed +Ġsen ator +Ġopp osing +Er ror +C ount +Ġconflic ts +Ġo w +ĠAr gent +Ġmatch ing +h h +ĠTre k +st arter +" ), +ĠA F +od er +xx xx +ĠAl t +ac re +ĠP ick +ĠSol ar +ĠD al +O ct +ĠB att +Ġs rc +Ġeng agement +Ġexecut ives +Ġliber ty +j ava +Ġtal ented +igen ous +Ġcon secut +.. ... +In fo +Ġhor rible +Ġsurprising ly +f eed +ic ating +ĠL ED +Ġfem ales +St ation +ell er +ĠOak land +Ġmechan ical +i ology +ĠV ar +Ġrob ust +ett ings +ott a +Ġthe oret +Ġret ain +k ward +Ġd a +Ġdeploy ed +d el +ĠAnd y +Ġsubsc ribe +we b +Ġn a +ĠMic hel +Ġpart ially +ĠCome y +Ġc rown +ĠM aj +ĠBl u +r ator +D ay +IN T +Ġdocument ed +ĠG DP +g i +che ll +Ġbrut al +ĠB ab +st ration +Ġthe ft +Ġt ube +@ @ +Ġqu ery +ĠL incoln +Ġpublish ing +Ġw ore +or ical +Ġr ic +Ġnot able +Ġsubsequ ently +ne x +Ġobser ve +ĠB oe +Ġc odes +m ain +W H +ĠS L +Ġresident ial +av an +Ġm as +are st +ade on +OU T +Ġsoph istic +ant e +Ġc ens +Ġ ** +Ġmort ality +Ġyour s +Ġoccas ions +Ġrec alled +ĠDri ver +Ġv ocal +Ġbath room +Ġsh ops +Ġcollabor ation +ĠOb amacare +ĠC ell +Ch ar +Su per +C re +Ġt ends +Ġt orn +Ġeconom ics +a very +ĠR aid +ĠS em +Ġshould ers +Ġexpect ing +Ġexam ination +en ame +ĠU I +i ability +ol as +ĠAm b +ĠD ra +Ġmid field +ĠI C +Ġlay out +Ġflo ating +f i +it ative +Ġtremend ous +Ġ Ð +Ġab und +W ork +ĠLight ning +Ġsimilar ly +Ġconserv atives +Ġpr ay +B E +iz arre +Ġt empt +Ġemphas is +ĠMet ro +Ġf ishing +Ġmar ry +ne g +ĠStud y +Ġrec k +Ġdis pos +on ing +bs ite +Ġsusp ic +Ġmer ch +ĠG ib +ĠDes cription +ĠD VD +w he +ĠY emen +Ġen vironments +oot ing +ĠMod ern +e u +Ġreflect s +Ġh oney +Ġanaly st +Ġg ut +d ec +A ction +Ġhousehold s +Ġst er +Ġtem ple +Ġreform s +Ġfavour ite +Ġdead line +ĠL E +Th ree +ĠWith in +A ug +Ġnight s +elt a +Ġinv alid +ĠEx change +ĠDel hi +w hen +inc ome +Ġ ðŁ +Ġwire less +sc ribe +ist a +Ġhost ile +Ġall y +Ġg ig +Ġout lets +ĠD or +EM ENT +Ġas h +Ġab stract +OR D +ĠMot or +Ġadv iser +ist le +Ġb ases +Ġcourt esy +Ġcross ing +Ġcle ared +Ġrefuge e +cos ystem +Ġthrow s +f un +bour ne +d ays +Ġdisag ree +ĠN ative +Ġreflect ed +ĠF ast +ĠY ellow +ĠSing apore +ĠR aven +Ġembr ace +ĠK u +ĠC hen +ĠEar ly +Ġappoint ment +ĠMin i +it ement +Ġpl acing +Ġb icy +S R +Ġwh is +S U +Ġinvestig ated +Ġphotograph s +g ithub +ĠBe at +ĠR ing +ig hed +i ar +Ġev olved +eral d +Ġd un +Ġh ub +I AL +Ġencour aging +ĠPr int +ĠD ays +Ġpro secution +Ġp ants +az y +l ive +Ġfoss il +ĠJ u +Ġro cks +ud ge +ĠR ace +Ġg reet +b ie +Ġf illing +ĠL en +Ġdi abetes +Ġfire arms +um ing +enez uel +ĠB B +Ġaccept ing +AT H +Ġres ort +Ġh unt +ri k +uck er +am ents +Ġsust ained +Ġcross ed +Ġbreak fast +Ġatt ributes +lect ed +at ile +Ġv ibr +ĠK al +ars on +op les +Ġtou ched +Ġdam ages +Ġimp ressed +ru p +Ġan ch +ĠAd ams +H el +ĠVict or +Ġmount ed +ĠC C +Ġdelic ious +sp an +ell a +Ġel abor +am ples +Ġdef ic +Ġconstit u +u ates +ĠM ission +ĠT her +ĠMon ster +b es +Re uters +ĠInd ones +h ill +mun ition +Ġconfirm ation +ĠCons ider +ac ent +Ġj et +ĠEm ploy +ĠGT X +n an +ĠSp ider +Ġprocess or +Ġpat ri +ĠPent agon +ĠRob inson +Ġreal istic +à ± +Ġappear ing +Ġp ipe +om ed +Ġf ru +Ġaw ful +Ġeval uation +Ġintellig ent +ĠC itiz +Ġfund ra +od ium +Ġtwe ets +Ġwor n +pr ing +Ġkid n +Ġreb els +ĠK am +ĠNether lands +ĠS W +Ġacqu isition +ĠM ale +ãĥ ª +omb ies +Ġtrad em +ĠStat us +B re +ĠTH IS +Ġad verse +ĠN EW +s ign +Ġorgan isation +en c +ĠHar per +ap or +ĠMem bers +ĠPe ace +ĠAir port +ĠOther s +Ġscr atch +ĠP il +Ġsens or +Ġadop tion +ĠHot el +ĠDr ag +Ġhonest ly +Ġy ard +ĠFor ces +Ġpat ent +Ġb ass +Ġquiet ly +Ġbreat hing +Ġp ose +i ors +ĠJ ess +st atic +IT E +O ffic +Ġj ew +w cs +Ġ14 0 +Ġpre view +ipp i +Ġunf ortunately +oke mon +Ġh orn +Ġre ass +Ġpe er +ock er +Ġunt o +ĠGr ay +Ġclean ing +Ġattract ed +200 7 +P oint +k ill +ĠAg reement +ur ches +Ġhor r +ĠMiss iss +Ġworth y +Ġfl owers +t own +d ll +Ġre actions +Ġde ce +Ġindic ating +M D +Ġpre ference +ĠM VP +ess ional +ĠT arget +g ence +ĠInd ians +Ġm isc +Ġfree ly +Ġmus cles +Ġline up +Ġimpact s +ous ing +om i +ac ular +Ġcontro lling +ag ine +c ery +he ll +Ġrank ing +ĠN ich +ĠA ve +12 8 +Ġhigh way +Ġinc ons +Ġb inding +Ġstrugg les +ĠPitt sburgh +Ġgr ay +r in +Ġcom ics +ĠS port +Ġrel atives +Ġfr ight +Ġpro be +ĠPort ug +Ġv oc +Ġt u +ĠCor ps +Ġposs ibilities +Ġqual ify +wcs store +Ġl ibraries +Ġm igrants +Ġent ries +Ġconsecut ive +v als +ĠChair man +Ġh ill +IM E +ĠG ard +Ġinequ ality +f ox +ĠS ave +Ġc ort +claim ed +Ġtra its +Ġp our +Ġmiss iles +Ġess ence +Ġs ends +Ġall iance +Ġw ishes +ĠChrist opher +B ig +N Y +ĠJac ob +s an +ur red +ĠS O +ll y +Ġadvoc ate +ĠB ond +Ġ" / +Us ing +Ġdistrict s +ĠG ate +ĠB ir +r idge +ĠN az +ĠR s +bo ards +ĠG a +ĠRe agan +Ġinflu enced +1 000 +ap y +Ġchalleng ed +Ġb arg +Ġfac ulty +ĠF if +Ġacqu ire +A c +Ġin sect +Ġinstr uments +Ġle af +th odox +M essage +Ġt ale +Ġthere by +Ġtra p +Ġstrong est +ĠMil itary +is ible +Ġ198 4 +ethe less +Ġflex ible +Ġkill s +Ġfin ishing +ĠS ize +Ġredu ces +Ġep id +Ġorient ation +f ull +Ġtr ace +Ġl aser +Ġopp ose +Ġed iting +Ġmoment um +ä º +sh ow +V I +ĠL ad +Ġ198 5 +Ġmurd ered +9 00 +ut her +Ġprob ability +ĠP oll +Ġrel uct +ĠChe m +ĠMont real +Ġadequ ate +ĠPol and +ĠSher iff +um ph +Ġo k +Ġ 000 +Ġ" [ +Ġoper ators +ĠF er +Ġmod es +ĠE ve +Ġdiscipl ine +N ET +H and +Ġor al +ĠW E +em ail +J P +ĠPalestin ians +Ġhe nce +ĠL ess +Ġover l +d ig +Ġintim id +ĠCo al +Ġr anging +th a +Ġdist ant +Ġf ib +ĠInd ex +ĠW onder +ĠP el +hatt an +ĠH ug +Ã Ĺ +ra it +Ġwra pped +ĠR PG +Ġchemical s +ĠM oney +Ġfro zen +Ġind irect +ĠAgain st +E nd +Ġuncom fortable +ĠGall ery +ĠPost ed +Ø § +ond uct +Ġconsequ ence +Ġbit ter +Ġ198 7 +p op +Ġcount less +ĠAl aska +ff ff +Ġdepart ure +Ġref und +ĠI an +i ated +Ġsee ks +Ġmechan ics +Ġjurisd iction +lyn n +Ġal ike +ĠH unt +ath on +Ġres olved +Ġc ache +Ġdist inction +d irect +Ġenc ount +ou b +be at +ĠCount ry +se arch +Ġcontin uous +Ġmod est +ĠR ail +th ood +1 30 +B UG +Ġcrim inals +Ġindic ation +Ġencount ered +l ast +ĠW y +Ġide ology +ĠP DF +sec urity +] ) +ĠJim my +ĠE N +Ġh iring +T em +Ġp ig +aun t +ĠCry stal +Ġpen alties +Ġcap ability +Ġp y +Ġproduct ive +Ġbal anced +ĠGe Force +cl ick +olit an +od s +Ġafter wards +Ġplay offs +ĠG ill +U ser +Ġback s +p ub +t ag +Ġabs urd +p iring +Ġc iting +Ġtr illion +Ġoblig ation +Ġmax im +ah oo +c f +um i +ĠAl pha +ĠN elson +Ġpursu ant +in itely +Ġf ract +ent ry +ber y +ĠTh or +Add ed +ĠD J +ĠG ene +Ġaw kward +St ud +Ġwal let +ĠDiv ine +ari os +Ġrele asing +Ġed ited +Ġaccompl ished +B est +Ġed ges +Ġplan es +Ġfeed ing +" }," +Ġdiscl osure +Ġgr ain +air y +o ons +ern and +V R +Ġreason ably +Ġdr um +Ġpart ial +Ġgraph ic +Ġunpre cedented +Ġadv ised +M icro +ĠAss ad +point s +sc ar +ĠZ one +tt es +Ġ7 00 +v o +ĠH amp +Ġfix es +Ġca ution +Ġstr ings +Ġpan els +Ġle ak +Ġpr icing +row th +ĠEr ror +ĠS aints +f ix +Ġobserv ations +ĠA bs +Ġsuggest ion +ĠUkrain ian +Ġbar rier +Ġpain ted +B et +im ir +ĠS pect +p ot +orne ys +Ġcomp ound +Ġbe ars +ĠR ush +Ġlux ury +S um +Ġor bit +ĠMar c +Ġex empt +ĠTra il +ĠM O +ĠH ans +ĠWe apon +oc used +umin um +ĠJer ry +Ġb ust +ĠA G +ĠW iki +Ġend less +ĠV lad +ĠB ah +ĠR adeon +ke ys +ĠSur vey +ĠV iol +def ine +le an +Ġcomm od +Ġreven ues +Å į +Ġfurn iture +Ġcast ing +Ġdiplom atic +ĠPlay ers +ĠK illed +Ġmod ify +Ġinnov ative +ĠAb u +n or +Ġbond s +Ġcoach ing +M er +Ġmod ules +ĠPatri ots +Ġenh anced +Ġproceed ings +Ġteam mates +Ġ12 8 +ard o +Ġcomprom ise +ĠM uch +Ġfle w +ĠEd ge +Ġunnecess ary +Ġdoct rine +re port +ĠOr lando +ĠProf ile +Ġplay off +friend ly +Ġcompl ain +ĠM C +ĠO pt +ĠG B +Ġbeat en +Ġg olf +Ġpl acement +B it +Ġnews letter +Ġ201 9 +vis or +raw l +ĠiP ad +Ġact ed +Ġju ice +Ġdec ks +P N +su ccess +ĠH alf +Ġdele ted +Ġsec rets +Ġas ylum +M art +ĠAct iv +ĠGu y +ĠT s +Ġd ys +Ġassum ing +Ġman a +Ġsub ur +Ġ12 5 +M edia +AR Y +r ide +c p +Ġdifficult ies +Ġcollect ing +Ġbank rupt +n on +Ġcomp osed +Ġvol t +Ġmilit ants +Ġ> >> +ĠM ormon +t or +Ġpartic les +ĠB art +ry ption +Ġad min +Ġsqu ee +VID IA +Ġcreat or +iam eter +ic ular +N BC +Ġgrab bed +Ġn odd +Ġr ated +Ġrot ation +Ġgr asp +Ġexcess ive +ĠE C +ĠWh it +Ġinvent ory +ault s +ĠF B +Ġe cosystem +Ġbill ions +Ġvent ure +n amed +Ġdef ender +out e +Inst ead +ir able +W ar +Ġassum ption +Ġb ite +Ġearth qu +t ail +sp ace +Ġgif ts +boy s +Ġinev itable +Ġstruct ural +Ġbenef icial +Ġcompe lling +h ole +erv ation +Ġco at +o j +inc arn +ĠY ears +Ġdetermin ing +Ġrhet oric +Ġbound aries +Ġwh ites +A nt +add y +) - +ra ham +eter min +Ġhar vest +ĠCon c +Ġlapt op +ĠM atch +Ġenjoy ing +cc a +oll ar +Ġtri ps +Ġadd iction +ĠS ak +Ġpow ered +Ġc ous +ĠRuss ians +ie re +Ġret rie +qu ality +Ġdiff er +Ġking dom +ĠL aur +ĠCap itol +Ġcon clusions +ĠAl tern +ĠN av +Ġtrans parent +B ER +G roup +ĠCom plete +Ġinf er +Ġint rig +Ġins ane +R O +oph ob +is en +qu al +Mich ael +Ġm useum +ĠP ope +Ġres et +r ative +f ive +Ġagg reg +itte es +osit ory +Ġcar b +ĠRec ord +Ġdec ides +ĠF ix +Ġexcept ions +ĠCommission er +un s +ĠEnvironment al +Ġlegend ary +ist ence +Ġtun nel +k m +Ġins ult +Ġt roll +Ġsh ake +Ġdet ention +qu es +ĠCh rome +ĠF iles +Ġsub t +Ġprospect s +Ġpro l +re nder +pro of +Ġperform ances +St r +Ġh ref +ern ame +Ġachieve ment +Ġf ut +F ull +ĠLe ban +go ogle +ãĥ Ī +amp a +May be +Ġproject ed +ĠE mb +Ġcol leg +Ġa wards +Ġâ Ķ +G old +ĠBl ake +ĠR aj +if ting +Ġp ending +Ġinst inct +Ġdevelop ments +Con nect +ĠM and +ĠW ITH +ĠPhilipp ines +prof ile +Ġalt ogether +ĠB und +ĠT D +oo oo +amp ed +ip h +Ġste am +Ġold est +Ġdet ection +ul pt +Ġ ç +ĠWay ne +200 6 +f a +Ġcir cles +ĠF u +Ġdon ors +appropri ate +ĠDak ota +j amin +Ġmotiv ated +Ġpurch ases +ĠLouis iana +ĠS pl +Ġgl obe +Ġ10 5 +z ip +c all +Ġdepart ments +Ġsustain able +10 5 +ĠO P +if iers +Ġprevent ed +Ġinc omp +ĠComm ander +Ġdom inated +Ġ » +Ġinvest ed +Ġcomplex ity +Ġin cl +Ġens uring +Ġreal m +yn c +ĠInd ependent +r ained +ĠJ en +ĠFl ight +Ġat he +Ġspec ulation +ĠT E +oc ate +t ic +Ġpl aint +her ry +Ġto y +Ġ1 11 +Ġpl ates +st atus +ĠIs a +Ġdev oted +C op +ĠE S +25 5 +ur rency +M ain +Ġsl aves +Ġpe pper +Ġqu otes +Ġce iling +ĠF ish +Ġtrans formation +Ġfra ction +Ġadvant ages +Ġto ile +Ġstun ning +Ġmo ist +bre aking +s i +ĠL ocation +ĠMed ium +Ġtext s +Ġu gly +Ġb io +. âĢĶ +ĠB ased +Ġtr ains +ĠW ing +ĠAn cient +ĠRec ords +ĠH ope +Spe cial +ades h +ob i +[ / +Ġtempor arily +V er +h u +os er +Ġover night +Ġm amm +ĠTre asury +ĠV enezuel +ĠMeg a +Ġt ar +Ġexpect s +bl ack +or ph +\\ \\ +Ġaccept ance +Ġrad ar +s is +Ġjun ior +Ġfram es +Ġobserv ation +ac ies +P ower +ĠAdv anced +M ag +olog ically +ĠMe chan +Ġsent ences +Ġanaly sts +augh ters +force ment +Ġv ague +Ġcl ause +Ġdirect ors +Ġeval uate +Ġcabin et +M att +ĠClass ic +A ng +Ġcl er +ĠB uck +Ġresear cher +Ġ16 0 +Ġpoor ly +Ġexperien cing +ĠP ed +ĠMan hattan +Ġfre ed +Ġthem es +ad vant +Ġn in +Ġpra ise +10 4 +ĠLib ya +b est +Ġtrust ed +Ġce ase +Ġd ign +D irect +Ġbomb ing +Ġm igration +ĠSci ences +Ġmunicip al +ĠA verage +Ġgl ory +Ġreve aling +Ġare na +Ġuncertain ty +Ġbattle field +ia o +G od +Ġc inem +ra pe +el le +ap ons +Ġlist ing +Ġwa ited +Ġsp otted +ke ley +ĠAud io +e or +ard ing +idd ing +ig ma +ĠN eg +Ġl one +Ġ ---- +ex e +d eg +Ġtrans f +Ġwas h +Ġsl avery +Ġexpl oring +ĠW W +ats on +Ġen cl +l ies +ĠC reek +Ġwood en +Man ager +ĠBr and +um my +ĠAr thur +Ġbureau cr +Ġbl end +ar ians +F urther +Ġsupposed ly +Ġwind s +Ġ19 79 +Ġgrav ity +Ġanalys es +ĠTra vel +ĠV eter +Ġd umb +Ġaltern ate +g al +Ġconsum ed +Ġeffect iveness +.' ' +Ġpath s +ond a +L A +ĠStr ong +Ġen ables +Ġesc aped +Ġ" " +Ġ1 12 +Ġ198 3 +Ġsm iled +Ġtend ency +F ire +Ġp ars +ĠR oc +Ġl ake +Ġf itness +ĠA th +ĠH orn +Ġh ier +Ġimp ose +m other +Ġp ension +ic ut +bor ne +ic iary +. _ +ĠS U +Ġpol ar +is y +eng u +itial ized +AT A +w rite +Ġexerc ises +ĠD iamond +ot ypes +Ġharm ful +on z +Ġprint ing +st ory +Ġexpert ise +ĠG er +Ġtraged y +ĠF ly +Ġd ivid +amp ire +st ock +M em +Ġre ign +Ġun ve +Ġam end +ĠProp het +Ġmut ual +ĠF ac +Ġrepl acing +H ar +ĠCirc uit +Ġthro at +ĠSh ot +Ġbatter ies +Ġto ll +Ġaddress ing +ĠMedic aid +Ġp upp +ĠN ar +ol k +Ġequ ity +M R +ĠHis pan +ĠL arge +m id +D ev +Ġexp ed +Ġdem o +ĠMarsh all +erg us +Ġf iber +Ġdiv orce +ĠCre ate +Ġsl ower +ĠPark er +ĠStud ent +ĠTr aining +Ret urn +ĠT ru +Ġc ub +ĠRe ached +Ġpan ic +Ġqu arters +Ġre ct +Ġtreat ing +Ġr ats +ĠChristian ity +ol er +Ġsac red +Ġdecl are +ul ative +et ing +Ġdeliver ing +est one +Ġt el +ĠL arry +Ġmet a +ac cept +art z +ĠRog er +hand ed +Ġhead er +Ġtra pped +ĠCent ury +Ġkn ocked +ĠOx ford +Ġsurviv ors +b ot +Ġdemon stration +Ġd irt +Ġass ists +OM E +ĠD raft +ortun ate +fol io +pe red +ust ers +g t +ĠL ock +Ġjud icial +ver ted +Ġsec ured +out ing +ĠBook s +Ġhost ing +Ġlif ted +l ength +Ġj er +Ġwhe els +ĠR ange +umbn ails +Ġdiagn osis +te ch +ĠStew art +ĠP ract +Ġnation wide +Ġde ar +Ġoblig ations +Ġgrow s +Ġmand atory +Ġsusp icious +! ' +A pr +G reat +Ġmort gage +Ġprosecut or +Ġeditor ial +ĠK r +Ġprocess ed +ung le +Ġflex ibility +Ear lier +ĠC art +ĠS ug +Ġfoc uses +Ġstart up +Ġbre ach +ĠT ob +cy cle +ãĢ Į +ro se +Ġb izarre +ãĢ į +Ġveget ables +$ $ +Ġret reat +osh i +ĠSh op +ĠG round +ĠSt op +ĠHawai i +ĠA y +Per haps +ĠBe aut +uff er +enn a +Ġproduct ivity +F ixed +cont rol +Ġabs ent +ĠCamp aign +G reen +Ġident ifying +Ġreg ret +Ġpromot ed +ĠSe ven +Ġer u +ne ath +aug hed +ĠP in +ĠL iving +C ost +om atic +me ga +ĠN ig +oc y +Ġin box +Ġem pire +Ġhor izont +Ġbr anches +Ġmet aph +Act ive +ed i +ĠFil m +ĠS omething +Ġmod s +inc ial +ĠOrig inal +G en +Ġspir its +Ġear ning +H ist +Ġr iders +Ġsacr ific +M T +ĠV A +ĠS alt +Ġoccup ation +ĠM i +Ġdis g +lic t +Ġn it +Ġn odes +e em +ĠP ier +Ġhat red +ps y +ãĥ ī +Ġthe ater +Ġsophistic ated +Ġdef ended +Ġbes ides +Ġthorough ly +ĠMedic are +Ġbl amed +arent ly +Ġcry ing +F OR +pri v +Ġsing ing +ĠI l +Ġc ute +o ided +olit ical +ĠNe uro +å ¤ +Ġdon ation +ĠEag les +ĠG ive +T om +Ġsubstant ially +ĠLic ense +ĠJ a +Ġg rey +ĠAn imal +ĠE R +ĠU nd +Ġke en +Ġconclud e +ĠMississ ippi +Eng ine +ĠStud ios +P ress +o vers +ll ers +Ġ3 50 +ĠR angers +Ġr ou +ert o +E p +iss a +iv an +Ġse al +ĠReg ist +dis play +Ġwe aken +u um +ĠComm ons +ĠS ay +Ġcult ures +Ġl aughed +Ġsl ip +Ġtreat ments +iz able +m art +ĠR ice +Ġbe ast +Ġob esity +ĠLa ure +ig a +Wh ich +hold er +Ġelder ly +Ġp ays +Ġcompl ained +Ġc rop +Ġpro c +Ġexplos ive +ĠF an +ĠAr senal +A uthor +ef ul +Ġme als +Ġ( - +id ays +Ġimag ination +Ġann ually +Ġm s +as ures +H ead +ik h +m atic +Ġboy friend +ĠCom puter +Ġb ump +Ġsur ge +ĠCra ig +ĠKir k +D el +medi ate +Ġscen arios +ĠM ut +ĠSt ream +Ġcompet itors +Ù Ħ +ĠStan ford +ĠRes ources +az ed +b age +Ġorgan is +ĠRe lease +Ġsepar ately +Ġha bits +Ġmeasure ments +ĠCl ose +Ġaccomp any +Ġg ly +Ġt ang +ĠR ou +Ġplug in +Ġcon vey +ĠChall enge +oot s +j an +Ġcur s +ĠRel ations +ke eper +Ġapproach ing +p ing +Spe aking +Ġarrang ement +ĠV I +are ttes +Ġaffect ing +Ġperm its +b ecause +Ġu seless +ĠH us +!! !! +Ġdestro ying +Un fortunately +Ġfasc inating +S em +Ġelect oral +Ġtrans parency +ĠCh aos +Ġvolunte er +Ġstatist ical +Ġactiv ated +ro x +We b +H E +ĠHamp shire +is ive +M ap +Ġtr ash +ĠLaw rence +st ick +C r +Ġr ings +EX T +Ġoper ational +op es +D oes +ĠEv ans +Ġwitness ed +P ort +Ġlaunch ing +ec onom +w ear +ĠPart icip +um m +cul es +ĠR AM +ĠT un +Ġass ured +Ġb inary +Ġbet ray +Ġexpl oration +ĠF el +Ġad mission +it ated +S y +Ġav oided +ĠSim ulator +Ġcelebr ated +ĠElect ric +¥ ŀ +Ġcl uster +itzer land +he alth +L ine +ĠN ash +at on +Ġsp are +Ġenter prise +ĠD IS +clud es +Ġfl ights +Ġreg ards +ĠÃ Ĺ +h alf +Ġtr ucks +Ġcontact s +Ġunc ons +ĠCl imate +Ġimm ense +N EW +oc c +ect ive +Ġemb od +Ġpat rol +Ġbes ide +Ġv iable +Ġcre ep +Ġtrig gered +ver ning +Ġcompar able +q l +Ġg aining +ass es +Ġ( ); +ĠG rey +ĠM LS +s ized +Ġpros per +" ? +Ġpoll ing +Ġsh ar +ĠR C +Ġfire arm +or ient +Ġf ence +Ġvari ations +g iving +ĠP i +osp el +Ġpled ge +Ġc ure +Ġsp y +Ġviol ated +Ġr ushed +Ġstro ke +ĠBl og +sel s +ĠE c +,' ' +Ġp ale +ĠColl ins +ter ror +ĠCanad ians +Ġt une +Ġlabor atory +Ġn ons +t arian +Ġdis ability +ĠG am +Ġsing er +al g +ĠSen ior +Ġtrad ed +ĠWar rior +Ġinf ring +ĠFrank lin +Ġstr ain +ĠSwed ish +Ġsevent h +ĠB enn +ĠT ell +Ġsynd rome +Ġwond ered +id en +++ ++ +ig o +Ġpur ple +Ġjournal ism +Ġreb el +Ġf u +bl og +Ġinv ite +ren cies +ĠCont act +Is rael +ĠCont ent +Ġche er +Ġbed room +ĠEngine ering +ĠQue ens +Ġd well +ĠPlay Station +ĠD im +ĠCol on +l r +Ġoper ates +Ġmotiv ation +US A +ast ered +C ore +ĠTr uth +ol o +OS E +ĠMem ory +Ġpred ec +Ġan arch +Ġ19 20 +ĠY am +à ¨ +b id +Ġgr ateful +Ġexc itement +Ġtre asure +Ġlong est +ct ive +Ġdes erves +Ġreserv es +Ġcop s +ĠOtt awa +ĠEgypt ian +ank ed +Ġart if +Ġhypot hesis +: / +Ġpurch asing +Ġlove ly +H P +Ġdiv ide +Ġstrict ly +Ġquestion ing +Ġtaxp ayers +ĠJ oy +Ġroll s +ĠHe avy +Ġp orts +Ġmag netic +Ġinf lamm +Ġbr ush +t ics +â ĪĴ +Ġbott les +pp y +Ġp add +ãĤ ¯ +m illion +Ġdevast ating +Ġcomp iled +Ġmed ication +Ġtw elve +ĠPer ry +Sp ace +im b +y our +Ġle aked +ĠT ar +Ġun ity +Ġinfect ed +Ġtravel ed +ID E +ĠMc Donald +t xt +ĠPr inc +Ġinter ven +ĠTai wan +ĠP ow +Ġbe aring +ĠTh read +Ġz ones +iz ards +un ks +Ch apter +ll or +Ġ · +Ġw ounds +Ġdisc retion +Ġsucceed ed +ik ing +Ġicon ic +C all +Ġscreen ing +ĠM is +ict s +Ġmin isters +Ġsepar ation +Pl ayer +Ġb ip +Ġbel oved +Ġcount ing +ĠE ye +ar ound +ing ing +Ġtable t +Ġoff ence +in ance +h ave +ĠInf o +ĠNin ja +Ġprotect ive +ĠC ass +M ac +ĠQual ity +N orth +Ġ ic +ĠCub a +ĠChron icle +ĠPro perty +Ġfast est +ot os +ĠG erm +OW N +Ġbo om +ĠStan ley +ergus on +Ġcle ver +Ġent ers +m ode +ter ior +ĠS ens +Ġlin ear +AR K +Ġcomp aring +Ġpure ly +Ġsaf er +ĠPot ter +Ġc ups +R T +Ġgl uc +Ġatt ributed +Ġdu pl +ĠP ap +Ġprec ious +Ġp a +iction ary +ĠT ig +ĠTo o +ol utions +st an +Ġrob ots +Ġlob b +Ġstat ute +Ġprevent ion +w estern +16 0 +ĠAct ive +ĠMar ia +h al +N one +ell ar +ĠK B +ĠPart ners +ĠSing le +ĠFollow ing +ang o +ac ious +Ġth ou +Ġk g +Ġinflu ential +ĠFriend s +S ur +ain ted +Ġfor ums +Ġst arter +Ġcitizens hip +ĠE lection +on ge +ot ation +os ph +;; ;; +ut ical +p ur +ere n +Ġaccus ations +bit ious +ab bit +ĠOr d +Post ed +ir k +Ġsens itivity +ic he +ĠAm y +ĠF ab +Ġsum mit +Ġped est +Ġrub ber +Ġagric ultural +Ġcan cel +A E +Ġin aug +Ġcont am +Ġfirm ly +i w +st age +ĠK an +Ġt ier +Ġinv ention +Ġtransl ated +ĠR ules +B ox +Tw itter +ID S +Ġp izza +Ġdeb ug +ĠD rop +v s +Ġh orses +b ig +Ġb oring +Ġh ood +ĠMcC ain +at ched +ĠBro s +Ġsk ip +Ġess ay +st at +ĠLeg ends +Ġam munition +au c +Ġshoot er +Ġun h +Ġsuppl ied +Ġgener ic +ĠS K +ib an +yr ics +Ġ25 5 +Ġclim bing +Form er +Ġfl ip +Ġjump ing +Ġfrust ration +ĠTer ry +Ġneighborhood s +Ġmed ian +be an +Ġbr ains +Follow ing +Ġsh aped +Ġdraw s +Ġal tered +J ack +Ġrecip es +Ġsk illed +we alth +ach i +e lection +Ġbehavi ors +de als +ĠU ntil +F e +Ġdecl aration +mar ks +ĠBet ween +cel ona +Ġres on +Ġbub ble +Am ong +Ġim perial +G S +Ġfemin ist +200 5 +ĠK yle +Ġaccount ing +ĠTe le +ĠT yr +Ġconnect ing +Ġre hab +ĠP red +s im +Ġmeant ime +Ġphys ician +M W +ĠCamp bell +ĠBr andon +Ġcontribut ing +ĠR ule +ĠWe ight +ĠN ap +Ġinter active +Ġv ag +Ġhel met +ĠCom b +f our +Ġsh ipped +Ġcomple ting +ĠP D +PD ATE +Ġspread ing +Ġsc ary +erv ing +ĠG as +Ġfr ank +s chool +Ġrom antic +Ġstab il +R ob +Ġaccur ately +Ġac ute +ĠH ann +Ġsymbol s +Ġcivil ization +ĠA W +Ġlight ning +Ġcons iders +Ġven ue +Ġ × +Ġo ven +ĠS F +h is +Ġn u +ĠLear n +Ġpe oples +Ġst d +Ġsle e +Ġs lic +ĠStat istics +Ġcor ners +ĠB aker +Ġ: ) +ment ation +ol ver +Ġlaugh ing +ĠT odd +ond e +ĠH ills +Ġn uts +ĠW oman +pl ane +Ġl iver +ĠIn side +S orry +Ġagre es +Ġfund ament +ĠF isher +Ġa uction +Ġthread s +gl as +ĠBas ic +ĠN at +Ġlack ing +Ġceleb ration +j u +Ġs illy +E uro +Ġt att +ight y +cont rolled +T est +ĠSing h +Ġr age +Ġrh yth +o ffic +ĠPh antom +Ġhead lines +Ġrespond ing +ĠMor ning +Ġvit amin +Ġboot s +ĠS ite +al in +p i +Ġvir al +ĠU C +D ER +ĠSe x +Ġst ocks +c urrent +Ġch urches +ĠR are +ĠMur phy +Ġden ial +ĠG aming +Ġtou g +Ġn ick +Ġm akers +ĠRon ald +Ġgener ous +ĠD oc +ĠMor ris +Ġtransform ed +ĠN ormal +Ġ10 4 +ĠKick starter +ĠUp on +On line +ĠI RS +Ġw rap +Ġl oving +Ġarri ves +ĠD ue +Ġhe ter +ĠM ade +Ġrent al +Ġbelong s +Ġatt orneys +Ġcro ps +Ġmat ched +ul um +ol ine +10 9 +Ġdis par +Ġbuy ers +ĠCam bridge +Ġeth ics +rou ps +Ġjust ified +Ġmarg inal +Ġrespect ed +win ning +Ġnodd ed +ĠSer ge +ĠForm er +C raft +######## ######## +ĠWar ner +Ġd ash +et e +Ġent ert +ĠE scape +out heast +Ġkn ees +ĠB omb +Ġr ug +P ass +Ġatt itudes +go vernment +ĠPri or +Ġqual ities +Ġnot ification +ĠPh one +l ie +Ġanticip ated +ĠCom bat +ĠBar ry +Ġ198 2 +Us ers +on er +Ġcomput ing +ĠConnect icut +Ġless er +Ġpe ers +ĠC u +Ġtechn ically +Ġsub mission +ĠUn iversal +Ġman ually +our ge +Ġrespond ents +ĠB TC +ĠH ost +Ġf are +ĠB ird +Ġrece ipt +al so +Ġj ack +Ġagric ulture +Ġsk ull +Ġ! = +Ġpass ive +ĠC I +Ġsoc ieties +Ġremind ed +Ġinter ference +B uy +Ġâ ľ +g on +Ġscrut iny +ĠW itch +Ġconduct ing +Ġ ãĥ +Ġexch anges +ĠMit chell +Ġinhab it +Ġtw ist +B D +Ġwhere ver +group on +Ġj okes +ĠBen jamin +ĠR andom +fr ame +ĠL ions +Ġhighlight ed +ĠArk ansas +E nt +Ġp ile +Ġpre lim +g s +mind ed +Ġfel ony +ĠG A +ĠL uck +Ġpract ically +ĠB os +Ġact ress +D am +ĠB ou +Ġvis a +Ġembed ded +Ġhy brid +Ġear liest +Ġsoon er +s ocial +ĠH A +Ġste ep +Ġdis advant +Ġexplo it +ĠE gg +ĠUlt ra +Ġnecess ity +L ocal +ie ge +Ġd ated +Ġmass es +Ġsubsc ription +pl ess +Ġan onym +Ġpresum ably +Bl ue +The ir +asket ball +ĠPhil ip +Ġcom ed +load ed +r ane +Ġref lection +Ch ina +Ġext ends +Ġform ing +Ġund ers +200 1 +Ġgr at +Ġconcent rations +Ġins ulin +Ġsec ular +Ġwh ilst +Ġwin ners +Ad vertisements +Ġdeliber ately +ĠWork ing +Ġs ink +et ics +d ale +Ġmand ate +Ġg ram +Ġvac ation +Ġwarn ings +ri pp +ĠTH AT +Ġcomment ary +Ġint u +Ġa est +Ġreason ing +Ġbreak down +ĠZ ombie +Ġ-- > +ĠPolit ical +c ott +Ġthr ust +Ġtechn ological +Ġdec iding +Ġtraff icking +L ong +W elcome +pr ising +ĠCommun ications +Ġend ors +Ġsw ift +Ġmetab ol +co ins +res a +ĠHT TP +Ġen roll +ĠH appy +us r +int age +Ġ[ " +u ably +ĠM aterial +Ġrepe al +Se pt +k h +ĠMod i +Ġunder neath +ĠI L +sh ore +Ġdiagn osed +ace utical +Ġsh ower +au x +ĠSw itch +ĠStre ngth +Ġj ihad +n ational +Ġtra uma +uss y +on i +Ġcons olid +Ġcal ories +ĠF lynn +ag ged +16 8 +ĠP ink +Ġfulf ill +Ġch ains +Ġnot ably +ĠA V +L ife +ĠCh uck +m us +ĠUr ban +ĠH end +Ġdep osit +ĠS ad +Ġaff air +OR K +ie val +ĠF DA +Ġt rop +ĠOver all +Ġvirt ue +Ġsatisf action +au nd +Ġl un +ĠSw itzerland +ĠOper ation +pro cess +Ġsh ook +Ġcount ies +le ased +ĠCharl otte +1 12 +Ġtrans cript +Ġre dd +p ush +ĠHe y +ĠAn alysis +[ " +Ġaltern atives +ard less +Ġele ph +Ġpre jud +ĠLe af +H aving +ĠH ub +Ġexpress ions +ĠVol ume +Ġshock ing +ĠRed s +Ġread ily +Ġplan ets +ad ata +Ġcollaps ed +ĠMad rid +Ġir rit +i pper +ĠEn c +ĠW ire +Ġbu zz +ĠG P +ash a +Ġaccident ally +ur u +Ġfrust rated +ĠS A +Ġhung ry +ĠH uff +Ġlab els +ant o +ĠE P +Ġbar riers +) | +ĠBer keley +ĠJ ets +Ġp airs +ĠL an +J ames +ĠB ear +Ġhum or +ĠLiber ty +Ġmagn itude +Ġag ing +ĠM ason +Ġfriends hip +umb ling +Ġemer ge +Ġnewsp apers +Ġam bitious +ĠRich ards +atern al +Ġ198 1 +Ġcook ies +Ġsc ulpt +Ġpur suit +L ocation +Ġscript s +p c +Ġarrang ements +Ġd iameter +Ġl oses +am ation +Ġl iqu +ĠJ ake +aret te +Ġunderstand s +ĠZ en +v m +Ġappro ve +Ġw ip +Ġult ra +Ġint end +ĠD I +asc ular +Ġst ays +ĠK or +ĠK l +Ġinvest ing +L a +Ġbelie ving +b ad +m outh +Ġtaxp ayer +ãĥ ĥ +ĠQue bec +Ġl ap +ĠSw iss +d rop +Ġdr ain +ir i +et c +ft en +ĠN ex +Ġst raw +Ġscream ing +Ġcount ed +Ġdam aging +Ġamb assador +cent ury +Ġpro x +Ġarrest s +u v +il ateral +ĠCh arg +Ġpresc ribed +Ġindepend ently +Ġf ierce +ĠB aby +Ġb rave +Ġsu its += > +Ġbas eline +ĠR ate +Ġis lands +Ġ( ( +g reen +ix els +Ġname ly +ĠVill age +th an +am y +V ersion +g mail +ential s +ĠS ud +ĠMel bourne +Ġarri ving +Ġquant um +e ff +rop olitan +T ri +Ġfun eral +ĠI R +ÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤ ÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤ +ĠC ob +it ably +Ġt urb +Ġcomb o +Re view +Ġdeploy ment +u ity +ĠB ott +Ġinv isible +Ġrender ing +Ġunl ocked +Ġa qu +ĠVlad imir +Ġp ad +ĠBr ain +ĠLeg acy +dr agon +ĠKurd ish +Ġsound ed +Ġdet ained +ĠD M +g ary +Ġd aughters +Ġdistur bing +uk a +ĠPar ad +Ġt ast +Ġunf ortunate +Ġu l +em in +Ġattend ance +tr l +Ġpar ks +ĠMem orial +ĠAl ice +oth y +gu ard +ĠD ise +ĠSh an +ĠFor um +R ich +Ġshif ted +ue z +Ġl ighter +ĠMag n +Ġc od +S ch +ham mad +P ub +3 50 +ĠP okemon +Ġprot otype +Ġun re +B ase +ĠStud ents +ĠRep ly +ĠCommun ist +Ġg au +ĠTy ler +I Z +Ġparticip ated +Ġsup rem +ĠDet ails +Ġvessel s +ro d +Ġt ribe +ke ep +Ġassum ptions +Ġp ound +Ġcr ude +ĠAv ailable +Ġswim ming +Ġin clusion +Ġadv ances +c ulation +Ġconserv ation +Ġover d +ĠBuff alo +Art icle +ed ge +Ġaw a +ĠMad ison +Ġsid ew +Ġcat ast +ĠK rist +uc le +ĠHigh way +ĠTer ror +Ġactiv ation +Ġuncons cious +ĠSat an +ĠSus an +ill ery +Ġarr anged +i op +Ġrum ors +ur ring +th ink +ĠKe ith +ĠK ind +Ġavoid ing +by n +n ut +ĠSpe aker +r us +n ames +Ġgu ilt +ĠOlymp ics +Ġsa il +ĠM es +lev ant +ĠColumb us +a ft +C ity +S outh +ĠHar vey +ĠP un +S everal +Ġment ally +Ġimp ress +m ount +ĠUb untu +âĢĶâĢĶâĢĶâĢĶ âĢĶâĢĶâĢĶâĢĶ +ĠSuper man +ĠMP s +Ġintent ions +ĠR acing +Ġlike lihood +Ġ2 40 +T otal +Ġto ys +ĠW atson +Ġur ge +L ear +ĠP aper +Ġoccur ring +ĠB eng +ĠC ert +Ġst ones +T im +ĠTw in +z b +ĠD ynam +Ġpolit ician +k ens +ĠEnter prise +UT ERS +Ġab ol +Ġref resh +Ġarbit rary +pe ction +Ġtrou bles +Ġ} ); +t v +Ġpil ots +Ġdist ribute +Ġaud it +Ġp ause +orig inal +Ġr ivals + £ +F ig +T L +ab il +ry ing +L in +ion ed +l on +Ġf ancy +Ġcr ashed +Ġt ract +Ġshe d +Ġcons ume +B ased +down load +in it +Ġvolt age +Int rodu +Ġcondem ned +ĠFin ance +res pect +Ġex cluded +Ġestablish ing +her ic +Ġher itage +Ġspect acular +Ġun st +ĠSnow den +ĠL ane +S an +Ġprotect ions +st ruction +inc inn +Ġmac ro +C ustom +ios ity +Ġes p +Ġfunction ing +Ġm ush +Ġp uzzle +Ġeth ical +M al +Ġgo verning +ĠF erguson +Ġrest ored +Ġst ressed +ĠCoun ter +ĠK as +cl ip +AN S +Ġse iz +U K +by ss +old own +ap i +Ġperman ently +oun ters +W est +Th rough +L ight +at oes +Ġne at +Ġc ord +ure r +Ġsevere ly +ĠA ven +Ġinter rog +Ġtri ple +G iven +N umber +Ġar ise +Ġs her +pl ant +Ġfl ower +ĠC ou +Ġat e +Ġnew er +b ul +Ġmean while +ĠL air +Ġadjust ment +ĠCop yright +Ġd ivers +i ological +Ġgam ers +o at +Ġhistor ically +Ġanal og +Ġlong time +Ġpres cription +ĠM ist +ĠHy per +ĠM aine +ĠDe ity +Ġmulti pl +ĠRe incarn +ĠH yd +ĠP ic +S il +r ants +ĠC ris +. ; +( { +epend ence +Ġrec y +ate ur +Ġqu ad +Ġgl ob +Ġcon ced +te am +Ġcapital ist +ĠL ot +Ġroy al +ĠCy ber +Ġblack s +met ic +ri v +ĠD anny +Ġsp o +ĠR O +Ġanim ated +rypt ed +ĠDep uty +Ġrend ered +F E +Ġstre ak +Ġcloud s +ĠDou g +~~~~ ~~~~ +Ġdisc our +ĠVe h +Ġpsych ology +ĠJ ourney +Ġcry stal +ĠFro st +Ġsuspic ion +Ġrel ate +or us +ĠC rypt +ĠN VIDIA +com ed +ut ing +incinn ati +Ġvulner ability +ost ic +Ġisol ation +Ġcool ing +ĠCoal ition +Ġ1 19 +F our +ĠDe al +Ġâ ī +se mble +ram ent +ĠBar celona +Ġ10 2 +Ġcoc aine +ocaly pse +F eb +ogen ic +Ġmut ation +Ġcrypt oc +ĠK el +ĠG it +a is +Ġs isters +AN K +Ġactiv ate +T er +Ġd read +yl on +Ġprop ri +A ust +ĠDef ault +Ġout door +Ġshe er +ce ive +Ġg ently +Ð ¾ +Pro gram +Ġâ ĨĴ +Ġve gan +ĠCr us +Ġrespons ibilities +ĠH R +OL D +Ġprev ents +Ġst iff +ĠW ere +Ġathlet ic +ĠSc ore +Ġ) : +Ġcolumn s +ĠL oc +av ailable +ĠF ram +ĠS essions +Ġcompan ion +Ġpack s +14 0 +ĠKn ights +Ġf art +Ġstream s +Ġsh ore +Ġapp eals +ĠPer formance +h aul +ĠSt ra +ĠN ag +10 3 +ĠTrans portation +B B +E v +z an +P ublic +Ġtw in +uls ion +M ult +Ġelect ro +Ġstat ue +ation ally +ĠN ort +Ġins pection +/ * +ig ue +Ġcomp assion +ĠT ales +ĠSte in +ĠSc reen +ĠB ug +ĠL ion +g irl +Ġwithdraw al +Ġobject ives +Ġblood y +Ġprelim inary +Ġj acket +Ġdim ensions +ĠC ool +ĠOcc up +Ġw reck +Ġdoub led +ank ing +Ġ19 75 +Ġglass es +ĠW ang +pro v +P ath +connect ed +ĠMult i +ĠNor way +agon ist +Ġfe ared +Ġtouch ing +Ġarg uably +¯¯¯¯ ¯¯¯¯ +ĠNC AA +che m +Ġsp at +ĠW WE +ĠC el +ig ger +Ġattack er +ĠJo in +ob ject +ett a +Ġelim inated +d et +Ġdest ruct +ĠLuc as +ct uary +18 0 +ĠBr ady +ĠBl ues +B ay +au kee +Ġtim eline +Ġdeleg ates +w ritten +uff icient +Ġsh apes +Cop yright +ou ble +serv ice +Ġp ione +Ġcolleg es +Ġrow s +Ġsp ite +Ġassess ed +3 60 +Ġle ase +Ġconfident ial +ck er +ĠMan ning +ĠV oice +Ġse aled +Ġcalcul ate +N O +ĠAss istant +Ġteen ager +ul ent +ather ine +Ġm ock +Ġd iamond +Ġf est +Ġsw itched +Ġres ume +ĠPu erto +Ġl anes +ir ation +ĠSimilar ly +Ġro d +ĠS el +ĠPal ace +ĠLim ited +e ous +Ġvar iant +Ġw ard +Ġ) ) +Sh ow +OO K +A lex +ĠN ep +br is +ĠWik ipedia +Ġexcept ional +Ġman ages +ĠD raw +Ag ain +Ġco pper +ut t +Ġex ports +Ġport folio +Ġelev ated +R ated +ĠOther wise +ĠT act +ĠShe l +ĠT X +" âĢĶ +Ġres ur +ĠW a +ven ant +Ġmon etary +pe ople +E mail +Ġfif ty +ĠS weet +ĠMalays ia +Ġconf using +ĠR io +ud a +uten ant +" ); +Ġpra ised +Ġvol umes +t urn +Ġm ature +Ġnon profit +Ġpassion ate +ĠPriv ate +Ġ10 3 +Ġdesc end +ç ¥ŀ +uff y +head ed +Whe ther +ri en +ze ch +be it +Ġch rom +ĠMc M +Ġd ancing +Ġe leg +ĠNot iced +11 5 +Ġadvoc acy +ENT S +amb ling +ĠMin or +ĠF inn +Ġprior ities +Ġthere of +ĠSt age +ĠRog ers +Ġsubst itute +ĠJ ar +ĠJeff erson +Ġlight ly +10 2 +ĠL isa +u its +ys ical +Ġshif ts +Ġd rones +Ġwork place +Ġres id +ens ed +ah n +Ġpref erences +ser ver +Ġdeb ates +d oc +ĠGod s +Ġhelicop ter +Ġhon our +Ġconsider ably +ed ed +ĠF emale +ĠAn ne +Ġre un +ĠF ace +ĠHall ow +ĠBud get +Ġcondem n +Ġt ender +Pro f +ocr atic +ĠTurn er +ĠAg ric +Ġ19 76 +Ġa pt +d isc +ĠF ighter +ĠA ur +Ġgar bage +in put +ĠK arl +ĠOl iver +ĠL anguage +k n +N on +ĠCl ar +Ġtrad itions +Ġad vertisement +ĠS or +Ġarch ive +Ġvill ages +7 50 +Ġimplement ing +w aukee +Ġdiet ary +Ġswitch ing +Rep ublic +Ġvel ocity +Ġc it +ĠA wards +Ġfin ancing +Ġlast ed +) ] +Ġrem inder +P erson +Ġprec ision +Ġdesign ers +ĠF ried +ĠB order +Ġtr agic +Ġw ield +Ġiniti atives +ĠT ank +w er +Ġjo ins +R o +in ery +Ġar row +Ġgener ating +found er +Ġsear ches +Ġrandom ly +A ccess +Ġb atch +Ġp osed +l at +Ġpursu ing +as a +Ġtest ified +form ing +ĠSh ar +w iki +ĠE ither +S ometimes +Ġsen ators +ĠJohn ny +ĠTal iban +ĠG PS +":" / +ãģ® å +Ġanaly zed +ĠRub io +ĠMove ment +op ard +ii i +St and +f ight +Ġign oring +i ang +ĠG N +so ever +ĠST AT +Ġref using +Ġswe at +Ġb ay +P ORT +ir med +ak y +Ġdis pro +Ġlabel ed +Ġ10 8 +H ello +Ġple asant +ab a +Ġtri umph +Ġab oard +Ġinc om +ĠC row +le tt +Ġfol k +Ġch ase +` ` +ĠBr us +Ġte ens +c ue +Ġter rain +h yd +il ight +OR Y +Su pport +ew s +ll i +rain ts +ĠC and +Ġab used +ach ment +l arg +B as +ĠC ancer +Ġ19 78 +Ġsupp orter +ac cess +ĠTer min +ĠT ampa +ĠAN Y +Ġnew est +ĠCrim inal +ed u +Ġ19 30 +Ġadm its +Ġend e +Ġfail ures +ur ate +ful ness +cy cl +ĠSub ject +Ġinf inite +th ree +W A +p it +ĠInst all +R ad +ili ation +G M +Ġcontin ent +Ġaccommod ate +ĠCl ay +Ġp up +ĠF unction +Ġham mer +ĠAlbert a +Ġrev ised +Ġminor ities +Ġmeasure ment +Con nell +Ġdis able +ĠM ix +In cre +Ġfor k +ĠR osen +Ġimpl ies +umb lr +AN G +Ġprote ins +Ġagg ression +Ġfacilit ate +S N +Ġilleg ally +u er +Ġacad em +Ġp uzz +ĠSh ift +p ay +oll o +Ġaud iences +B uild +Ġno ble +Ġsynt ax +â ĺħ +Ġbe am +ĠB ed +ĠA ld +Ġorig ins +v ideo +Ġ19 77 +ĠAss ault +Ġgar age +Te am +Ġver dict +Ġd war +ĠVirt ual +e vent +Ke ep +Ġsent iment +Ġwild life +sh irt +Ġb urg +Ġrecommend ation +rep resent +Ġgall ery +own ers +Ġsch olar +Ġconven ience +ĠSw ift +Ġconv inc +C ap +Ġwar fare +ĠVis ual +Ġconst itute +Ġab ort +ĠWe ather +ĠLook ing +ĠH em +Ġmart ial +Ġinc oming +et ition +Ġtoler ance +ĠCre ated +Ġfl ows +ĠE lder +Ġsoul s +Ġf oul +ĠP ain +ĠC AN +Ġ2 20 +b c +he nd +Ġgen ius +R eal +ĠW r +omet er +p ad +Ġlim iting +ĠS i +ĠL ore +ĠAd ventures +Ġvar ied +D isc +f in +ĠPerson al +Ch ris +Ġinv ented +Ġd ive +ĠR ise +Ġo z +ĠCom ics +Ġexp ose +ĠRe b +let ters +s ite +im ated +Ġh acking +Ġeduc ated +ĠNob ody +Ġdep ri +Ġincent ive +ãĤ · +Ġovers ight +Ġtrib es +ĠBelg ium +Ġlicens ing +our t +Produ ct +ah l +ĠG em +Ġspecial ist +Ġc ra +ann ers +ĠCor byn +Ġ19 73 +RE AD +Ġsum mar +Ġover look +ĠApp lication +Ġin appropriate +Ġdownload ed +Q ue +ĠB ears +Ġth umb +ĠChar acter +ĠReincarn ated +ĠS id +Ġdemonstr ates +s ky +ĠBloom berg +ĠAr ray +ĠRes ults +ĠFour th +ĠED T +ĠO scar +c end +Ġ10 6 +ĠN ULL +ĠH ERE +m atch +ĠBr un +Ġgluc ose +ie g +eg u +Ġcert ified +Ġrel ie +Ġhuman itarian +Ġpr ayers +K ing +Ġn an +h ou +10 8 +ul u +Ġrenew able +Ġdistingu ish +Ġd ense +ĠV ent +ĠPack age +ĠB oss +Ġedit ors +Ġm igr +T ra +ĠPet ers +ĠAr ctic +200 4 +ĠC ape +Ġloc ally +Ġlast ing +Ġhand y +. ). +P an +ĠR ES +Ind ex +Ġt ensions +Ġformer ly +Ġide ological +Ġsens ors +Ġdeal ers +Ġdef ines +S k +Ġproceed s +Ġpro xy +az ines +ĠB ash +ĠP ad +ĠC raft +eal ous +Ġshe ets +omet ry +J une +cl ock +T T +ĠThe atre +ĠB uzz +Ġch apters +Ġmill enn +Ġd ough +ĠCongress ional +Ġimag ined +av ior +Ġclin ic +Ġ19 45 +Ġhold er +ro ot +oles ter +Ġrest art +B N +ĠHam as +ĠJ ob +Ġor b +Ġr am +Ġdiscl ose +Ġtransl ate +Ġimm igrant +Ġannoy ing +Ġtreat y +an ium +ĠTe a +ĠLeg ion +Ġcrowd s +ĠB ec +ĠA er +oh yd +B ro +Look ing +Ġl bs +Ġagg ress +Ġse am +Ġinter cept +ĠM I +mer cial +act iv +ĠC it +Ġdim ension +Ġconsist ency +Ġr ushing +ĠDou glas +Ġtr im +Inst all +ick er +Ġsh y +10 6 +Ġment ions +pe lled +ĠT ak +c ost +Ġclass room +Ġfort une +dri ven +Ġun le +ĠWhe el +Ġinvest or +ĠM asters +k it +Ġassoci ations +ĠEv olution +op ing +us cript +Ġprov incial +ĠWal ter +av i +S O +Ġun limited +Eng lish +ĠC ards +ĠEb ola +ne red +Ġreven ge +Ġout right +um per +Ġf itting +ĠSol id +Ġform ally +Ġproblem atic +Ġhaz ard +Ġenc ryption +Ġstraight forward +ĠA K +Ġp se +ĠOr b +ĠCh amber +ĠM ak +Cont ents +Ġloyal ty +Ġl yrics +ĠSy m +Ġwel comed +Ġcook ed +Ġmon op +Ġn urse +Ġmis leading +Ġe ternal +Ġshif ting +Ġ+ = +V is +Ġinst itutional +ill ary +Ġp ant +VER T +ĠA CC +ĠEn h +Ġinc on +ĠRE UTERS +Ġdon ated +â̦â̦ â̦â̦ +In tern +Ġexhib it +Ġt ire +ĠR ic +ĠCh ampion +ĠMu hammad +N ING +ĠSoc cer +Ġmob ility +Ġvary ing +ĠM ovie +Ġl ord +o ak +F ield +Ġve ctor +us ions +Ġsc rap +Ġen abling +m ake +T or +. * +| | +ĠWe bsite +ĠN PC +Ġsocial ist +ĠBill y +ĠAdd itional +Ġc argo +Ġfar ms +ĠSo on +ĠPri ze +Ġmid night +Ġ9 00 +se en +ĠSp ot +Ġshe ep +Ġspons ored +ĠH i +ĠJ ump +Ġ19 67 +Micro soft +ĠAg ent +Ġch arts +d ir +Ġadj acent +Ġtr icks +Ġman ga +Ġex agger +/ > +foot ball +ĠF CC +G C +ĠT ier +and ra +OU ND +% ), +Ġfru its +V C +ĠA A +R ober +Ġmid st +â Ĺ +ank a +Ġlegisl ature +ĠNe il +Ġtour ists +" " +ĠWar ning +ĠNever theless +ĠOffic ial +ĠWh atever +Ġm old +Ġdraft ed +Ġsubst ances +Ġbre ed +Ġt ags +ĠT ask +Ġver b +Ġmanufact ured +com ments +ĠPol ish +Pro v +Ġdetermin es +Ob ama +k ers +Ġutter ly +Ġse ct +sc he +ĠG ates +ĠCh ap +Ġal uminum +Ġz ombie +ĠT ouch +ĠU P +Ġsatisf y +Ġpred omin +asc ript +Ġelabor ate +Ġ19 68 +Ġmeas uring +ĠV ari +any ahu +Ġs ir +ul ates +id ges +ick ets +ĠSp encer +T M +oub ted +Ġpre y +Ġinstall ing +ĠC ab +re ed +re ated +Su pp +Ġwr ist +ĠK erry +10 7 +ĠK le +ĠR achel +Ġc otton +ĠA RE +ĠE le +Cont rol +Ġload s +ĠD od +an as +b one +Ġclass ical +ĠReg ional +ĠInt eg +V M +Ġdes ires +Ġaut ism +support ed +ĠM essage +Ġcomp act +writ er +Ġ10 9 +ĠHur ricane +c ision +Ġcy cles +Ġdr ill +Ġcolle ague +Ġm aker +G erman +Ġmist aken +S un +ĠG ay +Ġwhat soever +Ġsell s +ĠA irl +l iv +ĠO ption +Ġsol ved +Ġse ctors +Ġhorizont al +Ġequ ation +ĠSk ill +ĠB io +g ement +ĠSn ap +ĠLeg al +Ġtradem ark +Ġmake up +Ġassemb led +Ġsa ves +ĠHallow een +ĠVer mont +ĠFR OM +Ġfar ming +ĠP odcast +accept able +ĠHig her +Ġas leep +ull ivan +Ġrefere n +ĠLe v +Ġbul lets +ok o +H C +Ġst airs +Ġmain tains +ĠL ower +ĠV i +Ġmar ine +Ġac res +Ġcoordin ator +ĠJ oh +Ġcounterpart s +ĠBrother s +Ġind ict +b ra +Ġch unk +Ġc ents +H ome +ĠMon th +Ġaccording ly +if les +ĠGerm ans +ĠSy n +H ub +Ġey eb +âĶĢâĶĢ âĶĢâĶĢ +Ġr anges +ĠHoll and +ĠRob ot +f c +M ike +Ġpl asma +Ġsw ap +Ġath lete +ĠR ams +,' " +Ġinfect ions +Ġcor rid +Ġv ib +Ġpat ches +Ġtradition ally +Ġrevel ation +Ġswe ep +Ġgl ance +Ġin ex +200 3 +ĠR aw +work ing +os ures +ĠD at +ĠLyn ch +Ġle verage +ĠRe id +Ġcorrel ation +ian ces +av ascript +Ġrep ository +ret ty +Ġ19 72 +24 0 +Ġo un +p ol +ĠRe ed +Ġtact ical +is ite +App le +ĠQu inn +Ġrap ed +ill o +Euro pe +Ġalgorith ms +ĠRod rig +i u +Ġill um +Ġf ame +Ġintrodu cing +Ġdel ays +ĠRaid ers +Ġwh istle +Ġnovel s +ĠRe ally +Ġder iv +Ġpublic ations +ĠNe ither +ĠCom merce +Ġa ston +l anguage +Not es +ĠR oth +ĠF ear +Ġm ate +Ġpar ade +ĠQ B +Ġman eu +ĠC incinnati +m itting +Ġwa ist +ĠR ew +Ġdisc ont +Ð ° +Ġst aring +Ġal ias +Ġsec urities +Ġtoile t +ĠJ edi +Ġun law +v ised +//// //// +] ( +ĠWe iss +Ġpre st +ĠComp an +Ġmem o +ĠGr ace +J uly +ĠEl ite +cent er +ĠSt ay +Ġgal axy +Ġto oth +ĠS ettings +Ġsubject ed +ãĤ ¦ +Ġline back +Ġretail ers +ĠW ant +Ġd angers +A ir +Ġvolunt ary +ew ay +Ġinterpret ed +ot ine +à § +Ġp el +Serv ice +ĠEvent ually +Ġcare ers +Ġthreat en +Ġmem or +ĠBrad ley +anc ies +s n +ĠUn known +N ational +Ġsh adows +ail and +ĠD ash +Every one +izz ard +M arch += ( +Ġpull s +Ġstr anger +Ġback wards +ĠBern ard +imens ional +Ġch ron +Ġtheoret ical +k top +Ġw are +ĠInvest ig +ĠIn iti +ĠOper ations +o ven +oc ide +* / +Ġfl ames +ĠC ash +sh it +Ġc ab +ĠAn aly +ĠSe ah +Ġdefin ing +Ġorder ing +Ġimm un +Ġpers istent +AC H +Russ ian +m ans +Ġh ind +Ġphot ography + © +Ġh ug +Ġ10 7 +ĠH ence +i ots +ude au +Ġsubsid ies +Ġroutine ly +ĠDev ice +it ic +Ġdisg ust +land er +Ġ19 40 +Ġassign ment +ĠB esides +w ick +ĠD ust +us c +struct ed +11 1 +de velop +Ġf ond +Ġinter section +Ġdign ity +Ġcommission er +With out +re ach +Ġcart oon +Ġsc ales +ãĥ Ń +F IG +Ġsurve ys +ĠIndones ia +Ġart work +Ġun ch +Ġcy cling +un ct +au er +or ate +ĠOb viously +Ġcharacter ized +fe ld +Ġaff irm +Ġinn ings +Ġ é +Ġal iens +Ġcl oth +et ooth +ĠC ertain + § +Ġdig est +k now +ĠX L +Ġpredict ions +Ġd in +W AR +Ġafter math +Ex ample +ĠSu ccess +ĠTh r +IG N +Ġmin er +B us +Ġcl arity +heim er +ĠO UT +ĠS end +ĠCirc le +ĠD iet +Ġpron ounced +Ġcreat ors +Ġearthqu ake +atter y +ge ons +Ġo d +Ġlay ing +or p +U lt +pro ject +Ġunder min +Ġsequ el +S am +ĠDark ness +Ġre ception +b ull +Y S +ĠV ir +Ġsequ ences +ĠCo in +Ġout fit +ĠW ait +1 19 +Ġdel ivers +.... .. +Ġbl own +ĠE sc +ĠM ath +per m +ĠU l +Ġgl im +Ġfac ial +Ġgreen house +Ġto kens +/ - +ĠAnn ual +ĠON E +Ġteen age +ĠPhys ical +ĠL ang +ĠC elt +Ġsu ed +ivid ually +Ġpat ience +ch air +reg ular +Ġa ug +in v +ex cept +ĠL il +Ġn est +f d +s um +ĠCh ase +Russ ia +ĠJenn ifer +Ġoff season +Over all +F ore +Ġr iot +A ud +form er +Ġdefend ers +ĠC T +iot ic +rib ly +Ġautom ated +Ġpen is +Ġins ist +Ġdi agram +ĠS QL +ĠG arc +Ġw itch +cl ient +ier ra +am bers +Ġrec ount +f ar +V ery +oster one +Ġappreci ated +ĠPer fect +S ection +Ġd oses +oca ust +Ġcost ly +Ġg rams +ĠSh i +Ġwrest ling +Ġ19 71 +Ġtro phy +Ġn erve +ĠK az +ĠExper ience +Ġpled ged +Ġplay back +Ġcreat ivity +by e +Ġattack ers +Ġhold ers +ĠCo ach +ĠPh D +Ġtransf ers +Ġcol ored +ĠH indu +Ġd rown +Ġlist ened +ĠW A +ias m +P O +Ġappeal ing +Ġdiscl osed +ĠCh icken +ag ging +Ġple aded +Ġnav igation +ĠReturn s +Ġ[ [ +R OR +E A +Ġphotograp her +ĠR ider +ipp ers +Ġsl ice +Ġe rect +Ġhe d +iss ance +ĠVik ings +ur ious +Ġapp et +oubted ly +Ch ild +Ġauthent ic +o os +ĠM aking +Ġannoun cing +Ġb od +Ġmet er +ĠN ine +ĠR ogue +Ġwork force +Ġrenew ed +Ġorganis ations +ac s +P LE +Sh ort +Ġcomp ounds +ĠVis it +Ġen velop +ear th +Ġsupport ive +gg le +ĠBrus sels +ĠGu ild +Cre ate +RE L +Ġaver aged +Ġ19 69 +ri ages +Ġlength y +Ġforg ot +O kay +ĠE rd +Ġdeal er +Ġrec ession +D D +Ġdesper ately +Ġhun ger +Ġst icks +Ġm ph +ĠF aith +Ġintention ally +Ġdem ol +ue ller +ĠS ale +Ġde bris +s pring +Ġle ap +>> >> +Ġcontain ers +se lling +rane an +atter ing +Ġcomment ed +ĠC M +on ut +Ġwood s +es pecially +Ġorgan ize +iv ic +ĠWood s +ang a +s qu +Ġm aj +am on +Ġax is +Ġ19 74 +ĠDen mark +Ġwar rior +ĠP and +Ġout lined +ĠB O +ins ula +z illa +eb ook +Ġd are +Ġsear ched +Ġnav igate +S n +writ ing +Ġun ited +J apan +ĠHe brew +Ġfl ame +Ġrel ies +Ġcatch ing +ĠSh o +Ġimprison ment +Ġp ockets +Ġclos ure +ĠF am +t im +ade qu +Act ivity +Ġrecru iting +ĠW ATCH +ĠArgent ina +d est +Ġapolog ize +or o +Ġlack s +Ġtun ed +ĠGriff in +Ġinf amous +Ġcelebr ity +ss on +Ġ ---------------------------------------------------------------- +ĠIs is +ĠDis play +Ġcred ibility +Ġeconom ies +Ġhead line +ĠCow boys +Ġind ef +Ġl ately +Ġincent ives +but ton +ĠM ob +A ut +Ġres igned +ĠO m +c amp +Ġprof iles +Ġsche mes +olph ins +ay ed +Cl inton +en h +ĠY ahoo +Ġab st +Ġan k +su its +Ġw ished +ĠMar co +udd en +Ġsp here +ĠB ishop +Ġincorpor ated +ĠPl ant +11 4 +Ġh ated +p ic +Ġdon ate +Ġl ined +Ġbe ans +Ġsteal ing +Ġcost ume +Ġsher iff +Ġfor ty +Ġint act +Ġadapt ed +Ġtrave lling +b art +Ġnice ly +Ġdri ed +Ġsc al +os ity +NOT E +ĠB h +ĠBron cos +ĠI gn +Ġint imate +Ġchem istry +Ġopt imal +D eb +ĠGener ation +Ġ] , +ich i +ĠW ii +ĠYOU R +vent ions +W rite +Ġpop ul +un ning +ĠW or +V ol +Ġqu een +head s +K K +Ġanaly ze +op ic +ear chers +Ġd ot +leg raph +ast ically +Ġupgr ades +Ġca res +Ġext ending +Ġfree ze +Ġin ability +Ġorg ans +Ġpret end +Ġout let +11 3 +ol an +ĠM all +ul ing +t alk +Ġexpress ing +ĠAl ways +ĠBe gin +f iles +Ġlic enses +% % +ĠM itt +Ġfil ters +ĠMil waukee +G N +Ġunf old +M o +Ġnut rition +pp o +B o +Ġfound ing +Ġunder mine +Ġeas iest +ĠC zech +ĠM ack +Ġsexual ity +ĠN ixon +W in +ĠAr n +ĠK in +ãĤ £ +ic er +Ġfort un +Ġsurf aces +agh d +Ġcar riers +ĠP ART +ĠT ib +Ġinter val +Ġfrust rating +ĠSh ip +ĠAr med +ff e +Ġbo ats +ĠAb raham +in is +Ġsu ited +th read +i ov +ab ul +ĠVenezuel a +Ġto m +su per +Ġcast le +alth ough +iox ide +ec hes +Ġevolution ary +Ġnegoti ate +Ġconfront ed +Rem ember +Ġ17 0 +S uch +Ġ9 11 +m ult +ĠA byss +ur ry +ke es +spe c +ĠBarb ara +Ġbelong ing +Ġvill ain +ist ani +Ġaccount able +Ġport ions +ĠDe cl +U r +ĠK ate +g re +Ġmag azines +UC K +Ġregul ate +om on +ĠAl most +Ġover view +Ġsc ram +Ġl oot +ĠF itz +Ġcharacter istic +ĠSn ake +s ay +ĠR ico +Ġtra it +ĠJo ined +au cus +Ġadapt ation +ĠAirl ines +Ġarch ae +ĠI de +Ġb ikes +Ġliter ary +Ġinflu ences +ĠUs ed +C reat +Ġple a +ĠDef ence +ĠAss ass +Ġp ond +UL T +) " +Ġeval uated +Ġob taining +Ġdem ographic +Ġvig il +ale y +Ġsp ouse +ĠSeah awks +resp ons +ĠB elt +um atic +Ġr ises +run ner +ĠMichel le +Ġpot ent +r ace +ĠP AC +F ind +olester ol +IS S +ĠIntrodu ced +ress es +ign ment +O s +ĠT u +ĠDe x +ic ides +Ġspark ed +ĠLaur a +ĠBry ant +Ġsm iling +ĠNex us +Ġdefend ants +ĠCat al +Ġdis hes +sh aped +Ġpro long +m t +( $ +ãĢ Ĥ +Ġcalcul ations +ĠS ame +Ġp iv +H H +Ġcance lled +Ġgr in +Ġterrit ories +ist ically +C ome +ĠP arent +Pro ject +Ġneg lig +ĠPriv acy +Ġam mo +LE CT +olute ly +ĠEp ic +Ġmis under +w al +Apr il +m os +path y +ĠC arson +Ġalbum s +ĠE asy +Ġpist ol +< < +Ġ\ ( +t arget +hel p +Ġinter pre +cons cious +ĠH ousing +ĠJ oint +12 7 +Ġbe ers +s cience +ĠFire fox +effect ive +ĠC abin +ĠO kay +ĠApp lic +Ġspace craft +ĠS R +ve t +ĠStr ange +S B +Ġcor ps +iber al +e fficient +Ġpreval ence +Ġeconom ists +11 8 +Th read +ord able +OD E +ĠC ant +=- =- +if iable +ĠA round +Ġpo le +Ġwilling ness +CL A +ĠK id +Ġcomple ment +Ġsc attered +Ġin mates +Ġble eding +e very +Ġque ue +ĠTr ain +Ġh ij +Ġme lee +ple ted +Ġdig it +Ġg em +offic ial +Ġlif ting +Ð µ +Re qu +it utes +Ġpack aging +ĠWork ers +h ran +ĠLeban on +ol esc +Ġpun ished +ĠJ uan +Ġj am +ĠD ocument +Ġm apping +ic ates +Ġinev itably +Ġvan illa +ĠT on +Ġwat ches +Ġle agues +Ġiniti ated +deg ree +port ion +Ġrec alls +Ġru in +Ġm elt +I AN +Ġhe m +Ex p +Ġb aking +ĠCol omb +at ible +Ġrad ius +pl ug +ĠI F +et ically +Ġf ict +H ER +ĠT ap +atin um +Ġin k +Ġco h +ĠW izard +b oth +te x +Ġsp ends +ĠCurrent ly +ĠP it +Ġneur ons +ig nt +Ġr all +Ġbus es +b uilding +Ġadjust ments +Ġc ried +ibl ical +att ed +ĠZ ion +ĠM atter +Ġmed itation +ĠD ennis +Ġour s +ĠT ab +Ġrank ings +ort al +Ġad vers +Ġsur render +ĠG ob +ci um +om as +im eter +Ġmulti player +Ġhero in +Ġoptim istic +Ġindic ator +ĠBr ig +Ġgro cery +Ġapplic ant +ĠRock et +v id +Ex ception +p ent +Ġorgan izing +Ġenc ounters +ĠT OD +Ġjew el +S ave +ĠChrist ie +Ġhe ating +Ġl azy +ĠC P +Ġcous in +Con fig +Ġreg ener +Ġne arest +Ġachie ving +EN S +th row +ĠRich mond +ant le +200 2 +Ġan ten +b ird +13 3 +Ġn arc +r aint +un ny +ĠHispan ic +ourn aments +Ġprop he +ĠTh ailand +ĠT i +Ġinject ion +Ġinher it +rav is +Ġmed i +Ġwho ever +ĠDE BUG +G P +ĠH ud +C ard +p rom +Ġp or +Ġover head +L aw +Ġviol ate +Ġhe ated +Ġdescript ions +Ġachieve ments +ĠBe er +ĠQu ant +W as +Ġe ighth +ĠI v +Ġspecial ized +U PDATE +ĠD elta +P op +J ul +ĠAs k +oph y +Ġnews letters +ĠT ool +Ġg ard +ĠConf eder +ĠGM T +ĠAb bott +Ġimm unity +ĠV M +Is lam +Ġimpl icit +w d +Ġ19 44 +rav ity +omet ric +Ġsurv iving +ur ai +ĠPr ison +Ġr ust +ĠSk etch +Ġbe es +ĠThe ory +Ġmer it +T ex +ch at +Ġm im +Ġpast e +ĠK och +Ġignor ance +ĠSh oot +Ġbas ement +Un ited +ĠAd vis +he ight +Ġf oster +Ġdet ain +in formation +Ġne ural +' ; +Ġprov es +all ery +Ġinv itation +um bers +Ġc attle +Ġbicy cle +z i +Ġconsult ant +Ġap ology +ĠT iger +Ġ12 3 +99 9 +Ġind ividually +r t +ig ion +ĠBrazil ian +Ġdist urb +Ġentreprene urs +Ġfore sts +cer pt +pl ates +p her +clip se +Ġtw itter +Ġac ids +ograph ical +h um +ĠB ald +if ully +Ġcomp iler +ĠD A +Ġdon or +as i +Ġtrib al +l ash +ĠCon fig +Ġapplic ants +Ġsal aries +13 5 +Put in +ĠF ocus +ir s +Ġmisc onduct +ĠH az +Ġeat en +M obile +Mus lim +ĠMar cus +v iol +Ġfavor able +Ġst ub +ad in +ĠH ob +Ġfaith ful +Ġelectron ics +Ġvac uum +w ait +back ed +econom ic +d ist +Ġten ure +Ġsince re +ĠT ogether +ĠW ave +Ġprog ression +Ġden ying +Ġdist ress +br aska +th ird +Ġmix ing +Ġcolon ial +Ġpriv ately +Ġun rest +atern ity +Ġprem ises +ant i +greg ation +Ġlic ence +ĠH ind +ĠSam uel +Ġconvinc ing +ĠA ce +ĠR ust +ĠNet anyahu +Ġhand les +ĠP atch +orient ed +ah o +ĠG onz +Ġhack ers +claim er +Ġcustom s +ĠGr an +f ighters +Ġl uc +Ġman uscript +aren thood +Ġdev il +Ġwar riors +Ġoff enders +Will iam +Ġhol idays +Ġnight mare +Ġle ver +iff erent +St at +Ġexhib ition +put ed +ĠP ure +Ġal pha +Ġenthus iasm +ĠRepresent atives +E AR +ĠT yp +Ġwhe at +ĠAl f +Ġcor rection +Ġev angel +AT T +M iss +Ġs oup +Ġimpl ied +par am +Ġsex y +ĠL ux +Ġrep ublic +p atch +ab lish +Ġic ons +Ġfather s +ĠG ET +ĠCar ib +Ġregul ated +ĠCo hen +ĠBob by +Ġn er +Ġb ent +vent ory +ĠAl ong +ĠE ST +ĠWall ace +Ġmurd ers +r ise +ke ll +ĠCommon wealth +Ġn asty +et a +ĠM IT +Ġadminist ered +Ġgenuine ly +Ed itor +n ick +Ġhyd ro +**************** **************** +ĠB le +Ġfin es +Ġg orge +aus ible +r h +Ġapp le +ment ioned +Ġro pe +ot yp +H R +Ġdisappoint ing +Ġc age +n ik +Ġdoub ts +ĠF REE +print s +ĠM UST +Ġvend ors +ĠIn qu +Ġliber als +Ġcontract or +Ġup side +child ren +Ġtrick y +Ġregul ators +charg ed +l iter +Ġ *** +Ġreb ell +l ang +Ġloc als +Ġphys icians +Ġhe y +ar se +t m +ĠLe x +Ġbehavior al +success ful +F X +Ġbr ick +ov ic +Ġcon form +Ġreview ing +Ġins ights +Ġbi ology +ĠRem ove +ĠExt ra +Ġcomm itting +indu ced +ignt y +ig m +Ġat omic +Comm on +ĠE M +ĠP ere +ĠIt ems +e h +Ġpres erved +ĠH ood +Ġprison er +Ġbankrupt cy +Ġg ren +us hes +Ġexplo itation +Ġsign atures +Ġfin an +] ," +ĠM R +Ġme g +rem lin +Ġmusic ians +Ġselect ing +Ġexam ining +IN K +l ated +H i +Ġart ic +Ġp ets +Ġimp air +ĠM AN +Ġtable ts +in clude +R ange +Ġca ut +Ġlog s +Ġmount ing +Ġun aware +Ġdynam ics +ĠPalest ine +ĠQu arter +ĠPur ple +Ġm a +ĠIm port +Ġcollect ions +ci ation +Ġsuccess or +Ġcl one +Ġaim ing +Ġposs essed +Ġstick ing +Ġsh aking +Ġloc ate +ĠH ockey +T urn +17 0 +Ġfif teen +ĠHar rison +Ġcontinu ously +ĠT C +ĠVal ent +ĠRes cue +Ġby pass +am ount +Ġm ast +Ġprotect s +Ġart istic +Ġsomet ime +Ġsh oe +Ġshout ed +ific ant +et itive +ĠReg ister +ĠJ in +Ġconcent rated +ling ton +on ies +Ġgener ator +yr im +ĠAr men +Ġclear ing +id o +ĠT W +al ph +Ġlad ies +H ard +Ġdial og +Ġinput s +æ ľ +Ġpos es +Ġsl ots +ĠPrem ium +Ġle aks +Ġboss es +Ġ11 3 +c ourse +A cc +ĠNew ton +ĠAust ria +ĠM age +Ġte aches +ab ad +Ġwe ars +Ġc yl +Ġcur se +ĠS ales +ĠW ings +Ġp sy +Ġg aps +ĠIce land +ĠP interest +Ġland lord +Ġdefin itions +ĠK er +Ġsufficient ly +ĠP ence +ĠArch itect +Ġsur pass +Ġ11 4 +Ġsuper hero +ĠDise ase +Ġpri ests +ĠC ulture +Ġdefin itive +Ġsecret ly +ĠD ance +inst all +ch ief +ĠJess ica +W ould +Up dated +Ġlock er +ĠK ay +Ġmem orial +è ¦ +f at +Ġdis gu +Ġflav ors +ĠBase ball +ĠRes istance +Ġk icks +Ġen v +Ġteen agers +D ark +ĠC AR +Ġh alt +ĠL G +ĠGab riel +Ġfe ver +Ġs atur +Ġm all +Ġaffili ate +ĠS leep +ĠSpe cific +ĠV el +Ġj ar +ĠSac red +ĠEd wards +ĠA CL +Ġret ained +ĠG iant +Ġlim itation +in ces +Ġref usal +ĠT ale +ĠBut ler +Ġacc idents +ĠC SS +Ġimport ed +ĠCop y +Î ± +ER T +z el +Ġdiv isions +h ots +ĠAl b +ĠD S +Load er +W ashington +at isf +ĠCreat ive +\ . +ĠAut om +red ict +Ġrecept or +ĠCarl os +Met hod +ok a +Ġmal icious +Ġste pping +, [ +ĠD ad +Ġatt raction +ĠEffect s +ĠPir ate +ĠC er +ĠIndust ry +ĠR ud +Ġchar ter +Ġd ining +Ġins ists +Ġconfig ure +Ġ( # +ĠSim ple +ĠSc roll +UT C +17 5 +ĠK on +Ġmarket place +Ġ ãĤ +Ġref res +Ġg ates +er red +ĠP od +Ġbeh ave +Fr ank +n ode +Ġendors ed +he tt +as ive +ĠHom eland +Ġr ides +ĠLe ave +er ness +Ġflood ing +A FP +Ġris en +Ġcontin ually +Ġun anim +ĠCont ract +ĠP as +Ġgu ided +ĠCh ile +b d +Ġsu cc +pt ic +Ġcomm ittees +ĠL uther +ĠAny one +Ġs ab +12 4 +Ġp ixel +ĠB ak +ĠT ag +ĠBenn ett +En ter +sm all +ĠPresident ial +Ġp ul +Ġcontr ace +arch ive +Ġcoast al +ĠK ids +19 2 +âĢ ² +ick y +ING TON +Ġw olf +ĠSt alin +T ur +id get +am as +ĠUn less +Ġspons or +Ġmor ph +ĠCho ose +Ġrun ner +Ġun bel +Ġm ud +ĠMan a +Ġdub bed +Ġg odd +ure rs +wind ow +Ġrel ied +Ġcelebr ating +os c +Ġ13 5 +Ġlobb ying +Ġincom plete +Ġrestrict ion +Ġinc ap +it us +Ġexpect ation +ĠAp ollo +Ġint ens +Ġsyn c +G H +Ġmanip ulation +B Y +Ġspe ar +Ġbre asts +Ġvol can +il ia +M aterial +Ġform ats +ĠB ast +Ġparliament ary +Ġsn ake +Ġserv ants +ĠTr udeau +ĠGr im +ĠArab ic +ĠSC P +ĠBoy s +st ation +Ġprospect ive +ord e +in itialized +Ġb ored +AB LE +Ġaccess ed +Ġtax i +ĠShe ll +aid en +urs ed +in ates +ĠIns urance +ĠPet e +Sept ember +6 50 +Ġad ventures +ĠCo ver +Ġt ribute +Ġsk etch +Ġem power +Ġ Ø +ĠGl enn +ĠD aw += \" +ĠPolit ics +Ġgu ides +Ġd ioxide +ĠG ore +ĠBr ight +ĠS ierra +Ġval ued +c ond +Ġpo inter +Se lect +Ġrisk y +Ġabsor b +im ages +Ġref uses +Ġbon uses +__ _ +Ġh ilar +ĠF eatures +2 20 +ĠCollect or +F oot +Ġ19 64 +cul us +Ġd awn +Ġwork out +ĠL O +Ġphilosoph ical +ĠSand y +ĠYou th +Ġl iable +A f +bl ue +Ġovert urn +less ness +ĠTrib une +ĠIn g +Ġfact ories +Ġcat ches +Ġpr one +Ġmat rix +Ġlog in +Ġin acc +Ġex ert +s ys +Ġneed le +ĠQ ur +Ġnot ified +ould er +t x +Ġremind s +Ġpublisher s +Ġn ort +Ġg it +Ġfl ies +ĠEm ily +Ġflow ing +ĠAl ien +ĠStr ateg +Ġhard est +Ġmod ification +AP I +ĠM Y +Ġcr ashes +st airs +n umber +Ġur ging +ch annel +ĠFal con +Ġinhabit ants +Ġterr ifying +Ġutil ize +Ġban ner +Ġcig arettes +Ġsens es +ĠHol mes +Ġpract ition +ĠPhill ips +ott o +Ġcomp ile +Mod el +ĠK o +Ġ[ ] +Americ ans +ĠTer ms +Ġmed ications +ĠAn a +Ġfundament ally +ĠNot ice +Ġwe aker +Ġ 0000 +Ġgar lic +Ġout break +Ġeconom ist +ĠB irth +Ġobst acles +ar cer +ĠOr thodox +Ġplace bo +ĠC rew +asp berry +ĠAng els +Ġdis charge +Ġdestruct ive +11 7 +ĠR ising +Ġd airy +l ate +Ġcoll ision +ĠTig ers +ean or +ocument ed +ĠIn valid +Ġd ont +ĠL iter +ĠV a +Ġhyd rogen +Ġvari ants +ĠBrown s +Ġ19 65 +Ġind igenous +Ġtrad es +Ġremain der +Ġswe pt +ĠImp act +Ġred ist +Ġun int +grad uate +ãĥ ķ +ĠW ILL +ãģ® ç +ĠCrit ical +Ġf isher +Ġv icious +Ġrevers ed +Y ear +ĠS ox +Ġshoot ings +Ġfil ming +Ġtouchdown s +ai res +m el +Ġgrand father +Ġaffect ion +ing le +Ġover ly +Add itional +Ġsup reme +ĠGr ad +Ġsport ing +Ġmer cy +ĠBrook s +ount y +Ġperform s +Ġtight ly +Ġdem ons +Ġkill ings +Ġfact ion +ĠNov a +aut s +Ġund oubtedly +ar in +Ġunder way +ra k +Ġl iv +ĠReg ion +Ġbrief ing +s ers +cl oud +ĠM ik +us p +Ġpred iction +az or +Ġport able +ĠG and +Ġpresent ing +Ġ10 80 + » +ush i +ĠSp ark +there um +Ġjust ification +ĠN y +Ġcontract ors +ming ham +ĠSt yle +å ħ +ĠChron icles +ĠPict ure +Ġprov ing +Ġw ives +set t +Ġmole cules +ĠFair y +Ġconsist ing +Ġp ier +al one +in ition +Ġn ucle +j son +Ġg otta +Ġmob il +Ġver bal +ar ium +Ġmon ument +uck ed +Ġ25 6 +T ech +mine craft +ĠTr ack +Ġt ile +Ġcompat ibility +as is +Ġs add +Ġinstruct ed +ĠM ueller +Ġle thal +Ġhorm one +Ġor che +el se +Ġske let +Ġentert aining +Ġminim ize +ag ain +Ġunder go +Ġconst raints +Ġcig arette +ĠIslam ist +Ġtravel s +ĠPant hers +l ings +C are +Ġlaw suits +ur as +Ġcry st +Ġlow ered +Ġaer ial +Ġcomb inations +Ġha un +Ġch a +Ġv ine +Ġquant ities +Ġlink ing +b ank +Ġso y +B ill +ĠAngel a +Ġrecip ient +ĠProt est +Ġs ocket +Ġsolid arity +Ġâ Ĩ +m ill +Ġvar ies +ĠPak istani +Dr agon +Ġun e +Ġhor izon +³³³³ ³³³³ +Ġprov inces +Ġfrank ly +Ġenact ed +not es +[ ' +Ġ19 2 +ocr acy +Ġendorse ment +Ġover time +Tr ue +L ab +lic ted +ĠD NC +Ġbe ats +ĠJam ie +15 2 +ĠIN T +Cont act +Ġaccount ed +h ash +ĠPack ers +p ires +Ġles bian +Ġamend ments +Ġhop eful +ĠFin land +Ġspot light +Ġconfig ured +Ġtrou bled +Ġg aze +ĠCal gary +Ġrel iability +Ġins urg +sw er +b uy +ĠSk in +Ġp ixels +Ġhand gun +Ġpar as +Ġcateg or +ĠE L +ĠRe x +Ind eed +Ġkind a +Ġconj unction +ĠBry an +ĠMan ufact +y ang +Pl us +S QL +ish ment +Ġdom inate +Ġn ail +Ġo ath +Ġeru pt +ĠF ine +it bart +ĠCh ip +ĠAb d +ĠN am +Ġbuy er +Ġdiss ent +Le aks +Cont in +Ġr ider +ĠSome one +Ġill usion +c in +ĠBoe ing +Ġin adequ +ov ation +i ants +Ġreb uild +4 50 +ĠDest iny +S W +ĠT ill +H it +ia z +ĠBang l +acher s +ĠRe form +Ġse gments +Ġsystem atic +d c +ĠConserv atives +Ġport al +h or +ĠDragon bound +Ġdrag ged +om o +Ġthe e +ad vert +ĠRep orts +ĠE t +Ġbarrel s +Aug ust +Ġcompar isons +Ġhe x +Ġan throp +" [ +bor ough +ab i +Ġpict ured +play ing +ĠAdd ress +ĠMir ror +Sm ith +Ġt ires +ĠN PR +AA AA +Ġclass ification +ĠTh an +ĠH arm +ĠR A +Ġreject ion +min ation +Ġr anged +ĠF alls +D I +H ost +ãĤ ´ +ĠEx ample +list ed +th irds +Ġsaf egu +br and +Ġprob able +Can ada +IT ION +ĠQ aeda +Ġch ick +Ġimport s +h it +l oc +W W +Ġble w +Ġany time +Ġwh oles +ik ed +Ġcal culation +cre ate +ĠO ri +Ġupgr aded +Ġapp ar +ut ory +ĠM ol +B rit +ĠJ ong +IN AL +ĠStart ing +Ġd ice +urt le +Ġre lying +cl osure +Ġprof itable +Ġsl aughter +ĠMan ual +c aster +Ġ" $ +Ġfe ather +ĠSim ply +ie ves +Ġdeter ior +ĠPC I +Ġst amp +Ġfl aws +Ġsh ade +ham mer +Ġpass port +Ġcont ing +am el +Ġobser vers +Ġneg lect +ĠR B +ĠBrother hood +Ġskept ical +f amily +us k +Ġemotion ally +â Ļ +ĠBet a +ason able +id ity +ĠM ul +Ġkick ing +ĠC arm +oll ah +VERT IS +ĠAt hen +Ġlad der +ĠBul let +å £ +00 01 +ĠWild life +ĠM ask +ĠN an +R ev +Ġun acceptable +leg al +Ġcrowd ed +ag i +ĠC ox +j e +Ġmor ality +Ġfu els +Ġc ables +Ġman kind +ĠCarib bean +Ġanch or +Ġby te +ĠO ften +ĠO z +Ġcraft ed +Ġhistor ian +ĠW u +Ġtow ers +ĠCitiz ens +Ġhel m +Ġcred entials +Ġsing ular +ĠJes se +Ġtack les +Ġcont empt +Ġa fore +ĠSh adows +Ġn il +Ġur gent +app le +bl ood +Ġv on +Ġoff line +Ġbreat he +Ġj umps +Ġirre levant +ox ic +om al +import ant +J im +Ġgl oves +arm ing +dep th +Ġtal ents +ook ie +ĠS B +Ġpal m +uff s +est a +IG H +Ġcan on +ĠVer izon +ĠP le +Ġcou pled +vel t +Ġfundra ising +ĠGet ting +ĠD LC +Ġmathemat ical +ĠH S +ĠCard inals +te lling +Ġspons ors +Ġ Ï +ĠBull s +op tion +Ġprop ose +Ġmem orable +Ġembr aced +Ġdecl ining +He alth +ed a +Ġ} ; +Ġsp am +m ile +Ġpit cher +ĠE ight +Ġcar ing +ut ic +ro le +Ġair line +ernand ez +ĠAth let +Ġcert ification +ux e +rig er +Ġem pir +Ġsens ation +Ġdis m +Ġb olt +Ġev olve +H ouse +Ġconsult ation +ĠD uty +Ġtou ches +ĠN athan +Ġf aint +h ad +" ( +ĠCons umer +ĠExt reme +Ġ12 7 +ĠHer m +ĠSac rament +iz oph +Ġanx ious +ul ously +Ġsoc ially +ĠU TC +Ġsol ving +ĠLet ter +Hist ory +ed uc +Pr ice +) ); +Ġrel oad +am ic +Ġp ork +Ġdisc ourse +Ġt ournaments +ai ro +ĠK ur +ĠCost a +Ġviol ating +Ġinterf ere +Ġrecre ational +uff le +Ġspe eches +Ġneed ing +Ġremem bers +Ġcred ited +n ia +f ocused +amer a +Ġb ru +um bs +ĠCub an +Ġpreced ing +Ġnons ense +ac ial +Ġsmart phones +ĠSt ories +S ports +ĠEmer gency +oun cing +ef ined +Ġb er +Ġconsult ing +Ġm asters +he astern +." [ +ĠRun ning +Ġsus cept +ĠF eng +Americ a +pr ises +st itial +ĠWeek ly +ĠGreat er +mod ules +if ter +G raphics +ul er +Ġwho lly +Ġsupp ress +Ġconce aled +Ġhapp ily +Ġaccept s +ĠEn joy +Ġr ivers +ĠEx cept +2 25 +ĠN HS +ĠMc Connell +Ġp ussy +fer red +ut able +Ġatt ain +Ġ> = +Ġdepos its +roph ic +Ġnot orious +ĠSh aw +il itation +Ġepid emic +all ic +Ġsmall est +ov ich +Ġaccess ories +per ties +Ġsur plus +ĠMe ch +Ġamb ig +ĠImm igration +Ġch im +ev al +Ġpract icing +ĠMyster y +Ġdom ains +ĠSil icon +app s +Ġkilomet ers +e a +ĠSm ash +Ġwarrant y +Ġn ost +s il +re v +J on +ĠDub lin +Ġtast es +Ġb out +g reat +er ror +Ġsw itches +ĠB apt +D O +ok i +Ġsour ced +pro du +Ġattach ment +ĠIss ue +ĠQuest ion +Jo in +Ġf itted +Ġunlaw ful +^ ^ +ere k +Ġauthent ication +Ġst ole +Ġaccount ability +l abel +S earch +Ġal beit +atic an +fund ed +ĠAdd ing +ĠI Q +Ġsub mar +l it +a que +ĠLear ning +Ġint eger +M aster +ĠCh rom +Ġprem ier +O p +ĠLi u +Ġbl essed +ĠGl obe +ĠResp onse +Ġlegit im +ĠMer kel +Ġdispos al + ´ +Ġgau ge +pe at +Ġindu ced +Ġquestion able +arth y +ĠV it +ĠF eed +U ntil +U t +worth y +R Y +ĠH erald +ĠHam mer +Ġmed al +ĠR ivers +ĠH ack +Ġclar ify +Ġtrack ed +Ġautonom ous +Ġten ant +ĠQ atar +er ie +Ġgr im +ĠMon itor +Ġresist ant +ĠSpe c +ĠWell s +N AS +14 8 +Ġmin ers +iot ics +Ġmiss es +11 6 +g ian +g it +ĠE yes +p res +Ġgrad uated +Ġang el +Ġsyn chron +Ġefficient ly +Ġtrans mitted +H arry +Ġglob ally +EN CE +ĠMont ana +r aged +ĠPre vention +Ġp iss +ĠL l +Ġshe lf +ĠB JP +ĠTest ament +ĠL ate +ik er +ĠH app +ĠJul ian +h all +Ġsp ont +Ġshut down +Ġincons istent +Ġsubscrib ers +Ġske leton +ĠNe braska +Ġins pire +ĠV oid +F eed +Ġang les +ĠSpr ings +Ġbench mark +Ġvacc ines +izoph ren +se xual +uff ed +Ġsh ine +ĠK ath +Ġgest ure +ine a +Ġr ip +Ġopp ression +Ġcons cience +b t +ĠL um +Ġinc idence +ĠF a +w r +Ġmin eral +ĠSp urs +alk y +Ġth under +Ġop io +Be ing +ĠPal m +Ġwas ted +Ġl b +i aries +ĠIniti ative +Ġcur ric +Ġmark er +ĠMc L +Ġext ensions +ĠP v +ĠAr ms +Ġoffer ings +Ġdef enses +Ġvend or +Ġcontrad ict +ĠCol in +Ġredd it +Ġper ipher +12 2 +Ġs ins +E dit +IC T +So ft +ĠSh ah +Ġadministr ator +ĠT rip +Ġporn ography +Ġtu ition +in ence +ĠPro gress +Ġcat alog +Ġsu ite +Ġh ike +Ġreprodu ctive +eng ine +Ġd rought +ĠNo ah +Ġ2 30 +Ġd ude +Ġrelax ed +Ġpart ition +Ġparticip ant +Ġtel esc +Ġfe as +ĠF F +own er +Ġswe eping +Ġl enses +Ġmatch up +ĠRe pl +ourn als +Ġcred ible +Ġgrand mother +Ġther mal +Ġsubscrib ing +Ġident ities +col m +U CT +Ġreluct ant +us ers +ĠC ort +Ġassist ed +OS S +ATION S +IS H +Ġpharm aceutical +ic able +ad ian +ĠSon ic +ĠF ury +ĠM ong +A H +ĠPsych ology +Ġph osph +Ġtreat s +Ń Ķ +Ġstead ily +ĠHell o +Ġrel ates +Ġcl ue +Ex pl +a uth +Ġrev ision +Ġe ld +os ion +Ġbr on +14 4 +ri kes +Ġmin es +Ġblank et +ĠF ail +el ed +ĠIm agine +ĠPl anned +a ic +Re quest +M ad +ĠHor se +ĠEag le +Ġcap ac +15 7 +Ġl ing +ĠN ice +ĠP arenthood +min ster +og s +ens itive +Not hing +Ġcar n +F in +ĠP E +Ġr ifles +ĠL P +S and +Ġgui Active +Ġtour ist +C NN +Ġunve iled +Ġpredec essor +} { +u ber +Ġoff shore +Ġopt ical +ĠR ot +ĠPear l +et on +Ġst ared +Ġfart her +at ility +cont in +ĠG y +ĠF oster +ĠC oc +ri ents +Ġdesign ing +ĠEconom y +ON G +W omen +ĠN ancy +er ver +Ġmas cul +Ġcasual ties +Ġ2 25 +ĠS ullivan +ĠCh oice +Ġa ster +w s +Ġhot els +Ġconsider ations +Ġcou ch +ĠSt rip +ĠG n +Ġmanip ulate +l ied +Ġsynt hetic +Ġassault ed +Ġoff enses +ĠDra ke +Ġim pe +Oct ober +ĠHer itage +h l +ĠBl air +Un like +Ġg rief +Ġ4 50 +Ġopt ed +Ġresign ation +il o +Ġver se +ĠT omb +Ġu pt +Ġa ired +ĠH ook +ĠML B +Ġassum es +out ed +ĠV ers +Ġinfer ior +Ġbund le +ĠD NS +ograp her +Ġmult ip +ĠSoul s +Ġillust rated +Ġtact ic +Ġdress ing +Ġdu o +Con f +Ġrel ent +Ġc ant +Ġscar ce +Ġcand y +ĠC F +Ġaffili ated +Ġspr int +yl an +ĠGarc ia +Ġj unk +Pr int +ex ec +C rit +Ġport rait +ir ies +ĠOF F +Ġdisp utes +W R +L ove +ãģ Ħ +ĠRe yn +Ġh ipp +op ath +Ġflo ors +ĠFe el +Ġwor ries +Ġsett lements +ĠP os +Ġmos que +Ġfin als +Ġcr ushed +ĠPro bably +ĠB ot +ĠM ans +ĠPer iod +Ġsovere ignty +Ġsell er +Ġap ost +Ġam ateur +Ġd orm +Ġconsum ing +Ġarm our +ĠRo ose +Ġint ensive +Ġelim inating +ĠSun ni +ĠAle ppo +j in +Ġadv ise +p al +ĠH alo +Ġdes cent +Ġsimpl er +Ġbo oth +ST R +L ater +ĠC ave +== = +Ġm ol +Ġf ist +Ġshot gun +su pp +Ġrob bery +E ffect +Ġobsc ure +ĠProf essional +Ġemb assy +Ġmilit ant +Ġinc arcer +Ġgener ates +Ġlaun ches +Ġadministr ators +Ġsh aft +Ġcirc ular +Ġfresh man +ĠW es +ĠJo el +ĠD rew +ĠDun can +ĠApp arently +s ight +ĠIntern al +ĠInd ividual +ĠF E +Ġb ore +ĠM t +Ġbroad ly +ĠO ptions +ount ain +ip es +ĠV ideos +20 4 +Ġh ills +Ġsim ulation +Ġdisappoint ment +it an +ĠLabor atory +Ġup ward +Ġbound ary +Ġdark er +h art +Ġdomin ance +C ong +ĠOr acle +ĠL ords +Ġscholars hip +ĠVin cent +ed e +ĠR ah +Ġencour ages +ro v +Ġqu o +Ġprem ise +ĠCris is +ĠHol ocaust +Ġrhyth m +Ġmet ric +cl ub +Ġtransport ed +Ġn od +ĠP ist +Ġancest ors +ĠFred er +th umbnails +ĠC E +ON D +Ph il +ven ge +ĠProduct s +cast le +Ġqual ifying +ĠK aren +VERTIS EMENT +Ġmight y +Ġexplan ations +Ġfix ing +D i +Ġdecl aring +Ġanonym ity +Ġju ven +ĠN ord +ĠDo om +ĠAct ually +O k +ph is +ĠDes ert +Ġ11 6 +I K +ĠF M +Ġinc omes +V EL +ok ers +Ġpe cul +Ġlight weight +g ue +Ġacc ent +Ġincre ment +ĠCh an +Ġcompl aining +ĠB aghd +Ġmidfield er +Ġover haul +Pro cess +ĠH ollow +ĠTit ans +Sm all +man uel +ĠUn ity +ĠEv ents +S ty +Ġdispro portion +n esty +en es +ĠC od +Ġdemonstr ations +ĠCrim son +ĠO H +Ġen rolled +Ġc el +ĠBre tt +Ġa ide +Ġhe els +Ġbroad band +Ġmark ing +Ġw izard +ĠN J +ĠChief s +Ġingred ient +Ġd ug +ĠSh ut +urch ase +end or +Ġfar mer +ĠGold man +12 9 +15 5 +Or der +Ġl ion +i ably +Ġst ain +ar ray +ilit ary +ĠFA Q +Ġexpl oded +ĠMcC arthy +ĠT weet +ĠG reens +ek ing +l n +ens en +Ġmotor cycle +Ġpartic le +Ġch olesterol +B ron +Ġst air +Ġox id +Ġdes irable +ib les +Ġthe or +for cing +Ġpromot ional +ov o +b oot +ĠBon us +raw ling +Ġshort age +ĠP sy +Ġrecru ited +Ġinf ants +Ġtest osterone +Ġded uct +Ġdistinct ive +Ġfirm ware +bu ilt +14 5 +Ġexpl ored +Ġfact ions +Ġv ide +Ġtatt oo +Ġfinan cially +Ġfat igue +Ġproceed ing +const itutional +Ġmis er +Ġch airs +gg ing +ipp le +Ġd ent +Ġdis reg +ç Ķ +st ant +ll o +b ps +aken ing +Ġab normal +ĠE RA +å£ « +ĠH BO +ĠM AR +Ġcon cess +Ġserv ant +Ġas pir +l av +ĠPan el +am o +Ġprec ip +Ġrecord ings +Ġproceed ed +Ġcol ony +ĠT ang +ab lo +Ġstri pped +Le ft +to o +Ġpot atoes +Ġfin est +% ). +Ġc rap +ĠZ ach +ab ases +ĠG oth +Ġbillion aire +w olf +Ġsan ction +S K +Ġlog ged +P o +ey ed +un al +Ġcr icket +Ġarm ies +Ġunc overed +Cl oud +ó n +Ġreb ounds +Ġm es +O per +P ac +Ġnation ally +Ġinsert ed +p ict +Ġgovern ance +Ð ¸ +Ġprivile ges +G ET +Ġfavor ites +im ity +Ġlo ver +the m +em pl +Ġgorge ous +An n +Ġsl ipped +Ġve to +B ob +Ġsl im +u cc +ĠF ame +udden ly +Ġden ies +ĠM aur +Ġdist ances +Ġw anna +t ar +ĠS ER +Ġâ Ī +Ġle mon +at hetic +Ġlit eral +Ġdistingu ished +Ġansw ering +G I +Ġrelig ions +ĠPhil os +ĠL ay +Ġcomp os +ire ments +ĠK os +ine z +roll ing +Ġyoung est +and ise +ĠB orn +Ġalt ar +am ina +ĠB oot +v oc +Ġdig ging +Ġpress ures +Ġl en +26 4 +Ġassass ination +ĠBir mingham +ĠMy th +Ġsovere ign +ĠArt ist +ĠPhot ograph +Ġdep icted +Ġdisp ens +orth y +Ġamb ul +int eg +ĠC ele +ĠTib et +Ġhier archy +Ġc u +Ġpre season +ĠPet erson +Ġcol ours +Ġworry ing +Ġback ers +ĠPal mer +ĠÎ ¼ +Ġcontribut or +Ġhear ings +Ġur ine +Ġ Ù +ourge ois +Sim ilar +ĠZ immer +s omething +ĠUS C +Ġstrength s +ĠF I +Ġlog ging +As ked +ĠTh ai +in qu +ĠW alt +Ġcrew s +it ism +3 01 +Ġshar ply +um ed +Ġred irect +r ators +In f +ĠWe apons +Ġte asp +19 99 +L ive +ĠEs pecially +ĠS ter +ĠVeter ans +Ġint ro +other apy +Ġmal ware +Ġbre eding +Ġmole cular +ĠR oute +ĠCom ment +oc hem +Ġa in +Se ason +Ġlineback er +Ä « +ĠEconom ics +es ar +ĠL ives +ĠEm ma +Ġk in +ĠTer rit +Ġpl anted +ot on +ĠBut ter +ĠSp ons +P ER +Ġdun geon +Ġsymb olic +Ġfil med +Ġdi ets +Ġconclud es +Ġcertain ty +ĠForm at +Ġstr angers +form at +ĠPh ase +Ġcop ied +Ġmet res +ld a +ĠUs ers +Ġdeliber ate +Ġwas hed +ĠL ance +im ation +Ġimpro per +ĠGen esis +ick r +ĠK ush +Ġreal ise +Ġembarrass ing +alk ing +b ucks +Ġver ified +Ġout line +year s +ĠIn come +20 2 +Ġz ombies +F inal +ĠMill enn +Ġmod ifications +ĠV ision +ĠM oses +ver b +iter ranean +ĠJ et +Ġnav al +ĠA gg +Ġur l +Ġvict ories +Ġnon etheless +Ġinj ust +ĠF act +ç ļ +Ġins ufficient +re view +face book +Ġnegoti ating +Ġguarant ees +im en +uten berg +Ġg ambling +Ġcon gr +Load ing +Ġnever theless +Ġpres idents +ĠIndust rial +Ġ11 8 +Ġp oured +ĠT ory +Ġ17 5 +Ġ: = +Sc ott +ange red +T ok +Ġorgan izers +M at +ĠG rowth +Ġad ul +Ġens ures +Ġ11 7 +é¾į å +Ġmass acre +Ġgr ades +be fore +AD VERTISEMENT +ĠSl ow +ĠM MA +âĢĶ " +ĠV atican +Q aeda +Ġo we +66 66 +ĠS orry +ĠGr ass +Ġbackground s +Ġexha usted +Ġcl an +Ġcomprom ised +ĠE lf +ĠIsa ac +ens on +In vest +IF A +Ġinterrupt ed +ãĥī ãĥ© +Ġtw isted +ĠDrag ons +M ode +ĠK remlin +Ġfert il +he res +ph an +ĠN ode +f ed +ĠOr c +Ġunw illing +C ent +Ġprior it +Ġgrad uates +Ġsubject ive +Ġiss uing +ĠL t +Ġview er +Ġw oke +Th us +bro ok +Ġdep ressed +Ġbr acket +ĠG or +ĠFight ing +Ġstri ker +Rep ort +ĠPortug al +Ġne o +w ed +19 9 +Ġflee ing +sh adow +ident ified +US E +Ste am +Ġstret ched +Ġrevel ations +art ed +ĠD w +Ġalign ment +est on +ĠJ ared +S ep +Ġblog s +up date +g om +r isk +Ġcl ash +ĠH our +Ġrun time +Ġunw anted +Ġsc am +Ġr ack +Ġen light +on est +ĠF err +Ġconv ictions +Ġp iano +Ġcirc ulation +ĠW elcome +Ġback lash +ĠW ade +Ġrece ivers +ot ive +J eff +Ġnetwork ing +ĠPre p +ĠExpl orer +Ġlect ure +Ġupload ed +ĠMe at +B LE +ĠNaz is +ĠSy nd +st ud +ro ots +ri ans +Ġportray ed +Ġ ?? +ĠBudd ha +s un +Rober t +ĠCom plex +Ġover see +Ġste alth +T itle +ĠJ obs +ĠK um +Ġappreci ation +ĠM OD +Ġbas ics +Ġcl ips +Ġnurs ing +Ġpropos ition +Ġreal ised +ĠNY C +Ġall ocated +ri um +ar an +ĠPro duction +ĠV ote +Ġsm ugg +Ġhun ter +az er +ĠCh anges +Ġfl uct +y on +Ar ray +Ġk its +W ater +Ġuncom mon +Ġrest ing +ell s +w ould +Ġpurs ued +Ġassert ion +omet own +ĠMos ul +ĠPl atform +io let +Ġshare holders +Ġtra ils +P ay +ĠEn forcement +ty pes +ĠAn onymous +Ġsatisf ying +il ogy +Ġ( ' +w ave +c ity +Ste ve +Ġconfront ation +ĠE ld +C apt +ah an +ht m +ĠC trl +ON S +2 30 +if a +hold ing +Ġdelic ate +Ġj aw +ĠGo ing +or um +S al +Ġd ull +ĠB eth +Ġpr isons +Ġe go +ĠEl sa +avor ite +ĠG ang +ĠN uclear +Ġsp ider +ats u +Ġsam pling +Ġabsor bed +ĠPh arm +iet h +Ġbuck et +ĠRec omm +O F +ĠF actory +AN CE +Ġb acter +H as +ĠObs erv +12 1 +Ġprem iere +De velop +Ġcur rencies +C ast +Ġaccompany ing +ĠNash ville +Ġfat ty +ĠBre nd +Ġloc ks +Ġcent ered +ĠU T +augh s +or ie +ĠAff ordable +v ance +D L +em et +Ġthr one +ĠBlu etooth +Ġn aming +if ts +AD E +Ġcorrect ed +Ġprompt ly +ĠST R +Ġgen ome +Ġcop e +Ġval ley +Ġround ed +ĠK end +al ion +p ers +Ġtour ism +Ġst ark +v l +Ġblow ing +ĠSche dule +st d +Ġunh appy +Ġlit igation +ced es +Ġand roid +Ġinteg ral +ere rs +ud ed +t ax +Ġre iter +ĠMot ors +oci ated +Ġwond ers +ĠAp ost +uck ing +ĠRoose velt +f ram +Ġyield s +Ġconstit utes +aw k +Int erest +Ġinter im +Ġbreak through +ĠC her +Ġpro sec +ĠD j +ĠM T +Res p +ĠP T +Ġs perm +ed it +B T +Lin ux +count ry +le ague +Ġd ick +Ġo ct +Ġinsert ing +Ġsc ra +ĠBrew ing +Ġ19 66 +Ġrun ners +Ġpl un +id y +ĠD ian +Ġdys function +Ġex clusion +Ġdis gr +Ġincorpor ate +Ġrecon c +Ġnom inated +ĠAr cher +d raw +achel or +Ġwrit ings +Ġshall ow +Ġh ast +ĠB MW +ĠR S +Ġth igh +Ġ19 63 +Ġl amb +Ġfav ored +ag le +Ġcool er +ĠH ours +ĠG U +ĠOrig in +Ġglim pse +---------------- ---- +L im +Ġche ek +Ġj ealous +- ' +Ġhar ness +ĠPo ison +Ġdis abilities +ne apolis +Ġout look +Ġnot ify +ĠIndian apolis +Ġab rupt +ns ic +Ġenc rypted +Ġfor fe +reat h +Ġr abb +Ġfound ations +Ġcompl iment +ĠInter view +ĠS we +Ġad olesc +Ġmon itors +ĠSacrament o +Ġtime ly +Ġcontem pl +Ġposition ed +Ġpost ers +ph ies +iov ascular +v oid +ĠFif th +Ġinvestig ative +OU N +Ġinteg rate +ĠIN C +ish a +ibl ings +ĠRe quest +ĠRodrig uez +Ġsl ides +ĠD X +Ġfemin ism +Ġdat as +Ġb end +ir us +ĠNig eria +F ox +Ch ange +Ġair plane +ĠLad en +Ġpublic ity +ixt y +Ġcommit ments +Ġaggreg ate +Ġdisplay ing +ĠAr row +Ġ12 2 +Ġrespect s +and roid +s ix +ĠSh a +Ġrest oration +) \ +W S +oy s +Ġillust rate +with out +12 6 +ĠâĶ Ĥ +Ġpick up +n els +Ġ .... +f ood +ĠF en +) ? +Ġphenomen a +Ġcompan ions +ĠW rite +Ġsp ill +Ġbr idges +ĠUp dated +ĠF o +Ġinsect s +ASH INGTON +Ġsc are +il tr +ĠZh ang +Ġsever ity +Ġind ul +14 9 +ĠCo ffee +Ġnorm s +Ġp ulse +ĠF T +Ġhorr ific +ĠDest roy +ĠJ SON +Ġo live +Ġdiscuss es +R est +E lect +ĠW inn +ĠSurv iv +ĠH ait +S ure +op ed +Ġro oted +ĠS ke +ĠBron ze +Ġl ol +Def ault +Ġcommod ity +red ited +Ġliber tarian +Ġforb idden +Ġgr an +à ¨ +Ġl ag +en z +dri ve +Ġmathemat ics +Ġw ires +Ġcrit ically +Ġcarb ohyd +ĠChance llor +ĠEd die +Ġban ning +ĠF ri +Ġcompl ications +et ric +ĠBangl adesh +Ġband width +St op +ĠOrig inally +Ġhalf way +yn asty +sh ine +Ġt ales +rit ies +av ier +Ġspin ning +ĠWH O +Ġneighbour hood +b ach +Ġcommer ce +ĠS le +B U +Ġentreprene ur +Ġpecul iar +ĠCom ments +f re +3 20 +IC S +Ġimag ery +ĠCan on +ĠElect ronic +sh ort +( ( +D ig +Ġcomm em +u ced +Ġincl ined +ĠSum mon +Ġcl iff +ĠMed iterranean +Ġpo etry +Ġprosper ity +ĠRe ce +Ġp ills +m ember +Ġfin ale +un c +ĠG ig +ä ½ +Ġl od +Ġback ward +- + +ĠFor ward +Ġth ri +s ure +Ġso ap +ĠF X +R ES +ĠSe xual +oul os +Ġfool ish +Ġright eous +Ġco ff +terror ism +ust ain +ot er +Ġab uses +ne xt +Ġab usive +Ġthere after +Ġprohib ition +ĠS UP +Ġd ip +Ġr ipped +Ġinher ited +Ġb ats +st ru +G T +Ġflaw ed +ph abet +Ġf og +do ors +Ġim aging +Ġdig its +ĠHung ary +Ġar rog +Ġteach ings +Ġprotocol s +ĠB anks +à ¸ +p ound +ĠC urt +." ) +. / +Ġex emption +end ix +ĠM ull +Ġimpro ves +ĠG amer +d imensional +I con +ĠMarg aret +St atus +d ates +Ġint ends +Ġdep ict +Ġpark ed +J oe +ĠMar ines +chn ology +! ). +Ġjud ged +Ġwe ights +R ay +Ġapart ments +he ster +Ġrein force +Ġoff ender +occ up +Ġs ore +e pt +ĠPH P +ĠB row +Ġauthor ization +ĠR isk +ĠDel aware +ĠQ U +Ġnot ifications +Ġsun light +Ġex clude +d at +Ġm esh +ĠSud an +Ġbelong ed +Ġsub way +Ġno on +ĠInter ior +ol ics +ĠL akers +Ġc oding +Dis claimer +Cal if +O ld +Ġdis l +???? ? +Ġconfir ms +Ġrecruit ment +Ġhom icide +Cons ider +ĠJeff rey +ft y +} ; +Ġobject ion +do ing +ĠLe o +W ant +Ġgl ow +ĠClar ke +ĠNorm an +Ġver ification +Ġpack et +ĠForm ula +Ġpl ag +es ville +Ġshout ing +Ġo v +ĠR EC +ĠB ub +Ġn inth +Ġener g +Ġvalid ity +Ġup s +j ack +Ġneighbor ing +ĠN ec +ew orks +ĠH ab +are z +Ġsp ine +Ġevent ual +ĠLe aders +ĠC arn +Ġprob ation +Ġrom ance +ms g +ĠMechan ical +ER Y +R ock +Ġpart isan +N ode +ass ets +min ent +Ġforeign ers +Ġtest ify +ĠUs ually +l ords +ĠG ren +ĠPow ell +BI L +Ġs r +Ġadd ict +Ġshell s +Ġs igh +ĠY ale +tern ity +Ġ7 50 +E U +ĠR ifle +Ġpat ron +em a +ĠB annon +an ity +Ġtrop ical +ĠV II +c ross +Every thing +ĠIS O +Ġhum ble +ass ing +ĠF IG +Ġupd ating +ys on +Ġcal cium +Ġcompet ent +Ġste ering +Pro t +ĠS Y +ĠFin als +ĠR ug +15 9 +13 7 +ĠG olf +Ġ12 6 +Ġaccommod ation +ĠHug hes +Ġaest hetic +art isan +ĠTw ilight +Ġpr ince +ĠAgric ulture +ĠDis co +Ġpreced ent +Ġtyp ing +author ized +O ption +ĠA ub +l ishes +ach t +m ag +P eter +ĠU FO +mont on +ĠL ith +Ġa rom +Ġsec uring +Ġconf ined +priv ate +Ġsw ords +Ġmark ers +Ġmetab olic +se lect +ĠCur se +ĠO t +g ressive +Ġinc umb +ĠS aga +Ġpr iced +Ġclear ance +Cont ent +Ġdr illing +Ġnot ices +Ġb ourgeois +Ġv est +Ġcook ie +ĠGuard ians +ry s +in yl +Ġ12 4 +Ġpl ausible +on gh +ĠOd in +Ġconcept ion +ĠY uk +ĠBaghd ad +ĠFl ag +Aust ral +ĠI BM +Ġintern ationally +ĠWiki Leaks +I ED +Ġc yn +Ġcho oses +ĠP ill +Ġcomb ining +Ġrad i +ĠMoh ammed +def ense +atch ing +Sub ject +ic iency +Fr ame +Ġ{ " +Ġche ss +Ġtim er +19 0 +Ġt in +Ġord inance +emet ery +Ġacc using +Ġnotice able +Ġcent res +Ġl id +ĠM ills +img ur +Ġz oom +erg ic +Ġcomp ression +pr im +f ind +Ġsur g +Ġp and +ĠK ee +ĠCh ad +cell ence +oy le +Ġsocial ism +ĠT ravis +ĠM Hz +Ġgu ild +ALL Y +ĠSub scribe +ĠRel ated +Ġoccur rence +itch ing +Ġfict ional +Ġcr ush +ĠE A +c od +m ix +ĠTri ple +Ġretrie ve +Ġstimul us +Ġpsych iat +ĠDo or +Ġhomosexual ity +Ġelement ary +Ġcell ular +id ian +ĠL aun +Ġintrig uing +Ġfo am +ĠB ass +id i +its u +Ġass ure +Ġcongr at +Ġbusiness man +ĠBo ost +cl ose +Ġl ied +Ġsc iences +ĠO mega +ĠG raphics +Ġ< = +sp oken +Ġconnect ivity +S aturday +ĠAven gers +Ġto ggle +Ġank le +Ġnational ist +mod el +ĠP ool +ophob ia +V ar +ĠM ons +ator ies +Ġaggress ively +C lear +For ge +act ers +Ġhed ge +Ġpip es +Ġbl unt +Ġs q +Ġremote ly +W ed +as ers +Ġref riger +Ġt iles +Ġresc ued +Ġcompr ised +ins ky +Ġman if +avan augh +Ġprol ifer +Ġal igned +x ml +Ġtri v +Ġcoord ination +ĠP ER +ĠQu ote +13 4 +b f +ĠS aw +Ġtermin ation +Ġ19 0 +Ġadd itions +Ġtri o +Ġproject ions +Ġpositive ly +Ġin clusive +Ġmem br +19 90 +old er +Ġpract iced +ink le +Ar ch +Ġstar ters +ari us +Ġinter mediate +ĠBen ef +ĠK iller +Ġinter ventions +ĠK il +ĠF lying +In v +Ġprem ature +Ġpsych iatric +Ġind ie +Ġcoll ar +ĠRain bow +af i +Ġdis ruption +ĠFO X +cast ing +Ġmis dem +c ro +Ġw ipe +ard on +Ġb ast +ĠTom my +ĠRepresent ative +Ġbell y +ĠP O +ĠBre itbart +13 2 +Ġmess aging +Sh ould +Ref erences +ĠG RE +ist ical +L P +ĠC av +ĠC razy +Ġintu itive +ke eping +ĠM oss +Ġdiscont in +ĠMod ule +Ġun related +ĠPract ice +ĠTrans port +Ġstatist ically +orn s +Ġs ized +p u +Ġca f +ĠWorld s +ĠRod gers +ĠL un +ĠCom ic +l iving +Ġc ared +Ġclim bed +) { +Ġconsist ed +Ġmed ieval +fol k +Ġh acked +Ġd ire +ĠHerm ione +Ġt ended +ce ans +D aniel +w ent +Ġlegisl ators +Ġred es +g ames +Ġg n +am iliar +Ġ+ + +gg y +th reat +Ġmag net +Ġper ceive +Ġz ip +Ġindict ment +Ġcrit ique +g ard +ĠSaf e +ĠC ream +Ġad vent +ob a +Ġv owed +ous ands +Ġsk i +Ġabort ions +u art +Ġstun ned +Ġadv ancing +Ġlack ed +Ġ\ " +Ġsch izophren +Ġeleg ant +Ġconf erences +Ġcance led +ĠHud son +ĠHop efully +Ġtr ump +Ġfrequ encies +Ġmet eor +ĠJun ior +ĠFle et +ĠMal colm +ĠT ools +Ġ ........ +Ġh obby +ĠEurope ans +Ġ15 00 +ĠInt o +Ġs way +ĠApp ro +ĠCom pl +Comm unity +Ġt ide +ĠSum mit +ä » +Ġinter vals +ĠE ther +Ġhabit at +ĠSteven s +lish ing +ĠDom ain +Ġtrig gers +Ġch asing +Ġchar m +ĠFl ower +it ored +Ġbless ing +Ġtext ures +F ive +Ġliqu or +R P +F IN +Ġ19 62 +C AR +Un known +Ġres il +ĠL ily +Ġabund ance +Ġpredict able +r ar +Ġbull shit +le en +che t +M or +M uch +ä ¹ +Ġemphas ized +Ġcr ust +Ġprim itive +Ġenjoy able +ĠPict ures +Ġteam mate +pl er +ĠT ol +ĠK ane +Ġsummon ed +th y +ram a +ĠH onda +Ġreal izing +Ġquick er +Ġconcent rate +cle ar +Ġ2 10 +ĠErd ogan +ar is +Ġrespond s +ĠB I +Ġelig ibility +Ġpus hes +ĠId aho +Ġagg rav +Ġru ins +ur ations +Ġb ans +Ġan at +sh are +Ġgr ind +h in +um en +Ġut ilities +ĠYan kees +Ġdat abases +ĠD D +Ġdispl aced +Ġdepend encies +Ġstim ulation +h un +h ouses +ĠP retty +ĠRaven s +ĠTOD AY +Ġassoci ates +Ġthe rape +cl ed +Ġde er +Ġrep airs +rent ice +Ġrecept ors +Ġrem ed +ĠC e +Ġmar riages +Ġball ots +ĠSold ier +Ġhilar ious +op l +13 8 +Ġinherent ly +Ġignor ant +Ġb ounce +ĠE aster +REL ATED +ĠCur rency +E V +ãĥ ŀ +ĠLe ad +Ġdece ased +B rien +ĠMus k +J S +Ġmer ge +heart ed +c reat +m itt +m und +ĠâĢ ĭ +ĠB ag +Ġproject ion +Ġj ava +ĠStand ards +ĠLeon ard +Ġcoc onut +ĠPop ulation +Ġtra ject +Ġimp ly +Ġcur iosity +ĠD B +ĠF resh +ĠP or +Ġheav ier +ne ys +gom ery +Ġdes erved +Ġphr ases +ĠG C +Ġye ast +d esc +De ath +Ġreb oot +Ġmet adata +IC AL +Ġrep ay +ĠInd ependence +Ġsubur ban +ical s +Ġat op +Ġall ocation +gener ation +ĠG ram +Ġmoist ure +Ġp ine +ĠLiber als +Ġa ides +Ġund erest +ĠBer ry +Ġcere mon +3 70 +ast rous +ĠPir ates +Ġt ense +ĠIndust ries +ĠApp eals +ĠN ear +Ġè£ı ç +Ġlo vers +ĠC AP +ĠC raw +Ġg iants +Ġeffic acy +E lement +ĠBeh avior +ĠToy ota +Ġint est +P riv +A I +Ġmaneu ver +Ġperfect ion +Ġb ang +p aper +r ill +Ge orge +b order +in ters +ĠS eth +Ġcl ues +ĠLe vi +ĠRe venue +14 7 +Ġv apor +Ġfortun ate +Ġthreat ens +Ġve t +Ġdepend ency +ers ed +art icle +ĠBl izzard +Ġch lor +Ġmin us +ĠB ills +Ġcryptoc urrency +Ġmetabol ism +ter ing +Ġp estic +step s +ĠTre asure +ract ed +ĠConst ant +Ġtem p +13 9 +ĠDet ective +ur ally +Ġrecover ing +Ġcort ex +Ġ14 4 +cl osed +Ġprejud ice +aun ted +Ġstorm s +ĠN OW +Ġmach inery +Add ress +Ġcompe lled +27 0 +Ġdesp air +b ane +Ġveget able +Ġbed s +Lear n +Ġcolor ful +Ġsp ike +Ġmarg ins +Ġsymp athy +Ġworks hop +ĠC BC +S at +Ġburn s +ĠG ender +Ġ12 9 +ĠC able +Ġdeb ts +ĠThe resa +Ġreflect ing +Ġa irst +Ġr im +ram id +Ġweakness es +W rit +ogg le +t i +ĠCh arge +Ġwe ighed +Ġ( . +Ġl aughter +Ġrou ter +ĠDemocr acy +D ear +Ġhas ht +Ġd y +Ġhint s +run ning +Ġfin ishes +ar us +M ass +res ult +asc us +Ġv intage +Ġcon qu +Ġwild ly +ac ist +Ġl ingu +Ġprot agonist +st rom +te enth +ĠSol o +m ac +f illed +Ġre nown +it ives +Ġmot ive +ĠAnt ar +ĠM ann +ĠAd just +Ġrock ets +Ġtrou bling +e i +Ġorgan isms +ass is +Christ ian +Ġ14 5 +ĠH ass +Ġsw all +Ġw ax +ĠSurv ival +V S +ĠM urd +v d +stand ard +Ġdrag ons +Ġacceler ation +r ational +f inal +Ġp aired +ĠE thereum +Ġinterf aces +Ġres ent +Ġartif acts +Å « +are l +Ġcompet itor +ĠNich olas +ĠSur face +c pp +ĠT ot +Ġeconom ically +Ġorgan ised +Ġen forced +in ho +Ġvar ieties +Ġab dom +ĠBa iley +id av +ĠSal v +p aid +Ġalt itude +ess ert +ĠG utenberg +are a +op oulos +Ġprofess ors +igg s +ĠF ate +he y +Ġ3 000 +D ist +Ġtw ins +c ill +ĠM aps +Ġtra ps +Ġwe ed +ĠK iss +Ġy oga +Ġrecip ients +ĠWest minster +Ġpool s +ĠWal mart +18 8 +ĠSchool s +att ack +ĠAR M +par agraph +W arning +j l +Ġself ish +anche z +ĠHe ights +F re +ĠS oph +Ġ -------------------------------- +t ml +33 3 +Ġraid s +Ġsatell ites +KE Y +Ġlast s +Ñ Ĥ +In s +ĠD ame +Ġunp redict +// / +gh ai +Ġart illery +Ġcru ise +Ġg el +ĠCabin et +Ġbl ows +ĠE sp +Ġprox imity +ot he +ĠSk ills +ĠU pper +ob o +ĠN DP +Ġenjoy s +Ġrepe ating +ĠConst ruction +ĠQuest ions +H illary +Ġu int +Ġprocess ors +ĠGib son +ĠMult iple +q a +ĠB om +ĠM iles +vent ional +Ġhur ts +s kin +ĠA IDS +Ġadvis ers +ĠR oot +Ġmethod ology +ĠD ale +Ġdet on +ĠKnow ledge +sequ ently +Ġ12 1 +Ġconnect s +C y +ĠD anger +Ġcontribut ors +ĠB ent +Ġbr ass +ĠGun s +int o +ĠFort une +Ġbro ker +bal ance +Ġlength s +Ġv ic +Ġaver aging +Ġappropri ately +ĠCamer a +Ġsand wich +ĠCD C +Ġcoord inate +Ġnav ig +Ġgood ness +l aim +Ġbra ke +Ġextrem ist +ĠW ake +ĠM end +ĠT iny +ĠC OL +ĠR F +ĠD ual +ĠW ine +C ase +Ġref ined +Ġl amp +L ead +Ġb apt +ĠCar b +ĠS add +ĠMin neapolis +PD F +Ear ly +ĠH idden +I ts +ĠT IME +Ġp ap +Ġcommission ed +ĠF ew +ĠCol ts +ĠB ren +Ġbot hered +Ġlike wise +Ex per +ĠSch w +c ry +n n +ĠM itch +im on +M G +b m +UM P +r ays +Ġregist ry +Ġ2 70 +ach ine +re lla +ant ing +00 000 +Ġru ined +sp ot +Ġt a +Ġmaxim ize +Ġincon ven +D ead +H uman +En abled +ĠMar ie +Ġch ill +ĠParad ise +Ġstar ring +ĠLat ino +ĠProt ocol +ĠE VER +Ġsuppl iers +m essage +ĠBro ck +Ġser um +âĸĪâĸĪ âĸĪâĸĪ +Ġen comp +Ġamb ition +ues e +Ġar rows +And rew +Ġanten na +Ġ19 61 +ĠB ark +Ġb ool +ãĤ ª +ĠSt orage +Ġrail way +Ġtoug her +ĠC ad +Ġwas hing +P y +' ] +em bed +ĠMem phis +ack le +Ġfam ously +ĠF ortunately +ov ies +Ġmind set +Ġsne ak +ĠD h +RA W +ĠSim pson +Ġliv est +Ġland mark +Ġc ement +L ow +Ġthr illed +ĠCour se +in el +Ġch uck +id ate +gl obal +Ġwh it +Ġ � +ad ays +s ki +ĠS V +Ġvir uses +30 6 +ĠResp ons +Ġthe aters +ĠBr anch +ĠGene va +ĠM K +Ġunbel iev +Ġcommun ist +Orig inal +ĠRe ceived +ĠTrans fer +ĠAr g +In put +ĠStr ategy +Ġpal ace +the ning +D ri +Ġsent encing +umbn ail +Ġp ins +re cy +Ġs iblings +Get ting +ĠB U +ĠNorth west +Ġprolong ed +ĠSak ura +C omb +ĠB our +Ġinadequ ate +ĠK ash +Ġus ername +ĠImpro ve +Ġbatt ling +ĠM AC +Ġcurric ulum +Ġs oda +ĠC annon +Ġsens ible +sp ons +De cember +Ġw icked +ĠP engu +Ġdict ators +ĠHe arts +og yn +Ġsimilar ities +ĠSt ats +Ġh ollow +it ations +": [ +Ġh over +ĠList en +s ch +S und +Ġc ad +ĠPar ks +Ġl ur +Ġhy pe +ĠL em +N AME +is ure +Fr iday +Ġshoot s +Ġclos es +Ġd b +ĠR idge +ĠDiff erent +Ġrepl ies +ĠBroad way +op ers +Ġint oler +ĠZe us +akes pe +Ġpropri etary +Ġrequest ing +Ġcontro llers +ĠM IN +im edia +be cca +Ġexp ans +Ġoil s +B ot +ĠCh and +Ġpr inter +Ġto pped +ĠP OL +ĠEar lier +S ocial +av in +Ġdecre ases +ĠSe b +Ġspecific ations +ĠBl ast +ĠK urt +Ġfre el +B rown +Ġdil ig +ro e +ĠPro blem +ĠQu ad +Ġdecent ral +ĠV ector +an ut +Ġplug ins +ĠGreg ory +Ġfuck ed +el ines +ĠAmb assador +t ake +Ġcle ans +ong yang +An onymous +st ro +" } +al ine +ĠO dd +ĠE ug +2 16 +Ġbo il +ĠP owers +Ġnurs es +Ob viously +ĠTechn ical +Ġexceed ed +OR S +Ġextrem ists +Ġtr aces +ex pl +Ġcom r +ĠS ach +) / +Ġm asks +Ġsc i +B on +Ġreg ression +we gian +Ġadvis or +it ures +ĠV o +ex ample +ĠInst ruct +Ġs iege +Ġredu ctions +pt r +Ġstat utory +Ġrem oves +Ġp uck +red its +Ġbe e +Ġsal ad +Ġpromot ions +ĠJosh ua +with standing +ET H +ĠCh a +im us +Ġexpend iture +aun ting +Ġdelight ed +Ġ15 5 +be h +Ġcar pet +ĠSp art +Ġj ungle +l ists +Ġbull ying +ĠNob el +ĠGl en +Ġreferen ced +Ġintrodu ces +se in +Ġcho pped +gl ass +ĠW rest +Ġneutral ity +Ġâ Ļ +Ġinvestig ator +Ġshel ves +Ġun constitutional +Ġreprodu ction +Ġmer chant +m ia +Ġmet rics +Ġexplos ives +ĠSon ia +Ġbod ily +Ġthick ness +Ġpredomin antly +ĠAb ility +Ġmon itored +IC H +Ġ] . +ĠMart inez +Ġvis ibility +Ġqu eries +Ġgen ocide +ĠWar fare +Qu ery +Ġstud ios +Ġemb ry +Ġcorrid or +Ġclean ed +com plete +ĠM H +Ġenroll ment +ING S +Ġimpact ed +Ġdis astrous +ĠY un +ĠCl aire +ĠBas ically +y t +uster ity +Ġindirect ly +w ik +Ġd od +ĠCar r +Ġam p +Ġprohib it +ĠIn itial +ĠR d +ij i +Ġeduc ate +c orn +i ott +ĠBeaut y +Ġdetect ive +ĠCon n +s ince +Ġst agger +Ġob ese +Ġb ree +olog ic +is se +walk er +Ġbl ades +Ġlaw ful +fun c +ĠBeh ind +Ġappet ite +Ġ( * +Ġt ennis +Ġoff spring +Ġj ets +Ġstruct ured +Ġafore mentioned +N ov +Ġsc aling +f ill +Ġst ew +Ġcur b +ĠStep han +ed In +S F +ob ic +é ŃĶ +ou g +ĠM M +Ġgen etically +ope z +13 6 +Ġu mb +anc ers +Ġcoh ort +Ġmerch andise +Ġimp osing +ĠLegisl ature +ĠArch ive +iv ia +ĠN aval +Ġoff ences +Ġmir acle +Ġsn apped +Ġf oes +Ġextensive ly +ĠR af +Ġc ater +ed ience +K it +ĠB in +Ġrecomm ends +ĠC ities +Ġrig id +ĠRE AD +ĠNob le +ĠT ian +Ġcertific ates +ant is +o iler +ĠBudd hist +d id +Ġsurvey ed +Ġdown ward +Ġprint s +ĠMot ion +ron ics +ĠS ans +oss ibly +u ctions +Ġcolon ies +ĠDan ish +un it +Ġsp oil +Ġadvis ory +ber ries +Pl an +Ġspecific ation +op hers +ĠRes ource +Ġsh irts +prising ly +commun ications +Ġtriv ial +Ġmention ing +ise xual +Ġsupp lements +Ġsuper vision +B P +v or +Ġw it +Ġco oldown +Ġplaint iff +ĠReview s +ĠS ri +ĠM int +ĠSug ar +Ġafter ward +ĠPri est +ĠInvest ment +og ene +ĠT aking +Ġstretch ing +Ġinflamm ation +ĠTe hran +Ġl ining +Ġfree zing +ĠEnt ity +Ġins piring +spe cial +pr ice +Ġsu e +ĠP orter +oun ge +ET A +ĠD erek +ĠLu is +u o +ym ph +Ġex terior +ih il +ĠAsh ley +in ator +Ġnut rients +ĠTh rones +Ġfin ances +ĠIn spect +Ġspe cially +ĠRequ ired +ĠP TS +ĠViol ence +oint ed +sh ots +Ġex cerpt +co on +IN S +ĠG ri +Ġrecogn ised +We ek +You ng +Ġv om +is le +ĠCur ry +ĠBudd h +Ġnot ebook +Ġd urable +/ ? +ĠG ad +ĠP upp +Ġforg ive +p ark +Ġpersonal ities +an alysis +cl amation +Ġelev ator +Ġware house +ĠR ole +un n +Ġillust ration +ĠSc an +Ġatmosp heric +Im port +AN C +rict ed +f u +01 0 +Ġar che +Ġreward ed +akespe are +Ġintern ally +ĠR BI +alk er +Ġeleph ant +ow itz +ĠP izza +Ġbip artisan +é s +Ġslow ed +ĠSt ark +Ġover ride +OU S +Ġ3 20 +undred s +ĠDe ck +ĠC ensus +be e +14 6 +ot or +Ġ ip +Ġu b +oc ations +ĠBut ton +r ice +Ġc ripp +ff f +Ġorig inated +Ġoverwhel med +app a +Ġfore most +âĢ ij +ĠL EG +re lease +eat ured +at ches +Ġre ps +Ġl ending +ĠRe ference +ĠCl ient +16 5 +vent h +Com plete +ĠPat rol +Ġsw orn +c am +Ġshut tle +ĠR alph +Ġh ometown +- , +on al +ĠB P +å ı +Ġpersu ade +ĠAlex and +Ġcomb ines +Ġv ivid +ĠL ag +Ġenc oding +Ġsal vation +w en +ĠRec overy +i ya +Un iversity +ĠB iden +Ġbud gets +ĠTex ans +f its +Ġhon ored +Ġp ython +T D +## # +cl one +Ġbl ink +ĠL iquid +Ġunemploy ed +Ġcl ashes +ĠCoun sel +Ġdirect ing +Ġpun ct +ĠFal cons +Ġsh ark +ĠDam ascus +Ġje ans +Ġemb ark +Ġse ize +Ġup wards +2 80 +ĠE z +ĠAny thing +Ġex otic +l ower +ĠCreat or +ĠU m +Ġsubur bs +ber ger +ĠW end +Ġm int +ĠX X +ĠD ro +Ġsuff ers +Ġher b +t ree +Ġfrag ile +Ġflood ed +ĠAl cohol +ole an +ny der +ĠK O +F ram +Ġ13 6 +Ġow ed +ĠMe lee +ĠH ash +Ġwh isk +Ġsu do +r r +Qu ick +app ro +Ġi i +ĠEx amples +he e +Ġpromot es +per ature +k ar +ĠHon or +Ġs odium +ĠL if +ros so +intend ent +Ġcorrespond ent +F ound +sec ret +Ġident ifies +ag ne +Ġl ou +ĠP P +Ġcoinc idence +m ove +Ġmilit ia +Ġinf iltr +ĠPrim ary +Ġpitch ing +ĠI b +ĠGO OD +ãĤ ¸ +ĠW izards +ir al +ĠVen us +R R +ĠâĢ ķ +ĠCase y +Ġsad ly +Ġadm ire +Ġembarrass ed +c b +M el +Ġtub es +Ġbeaut ifully +ĠQueens land +Bel ow +re z +qu et +ple asant +Ġ « +C amp +Ġdec isive +19 98 +ĠL amb +ut ton +h n +ĠJ agu +au nder +ĠC ord +Ġcl erk +Ġca ffe +Ġwip ed +Ġre im +ĠMount ains +Ġimprison ed +Ġdevelop s +ĠP ra +Ġmodel ing +Any one +ance l +ĠS it +Ġshield s +Ġl awn +Ġcard iovascular +Ġdemonstr ating +Ġpar se +ĠIsrael is +Ġeuro s +14 3 +Ġgl orious +ins ki +ec d +Ġcondition ing +Ġhel pless +Ġmicro sc +ĠHar bor +Ġst akes +Ġ2 60 +Ġun equ +ĠFl oyd +Ġd amp +Ġappar atus +ĠLaw s +Ġcoun ters +Ġindu ce +at able +ĠAh med +Ġsl am +N ovember +Ġpers ist +Ġim minent +á n +Ġsh red +Ġph ases +ĠEd monton +ĠArm strong +ĠMe et +ĠK itty +Ñ Ģ +c irc +ĠAd ult +Ġa rose +ĠX en +D an +g ow +Ġsuper f +ĠAd mir +Ġend ure +Ġkey word +yr us +Ġy arn +Ġpath way +ĠHop kins +mid t +Ġcens orship +d ependent +Ġinstruct or +S ources +Ġto e +Ġball oon +N ob +Ġsw ear +ĠCast ro +Ġgl oss +ĠK avanaugh +Ġremark ably +Ph otos +ĠN om +ĠS outheast +y ers +Ġvalid ation +Ġcann on +ĠVict ory +ĠPier re +Ġcaut ious +Aud io +Ġf etch +ĠG ift +ĠH yp +Ġrem edy +Z E +Ġsc ent +Ġbe ard +ĠR ut +- " +Ġpat ents +H y +Ġun just +Ġpot ato +Ġforth coming +Ġche f +ĠR ift +aff e +ĠR OM +ĠL aunch +Ġp ads +ĠNe o +Ġon set +Ġsquee ze +s afe +Ġpref ix +ĠT M +ĠN early +ĠClin ical +ĠM ental +ot iation +ĠUn ic +ant ry +ĠC ir +Ġep it +à ¦ +Ġextract ed +verse ly +ri ad +Ġstr ains +Ġto ps +Ġpo em +ĠRand y +ĠMap le +TH ER +up iter +ĠSS D +ļ é +Ġun con +per ing +Ġsle pt +in ers +Ġunder water +ĠEv idence +g one +20 5 +Ġhistor ians +Ġsynt hesis +Ġf rog +b asketball +Ġvibr ant +Ġsub ord +Ġ3 65 +ĠD ial +Ġcooper ate +HA HA +Ġgreet ed +15 8 +Ġj azz +Ġinto x +ĠWalk ing +Ġsuper visor +ĠF usion +ĠMer cedes +s end +H am +s d +n l +Ġtour s +ĠF IFA +Ġcul p +g d +30 4 +Ġple as +Ġillust rates +ĠColomb ia +Ġhighlight ing +ĠSum mary +Ġexp osing +ĠD ru +Ġir ony +r itional +ĠCar roll +ĠEll is +P ict +ĠR apt +Ġad apter +Ġun m +Ġcor pse +Ġceleb rities +D en +at um +ĠAp ocalypse +ĠW ag +lin ing +Ġhorm ones +R ub +ĠX i +ĠV aults +20 8 +alky rie +inos aur +Ġfeed s +v ity +Ġdefe ating +W ait +Ġemphas ize +ĠSteel ers +yr inth +le ys +ĠWhe never +Current ly +ĠCl ock +Ġcollect ively +any on +ĠJ P +Ġment ality +Ġdownload s +Ġsurround ings +ĠBarn es +Ġflags hip +Ġindic ators +Ġgra pp +Jan uary +ĠElement al +ĠAthen a +ib al +Ġs ights +Ġcap ita +ĠTreat y +Ġvo iced +ĠG az +let te +Ġy a +Ġexp ired +Leg end +H ot +n ature +Ġunst able +Ġ2 80 +à º +Com ment +AL E +Ġquest s +Ġhand ler +n is +Ġvers atile +Ġconce al +enge ance +ĠInter active +Ġobs essed +ĠDog s +Ġcr acked +S ound +s v +ĠD ylan +ro ads +f x +ĠCath olics +ĠH ag +Ġsl ammed +Ġgl owing +s ale +Ġtiss ues +ĠCh i +ne e +Ġc her +s ic +ur rection +Ġb acon +ul atory +) ." +Ġir regular +FOR M +ass ed +Ġintention al +Ġcompens ate +ĠSpe aking +ĠS ets +15 3 +Ġconvent ions +b ands +em ade +Ġe cc +ĠWin ston +ĠAssass in +ĠBelg ian +Ġdepend ence +Ġnic he +Ġb ark +ĠJ azz +Ġdisadvant age +Ġgas oline +Ġ16 5 +çļ Ħ +ess a +mod ule +ang ular +O Y +ĠTreat ment +it as +ol ation +ĠArn old +Ġfe ud +ĠN est +Ġthe atre +ew ater +Ġmin ors +olic y +ĠH aven +div ision +Ġtr unk +F ar +ĠP ull +Ġcapt uring +Ġ18 00 +ĠTe en +Ġex empl +Ġclin ics +ĠB urg +Ġsubst it +Ġpay load +ĠL av +ĠT roy +ĠW itness +Ġfrag ments +Ġpass words +Ġg ospel +ĠG in +Ġten ants +ol ith +S ix +Pre vious +ĠAg es +ĠDar win +Ġbl at +Ġem pathy +sm ith +b ag +ĠE cho +ĠC amb +ĠM add +ĠB oo +Ġred e +ĠBurn ing +Ġsmooth ly +ĠAd rian +ĠV ampire +ĠMon sters +ste am +Sty le +M a +re a +ĠD war +aly st +urs or +Ġelim ination +Ġcrypt o +ch t +ĠE ternal +â̦ ] +ĠS orce +I ll +N ER +Ġu h +Con clusion +w age +Ġresp ir +Ġrem inis +het ical +Ġg y +Ġutil ized +ic idal +Ġ19 00 +Ġhun ters +ĠSw an +ĠRe act +Ġvis itor +ĠThanks giving +30 8 +Post s +Ġh ips +19 97 +om ers +Ġkn ocking +ĠVeh icle +Ġt il +Ġ13 8 +Ġm i +ĠInvest igation +ĠKen ya +Ġcas ino +Ġmot ives +Ġreg ain +re x +Ġweek ends +Ġstab bed +bor o +Ġexplo ited +ĠHA VE +ĠTe levision +c ock +Ġprepar ations +Ġende av +ĠRem ote +ĠM aker +ĠPro du +ĠEv an +Ġinform ational +ĠLouis ville +15 4 +ĠDream s +Ġpl ots +ĠRun ner +Ġhur ting +Ġacad emy +ĠMont gomery +n m +ĠL anc +ĠAl z +2 10 +el ong +Ġretail er +Ġar ising +Ġrebell ion +Ġbl onde +play ed +Ġinstrument al +C ross +Ġret ention +Ġtherape utic +Ġse as +Ġinfant ry +ĠCl int +Ġprompt ing +Ġbit ch +Ġst ems +ĠK ra +Ġthe sis +ĠB og +ru ed +Ġk ings +Ġcl ay +ific ent +ĠY ES +ĠTh ing +ĠCub s +vey ard +els h +in arily +ĠE y +ĠRoll ing +Ġev olving +Ind ia +Ġrecogn izes +Ġgrad uation +is ers +Ġfert ility +ĠMil an +Comm and +Ġbox ing +Ġ19 43 +Ġgl uten +ĠEm ir +Ġid ol +Ġcon ceived +ĠCre ation +Mer it +udd y +uss ions +ĠLie utenant +iet al +Ġunch anged +ĠSc ale +ĠCrime a +ball s +ator ial +Ġdepth s +Ġempir ical +Ġtrans m +Ġuns afe +miss ible +com fort +15 6 +Ġmechan ic +00 2 +l ins +Ġsm oked +P os +Ġslow ing +Ġl av +Tex as +Ġche ating +ĠMet ropolitan +eth yl +Ġdiscover ing +as se +Ġpen cil +ĠPy ongyang +Ġclos et +ĠShe et +ĠEnt ry +ou stic +Ġmy st +er ate +ari at +Ġminer als +Ġmusic ian +ĠP ul +ĠM az +24 9 +Ġper missions +Ġ iv +en ary +ick ers +ĠB ing +he a +en able +Ġgri ev +Ġassert ed +ĠColon el +Ġaff idav +w o +Ġse ated +ĠR ide +Ġpaint ings +ĠP ix +Ġ13 7 +ish i +umb ai +g otten +ĠEar l +Ġin ning +Ġc ensus +Ġtrave lled +ĠCons ult +18 5 +b ind +Ġsimpl icity +Ġoverlook ed +ĠHelp ful +Ġmon key +Ġoverwhelming ly +Bl ood +ĠFl int +ĠJ ama +ĠPres ent +ĠR age +ĠT A +pt ive +Ġturn out +w ald +ĠD olphins +ĠV PN +Ġon ion +Ġcraft ing +m ma +ĠMerc ury +Ġarr ange +Ġalert s +ĠO T +zb ollah +Ġg ases +ĠRichards on +s al +l ar +Ġfro st +Ġlower ing +Ġacc laim +Ġstart ups +ĠG ain +ess ment +Ġguard ian +äº º +ĠP ie +ĠL inks +Ġmer its +Ġaw ake +Ġparent al +Ġexceed s +Ġid le +ĠPil ot +Ġe Bay +ĠAc cept +ipe g +C am +ĠK ot +Ġtrad ers +olit ics +unk er +ĠP ale +os i +an mar +Ġ19 47 +ĠF ell +est ial +it ating +G F +ĠS r +if ted +Ġconnect or +ĠB one +ill es +2 60 +h ma +Ġoverl ap +ĠGit Hub +Ġclean er +ĠBapt ist +ĠW AS +Ġlung s +Ñ ģ +ĠB UT +Ġc ite +Ġpit ched +reat ment +Ġtro phies +ĠN u +38 6 +ĠPr ide +Ġattend ees +[ ] +17 9 +Ġspat ial +Ġpri zes +ĠRel igion +Ġshow case +ĠC ategory +vid ia +T arget +Pro perty +? , +Ġf usion +p ie +ĠU CLA +Ġsound track +Ġprin cess +ĠC aval +sh ould +Ġlim bs +Back ground +Ġlone ly +Ġc ores +ĠT ail +she et +Ġ13 2 +R a +ãĤ « +ĠB olt +Ġbook ed +Ġadmin ister +Ġequ als +w y +Ġobserv ing +ĠBar on +ĠAd obe +Ġv irgin +ĠSocial ist +M ove +gh azi +ĠLind a +2 12 +Ġbre wing +Ġmerch ants +bur se +Ġdiv or +Ġmet als +ĠN er +Ġsum s +ĠEn emy +Ġen vision +Ġgrant ing +ĠH oney +ĠSk yrim +Ġsoc io +gr aded +Ġselect ive +W ASHINGTON +Ġ19 48 +ĠSir ius +ĠG ross +act ivity +ĠI van +Ġfur ious +BS D +ĠPre vious +Ġrespons ive +Ġchar itable +Ġle aning +ĠP ew +Ġviol ates +\\\\ \\\\ +ĠCom ing +w ire +Ġpo et +Ġres olutions +comm and +ĠPortug uese +Ġnick name +Ġde af +Feb ruary +Ġrecogn ise +Ġentire ty +Ġseason al +pl aced +ĠTe legraph +Ġmicro phone +our ing +Ġgr ains +Ġgovern ed +Ġpost p +ĠW aters +in ement +Ġund ocumented +ĠCom cast +Ġf ox +Ġassault s +re on +man y +ĠJen kins +ĠAny way +Ġassess ments +Ġdown s +ĠM ouse +Ġsuper b +k t +ĠD ow +Ġtax ation +4 01 +Ġsm iles +Ġundert aken +Ġex h +Ġenthusi astic +Ġtw ent +Ġgovernment al +Ġautonom y +ĠTechn ologies +ĠCh ain +Ġpreval ent +f b +Ġnic otine +og ram +j ob +Ġawa iting +ĠMen u +Ġdep uties +k ov +ish ops +But ton +ĠShan ghai +Ġdies el +ĠD uck +R yan +ĠPC s +N F +j ury +ent e +Ġinacc urate +edd y +Wh atever +Ġshow c +ĠN ad +od us +et r +Ġplaint iffs +ĠW OR +ĠAss ange +Ġpriv at +Ġpremium s +Ġt am +UR L +Ġel ites +ĠR anger +otten ham +ĠH off +ĠAt hens +Ġdefin ite +Ġs ighed +Ġeven ly +2 11 +ĠAm ber +ak ia +Ġmail ing +Ġcr ashing +ĠConfeder ate +ru gged +W al +ĠDep ths +Ġjuven ile +Ġreact or +Introdu ction +ĠDel uxe +19 95 +ĠS anchez +ĠM ead +iv able +: - +ĠPlan ning +ĠT rap +qu in +ĠProt ect +ve red +In formation +Ġkid ney +inn amon +l as +Ġpolic ing +Ġtoler ate +ĠQ i +Ġbi ased +F ort +ĠK i +s ave +Ġprivile ged +Ġbe asts +ĠGl as +ĠC inem +Ġcome back +Sund ay +Ġext inction +h ops +Ġtrans mit +Ġdoub les +ĠFl at +16 7 +Ġdis puted +Ġinjust ice +f oo +V ict +role um +ĠJul ie +Con text +ĠR arity +iss ue +Comp onent +Ġcounsel ing +an ne +d ark +Ġobject ions +u ilt +Ġg ast +Ġpl ac +Ġun used +ãĥ ĩ +ĠT rial +ĠJ as +hed ral +ob b +Ġtempor al +ĠPR O +ĠN W +ĠAnn iversary +L arge +Ġther m +Ġd avid +Ġsystem ic +ĠSh ir +m ut +ĠNe pt +add ress +Ġscan ning +Ġunderstand able +Ġcan vas +C at +ĠZ oo +Ġang els +L O +ĠStat ement +ĠS ig +ov able +ĠA way +sh aring +ocr ats +st ated +Ġweigh ing +N or +w ild +B ey +Ġaston ishing +ĠReyn olds +Ġop ener +Ġtrain er +Ġsurg ical +p n +Ġadjust ing +whe el +Ġf rown +erv ative +Ġsusp end +With in +te in +Ġobst acle +Ġliber ties +ym es +Ġur anium +ans om +an ol +ub a +ĠL oss +Ġa rous +ĠHend erson +W ow +s pl +c ur +ĠÂ Ń +Ġtheir s +Dam age +Ġdownload ing +Ġdisc ern +ĠSt o +ĠFl a +Ġh ath +ĠA j +Ġun pleasant +Europe an +exp ensive +Ġscreens hot +ĠU V +Ġall ied +ĠPers ian +Ġmonop oly +Ġat om +ĠReds kins +"> < +Ġcan cell +Ġcinem a +13 1 +f air +ĠAlf red +Ġd uck +arg s +22 3 +ĠIS I +Ġsign aling +in ar +Ġlaugh s +Ġfor wards +Ġreck less +Ġlisten ers +at ivity +Ġvast ly +n ant +L ess +ĠHun ting +ĠScient ific +IT ED +Ġkn ight +ĠH TC +us a +t mp +Ġr ude +ĠLegend ary +Ġar ises +B ad +ĠCl aim +pe g +Ġreal ities +Th ink +Ġ ° +Ġro de +Ġstri ve +Ġan ecd +Ġshort s +Ġhypot hes +Ġcoord inated +ĠGand hi +ĠF PS +R ED +Ġsuscept ible +Ġshr ink +ĠCh art +Hel p +Ġ ion +de ep +rib es +ĠK ai +ĠCustom er +Sum mary +Ġc ough +w ife +Ġl end +Ġposition ing +Ġlot tery +ĠC anyon +Ġf ade +Ġbron ze +ĠKenn y +Ġbo asts +ĠEnh anced +rec ord +Ġemer gence +Ġa kin +ĠB ert +it ous +âĸ ij +Ġst ip +Ġexch anged +om ore +als h +Ġreserv oir +Ġstand point +W M +Ġiniti ate +Ġdec ay +Ġbrew ery +Ġter ribly +Ġmort al +lev ard +Ġrev is +N I +el o +Ġconf ess +ĠMS NBC +Ġsub missions +Cont roller +Ġ20 2 +ĠR uth +} ); +ĠAz ure +Ġ ." +20 6 +ĠMarket ing +Ġl aund +ien cies +Ġrenown ed +ĠT rou +ĠN GO +ble ms +Ġterr ified +Ġwar ns +Ġper t +Ġuns ure +4 80 +ale z +ult z +ĠOut side +Ġst yl +ĠUnder ground +Ġp anc +Ġd ictionary +Ġf oe +rim inal +ĠNor wegian +Ġj ailed +Ġm aternal +é e +ĠLu cy +c op +Ch o +Ġuns igned +ĠZe lda +ĠIns ider +ĠContin ued +Ġ13 3 +ĠNar uto +ĠMajor ity +16 9 +ĠW o +ãĤ ĵ +Ġpast or +Ġinform al +Ð ½ +an throp +jo in +ãģ Ĺ +it ational +N P +ĠWrit ing +f n +ĠB ever +19 5 +Ġy elling +Ġdr astically +Ġe ject +Ġne ut +Ġth rive +ĠFre qu +ou x +Ġpossess es +ĠSen ators +ĠD ES +ĠSh akespeare +ĠFran co +ĠL B +uch i +Ġinc arn +Ġfound ers +F unction +Ġbright ness +ĠB T +Ġwh ale +ĠThe ater +m ass +ĠD oll +S omething +Ġecho ed +ĠHe x +c rit +af ia +Ġgodd ess +Ġele ven +ĠPre view +ĠAur ora +Ġ4 01 +uls ive +ĠLog an +in burgh +ĠCent ers +ĠON LY +ĠA id +Ġparad ox +Ġh urd +ĠL C +D ue +c ourt +Ġoff ended +Ġeval uating +ĠMatthew s +Ġto mb +Ġpay roll +Ġextra ction +ĠH ands +if i +Ġsuper natural +ĠCOM M +] = +dog s +Ġ5 12 +ĠMe eting +Rich ard +ĠMax imum +Ġide als +Th ings +m and +ĠReg ardless +Ġhum ili +b uffer +L ittle +ĠD ani +ĠN ak +Ġliber ation +ĠA be +ĠO L +Ġstuff ed +ac a +ind a +raph ic +Ġmos qu +Ġcampaign ing +Ġoccup y +S qu +r ina +ĠW el +ĠV S +Ġphys ic +Ġp uls +r int +oad ed +ET F +ĠArch ives +Ġven ues +h ner +ĠTur bo +Ġl ust +Ġappeal ed +que z +il ib +ĠTim othy +Ġo mn +d ro +Ġobs ession +ĠSav age +19 96 +Gl obal +J es +2 14 +Ġsl iding +Ġdisapp ro +ĠMag ical +Ġvolunt arily +g b +ane y +Ġprop het +ĠRe in +ĠJul ia +ĠW orth +aur us +Ġb ounds +ie u +)) ) +Ġcro re +ĠCitiz en +S ky +Ġcolumn ist +Ġseek ers +ond o +IS A +ĠL ength +Ġnost alg +Ġnew com +Ġdet rim +ent ric +3 75 +ĠG E +Ġaut op +Ġacadem ics +App Data +ĠS hen +Ġid iot +ĠTrans it +Ġteasp oon +W il +K O +ĠCom edy +> , +Ġpop ulated +W D +Ġp igs +ĠO culus +Ġsymp athetic +Ġmar athon +19 8 +Ġseiz ure +s ided +Ġd op +irt ual +L and +ĠFl oor +osa urs +... ] +Ġl os +Ġsubsid iary +E Y +ĠPart s +ĠSt ef +ĠJud iciary +Ġ13 4 +Ġmir rors +Ġk et +t imes +Ġneuro log +Ġc av +ĠGu est +Ġtum or +sc ill +ĠLl oyd +E st +Ġcle arer +Ġstere otypes +Ġd ur +not hing +Red dit +Ġnegoti ated +---------------- -------- +23 5 +Ġfl own +ĠSe oul +ĠRes ident +ĠS CH +Ġdisappear ance +ĠV ince +g rown +Ġgrab s +r il +ĠInf inite +ĠTw enty +Ġpedest rian +Ġjer sey +ĠF ur +ĠInf inity +ĠEll iott +Ġment or +Ġmor ally +Ġob ey +sec ure +iff e +Ġantib iotics +ang led +ĠFre eman +ĠIntrodu ction +J un +Ġm arsh +ic ans +ĠEV ENTS +och ond +W all +icult y +Ġmisdem eanor +Ġl y +Th omas +ĠRes olution +Ġanim ations +ĠD ry +Ġinter course +ĠNew castle +ĠH og +ĠEqu ipment +17 7 +Ġterrit orial +Ġarch ives +20 3 +Fil ter +ĠMun ich +Ġcommand ed +ĠW and +Ġpit ches +ĠCro at +Ġrat ios +ĠM its +Ġaccum ulated +ĠSpecific ally +Ġgentle man +acer b +Ġp enn +Ġa ka +ĠF uk +Ġinterven e +ĠRef uge +ĠAlz heimer +Ġsuccess ion +oh an +d oes +L ord +Ġsepar at +Ġcorrespond ence +Ġsh iny +P rior +Ġs ulf +Ġmiser able +Ġded ication +( ). +Ġspecial ists +Ġdefect s +ĠC ult +ĠX ia +Ġje opard +ĠO re +Ab ility +Ġle ar +Ġamb itions +ĠB MI +ĠArab s +Ġ19 42 +Ġpres ervation +ific ate +Ġash amed +l oss +ĠRest aur +Ġrese mble +Ġen rich +ĠK N +ĠCl an +fl oat +Ġplay able +IT T +Ġharm ony +arr ison +ĠWe instein +w ere +Ġpoison ing +ĠCom put +ĠWord Press +m ajor +ĠVal ve +F an +ĠTh row +ĠRom ans +ĠDep ression +ad os +Ġtort ured +Ġbal ancing +bott om +Ġacqu iring +ĠMon te +ard i +Ġa ura +Ġ# # +ĠStand ing +ĠAtl as +C F +Ġintr ins +ĠBen ghazi +Ġcamp ing +Ġt apped +bl ade +st rous +ĠR abb +ĠW ritten +t ip +ĠNe igh +ster dam +ĠAll ow +ĠHe aling +ĠR hod +n um +Ġcaffe ine +ĠPer cent +Ġbo o +Ġapp les +30 5 +Ġwel coming +Ġappl aud +Ġa usterity + ± +ĠRe ality +ef e +å ® +Ġsu cks +Ġtab s +ĠPay Pal +Ġback pack +Ġgif ted +abul ary +ĠSc out +ir teen +Ġch in +Ġo mitted +Ġnegative ly +Ġaccess ing +ĠE arn +Ġambul ance +Ġhead phones +Ġ20 5 +ĠRef resh +p resident +ĠKit chen +ĠEnt ered +ĠS nyder +00 5 +om ical +Ġborrow ed +ĠN em +Ġav iation +Ġst all +rim ination +Ġuniform s +it ime +ĠSim mons +ener gy +ab lished +y y +qual ified +Ġrall ies +ĠSt uart +fl ight +Ġgang s +r ag +Ġv ault +lu x +ĠCom par +Ġdesign ation +20 9 +ĠJ os +d ollar +z ero +Ġwell s +30 3 +Ġconstitu ents +Ġhe ck +Ġc ows +Ġcommand ers +Ġdifferent ial +ĠC atherine +29 9 +Ġval ve +Ġbr ace +Ġperspect ives +c ert +f act +icular ly +ĠMc N +pl anes +Ġint ric +Ġpe as +ov an +Ġtoss ed +ret ch +ĠL opez +Ġunf amiliar +de ath +ĠA part +ĠCh ang +Ġrelie ved +rop he +Ġair ports +Ġfre ak +ut il +M ill +ĠCh in +ĠOw en +m ale +ĠBro ken +ĠWind s +ro b +r ising +Ġfire fighters +Ġauthor itarian +Ġ14 8 +Bit coin +ex ternal +Ġbrow sers +iche ver +or ian +Ġun b +Ġpo ke +ĠZ ot +M id +ĠPop ular +Ġco vert +Ġcont ributes +Ġ6 50 +Ġcont ention +G ate +Ġcons oles +Ġchrom os +ĠI X +Ġvis ually +ĠE isen +Ġjewel ry +Ġdeleg ation +Ġacceler ate +ĠR iley +Ġsl ope +Ġind oor +it ially +Ġhuge ly +Ġtun nels +Ġfin ed +Ġdirect ive +Ġfore head +ustom ed +Ġsk ate +Mus ic +g as +Ġrecogn izing +am bo +Ġover weight +ĠGr ade +Ù Ĭ +Ġsound ing +Ġlock ing +ĠR EM +St ore +Ġexc av +ĠLike wise +ĠL ights +Ġel bow +ĠSupp ly +w ic +Ġhands ome +19 94 +C oll +Ġadequ ately +ĠAssoci ate +Ġstri ps +Ġcrack down +Ġmar vel +ĠK un +Ġpass ages +@@ @@ +ĠT all +Ġthought ful +names e +Ġprost itution +bus iness +Ġball istic +person al +c ig +iz ational +R ound +ĠÂłĠÂł ĠÂłĠÂł +ĠCole man +Ġadm itting +ĠPl ug +Ġbit coins +ĠSu z +Ġfair ness +Ġsupp lier +Ġcatast rophic +ĠHel en +o qu +M arc +ĠArt icles +g ie +Ġend angered +Ġdest iny +ĠVol t +ol ia +ax is +Ġche at +Ġun ified +IC O +qu ote +30 2 +ĠS ed +Ġsupp ression +Ġanaly zing +Ġsqu at +Ġfig uring +Ġcoordin ates +Ġch unks +Ġ19 46 +Ġsub p +Ġw iki +ĠFor bes +ĠJ upiter +ĠE rik +im er +ĠCom mercial +\ ) +Ġlegitim acy +Ġd ental +ĠMe an +Ġdefic its +5 50 +Orig inally +ĠHor ror +Ġcontam ination +ll ah +Ġconf isc +ĠCl are +T B +ĠF ailed +an ed +Ġrul er +ĠCont roller +Ġfemin ists +F ix +g ay +20 7 +Ġr abbit +Th ird +ownt own +Ġgl ue +Ġvol atile +Ġsh ining +Ġf oll +Ġimp aired +Ġsup ers +æ Ī +Ġcl utch +ļé ĨĴ +Ġpro let +Ġ( ! +Ġy elled +ĠK iev +ĠEr n +ĠSh ock +K B +Ġsit uated +qu ery +ĠN as +Ġan nex +char acter +ĠHol iday +Ġautom ation +ĠJ ill +ĠRem astered +Ġl inem +Ġwild erness +ĠHor izon +ĠGu inea +A Z +Ġmain land +Ġsec recy +LE ASE +Ġp unk +ĠProv ince +( ), +Spe ed +Ġhand ing +ĠSeb ast +S ir +r ase +Ġj ournals +Ġcon gest +ĠT ut +ir rel +Ġschizophren ia +Ġmis ogyn +health y +I ron +Ġreact ed +- $ +25 2 +Ġpl ural +Ġpl um +Ġbarg ain +Ġground ed +f inder +Ġdis se +ĠL az +O OD +Ġat roc +F actory +Ġmin ions +Ġo ri +ĠB rave +ĠP RE +ĠMy anmar +ĠH od +Ġexped ition +Ġexpl ode +ĠCo ord +Ġext r +ĠB rief +ĠAD HD +Ġhard core +feed ing +Ġd ile +ĠF ruit +Ġvacc ination +ĠM ao +osp here +Ġcont ests +- | +Ġf ren +isp here +R om +ĠSh arp +ĠTre nd +Ġdis connect +âĢ¢ âĢ¢ +Ġper secution +Ear th +Ġhealth ier +38 4 +Ġc ob +ĠTr inity +OW S +AN N +Ġspecial ty +Ġg ru +Ġcooper ative +wh y +Start ing +ĠIss ues +st re +ens or +Ġ18 5 +Ad v +! ? +ĠRe vel +em ia +ĠH ulk +Ġcelebr ations +ĠS ou +ra ud +ĠKle in +Ġun real +con text +Ġpartners hips +Ġadop ting +t ical +Ġspl ash +ĠHe zbollah +c ategory +cycl op +xt on +ĠD ot +urd y +t z +Ġenvelop e +ĠN L +â ķ +Ġwhere in +Spe c +18 4 +Ġte lev +al iation +Ġmyth s +å ° +Ġrig orous +Ġcommun icating +Ġobser ver +Ġre he +ĠW ash +Ġapolog ized +ĠT in +Ġexpend itures +work ers +d ocument +Ġhes itate +ĠLen in +Ġunpredict able +Ġrenew al +cl er +ok ia +ĠCON T +Ġpost season +Tok ens +Ġex acerb +Ġbet ting +Ġ14 7 +Ġelev ation +W ood +ĠSol omon +19 4 +00 4 +out put +Ġredu nd +ĠM umbai +Ġp H +Ġreprodu ce +ĠD uration +MA X +Ġb og +C BS +ĠBal ance +ĠS gt +ĠRec ent +Ġc d +Ġpo pped +Ġincomp et +pro p +ay an +g uy +Pac ific +Ġty r +Ġ{ { +ĠMy stic +ĠD ana +Ġmast urb +Ġge ometry +à ¢ +ĠCor rect +Ġtraject ory +Ġdistract ed +Ġf oo +ĠW elsh +L uc +m ith +Ġrug by +Ġrespir atory +Ġtri angle +Ġ2 15 +Ġunder graduate +ĠSuper ior +ch anging +_ - +Ġright ly +Ġrefere e +Ġluc rative +Ġun authorized +Ġresemb les +ĠGN U +ĠDer by +Ġpath ways +ĠL ed +Ġend urance +Ġst int +Ġcollect or +F ast +Ġd ots +Ġnational s +ĠSec urities +Ġwh ip +Par am +Ġlearn s +M agic +Ġdetail ing +m oon +Ġbroadcast ing +Ġb aked +26 5 +hol m +ĠS ah +ĠHus sein +ĠCourt esy +17 4 +Ġ14 6 +Ġge ographic +pe ace +Ġjud ging +ĠS tern +B ur +Ġstory line +G un +ĠSt ick +24 5 +30 7 +ãĤ´ ãĥ³ +ĠAdminist rator +Ġbur nt +Ġp ave +ch oes +Ex ec +Ġcamp uses +Res ult +Ġmut ations +ĠCh arter +Ġcapt ures +Ġcomp ares +Ġbad ge +S cient +Ġer ad +ier y +o i +ett es +ĠE state +Ġst rap +Ġproud ly +Ġf ried +Ġwithd rawn +ĠV oy +ph ony +It ems +ĠP ierce +b ard +Ġann otation +ant on +ill on +Im pro +... ) +Ġhapp ier +---- -- +ad just +Ġstaff ers +Ġactiv ism +Ġper f +Ġal right +N eed +Ġcomm ence +Ġopio id +ĠAm anda +E s +ĠP ars +ĠK aw +W orks +24 8 +Ġind o +t c +end ant +ĠM oto +Ġlegal ization +OT E +Ġtask ed +Ġt sp +ĠACT IONS +16 6 +Ġrefres hing +ĠN R +ĠPere z +Ġinfring ement +S Y +List en +in ning +k u +Ġrot ate +pro gram +ar ah +Des ign +Ġ( £ +Ġst oring +Ġwar rants +Ġjud gement +ĠB rist +us ually +ph oto +ĠR an +ĠP ine +Ġoutrage ous +ĠValent ine +lu ence +ĠEvery body +Al tern +Ġrele vance +Ġtermin ated +Ġd essert +Ġfulf illed +Ġprosecut ed +ĠW ords +Ġm igrant +Ġcultiv ation +ÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤ ÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤ +idel ity +ĠV ern +ĠLog in +Ġmetaph or +ĠT ip +Ġrecru its +ĠP ig +rib ing +Ġenthusi asts +ex per +Ġfright ening +ĠH air +ans on +str ate +Ġh i +He ight +Ġown ing +n one +Ġdis like +Ġkn ives +pher d +Ġloud ly +ĠAP Is +Dis play +ĠL ac +ĠUS S +ab l +ver ages +J ew +Ġ17 2 +ĠHist orical +at oon +ĠPhys ics +in tern +Ġwarm th +Ġto pp +D M +Ġgun man +Ġem peror +od i +ãĥ £ +in atory +ĠR ib +Ġ13 1 +ĠSat urn +ĠSh ining +Ġw aking +Qu otes +Ġcomed ian +en berg + ½ +Ġbelie vers +Ġpaper work +c ustom +Ġle v +Ġl ament +Ġpour ing +22 2 +p olitical +ĠSupp lement +m aid +Ġcruel ty +Ġt read +ys ics +A w +rit es +Ġmod ifier +ĠP osition +Ad am +l b +ub s +Ġimper fect +Ġcl usters +ĠEngine er +ĠC herry +Ġinaug uration +ĠS au +Ġembod iment +ĠUn cle +Ġover r +Ġexplos ions +c ule +ĠPrinc eton +ĠAndre a +Ġincorrect ly +Ġearn est +Ġpil gr +ĠS print +Ġslee ve +Ġhe ars +ĠAm azing +Ġbrow sing +ag in +Ġhom eland +Ġha w +Ġd iving +ist ered +17 8 +Ġbarg aining +ĠArc ade +Ġdeleg ate +ters on +................................ ................................ +ĠJackson ville +27 5 +Ġst agn +Ġad am +ĠSher man +C B +Ġsub urb +ĠFood s +Ġconver ting +ĠAr ist +Ġch ambers +l ove +Ġam ino +ĠG an +Ġmad ness +m c +ĠUS E +def ined +Ġul tr +ind ust +Ġw olves +l ance +Add itionally +Ġcr acks +as ia +ĠRe ason +ĠP ump +Ġaccident al +ĠL aser +ĠR id +Ġinitial ized +ell i +Ġun named +Ġn oun +ĠPass ed +Ġhost age +ĠEth iop +sh irts +Ġun rel +ĠEmb assy +Ġ19 41 +Ġat oms +Ġpur ported +16 4 +ĠF i +Ġgall ons +ĠMon ica +Ġp g +en ment +Ġsort ed +ĠG ospel +Ġhe ights +Ġtr aced +Ġunder going +She ll +Ġs acks +Ġproport ions +Ġhall uc +F ont +ac et +Ġwar mer +ĠIN TER +Ġgrab bing +Pl ug +Ġreal ization +ĠBur ke +Ġen chant +AT ER +ĠSe ed +Ġabund ant +F M +Ġc ivic +V s +is i +Ġv ow +Ġre per +ĠPartners hip +Ġpenet ration +Ġax e +Ġsh attered +ĠZ ombies +Ġv inyl +ĠAl ert +e on +Ġoblig ed +ĠIll ust +ĠPl aza +ĠFront ier +Ġdavid jl +ĠSer ial +ĠH av +ĠNut rition +B i +Ġâĸ Ī +ĠJ ays +lin ux +Ġhur ry +Ġv oy +Ġhop eless +ĠSte alth +Ġ ãģ +ess ors +tt le +b org +ĠSaf ari +f ell +Ġw ary +d ue +ĠAb ove +H a +E LL +Ġnot or +ĠW on +T oo +Ġoccup ations +Ġposs essions +Ġinv iting +Ġpred ators +Ġacceler ated +Ġ15 7 +uter te +ĠC ube +e ast +acc ount +G ive +Ġtrans plant +red ients +id able +Ġscreens hots +ĠG und +ĠF S +Ġtravel ers +Ġsens ory +ĠF iat +ĠRock ets +İ ĭ +_ { +F riend +Ġchar ming +AL S +Ġenjoy ment +m ph +Ġ5 000 +ĠRE G +Ù Ĩ +b ia +Ġcomp ilation +ro st +ĠV P +ĠSch ne +201 9 +Ġcop ying +M ORE +ĠFl ore +f alls +2 15 +t otal +Ġdis ciples +d ouble +Ġexceed ing +Ġsm ashed +Ġconcept ual +ĠRom ania +ĠB rent +ĠI CE +ĠT ou +Ġg rap +Ġn ails +18 9 +ãĥ ĺ +Ġproc ure +e ur +Ġconfir ming +ĠC ec +aw i +ĠEd en +Ġn g +Ġengine ered +at ics +Ġhook ed +Ġdisgust ing +ĠMur der +ãĤ ¿ +L ibrary +Ġ16 8 +Al most +hem atic +Men u +ĠNot re +ĠJ ur +Ġkidn apped +Ġhack er +ĠJ ade +Ġcreep y +Ġdraw ings +ĠSpons or +Ġcycl ists +ĠGob lin +Ġoptim ized +Ġst aged +ĠMc D +bet ween +A ge +en o +S ex +ĠW ide +n ings +av is +Ġincap able +ĠK ob +Ġreward ing +ĠL one +oles cent +Ġcontract ed +Ġstick y +J ose +B all +f est +ĠIn put +ĠRec ently +Ġto mat +squ are +App lication +Ġnit rogen +Ġdupl icate +ĠRec on +ĠD ear +L ondon +Ġint ra +Ġd ock +Ġout reach +ĠM illion +Ġmamm als +am pton +V AL +Ġsn aps +Ġd os +ĠWh ole +ĠRead y +T ry +ĠWinn ipeg +ear ance +Ġinc urred +ren ched +ĠNS W +il ot +rain e +Ġc ube +g ot +Ġrun way +etermin ed +ĠHaw ks +Ġsurviv or +ĠW ish +ĠD in +ĠDE F +ĠV ault +18 7 +Ġmush rooms +Ġcris p +be y +ĠDisco very +Ġdevelopment al +Ġparad igm +Ġcha otic +ĠT su +Ġ3 33 +b ons +Ġbacter ial +Ġcomm its +Ġcos mic +Ġme ga +oc ative +ĠP aint +ophob ic +Ġv ain +Ġcar ved +ĠTh ief +ĠG ul +ows hip +Ġc ites +ĠEd inburgh +Ġdimin ished +Ġacknowled ges +ĠK ills +Ġmic row +ĠHer a +Ġsen iors +Ġwhere by +H op +at ron +Ġun available +ĠN ate +Ġ4 80 +Ġsl ated +ĠRe becca +ĠB attery +Ġgram mar +Ġhead set +Ġcurs or +Ġex cluding +any e +aunder ing +eb in +Ġfeas ible +ĠPub lishing +ĠLab s +ĠCl iff +ĠFerr ari +Ġp ac +vis ible +mark ed +pe ll +Ġpol ite +Ġstagger ing +ĠGal actic +Ġsuper st +Ġpar an +ĠOffic ers +ãĢ ģ +Ġspecific s +ul us +23 9 +ĠP aste +AM P +ĠPan ama +ĠDe lete +angu ard +rest rial +Ġhero ic +ĠD y +ا ÙĦ +Ġincumb ent +Ġcr unch +t ro +Ġsc oop +Ġblog ger +Ġsell ers +ure n +Ġmedic ines +ĠC aps +ĠAnim ation +ox y +Ġout ward +Ġinqu iries +22 9 +Ġpsych ologist +ĠS ask +ev il +Ġcontam inated +ãĤ ¨ +he rence +Ġbrand ed +ĠAbd ul +z h +Ġparagraph s +Ġmin s +Ġcor related +er b +Ġimp art +Ġmil estone +ĠSol utions +ot le +Ġunder cover +Ġmar ched +ĠCharg ers +f ax +ĠSec rets +Ġr uth +we ather +Ġfemin ine +Ġsh am +Ġprest igious +igg ins +Ġs ung +hist ory +ett le +gg ie +Ġout dated +ol and +Ġper ceptions +ĠS ession +ĠDod gers +u j +ĠE ND +D oc +Ġdefic iency +Gr and +ĠJ oker +Ġretro spect +Ġdiagn ostic +Ġharm less +Ġro gue +ĠA val +E qu +Ġtrans c +ĠRoberts on +ĠDep ending +ĠBurn s +iv o +Ġhost ility +F eatures +ĵ ĺ +Ġdis comfort +ĠL CD +spec ified +ĠEx pect +3 40 +Ġimper ative +ĠReg ular +Ch inese +Ġstate wide +Ġsy mm +Ġlo ops +Ġaut umn +N ick +Ġsh aping +Ġqu ot +Ġc herry +ĠCross ref +è¦ ļéĨĴ +Stand ard +he ed +ĠD ell +ĠViet namese +Ġo st +ĠV alkyrie +O A +Ass ad +Ġreb ound +ĠTra ffic +pl aces +æ ĺ +ĠB uc +17 2 +Ġshel ters +Ġins isting +ĠCertain ly +ĠKenn eth +ĠT CP +Ġpen al +ĠRe play +he ard +Ġdial ect +iz a +ĠF Y +it cher +ĠD L +Ġspir al +Ġquarterback s +Ġh ull +Ġgo ogle +Ġto dd +ĠSter ling +ĠPl ate +Ġsp ying +mb ol +ĠReal m +ĠPro ced +ĠCr ash +Ġtermin ate +Ġprotest ing +C enter +gu ided +Ġun cover +Ġboy cott +Ġreal izes +s ound +Ġpret ending +ĠV as +19 80 +Ġfram ed +Ġ13 9 +Ġdesc ended +Ġrehab ilitation +Ġborrow ing +ĠB uch +Ġbl ur +R on +ĠFro zen +en za +Ch ief +ĠP oor +Ġtransl ates +M IN +Ġ2 12 +J ECT +Ġerupt ed +Ġsuccess es +S EC +Ġpl ague +Ġg ems +d oms +Ġstret ches +ĠSp y +Ġstory telling +C redit +ĠP ush +Ġtra ction +Ġin effective +ĠL una +Ġt apes +Ġanaly tics +erc ise +Ġprogram mes +ĠCar bon +Ġbeh old +he avy +ĠConserv ation +ĠF IR +Ġs ack +ter min +ric ks +Ġhous ed +Ġunus ually +I ce +Ġexecut ing +ĠMor oc +ed ay +Ġed itions +Ġsm arter +ĠB A +Ġout law +Ġvan ished +ib a +AL SE +ĠSil va +23 8 +C ould +Ġphilos opher +Ġevac uated +Sec ret +14 2 +Ġvis as +ãĤ ¬ +ĠM alt +ĠClear ly +ĠN iger +ĠC airo +ĠF ist +3 80 +ĠX ML +aut o +it ant +Ġrein forced +Rec ord +ĠSurviv or +G Hz +Ġscrew s +parent s +Ġo ceans +ma res +Ġbra kes +vas ive +Ġhell o +ĠS IM +rim p +Ġo re +ĠArm our +24 7 +Ġterr ific +Ġt ones +14 1 +ĠMin utes +Ep isode +Ġcur ves +Ġinflamm atory +Ġbat ting +ĠBeaut iful +L ay +Ġunp op +v able +Ġr iots +ĠTact ics +b augh +ĠC ock +Ġorg asm +ĠS as +Ġconstruct or +et z +G ov +Ġant agon +Ġthe at +Ġde eds +ha o +c uts +ĠMc Cl +Ġu m +ĠScient ists +Ġgrass roots +ys sey +"] => +Ġsurf aced +Ġsh ades +Ġneighb ours +Ġad vertis +oy a +Ġmer ged +Up on +Ġg ad +Ġanticip ate +Any way +Ġsl ogan +Ġdis respect +I ran +ĠT B +act ed +Ġsubp oen +medi ately +OO OO +Ġwa iver +Ġvulner abilities +ott esville +ĠHuff ington +J osh +ĠD H +M onday +ĠEll en +K now +x on +it ems +22 8 +Ġf ills +ĠN ike +Ġcum ulative +and als +I r +Ġ ì +Ġfr iction +ig ator +Ġsc ans +ĠVi enna +ld om +Ġperform ers +P rim +Ġb idding +M ur +Ġlean ed +ĠPri x +al ks +Ġ[ â̦] +ĠTw itch +ĠDevelop er +ĠG ir +Ġcall back +Ab stract +Ġacc ustomed +Ġfreed oms +ĠP G +ur acy +Ġl ump +is man +,, ,, +19 92 +ĠR ED +Ġwor m +M atch +ĠPl atinum +I J +ĠOwn er +Tri via +com pl +Ġnew born +Ġfant as +O wn +Ġ19 59 +Ġsymp ath +Ġub iqu +Ġoutput s +Ġal lev +Ġpr ag +K evin +Ġfav ors +Ġbur ial +Ġn urt +so lete +c ache +Ġ15 6 +Ġunl ocks +te chn +M aking +Ġcon quer +ad ic +æ ĸ +Ġel f +Ġelect orate +ĠKurd s +ĠSt ack +ĠSam urai +Ġâ ĺħ +Ġ{ } +ĠS aid +ĠFall out +Ġkind ness +ĠCustom s +ĠBou levard +Ġhelicop ters +ot ics +ĠVe get +com ment +Ġcritic ised +Ġpol ished +ĠRem ix +ĠC ultural +Ġrec ons +Ġdo i +at em +Sc reen +Ġbar red +Com ments +ĠGener ally +Ġsl ap +7 20 +V ari +p ine +Ġem pt +Ġh ats +ĠPlay ing +l ab +a verage +form s +ĠC otton +Ġcan s +ĠD ON +ĠSom alia +C rypt +ĠIncre ases +E ver +mod ern +Ġsur geon +3 000 +Ġrandom ized +================================ ================================ +B ern +im pl +ĠC OR +Ġpro claim +th ouse +Ġto es +Ġam ple +Ġpres erving +Ġdis bel +gr and +B esides +Ġsil k +ĠPat tern +h m +Ġenter prises +Ġaffidav it +ĠAdvis ory +Ġadvert ised +ĠRel igious +se ctions +psy ch +ĠField s +aw ays +Ġhasht ag +ĠNight mare +Ġv ampire +Ġfore nsic +rosso ver +n ar +Ġn avy +Ġvac ant +ĠD uel +Ġhall way +Ġface book +ident ally +ĠN RA +Ġm att +Ġhur ricane +ĠKir by +ĠP uzzle +Ġsk irt +ou st +du llah +Ġanal ogy +in ion +Ġtomat oes +ĠN V +ĠPe ak +ĠMe yer +Ġappoint ments +Ġm asc +Ġal ley +re hend +Ġchar ities +Ġund o +Ġdest inations +ĠTest ing +"> </ +Ġdest ined +Ġimp lements +ĠHar old +RE CT +Ġoptim ization +Ġkilomet res +Ġc md +Ġimpair ment +Ġun successful +Ġswift ly +ĠGlas gow +art en +ĠSh ares +ĠAn swer +ĠAl bum +Ġnut ritional +ãĥ ĸ +ĠF ut +Ġbl oc +ĠN FC +Ġwholes ale +ĠC W +Ġneg lected +Ġlaun cher +Ġannounce ments +OU LD +com b +Ġrot ating +Ġrest s +ĠT icket +ched el +L ou +ĠV ic +Ġ" ' +Ġtem plates +Ġrepl aces +Ar c +:: :: +ĠGil bert +Ġillness es +Ġsched ules +Ġheter osexual +L INE +Ġhere in +Ġco erc +Ġdecre asing +Ġde portation +s udo +ĠInd igenous +Ġweigh s +Al ong +' ); +ĠBeng als +70 7 +Ġjoint s +ver ts +Ġ14 9 +na ire +Ġsimpl est +Ġl ore +10 80 +f iction +ĠDat abase +Ġreserv ation +Ġs ou +Ġsan ctuary +aud io +ap le +Ġveget arian +Ġanticip ation +m icro +Ġend uring +Ġdepart ed +Ġsidew alk +Ġprohib its +ĠF ont +Ġcomp ute +ĠS ect +Ġ15 8 +B attle +Ġbom ber +Ġdist raction +Ġend ured +Ġpractition ers +Ġdistur bed +Ġdr ank +ord ered +Ġsurpr ises +se at +Sec urity +ĠW isdom +og o +Ġsub paragraph +ĠPen insula +ĠOrig ins +ire n +ĠP av +igg le +Ġgrat itude +ĠG ravity +over ty +im an +ct r +ĠCa esar +c ould +g em +Ġsk ies +Ġch amp +Ġagree ing +F amily +D iv +17 6 +Ġmess y +um ption +F ederal +ern o +ĠCh at +Bey ond +Ġdev ote +ĠW alsh +Ġdump ed +Ġaccum ulation +st ad +hib ition +Ġsm okers +Ġinspect or +F rench +iss an +ĠV ita +Ġresearch ing +R AM +ĠCelt ics +Ġcl oak +ĠTer ra +M ary +so ld +ĠD OM +mod s +Int el +Ġmult itude +ĠImpro ved +Ġrel iance +Ġartif act +Ġalarm ing +P rom +h on +T ION +med ium +Ġref lex +ĠEx cel +Ġweaken ed +16 3 +2 24 +Ġcost umes +Ġunique ly +Ġs orrow +Ġm ansion +w p +Ġsal v +ĠGro ve +bs p +ĠSn iper +ĠSh ipping +ĠP OW +Ġund is +Ġbrand ing +G irl +ĠAh mad +ĠL akes +ĠCore y +Ġinherit ance +ener y +Ġpack ing +ĠP rest +D est +F W +Ġregul ator +l ocked +Ġcont ested +ĠMel issa +ĠD uc +Ġunpop ular +Ġst acked +Ġ19 17 +Ġyear ly +Ġst are +Ġassess ing +à ¸ +Ġbe verages +Ġcompet itions +Ġstreng thening +al ong +ĠL ud +Ġmel ted +stan bul +Ġb ounty +EN C +ĠL ands +Ġdecl ares +Ġcustom ize +Ġcomp osite +ãĥ ¬ +C M +ograph ics +ĠTem p +Ġcont ender +Ġins ign +ĠL AN +Ġdis asters +ins pired +Ġjud gments +ustain able +urs ion +Ġvar iance +ĠUlt imately +Ġ -------- +u ador +ĠR X +Ġmel ting +ĠExt ended +ĠT we +M ajor +ĠB il +Ġsy rup +qu ick +ĠHold er +Ġinnoc ence +U LE +ĠM ight +99 99 +Ġf al +Ġcontinu ity +Ġ19 53 +ĠB S +st ill +L at +ĠAb use +Ġun supported +xxxx xxxx +Ġinst itute +Ġfrag ment +ĠP ep +W estern +ĠC ause +ĠFr ag +ĠAr s +à ¥ +ast ics +Ġb ishop +Ġcross es +Ġ15 4 +ĠUp grade +Ġmit igate +ĠRay mond +Mod s +Ġtom ato +Ġst umbled +Ġdiff ers +In itial +ĠR aspberry +Ġign ores +Ġt ant +à ł +Ġrel ay +Ġb isexual +Ġconf ession +Ġd ement +in as +ĠHe ather +pl atform +dri ving +bour g +ĠM ush +Ġhy ster +Det ails +Ġdr ift +ĠW ald +ĠLuck ily +or f +Ġexp ire +ĠP unch +zy me +g old +Ġunp aid +ĠT rent +Ġun armed +Ġill icit +ĠT ottenham +Ġsm ash +Intern ational +ink er +Ġst ing +ĠSadd am +ĠAR T +Ġtruth s +b irth +Ġso ber +ĠN it +Ġ ib +Ġus able +Ġst acks +ĠSy lv +Ġnort heast +Ġdom ination +ĠM our +EN SE +ĠMe asure +Ġprogram mer +Ġ< - +18 2 +ĠCond ition +Ġback yard +ir ling +ĠJ eb +ĠCre ed +ĠH ang +ĠCOM P +F ER +ĠIs h +Ġdetect ives +------------ --- +ĠMess enger +Ġlo oph +Ġgate way +15 1 +ĠMaterial s +ĠD T +Ġdo omed +od o +Ġslic es +Ġemail ed +ĠPer l +Ġren ov +UT H +ody nam +ĠSouth west +get ic +ĠT PP +Ġoptim ism +ĠT ow +ul ators +prot ected +y les + « +Ġex ile +en v +P rop +ĠZimmer man +Ù İ +C a +om aly +ãĥ Ĩ +Ġrail road +L ee +23 2 +Ġrepl icate +Ġcomfort ably +act ly +Ġr av +Ġtelesc ope +Ġhonest y +ĠPe pper +ĠBr ing +Ġric hest +Ġout doors +Ġh alls +Ġcont end +IS E +Ġsub mitting +Ġna ive +ar ations +Ġ14 3 +Ġpo ised +respons ible +Ġsoc ks +ĠSk ull +Quest ion +Ġdiscover ies +Jo ined +ĠEn emies +ĠWire less +ĠRe venge +Ġpuzz les +Ġce ased +29 0 +cript ions +ĠCon sole +Ġbo iling +Ġdisc rep +Ġded uction +Ġar senal +XX XX +ĠAm sterdam +rox imately +ĠSh ane +Ġpos ing +ĠACL U +ĠCompan ies +Ġthe ology +ĠU g +qu arter +ĠH ank +Co in +ĠL v +Ġalleg ation +ĠAv oid +Ġindef initely +Ġcommod ities +Ġbr ig +ĠMan it +Ġt enth +met hod +ĠKn icks +ĠâĢ İ +Ġinv oked +D ial +AR A +Ġc aucus +22 7 +ĠJ ab +Ġoun ces +b ay +Ġbud dy +f an +23 4 +ĠH il +ad h +ĠT Y +ĠIN D +Ġ19 39 +Ġiter ation +ĠGonz alez +ĠV ert +ĠI O +em b +re ra +en ch +ĠRequ irements +ĠW ins +Ġlivest ock +h ours +" â̦ +b ral +M arg +ĠD one +Ġwas ting +ing ed +g roups +Ġw ishing +ĠT umblr +Ġt apping +Ġnational ism +ĠB yr +Ġsqu ares +ĠAct ions +ãĥ ¥ +In side +deb ug +Ġapp end +Ġstub born +ĠC ind +T ell +Ġt earing +ĠRe y +or c +ĠDay ton +ĠN H +ĠMad ness +Ch arl +ĠMor rison +fil ter +Ġacc use +Ġ. / +Ġtor rent +Ġdecl ines +g allery +M ine +Ġneg otiation +ĠBash ar +op ia +19 93 +em ort +ĠNo vel +ĠF ang +ers ive +ĠInst ant +Ġroll er +A round +ĠElect ions +G ames +Ġin expensive +Ġwor s +Ġv ul +ĠH ole +Ġunbeliev able +Ġn ause +Ġent r +bo at +ĠST E +Ġbus h +ĠHass an +Ġw o +Ġpa used +ĠM ig +l ived +Ġsc out +Ġl ith +Pub lished +du ino +c ool +Ġcirc ulating +id as +ĠP am +viol ent +ĠCraw ford +udd le +ĠLet ters +Gu ard +mor ph +Ġwand ering +Ġsoph omore +Ġque er +ĠBl ind +r ue +ĠMar riage +D om +Ġpadd ing +Ġfold ers +Ġmeaning less +Ġcandid acy +af ort +Ġwhistle bl +ĠIdent ified +Ġcig ar +Ġh id +ĠDub ai +Ġpost ure +Ġh iking +ĠTermin al +Legend ary +ĠT P +ĠAT K +ĠStar bucks +ĠR iot +19 91 +ĠBott om +e ffic +ĠEug ene +ĠWy oming +ĠRock y +Ġsal mon +Ġmet ro +Ġb ilateral +Ġcelebr ates +L ength +b illion +B at +Ġre leg +Ġpse udo +D T +ĠRh ode +P arent +ple tion +Ġatt ribut +Ġtun ing +ĠNOT E +ĠRe bel +ic us +F und +Ġcock tail +Ġ5 01 +Ġsp oon +Ġbrut ality +Ġun ite +Ġmicro bi +ĠRe ich +pos itive +Ġam azed +ĠN T +D esc +ECT ION +Ġfalse ly +ĠHigh lander +ĠC rist +ĠVictor ian +Ġdistribut ions +the ir +ĠE instein +Ġp od +Ġepid em +Ġhe ap +ĠR anch +Ġan them +Ġre app +ĠAub urn +Ġconc urrent +ĠThrough out +ĠP OST +â ĺ +Ġhom emade +k ick +B eg +Ġch assis +c ounter +Ġmer ger +Ġl aps +2 17 +un ion +ĠTr igger +Ġdeb ated +Ġsil ently +Ġrest raint +B al +0000 000 +Ġform idable +ĠFil ip +Ġsacrific es +F ood +Ġdwar f +ĠSe qu +in ian +More over +Ġtang ible +ops is +ĠMine craft +ĠRegist ration +o an +Ġrepresent ations +Ġth irst +Ġcor p +ire ment +M ade +l oe +> " +c ats +* . +Ġgest ures +gener al +Le ague +Ġpack ets +ĠInspect or +ĠBer g +Ġfraud ulent +Ġcritic ize +F un +Ġbl aming +nd ra +Ġsl ash +ĠE ston +Ġpropos ing +Ġwh ales +Ġtherap ist +Ġsub set +Ġle isure +EL D +ĠC VE +ĠAct ivity +Ġcul min +sh op +ĠD AY +is cher +ĠAdmir al +ĠAtt acks +Ġ19 58 +Ġmem oir +Ġfold ed +Ġsex ist +Ġ15 3 +ĠL I +Ġread ings +Ġembarrass ment +ĠEmploy ment +w art +ch in +Ġcontin uation +l ia +Rec ently +Ġd uel +Ġevac uation +ĠKash mir +Ġdis position +ĠR ig +Ġbol ts +Ġins urers +4 67 +M ex +Ġret aliation +Ġmis ery +Ġunre asonable +r aining +I mm +ĠP U +em er +Ġgen ital +ãĤ ³ +ĠC andy +Ġon ions +ĠP att +lin er +Ġconced ed +Ġf a +Ġfor c +ĠH ernandez +ĠGe off +deb ian +ĠTe ams +Ġc ries +Ġhome owners +23 7 +A BC +Ġst itch +Ġstat istic +Ġhead ers +ĠBi ology +Ġmot ors +ĠG EN +ĠL ip +Ġh ates +Ġhe el +S elf +i pl +ED IT +ort ing +Ġann ot +ĠSpe ech +old emort +ĠJ avascript +ĠLe Bron +Ġfoot print +Ġf n +Ġseiz ures +n as +h ide +Ġ19 54 +ĠBe e +ĠDecl aration +ĠKat ie +Ġreserv ations +N R +f emale +Ġsatur ated +Ġb iblical +Ġtroll s +Dev ice +ph otos +Ġdr ums +ãĥīãĥ© ãĤ´ãĥ³ +N ight +f ighter +ĠH ak +ri ber +Ġc ush +Ġdiscipl inary +ba um +ĠG H +ĠSch midt +ilib rium +Ġs ixty +ĠKush ner +ro ts +Ġp und +ĠR ac +Ġspr ings +Ġcon ve +Bus iness +F all +Ġqual ifications +Ġvers es +Ġnarc iss +ĠK oh +ĠW ow +ĠCharl ottesville +ed o +Ġinterrog ation +ĠW ool +36 5 +B rian +Ġâľ ĵ +Ġalleg es +ond s +id ation +ĠJack ie +y u +Ġl akes +Ġworth while +Ġcryst als +ĠJud a +Ġcomp rehend +Ġfl ush +Ġabsor ption +ĠO C +Ġfright ened +ĠCh ocolate +Mart in +Ġbu ys +Ġbu cks +Ġapp ell +ĠChampions hips +Ġlist ener +ĠDef ensive +Ġc z +ud s +ĠM ate +Ġre play +Ġdecor ated +Ġs unk +ĠV IP +ĠAn k +Ġ19 5 +aa aa +Nob ody +ĠMil k +ĠG ur +ĠM k +ĠS ara +Ġse ating +ĠW id +Tr ack +Ġemploy s +Ġgig antic +AP P +ãĤ § +in ventory +Ġtow el +at che +l asting +ĠT L +Ġlat ency +Ġkn e +B er +me aning +Ġup held +Ġplay ground +Ġm ant +S ide +Ġstere o +Ġnorth west +Ġexception ally +Ġr ays +Ġrec urring +D rive +Ġup right +Ġab duct +ĠMar athon +Ġgood bye +Ġal phabet +h p +Ġcourt room +ring ton +ot hing +T ag +Ġdiplom ats +Ġbar bar +ĠAqu a +18 3 +33 33 +Ġmat urity +Ġinst ability +ĠAp ache +Ġ= == +Ġfast ing +ĠGr id +Mod Loader +Ġ15 2 +A bs +ĠOper ating +ett i +Ġacqu aint +Don nell +ĠK em +ĠFor ge +Ġarm ored +M il +Ġphilos ophers +in vest +Pl ayers +â Ī +Ġmy riad +Ġcomr ades +R ot +Ġremember ing +Ġcorrespond s +Ġprogram mers +ĠLyn n +Ġo lig +Ġco herent +yn chron +ĠChem ical +Ġj ugg +p air +post s +E ye +ĠIn ner +Ġsem ester +ott est +ĠEmir ates +ric anes +or ously +m its +ĠW is +Ġd odge +l ocation +Ġf aded +Am azon +ĠPro ceed +ĠIN FO +j ournal +ĠTru ck +T en +Ġ2 17 +Ġstat utes +m obile +ĠT ypes +Rec omm +b uster +pe x +Ġleg ends +Ġhead ache +f aced +ĠWi Fi +if ty +ĠH ER +Ġcirc uits +ER ROR +22 6 +ol in +Ġcyl inder +osp ace +ik ers +P rem +Qu ant +Ġconflic ting +Ġslight est +Ġfor ged +ion age +Step hen +ĠK ub +ĠOpp ortun +ĠHe al +Ġbl o +Ġrul ers +Ġh uh +Ġsubmar ine +f y +ass er +Ġallow ance +ĠKas ich +ĠT as +ĠAustral ians +Forge ModLoader +ĠâĨ ij +ĠMat rix +am ins +Ġ12 00 +ĠAc qu +23 6 +D ocument +ĠBre aking +19 3 +ĠSub st +ĠRoll er +ĠPro perties +ĠN I +t ier +Ġcr ushing +Ġadvoc ating +Further more +keep ers +Ġsex ism +x d +Ġcall er +ĠS ense +chie ve +ĠT F +Ġfuel ed +Ġreminis cent +Ġobs ess +ur st +Ġup hold +ĠF ans +het ics +Ġâ Ĺ +ĠB ath +Ġbe verage +Ġo scill +25 4 +Ġpol es +Ġgrad ual +Ġex ting +ĠS uff +ĠS uddenly +Ġlik ing +Ġ19 49 +un ciation +am ination +ĠO mar +ĠL V +ĠCon sequently +Ġsynt hes +ĠG IF +Ġp ains +Ġinteract ing +u ously +inc re +Ġrum or +ĠScient ology +19 7 +ĠZ ig +Ġspe lling +ĠA SS +Ġexting u +ms on +Ġg h +Ġremark ed +ĠStrateg ic +ĠM ON +å ¥ +g ae +ĠWH AT +E ric +ĠCamp us +Ġmeth ane +Ġimag in +J UST +ĠAl m +X T +i q +ĠR SS +Ġwrong doing +att a +Ġbig ot +Ġdemonstr ators +ĠCal vin +ĠV illa +Ġmembr ane +ĠAw esome +Ġbenef ic +26 8 +Ġmagn ificent +ĠL ots +G reg +ĠBor is +Ġdetain ees +ĠH erman +Ġwhis pered +Ġa we +Prof essor +fund ing +Ġphys iological +ĠDest ruction +Ġlim b +Ġmanip ulated +Ġbub bles +Ġpse ud +Ġhyd ra +ĠBrist ol +Ġst ellar +ĠExp ansion +ĠK ell +ĠInterest ingly +Ġm ans +Ġdrag ging +Ġec ological +ĠF it +Ġg ent +Ġbenef ited +ĠHait i +Ġpoly g +ãĥ İ +Ġ20 30 +Ġpro w +Ġrecon struction +Ġwas t +Ġpsych ic +ĠGree ks +Hand ler +16 2 +ĠP ulse +Ġsol icit +Ġsy s +Ġinflu x +ĠG entle +per cent +Ġprolifer ation +Ġtax able +Ġdisreg ard +Ġesc aping +Ġg inger +Ġwith stand +Ġdevast ated +ĠD ew +ser ies +Ġinject ed +ela ide +Ġturn over +he at +Ļ Ĥ +H appy +ĠSil ent +ãĤ Ń +iv ism +Ġir rational +AM A +Ġre ef +r ub +Ġ16 2 +Ġbank ers +ĠEth ics +v v +Ġcritic isms +K n +18 6 +M ovie +ĠT ories +Ġno od +Ġdist ortion +F alse +od ore +Ġt asty +Res earch +ĠU ID +- ) +Ġdivor ced +ĠM U +ĠHay es +ĠIs n +ian i +ĠH Q +Ġ" # +ign ant +Ġtra umatic +ĠL ing +H un +Ġsab ot +on line +r andom +Ġren amed +ra red +K A +d ead +é t +ĠAss istance +Ġse af +++++ ++++ +Ġse ldom +ĠWeb b +Ġbo olean +u let +Ġref rain +ĠDI Y +ru le +Ġshut ting +Ġutil izing +load ing +ĠPar am +co al +oot er +Ġattract ing +ĠD ol +Ġher s +ag netic +ĠRe ach +im o +Ġdisc arded +ĠP ip +01 5 +ü r +Ġm ug +Im agine +C OL +Ġcurs ed +ĠSh ows +ĠCurt is +ĠSach s +spe aking +ĠV ista +ĠFram ework +ong o +Ġsub reddit +Ġcr us +ĠO val +R ow +g rowing +Ġinstall ment +Ġgl ac +ĠAdv ance +EC K +ĠLGBT Q +LE Y +Ġac et +Ġsuccess ive +ĠNic ole +Ġ19 57 +Qu ote +Ġcircumst ance +ack ets +Ġ14 2 +ort ium +Ġguess ed +ĠFr ame +Ġperpet rators +ĠAv iation +ĠBen ch +Ġhand c +A p +Ġ19 56 +25 9 +r and +Net Message +d in +urt les +h ig +ĠV III +ff iti +ĠSw ords +b ial +Ġkidn apping +dev ice +Ġb arn +ĠEl i +auc as +S end +Con structed +Ġ ½ +Ġneed les +Ġad vertisements +Ġv ou +Ġexhib ited +ĠFort ress +As k +B erry +TY PE +Ġcan cers +ump ing +ĠTerrit ory +Ġpr ud +Ġn as +Ġathe ist +Ġbal ances +ãģ Ł +ĠSh awn +& & +Ġland sc +ĠR GB +Ġpet ty +Ġex cellence +Ġtransl ations +Ġpar cel +ĠChe v +E ast +ĠOut put +im i +Ġamb ient +ĠTh reat +Ġvill ains +Ġ5 50 +IC A +Ġtall er +Ġle aking +c up +Ġpol ish +Ġinfect ious +ĠK C +Ġ@ @ +back ground +Ġbureaucr acy +ĠS ai +un less +it ious +ĠSky pe +At l +ID ENT +00 8 +Ġhyp ocr +Ġpit chers +Ġguess ing +ĠF INAL +Bet ween +Ġvill agers +Ġ25 2 +f ashion +ĠTun is +Be h +ĠEx c +ĠM ID +28 8 +ĠHas kell +19 6 +ĠN OR +Ġspec s +Ġinv ari +Ġgl ut +ĠC ars +Ġimp ulse +Ġhon ors +g el +Ġjurisd ictions +ĠBund le +ul as +Calif ornia +ĠIncre ase +Ġp ear +Ġsing les +Ġc ues +Ġunder went +ĠW S +Ġexagger ated +Ġdub ious +Ġfl ashing +L OG +) ]. +J ournal +t g +V an +ĠI stanbul +ĠIn sp +ĠFrank en +D raw +Ġsad ness +Ġiron ic +ĠF ry +x c +Ġ16 4 +is ch +W ay +ĠProtest ant +h orn +Ġun aff +ĠV iv +ill as +ĠProduct ions +ĠH ogan +Ġper imeter +ĠS isters +Ġspont aneous +Ġdown side +Ġdescend ants +Ġor n +w orm +Japan ese +Ġ19 55 +Ġ15 1 +ĠDo ing +els en +umb les +Ġrad ically +ĠDr um +ĠB ach +Ġli abilities +ĠO B +ĠElement ary +Ġmem e +yn es +Ġfinger print +ĠGr ab +Ġundert ake +Mem bers +ĠRead er +ĠSim s +g od +Ġhypot hetical +s cient +ĠA J +Ġchar ism +Ġad missions +ĠMiss ile +tr ade +Ġexerc ising +ĠBack ground +W ritten +Ġvoc als +whe ther +Ġv i +ĠW inner +Ġl itter +ĠSh ooting +ST EM +ãĤ ¡ +ĠA FL +Ġvari ability +Ġe ats +ĠD PS +b row +Ġeleph ants +Ġstr at +Ġ Å +Ġsett lers +Matt hew +Ġin advert +H I +ĠIM F +ĠGo al +Ġnerv es +John son +ey e +ablish ment +Th ursday +BIL ITY +H ad +am oto +het amine +ep s +Ġmit ochond +Ġcomp ressed +ĠTre vor +ĠAnim als +T ool +L ock +Ġtwe ak +Ġpin ch +Ġcancell ation +P ot +Ġfoc al +ĠAst ron +17 3 +ĠA SC +ĠO THER +umn i +Ġdem ise +d l +Ù ħ +Sem itism +Ġcr acking +Ġcollabor ative +Ġexpl ores +s ql +Ġher bs +Ġconfig urations +m is +ĠRes ult +ace y +ĠSm oke +Ġsan ct +el ia +Ġdeg ener +Ġdeep est +Ġscream ed +Ġn ap +Soft ware +ĠST AR +E F +ĠX in +spons ored +mans hip +23 3 +Ġprim aries +Ġfilter ing +Ġas semble +m il +ĠMy ers +b ows +Ġpun ched +M ic +Ġinnov ations +Ġfun c +and o +Ġfr acking +ĠV ul +о Ð +osh op +ĠIm mun +Ġsett ling +Ġadolesc ents +Ġreb uilding +Ġtransform ing +Ġpar ole +Ġhar bor +Ġbook ing +ot ional +onge vity +ĠY o +b ug +Ġemer ges +ĠMethod s +ĠCh u +P res +ĠDun geons +Ġtra iling +ĠR um +ĠH ugh +å¤ © +ĠE ra +ĠBatt les +Res ults +ĠTr ading +Ġvers a +c ss +ax ies +he et +Ġgre ed +19 89 +Ġgard ens +Ġconting ent +P ark +ĠLeaf s +h ook +ro be +Ġdiplom acy +ĠF uel +ĠInv asion +Ġupgr ading +M ale +Ġe lic +Ġrelent less +ĠCo venant +ap esh +ĠT rop +T y +pro duction +art y +Ġpun ches +ak o +cyclop edia +ĠR abbit +ĠHD MI +Ġ14 1 +Ġf oil +Item Image +ĠF G +Ġimplement ations +ĠP om +ixt ures +Ġaw ait +Ġ3 30 +am us +Ġumb rella +Ġfore see +se par +Ġcircum cision +Ġperipher al +S ay +ĠExper t +In c +Ġwithd rew +ĠAnd ers +f ried +Ġradio active +ĠOp ening +Ġboard ing +ĠN D +Ġover throw +Act iv +W P +ĠAct s +× Ļ +Ġmot ions +v ic +ĠM ighty +ĠDef ender +a er +Ġthank ful +ĠK illing +ĠBr is +mo il +Ġpredict ing +26 6 +ch oice +Ġkill ers +Ġinc ub +ĠChe st +ather ing +Ġpro claimed +fl ower +oss om +umbled ore +ĠCy cling +ĠOccup y +AG ES +P en +ĠY ug +Ġpack aged +Ġheight ened +c ot +st ack +C ond +Ġst amps +m age +Ġpersu aded +Ġens l +ĠCard inal +Ġsol itary +Ġpossess ing +ĠC ork +Ġev id +ĠT ay +Ġbl ues +Ġextrem ism +Ġlun ar +Ġcl own +Te chn +Ġfest ivals +ĠPv P +ĠL ar +Ġconsequ ently +p resent +Ġsom eday +ç İĭ +ĠMet eor +Ġtour ing +c ulture +Ġbe aches +S hip +c ause +ĠFl ood +ãĥ ¯ +Ġpur ity +th ose +Ġem ission +b olt +Ġch ord +ĠScript ure +L u +Ġ$ { +cre ated +Other s +25 8 +Ġelement al +Ġannoy ed +ĠA E +d an +ĠS ag +Res earchers +Ġfair y +âĢĵ âĢĵ +======== ==== +Sm art +GG GG +Ġskelet ons +Ġpup ils +link ed +Ġur gency +en abled +ĠF uck +Ġcoun cill +r ab +U AL +T I +Ġlif es +Ġconf essed +B ug +Ġharm on +ĠCON FIG +ĠNe utral +D ouble +Ġst aple +ĠSH A +Brit ish +ĠSN P +AT OR +oc o +Ġswing ing +ge x +ole on +pl ain +ĠMiss ing +ĠTro phy +v ari +ran ch +Ġ3 01 +4 40 +00000000 00000000 +Ġrest oring +Ġha ul +uc ing +ner g +Ġfut ures +Ġstrateg ist +quest ion +Ġlater al +ĠB ard +Ġs or +ĠRhod es +ĠD owntown +????? - +ĠL it +ĠB ened +Ġco il +st reet +ĠPort al +FI LE +ĠG ru +* , +23 1 +ne um +Ġsuck ed +Ġr apper +Ġtend encies +ĠLaure n +cell aneous +26 7 +Ġbrow se +Ġover c +head er +o ise +Ġbe et +ĠG le +St ay +Ġm um +Ġtyp ed +Ġdiscount s +T alk +ĠO g +ex isting +ĠS ell +u ph +C I +ĠAust rian +ĠW arm +Ġdismiss al +Ġaver ages +c amera +Ġalleg iance +L AN +=" # +Ġcomment ators +ĠSet ting +ĠMid west +Ġpharm ac +ĠEX P +Ġstain less +Ch icago +Ġt an +24 4 +Ġcountry side +ĠV ac +29 5 +Ġpin ned +Ġcr ises +Ġstandard ized +T ask +ĠJ ail +ĠD ocker +col ored +f orth +" }, +Ġpat rons +Ġsp ice +Ġm ourn +ĠM ood +Ġlaund ry +Ġequ ip +ĠM ole +y ll +ĠTH C +n ation +ĠSher lock +Ġiss u +ĠK re +ĠAmeric as +ĠA AA +Ġsystem atically +Ġcont ra +ĠS ally +Ġrational e +Ġcar riage +Ġpe aks +Ġcontrad iction +ens ation +ĠFail ure +Ġpro ps +Ġnames pace +Ġc ove +field s +ãĤ ĭ +Ġw ool +ĠC atch +Ġpresum ed +ĠD iana +r agon +ig i +Ġh amm +Ġst unt +ĠG UI +ĠObserv atory +ĠSh ore +Ġsmell s +ann ah +Ġcock pit +ĠD uterte +8 50 +Ġopp ressed +bre aker +ĠCont ribut +ĠPer u +ĠMons anto +ĠAtt empt +Ġcommand ing +Ġfr idge +ĠR in +ĠChe ss +ual ity +Ġo l +Republic an +ĠGl ory +ĠW IN +.... ... +ag ent +read ing +Ġin h +J ones +Ġcl icks +al an +Ġ[ ]; +ĠMaj esty +ĠC ed +op us +ate l +à ª +AR C +ĠEc uador +ãĥ ł +ĠK uro +Ġritual s +Ġcapt ive +Ġoun ce +Ġdisag reement +Ġsl og +f uel +P et +M ail +Ġexerc ised +Ġsol ic +Ġrain fall +Ġdev otion +ĠAss essment +Ġrob otic +opt ions +ĠR P +ĠFam ilies +ĠFl ames +Ġassign ments +00 7 +aked own +Ġvoc abulary +Re illy +Ġc aval +g ars +Ġsupp ressed +ĠS ET +ĠJohn s +Ġwar p +bro ken +Ġstat ues +Ġadvoc ated +Ġ2 75 +Ġper il +om orph +ĠF emin +per fect +Ġh atch +L ib +5 12 +Ġlif elong +3 13 +Ġche eks +Ġnum bered +ĠM ug +B ody +ra vel +We ight +ĠJ ak +ĠHe ath +Ġkiss ing +ĠJ UST +Ġw aving +u pload +Ġins ider +ĠPro gressive +ĠFil ter +tt a +ĠBe am +Ġviol ently +ip ation +Ġskept icism +Ġ19 18 +ĠAnn ie +ĠS I +Ġgen etics +Ġon board +at l +ĠFried man +ĠB ri +cept ive +Ġpir ate +ĠRep orter +27 8 +Ġmyth ology +Ġe clipse +Ġsk ins +Ġgly ph +ing ham +F iles +C our +w omen +Ġreg imes +Ġphotograp hed +K at +ĠMA X +Offic ials +Ġunexpected ly +Ġimpress ions +F ront +;;;; ;;;; +Ġsuprem acy +Ġs ang +Ġaggrav ated +Ġabrupt ly +ĠS ector +Ġexc uses +Ġcost ing +ide press +St ack +ĠR NA +ob il +Ġghost s +ld on +at ibility +Top ics +Ġreim burse +ĠH M +ĠDe g +Ġth ief +y et +ogen esis +le aning +ĠK ol +ĠB asketball +Ġf i +ĠSee ing +Ġrecy cling +Ġ[ - +Cong ress +Ġlect ures +P sy +Ġne p +Ġm aid +Ġori ented +A X +Ġrespect ful +re ne +fl ush +ĠUn loaded +re quest +gr id +ĠAltern atively +ĠHug o +Ġdec ree +ĠBuddh ism +and um +And roid +ĠCong o +ĠJoy ce +Ġacknowled ging +hes ive +ĠTom orrow +ĠH iro +th ren +ĠM aced +Ġho ax +ĠIncre ased +ĠPr adesh +W ild +____ __ +16 1 +Ġa unt +Ġdistribut ing +ĠT ucker +ĠSS L +ĠW olves +B uilding +ou lt +ĠLu o +ĠY as +ĠSp ir +ĠSh ape +ĠCamb od +ĠIP v +Ġm l +Ġext rad +39 0 +ĠPenn y +d ream +Ġstation ed +opt ional +ew orthy +. </ +Ġundert aking +Ġchick ens +Ġstimul i +ĠEl se +ig ators +ĠBegin ning +ct ory +Ġprep ares +Ġdel ta +Ġvic inity +t ool +Ġworks hops +M Hz +Ġaccus ation +Ġhist ories +rop olis +ĠChurch ill +Ġne on +Ġb aff +d ies +may be +Ġè£ı è¦ļéĨĴ +Ġsympt om +EC H +ĠMan uel +Ġban ana +ĠH B +Ġ **** +ĠKore ans +c oll +F B +Ġpr aying +ĠCann ot +ĠM ile +Ġembr acing +ĠSil k +39 3 +ot ers +F D +Ġday light +al ias +ĠBrig ade +ĠHann ah +Ġcler gy +Ġs outheast +Ġalcohol ic +Ġpropos es +liv ion +Ġcalcul ating +Ġstim ulate +Ġspl itting +e ight +ĠInd y +pl ays +ĠP ik +Ġdom est +Ġforg iveness +ĠR ings +pat ient +kins on +M ont +ig ible +; " +Ġperiod ically +amm ad +ĠBr itt +p ard +Ġarbit ration +ĠSchne ider +ĠCorpor ate +ĠMay a +Ġsn akes +a um +Ġbl asted +Ġmyster ies +Ġrev ive +oc amp +ĠD odge +ĠOper a +27 9 +Ġor phan +Ġspec ifies +ĠM ets +D uration +H en +Ġfire works +Ġprosec ute +ĠTill erson +d p +us age +l iness +ĠDeb ian +Ġ2 24 +ris es +ĠIn fect +at ra +ĠR R +ĠL or +d iff +ĠCharl eston +Ġac oustic +Ġam use +3 30 +Ġc er +ĠT ac +Ġ[ + +Ġcard iac +ĠRestaur ant +er gy +Ġf uzz +Ġbit es +Ġhazard ous +Ġbr ighter +r ans +ĠStephan ie +ext ra +RE T +ĠChrist ine +ĠS ue +stat ement +Ġbol ster +Ġant it +Rad io +B IT +ãĤ ° +Ġvis ions +ĠCon cept +Ġin line +ĠPhilos ophy +is ans +ĠIr ving +à £ +t aking +Ġincons ist +ĠKum ar +Ġl ig +ĠSch umer +ĠReg ulations +ĠH z +th ro +ĠV oldemort +ĠM ED +ĠFreder ick +P ad +22 1 +Ġalleg ing +ĠCommun ication +Ġ16 7 +Ġforecast s +Ġsp iders +Or gan +ĠParticip ants +ĠO ps +des ign +Cl ose +Ġfact o +Ġbom bers +res istant +ateg ories +S chool +Ġhom ework +Ġcor ro +T uesday +ĠBrend an +ĠM X +ĠT S +ĠSt ri +Ġstake holders +ĠMillenn ium +Ġtransfer ring +J ud +Ġt ac +Ġ16 00 +ĠSD K +r b +Ġinterpret ations +ĠS G +Ġup stairs +ĠHar vest +Ġvag ina +Ġing est +x f +ĠOr ion +ĠJoe y +Ġsand wic +Ġimm ortal +Ġfl ipped +ort ex +threat ening +Ġsn iper +Ġconver ts +Ġinstall ations +ĠBul gar +ors che +m ails +Ġl ure +Ġnarrow ly +Ġgren ade +ĠG ing +Ġunder wear +------------ -- +Ġch ased +ĠV AL +Ġparent ing +ĠH amb +ĠBl az +Ġanarch ist +ĠMed ian +ĠProgram s +Î ½ +Ġob j +ĠN okia +orm an +an qu +at ism +op a +Ġfulf illing +Ġpupp y +Ġent it +ĠSebast ian +Ġshoot ers +Ġric her +è ¡ +Ġtempt ed +ĠAT T +ĠC V +Ġto re +Res ource +ĠDevil s +40 8 +in ational +Ġass urance +ĠDar ren +Ġwh ichever +pos ure +Ġf ury +St ock +Ġunivers ally +resp onse +Ġo ak +Ġwork load +ĠCor ner +ee le +" ... +Ġdepri ved +k owski +Ġcast s +Ġaffili ation +ĠA ch +ĠAs ked +at he +Ġl act +ĠTh u +r m +Ġair lines +Ġnot ions +Form at +ĠF AA +ãĥ Ĭ +dri ver +Ġtrans cend +S ettings +ĠPro secut +Ġsp inal +Ġdefault s +F K +Ġpref ers +rend ered +th us +fil m +Ġt iger +ĠSp icer +rec ogn +ĠRug by +Net work +Ġp ity +Ġcomp artment +c asters +ĠMon roe +Ġ7 20 +Ġcorrect ions +Ġdop amine +ĠA Z +C ut +Ġro omm +Ġspec ulate +H ash +Ġrestrict ive +11 11 +red ible +on el +Ġramp ant +re ported +ĠSu ite +ĠMin imum +al ys +az ard +lo op +Ġl ent +sh a +Ġv andal +men u +ĠBoe hner +Ġnarr atives +Ġauthent icity +26 9 +an ic +d uty +28 5 +Ġthank ed +Ġbetray ed +l ift +Ġsouth west +ĠDex ter +ĠB od +Ġkey words +A verage +D IS +Ġethnic ity +! ), +ĠNational s +á ¹ +ĠT ah +iox id +Ġwid get +Ġpast a +Ġbill ing +Ġtr ilogy +ĠL ines +Ġsn iff +Ġnep hew +L ate +Ġprinc ip +ĠLo op +ĠMarx ist +Ġdiss olved +Ġcontext s +ĠAm ount +ĠSp ike +Ġtot als +Ġorgan izer +Ġup rising +s hips +Y Y +ĠNort heast +m oney +grad ation +Ġgoal keeper +ĠH ear +Ġste ak +ĠBuzz Feed +Ġsole mn +ĠSc and +Ġpo pping +Ġad here +ĠAl leg +by te +ĠW olver +Ġun in +Ġrec ol +it ud +Ġmim ic +ib us +Ġpredict s +ĠKee per +i ating +Ġde ception +Ġlear nt +Ġdi ary +Ġcond itional +Ġre lic +Ġinv oke +ien ced +å Ī +ĠP ont +Ġcell phone +Ġspeed ing +Ġtack ling +Ġn ude +op ened +ĠMan afort +Ġ19 52 +Ġmaj ors +ĠSil ence +Ġlog istics +Ġweight ed +ĠPsych iat +": [" +Ġsick ness +Ġdivid ends +z on +Re lease +ĠKe ys +ĠI ch +Ġen z +ĠF ernand +ĠÎ ± +Ġmean ings +Ġp enny +Ġst ern +Ġl ar +ĠPub lished +Ġback drop +K im +ĠSy nt +Ġdeb uted +w m +ĠIs le +Ġregul ating +ott i +ĠSch olars +ices ter +ĠChe f +Ġpop s +ĠLaun cher +ĠVar ious +Ġcomment ing +os lav +enz ie +Ġrival ry +â Ĥ¬ +Re ally +Ġor c +Ġbe an +ĠJud y +Not ice +ĠB ike +? ] +Ġrent ed +st en +Ġfore front +ĠBald win +Ġyield ed +t ails +Pr ime +ĠS ources +ic ator +Se an +Ġmarch ing +Out put +ĠJ ungle +Ġres ide +zz le +ĠAndrew s +Ġtor que +Bas ic +Act ually +st rap +p enter +Ġexam s +ĠY a +Ġ15 9 +ĠDec ision +Ġr ansom +ete enth +ens ing +2 13 +Ġsun set +40 4 +ĠRap id +ĠHe in +ĠAb original +Ġorgan ism +ĠS ever +Ġcl a +aj i +Sim ple +ĠFl avor +ĠE val +pr us +Ġch orus +D AY +Ġden ounced +Ġbi ography +ĠTurn bull +Rec ent +N ormal +lect ions +W ord +Ġf erry +ĠWag ner +h om +Un it +Ġsuper market +ĠS ith +Ġnomine es +Ġdictators hip +idd ler +Ġannoun ces +ĠThe m +ĠNept une +Ġde ity +ĠY i +Ġmon arch +AR R +Ġinv aded +ĠH ok +unt ary +C ertain +eg a +Ġk idding +ĠReg ulation +Ġtr ay +Ġphotograp hers +ĠArc ane +Ġdis charged +Ġevangel ical +Ġinter change +Ġfilm maker +ĠEnd less +Ġ29 0 +ĠSalv ador +AS Y +ĠSign al +Ġwr ath +â ľ +l ot +' / +Ġproject ile +Ġemploy ing +ĠInter face +19 1 +atell ite +ĠR ath +pack age +Ġindic ations +J ason +Ġarg s +ĠG Hz +Ġt ilt +n ants +w on +ãĤ µ +red d +res cent +ĠCal endar +Ġmod ular +Ġassist ing +Ġred eem +ĠBe an +Ġwor sh +Ġdecentral ized +) ... +37 7 +Ġarr ays +Ġaccomplish ments +Î ¿ +d ot +Ġmut ually +Ġob struct +Ġmis represent +ore st +ion ic +ru ce +% ; +Ġknow ingly +port ing +in ently +A ri +ĠSch ultz +D a +ĠC ere +Ġob solete +ħ ĭ +g ive +Ġb ait +Ġen larg +Ne ill +Ġ19 33 +Ġrecons ider +ĠSerge ant +ĠDian e +ĠC ogn +ĠI con +P osition +Ġf ost +Ġstir ring +se ven +ĠSpace X +ugg ets +Ġmed d +G al +ĠS ister +B oy +Ġtrigger ing +T aking +Ġscream s +Ġca usal +Ġaw aken +Ar m +29 7 +Ġdisp atched +ĠF ALSE +Ġorgan izational +ĠT ong +Ġdile mma +d emon +S pl +Ġhook s +ud ing +Ġvalid ate +Ġpot ion +Ġcl aw +Ġburg l +Ġqu ir +AC A +ĠBren nan +Ġdur ability +Ġbomb ings +ĠWind ow +Ġculp rit +3 25 +There fore +umb ered +per formance +w arts +Ġen forcing +ĠBl ow +Ġre print +if ax +al pha +Ġsin ister +Ġbur ger +fight ing +Sc ore +ĠSt ones +i em +40 5 +che my +Ġvine gar +n om +Ġprev ailing +ĠLat est + ¶ +Ġb a +ĠWrit er +Ġ17 7 +ĠCon way +Ġcollect s +Ġquant itative +Ġhor rors +og ens +ĠSl ov +Ġl ays +h aw +ĠSl ash +Ġnight club +ĠDav ies +Ġbr ide +ĠScar let +y mm +ĠApplic ations +vel ength +Ġrev ival +Ġsoft ly +Ġz oo +ita ire +C ur +Ġelect rom +Ġplant ing +OT O +ĠE lements +Ġsw allow +por ter +Ġlapt ops +Ġpe anut +Ġlobby ists +Î ² +Pan el +ĠJo an +im il +t nc +Ġresist ed +Ġout we +Ġret aining +at ri +Ġpo orer +ĠSyri ans +ĠHam mond +Ġwe ld +ud er +top ic +ĠT T +ric ia +Ġth ieves +L ic +ĠG ust +ĠW ays +are th +24 3 +Ġbroad caster +sh ield +ass ium +ub le +Ġairst rikes +on so +Ġped al +Ġcollect ors +ĠV ander +ĠMes a +Ġdict ator +Ġd ir +ent on +c art +sc ore +ad der +C ry +Ġs sh +gg er +Ġdrunk en +ĠG S +ĠSe at +Ġcorner back +Ġsk ipped +ĠRes earchers +ĠAud i +Ref erence +Ġhaun ted +à « +ĠClin ic +c z +Ġp s +ĠPal adin +ĠRec ipe +Ġst igma +opp y +Ġmon keys +ĠHaw k +S ad +" /> +ĠWorks hop +ĠRet ail +ĠAv atar +6 25 +N a +ĠV C +ĠSec ure +M Y +19 88 +oss ip +Ġpro state +Ġund en +Ġg amer +ĠCont ents +ĠWar hammer +ĠSent inel +3 10 +Ġse gregation +ĠF lex +ĠM AY +Ġdr ills +ĠDrug s +Islam ic +Ġsp ur +Ġca fe +Ġimag inary +Ġgu iding +Ġsw ings +ĠThe me +ob y +Ġn ud +Ġbe gging +Ġstr ongh +Ġreject ing +Ġpedest rians +ĠPro spect +R are +s le +Ġconcess ions +ĠConst itutional +Ġbe ams +Ġfib ers +p oon +Ġinstinct s +pro perty +ĠB IG +Sand ers +im ates +Ġco ating +Ġcorps es +ĠTR UE +check ed +Ġ16 6 +A sh +ĠJ S +ĠF iction +Ġcommun al +Ġener getic +oooo oooo +Ġnow adays +IL D +ib o +ĠSU V +R en +Ġdwell ing +Sil ver +Ġt ally +ĠM oving +Ġcow ard +Ġgener als +Ġhorn s +Ġcirc ulated +Ġrob bed +ĠUn limited +Ġharass ed +Ġinhib it +Ġcomp oser +ĠSpot ify +Ġspread s +3 64 +Ġsu icidal +Ġno ises +ĠSt ur +Ġs aga +ĠK ag +is o +Ġtheoret ically +M oney +Ġsimilar ity +Ġslic ed +ut ils +ing es +" - +Ġan th +Ġimp ed +Mod ule +Through out +Ġmen us +comm ittee +and i +ob j +in av +f ired +ĠAb dullah +Ġund ead +Ġfont s +H old +EN G +Ġsustain ability +Ġfl ick +Ġr azor +ĠF est +ĠChar acters +Ġword ing +Ġpopul ist +Ġcritic izing +Ġm use +v ine +Ġcard board +Ġkind ly +Ġfr inge +ĠThe ft +icult ural +Ġgovern ors +Ġ ���� +Ġ16 3 +Ġtime out +ĠA uth +Child ren +A U +Ġred emption +ĠAl ger +Ġ19 14 +Ġw aved +Ġastron auts +og rams +Ġsw amp +ĠFinn ish +Ġcand le +Ġton nes +ut m +Ġr ay +Ġsp un +Ġfear ful +art icles +Ġca us +or ically +ĠRequ ires +ĠG ol +Ġpop e +Ġinaug ural +Ġg le +AD A +ĠIS IL +ĠOff ensive +Ġwatch dog +Ġbal con +ent ity +ĠH oo +Ġgall on +AC C +Ġdoub ling +Ġimpl ication +ĠS ight +Ġdoct r +---- --- +Ġ\ \ +Ġm alt +R oll +Ġâī ¥ +Ġrec ap +add ing +u ces +ĠB end +fig ure +Ġtur key +Ġsoc ietal +ĠT ickets +Ġcommer cially +Ġsp icy +Ġ2 16 +ĠR amp +Ġsuperior ity +à ¯ +ĠTr acker +C arl +ĠC oy +ĠPatri ot +Ġconsult ed +Ġlist ings +Ġsle w +reens hot +ĠG one +Ġ[ ...] +30 9 +Ġh ottest +Ø ± +Ġrock y +ĠD iaz +Ġmass age +Ġpar aly +Ġp ony +A z +Ġcart ridge +ĠN Z +Ġsn ack +ĠLam ar +ple ment +ĠLes lie +Ġm ater +Ġsn ipp +24 6 +Ġjoint ly +ĠBris bane +ĠiP od +Ġpump ing +Ġgo at +ĠSh aron +eal ing +Ġcor on +Ġan omal +rah im +ĠConnect ion +Ġsculpt ure +Ġsched uling +ĠD addy +at hing +Ġeyeb rows +Ġcur ved +Ġsent iments +Ġdraft ing +D rop +( [ +Ġnom inal +ĠLeaders hip +ĠG row +Ġ17 6 +Ġconstruct ive +iv ation +Ġcorrupt ed +ger ald +ĠC ros +ĠChe ster +ĠL ap +ãģ ª +OT H +D ATA +Ġal mond +pro bably +I mp +Ġfe ast +ĠWar craft +F lor +Ġcheck point +Ġtrans cription +Ġ20 4 +Ġtwe aks +Ġrel ieve +S cience +Ġperform er +Z one +Ġtur moil +ig ated +hib it +ĠC afe +the med +Ġflu or +ben ch +Ġde com +ĠU nt +ĠBar rett +ĠF acts +Ġt asting +ĠPTS D +ĠSe al +ĠJuda ism +ĠDynam ic +ĠC ors +V e +ĠM ing +ĠTrans form +v on +ĠDef enders +ĠTact ical +ĠV on +ĠUn ivers +Ġdist orted +ĠB reath +?' " +Ġag on +ĠDead ly +Ġl an +ĠCy cle +orn ed +Ġrel iably +Ġgl or +ĠMon key +ãĥ ¡ +Ġad ren +Ġmicrow ave +ĠAl ban +irc raft +dig it +sm art +ĠD read +¯¯¯¯¯¯¯¯ ¯¯¯¯¯¯¯¯ +{ { +ĠRoc hester +Ġsimpl ified +Ġinf licted +Ġtake over +Ġyour selves +ad itional +Ġmus cular +K S +Ġing en +T ax +ĠFe ature +27 7 +Ġcru c +Ġcr ate +Ġun identified +Ġacclaim ed +ĠM anga +ĠFr ances +ĠNep al +ĠG erald +ĠKu wait +Ġsl ain +ĠHe b +ĠG oku +ãģ® æ +28 6 +M rs +ĠC ody +ĠSan ctuary +01 6 +Ġdism ant +Ġdatas et +ĠH ond +b uck +ĠPat terson +Ġpal ette +ĠG D +ic ol +ĠL odge +Ġplanet ary +ak in +ĠRegist ered +ab we +ĠPeters burg +Ġha iled +ĠP iece +S che +ĠDO J +Ġen umer +18 1 +ĠObs erver +ĠB old +f ounded +com merce +Ġexplo its +ĠF inding +UR N +ĠS ne +ĠAc id +ay ette +ĠVal ues +Ġdr astic +Ġarchitect ural +Ġ" . +× ķ +ump ed +Ġwra pping +Ġwid ow +ĠSl ayer +l ace +on ce +German y +av oid +Ġtem ples +P AR +à ´ +ĠLuc ifer +ĠFl ickr +l ov +for ces +Ġsc outing +Ġlou der +tes y +Ġbefore hand +Ä ĵ +ĠNe on +ĠW ol +ĠTyp ically +ĠPolit ico +-+ -+ +Ġbuild er +Ġder ive +K ill +Ġp oker +Ġambig uous +Ġlif ts +Ġcy t +Ġrib s +ood le +ĠS ounds +h air +ĠSynd rome +t f +Ġproport ional +u id +Ġper taining +ĠKind le +ĠNeg ro +Ġreiter ated +ĠTon ight +oth s +ĠCorn ell +Ġo wing +Ġ20 8 +elf are +oc ating +ĠB irds +Sub scribe +Ġess ays +Ġburd ens +Ġillust rations +ar ious +ER AL +ĠCal cul +Ġx en +ĠLink edIn +ĠJ ung +Ġredes ign +Con nor +29 6 +Ġrevers al +ĠAd elaide +ĠL L +Ġs inking +Ġg um +US H +c apt +ĠGr imm +Ġfoot steps +ĠCB D +isp ers +Ġpro se +Wed nesday +ĠM ovies +ed in +Ġoverturn ed +Ġcontent ious +US B +~~~~~~~~ ~~~~~~~~ +ĠCo pper +Ġpoint less +N V +val ues +olph in +d ain +Ġdepos ited +ĠG W +Ġpreced ed +ĠCl a +ĠGo lem +ĠN im +ĠÎ ² +ĠEngine ers +m iddle +Ġfl att +oper ative +Ġcouncil s +imb abwe +el in +Ġstress ful +ĠL D +Ġres h +l ake +Ġwheel chair +ĠAltern ative +Ġoptim ize +oper ation +Ġpe ek +Ġones elf +ig il +Ġtrans itions +op athy +bl ank +Ġ16 9 +17 1 +________________________________ ________________________________ +Ġl aundering +En c +ĠD EC +Ġwork outs +Ġsp ikes +Ġdin osaurs +Ġdiscrim inatory +P ool +R ather +38 5 +R NA +tes ters +et o +ĠIdent ity +Ġve in +ĠBur ton +Ġarc ade +4 20 +Ult imately +ĠSad ly +à ° +p ill +Ġcub ic +ĠSpect rum +the se +st ates +Ġun official +h awks +ĠEVER Y +Ġrain bow +Ġincarcer ation +and ing +Ġsy ll +ĠEver ton +Ġ17 9 +ĠSer bia +Ġ18 9 +m eter +ĠMic key +Ġant iqu +Ġfact ual +ne ck +ĠN are +n orm +m ust +Ġhigh ways +Ġgl am +Ġdivid ing +ĠSquad ron +ĠMar tha +Ġbirth s +C over +//////// //////// +ĠW ong +Ph ot +ĠA LS +ri o +ĠNon etheless +ĠL emon +Ġ20 6 +ĠE E +Ġderiv ative +ĠWW II +v ote +Ġthere in +Ġsepar ating +44 6 +sy nc +ĠStre ets +Ġr att +Ġmunicip ality +ĠShort ly +Ġmon k +) ," +Ġscr ub +Ġoper atives +Ne ither +Pl ace +ĠLim it +F emale +ĠAct or +Char acter +Ġconstit uted +35 7 +Ġprotest ed +ĠSt raw +ĠHe ight +ild a +ĠTy ph +Ġflood s +Ġcos metic +W AY +pert ure +up on +t ons +ess ing +ĠP ocket +Ġro oft +ĠC aucas +Ġant idepress +Ġincomp atible +EC D +Ġoper a +ĠCont est +Ġgener ators +l ime +Def ense +19 87 +for um +Ġsav age +ĠHung arian +n z +Ġmet allic +Ġex pelled +Ġres idency +Ġdress es +66 6 +ĠC lement +f ires +C ategory +Ġge ek +al is +Ġc emetery +educ ated +Ġc rawl +ĠUn able +ĠT yson +ak is +Ġp ardon +ĠW ra +Ġstrengthen ed +ĠF ors +33 5 +ĠH C +ĠM ond +Ġvisual s +ĠBeat les +ett lement +Ġ ï +g ro +Ġb ash +Ġpo orest +Ġex cel +Ġaspir ations +ĠM unicip +ens ible +Ġceremon ies +Ġintimid ation +ĠCON TR +be ck +ĠK ap +as u +Ġtradem arks +ĠS ew +ĠComp etition +net work +ĠAr ri +ĠT et +Ro aming +W C +D at +Ġso b +Ġpair ing +Ġoverd ose +SA Y +ab er +Ġrev olt +ĠF ah +act ing +e q +est ation +F ight +ĠMar ks +27 3 +Ġ17 8 +R aw +ãģ ĭ +34 9 +bl ocks +Ġver ge +est ine +ĠPod esta +Ġinv asive +Ġprofound ly +ĠA o +e ach +Ġl est +inter pret +Ġshr inking +Ġerr one +Ġche es +ly s +ĠI vy +ĠDirect ory +Ġhint ed +V ICE +Ġcontact ing +ĠG ent +he i +Ġlabel ing +Ġmerc ury +ĠL ite +Ġexp ires +Ġdest abil +rit is +c u +Ġfeather s +Ġste er +Ġprogram med +ĠV ader +Go ing +ĠE lim +Ġy o +ĠMic he +Ġ20 3 +Ġslee ves +Ġb ully +ĠHum ans +36 8 +Ġcomp ress +ĠBan ner +AR S +Ġa while +Ġcal ib +Ġspons orship +ĠDiff iculty +ĠP apers +Ġident ifier +} . +Ġy og +ĠSh ia +Ġclean up +Ġvib e +int rodu +im ming +Austral ia +Ġout lines +ĠY outube +tr ain +ĠM akes +Ġde ported +Ġcent r +ĠD ug +ĠB oulder +ĠBuff y +Ġinj unction +ĠHar ley +ĠG roups +ĠD umbledore +ĠCl ara +Ġ" - +Ġsacrific ed +ep h +Sh adow +ib ling +Ġfreel ance +Ġevident ly +ph al +Ġret ains +M ir +Ġfin ite +d ar +ĠC ous +Ġrep aired +Ġperiod ic +Ġchampions hips +Ġaster oid +bl ind +Ġexpress ly +ĠAst ros +Ġsc aled +Ġge ographical +ĠRap ids +En joy +Ġel astic +ĠMoh amed +Mark et +be gin +Ġdisco vers +Ġtele communications +Ġscan ner +Ġen large +Ġsh arks +Ġpsy chedel +ĠRou ge +Ġsnap shot +is ine +X P +Ġpestic ides +ĠL SD +ĠDist ribution +re ally +Ġde gradation +Ġdisgu ise +Ġbi om +ĠEX T +Ġequ ations +Ġhaz ards +ĠComp ared +) * +Ġvirt ues +Ġeld ers +Ġenh ancing +ĠAc ross +er os +ang ling +Ġcomb ust +ucc i +Ġconc ussion +Ġcontrace ption +ĠK ang +Ġexpress es +Ġa ux +ĠP ione +Ġexhib its +Deb ug +OT AL +ĠAl ready +ĠWheel er +Ġexp ands +? : +Ġreconc iliation +Ġpir ates +Ġpur se +Ġdiscour age +Ġspect acle +R ank +Ġwra ps +ĠTh ought +Ġimp ending +O pp +ĠAng lo +ĠE UR +Ġscrew ed +ret ched +Ġencour agement +mod els +Ġconf use +mm m +ĠVit amin +âĸij âĸij +C ru +Ġkn ights +Ġdisc ard +Ġb ishops +ĠW ear +ĠGar rett +k an +ãĥ Ł +Ġmascul ine +cap ital +ĠA us +Ġfat ally +th anks +ĠA U +ĠG ut +12 00 +Ġ 00000000 +Ġsur rog +ĠBI OS +ra its +ĠWat ts +Ġresur rection +ĠElect oral +ĠT ips +4 000 +Ġnut rient +Ġdepict ing +Ġspr ink +Ġm uff +ĠL IM +ĠS ample +ps c +ib i +gener ated +Ġspec imens +Ġdiss atisf +Ġtail ored +Ġhold ings +ĠMonth ly +ĠE at +po ons +Ġne c +ĠC age +ĠLot us +ĠLan tern +Ġfront ier +Ġp ensions +Ġj oked +ĠHard y +=-=- =-=- +r ade +U ID +Ġr ails +Ġem it +Ġsl ate +Ġsm ug +Ġsp it +ĠCall s +ĠJac obs +f eat +ĠU E +Ġrest ruct +Ġregener ation +Ġenerg ies +ĠCon nor +OH N +ĠChe ese +Ġg er +Ġresur rect +man agement +N W +Ġpres ently +ĠBru ins +M ember +ĠM ang +id an +Ġboost ing +w yn ++ . +requ isite +ĠNY PD +ĠMe gan +ĠCond itions +Ġp ics +nes ium +ĠR ash +Ġ17 4 +ĠD ucks +Ġemb ro +z u +on ian +rel igious +Ġc raz +ĠAC A +ĠZ ucker +EM A +ĠPro s +We apon +ĠKn ox +ĠAr duino +Ġst ove +Ġheaven s +ĠP urchase +Ġher d +Ġfundra iser +Dig ital +5 000 +Ġprop onents +/ âĢĭ +Ġj elly +ĠVis a +Ġmon ks +Ġadvance ment +ĠW er +Ġ18 7 +e us +ert ility +Ġfet al +Ġ19 36 +L o +Ġout fits +Ġstair case +b omb +Ġcustom ized +cl air +T ree +Ġm apped +ĠConsider ing +ĠTor res +Ġmeth yl +Ġapprox imate +Ġdo om +ĠHans en +Ġc rossover +Ġstand alone +ä ¼ +Ġinv ites +Ġgra veyard +Ġh p +Donald Trump +Ġesc ort +G ar +Ġpredec essors +Ġh ay +Ġen zyme +ĠStra ight +vis ors +I ng +ane ously +ĠApp lied +Ġf ec +ĠDur ant +Ġout spoken +or b +Ġz eal +Ġdisgr ace +' ). +ĠChe ng +28 9 +ĠRen a +ĠSu icide +29 4 +Ġout raged +ĠNew man +ĠN vidia +ĠA ber +ĠB ers +Ġrecre ation +Wind ow +ĠD P +x e +Ġped oph +Ġfall out +ambo o +Ġpresent ations +ĠApp s +Ġh tml +3 45 +ĠX XX +Ġrub bing +ĠLe ather +Ġhum idity +se ys +est ablished +ĠUn its +64 6 +Ġrespect able +A uto +Ġthri ving +ĠInn ovation +ang s +Ext ra +reg ulation +29 8 +p ick +Ex amples +ĠC J +Att ack +Ġdr acon +L T +Ġstick er +re rs +Ġsun ny +I ss +reg ulated +d im +ĠAb stract +Ġhus bands +Off ice +om ination +it ars +AN GE +asc al +ĠK ris +ĠInf antry +Ġm alf +ĠA the +ĠR ally +bal anced +................ ........ +OU P +Ġmole cule +met ics +ĠSpl it +ĠInstruct ions +ĠN ights +c ards +Ġt ug +Ġcon e +å Ń +Ġt x +ĠDisc ussion +Ġcatast rophe +pp e +g io +Ġcommun ism +Ġhal ted +ĠGu ant +cle an +ĠSc hed +ĠK anye +Ġw ander +ĠSer iously +Ġ18 8 +enn ial +f ollow +product ive +ĠFl ow +ĠS ail +Ġc raw +Ġsim ulations +or u +ang les +ĠN olan +Ġmen stru +4 70 +Ġ20 7 +aj a +Ġcas ually +board ing +Ġ2 22 +ov y +ĠN umbers +um at +O E +28 7 +ĠCle mson +Ġcert s +Ġsl id +ĠT ribe +Ġto ast +Ġfort unes +Ġf als +ĠComm ittees +Ġg p +Ġf iery +ĠN ets +ĠAn ime +Pack age +ĠComp are +l aughter +in fect +Ġatroc ities +Ġjust ices +Ġins ults +ĠVern on +Ġsh aken +Ġperson a +est amp +36 7 +br ain +Ġexperiment ing +K en +ĠElect ronics +Ġ16 1 +dom ain +Ġgraph ical +b ishop +Ġwho pping +ĠEv angel +Ġadvertis ers +ĠSpe ar +Ġb ids +Ġdestro ys +ut z +Ġunders c +ĠAD D +Ġan ts +ĠC um +ipp les +ĠF ill +Ġgl anced +Ġind icted +ĠE ff +Ġmis con +ĠDes ktop +Ġab ide +ãĥ Ģ +ĠI o +ĠC oul +Ġcaps ule +ĠCh rys +M ON +Ġund es +ĠI RA +Ġc itation +Ġdict ate +ĠNet works +ĠConf lict +ĠSt uff +x a +is ec +ĠChem istry +Ġquarter ly +William s +an an +O pt +ĠAlexand ria +out heastern +ĠSpring field +ĠBlack s +Ġge ography +24 2 +Ġut most +ĠEx xon +ab outs +E VA +ĠEn able +ĠBar r +Ġdisag reed +ĠCy prus +Ġdement ia +Ġlab s +Ġubiqu itous +ĠLO VE +Ġconsolid ated +s r +Ġcream y +ĠTim ber +Reg ardless +ĠCert ificate +Ġ" ... +ogen ous +Capt ain +Ġinsult ing +ĠSor os +ĠInst r +ĠBulgar ia +bet ter +Ġsuck ing +ĠDavid son +at z +Ġcoll ateral +g if +Ġplag ued +ĠC ancel +ĠGard ner +R B +Ġsix teen +Rem ove +ur istic +c ook +R od +Ġcompr ising +f le +) âĢĶ +ĠVik ing +g rowth +agon al +Ġsr f +af ety +m ot +N early +st own +ĠF actor +Ġautom obile +Ġproced ural +m ask +amp ires +Ġdisapp ears +j ab +3 15 +Ġ19 51 +ne eded +Ġd aring +le ader +Ġp odium +Ġun healthy +Ġm und +Ġpy ramid +oc re +Ġkiss ed +Ġdream ed +ĠFant astic +ĠG ly +å Ĭ +Ġgreat ness +Ġsp ices +Ġmet ropolitan +Ġcomp uls +i ets +101 6 +ĠSh am +ĠP yr +fl ies +ĠMid night +Ġswall owed +Ġgen res +ĠL ucky +ĠRew ards +Ġdisp atch +ĠI PA +ĠApp ly +Ġa ven +al ities +3 12 +th ings +Ġ( ). +Ġm ates +ĠS z +ĠC OP +ol ate +O FF +Ġre charge +c aps +ĠYork er +ic one +Ġgal axies +ile aks +D ave +ĠP uzz +ĠCelt ic +ĠA FC +27 6 +ĠS ons +Ġaffirm ative +H or +Ġtutorial s +ĠC ITY +ĠR osa +ĠExt ension +Ser ies +Ġf ats +Ġr ab +l is +Ġun ic +Ġe ve +ĠSp in +Ġadul thood +ty p +Ġsect arian +Ġcheck out +ĠCy cl +S ingle +Ġmart yr +Ġch illing +88 8 +ou fl +Ġ] ; +Ġcongest ion +m k +ĠWhere as +Ġ19 38 +ur rencies +er ion +Ġbo ast +ĠPat ients +Ġch ap +ĠB D +real DonaldTrump +Ġexam ines +h ov +Ġstart ling +ĠBab ylon +w id +om ew +br ance +ĠOd yssey +w ig +Ġtor ch +ĠV ox +ĠMo z +ĠT roll +ĠAn s +Similar ly +ĠF ul +00 6 +Un less +ĠAl one +st ead +ĠPub lisher +r ights +t u +ĠDoes n +Ġprofession ally +Ġcl o +ic z +Ġste als +Ġ á +19 86 +Ġst urdy +ĠJoh ann +Ġmed als +Ġfil ings +ĠFr aser +d one +Ġmult inational +Ġf eder +Ġworth less +Ġp est +Yes terday +ank ind +Ġg ays +Ġb orne +ĠP OS +Pict ure +Ġpercent ages +25 1 +r ame +Ġpot ions +AM D +ĠLeban ese +Ġr ang +ĠL SU +ong s +Ġpen insula +ĠCl ause +AL K +oh a +ĠMac Book +Ġunanim ous +Ġl enders +Ġhang s +Ġfranch ises +ore rs +ĠUp dates +Ġisol ate +and ro +S oon +Ġdisrupt ive +ĠSur ve +Ġst itches +ĠSc orp +ĠDomin ion +Ġsupp lying +Ar g +Ġtur ret +ĠL uk +Ġbr ackets +* ) +ĠRevolution ary +ĠHon est +Ġnot icing +ĠSh annon +Ġafford ed +Ġth a +ĠJan et +! -- +ĠNare ndra +ĠPl ot +H ol +se ver +e enth +Ġobst ruction +Ġ10 24 +st aff +j as +or get +sc enes +l aughs +ĠF argo +cr ime +Ġorche str +Ġde let +ili ary +rie ved +Ġmilit ar +ĠGreen e +âĹ ı +ãģ ¦ +ĠGu ards +Ġunle ashed +ĠWe ber +Ġadjust able +Ġcal iber +Ġmotiv ations +Ġà ł +m Ah +ĠL anka +hand le +Ġp ent +ĠR av +ĠAng ular +ĠK au +umb ing +Ġphil anthrop +Ġde hyd +Ġtox icity +e er +ĠY ORK +w itz +å ¼ +ĠI E +commun ity +ĠA H +Ġret ali +Ġmass ively +ĠDani els +ĠD EL +Ġcar cin +Ur l +Ġrout ing +ĠNPC s +ĠR AF +ry ce +Ġwa ived +ĠGu atem +Every body +Ġco venant +Ġ17 3 +Ġrelax ing +Ġqu art +al most +Ġguard ed +ĠSold iers +ĠPL AY +Ġout going +L AND +Ġre write +ĠM OV +ĠIm per +ĠS olution +Ġphenomen al +Ġl ongevity +Ġimp at +ĠN issan +ir ie +Ġod or +ĠZ ar +ok s +Ġmilit ias +ĠSP EC +Ġtoler ated +ars er +ĠBrad ford ++ , +Ġsur real +s f +Can adian +Ġresemb lance +Ġcarbohyd rate +VI EW +Ġaccess ory +me al +larg est +ieg el +Some one +Ġtoug hest +os o +Ġfun nel +Ġcondemn ation +lu ent +Ġw ired +ĠSun set +Jes us +ĠP ST +ĠP ages +ĠTy coon +ĠP F +Ġselect ions +Ġ ठ+part isan +Ġhigh s +ĠR une +Ġcraft s +le ad +ĠParent s +Ġre claim +ek er +ĠAll ied +ae per +Ġlo oming +Ġbenefic iaries +ĠH ull +Stud ents +Jew ish +d j +Ġp act +tem plate +ĠOffic ials +ĠBay lor +Ġhe mp +Ġyouth s +ĠLevel s +ĠX iao +ĠC hes +Ġende avor +ĠRem oved +Ġhipp ocamp +H ell +ãĤ Ĭ +80 5 +Ġd inosaur +ĠWr ath +ĠIndones ian +Ġcalcul ator +ĠD ictionary +Ġ4 20 +ĠM AG +( _ +! , +t arians +Ġrestrict ing +rac use +Ġweek day +OU NT +Ġsh rugged +leg round +Ġb ald +ĠDo ctors +Ġt outed +ĠMax well +Ġ2 14 +Ġdiplom at +Ġrep ression +Ġconstitu ency +v ice +r anked +ĠNap oleon +g ang +ĠFore ver +t un +Ġbul b +ĠPD T +ĠC isco +V EN +Ġres umed +Ste ven +ĠManit oba +Ġfab ulous +ĠAg ents +19 84 +Ġam using +ĠMyster ies +Ġor thodox +fl oor +Ġquestion naire +Ġpenet rate +Ġfilm makers +ĠUn c +Ġst amped +Ġth irteen +Ġout field +Ġforward ed +Ġapp ra +Ġa ided +t ry +Ġunf ocused +ĠL iz +ĠWend y +ĠSc ene +Ch arg +Ġreject s +Ġleft ist +ĠProv idence +ĠBr id +reg n +Ġprophe cy +ĠL IVE +4 99 +Ġfor ge +ĠF ML +Ġintrins ic +ĠF rog +Ġw ont +ĠH olt +Ġfam ed +CL US +aeper nick +ĠH ate +ĠC ay +Ġregister ing +ort ality +rop y +ocaly ptic +a an +n av +Ġfasc ist +IF IED +Ġimpl icated +ĠRes ort +ĠChand ler +ĠBr ick +P in +ys c +Us age +ĠHel m +us ra +âĺħ âĺħ +ĠAb bas +Ġunanim ously +Ġke eper +Ġadd icted +?? ? +Ġhelm ets +Ġant ioxid +aps ed +80 8 +gi ene +Ġwa its +Ġmin ion +ra ved +ĠP orsche +Ġdream ing +Ġ17 1 +ĠC ain +Ġun for +ass o +ĠConfig uration +k un +hard t +Ġn ested +ĠL DS +L ES +Ġt ying +en os +Ġc ue +ĠMar qu +sk irts +Ġclick ed +Ġexp iration +ĠAccording ly +ĠW C +Ġbless ings +Ġaddict ive +ĠN arr +y x +ĠJagu ars +Ġrent s +ĠS iber +Ġt ipped +ous se +ĠFitz gerald +Ġhier arch +out ine +Ġwa velength +> . +ch id +ĠProcess ing +/ + +r anking +E asy +ĠConst ruct +Ġt et +ins ured +H UD +Ġqu oting +Ġcommun icated +in x +Ġin mate +Ġerect ed +ĠAbs olutely +ĠSure ly +Ġun im +ĠThr one +he id +Ġcl aws +Ġsuper star +ĠL enn +ĠWh is +U k +ab ol +Ġsk et +ĠN iet +Ġper ks +Ġaff inity +Ġopen ings +phas is +Ġdiscrim inate +T ip +v c +Ġgr inding +ĠJenn y +Ġast hma +hol es +ĠHom er +Ġreg isters +ĠGl ad +Ġcre ations +Ġlith ium +Ġappl ause +unt il +Just ice +ĠTur ks +Ġsc andals +Ġb ake +t ank +M ech +ĠMe ans +ĠM aid +Republic ans +is al +wind ows +ĠSant os +Ġveget ation +33 8 +t ri +Ġfl ux +ins ert +Ġclar ified +Ġmort g +ĠCh im +ĠT ort +Ġdiscl aim +met al +ĠAs ide +Ġindu ction +Ġinf l +Ġathe ists +amp h +Ġe ther +ĠV ital +ĠBu ilt +M ind +Ġweapon ry +S ET +Ġ18 6 +ad min +g am +cont ract +af a +Ġderiv atives +Ġsn acks +Ġch urn +E conom +Ġca pped +ĠUnder standing +ĠH ers +ĠI z +Ġd uct +I ENT +augh ty +Ġâľ Ķ +ĠN P +Ġsa iling +In itialized +Ġt ed +Ġreact ors +ĠL omb +Ġcho ke +ĠW orm +Ġadm iration +Ġsw ung +ens ibly +Ġr ash +ĠGo als +ĠImport ant +Sh ot +ĠR as +Ġtrain ers +ĠB un +Work ing +Ġhar med +ĠPand ora +ĠL TE +Ġmush room +ĠCH AR +ĠF ee +ĠM oy +B orn +ol iberal +ĠMart ial +Ġgentle men +Ġling ering +Offic ial +Ġgra ffiti +ĠN ames +D er +Ġqu int +ist rate +aze era +ĠNOT ICE +ĠFlore nce +Ġpay able +Ġdep icts +ĠSpe cies +He art +âĶĢâĶĢâĶĢâĶĢ âĶĢâĶĢâĶĢâĶĢ +Ġencl osed +Incre ases +D aily +ĠL is +Ġenact ment +ĠB acon +ĠSt eele +dem and +Ġ18 3 +Ġmouth s +Ġstr anded +Ġenhance ment +01 1 +ĠWh ats +Ġhe aled +en y +ĠR ab +Ġ3 40 +ĠLab yrinth +ro ach +ĠY osh +ĠCl ippers +Ġconcert s +Intern et +35 5 +Ġstick ers +Ġter med +ĠAx e +Ġgrand parents +Fr ance +ĠCl im +ĠU h +ul ic +Ġthr ill +cent ric +ĠOver view +ĠCond uct +Ġsubstant ive +Ġ18 2 +m ur +Ġstr ay +ĠCo ff +Ġrep etitive +ĠFor gotten +Ġqual ification +ew itness +ĠZ imbabwe +Ġsim ulated +ĠJ D +25 3 +ĠW are +Ġun sc +T imes +Ġsum mons +Ġdis connected +Ġ18 4 +ci us +ĠGu jar +od ka +Ġer ase +ĠTob acco +elect ed +Ġun cont +ĠShe pard +ĠL amp +Ġalert ed +Ġoper ative +arn a +u int +Ġneglig ence +ac ements +Ġsup ra +Ġprev ail +ĠSh ark +Ġbel ts +ãģ « +Ġt ighter +Engine ers +Ġin active +Ġexp onent +ĠWill ie +a ples +Ġhe ir +ĠH its +ian n +ĠS ays +Ġcurrent s +ĠBeng al +Ġar ist +B uffer +Ġbree ze +ĠWes ley +Col a +Ġpron oun +Ġde ed +ĠK ling +Ġof t +Ġinf lict +Ġpun ishing +Ġn m +ik u +OD UCT +01 4 +Ġsubsid y +ĠDE A +ĠHer bert +ĠJ al +B ank +Ġdef erred +Ġship ment +B ott +Ġal le +b earing +HT ML +Off line +Ġ2 13 +Ġscroll ing +Ġsc anned +ĠLib yan +ĠT OP +ch rom +d t +col umn +Psy NetMessage +Z ero +Ġtor so +0 50 +âķ IJ +Ġimp erson +ĠSchw artz +ud ic +Ġpiss ed +ĠS app +25 7 +ĠIS Ps +og l +Ġsuper vised +Ġad olescent +Ġatt ained +ĠDel ivery +ĠB unny +Ġ19 37 +Ġmini ature +Ġo s +Ġ3 70 +60 8 +ĠMour inho +Ġinn ate +Ġtem po +ĠN M +ĠFall en +00 9 +Ġprov ocative +Stream er +ĠBened ict +ĠBol she +Ġt urtle +ĠPC B +ĠEqu al +Direct or +ĠR end +Ġflu ids +Author ities +Ġcous ins +requ ency +ĠNeigh bor +s ets +sh ared +Char les +pass word +Ġg ears +Ġ2 11 +ĠHard ware +ri ka +Ġup stream +H om +Ġdisproportion ately +iv ities +Ġund efined +Ġelect rons +Ġcommem or +Event ually +Ġ> < +Ġir responsible +2 18 +ĠRe leased +ĠO VER +ĠI GN +ĠB read +st ellar +ĠS age +tt ed +dam age +ed ition +ĠPre c +Ġl ime +Ġconf inement +Ġcal orie +we apon +Ġdiff ering +ĠS ina +m ys +am d +Ġintric ate +k k +ĠP AT +ã o +st ones +lin ks +Ġr anch +Sem itic +Ġdifferent iate +ĠS inger +occup ied +Ġfort ress +c md +Ġinter ception +ĠAnk ara +Ġre pt +ĠSol itaire +Ġrem ake +p red +Ġd ared +aut ions +ĠB ACK +Run ning +Ġdebug ging +Ġgraph s +3 99 +ĠNig el +Ġb un +Ġpill ow +Ġprog ressed +fashion ed +Ġob edience +ER N +Ġrehe ars +C ell +t l +S her +Ġher ald +ĠPay ment +ĠC ory +ĠDe pt +Ġrep ent +ĠWe ak +uck land +Ġple asing +Ġshort ages +Ġjur ors +ĠK ab +q qa +Ant i +Ġw ow +ĠRC MP +Ġt sun +ĠS ic +Ġcomp rises +Ġsp ies +Ġprec inct +n u +Ġur ges +Ġtim ed +Ġstrip es +ĠB oots +Ġy en +Adv anced +Ġdisc rete +ĠArch angel +employ ment +D iff +Ġmon uments +Ġ20 9 +work er +Ġ19 6 +ĠI g +utter stock +T PS +J ac +Ġhomeless ness +Ġcomment ator +Ġrac ially +f ing +se ed +E le +ell ation +Ġeth anol +Ġpar ish +ĠD ong +ĠAw akening +Ġdev iation +ĠB earing +ĠTsu k +Ġrec ess +Ġl ymph +ĠCann abis +å ľ +ĠNEW S +Ġd ra +ĠStef an +ĠWr ong +ĠS AM +Ġloose ly +Ġinterpre ter +ĠPl ain +Go vernment +Ġbigot ry +Ġgren ades +ave z +pict ured +Ġmand ated +ĠMon k +ĠPed ro +Ġl ava +27 4 +Ġcyn ical +ĠScroll s +l ocks +M p +Ġcon gregation +orn ings +ph il +ĠI bid +Ġf erv +Ġdisapp earing +Ġarrog ant +sy n +ĠMa ver +ĠSu it +24 1 +Ġab bre +ack ers +P a +ĠY el +Whe never +Ġ23 5 +ĠV ine +ĠAn at +Ġext inct +LE T +Ġexecut able +V ERS +ox ide +D NA +ĠP rel +Ġresent ment +Ġcompr ise +ĠAv iv +Ġinter ceptions +Ġprol ific +IN A +ĠEr in +though t +2 19 +ĠPsychiat ry +un ky +chem ist +H o +ĠMcC oy +Ġbr icks +L os +ri ly +ĠUS SR +Ġr ud +Ġl aud +ĠW ise +ĠEmer ald +Ġrev ived +Ġdam ned +ĠRep air +id em +ct ica +Ġpatri arch +ĠN urs +me g +Ġcheap est +re ements +empt y +ĠCele br +Ġdepri vation +ch anted +ĠTh umbnails +E nergy +ĠEth an +ĠQ ing +Ġopp oses +W IND +v ik +ĠM au +ĠS UB +66 7 +G RE +ĠVol unte +nt on +C ook +å IJ +es que +Ġplum met +Ġsu ing +Ġpron ounce +Ġresist ing +ĠF ishing +ĠTri als +Ġy ell +Ġ3 10 +Ġin duct +Ġpersonal ized +oft en +R eb +EM BER +Ġview point +Ġexist ential +() ) +rem ove +MENT S +l asses +Ġev apor +Ġa isle +met a +Ġreflect ive +Ġentit lement +Ġdev ised +mus ic +asc ade +Ġwind ing +off set +Ġaccess ibility +ke red +Bet ter +ĠJohn ston +th inking +S now +ĠCroat ia +ĠAt omic +27 1 +34 8 +Ġtext book +ĠSix th +Ġ اÙĦ +Ġsl ider +ĠBur ger +b ol +S ync +Ġgrand children +Ġc erv ++ ) +Ġe ternity +Ġtweet ing +Ġspec ulative +Ġpiv otal +ĠW P +ĠT ER +ynam ic +Ġu pl +ĠC ats +per haps +Ġclass mates +Ġblat ant +' - +Ġl akh +ant ine +ĠB org +i om +/ ( +ĠAthlet ic +Ġs ar +OT A +ĠHoff man +Never theless +Ġad orable +Ġspawn ed +Ass ociated +ĠDom estic +Ġimpl ant +ĠLux em +ĠK ens +Ġp umps +ĠS AT +Att ributes +50 9 +av our +Ġcentral ized +ĠT N +Ġfresh ly +ĠA chieve +Ġouts iders +her ty +ĠRe e +ĠT owers +ĠD art +ak able +Ġm p +ĠHeaven ly +Ġr ipe +ĠCarol ine +ry an +Ġclass ics +Ġret iring +Ġ2 28 +Ġa h +Ġdeal ings +Ġpunch ing +ĠChap man +O ptions +max well +vol ume +Ġst al +Ġex ported +ĠQu ite +Ġnumer ical +B urn +F act +ĠKey stone +Ġtrend ing +Ġalter ing +ĠAfric ans +47 8 +ĠM N +ĠKn ock +Ġtempt ation +Ġprest ige +Over view +ĠTrad itional +ĠBah rain +Priv ate +ĠH OU +Ġbar r +ĠT at +C ube +US D +ĠGrand e +ĠG at +ĠFl o +Ġres ides +Ġind ec +vol ent +Ġperpet ual +ub es +Ġworld view +ĠQuant um +Ġfil tered +Ġen su +orget own +ERS ON +ĠM ild +37 9 +OT T +à ¥ +Ġvit amins +Ġrib bon +Ġsincere ly +ĠH in +Ġeight een +Ġcontradict ory +Ġgl aring +Ġexpect ancy +Ġcons pir +Ġmon strous +Ġ3 80 +re ci +Ġhand ic +Ġpump ed +Ġindic ative +Ġr app +Ġav ail +ĠLEG O +ĠMar ijuana +19 85 +ert on +Ġtwent ieth +################ ################ +ĠSw amp +Ġval uation +Ġaffili ates +adjust ed +ĠFac ility +26 2 +Ġenz ymes +itud inal +Ġimp rint +S ite +Ġinstall er +ĠT RA +m ology +lin ear +ĠCollect ive +ig ating +ĠT oken +Ġspec ulated +K N +ĠC ly +or ity +Ġdef er +Ġinspect ors +appro ved +R M +ĠSun s +Ġinform ing +ĠSy racuse +ib li +7 65 +Ġgl ove +Ġauthor ize +â̦â̦â̦â̦ â̦â̦â̦â̦ +ĠCru ise +Ġcontract ing +she ll +IF E +ĠJew el +p ract +ĠPhot oshop +ĠKnow ing +h arm +Ġattract ions +ad an +et us +01 8 +w agen +Al t +Ġmultip ly +Ġequ ilibrium +: { +ĠF ighters +ĠEd gar +Ġfour teen +Go vern +Ġmis use +Ġab using +Ġancest ry +ram er +64 4 +Ġwor ms +Ġthick er +ĠComb ine +Ġpeas ants +Ġv ind +Ġcon quest +Ġm ocked +Ġc innamon +ĠC ald +ĠGall up +Ġavoid ance +Ġincarn ation +ĠStr at +Ġt asted +ent a +ĠN eal +p ared +Ġtermin ology +ject ion +Scient ists +ĠIN S +ĠDe e +Ġdirect ories +R oad +ĠSh ap +br ight +ĠDirect ors +ĠCol umn +Ġb ob +Ġprefer ably +Ġgl itch +f urt +Ġe g +id is +C BC +Ġsur rendered +Ġtest ament +33 6 +ug gest +ĠN il +an other +Ġpat hetic +ĠDon na +Ġ2 18 +ĠA very +Ġwhis key +Ġf ixture +ĠCon quest +Ġbet s +O cc +ĠLe icester +] ." +Ġ) ); +Ġfl ashes +45 6 +Ġmask ed +ge bra +Ġcomput ed +che l +aud er +Ġdefe ats +ĠLiber ation +ĠOs ama +ĠV ive +Ch anges +Ch annel +Ġtar iffs +Ġm age +ĠS ax +Ġinadvert ently +ĠC RE +ĠRe aper +ink y +gr ading +Ġstere otyp +Ġcur l +ĠF ANT +Ġfram eworks +M om +ĠAn ch +Ġflav our +car bon +Ġperm itting +let cher +ĠMo zilla +ĠPark ing +ĠCh amp +Sc roll +Ġmurd erer +Ġrest ed +Ġow es +ĠP oss +AD D +IF F +res olution +ĠMin ing +Ġcompar ative +D im +Ġneighbour ing +ĠA ST +ĠT oxic +Ġbi ases +Ġgun fire +ur ous +ĠMom ent +19 83 +Ġper vasive +tt p +ĠNorm ally +r ir +S arah +ĠAlb any +Ġun sett +ĠS MS +ip ers +l ayer +ĠWh ites +up le +Ġtur bo +ĠLe eds +Ġthat s +ĠMin er +M ER +ĠRe ign +Ġper me +ĠBl itz +Ġ19 34 +Ġintimid ating +t ube +Ġecc entric +ab olic +box es +ĠAssoci ates +v otes +Ġsim ulate +um bo +aster y +Ġship ments +FF FF +an th +Ġseason ed +Ġexperiment ation +âĸ ł +law s +Me et +idd les +ant ics +R ating +IS IS +h ift +Ġfront s +b uf +01 7 +Ġun att +ĠD il +le ases +ĠGard ens +77 7 +t ouch +ve ll +45 8 +Ġ= ==== +s aving +Ġer osion +ĠQu in +Ġearn s +Ġaccomplish ment +ĠWe i +Ġ< [ +____ _ +Ġir rig +ĠT eddy +Ġconqu ered +ĠArm ored +Ġassert s +Ġmanip ulating +r é +Ġtranscript s +G allery +Ġplot ting +Ne il +Ġbetray al +load er +ĠS ul +Ġdispl acement +Ġroy alty +ĠW I +he it +ĠDev ices +alle l +Ġmunicipal ities +Ġcan al +St ars +ĠU AE +Ġ" â̦ +ĠC U +ab ove +Ġreson ance +ĠguiActive Un +add ed +ĠBra ves +ĠI bn +Ġhere by +ĠB RE +Ġshare holder +ĠH ir +ĠJ i +Ġstrange ly +Ġadm ired +Ġpl ight +Ġb achelor +ĠP ole +cipl inary +T ony +ĠArmen ian +Ġun man +ĠZion ist +St age +isco ver +Ġautom otive +Ġs idelines +Ġsl ick +ĠRena issance +ĠF UN +Im ages +ĠH aj +Ġp ing +Ġshort cut +ĠBl vd +ĠLook s +Ġbur sts +Ġcl amp +Ġm ish +Ġsort ing +Ġpatri ot +Ġcorrect ness +ĠScand inav +ĠCaval iers +p ython +az ar +Ġ3 75 +ĠJa une +40 9 +Ġdetrim ental +Ġstab bing +Ġpoison ed +Ġf ountain +oc ent +or st +ĠMar i +Ġr ains +ĠO vers +ĠInst itution +ud get +AM Y +t ale +ĠK R +ĠPr ices +Ġhead aches +Ġlands l +ĠA ura +Bon us +ĠZ hao +ĠH ip +Ġhop s +ĠKurd istan +Ġexplo iting +ry n +Ġhypocr isy +op ening +Ġgun shot +Ġw ed +inter stitial +Inter stitial +Ġam en +Bre aking +Ġmarket ed +W ire +ĠC rowd +Contin ue +ĠK nown +ĠEffect ive +ore an +iz ons +Jose ph +Ġescal ation +us ername +Ġcur tain +AT ES +ĠP AR +ĠM iy +Ġcounter fe +l ene +Ġcont enders +d aily +ĠAs c +ĠPhill ip +most ly +Ġfil ename +he ne +Ġresemb ling +Ġst aging +ĠCh loe +Ġw iring +H on +ĠRen ew +ott age +ĠHy brid +m uch +Ġstro kes +Ġpolicy makers +AP TER +ĠArk ham +pl ot +Ġassist ants +Ġde port +ĠSe ga +Ġinflu enza +ĠC ursed +ĠK obe +Ġskin ny +Prov ider +ĠR ip +Ġincrement al +product s +B F +Ġd ome +ĠC redits +Ġlos ers +int s +ĠBet ty +ĠTal ent +ĠD AM +L v +E ss +Ġd ens +tem p +J udge +od ic +Ġ' ( +UR ES +ets k +V O +Ġretrie ved +Ġarchitect s +Ù ĩ +Ġeth ic +ĠSecond ary +st ocks +ad ia +Ġ3 25 +ĠOp inion +Ġsimultane ous +Ġd izz +ul p +Ġsmugg ling +ipp ery +R andom +f acing +ĠD as +Ġstock p +Ġdiscl osures +po inter +Ġcor al +ĠSe lection +ĠP ike +ival ent +Ġruth less +ĠR im +Ġensu ing +ĠExper iment +Ġcongress man +Ġbelie ver +Ġun specified +ĠM ord +Ġknowledge able +ĠV ERY +T X +Ġstra ps +Ġtur f +apesh ifter +Ġmar ital +Ġfl ock +ãģ Ĩ +26 3 +AM ES +ĠOpp osition +Ġtre asures +ĠG OD +Ġmodel ed +ĠWOR LD +Ġ( [ +ĠUs age +H F +Ġ$ ( +uss ed +Ġpione er +E ight +par se +b read +rit z +ĠMir anda +ĠK ant +++ ) +ore n +Ġprov oked +Ġbre eds +ĠIn cludes +ĠPast ebin +ĠFl ip +J ava +Ġbr ink +Ġrum ored +Ġun seen +Ġgar nered +ĠDef in +al ted +Ġtatt oos +Ġhes itation +is itions +ĠWe aver +ĠReport ing +Ġtherap ies +Ġconsult ants +Ġresid ual +ĠMal i +ĠRom a +i ago +ĠRes idents +ub i +Ġremed ies +Ġadapt ive +ĠAl ive +ĠBar cl +Ġwal lets +c rypt +etermin ation +ĠPel osi +Ġsl ipping +oton in +Ġall iances +pat rick +ir is +Ġor th +ĠPer kins +ĠDe V +ĠG ets +Ġdry ing +ge e +fore st +ĠFor get +ore m +33 9 +Ġvague ly +ĠD ion +ĠP orn +ĠH OW +Ġp neum +Ġrub ble +ĠT aste +enc ia +ĠG el +Ġd st +Ġ24 5 +ĠMoroc co +inf lamm +ĠTw ins +Ġb ots +d aughter +ĠB alk +Ġbre thren +Ġlog os +Ġgo bl +f ps +Ġsub division +Ġp awn +Ġsquee zed +Ġmor ale +ĠD W +' " +Ġkn ot +ook y +Ġdiv isive +Ġboost ed +ch y +ãĥ IJ +if act +Ġnewcom ers +ĠWrest ling +Ġsc outs +w olves +R at +Ġnin eteenth +ĠOs borne +St ats +Ġem powered +Ġpsych opath +ĠO EM +ugg age +ĠP K +ĠMoh ammad +P ak +Ġanarch ists +ĠExt ract +est hes +ĠStock holm +l oo +ĠG raph +Ġdeploy ing +ĠStr anger +ĠM old +Ġstaff er +Ġdiscount ed +uck le +ple ase +ĠLand ing +ÃŃ a +Ġ19 3 +Ġan te +Ġrep etition +Ġ+ /- +Ġpar ody +Ġlive ly +AA A +ĠHor us +Ġp its +ind ers +L OC +ĠVen ice +40 6 +ĠDis cover +â Ĩ +ellect ual +Ġp ens +Ġey el +ig uous +Im pl +Ġj oking +Ġinv al +ĠBel fast +Ġcredit ors +ĠSky walker +ov sky +Ġcease fire +Ġse als +is oft +) ). +ĠFel ix +IT S +Ġt resp +ĠBlock chain +ew are +ĠSch war +en ne +mount ed +ĠBe acon +les h +Ġimmense ly +Ġche ering +Em ploy +sc ene +ish ly +atche wan +ĠNic olas +Ġdr ained +ĠEx it +ĠAz erb +j un +Ġflo ated +u ania +De ep +Ġsuper v +Ġmyst ical +ĠD ollar +ĠApost le +ĠR EL +ĠProv ided +ĠB ucks +ãĥ ´ +cut ting +Ġenhance ments +ĠPengu ins +ĠIsa iah +Ġj erk +ĠW yn +Ġst alled +Ġcryptoc urrencies +ĠR oland +sing le +Ġl umin +ĠF ellow +ĠCap acity +ĠKaz akh +W N +Ġfin anced +38 9 +Ġt id +Ġcoll usion +ĠMy r +î Ģ +Sen ator +Ġped iatric +Ġneat ly +Ġsandwic hes +ĠArchitect ure +Ġt ucked +Ġbalcon y +Ġearthqu akes +qu ire +F uture +Ġhe fty +é Ĺ +Ġspecial izes +Ġstress es +Ġs ender +Ġmisunder standing +Ġep ile +Ġprov oke +ĠCol ors +Ġdis may +uk o +[ _ +58 6 +ne utral +Ġdon ating +ĠRand all +Mult i +Ġconvenient ly +ĠS ung +ĠC oca +Ġt ents +ĠAc celer +Ġpart nered +27 2 +ir ming +ĠB AS +s ometimes +Ġobject ed +ub ric +p osed +LC S +gr ass +Ġattribut able +V IS +Israel i +Ġrepe ats +ĠR M +v ag +ut a +in ous +Ġin ert +ĠMig uel +æ Ń +ĠHawai ian +B oard +Ġart ific +ĠAzerb ai +as io +ĠR ent +A IN +Ġappl iances +Ġnational ity +Ġass hole +ĠN eb +Ġnot ch +h ani +ĠBr ide +Av ailability +Ġintercept ed +Ġcontin ental +Ġsw elling +ĠPers pect +b ies +. < +ith metic +ĠL ara +Ġtempt ing +add r +Ġoversee ing +cl ad +ĠD V +ĠGing rich +Ġm un +ĠApp ropri +Ġalter ations +ĠPat reon +Ġha voc +Ġdiscipl ines +Ġnotor iously +aku ya +ier i +? ). +ĠW ent +Ġsil icon +Ġtre mb +Cont ainer +K nown +Ġmort ar +est e +ick a +Ar thur +ĠPre viously +ĠMart y +Ġsp arse +g ins +Ġin ward +ĠParticip ant +C opy +ĠM isc +Ġantib iotic +ĠRet ro +Ġel usive +Ġass ail +ĠBatt alion +ĠB ought +Ġdimin ish +ĠEuro pa +s ession +ĠDanger ous +ies el +Ġdisbel ief +Ġbl asts +ext reme +ĠBoy d +ĠProject s +ĠGu ys +Ġunder gone +Ġgr ill +ĠDw ight +Ġ19 7 +US ER +Ġfiles ystem +Ġcl ocks +T aylor +Ġwra pper +Ġfold ing +ous and +ĠPhilipp ine +ATION AL +ĠPer th +Ġas hes +Ġaccum ulate +ĠGate way +Sh op +orks hire +H an +ĠBar rel +ĠLe h +ĠX V +Ġwh im +Ġrep o +ĠC G +ĠM am +Ġincorpor ating +Ġbail out +Ġlingu istic +Ġdis integ +C LE +Ġcinem atic +ĠF iber +S yn +il ion +ĠCom pos +c hens +Ġne oc +Ġbo iled +F INE +on o +un cle +ik en +ĠB M +Î ¹ +Ġreceipt s +Ġdisp osed +ĠTh irty +ĠR ough +ĠA BS +Ġnot withstanding +oll en +# $ +Ġunrel iable +Ġbl oom +Ġmedi ocre +Ġtr am +ĠTas man +Ġsh akes +Ġmanifest o +ĠM W +Ġsatisf actory +Ġsh ores +Ġcomput ation +Ġassert ions +orm ons +ar ag +ab it +Dem ocrats +ĠL oot +ĠVol ks +ha ired +Ġgrav itational +S ing +ĠM iz +Ġthro ttle +Ġtyr anny +ĠView s +Ġrob ber +ĠMinor ity +Ġsh rine +sc ope +pur pose +Ġnucle us +our cing +ĠUS DA +ĠD HS +w ra +ĠBow ie +Sc ale +ĠB EL +x i +I ter +Ġ( ), +w right +Ġsail ors +ous ed +NAS A +ĠPro of +ĠMin eral +t oken +ĠF D +R ew +Ġe ll +6 30 +Ġchance llor +ĠG os +Ġamount ed +ĠRec re +ome z +ĠOpt im +ĠOl ive +Ġtrack er +ow ler +ĠUn ique +R oot +Ġmar itime +ĠQur an +ĠAd apt +Ġecosystem s +ĠRe peat +ĠS oy +ĠI MP +Ġgrad uating +and em +P ur +ĠRes et +ĠTr ick +ĠPh illy +ĠT ue +ĠMalays ian +Ġclim ax +Ġb ury +Ġcons pic +ĠSouth ampton +ĠFl owers +Ġesc orted +ĠEduc ational +ĠI RC +Ġbrut ally +e ating +Ġpill ar +ĠS ang +ĠJ ude +ar ling +ĠAm nesty +Ġrem inding +ĠAdminist rative +hes da +Ġfl ashed +ĠP BS +per ate +fe ature +Ġsw ipe +Ġgra ves +oult ry +26 1 +bre aks +ĠGu er +Ġsh rimp +ĠV oting +qu ist +Ġanaly tical +Ġtables poons +ĠS OU +Ġresear ched +Ġdisrupt ed +Ġj our +Ġrepl ica +Ġcart oons +b ians +} ) +c opy +G ot +ou ched +P UT +Ġsw arm +not ations +s aid +Ġreb uilt +Ġcollabor ate +Ġr aging +Ġn ar +Ġdem ographics +ĠD DR +Ġdist rust +oss ier +ĠK ro +Ġpump kin +Ġreg rets +Ġfatal ities +ĠL ens +ĠO le +p d +Ġpupp et +ĠOut look +ĠSt am +O l +F air +U U +Ġre written +Ä ± +Ġfasc inated +Ġve ctors +Ġtrib unal +u ay +ĠM ats +ĠCo ins +[ [ +Ġ18 1 +Ġrend ers +ĠK aepernick +Ġesp ionage +Ġsum m +Ġd itch +Acc ount +Ġspread sheet +Ġmut ant +p ast +40 7 +Ġd ye +Ġinit iation +Ġ4 000 +Ġpunish able +Ġth inner +ĠKh al +Ġinter medi +D un +ĠGoth am +Ġeager ly +Ġvag inal +p owers +V W +ĠWATCH ED +Ġpred ator +ams ung +Ġdispar ity +Ġ[ * +Ġam ph +Ġout skirts +ĠSpir its +Ġskelet al +Ð » +ĠR ear +Ġissu ance +ĠLog ic +re leased +Z Z +ĠB ound +Ent ry +Ġex its +is ol +ĠFound er +Ġw re +ĠGreen land +ĠM MO +t aker +IN C +ãģ ¾ +Ġhour ly +hen ko +Ġfantas ies +Ġdis ob +Ġdemol ition +ãĥ ĭ +Ġen listed +rat ulations +Ġmis guided +Ġens ured +Ġdiscour aged +m ort +Ġfl ank +Ġc ess +Ġreact s +ĠS ere +s ensitive +ĠSer pent +ass ad +Ġ24 7 +Ġcalm ly +b usters +Ġble ed +ĠSt ro +Ġamuse ment +ĠAntar ctica +Ġs cept +ĠG aw +a q +ason ic +Ġsp rawling +n ative +atur ated +ĠBattle field +IV ERS +E B +ĠG ems +ĠNorth western +ĠFil ms +ĠAut omatic +Ġappre hend +ãģ ¨ +Ġgui Name +Ġback end +Ġevid enced +ge ant +01 2 +ĠS iege +Ġexternal To +Ġunfocused Range +ĠguiActiveUn focused +Ġgui Icon +ĠexternalTo EVA +ĠexternalToEVA Only +F ri +ch ard +en aries +Ġchief s +Ġc f +ĠH UD +Ġcorro bor +Ġd B +ĠT aken +ĠPat ricia +ra il +ĠCh arm +ĠLiber tarian +rie ve +Person al +ĠO UR +ger ies +Ġdump ing +Ġneurolog ical +it imate +ĠClint ons +raft ed +ĠM olly +Ġtermin als +reg ister +Ġfl are +Ġenc oded +Ġautop sy +p el +m achine +Ġexempt ions +ĠRoy als +d istance +Ġdraft s +Ġl ame +ĠC unning +Ġsp ouses +ĠMark ets +ĠCar rier +Ġimp lying +ĠY ak +s id +Ġl oser +Ġvigil ant +Ġimpe achment +Ġaug mented +ĠEmploy ees +Ġunint ended +tern ally +ĠW att +Ġrecogn izable +ess im +æ Ŀ +Ġco ated +r ha +Ġlie utenant +ĠLegisl ation +pub lished +44 4 +01 3 +Ġide ally +ĠPass word +Ġsimpl ify +ĠMet a +ĠM RI +Ġple ading +organ ized +hand ler +Ġun ravel +cor rect +Ġ icy +Ġparan oid +Ġpass er +Ġinspect ions +of er +ĠHealth care +28 3 +ĠBr ut +iol a +for ge +ĠMed ieval +MS N +ie vers +ĠProgram ming +å ī +Ġ2 23 +m u +ĠC LE +ug a +Ġsho ppers +Ġinform ative +ĠPl ans +Ġsupplement ation +ĠT ests +ty ard +ocy tes +ĠVeg a +ĠGujar at +erman ent +Ex cept +ĠL OT +all a +ĠC umm +ĠO sw +Ġven om +ĠDeb t +ĠD OWN +Ġreun ion +Ġm uc +ĠRel ief +Ġge op +ĠðŁ ĺ +al ogue +An th +ech o +Ġcor ros +Ġrepl ication +ĠBl azing +ĠD aughter +Ġinf lic +ĠLind sey +Ù Ī +28 4 +Ex it +Ġgl oom +TA IN +Ġundermin ing +Ġadv ising +h idden +Ġover flow +Ġg or +urd ue +Ġe choes +enh agen +Ġimp uls +d rug +c ash +Ġas ync +Ġmir ac +at ts +p unk +Ġpiv ot +ĠLegisl ative +Ġblog gers +ĠCl aw +s burg +d yl +ĠRecomm end +Ġver te +Ġprohib iting +ĠPant her +Jon athan +Ġo min +Ġhate ful +28 1 +ĠOr che +ĠMurd och +down s +Ġas ymm +G ER +Al ways +Ġinform s +ĠW M +ĠP ony +ĠApp endix +ĠAr lington +J am +Ġmedic inal +ĠS lam +IT IES +Ġre aff +ĠR i +F G +S pring +b ool +Ġthigh s +Ġmark ings +ĠRa qqa +ĠL ak +p oll +ts ky +ĠMort y +ĠDef inition +Ġdeb unk +end ered +ĠLe one +a vers +Ġmortg ages +App arently +N ic +ha us +ĠTh ousands +au ld +Ġm ash +sh oot +Ġdi arr +Ġconscious ly +H ero +e as +ĠN aturally +ĠDestroy er +Ġdash board +serv ices +R og +Ġmillenn ials +Ġinv ade +- ( +Ġcomm issions +ĠA uckland +Ġbroadcast s +Ġfront al +Ġcr ank +ĠHist oric +Ġrum ours +CT V +Ġster il +Ġboost er +rock et +ãĤ ¼ +ut sche +ĠP I +Ġ2 33 +ĠProdu cer +ĠAnaly tics +Ġinval uable +Ġunint ention +ĠC Y +Ġscrut in +Ġg igg +Ġeng ulf +Ġprolet ariat +Ġh acks +ĠH ew +ar ak +ĠSl ime +ield ing +ag her +ĠEll iot +Ġtele com +Ġ2 19 +ult an +ĠAr bor +ĠSc outs +B an +Ġlifes pan +Ġbl asp +38 8 +Ġjud iciary +ĠContin ental +ask ing +Mc C +L ED +Ġbag gage +ĠSorce rer +Ġrem nants +ĠGriff ith +ets u +ĠSub aru +ĠPerson ality +des igned +ush ima +agn ar +Ġrec oil +Ġpass ions +\ ": +Ġte e +Ġabol ition +ĠCreat ing +j ac +Ġ19 4 +01 9 +Ġpill ars +ric hed +/ " +t k +Ġlive lihood +Ġro asted +ah on +ĠH utch +ass ert +Ġdivid end +Ġkn it +Ġd aunting +Ġdisturb ance +Ġsh ale +Ġcultiv ated +Ġrefriger ator +L B +ĠN ET +Ġcommercial s +Ġthink ers +45 5 +Ġch op +B road +Ġsuspic ions +Ġtag ged +l ifting +Ġsty lish +ĠShield s +Short ly +Ġt ails +A uth +ST E +ĠG AME +Ġse ism +ĠK is +olog ne +Ġcow ork +Ġforc ibly +Ġthy roid +ĠP B +AN E +mar ried +h orse +Ġpoly mer +ĠCh al +od or +DE BUG +ĠCon text +Ġbl iss +Ġpin point +ĠMat hemat +leg ram +ĠWeek end +Ġlab elled +Ġb art +it les +Ġest rogen +âĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶ âĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶ +" ' +Ġvis ibly +Ġouts ider +aid a +Are a +Ġdisse min +Ġdish onest +ĠCl osed +ĠBullet in +ĠRam sey +sw ord +ĠX I +our ced +S ame +34 6 +ĠRe pe +ĠK ou +c ake +em is +C ache +ĠMe aning +ĠEn light +onom y +Ġmanifest ation +sw orth +J ay +Ġch ore +ö r +D ream +Ġsanction ed +Ġcult urally +ĠA ra +N av +Ġthe ological +Ġstr ut +ĠV O +ĠHand book +Ġconstruct ing +Ġ ¶ +ĠBenef its +ĠPsych ological +s ac +å ¸ +p olicy +ĠMat ters +ĠReport ed +ĠBy te +Ġvit ro +ĠM aiden +Ġl am +ĠJenn ings +Ġgar ment +ĠRut gers +ĠStaff ord +ĠWell ington +Ġinter mitt +Ġn pm +Ġord eal +Ġplug ged +o oming +in ished +fram ework +Ġtim ber +Ġc ass +Ġ8 50 +il ess +ĠRed ux +7 68 +St re +Ġsurpass ed +w hel +Ġparalle ls +Ġve il +ĠG I +ĠR EST +Ġread iness +s ort +Ġmod ifying +ĠSl ate +ru ff +Ġmar ble +Ġinf rared +Ġaud itor +ĠFANT ASY +ĠP overty +ĠS PD +Ġ" ( +K y +RA Y +Ġexecut ions +ĠBever ly +ĠMarx ism +ĠBur st +ĠK ali +est ones +Clear ly +E ll +ãģ § +ĠProceed ings +T oken +IF IC +ñ a +Cent ral +ĠH aley +ĠD rama +Ġform ations +OR N +Book s +Ġdom inating +ĠFly ers +ĠCompan ion +Ġdiscipl ined +ĠYug oslav +ĠSpell s +Ġv engeance +Ġland lords +L en +ĠO gre +ano ia +Ġpier cing +Ġcon greg +Ġscore r +ob ia +Ġnic kel +ĠLear ns +Ġre jo +Ġmaster piece +Fl ash +Ġinhab ited +ĠOpen GL +ĠD ud +ĠI CO +Ġar ter +Ġpl ur +Ġmaster y +Ġlong standing +st ed +Ġw ines +Ġtelev ised +ĠSh rine +ĠBay ern +Ġâ ĵĺ +Ġencl osure +j ohn +Ġprophe ts +ĠRes urrection +ĠOrd ers +Ġun even +r als +Ġd wind +ĠL ah +ĠSl oven +37 8 +Ġins istence +aff le +ĠCl one +Ġhard ship +ĠCongress man +Ġple ad +Ġreview ers +Ġc ured +Ġ19 35 +as ley +f ake +ĠTh inking +yd ia +P ART +ĠD ota +o it +Ġwh ipped +Ġb ouncing +ĠHispan ics +com ings +Ġcann abin +ĠCh ambers +ĠZ ack +Option al +Ġco ats +Ġprow ess +ĠNort on +Ġplain ly +Ġfre ight +Ġinhib ition +Ġcl am +Ġ30 3 +ke f +ale igh +L uke +Ġpsych o +ator ium +M ED +Ġtreat ies +Ġind isc +Ġd c +OP S +Ġresil ient +ĠInter state +Ġsl ack +Ġmund ane +Ġestab lishes +35 9 +Ġstr ained +Ġn ond +S us +Ġcast e +ar ate +ie ving +Ġunfair ly +Ġpars er +on ial +urs ive +V ia +ĠOtt o +ĠAuthor ities +stro ke +K R +ĠMer cy +Ġfurn ished +Ġout set +Ġmet ic +19 82 +olith ic +ĠT ent +og ical +ĠA ircraft +Ġh ides +ĠBec ame +Ġeduc ators +re aching +Ġvol atility +Ġtodd ler +ĠNAS CAR +ĠTw elve +ĠHigh lights +Ġgra pe +Ġspl its +Ġpe asant +Ġre neg +ĠMS I +Tem p +st ars +Ġtre k +ĠHy de +b inding +Ġreal ism +Ġox ide +ĠH os +Ġmount s +Ġbit ing +Ġcollaps ing +Ġpost al +Ġmuse ums +Ġdet ached +Ġrespect ing +Ġmonop ol +Ġwork flow +ĠC ake +Tem plate +ĠOrgan isation +Ġpers istence +36 9 +C oming +B rad +Ġredund ant +ĠG TA +Ġb ending +Ġrev oked +Ġoff ending +Ġfram ing +Ġprint f +Comm un +mem bers +Out side +Ġconst rued +Ġc oded +F ORE +Ġch ast +Ch at +Ind ian +ĠY ard +? !" +ĠP orts +ĠX avier +ĠR ET +' ." +ĠBo at +iv ated +ich t +umer able +D s +ĠDun n +Ġcoff in +Ġsecure ly +ĠRapt ors +ĠB es +Install ation +Ġin ception +ĠHealth y +end ants +Ġpsych ologists +ĠShe ikh +c ultural +ĠBlack Berry +sh ift +F red +oc he +Ġc akes +ĠS EO +ĠG ian +ĠAs ians +og ging +e lement +Ġpund its +ĠV augh +ĠG avin +Ġh itter +Ġdrown ed +Ġch alk +ĠZ ika +Ġmeas les +80 2 +â̦ .. +ĠAW S +] " +Ġdist ort +ĠM ast +Ġantib odies +ĠM ash +Mem ory +ĠUg anda +ĠPro b +Ġvom iting +ĠTurn s +Ġoccup ying +Ġev asion +ĠTher apy +Ġprom o +Ġelect r +Ġblue print +ĠD re +pr iced +ĠDep ot +Ġallev iate +ĠSom ali +m arg +n ine +Ġnostalg ia +ĠShe pherd +Ġcaval ry +Ġtor ped +ĠBlood y +x b +Ġs ank +Ġgo alt +report print +embed reportprint +clone embedreportprint +ĠIn itially +ĠF ischer +Ġnot eworthy +c ern +Ġin efficient +raw download +rawdownload cloneembedreportprint +c ation +ĠD ynasty +l ag +D ES +Ġdistinct ly +ĠEston ia +Ġopen ness +Ġg ossip +ru ck +W idth +ĠIb rahim +Ġpet roleum +Ġav atar +ĠH ed +ath a +ĠHog warts +Ġc aves +67 8 +Ġsafegu ard +ĠM og +iss on +ĠDur ham +sl aught +ĠGrad uate +Ġsub conscious +ĠEx cellent +ĠD um +---- - +Ġp iles +ĠW ORK +ĠG arn +ĠF ol +ĠAT M +Ġavoid s +ĠT ul +Ġble ak +EL Y +iv ist +light ly +P ers +ĠD ob +ĠL S +Ġins anity +Î µ +atal ie +En large +Ġtw ists +Ġfault y +Ġpir acy +Ġimp over +Ġrug ged +ĠF ashion +Ġs ands +' ? +sw ick +Ġn atives +Ġhe n +ĠNo ise +ãĥ Ĺ +Ġg reens +Ġfree zer +Ġd ynasty +ĠFather s +ĠNew ark +Ġarchae ological +Ġo t +ob ar +Ġblock ade +Ġall erg +L V +Ġdeb it +ĠR FC +ĠMil ton +ĠPress ure +Ġwill ingly +Ġdisproportion ate +Ġopp ressive +Ġdiamond s +Ġbelong ings +19 70 +Ġbell s +Ġimperial ism +Ġ2 27 +Ġexpl oding +ĠE clipse +Ġ19 19 +Ġr ant +Ġnom inations +34 7 +Ġpeace fully +ric a +ĠF UCK +Ġvib ration +mal ink +Ġro pes +ĠIv anka +ĠBrew ery +ĠBook er +ĠOw ens +go ers +Serv ices +ĠSn ape +Ġ19 1 +39 5 +Ġ2 99 +just ice +Ġb ri +Ġdisc s +Ġprom inently +Ġvul gar +Ġsk ipping +l ves +Ġtsun ami +37 4 +ĠU rug +ĠE id +rec ated +p hen +Ġfault s +ĠStart ed +9 50 +Ġp i +Ġdetect or +Ġbast ard +Ġvalid ated +Space Engineers +OUR CE +Ġ( ~ +Ġuns ur +Ġaff irmed +Ġfasc ism +Ġres olving +ĠCh avez +ĠC yn +Ġdet ract +L ost +Ġrig ged +Ġhom age +ĠBrun o +55 5 +ec a +Ġpress es +Ġhum our +Ġsp acing +Ġ' / +olk ien +C oun +OP ER +T re +S on +ĠCambod ia +ier re +m ong +o zy +Ġliquid ity +ĠSov iets +ĠFernand o +Ġ2 29 +Ġsl ug +ĠCatal an +elect ric +Ġsc enery +ĠH earth +Ġconst rained +Ġgoal ie +ĠGu idelines +ĠAm mo +ĠPear son +Ġtax ed +Ġfet us +Resp onse +ĠAlex is +th ia +G uy +Ġrecon struct +Ġextrem es +Ġconclud ing +ĠP eg +ook s +Ġded uctions +R ose +Ġground breaking +ĠT arg +ãĥ ģ +ĠRe ve +res ource +Ġmo ons +Ġelectrom agnetic +Ġamid st +ĠVik tor +N ESS +B ACK +Ġcomm ute +ĠAna heim +Ġfluct uations +6 40 +Ġnood les +ĠCop enhagen +ĠT ide +ĠGri zz +ĠS EE +Ġpip elines +Ġsc ars +end o +ag us +ĠE TF +/ # +ĠBec ome +44 8 +Ġvis c +ĠRecomm ended +Ġj umper +Ġcogn ition +Ġassass in +Ġwitness ing +ĠSet up +Ġl ac +v im +IS M +p ages +SS L +35 8 +Ġad ject +indust rial +l ore +cher y +Ġgl itter +Ġc alf +Flor ida +Ġspoil ers +Ġsucceed s +Ġch anting +Ġslog ans +ĠTr acy +Vis it +rol ogy +Ġm ornings +Ġline age +Ġs ip +Ġintense ly +Ġflour ish +ĠSle eping +ĠF em +or por +ĠK lan +ĠDar th +h ack +ĠNi elsen +Ġtum ors +Ġprocure ment +ĠY orkshire +Ġra ided +K Y +An na +Ġ// [ +ĠDis order +ĠMust ang +ĠW en +ĠTry ing +s q +Ġdeliver ies +Ġshut ter +Ġcere bral +Ġbip olar +ĠC N +l ass +j et +Ġdeb ating +> : +Ġe agle +gr ades +ĠD ixon +UG C +M AS +ĠDr aco +ĠMach ines +aff er +Ġem an + ² +pr on +ĠG ym +Ġcompar atively +ĠTrib unal +PR O +Ġle x +Ġfert ile +Ġdep ressing +Ġsuperf icial +ess ential +ĠHun ters +g p +Ġprom inence +L iber +ĠAn cest +ote chnology +Ġm ocking +ĠTra ff +ĸ ļ +Med ium +I raq +Ġpsychiat rist +Quant ity +ĠL ect +Ġno isy +5 20 +G Y +Ġsl apped +ĠM TV +Ġpar a +p ull +Mult iple +as her +Ġn our +ĠSe g +Spe ll +v ous +ord ial +Sen ior +ĠGold berg +ĠPl asma +ne ed +Ġmess enger +ere t +Ġteam ed +Ġliter acy +ĠLe ah +ĠD oyle +Ġem itted +U X +Ġev ade +Ġm aze +Ġwrong ly +ĠL ars +Ġstere otype +Ġpled ges +Ġarom a +ĠM ET +Ġac re +ĠO D +Ġf f +Ġbrew eries +ĠH ilton +und le +ĠK ak +ĠThank fully +ĠCan ucks +in ctions +ĠApp ears +Ġco er +Ġundermin ed +ro vers +And re +Ġbl aze +um ers +Ġfam ine +amp hetamine +ulk an +Am ount +Ġdesper ation +wik ipedia +develop ment +ĠCor inth +uss ia +Jack son +L I +N ative +R s +Oh io +ĠKath leen +F ortunately +Ġattend ant +ĠPre ferred +ĠDid n +ĠV s +M is +Ġrespond ent +Ġb oun +st able +Ġp aved +Ġunex pl +ĠChe ney +L M +ĠC ull +bl own +Ġconfront ing +oc ese +serv ing +W i +ĠLith uania +ann i +Ġst alk +h d +Ġv ener +AP H +ynchron ous +UR R +um ably +hist oric +H alf +H ay +Ġresil ience +spe ction +Ġabandon ing +O bs +ĠDeb bie +Ġgrad ient +ĠPl aint +ĠCan al +AR CH +Ġexpans ive +Ġfun g +Ġb ounced +U nd +Ġprec autions +Ġclar ification +Ġd agger +Ġgri ps +Ġ µ +ĠRiver a +ĠUnd ead +is ites +ĠFIR ST +ñ o +aud i +Ġhost ages +Ġcompl iant +Ġal umni +Se ven +Ġcyber security +e ither +Col lect +Ġinvari ably +ĠS oci +Ġlaw maker +Ġa le +ĠPerson ally +N azi +Ġcustom ization +ĠPro c +ĠSask atchewan +eat uring +Ġsp ared +Ġdiscontin ued +Ġcomput ational +ĠMotor ola +Ġsuprem acist +government al +Ġparad ise +ĠDown ing +ĠNik on +Ġcat alyst +ber ra +Tor onto +8 75 +bet a +ĠMac ron +Ġunreal istic +ve ctor +ĠVeh icles +it iveness +ĠR V +ĠCol bert +s in +o ji +ent in +ĠKr ish +hell o +ff ield +ok y +ĠT ate +Ġmap le +Ġa ids +chem ical +33 4 +n uts +ĠWar p +Ġx x +ĠRob b +umer ous +_- _ +ft ime +ĠV W +Ġw inger +ĠD ome +t ools +ĠP V +ĠGe orgetown +Ġg eared +Ġjihad ists +Ġc p +Ġster oids +M other +cler osis +ĠDR M +nes ia +Ġl inger +Ġimm ersive +ĠC OUN +Ġoutwe igh +ens ual +B and +Ġtransform s +mat ched +ps ons +ĠJud icial +f actor +Ġrefer ral +Ġodd ly +ĠW enger +B ring +ĠB ows +60 2 +IC LE +Ġl ions +ĠAcad emic +ĠTh orn +ĠRa ider +kef eller +St orage +L ower +ĠOr t +ĠEqu ality +AL T +ĠS OC +T ypes +Ġl yn +ĠAss et +co at +TP P +C VE +ĠPione er +app lication +Mod ern +ĠH K +En vironment +Al right +R ain +IP P +ĠShi ite +Ġm ound +ĠAb ilities +cond ition +St aff +Ġcompet ence +ĠM oor +ĠDi ablo +Ġwith held +Ġost ensibly +ĠB rom +Ġms g +Ġden omin +ĠRef erences +ĠF P +Ġplun ged +Ġp amph +m oving +cent ral +Ġdown right +Ġf ading +T al +T yp +ĠTh y +uk es +it he +Ġo ve +Ġbatt led +Ġseaf ood +Ġfig ur +ĠR D +c rop +Ġsqu ads +{ \ +à ¹ +ĠE h +Ġinterview ing +ĠQ in +Ġas piring +PL IC +Ġcla uses +ĠG ast +ĠN ir +Ġl uggage +Ġh ose +Ġsystem d +Ġdesc ending +ĠRev ised +ĠR ails +al ign +70 9 +33 7 +Ġf ug +charg ing +t ags +Ġut er +k ish +WAR NING +49 0 +prof its +Ġvoy age +Ġa ce +ĠV anguard +ĠT anks +ĠM uk +Ġ2 26 +S afe +Ar mor +Ġvolcan ic +Ġwom b +ĠM IL +Ġbegin ner +ĠRec ogn +ĠA AP +PL AY +) ! +Ġdetect ing +c n +Ġbre aches +Bas ically +ĠP ag +ĠMunicip al +ĠInd ie +ĠL af +ĠDis able +ĠOl son +Ġrest rained +Ġrul ings +Ġhum ane +ev ents +ĠCinem a +display Text +ĠH atch +action Date +onna issance +Ġassault ing +ĠL ug +CH AT +Ġvig orous +ĠPer se +Ġintoler ance +ĠSnap chat +ĠSh arks +Ġd ummy +ĠDi agn +ĠGu itar +im eters +40 3 +RE G +A x +Ġsepar ates +ĠMah m +Ġt v +j ah +O OL +C irc +ĠWinds or +uss ian +Ġintu ition +Ġdis dain +ĠDon ovan +Ġ2 21 +E mb +Ġcondem ning +Ġgener osity +zz y +Ġpant ies +ĠPre vent +Action Code +AN A +34 2 +external ActionCode +Ġspec ifying +Ġcryst all +J ere +Ġru pt +ĠApp rentice +Ġprof iling +Ð º +St rike +Ġsid eline +Ġoblig ated +Ġocc ult +Ġbureaucr atic +ant ically +rupt ed +neg ative +ĠEthiop ia +ĠC ivic +Ġins iders +el igible +ĠTV s +ĠB AR +ĠT I +i ologist +ĠA IR +Ġsubstit uted +Ar ab +ĠS aul +ĠY og +p rem +Ġbuild ers +Ġstation ary +Ġdoubt ful +Ġvig orously +Ġthr illing +Ph ysical +ĠCare y +ĠHyd ra +geon ing +ĠS ly +y ton +Ġborrow ers +ĠPark inson +Ġ ë +ĠJama ica +Ġsat ir +Ġinsurg ents +ĠF irm +Ġis ot +ĠK arn +our ning +ak ens +doc s +l ittle +ĠMon aco +CL ASS +Tur key +L y +ĠCon an +ass ic +Ġstar red +ĠPac ers +et ies +Ġt ipping +M oon +ĠR w +s ame +Ġcav ity +Ġgo of +ĠZ o +Sh ock +um mer +Ġemphas izes +Ġreg rett +Ġnovel ty +Ġen vy +ĠPass ive +r w +50 5 +Ġind ifferent +ĠR ica +ĠHim self +ĠFred die +Ġad ip +ä¸ Ģ +Ġbreak out +Ġhur ried +ĠHu ang +ĠD isk +Ġro aming +?????- ?????- +U V +ĠRick y +ĠS igma +Ġmarginal ized +Ġed its +Ġ30 4 +mem ory +Ġspec imen +29 3 +ãģ ¯ +Ġvert ically +Ġaud ition +ĠHe ck +Ġc aster +ĠHold ings +ad al +ĠC ron +ĠL iam +Ġdef lect +P ick +ĠDeb ug +RE F +Ġvers atility +ot hes +class ified +ĠMah ar +ĠH ort +C ounter +st asy +not iced +33 1 +ĠSh im +f uck +ĠB ie +Ġair ing +ĠPro tein +ĠHold ing +Ġspect ators +ili ated +ĠThat cher +n osis +ãĥ¼ ãĥ³ +Te le +B oston +ĠTem pl +st ay +Ġdecl arations +47 9 +Vol ume +ĠDesign er +ĠOver watch +id ae +Ġon wards +Ġn ets +ĠMan ila +part icularly +Ġpolit ic +o other +Ġport raits +Ġpave ment +c ffff +Ġs aints +Ġbegin ners +ES PN +Ġshort comings +âķIJ âķIJ +Ġcom et +ĠOrgan ic +qu el +Ġhospital ized +Bre ak +Ġpe el +dyl ib +asp x +ur ances +ĠT IM +P g +Ġread able +ĠMal ik +Ġm uzzle +Ġbench marks +d al +ĠV acc +ĠH icks +60 9 +ĠB iblical +he ng +Ġover load +ĠCivil ization +Ġimm oral +Ġf ries +ãĤ Ĵ +Ġreprodu ced +Ġform ulation +j ug +ire z +g ear +Ġco ached +Mp Server +ĠS J +ĠK w +In it +d eal +ĠO ro +ĠL oki +ĠSong s +Ġ23 2 +ĠLou ise +asion ally +Ġunc ond +olly wood +Ġprogress ives +ĠEn ough +ĠDo e +Ġwreck age +Ġbr ushed +ĠBase Type +Ġz oning +ish able +het ically +ĠC aucus +ĠH ue +Ġk arma +ĠSport ing +Ġtrad er +Ġseem ing +ĠCapt ure +4 30 +b ish +Ġt unes +Ġindo ors +ĠSp here +ĠD ancing +TER N +Ġno b +ĠG ST +m aps +Ġpe ppers +F it +Ġoverse es +ĠRabb i +ĠR uler +vert ising +off ice +xx x +Ġra ft +Ch anged +Ġtext books +L inks +ĠO mn +ãĢ ij +Ġinconven ience +ĠDon etsk += ~ +Ġimplicit ly +Ġboost s +ĠB ones +ĠBo om +Cour tesy +Ġsens ational +AN Y +Ġgre edy +ed en +Ġinex per +ĠL er +ĠV ale +Ġtight en +ĠE AR +ĠN um +Ġancest or +S ent +ĠH orde +urg ical +all ah +Ġsa p +amb a +ĠSp read +tw itch +Ġgrand son +Ġfract ure +Ġmoder ator +ĠSe venth +ĠRe verse +Ġestim ation +Cho ose +Ġpar ach +Ġbar ric +ãĢ IJ +Ġcomp ass +Ġall ergic +âĢ ķ +OT HER +err illa +Ġw agon +Ġz inc +Ġrub bed +ĠFull er +ĠLuxem bourg +ĠHoo ver +Ġli ar +ĠEven ing +ĠCob b +est eem +Ġselect or +ĠB rawl +is ance +ĠE k +Ġtro op +Ġg uts +ĠApp eal +ĠTibet an +Ġrout ines +ĠM ent +Ġsummar ized +steam apps +Ġtr anqu +Ġ19 29 +or an +ĠAut hent +Ġg maxwell +Ġappre hens +Ġpo ems +Ġsa usage +ĠWeb ster +ur us +Ġthem ed +Ġl ounge +Ġcharg er +Sp oiler +Ġsp illed +h og +ĠSu nder +ĠA in +ĠAng ry +Ġdis qual +ĠFrequ ency +ĠEther net +Ġhel per +Per cent +Ġhorr ifying +Ġa il +ĠAll an +EE E +ĠCross ing +44 9 +Ġh olog +ĠPuzz les +ĠGo es +eren n +60 4 +ãģ ı +ĠRaf ael +Ġatt en +ĠE manuel +Ġup ro +ĠSus p +P sych +ĠTr ainer +ĠN ES +ĠHun ts +bec ue +Ġcounsel or +R ule +Ġtox ins +Ġb anners +r ifice +Ġgreet ing +Ġfren zy +Ġall ocate +Ġ* ) +ex pr +50 3 +ĠCh ick +ĠT orn +Ġconsolid ation +ĠF letcher +sw itch +fr ac +cl ips +ĠMcK in +ĠLun ar +Mon th +IT CH +Ġscholar ly +rap ed +39 8 +Ġ19 10 +Ġe greg +Ġin secure +Ġvict orious +cffff cc +Ġsing led +Ġel ves +ĠW ond +bur st +Ġcam oufl +ĠBL ACK +Ġcondition ed +ç ī +ans wered +Ġcompuls ory +asc ist +Ġpodcast s +ĠFrank furt +bn b +Ġne oliberal +ĠKey board +ĠBel le +w arm +Ġtrust s +Ġins ured +ĠBu cc +us able +60 7 +ĠPl ains +Ġ18 90 +Ġsabot age +Ġlod ged +f elt +Ġg a +ĠN arc +ĠSal em +Ġsevent y +ĠBl ank +p ocket +Ġwhis per +Ġm ating +om ics +ĠSal man +ĠK ad +Ġan gered +Ġcoll isions +Ġextraord inarily +Ġcoerc ion +G host +b irds +è Ģ +k ok +Ġper missible +avor able +Ġpo inters +Ġdiss ip +ac i +Ġtheat rical +ĠCos mic +Ġforget ting +Ġfinal ized +å¤ § +y out +l ibrary +Ġbo oming +ĠBel ieve +ĠTe acher +ĠL iv +ĠGOOD MAN +ĠDomin ican +OR ED +ĠPart ies +Ġprecip itation +ĠSl ot +R oy +ĠComb ined +Ġinteg rating +Ġch rome +Ġintest inal +ĠRe bell +Ġmatch ups +Ġblock buster +ĠLore n +ĠLe vy +Ġpre aching +ĠS ending +ĠPur pose +ra x +f if +Ġauthor itative +ĠP ET +ast ical +Ġdish on +Ġchat ting +Ġ"$ :/ +Connect ion +Ġrecre ate +Ġdel inqu +Ġbro th +ĠD irty +ĠAd min +z man +Ġscholars hips +Ġ25 3 +cont act +als a +7 67 +c reen +abb age +Ġ19 15 +Ġbl ended +Ġal armed +L anguage +35 6 +Ġbl ends +ĠCh anged +W olf +Ġhe pat +Creat ing +Ġper secut +Ġsweet ness +art e +Ġforfe iture +ĠRober to +im pro +N FL +ĠMag net +Det ailed +Ġinsign ificant +ĠPOL IT +ĠBB Q +ĠC PS +Ġse aw +amin er +m L +end if +f inals +Ġ26 5 +u ish +Ġ} ) +ĠPro blems +Ġem blem +Ġserious ness +Ġpars ing +Ġsubst itution +Ġpress ured +Ġrecy cled +ale b +Rub y +Ġprof iciency +Dri ver +ĠW ester +: ' +AF TA +Ġm antle +ĠClay ton +fl ag +Ġpractition er +c overed +ĠSt ruct +add afi +4 25 +ĠTown ship +ĠHyd ro +Lou is +34 3 +Ġcond o +ĠT ao +Ġutil ization +Ġnause a +ĠDem s +rid ges +p ause +Ġform ulas +Ġchall enger +37 6 +Ġdefect ive +ĠRail way +ĠPub Med +Ġyog urt +l bs +ĠNor folk +OP E +ĠMood y +Ġdistribut or +Ġscroll s +Ġextract s +St an +Ġv iability +Ġexp oses +Ġstar vation +ĠStep s +ĠD odd +f ew +ST D +33 2 +Ġclos ures +Ġcomplement ary +ĠS asha +ump y +Ġmon et +Ġartic ulate +ĠDo ct +k iller +Ġsc rim +Ġ2 64 +Ġprost itutes +Ġse vered +Ġattach ments +Ġcool ed +L ev +ĠF alk +f ail +Ġpolic eman +ĠD ag +Ġpray ed +ĠK ernel +Ġcl ut +Ġc ath +Ġan omaly +St orm +em aker +ĠBreak fast +ul i +o ire +J J +h z +Oper ation +ĠS ick +35 4 +ĠGuatem ala +R ate +Ġexp osures +f aces +ĠArch ae +ra f +ĠM ia +Ġ20 25 +Ġop aque +Ġdisgu ised +ĠHead quarters +S ah +Ġp ots +9 78 +ĠM alf +Ġfrown ed +Ġpoison ous +ĠCon vers +ee ks +Ġcr ab +." " +Ġtre ason +Ġr anc +Ġescal ating +Ġwar r +Ġmob s +Ġl amps +ĠSun shine +ĠBrun swick +Ph ones +Ġspe lled +ĠSk ip +Ġ20 50 +Ġ19 11 +ĠPl uto +ĠAm end +Ġme ats +38 7 +Ġst omp +ĠZh ou +ĠLevi athan +ĠHaz ard +ad v +ĠOr well +Ġal oud +Ġb umper +ĠAn arch +ub untu +ĠSer ious +f itting +ĠOption al +ĠCec il +RE AM +Ġser otonin +Ġcultiv ate +ag ogue +} \ +Ġmos ques +ĠSun ny +Ġre active +rev olution +ĠL up +ĠFed ora +Ġdefense man +ĠV ID +ist ine +Ġdrown ing +ĠBroad casting +Ġthr iller +ĠS cy +Ġacceler ating +Ġdirect s +od ied +b ike +d uration +Ġpain fully +R edd +Ġproduct ions +Ġg ag +Ġwh ist +Ġs ock +Ġinf initely +ĠConc ern +ĠCit adel +Ġlie u +Ġcand les +ogene ous +arg er +Ġheaven ly +inflamm atory +Per formance +C s +ruct ose +az aki +Ġp essim +Ġinf erence +Ġpow d +ĠZ oe +Ġpain ts +Ġd azz +pt a +-------- --- +Ġins pir +ĠExper imental +ĠKn ife +reg or +b ors +Ġshow ers +rom eda +Ġs aint +Ġben ign +ĠJ iang +Ġenvision ed +Ġsh roud +IF T +H O +Ġsh uff +ĠI CC +Ġse greg +Ġrevis it +ighth ouse +L i +Ġsub strate +ĠSe as +ĠRew ard +ĠH ep +ĠBr ass +s bm +Ġelim inates +Ġst amina +ĠV AT +ĠLo an +Ġconst raint +Ġappropri ated +Ġp es +ĠA LE +r anging +Ġ40 4 +39 2 +Ġintellectual s +ach u +Ġrestruct uring +ĠLe vin +Ġrun es +Ġdelight ful +Ġcarbohyd rates +ĠMod els +ĠExp o +Ġtransport ing +all oc +Ġring ing +S amsung +Ġscarce ly +ĠURL s +ĠM AS +Ġprot otypes +Ġnarr ator +ĠCPU s +cd n +ĠBart on +Ġdecided ly +ĠSh u +ix ir +oc ious +ĠMy st +N intendo +Ġre use +Ġforg iven +F ew +in ical +n at +Ġseam less +ĠEv a +ĠE VE +ĠJ O +land ers +Ġso fter +neg ie +Ġtrans ient +Ġorb ital +Ġfulf il +ĠK om +Hop efully +Ġdynam ically +ĠHun ger +å Ľ +ĠArmen ia +el man +ber to +Ġp ige +ĠID s +lim it +Ġve ins +Ġso aring +p acks +Gold en +ĠCr ab +ist or +ĠR PM +Ġ$ $ +g ression +Ġjihad ist +Ġgam ble +Ġcare g +Ġinf lated +F ace +ĠFire arms +ĠEm manuel +â Ŀ +Ġsh ocks +gr ab +Ġspl end +ĠHP V +ab ortion +Ab ove +Ent ity +play ers +Ġcomm enced +ul ence +Ġfulfill ment +Ġembod iments +ĠW elfare +Ġha il +Ġ< @ +tt en +Ġcat cher +ĠJ azeera +Ġvolcan o +Ġstabil ize +ĠHand ler +Ġintens ified +ĠAb rams +Ġhum iliation +p aced +60 5 +ĠCent OS +Spe cific +Ġhe ed +ĠC AM +ĠGal ile +D ie +Ġabol ished +ĠThom son +ĠTe achers +ĠW ass +j ong +ĠIS BN +ĠAll ies +sh ake +å · +v ict +How ard +Ġde em +Ġexceed ingly +ĠSmart stocks +ib e +Ġdoor way +Ġcompet ed +ig mat +Ġnational ists +Ġg room +ĠKe en +Ġdispos able +de cl +ĠT olkien +ĠSche me +Ġb iod +Ġav id +ĠEl on +ag ar +ĠT SA +R oman +Ġartific ially +Ġadvis ors +X L +ĠInf erno +36 6 +Ġted ious +ĠPhot ography +ĠCar rie +Ġtro pe +ĠSand ra +Ġdec imal +Que en +ĠGund am +ĠO M +ote ch +N BA +Ġ19 32 +Ġent renched +ĠMar ion +Ġfr aternity +Lab our +Hen ry +Ġlat itude +E ither +Ġenh ances +ĠPot ential +Ġsh ines +id ad +Ġbread th +Ġcapac ities +ĠðŁ ĻĤ +ĠBron x +Ġsex es +Ġdifferent iation +Ġheavy weight +ĠT aj +d ra +Ġmigr ate +Ġexhaust ion +ĠR UN +els ius +ĠCu omo +Ġgu itars +Ġcl ones +ĠSom ew +ĠP ry +------------ - +Ġwarr anted +cy cles +Ġsalv age +Ġdis ks +R ANT +ĠNGO s +ĠMart ian +":[ {" +Ġadd icts +oj ure +il let +Ġamazing ly +art ments +p ixel +ĠGPU s +Lay out +è £ +ĠTam il +ĠBas il +Ġimpart ial +ĠSt ructure +f ork +b ryce +Ġr idge +ĠHamb urg +ri ous +Ġbl itz +cig arettes +Ġcan ned +40 2 +Ġiron ically +Ġcompassion ate +ĠHaw kins +. # +ĠCat hedral +Ġrall ied +in ternal +Ġqu ota +st akes +T EXT +m om +Ġcomple tes +Ġ23 8 +Ġsh rug +ãĥ ij +ĠN inth +Ġrev ise +ĠProv ider +Ġtre acher +Ġqu asi +ĠPR ES +Ġdep osition +Ġconfidential ity +iss ors +Ġim balance +Ġspan ning +Ġang ular +ĠC ul +commun ication +ĠNor a +ĠGen ius +op ter +Ġs acked +Sp ot +Ġfine ly +ĠCH R +28 2 +w aves +Pal est +ĠRo hing +N L +è ¿ +Ġsh itty +ĠSc alia +4 75 +Pro gress +Ġreferen cing +Ġclass rooms +ab ee +Ġs od +hes ion +70 8 +ĠZucker berg +ĠFin ish +ĠScot ia +ĠSav ior +ĠInstall ation +an tha +( - +Ġ30 2 +ĠP unk +Ġcr ater +yout u +Ġro ast +Ġinflu encing +Ġd up +ĠJ R +ĠG rav +Ġstat ure +Ġbath rooms +A side +W iki +me an +ĠZ ak +ĠOn es +ĠN ath +Ġhyper t +Ġcommence ment +C ivil +Ġmoder ately +Ġdistribut ors +Ġbreast feeding +Ġ9 80 +ĠS ik +ĠC ig +ĠAM ER +R IP +ĠCare er +ust ing +Ġmess ed +Ġe h +ĠJ ensen +/ $ +Ġblack mail +Ġconvers ions +Ġscientific ally +Ġmant ra +p aying +Ġiv ory +ĠCour ts +OU GH +aunt let +Ser ial +B row +ĠH undreds +3 23 +Ġpe e +Ġlin ux +Ġsub mer +ĠPrinc ipal +48 5 +ĠD SL +ĠCous ins +Ġdoctr ines +ĠAthlet ics +Ġ3 15 +ĠK arma +Ġatt ent +ur ger +Ġpresc ribe +Ġenc aps +ĠC ame +Ġsecret ive +ĠCr imes +d n +C lean +ĠEgypt ians +ĠCar penter +Ġ ll +H um +ĠMil o +Ġcapital ists +Ġbrief ed +T we +ĠBas in +elve t +M os +Ġplun ge +ĠKa iser +ĠFu j +ill in +Ġsafegu ards +Ġo ste +ĠOpportun ity +ĠM afia +ĠCall ing +ap a +ur ban +br ush +ill ard +c é +int elligence +ĠL ob +ĠDru id +Ġsm oother +Ġfoot ing +Ġmotor ists +arc ity +Ġmascul inity +Ġm ism +Ġabdom inal +ĠTa vern +ĠR oh +Ġesc apes +s igned +Anth ony +Ġsacrific ing +Ġintim acy +Ġan terior +ĠK od +Ġmot if +Ġg raz +Ġvisual ization +Ġguitar ist +ĠTro tsky +m agic +D ar +ĠMor i +Ġw ards +Ġtoile ts +l est +Ġtele port +ĠSund ays +ĠPl at +ET S +Ġe Sports +Pat rick +ĠK atherine +en ko +Ġhas sle +ĠM ick +gg les +Ġh ob +aint ain +Ġair borne +Ġsp ans +Ġch ili +Ġa perture +Ġvolunte ered +ĠInc ident +ĠF res +ĠVeter an +augh tered +ing o +Ġun insured +CL OSE +Ġf use +Ġer otic +Ġadvert ise +ra ising +Text ure +Ġatt ends +ĠRE AL +udd led +Ġsm oot +Ġ30 5 +ĠWill is +Ġbl ond +An alysis +ĠV T +on ica +Ġstrongh old +R F +N M +. >> +Ġprosper ous +Ġbo asted +29 2 +ĠManufact uring +PR ESS +g ren +Ġpharm acy +ĠRoc kefeller +k ai +Ġth umbs +ĠH ut +Ġmother board +Ġguard ians +ĠAl ter +ll ular +Ġsh ack +Ġwise ly +Ġback bone +erv a +Ġsu icides +ĠMcG regor +ij ah +E mer +ĠB rav +Ġdesign ate +P OST +produ ced +Ġcleans ing +irl wind +ex istent +ĠHum ph +ĠPay ne +Ġv ested +Å ¡ +Ġstring ent +ion a +Ġuns ub +Ġsum med +ĠHer cules +sub ject +ĠR agnar +ĠN os +Ġcharacter ization +Ġsav vy +ĠDaw son +ĠCas ino +Ġf ri +ĠBar rier +Ġmis information +Ġins ulation +Ġcorrid ors +Ġair planes +ĠNo ct +ah i +Ġ19 16 +k b +arm ac +Ġsh un +Ġsche ma +Ġhorr ified +Ġ23 9 +aund ers +N B +i ates +er ity +ĠSh ard +Ġr arity +Ġgroup ed +ĠGh ana +again st +ĠBi ological +ĠA ware +ow ell +Ï Ħ +ĠBe au +sh aw +H ack +ĠJul ius +US S +ol son +aun a +c ru +ĠMaur ice +ĠI k +Ġsequ encing +Ġradical s +Ġ( ?, +v irtual +Ġany ways +Ġreper c +Ġhand lers +Ġhes itant +é ĥ +ĠM F +ple mentation +ass ociated +Ġcampaign ed +ĠY ue +ut ations +ĠY oga +Ġsim mer +Ġro ds +Ġmel ody +Ġconv oy +v ideos +Ġscreen ed +N eg +ochem ical +Ġ( )) +Ġultr as +Ġant ip +ĠIsland ers +70 4 +Ġfet ish +Ġridic ulously +ĠK art +Ġmitochond rial +Ġinterf ering +Build er +Ġover fl +Ġac ne +ĠM ud +ĠK err +f lex +ĠPost al +ĠBalt ic +47 7 +ĠPers ons +our age +H B +ĠM use +ĠImm ortal +ĠDri ving +Ġpet itions +Ġsubsc ript +Ġs orce +ĠProcess or +ut on +S ony +Ġph on +Ġr aced +ĠAnth rop +Ġday time +ĠEx ercise +Add ing +Ġeng ages +ĠQual comm +Ġmir acles +Ġmem es +ĠDr ink +ĠOri oles +Ġhair s +ĠPol ar +ath om +Ġsl ippery +ĠR emy +Ġcar amel +ĠY EAR +Ġal k +I gn +a ution +ĠMer lin +ĠC ran +Ġap ologies +Ġ4 10 +Ġout ing +ĠMem ories +app ointed +Ġcount ered +u ld +pos ing +Ġfire wall +ĠW ast +ĠW et +work ed +se ller +Ġrepe aled +ere o +ass uming +BL IC +m ite +ĠCEO s +ĠChap el +ellig ent +________________ ________ +D og +Ġw art +Ġsubsc riber +s ports +Ġbe gged +ĠM V +Ġsem if +eth ical +Ġpre ach +Ġrev ital +Ġpun itive +Ġshort cuts +Ġinstit uted +ĠWars aw +Ġabdom en +ĠK ING +Ġsuper intendent +Ġf ry +ĠGe o +T OR +Ġcontrad ictions +apt ic +Ġlandsc apes +b ugs +Ġcl ust +Ġvol ley +c ribed +Ġt andem +Ġrob es +WH AT +Ġpromot er +Ġel oqu +review ed +ĠD K +ĠPl ato +Ġf ps +T ank +ĠDer rick +Ġpriorit ize +as per +ĠHond uras +ĠCom pleted +ne c +Ġm og +n ir +ĠMay o +DE F +st all +in ness +ĠVolks wagen +Ġprec aution +ĠM ell +i ak +ist ries +Ġ24 8 +Ġoverl apping +Sen ate +ĠEnh ance +res y +rac ial +OR TS +ĠM ormons +Str ong +ĠCo ch +Mex ico +ĠMad uro +Ġj ars +Ġcan e +W ik +oll a +iff erence +Ġphysic ist +ĠMag gie +Ġ28 5 +Ġdep iction +ĠMcL aren +J u +Ġsl ows +Ġcommission ers +ĠWill ow +ĠExpl os +hov ah +Ġtechn ician +Ġhom icides +ĠFl av +ĠTr uman +Ġ100 00 +u ctor +Ġsh ader +News letter +45 7 +Ġre ver +Ġhard ened +Ġwhere abouts +Ġrede velop +Ġcar bs +Ġtra vers +Ġsqu irrel +Ġfoll ower +Ġs ings +50 8 +Ġrabb its +emon ium +Ġdocument ing +Ġmisunder stood +) ' +R ick +gg ies +Ġprem ie +Ġsk ating +Ġpass ports +Ġf ists +aged don +H aw +AC P +0 80 +ĠThough ts +ĠCarl son +Ġpriest hood +h ua +Ġdun geons +ĠLo ans +Ġant is +Ġfamiliar ity +ĠS abb +op al +ĠIn k +st rike +Ġc ram +Ġlegal ized +Ġcu isine +Ġfib re +Tra vel +ĠMon ument +OD Y +eth y +Ġinter state +ĠP UR +em porary +ĠArab ian +develop ed +Ġsadd le +Ġg ithub +ĠOff er +ĠIS P +ro let +ĠSUP ER +ĠDen is +Ġmultipl ier +Ġstir red +Interest ingly +Ġcustom ary +Ġbill ed +he x +Ġmultipl ied +Ġfl ipping +ĠCros by +Ġfundament als +ia e +ĠPlay ed +ĠAt om +am azon +ĠFl am +ee z +activ ated +Ġtables poon +Ġliberal ism +ĠPal in +ĠP atel +N um +ĠT AM +Ġs urn +ĠRel oaded +Ġco ined +" ], +ĠCl ash +ĠAg u +Ġprag matic +ĠActiv ate +Ġ8 02 +Ġtrail ers +Ġsil hou +Ġprob es +Ġcirc us +ĠB ain +ĠLind say +ĠAb bey +Del ivery +Ġconcess ion +Ġgast ro +ĠSpr ite +Ä Ł +and el +Ġg imm +Ġaut obi +ĠT urtle +Ġwonder fully +ĠHar am +ĠWorld wide +ĠHand le +Ġtheor ists +Ġsle ek +ĠZh u +ograph ically +EG A +ĠOwn ers +ath s +ĠAntar ctic +n atal +=" " +fl ags +`` `` +Ġs ul +K h +Ġpot assium +Ġlinem an +Ġcere al +ĠSe asons +Ġ20 22 +Ġmat hematic +Ġastron omers +prof essional +Ġf ares +cknow led +Ġch i +Ġyoung sters +Ġmistaken ly +Ġhem isphere +ĠDiv inity +r one +Ġ" , +r ings +Ġattract s +v ana +å ¹ +C AP +Ġplay list +Ġpor ch +ãģ £ +Ġincorpor ates +Ġso ak +Ġassert ing +ĠTerror ism +ĠP ablo +J a +ces ter +Ġfear ing +ĠPr ayer +Ġescal ated +G W +Ġro be +ĠBright on +ac ists +ĠSym phony +ĠDwar f +ĠPar ade +ĠLe go +Ġinex pl +Ġl ords +le af +RA G +l iber +Ġcig ars +ĠJe hovah +60 6 +WIND OWS +ĠLiber ia +eb us +He avy +Ġl ubric +ĠR W +angu ages +Ġnarrow ed +com puter +ĠE mber +Ġmurder ing +Ġdown stream +ĠT uls +ĠT ables +Top ic +ĠAcc uracy += / +l ost +ĠRe i +Ġprogress es +b ear +Ġestablish ments +Just in +ĠPe ach +ĠG omez +å ¿ +ĠTri angle +Id ent +ĠH ive +Res ources +Ġmix es +ĠAss uming +M u +Ġhyp oc +Ġs ane +ĠW an +id ious +Su ccess +Ġ io +Ang el +Ġdanger ously +ĠCreat ure +W ORK +: [ +ĠKat rina +List ener +M iller +ĠId lib +h ang +Ġcircum vent +h ref +Ġcel estial +ĠWe eks +ĠP ug +ĠDal ton +Ġsubpoen a +uk u +Ġpers isted +pe i +old ing +ĠDoc uments +ĠH ast +ĠC ENT +Ġprim er +Ġsyn onymous +Ġn ib +om bs +Ġnot ation +ĠD ish +ĠAt mosp +Ġforb id +ĠAN G +pat tern +l os +Ġproject iles +b rown +." , +ĠVen om +Ġfierce ly +ub lished +ĠU ran +ĠNic arag +4 10 +ĠC AL +OT OS +ĠMir acle +ĠEn chant +Ġguard ing +app end +Att ach +Ġlevel ed +Ġcond oms +ih ilation +64 9 +Ġnight mares +ĠTHE Y +ĠST ART +ĠK inn +Ġroomm ate +Ġhy giene +o pping +J ob +Ġl vl +ĠV ER +ĠKe eping +ab etic +Ġformat ting +eral a +Ġrev isions +Ġres urg +T el +ĠGood man +35 3 +p od +Ġind isp +ĠTrans lation +Ġg own +ĠM und +Ġc is +Ġby stand +col lect +ĠPun jab +act ively +ĠG amb +te ll +Ġimport ing +g encies +Ġloc om +ĠBr ill +H oly +ĠBer ger +Ġshow down +Ġrespond ers +IL Y +Ġt akedown +le ted +Ġmat tered +Ġpredict ive +Ġover lay +G PU +ĠV ick +Ġconvey ed +T ab +pe er +Sc an +Ġdefensive ly +v ae +Ġappro ving +Ġt iers +ĠV ia +quer ade +ĠSaud is +Ġdemol ished +ĠProp he +Ġmon o +Ġhospital ity +H AM +ĠAri el +M OD +ĠTor ah +Ġbl ah +ĠBel arus +erent ial +ĠT uc +Ġbank er +39 7 +Ġmosqu it +ĠScient ist +ĠMus ical +Ġh ust +Sh ift +Ġtor ment +Ġstand off +E duc +ĠF og +Ġampl ifier +Sh ape +Inst ance +ĠCrit ics +Ġda emon +H ouston +Ġmatt ress +ĠID F +Ġobsc ene +ĠA mer +hett i +Ġcomp iling +35 2 +vere tt +ĠRed uction +ist ration +ĠBl essed +ĠB achelor +3 16 +Ġpr ank +ĠVul can +dd ing +Ġm ourning +ĠQu int +ĠBl aster +test ing +Ġsed iment +>> > +ĠE ternity +ĠWH ERE +ĠM aze +Ġreact ing +ĠAl v +oms day +ĠC RA +Ġtransl ator +Ġbog us +at u +We bsite +oll s +Ġbapt ism +Ġs ibling +ĠAut umn +ve z +ãģ® é +gu ards +Ge org +assad ors +ĠFre ud +Ġcontin ents +ĠReg istry +Bern ie +ĸļ 士 +Ġtoler ant +ĠU W +Ġhor ribly +99 5 +ĠMID I +Ġimpat ient +oc ado +er i +ĠWor st +ĠNor ris +ĠTalk ing +Ġdef ends +ens able +Ġ20 21 +Ġanat omy +L ew +Ġdraw er +ĠCan berra +Ġpatri otic +é¾įå ĸļ士 +ĠAv g +AR M +Ġundis closed +Ġfare well +45 9 +b able +ĠAll ison +OL OG +Ġcon co +t ight +ĠAC PI +ĠM ines +l ich +ĠâĶ ľ +represent ed +200 000 +Ġenthusi ast +OT S +b il +ĠIng redients +Ġinvent or +ĠMy SQL +³³ Âł +ĠAB OUT +with in +Ġm k +B ul +ĠF ake +Ġdracon ian +W a +hel m +ĠTer ran +erv ille +Ġcommon place +SI ZE +Ġ" < +re place +ograph s +ĠSE LECT +inc ible +ĠMost ly +ĠShe ffield +ĠID E +ugg le +Ġcit ations +h urst +ĠUn ix +Ġunle ash +ĠP iper +ĠN ano +Ġsucc umb +Ġreluct ance +Ġ25 00 +ĠMer chant +Ġwire t +Ġcomb os +ĠBirth day +Ġchar coal +ĠU PS +ĠFair fax +Ġdrive way +ĠT ek +ĠP itch +ove re +Ġtechn icians +ĠAct ual +fl ation +ĠF iscal +ĠEm pty +an amo +Ġmag nesium +Ġsl ut +Ġgrow ers +Invest igators +( ): +ĠS atellite +ĠKe ynes +miss ive +l ane +Ġb orough +3 44 +ĠTE AM +ĠBet hesda +C V +h ower +ĠR AD +Ġch ant +ĠR iy +Ġcompos itions +Ġmild ly +Ġmedd ling +Ġag ility +ane ers +5 01 +Ġsyn th +ling er +29 1 +Ġex claimed +Part y +Ġcont amin +ĠMan or +ĠResp ond +Ġpra ising +Ġman ners +fle et +Sum mer +ĠLy nd +ĠDef initely +gr im +Ġbow ling +st ri +ç Ľ +y nt +Ġmand ates +D IV +Ġreconc ile +view s +ĠDam on +vet te +F lo +ĠGreat est +il on +ic ia +Ġportray al +Ġcush ion +50 4 +19 79 +oss al +App lic +sc ription +Ġmit igation +AT S +p ac +Ġer ased +Ġdefic iencies +ĠHolland e +ĠX u +Ġb red +Ġpregn ancies +f emin +Ġem ph +Ġpl anners +Ġout per +utter ing +Ġperpet rator +Ġm otto +ĠEll ison +ĠNE VER +Ġadmitted ly +AR I +ĠAzerbai jan +Ġmill isec +Ġcombust ion +ĠBott le +ĠL und +ĠP s +ĠD ress +Ġfabric ated +Ġbat tered +Ġs idel +ĠNot ting +Fore ign +ĠJer ome +0 20 +ĠAr bit +Ġkn ots +ĠR IGHT +M oving +ãģ Ļ +Ġsur geries +Ġcour thouse +Ġm astered +Ġhover ing +ĠBr an +ĠAl ison +Ġsaf est +m ilitary +Ġbull ied +Ġbar rage +Read er +ES E +ĠGe ographic +T ools +3 14 +ĠGe ek +ro th +gl ers +ĠF IN +Ï ģ +ĠA ston +al tern +48 8 +Ġveter in +G amer +Ġint el +ren ches +Sh ield +Ġam nesty +ĠB har +Ġp iled +Ġhonor able +ĠInst itutes +Ġso aked +Ġcom a +ĠE FF +34 1 +by tes +ĠG mail +le in +ĠCanad iens +m aterial +I l +Ġinstruct ors +ĠK Y +Ġconce ive +ub b +ĠP ossible +Ġeas ing +ĠChrist ina +Ġcar ic +ĠHD R +R OM +Ġsho vel +de lete +Ġp uff +ĠCh anging +Ġseam lessly +Att ribute +Ġacqu isitions +ak ery +ĠE F +Ġaut istic +ĠT akes +ĠPow der +ĠSt ir +5 10 +ĠBub ble +sett ings +ĠF owler +Ġmust ard +Ġmore over +Ġcopyright ed +ĠLED s +15 00 +æ ī +ĠH IS +en f +Ġcust od +ĠH uck +G i +Ġim g +An swer +C t +j ay +ĠInf rastructure +Ġfeder ally +L oc +Ġmicro bes +Ġover run +dd s +ot ent +adi ator +>>>> >>>> +Ġtorn ado +Ġadj ud +Ġintrig ued +Ġs i +ĠRevel ation +pro gress +Ġburgl ary +ĠSai yan +ĠK athy +Ġser pent +ĠAndre as +Ġcomp el +ess ler +ĠPl astic +ĠAd vent +ĠPos itive +ĠQ t +ĠHind us +reg istered +ular ity +Ġrighteous ness +Ġdemon ic +u itive +ĠB DS +ĠGre gg +c ia +ĠCrus ade +ĠSina i +W ARE ++ ( +Ġme ll +Ġder ail +y ards +A st +Ġnotice ably +ĠO ber +R am +Ġun noticed +Ġse q +av age +T s +Ġ6 40 +Ġconced e +Ġ] ) +F ill +Ġcapt ivity +ĠImprove ment +ĠCrus ader +ara oh +M AP +æ Ĺ +Ġstr ide +al ways +F ly +N it +Ġal gae +ĠCook ing +ĠDo ors +Mal ley +Ġpolic emen +ãģ į +Ġastron aut +access ible +49 5 +ĠR AW +cl iffe +udic rous +Ġdep ended +al ach +Ġvent ures +ra ke +Ġt its +ĠH ou +Ġcond om +ormon al +Ġind ent +Ġupload ing +Foot note +Import ant +Ġ27 1 +Ġmind ful +Ġcont ends +C ra +Ġcal ibr +ĠO ECD +plug in +F at +ĠIS S +ĠDynam ics +ans en +68 6 +' ), +Ġsp rite +Ġhand held +ĠH ipp +=~ =~ +Tr ust +Ġsem antics +ĠBund es +ĠRen o +ĠLiter ature +s ense +G ary +ĠA eg +ĠTr in +EE K +Ġcler ic +ĠSS H +Ġch rist +Ġinv ading +ib u +Ġen um +aur a +Ġal lege +ĠInc redible +B BC +Ġth ru +Ġsa iled +Ġem ulate +Ġin security +Ġc rou +Ġaccommod ations +Ġincompet ent +Ġsl ips +ĠEarth qu +s ama +IL LE +Ġi Phones +as aki +Ġby e +Ġar d +Ġext ras +Ġsl aughtered +Ġcrowd funding +res so +Ġfil ib +ĠER ROR +ĠT LS +e gg +ĠIt al +Ġen list +ĠCatal onia +ĠSc ots +Ġser geant +Ġdiss olve +N H +Ġstand ings +ri que +I Q +Ġbenef iciary +Ġaqu arium +You Tube +ĠPower Shell +Ġbright est +ĠWar rant +S old +Writ ing +Ġbegin nings +ĠRes erved +ĠLatin os +head ing +Ġ4 40 +Ġrooft op +AT ING +Ġ3 90 +VP N +G s +k ernel +turn ed +Ġprefer able +Ġturn overs +ĠH els +S a +ĠShin ji +ve h +ĠMOD ULE +V iol +Ġex iting +Ġj ab +ĠVan illa +Ġac ron +ĠG ap +ber n +A k +ĠMc Gu +Ġend lessly +ĠFar age +ĠNo el +V a +M K +Ġbr ute +ĠK ru +ĠES V +ĠOl ivia +âĢ ł +ĠK af +Ġtrust ing +Ġh ots +3 24 +Ġmal aria +Ġj son +Ġp ounding +ort ment +Count ry +Ġpostp oned +Ġunequ iv +? ), +ĠRo oney +udd ing +ĠLe ap +ur rence +sh apeshifter +ĠH AS +os ate +Ġca vern +Ġconserv atism +ĠB AD +Ġmile age +Ġarrest ing +V aults +Ġmix er +Dem ocratic +ĠB enson +Ġauth ored +8 000 +Ġpro active +ĠSpirit ual +t re +Ġincarcer ated +ĠS ort +Ġpe aked +Ġwield ing +re ciation +×Ļ × +P atch +ĠEm my +Ġex qu +tt o +ĠRat io +ĠP icks +ĠG ry +ph ant +Ġf ret +Ġeth n +Ġarch ived +% - +c ases +ĠBl aze +Ġim b +c v +y ss +im ony +Ġcount down +Ġaw akening +ĠTunis ia +ĠRe fer +ĠM J +Ġun natural +ĠCar negie +iz en +ĠN uggets +he ss +Ġev ils +64 7 +Ġintrodu ctory +l oving +ĠMcM ahon +Ġambig uity +L abel +ĠAlm ighty +Ġcolor ing +ĠCl aus +set ting +N ULL +ĠF avorite +ĠS IG +> ( +ĠSh iva +ĠMay er +Ġstorm ed +ĠCo verage +we apons +igh am +Ġun answered +Ġle ve +Ġc oy +c as +b ags +as ured +Se attle +ĠSant orum +ser ious +Ġcourage ous +ĠS oup +Ġconfisc ated +Ġ// / +Ġuncon ventional +Ġmom s +ĠRohing ya +ĠOrche stra +ĠPot ion +Ġdisc redit +ĠF IL +f ixed +ĠDe er +do i +ĠDim ension +Ġbureaucr ats +et een +Ġaction Group +oh m +Ġb umps +ĠUt ility +Ġsubmar ines +ren heit +re search +ĠShap iro +Ġsket ches +Ġde ceptive +ĠV il +es ame +ĠEss entially +Ġramp age +isk y +Ġmut tered +th ritis +Ġ23 6 +f et +b ars +Ġpup il +ĠTh ou +o S +s ong +Ġfract ured +Ġre vert +pict ure +Ġcrit erion +us her +Ġreperc ussions +ĠV intage +ĠSuper intendent +Offic ers +Ġflag ged +Ġbl ames +Ġin verse +ograp hers +Ġmakes hift +Ġdev oid +Ġfoss ils +ĠArist otle +ĠFund s +Ġde pleted +ĠFl u +ĠY uan +Ġw oes +Ġlip id +Ġsit u +requ isites +Ġfurn ish +ĠSam ar +Ġshame ful +Ġadverse ly +Ġad ept +Ġrem orse +Ġmurder ous +uck les +ĠE SL +Ġ3 14 +s ent +Ġred ef +ĠC ache +ĠP urs +ig ans +Ġ4 60 +Ġpres criptions +Ġf res +F uck +ocr ates +Tw enty +ĠWe ird +ĠT oggle +ĠC alled +itiz ens +Ġp oultry +Ġharvest ing +ãĤ¦ ãĤ¹ +Bott om +Ġcaution ed +t n +39 6 +ĠNik ki +Ġeval uations +Ġharass ing +Ġbind ings +ĠMon etary +Ġhit ters +Ġadvers ary +un ts +Ġset back +Ġenc rypt +ĠC ait +Ġl ows +eng es +ĠN orn +Ġbul bs +Ġbott led +ĠVoy ager +3 17 +Ġsp heres +p olitics +Ġsubt ract +Ġsens ations +Ġapp alling +Ġ3 16 +Ġenvironment ally +ĠST EM +Ġpub lishes +5 60 +Ġdilig ence +48 4 +Ġadv ises +Ġpet rol +Ġimag ining +Ġpatrol s +ĠInt eger +ĠAs hes +act us +ĠRad iant +ĠL T +it ability +ht aking +Set ting +Ġnu anced +ĠRe ef +ĠDevelop ers +N i +pie ces +99 0 +Lic ense +Ġlow ers +ĠOtt oman +3 27 +oo o +Ġqu itting +mark ets +Beh ind +Ġbas in +Ġdoc s +an ie +fl ash +ct l +Ġcivil ized +ĠFuk ushima +"] ," +ĠK S +ĠHonest ly +ar at +Ġconstruct s +ĠL ans +ĠD ire +ĠLI KE +ĠTrou ble +Ġwith holding +ĠOb livion +Ġsan ity +any a +Con st +Ġgro cer +ĠC elsius +Ġrecount ed +ĠW ife +B order +ate red +h appy +Ġspo iler +Ġlog ically +H all +Ġsucceed ing +Ġpoly morph +Ġax es +ĠShot gun +ĠS lim +ĠPrin ciples +ĠL eth +art a +Ġsc or +Sc reenshot +Ġrelax ation +#$ #$ +Ġdeter rent +idd y +Ġpower less +Ġles bians +Ġch ords +ĠEd ited +se lected +Ġseparat ists +000 2 +Ġair space +Ġturn around +Ġc unning +P ATH +P oly +Ġbomb ed +Ġt ion +x s +Ġwith hold +Ġw aged +ĠLiber ties +Fl ag +Ġcomfort ing +45 4 +ĠI ris +are rs +Ġr ag +Ġrel ocated +ĠGu arant +Ġstrateg ically +Ġgam ma +uber ty +ĠLock heed +g res +Ġgr illed +ĠLow e +st ats +ĠR ocks +Ġsens ing +Ġrent ing +ĠGe ological +ا Ø +ot rop +Ġse w +Ġimproper ly +48 6 +Ġâĸ ł +Ġstar ving +ĠB j +Disc ussion +3 28 +ĠCom bo +ĠFix es +N AT +Ġstri ving +th ora +Ġharvest ed +ĠP ing +Ġplay ful +Ġaven ues +Ġoccup ational +Ġw akes +ĠCou rier +Ġdrum mer +ĠBrow ser +ĠH outh +it u +Ġapp arel +p aste +Ġhun ted +ĠSecond ly +l ain +X Y +ĠP IN +ic ons +Ġcock tails +Ġs izable +Ġhurd les +est inal +ĠRecre ation +Ġe co +64 8 +ĠD ied +m int +Ġfinger prints +Ġdis pose +ĠBos nia +ts y +22 00 +Ġins pected +ĠF ou +Ġf uss +Ġamb ush +ĠR ak +Ġmanif ested +Pro secut +Ġsuff ice +ren ces +Ġcompens ated +ĠC yrus +Ġgen us +ĠWolver ine +ĠTrend s +Ġh ikes +ĠSe en +Ġen rol +C old +Ġpol itely +ĠSl av +ĠRu pert +Ġey ewitness +ĠAl to +Ġun comp +Ġposter ior +M ust +ĠHer z +Ġprogress ively +Ġ23 4 +Ġind ifference +ĠCunning ham +Ġacadem ia +Ġse wer +Ġast ounding +ĠA ES +r ather +Ġeld est +Ġclim bs +ĠAdd s +Ġout cry +Ġcont ag +ĠH ouses +Ġpe pt +ĠMel ania +interest ed +ĠU CH +ĠR oots +ĠHub bard +ĠT BD +ĠRoman ian +fil ename +St one +ĠIm pl +Ġchromos ome +C le +d x +Ġscram bled +ĠP t +Ġ24 2 +OP LE +Ġtremend ously +St reet +Ġcra ving +Ġbund led +ĠR G +p ipe +Ġinj uring +Ġarc ane +Part icip +ĠHero ic +st y +Ġto pping +ĠTemp est +rent ices +b h +Ġpar anoia +ĠUnic ode +Ġegreg ious +Ġ\ ' +ĠOsw ald +Ġgra vel +ĠSim psons +Ġbl and +ĠGuant anamo +Writ er +lin ers +ĠD ice +J C +Ġpar ity +Ġs ided +Ġ23 7 +ĠPyr rha +at ters +d k +F ine +comp an +Ġform ulated +ĠId ol +il ers +hem oth +ĠF av +Ġintr usion +Ġcar rots +ĠL ayer +ĠH acker +Ġ ---------------- +Ġmoder ation +é ģ +oc oc +Ġcharacter ize +ĠTe resa +Ġsocio economic +Ġper k +ĠParticip ation +tr aining +ĠPaul o +ph ys +Ġtrust worthy +Ġembod ied +ĠMer ch +c urrency +ĠPrior ity +Ġte asing +Ġabsor bing +Ġunf inished +ĠCompar ison +Ġdis ple +writ ers +Ġprofess ions +ĠPengu in +Ġang rily +ĠL INK +68 8 +ĠCor respond +Ġprev ailed +Ġcart el +l p +as ms +ĠRed emption +ĠIslam ists +effect s +d ose +ĠL atter +ĠHal ifax +Ġv as +ĠTop ics +ĠN amed +advert ising +zz a +IC ES +Ġret arded +ach able +ĠPupp et +ĠItem Level +Ġret ract +Ġident ifiable +A aron +ĠB uster +s ol +hel le +as semb +H ope +r anged +B a +ĠP urch +é Ģ +ĠSir i +Ġarri vals +Ġ19 12 +Ġshort ened +Ġ3 12 +Ġdiscrep ancy +ĠTem perature +ĠWal ton +Ġkind erg +p olit +Ġrem ix +Ġconnect ors +ãĥĺ ãĥ© +ĠKazakh stan +dom inated +Ġsu gars +im ble +ĠPan ic +ĠDem and +ĠCol ony +on en +ĠM ER +7 75 +ur ia +aza ar +ĠDeg ree +P ri +Ġsun shine +Ġ25 1 +Ġpsychedel ic +Ġdigit ally +ĠBra un +Ġsh immer +Ġsh ave +ĠTel esc +ĠAst ral +ĠVenezuel an +ĠO G +Ġc rawling +Int eg +ĠFe ather +Ġunfold ing +Ġappropri ation +Ġè£ı è +ĠMob ility +ĠN ey +- . +b ilt +L IN +ĠT ube +ĠCon versely +Ġkey boards +ĠC ao +Ġover th +Ġla ure +>> \ +ĠV iper +ach a +Off set +ĠR aleigh +ĠJ ae +J ordan +j p +Ġtotal itarian +Connect or +Ġobserv es +ĠSpart an +ĠIm mediately +ĠSc al +C ool +Ġt aps +Ġro ar +P ast +Ġch ars +ĠB ender +ĠShe ldon +Ġpain ter +Ġbe acon +ĠCreat ures +Ġdownt urn +Ġh inder +ĠAnd romeda +à Ľ +cc oli +ĠF itness +et rical +Ġutil izes +Ġsen ate +Ġen semble +Ġche ers +T W +Ġaff luent +k il +ry lic +ord ering +Com puter +Ġgru esome +ost ics +ĠUb isoft +ĠKel ley +Ġw rench +Ġbourgeois ie +IB LE +ĠPrest on +w orn +ar ist +reat ing +Ġst ained +ar ine +Ġsl ime +EN N +Ġche sts +Ġground water +ann ot +ĠTr ay +ĠLoc ke +ĠC TR +Ġd udes +ĠEx ternal +ĠDec oder +Ġpar amed +ĠMed line +80 9 +ĠD inner +rup al +g z +ĠG um +ĠDem o +j ee +Ġd h +ber man +arch s +Ġen qu +ĠEp stein +Ġdevast ation +Ġfriends hips +ĠAr d +Ġ23 1 +ĠRub in +ĠDist ance +Ġsp urred +Ġd ossier +Ġover looking +\\\\\\\\ \\\\\\\\ +Fore st +ĠCom es +\ ", +ĠIran ians +Ġf ixtures +L aughs +Ġcur ry +ĠKing ston +Ġsqu ash +Ġcat alogue +Ġabnormal ities +Ġdigest ive +.... ..... +Ġsubord inate +og ly +Ġ24 9 +M iddle +Ġmass ac +Ġburg ers +Ġdown stairs +Ġ19 31 +39 4 +ĠV G +Ġl asers +ĠS ikh +ĠAlex a +der ived +Ġcycl ist +ãģ® éŃĶ +onel iness +!!!! !!!! +Ġbuff s +leg ate +Ġrap ing +Ġrecomm ending +ro red +Ġmult icultural +un ique +Ġbusiness men +Ġune asy +ĠM AP +Ġdisp ersed +cipl ine +J ess +ĠK erala +å § +Ġabst raction +Sur v +U h +Ġprin ters +ij a +ow der +Ġanalog ous +ĠA SP +af er +Ġunfold ed +Ġlevel ing +Ġbre ached +ĠH earing +Ġn at +Ġtransl ating +crit ical +Ġant agonist +ĠYes terday +Ġfuzz y +w ash +m ere +Ġbe wild +ĠM ae +V irgin +ph rase +Ġsign aled +ĠH IGH +Ġprot ester +Ġgar ner +unk nown +Ġk ay +Ġabduct ed +Ġst alking +am n +Ġdes erving +ĠR iv +ĠJ orge +Ġscratch ing +ĠS aving +ip ing +Ġte ase +Ġmission ary +ĠMor row +T IME +P resent +Ġchem otherapy +tern ess +ĠH omes +ĠP urdue +Ġst aunch +ĠWhit ney +ĠTH ERE +Î ¼ +iat us +ĠErn est +ĠDe ploy +Ġcove ted +F ML +ĠDial ogue +Ġex ited +f ruit +Ġner d +":" "," +Ġv ivo +ru ly +4 60 +ĠAm en +rehens ible +Ġâ ĺ +D IR +Ġad herence +Ġche w +ĠCo ke +ĠSerge i +dig ital +ĠNe ck +g ently +enth al +/ ) +Ġwe ary +Ġgu ise +ĠConc ord +ĠOn ion +at cher +Ġb inge +ĠDirect ive +Ġman ned +ans k +Ġill usions +Ġbillion aires +38 3 +oly n +odynam ic +ĠWhe at +ĠA lic +Ġcol oured +ĠN AFTA +ab o +Ġmac ros +ind ependent +s weet +Ġsp ac +ĠK abul +Ġ Ä +em e +Ġdict ated +Ġsh outs += { +Ġr ipping +ĠSh ay +ĠCr icket +direct ed +Ġanalys ed +ĠWAR RANT +ag ons +ĠBlaz ers +Ġche ered +Ġar ithmetic +ĠTan z +37 3 +ĠFl ags +Ġ29 5 +Ġw itches +ĠIn cluded +ĠG ained +ĠBl ades +G am +ĠSam antha +ĠAtl antis +ĠPr att +Ġspo iled +ĠI B +ĠRam irez +Pro bably +re ro +ĠN g +ĠWar lock +t p +Ġover he +Ġadministr ations +Ġt int +Ġreg iment +Ġpist ols +Ġblank ets +Ġep ist +Ġbowl s +Ġhydra ulic +Ġde an +Ġj ung +Ġasc end +70 5 +ĠSant iago +à ® +Ġun avoid +ĠSh aman +re b +Ġstem ming +99 8 +ĠM G +st icks +esthes ia +ER O +Ġmor bid +ĠGr ill +ĠP oe +any l +Ġdele ting +ĠSurve illance +Ġdirect ives +Ġiter ations +ĠR ox +ĠMil ky +F ather +Ġpat ented +44 7 +Ġprec ursor +Ġm aiden +ĠP hen +ĠVe gan +ĠPat ent +K elly +Redd itor +Ġn ods +Ġvent ilation +ĠSchwar z +Ġw izards +Ġomin ous +ĠHe ads +ĠB G +Ġl umber +ĠSp iel +Ġis Enabled +Ġancest ral +ĠSh ips +Ġwrest ler +ph i +Ġy uan +ĠRebell ion +Ġice berg +Ġmag ically +Ġdivers ion +ar ro +yth m +ĠR iders +ĠRob bie +ĠK ara +ĠMain tenance +ĠHer b +Ġhar ms +p acked +ĠFe instein +Ġmarry ing +Ġbl ending +ĠR ates +Ġ18 80 +Ġwr ink +ĠUn ch +ĠTor ch +desc ribed +Ġhuman oid +ilit ating +ĠCon v +ĠFe ld +IGH TS +Ġwhistlebl ower +ort mund +ets y +arre tt +ĠMon o +ĠI ke +ĠC NBC +ĠW AY +ĠMD MA +ĠIndividual s +Ġsupplement al +Ġpower house +ĠSt ru +F ocus +aph ael +ĠCol leg +att i +Z A +Ġp erenn +ĠSign ature +ĠRod ney +Ġcub es +idd led +ĠD ante +ĠIN V +iling ual +ĠC th +Ġso fa +Ġintimid ate +ĠR oe +ĠDi plom +ĠCount ries +ays on +Ġextrad ition +Ġdis abling +ĠCard iff +Ġmemor andum +ĠTr ace +Ġ?? ? +se ctor +ĠRou hani +ĠY ates +ĠFree ze +Ġbl adder +M otor +ĠProm ise +ant asy +Ġforesee able +ĠC ologne +cont ainer +ĠTre es +ĠG ors +ĠSin clair +Ġbar ring +key e +Ġsl ashed +ĠStat istical +é ĩ +Ġâĸ º +All ows +Ġhum ility +Ġdr illed +ĠF urn +44 3 +Ġse wage +Ġhome page +Ġcour tyard +Ġv ile +Ġsubsid iaries +aj o +direct ory +Ġam mon +V ers +charg es +Ġ} } +ĠCh ains +Ġ24 6 +n ob +Ġper cept +Ġg rit +Ġfisher men +ĠIraq is +ĠDIS TR +ĠF ULL +ĠEval uation +g raph +at ial +Ġcooper ating +Ġmel an +Ġenlight ened +Ġal i +t ailed +Ġsal ute +Ġweak est +ĠBull dogs +U A +ĠAll oy +Ġsem en +oc ene +ĠWilliam son +s pr +, âĢĶ +ĠG F +itt ens +Be at +ĠJ unk +iph ate +ĠFarm ers +ĠBit coins +ig ers +d h +ĠL oyal +p ayer +Ġentert ained +Ġpenn ed +Ġcoup on +Que ue +Ġweaken ing +c arry +Ġunderest imate +Ġshoot out +Ġcharism atic +ĠProced ure +Ġprud ent +in ances +Ġric hes +Ġcort ical +Ġstr ides +Ġd rib +ĠOil ers +5 40 +ĠPer form +ĠBang kok +Ġe uth +S ER +Ġsimpl istic +t ops +camp aign +Q uality +Ġimpover ished +ĠEisen hower +Ġaug ment +ĠH arden +Ġinterven ed +Ġlist ens +ĠK ok +Ġs age +Ġrub bish +ĠD ed +Ġm ull +pe lling +Ġvide ot +Produ ction +D J +m iah +Ġadapt ations +Ġmed ically +Ġboard ed +Ġarrog ance +Ġscra pped +Ġopp ress +FORM ATION +Ġj unction +4 15 +EE EE +S kill +Ġsub du +ĠSug gest +ĠP ett +Ġle tt +ĠMan ip +ĠC af +ĠCooper ation +T her +Ġreg ained +¶ æ +ref lect +Ġth ugs +ĠShel by +Ġdict ates +ĠWe iner +ĠH ale +Ġbatt leground +s child +Ġcond ol +h unt +osit ories +Ġacc uses +Fil ename +Ġsh ri +Ġmotiv ate +Ġreflect ions +N ull +ĠL obby +¥ µ +ĠS ATA +ĠBack up +Ñ ĥ +n in +ĠCor rection +Ġju icy +ut ra +ĠP ric +Ġrest raining +ĠAir bnb +ĠAr rest +Ġappropri ations +Ġsl opes +Ġmans laughter +Ġwork ings +ĠH uss +ĠF rey +Le ave +ĠHarm ony +ĠF eder +Ġ4 30 +Ġt rench +Ġglad ly +Ġbull pen +ĠG au +b ones +Ġgro ove +Ġpre text +ã ħĭ +Ġtransm itter +ĠComp onent +Ġunder age +ĠEm pires +T ile +Ġo y +ĠMar vin +ĠC AS +Ġbl oss +Ġrepl icated +ĠMar iners +Marc us +ĠBl ocks +Ġliber ated +Ġbutter fly +Fe el +Ġfer mentation +Ġyou tube +Ġoff end +ĠTer m +res ist +Ġcess ation +Ġinsurg ency +Ġb ir +ĠRa ise +59 5 +Ġhypothes es +50 2 +Ġpl aque +ocr at +Ġjack ets +ĠHuff Post +am ong +Ġconf er +48 7 +ĠL illy +Ġadapt ing +ĠF ay +Ġsh oved +ve c +Ġref ine +Ġg on +Ġgun men +z ai +ĠShut tle +ĠI zan +Ġ19 13 +Ġple thora +· · +Ġ5 10 +Ġp uberty +Ġ24 1 +ĠWe alth +ĠAl ma +ĠM EM +ĠAd ults +C as +pr ison +R ace +Ġwater proof +Ġathlet icism +Ġcapital ize +ĠJu ice +Ġillum inated +ĠP ascal +Ġirrit ation +ĠWitness es +ad le +ĠAst ro +Ġf ax +ĠEl vis +Prim ary +ĠL ich +ĠEl ves +Ġres iding +Ġst umble +3 19 +ĠP KK +Ġadvers aries +D OS +ĠR itual +Ġsm ear +Ġar son +ident al +Ġsc ant +Ġmon archy +Ġhal ftime +Ġresid ue +Ġind ign +ĠSh aun +ĠEl m +aur i +A ff +W ATCH +ĠLy on +hel ps +36 1 +Ġlobby ist +Ġdimin ishing +Ġout breaks +Ġgo ats +f avorite +ĠN ah +son ian +ĠBo oster +Ġsand box +ĠF are +ĠMalt a +Ġatt Rot +ĠM OR +ld e +Ġnavig ating +T ouch +Ġunt rue +ĠDis aster +Ġl udicrous +Pass word +ĠJ FK +blog spot +4 16 +ĠUN DER +ern al +Ġdelay ing +T OP +Ġimpl ants +ĠAV G +ĠH uge +att r +Ġjournal istic +ĠPe yton +ĠI A +R ap +go al +ĠProgram me +Ġsm ashing +w ives +print ln +ĠPl ague +in us +EE P +Ġcru iser +ĠPar ish +umin ium +Ġoccup ants +ĠJ ihad +m op +Ġp int +Ġhe ct +ĠMe cca +direct or +ĠFund ing +ĠM ixed +Ġst ag +T ier +Ġg ust +Ġbright ly +ors i +Ġup hill +R D +Ġles ions +ĠBund y +liv ious +Ġbi ologist +ĠFac ulty +ĠAuthor ization +Ġ24 4 +All ow +ï ¸ +ĠGi ul +Ġpert inent +ot aur +es se +ĠRo of +Ġunman ned +35 1 +ĠSh ak +ĠO rient +Ġend anger +D ir +Ġrepl en +ed ient +Ġtail or +Ġgad gets +Ġaud ible +âĺ Ĩ +N ice +Ġbomb ard +ĠR ape +Ġdef iance +ĠTW O +ĠFilip ino +Ġunaff ected +erv atives +Ġso ared +ĠBol ton +Ġcomprom ising +ĠBrew ers +R AL +ĠA HL +icy cle +Ġv ampires +Ġdi pped +oy er +ĠX III +Ġsidew ays +ĠW aste +ĠD iss +ĠâĶľ âĶĢâĶĢ +$ . +Ġhabit ats +ĠBe ef +tr uth +tr ained +spl it +R us +And y +ĠB ram +RE P +p id +è£ ħ +ĠMut ant +An im +ĠMar ina +Ġfut ile +hig hest +f requency +Ġepile psy +Ġcop ing +Ġconc ise +Ġtr acing +ĠS UN +pan el +ĠSoph ie +ĠCrow ley +ĠAd olf +ĠShoot er +Ġsh aky +ĠI G +ĠL ies +ĠBar ber +p kg +Ġupt ake +Ġpred atory +UL TS +/ ** +Ġintox icated +ĠWest brook +od der +he ment +Ġbas eman +AP D +st orage +ĠFif ty +ed itor +G EN +UT ION +ir ting +Ġse wing +r ift +Ġag ony +ĠS ands +Ġ25 4 +C ash +Ġl odge +Ġp unt +N atural +ĠIde as +Ġerrone ous +ĠSens or +ĠHann ity +Ġ19 21 +Ġm ould +ĠG on +kay a +Ġanonym ously +ĠK EY +Ġsim ulator +W inter +Ġstream ed +50 7 +? ", +Ġte ased +Ġco efficient +Ġwart ime +ĠTH R +' '. +ĠBank ing +mp ire +Ġf andom +Ġl ia +G a +Ġdown hill +Ġinterpre ting +Ind ividual +N orm +Ġjealous y +bit coin +Ġple asures +ĠToy s +ĠChev rolet +ĠAd visor +IZ E +Ġrecept ions +70 6 +C ro +Ġ26 2 +Ġcit rus +ir u +Review er +ject ed +U ES +an z +19 81 +ĠWork er +Ġcompl ied +ores cent +contin ental +T on +ĠPr ism +ĠShe ep +Ġ28 8 +n ox +ĠV og +O rd +Ġreal ms +te k +Ġirrig ation +Ġbicy cles +Ġelectron ically +p oly +t all +() ); +Ġaest hetics +ĠInteg rated +Expl ore +Ġd unk +47 6 +p ain +ĠJac ques +ĠD mit +Fram es +Ġreun ited +Ġhum id +D ro +P olitical +Ġyouth ful +Ġent ails +Ġmosqu ito +36 3 +spe cies +Ġcoord inating +ĠMay hem +ĠMagn us +M ount +Impro ved +ĠST ATE +ATT LE +Ġflow ed +Ġtack led +Ġfashion ed +Ġre organ +iv ari +f inger +Ġreluct antly +et ting +ĠV and +you ng +ĠGar land +Ġpresum ption +Ġamen ities +ĠPle asant +on ential +ĠO xy +Ġmor als +ĠY ah +Read y +Sim on +En h +D emon +Ġcl ich +Mon itor +ĠD U +Ġwel comes +Ġstand out +Ġdread ful +Ġban anas +Ġball oons +h ooting +bas ic +Ġsuff ix +Ġd uly +can o +Ch ain +at os +Ġgeop olitical +Ġ( & +ĠGem ini +ÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤ ÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤ +Ġacqu itted +L uck +prot ect +10 24 +Ġsc arcity +Ġmind fulness +ec ided +D N +pr ime +ĠPres idents +ĠVID EO +Ġ( âĪĴ +add ock +N OR +ĠP ru +p un +ĠL OL +)) )) +ĠL iqu +ĠS AS +Ġsty ling +Ġpunish ments +Ġnum b +Ġasc ertain +ĠRock ies +f lu +Th umbnail +Ġperpet rated +ĠSem i +Ġdis arm +ĠOld er +ĠEx ception +Ġexponent ially +ĠCommun ities +Ġabol ish +ĠPart ner +pt oms +Ġ7 77 +ĠFo ley +ĠC ases +Ġgre ase +ĠReb irth +G round +Ġ; ) +ĠDoct rine +ik ini +Y e +ĠBl ossom +Ġpers ists +b ill +Ġinf usion +Ġbud dies +9 11 +ĠPat ient +Ġdem os +Ġacquaint ance +ĠP aw +at ari +Ġx ml +Ġfasc ination +ĠSer ve +Ï Ĥ +br anded +Ġa z +Return s +Ġover shadow +Ġro am +Ġspeed y +n umbered +hel ial +Ġdisc iple +Ġass urances +g iven +pect ing +ĠN atalie +çĶ ° +Ġmosquit oes +rote in +Ġnumer ic +Ġindepend ents +Ġtrans itional +Ġreaction ary +ĠMech dragon +do ctor +Ġshort est +Ġsequ ential +ĠB ac +ĠAccount s +ãģ Į +ach y +ract ive +ĠReg iment +Ġbreat htaking +ffic iency +ĠB ates +Ġ3 11 +Ġward robe +ft s +ĠBer k +Sim ply +ĠRivers ide +iver ing +ident ial +lu cent +Ġen riched +ĠCon ver +ĠG iving +ãĥ Ļ +Ġlegal ize +ĠF TC +Ġfre aking +M ix +Ġter restrial +es ian +ci ents +W ing +LO AD +Ġled ge +ĠViol ent +ĠMet all +Ġ30 8 +Ġs outheastern +hett o +M eat +Ġslow down +Ġret reated +Jere my +end as +**** * +er ic +Ġre ins +opp able +ĠHuman ity +ear ances +rig an +C amera +Ġwa ivers +s oc +Ġalter ation +trans form +ĠC emetery +50 6 +Ġindef inite +Ġstim ulating +y g +60 3 +ĠS op +Ġdescript ive +Ph ase +ĠEd mund +Ġpneum onia +vent us +A mb +Ġlabor atories +ĠEx clusive +ug ar +W ere +Ġmalf unction +Ġhomosexual s +Ġ---- --- +un i +Ġturb ines +ĠEqu ity +D u +Ġmind ed +ĠR H +ĠBlack hawks +Ġfe ats +Ġ17 00 +re pl +36 2 +lad en +Ġindisp ensable +ly ss +tt i +Ġre el +Ġdiver ted +Ġlik eness +Ġsubscript ions +Ġfing ert +Ġfil thy +dest ruct +d raft +ĠBernard ino +l aunch +Ġper plex +ĠS UM +car b +Ġswe ater +ĠVent ure +ĠJ ag +ĠCele b +ĠV oters +Ġstead fast +Ġathlet ics +ĠHans on +ĠDr ac +Tr acker +Ġcomm end +ĠPres idency +ĠD ID +in formed +Ġweb page +P retty +Ġforce fully +ãĥĥ ãĤ¯ +Ġrel ocation +Ġsat ire +â ī +ĠSunder land +æ Ħ +V oice +???? ???? +Ġinform ant +Ġbow el +ĠUn iform +Ġ ..." +Ġpur ge +Ġpic nic +ĠU mb +ĠU PDATE +ĠSapp hire +ĠSt all +le arn +Ġobject ively +Ġob liter +Ġlooph ole +Ġjour neys +Ġo mission +Pro s +ĠSid ney +pl oma +Ġspray ed +Ġg uru +Ġtra itor +Ġtim et +Ġsn apping +ĠSe vent +urn al +ĠUk ip +Ġb owed +por al +l iberal +R os +Quest ions +i OS +Ġsummar ize +ST AT +Ġ18 50 +ap est +Ġl ender +ĠVari able +br inging +ĠL ORD +, ) +Ġcollaps es +x iety +ĠN ed +Y D +ĠSch a +Ġantib ody +Ġdis band +y re +ill usion +Ġro ver +s hed +ĠHiro sh +cc i +Ġcal am +ĠMort on +P interest +Ġ19 28 +ĠE uras +ord es +Ġf ences +ĠIn ventory +ĠVal encia +ĠU d +ĠT iff +Ġsqu e +Ġqu otation +Ġtroubles ome +er ker +QU EST +ĠKing doms +s outh +Ġle vy +Pr ince +ĠSt ing +Ġnick named +Ġapp e +Ġphot ographic +Ġcorp us +re ference +ĠT rog +U nt +) =( +ĠLat via +Ġactiv ating +Ġlicense e +Ġdispar ities +ĠNews letter +ãĥĥ ãĥĪ +Ġfree ing +ĠJe ep +ĠPer ception +ins k +Ġsil icone +ĠHay den +Le an +ĠSuz uki +ibr arian +66 8 +Ġsp or +Ġcorrel ations +ag hetti +Ġtu ber +ĠIP CC +il us +ĠV u +Ġwealth iest +ĠCarb uncle +an za +Ġfool ed +ĠZ ur +Ġd addy +ran o +il ian +Ġknock out +f man +requ ired +ĠWik ileaks +ĠD uffy +ON T +Ġins ol +ĠObject s +Ġb ou +ĠNord ic +ĠIns ert +sc an +Ġd ancers +Ġid iots +major ity +ĠNev ille +ĠFree BSD +Ġt art +pan ic +69 0 +Ġcoc oa +Ġsam pled +Ġlook up +Ind ust +Ġinject ions +gen re +Ġa u +Ġroad way +Ġgen itals +K ind +ĠEx aminer +ĠY az +F resh +Ġpar alysis +ĠAl uminum +Ġre ap +ok é +Ġsl oppy +ĠTun nel +pos ium +ner y +en ic +Ġher bal +ĠOut er +ĠBuild er +Ġinc ur +Ġide ologies +Ġback ups +cons uming +ĠDet ect +de ck +ĠKN OW +ĠG ret +ĠM IC +Ġtough ness +ĠEx hibit +Ġh ive +L es +ĠSCH OOL +ĠAt ari +ald e +ĠN ull +and estine +m ouse +Ġbrig ade +48 9 +Ġrev ol +ĠLaw son +ĠW ah +op oly +eb ted +ĠS aunders +Ġ3 13 +ĠW inc +Ġtab oo +ĠHel met +Ġw edge +ch ip +ĠT ina +b g +Ġinf uri +r n +Ġanomal ies +ĠSy nc +ĠEx am +ĠComm it +ĠDi ary +ĠALS O +ĠDe bor +omed ical +Ġcomprehens ion +6 55 +Ġempower ing +Ġ ire +Ġju ices +ĠE TH +ĠBox ing +=" / +Ġfacilit ated +p oke +ĠPars ons +ĠMod er +tra vel +Ġcivil izations +Ġliber tarians +Ġrun e +ĠCl arks +at hed +Ġcampaign ers +ĠDis patch +ĠFah renheit +ĠCap com +-------- -- +Ġl ace +Ġdr aining +Ġl iner +ĠArt ificial +é n +t ask +] ). +ĠGM O +ĠOper ator +ord inary +ĠInf luence +ĠU ps +Ġpot ency +uss en +osp ons +ĠSw im +ĠDead line +Un ity +Ġcul inary +Ġenlight enment +Ġwe arer +Ġmin ed +Ġp ly +Ġinc est +ĠDVD s +W alk +B TC +Tr ade +Ġdev al +ib and +ĠOvers ight +Palest inian +Ġd art +Ġm ul +L R +Ġrem ovable +ĠReal ms +ì Ŀ +Ġmisc ar +ĠV ulkan +68 5 +è re +ĠS ap +Ġmer ging +ĠCar ly +che ster +Ġbr isk +Ġlux urious +ĠGener ator +Ġbit terness +Ġed ible +Ġ24 3 +T G +Ġrect angle +With No +bel ow +J enn +Ġdark est +Ġh itch +Ġdos age +Ġsc aven +ĠK eller +ĠIllust rated +Certain ly +ĠMaver icks +Marg inal +Ġdiarr hea +Ġenorm ously +Ġ9 99 +sh r +qu art +Ġadam ant +ĠM ew +Ġren ovation +Ġcerv ical +ĠPercent age +en ers +ĠKim ber +Ġflo ats +Ġde x +ĠW itcher +ĠSwan sea +d m +Ġsal ty +y ellow +Ġca pe +ĠDr ain +ĠPaul a +ĠTol edo +les i +Mag azine +ĠW ick +ĠM n +ĠA ck +ĠR iding +AS ON +Ġhom ophobic +AR P +Ġwand ered +C PU +ood oo +ĠP ipe +Ġtight ening +ĠBut t +3 18 +Ġdesert ed +S ession +Ġfacilit ating +J ump +Ġemer gencies +OW ER +Ġexhaust ive +ĠAF TER +Ġheart beat +ĠLab el +ack y +ĠCert ified +ilt ration +Z e +ĠU tt +Ġ13 00 +Ġpres ume +ĠDis p +Ġsur ged +Ġdoll s +Col umb +Ġchim pan +ĠR azor +Ġt icks +Ġcouncill or +Ġpilgr image +ĠReb els +ĠQ C +ĠA uction +x ia +ik k +b red +Ġinsert ion +Ġco arse +d B +SE E +ĠZ ap +ĠF oo +Ġcontem por +ĠQuarter ly +ot ions +ĠAl chemist +ĠT rey +ĠDu o +S weet +80 4 +ĠGi ov +Ġfun n +N in +h off +Ġram ifications +Ġ19 22 +ĠExper ts +az es +Ġgar ments +ar ial +ĠN ab +Ġ25 7 +ĠV ed +Ġhum orous +ĠPom pe +Ġn ylon +Ġlur king +ĠSerge y +ĠMatt is +Ġmisogyn y +ĠComp onents +ĠWatch ing +ĠF olk +ract ical +B ush +Ġt aped +Ġgroup ing +Ġbe ads +Ġ20 48 +Ġcon du +quer que +Read ing +Ġgriev ances +Ult ra +Ġend point +H ig +ĠSt atic +ĠScar borough +L ua +ĠMess i +a qu +ĠPsy Net +ĠR udd +Ġa venue +v p +J er +Ġsh ady +ĠRes ist +ĠArt emis +Ġcare less +Ġbro kers +Ġtemper ament +Ġ5 20 +T ags +ĠTurn ing +Ġut tered +Ġp edd +Ġimpro vised +Ġ: ( +Ġtab l +Ġpl ains +16 00 +press ure +ĠEss ence +marg in +friend s +ĠRest oration +Ġpoll ut +ĠPok er +ĠAugust ine +ĠC IS +ĠSE AL +or ama +Ġth wart +se ek +Ġp agan + º +cp u +Ġg arn +Ġass ortment +ĠI LCS +t ower +Recomm ended +Ġun born +ĠRandom Redditor +ĠRandomRedditor WithNo +Ġparaly zed +Ġeru ption +Ġinter sect +ĠSt oke +ĠS co +B ind +å ¾ +ĠP NG +ĠNeg ative +ĠNO AA +Le on +Ġall oy +ĠL ama +ĠD iversity +5 75 +Ġunderest imated +ĠSc or +Ġm ural +Ġb usted +so on +l if +Ġnone x +Ġall ergy +ĠUnder world +ĠR ays +ĠBl asio +Ġh rs +ĠD ir +Ġ3 27 +by ter +Ġrepl acements +Ġactiv ates +ri ved +M H +Ġp ans +ĠH I +Ġlong itudinal +Ġnu isance +al er +Ġsw ell +ĠS igned +s ci +ĠIs les +ĠA GA +Ġdef iant +Ġson ic +oc on +K C +ĠA im +t ie +ah ah +Ġm L +D X +Ġb isc +ĠBill board +ĠSY STEM +NE Y +ga ard +Ġdist ressed +former ly +Al an +Ġche fs +Ġopt ics +ĠC omet +ĠAM C +Ġredes igned +irm ation +Ġsight ings +38 2 +3 11 +ĠW B +Ġcont raction +ĠT OTAL +D ual +Ġstart led +Ġunderstand ably +Ġsung lasses +ETH OD +Ġd ocker +Ġsurf ing +ĠH EL +ĠSl ack +ton es +Ġsh alt +Vis ual +49 8 +Dep artment +c ussion +Ġunrest ricted +Ġt ad +Ġre name +employ ed +Ġeduc ating +Ġgrin ned +bed room +ĠActiv ities +ĠV elvet +ĠSW AT +Ġsh uffle +ig or +Ġsatur ation +F inding +c ream +ic ter +Ġv odka +tr acking +te c +Ġfore ground +iest a +Ġve hement +ĠEC B +ĠT ie +E y +Ġt urtles +ĠRail road +ĠKat z +ĠFram es +Ġmen ace +ĠFell owship +ĠEss ential +ugg ish +Ġdri p +ch witz +ĠKy oto +s b +ĠN ina +Param eter +Ġal arms +ĠCl aud +Ġpione ering +Ġchief ly +ĠSc ream +Col lection +Ġthank fully +ĠRonald o +åŃ IJ +st rip +ĠDisney land +com mercial +See ing +S oul +Ġevac uate +Ġc iv +ĠAs he +Ġdiv ides +ĠD agger +rehens ive +Ġber ries +ĠD F +Ġs ushi +Ġplur ality +W I +Ġdisadvant aged +Ġbatt alion +ob iles +45 1 +Ġcl ing +Ġunden iable +ĠL ounge +Ġha unt +p he +Ġquant ify +Ġdiff ered +Ġ[* ] +ĠV iz +c um +sl ave +Ġvide og +Ġqu ar +Ġbund les +ĠAl onso +t ackle +Ġneur onal +Ġlandsl ide +conf irmed +ĠDep th +Ġrenew ables +B ear +ĠMaced onia +Ġjer seys +Ġb unk +ĠSp awn +ĠControl s +ĠBuch anan +Ġrobot ics +Ġemphas izing +ĠTut orial +h yp +ist on +Ġmonument al +æ ° +ĠCar ry +Ġt bsp +en ance +H ill +art hed +Ġro tten +De an +Ġtw isting +Ġgood will +Ġimm ersion +L iving +Ġbr ushes +ĠC GI +ĠAt k +tr aditional +Ġph antom +ĠSt amina +Ġexpans ions +ĠMar in +Ġembark ed +ĠE g +int estinal +ĠPE OPLE +ĠBo oth +ĠApp alach +Ġreleg ated +V T +M IT +Ġmust er +Ġwithdraw ing +Ġmicrosc ope +ĠG athering +ĠC rescent +ĠArgent ine +ĠDec re +ĠDomin ic +Ġbud s +ant age +ĠI on +Ġwid ened +ONS ORED +ĠGl oves +iann opoulos +raz en +fe el +Ġrepay ment +Ġhind sight +ĠRE ALLY +ĠPist ol +ĠBra h +Ġwat ts +Ġsurv ives +Ġfl urry +iss y +Al ert +ĠUrug uay +Ph oenix +S low +ĠG rave +ĠF ir +Ġmanage able +Ġtar iff +ĠU DP +ĠPist ons +ĠNiger ian +Ġstrike outs +Ġcos metics +whel ming +f ab +c ape +pro xy +Ġre think +Ġover coming +sim ple +Ġw oo +Ġdistract ing +ĠSt anton +ĠTuls a +ĠD ock +65 9 +Ġdisc ord +ĠEm acs +ĠV es +ĠR OB +Ġreass uring +Ġcons ortium +Muslim s +3 21 +Ġprompt s +se i +ĠH itch +imp osed +ĠF ool +Ġindisc rim +wr ong +bu querque +D avis +! ] +Ġtim eless +ĠNE ED +Ġpestic ide +Ġrally ing +ĠCal der +Ġå ¤ +Ġx p +ĠUn le +ĠEx port +lu aj +B uff +) </ +B oot +ĠChrys ler +or ative +M ess +Ġneglig ible +ert odd +ĠMush room +ĠG ale +g c +ĠCos by +ĠR ural +rit ical +B ell +Ġturb ine +00 200000 +Ġlegit imately +ĠAnim ated +T ED +ĠThe odore +c onduct +ĠH ier +Ġcounterfe it +ĠAlger ia +Ġun beat +cont roller +Ġun res +Ġscram bling +ĠFall on +T es +Ġam ber +Ġroy alties +ĠShel ter +ĠL ester +Ġclass ify +Rem ote +Ġun heard +Ġcontrovers ies +Ġenrich ment +ĠYan kee +g amer +Ġpl atinum +Ġec ology +ĠS ark +Ġunt ouched +Ġsuper visors +Ġ" % +Ġf ooth +Ġcomm ons +Ġnarc otics +Ġind ices +ĠP ly +Ġaddition ally +ĠGaw ker +ĠE Q +Pl aying +Ġcave at +ĠAbs olute +oss us +B aby +Ġr ation +Ġres in +Ġcalib ration +ĠNew port +Ġkn ocks +v t +Ġcomp ost +Sc ene +Ġsar cast +Ġkiss es +Ġn s +all i +ĠMar cel +ĠP iet +iat rics +Ġsurround s +ĠRep rodu +ĠPhill ies +Ġuncertain ties +ĠE ur +ĠRom ance +ĠH ath +ĠNeed s +ĠCl oak +Ġcre m +que ue +Ġ3 55 +Ġup front +] ); +Ġrecip roc +Ġ19 27 +Ġ11 00 +ut su +Ġdep ressive +ow ment +F ans +Ġme ch +Ġann ihil +Ġcounter terrorism +ĠFig ures +b old +ĠMo ines +ĠDri vers +Ġmanuscript s +ĠCrypt o +Ġhyp not +redd its +Ġprosec utions +Ġdiver t +CR IP +ĠB ene +ĠRe ggie +Ġtax ing +ĠMor ales +ent ing +t ur +sign ificant +ĠPR OV +Ġstr ands +Ġp ouch +ĠR ookie +» Ĵ +Ġnic er +he my +h w +EC A +Ġintimid ated +Ġstr icter +Ġmicro bial +det ails +Ġv ows +Ġqu ake +hh hh +Ġrein vent +U b +Ġrel inqu +ĠBuff ett +lic ensed +itte red +ĠPic ard +Ġche wing +u cl +organ ic +Ġlocal ized +ĠEconom ist +Ġacqu ainted +Def inition +s ed +Crit ics +Ġc c +45 3 +38 1 +Ġfell ows +Ġcheck points +0 25 +Ġre election +Ġmed iated +ĠK DE +Ġhurd le +Ġtext ing +Per fect +Ġtrust ees +fect ure +Ġd ich +mon ary +Ġdist inctions +Ġ14 00 +Ġus her +Ġparas ites +ĠSh aring +ĠV im +Ġbar becue +ĠMin isters +ere lla +Ġe b +Ġm c +ĠSome how +ĠIn sect +ch anges +b road +ĠBy z +Ġgrap es +66 9 +Ġ= ================ +Ġass imil +Ġhaun ting +Ġfire power +Ġdef amation +em phasis +Ġcomp ose +Ġallerg ies +Ġstr ang +roll ers +b ang +Ġbrew ers +ron gh +ri ot +p oor +c old +S ample +Ġbu oy +0 40 +ĠCourt ney +Ġ26 8 +ĠWed ding +70 2 +Ġobsess ive +Ġbra king +ĠL al +an ical +å ¦ +at en +Con struction +Ġclin ically +iers hip +N ames +ĠDisc uss +ĠRam os +Ġloc ale +ĠAgric ultural +En able +Ġhorse power +ent ure +P ref +C ourt +Ġstaff ing +Ġfut uristic +dri vers +ĠMarket place +æĪ ¦ +Friend s +Ġdam ning +ĠCustom ers +Ġwe eds +ĠM ai +Ġag ile +ĠT att +ic ent +R anked +cro ft +ĠKat y +Ext reme +Ġcar ve +ĠR over +ĠBy ron +37 2 +Ġconduct s +r atch +it ia +ĠPump kin +Sad ly +Rel oaded +P olicy +Ġl ick +pe ak +is ks +ĠCD s +ĠEn cyclopedia +in itial +C os +ĠAware ness +ĠD ram +$$ $$ +Ġr iff +Ġscript ure +run ners +Ġbo iler +ons on +o in +Ġham string +Ġcat aly +ĠArch bishop +ch all +Ġf aux +ok in +local host +ĠN AME +ad obe +S AN +am ate +Ġscram ble +Ġcar c +ĠMan ifest +ĠCed ar +ĠSer gio +l ater +ff er +Ġgrapp ling +ĠDe utsche +agon ists +ĠNew sp +Ġpret ended +arch ment +Ġcur ated +Ġhead phone +ĠUn common +ĠS IGN +A gent +Ġdead lines +Ġhorizont ally +ĠM AT +ĠSum mers +Ġord ained +ĠLast ly +ĠKend all +Ġfr ig +ĠMach ina +ĠWater loo +ĠMex icans +Ġprotect or +Ġgl are +} " +Prem ium +Ġr ift +ĠTelesc ope +Met al +Ġrec apt +Ġ; ; +Ġincl ination +Ġimp oses +ing en +^ { +Ġh aste +Ġd olphins +Ġcomm uters +pl anned +c ong +m x +ĠU pload +Ġext rap +ĠTuc son +ĠExpl oration +efe ated +Ġsl ender +70 3 +ĠB uk +is el +Ġcompet itiveness +ch lor +ĠP ermanent +ĠE verett +ĠSpecial ist +ĠS OL +Ġcy an +ĠEx actly +U F +ĠL IFE +ary l +on et +ĠEmploy ee +aw ed +ĠRat ings +Ġextra vag +ul hu +ĠPl ane +Ġelev ate +ĠCoord inator +ĠWat kins +Ġex cludes +Ġsent ient +Ġep och +Ġall oc +Pre viously +ĠSh y +ĠSlov akia +L OCK +Ġmarked ly +Ġkn ob +Ġadventure rs +ĠBe en +ĠCost s +amm ers +Ġon slaught +ĠSupport ed +ĠT au +ik arp +ĠS overe +ĠHam pton +ãĤ ī +Pre v +ĠW orse +Ġc ottage +ĠH ades +le z +b owl +Ġfrag rance +ĠL ok +EM OTE +ĠPet ro +Ġ19 25 +ĠP end +produ cing +Ġrel ocate +v ati +p ole +Ġsem in +ĠN UM +Ġrock ed +b uff +b ly +Rep ly +ĠH ai +Ġartic ulated +ĠIslam abad +66 5 +ĠClaim s +Des ktop +Ġtrust ee +Ġscript ing +ĠS ob +ĠAs ylum +STD OUT +ĠCl own +ĠD ortmund +ĠDev on +l ite +ĠMar ble +Ġb unker +Ġcre st +Ġarous al +ĠS ears +ĠBudd y +ered ith +ĠP olly +Ġdec ode +ĠV ish +ĠRef lect +an on +Ġrefund s +imm ers +H M +Ġwip ing +Ġpuzz led +Ġmat te +un o +P ierre +) ), +Ġt ainted +Ġsymbol ism +ĠF raz +Ġprotest ors +ethe us +%% %% +W ra +Ġl ax +ad em +atur ation +ãĥ ĵ +ĠTra iler +ĠE NG +ĠBows er +Ġatt m +D ur +80 7 +Ġsid x +Ġc ider +ĠA ffect +Ġw oven +ĠBark er +ben ef +Ġdst g +ĠRy u +> [ +Ġsq or +S audi +Ġis tg +Ġindul ge +pro c +Ġdisg usted +Ġcomp ounded +Ġn em +Ġschool ing +ĠC ure +process ing +S ol +Ġpro verb +it ized +ĠAlv arez +Ġscar f +Ġrect angular +re ve +Ġh ormonal +ĠSt ress +itiz en +Ġ4 25 +girl s +ĠNo ir +ĠR app +Ġmar ches +ch urch +ĠUs es +Ġ40 5 +ĠBer m +Ġord inances +ĠJud gment +Charg es +ĠZ in +Ġdust y +Ġstraw berries +Ġper ce +ĠTh ur +ĠDebor ah +net flix +ĠLam bert +Ġam used +ĠGu ang +Y OU +R GB +ĠC CTV +Ġf iat +r ang +Ġf ederation +ĠM ant +ĠB ust +ĠM are +respect ive +ĠM igration +ĠB IT +59 0 +Ġpatriot ism +Ġout lining +reg ion +ĠJos é +Ġbl asting +ĠEz ra +B s +Ġundermin es +ĠSm ooth +Ġcl ashed +rad io +Ġtransition ing +ĠBucc aneers +ĠOw l +Ġplug s +Ġh iatus +ĠPin ball +Ġm ig +ĠNut r +ĠWolf e +Ġinteg ers +Ġor bits +ĠEd win +ĠDirect X +b ite +Ġbl azing +v r +Ed ge +ĠP ID +ex it +ĠCom ed +ĠPath finder +ĠGu id +ĠSign s +ĠZ er +ĠAg enda +Ġreimburse ment +M esh +i Phone +ĠMar cos +ĠS ites +h ate +en burg +Ġs ockets +p end +Bat man +v ir +ĠSH OW +Ġprovision al +con n +ĠDeath s +AT IVE +Pro file +sy m +J A +Ġnin ja +inst alled +id ates +eb ra +ĠOm aha +Ġse izing +ĠBe asts +Ġsal ts +M ission +Gener ally +ĠTr ilogy +he on +leg ates +Ġd ime +Ġf aire +par able +G raph +Ġtotal ing +Ġdiagram s +ĠYan uk +ple t +ĠMe h +Ġmyth ical +ĠStep hens +aut ical +ochem istry +Ġkil ograms +Ġel bows +anc ock +ĠB CE +ĠPr ague +Ġimpro v +ĠDev in +Ġ" \ +par alle +Ġsuprem acists +ĠB illion +Ġreg imen +inn acle +Ġrequ isite +ang an +ĠBur lington +ain ment +ĠObject ive +oms ky +G V +Ġun ilateral +Ġt c +Ġh ires +ment al +Ġinvol untary +Ġtrans pl +ĠASC II + ¨ +Ev ents +Ġdoub ted +ĠKa plan +ĠCour age +ig on +ĠMan aging +ĠT art +Ġfalse hood +ĠV iolet +Ġair s +Ġfertil izer +Brit ain +Ġaqu atic +ou f +W ords +ĠHart ford +Ġeven ings +ĠV engeance +qu ite +G all +ĠP ret +Ġp df +ĠL M +ĠSo chi +ĠInter cept +9 20 +Ġprofit ability +ĠId le +ĠMac Donald +ĠEst ablishment +um sy +Ġgather ings +ĠN aj +Charl ie +Ġas cent +ĠProt ector +Ġal gebra +Ġbi os +for ums +EL S +Introdu ced +Ġ3 35 +Ġastron omy +Cont ribut +ĠPol ic +Pl atform +Ġcontain ment +w rap +Ġcoron ary +ĠJ elly +man ager +Ġheart breaking +c air +ĠChe ro +c gi +Med ical +ĠAccount ability +! !" +oph ile +Ġpsych otic +ĠRest rict +Ġequ itable +iss ues +Ġ19 05 +ĠN ek +c ised +ĠTr acking +Ġo zone +Ġcook er +ros is +Ġre open +Ġinf inity +ĠPharm aceutical +ens ional +Att empt +ĠR ory +Mar co +Ġawa its +H OW +t reated +Ġbol st +Ġreve red +Ġp ods +opp ers +00 10 +Ġampl itude +ric an +SP ONSORED +Ġtrou sers +Ġhal ves +ĠK aine +ĠCut ler +ĠA UTH +Ġsplend id +Ġprevent ive +ĠDud ley +if acts +umin ati +ĠY in +Ġad mon +ĠV ag +Ġin verted +Ġhast ily +ĠH ague +L yn +Ġled ger +Ġastron omical +get ting +Ġcirc a +ĠC ic +ĠTenn is +Lim ited +Ġd ru +ĠBY U +Ġtrave llers +Ġp ane +ĠInt ro +Ġpatient ly +Ġa iding +Ġlo os +ĠT ough +Ġ29 3 +Ġconsum es +Source File +Ġ"" " +Ġbond ing +Ġtil ted +Ġmenstru al +ĠCel estial +UL AR +Plug in +Ġrisk ing +N az +ĠRiy adh +Ġacc redited +Ġsk irm +é Ľ +Ġexam iner +Ġmess ing +Ġnear ing +ĠC hern +ĠBeck ham +Ġsw apped +Ġgo ose +K ay +Ġlo fty +ĠWal let +Ġ[ ' +Ġap ocalypse +Ġb amboo +ĠSP ACE +ĠEl ena +Ġ30 6 +ac ons +Ġtight ened +Ġadolesc ence +Ġrain y +Ġvandal ism +ĠNew town +Ġcon ject +c akes +Ġche ated +Ġmoder ators +par ams +E FF +Ġdece it +ĠST L +ĠTanz ania +ĠR I +Ġ19 23 +ĠEx ile +the l +Ġthe olog +Ġquir ky +ĠIr vine +Ġneed y +or is +U m +K a +Ġmail box +3 22 +Ġb os +ĠPet ra +K ING +Ġenlarg ed +O ften +Ġbad ass +Ġ3 43 +ĠPl aces +ĠC AD +Ġpr istine +Ġinterven ing +d irection +Ġl az +ĠD SM +Ġproject ing +ĠF unk +ag og +pay ment +n ov +Ġch atter +AR B +Ġexam inations +ĠHouse hold +ĠG us +F ord +4 14 +B oss +Ġmy stic +Ġle aps +ĠB av +ul z +b udget +Foot ball +Ġsubsid ized +Ġfirst hand +Ġcoinc ide +oc ular +Con n +ĠColl abor +Ġfool s +am ura +ah ar +r ists +Ġsw ollen +Ġexp ended +ĠP au +s up +Ġsp ar +Ġkey note +s uff +Ġunequ al +Ġprogress ing +str ings +ĠGamer gate +Dis ney +ĠEle ven +om nia +Ġscript ed +Ġear ners +bro ther +ĠEn abled +æ ³ +Ġlar vae +ĠL OC +m ess +Wil son +ĠTem plate +success fully +Ġparam ount +Ġcamoufl age +Ġbind s +ĠQu iet +ĠSh utterstock +r ush +Ġmasc ot +fort une +ĠCol t +ĠBe yon +hab i +Ġha irc +Ġ26 7 +ĠDe us +Ġtw itch +Ġconcent rating +Ġn ipples +c ible +Ġg ir +N Z +M ath +n ih +Requ ired +Ġp onder +ĠS AN +Ġwedd ings +Ġl oneliness +N ES +ĠMah jong +69 5 +add le +ĠGar ner +ĠC OUR +Br idge +Ġsp ree +ĠCald well +Ġbri bery +Ġ���� ���� +plug ins +Ġr acket +Ġchamp agne +vers ible +V ote +Ġmod ifiers +May or +6 80 +Ġassemb lies +ĠS ultan +ĠN ing +ĠLad ies +Ġsulf ur +Ġor bs +Ġ---- - +____ ___ +ĠJournal ism +Ġes ports +Ġl ush +Ġh ue +Ġspect ral +H onest +ãĥ ı +Ġbus hes +Ġrein forcement +Ġre opened +ĠWhe els +ĠM org +rie ving +Ġaux iliary +Ġj Query +ĠB AT +tes que +Ġver tex +p ure +f rey +ãĤ º +d os +Ġty ph +Ġc ull +Ġe q +Ġdec on +Ġtoss ing +Ġdispar ate +ĠBr igham +print f +led ged +Ġsu nd +Ġco zy +Ġhepat itis +per forming +Ġav al +ĠG G +f uture +Ġpet ertodd +ĠKos ovo +Ġmagn ets +Al ready +ĠEd ison +ĠCe res +ĠRA ID +Ġbrill iance +57 6 +Ġder ives +Ġhypert ension +ĠÎ Ķ +Ġlamb da +Ġfl air +Ġmission aries +Ġrap es +ĠSt arter +ĠMon ths +Ġdef y +Ġseism ic +ĠR aphael +Ġeuro zone +65 6 +z sche +Ġscr atched +Ġb ows +ĠLenn on +ĠGa ia +Ġdri pping +f acts +A le +Ġfrog s +ĠBre ast +ogene ity +ĠProsecut or +Ġampl ified +ĠHod g +ĠF n +Th ousands +ĠNI H +ĠMonitor ing +FT WARE +ĠPri ebus +ĠG rowing +hun ter +Ġdiagn ose +ĠM ald +ĠL R +Ġcrown ed +Ġburst ing +Ġdiss olution +j avascript +Ġuseful ness +ĠExec ution +: ( +ĠIv ory +a ah +Ġpersecut ed +viol ence +ist as +ĠCr ate +Ġimpuls es +ĠSp ani +ed es +Hand le +ĠZ erg +think able +Last ly +Ġspont aneously +Ġinconven ient +Ġdismiss ing +Ġpl otted +Ġeight y +Ġ7 37 +r ish +ĠThor nton +ath am +Ġsit com +V en +Rec ipe +t el +l und +Ġcle ars +ĠSas uke +Ġ25 8 +Ġopt ing +Ġen raged +est hetic +ĠA e +uch s +Pre p +Fl ow +Ġrun off +ĠE ating +ĠG iles +ĠAct ing +res ources +ib aba +Ġr pm +Ġske wed +ĠBl anc +ĠS akuya +Ġhot ter +Ġ19 24 +op ian +ck o +Ġcr umbling +Ġcapt ains +ĠAppropri ations +le aders +dro pping +an uts +Ġrevers ing +ĠP ose +ĠS ek +Sc ot +ĠIde a +c ise +ĠSloven ia +Ġ3 17 +Do ctor +Ġcro cod +ald i +Se a +ĠFar rell +Ġmerc enaries +ĠR NC +ĠGu ess +Ġp acing +M achine +Streamer Bot +ĠChar ity +Ġ29 8 +Ġcann ons +ĠTob y +TPP StreamerBot +ĠPass ion +cf g +Th om +Ġbad ges +ĠBern stein +. âĢĵ +ĠP OP +ĠCon j +Ġinitial ization +Ġbiod iversity +D ub +Ġfeud al +Ġdisclaim er +Ġc row +Ġign ition +ar f +S HA +Ġk Hz +h azard +ĠArt ists +oe uv +67 9 +ĠRud y +N ine +ĠRam adan +å ½ +itt o +Ġadren aline +C ert +Ġsmell ed +Ġimp unity +Ġag endas +ĠRe born +ĠCon cent +ĠSe ems +Ġo mega +ĠDust in +Ġback er +ĠSau ce +ĠBoy le +W IN +Ġsp ins +Ġpa uses +u pt +Ġshred ded +Ġstra pped +ĠCor ruption +Ġscr atches +Ġn i +Ġatt ire +ĠS AF +Factory Reloaded +ĠI PS +Ġ( % +Ġsem inar +f ocus +c ivil +Ġ18 60 +int osh +Ġcontin ual +Ġabbre vi +ĠS ok +oc obo +X M +Ġfr antic +Ġunavoid able +Ġar tery +Ġannot ations +b ath +Cl imate +Ġd ors +ĠSl ide +co ord +ĠRel oad +ĠL DL +ĠLove craft +Ġunim agin +Ġresemb led +Ġbarr acks +n p +Ġsurrog ate +Ġcategor ized +ãĤ © +Ġvacc inated +Ġdrain age +Ġind ist +ĠWhats App +Ġ18 70 +oler ance +inv oke +am orph +Ġrecon nect +Ġem anc +Ġblind ness +Ġ12 80 +intern et +c ollar +Ġalt ru +Ġab yss +ĠT RI +65 7 +Ġinf used +HE AD +Ġforest ry +ĠWood y +ĠC i +w i +s am +78 4 +hol iday +Ġmog ul +ĠF ees +ĠD EN +In ternal +ur bed +f usc +at om +ĠIll usion +Ġpoll ed +Ġfl ap +Ġco ax +L GBT +An aly +ĠSect ions +ĠCalif orn +em n +Ġh ither +ĠN IGHT +Ġn ailed +ĠPip eline +39 1 +o of +ĠPr imal +vere nd +Ġsl ashing +Ġret ri +avi our +Ġdepart ing +g il +IS C +Ġmid way +Ġultras ound +Ġbeh aving +ĠT ara +class es +V irtual +ĠColon ial +Ġstri pping +Ġorchestr ated +ĠGra ves +45 2 +ĠIron ically +ĠWrit ers +Ġl ends +ĠMan z +Ġra ven +Ġoxid ative +Ġ26 6 +EL F +act ually +asc ar +D raft +Ġfavour able +Ġhumili ating +Ġf idelity +ĠH of +ĠX uan +49 6 +Ġlay ered +at is +79 0 +Ġpay check +it on +K ar +ĠVM ware +ĠFar mer +Ġserv ic +gl omer +Ġsl ump +ĠFab ric +ĠD OC +est ing +Ġreass ure +Ġph yl +v olt +it ory +R ules +Ġoxid ation +Ġpri zed +Ġmist ress +ĠDj ango +WAR N +å ij +Ġenc ode +ĠFeed back +Ġstupid ity +I an +ĠYugoslav ia +× ¨ +ac l +UT E +19 77 +Ġqual ifies +Ġpuls es +pret ty +Ġfro ze +Ġs s +Iter ator +Ġur gently +Ġm ailed +ĠCh am +Ġsust aining +Ġbas il +Ġpupp ies +il ant +ĠP LEASE +l ap +ace ous +F ear +ĠMaster y +aut omatic +ĠT AG +Ġant im +ag les +47 3 +fram es +Ġwh ispers +ĠWho ever +Ġbra very +ĠUK IP +ract ions +"" " +Ġt ame +Ġpart ed +every thing +CON T +Ġind ebted +Ġadd r +re k +IR ED +Ġem inent +cl inton +Ġo usted +Ġreview er +Ġmelt down +Ġre arr +ĠY ao +the real +aby te +Ġst umbling +Ġbat ches +Ġ25 9 +Ġcontrace ptive +Ġprost itute +ens is +De cl +ĠSt rikes +M ilitary +ĠO ath +v acc +pp ings +05 2 +Ġpart Name +amp ing +Rep orts +K I +CH R +Ġsubt ly +sw ers +Bl ake +us ual +Ġcontest ants +Ġcart ridges +ĠGRE AT +Ġbl ush +ĠâĢ º +47 2 +Ġreason ed +ãĥ ¤ +paralle led +Ġd yn +ag ate +Ġnight ly +å Ĩ +55 6 +Ġsem antic +ĠAdv oc +Ġ !! +Ġdisag rees +ĠB W +V eh +Ġharm ing +Ġembr aces +Ġstri ves +Ġin land +ĠK ard +Ġhe ats +ĠGin ny +ut an +ern aut +yl ene +ĠE lev +J D +Ġh ars +ĠStar r +Ġsk ysc +Ġcollabor ators +Us ually +Ġrev olutions +ĠSTAT S +Ġdism antle +Ġconfident ly +Ġkin etic +Al i +Ġpercent ile +Ġextract ing +ill ian +est ead +Ġphysic ists +ĠMarsh al +Ġfell owship +Ġd ashed +ĠU R +ĠSi oux +ĠComp act +am ide +P ython +ĠLe igh +ĠPharm ac +ist rates +her ical +Ġf ue +ĠE min +Ġ( { +ĠNeighbor hood +Ġdisrupt ing +ĠD up +Ġg land +ĠSe v +ĠMar ian +arg on +ĠD und +Ġ< !-- +Ġstr and +Ġstadium s +z os +Ġpsych osis +ĠR ack +Ġbrilliant ly +ï¸ ı +Ġsubmer ged +ĠInst it +ĠCh ow +Ġc ages +ĠH ats +ĠU rs +Ġdil uted +us at +ien ne +ĠMembers hip +ĠBur k +Ġ ie +Ġarche type +D rug +ult on +ĠSp ock +ĠMcK ay +ĠDep end +F eatured +S oc +19 78 +ĠB ere +Ġrelent lessly +Ġcripp ling +Ġar thritis +çĶ Ł +ĠTrop ical +ĠBul g +ĠCher yl +Ġadm irable +Ġsub title +Over ride +Ġorig inating +ĠC CP +Ġsw ore +ĠSo le +ĠDis orders +3 29 +Ġprocess ion +Ġref urb +Ġimm ersed +requ ently +Ġskept ics +Ġcer amic +m itter +en stein +b elt +ĠT IT +b idden +Ġf ir +m ist +> ] +Ġwe ave +ĠParad ox +Ġentr usted +ĠBarcl ays +Ġnovel ist +og ie +80 6 +Ġnin ety +Ġdisag reements +@@@@ @@@@ +ĠAus chwitz +c ars +ĠL ET +t ub +arant ine +P OS +Ġback story +Ġcheer ful +ĠR ag +ek a +bi ased +Ġinexper ienced +ak ra +ĠW itt +t an +Ġrap ist +Ġplate au +ch al +ĠInqu is +exp ression +Ġc ipher +Ġsh aving +add en +re ly +( \ +ism a +ĠReg ulatory +CH AR +ily n +N VIDIA +G U +Ġmur m +la us +Christ opher +Ġcontract ual +ĠPro xy +ĠJa ime +ĠMethod ist +Ġstew ards +st a +per ia +Ġphys iology +Ġbump ed +Ġf ructose +Austral ian +ĠMet allic +ĠMas querade +ar b +Ġprom ul +Ġdown fall +Ġbut cher +Ġb our +ĠIN FORMATION +ĠB is +pect s +ad ena +Ġcontempl ating +ar oo +cent ered +ĠPe aks +Us ed +Ġmod em +Ġg enders +Ġ8 000 +37 1 +Ġm aternity +ĠR az +Ġrock ing +Ġhandgun s +ĠD ACA +Aut om +ĠN ile +Ġtum ult +ĠBenef it +ĠAppro ach +works hop +ĠLe aving +G er +inst ead +Ġvibr ations +Ġrep ositories +49 7 +ĠA unt +ĠJ ub +ĠExp edition +Al pha +Ġs ans +Ġoverd ue +Ġoverc rowd +Ġlegisl atures +Ġp aternal +ĠLeon ardo +Ġexp ressive +Ġdistract ions +Ġsil enced +tr ust +Ġb iking +Ġ5 60 +Ġpropri et +Ġimp osition +Ġcon glomer +Ġ= ================================================================ +ĠTe aching +ĠY ose +int ensive +T own +Ġtroll ing +ĠGr ac +ĠAS US +Y o +Ġspecial s +ĠNep h +ĠGod zilla +Dat abase +ĠHe gel +Ġ27 2 +19 76 +ĠGl oria +Ġdis emb +ĠInvestig ations +ĠB ane +ag ements +St range +Ġtre asury +ĠPl ays +Ġundes irable +Ġwid ening +Ġverb ally +Ġinf ancy +Ġcut ter +f ml +Ġ21 00 +prot otype +f ine +Ġdec riminal +Ġdysfunction al +Ġbes ie +ĠErn st +z eb +Ġnort heastern +Ġa ust +por ate +ĠMar lins +Ġsegreg ated +ew orld +ĠMa her +Ġtra verse +Ġmon astery +ur gy +G ear +s and +Com pl +ĠE MP +Ġpl ent +ĠMer cer +Ġ27 6 +TA BLE +Config uration +H undreds +Ġpr ic +Ġcollabor ating +ĠPar amount +ĠCumm ings +Ġ( < +Ġrecord er +Ġfl ats +Ġ4 16 +wh ose +Font Size +ĠOr bit +Y R +Ġwr ists +Ġb akery +) } +ĠB ounty +ĠLanc aster +Ġend ings +acc ording +ĠSal am +e asy +75 5 +ĠBur r +ĠBarn ett +onom ous +Un ion +Ġpreced ence +ĠScholars hip +ĠU X +Ġroll out +Ġbo on +al m +ĠCan ter +æ µ +Ġround ing +Ġcl ad +Ġv ap +ĠF eatured +is ations +Ġ5 40 +pol ice +Ġunsett ling +Ġdr ifting +ĠLum ia +ĠObama Care +ĠF avor +Hy per +ĠRoth schild +ĠMil iband +an aly +ĠJul iet +H u +Ġrec alling +a head +69 6 +Ġunf avorable +Ġd ances +O x +Ġleg ality +Ġ40 3 +rom ancer +Ġinqu ire +ĠM oves +\ "> +ĠVari ant +ĠMess iah +ĠL CS +ĠBah á +75 6 +Ġeyeb row +Ġ ¥ +ĠMc F +ĠFort y +M as +Ġpan icked +Ġtransform ations +q q +Ġrev olves +ring e +ĠA i +ax e +Ġon ward +ĠC FR +ĠB are +log in +Ġliqu ids +Ġde comp +second ary +il an +ĠCon vert +ami ya +Ġprosecut ing +Ġâī ¡ +ĠYork ers +ĠByr ne +sl ow +aw ei +J ean +Ġ26 9 +ĠSky dragon +Ġ é +ĠNicarag ua +ĠHuck abee +ĠHigh ly +Ġamph ib +ĠPast or +ĠL ets +Ġbl urred +Ġvisc eral +ĠC BO +Ġcollabor ated +z ig +Leg al +Ġapart heid +Ġbr id +Ġpres et +ĠD ET +ĠAM A +× Ķ +arch ing +auc uses +build er +Ġpo etic +Ġem ulator +ĠMole cular +Ġhon oring +ise um +Ġtract or +ĠCl uster +ĠCal m +ared evil +Ġsidew alks +Ġviol in +Ġgeneral ized +ĠAle c +Ġemb argo +Ġfast ball +ĠHT TPS +ĠL ack +ĠCh ill +ri ver +C hel +ĠSw arm +ĠLev ine +ro ying +L aunch +Ġkick er +Ġadd itive +ĠDe als +W idget +cont aining +Ġescal ate +ĠOP EN +Ġtwe aked +Ġst ash +Ġsp arks +ĠEs sex +ĠE cc +Ġconv ict +Ġblog ging +I ER +ĠH L +Ġmurd erers +75 9 +ĠH ib +Ġde pl +ĠJ ord +S ac +Ġdis sect +ĠHow e +os her +Ġcustom izable +ĠFran z +Ġat ro +Ä ĩ +Ġ000 4 +Ġout post +R oss +Ġglyph osate +ĠHast ings +ĠBE FORE +Ġsh ove +o pped +ĠSc ala +Ġam ulet +an ian +Ġexacerb ated +Ġe ater +47 1 +UM E +Ġpul p +izont al +ĠZ am +ĠAT I +imm une +aby tes +Ġunnecess arily +ĠC AT +ĠAx is +Ġvisual ize +à ī +ĠRad ical +f m +Doc uments +ĠFor rest +Ġcontext ual +ĠSy mbol +Ġtent ative +ĠDO ES +ĠGood s +Ġintermitt ent +} : +medi ated +Ġridic ule +Ġathe ism +Ġpath ogens +ĠM um +Ġre introdu +Ġ30 7 +i HUD +Ġflash light +Ġsw earing +Ġp engu +B u +Ġrot ated +ĠCr ane +Ġ() ); +Ġfashion able +Ġendors ing +46 3 +) [ +Ġingest ion +Ġcook s +Ġ9 50 +ot omy +ĠIm am +Ġk a +Ġte aser +ĠGhost s +ĠãĤ µ +19 69 +Ï ĥ +ub by +Ġconver ter +zan ne +end e +ĠPre par +ĠNic kel +ĠChim era +h im +ĠTyr ann +ĠSabb ath +ĠNich ols +Ġra pt +ih ar +Ġshe lling +Ġillum inate +Ġdent ist +ut or +ĠInteg ration +Ġwh ims +ĠLiter ary +Be aut +Ġp archment +ag ara +Br and +Ġder og +â̦ ) +ĠNor se +Ġunw itting +Ġc uc +Ġborder line +Ġupset ting +Ġrec ourse +Ġd raped +ĠRad ar +Ġcold er +ĠPep si +im inary +], [ +65 8 +V i +ĠF rem +ĠP es +Ġveter inary +ĠT ED +ĠEp idem +n ova +k id +Ġdev out +o ct +j ad +M oh +ĠP AY +Ġge ometric +Ġ3 23 +Ġcircum ference +ich ick +19 75 +ĠY uri +ĠSh all +ĠH over +un in +S pr +Ġg raft +ĠHapp iness +Ġdisadvant ages +att acks +Ġhub s +ĠStar Craft +é ĸ +Ġgall eries +ĠKor ra +Ġgrocer ies +ĠGors uch +Ġrap ists +Ġfun gi +ĠTyph oon +V ector +ĠEm press +b attle +4 68 +Ġparas ite +ĠBom ber +S G +ex ist +ĠP f +Ġun se +Ġsurge ons +B irth +ĠUn sure +ĠPrint ed +ĠBehavior al +ĠA ster +Pak istan +Ġun ethical +Ġs v +ĠIo T +Ġlay outs +P ain +Ġconst ants +ĠL W +ĠB ake +Ġtow els +Ġdeterior ation +ĠBol ivia +Ġblind ed +ĠW arden +ĠMist ress +Ġon stage +Ġcl ans +ĠB EST +19 60 +Ġant ique +Ġrhet orical +ĠPer cy +ĠRw anda +, . +B ruce +Ġtra umat +ĠParliament ary +Ġfoot note +id ia +ĠLear ned +se eking +gen ic +Ġdim ensional +H ide +èĢ ħ +Ġintrig ue +in se +Ġle ases +Ġapp rentices +w ashing +Ġ19 26 +V ILLE +Ġsw oop +s cl +Ġbed rooms +on ics +ĠCr unch +comp atible +Ġincap ac +ĠYemen i +ash tra +z hou +d anger +Ġmanifest ations +ĠDem ons +AA F +Secret ary +ACT ED +L OD +Ġam y +ra per +eth nic +4 17 +Ġpos itives +Ġ27 3 +ĠRefuge es +Ġus b +ĠV ald +odd y +ĠMahm oud +As ia +Ġskull s +ĠEx odus +ĠComp et +ĠL IC +ĠM ansion +ĠA me +Ġconsolid ate +storm s +ont ent +99 6 +Ġcl en +Ġm ummy +fl at +75 8 +ĠV OL +oter ic +n en +ĠMin ute +S ov +Ġfin er +R h +ly cer +Ġreinforce ments +ĠJohann es +ĠGall agher +Ġgym n +S uddenly +Ġext ortion +k r +i ator +T a +Ġhippocamp us +N PR +ĠComput ing +Ġsquare ly +Ġmod elling +ĠFor ums +ĠL isp +ĠKrish na +Ġ3 24 +Ġr ushes +Ġens ued +Ġcre eping +on te +n ai +il ater +ĠHorn ets +Ġob livious +IN ST +55 9 +Ġjeopard y +Ġdistingu ishing +j ured +Ġbeg s +sim ilar +ph ot +5 30 +ĠPark way +Ġs inks +ĠHearth stone +ib ur +ĠBat on +Av oid +Ġd ancer +Ġmag istrate +ary n +Ġdisturb ances +ĠRom ero +Ġpar aph +Ġmis chief +âĸ ĵ +ĠSh aria +Ġur inary +r oute +iv as +f itted +Ġeject ed +ĠAl buquerque +Ġ4 70 +Ġirrit ated +ĠZ ip +ĠB iol +à į +Ġden ounce +Ġbin aries +ĠVer se +Ġopp os +ĠKend rick +ĠG PL +Ġsp ew +ĠEl ijah +ĠE as +Ġdr ifted +so far +Ġannoy ance +ĠB ET +47 4 +ĠSt rongh +it ates +ĠCogn itive +oph one +ĠIdent ification +ocr ine +connect ion +Ġbox er +ĠAS D +ĠAre as +Y ang +t ch +ull ah +Ġdece ive +Comb at +ep isode +cre te +W itness +Ġcondol ences +ht ar +Ġhe als +Ġbuck ets +ĠLA W +B lu +Ġsl ab +ĠOR DER +oc l +att on +ĠSteven son +ĠG inger +ĠFriend ly +ĠVander bilt +sp irit +ig l +ĠReg arding +ĠPR OG +Ġse aling +start ing +Ġcard inal +ĠV ec +ĠBe ir +Ġmillisec onds +we ak +per se +Ġster ile +ĠCont emporary +ĠPh ant +ĠCl o +Ġout p +Ġex iled +Ġ27 7 +Ġself ie +Ġman ic +Ġn ano +ter ms +Alex ander +Ġres olves +Ġmillenn ia +Ġexpl odes +Ġconst ellation +Ġadul tery +m otion +D OC +Ġbroad casters +Ġkinderg arten +ĠMay weather +ĠE co +ich o +Ġ28 7 +l aun +Ġm ute +Ġdisc reet +Ġpres chool +Ġpre empt +De lete +ĠFre ed +P i +H K +Ġblock er +ĠC umber +Ġw rought +d ating +Ġins urer +Ġquot as +Ġpre ached +Ġev iction +ĠReg ina +ĠP ens +Ġsevent een +ĠN ass +D ick +Ġfold s +Ġd otted +ĠA ad +Un iversal +Ġp izz +ĠG uru +Ġso ils +Ġno vice +ĠNe ander +Ġst ool +Ġdeton ated +ĠPik achu +ĠMass ive +IV ER +ĠAb del +Ġsubdu ed +Ġtall est +Ġprec arious +Ġa y +r ification +ĠOb j +c ale +Ġun question +cul osis +ad as +igr ated +D ays +Ġque ens +ĠGaz ette +ĠCol our +ĠBow man +ĠJ J +ï ve +Ġdomin ates +Stud ent +Ġm u +Ġback log +ĠElect ro +Tr uth +48 3 +Ġcond ensed +r ules +ĠCons piracy +Ġacron ym +hand led +ĠMat te +j ri +ĠImp ossible +l ude +cre ation +Ġwar med +ĠSl ave +Ġmis led +Ġfer ment +ĠK ah +ink i +ke leton +cy l +ĠKar in +Hun ter +Reg ister +ĠSur rey +Ġst ares +ĠW idth +ĠN ay +ĠSk i +Ġblack list +uck et +Ġexp ulsion +im et +Ġret weet +vant age +Fe ature +Ġtro opers +Ġhom ers +9 69 +Ġconting ency +ĠW TC +ĠBrew er +fore ign +W are +S olar +Ġund ue +RE C +ulner able +path ic +ĠBo ise +Ġ3 22 +Ġarous ed +ĠY ing +ä¸ į +uel ess +Ġp as +Ġmor p +Ġfl oral +Ex press +ud ging +k B +ĠGr anted +Ø ¯ +ĠMich a +ĠGoth ic +ĠSPEC IAL +ĠRic ardo +F ran +Ġadminister ing +6 20 +por a +Ġ ® +Ġcomprom ises +Ġb itten +Ac cept +Th irty +Ð ² +Ġmater ially +ĠTer r +ig matic +ch ains +Ġdo ve +stad t +Mar vel +FA ULT +Ġwind shield +Ġ3 36 +ad ier +Ġsw apping +Ġflaw less +ĠPred ator +ĠMiche le +Ġprop ulsion +ĠPsych ic +Ġassign ing +Ġfabric ation +Ġbar ley +l ust +Ġtow ering +Ġalter cation +ĠBent ley +Sp here +Ġtun a +ĠClass es +Fre edom +un er +L ady +v oice +Ġcool est +or r +Ġpal p +$ { +Ġhyster ia +ĠMet atron +p ants +Ġspawn ing +Exper ts +ĠInvest ors +ĠAn archy +Ġshr unk +ĠVict im +Ġ28 9 +Ġec stasy +ĠB inding +58 5 +ĠMel ody +57 8 +ot ally +ĠE tsy +lig a +Ġapplaud ed +Ġswe ating +Ġredist ributed +Ġpop corn +Ġsem inal +f ur +ĠNeuro science +R and +ĠO st +ĠMadd en +ĠIncre asing +ĠDaw kins +ĠSub way +Ġar sen +cons erv +B UR +Ġsp iked +ĠLy ft +ĠImper ium +ĠDrop box +Ġfav oured +Ġencomp asses +gh ost +Ġins pires +Ġbur geoning +ĠY oshi +ĠVert ical +ĠAud itor +Ġint ending +Ġfilib uster +Bl oom +f ac +ĠCav s +ign ing +Ġcowork ers +ĠBarb arian +rem ember +FL AG +Ġaudit ory +ason ry +Col lege +Ġmut ed +gem ony +ob in +ĠPsych o +9 68 +Ġlav ish +Ġhierarch ical +ĠDr one +ou k +Ġcripp led +ĠMax im +Sl ot +Ġqu iz +ĠV id +if ling +Ġarchae ologists +Ġabandon ment +d ial +le on +ĠF as +T ed +Ġr aspberry +Ġmaneu vers +Ġbehavi ours +Ġins ure +Ġrem od +Sw itch +h oe +Ġsp aced +Ġafford ability +ĠF ern +not ation +ĠBal anced +Ġoccup ies +en vironment +Ġneck lace +Ġsed an +F U +ĠBrav o +Ġab users +ĠAn ita +met adata +ĠG ithub +ait o +ĠF aster +ĠWass erman +ĠF lesh +Ġth orn +r arily +ĠMer ry +w ine +Ġpopul ace +ĠL ann +Ġrepair ing +Ġpsy che +Ġmod ulation +aw aru +âĢĭ âĢĭ +ari j +Ġdecor ations +Ġapolog ise +ĠG arg +app ly +Ġgive away +ĠFl an +ĠWy att +U ber +Ġauthor ised +ĠMor al +HAHA HAHA +activ ate +Ġtorped o +ĠF AR +Ġam assed +ĠA ram +ark in +ĠVict ims +st ab +Ġo m +ĠE CO +Ġopio ids +Ġpurpose ly +ĠV est +Ġer g +at an +ĠSur gery +Ġcorrect ing +ĠOrt iz +ĠBe et +Ġrev oke +Ġfre eway +ĠH iggins +F ail +ĠFar ms +ĠAT P +h ound +Ġp oking +ĠCommun ists +mon ster +iment ary +Ġunlock ing +Ġunf it +we ed +en ario +at ical +ĠEnlight enment +ĠN G +ĠComp ensation +de en +ĠWid ow +ĠCind y +ĠAfter wards +Ġ6 000 +ikh ail +ag ically +Ġrat ified +Ġcasual ty +H OME +p sey +f ee +Ġspark ling +Ġd é +Ġconcert ed +C atal +Ġcomp lying +ĠA res +ĠD ent +Sh ut +Ġsk im +ad minist +Ġhost ilities +ĠG ins +Ġ6 08 +Ġm uddy +ĠMc Int +ĠDec ay +5 25 +Ġconspic uous +ĠEx posure +Ġresc ind +Ġwear able +Ġ3 28 +our met +ah s +ĠRob ots +Ġe clips +inst ance +ĠRE PORT +ĠApp l +0 30 +ĠSk ies +01 00 +Ġfall acy +S ocket +ĠRece iver +Ġsol ves +ĠButter fly +ĠSho pping +ĠFI RE +65 4 +Med ic +Ġsing ers +ĠNeed less +'' '' +isher s +ĠD ive +58 8 +Ġselect ively +Ġcl umsy +88 9 +Ġpurch aser +ear ned +ard y +Ġbenef iting +eng lish +Ġyield ing +ĠP our +Ġspin ach +Ġdel ve +ĠC rom +6 10 +Ġexport ing +ĠMA KE +Ġ26 3 +Ġg rop +Ġenv oy +ĠInqu iry +ĠLu igi +d ry +ĠT uring +Thumbnail Image +ĠVar iety +Ġfac et +Ġfl uffy +Ġexcerpt s +Ġsh orth +ĠOl sen +CL UD +Ġrel iant +ĠUN C +T our +Ġbat hing +Comp any +Ġglobal ization +P red +ĠMalf oy +Ġh oc +j am +craft ed +ĠBond s +ĠKiss inger +Eng land +Ġorder ly +cat entry +Ġ26 1 +Ġexch anging +ĠInt ent +ĠAmend ments +D OM +Ġst out +³³³³³³³³ ³³³³³³³³ +ĠAir bus +Ġ27 8 +hy de +P oll +Item ThumbnailImage +Ġlooph oles +ĠPill ar +Ġexpl or +St retch +A part +Ġun married +Lim it +ĠTransform ers +Ġintellect ually +unct ure +18 00 +Ġd arn +B razil +Ġleft over +ber us +f red +Mine craft +3 26 +ĠForm s +Ġproof s +ĠDes igned +Ġindex es +ĠSupp ose +EM S +ĠL oving +ĠBon nie +im ating +OT US +Ġconduct or +Ġbehav ed +ĠF ren +Ġsy nerg +Ġmillenn ium +Ġcater ing +ĠL auder +W r +ĠY iannopoulos +ĠAT F +Ġensl aved +Ġawaken ed +D VD +ĠED ITION +ĠConc ert +ĠChall enger +ĠH aku +umer ic +Ġdep recated +ĠSH AR +4 12 +Ġdy stop +Ġtremb ling +Ġdread ed +ĠSp ac +p adding +Re pl +ĠG arrison +M ini +Ġun paralleled +am ar +URR ENT +w reck +c ertain +t al +ĠC LS +app ings +Ġsens ed +Ġf encing +ĠPas o +ĠDes k +Ġsc off +Ġcontem plate +ĠL iga +l iquid +75 7 +Ġapp rentice +ĠUCH IJ +5 70 +ĠTh ousand +ĠIll um +Ġchampion ed +ãĤ Į +Ġelect ors +Ġ3 98 +ĠH ancock +round ed +ĠJ OHN +Ġuns atisf +Ġqual ifier +ĠGad get +EN E +Ġdead liest +ĠPl ants +Ġ ions +Ġacc ents +Ġtwe aking +Ġsh aved +F REE +ĠCh aser +Again st +9 60 +Ġmeth amphetamine +Ġnormal ized +Ġ$ \ +ĠPre cision +ĠGu am +Ġch oked +ĠX II +ĠCast ing +Tor rent +Ġscal p +ĠJagu ar +w it +Ġsem ic +ix ie +ĠG ould +Ġconf ines +N usra +ĠL on +ĠJ ugg +y cle +ĠCod ec +E gypt +Ġrest rain +ĠAl iens +Ġch oking +ĠD unk +ĠBell a +ab c +Ġsl ang +Ġneuro trans +s av +Ġempower ment +â ĨĴ +Ġclim bers +ĠM im +ĠF ra +ros se +Cap ital +ĠCth ulhu +Inter face +Ġprof icient +ĠIN TO +Ġ3 18 +ront al +5 80 +ĠDes pair +K enn +Ġscrim mage +ĠCo at +as ions +Ġwall paper +ĠJ ol +Ġresurg ence +Ġant iv +ĠB alls +² ¾ +Ġbuff ers +Ġsub system +ĠSt ellar +ĠL ung +A IDS +Ġerad icate +Ġblat antly +Ġbehav es +ĠN un +Ġant ics +ex port +DE V +w b +Ġph p +ĠInteg rity +Ġexplore r +Ġrev olving +auth ored +g ans +Ġbas k +Ġas ynchronous +å į +TH ING +69 8 +G ene +ĠR acer +ĠN ico +iss ued +Ġser mon +p ossibly +Ġsize of +Ġentrepreneur ial +ox in +ĠMin erva +Ġpl atoon +n os +ri ks +A UT +ĠAval anche +ĠDes c +ij 士 +ĠP oc +Ġconf erred +Î » +Ġpat ched +F BI +66 2 +Ġfract ures +Ġdetect s +Ġded icate +Ġconstitu ent +Ġcos mos +W T +Ġswe ats +Ġspr ung +b ara +s olid +Ġuns us +Ġbul ky +ĠPhilipp e +ĠFen rir +Ġtherap ists +ore al +^^ ^^ +Ġtotal ed +Ġboo ze +ĠR PC +Prosecut ors +Ġdis eng +ĠSh ared +Ġmotor cycles +Ġinvent ions +Ġlett uce +ĠMer ge +ĠJ C +Ġspiritual ity +ĠWAR NING +Ġunl ucky +ĠT ess +Ġtong ues +ĠD UI +T umblr +Ġle ans +Ġinv aders +Ġcan opy +ĠHur ricanes +ĠB ret +ĠAP PLIC +id ine +ick le +Reg arding +Ġve ggies +Ġe jac +ju ven +F ish +D EM +ĠD ino +Th row +ĠCheck ing +be ard +( & +Ġj ails +Ġh r +trans fer +iv ating +Ġfle ets +ĠIm ag +ĠMc Donnell +Ġsnipp et +Is a +ĠCh att +ĠSt ain +ĠSet FontSize +ĠO y +ĠMathemat ics +49 4 +Ġelectro ly +ĠG ott +ĠBr as +B OOK +ĠF inger +d ump +Ġmut ants +Ġrent als +Ġinter tw +Ġc reek +ail a +Bro ther +ĠDisc ord +pe e +raw ler +Ġcar p +Ġ27 9 +ãĤ· ãĥ£ +rel ations +Ġcontr asts +Col umn +Ġrec onnaissance +Ġun know +Ġl ooting +Ġregul ates +Ġopt imum +ĠChero kee +ĠA ry +Lat est +Ġroad side +Ġd anced +ĠUnic orn +A cknowled +Ġuncont roll +ĠM US +at io +ch ance +ha ven +VAL UE +Ġfavour ites +Ġceremon ial +b inary +pe ed +wood s +EM P +Ġv ascular +Ġcontempl ated +Ġbar ren +ĠL IST +Y ellow +ospons ors +Ġwhisk y +ĠM amm +ĠDeV os +min imum +H ung +44 2 +P ic +ĠSnap dragon +77 6 +Ġcar ving +Ġund ecided +Ġadvantage ous +Ġpal ms +ĠA Q +Ġst arch +L oop +Ġpadd le +Ġfl aming +ĠHor izons +An imation +bo ost +Ġprob abilities +ĠM ish +Ġex odus +ĠEditor ial +Ġfung us +Ġdissent ing +ĠDel icious +rog ram +ĠD yn +d isk +t om +Ġfab rics +ĠC ove +ĠB ans +Ġsoft en +ĠCON S +Ġin eligible +Ġestim ating +ĠLex ington +pract ice +of i +Ġshe dding +ĠN ope +Ġbreat hed +ĠCorinth ians +y ne +ek i +B ull +Ġatt aching +reens hots +Ġanaly se +ĠK appa +Ġuns ustainable +Ġinter pol +ank y +he mer +Ġprot agonists +Ġform atted +ĠBry ce +ĠAch illes +ĠAb edin +sh ock +Ġb um +b os +qu a +ĠW arn +q t +ĠDi abetes +8 64 +ĠIn visible +Ġvan ish +Ġtrans mitting +Ġmur ky +ĠFe i +Ġawa ited +ĠJur assic +umm ies +Ġmen acing +g all +C ath +B uilt +ild o +ĠV otes +Ġon t +Ġmun itions +ĠFre em +ÃŃ n +Ġdec ency +lo pp +ie ved +ĠG ord +Ġun thinkable +ĠNews week +Ġ3 21 +He at +Ġpresent er +ji ang +Ġpl ank +ĠAval on +Ġben z +ĠR out +Ġslam ming +ĠD ai +ou ter +ĠCook ie +ĠAlic ia +ge y +Ġvan ity +Ġow l +á µ +t ested +ĠAw akens +Ġcan v +Ġblind ly +ĠRid ley +ĠEm ails +Requ ires +ĠSer bian +ograp hed +if rame +eter ia +Ġaltern ating +qu iet +Ġsoc iology +ĠUn lock +ĠCommun ism +Ġo ps +Ġatt ribution +Ġab duction +ĠAb ram +Ġsidel ined +ĠB OOK +Ġref ining +ĠFe eling +ĠOs lo +ĠPru itt +r ack +ang ible +Ġcaut iously +ĠM ARK +eed s +M ouse +ĠStep h +ĠP air +S ab +99 7 +ĠBa al +B ec +Ġcomm a +ĠP all +ĠG ael +Ġmisunder stand +ĠP esh +Order able +Ġdis mal +ĠSh iny +% " +Ġreal istically +Ġpat io +ĠG w +ĠVirt ue +Ġexhaust ing +wh atever +oph ys +y ip +4 18 +Ad just +ĠWa iting +ess on +ĠMaz da +ĠDo zens +Ġstream lined +Ġincompet ence +ĠM eth +Ġeth os +ON ES +Ġincent iv +Ġgr itty +ĠBut cher +Head er +Ġexp onential +Ã Ł +Ġcorrel ate +Ġcons ensual +s ounding +R ing +Orig in +Ġcon clusive +fe et +ac ly +ĠF ernandez +Buy able +Ġd ucks +aunt lets +Ġel ong +Ġ28 6 +Ġsim ul +G as +ĠK irst +Ġprot r +ĠRob o +ĠAo E +op ol +Ġpsych ologically +sp in +ilater ally +ĠCon rad +W ave +44 1 +ĠAd vertisement +ĠHarm on +ĠOri ental +is Special +Ġpresum ptive +Ġw il +ĠK ier +ne a +Ġp pm +Ġhar bour +ĠW ired +comp any +Ġcor oner +atur days +ĠP roud +ĠN EXT +ĠFl ake +val ued +ce iver +Ġfra ught +Ġc asing +Ġrun away +Ġg in +ĠLaure nt +ĠHar lem +ĠCur iosity +qu ished +Ġneuro science +ĠH ulu +Ġborrow er +Ġpetition er +ĠCo oldown +W ARD +Ġinv oking +conf idence +For ward +Ġst s +pop ulation +Delivery Date +Fil m +ĠC ov +quick Ship +quickShip Available +prim ary +isSpecial Orderable +inventory Quantity +channel Availability +BO X +ĠMulti player +ĠJen ner +77 8 +ĠM d +Ġ~ /. +M N +Ġchild ish +Ġantioxid ant +ĠChrom ebook +Ġ27 4 +Ġscreen play +Ġadvent urous +ĠRelations hip +respons ive +ming ton +Ġcorner stone +ĠF ey +F IR +Ġrook ies +ĠF eaturing +Ġorig inate +Ġelectro des +ant es +Ġscript ures +Ġgl ued +Ġdiscont ent +Ġaff licted +lay out +B rave +Ġm osa +ĠQuant ity +ĠH ik +w inner +H ours +Ġent ail +ĠCell s +olog ue +Ġv il +Ġpre acher +Ġdecor ative +d ifferent +Ġprejud ices +ĠSm oking +ĠNotting ham +so Type +Ġrhyth ms +ĠAl ph +bl ast +Ste el +ĠDaniel le +Ġstr ife +Ġrem atch +so DeliveryDate +ĠF ork +t rip +ol ulu +hes es +C G +ĠPOLIT ICO +ost a +ĠDr ift +é¾įå ¥ +é¾įå¥ ij士 +Ġvet ting +ĠJin ping +ĠRec ession +Min or +ĠF raud +enf ranch +Ġconven ed +ĠNA ACP +ĠMill ions +ĠFarm ing +ĠW oo +ĠFl are +rit o +imm igrant +Ġvac ancy +ĠHE AD +ĠV aj +eg al +ĠV igil +Stud y +Ġru ining +Ġr acks +Ġhe ater +ĠRand olph +ĠBr ush +ĠT ir +Ø ¨ +Ġc ov +% ] +Ġrecount s +ĠO PT +ĠM elt +Ġtr uce +Ġcas inos +Ġcrus ade +Ġcarn age +Ġstri pe +ĠK yl +Text ures +Ġ6 98 +Ġpro clamation +Ġgood ies +Ġ........ .. +pro claimed +P olit +Ġtop ical +Ġspecial ize +ĠA min +g m +Ġanch ored +Ġbear ings +s ample +ĠHigh land +ĠAut ism +Ġmerc enary +Ġinterview er +L ER +ĠSom ers +Ġembry o +ĠAss y +Ġ28 1 +ĠEd iting +ĠCh osen +6 60 +Ġp ci +ĠThunder bolt +BI LL +Ġchuck led +jri wal +h of +Ġearth ly +() { +ind ependence +Ġdisp ers +ĠV endor +ĠG areth +Ġp als +P enn +ĠSub mit +ic um +Th u +Ġcl andestine +Ġcann ibal +ĠCl erk +E Stream +gal itarian +âĻ ¥ +g ew +Ġhor rend +ĠL ov +ĠRe action +ocr in +Class ic +Ġecho ing +Ġdiscl osing +ĠIns ight +og un +ĠInc arn +upload s +pp erc +guy en +Ġ19 01 +ĠB ars +68 7 +Ġb ribes +ĠFres no +ur at +ĠRe ese +Ġintr usive +Ġgri pping +ĠBlue print +ĠR asm +un ia +man aged +ĠHeb do +Ġ3 45 +Ġdec oding +Ġpo ets +Ġj aws +ĠF IGHT +am eless +ĠMead ows +ĠHar baugh +Inter view +ĠH osp +ĠB RA +Ġdelet ion +m ob +W alker +ĠMoon light +ĠJ ed +ĠSoph ia +Ġus ur +Ġfortun ately +ĠPut ting +ĠF old +Ġsan itation +Ġpart isans +IS ON +B ow +ĠCON C +ĠRed uced +ĠS utton +Ġtouch screen +Ġembry os +âĢ¢âĢ¢ âĢ¢âĢ¢ +ĠK rug +com bat +ĠPet roleum +Ġam d +ĠCos mos +Ġpresc ribing +Ġconform ity +ours es +Ġplent iful +Ġdis illusion +ĠEc ology +itt al +Ġf anc +Ġassass inated +regn ancy +Ġperenn ial +ĠBul lets +Ġst ale +Ġc ached +ĠJud ith +ĠDise ases +All en +Ġl as +Ġsh ards +ĠSu arez +ĠFriend ship +inter face +ĠSupp orters +add ons +46 2 +ĠIm ran +ĠW im +Ġnew found +ĠM b +An imal +Ġd arling +and e +Ġrh y +ĠTw isted +pos al +yn ski +Var ious +× ľ +ĠK iw +uy omi +Ġwell being +ĠL au +an os +Ġunm ist +Ġmac OS +Ġrest room +ĠOl iv +ĠAir ways +Ġtimet able +9 80 +Ġrad ios +v oy +ias co +Ġcloud y +ĠDraw ing +Any thing +Sy ria +ĠH ert +st aking +Ġun checked +Ġb razen +ĠN RS +69 7 +onom ic +est ablish +Ġl eng +Ġdi agonal +ĠF ior +L air +ĠSt ard +Ġdef icient +jo ining +be am +Ġomn ip +Ġbl ender +Ġsun rise +Mo ore +ĠF ault +ĠCost ume +ĠM ub +Fl ags +an se +Ġpay out +ĠGovern ors +ĠD illon +ĠBan ana +N ar +Ġtra iled +Ġimperial ist +um ann +ats uki +4 35 +ĠRoad s +Ġsl ur +ĠIde ally +Ġt renches +C trl +Ġmir rored +ĠZ el +ĠC rest +Comp at +ĠRoll s +sc rib +ĠTra ils +omet ers +w inter +Ġimm ortality +il ated +Ġcontrad icts +un iversal +ill ions +ĠM ama +opt im +AT URE +Ġge o +et ter +ĠCar lo +4 24 +Ġcanon ical +ĠStrongh old +n ear +Ġperf ume +Ġorche stra +od iac +Ġup he +Ġreign ing +vers ive +Ġc aucuses +ĠD EM +Ġinsult ed +Ġ---- -- +ĠCr ush +Ġroot ing +ĠWra ith +Ġwh ore +Ġto fu +C md +ĠB ree +Ġ$ _ +Ġr ive +ĠAd vertising +Ġw att +ĠH O +Ġpersu asive +ĠParam eters +Ġobserv ational +ĠN CT +ĠMo j +ĠSal on +Ġtr unc +Ġexqu isite +ĠMar a +Ġpo op +ĠAN N +Ex c +ĠWonder ful +ĠT aco +Ġhome owner +ĠSmith sonian +orpor ated +mm mm +Ġlo af +ĠYam ato +ĠInd o +Ġcl inging +á s +Ġimm utable +h ub +Or ange +Ġfingert ips +ĠWood en +ĠK idd +ĠJ PM +ĠDam n +C ow +c odes +48 2 +Ġiniti ating +ĠEl k +ĠCut ting +Ġabsent ee +ĠV ance +ĠLil ith +G UI +Ġobsc ured +Ġdwar ves +ĠCh op +ĠB oko +Val ues +Ġmult imedia +Ġbrew ed +Reg ular +CRIP TION +ĠMort al +Ġa pex +Ġtravel er +Ġbo ils +Ġspray ing +Rep resent +ĠStars hip +4 28 +Ġdisappro val +Ġshadow y +Ġlament ed +ĠRe place +ĠFran ç +67 7 +d or +Ġunst oppable +Ġcoh orts +gy n +ĠClass ics +ĠAm ph +Ġsl uggish +ĠAdd iction +ĠPad res +Ġins cription +Ġin human +min us +ĠJere miah +at ars +Ter ror +ĠT os +ĠSh arma +ast a +c atch +Ġpl umbing +ĠTim bers +Sh ar +H al +ĠO sc +Ġcou pling +hum ans +Ġsp onge +Ġid ols +ĠSp a +ĠAdv ocate +ĠBe ats +lu a +Ġtick ing +Ġload er +ĠG ron +8 10 +Ġstim ulated +Ġside bar +ĠManufact urer +ore And +19 73 +Ġpra ises +ĠFl ores +dis able +ĠElect rical +ra ise +E th +Ġmigr ated +Ġlect urer +K ids +ĠCa vern +Ġk ettle +Ġgly c +ĠMand ela +ĠF ully +å§ « +FIN EST +Ġsquee zing +ĠRy der +amp oo +oreAnd Online +Inst oreAndOnline +Buyable InstoreAndOnline +Ġcommem orate +ĠRamp age +Aust in +ĠSh roud +ĠRu ins +9 15 +ĠK H +Ġwater front +ĠE SC +b aby +ĠC out +ĠEm blem +Ġequival ents +49 2 +Un ique +ĠNiet zsche +brow ser +Ġim itation +ĠWere wolf +ĠKir in +ac as +' ," +Ġà ¾ +Review ed +Ġc unt +Ġvo ic +ĠLen ovo +Ġbond ed +48 1 +Ġinhib itors +Ġendeav ors +ĠHav ana +ĠSt out +ĠJ olly +A ctor +*/ ( +Ġoccur rences +ĠT ens +Incre ased +ĠACT ION +Ġ ãĢĮ +ĠRank ings +ĠB reat +Ġ30 9 +D ou +Ġimpact ing +ĠDuc hess +pre fix +Q B +Ġsummon ing +Ġbest owed +ĠKe pler +ĠPOW ER +c ube +ĠK its +ĠG rip +Ġop ium +Ġrep utable +t oc +ich ael +ĠR ipple +Ġcaf é +ĠZ oom +ĠBur ma +Ġwa ive +Ġst alls +Ġdem eanor +inc erity +Ġfluor ide +ĠSH OULD +Par is +Ġlong ing +Ġpl at +Ġgross ly +Ġbull s +Ġshowc asing +ex pected +ĠG addafi +engine ering +Re peat +ĠK ut +Ġconce ivable +Ġtrim med +osc ope +ĠCand idate +ĠT ears +rol og +Lew is +S UP +Ġroad map +Ġsal iva +Ġtrump et +Jim my +Ġmirac ulous +Ġcolon ization +Ġam put +ĠGN OME +ate ch +D ifferent +ĠE LE +ĠGovern ments +ĠA head +ãħĭ ãħĭ +word press +L IB +ĠIn clude +ĠDor othy +0 45 +ĠColomb ian +Ġle ased +88 4 +Ġde grading +ĠDa isy +i ations +Ġbapt ized +Ġsurn ame +co x +Ġblink ed +ãĥ ¢ +Ġpoll en +Ġder mat +Ġre gex +ĠNich olson +ĠE ater +ç ľ +rad or +Ġnarrow er +Ġhur ricanes +Ġhalluc inations +r idden +ISS ION +ĠFire fly +Ġattain ment +Ġnom inate +Ġav ocado +ĠM eredith +Ġt s +Ġreve rence +Ġe uph +Ġcr ates +ĠT EXT +Ġ4 43 +Ġ3 19 +J SON +iqu ette +Ġshort stop +ic key +Ġpro pelled +Ġap i +ĠTh ieves +77 9 +Ġovers aw +Ġcol i +ĠNic ola +Ġover cl +ik awa +ĠC yr +Ġ38 4 +78 9 +ĠAll ows +10 27 +Det roit +TR Y +set up +ĠSocial ism +Sov iet +s usp +ĠAP R +ĠShut down +Ġal uminium +zb ek +ĠL over +GGGG GGGG +Ġdemocr acies +Ġ19 08 +ĠMer rill +ĠFranco is +gd ala +Ġtraff ickers +ĠT il +ĠGo at +Ġsp ed +ĠRes erv +Ġpro d +55 2 +Ġc ac +ĠUn iv +ĠSch we +Ġsw irling +ĠWild erness +ĠEgg s +Ġsadd ened +Ġarch aic +H yd +Ġexcess ively +B RE +Ġaer ospace +ĠVo ices +Cra ig +Ġign ited +In itially +ĠMc A +Ġhand set +Ġreform ing +Ġfrust rations +ĠDead pool +ĠBel ichick +ract or +ĠRagnar ok +ĠD rupal +ĠApp roximately +19 20 +ĠHub ble +arm or +ĠSar as +ĠJon as +Ġnostalg ic +Ġfeas ibility +Sah aran +Ġorb iting +Ġ9 70 +R u +Ġsh in +ĠInvestig ators +Ġinconsist encies +ĠP AN +B G +Ġgraz ing +Ġdetect ors +ĠStart up +ĠFun ny +ĠNa omi +Consider ing +Ġh og +ut f +ce mic +Ġfort ified +ĠFun ctions +Ġcod ec +nut rition +H at +" ! +micro soft +55 8 +ĠTh in +ĠA CE +Al ias +ĠO PS +p apers +P K +ãĢ İ +Ġimpro bable +N orthern +equ al +Ġlook out +Ġty res +ĠMod ified +ĠK op +Abs olutely +Ġbuild up +sil ver +Ġaud i +Ġgro tesque +ĠSab er +ĠPres byter +ON Y +Ġglac iers +ĠSho als +ĠK ass +ĠH RC +ĠNic ol +ĠL unch +ĠF oss +âĸ Ĵ +AD RA +ĠOne Plus +o ing +ground s +Ġincident al +Ġdatas ets +68 9 +ĠClarks on +Ġassemb ling +ĠCorrect ions +Ġdrink ers +Ġqual ifiers +Ġle ash +Ġunf ounded +ĠH undred +Ġkick off +T i +Ġrecon cil +ĠGr ants +ĠCompl iance +ĠDexter ity +Ġ19 06 +w arn +D allas +Max imum +n ard +av ia +be aut +ens itivity +tr ace +Ġpione ers +ĠF ract +ãĢ ı +Ġpre cept +Ġgloss y +ĠI EEE +Ac ross +Ġ6 80 +S leep +che on +Ġsatir ical +ĠMin otaur +ĠCla ude +Ġr é +ape go +Ġcar rot +ĠSem in +ino a +Ġz o +Ind ependent +Ġdiagn oses +ĠC ue +M AR +Ġrend ition +ĠK ik +Ġpath ology +Ġselect s +Link edIn +Ġass ay +ĠD res +Ġtext ual +post ed +IT AL +ĠM aul +N eal +Ġinter connected +Ġerr atic +ĠVir us +Ġ5 30 +Ġenvironmental ists +ĠP helps +Ġeng agements +ĠIN ST +Ġeconom ical +nox ious +Ġg earing +izz y +Ġfavor ably +ĠMcG ill +T erm +Ġh anged +Ġball park +ĠRe yes +Ġbe ware +ĠP sal +ĠMass acre +q i +Ġin accessible +acly sm +Ġfr ay +ill ac +Ġbitter ly +ĠCert ification +Mich igan +Ġir respective +al ore +Em pty +Ġendorse ments +Ġund et +f g +equ ipped +Ġmerc iless +ĠC ust +Ġimm ature +Ġvou cher +ĠBlack well +Ñ ı +h awk +dis ciplinary +ile e +ĠMak oto +ĠD ude +ãĥĩ ãĤ£ +Y ears +Ġin ver +Ġsh aman +ĠY ong +ip el +ell en +ĠCath y +br ids +Ġs arc +65 1 +N ear +Ġground work +Ġam az +Ġ4 15 +ĠHunting ton +hew s +ĠB ung +Ġarbit rarily +ĠW it +ĠAl berto +Ġdis qualified +best os +46 1 +Ġp c +Ġ28 4 +ro bat +Rob in +Ġh ugs +ĠTrans ition +ĠOcc asionally +Ġ3 26 +ĠWh ilst +ĠLe y +Ġspaces hip +cs v +Ġun successfully +ĠA u +le ck +ĠWing ed +ĠGrizz lies +. � +Ġne arer +ĠSorce ress +ĠInd igo +El se +8 40 +let es +Co ach +Ġup bringing +ĠK es +Ġseparat ist +Ġrac ists +Ġch ained +Ġabst inence +lear ning +Ġrein stated +Ġsymm etry +Ġremind ers +ĠChe vy +Ġm ont +Ġexempl ary +ĠT OR +Z X +Ġqual itative +ĠSt amp +ĠSav annah +ĠRoss i +Ġp aed +Ġdispens aries +ĠWall s +ĠCh ronic +Ġcompliment ary +ĠBeir ut +Ġ+ --- +igs list +Ġcrypt ographic +mas ters +ĠCap itals +Ġmax imal +Ġent ropy +Point s +Ġcombat ants +l ip +ĠGl ob +ĠB MC +ph ase +th ank +HT TP +Ġcomm uter +Ġ\( \ +.. / +ĠReg ener +ĠDO I +ĠActiv ision +Ġsl it +os al +RE M +Ġch ants +Y u +Ke ys +Bre xit +ĠFor ced +Ari zona +Ġsquad ron +IS O +ĠMal one +Ġ3 38 +Ġcontrast ing +Ġt idal +Ġlib el +Ġimpl anted +Ġupro ar +ĠC ater +Ġpropos itions +M anchester +ĠEuro s +it amin +G il +ĠEl ven +ĠSe ek +ĠB ai +Ġredevelop ment +ĠTown s +ĠL ub +! ", +al on +K rist +Ġmeas urable +Ġimagin able +Ġapost les +Y N +7 60 +Ġster oid +Ġspecific ity +ĠL ocated +ĠBeck er +ĠE du +ĠDiet ary +uts ch +ĠMar ilyn +Ġbl ister +ĠM EP +ĠK oz +ĠC MS +y ahoo +ĠCar ney +Ġbo asting +ĠC aleb +By te +read s +ad en +Pro blem +ĠWood ward +S we +S up +ĠK GB +Set up +Ġtac it +Ġret ribution +Ġd ues +ĠM ü +. ? +ä¸ Ń +p ots +Ġcame o +ĠP AL +educ ation +A my +like ly +g ling +Ġconstitution ally +ĠHam m +ĠSpe ak +Ġwid gets +br ate +Ġcra ppy +ĠI ter +Ġanticip ating +ĠB out +P ixel +ĠY ep +ĠLaur ie +Ġh ut +Ġbullet in +ĠSal vation +Ġch ats +ear able +Honest ly +AL TH +onse qu +c ult +isco very +ovy ch +Ġse lves +ĠSat oshi +S ounds +Ġconver gence +ĠRosen berg +19 74 +Ġnas al +Ġfull est +Ġfer ocious +x us +ist e +AM S +Ġlobb ied +Ġso othing +ĠGun n +t oday +0 24 +Ġinspir ational +ĠN BN +p b +g ewater +or ah +all owed +ĠCol iseum +Ġspecial izing +Ġinsane ly +ĠT ape +del ay +Ġt arn +ĠP ound +Ġmel anch +Ġdeploy ments +il and +Ġless en +Ġfur ry +ĠUE FA +Ġblood shed +ĠMe ier +ither ing +Ġhe irs +ĠJ aw +ax ter +ĠPublic ations +Ġal ters +int ention +ĠWinc hester +d etermination +ĠLif etime +th in +Mon ster +7 80 +Ġapprox imation +Ġsuper markets +ĠSecond s +or os +h uge +Ġb ribe +ĠLIM ITED +un ed +Ġmis interpret +ĠIn jury +Ġ3 67 +Ġthreshold s +ĠCarn ival +Ġgastro intestinal +Ġguid eline +Ġde ceived +f eatures +Ġpurported ly +ĠRon nie +ĠNew t +Ġsp acious +as us +Ġsuperhero es +ĠCyn thia +le gged +k amp +ch io +Ġth umbnail +ĠShir ley +ill ation +Ġshe ds +ĠZ y +E PA +Ġdam s +Ġy awn +n ah +ĠPe ggy +ĠE rie +ĠJu ventus +ĠF ountain +r x +don ald +al bum +ĠComp rehensive +Ġc aching +ĠU z +ulner ability +ĠPrinc iple +ĠJ ian +ing ers +cast s +ĠOs iris +ch art +t ile +ĠTiff any +ĠPatt on +ĠWh ip +Ġovers ized +J e +ĠCind erella +ĠB orders +ĠDa esh +M ah +Ġdog ma +Ġcommun ists +v u +Coun cil +Ġfresh water +Ġw ounding +Ġdeb acle +Ġyoung ster +Ġthread ed +ĠB ots +ĠSav ings +ãģ Ĥ +ol ing +oh o +Ġillum ination +M RI +Ġlo osen +tr ump +ag ency +ur ion +Ġmoment arily +ĠCh un +ĠBud apest +ĠAl ley +D isk +Ġaston ished +ĠCon quer +ĠAccount ing +h aving +ĠWe in +ĠAl right +Ġrev olver +Ġdel usion +Ġrelic s +Ġad herent +qu ant +Ġhand made +or io +Ġcomb ating +c oded +Ġquad ru +re th +N ik +ĠTrib al +ĠMyster ious +Ġin hal +ĠWin ning +ĠClass ification +ch anged +Ġun ab +Ġsc orn +icip ated +w l +ond uctor +Ġrein forcing +ĠChild hood +an ova +Ġadventure r +Ġdoctor al +ĠStrateg ies +Ġengulf ed +ĠEnc ounter +Ġl ashes +Crit ical +ric ular +ĠU TF +oci ation +check ing +ĠConsult ing +Run time +per iod +ĠAs gard +Ġdist illed +ĠPas adena +ĠD ying +ĠCOUN TY +Ġgran ite +Ġsm ack +Ġparach ute +ĠS UR +Virgin ia +ĠF urious +78 7 +ĠO kin +Ġcam el +ĠM bps +19 72 +ĠCh ao +ĠC yan +j oice +ef er +ĠW rap +ĠDeb ate +S eg +Ġfore arm +ĠIgn ore +Ġtim estamp +Ġprob ing +ĠNo on +ĠGra il +f en +Ġdorm ant +ĠFirst ly +ĠE ighth +ĠH UN +ĠDes ire +or as +Girl s +ĠDes mond +z ar +am ines +O AD +exec ute +Ġbo obs +ĠAT L +_ ( +Chel sea +Ġmasturb ation +ĠCo C +Ġdestroy er +ĠCh omsky +Ġsc atter +ĠAss ets +79 6 +ĠC argo +Ġrecept ive +ĠSc ope +Ġmarket ers +Ġlaun chers +Ġax le +ĠSE A +se q +ĠM off +f inding +ĠGib bs +Georg ia +extreme ly +N J +Ġlab orers +st als +Ġmed iation +ĠH edge +at own +Ġi od +des pite +v ill +J ane +ex istence +Ġcoinc ided +ĠUt ilities +ĠChe ap +Ġlog istical +Ġcul mination +ĠNic otine +p ak +F older +Ġrod ents +st uff +Ġlaw fully +Ġreper to +io ch +j j +Dial ogue +HH HH +lic tion +Look s +Ġ29 7 +Ġtur rets +ĠAb andon +Ġinc ess +ĠTraff ord +Ġcur led +Ġprefer ring +Ġprivat ization +Ġir resist +ĠP anda +ĠSh ake +ĠMc Gr +ãĥ Ħ +und ers +Ġdiscrim inated +Ġbart ender +I LE +Atl antic +Ġprop ensity +ĠW iz +ĠG im +con ference +Ġrein forces +G h +w agon +Ġe erie +F al +Ġhug ged +rac ist +R IC +F u +Ġf iller +ĠSt ub +Ġeng raved +ĠWrest le +Ġimagin ative +ĠPe er +ĠFact ors +an us +ĠDrac ula +mon itor +Ġrou ters +ib ia +ĠBoo lean +end ale +ĠSl aughter +ĠSh ack +R FC +ĠSpiel berg +S ax +ĠPH OTO +ĠCl over +ĠR ae +Dep ending +ĠMem or +ar am +Ġpier ced +Ġcur tains +v ale +ĠInqu isition +ĠP oke +Ġforecast ing +Ġcompl ains +S ense +ĠHer mes +isc overed +Ġb ible +ĠMor ph +Ġg erm +78 5 +D ON +Ġcon gen +Ġcr ane +ĠD PR +Ġrespect fully +R oom +ĠN aw +ĠDal ai +re ason +ĠAng us +Educ ation +ĠTitan ic +Ë ľ +Ġo val +un ited +Ġthird s +Ġmoist ur +ĠC PC +M iami +Ġtent acles +ĠPol aris +ex c +ex clusive +ĠPra irie +Ġcol ossal +ĠBl end +sur prisingly +ÃŃ s +Ġindo ctr +Ġbas al +ĠMP EG +und o +Spl it +Develop ment +Ġlan tern +19 71 +Ġprov ocation +Ġang uish +ĠB ind +ĠLe ia +duc ers +ipp y +conserv ancy +Ġinitial ize +ĠTw ice +ĠSu k +Ġpred ic +Ġdi ploma +Ġsoc iop +Ing redients +Ġhamm ered +ĠIr ma +Q aida +Ġglim ps +ĠB ian +Ġst acking +Ġf end +gov track +Ġun n +dem ocratic +ig ree +Ġ5 80 +Ġ29 4 +Ġstraw berry +ID ER +Ġcher ished +ĠH ots +Ġinfer red +Ġ8 08 +ĠS ocrates +O regon +ĠR oses +ĠFO IA +Ġins ensitive +Ġ40 8 +Recomm end +ĠSh ine +Ġpain staking +UG E +ĠHell er +ĠEnter prises +I OR +ad j +N RS +L G +Ġalien ated +Ġacknowled gement +ĠA UD +ĠRen eg +Ġvou chers +Ġ9 60 +Ġm oot +ĠDim ensions +Ġc abbage +B right +g at +ĠK lu +Ġlat ent +Ġz e +ĠM eng +Ġdis perse +Ġpand emonium +H Q +Ġvirt uous +ĠLoc ations +ee per +prov ided +Ġse ams +ĠW T +iz o +PR OV +Ġtit anium +Ġrecol lection +Ġcr an +Ġ7 80 +ĠN F +49 1 +64 2 +p acking +59 8 +text ure +Sp ider +fre edom +cipl ed +ĠTAM ADRA +âĻ ¦ +aut hent +ĠW ANT +r ified +Ġr ites +Ġuter us +k iss +Ġâī ¤ +Ġsk illet +Ġdis enfranch +ĠGa al +Comp an +Ġage ing +gu ide +B alt +Ġiter ator +Ġdiscretion ary +t ips +Ġprim ates +ĠTechn ique +ĠPay ments +az el +ĠR OCK +stant ial +0 60 +Ġd mg +ĠJack ets +ĠPlay off +Ġnurs ery +ĠSy mb +art on +Ġannex ation +Color ado +Ġco ils +ĠSh oes +âĦ¢ : +ĠRo z +COM PLE +ĠEve rest +ĠTri umph +J oy +G rid +à ¼ +process or +ĠPros per +ĠSever us +ĠSelect ed +r g +ĠTay yip +St ra +Ġski ing +Ġ? ) +Ġpe g +Tes la +Ġtime frame +Ġmaster mind +ĠN B +scient ific +ĠSh it +gener ic +IN TER +N UM +Ġst roll +ĠEn ix +ĠM MR +ĠE MS +m ovie +Ĥ ª +Ġminim izing +idd ling +Ġilleg itimate +Ġprot otyp +Ġpremature ly +Ġmanual s +obb ies +ĠCass idy +D EC +des ktop +Ġaer os +Ġscreen ings +Ġdeb ilitating +ĠGr ind +nature conservancy +Ġf ades +ter mination +assets adobe +F actor +Ġdefinitive ly +P oké +ap ult +ĠLaf ayette +C orn +ĠCor al +Ġstagn ant +T ue +Ġdissatisf action +G ender +Ġkid neys +ĠG ow +ĠDef eat +ĠAsh ton +Ġcart els +Ġfore closure +ĠExpl ore +stre ngth +ot in +Ġveterin arian +Ġf umble +Ġpar ap +ĠSt rait +r ils +Ġpr ick +ĠBerm uda +ĠAm munition +skin ned +Ġab ound +ĠB raz +Ġshar per +ĠAsc ension +Ġ9 78 +Ġpreview s +Ġcommun ion +ĠX Y +Ġph ony +Ġnewcom er +Ġ3 32 +." ," +Ġredist ribution +Prot ect +ĠSo f +K al +Ġlip stick +w orst +Ġtang led +Ġretrospect ive +int eger +Ġvolunte ering +Ġ19 07 +Ġ -------------------- +ic hen +Ġunve iling +Ġsen seless +Ġfisher ies +\ - +Ġh inges +Ġcalcul us +My th +Ġund efeated +Ġoptim izations +Ġdep ress +Ġbill board +ĠY ad +ĠPy ramid +Is n +I de +Ġleg ion +ĠK ramer +ent anyl +Ġpenet rating +ĠHaw th +ĠPR ODUCT +ĠGer ard +ĠP act +ĠIn cluding +ĠEl ias +ĠEl aine +vis ual +Ġhum ming +Ġcond esc +ĠF asc +ä¸ Ĭ +Ġe galitarian +Ġdev s +ĠD ahl +O ps +D H +ĠB ounce +id ated +ald o +Ġrepublic an +Ġh amb +ĠS ett +ograph ies +CH APTER +Ġtrans sexual +Ġsky rocket +ans wer +Ġmark up +Ø ª +Ġhero ine +Comp are +ĠT av +Be ast +Ġsuccess ors +Ġna ïve +ĠBuck ley +st ress +me at +Ġdownload able +Ġindex ed +Ġsc aff +ĠL ump +ĠHom o +Stud io +In sp +Ġr acked +far ious +ĠPet ty +Ex ternal +Ġ19 09 +W ars +com mit +put ers +Ġun ob +ĠEr r +ĠE G +ĠAl am +ĠSiber ia +ĠAtmosp heric +IS TER +ĠSatan ic +trans lation +ĠL oud +tra umatic +l ique +Ġreson ate +ĠWel ch +Ġspark ing +ĠT OM +t one +Ġout l +Ġhandc uffed +ĠSer ie +8 01 +Ġland marks +ĠRee ves +Ġsoft ened +Ġdazz ling +ĠW anted +month s +Mag ikarp +Ġunt reated +ĠBed ford +M i +ĠDynam o +O re +79 5 +Ġwrong ful +Ġl ured +Ġcort isol +Ġve x +d rawn +ile t +Download ha +ĠF action +Ġlab yrinth +Ġhij acked +w aters +er ick +Ġsuper iors +ĠRow ling +ĠGu inness +Ġt d +99 2 +Ġune arthed +Ġcentr if +Ġsham eless +P od +ĠF ib +Ġ icing +Ġpredict or +Ġ29 2 +fore station +con struct +C and +@ # +Ġag itated +Ġre pr +OV A +Ġkn itting +ĠLim a +Ġf odder +68 4 +ĠPerson a +k l +7 01 +Ġbreak up +á ¸ +Ġapp alled +Ġantidepress ants +ĠSus sex +Har ris +ĠTher mal +ee ee +U pload +Ġg ulf +Ġdoor step +ĠSh ank +L U +ĠM EN +ĠP ond +s orry +Ġmis fortune +n ance +Ġb ona +M ut +Ġde graded +ĠL OG +ĠN ess +an imal +Ġa version +und own +Ġsupplement ed +ĠC ups +Ġ50 4 +Ġdep rive +ĠSpark le +Å Ĥ +ĠMed itation +auth ors +ĠSab an +ĠN aked +air d +ĠMand arin +ĠScript ures +ĠPerson nel +ĠMahar ashtra +Ġ19 03 +ĠP ai +ĠMir age +omb at +Access ory +Ġfrag mented +T ogether +Ġbelie vable +ĠGl adiator +al igned +ĠSl ug +M AT +Ġconvert ible +ĠBour bon +amer on +ĠRe hab +nt ax +Ġpowd ered +pill ar +Ġsm oker +ĠMans on +ĠB F +5 11 +ĠGood ell +ĠD AR +m ud +g art +Ġob edient +ĠTrans mission +ĠDon ation +8 80 +Ġbother ing +Material s +ãĤ ± +dest roy +Ġfore going +Ġanarch ism +ĠK ry +ice ps +Ġl ittered +ĠSch iff +Ġanecd otal +un its +Ġf ian +ĠSt im +ĠS OME +ĠInv aders +Ġbehaviour al +ĠVent ures +Ġsub lime +Ġfru ition +ĠPen alty +Ġcorros ion +¶ ħ +Ġlik ened +Ġbesie ged +ween ey +ĠCre ep +Ġlinem en +mult i +ic ably +ud der +Ġvital ity +Ġshort fall +ĠP ants +ap ist +H idden +ĠDro ps +med ical +Ġpron unciation +ĠN RL +Ġinsight ful +J V +ĠBe ard +ĠCh ou +Ġchar ms +Ġb ins +Ġamb assadors +ĠS aturdays +Ġinhib itor +ĠFr anch +6 01 +', ' +ĠCon or +art ney +ĠX peria +g rave +be es +ĠProtest ants +Ġso aking +ĠM andal +Ġph ased +Ġ6 60 +Ġsc ams +Ġbuzz ing +ĠItal ians +ĠLoren zo +ĠJ A +Ġhes itated +Ġcl iffs +ĠG OT +ingu ishable +Ġk o +Ġinter ruption +Z ip +Lear ning +Ġundersc ores +ĠBl ink +K u +57 9 +ĠAut ob +I RE +Ġwater ing +Ġpast ry +8 20 +Ġvision ary +ĠTempl ar +awa ited +Ġpist on +Ġant id +current ly +Ġp ard +Ġw aging +Ġnob ility +ĠY us +Ġinject ing +f aith +ĠP ASS +å º +Ġret ake +ĠPR OC +Ġcat hedral +b ash +Ġwrest lers +Ġpartner ing +Ġn oses +Ġ3 58 +Trans form +am en +Ġb outs +ĠId eal +ĠConstant in +Ġse p +ĠMon arch +att en +ĠPe oples +mod ified +Ġmor atorium +Ġpen chant +Ġoffensive ly +Ġprox ies +ok ane +ĠTaiwan ese +ĠP oo +ĠH OME +us ional +Ġver bs +ĠO man +vis ory +Ġpersu asion +Ġmult it +Ġsc issors +G ay +ow ay +oph ysical +l us +gn u +Ġap ocalyptic +Ġabsurd ity +Ġplay book +Ġautobi ography +I UM +Ġsne aking +ĠSim ulation +pp s +ell ery +Plan et +Ġright fully +Ġn iece +ĠN EC +ĠIP O +ĠDis closure +lean or +ous y +ST ER +Ġ28 2 +Cru z +Ch all +64 3 +ĠSurv ive +ĠF atal +ĠAm id +ap o +We apons +D EN +7 70 +ĠGreen wald +Ġlin en +al os +Ġpollut ants +ĠPCI e +k at +Ġp aw +ĠK raft +C hem +ĠTermin ator +Ġre incarn +Ġ] [ +ĠSe eds +Ġsilhou ette +ĠSt ores +Ġgro oming +ĠD irection +ĠIs abel +ĠBr idges +ðŁ ij +E ED +ĠM orsi +Ġval ves +ĠRank ed +ĠPh arma +ĠOrgan izations +Ġpenet rated +ĠRod ham +ĠProt oss +Ġove rest +Ġex asper +ĠT J +Ġ 000000 +Ġtrick le +Ġbour bon +WH O +Ġw retched +Ġmicrosc opic +Ġcheck list +Ġad orned +R oyal +Ad minist +ĠRet irement +ĠHig hest +We ather +ile ge +Ġincre ments +ĠC osponsors +Ġmas se +ĠS inn +r f +Ġh ordes +as sembly +75 4 +ĠNat asha +ĠTY PE +ĠGEN ERAL +Ġarr anging +Ġ40 7 +l ator +Ġg lean +Ġdisc redited +Ġclin icians +UN E +Ġachie ves +ĠEm erson +com plex += [ +Ġprincip ally +Ġfra il +p icked +Ġthan king +Ġre cl +ĠL AST +Ġsupp ressing +il ic +Ġantidepress ant +ĠLis bon +Ġth or +Ġsp a +Ġking doms +ĠPear ce +em o +Ġpl ung +Ġdiv est +Ġ ******************************** +b is +osp els +ad r +Sp irit +hall a +P ink +end ez +Ġresurrect ed +esc ape +ĠRosen stein +Ġge ological +Ġnecess ities +Ġcarn iv +ĠE lys +ĠBar ney +Ġ29 6 +dig y +ST ON +D OWN +Ġmil estones +Ġk er +Ġdismant ling +Ġre prim +Ġcross ings +19 45 +Ġpatri archy +Ġblasp hemy +Ġ3 59 +met ry +ĠOb esity +ĠDiff erences +bl ocking +ãĥķ ãĤ¡ +ich ita +ĠSab ha +ph alt +ĠCol o +ual a +effic ients +ĠMed ina +con sole +55 7 +ĠHann ibal +ĠHab it +ĠF ever +Ġthen ce +Ġsyn agogue +Ġessential s +Ġw ink +ĠTr ader +ID A +ĠSp oiler +ĠIceland ic +ĠHay ward +Ġpe ac +Ġmal ice +Ġflash back +Ġth w +Ġlay offs +L iquid +Ġtro oper +Ġh inge +ĠRead ers +Ph ill +ĠB auer +Cre ated +Ġaud its +ac compan +Ġunsus pecting +ier a +6666 6666 +Ġbro ch +Ġapprehend ed +ĠM alk +cer ning +ĠCod ex +O VER +M arsh +ĠD eng +ĠExp ression +Ġdisrespect ful +Ġasc ending +t ests +ĠPlaint iff +ster y +ĠAl ibaba +din and +ĠDem psey +Applic ations +mor al +Ġthrough put +Ġquar rel +Ġm ills +Ġhe mor +ĠC ASE +terror ist +st im +ifest yle +ro zen +CE PT +Ar k +u ci +lect ic +Ġirrit ating +she ets +A y +Ġrede emed +Ġhorn y +ĠTe ach +ĠS ear +dem ocracy +4 65 +ĠRest ore +Ġstand by +ĠP is +iff in +Ġsleep y +Ġextr ater +Ġcompl iments +Fram eworks +Ġinstall s +Ġb anging +sur face +found land +Ġmetaph ysical +Ġ28 3 +oul s +dev ices +Ar gs +ĠSac rifice +ĠMcC orm +es on +Cons ervative +ĠM ikhail +see ing +is ively +ĠRo oms +ĠGener ic +Ġenthusi astically +Ġgri pped +Ġcomed ic +ĠElectric ity +Ġgu errilla +Ġdec oration +ĠPerspect ive +Ġconsult ations +Ġun amb +Ġplag iar +Ġmagic ian +Ġe rection +ĠTour ism +or ied +ro xy +11 00 +T am +Ī è +Î ³ +× ª +ĠPred ators +Nit rome +Ġtelesc opes +project s +Ġun protected +Ġst ocked +ĠEnt reprene +nex pected +Ġwast ewater +V ill +Ġint imately +Ġi Cloud +ĠConst able +Ġspo of +Ġne farious +Ġfin s +Ġcens or +ĠMod es +ĠEs per +ar bon +Ġinter sections +Ġlaud ed +Ġphys i +Ġgener ously +ĠThe Nitrome +ĠTheNitrome Fan +Ġar isen +ĠÙ Ī +Ġg lands +ĠPav ilion +ĠGu pta +Ġuniform ly +Ġr amps +ri et +ĠWH EN +ĠVan essa +Ġrout ed +Ġlim p +ĠC PI +p ter +int uitive +Ġv aping +Ġexperiment ed +ĠOlymp us +ĠAm on +Ġsight ing +Ġinfiltr ate +ĠGentle man +Ġsign ings +ĠMe ow +ĠNav igation +che cks +4 33 +Ġel apsed +ĠBulg arian +esp ie +ĠS OM +d uring +Ġsp ills +anc a +ĠPly mouth +M AL +Ġdomest ically +ĠWater gate +ĠF AM +k illed +ed ited +ĠYour self +Ġsynchron ization +ĠPract ices +ST EP +Ġgen omes +ĠQ R +not ice +Ġloc ating +z in +Ġ3 29 +al cohol +Ġk itten +V o +Ġr inse +Ġgrapp le +ĠSc rew +ĠD ul +A IR +Ġle asing +ĠCaf é +Ġro ses +ĠRes pect +Ġmis lead +Ġperfect ed +Ġnud ity +Ġnon partisan +ĠCons umption +Report ing +Ġnu ances +Ġdeduct ible +ĠSh ots +Ġ3 77 +Ġæ ľ +ano oga +Ben ef +ĠB am +ĠS amp +if ix +Ġgal van +ĠMed als +rad ius +Ġno bles +Ġe aves +igr ate +K T +ĠHar bour +u ers +Ġrisk ed +re q +Ġneuro t +get table +ain a +Rom ney +Ġunder pin +Ġlo ft +ĠSub committee +ĠMong ol +b iz +Ġmanif ests +ass isted +ĠG aga +Ġsy nergy +Ġreligious ly +ĠPre f +ĠG erry +T AG +ĠCho i +4 66 +beh ind +ĠO u +Gold Magikarp +Ġhemor rh +R iver +Ġtend on +Ġinj ure +ĠF iona +Ġp ag +Ġag itation +|| || +ur an +ĠE SA +Ġest eem +Ġdod ging +Ġ4 12 +r ss +Ġce ases +ex cluding +Ġint akes +Ġinsert s +Ġemb old +ĠO ral +up uncture +4 11 +ĠUn ified +ĠDe le +Ġfurn ace +ĠCoy otes +ĠBr ach +L abor +Ġhand shake +Ġbru ises +Gr ade +éĹ ĺ +ĠGram my +ile en +St ates +ĠScandinav ian +ĠKard ash +8 66 +Ġeffort lessly +ĠDI RECT +ĠTH EN +ĠMe i +ert ation +19 68 +Ġgro in +w itch +Requ irements +98 5 +Ġroof s +Ġest ates +ĠH F +Ġha ha +Ġdense ly +ĠO CT +Ġpl astics +Ġincident ally +ĠTr acks +ĠTax es +Ġch anted +Ġforce ful +ĠBie ber +ĠK ahn +K ent +ĠC ot +lic ts +F ed +Ġhide ous +ĠVer d +ĠSynd icate +ĠIl legal +J et +ĠD AV +re asonable +c rew +Ġfundamental ist +Ġtruth ful +ĠJ ing +Ġl il +Ġdown ed +Ġen chanted +ĠPolic ies +ĠMcM aster +ĠH are +ides how +Ġpar ams +en cers +gorith m +Ġallow ances +Ġturb ulent +Ġcomplex ities +ĠK T +Ġ3 37 +ĠGen etic +F UN +D oug +t ick +Ġg igs +ument hal +Ġpatriarch al +Ġcal c +, ... +Ġc out +ĠGu an +Ġpath ological +ĠR ivals +Ġunder rated +Ġflu orescent +ĠJ iu +arna ev +ĠQu an +Ġ4 29 +Ġ ਠ+M ario +Con struct +ĠC itation +ĠR acial +ĠR SA +ĠF idel +Ġ3 95 +Person ally +C ause +à » +rad ical +in en +Ġvehement ly +ĠPap a +Ġintern ship +Ġfl akes +ĠRe ck +Luck ily +B ra +20 20 +rav ings +R N +W onder +Ser iously +Ġre usable +Ġpoll uted +ĠP eng +le igh +ind le +Ġcircuit ry +ĠMad onna +ĠB ART +Res idents +att ribute +Phil adelphia +Cl ub +Ġplan ner +Ġfr antically +Ġfaith fully +ĠTerrit ories +ĠL AT +ĠAnders en +an u +ĠP ARK +ĠS ora +i age +ĠPlay offs +ĠG CC +4 27 +Ġab norm +ĠL ever +Ġdisob edience +As ync +ĠShe a +V ert +Ġsk irts +ĠSaw yer +x p +Ġwors ening +Ġsc apego +ĠAng le +oth al +Ġtro ve +ĠSt y +ĠN guyen +mar ine +ide on +Dep ths +Bl og +ĠIll uminati +Ġtract s +Ġorgan ise +Ġo str +F s +Ġlever aging +ĠD aredevil +as ar +Ġl ang +Ġex termin +urs ions +ĠRom o +ãĤ¤ ãĥĪ +Ġcont ended +Ġencounter ing +ĠTable t +ĠAltern ate +sk ill +Ġswe ets +Ġco hesive +cap acity +Ġrep ud +Ġl izard +ro o +Ġpilgr ims +ĠR uff +ĠInstr ument +ĠLog o +uit ous +E H +Ġsales man +Ġank les +L ed +ĠPat ty +ud os +Own er +Ġdiscrep ancies +k j +M U +Ġuncond itional +Dragon Magazine +i ard +O ak +ĠConvers ation +be er +ĠOs aka +D elta +us ky +Ġsecret ion +Ġpl aza +Ġm ing +Ġde pletion +ĠM ous +ĠI TS +ĠH imal +ĠFle ming +Ġcyt ok +ĠH ick +Ġbat ters +ĠInt ellectual +6 75 +é r +IS ION +ĠQu entin +ĠCh apters +ih adi +Ġco aster +WAY S +ĠL izard +ĠY or +and ering +S kin +ha ust +ab by +Ġportray ing +Ġwield ed +d ash +Ġprop onent +Ġr ipple +Ġgrap hene +Ġfly er +Ġrec urrent +Ġdev ils +Ġwater fall +æĺ ¯ +go o +Text Color +Ġtam pering +IV ES +TR UMP +ĠAb el +ĠS AL +ĠHend ricks +ĠLu cius +b ots +Ġ40 96 +IST ORY +Gu est +ĠN X +in ant +Ben z +ĠLoad ed +ĠCle ver +t reatment +Ġta vern +Ġ3 39 +ĠT NT +ific antly +Tem perature +F el +Ġunder world +ĠJud ges +Ġ< + +Ġst ump +Ġoccup ancy +Ġab er +ĠF inder +) ", +ĠN unes +res et +in et +ect omy +Ġwell ness +ĠP eb +quart ered +and an +Ġneg atives +ĠTh iel +ĠCl ip +ĠL TD +Ġbl ight +Ġreperto ire +K yle +Ġqu er +ĠC es +Ġha pl +98 9 +ĠTh ames +isc opal +Des k +ivari ate +ĠEx cellence +found ation +Ġâ ĩ +X i +Ġmyster iously +esty les +Ġper ish +ĠEng els +ĠDE AD +09 0 +}} } +ĠUn real +Ġrest less +ID ES +orth odox +ĠInter mediate +Ġdin ners +ĠTr out +ĠSe ym +ĠHall s +og ged +Ġtraged ies +Ġdid nt +67 6 +Ġail ments +Ġobserv able +ĠV ide +ad apt +ĠD usk +Ġprofessional ism +ĠPres cott +ĠInd ies +p ox +ĠMe hran +W ide +Ġend emic +ĠPar an +B ird +Ġped als +ĠI U +ĠAdam ant +ĠH urt +Ġcorrel ates +urd en +Ġspons oring +cl imate +ĠUnivers ities +ĠK not +enn es +ĠDam ian +ĠAx el +S port +Ġbar b +ĠS no +sh own +ste en +ud ence +Ġnon violent +Ġhom ophobia +Ġbiom ass +ĠDet ail +Ġsrf N +ĠT une +accompan ied +I ENCE +Al bert +ĠMong o +z x +ĠCer berus +or bit +c ens +Ġsl ay +SH ARE +H Y +Ġb rawl +ĠPro be +Ġnonex istent +ĠClare nce +ĠBlack burn +Ġport als +ĠR ita +ĠRem ain +ĠLe vant +Ġtrick ed +ĠF erry +aver ing +ĠStraw berry +ĠAn swers +Ġhorrend ous +ĠA man +Supp lement +ĠT oad +Ġpe eled +Ġman oeuv +ĠU zbek +mond s +ĠH ector +Ġ40 2 +pe es +fix es +Ġd j +Ġres umes +Ġaccount ant +Ġadvers ity +Ġham pered +ĠL arson +Ġd oping +part s +H ur +Ġbe arded +Ġy r +ĠPlug in +å¥ ³ +Ġ/ ** +rol ley +Ġwaters hed +ĠSub mission +if lower +AS C +Ġcho ir +Ġsculpt ures +m A +incre asing +ai i +Ġsne akers +Ġconfront s +ĠEle phant +ĠEl ixir +Ġrec al +ĠT TL +w idget +ĠW ax +ĠGr ayson +Ġha irst +Ġhumili ated +ĠWAR N +app iness +ĠT TC +F uel +Ġpol io +Ġcomplex es +Ġbab e +ĠX IV +P F +). [ +P arts +Ġ4 35 +M eg +ĠY ards +ĠAL P +Ġy ells +Ġprin ces +Ġbull ies +ĠCapital ism +ex empt +FA Q +ĠSp onge +ĠAl a +Ġpleas antly +Ġbu f +Ġden ote +Ġunp ublished +Ġkne eling +asc a +Ġl apse +al ien +99 4 +Ġrefere es +ĠLaw yers +S anta +Ġpuzz ling +ĠProm etheus +ĠPh araoh +ĠDel ay +Ġfacilit ates +ĠC ES +Ġjew els +Ġbook let +ond ing +Ġpolar ization +ĠMor an +ĠSal ad +ĠS OS +ĠAdv ice +PH OTOS +IC AN +iat ures +ex press +ĠWonder land +ĠC ODE +ĠCL ASS +9 75 +Ġg rep +ĠD iesel +ĠGl ac +! ?" +Ġr m +o ine +disc rimination +ĠN urse +m allow +Ġv ortex +ĠCons ortium +Ġlarge Download +stra ight +augh lin +G rad +Ġpublic ized +ĠW aves +ĠRed d +Ġfest ivities +ĠM ane +ar ov +Ġfleet ing +ĠDr unk +ug en +C ele +Ġchromos omes +ĠD OT +-+-+ -+-+ +Ġbus iest +ĠBe aver +Sy rian +ĠK yr +k as +ĠCross Ref +19 50 +76 01 +Ġrepe aling +ĠWin ners +ĠMac ro +ĠD OD +bl ance +S ort +64 1 +Ġmet re +ĠD irk +Ġgo ggles +Ġdraw backs +Ġcomplain ant +Ġauthor izing +Ġantit rust +oper ated +Ġm ah +Ġexagger ation +Am azing +ĠSer aph +Ġha ze +w ow +Ġextingu ished +Ġcan yon +ĠB osh +Ġv ents +Ġsc rape +Cor rect +4 26 +Ġav g +Dem and +ĠâĪ ¼ +Ġmicrobi ota +"} ]," +ĠSt ev +B io +ĠPlan es +Ġsuggest ive +Ġdec ipher +ĠRefuge e +ĠKe jriwal +ĠGreen peace +Ġdecl ass +ĠSound ers +Ġth o +Ġdec rypt +Ġbr ushing +ĠJane iro +ip op +S i +8 77 +ĠGeoff rey +Ġc pu +ĠHaz el +Ġview points +Ġcris py +ĠNot ification +Ġsold er +ĠMod est +ĠHem isphere +Ġcass ette +in cludes +Ġident ifiers +ĠC ALL +in cent +T odd +ĠSwe ep +Ġ3 34 +b oss +Ġsm ir +gin x +Ġtown ship +Ġg rieving +ĠMos que +Net flix +AS ED +ĠMillenn ials +oc om +19 67 +Ġbold ly +s leep +Ġes che +arij uana +Ġsw irl +ĠPen al +Ġneglig ent +ĠStephen son +K ER +ĠZ oro +ris is +Ġlocal ization +ĠSeym our +ĠAng lic +red itation +prot ection +ĠPa ige +Ġo mit +ĠR ousse +ĠT ub +Ġinv itations +t ty +Ġm oss +ph ysical +C redits +Ġan archy +Ġchild care +Ġl ull +ĠM ek +ĠL anguages +lat est +ĠSan ford +Ġus ability +Ġdiff use +ĠD ATA +Ġsp rites +ĠVeget a +ĠProm otion +ãĥ¼ ãĤ¯ +rict ing +z ee +Tur kish +ĠTD s +pro ven +57 1 +Ġsmug glers +707 10 +Ġreform ed +ĠLo is +Ġun fl +ĠWITH OUT +ĠReturn ing +ann ie +ĠTom as +Fr anc +ĠProf it +ĠSER V +ĠR umble +ik uman +es an +Ġt esters +Ġgad get +Ġbrace let +ĠF SA +comp onent +Ġparamed ics +Ġj an +ĠRem em +ĠSk inner +Ġl ov +ĠQu ake +rom a +Ġfl ask +Pr inc +Ġover power +Ġlod ging +ĠK KK +ret te +Ġabsor bs +w rote +Ġ ," +K ings +ĠH ail +ĠFall ing +xt ap +ĠHel ena +ire ns +L arry +Ġpamph let +ĠC PR +G ro +ĠHirosh ima +Ġhol istic +". [ +Ġdet achment +Ġas pire +Ġcompl icit +ĠGreen wood +Ġresp awn +ĠSt upid +ĠFin ished +f al +b ass +Ġab hor +Ġmock ery +ĠFe ast +VID EO +Ġcon sec +ĠHung ry +P ull +ĠH ust +it ance +? ãĢį +) -- +ĠPar allel +con v +4 69 +ha ar +w ant +P aper +m ins +ĠTor o +ĠTR UMP +ĠR ai +D W +ĠW icked +ĠL ep +Ġfun ky +Ġdetrim ent +ios is +ache v +Ġde grade +im ilation +Ġret ard +Ġfrag mentation +Ġcow boy +ĠY PG +ĠH AL +Parent s +ĠS ieg +ĠStra uss +ĠRub ber +× IJ +Fr ag +Ġp t +Ġoption ally +ĠZ IP +ĠTrans cript +ĠD well +88 2 +M erc +ĠM OT +ãĥ¯ ãĥ³ +Ġhun ts +Ġexec utes +In cludes +Ġacid ic +ĠRespons ibility +ĠD umb +we i +And erson +ĠJas per +ight on +abs olutely +Ad ult +Ġpl under +Mor ning +ĠT ours +ĠD ane +Î º +ĠT EST +ĠG ina +Ġcan ine +aw an +Ġsocial ists +ĠS oda +Ġimp etus +ĠSupplement ary +oli ath +ĠKinn ikuman +mitted ly +second s +Ġorganis ers +Ġdocument aries +Vari able +GRE EN +Ġres orts +Ġbr agging +Ġ3 68 +Art ist +w k +bl ers +Un common +ĠRet rieved +Ġhect ares +Ġtox in +r ank +Ġfaith s +ĠG raphic +Ġve c +ĠL IA +Af rican +Ġard ent +end iary +L ake +ĠD OS +cient ious +ĠOk awaru +ĠAll y +ĠTim eline +D ash +ĠI c +contin ue +Ġt idy +Ġinstinct ively +ĠP ossibly +ĠOut door +ĠWould n +Ġl ich +ĠBr ay +ĠA X +Ġà ī +Ġ+ # +\ ' +Direct ory +ab iding +Ġf eral +ic ative +but t +Ġper verse +S alt +Ġwar ped +Ġnin eteen +Ġcabin ets +Ġsrf Attach +ĠSl oan +Ġpower ing +reg ation +F light +se vere +Ġst ren +Ġc og +ap ache +Ġâ Ŀ +Ġcaf eteria +p aces +ĠGrim oire +uton ium +Ġr aining +Ġcir cling +Ġlineback ers +c redit +Ġrep atri +ĠCam den +lic ense +Ġly ric +Ġdescript or +Ġval leys +Ġre q +Ġback stage +ĠPro hibition +ĠK et +Op ening +S ym +æĸ ¹ +Ġserv ings +Ġoverse en +Ġaster oids +ĠMod s +ĠSpr inger +ĠCont ainer +è » +ĠM ens +Ġmult im +Ġfire fighter +pe c +Ġchlor ine +Ð ¼ +end i +Ġsp aring +Ġpolyg amy +ĠR N +ĠP ell +Ġt igers +Ġflash y +ĠMad ame +S word +Ġpref rontal +Ġpre requisite +uc a +Ġw ifi +Ġmiscon ception +Ġharsh ly +ĠStream ing +ot om +ĠGiul iani +foot ed +Ġtub ing +ind ividual +z ek +n uclear +m ol +Ġright ful +49 3 +Ġspecial ization +Ġpassion ately +ĠVel ocity +ĠAv ailability +T enn +Ġl atch +ĠSome body +Ġhel ium +cl aw +Ġdi pping +XX X +Ġinter personal +7 10 +Ġsub ter +Ġbi ologists +ĠLight ing +Ġopt ic +Ġden im +end on +ĠC orm +Ġ3 41 +ĠC oup +Ġfear less +Ġal ot +ĠCliff ord +ĠRun time +ĠProv ision +up dated +lene ck +Ġneur on +Ġgrad ing +ĠC t +sequ ence +in ia +con cept +Ġro aring +ri val +ĠCaucas ian +Ġmon og +key es +Ġappell ate +Ġlia ison +EStream Frame +ĠPl um +! . +Ġsp herical +Ġper ished +Ġbl ot +Ġben ches +Ġ4 11 +Ġpione ered +Ġhur led +Jenn ifer +ĠYose mite +Ch air +Ġreef s +Ġelect or +ĠAnt hem +65 2 +Ġun install +Ġimp ede +Ġbl inking +Ġgot o +Dec re +A ren +Ġstabil ization +ĠDis abled +ĠYanuk ovych +Ġoutlaw ed +ĠVent ura +ten ess +Ġplant ation +Ġy acht +ĠHu awei +Ġsol vent +Ġgr acious +Ġcur iously +Ġcapac itor +Ġc x +ĠRef lex +Ph ys +ĠC f +pt in +cons ervative +Ġinv ocation +c our +F N +ĠNew ly +H our +As ian +ĠLe ading +ĠAer ospace +An ne +Ġpre natal +Ġdeterior ating +H CR +ĠNorm andy +ol ini +ĠAm bro +9 10 +Ġset backs +ĠT RE +Ġs ig +ĠSc ourge +59 7 +79 8 +Game play +Ġm sec +M X +Ġprice y +ĠL LP +aker u +Ġover arching +ĠB ale +Ġworld ly +Cl ark +Ġscen ic +Ġdisl iked +ĠCont rolled +T ickets +ĠE W +ab ies +ĠPl enty +Non etheless +Ġart isan +Trans fer +ĠF amous +Ġinf ield +ble y +Ġunres olved +ĠML A +ãĤ Ĥ +Cor rection +Ġdemocr at +ĠMore no +ro cal +il ings +Ġsail or +Ġr ife +h ung +Ġtrop es +Ġsn atched +ĠL IN +ĠB ib +ES A +ĠPre v +ĠCam el +run time +Ġob noxious +4 37 +Ġsum mers +Ġunexpl ained +ĠWal ters +cal iber +Ġg ull +ĠEnd urance +ä½ ľ +Ġ3 47 +Ir ish +Ġaer obic +Ġcr amped +ĠHon olulu +à © +us erc +ec ast +AC Y +ĠQu ery +ãĤ¹ ãĥĪ +Bet a +Ġsuscept ibility +ĠSh iv +ĠLim baugh +Ġà ĸ +ĠN XT +ĠM uss +ĠBrit ons +ES CO +EG IN +Ġ% % +Ġsec ession +ĠPat ron +ĠLu a +n aires +ĠJPM organ +us b +ocy te +Ġcouncill ors +ĠLi ang +f arm +Ġnerv ously +Ġattract iveness +ĠK ov +j ump +Pl ot +Ġst ains +ĠStat ue +ĠApost les +he ter +ĠSUP PORT +Ġoverwhel m +Y ES +Ġ29 1 +d ensity +Ġtra pping +M it +Ġf ide +ĠPam ela +atl antic +Dam n +Ġp ts +OP A +Ġserv icing +Ġoverfl owing +ul o +ĠE rit +t icket +light ing +ĠH mm +ãĥ¼ ãĥ« +im oto +Ġchuck le +4 23 +ãģ ķ +sh ape +Ġque ues +Ġanch ors +ãĤ¼ ãĤ¦ãĤ¹ +F er +Ġaw oke +Ġ6 66 +h ands +Ġdiver gence +Ġ50 5 +T ips +Ġdep ot +Ġske w +ĠDel iver +op ot +Ġdiv ul +ĠE B +uns igned +ĠUn i +X box +Ġfor ks +Ġ7 02 +å ¯ +Ġpromot ers +ĠV apor +Ġlev ied +sl ot +Ġpig ment +Ġcyl inders +C RE +Ġsn atch +Ġperpet ually +Ġl icking +ĠFe et +ĠKra ken +ĠHold en +ĠCLS ID +m r +Ġproject or +Ġden otes +Ġchap el +ĠTor rent +b ler +R oute +ĠDef endant +ĠPublisher s +ĠM ales +ĠInn ov +ĠAg ility +rit er +ty mology +st ores +L ind +Ġf olly +ĠZur ich +B le +Ġnurt ure +Ġcoast line +uch in +D omin +Ġfri vol +ĠCons olid +res ults +M J +Ġphyl ogen +Ġha uled +ĠW iley +ĠJess ie +ĠPrep are +ĠE ps +Ġtreasure r +I AS +Ġcolon ists +Ġin und +ĠWW F +ĠCon verted +6 000 +out side +ĠApp earance +ĠRel ic +ĠM ister +s aw +Ġresult ant +Ġadject ive +ĠLaure l +ĠHind i +b da +Pe ace +Ġreb irth +Ġmembr anes +Ġforward ing +Ġcoll ided +ĠCar olyn +K ansas +5 99 +ĠSolid GoldMagikarp +Be ck +Ġstress ing +ĠGo o +ĠCooper ative +Ġf s +ĠAr chie +L iter +ĠK lopp +J erry +Ġfoot wear +War ren +Ġsc ree +h are +Under standing +P ed +Ġanth ology +ĠAnn ounce +M ega +Ġflu ent +Ġbond age +ĠDisc ount +il ial +C art +ĠNight mares +Sh am +ĠB oll +uss ie +H ttp +Atl anta +Ġun recogn +ĠB id +Ġunder grad +Ġforg iving +ĠGl over +AAAA AAAA +4 45 +V G +pa io +kill ers +Ġrespons ibly +Ġmobil ize +Ġeffect ed +ĠL umin +Ġk ale +Ġinfring ing +ann ounced +Ġf itt +b atch +ĠT ackle +ĠL ime +ĠAP P +uke mia +Ġrub y +Ġex oner +ĠCas ual +0 70 +Ġpel vic +Ġautom ate +ĠK ear +ĠCoast al +Ġcre ed +Ġbored om +ĠSt un +ri ott +Ĥ İ +Ġregener ate +Ġcomed ians +ĠOP ER +Sp ons +id ium +on is +L ocated +05 7 +Ġsusp ense +ĠD ating +C ass +Ġneoc ons +ĠShin zo +Ġaw oken +ch rist +ĠMess ages +att led +ĠSpr ay +ĠSp ice +C W +Ġshield ing +ĠG aul +Am id +Ġparam ilitary +Ġmult if +ĠTan ner +il k +Ġgodd amn +g ements +Ġbe friend +m obi +Ġ3 88 +fold er +acc a +Ġins in +g ap +N ev +fif th +Ġpsychiat ry +b anks +TH IS +Ġhar b +ac qu +Ġfac ade +ĠPower Point +80 3 +Ġbl uff +Sh ares +Ġfavor ing +El izabeth +Ãį Ãį +Ġr anger +77 2 +ĠAr che +h ak +ĠGen etics +ĠF EMA +Ġev olves +Ġest e +ĠP ets +ĠM é +ĠInterest ing +ĠCanter bury +ch apter +ĠStar fleet +Sp anish +Ġdraw back +ĠNor wich +9 70 +n orth +ag anda +Ġtransform ative +ram ids +bi ology +ad ay +Ġpropag ation +ĠGam ma +ĠDen ise +ĠCalcul ator +ent imes +ĠB ett +Ġapp endix +ĠHD D +AK ING +Ġst igmat +Ġhol ster +Ġord inarily +Ch ance +ĠCont rary +Ġad hesive +Ġgather s +6 12 +re au +ony ms +ew ays +Ġindu ces +Ġinterchange able +se m +Wh it +Ġtr ance +Ġincorpor ation +ĠExt ras +Fin ancial +Ġawkward ly +ĠStur geon +ĠH Y +Norm ally +ĠEnd ing +ĠAss ist +enc rypted +Ġsub jug +Ġn os +Ġfan atic +C ub +C U +?" . +Ġirre versible +å Ĥ +03 1 +ĠH AR +sp read +ul ia += $ +Sc ope +L ots +Ġlif estyles +ol on +Ġf eds +Ġcongrat ulate +web kit +Ġindist inguishable +ĠSw ing +Ġcommand ments +qu ila +ab ella +m ethyl +ann abin +Ġo vere +Ġlob ster +ĠQU EST +ĠCONT IN +bern atorial +:::: :::: +ĠTra ve +ĠSam oa +AN I +75 2 +Ð ´ +userc ontent +ĠMod erate +y eah +ĠK itt +Ġwe e +Ġstuff ing +ĠInter vention +ĠD ign +Ġware houses +ĠF iji +Ġpel lets +Ġtake away +ĠT ABLE +ĠClass ical +col lection +Ġland fall +ĠMus cle +Ġsett les +ĠAD V +Ġ3 44 +L aura +Ġf ared +ĠPart ial +4 36 +oss ibility +ĠD aly +ĠT arant +ĠFu ji +am l +c ence +55 1 +ĠProced ures +ĠO CD +ĠU D +t in +Q UI +ach o +4 38 +Ġgl itches +Ġenchant ment +Ġcalcul ates +IR O +ĠH ua +alys es +ĠL ift +um o +Ġle apt +Ġhypothes ized +ĠGust av +it ans +VERS ION +æ ł +Rog er +Ġr and +ĠAd apter +Ġ3 31 +ĠPet ition +k ies +M ars +Ġunder cut +ze es +ĠLy ons +ĠDH CP +Miss ing +Ġretire es +Ġins idious +el i +> ) +. ãĢį +Ġfinal ists +ĠA ure +Ġacc user +Ġwas tes +ĠY s +ĠL ori +Ġconstitu encies +Ġsupp er +Ġmay hem +or ange +Ġmis placed +Ġmanager ial +Ġex ce +ĠCL I +Ġprim al +ĠL ent +Cry stal +h over +ĠN TS +end um +Ġd w +ĠAl c +n ostic +Ġpres erves +ĠTs arnaev +Ġtri pled +rel ative +Arc ade +k illing +ĠW EEK +ĠH anna +D ust +Com pleted +ģ « +Ġappro ves +ĠSur f +ĠLuther an +ven ants +Ġrobber ies +we ights +soft ware +at ana +ug al +Ġgrav y +ĠC ance +OLOG Y +ly ak +Ton ight +Ġunve il +Ġ19 04 +ĠMin ion +ent ious +st ice +pack ages +ĠG EAR +Ġg ol +ĠHutch inson +ĠProf ession +ĠG UN +ĠDiff erence +ĠTsuk uyomi +ĠLes bian +6 70 +Ġfug itive +ĠPlan etary +-------------------------------- ------------------------ +Ġacc rued +Ġch icks +Ġsto pp +Ġblock ers +C od +Ġcomment ers +ĠSomew here +ĠPhot ographer +the me +Ġmay oral +w u +Ġanten nas +Ġrev amped +ĠSubject s +it é +im ura +Ġentr ances +liter ally +Ġten ets +ĠO MG +ĠMP H +ĠDon key +ĠOff ense +Ġ" + +Sn ap +ĠAF B +Ġan imate +ĠS od +His panic +Ġinconsist ency +D b +F Y +Ex port +Ġa pe +Ġpear l +ib el +ĠPAC s +Ġ{ \ +Ġact u +ĠHS BC +camp us +Ġpay off +Ġde ities +ĠN ato +ou ple +Ġcens ored +ĠCl ojure +Ġconf ounding +en i +Ġreck on +op he +Ġspot ting +Ġsign ifies +Ġprop el +Ġfest ive +S uggest +Ġpled ging +ĠB erman +Ġrebell ious +Ġovershadow ed +Ġinfiltr ated +j obs +67 2 +Ġscal able +Ġdomin ion +ĠNew foundland +ĠMead ow +Ġpart itions +AM I +Ġsupplement ary +str ument +Ġhair y +Ġperpet uate +Ġnuts hell +ĠPot ato +ĠHob bit +Ġcur ses +Flo at +Ġquiet er +Ġfuel ing +Ġcaps ules +ĠL ust +ĠH aunted +Exec utive +Ġchild birth +G re +Ġrad iant +å İ +Ġm alls +Ġin ept +ĠWarrant y +Ġspect ator +E h +t hens +Ġculmin ating +æ © +ary a +ãĤ ® +ilit arian +ĠOR IG +ĠSp ending +pt ives +ĠS iren +ĠRec ording +ay ne +Ġv im +Ġspr ang +T ang +ĠM FT +mor ning +ĠWe ed +m peg +cess ion +ĠCh ung +7 30 +w arning +56 2 +handed ly +P oor +P olitics +: # +Ġp ian +Ġfec es +ĠDocument ation +Ġban ished +Ġ3 99 +ĠAR C +Ġhe inous +J ake +ĠAm ir +way ne +v re +os henko +Ġnotebook s +Ġfound ational +Ġmarvel ous +ixt ape +Ġwithdraw als +Ġh orde +ĠD habi +is able +ĠK D +Ġcontag ious +ĠD ip +ĠAr rows +Ġpronoun s +Ġmorph ine +ĠB US +68 2 +Ġk osher +fin ished +ĠInstr uments +Ġf used +yd en +ĠSal mon +F ab +aff ected +K EN +C ENT +Dom ain +Ġpoke mon +ĠDr inking +G rowing +ĠInvestig ative +ĠA ether +em i +Ġtabl oid +Ġrep ro +ĠNot withstanding +ĠBers erker +Ġdram as +Ġclich é +Ġb ung +ĠU RI +ĠD os +0 44 +Ġpast ors +Ġl s +Ġac rylic +aun ts +Ed ward +Ġmajor ities +B ang +Ġfield ing +ĠRepl acement +ĠAl chemy +pp ard +ĠRome o +ĠSan ct +ĠLav rov +ib ble +Inst ruct +Ġimp ractical +ĠPlay boy +ce phal +Ġsw aps +Ġk an +ĠThe o +Ġillust rating +Ġdismant led +ĠTrans gender +ĠG uth +UG H +Ġtriumph ant +Ġencomp ass +Ġbook mark +udd in +j er +Ġpred icate +ES H +Ġwhen ce +ĠAB E +Ġnon profits +Se qu +Ġdi abetic +Ġp end +Ġheart felt +sh i +Ġinter acts +ĠTele com +Ġbombard ment +dep ending +ĠLow ry +ĠAd mission +ĠBl ooming +ust ration +ene gger +B rew +Ġmol ten +ĠNer d +P IN +âĸ Ģ +ave ment +Ġtou red +Ġco efficients +ĠTray von +ans son +Ġsand y +t old +fl ows +Ġpop ulous +ĠT inder +ĠBl iss +R achel +Min imum +Ġcontest ant +ĠRed uce +ĠMor se +ĠGrass ley +ĠClick er +Ġexp r +Ġs incerity +Ġmar qu +Ġelic it +ĠPro position +ĠDemon ic +Ġtac os +G reek +Ġpost war +Ġin sofar +ĠP ork +Ġ35 2 +doctor al +walk ing +Ġmid term +ĠSam my +sight ed +ĠTR ANS +ic i +AL D +ĠUS L +ĠF ISA +ĠAm pl +ĠAlex andra +ine lli +Tr ain +Ġsign ify +ĠVers us +Ġob fusc +Ġk h +Ġagg ro +ĠRen ault +Ġ3 48 +5 18 +ox icity +0 22 +ĠTw ist +Ġgoof y +D ynamic +Ġbrief ings +m ight +8 99 +Ġderog atory +T ro +Ġfor ging +ĠKor an +ĠMar ried +ĠBuc s +Ġpal ate +ĠCon version +m able +4 13 +Ġ( _ +Ġs iph +ĠN EO +col lege +Ġmarg inally +Ġfl irt +ĠTra ps +ĠP ace +é »Ĵ +Ġgoalt ender +Ġforb ids +Ġcler ks +ĠT ant +ĠRobb ins +ĠPrint ing +Ġpremie red +Ġmagn ification +ĠT G +ĠR ouse +ĠM ock +odynam ics +Ġpre clude +ism o +ĠPul itzer +Ġaval anche +ĠK odi +rib une +ĠL ena +Elect ric +Ġref inery +Ġend owed +Ġcounsel ors +Ġd olphin +ĠM ith +Ġarm oured +hib ited +Beg in +ĠP W +O il +ĠV or +ĠShar if +ĠFraz ier +est ate +Ġj ams +Pro xy +Ġband its +ĠPresbyter ian +ĠPrem iere +t iny +ĠCru el +Test ing +Ġhom er +ĠV ERS +ĠPro l +ĠDep osit +ĠCoff in +Ġsemin ars +Ġs ql +ĠDef endants +Altern atively +ĠR ats +ç « +ethy st +' > +Ġiss uer +58 9 +Ġch aired +ĠAccess ories +man ent +Ġmar row +ĠPrim ordial +C N +Ġlimit less +ĠCarn age +Ġund rafted +q v +IN ESS +on ew +Ġco hesion +98 7 +Ġne cks +Ġfootball er +ĠG ER +Ġdetect able +ĠSupport ing +ĠCS V +oc ally +k Hz +Ġund e +Ġsh one +Ġbud ding +tra k +Stand ing +ĠStar craft +ĠKem p +Ben ch +Ġthw arted +ĠGround s +ath i +L isa +Dial og +ĠS X +V ision +Ġingen ious +Ù IJ +Ġfost ering +ĠZ a +ĠIn gram +Ġ" @ +N aturally +6 16 +0 35 +ĠF AC +H mm +55 4 +Ġacceler ator +ĠV end +Ġsun screen +Ġtuber culosis +rav iolet +ĠFunction al +ĠEr rors +ed ar +19 66 +ĠSpect re +ĠRec ipes +88 5 +ĠM ankind +L iverpool +Ġ| -- +Ġsubst itutes +ĠX T +w ired +Ġinc o +ĠAf gh +E va +ic c +S ong +K night +Ġdilig ently +ĠBroad cast +A id +Ġaf ar +ĠH MS +aton in +ĠGr ateful +Ġfire place +ĠOm ni +e uro +ĠF RE +ĠSh ib +ĠDig est +t oggle +Ġheads ets +Ġdiff usion +ĠSqu irrel +ĠF N +Ġdark ened +out her +Ġsleep s +ĠX er +gun s +Ġset ups +Ġpars ed +Ġmamm oth +ĠCur ious +g ob +ĠFitz patrick +ĠEm il +im ov +........ ..... +ĠB enny +Second ly +Ġheart y +Ġcons on +st ained +Ġgal actic +cl ave +Ġplummet ed +Ġp ests +Ġsw at +Ġrefer rals +ĠLion el +h oly +Ġunder dog +ĠSl ater +ĠProv ide +ĠAm ar +ress or +å Į +ong a +Ġtim id +Ġp iety +ĠD ek +Ġsur ging +az o +Ġ6 10 +Ġdes ks +ĠSp okane +ĠAn field +Ġwars hips +ĠCob ra +Ġar ming +clus ively +ĠBad ge +ag ascar +ĠPR ESS +ĠMcK enzie +ĠFer dinand +burn ing +Af ee +Ġtyr ann +ĠI w +ĠBo one +100 7 +ĠRe pt +Ċ Âł +Ġcar avan +ĠD ill +ĠBundes liga +Ch uck +Ġheal er +ãĥ¼ãĥ Ĩ +ĠH obby +Ġneg ate +Ġcrit iques +section al +mop olitan +Ġd x +Ġouts ourcing +ĠC ipher +t ap +Sh arp +Ġup beat +Ġhang ar +Ġcru ising +ĠNi agara +Ġ3 42 +ill us +ĠS v +Ġsubt itles +Ġsqu ared +Ġbook store +Ġrevolution aries +ĠCarl ton +ab al +Ut ah +Ġdesp ise +ĠU M +cons ider +aid o +Ġc arts +ĠT urtles +Tr aining +Ġhonor ary + ¢ +Ġtri angles +4 22 +Ġreprint ed +Ġgrace ful +ĠMong olia +Ġdisrupt ions +ĠB oh +Ġ3 49 +Ġdr ains +Ġcons ulate +Ġb ends +Ġm afia +ur on +ĠF ulton +m isc +Ġren al +Ġin action +ck ing +Ġphot ons +Ġbru ised +ĠC odes +og i +Ġn ests +ĠLove ly +ĠLib re +ĠD aryl +Ġ# ## +S ys +. ," +Ġfree zes +est ablishment +and owski +Ġcum bers +ĠSt arg +ĠBom bs +Ġleg ions +Ġhand writing +Ġgr un +ĠC ah +sequ ent +Ġm oth +ĠMS M +Ins ert +F if +Ġmot el +Ġdex ter +ĠB ild +hearted ly +Ġpro pe +ĠText ure +ĠJ unction +ynt hesis +oc ard +ĠVer a +ĠBar th +Ġμ g +Ġl ashed +Ġ35 1 +ĠZ amb +ĠSt aples +ĠCort ex +ĠCork er +Ġcontinu um +ĠWR ITE +unt a +rid or +Ġde ems +0 33 +ĠG OLD +p as +Ġrep ressive +ãĥĨ ãĤ£ +Ġbaff led +Sc ar +Ġc rave +Ġ ______ +Ġentrepreneurs hip +ĠDirector ate +Ġ' [ +Ġv ines +Ġasc ended +ĠGR OUP +ĠGood bye +Ġdo gged +ãĥ´ ãĤ¡ +Man ufact +Ġunimagin able +ri ots +ier rez +Ġrel ativity +ĠCraft ing +ra ught +ud en +c ookie +Ġassass ins +Ġdissatisf ied +ac ci +Ġcondu it +Sp read +ĠR ican +n ice +izz le +Ġsc ares +ĠWH Y +ph ans +5 35 +Ġprot racted +ĠKrist en +5 36 +ĠSc rib +ĠNe h +Ġtwent ies +Ġpredic ament +Ġhandc uffs +Ġfruit ful +ĠU L +ĠLud wig +Ġatt est +ĠBre aker +Ġbi ologically +ĠDeal er +Ġrenov ations +f w +ess en +Al ice +ĠHen ri +Ġun ilaterally +ĠS idd +h ai +ĠSt retch +S ales +Ġcumbers ome +ĠJ avier +Ġtrend y +Ġrot ting +ĠChall enges +Ġscra ps +Ġfac ets +ĠVer onica +ĠVer ge +ĠS ana +Al ien +ĠR ih +Ġrad ial +ect ar +Ġ6 30 +cl i +Mar ie +Ġwild fire +ĠCat o +h ander +Ġwait ress +Ġch ops +ĠS ECTION +Ġblunt ly +ĠCat alog +n ian +stud y +Ġpat rolling +ĠT enth +nex us +ĠN ON +op sy +Ġsc athing +s ie +Ġdeterior ated +V B +Naz is +Ġdep ictions +Ġauthent icated +ĠCon ce +k rit +Ġpromul g +ĠL ONG +U FC +ĠVis itors +ĠRec all +Ġrehab ilit +ĠSL I +Ġglac ier +ĠB ite +Ġ50 3 +Ġvom it +Ġfer mented +ĠKh alid +Ġgrad ed +ĠMag icka +ĠIch igo +power ful +ic ators +75 3 +Ġsh rew +Ġ35 6 +Ġlegal izing +Ġall otted +ĠArch demon +ith ing +igg urat +V OL +Le od +Ġo ily +Ġindu cing +Ġamy gdala +Ġadm ins +ĠAcqu isition +C AN +Ġsche matic +Ġmo an +ĠCamer oon +Ġt ink +Ġmer ry +Ġbutter flies +ĠGo ff +Ġworks pace +ĠCor ona +Ġj avascript +ĠD olphin +ĠCant or +4 64 +to e +AP S +ĠAg ing +Ġpadd ed +ĠZ heng +ĠHe ld +Ġest ranged +Ġ7 70 +. } +ĠDun ham +Ġsm okes +Ġcap itals +und ai +Sh in +ĠFound ing +Ġent itle +Ġcenter piece +D iscover +Ġthere to +al ert +ĠN ou +ĠAnaly st +l c +F H +FI ELD +ĠP OV +gr ay +Ġar cs +ĠH OT +Ġr s +Ġoblig atory +ĠArchitect s +ĠS ven +ĠF EC +0 200 +Christ mas +ĠAlban ia +rat om +58 7 +Ġhard ships +Ġaut os +ĠCharg es +Ġap es +Ġ3 76 +wal let +Ġintox ication +Ġgobl in +Ġ5 70 +++++++++ ++++++++ +ĠYel p +ĠMag netic +ĠBr iggs +R ail +Ġspawn s +ĠW iggins +Ġshowc ased +Ġres orted +ub en +Ġwh ipping +Ġim itate +Ġdigest ion +ĠUS PS +ĠG est +Ġye a +ĠT ight +ind al +ic as +` . +C AST +'' ; +ĠF et +opath ic +In valid +Ġregrett ed +Ġbro ccoli +ĠSc ores +e ve +Ġpost ings +Ġaccum ulating +Ġneed less +elf th +Ġmay ors +Ġsc rib +Ġanecd otes +Ġbot ched +ĠRib bon +ĠConstant ine +i uses +ess es +Ġdev ise +Comp ared +Ġp udding +Ġg arg +Ġev oke +79 7 +Ġdet ox +9 09 +ĠPie ces +ĠMcC artney +Ġmet ast +ĠK rypt +P OR +Ġt ending +ĠMerch ants +Pro of +ĠV arg +ĠPort able +ãĥ¼ãĥĨ ãĤ£ +B rain +25 00 +Ġfol iage +Ø ¹ +Ġment ors +ĠA ires +Ġminimal ist +Ġing ested +ĠTro jan +ĠQ ian +inv olved +0 27 +Ġer oded +RA FT +Ġbl urry +M ob +Ġbuff et +ĠFn atic +ae a +KN OWN +ĠIn it +s afety +en um +ACT ION +ĠCrus her +ĠD ates +Ġ ................ +c alling +ak ov +Ġvent ured +Ġ5 55 +au ga +H art +ĠA ero +M AC +Ġthin ly +Ġar ra +ST ATE +ild e +ĠJac qu +ĠFem ales +Ġthe orem +Ġ3 46 +Ġsmart est +ĠPU BLIC +ĠK ron +ĠB its +ĠV essel +ĠTele phone +Ġdec ap +Ġadj unct +ĠS EN +mer ga +Ġred acted +Ġpre historic +Ġexplan atory +ĠRun s +ĠUtt ar +ĠM anny +ĠAUTH OR +ĠUnle ashed +ĠBow ling +be ans +79 3 +Ġunivers es +Ġsens it +ĠK ung +re peat +ctr l +Ġp aced +Ġfull er +Cl ock +Ġrec omb +ĠF aul +ĠB unker +Ġpool ed +Ġan a +ĠM outh +LL OW +hum ane +Ġbull do +ĠMicha els +f am +Ġwreck ed +Ġport rays +ĠWh ale +ĠH es +Ġguess es +ĠBrow se +ĠL APD +Ġconsequ ential +ĠInn ocent +ĠD RAG +Ġtrans gress +ĠO aks +Ġtri via +ĠRes on +ĠA DS +-- + +ĠT oll +Ġgrasp ing +ĠTHE M +ĠT ags +ĠCon clusion +Ġpract icable +Ġho op +Ġunintention ally +Ġign ite +ĠM ov +ur ized +le hem +Ter min +Ġcolour ful +ĠLin ear +ĠEll ie +G y +Ġman power +Ġj s +Ġem oji +ĠSHAR ES +_ . +0000 7 +Ġsophistic ation +Ġunders core +Ġpract ise +Ġbl ob +op ens +Uk raine +Ke eping +Y C +J R +ult imate +Cl aim +Ġautom obiles +99 3 +ste el +Ġpart ing +ĠL ank +... ? +Ġ38 5 +Ġremem brance +Ġe ased +Ġcov ari +ĠS ind +Effect ive +Ġdisse mination +ĠMo ose +ĠCl apper +br ates +App ly +Ġinv is +Ġwors ened +âĢĶ - +Ġlegisl ator +ĠL ol +ĠRow e +Ġdealers hip +um ar +id ences +Ġinvestig ates +Ġc ascade +Ġbid der +ĠB EN +Iron ically +Ġpres iding +Ġd ing +Ġcontrad icted +Ġshut s +ĠF IX +Ġ3 66 +Dist rict +Ġsin ful +ĠChar isma +o ops +Ġtot ality +Ġrest itution +ĠOpt imus +ĠD ah +Ġcl ueless +urn ed +Ġnut rit +Ġland owners +Ġfl ushed +Ġbroad en +m ie +Ġprint ln +Ġn ig +ĠCorp us +J en +Ġprot o +ĠWik imedia +ĠPal o +C OR +Ġstory lines +Ġevangel icals +ĠDar rell +Ġrot or +ĠH W +sk illed +ery l +Ġbe gg +ĠBl umenthal +Ġwe aving +Ġdown wards +ĠJack et +ĠANG EL +Te chnology +Ġes oteric +alde hyde +Ġfur iously +Ġforeign er +We ak +CH O +ĠH ound +Exper ience +ĠPlay station +ĠM IA +ĠU ng +cl oth +ag all +Ġcal ming +iz ens +St ruct +ĠW itches +ĠCeleb ration +Ġ........ ...... +pt roller +ĠTC U +Ġb unny +ãĥ į +ut orial +Ġup scale +ĠSt a +ĠCol ossus +Ġchlor ide +ĠZ ac +ĠRe asons +ĠBrook ings +ĠWH ITE +][ / +ĠL ose +9 05 +Ġunders ide +ern els +Ġv ape +do zen +upp et +ĠST OP +mat ical +ĠStat ements +hed dar +P AC +Custom er +Ġmem os +ĠP J +end ars +ĠLim its +l augh +Ġstabil ized +ĠALE C +Y A +Up grade +al am +Ġtechn o +Ġan ew +fore seen +Ġcolleg iate +ĠPy ro +ĠD ism +Ġfront line +Ġammon ia +I U +Qu ite +John ny +ass in +G OP +ĠSt yles +ĠSovere ign +acter ial +5 49 +ĠR IP +ĠL ists +Ġ3 64 +ĠRece p +s ocket +ĠByr d +ĠCand le +An cient +Ġappell ant +en forcement +ace a +ans ki +Ġold s +88 6 +Ġsl urs +Ġem pires +Ġbuck le +Ġalien ation +ĠAber deen +Ġunic orn +Ġoverr iding +ĠL X +pp a +Ġdesp ised +ĠB ugs +ĠB ST +S outhern +5 33 +Ġhall mark +ĠPost er +Ġstem med +Ġprincip als +ĠT ECH +ĠSand wich +It aly +Ġche esy +ĠSet TextColor +ĠProt ective +ĠC ohn +J O +apt op +Re ason +Lead er +ĠUnder stand +ĠFr idays +ĠContin uous +Ġcl ipping +ĠR ye +Ġber th +tim er +ann is +re act +Ġbuff alo +ĠPar as +Ġ6 55 +Ġpres ided +ĠSun rise +Ġve ts +Ġcl oves +ĠMcC ull +Stre ngth +G AN +Ġill iter +ĠPric ing +l é +Ġresist or +Ġbr un +ĠSuff olk +Ñ ĭ +ĠL iver +Re leased +Ġwhat s +8 60 +ĠMe asures +Ġden ouncing +ĠRy zen +Ġsou ven +Ġcareg ivers +ch ini +ĠScar lett +Ġt rough +Cong ratulations +Ġtax is +ĠTrad ition +j it +Ġtable top +Ġhither to +Ġdis information +off ensive +h ra +ĠDISTR ICT +Ġcompl icate +chen ko +ĠRecon struction +Ġpalp able +Ġa usp +Ġ4 28 +Ġshowc ases +ĠPublic ation +know ledge +inn on +4 19 +Ġretri eval +and ers +Ġref ute +Ġinqu ired +g ur +Ġneg ativity +Ġcons erve +Ġafter life +Ġpres upp +ĠGill espie +Ġm t +ĠD N +T ap +Ġper pend +ĠS my +does n +Ġsp illing +Ġhyp ers +K ate +® , +ke pt +ĠP owered +Ġj a +ĠK lux +ard e +ab an +Ġ4 44 +Ġflatt ened +ĠImprove ments +urg a +ĠK und +Ġins cribed +Ġfac ult +Ġunpre pared +ĠCons umers +Ġsatisf ies +Ġpul monary +Ġinf iltration +Ġex ternally +Ġcongrat ulations +ag han +Ġair liner +Ġfl ung +Ġfly ers +G D +Ġsnipp ets +Ġrec ursive +Ġmaster ing +L ex +Ġovert ly +v g +Ġluck ily +Ġenc ro +ĠLanc et +ĠAbyss al +function al +Ġs ow +Ġsqu id +Ġnar ration +Ġn aughty +ĠHon our +ĠSpart ans +Ġsh atter +ĠTac oma +ĠCal ories +ĠR aces +Sub mit +Ġpurpose fully +w av +ĠY ok +F est +ĠG err +Met ro +Ġit iner +f amous +Ġ" { +in line +was her +Iss ue +ĠCL IENT +oz o +Vers ions +7 25 +ĠGl ock +Ġshield ed +ĠPC R +ENC Y +ĠWe ld +ĠSim pl +Ġredirect ed +ĠK ham +Ġ( > +Ġlab ou +Ġdi apers +ss l +Ġcell ar +organ isms +ore sc +ĠBer ks +did n +Sh ipping +C hest +Ġund one +Ġmillion aire +Ġc ords +ĠYoung er +appropri ately +Ġsequ els +u ve +ant icipated +Ġle wd +ĠSh irt +ĠDmit ry +V eter +Ġsl aying +ĠY ar +Ġcompl ication +I owa +ĠEric a +ĠBL M +g irlfriend +b odied +6 26 +19 63 +Ġintermedi ary +Ġcons olation +M ask +ĠSi em +ow an +Beg inning +Ġfix me +Ġculmin ated +Ġcon duc +ĠVolunte er +Ġpos itional +Ġgre ets +ĠDefin itions +Ġthink er +Ġingen uity +Ġfresh men +ĠMom ents +Ġ35 7 +ate urs +ĠFed Ex +s g +69 4 +Ġdwind ling +ĠBO X +sel age +Ġt mp +Ġst en +ĠS ut +Ġneighbourhood s +Ġclass mate +f ledged +Ġleft ists +Ġclim ates +ATH ER +ĠScy the +ul iffe +Ġs ag +Ġho pped +ĠF t +ĠE ck +ĠC K +ĠDo omsday +k ids +Ġgas ped +Ġmon iker +ĠL od +ĠC FL +t ions +r ums +fol ios +Ġm d +Ġunc anny +Ġtrans ports +ĠLab rador +Ġrail ways +Ġappl iance +ĠCTR L +æ Ģ +Pop ulation +ĠConfeder acy +Ġunb earable +Ġdors al +ĠIn form +op ted +ĠK ILL +Mar x +Ġhypoc ritical +q us +ĠN umerous +ĠGeorg ian +ĠAmbro se +ĠL och +Ġgu bernatorial +ĠX eon +ĠSupp orts +ens er +ee ly +ĠAven ger +19 65 +Ar my +Ġju xtap +Ġcho pping +ĠSpl ash +ĠS ustainable +ĠFin ch +Ġ18 61 +ict ive +at meal +ĠG ohan +Ġlights aber +ĠG PA +ug u +ĠRE PL +vari able +Ġher pes +Ġdesert s +ac iously +Ġsitu ational +week ly +ob l +Ġtext ile +ĠCorn wall +Ġcontrace ptives +ĠA ke +] - +ä¹ ĭ +: , +ĠW em +ĠB ihar +Ġ' . +Ġbe re +Ġanal ogue +ĠCook ies +Ġtake off +Whe el +Ġmaj estic +Ġcomm uting +0 23 +ĠCor pse +ass ment +min i +Ġgor illa +ĠAl as +ere e +Ġacquaint ances +ĠAd vantage +Ġspirit ually +Ġey ed +pm wiki +ĠE nder +Ġtrans lucent +Ġnight time +ĠIM AGES +5 45 +ĠK amp +ĠFre ak +Ġ ig +Port land +4 32 +ĠM ata +Ġmar ines +Ġh ors +ater asu +ĠAtt ribution +Ġ-------- - +Ġk ins +ĠBEL OW +++ + +Ġre eling +ol ed +Ġcl utter +ĠRel ative +Ġ4 27 +B US +Ġa vert +ĠChe ong +ĠA ble +ĠPry or +Develop er +Ġen cyclopedia +ĠUSA F +ĠG arry +Sp ain +Bl ocks +Ġexp osition +ĠGamer Gate +W OR +Ġstockp ile +Ġclot hed +ĠT one +ĠR ue +t umblr +Ġtreacher ous +Ġf rying +Ñ Į +ĠS ph +Ġrest raints +Ġemb odies +ĠG es +S afety +Ġnegoti ators +min ing +ĠAppalach ian +L OS +ĠJenn a +Ġpass ers +ç ĭ +sn ap +Ġshort en +creat or +Ġinn umerable +uther land +67 4 +ĠW OM +ĠAs cend +ĠArm ory +ĠTrans action +K ick +Ġsuit case +day Name +Ġwaste ful +mar riage +ĠMcC abe +ite ch +ĠO ss +Cl osure +ĠTreasure r +Ġindec ent +ĠD ull +Ġresid ences +19 59 +ĠS ettlement +Ham ilton +Ġself ies +ĠRank ing +ĠBark ley +ĠB ore +ĠW CS +ĠMar itime +ĠH uh +ĠForest ry +Ġcultiv ating +ĠBall ard +Ġg arrison +ĠSD L +9 30 +Ġnas cent +Ġirresist ible +Ġaw fully +\/ \/ +Ġequ ate +Ġanthrop ology +ĠSylv ia +Ġintest ine +Ġinnoc uous +cess ive +ag ra +ĠMet roid +G rant +8 55 +ģ ĸ +Ġ" _ +ãĥĥ ãĥī +Ġappra isal +ĠFred dy +04 6 +Ġ40 6 +Ġ18 30 +Ġd ocking +St atic +Ġp ont +ĠVolt age +ĠSt ead +ĠMort gage +ĠJon ah +Y L +CLASS IFIED +Ġas bestos +nik ov +Ġcoll agen +ĠOrb ital +P ocket +7 99 +Ġhy brids +inc hes +Ġinv oice +und y +Ġinequ alities +T rend +w ashed +B ALL +Ġluc id +ĠComment ary +Ġw itty +Br andon +Ġbru ising +Ġ6 20 +es cent +box ing +P OL +Ġ3 78 +R ect +Ġlic ences +ĠMcG ee +p ressed +D anny +Ġj ammed +ord inate +Ġle th +Ġdistingu ishes +ĠYam aha +IL S +ĠH ume +ĠC ategories +Rober ts +Ch art +Ġbeet le +ĠGra veyard +Ġ($ ) +o ÄŁ +Ġtw ilight +are lla +á ½ +Ġbooth s +ĠH HS +ĠFeld man +Ġexcav ation +Ġphilosoph ies +at ography +ĠGar age +te chnology +Ġunfor gettable +Ġver ifying +Ġsubord inates +E ls +Ġne b +G aming +EN A +ĠAchieve ment +it ters +ĠG abe +Ġd umps +for cer +Ġpo ignant +ĠM BA +ĠHe idi +ime i +Ġm ages +Ġliber ate +Ġcircum cised +ĠMer maid +ĠMat th +t ogether +ĠW ichita +Ġstore front +ĠAd in +V II +Four th +Ġexplore rs +W ER +Not able +Bro ok +m ens +F aith +-------- - +ĠJ ou +¬ ¼ +Ġpine apple +Ġam alg +el n +ark able +ĠãĤµ ãĥ¼ãĥĨãĤ£ +ĠãĤµãĥ¼ãĥĨãĤ£ ãĥ¯ãĥ³ +Ġov arian +ĠE choes +Ġhairc ut +Ġp av +Ġch illed +anas ia +Ġsty led +Ġd ab +ni per +Ġminister ial +ĠD UP +T an +Ġsul ph +ĠD eter +ĠBo hem +od an +Ġeduc ator +â ĵĺ +sp ir +Ch icken +ĠE leanor +Ġqu i +Ġheav iest +Ġgrasp ed +U RA +Ġcro oked +Jess ica +pro blem +Ġpred etermined +Ġman iac +Ġbreath s +ĠLauder dale +Ġh obbies +y z +Cr ime +Ġcharism a +d L +Ġle aping +Ġk ittens +Ang elo +ĠJ ACK +ĠSu zanne +Ġhal ting +ENT ION +Ġswall owing +ĠEarthqu ake +Ġeight eenth +ĠN IC +ĠIN F +ĠCons cious +Ġparticular s +circ le +7 40 +Ġbene volent +Ġ7 47 +Ġ4 90 +Ġr undown +ĠVal erie +ĠB UR +Ġcivil isation +ĠS chn +W B +ot ide +intern ational +Ġj ohn +Ġ19 02 +Ġpe anuts +Ġflav ored +k us +Ġro ared +Ġcut off +é £ +Ġorn ament +Ġarchitect ures +Ġ3 69 +ol or +ĠWild e +ĠC RC +ĠAdjust ed +Ġprov oking +land ish +Ġrational ity +Ġjust ifies +Ġdisp el +Ġa meric +ĠPol es +Ø © +Ġen vis +ĠD oodle +ä½ ¿ +igs aw +auld ron +Techn ical +T een +up hem +ĠX iang +Ġdetract ors +ĠZ i +ĠJournal ists +Ġconduc ive +ĠVolunte ers +Ġs d +Know ing +Ġtrans missions +ĠPL AN +ĠL IB +Ġall uded +Ġob e +Ġd ope +ĠGold stein +Ġwavelength s +ĠDest ination +nd a +ug i +Ġattent ive +ĠLe an +ral tar +Ġman g +mb uds +ak ings +b ender +Ġacc ol +Ġcraw led +N OW +Min nesota +Ġflour ished +ĠZ up +ĠSuper visor +ĠOliv ier +Ex cellent +Ġwid en +D one +Ġw ig +Ġmiscon ceptions +Cor p +W an +Ġvener able +ĠNot ably +ĠKling on +an imate +Bo ost +ĠS AY +miss ing +ibli ography +mel on +Ġpay day +Ø ³ +bo le +Ġve iled +ĠAl phabet +It alian +Ġever lasting +ĠR IS +ĠC ree +rom pt +Ġh ating +Ġgrin ning +Ġge ographically +OS H +Ġwe eping +ĠÂłĠÂłĠÂłĠÂł ĠÂłĠÂłĠÂłĠÂł +Ġimpe cc +Let ter +Ġblo ated +PL A +ĠFe in +Ġper sever +Th under +Ġa ur +ĠR L +Ġpit falls +âĸ º +Ġpredomin ant +Ġ5 25 +7 18 +AP E +7 14 +Ġfarm land +ĠQ iao +Ġv iolet +ĠBah amas +Ġinflic ting +ĠE fficiency +Ġhome brew +Ġundert ook +Ġcur ly +ĠHard ing +man ia +59 6 +Ġtem pered +Ġhar rowing +ĠP ledge +ĠFranken stein +è ª +M otion +Ġpredict ably +ĠExpl osion +oc using +er d +col o +FF ER +Ġback field +ĠV IDE +ue bl +N arr +ĠArg ument +Ġgen omic +Ġbout ique +Ġbatt ed +ĠB inary +Ġg amb +ĠRh ythm +67 3 +Ġa float +ĠOlymp ia +Y ING +Ġend if +is in +Ġwin ters +Ġsc attering +I v +D istance +Ġtr u +ĠCom fort +Ġne xus +Ġair flow +ĠByz antine +p ayers +con i +ĠB etsy +D eal +ĠN ug +ĠContin ent +red ibly +Ġoptim izing +al beit +Ġec static +ĠPro to +ç · +iv ot +âĸ Ħ +em p +rou nder +Ġcl out +ĠI ST +66 3 +ĠDoll ars +ĠD AC +Ġsubsc ribed +Ġrehears al +Ġam ps +ĠSh ang +es m +Ġspr inkle +Ġassail ant +ĠO o +ĠCoin base +T act +Ġret ina +Ġn uns +R ON +att o +Ġj ug +ĠSV G +Ġb ikini +ĠFI LE +ĠFound ers +ep ort +ĠK P +Ġrest ores +ĠTh ick +Ġash ore +Ġappro vals +R ender +M AG +G raham +ĠCort ana +ãĥ³ ãĤ¸ +ss h +or ians +ars ity +ĠInsp ired +u pper +Ġsign alling +Ġreb uke +Ġfl ares +Ġdownt ime +Stud ies +Ġstagn ation +ĠSequ ence +Ġgr unt +Ġass ures +ĠPL A +59 2 +Ġintra ven +d epend +Sus an +ĠManz iel +Man ia +Cont ract +Ġsl ams +Ġcult ured +Ġcred itor +L IST +ĠH UM +ĠChatt anooga +serv ed +Ġclo aked +ĠF TP +p owder +ĠSt ella +uct ive +Ġcheap ly +ĠMU CH +ĠGalile o +Ġsu ites +spe ech +Ġdeliber ations +ĠCh ips +« ĺ +Bal ance +ĠWyn ne +ĠAk ron +Ass et +Ġhon oured +Ġed ged +Like wise +anim ous +ĠW age +ĠEz ek +ad vertisement +ĠRT X +ĠM AD +Ġmigr ating +ĠS QU +Ġ4 75 +Ed ited +Ġshorth and +ĠBas ics +Ġcro tch +ĠEV EN +Ġv m +effic iency +Ġcal ves +ĠF rie +ĠBrill iant +Ġstri kers +Ġrepent ance +Ġarter ies +r l +B ed +h ap +Ġcrypt ography +ĠSab res +Ġ4 14 +vi ks +ih ara +aps es +T alking +Ġintertw ined +Ġdoc ks +Ġalle le +ĠArt ifact +ĠH IM +t orn +ç ķ +Ġop acity +ĠE ly +os uke +Ġn ipple +Ġhand written +ĠV K +ĠChamber lain +ĠLa os +ig raph +g row +Ġtr illions +Ġdescend ant +ĠSail or +as uring +Ġce ilings +ĠWare house +f lying +ĠGl ow +Ġn ont +Ġmiscar riage +Ġrig s +Ġmin istries +Ġelabor ated +Ġdel usional +ĠHum ane +Ġ3 79 +n ets +Ġblack out +add ers +Ġn p +ĠT ire +ro sc +Ġsub div +Ġlink age +Ġchron ological +ĠHER O +Ġres ettlement +ĠVin yl +Ġpast oral +ĠMob il +ĠBar bar +Co oldown +ĠF ritz +c riminal +re pe +Ġbell ig +ĠBre ed +Ġ4 18 +Ġsem blance +ij k +Ġcur tail +Ġclin ch +cont ained +ĠProm pt +ast on +Ġw i +Ġpursu its +5 15 +ĠGl oss +Ġfl ips +Ġcoup ons +Ġcl oning +ĠLike ly +Rem oved +ĠQu artz +r ices +ĠSpe ars +Ġp ious +Ġdep reciation +ĠD are +oun ces +am az +O nt +Ġp innacle +d ocker +0 26 +ĠW yr +ĠPro per +Ë Ī +n il +By tes +Ġseek er +t rial +Ġunf olds +ĠMar se +Ġextravag ant +ĠSurviv ors +RED ACTED +ĠSpeed way +ĠCra igslist +sub mit +ĠGener ations +Ġup holding +Ġblood stream +ĠMiss ions +ĠL awn +Ġlim bo +ene i +H uh +ĠWild cats +pre p +ĠMark us +ĠFor bidden +rit ic +IN O +Ġexhib iting +requ ent +ch uk +Ġhabit ual +ĠComp atibility +Dr ag +RIP T +uj ah +GR OUND +Ġdelinqu ent +Ġburn er +Ġcontempor aries +Ġgimm ick +load s +Ġno zzle +p odcast +ĠW ak +ĠStat en +ĠK uh +ãģ ĵ +inter rupted +Ġinv incible +ĠBurn ett +cig arette +ĠPeb ble +ĠTem porary +ĠMar ino +58 2 +Ġwast eland +ident ly +T x +Ġr ite +ĠPan asonic +ĠM iddles +ĠHort on +ae us +Ġc uring +Ġm ats +Ġadj ourn +Ġfears ome +pe z +bo ats +Ġpro pell +Ġconflic ted +ĠAng er +Ġinsurg ent +K arl +Ġco ales +Ġsouth western +Ġdis su +ĠO vert +******** **** +Ġbox ed +ĠBr une +aa a +Ġgard ening +ĠEng el +tr acks +Ġpur ified +Ġplace holder +ĠL ikes +Ġd an +G ab +Ġe ct +ĠF aw +ĠEl iot +Ġ' , +otrop ic +ĠRu in +hed on +Ġca ul +Ġa ft +ĠCad illac +gh a +ass ian +ud eb +ĠT ick +Ġadjust s +AR GET +5 37 +isc he +ant y +ĠFried rich +ĠBl izz +ĠA OL +Camp aign +Ġmamm al +ĠVe il +ĠK ev +ĠMaur it +ĠDam ien +N ation +E astern +Ġ{ : +Ġ= ================================ +Ġstereotyp ical +Ġatt ic +ĠCy borg +requ ire +Ġaward ing +ĠPap ua +bt n +b ent +B oo +Ġ( = +ĠX ander +ĠSomers et +Ġcatch y +Ġcert ify +STR UCT +Ġit al +Ġt ides +ĠBr ands +G ray +comp etitive +Ġcur ator +ĠD G +omin ium +ĠGM Os +ci ating +ĠCarm en +ow ard +Balt imore +Ġr gb +C u +Ġwip es +spe ll +IT NESS +Ġsummar izes +ĠRe vis +Ġwhistlebl owers +ĠBre ach +Ġcro chet +k os +ews ki +Ġrep et +Ġcrim son +ĠKar achi +read able +dim ension +ĠI gor +ild ed +ĠZ ed +ĠKe ane +ĠCos metic +DE P +Ġretreat ing +ĠU A +ens ical +Ġd usk +ĠDick ens +Ġaren as +ĠPass age +level s +Ġcur v +P ope +Ġch ores +ĠEl ise +ĠComp ass +b ub +Ġmamm alian +ĠSans krit +ĠAN C +ĠCr ack +Q ual +L aun +amp unk +Ġlearn ers +Ġglam orous +Ġfur the +erm ott +c and +Gener ic +Ġnarr ated +Ġdisorder ly +ĠTrans actions +ĠDet ention +ĠR oku +Ä į +Ġunder statement +ĠS aur +ĠRodrig o +ĠAS AP +S in +Ġre joice +Method s +Ġelectro de +Ġworsh ipped +Ġid i +ĠPhys icians +Ġpop up +Ġde ft +ĠRem oval +ĠBu enos +ver bs +Ġfun k +ush a +rict ion +ore a +ĠBang alore +ĠKen obi +zz i +Ġnorm ative +Ġgobl ins +Ġcaf es +ĠUN CLASSIFIED +ĠF ired +S IGN +Ġs clerosis +ĠV oter +ĠSon ny +ĠExt end +ĠEV s +Ar senal +Ġp si +Ġwid est +ĠT us +Ġlo oms +Ġjust ifying +ĠGr anger +è ¯ +Ref er +58 3 +Ġflour ishing +ab re +Ġr ave +ĠCont ra +Ġ18 98 +Add s +Ġf ul +ĠCo oke +some one += # +67 1 +Ġy ak +Ġar te +ĠMis cellaneous +ĠDet ection +ĠCl ancy +â ģ +ass ies +Ġval iant +ĠFemin ist +cor ruption +V el +P ear +Ġsucc inct +Ġquick est +k w +Ġsp itting +ĠL ibraries +åħ ī +ant z +D ad +ĠSpec ifications +rup ulous +and r +RES ULTS +Ġsnow ball +Ġpred is +ĠB axter +ĠNurs ing +ĠCh aff +s we +Ġout age +Ġnest ing +Ġnotor iety +tr igger +on ite +j on +Ġf ou +ook ed +ĠCelebr ity +re ality +Ġfat ig +Ġhug ging +Ġbother s +ĠPan zer +ĠCh andra +fig ured +Ġvol ts +ĠCloud s +Ġfee ble +ĠCur ve +ĠAs us +78 6 +abs or +ĠV ICE +ĠH ess +Ġmanufact ures +Ġgri zz +ĠPower ful +ac id +Ġsub sections +ĠKrug man +ĠAl ps +is u +Ġsequ est +ĠUlt ron +ĠT inker +ĠGo ose +Ġmism atch +Att orney +Ġmorph ology +ĠSix ers +ut tered +ĠE LECT +gr an +Rus sell +ĠG SL +Ġfort night +Ġ. ) +Ġapost le +pr one +el ist +Unt itled +ĠIm plementation +ist ors +Ġtank er +Ġpl ush +Ġattend ants +ĠT ik +ĠGreen wich +ĠY on +ĠSP L +cell s +unt led +S olution +ĠQu é +Ġvac ated +Ġupt ick +ĠMer idian +æ ĥ +ĠDr ill +9 25 +58 4 +Ġrenov ated +ĠKub rick +zy k +Ġl ousy +pp el +ohyd rate +ĠI zzy +lesi astical +CC C +ĠAj ax +Ġad apters +ĠPetra eus +Ġaffirm ation +ĠST OR +le ms +ad oes +ĠConstantin ople +Ġp onies +Ġl ighthouse +Ġadherent s +ĠBre es +omorph ic +Fight ing +Ġpl aster +ĠP VC +ĠOb st +Ġdear ly +ĠTo oth +icks on +Ġsh aming +P lex +A gg +Ġâ̦ " +Ġsub reddits +Ġpige on +ĠResident ial +ĠPass ing +Ġl um +ĠP ension +Ġpessim istic +Ġ4 32 +z inski +c ade +0 75 +Ġapolog ised +iy ah +Put ting +Ġgloom y +ĠLy me +=-=-=-=- =-=-=-=- +ĠT ome +ĠPsych iatric +ĠH IT +c ms +ap olog +Ġbreak er +Ġdeep en +Ġtheor ist +ĠHigh lands +Ġb aker +Ġst aples +Ġinterf ered +ĠAb ortion +jo ined +ch u +Ġform ulate +Ġvacc inations +Ġban ter +phe us +Ġoutfield er +ĠM eter +Ġ# #### +Ġ18 95 +Ġnarrow ing +ĠST ORY +f p +ĠC ST +ign ore +Ġproclaim ing +ĠR U +ĠB ALL +yn a +65 3 +Ġpos it +P RE +59 4 +ĠRegist rar +ĠPil grim +ic io +Ġpre tt +Ġlif eless +Ġ__ _ +Ne igh +ĠCh urches +orn o +Ġor cs +Ġkind red +ĠAud it +Ġmillenn ial +ĠPers ia +g ravity +ĠDis ability +ĠD ARK +W s +od on +Ġgrand daughter +ĠBro oke +ĠA DA +ER A +Ġpick ups +ĠWil kinson +ĠSh ards +ĠN K +Ġexp el +ĠKis lyak +Ġj argon +Ġpolar ized +ian e +Pub lisher +Ġreb utt +Ġapprehens ion +ĠK essler +Ġpr ism +F UL +19 64 +ĠL oll +ä ¿ +le thal +Å Ł +Ġg hetto +Ġb oulder +ĠSlow ly +ĠOsc ars +ĠInst ruction +ĠUl tr +ĠM oe +N ich +ĠP ATH +( * +ĠRE LEASE +un ing +rou se +en eg +Ġre imb +ĠDet ected +Do S +Ġster ling +Ġaggreg ation +ĠLone ly +ĠAtt end +hig her +Ġairst rike +ks on +SE LECT +Ġdef lation +ĠHer rera +C ole +rit ch +Ġadvis able +F ax +Ġwork around +Ġp id +mort em +ers en +Ġtyp o +Ġal um +78 2 +ĠJam al +script s +Ġcapt ives +ĠPres ence +ĠLie berman +angel o +Ġalcohol ism +ass i +Ġrec ite +Ġgap ing +Ġbask ets +ĠG ou +Brow ser +ne au +Ġcorrect ive +und a +sc oring +ĠX D +Ġfil ament +Ġdeep ening +ĠStain less +Int eger +Ġbu ggy +Ġten ancy +ĠMub arak +Ġt uple +ĠD roid +ĠS itting +Ġforfe it +ĠRasm ussen +ixt ies +es i +ĠKim mel +Ġmetic ulously +Ġap opt +ĠS eller +08 8 +ec ake +hem atically +T N +Ġmind less +Ġdig s +ĠAcc ord +ons ense +em ing +br ace +Ġe Book +ĠDist ribut +ĠInvest ments +w t +] ), +beh avior +56 3 +Ġbl inding +ĠPro testers +top ia +Ġreb orn +ĠKel vin +ĠDo ver +ĠD airy +ĠOut s +Ġ[ / +Ï Ģ +b p +ĠVan ity +ĠRec ap +ĠHOU SE +ĠF ACE +Ġ4 22 +69 2 +ĠAnt ioch +cook ed +Ġcoll ide +Ġa pr +Ġsle eper +ĠJar vis +Ġalternative ly +ĠLe aves +ĠM aw +Ġantiqu ity +ĠAdin ida +Ġab user +Poké mon +Ġass orted +ĠRev ision +ĠP iano +ĠG ideon +O cean +Ġsal on +Ġbust ling +ogn itive +ĠRah man +Ġwa iter +Ġpres ets +ĠO sh +ĠG HC +oper ator +Ġrept iles +Ġ4 13 +ĠG arr +ĠCh ak +Ġhas hes +Ġfail ings +Ġfolk lore +Ġab l +ĠC ena +ĠMac Arthur +ĠCOUR T +Ġperipher y +app ers +Ġreck oned +ĠInf lu +ĠC ET +Ġ3 72 +ĠDefin itive +ass ault +4 21 +Ġreservoir s +Ġd ives +ĠCo il +DA Q +Ġvivid ly +ĠR J +ĠBel lev +Ġec lectic +ĠShow down +ĠK M +ip ed +reet ings +ĠAs uka +L iberal +ĠÏ Ħ +Ġbystand ers +ĠGood win +uk ong +S it +ĠT rem +Ġcrim inally +ĠCirc us +ch rome +88 7 +Ġnan op +ĠOb i +ĠL OW +o gh +ĠAuth ors +ob yl +Ur ban +Ġt i +ĠWe ir +t rap +ag y +Ġparent heses +Ġout numbered +Ġcounter productive +ĠTob ias +ub is +P arser +ST AR +Ġsyn aptic +ĠG ears +Ġh iber +Ġdebunk ed +Ġex alted +aw atts +H OU +Ch urch +ĠPix ie +ĠU ri +ĠForm ation +ĠPred iction +C EO +Ġthro tt +ĠBrit ann +ĠMad agascar +ë ĭ +Ġbill boards +ĠRPG s +ĠBe es +complete ly +F IL +Ġdoes nt +ĠGreen berg +re ys +Ġsl ing +Ġempt ied +ĠPix ar +ĠDh arma +l uck +ingu ished +Ġend ot +Ġbab ys +05 9 +che st +r ats +Ġr idden +Ġbeet les +Ġillum inating +Ġfict itious +ĠProv incial +Ġ7 68 +Ġshe pherd +ĠR ender +Ġ18 96 +C rew +Ġmold ed +ĠXia omi +ĠSp iral +Ġdel im +Ġorgan ising +Ġho ops +ĠBe i +z hen +Ġfuck in +Ġdec ad +Ġun biased +am my +sw ing +Ġsmugg led +Ġk ios +ĠP ERSON +ĠInquis itor +Ġsnow y +Ġscrap ing +ĠBurg ess +P tr +ag ame +R W +Ġdro id +ĠL ys +ĠCass andra +Jac ob +Ġ35 4 +Ġpast ure +Ġfr anc +ĠScot ch +ĠEnd s +ĠI GF +def inition +Ġhyster ical +ĠBrown e +77 1 +Ġmobil ization +æ ķ +iqu eness +Th or +Ġspear headed +Ġembro iled +Ġconject ure +jud icial +Ch oice +Ġpaper back +P ir +Ġrec overs +ĠSur ge +ĠSh ogun +ĠPed iatrics +ãģ ł +Ġsweep s +ĠLabor atories +ĠP acks +al us +add in +Ġhead lights +g ra +Ev idence +COL OR +Ad min +Ĭ ± +Ġconco ct +s ufficient +Ġun marked +Ġrich ness +Ġdiss ertation +Ġseason ing +Ġg ib +ĠM ages +un ctions +ĠN id +che at +ĠTM Z +c itizens +ĠCatholic ism +n b +Ġdisemb ark +ĠPROG RAM +a ques +Ty ler +Or g +ĠSl ay +ĠN ero +ĠTown send +IN TON +te le +Ġmes mer +9 01 +Ġfire ball +ev idence +aff iliated +ĠFrench man +ĠAugust a +0 21 +Ġs led +Ġre used +ĠImmun ity +Ġwrest le +assemb led +Mar ia +Ġgun shots +ĠBarb ie +Ġcannabin oids +ĠTo ast +ĠK inder +IR D +Ġre juven +Ġg ore +Ġrupt ure +Ġbre aching +ĠCart oon +Ġ4 55 +ĠPale o +6 14 +Ġspe ars +ĠAm es +ab us +Mad ison +GR OUP +Ġab orted +y ah +Ġfel on +Ġcaus ation +Ġprep aid +Ġp itted +op lan +ĠShel ley +ĠRus so +ĠP agan +Ġwill fully +ĠCan aver +und rum +ĠSal ary +ĠAr paio +read er +ĠR ational +ĠOver se +ĠCa uses +Ġ* . +Ġw ob +Ke ith +ĠCons ent +man ac +77 3 +6 23 +Ġfate ful +et imes +Ġspir ited +ĠD ys +Ġhe gemony +Ġboy cot +ĠEn rique +em outh +Ġtim elines +ĠSah ara +ĠRel ax +ĠQuin cy +ĠLess ons +ĠE QU +SE A +N K +ĠCost co +Incre ase +Ġmotiv ating +ĠCh ong +am aru +ĠDiv ide +Ġped igree +ĠTasman ia +ĠPrel ude +L as +9 40 +57 4 +Ġch au +ĠSp iegel +un ic +-- > +ĠPhil ips +ĠKaf ka +Ġuphe aval +Ġsent imental +Ġsa x +ĠAk ira +ser ial +Mat rix +Ġelect ing +Ġcomment er +ĠNeb ula +ple ts +ĠNad u +ĠAd ren +Ġen shr +ĠR AND +fin ancial +ĠCly de +uther ford +Ġsign age +Ġde line +Ġphosph ate +rovers ial +f ascist +ĠV all +ĠBeth lehem +Ġfor s +Ġeng lish +S olid +N ature +Ġv a +ĠGu ests +Ġtant al +Ġauto immune +;;;;;;;; ;;;; +ĠTot ally +ĠO v +Ġdef ences +ĠCoc onut +Ġtranqu il +Ġpl oy +Ġflav ours +ĠFl ask +ãĤ¨ ãĥ« +ĠWest on +ĠVol vo +8 70 +Ġmicro phones +ver bal +R PG +Ġi ii +; } +0 28 +Ġhead lined +Ġprim ed +Ġho ard +ĠSh ad +ĠEN TER +Ġtri angular +Ġcap it +l ik +ĠAn cients +Ġl ash +Ġconv ol +Ġcolon el +en emy +G ra +Ġpub s +ut ters +Ġassign s +ĠPen et +ĠMon strous +ĠBow en +il ver +H aunted +ĠD ing +start ed +pl in +Ġcontamin ants +ĠDO E +ff en +ĠTechn ician +R y +Ġrob bers +Ġhot line +ĠGuard iola +ĠKau fman +row er +ĠDres den +ĠAl pine +E lf +Ġf mt +ĠS ard +urs es +g pu +Un ix +Ġunequiv ocally +ĠCitizens hip +qu ad +m ire +ĠS weeney +B attery +6 15 +Ġpanc akes +Ġo ats +M aps +ĠCont rast +mbuds man +ĠE PS +Ġsub committee +Ġsour cing +Ġs izing +ĠBuff er +ĠMand atory +Ġmoder ates +ĠPattern s +ĠCh ocobo +ĠZ an +ĠSTAT ES +ĠJud ging +ĠIn her +* : +Ġb il +ĠY en +Ġexh ilar +oll ower +z ers +Ġsn ug +max imum +Ġdesp icable +ĠP ACK +ĠAn nex +Ġsarcast ic +Ġlate x +Ġt amp +ĠS ao +b ah +ĠRe verend +ĠChin atown +ĠA UT +d ocumented +ĠGA BA +ĠCan aan +ĠÙ ħ +Ġgovern s +pre v +E sc +ĠEst imates +OS P +Ġendeav our +ĠCl osing +omet ime +every one +Ġwor sen +Ġsc anners +Ġdev iations +ĠRobot ics +ĠCom pton +Ġsorce rer +Ġend ogenous +Ġem ulation +ĠPier cing +ĠA ph +ĠS ocket +Ġb ould +ĠO U +ĠBorder lands +Ġ18 63 +G ordon +ĠW TO +Ġrestrict s +Ġmosa ic +Ġmel odies +ç Ħ +T ar +Ġdis son +ĠProv ides +Ġ ...... +b ek +F IX +Ġbro om +ans hip +Do ctors +Ġner ds +ĠReg ions +na issance +Ġmet e +Ġcre pt +pl ings +Ġgirlfriend s +kn it +ig ent +ow e +Ġus hered +ĠB az +M obil +4 34 +ĠPres ents +orig in +Ġins omnia +ĠA ux +4 39 +ĠCh ili +irs ch +G AME +Ġgest ation +alg ia +rom ising +$ , +c row +ĠIn spection +at omic +Rel ations +J OHN +rom an +ĠClock work +ĠBak r +m one +M ET +Ġthirst y +Ġb c +Ġfacult ies +R um +Ġnu ance +ĠD arius +ple ting +fter s +etch up +Reg istration +ĠK E +R ah +Ġpref erential +ĠL ash +ĠH H +Val id +ĠN AV +Ġstar ve +ĠG ong +z ynski +ĠAct ress +Ġw ik +Ġun accompanied +lv l +Br ide +AD S +ĠCommand o +ĠVaugh n +Wal let +Ġho pping +ĠV ie +Ġcave ats +Ġal as +if led +ab use +66 1 +Ġib n +Ġg ul +Ġrob bing +t il +IL A +Ġmit igating +Ġapt ly +Ġty rant +Ġmid day +ĠGil more +ĠDe cker +Ġ§ § +part ial +Ex actly +Ġphen otype +Ġ[+ ] +ĠP lex +ĠI ps +vers ions +Ġe book +Ġch ic +g ross +":" "},{" +ĠSur prisingly +M organ +Ġresid ues +ĠConf ederation +in feld +Ġl yr +mod erate +Ġperpend icular +V K +Ġsynchron ized +Ġrefres hed +Ġad ore +ĠTor ment +ol ina +Ġ26 00 +Item Tracker +Ġp ies +ĠF AT +ĠR HP +0 48 +ĠRES P +ĠB J +all ows +P and +Ġunw elcome +ĠV oc +ĠBast ard +ĠO W +ĠL AR +ĠHeal er +Environment al +ĠKen yan +ĠTr ance +ĠP ats +Ġali ases +ĠGar field +Ġcampaign er +Ġadvance ments +ĠOkin awa +ĠC oh +ows ky +Ġstar ved +Ġsize able +Ġ: -) +Ġm RNA +Ġsusp ensions +ist ar +Scot land +Pr in +-------------------------------- ---------------- +Ġ50 2 +Ġteasp oons +Ġ10 50 +Ġcoerc ive +ĠMason ic +edd ed +ĠPass enger +Ġl att +Ġbr aces +ĠSt eal +ĠNY T +ĠK ats +ĠCel est +ae z +T u +ĠCoul ter +ðŁ ĺ +Fl ickr +ĠWil mington +ith s +++ ; +Ġv ending +Ġneg ro +ĠPh i +ĠYellow stone +Call back +Ġsh ampoo +ĠSh ades +w at +Ġsuper human +Ġridic uled +Ġhol iest +om bo +Ġintern s +Ġh one +ĠPar agu +UR I +Ġd angling +ãĤ » +so v +ict ional +av ailability +Ġrev ocation +Ġd ow +in ic +ĠTHE IR +Ġis o +Ġout ings +ĠLeth al +Ġ) )) +Ġinacc ur +Ġout landish +Ġan us +let ico +id on +l ol +Ġun regulated +Ġsuccumb ed +Ġc uff +ĠWast eland +let al +Ġsub str +Ġcoff ers +Ġautom akers +ov i +ĠX ue +ĠDayton a +Ġjar ring +Ġf umes +Ġdisband ed +z ik +itt on +Ġstriking ly +Ġsp ores +Ad apter +.) : +ĠLynd on +ival ry +Ġor ally +Ġtumult uous +Ġdisple asure +Ġcon es +or rect +Ġappe ase +Ġder by +ĠTrip oli +ĠAl ess +Ġp oked +ĠGu ilty +v P +En ough +Ġorig inals +6 99 +Ġrabb i +Ġproverb ial +Ġpostp one +el ope +ĠMist y +Ġstaff ed +ĠUn employment +redit ary +Ġdilig ent +re comm +me asures +as in +8 25 +Ġpond s +Ġmm ol +ĠS AR +ĠC ARE +Ġ3 71 +Ġclen ched +ĠCors air +Ġcaric ature +z n +att ach +ĠSch ro +spe ak +p ainted +ĠS uc +ĠE NT +Ġcell ul +ĠP aid +di agn +WH ERE +Ġtext ed +B arn +Ġret racted +ĠRe ferred +S av +Ġup keep +Ġwork places +ĠTok ens +Ġampl ify +cl inical +Ġmult ic +mber g +Ġconvol uted +Reg ion +5 65 +ĠTop ic +Ġsn ail +Ġsal ine +Ġins urrection +ĠPet r +f orts +B AT +ĠNav ajo +Ġrud imentary +ĠLak sh +OND ON +Me asure +Ġtransform er +ĠGodd ard +Ġcoinc ides +ir in +R ex +ĠB ok +qu it +Ġshotgun s +Ġprolet arian +Ġsc orp +ĠAd a +5 14 +Ġsl ander +record ed +Ġemb ell +ris ome +Ġapolog izing +ĠMul cair +ĠGib raltar +Cl a +Ġall ot +ĠAtt ention +Ġ4 33 +le ave +Ġwh ine +ĠIss a +ĠFa ust +ĠBar ron +hen y +Ġvictim ized +J ews +Ġnurt uring +ett el +W inged +ĠSub tle +Ġflavor ful +ĠRep s +eng ed +call back +Ġdirection al +Ġcl asp +ĠDirect ions +plan et +icult ure +Hel per +ic ion +ac ia +Ġç ¥ŀ +Ġsur ges +Ġcan oe +ĠPrem iership +be en +Ġdef ied +ĠTro oper +Ġtrip od +Ġgas p +ĠE uph +ĠAd s +vern ight +high ly +R ole +Ġent angled +ĠZe it +6 18 +ĠRust y +Ġhaven s +ĠVaugh an +HA EL +ĠSER VICE +/ , +Ġstr icken +Ġdel usions +Ġb is +ĠH af +Ġgrat ification +Ġent icing +UN CH +Ad ams +ĠOL ED +ĠBeet le +Ġ18 99 +ĠSO FTWARE +ateg or +V L +ĠTot em +ĠG ators +AT URES +Ġimped ance +Reg istered +ĠC ary +ĠAer ial +on ne +en ium +Ġd red +ĠBe g +Ġconcurrent ly +Ġsuper power +ĠX an +j ew +imes ter +ĠDick inson +âĶ ģ +F la +Ġp ree +ĠRoll ins +© ¶æ +Ġden omination +ĠL ana +5 16 +Ġinc iting +sc ribed +j uries +ĠWond ers +app roximately +Ġsusp ending +Ġmountain ous +ĠL augh +oid al +N s +Det ect +) = +ĠL uthor +ĠSchwarz enegger +ĠMull er +ĠDev i +ec ycle +J ar +6 13 +ĠL ongh +B ah +ĠSP ORTS +n w +Ġref inement +Ġwater ways +Ġd iner +Bl ade +68 3 +F ac +Ġinitial s +Ġro g +Ġparan ormal +B UT +Ġ[ ( +ĠSw anson +ĠM esh +âĸ ¬ +Impro ve +ĠRad iation +ĠEst her +ĠE sk +ĠA ly +ik y +Ġir rad +ĠBuck ingham +Ġref ill +Ġ. _ +Re pe +CON CLUS +Ġdifferent iated +Ġchi rop +ĠAt kins +Pat tern +Ġexc ise +Ġcab al +N SA +ĠST A +ĠS IL +ĠPar aly +Ġr ye +ĠHow ell +ĠCount down +ness es +alys ed +Ġres ize +ãĤ ½ +Ġbudget ary +ĠStr as +w ang +Ġap iece +Ġprecinct s +Ġpe ach +Ġsky line +Ġ35 3 +pop ular +App earances +ĠMechan ics +ĠDev Online +S ullivan +Z en +Ġp u +op olis +5 44 +Ġde form +Ġcounter act +ĠL ange +Ġ4 17 +Con sole +77 4 +Ġnodd ing +Ġpopul ism +Ġhe p +Ġcoun selling +compl iance +U FF +Ġunden iably +Ġrail ing +ĠHor owitz +ĠSim one +ĠBung ie +Ġa k +ĠTal ks +x ff +fl ake +Cr ash +Ġsweat y +Ġban quet +ĠOFF IC +Ġinvent ive +Ġastron omer +ĠStam ford +ĠSc are +ĠGRE EN +olic ited +Ġr usher +Ġcent rist +ight ing +Ġsub class +Ġdis av +Ġdef und +ĠN anto +oci ate +m ast +Ġpac if +Ġm end +e ers +imm igration +ESS ION +Ġnumber ing +Ġlaugh able +ĠEnd ed +v iation +em ark +P itt +Ġmetic ulous +ĠL F +Ġcongrat ulated +ĠBir ch +Ġsway ed +Ġsemif inals +Ġhum ankind +m atter +ĠEqu ip +opa usal +S aid +ĠLay out +Ġvo icing +Ġth ug +Ġporn ographic +I PS +Ġmo aning +Ġgriev ance +Ġconf essions +esc al +TEXT URE +Aut hent +os aurus +P urchase +Ġreleg ation +al ter +ĠÂł Âł +Ġr iddled +Ġo gre +ĠLow ell +Occ up +E at +ĠHy der +ĠAdvis er +Com merce +H unt +ĠOr th +ĠComp etitive +ĠCL A +CD C +Ġsal ads +F le +Ġindustrial ized +` , +ĠO WN +Ġbec k +ĠPart icularly +oub t +Ġm M +ĠHuss ain +ĠChen nai +Ġ9 20 +Ġappoint ing +ĠCull en +,,,, ,,,, +Ġp ores +ver ified +Ġbi ochemical +em ate +Ġcoward ly +ĠHels inki +ĠEthiop ian +S OURCE +ER C +est ro +Ġbi otech +ĠS our +Ġbrew er +Bloom berg +Ġintens ify +Gl ass +an co +ĠF DR +gre SQL +ĠF ires +©¶æ ¥µ +ec o +100 1 +ĠHom eless +Ġinstant aneous +ĠH aste +ig el +D iamond +Ġp aving +Ġland fill +Ġd ads +h oun +: ] +Ġinc endiary +ĠLiving ston +ĠHil bert +ĠChe cks +st yles +in ators +ĠCl ive +ph rine +Ġchimpan zees +Ġp all +ĠJ M +ĠAad haar +ð Ŀ +Ġachie vable +dis abled +P ET +OOOO OOOO +M ot +Ġint angible +Ġbal let +ĠWe bs +ĠEst imated +Effect s +Ġb ailed +Josh ua +Ġturb ulence +Ġoccup ant +ĠDay light +Ġ36 1 +me et +Ġstat ically +Ġon look +Ġk i +il legal +Ġvel vet +Ġdehyd ration +Ġacqu ies +ĠRe z +ak ura +ĠU pton +at ro +Ġincomp rehensible +Ġback door +ĠRh ino +7 27 +Ġmath s +) + +Ġhe resy +Ġd f +ĠRoc he +ĠL ydia +Ġpanc reat +re ply +arre ll +Ġsolicit ation +Ġcirc adian +BI P +Ġfor ay +Ġcrypt ic +iz u +ime o +ĠTom ato +ĠH oms +ex amination +Ġqu arry +ĠVal iant +ĠJer icho +ĠIN CLUD +Ġ18 40 +5 19 +Ġres ists +Ġsnap shots +ĠSp ur +ĠAnt iqu +Log in +Ġbest selling +Ġant ic +ĠS utherland +ãĤ¢ ãĥ« +Ġ~ / +ĠP arm +è ĥ +P ages +int ensity +Ġimm obil +Ġ18 65 +zz o +Ġn ifty +Ġf entanyl +ĠPres ervation +op hen +Ġd arts +ĠD inosaur +po inters +ĠR ite +s uggest +aware ness +ĠSher idan +Ġst ances +Ġsor cery +Ġper jury +ĠNik ola +ie ver +Ġf iance +ĠJordan ian +ĠBall oon +Ġn ab +Ġk b +Ġhuman ities +ĠTan aka +hill ary +Ġconsult ancy +ĠZ ub +Ġrem ission +Ġconf id +CH Q +ĠF ug +Ġimpro vis +Y ep +/ _ +Ġunwilling ness +Ġport folios +05 5 +ĠInstruct or +aim an +Ġclaim ants +M bps +ĠBy e +re ceived +T weet +Ġind emn +ri z +am ara +N at +Ġeval uates +ĠL ur +ep ad +FO X +ĠTh ro +Ġrust y +Ġbed rock +ĠOp rah +J B +Ġmanip ulative +Ġwill ful +Ġrel apse +Ġext ant +The me +S ensor +ĠSt ability +go vern +Ġpo ppy +Ġkn ack +Ġins ulated +ĠT ile +ĠExt rem +Ġunt old +Ġconver ge +Ġref uel +ig roup +Ġdistort ions +Ġrav aged +Ġmechan ically +ĠRe illy +ĠN ose +ĠIncarn ation +ĠBeck y +abb ling +Ġt aco +Ġr ake +Ġmelanch oly +Ġillust rious +ĠDart mouth +Gu ide +ĠR azer +ĠBen z +Ult imate +ĠSur prise +Ġpage ant +off er +Who ever +Ġw iser +Ġchem ist +ĠHE LL +ĠBul k +Ġpl utonium +ĠCO VER +Ö ¼ +f ailed +Ġtire lessly +Ġinf ertility +ĠTr ident +ĠShow time +ĠC iv +V ice +requ ires +itt ance +Ġun controlled +interest ing +56 1 +Ġinnov ate +ateg ic +L ie +ĠS elling +U l +Ġsav ior +ĠT osh +Ġsw ast +P ASS +Ġr ink +Ġcard io +ĠI ro +ud i +Ġv antage +Ġv ans +ĠNi ño ++ = +Ġpropag ate +< ? +Ġmethod ological +204 39 +Ġtrig lycer +Ġing rained +ĠAn notations +arr anted +6 17 +ĠS odium +ĠA AC +techn ical +mult ipl +Ġ3 73 +å ĭ +Ġdec isively +Ġboost ers +Ġdessert s +ĠGren ade +Ġtest ifying +ĠSc ully +ID s +Ġlock down +ĠSc her +ĠR é +ĠWhit man +ĠRams ay +rem ote +Ġh ikers +ĠHy undai +Ġcons cientious +Ġcler ics +ĠSiber ian +ut i +is bury +Ġrel ayed +Ġqu artz +ĠC BI +seek ers +ull a +Ġweld ing +ĠSh al +ble acher +T ai +ĠSam son +Ġt umble +ĠInvest or +Ġsub contract +ĠShin ra +ow icz +j andro +d ad +Ġtermin ating +ĠNe ural +ä» £ +Ġleak age +ĠMid lands +ĠCaucas us +í ķ +c it +ll an +iv ably +ĠAlb ion +Ġ4 57 +Ġregist rations +Ġcomr ade +Ġclip board +0 47 +Ġdiscour aging +ĠO ops +Ad apt +Ġem path +n v +ĠPR OT +ĠDon n +ĠP ax +ĠB ayer +t is +Squ are +Ġfoot prints +part icip +ĠChile an +B rend +ind ucing +M agn +Ġclub house +ĠMagn um +Ġenc amp +ĠEth nic +uch a +ere y +Ġw atered +ĠCal ais +Ġcomplex ion +Ġsect s +Ġren ters +Ġbr as +oÄŁ an +Time out +Man agement +Ġinf ographic +P okemon +Cl ar +Ġloc ality +Ġfl ora +as el +P ont +Ġpop ulate +ĠO ng +Ġsubs istence +Ġa uctions +ĠMcA uliffe +ĠL OOK +br inger +Ġtit an +Ġmanif old +ĠâĹ ı +Ġcalibr ated +Ġcal iphate +ĠSH E +ĠCommission ers +ce ivable +j c +W inner +5 24 +Ġcond one +Other wise +Ġp iling +Ġem body +ĠCrime an +ut ics +ĠEx hibition +Ġ4 26 +e ering +Ġv ying +ĠH UGE +* =- +Ġprin cipled +à ¦ +Ġquir ks +ĠEdit ors +put ing +G ES +ĠF TA +ठ¾ +add on +ĠH AM +ĠFrie za +W oman +. $ +Ġc rib +ĠHer od +Ġtim ers +ĠSp aces +ĠMac intosh +at aka +Ġgl ide +Ġsmell ing +ĠB AL +Ġun su +Ġcond os +Ġbicy cl +ĠRev ival +55 3 +Ġjugg ling +H ug +ĠKardash ian +ĠBalk ans +mult iple +Ġnutrit ious +oc ry +19 00 +Ġinteg rates +Ġad joining +ĠF older +roll ment +ven ient +Ġu ber +y i +Ġwh iff +ĠJu ven +ĠB orough +net te +Ġb ilingual +ĠSp arks +ph thal +man ufact +Ġt outing +ĠPH I +Ke efe +Rew ard +Ġinf all +ĠTem per +typ ically +ĠNik ol +Ġregular s +Ġpseud onym +Ġexhib itions +Ġbl aster +Ġ40 9 +w arming +Ġrever ber +Ġrecip rocal +Ġ6 70 +ip ient +b ett +ĠBe gins +Ġit ching +ĠPh ar +Ass uming +Ġem itting +ĠML G +Ġbirth place +Ġt aunt +ĠL uffy +ĠAm it +Ġcir cled +ĠN ost +enn ett +Ġde forestation +ĠHist orically +ĠEvery day +Ġovert ake +79 2 +Ġn un +ĠLuc ia +Ġaccompan ies +ĠSe eking +ĠTr ash +an ism +R ogue +Ġnorth western +ĠSupplement al +ĠNY U +ĠF RI +ĠSat isf +x es +5 17 +Ġreass ured +Ġspor adic +Ġ7 01 +Ġmed ial +Ġcannabin oid +Ġbarbar ic +Ġep is +ĠExplos ive +ĠD ough +Ġuns olved +Support ed +Ġacknowled gment +sp awn +Ġkit chens +Ġ- = +talk ing +ic ist +ĠPeg asus +ĠPS U +Ġphot on +ĠAuthent ication +R G +@# & +76 2 +ĠCl air +Ġdi aper +Ġbr ist +ĠProsecut ors +ĠJ em +6 28 +ĠEvery where +ĠJean ne +equ ality +ãĥ© ãĥ³ +object s +ĠPel icans +Ġ39 2 +Ġbl u +b ys +ĠA go +Ġinstruction al +Ġdiscrim inating +ĠTR AN +ĠCorn el +ag os +Ġty re +Ġas piration +ĠBrid gewater +": - +! ". +ĠEn s +ĠCoc o +P ie +Ġdet ach +ĠC ouch +Ġphys ique +ĠOccup ations +osc opic +en ough +B uzz +App earance +Y P +Ġrac er +Ġcompl icity +r pm +T oy +Ġinterrupt s +ĠCat alyst +Ġut ilitarian +imp act +Ġsp aghetti +Ġp orous +Ġeste emed +Ġinc iner +ĠI OC +7 48 +Ġesp resso +ĠSm ile +abil ia +6 35 +Ġmathematic ian +Ġ4 24 +ĠK L +ĠH IP +Ġover heard +ĠT ud +ĠT ec +Ġqu izz +Ġfl attering +Ġcon n +âĢ İ +Ġatt aches +ĠR OS +ĠAC S +Ġt cp +ĠSh ame +sk ip +res pected +ĠTrin idad +gr ain +Ġfooth old +ĠUnch arted +ĠJul io +z l +av ored +ĠAn xiety +er rors +ĠCent auri +its ch +D addy +Ġclutch ing +ĠIm plement +ĠGut ierrez +Ġ7 60 +Ġtele portation +end ra +Ġrevers ible +st ros +Ad venture +08 3 +Ġliber ating +Ġas phalt +ĠSp end +AR DS +im sy +PR ES +ĠEmer ging +Ġwild fires +Ġtechn ologically +Ġem its +ĠART ICLE +Ġirregular ities +Ġcher ish +çī Ī +Ġst ink +ĠR ost +Econom ic +Ġcough ing +ĠMcC ann +pro perties +ilant ro +Ġreneg oti +Trans lation +Ġin quest +ĠGra pe +oot ers +gu i +ĠSwords man +ace ae +h itting +Ġr c +Ġexert ed +ĠS AP +it ent +Ġperil ous +Ġobsc urity +Ġassass inate +Ġab original +Ġresc uing +ĠSh attered +lock ing +all ion +Ch anging +ĠHar rington +ĠB ord +ĠAfgh ans +Jam ie +aret z +ĠAugust us +Ġ38 6 +8 30 +Ġj og +ok ingly +Tr igger +ĠH OR +Stat istics +Ġviewers hip +Ġadd itives +h ur +Ġmaxim izing +ĠR ove +ĠLou ie +ĠBuck et +ĠCHR IST +ou sel +Ġstre aks +ir ted +Ġt ert +Ġcolonial ism +Ġbur ying +y k +Cond ition +ĠDPR K +By Id +75 1 +âĹ ¼ +Ġwor risome +Ġvoc ational +sl ice +Ġsa ils +ĠCorrection al +95 4 +Ġt ul +K id +l uster +Ġfam ilial +ĠSp it +ĠEp iscopal +Specific ally +ĠVol cano +run s +q s +Ġve tted +Ġcram med +t rop +here r +Thank fully +Ġper cussion +Ġor anges +Ġround up +Ġ4 99 +x ious +Char acters +ĠZion ism +ĠR ao +ÃĽ ÃĽ +W F +Ġunintention al +ONE Y +Gr ab +Com mercial +Ġglut amate +ĠMcK enna +ru ciating +ning ton +ih u +Ch an +ĠSw ap +Ġleaf lets +Ġfunction ally +er ous +F arm +Ġcal oric +ĠLiter ally +con cert +Ġshe nan +Ġrep aid +ey es +Ġbas hing +ĠG orge +Ġcollabor ations +Ġun account +itch ie +Ġteam work +pp elin +Ġpip ing +Ġmin ced +Ġd iam +ri eg +Ġmasc ara +Ġsuck er +ĠMo ons +App s +ĠPe ck +Ġper v +ĠFl oat +o ley +ĠN ish +im ize +Ġarom atic +u in +end ish +! / +ĠB icycle +ĠAS IC +ile ged +ĠQuad ro +ios yn +Ġlock out +ĠW ink +SP EC +Attempt s +Ġseed ed +red o +ias is +Ġsn ag +ãĥķ ãĤ© +ãĤ ¶ +Ġground ing +Ġrelie ver +Ġfrivol ous +ĠG ifts +ĠF aces +Es pecially +Ġmicrobi ome +im ag +ĠSch l +ĠP les +ĠBle ach +ĠIr win +ĠE aton +ĠDisc iple +Ġmultipl ication +Ġcoer ced +Ġ4 19 +st h +E vil +B omb +Ġex orc +Ġstag gered +L ESS +Ġinert ia +ĠED IT +Ġgo b +Tr aditional +Ġclass y +Lear y +ĠP AGE +yr s +Ġtrans porter +Ġmat ured +Ġhij ab +Ġbi ome +Where as +Ġex termination +ĠT ues +ĠT akeru +ĠAud rey +er ial +ĠAd en +aff les +Ġnarciss istic +ĠB aird +UT F +I re +ĠCon nie +Ch amp +Ġwhis pering +ĠH att +D K +Ġdis infect +Ġdeduct ed +Ġpart ake +Ġdown grade +ĠEs ports +ĠContin uing +Ġdemocr atically +icro bial +itt a +Ġlim estone +Ġexempt ed +ĠFren zy +H erm +7 28 +Ġfled gling +Met a +765 61 +69 3 +% : +w ake +5 26 +ĠDis cipline +Ġvirgin ity +ĠLeg ions +ĠFrank ie +int ent +Ġrest rooms +ĠRou ter +da q +Ġobjection able +âĨ ij +w ark +ĠRah ul +g ain +activ ation +abs olute +ĠAccess ed +Ġ24 00 +ogg les +Ġsecond ly +ĠDEF ENSE +Ġpost age +wra pper +sh arp +7 29 +Ġcommun icates +Ġadd on +ĠMil itia +H ong +Ġsl umped +ĠJP EG +ĠI car +ad ish +68 1 +Ġmaj esty +ĠWolf gang +ĠEl astic +u per +Ġv iz +Ġunconscious ly +ĠST D +ĠS ass +Ġflower ing +ĠHel ic +ĠDra per +ĠAm ateur +Ġman ure +Ġdis ingen +ĠLe i +br ing +9 49 +Ġinhib ited +Ġhead quartered +Ġen igmatic +�� � +Ġred ress +R H +Ġratt led +Ġd iction +l io +ĠT BA +ĠSN AP +C alling +Ġfasc ists +ĠD ove +iew icz +0 36 +Ġco asts +ĠR ect +Ġ) ] +L ot +6 29 +ĠS EM +ĠPeters en +ĠExpl ain +ĠBo ards +ĠBe zos +ĠJ ournals +Ġ20 24 +p arser +Ġmist rust +Ġgr ate +ĠL ocked +bo a +S aint +g aming +Ġvow el +in ately +bl ow +All ah +Ġun matched +Ġb ordering +ĠExp end +n r +Or acle +rou ch +Ġcont iguous +ac us +Ġdist raught +58 1 +Ġanat omical +O X +ap ixel +8 33 +ĠPL US +Ġres usc +Ġab iding +57 3 +Ġvac ancies +Em ily +Ġhyp othal +ĠWer ner +ĠWe e +ĠDJ s +5 13 +Ġwitch craft +Ġac upuncture +ent ary +benef it +Product s +ĠP SP +ĠMP G +ĠJ inn +ĠJ arrett +Ġ4 45 +ĠIm aging +ĠP yth +Fin ish +Ġte x +Ġjuven iles +Ġhero ism +Ġdoubt less +ĠA ki +ĠT end +ĠPatri arch +Ġbit ters +ĠTele communications +it atively +ag na +Ġr g +ĠS OLD +Ġcomp ulsion +ĠN asa +ĠKath ryn +Ġmillion aires +Ġintrins ically +Ġbolst ered +time out +fl o +Ġtut or +p our +Stat ement +Ġ{ * +ĠRud olph +ĠKimber ly +rog ens +adi q +] + +Ġindign ation +Ġfract uring +ĠRe leases +ĠGr ain +pro tein +L ago +Ġvac ations +Ġboot ed +ĠTH REE +ĠH G +oresc ence +Ġt f +Ġso ar +iosyn cr +Ġgl ances +ĠSp oon +ĠJ ury +ĠCow boy +Ġcreat ively +Hig her +Ġsolic itor +Ġhaw k +ac io +89 6 +Ġsuperf lu +Ġbombs hell +ct ure +Ġbroker age +Ġraid ing +Ġf rench +Ġang led +Trans action +ĠGen ocide +u pe +ĠHait ian +57 2 +! : +Ġunwitting ly +iter ator +sc roll +Ġtall ied +Ġbi omedical +ĠC ARD +Ġe uphem +Ġbrain storm +a quin +K o +Mic helle +ĠR unes +ĠBall istic +ud ers +Ġmod esty +ĠiP ads +ĠEzek iel +Y E +Ġstars hip +Ġpower fully +Ġper l +ĠSh ade +ĠQu art +ĠE EG +Ġfisher man +OS ED +ĠTyp ical +df x +Ġmes hes +Ġet ched +worth iness +Ġtopp led +Ġ3 96 +or ius +We iss +Ġmy sql +ĠVal halla +Ù Ĵ +le asing +Ġrec omp +rap nel +S el +04 3 +Ġder ailed +ĠGu ides +IR T +Ġde human +ĠBritt any +" )) +Ġex claim +Ġb alk +Ġ8 40 +CLA IM +int el +L AB +Ġpe gged +Ġast roph +sm oking +Ġrig ging +Ġfix ation +Ġcat apult +ins ide +ĠC ascade +ĠBolshe vik +G aza +Dep th +Ġloud spe +Ġalmond s +me yer +l eness +j en +f resh +Ġunbeat en +ĠSqu id +ĠPres umably +Tim er +B W +Ġro sters +Ġell ipt +ĠHar riet +dat abase +ĠMut ual +ĠComm odore +uk ed +kn ife +ĠCOMM UN +h ya +Ġmel ts +arch ives +Ġrat ification +Ġmultip lying +Ġinter oper +Ġasc ert +w ings +ver ting +ĠScorp ion +ay e +ĠPorts mouth +ĠM TA +n it +iaz ep +Ġqu arantine +Ġslides how +Ġcent imeters +Ġsyn opsis +Ġsp ate +th irst +Ġnom inating +ĠMel vin +Pre view +Ġthro b +Ġgener ational +ĠRad ius +rest ling +put able +aw ar +N ECT +Ġunlaw fully +ĠRevel ations +Wik ipedia +sur v +Ġeye ing +ij n +ĠF W +Ġbr unt +Ġinter stellar +Ġcl itor +ĠCroat ian +ĠCh ic +ev a +ĠDis app +ĠA kin +iner ies +d ust +Interest ed +Ġgen esis +ĠE ucl +ö n +p icking +Ġmut ated +Ġdisappro ve +ĠHD L +Ġ6 25 +Ì ¶ +c ancer +Ġsqu ats +Ġle vers +Disc uss += ] +D ex +ĠVIDE OS +A UD +Ġtrans act +ĠKin ect +ĠK uala +ĠC yp +7 47 +Ġsh attering +Ġarsen ic +ĠInt ake +ĠAngel o +ĠQu it +ĠK he +Ġ18 93 +M aker +0 29 +ĠPain ting +Dis able +9 16 +Ġanal ges +Ġtact ile +Ġprop hes +Ġd iced +ĠTravel s +ĠHe ader +ĠClub s +Ass istant +Ġinc rim +Ġd ips +Ġcruc ifix +ĠShan ahan +ĠInter pret +Ġ40 90 +al ogy +abb a +Ġsimul ac +hus band +S IM +Ġrecy cle +uc er +ed ged +Ġre naissance +ĠBomb ay +Cath olic +ĠL INE +ĠCl othing +re ports +Ġpl aus +Ġd ag +ĠM ace +Z I +Ġintr uder +ĠVeter inary +g ru +Ġsne aky +ĠS ie +ĠC innamon +P OSE +Ġcou rier +ĠC NS +Ġemanc ipation +s it +Ġplay through +ĠFac ilities +v irt +ĠG auntlet +Thom pson +Ġunbeliev ably +Param eters +Ġst itching +ign e +ĠTH ESE +Priv acy +Ġshenan igans +Ġvit ri +ĠVal id +59 1 +Ń · +ĠProt otype +ink a +SC P +ĠT id +è Ī +old ed +Ġindividual ity +Ġbark ing +Ġm ars +ĠW D +Ġ8 20 +Ġt ir +Ġsl apping +Ġdisgr untled +ĠAng ola +ri us +ĠTorn ado +ĠTh urs +Ġcapt cha +Ġang st +ĠP og +ĠAssass ins +ĠAd idas +Ġjoy ful +Ġwh ining +Emer gency +Ġphosph orus +Ġatt rition +oph on +ĠTimber wolves +ĠJ ah +ĠBr inging +ĠW ad +ĠEn sure +oh l +ĠX ie +omm el +c mp +Ġz ipper +Ġrel at +ĠCor ridor +m ilo +T ING +Av g +Ġcro pped +] } +Ġr aged +ĠLump ur +ĠGuer rero +our ke +N ut +Ġoff sets +og lu +dr m +Ġmort als +lat able +Ġdismiss ive +ä¸ ī +Ġthro ats +Ġchips et +ĠSpot light +Catal og +art ist +G b +Ġch illy +Ġst oked +Ġ3 74 +W ard +L atin +Ġf iasco +Ġble ach +Ġb rav +Enh anced +Ġin oc +ĠFior ina +_ > +Ġle ukemia +Ġel uc +Ġannoun cer +ĠLith uan +ĠArm ageddon +å ĩ +Len in +ĠR uk +Ġpe pp +ĠRom antic +ĠP IT +ĠInter stellar +ĠAt kinson +R aid +J s +Go al +C ourse +Ġvan ishing +es ley +ĠR ounds +Els a +59 3 +Ġredund ancy +ĠST AND +Ġprop hetic +Ġhabit able +ry u +Ġfaint ly +M ODE +Ġfl anked +IR C +Aw esome +Ġsp urious +ĠZ ah +ĠMS G +Ġsh ading +Ġmotiv ational +ĠSant ana +ĠS PR +Ġexc ruciating +om ial +ĠM iko +ĠLe opard +A byss +Ġ[ | +d irty +Ġbath s +Ġdem oral +and re +P B +Ġun ification +Ġsac rament +Ġ[ & +Ġpric eless +Ġgel atin +Ġeman ating +ĠAll aah +98 6 +Ġout burst +Ġer as +ĠX VI +ĠSP I +O tt +ĠLaz arus +PL IED +F lying +blog s +W isconsin +R aven +Ġreb ate +Ġcreep s +ĠSp an +ĠPain ter +ĠKir a +ĠAm os +ĠCor vette +Cons umer +ĠRec over +ck i +Ġpes ky +ĠIn vention +Compan ies +Ġchalleng ers +ad emic +ĠUkrain ians +ĠNeuro log +ĠFors aken +Ġent rants +Ġemb attled +Ġdef unct +ĠGlac ier +Ġpo isons +ĠH orses +m akes +ĠD irt +Ġ4 23 +hh h +ĠTrans formation +QUI RE +................ .. +Ġtrave ller +ĠSe xy +ĠK ern +ip olar +Ġransom ware +oooooooo oooooooo +E c +rub y +Prof essional +ĠOut break +arg ument +G rey +ĠFif a +ĠCH O +ĠFOR M +ĠAm trak +- [ +Ġcr adle +Ġantioxid ants +ãģ®å ® +7 36 +ĠNAS L +ĠContribut ions +Ind iana +ĠST EP +C SS +Ġsal ient +Ġall ocations +yr ights +Ġm ashed +ĠCut ter +Sex ual +Ġp ounded +Ġfan base +Ġc asc +ĠTrans parency +Ġanaly tic +ĠSummon er +× ŀ +ĠAD C +det ail +Ġvan quished +Ġcr abs +ar ie +Dest roy +ĠS ack +Ġtrans istor +Al abama +ĠK oen +ĠFisher ies +c one +Ġannex ed +ĠM GM +es a +Ġf aked +ĠCong ratulations +Ġhind ered +Ġcorrection al +ĠI TV +lee ve +Ġin appropriately +lic ks +Ġtresp ass +Ġp aws +Ġnegoti ator +ĠChrist ensen +lim its +ĠDian ne +Ġeleg ance +ĠContract s +an ke +Ob j +Ġvigil ance +Ġcast les +ĠN AD +ĠHol o +Ġemph atically +ĠTit us +ĠServ ing +ĠRich ie +ĠP igs +5 68 +Ġanim osity +ĠAtt ributes +ĠU riel +M Q +my ra +ĠApplic ant +Ġpsychiat rists +ĠV ij +ĠAb by +ag ree +P ush +Ġk Wh +hib a +Ġinc ite +ĠWe asley +ĠTax i +minist ic +hy per +ĠF arn +Ġ6 01 +ĠNation wide +F ake +95 2 +Ġma ize +Ġinteract ed +Ġtransition ed +Ġparas itic +Ġharm onic +Ġdec aying +Ġbas eless +ns ics +Ġtrans pired +Ġabund antly +ĠFore nsic +Ġtread mill +ĠJ av +ab and +Ġssh d +Ġfront man +ĠJak arta +oll er +dro ps +ĠSERV ICES +rompt u +oph ical +h ospital +bled on +6 45 +Ġmid range +ĠEV ENT +cul ated +raw led +Ġper ched +Ġover board +ĠPe el +ĠP wr +ĠCar th +ĠCOM PLE +co e +sh all +Ġdeter rence +M ETHOD +ĠAbs ent +M EN +Ġs ill +ĠLE VEL +Y ork +Ġsin ners +ĠOP EC +ĠN ur +ĠDesign s +se lection +Ġunw orthy +CH A +Ġstreng thens +88 3 +ed ly +Ġslic ing +Ġmal nutrition +Ġfilm making +ĠPol k +ur ated +Ġ4 21 +bre akers +!' " +Ġwet lands +ĠDisc rimination +Ġallow able +Ġste ered +ĠSic ily +S AM +Ġmust ache +Ġm ids +Ġcl ipped +Ġcirc ulate +Ġbr ittle +ĠBuild ings +ra ised +ĠRound up +Ġwealth ier +Ġoverw rite +Ġover powered +ĠGerr ard +s ites +PD ATED +Ġacute ly +ĠGam ble +Ġp im +ĠK us +Typ ically +De ploy +ĠMoroc can +p otion +com be +Ġvigil ante +Ġ36 3 +St ew +ĠB agg +Ġres ided +ĠSp o +Ġrem nant +Ġempt iness +br ainer +Ġout patient +pri ority +Ġle ptin +ĠPay ton +ĠGle aming +ĠS hed +ĠPol o +ĠMormon ism +rest ricted +arl ane +w x +Ġcreat ine +ĠAn on +ĠST UD +ĠJ UL +ĠT ee +5 28 +08 9 +Ġhat ched +Dis patch +ĠCompos ite +Ġ45 1 +p uff +ĠX COM +ĠOr n +ĠTH ANK +END ED +ĠAshe ville +Ġà ľ +Ġman go +ĠS lightly +world ly +ĠW ander +ĠExp and +ĠCh r +M ist +Ġorthodox y +ĠUN ESCO +reg ate +Else where +k ie +ir led +Ġtopp le +Ġadopt ive +ĠLeg s +d ress +ĠS agan +b are +ĠGl ou +Cr unch +Ġhelp ers +Ġchron ically +ĠH uma +1 0000 +Ġaccommod ating +äº Ķ +Ġwrink les +Ġdod ged +four th +Ġpre con +Ġcompress or +ĠK are +Ġev ict +ĠWar wick +im ar +Ġmodern ization +Ġband wagon +Ġref uted +Ġnet ted +ĠNa ples +ĠGen ie +per ors +Ġfield ed +Ġde re +ĠPar ables +le es +Ġtr out +asp ers +Ġn ihil +Ġhapp iest +Ġflo ppy +ĠLo ft +ĠHe ard +Ġun ison +Ġl ug +ĠRed mond +class ic +Supp orters +SH IP +G MT +Ġfue lled +ç IJ +Ġd d +ĠEmin em +Ġ18 97 +NY SE +Ġsecret aries +ĠF IA +ĠCanaver al +F avorite +Ġp omp +Ġdetain ee +ers hip +aim on +i our +ĠA pex +Ġplant ations +am ia +ac ion +R ust +Ġtow ed +ĠTru ly +5 77 +Ġshel tered +r ider +W o +Ġl air +ĠInt elligent +impro ve +m atically +Ġet iquette +ad ra +all o +ĠJun o +any thing +ĠStru ggle +ĠPred ict +ĠGr imes +ĠAMER ICA +ct x +ĠSit uation +W OOD +Ġsol uble +me ier +Ġintoler able +ang ering +Ġun interrupted +Ġtool tip +Ġinterrog ated +Ġgun ned +ĠSne ak +æŃ ¦ +Ġt ether +Ġcr umble +L ens +Ġclust ered +ĠSy l +ĠHas an +Ġdystop ian +w ana +Ġjoy stick +ĠTh ib +amm u +Tom orrow +5 46 +Ġoverc ame +Ġminim ized +cept or +Run ner +ENG TH +ĠBrend a +ĠAchieve ments +Ġtor ches +Ġrapp ort +ĠInvestig ator +ĠHand ling +rel ation +g rey +8 15 +Ġk cal +ĠComm ands +d q +Ġcur ls +Ġbe arer +Ġcyn icism +it ri +ĠUse ful +B ee +D CS +Ġab ras +P ract +BIL ITIES +7 12 +Ġdebug ger +Ġdebt or +ĠL ia +ĠK ers +Ġexacerb ate +ĠSt acy +ĠB land +ĠSc enes +Ġbranch ing +âĸĪâĸĪâĸĪâĸĪ âĸĪâĸĪâĸĪâĸĪ +ape ake +Ġs alsa +Ġmish and +ĠKon ami +ĠN ib +Ġanecd ote +Ġagree able +Ï ī +ĠNath aniel +ĠHe isman +ĠB eware +Ġ18 86 +spect ive +69 1 +5 22 +Ġinhib its +Ġhas hing +Ġ18 89 +å° Ĩ +v ich +P ure +Ġsolid ly +Ġaspir in +im aru +Ġstreet car +ĠU CS +ĠJ udd +Ġflash backs +p ins +Ġ14 40 +ĠUN HCR +ĠSym ptoms +T IT +5 38 +F ra +% ); +Ġo oz +Ġcur few +Ġcal med +Ġparticip ates +Te X +Ġnons ensical +Ġfull back +ĠDe L +mon key +h ari +Ġmetabol ites +Ġloot ed +ĠAL WAYS +ĠB CC +L t +oc het +B one +Ġveto ed +Ġg cc +ĠCL ICK +Ġ18 88 +s af +Ġstiff ness +Ġlow ly +ĠGe h +vers on +ors et +Ġun foreseen +Ġan esthesia +ĠOpt ical +Ġrecon structed +ĠT up +sh ows +NEW S +ĠNewsp aper +ĠA SA +ter a +N umbers +Ġinexpl icable +× ij +Ġhard ness +unt arily +ĠA cer +grad ient +ARD IS +Ġwood land +Ġmetaph ors +ĠWem bley +ĠPa vel +phil is +Ġre writing +Ġpercept ual +Ġ10 70 +worm s +ĠDown s +Ġunsur prisingly +Ġtag ging +fl ame +Ġlit res +Ġboun ces +ĠB abe +sh ut +Ġoverd oses +ĠShe ila +ĠCh au +ĠBl ess +Capt ure +ĠSign ificant +ĠSc ion +Ġ38 9 +ĠMc H +ĠTitan ium +ĠMe al +amed a +ag ents +agg ressive +B illy +76 3 +ĠS aying +DER R +it one +Coll ins +B ound +Ġbol ted +ĠDM CA +95 3 +Ġun iqueness +Ġep igen +un ci +ant am +Ġreck oning +ch airs +OG R +ĠSen egal +Ġ18 62 +re levant +Ġ ¯ +Ġpharm acies +ĠG eral +v ier +Y an +OR PG +Ġrab id +b ending +ĠUN ITED +Ġ4 65 +As sembly +Ġwe ep +Ġbe hest +ĠMother s +ĠJ ace +h id +Ġwh irlwind +ĠUN IVERS +Ġut opian +Ġkidn ap +Ph ilipp +K in +89 3 +Ġlivest ream +ĠM ISS +Ġsub versive +ĠTechn iques +ĠJUST ICE +ĠB ASE +Ġ38 7 +Ġassail ants +ĠHard core +Ġsprink led +ĠP se +é ļ +print ed +ĠH au +OR GE +ĠT OUR +Ġl aced +Ġit ch +G iving +Ġport ed +78 1 +//////////////// //////////////// +bre eding +Ġlog ger +ĠH OL +inn ie +First ly +Ġembry onic +Ġdeleg ated +p ai +O IL +Ġcentr ally +ĠR x +ĠSc outing +D utch +Ġhe reditary +ĠCru iser +s at +5 29 +ĠMar riott +other mal +Ġprohib itions +E arn +ĠSt ab +ĠColleg es +ĠBel ief +st retched +ĠL H +ĠEntity Item +C IA +Ġun rem +Ġlaure ate +Ġdenomin ations +sum mary +h ler +S pect +ĠK laus +ĠBe ans +Ġins ur +ĠPA X +Ġfield er +ĠV et +ĠSp arrow +z ie +ĠS Q +ĠMond ays +ĠOff line +ĠLer ner +ĠExt ensions +Ire land +Ġpatron age +Ġcontrast ed +ĠMan ia +h irt +Mos cow +Ġcondem ns +ĠAn ge +Ġcomp osing +ĠPe pe +ĠP addock +Ġheter ogeneity +Ġide ologically +Ġf ishes +Ġcur sing +ĠR utherford +ĠFlo ating +ĠAm elia +Te a +Syn opsis +Ġstun ts +Ġbe ad +Ġstock ing +ĠM ILL +ob ook +mass ive +\ < +Ġh ump +ĠPref erences +Engine Debug +ge ist +ĠNiet o +ome ver +ish y +eval uate +col onial +Altern ative +ĠGo Pro +ĠV ortex +ĠNET WORK +ans ky +Sec ure +ĠTh rust +Sn ake +Ġparcel s +Ġsam urai +Ġactress es +N ap +M F +ifer ation +Be er +5 23 +ĠI ly +oint ment +P ing +Ġstri ped +ĠMell on +oss ession +Ġneut ron +end ium +Ġa ph +ĠFlav oring +Ġ38 3 +Ġrespons iveness +ĠJ indal +ĠHitch cock +Den ver +ĠDRAG ON +sm anship +ĠDu pl +Ġs ly +Ġweb cam +ĠTw ain +ĠDar ling +ili ate +cons umer +D IT +Ġnames ake +Ġun orthodox +Ġfun er +ĠPL oS +ĠCONTR OL +ozy g +ogl obin +F ACE +ER G +ĠD ia +ĠF iesta +ce le +0 34 +Ġencl ave +âĸ¬ âĸ¬ +on ement +al ist +M and +Ġhome grown +ĠF ancy +Ġconcept ions +ĠCont ains +ure en +Ġreiter ate +Ġme ager +Ġinstall ments +Sp awn +6 27 +Ġphot oc +ĠCab rera +ĠRos enthal +ĠLans ing +is ner +Ġinvest s +ĠUFO s +EX P +Hard ware +Ġtr agically +Ġconced es +ie ft +ch am +bor gh +ĠSch r +ĠMel anie +ĠH oy +Ġvisit ation +Ġid iosyncr +Ġfract ions +Ġfore skin +ob os +Ġpo aching +ĠVI EW +Ġstimul ates +ĠG ork +can on +M IC +ĠNem esis +ĠInd ra +ĠDM V +Ġ5 29 +Ġinspect ing +Ġgrand ma +ĠW hedon +ĠSh ant +ĠP urg +ik an +ĠT eg +ĠCL R +z ac +Vict oria +ĠVer ify +ion ics +Ġpart ying +ĠM ou +col our +Ġtestim onies +l ations +Ġpress uring +hi ro +ac ers +Ġf id +ang ler +ĠCS I +Ġhere after +Ġdiss idents +report ing +iph any +che v +Ġsol itude +Ġl obe +Ġind is +Ġcred ential +re cent +ad ult +ĠNir vana +ĠFranch ise +L ayer +H yp +ĠBerks hire +Ġwill s +t if +Ġtot em +ĠJud ah +rep air +Inst ant +5 48 +Ġemb assies +Ġbott leneck +Ġb ount +Ġtyp ew +ĠAl vin +j ing +im ilar +R ush +Ġbr im +ĠHEL P +A im +] ' +Ġpass ively +Ġbound ed +ĠR ated +Ġcriminal ity +Ġbiom ark +Ġdisp atcher +ĠTow ards +Ġ+ ++ +right eous +f rog +ĠP anc +C arter +0 32 +æ© Ł +Ġult raviolet +ĠLic ensed +ĠT ata +ĠBl essing +ĠG AM +Ġchem ically +ĠSe af +ĠRE LE +ĠMerc enary +capital ist +Ġform ulations +Ġann ihilation +ĠVer b +ĠAr gon +Ġun loaded +Ġmorp hed +Ġconqu ering +back er +I ELD +Ġtheft s +Ġfront runner +ĠRoy ale +ĠFund amental +el ight +C hip +necess ary +ay n +ĠSl ip +Ġ4 48 +cern ed +P ause +Ġshock ingly +ĠAB V +Ġcomp osure +7 33 +ĠMotors port +ah ime +Mur ray +M ach +Ġgr ids +Ġdeb ian +Ġfurther more +Ġdexter ity +ĠCollect ions +os lov +il age +b j +ĠMont eneg +Ġstrut Connector +Ġmassac res +Ġbrief s +fet ched +uv ian +ol ition +Fail ure +emon ic +Ġfl ared +Ġclaim ant +Ġc ures +Ġgive aways +ĠSubst ance +al ions +Ġcr inge +ĠK ul +Ġarist ocracy +ĠUl ster +ol ated +h ousing +ĠM IS +Ġgl ared +ĠWil helm +ne eds +lam bda +build ers +ĠV IS +Ġradi ator +ĠGhost busters +Ġ4 36 +act ual +Ġher ds +ç a +watch ing +Ġcounter ing +Ch arge +Ġchar red +Ġwar heads +Ġiod ine +ĠM acy +04 1 +Ġdepart ures +ĠS ins +Ġdy ed +ĠConcept s +g ado +7 13 +Ġquot ations +Ġg ist +ĠChrist y +Ġant igen +ĠHem p +ĠD rawn +ĠB arg +ez vous +Ġp aternity +Ġar du +ĠAnch orage +ĠR ik +Ġover loaded +ĠUs ername +ĠTam my +ĠN au +ĠCell ular +Ġw aning +Ġrod ent +ĠWor cester +il ts +ĠT ad +Ġdwell ings +Ġbull ish +4 31 +Ġretali ate +Ġmig raine +ĠChev ron +CH ECK +Ġdon key +c rim +SP A +ĠAn alog +Ġmarqu ee +ĠHa as +B ir +ĠGD DR +ĠDownload s +Ġwill power +ĠFor th +ĠRecord ed +Ġimp ossibility +ĠLog ged +ĠFr anks +ĠR att +in itions +Ġclean ers +Ġsore ly +Ġflick ering +ĠEx amination +c atching +allow een +Ms g +Ġdun no +F a +Ġdys ph +c razy +.' '. +Ġmain line +Ġc s +Ġp tr +ĠW ally +ig un +95 1 +ĠBig foot +f ights +Ġretrie ving +J r +Ġdupl ication +ĠExpl an +Ġrel ational +Ġqu aint +Ġbisc uits +Ġad o +Ġsh udder +Ġantid ote +blood ed +ks h +Ġsa uces +Ġrein vest +Ġdispens ary +ĠD iver +Ġ9 000 +stud ent +Ġin separ +esc ap +Ġtodd lers +ĠGP IO +ĠAss ignment +head ers +Ġlack luster +Ġab ack +95 6 +Ġtool bar +7 45 +Ġo ust +Ġcontempl ation +ĠPRES IDENT +Ġ4 58 +==== == +Ġguarantee ing +ĠHe ist +ĠCann es +Ļ ½ +Ġcollabor ator +ĠAm p +Ġg ou +ĠSH ALL +st ories +78 3 +Ġmobil ized +Ġbro od +ĠL U +ĠðŁ ij +Ġref in +ĠAnthrop ology +v ind +ill i +Ġwarrant ies +ĠB abel +Ġsw ath +Ġc aches +Ġantagon ists +art ifacts +Ġhot ly +ĠSt arts +ĠG ö +z ag +!! !!! +Ġsc ourge +Ġcons piring +ru its +re verse +ĠShe en +ĠJes uit +ĠGiov anni +ad ies +Ġbutt ocks +ear cher +ac an +Ġvolley ball +Ġshroud ed +Ġscore board +b ats +ĠI PM +Ġass es +Ġde regulation +ĠTe legram +ĠReb oot +Ġ7 000 +ĠCan ary +Ġk ernels +ĠFranç ois +ĠD uff +ĠP on +ĠLe ica +ĠGar min +Ġor phans +ĠClaud ia +Ġcal endars +ĠLe ilan +ent o +R ocket +Ġbr unch +ĠHaw king +ain ers +Ġsens ibilities +Ġk W +ĠK and +Ġre claimed +Ġinteresting ly +× © +rom y +J M +ĠEnhance ment +b ush +Sk ip +Ġrapp ers +Ġg azing +p edia +ath lon +Rev olution +Ġsn ipers +Ġre verted +Ġconglomer ate +T erry +79 4 +Ġhars her +Ġdes olate +ĠHit man +Comm ission +Ġ( / +â̦ ." +Com par +Ġampl ification +om inated +Ġreg ress +ĠColl ider +Ġinform ants +Ġg azed diff --git a/whisper/assets/gpt2/special_tokens_map.json b/whisper/assets/gpt2/special_tokens_map.json new file mode 100644 index 0000000000000000000000000000000000000000..817762d631ad6f9c799f6b9dc713c46420e65546 --- /dev/null +++ b/whisper/assets/gpt2/special_tokens_map.json @@ -0,0 +1 @@ +{"bos_token": "<|endoftext|>", "eos_token": "<|endoftext|>", "unk_token": "<|endoftext|>"} \ No newline at end of file diff --git a/whisper/assets/gpt2/tokenizer_config.json b/whisper/assets/gpt2/tokenizer_config.json new file mode 100644 index 0000000000000000000000000000000000000000..c92208ce39324aadabc239d73dd9bb3ec1cd45ca --- /dev/null +++ b/whisper/assets/gpt2/tokenizer_config.json @@ -0,0 +1 @@ +{"unk_token": "<|endoftext|>", "bos_token": "<|endoftext|>", "eos_token": "<|endoftext|>", "add_prefix_space": false, "model_max_length": 1024, "special_tokens_map_file": null, "name_or_path": "gpt2", "tokenizer_class": "GPT2Tokenizer"} \ No newline at end of file diff --git a/whisper/assets/gpt2/vocab.json b/whisper/assets/gpt2/vocab.json new file mode 100644 index 0000000000000000000000000000000000000000..84ef7fb594b5c0979e48bdeddb60a0adef33df0b --- /dev/null +++ b/whisper/assets/gpt2/vocab.json @@ -0,0 +1 @@ +{"!":0,"\"":1,"#":2,"$":3,"%":4,"&":5,"'":6,"(":7,")":8,"*":9,"+":10,",":11,"-":12,".":13,"/":14,"0":15,"1":16,"2":17,"3":18,"4":19,"5":20,"6":21,"7":22,"8":23,"9":24,":":25,";":26,"<":27,"=":28,">":29,"?":30,"@":31,"A":32,"B":33,"C":34,"D":35,"E":36,"F":37,"G":38,"H":39,"I":40,"J":41,"K":42,"L":43,"M":44,"N":45,"O":46,"P":47,"Q":48,"R":49,"S":50,"T":51,"U":52,"V":53,"W":54,"X":55,"Y":56,"Z":57,"[":58,"\\":59,"]":60,"^":61,"_":62,"`":63,"a":64,"b":65,"c":66,"d":67,"e":68,"f":69,"g":70,"h":71,"i":72,"j":73,"k":74,"l":75,"m":76,"n":77,"o":78,"p":79,"q":80,"r":81,"s":82,"t":83,"u":84,"v":85,"w":86,"x":87,"y":88,"z":89,"{":90,"|":91,"}":92,"~":93,"¡":94,"¢":95,"£":96,"¤":97,"¥":98,"¦":99,"§":100,"¨":101,"©":102,"ª":103,"«":104,"¬":105,"®":106,"¯":107,"°":108,"±":109,"²":110,"³":111,"´":112,"µ":113,"¶":114,"·":115,"¸":116,"¹":117,"º":118,"»":119,"¼":120,"½":121,"¾":122,"¿":123,"À":124,"Á":125,"Â":126,"Ã":127,"Ä":128,"Å":129,"Æ":130,"Ç":131,"È":132,"É":133,"Ê":134,"Ë":135,"Ì":136,"Í":137,"Î":138,"Ï":139,"Ð":140,"Ñ":141,"Ò":142,"Ó":143,"Ô":144,"Õ":145,"Ö":146,"×":147,"Ø":148,"Ù":149,"Ú":150,"Û":151,"Ü":152,"Ý":153,"Þ":154,"ß":155,"à":156,"á":157,"â":158,"ã":159,"ä":160,"å":161,"æ":162,"ç":163,"è":164,"é":165,"ê":166,"ë":167,"ì":168,"í":169,"î":170,"ï":171,"ð":172,"ñ":173,"ò":174,"ó":175,"ô":176,"õ":177,"ö":178,"÷":179,"ø":180,"ù":181,"ú":182,"û":183,"ü":184,"ý":185,"þ":186,"ÿ":187,"Ā":188,"ā":189,"Ă":190,"ă":191,"Ą":192,"ą":193,"Ć":194,"ć":195,"Ĉ":196,"ĉ":197,"Ċ":198,"ċ":199,"Č":200,"č":201,"Ď":202,"ď":203,"Đ":204,"đ":205,"Ē":206,"ē":207,"Ĕ":208,"ĕ":209,"Ė":210,"ė":211,"Ę":212,"ę":213,"Ě":214,"ě":215,"Ĝ":216,"ĝ":217,"Ğ":218,"ğ":219,"Ġ":220,"ġ":221,"Ģ":222,"ģ":223,"Ĥ":224,"ĥ":225,"Ħ":226,"ħ":227,"Ĩ":228,"ĩ":229,"Ī":230,"ī":231,"Ĭ":232,"ĭ":233,"Į":234,"į":235,"İ":236,"ı":237,"IJ":238,"ij":239,"Ĵ":240,"ĵ":241,"Ķ":242,"ķ":243,"ĸ":244,"Ĺ":245,"ĺ":246,"Ļ":247,"ļ":248,"Ľ":249,"ľ":250,"Ŀ":251,"ŀ":252,"Ł":253,"ł":254,"Ń":255,"Ġt":256,"Ġa":257,"he":258,"in":259,"re":260,"on":261,"Ġthe":262,"er":263,"Ġs":264,"at":265,"Ġw":266,"Ġo":267,"en":268,"Ġc":269,"it":270,"is":271,"an":272,"or":273,"es":274,"Ġb":275,"ed":276,"Ġf":277,"ing":278,"Ġp":279,"ou":280,"Ġan":281,"al":282,"ar":283,"Ġto":284,"Ġm":285,"Ġof":286,"Ġin":287,"Ġd":288,"Ġh":289,"Ġand":290,"ic":291,"as":292,"le":293,"Ġth":294,"ion":295,"om":296,"ll":297,"ent":298,"Ġn":299,"Ġl":300,"st":301,"Ġre":302,"ve":303,"Ġe":304,"ro":305,"ly":306,"Ġbe":307,"Ġg":308,"ĠT":309,"ct":310,"ĠS":311,"id":312,"ot":313,"ĠI":314,"ut":315,"et":316,"ĠA":317,"Ġis":318,"Ġon":319,"im":320,"am":321,"ow":322,"ay":323,"ad":324,"se":325,"Ġthat":326,"ĠC":327,"ig":328,"Ġfor":329,"ac":330,"Ġy":331,"ver":332,"ur":333,"Ġu":334,"ld":335,"Ġst":336,"ĠM":337,"'s":338,"Ġhe":339,"Ġit":340,"ation":341,"ith":342,"ir":343,"ce":344,"Ġyou":345,"il":346,"ĠB":347,"Ġwh":348,"ol":349,"ĠP":350,"Ġwith":351,"Ġ1":352,"ter":353,"ch":354,"Ġas":355,"Ġwe":356,"Ġ(":357,"nd":358,"ill":359,"ĠD":360,"if":361,"Ġ2":362,"ag":363,"ers":364,"ke":365,"Ġ\"":366,"ĠH":367,"em":368,"Ġcon":369,"ĠW":370,"ĠR":371,"her":372,"Ġwas":373,"Ġr":374,"od":375,"ĠF":376,"ul":377,"ate":378,"Ġat":379,"ri":380,"pp":381,"ore":382,"ĠThe":383,"Ġse":384,"us":385,"Ġpro":386,"Ġha":387,"um":388,"Ġare":389,"Ġde":390,"ain":391,"and":392,"Ġor":393,"igh":394,"est":395,"ist":396,"ab":397,"rom":398,"ĠN":399,"th":400,"Ġcom":401,"ĠG":402,"un":403,"op":404,"00":405,"ĠL":406,"Ġnot":407,"ess":408,"Ġex":409,"Ġv":410,"res":411,"ĠE":412,"ew":413,"ity":414,"ant":415,"Ġby":416,"el":417,"os":418,"ort":419,"oc":420,"qu":421,"Ġfrom":422,"Ġhave":423,"Ġsu":424,"ive":425,"ould":426,"Ġsh":427,"Ġthis":428,"nt":429,"ra":430,"pe":431,"ight":432,"art":433,"ment":434,"Ġal":435,"ust":436,"end":437,"--":438,"all":439,"ĠO":440,"ack":441,"Ġch":442,"Ġle":443,"ies":444,"red":445,"ard":446,"âĢ":447,"out":448,"ĠJ":449,"Ġab":450,"ear":451,"iv":452,"ally":453,"our":454,"ost":455,"gh":456,"pt":457,"Ġpl":458,"ast":459,"Ġcan":460,"ak":461,"ome":462,"ud":463,"The":464,"Ġhis":465,"Ġdo":466,"Ġgo":467,"Ġhas":468,"ge":469,"'t":470,"ĠU":471,"rou":472,"Ġsa":473,"Ġj":474,"Ġbut":475,"Ġwor":476,"Ġall":477,"ect":478,"Ġk":479,"ame":480,"Ġwill":481,"ok":482,"Ġwhe":483,"Ġthey":484,"ide":485,"01":486,"ff":487,"ich":488,"pl":489,"ther":490,"Ġtr":491,"..":492,"Ġint":493,"ie":494,"ure":495,"age":496,"Ġne":497,"ial":498,"ap":499,"ine":500,"ice":501,"Ġme":502,"Ġout":503,"ans":504,"one":505,"ong":506,"ions":507,"Ġwho":508,"ĠK":509,"Ġup":510,"Ġtheir":511,"Ġad":512,"Ġ3":513,"Ġus":514,"ated":515,"ous":516,"Ġmore":517,"ue":518,"og":519,"ĠSt":520,"ind":521,"ike":522,"Ġso":523,"ime":524,"per":525,".\"":526,"ber":527,"iz":528,"act":529,"Ġone":530,"Ġsaid":531,"Ġ-":532,"are":533,"Ġyour":534,"cc":535,"ĠTh":536,"Ġcl":537,"ep":538,"ake":539,"able":540,"ip":541,"Ġcont":542,"Ġwhich":543,"ia":544,"Ġim":545,"Ġabout":546,"Ġwere":547,"very":548,"ub":549,"Ġhad":550,"Ġen":551,"Ġcomp":552,",\"":553,"ĠIn":554,"Ġun":555,"Ġag":556,"ire":557,"ace":558,"au":559,"ary":560,"Ġwould":561,"ass":562,"ry":563,"ĠâĢ":564,"cl":565,"ook":566,"ere":567,"so":568,"ĠV":569,"ign":570,"ib":571,"Ġoff":572,"Ġte":573,"ven":574,"ĠY":575,"ile":576,"ose":577,"ite":578,"orm":579,"Ġ201":580,"Ġres":581,"Ġman":582,"Ġper":583,"Ġother":584,"ord":585,"ult":586,"Ġbeen":587,"Ġlike":588,"ase":589,"ance":590,"ks":591,"ays":592,"own":593,"ence":594,"Ġdis":595,"ction":596,"Ġany":597,"Ġapp":598,"Ġsp":599,"int":600,"ress":601,"ations":602,"ail":603,"Ġ4":604,"ical":605,"Ġthem":606,"Ġher":607,"ount":608,"ĠCh":609,"Ġar":610,"Ġif":611,"Ġthere":612,"Ġpe":613,"Ġyear":614,"av":615,"Ġmy":616,"Ġsome":617,"Ġwhen":618,"ough":619,"ach":620,"Ġthan":621,"ru":622,"ond":623,"ick":624,"Ġover":625,"vel":626,"Ġqu":627,"ĊĊ":628,"Ġsc":629,"reat":630,"ree":631,"ĠIt":632,"ound":633,"port":634,"Ġalso":635,"Ġpart":636,"fter":637,"Ġkn":638,"Ġbec":639,"Ġtime":640,"ens":641,"Ġ5":642,"ople":643,"Ġwhat":644,"Ġno":645,"du":646,"mer":647,"ang":648,"Ġnew":649,"----":650,"Ġget":651,"ory":652,"ition":653,"ings":654,"Ġjust":655,"Ġinto":656,"Ġ0":657,"ents":658,"ove":659,"te":660,"Ġpeople":661,"Ġpre":662,"Ġits":663,"Ġrec":664,"Ġtw":665,"ian":666,"irst":667,"ark":668,"ors":669,"Ġwork":670,"ade":671,"ob":672,"Ġshe":673,"Ġour":674,"wn":675,"ink":676,"lic":677,"Ġ19":678,"ĠHe":679,"ish":680,"nder":681,"ause":682,"Ġhim":683,"ons":684,"Ġ[":685,"Ġro":686,"form":687,"ild":688,"ates":689,"vers":690,"Ġonly":691,"oll":692,"Ġspe":693,"ck":694,"ell":695,"amp":696,"Ġacc":697,"Ġbl":698,"ious":699,"urn":700,"ft":701,"ood":702,"Ġhow":703,"hed":704,"Ġ'":705,"Ġafter":706,"aw":707,"Ġatt":708,"ov":709,"ne":710,"Ġplay":711,"erv":712,"ict":713,"Ġcould":714,"itt":715,"Ġam":716,"Ġfirst":717,"Ġ6":718,"Ġact":719,"Ġ$":720,"ec":721,"hing":722,"ual":723,"ull":724,"Ġcomm":725,"oy":726,"old":727,"ces":728,"ater":729,"Ġfe":730,"Ġbet":731,"we":732,"iff":733,"Ġtwo":734,"ock":735,"Ġback":736,").":737,"ident":738,"Ġunder":739,"rough":740,"sel":741,"xt":742,"Ġmay":743,"round":744,"Ġpo":745,"ph":746,"iss":747,"Ġdes":748,"Ġmost":749,"Ġdid":750,"Ġadd":751,"ject":752,"Ġinc":753,"fore":754,"Ġpol":755,"ont":756,"Ġagain":757,"clud":758,"tern":759,"Ġknow":760,"Ġneed":761,"Ġcons":762,"Ġco":763,"Ġ.":764,"Ġwant":765,"Ġsee":766,"Ġ7":767,"ning":768,"iew":769,"ĠThis":770,"ced":771,"Ġeven":772,"Ġind":773,"ty":774,"ĠWe":775,"ath":776,"Ġthese":777,"Ġpr":778,"Ġuse":779,"Ġbecause":780,"Ġfl":781,"ng":782,"Ġnow":783,"ĠâĢĵ":784,"com":785,"ise":786,"Ġmake":787,"Ġthen":788,"ower":789,"Ġevery":790,"ĠUn":791,"Ġsec":792,"oss":793,"uch":794,"Ġem":795,"Ġ=":796,"ĠRe":797,"ied":798,"rit":799,"Ġinv":800,"lect":801,"Ġsupp":802,"ating":803,"Ġlook":804,"man":805,"pect":806,"Ġ8":807,"row":808,"Ġbu":809,"Ġwhere":810,"ific":811,"Ġyears":812,"ily":813,"Ġdiff":814,"Ġshould":815,"Ġrem":816,"Th":817,"In":818,"Ġev":819,"day":820,"'re":821,"rib":822,"Ġrel":823,"ss":824,"Ġdef":825,"Ġright":826,"Ġsy":827,"),":828,"les":829,"000":830,"hen":831,"Ġthrough":832,"ĠTr":833,"__":834,"Ġway":835,"Ġdon":836,"Ġ,":837,"Ġ10":838,"ased":839,"Ġass":840,"ublic":841,"Ġreg":842,"ĠAnd":843,"ix":844,"Ġvery":845,"Ġinclud":846,"other":847,"Ġimp":848,"oth":849,"Ġsub":850,"ĠâĢĶ":851,"Ġbeing":852,"arg":853,"ĠWh":854,"==":855,"ible":856,"Ġdoes":857,"ange":858,"ram":859,"Ġ9":860,"ert":861,"ps":862,"ited":863,"ational":864,"Ġbr":865,"Ġdown":866,"Ġmany":867,"aking":868,"Ġcall":869,"uring":870,"ities":871,"Ġph":872,"ics":873,"als":874,"Ġdec":875,"ative":876,"ener":877,"Ġbefore":878,"ility":879,"Ġwell":880,"Ġmuch":881,"erson":882,"Ġthose":883,"Ġsuch":884,"Ġke":885,"Ġend":886,"ĠBut":887,"ason":888,"ting":889,"Ġlong":890,"ef":891,"Ġthink":892,"ys":893,"Ġbel":894,"Ġsm":895,"its":896,"ax":897,"Ġown":898,"Ġprov":899,"Ġset":900,"ife":901,"ments":902,"ble":903,"ward":904,"Ġshow":905,"Ġpres":906,"ms":907,"omet":908,"Ġob":909,"Ġsay":910,"ĠSh":911,"ts":912,"ful":913,"Ġeff":914,"Ġgu":915,"Ġinst":916,"und":917,"ren":918,"cess":919,"Ġent":920,"ĠYou":921,"Ġgood":922,"Ġstart":923,"ince":924,"Ġmade":925,"tt":926,"stem":927,"olog":928,"up":929,"Ġ|":930,"ump":931,"Ġhel":932,"vern":933,"ular":934,"ually":935,"Ġac":936,"Ġmon":937,"Ġlast":938,"Ġ200":939,"10":940,"Ġstud":941,"ures":942,"ĠAr":943,"self":944,"ars":945,"meric":946,"ues":947,"cy":948,"Ġmin":949,"ollow":950,"Ġcol":951,"io":952,"Ġmod":953,"Ġcount":954,"ĠCom":955,"hes":956,"Ġfin":957,"air":958,"ier":959,"âĢĶ":960,"read":961,"ank":962,"atch":963,"ever":964,"Ġstr":965,"Ġpoint":966,"ork":967,"ĠNew":968,"Ġsur":969,"ool":970,"alk":971,"ement":972,"Ġused":973,"ract":974,"ween":975,"Ġsame":976,"oun":977,"ĠAl":978,"ci":979,"Ġdiffere":980,"Ġwhile":981,"--------":982,"Ġgame":983,"cept":984,"Ġsim":985,"...":986,"Ġinter":987,"ek":988,"Ġreport":989,"Ġprodu":990,"Ġstill":991,"led":992,"ah":993,"Ġhere":994,"Ġworld":995,"Ġthough":996,"Ġnum":997,"arch":998,"imes":999,"ale":1000,"ĠSe":1001,"ĠIf":1002,"//":1003,"ĠLe":1004,"Ġret":1005,"Ġref":1006,"Ġtrans":1007,"ner":1008,"ution":1009,"ters":1010,"Ġtake":1011,"ĠCl":1012,"Ġconf":1013,"way":1014,"ave":1015,"Ġgoing":1016,"Ġsl":1017,"ug":1018,"ĠAmeric":1019,"Ġspec":1020,"Ġhand":1021,"Ġbetween":1022,"ists":1023,"ĠDe":1024,"oot":1025,"It":1026,"Ġear":1027,"Ġagainst":1028,"Ġhigh":1029,"gan":1030,"az":1031,"ather":1032,"Ġexp":1033,"Ġop":1034,"Ġins":1035,"Ġgr":1036,"Ġhelp":1037,"Ġrequ":1038,"ets":1039,"ins":1040,"ĠPro":1041,"ism":1042,"Ġfound":1043,"land":1044,"ata":1045,"uss":1046,"ames":1047,"Ġperson":1048,"Ġgreat":1049,"pr":1050,"Ġsign":1051,"ĠAn":1052,"'ve":1053,"Ġsomet":1054,"Ġser":1055,"hip":1056,"Ġrun":1057,"Ġ:":1058,"Ġter":1059,"irect":1060,"Ġfollow":1061,"Ġdet":1062,"ices":1063,"Ġfind":1064,"12":1065,"Ġmem":1066,"Ġcr":1067,"ered":1068,"ex":1069,"Ġext":1070,"uth":1071,"ense":1072,"co":1073,"Ġteam":1074,"ving":1075,"ouse":1076,"ash":1077,"att":1078,"ved":1079,"Ġsystem":1080,"ĠAs":1081,"der":1082,"ives":1083,"min":1084,"Ġlead":1085,"ĠBl":1086,"cent":1087,"Ġaround":1088,"Ġgovern":1089,"Ġcur":1090,"velop":1091,"any":1092,"Ġcour":1093,"alth":1094,"ages":1095,"ize":1096,"Ġcar":1097,"ode":1098,"Ġlaw":1099,"Ġread":1100,"'m":1101,"con":1102,"Ġreal":1103,"Ġsupport":1104,"Ġ12":1105,"....":1106,"Ġreally":1107,"ness":1108,"Ġfact":1109,"Ġday":1110,"Ġboth":1111,"ying":1112,"Ġserv":1113,"ĠFor":1114,"Ġthree":1115,"Ġwom":1116,"Ġmed":1117,"ody":1118,"ĠThey":1119,"50":1120,"Ġexper":1121,"ton":1122,"Ġeach":1123,"akes":1124,"Ġche":1125,"Ġcre":1126,"ines":1127,"Ġrep":1128,"19":1129,"gg":1130,"illion":1131,"Ġgrou":1132,"ute":1133,"ik":1134,"We":1135,"get":1136,"ER":1137,"Ġmet":1138,"Ġsays":1139,"ox":1140,"Ġduring":1141,"ern":1142,"ized":1143,"ared":1144,"Ġfam":1145,"ically":1146,"Ġhapp":1147,"ĠIs":1148,"Ġchar":1149,"med":1150,"vent":1151,"Ġgener":1152,"ient":1153,"ple":1154,"iet":1155,"rent":1156,"11":1157,"ves":1158,"ption":1159,"Ġ20":1160,"formation":1161,"Ġcor":1162,"Ġoffic":1163,"ield":1164,"Ġtoo":1165,"ision":1166,"Ġinf":1167,"ĠZ":1168,"the":1169,"oad":1170,"Ġpublic":1171,"Ġprog":1172,"ric":1173,"**":1174,"Ġwar":1175,"Ġpower":1176,"view":1177,"Ġfew":1178,"Ġloc":1179,"Ġdifferent":1180,"Ġstate":1181,"Ġhead":1182,"'ll":1183,"Ġposs":1184,"Ġstat":1185,"ret":1186,"ants":1187,"Ġval":1188,"Ġiss":1189,"Ġcle":1190,"ivers":1191,"anc":1192,"Ġexpl":1193,"Ġanother":1194,"ĠQ":1195,"Ġav":1196,"thing":1197,"nce":1198,"Wh":1199,"Ġchild":1200,"Ġsince":1201,"ired":1202,"less":1203,"Ġlife":1204,"Ġdevelop":1205,"ittle":1206,"Ġdep":1207,"Ġpass":1208,"ãĥ":1209,"Ġturn":1210,"orn":1211,"This":1212,"bers":1213,"ross":1214,"ĠAd":1215,"Ġfr":1216,"Ġresp":1217,"Ġsecond":1218,"oh":1219,"Ġ/":1220,"Ġdisc":1221,"Ġ&":1222,"Ġsomething":1223,"Ġcomple":1224,"Ġed":1225,"Ġfil":1226,"Ġmonth":1227,"aj":1228,"uc":1229,"Ġgovernment":1230,"Ġwithout":1231,"Ġleg":1232,"Ġdist":1233,"Ġput":1234,"Ġquest":1235,"ann":1236,"Ġprot":1237,"20":1238,"Ġnever":1239,"ience":1240,"Ġlevel":1241,"Ġart":1242,"Ġthings":1243,"Ġmight":1244,"Ġeffect":1245,"Ġcontro":1246,"Ġcent":1247,"Ġ18":1248,"Ġallow":1249,"Ġbelie":1250,"chool":1251,"ott":1252,"Ġincre":1253,"Ġfeel":1254,"Ġresult":1255,"Ġlot":1256,"Ġfun":1257,"ote":1258,"Ġty":1259,"erest":1260,"Ġcontin":1261,"Ġusing":1262,"Ġbig":1263,"201":1264,"Ġask":1265,"Ġbest":1266,"Ġ)":1267,"IN":1268,"Ġopp":1269,"30":1270,"Ġnumber":1271,"iness":1272,"St":1273,"lease":1274,"Ġca":1275,"Ġmust":1276,"Ġdirect":1277,"Ġgl":1278,"Ġ<":1279,"Ġopen":1280,"Ġpost":1281,"Ġcome":1282,"Ġseem":1283,"ording":1284,"Ġweek":1285,"ately":1286,"ital":1287,"Ġel":1288,"riend":1289,"Ġfar":1290,"Ġtra":1291,"inal":1292,"Ġpri":1293,"ĠUS":1294,"Ġplace":1295,"Ġform":1296,"Ġtold":1297,"\":":1298,"ains":1299,"ature":1300,"ĠTrump":1301,"Ġstand":1302,"Ġ#":1303,"ider":1304,"ĠFr":1305,"Ġnext":1306,"Ġsoc":1307,"Ġpur":1308,"Ġlet":1309,"Ġlittle":1310,"Ġhum":1311,"Ġi":1312,"ron":1313,"15":1314,"Ġ15":1315,"Ġcommun":1316,"Ġmark":1317,"ĠThere":1318,"Ġwr":1319,"ĠThat":1320,"Ġinformation":1321,"ways":1322,"Ġbus":1323,"app":1324,"Ġinvest":1325,"me":1326,"Ġhard":1327,"ained":1328,"ead":1329,"Ġimport":1330,"Ġappro":1331,"Ġtest":1332,"Ġtri":1333,"Ġrest":1334,"osed":1335,"Ġfull":1336,"Ġcare":1337,"ĠSp":1338,"Ġcase":1339,"ON":1340,"Ġsk":1341,"Ġless":1342,"Ġ+":1343,"Ġpartic":1344,"ĠPl":1345,"ably":1346,"uck":1347,"ished":1348,"chn":1349,"be":1350,"Ġlist":1351,"ator":1352,"Ġtop":1353,"Ġadv":1354,"ĠBe":1355,"ruct":1356,"Ġdem":1357,"ration":1358,"ling":1359,"gy":1360,"reen":1361,"ger":1362,"Ġhome":1363,"Ġleft":1364,"Ġbetter":1365,"Ġdata":1366,"Ġ11":1367,"Ġattack":1368,"Ġproble":1369,"line":1370,"ards":1371,"Ġbeh":1372,"ral":1373,"ĠHow":1374,"ĠShe":1375,"arge":1376,"Ġ--":1377,"://":1378,"Ġbro":1379,"ĠPh":1380,"ats":1381,"Ġbuild":1382,"ww":1383,"ided":1384,"aim":1385,"ases":1386,"ency":1387,"Ġmain":1388,"ined":1389,"Ġincluding":1390,"Ġ{":1391,"Ġgot":1392,"Ġinterest":1393,"Ġkeep":1394,"ĠX":1395,"Ġeas":1396,"aining":1397,"Ġclass":1398,"â̦":1399,"ĠNo":1400,"Ġvar":1401,"Ġsmall":1402,"ample":1403,"AT":1404,"Ġide":1405,"ĠSo":1406,"Ġrece":1407,"Ġpolit":1408,"Ġmov":1409,"Ġplan":1410,"Ġpercent":1411,"iving":1412,"Ġcamp":1413,"Ġpay":1414,"14":1415,"sc":1416,"ised":1417,"Ġunt":1418,"oney":1419,"ploy":1420,"====":1421,"Ġdidn":1422,"ĠInd":1423,"els":1424,"ertain":1425,"Ġpos":1426,"____":1427,"iver":1428,"Ġprocess":1429,"Ġprogram":1430,"ified":1431,"ĠRep":1432,"16":1433,"uro":1434,"ology":1435,"atter":1436,"ina":1437,"Ġname":1438,"ĠAll":1439,"Ġfour":1440,"Ġreturn":1441,"vious":1442,"bs":1443,"Ġcalled":1444,"Ġmove":1445,"ĠSc":1446,"ird":1447,"Ġgroup":1448,"Ġbre":1449,"Ġmen":1450,"Ġcap":1451,"ten":1452,"ee":1453,"Ġdri":1454,"leg":1455,"here":1456,"uthor":1457,"Ġpat":1458,"Ġcurrent":1459,"ides":1460,"Ġpop":1461,"to":1462,"ention":1463,"Ġalways":1464,"Ġmil":1465,"Ġwomen":1466,"Ġ16":1467,"Ġold":1468,"iven":1469,"raph":1470,"ĠOr":1471,"ror":1472,"ently":1473,"Ġnear":1474,"ĠEx":1475,"ream":1476,"sh":1477,"Ġ14":1478,"Ġfree":1479,"ission":1480,"stand":1481,"ĠCon":1482,"ality":1483,"used":1484,"13":1485,"Ġdesign":1486,"Ġchange":1487,"Ġchang":1488,"Ġbo":1489,"Ġvis":1490,"ember":1491,"Ġbook":1492,"ready":1493,"Ġkill":1494,"25":1495,"pped":1496,"Ġaway":1497,"Ġable":1498,"Ġcountry":1499,"Ġconst":1500,"arn":1501,"Ġorder":1502,"AR":1503,"ior":1504,"ium":1505,"orth":1506,"18":1507,"ailable":1508,"Ġsw":1509,"Ġmillion":1510,"Ġ13":1511,"atic":1512,"ted":1513,"ĠGo":1514,"Ġoper":1515,"eng":1516,"Ġthing":1517,"ajor":1518,"conom":1519,"ĠComm":1520,"Ġwhy":1521,"ured":1522,"ural":1523,"Ġschool":1524,"by":1525,"ĠMar":1526,"Ġaff":1527,"Ġdays":1528,"Ġann":1529,"ush":1530,"ane":1531,"If":1532,"eg":1533,"Ġprof":1534,"Ġhealth":1535,"outh":1536,"But":1537,"ional":1538,".,":1539,"Ġsol":1540,"Ġalready":1541,"Ġ30":1542,"Ġcharact":1543,"He":1544,"Ġfriend":1545,"ES":1546,"ians":1547,"icle":1548,"'d":1549,"ĠOn":1550,"Ġleast":1551,"Ġprom":1552,"Ġdr":1553,"Ġhist":1554,"ither":1555,"Ġest":1556,"iqu":1557,"17":1558,"son":1559,"Ġtell":1560,"Ġtalk":1561,"ohn":1562,"oint":1563,"lection":1564,"AN":1565,"Ġuntil":1566,"augh":1567,"Ġlater":1568,"Ġve":1569,"Ġview":1570,"ending":1571,"ived":1572,"Ġword":1573,"ware":1574,"Ġcost":1575,"Ġenough":1576,"Ġgive":1577,"ĠUnited":1578,"Ġtechn":1579,"arent":1580,"OR":1581,"Ġpar":1582,"ĠDr":1583,"Ġ2016":1584,"rist":1585,"ering":1586,"ĠÂ":1587,"Ġlarge":1588,"side":1589,"acy":1590,"ccess":1591,"Ġwin":1592,"Ġimportant":1593,"Ġ199":1594,"Ġdoesn":1595,"Ġ17":1596,"Ġbusiness":1597,"Ġclear":1598,"Ġrese":1599,"\",":1600,"ury":1601,"Ġequ":1602,"aster":1603,"alf":1604,"ĠAmerican":1605,"nect":1606,"Ġexpect":1607,"iversity":1608,"Ġocc":1609,"ĠFl":1610,"Ġkind":1611,"Ġmean":1612,"Ġpast":1613,"Ġdev":1614,"Ġbas":1615,"let":1616,"raft":1617,"Ġorgan":1618,"Ġdel":1619,"Ġperform":1620,"Ġstory":1621,"Ġseason":1622,"ĠCol":1623,"Ġclaim":1624,"Ġcame":1625,"Ġwithin":1626,"Ġline":1627,"Ġproject":1628,"ĠAt":1629,"Ġcontrol":1630,"ended":1631,"ĠSy":1632,"Ġair":1633,"ization":1634,"Ġ*":1635,"ley":1636,"Ġmoney":1637,"idd":1638,"You":1639,"for":1640,"Ġfamily":1641,"Ġmaking":1642,"Ġbit":1643,"Ġpolice":1644,"Ġhappen":1645,"Ġvers":1646,"ony":1647,"uff":1648,"ĠWhen":1649,"Ġsit":1650,"ideo":1651,"lf":1652,"ison":1653,"Ġsure":1654,"gin":1655,"Ġappear":1656,"Ġlight":1657,"Ġes":1658,"of":1659,"Ġwater":1660,"Ġtimes":1661,"not":1662,"Ġgrow":1663,"Ġcompany":1664,"ĠTe":1665,"ows":1666,"Ġmar":1667,"ource":1668,"iol":1669,"arm":1670,"br":1671,"Ġexample":1672,"Ġconc":1673,"Ġfore":1674,"ĠTo":1675,"pro":1676,"EN":1677,"ries":1678,"Ġ25":1679,"ĠCan":1680,"ney":1681,"Ġactually":1682,"Ġever":1683,"urity":1684,"aken":1685,"aps":1686,"Ġtax":1687,"Ġmajor":1688,"ama":1689,"Ġoften":1690,"eral":1691,"Ġhuman":1692,"Ġjob":1693,"ister":1694,"Ġavailable":1695,"ocr":1696,"enn":1697,"aid":1698,"ivid":1699,"Ġrecord":1700,"?\"":1701,"Ġsing":1702,"ĠAm":1703,"idence":1704,"Ġnews":1705,"ster":1706,"Ġeconom":1707,"Ġfollowing":1708,"ĠBr":1709,"ising":1710,"Ġhour":1711,"most":1712,"ument":1713,"Ġsex":1714,"Ġdesc":1715,"Ġbecome":1716,"ĠEd":1717,"Ġtook":1718,"Ġhaving":1719,"Ġproduct":1720,"ault":1721,"As":1722,"aring":1723,"Ġmeans":1724,"Ġhop":1725,"une":1726,"Ġcho":1727,"Ġcertain":1728,"Ġnon":1729,"Ġdeal":1730,"24":1731,"lement":1732,"oci":1733,"ene":1734,"Ġside":1735,"ĠPr":1736,"ĠMay":1737,"Ġreason":1738,"ued":1739,"ched":1740,"ulation":1741,"Ġelect":1742,"Ġofficial":1743,"Ġpossible":1744,"Ġhold":1745,"ands":1746,"ots":1747,"Ġcity":1748,"ories":1749,"Ġsever":1750,"Ġchildren":1751,"Ġonce":1752,"Ġactiv":1753,"ler":1754,"Ġnight":1755,"itions":1756,"ĠJohn":1757,"ape":1758,"play":1759,"Ġdone":1760,"Ġlim":1761,"Ġworking":1762,"ĠPres":1763,"orld":1764,"eb":1765,"ĠCo":1766,"Ġbody":1767,"ails":1768,"utes":1769,"ĠMr":1770,"Ġwhether":1771,"Ġauthor":1772,"rop":1773,"Ġproper":1774,"Ġseen":1775,");":1776,"Ġfac":1777,"ĠSu":1778,"Ġcond":1779,"iting":1780,"Ġcourse":1781,"Ġ}":1782,"----------------":1783,"aign":1784,"Ġevent":1785,"Ġeng":1786,"Ġpot":1787,"Ġintern":1788,"iam":1789,"Ġshort":1790,"empt":1791,"ãĤ":1792,"ĠGod":1793,"ilar":1794,"80":1795,"Ġorig":1796,"IS":1797,"ourn":1798,"ability":1799,"itive":1800,"Ġdam":1801,"Ġ100":1802,"Ġpress":1803,"Ġdoing":1804,"Ġprotect":1805,"ring":1806,"Ġthought":1807,"Ġquestion":1808,"rew":1809,"ĠWar":1810,"Ġseveral":1811,"ĠState":1812,"Ġgiven":1813,"Ġfund":1814,"ĠTw":1815,"Ġwent":1816,"ances":1817,"work":1818,"por":1819,"my":1820,"40":1821,"Ġarg":1822,"artment":1823,"ustom":1824,"Ġpolic":1825,"Ġmeet":1826,"Ġcreat":1827,"22":1828,"ĠStates":1829,"Ġgames":1830,"raw":1831,"uture":1832,"Ġunderstand":1833,"urs":1834,"ĠOb":1835,"lish":1836,"sy":1837,"Ġmakes":1838,"Ġwon":1839,"agon":1840,"Ġhtt":1841,"Ġlove":1842,"ential":1843,"Ġcomplete":1844,"par":1845,"ĠIm":1846,"AL":1847,"Ġaccount":1848,"Âł":1849,"ored":1850,"vert":1851,"Ġident":1852,"Ġ2015":1853,"Ġothers":1854,"ĠMin":1855,"iber":1856,"verage":1857,"There":1858,"itional":1859,"dd":1860,"Ġprob":1861,"Ġyoung":1862,"Ġalong":1863,"Ġaccording":1864,"Ġyet":1865,"Ġmembers":1866,"ĠWhat":1867,"oid":1868,"ĠMan":1869,"And":1870,"Ġamong":1871,"ai":1872,"Ġemploy":1873,"ĠRes":1874,"Ġ>":1875,"Ġinvol":1876,"Ġlow":1877,"af":1878,"ĠCar":1879,"Ġhig":1880,"ĠOne":1881,"ĠSec":1882,"ination":1883,"Ġlikely":1884,"Ġant":1885,"aged":1886,"ĠRuss":1887,"Ġben":1888,"Ġrele":1889,"For":1890,"back":1891,"ĠNot":1892,"Ġpresident":1893,"ball":1894,"Ġaccess":1895,"ividual":1896,"ĠDem":1897,"ĠEuro":1898,"60":1899,"Ġknown":1900,"irl":1901,"ĠGr":1902,"Ġearly":1903,"use":1904,"iety":1905,"âĢĵ":1906,"Ġfight":1907,"Ġsent":1908,"Ġtoday":1909,"Ġmarket":1910,"\".":1911,"Ġbased":1912,"Ġstrong":1913,"urther":1914,"Ġdeb":1915,"mber":1916,"Ġproblem":1917,"Ġdeath":1918,"Ġsocial":1919,"imate":1920,"AS":1921,"ortun":1922,"Ġcampaign":1923,"ery":1924,"Ch":1925,"Ġey":1926,"ially":1927,"Ġmus":1928,"wh":1929,"pos":1930,"Ġer":1931,"Ġsaf":1932,"Ġmonths":1933,"iron":1934,"Ġviol":1935,"Ġfive":1936,"Ġstre":1937,"Ġplayers":1938,"inc":1939,"ald":1940,"year":1941,"aun":1942,"Ġsuccess":1943,"Ġpresent":1944,"erence":1945,"Ġ2014":1946,"Ġsugg":1947,"Ġparticular":1948,"Ġtry":1949,"Ġsuggest":1950,"ĠChrist":1951,"ones":1952,"Ġpriv":1953,"23":1954,"Ġcrit":1955,"Ġland":1956,"Ġlocal":1957,"ify":1958,"29":1959,"Ġaut":1960,"ED":1961,"ĠGu":1962,"Ġmult":1963,"Ġpolitical":1964,"Ġasked":1965,"Ġformer":1966,"itter":1967,"ript":1968,"Ġclose":1969,"Ġpract":1970,"ĠYork":1971,"Ġgetting":1972,"Ġacross":1973,"Ġcomb":1974,"Ġbelieve":1975,"Ġz":1976,"Ġtoget":1977,"Ġtogether":1978,"ĠCent":1979,"irc":1980,"Ġindividual":1981,"ĠMc":1982,"27":1983,"isk":1984,"ĠEng":1985,"Ġface":1986,"Ġ24":1987,"Ġvalue":1988,"Ġarea":1989,"ev":1990,"Ġwrit":1991,"ĠPresident":1992,"Ġvot":1993,"Ġkey":1994,"Ġmom":1995,"put":1996,"Ġanything":1997,"Ġexperience":1998,"attle":1999,"Ġmind":2000,"aff":2001,"omm":2002,"Ġfuture":2003,"ged":2004,"Ġcut":2005,"Ġtot":2006,"itch":2007,"Ġvideo":2008,"Ġinvestig":2009,"Ġnet":2010,"ĠMy":2011,"rict":2012,"ien":2013,".)":2014,"Ġimpro":2015,"though":2016,"wards":2017,"Ġconnect":2018,"ĠMed":2019,"selves":2020,"ensive":2021,"mb":2022,"ober":2023,"ators":2024,"An":2025,"Ġ50":2026,"Ġredu":2027,"resent":2028,"Ġabove":2029,"Ġfre":2030,"ĠEurope":2031,"sw":2032,"Ġamount":2033,"ĠApp":2034,"Ġeither":2035,"Ġmilit":2036,"Ġanal":2037,"Ġfail":2038,"ĠEn":2039,"ales":2040,"Ġspecial":2041,"Ġblack":2042,"IT":2043,"cher":2044,"Ġlooking":2045,"Ġfire":2046,"yn":2047,"Ġalmost":2048,"oon":2049,"Ġstudy":2050,"Ġmiss":2051,"ches":2052,"rown":2053,"Ġtre":2054,"Ġcommunity":2055,"Ġmedia":2056,"Ġfood":2057,"Ġcomes":2058,"ĠUniversity":2059,"Ġsingle":2060,"What":2061,"uly":2062,"Ġhalf":2063,"ague":2064,"hod":2065,"ĠRepublic":2066,"Ġstarted":2067,"Ġquick":2068,"oto":2069,"book":2070,"Ġissue":2071,"itor":2072,"Ġelse":2073,"Ġconsider":2074,"26":2075,"rodu":2076,"Ġtaken":2077,"28":2078,"99":2079,"ĠWith":2080,"Ġtrue":2081,"Ġwa":2082,"Ġtrad":2083,"Ġago":2084,"Ġmess":2085,"ief":2086,"Ġadded":2087,"oke":2088,"Ġbad":2089,"Ġfav":2090,"33":2091,"Ġsimilar":2092,"ask":2093,"ĠDon":2094,"Ġcharacter":2095,"orts":2096,"ĠHouse":2097,"Ġreported":2098,"Ġtype":2099,"val":2100,"iod":2101,"ĠHowever":2102,"Ġtarg":2103,"Ġentire":2104,"pping":2105,"Ġhistory":2106,"Ġlive":2107,"ffic":2108,"........":2109,"ederal":2110,"Ġtrying":2111,"Ġdiscuss":2112,"ĠHar":2113,"aces":2114,"lished":2115,"Ġself":2116,"osp":2117,"rest":2118,"Ġroom":2119,"elt":2120,"Ġfall":2121,"olution":2122,"Ġet":2123,"Ġx":2124,"Ġisn":2125,"Ġidea":2126,"bo":2127,"Ġsound":2128,"ĠDep":2129,"Ġsomeone":2130,"cially":2131,"ully":2132,"Ġfoc":2133,"Ġobject":2134,"ift":2135,"aper":2136,"Ġplayer":2137,"Ġrather":2138,"Ġservice":2139,"ashing":2140,"ĠDo":2141,"ĠPart":2142,"rug":2143,"mon":2144,"ply":2145,"Ġmor":2146,"Ġnothing":2147,"Ġprovide":2148,"IC":2149,"ung":2150,"Ġparty":2151,"Ġexist":2152,"Ġmag":2153,"70":2154,"Ġrul":2155,"Ġhouse":2156,"Ġbehind":2157,"Ġhowever":2158,"ĠWorld":2159,"Ġsum":2160,"Ġapplic":2161,"Ġ;":2162,"Ġfunction":2163,"gr":2164,"ĠPol":2165,"Ġfront":2166,"200":2167,"Ġseries":2168,"Ġtem":2169,"Ġtyp":2170,"ills":2171,"Ġopt":2172,"Ġpoints":2173,"Ġbelow":2174,"itted":2175,"Ġspecific":2176,"Ġ2017":2177,"umb":2178,"Ġra":2179,"Ġprevious":2180,"Ġpret":2181,"reme":2182,"Ġcustom":2183,"Ġcourt":2184,"ĠMe":2185,"Ġrepl":2186,"Ġwhole":2187,"go":2188,"cer":2189,"Ġtreat":2190,"ĠAct":2191,"Ġprobably":2192,"Ġlearn":2193,"ender":2194,"ĠAss":2195,"Ġversion":2196,"now":2197,"Ġcheck":2198,"ĠCal":2199,"RE":2200,"minist":2201,"On":2202,"ources":2203,"Ġbenef":2204,"Ġdoc":2205,"Ġdeter":2206,"Ġenc":2207,"Ġsuper":2208,"Ġaddress":2209,"Ġvict":2210,"Ġ2013":2211,"Ġmeas":2212,"tr":2213,"Ġfield":2214,"When":2215,"Ġsignific":2216,"uge":2217,"Ġfeat":2218,"Ġcommon":2219,"load":2220,"Ġbegin":2221,"Ġbring":2222,"Ġaction":2223,"erman":2224,"Ġdescrib":2225,"Ġindust":2226,"Ġwanted":2227,"ried":2228,"ming":2229,"Ġattempt":2230,"45":2231,"fer":2232,"Ġdue":2233,"ression":2234,"##":2235,"Ġshall":2236,"Ġsix":2237,"oo":2238,"Ġstep":2239,"Ġpub":2240,"Ġhimself":2241,"Ġ23":2242,"Ġcop":2243,"Ġdest":2244,"Ġstop":2245,"AC":2246,"ibility":2247,"Ġlab":2248,"icult":2249,"Ġhours":2250,"Ġcreate":2251,"Ġfurther":2252,"ĠAmerica":2253,"ĠCity":2254,"Ġdou":2255,"head":2256,"ST":2257,"ĠNorth":2258,"cing":2259,"Ġnational":2260,"ule":2261,"ĠInst":2262,"Ġtaking":2263,"ĠQu":2264,"irt":2265,"Ġred":2266,"Ġresearch":2267,"viron":2268,"ĠGe":2269,"Ġbreak":2270,"ana":2271,"Ġspace":2272,"aterial":2273,"Ġrecent":2274,"ĠAb":2275,"Ġgeneral":2276,"Ġhit":2277,"Ġperiod":2278,"Ġeverything":2279,"ively":2280,"Ġphys":2281,"Ġsaying":2282,"anks":2283,"Ġcou":2284,"Ġcult":2285,"aced":2286,"eal":2287,"uation":2288,"Ġcoun":2289,"lu":2290,"Ġinclude":2291,"Ġposition":2292,"ĠAfter":2293,"ĠCanad":2294,"ĠEm":2295,"Ġimm":2296,"ĠRed":2297,"Ġpick":2298,"Ġcompl":2299,"Ġmatter":2300,"reg":2301,"ext":2302,"angu":2303,"isc":2304,"ole":2305,"aut":2306,"Ġcompet":2307,"eed":2308,"fect":2309,"Ġ21":2310,"ĠSen":2311,"ĠThese":2312,"asing":2313,"Ġcannot":2314,"Ġinit":2315,"Ġrelations":2316,"ached":2317,"Ġbar":2318,"Ġ40":2319,"ĠTH":2320,"Ġ2012":2321,"Ġvol":2322,"Ġground":2323,"Ġsecurity":2324,"Ġupd":2325,"ilt":2326,"35":2327,"Ġconcern":2328,"ĠJust":2329,"Ġwhite":2330,"Ġseems":2331,"ĠHer":2332,"pecially":2333,"ients":2334,"Ġannoun":2335,"Ġfig":2336,"ights":2337,"Ġstri":2338,"like":2339,"ids":2340,"Ġsus":2341,"Ġwatch":2342,"Ġâ":2343,"Ġwind":2344,"ĠCont":2345,"Ġitself":2346,"Ġmass":2347,"Al":2348,"yle":2349,"ique":2350,"ĠNational":2351,"Ġabs":2352,"Ġpack":2353,"Ġoutside":2354,"Ġanim":2355,"Ġpain":2356,"eter":2357,"Ġmanag":2358,"duct":2359,"ogn":2360,"Ġ]":2361,"ĠSept":2362,"sec":2363,"off":2364,"ĠJan":2365,"Ġfoot":2366,"ades":2367,"Ġthird":2368,"Ġmot":2369,"Ġevidence":2370,"inton":2371,"Ġthreat":2372,"apt":2373,"ples":2374,"cle":2375,"Ġlo":2376,"Ġdecl":2377,"Ġitem":2378,"medi":2379,"Ġrepresent":2380,"omb":2381,"amer":2382,"Ġsignificant":2383,"ograph":2384,"su":2385,"Ġcal":2386,"ires":2387,"0000":2388,"ID":2389,"AM":2390,"Ġsimply":2391,"Ġlonger":2392,"Ġfile":2393,"OT":2394,"che":2395,"So":2396,"ateg":2397,"org":2398,"ĠHis":2399,"Ġener":2400,"Ġdom":2401,"Ġupon":2402,"ili":2403,"\":\"":2404,"Ġthemselves":2405,"Ġcoming":2406,"Ġquite":2407,"Ġdifficult":2408,"ĠBar":2409,"ilities":2410,"rel":2411,"ends":2412,"cial":2413,"64":2414,"Ġwoman":2415,"rap":2416,"yr":2417,"Ġnecess":2418,"ips":2419,"Ġtext":2420,"Ġrequire":2421,"Ġmilitary":2422,"Ġreview":2423,"Ġrespons":2424,"75":2425,"Ġsubject":2426,"Ġinstead":2427,"Ġissues":2428,"Ġgen":2429,"\",\"":2430,"Ġminutes":2431,"Ġweap":2432,"ray":2433,"amed":2434,"time":2435,"bl":2436,"How":2437,"Ġcode":2438,"ĠSm":2439,"Ġhigher":2440,"ĠSte":2441,"ris":2442,"Ġpage":2443,"Ġstudents":2444,"ĠIntern":2445,"Ġmethod":2446,"ĠAug":2447,"ĠPer":2448,"ĠAg":2449,"Ġpolicy":2450,"ĠSw":2451,"Ġexec":2452,"Ġaccept":2453,"ume":2454,"ribut":2455,"Ġwords":2456,"Ġfinal":2457,"Ġchanges":2458,"ĠDemocr":2459,"Ġfriends":2460,"Ġrespect":2461,"Ġep":2462,"Ġcompan":2463,"ivil":2464,"Ġdamage":2465,"****":2466,"ogle":2467,"vironment":2468,"Ġneg":2469,"ental":2470,"Ġap":2471,"Ġtotal":2472,"ival":2473,"!\"":2474,"lim":2475,"Ġneeds":2476,"Ġagre":2477,"Ġdevelopment":2478,"Ġage":2479,"iple":2480,"21":2481,"Ġresults":2482,"ĠAf":2483,"Sh":2484,"Ġgun":2485,"ĠObama":2486,"roll":2487,"Ġ@":2488,"Ġrights":2489,"ĠBrit":2490,"Ġrunning":2491,"Ġwasn":2492,"Ġport":2493,"Ġrate":2494,"Ġpretty":2495,"Ġtarget":2496,"Ġsaw":2497,"Ġcirc":2498,"Ġworks":2499,"icro":2500,"alt":2501,"over":2502,"www":2503,"That":2504,"lier":2505,"Ġeveryone":2506,"ude":2507,"Ġpie":2508,"iddle":2509,"rael":2510,"Ġrad":2511,"Ġblock":2512,"Ġwalk":2513,"To":2514,"ãģ":2515,"nes":2516,"ĠAust":2517,"aul":2518,"rote":2519,"ĠSouth":2520,"ession":2521,"oph":2522,"Ġshows":2523,"Ġsite":2524,"Ġjo":2525,"Ġrisk":2526,"clus":2527,"lt":2528,"Ġinj":2529,"iding":2530,"ĠSpe":2531,"Ġchall":2532,"irm":2533,"Ġ22":2534,"itting":2535,"str":2536,"Ġhy":2537,"LE":2538,"key":2539,"Ġbegan":2540,"atur":2541,"ashington":2542,"lam":2543,"ĠDav":2544,"bit":2545,"Ġsize":2546,"ĠPar":2547,"38":2548,"ournal":2549,"face":2550,"Ġdecision":2551,"Ġlarg":2552,"Ġjud":2553,"rect":2554,"Ġcontinue":2555,"ĠOct":2556,"overed":2557,"ĠInt":2558,"========":2559,"Ġparent":2560,"ĠWill":2561,"Ġeasy":2562,"Ġdrug":2563,"anger":2564,"Ġsense":2565,"Ġdi":2566,"iday":2567,"Ġenergy":2568,"istic":2569,"Ġassoci":2570,"arter":2571,"obal":2572,"eks":2573,"ĠEl":2574,"urch":2575,"Ġgirl":2576,"oe":2577,"itle":2578,"Ġ28":2579,"ĠChe":2580,"Ġrequest":2581,"Ġsoon":2582,"Ġhost":2583,"ky":2584,"Ġstates":2585,"omes":2586,"Ġmaterial":2587,"lex":2588,"Ġmoment":2589,"Ġansw":2590,"onse":2591,"Ġespecially":2592,"Ġnorm":2593,"Ġservices":2594,"pite":2595,"ran":2596,"Ġrole":2597,"44":2598,"):":2599,"Ġcred":2600,"Cl":2601,"________":2602,"Ġmat":2603,"Ġlog":2604,"ĠClinton":2605,"OU":2606,"Ġoffice":2607,"Ġ26":2608,"Ġcharg":2609,"Ġtrack":2610,"ma":2611,"Ġheart":2612,"Ġball":2613,"Ġpersonal":2614,"Ġbuilding":2615,"na":2616,"set":2617,"body":2618,"ĠBlack":2619,"Ġincrease":2620,"itten":2621,"Ġneeded":2622,"36":2623,"32":2624,"=\"":2625,"Ġlost":2626,"Ġbecame":2627,"Ġgroups":2628,"ĠMus":2629,"Ġwrote":2630,"ĠPe":2631,"Ġprop":2632,"joy":2633,"é":2634,"ĠWhite":2635,"Ġdead":2636,".'":2637,"Ġhttp":2638,"Ġwebs":2639,"OS":2640,"Ġinside":2641,"Ġwrong":2642,"Ġstatement":2643,"Ġ...":2644,"yl":2645,"Ġfilm":2646,"Ġmusic":2647,"Ġshare":2648,"ification":2649,"Ġrelease":2650,"Ġforward":2651,"Ġstay":2652,"Ġcomput":2653,"itte":2654,"ser":2655,"Ġoriginal":2656,"Ġcard":2657,"Ġcand":2658,"Ġdiv":2659,"atural":2660,"Ġfavor":2661,"OM":2662,"Ġcases":2663,"uses":2664,"Ġsection":2665,"Ġleave":2666,"ging":2667,"oved":2668,"ĠWashington":2669,"39":2670,"ĠGl":2671,"Ġrequired":2672,"action":2673,"apan":2674,"oor":2675,"iter":2676,"ĠKing":2677,"Ġcountries":2678,"ĠGerman":2679,"lling":2680,"Ġ27":2681,"34":2682,"Ġquestions":2683,"Ġprim":2684,"Ġcell":2685,"Ġshoot":2686,"Ġanyone":2687,"ĠWest":2688,"Ġaffect":2689,"epend":2690,"Ġonline":2691,"ĠIsrael":2692,"ĠSeptember":2693,"Ġability":2694,"Ġcontent":2695,"ises":2696,"Ġreve":2697,"Ġlaun":2698,"Ġindic":2699,"Ġforce":2700,"cast":2701,"Ġsold":2702,"aving":2703,"fl":2704,"Ġsoft":2705,"Ġcompanies":2706,"ceed":2707,"Ġarticle":2708,"Ġaud":2709,"Ġrev":2710,"Ġeduc":2711,"Ġplaying":2712,"05":2713,"Ġheld":2714,"ctor":2715,"Ġreleased":2716,"Ġfederal":2717,"37":2718,"Ġadminist":2719,"Ġinterview":2720,"Ġinstall":2721,"Ġreceived":2722,"Ġsource":2723,"uk":2724,"Ph":2725,"Ġserious":2726,"Ġcreated":2727,"Ġcause":2728,"Ġimmedi":2729,"Ġdefin":2730,"uel":2731,"ĠDepartment":2732,"ctions":2733,"ĠCour":2734,"ĠNow":2735,"ze":2736,"ites":2737,"itution":2738,"Ġlate":2739,"Ġspeak":2740,"ners":2741,"Ġlegal":2742,"ari":2743,"ĠCor":2744,"Ġweeks":2745,"Ġmodel":2746,"Ġpred":2747,"Ġexact":2748,"BC":2749,"ĠBy":2750,"ING":2751,"osing":2752,"Ġtakes":2753,"Ġregard":2754,"Ġopportun":2755,"Ġprice":2756,"Ġ198":2757,"ĠApr":2758,"fully":2759,"Ġord":2760,"Ġproblems":2761,"ruction":2762,"ham":2763,"ĠCount":2764,"lege":2765,"Ġleaders":2766,"ET":2767,"lev":2768,"Ġdeep":2769,"ological":2770,"ese":2771,"haps":2772,"ĠSome":2773,"Ġpers":2774,"Ġcontract":2775,"Ġrelationship":2776,"sp":2777,"oud":2778,"Ġbase":2779,"48":2780,"mit":2781,"Ad":2782,"ancial":2783,"Ġconsum":2784,"Ġpotential":2785,"Ġlangu":2786,"rem":2787,"eth":2788,"Ġrelig":2789,"ressed":2790,"66":2791,"Ġlink":2792,"Ġlower":2793,"ayer":2794,"ĠJune":2795,"Ġfem":2796,"unt":2797,"erc":2798,"urd":2799,"Ġcontact":2800,"Ġill":2801,"Ġmother":2802,"Ġestab":2803,"htt":2804,"ĠMarch":2805,"ĠBro":2806,"ĠChina":2807,"Ġ29":2808,"Ġsqu":2809,"Ġprovided":2810,"Ġaverage":2811,"asons":2812,"Ġ2011":2813,"Ġexam":2814,"lin":2815,"55":2816,"ned":2817,"Ġperfect":2818,"Ġtou":2819,"alse":2820,"ux":2821,"Ġbuy":2822,"Ġshot":2823,"Ġcollect":2824,"Ġphot":2825,"Ġplayed":2826,"Ġsurpr":2827,"Ġofficials":2828,"Ġsimple":2829,"avy":2830,"Ġindustry":2831,"Ġhands":2832,"ground":2833,"Ġpull":2834,"Ġround":2835,"Ġuser":2836,"Ġrange":2837,"uary":2838,"Ġprivate":2839,"ops":2840,"ees":2841,"Ġways":2842,"ĠMich":2843,"Ġveh":2844,"Ġexcept":2845,"Ġterms":2846,"imum":2847,"pper":2848,"ION":2849,"ores":2850,"ĠDragon":2851,"oul":2852,"Ġden":2853,"Ġperformance":2854,"Ġbill":2855,"cil":2856,"47":2857,"Ġenvironment":2858,"Ġexc":2859,"add":2860,"Ġworth":2861,"Ġpict":2862,"Ġchance":2863,"Ġ2018":2864,"bor":2865,"Ġspeed":2866,"iction":2867,"Ġalleg":2868,"ĠJapan":2869,"atory":2870,"reet":2871,"Ġmatch":2872,"ĠII":2873,"Ġstru":2874,"order":2875,"Ġste":2876,"Ġliving":2877,"Ġstruct":2878,"ino":2879,"Ġsepar":2880,"hern":2881,"Ġresponse":2882,"Ġenjoy":2883,"Ġvia":2884,"AD":2885,"uments":2886,"acebook":2887,"Ġmember":2888,"ibr":2889,"izing":2890,"Ġtool":2891,"ĠMon":2892,"ĠWhile":2893,"hood":2894,"ĠAng":2895,"ĠDef":2896,"Ġoffer":2897,"Tr":2898,"aur":2899,"Ġturned":2900,"ĠJuly":2901,"down":2902,"anced":2903,"Ġrecently":2904,"ĠEar":2905,"Ġce":2906,"ĠStar":2907,"ĠCong":2908,"rought":2909,"Ġblood":2910,"Ġhope":2911,"Ġcomment":2912,"aint":2913,"Ġarri":2914,"iles":2915,"Ġparticip":2916,"ought":2917,"ription":2918,"08":2919,"49":2920,"Ġgave":2921,"Ġselect":2922,"Ġkilled":2923,"sych":2924,"Ġgoes":2925,"ij":2926,"Ġcoll":2927,"Ġimpact":2928,"atives":2929,"ĠSer":2930,"09":2931,"ĠAugust":2932,"Ġboy":2933,"de":2934,"ĠDes":2935,"Ġfelt":2936,"US":2937,"Ġexpected":2938,"Ġimage":2939,"ĠMark":2940,"ccording":2941,"oice":2942,"EC":2943,"ĠMag":2944,"ened":2945,"hold":2946,"ĠPost":2947,"Ġprevent":2948,"No":2949,"Ġinvolved":2950,"Ġeyes":2951,"Ġquickly":2952,"At":2953,"unk":2954,"Ġbehav":2955,"Ġur":2956,"Ġled":2957,"come":2958,"ey":2959,"Ġcandid":2960,"Ġearlier":2961,"Ġfocus":2962,"ety":2963,"Pro":2964,"ledge":2965,"ixed":2966,"illed":2967,"Ġpopular":2968,"AP":2969,"Ġsett":2970,"light":2971,"Ġvarious":2972,"inks":2973,"Ġlevels":2974,"Ġroad":2975,"ellig":2976,"ables":2977,"hel":2978,"ittee":2979,"ĠGener":2980,"ype":2981,"Ġheard":2982,"icles":2983,"Ġmis":2984,"Ġusers":2985,"ĠSan":2986,"Ġimprove":2987,"Ġfather":2988,"Ġsearch":2989,"They":2990,"vil":2991,"Ġprofess":2992,"Ġknew":2993,"Ġloss":2994,"Ġevents":2995,"65":2996,"Ġbillion":2997,"07":2998,"02":2999,"ĠNews":3000,"ĠAM":3001,"Ġcover":3002,"where":3003,"ension":3004,"Ġbott":3005,"Ġareas":3006,"ences":3007,"ope":3008,"ĠTwitter":3009,"ael":3010,"Ġgets":3011,"ĠGoogle":3012,"Ġsn":3013,"iant":3014,"Ġvote":3015,"Ġnearly":3016,"Ġincluded":3017,"Ġrecogn":3018,"zz":3019,"mm":3020,"aled":3021,"Ġhappened":3022,"04":3023,"Ġhot":3024,"Ġwhose":3025,"Ġcivil":3026,"Ġsuff":3027,"oes":3028,"itiz":3029,"ĠSyri":3030,"Ġrespond":3031,"Ġhon":3032,"Ġfeatures":3033,"Ġeconomic":3034,"ĠApril":3035,"rim":3036,"Ġtechnology":3037,"Ġoption":3038,"aging":3039,"Ġpurch":3040,"Re":3041,"Ġlat":3042,"chie":3043,"isl":3044,"Ġrecomm":3045,"uf":3046,"Ġtraining":3047,"Ġeffects":3048,"Ġfast":3049,"Ġ2010":3050,"Ġoccur":3051,"Ġwebsite":3052,"Ġemail":3053,"Ġsens":3054,"ech":3055,"Ġoil":3056,"Ġinflu":3057,"Ġcurrently":3058,"ĠSch":3059,"ĠAdd":3060,"Ġgoal":3061,"Ġscient":3062,"Ġconv":3063,"100":3064,"emy":3065,"Ġdecided":3066,"Ġtravel":3067,"Ġmention":3068,"LL":3069,"03":3070,"Ġelection":3071,"Ġphone":3072,"Ġlooks":3073,"Ġsituation":3074,"Ġcy":3075,"Ġhor":3076,"bed":3077,"ĠCourt":3078,"aily":3079,"aves":3080,"Ġquality":3081,"ĠComp":3082,"wise":3083,"Ġtable":3084,"Ġstaff":3085,"ĠWind":3086,"ett":3087,"Ġtried":3088,"idered":3089,"Ġaddition":3090,"Ġbox":3091,"Ġlack":3092,"arily":3093,"Ġwide":3094,"Ġmid":3095,"Ġboard":3096,"ysis":3097,"Ġanti":3098,"ha":3099,"Ġdig":3100,"ening":3101,"Ġdro":3102,"Con":3103,"68":3104,"Ġslow":3105,"based":3106,"sequ":3107,"Ġpath":3108,"Ex":3109,"aker":3110,"Ġworked":3111,"Ġpen":3112,"Ġengine":3113,"Ġlooked":3114,"ĠSuper":3115,"ĠServ":3116,"Ġvictim":3117,"Un":3118,"Ġproperty":3119,"Ġintrodu":3120,"Ġexecut":3121,"ĠPM":3122,"Le":3123,"Ġcolor":3124,"ĠMore":3125,"Ġ60":3126,"Ġnetwork":3127,"Ġdate":3128,"cul":3129,"idge":3130,"Ġextra":3131,"31":3132,"Ġsle":3133,"67":3134,"Ġwond":3135,"Ġreports":3136,"just":3137,"ĠAustral":3138,"Ġcapital":3139,"Ġens":3140,"Ġcommand":3141,"Ġallowed":3142,"Ġprep":3143,"Ġcapt":3144,"hib":3145,"Ġnumbers":3146,"chan":3147,"Ġfair":3148,"mp":3149,"oms":3150,"Ġreach":3151,"With":3152,"tain":3153,"Ġbroad":3154,"Ġcouple":3155,"ecause":3156,"lying":3157,"ĠFeb":3158,"Ġscreen":3159,"Ġlives":3160,"Ġprior":3161,"ĠCongress":3162,"Ar":3163,"Ġapproach":3164,"Ġemer":3165,"aries":3166,"ĠDis":3167,"serv":3168,"ĠNe":3169,"Ġbuilt":3170,"cies":3171,"Ġrepe":3172,"Ġrules":3173,"force":3174,"ĠPal":3175,"Ġfinancial":3176,"Ġconsidered":3177,"ĠChar":3178,"nces":3179,"ĠIS":3180,"Ġbrought":3181,"Ġbi":3182,"iers":3183,"ĠSim":3184,"OP":3185,"Ġproducts":3186,"Ġvisit":3187,"Ġdocument":3188,"Ġconduct":3189,"Ġcompletely":3190,"ining":3191,"ĠCalif":3192,"ibly":3193,"Ġwritten":3194,"ĠTV":3195,"ements":3196,"Ġdraw":3197,"One":3198,"Ġpublished":3199,"Ġsecret":3200,"rain":3201,"het":3202,"ĠFacebook":3203,"onday":3204,"ĠUp":3205,"Ġsexual":3206,"Ġthous":3207,"ĠPat":3208,"Ġess":3209,"Ġstandard":3210,"Ġarm":3211,"ges":3212,"ection":3213,"Ġfell":3214,"Ġforeign":3215,"ani":3216,"ĠFriday":3217,"Ġregular":3218,"inary":3219,"Ġincreased":3220,"Ġusually":3221,"Ġdemon":3222,"Ġdark":3223,"Ġadditional":3224,"rol":3225,"ĠOf":3226,"Ġproduction":3227,"!!":3228,"undred":3229,"Ġinternational":3230,"idents":3231,"ĠFree":3232,"roup":3233,"Ġrace":3234,"Ġmach":3235,"Ġhuge":3236,"All":3237,"lear":3238,"ovember":3239,"Ġtown":3240,"Ġattention":3241,"ĠOff":3242,"yond":3243,"ĠThen":3244,"field":3245,"Ġterror":3246,"raz":3247,"ĠBo":3248,"Ġmeeting":3249,"ĠPark":3250,"Ġarrest":3251,"Ġfear":3252,"Ġaw":3253,"ĠVal":3254,"oring":3255,"',":3256,"Ġextreme":3257,"arr":3258,"Ġworkers":3259,"After":3260,"Ġ31":3261,"net":3262,"ament":3263,"Ġdirectly":3264,"Ġpopulation":3265,"ube":3266,"ĠOctober":3267,"ĠIN":3268,"ĠJanuary":3269,"59":3270,"ĠDavid":3271,"Ġcross":3272,"cember":3273,"ĠFirst":3274,"Ġmessage":3275,"irit":3276,"Ġnation":3277,"Ġpoll":3278,"isions":3279,"Ġanswer":3280,"ny":3281,"isode":3282,"Ġcarry":3283,"ĠRussia":3284,"Ġhear":3285,"ength":3286,"roy":3287,"Ġnatural":3288,"inally":3289,"Ġdog":3290,"mitted":3291,"Ġtrade":3292,"Ġsubst":3293,"Ġmultiple":3294,"ĠAfric":3295,"Ġfans":3296,"Ġsort":3297,"Ġglobal":3298,"ication":3299,"ĠWed":3300,"ara":3301,"Ġachie":3302,"Ġlanguage":3303,"vey":3304,"Ġtal":3305,"Ġnecessary":3306,"Ġdetails":3307,"Ġsen":3308,"ĠSund":3309,"ĠReg":3310,"ĠRec":3311,"06":3312,"Ġsil":3313,"ressive":3314,"Ġmedical":3315,"unch":3316,"ornia":3317,"Ġund":3318,"fort":3319,"ocks":3320,"ĠMonday":3321,"uesday":3322,"craft":3323,"77":3324,"urt":3325,"Ġver":3326,"ĠHill":3327,"Ġreceive":3328,"Ġmorning":3329,"estern":3330,"Ġbank":3331,"Ġsat":3332,"irth":3333,"ĠHigh":3334,"Ġdevice":3335,"ĠTHE":3336,"ĠCenter":3337,"Ġsafe":3338,"Ġple":3339,"ĠCanada":3340,"Ġsystems":3341,"Ġassist":3342,"Ġsurv":3343,"Ġbattle":3344,"ĠSoc":3345,"vertis":3346,"She":3347,"Ġpaper":3348,"Ġgrowth":3349,"Ġcast":3350,"Sc":3351,"Ġplans":3352,"lled":3353,"Ġparts":3354,"Ġwall":3355,"Ġmovement":3356,"Ġpractice":3357,"imately":3358,"Ġdisplay":3359,"Ġsometimes":3360,"omp":3361,"ĠPaul":3362,"ĠYes":3363,"king":3364,"58":3365,"oly":3366,"Ġson":3367,"Ġavoid":3368,"okes":3369,"ĠJew":3370,"Ġtowards":3371,"asc":3372,"Ġ//":3373,"ĠKore":3374,"Ġtalking":3375,"Ġcorrect":3376,"Ġspent":3377,"icks":3378,"iable":3379,"eared":3380,"Ġterm":3381,"Ġwants":3382,"oming":3383,"Ġut":3384,"Ġdoub":3385,"Ġforces":3386,"Ġplease":3387,"69":3388,"ĠNovember":3389,"atform":3390,"ondon":3391,"Ġones":3392,"Ġimmediately":3393,"ĠRussian":3394,"ĠMet":3395,"Ġdeg":3396,"Ġparents":3397,"CH":3398,"ĠAmericans":3399,"aly":3400,"ĠMod":3401,"Ġshown":3402,"Ġconditions":3403,"Ġstuff":3404,"Ġreb":3405,"ĠYour":3406,"Ġincludes":3407,"nown":3408,"ĠSam":3409,"Ġexperien":3410,"mission":3411,"ĠEven":3412,"aught":3413,"Ġannounced":3414,"ĠRepublican":3415,"Ġdetermin":3416,"Ġdescribed":3417,"ĠCounty":3418,"()":3419,"Ġdoor":3420,"Ġchanged":3421,"Ġneigh":3422,"ĠHere":3423,"Ġclean":3424,"Ġpan":3425,"ĠDecember":3426,"ĠEuropean":3427,"iring":3428,"apter":3429,"Ġclub":3430,"ĠTuesday":3431,"Ġpaid":3432,"ĠNet":3433,"Ġattacks":3434,"Ġcharacters":3435,"Ġalone":3436,"Ġdirector":3437,"dom":3438,"Ġ35":3439,"Ġload":3440,"Ġrout":3441,"ĠCalifornia":3442,"Ġfinally":3443,"Ġrac":3444,"Ġcontr":3445,"Ġexactly":3446,"resh":3447,"pri":3448,"ĠIslam":3449,"Ġnature":3450,"Ġcareer":3451,"Ġlatest":3452,"Ġconvers":3453,"ĠSl":3454,"pose":3455,"cient":3456,"ĠInc":3457,"ivity":3458,"88":3459,"ĠAtt":3460,"ĠMor":3461,"nesday":3462,"Ġweight":3463,"ken":3464,"Ġnote":3465,"Ġteams":3466,"Ġ\\":3467,"airs":3468,"ĠGreen":3469,"Ġhundred":3470,"onent":3471,"Ġstreng":3472,"Ġconsist":3473,"icated":3474,"Ġregul":3475,"Ġlic":3476,"astic":3477,"Ġten":3478,"ursday":3479,"elligence":3480,"ously":3481,"ĠUK":3482,"BI":3483,"Ġcosts":3484,"Ġindepend":3485,"ĠAP":3486,"Ġnormal":3487,"Ġhom":3488,"Ġobvious":3489,"Ġswe":3490,"Ġstar":3491,"Ġready":3492,"acher":3493,"Ġimplement":3494,"gest":3495,"Ġsong":3496,"ĠGet":3497,"ĠLab":3498,"Ġinteresting":3499,"using":3500,"Ġgiving":3501,"ĠSunday":3502,"Ġetc":3503,"Ġmiddle":3504,"Ġremember":3505,"right":3506,"osition":3507,"utions":3508,"Ġmax":3509,"46":3510,"Ġyourself":3511,"Ġdemand":3512,"Ġtreatment":3513,"Ġdanger":3514,"ĠCons":3515,"Ġguy":3516,"ĠBritish":3517,"Ġphysical":3518,"Ġrelated":3519,"Ġremain":3520,"Ġcouldn":3521,"Ġrefer":3522,"Ġcitiz":3523,"box":3524,"ENT":3525,"board":3526,"Ġinn":3527,"IG":3528,"ero":3529,"ĠStreet":3530,"ospital":3531,"rench":3532,"chers":3533,"Ġstra":3534,"OL":3535,"ager":3536,"ĠAN":3537,"Ġeasily":3538,"IA":3539,"enge":3540,"iny":3541,"Ġclos":3542,"ocked":3543,"Ġuses":3544,"ĠCoun":3545,"Im":3546,"uild":3547,"??":3548,"more":3549,"Ġang":3550,"Ġwrite":3551,"olute":3552,"57":3553,"Ġleader":3554,"Ġreading":3555,"</":3556,"Ġautom":3557,"ests":3558,"43":3559,"Ġlegisl":3560,"ĠGold":3561,"Ġdesigned":3562,"ĠST":3563,"ĠLeg":3564,"ares":3565,"Ġbeaut":3566,"ĠTex":3567,"Ġappears":3568,"Ġstrugg":3569,"ĠRom":3570,"Ġ00":3571,"Ġchoice":3572,"Ġparticularly":3573,"ĠFrom":3574,"oper":3575,"ĠLondon":3576,"anned":3577,"Ġallows":3578,"obile":3579,"Ġdifference":3580,"âĢ¢":3581,"ĠView":3582,"ĠWednesday":3583,"Ġalthough":3584,"Ġrelative":3585,"Ġapplication":3586,"atever":3587,"Ġaren":3588,"Ġmyself":3589,"Ġimag":3590,"Ġdise":3591,"Ġsociety":3592,"Ġfrequ":3593,"ĠEnglish":3594,"Ġpoor":3595,"ĠDay":3596,"Ġwriting":3597,"Ġseven":3598,"Ġstarting":3599,"Ġbud":3600,"Ġprint":3601,"ĠTrans":3602,"ufact":3603,"ĠStud":3604,"new":3605,"Ġcrim":3606,"Ġgives":3607,"Ġcool":3608,"ae":3609,"iance":3610,"ĠGeneral":3611,"Ġthinking":3612,"Ġsave":3613,"Ġlimited":3614,"ĠParty":3615,"Ġmeaning":3616,"pen":3617,"owers":3618,"ĠJack":3619,"EM":3620,"Ġnice":3621,"rupt":3622,"Ġgas":3623,"Ġeight":3624,"Ġfeet":3625,"Ġeffort":3626,"Ġign":3627,"icit":3628,"Bl":3629,"coin":3630,"Ġopin":3631,"Ġbrain":3632,"While":3633,"hest":3634,"ĠThursday":3635,"Ġwouldn":3636,"aughter":3637,"Ġtouch":3638,"lements":3639,"Ġstudies":3640,"Ġcenter":3641,"cont":3642,"orge":3643,"Ġcomputer":3644,"Ġinvestigation":3645,"Pl":3646,"orks":3647,"Ġ2008":3648,"Ġincreasing":3649,"Ġstore":3650,"Ġcomments":3651,"Ġbal":3652,"men":3653,"Ġdoll":3654,"Ġliber":3655,"Ġwife":3656,"Ġlaws":3657,"aturday":3658,"itness":3659,"Ġmodern":3660,"ĠSk":3661,"Ġadministration":3662,"Ġopportunity":3663,"Ġsal":3664,"Ġpowerful":3665,"My":3666,"Ġclaims":3667,"ĠEarth":3668,"ords":3669,"Ġtitle":3670,"Ġesc":3671,"name":3672,"Not":3673,"omen":3674,"Ġbeyond":3675,"Ġcamer":3676,"Ġsell":3677,"itute":3678,"earch":3679,"Ġappl":3680,"iment":3681,"42":3682,"ĠArt":3683,"Ġunf":3684,"Ġviolence":3685,"urg":3686,"ĠEast":3687,"Ġcompared":3688,"Ġoptions":3689,"Ġthroughout":3690,"Ġvs":3691,"igr":3692,".[":3693,"aches":3694,"78":3695,"Ġfiles":3696,"FL":3697,"EL":3698,"arian":3699,"ĠJames":3700,"ĠAir":3701,"anch":3702,"Ġdetail":3703,"Ġpiece":3704,"PS":3705,"Ġnamed":3706,"Ġeducation":3707,"Ġdrive":3708,"Ġitems":3709,"Ġstudent":3710,"iced":3711,"::":3712,"ico":3713,"Ġthrow":3714,"Ġscene":3715,"Ġcomplex":3716,"Ġ2009":3717,"Ġprec":3718,"ĠBre":3719,"79":3720,"Ġconcept":3721,"Ġstatus":3722,"aming":3723,"Ġdied":3724,"Ġknowledge":3725,"Ġbeginning":3726,"OD":3727,"ruary":3728,"Ġcertainly":3729,"Ġguys":3730,"Ġslight":3731,"inn":3732,"ounds":3733,"Ġfine":3734,"Ġfat":3735,"ications":3736,"Ġperhaps":3737,"ĠAnt":3738,"Ġincome":3739,"Ġhttps":3740,"Ġmajority":3741,"ports":3742,"ston":3743,"Ġgreater":3744,"Ġfeed":3745,"entially":3746,"Ġsafety":3747,"Ġunique":3748,"andom":3749,"Ġgone":3750,"Ġshowed":3751,"Ġhistor":3752,"Ġcounter":3753,"ius":3754,"ida":3755,"Ġleading":3756,"ipe":3757,"Ġsend":3758,"ĠDonald":3759,"erve":3760,"Ġdefense":3761,"inese":3762,"Ġyes":3763,"ĠFire":3764,"ĠMuslim":3765,"raq":3766,"Ġcontinued":3767,"osh":3768,"Ġprovides":3769,"Ġprison":3770,"ĠPre":3771,"Ġhappy":3772,"Ġeconomy":3773,"Ġtrust":3774,"ags":3775,"ĠGame":3776,"Ġweapons":3777,"uman":3778,"ĠCle":3779,"itation":3780,"Ġanalysis":3781,"ĠTimes":3782,"Ġscience":3783,"->":3784,"Ġfigure":3785,"Ġdisapp":3786,"enty":3787,"Ġsoftware":3788,"Ġult":3789,"Ġofficers":3790,"New":3791,"Is":3792,"Ġremains":3793,"ĠIndia":3794,"Ġpsych":3795,"rief":3796,"Ġcat":3797,"esc":3798,"Ġobserv":3799,"Ġstage":3800,"ĠDark":3801,"Ġenter":3802,"change":3803,"Ġpassed":3804,"Ġdespite":3805,"ĠOut":3806,"Ġmovie":3807,"rs":3808,"Ġvoice":3809,"mine":3810,"ĠPlay":3811,"Ġtoward":3812,"ĠTer":3813,"Ġregion":3814,"Ġvalues":3815,"orters":3816,"Ġmount":3817,"Ġofficer":3818,"ĠOther":3819,"ban":3820,"Ġhous":3821,"wood":3822,"room":3823,"IV":3824,"ĠSun":3825,"see":3826,"ĠOver":3827,"rog":3828,"90":3829,"Ġlay":3830,"ĠTur":3831,"awn":3832,"Ġpressure":3833,"ĠSub":3834,"Ġbooks":3835,"edom":3836,"ĠSand":3837,"AA":3838,"ago":3839,"Ġreasons":3840,"ford":3841,"Ġactivity":3842,"UT":3843,"Now":3844,"ĠSenate":3845,"cell":3846,"night":3847,"Ġcalls":3848,"inter":3849,"Ġletter":3850,"ĠRob":3851,"ĠJe":3852,"Ġchoose":3853,"ĠLaw":3854,"Get":3855,"Be":3856,"Ġrob":3857,"Ġtypes":3858,"Ġplatform":3859,"Ġquarter":3860,"RA":3861,"ĠTime":3862,"Ġmaybe":3863,"ĠCr":3864,"95":3865,"pre":3866,"Ġmoving":3867,"Ġlif":3868,"Ġgold":3869,"Ġsom":3870,"Ġpatients":3871,"Ġtruth":3872,"ĠKe":3873,"urance":3874,"antly":3875,"mar":3876,"Ġcharge":3877,"ĠGreat":3878,"Ġcele":3879,"--------------------------------":3880,"Ġrock":3881,"roid":3882,"ancy":3883,"Ġcredit":3884,"aud":3885,"By":3886,"ĠEvery":3887,"Ġmoved":3888,"inger":3889,"ribution":3890,"Ġnames":3891,"Ġstraight":3892,"ĠHealth":3893,"ĠWell":3894,"Ġfeature":3895,"Ġrule":3896,"Ġsche":3897,"inated":3898,"ĠMichael":3899,"berg":3900,"41":3901,"iled":3902,"band":3903,"Ġclick":3904,"ĠAngel":3905,"onents":3906,"ÂŃ":3907,"ĠIraq":3908,"ĠSaturday":3909,"Ġaware":3910,"part":3911,"Ġpattern":3912,"OW":3913,"ĠLet":3914,"Ġgrad":3915,"igned":3916,"Ġassociated":3917,"Ġstyle":3918,"no":3919,"iation":3920,"aith":3921,"ilies":3922,"Ġstories":3923,"uration":3924,"Ġindividuals":3925,"Ġâ̦":3926,"miss":3927,"ĠAssoci":3928,"ishing":3929,"aby":3930,"Ġsummer":3931,"ĠBen":3932,"Ġ32":3933,"Ġarch":3934,"uty":3935,"ĠTexas":3936,"hol":3937,"Ġfully":3938,"Ġmill":3939,"Ġfollowed":3940,"ĠBill":3941,"ĠIndian":3942,"ĠSecret":3943,"ĠBel":3944,"ĠFebruary":3945,"Ġjobs":3946,"Ġseemed":3947,"ĠGovern":3948,"ipped":3949,"Ġreality":3950,"Ġlines":3951,"Ġpark":3952,"Ġmeasure":3953,"ĠOur":3954,"IM":3955,"Ġbrother":3956,"Ġgrowing":3957,"Ġban":3958,"Ġestim":3959,"Ġcry":3960,"ĠSchool":3961,"Ġmechan":3962,"ĠOF":3963,"ĠWindows":3964,"Ġrates":3965,"ĠOh":3966,"Ġpositive":3967,"Ġculture":3968,"istics":3969,"ica":3970,"Ġhar":3971,"ya":3972,"itely":3973,"ipp":3974,"Ġmap":3975,"encies":3976,"ĠWilliam":3977,"II":3978,"akers":3979,"56":3980,"ĠMart":3981,"ĠRem":3982,"Ġaltern":3983,"itude":3984,"Ġcoach":3985,"rowd":3986,"Don":3987,"Ġkids":3988,"Ġjournal":3989,"Ġcorpor":3990,"Ġfalse":3991,"Ġweb":3992,"Ġsleep":3993,"Ġcontain":3994,"Ġsto":3995,"Ġbed":3996,"iverse":3997,"ĠRich":3998,"ĠChinese":3999,"Ġpun":4000,"Ġmeant":4001,"known":4002,"Ġnotice":4003,"Ġfavorite":4004,"aven":4005,"Ġcondition":4006,"Ġpurpose":4007,"))":4008,"Ġorganization":4009,"Ġchalleng":4010,"Ġmanufact":4011,"Ġsusp":4012,"ĠAc":4013,"Ġcritic":4014,"unes":4015,"uclear":4016,"Ġmer":4017,"vention":4018,"Ġ80":4019,"Ġmist":4020,"ĠUs":4021,"ĠTor":4022,"http":4023,"olf":4024,"Ġlarger":4025,"Ġadvant":4026,"Ġresear":4027,"Ġactions":4028,"ml":4029,"Ġkept":4030,"Ġaim":4031,",'":4032,"col":4033,"Ġbenefits":4034,"ifying":4035,"Ġactual":4036,"ĠInternational":4037,"Ġvehicle":4038,"Ġchief":4039,"Ġefforts":4040,"ĠLeague":4041,"ĠMost":4042,"Ġwait":4043,"Ġadult":4044,"Ġoverall":4045,"Ġspeech":4046,"Ġhighly":4047,"Ġfemale":4048,"Ġerror":4049,"Ġeffective":4050,"54":4051,"Ġencour":4052,"well":4053,"Ġfailed":4054,"Ġconserv":4055,"Ġprograms":4056,"Ġtrou":4057,"Ġahead":4058,"500":4059,"vertisement":4060,"IP":4061,"ĠFound":4062,"pir":4063,"Ġ%":4064,"Ġcrime":4065,"ander":4066,"Ġlocation":4067,"ĠIran":4068,"Ġbehavior":4069,"azing":4070,"Ġrare":4071,"Ġemb":4072,"Ġcaused":4073,"Ġship":4074,"Ġactive":4075,"Ġcontribut":4076,"Ġgreen":4077,"Ġacqu":4078,"Ġreflect":4079,"venue":4080,"Ġfirm":4081,"Ġbirth":4082,"].":4083,"Ġclearly":4084,"Ġemot":4085,"Ġagency":4086,"riage":4087,"Ġmemory":4088,"98":4089,"SA":4090,"ĠSee":4091,"acing":4092,"CC":4093,"Ġbiggest":4094,"Ġrap":4095,"Ġbasic":4096,"Ġband":4097,"eat":4098,"Ġsuspect":4099,"ĠMac":4100,"Ġ90":4101,"mark":4102,"istan":4103,"Ġspread":4104,"ams":4105,"ki":4106,"asy":4107,"rav":4108,"ĠRober":4109,"Ġdemonstr":4110,"rated":4111,"Ġabsolute":4112,"Ġplaces":4113,"Ġimpl":4114,"ibrary":4115,"Ġcards":4116,"Ġdestroy":4117,"Ġvirt":4118,"vere":4119,"Ġappeared":4120,"yan":4121,"point":4122,"Ġbeg":4123,"Ġtemper":4124,"spe":4125,"anted":4126,"ears":4127,"ĠDirect":4128,"Ġlength":4129,"Ġblog":4130,"amb":4131,"Ġinteg":4132,"Ġresources":4133,"acc":4134,"iful":4135,"Ġspot":4136,"Ġforced":4137,"Ġthousands":4138,"ĠMinister":4139,"Ġqual":4140,"ĠFrench":4141,"atically":4142,"Ġgenerally":4143,"Ġdrink":4144,"Ġthus":4145,"IL":4146,"odes":4147,"Ġappropri":4148,"ĠRead":4149,"Ġwhom":4150,"Ġeye":4151,"Ġcollege":4152,"Ġ45":4153,"irection":4154,"Ġensure":4155,"Ġapparent":4156,"iders":4157,"Ġreligious":4158,"Ġminor":4159,"olic":4160,"Ġtro":4161,"ĠWhy":4162,"ribute":4163,"met":4164,"Ġprimary":4165,"Ġdeveloped":4166,"Ġpeace":4167,"Ġskin":4168,"ste":4169,"ava":4170,"Ġblue":4171,"Ġfamilies":4172,"Ġir":4173,"Ġapply":4174,"Ġinform":4175,"ĠSmith":4176,"CT":4177,"ii":4178,"Ġlimit":4179,"Ġresist":4180,"................":4181,"umn":4182,"Ġconflic":4183,"Ġtwe":4184,"udd":4185,"ĠTom":4186,"Ġliter":4187,"que":4188,"bon":4189,"Ġhair":4190,"Ġeventually":4191,"Ġpus":4192,"Ġhelped":4193,"Ġagg":4194,"orney":4195,"ĠApple":4196,"Ġfit":4197,"ĠSur":4198,"Ġprem":4199,"Ġsales":4200,"Ġseconds":4201,"Ġstrength":4202,"Ġfeeling":4203,"¿½":4204,"Ġtour":4205,"Ġknows":4206,"oom":4207,"Ġexerc":4208,"Ġsomew":4209,"�":4210,">>":4211,"Ġspokes":4212,"Ġideas":4213,"Ġregist":4214,"soft":4215,"ĠDel":4216,"ĠPC":4217,"Ġpropos":4218,"Ġlaunch":4219,"Ġbottom":4220,"TH":4221,"ĠPlease":4222,"vest":4223,"itz":4224,"ĠInter":4225,"Ġscript":4226,"Ġrat":4227,"arning":4228,"Ġil":4229,"ĠJer":4230,"ĠAre":4231,"Ġwhatever":4232,"oken":4233,"cience":4234,"Ġmode":4235,"Ġagree":4236,"Ġsources":4237,"Ġinitial":4238,"Ġrestrict":4239,"Ġwonder":4240,"usion":4241,"####":4242,"ĠSil":4243,"ville":4244,"Ġburn":4245,"tw":4246,"asion":4247,"Ġ£":4248,"Ġnor":4249,"uing":4250,"Ġreached":4251,"Ġsun":4252,"Ġcateg":4253,"igration":4254,"Ġcook":4255,"Ġpromot":4256,"Ġmale":4257,"Ġclimate":4258,"Ġfix":4259,"Ġalleged":4260,"UR":4261,"alled":4262,"Ġimages":4263,"Cont":4264,"ota":4265,"Ġschools":4266,"ios":4267,"Ġdrop":4268,"Ġstream":4269,"ĠMo":4270,"Ġpreviously":4271,"aling":4272,"Ġpet":4273,"Ġdouble":4274,"Ġ(@":4275,"annel":4276,"Ġdefault":4277,"ties":4278,"Ġrank":4279,"ĠDec":4280,"ĠCouncil":4281,"Ġweapon":4282,"Ġstock":4283,"Ġanaly":4284,"ĠStr":4285,"Ġpicture":4286,"ĠPolice":4287,"ference":4288,"Ġcentury":4289,"Ġcitizens":4290,"Ġonto":4291,"Ġexpand":4292,"Ġhero":4293,"ĠSol":4294,"Ġwild":4295,"Ġupdate":4296,"Ġcustomers":4297,"ront":4298,"def":4299,"Ġlik":4300,"Ġcriminal":4301,"ĠChristian":4302,"SP":4303,"76":4304,"Ġleaving":4305,"Ġotherwise":4306,"ĠDist":4307,"Ġbasis":4308,"52":4309,"53":4310,"icip":4311,"ĠBer":4312,"Ġrecommend":4313,"Ġfloor":4314,"Ġcrowd":4315,"oles":4316,"Ġ70":4317,"Ġcentral":4318,"ĠEv":4319,"Ġdream":4320,"Ġdownload":4321,"Ġconfir":4322,"ĠThom":4323,"Ġwindow":4324,"Ġhappens":4325,"Ġunit":4326,"Ġtend":4327,"Ġspl":4328,"Ġbecomes":4329,"Ġfighting":4330,"Ġpredict":4331,"ĠPress":4332,"ĠPower":4333,"Ġheavy":4334,"aked":4335,"Ġfan":4336,"orter":4337,"ategy":4338,"BA":4339,"izes":4340,"Ġspend":4341,"Here":4342,"Ġ2007":4343,"Ġadop":4344,"ĠHam":4345,"Ġfootball":4346,"ĠPort":4347,"oday":4348,"51":4349,"ampions":4350,"Ġtransfer":4351,"ht":4352,"Ġ38":4353,"term":4354,"acity":4355,"Ġbur":4356,"],":4357,"ternal":4358,"rig":4359,"but":4360,"Ġtherefore":4361,"ĠBecause":4362,"resp":4363,"rey":4364,"Ġmission":4365,"Some":4366,"Ġnoted":4367,"Ġassum":4368,"Ġdisease":4369,"Ġedit":4370,"Ġprogress":4371,"rd":4372,"ĠBrown":4373,"ocal":4374,"Ġadding":4375,"Ġraised":4376,"ĠAny":4377,"Ġtick":4378,"Ġseeing":4379,"ĠPeople":4380,"Ġagreement":4381,"Ġserver":4382,"Ġwat":4383,"Ġdebate":4384,"Ġsupposed":4385,"iling":4386,"Ġlargest":4387,"Ġsuccessful":4388,"ĠPri":4389,"ĠDemocratic":4390,"Ġjump":4391,"ĠSyria":4392,"Ġowners":4393,"Ġoffers":4394,"Ġshooting":4395,"Ġeffic":4396,"sey":4397,"Ġhaven":4398,"verse":4399,"tered":4400,"ĠLight":4401,"imal":4402,"ĠBig":4403,"Ġdefend":4404,"Ġbeat":4405,"Ġrecords":4406,"%)":4407,"Ġscen":4408,"Ġemployees":4409,"Ġdevices":4410,"hem":4411,"Ġcommer":4412,"ĠMex":4413,"Ġbenefit":4414,"ĠProf":4415,"Ġilleg":4416,"Ġsurface":4417,"ĠAlso":4418,"Ġharm":4419,"ingly":4420,"wide":4421,"ĠAlex":4422,"Ġshut":4423,"ĠCur":4424,"Ġlose":4425,"pm":4426,"Ġchallenge":4427,"semb":4428,"Ġstation":4429,"Ġintelligence":4430,"Ġaccur":4431,"ĠFlor":4432,"Ġrequires":4433,"ĠMal":4434,"bum":4435,"Ġhospital":4436,"Ġspirit":4437,"Ġoffered":4438,"Ġproduce":4439,"ĠCommun":4440,"Ġcreating":4441,"Ġcris":4442,"spect":4443,"Ġended":4444,"Ġdaily":4445,"Ġvoters":4446,"lands":4447,"ias":4448,"ih":4449,"ona":4450,"Ġsmart":4451,"ĠOffice":4452,"ĠLord":4453,"rial":4454,"ĠInternet":4455,"Ġcircum":4456,"Ġextremely":4457,"'.":4458,"Ġopinion":4459,"ĠMil":4460,"Ġgain":4461,"BS":4462,"ĠFin":4463,"yp":4464,"Ġuseful":4465,"Ġbudget":4466,"Ġcomfort":4467,"isf":4468,"Ġbackground":4469,"eline":4470,"Ġepisode":4471,"Ġenemy":4472,"Ġtrial":4473,"Ġestablish":4474,"date":4475,"ĠCap":4476,"Ġcontinues":4477,"Ġshowing":4478,"ĠUnion":4479,"with":4480,"Ġposted":4481,"ĠSystem":4482,"Ġeat":4483,"rian":4484,"Ġrise":4485,"ĠGermany":4486,"ils":4487,"Ġsigned":4488,"Ġvill":4489,"Ġgrand":4490,"mor":4491,"ĠEngland":4492,"Ġprojects":4493,"umber":4494,"Ġconference":4495,"za":4496,"Ġresponsible":4497,"ĠArab":4498,"Ġlearned":4499,"âĢĶâĢĶ":4500,"ipping":4501,"ĠGeorge":4502,"OC":4503,"Ġreturned":4504,"ĠAustralia":4505,"Ġbrief":4506,"Qu":4507,"Ġbrand":4508,"illing":4509,"abled":4510,"Ġhighest":4511,"Ġtrain":4512,"ĠCommission":4513,"while":4514,"Ġnom":4515,"ception":4516,"Ġmut":4517,"ĠBlue":4518,"Ġincident":4519,"vant":4520,"86":4521,"ĠID":4522,"Ġnuclear":4523,"74":4524,"ĠLike":4525,"ĠRE":4526,"ĠMicro":4527,"li":4528,"mail":4529,"Ġcharges":4530,"89":4531,"Ġadjust":4532,"ado":4533,"Ġearth":4534,"NA":4535,"Ġprices":4536,"PA":4537,"Ġdraft":4538,"Ġruns":4539,"Ġcandidate":4540,"enses":4541,"Ġmanagement":4542,"ĠPhil":4543,"ĠMiss":4544,"Ġteach":4545,"gram":4546,"Ġunderstanding":4547,"ait":4548,"icago":4549,"Add":4550,"ĠEp":4551,"secut":4552,"Ġseparate":4553,"Ġinstance":4554,"Ġeth":4555,"Ġunless":4556,"********":4557,"ĠFore":4558,"inate":4559,"Ġoperations":4560,"Sp":4561,"Ġfaith":4562,"gar":4563,"ĠChurch":4564,"ronic":4565,"Ġconfig":4566,"osure":4567,"Ġactivities":4568,"Ġtraditional":4569,"Ġ36":4570,"Ġdirection":4571,"Ġmachine":4572,"Ġsurround":4573,"Ġpush":4574,"unction":4575,"ĠEU":4576,"Ġeasier":4577,"Ġargument":4578,"GB":4579,"Ġmicro":4580,"Ġspending":4581,"izations":4582,"Ġtheory":4583,"adow":4584,"Ġcalling":4585,"ĠLast":4586,"Ġder":4587,"Ġinfluence":4588,"Ġcommit":4589,"Ġphoto":4590,"Ġunc":4591,"istry":4592,"gn":4593,"aste":4594,"acks":4595,"Ġdisp":4596,"ady":4597,"do":4598,"ĠGood":4599,"Ġ`":4600,"Ġwish":4601,"Ġrevealed":4602,"³³":4603,"lig":4604,"Ġenforce":4605,"ĠCommittee":4606,"Ġchem":4607,"Ġmiles":4608,"Ġinterested":4609,"Ġsolution":4610,"icy":4611,"inct":4612,"Ġ->":4613,"ĠDet":4614,"Ġremoved":4615,"Ġcompar":4616,"eah":4617,"Ġplant":4618,"ĠSince":4619,"Ġachieve":4620,"Ġadvantage":4621,"Ġslightly":4622,"bing":4623,"Ġplaced":4624,"under":4625,"2015":4626,"ĠMad":4627,"Ġtim":4628,"oses":4629,"Ġcru":4630,"ĠRock":4631,"Ġmostly":4632,"Ġnegative":4633,"Ġsetting":4634,"Ġproduced":4635,"Ġmur":4636,"Ġconnection":4637,"ĠMer":4638,"Ġdriver":4639,"Ġexecutive":4640,"Ġassault":4641,"Ġborn":4642,"ĠVer":4643,"tained":4644,"Ġstructure":4645,"Ġreduce":4646,"Ġdecades":4647,"Ġded":4648,"uke":4649,"ĠMany":4650,"idden":4651,"Ġleague":4652,"Se":4653,"Ġjoin":4654,"Ġdisco":4655,"Ġdie":4656,"cks":4657,"actions":4658,"Ġassess":4659,"agn":4660,"Ġgoals":4661,"ours":4662,"IR":4663,"Ġsenior":4664,"iller":4665,"mod":4666,"ipment":4667,"ocol":4668,"uy":4669,"ĠQue":4670,"Ġparties":4671,"irgin":4672,"Ġlearning":4673,"itable":4674,"Ġstreet":4675,"Ġcamera":4676,"App":4677,"Ġskills":4678,"bre":4679,"cious":4680,"Ġcelebr":4681,"ĠFranc":4682,"Ġexisting":4683,"Ġwilling":4684,"lor":4685,"Ġid":4686,"ĠSpace":4687,"Ġcritical":4688,"ĠLa":4689,"ortunately":4690,"Ġserve":4691,"Ġcold":4692,"Ġspecies":4693,"TS":4694,"Ġanimals":4695,"ĠBay":4696,"Ġolder":4697,"ĠUnder":4698,"estic":4699,"ĠTre":4700,"Ġteacher":4701,"Ġprefer":4702,"vis":4703,"Ġthread":4704,"ĠMatt":4705,"Ġmanager":4706,"ãĥ»":4707,"Ġprofessional":4708,"ĠVol":4709,"Ġnotes":4710,"These":4711,"ula":4712,"Ġfresh":4713,"ented":4714,"uzz":4715,"edy":4716,"clusion":4717,"ĠRel":4718,"Ġdoubt":4719,"EO":4720,"Ġopened":4721,"ĠBit":4722,"Advertisement":4723,"Ġguess":4724,"ĠUN":4725,"Ġsequ":4726,"Ġexplain":4727,"otten":4728,"Ġattract":4729,"aks":4730,"Ġstring":4731,"Ġcontext":4732,"ossible":4733,"ĠRepublicans":4734,"Ġsolid":4735,"Ġcities":4736,"Ġasking":4737,"Ġrandom":4738,"ups":4739,"uries":4740,"arant":4741,"dden":4742,"gl":4743,"ĠFlorida":4744,"Ġdepend":4745,"ĠScott":4746,"Ġ33":4747,"ĠiT":4748,"icon":4749,"Ġmentioned":4750,"Ġ2000":4751,"Ġclaimed":4752,"Ġdefinitely":4753,"ulf":4754,"Ġcore":4755,"Ġopening":4756,"ĠConst":4757,"which":4758,"ĠTra":4759,"AG":4760,"72":4761,"Ġbelieved":4762,"ada":4763,"Ġ48":4764,"ĠSecurity":4765,"yright":4766,"ĠPet":4767,"ĠLou":4768,"Ġholding":4769,"================":4770,"Ġice":4771,"Ġbrow":4772,"Ġauthorities":4773,"host":4774,"word":4775,"Ġscore":4776,"ĠDiv":4777,"Ġcells":4778,"Ġtransl":4779,"Ġneighbor":4780,"Ġremove":4781,"uct":4782,"Ġdistrict":4783,"ĠAccording":4784,"Ġworse":4785,"Ġconcerns":4786,"Ġpresidential":4787,"Ġpolicies":4788,"ĠHall":4789,"73":4790,"Ġhus":4791,"AY":4792,"Ġ2006":4793,"ĠJud":4794,"Ġindependent":4795,"ĠJustice":4796,"iliar":4797,"print":4798,"ighter":4799,"Ġprotection":4800,"zen":4801,"Ġsudden":4802,"house":4803,"ĠJes":4804,"PR":4805,"ĠInf":4806,"Ġbul":4807,"Ġ_":4808,"ĠService":4809,"ĠPR":4810,"Ġstrategy":4811,"ffect":4812,"Ġgirls":4813,"Ġmissing":4814,"oyal":4815,"ĠTeam":4816,"ulated":4817,"Ġdat":4818,"Ġpolitics":4819,"abor":4820,"According":4821,"Ġspell":4822,"Ġgraph":4823,"orthern":4824,"TC":4825,"Ab":4826,"Ġlabor":4827,"isher":4828,"Ġkick":4829,"ĠiTunes":4830,"Ġsteps":4831,"poses":4832,"Ġsmaller":4833,"En":4834,"bert":4835,"Ġroll":4836,"Ġresearchers":4837,"Ġclosed":4838,"Ġtransport":4839,"Ġlawy":4840,"________________":4841,"ĠChicago":4842,"Ġaspect":4843,"Ġnone":4844,"Ġmarriage":4845,"96":4846,"Ġelements":4847,"ĠFre":4848,"ĠSal":4849,"Ġdram":4850,"FC":4851,"top":4852,"equ":4853,"Ġhearing":4854,"Ġsupported":4855,"Ġtesting":4856,"cohol":4857,"Ġmassive":4858,"Ġstick":4859,"Ġguard":4860,"isco":4861,"phone":4862,"From":4863,"However":4864,"Ġborder":4865,"Ġcopy":4866,"ography":4867,"list":4868,"71":4869,"Ġowner":4870,"class":4871,"ruit":4872,"rate":4873,"ĠOnce":4874,"Ġdigital":4875,"Ġtask":4876,"ERS":4877,"Ġincred":4878,"tes":4879,"++":4880,"ĠFrance":4881,"Ġbreat":4882,"owl":4883,"Ġissued":4884,"ĠWestern":4885,"Ġdetect":4886,"Ġpartners":4887,"Ġshared":4888,"ĠCall":4889,"Ġcancer":4890,"ache":4891,"ribe":4892,"Ġexplained":4893,"Ġheat":4894,"{\"":4895,"Ġinvestment":4896,"ĠBook":4897,"Ġwood":4898,"Ġtools":4899,"ĠAlthough":4900,"Ġbelief":4901,"Ġcrisis":4902,"Ġge":4903,"ĠMP":4904,"Ġoperation":4905,"type":4906,"~~":4907,"ga":4908,"Ġcontains":4909,"anta":4910,"Ġexpress":4911,"ĠGroup":4912,"ĠJournal":4913,"ka":4914,"Ġamb":4915,"ĠUSA":4916,"Ġfinding":4917,"Ġfunding":4918,"how":4919,"Ġestablished":4920,"ideos":4921,"Ġdegree":4922,"Ġdangerous":4923,"anging":4924,"Ġfreedom":4925,"pport":4926,"outhern":4927,"Ġchurch":4928,"Ġcatch":4929,"ĠTwo":4930,"Ġpresence":4931,"ĠGuard":4932,"Up":4933,"Ġauthority":4934,"ĠProject":4935,"Ġbutton":4936,"Ġconsequ":4937,"Ġvalid":4938,"Ġweak":4939,"Ġstarts":4940,"Ġreference":4941,"ĠMem":4942,"\")":4943,"UN":4944,"orage":4945,"ĠOpen":4946,"Ġcollection":4947,"ym":4948,"gency":4949,"Ġbeautiful":4950,"ros":4951,"Ġtells":4952,"Ġwaiting":4953,"nel":4954,"Ġproviding":4955,"ĠDemocrats":4956,"Ġdaughter":4957,"Ġmaster":4958,"Ġpurposes":4959,"ĠJapanese":4960,"Ġequal":4961,"Ġturns":4962,"Ġdocuments":4963,"Ġwatching":4964,"Res":4965,"Ġran":4966,"2014":4967,"Ġreject":4968,"ĠKorea":4969,"Ġvictims":4970,"Level":4971,"erences":4972,"Ġwitness":4973,"Ġ34":4974,"Ġreform":4975,"coming":4976,"Ġoccup":4977,"Ġcaught":4978,"Ġtraffic":4979,"ading":4980,"Ġmodels":4981,"ario":4982,"Ġserved":4983,"Ġbatter":4984,"uate":4985,"ĠSecretary":4986,"Ġagreed":4987,"Ġtruly":4988,"ynam":4989,"ĠRet":4990,"Ġunits":4991,"ĠResearch":4992,"hand":4993,"azine":4994,"ĠMike":4995,"Ġvariety":4996,"otal":4997,"Ġamazing":4998,"Ġconfirmed":4999,"Ġentirely":5000,"Ġpurchase":5001,"Ġelement":5002,"Ġcash":5003,"Ġdetermine":5004,"De":5005,"Ġcars":5006,"ĠWall":5007,"âĸ":5008,"Ġviews":5009,"Ġdrugs":5010,"Ġdepartment":5011,"ĠStep":5012,"uit":5013,"Ġ39":5014,"asure":5015,"ĠClass":5016,"Ġcovered":5017,"ĠBank":5018,"Ġmere":5019,"uana":5020,"Ġmulti":5021,"Ġmix":5022,"Ġunlike":5023,"levision":5024,"Ġstopped":5025,"Ġsem":5026,"ĠGal":5027,"ules":5028,"Ġwel":5029,"ĠJohnson":5030,"la":5031,"Ġskill":5032,"Ġbecoming":5033,"rie":5034,"Ġappropriate":5035,"fe":5036,"ellow":5037,"ĠProt":5038,"ulate":5039,"ocation":5040,"Ġweekend":5041,"odies":5042,"Ġsites":5043,"Ġanimal":5044,"ĠTim":5045,"Ġscale":5046,"Ġcharged":5047,"Ġinstruct":5048,"illa":5049,"Ġmethods":5050,"Ġcert":5051,"Ġjudge":5052,"ĠHel":5053,"Ġdollars":5054,"Ġstanding":5055,"ĠSqu":5056,"Ġdebt":5057,"liam":5058,"Ġdriving":5059,"ĠSum":5060,"ĠEdition":5061,"Ġalbum":5062,"andon":5063,"IF":5064,"ĠUk":5065,"63":5066,"ader":5067,"Ġcommercial":5068,"esh":5069,"ĠGovernment":5070,"Ġdiscovered":5071,"Ġoutput":5072,"ĠHillary":5073,"ĠCarol":5074,"Ġ2005":5075,"Ġabuse":5076,"ancing":5077,"Ġswitch":5078,"Ġannual":5079,"Tw":5080,"Ġstated":5081,"agement":5082,"inner":5083,"Ġdemocr":5084,"Ġresidents":5085,"Ġallowing":5086,"Ġfactors":5087,"odd":5088,"Ġfuck":5089,"emies":5090,"Ġoccurred":5091,"oti":5092,"Ġnorth":5093,"ĠPublic":5094,"Ġinjury":5095,"Ġinsurance":5096,"CL":5097,"olly":5098,"ãĢ":5099,"Ġrepeated":5100,"Ġarms":5101,"anged":5102,"Ġconstruction":5103,"Ġfle":5104,"PU":5105,"icians":5106,"Ġforms":5107,"ĠMcC":5108,"antic":5109,"Ġmental":5110,"pire":5111,"Ġequipment":5112,"Ġfant":5113,"Ġdiscussion":5114,"Ġregarding":5115,"kin":5116,"arp":5117,"Ġchair":5118,"ogue":5119,"Ġproceed":5120,"ĠId":5121,"Our":5122,"Ġmurder":5123,"Man":5124,"Ġ49":5125,"asp":5126,"Ġsupply":5127,"Ġinput":5128,"Ġwealth":5129,"liament":5130,"Ġproced":5131,"orial":5132,"ĠStat":5133,"ĠNFL":5134,"hens":5135,"ĠInstitute":5136,"Ġputting":5137,"ournament":5138,"etic":5139,"Ġlocated":5140,"Ġkid":5141,"eria":5142,"run":5143,"Ġprinc":5144,"Ġ!":5145,"going":5146,"ĠBet":5147,"Ġclot":5148,"Ġtelling":5149,"Ġproposed":5150,"iot":5151,"orry":5152,"Ġfunds":5153,"gment":5154,"ĠLife":5155,"Ġbaby":5156,"ĠBack":5157,"Ġspoke":5158,"Image":5159,"Ġearn":5160,"ĠAT":5161,"gu":5162,"Ġexchange":5163,"ĠLin":5164,"oving":5165,"Ġpair":5166,"More":5167,"azon":5168,"Ġarrested":5169,"Ġkilling":5170,"can":5171,"ĠCard":5172,"yd":5173,"Ġidentified":5174,"Ġmobile":5175,"Ġthanks":5176,"onym":5177,"ĠForm":5178,"Ġhundreds":5179,"ĠChris":5180,"ĠCat":5181,"Ġtrend":5182,"hat":5183,"ĠAv":5184,"oman":5185,"Ġelectric":5186,"ĠWil":5187,"SE":5188,"Of":5189,"Ġrestaur":5190,"oted":5191,"Ġtrig":5192,"Ġnine":5193,"Ġbomb":5194,"Why":5195,"¯":5196,"Ġcoverage":5197,"Ġappeal":5198,"ĠRobert":5199,"ĠSup":5200,"Ġfinished":5201,"Ġflow":5202,"Ġdeliver":5203,"Ġcalcul":5204,"Ġphotos":5205,"Ġphil":5206,"Ġpieces":5207,"Ġappre":5208,"kes":5209,"Ġrough":5210,"Do":5211,"Ġpartner":5212,"Ġconcerned":5213,"Ġ37":5214,"ĠGen":5215,"Col":5216,"ctors":5217,"Ġ=>":5218,"state":5219,"Ġsuggested":5220,"ĠForce":5221,"CE":5222,"Ġherself":5223,"ĠPlan":5224,"works":5225,"ooth":5226,"rency":5227,"Ġcorner":5228,"Ġhusband":5229,"Ġinternet":5230,"ĠAut":5231,"ems":5232,"osen":5233,"ĠAtl":5234,"gen":5235,"Ġbalance":5236,"62":5237,"Ġsounds":5238,"text":5239,"Ġarr":5240,"oves":5241,"Ġmillions":5242,"Ġradio":5243,"Ġsatisf":5244,"ĠDam":5245,"Mr":5246,"Go":5247,"Spe":5248,"Ġcombat":5249,"rant":5250,"ĠGree":5251,"Ġfuel":5252,"Ġdistance":5253,"Ġtests":5254,"Ġdecre":5255,"ĠEr":5256,"Ġmanaged":5257,"DS":5258,"Ġtit":5259,"Ġmeasures":5260,"ĠLiber":5261,"Ġattend":5262,"ashed":5263,"ĠJose":5264,"ĠNight":5265,"dit":5266,"ĠNov":5267,"ĠEnd":5268,"outs":5269,"Ġgeneration":5270,"Ġadvoc":5271,"yth":5272,"Ġconversation":5273,"ĠSky":5274,"active":5275,"cel":5276,"rier":5277,"ĠFrank":5278,"Ġgender":5279,"Ġconcent":5280,"Ġcarried":5281,"anda":5282,"ĠVirgin":5283,"Ġarrived":5284,"icide":5285,"aded":5286,"Ġfailure":5287,"Ġminimum":5288,"lets":5289,"Ġworst":5290,"Ġkeeping":5291,"Ġintended":5292,"Ġillegal":5293,"Ġsubsc":5294,"Ġdetermined":5295,"Ġtrip":5296,"Yes":5297,"Ġraise":5298,"Ġ~":5299,"Ġfeels":5300,"Ġpackage":5301,"ĠJo":5302,"hi":5303,"2016":5304,"real":5305,"Ġfra":5306,"Ġsymb":5307,"Me":5308,"ucky":5309,"pret":5310,"ĠKh":5311,"ĠEdit":5312,"ĠWeb":5313,"emic":5314,"ĠColor":5315,"Ġjustice":5316,"Int":5317,"Ġfarm":5318,"cknow":5319,"\">":5320,"eless":5321,"Ġreduced":5322,"Ġ500":5323,"xx":5324,"ĠRad":5325,"ĠWood":5326,"Ġclin":5327,"Ġhyp":5328,"iler":5329,"ura":5330,"kins":5331,"85":5332,"61":5333,"ĠTheir":5334,"ĠMary":5335,"Ġsan":5336,"Ġnovel":5337,"ĠWho":5338,"Ġcapacity":5339,"Ġimpossible":5340,"Ġplays":5341,"Ġminister":5342,"ijuana":5343,"icate":5344,"ĠSet":5345,"Ġfram":5346,"Ġing":5347,"Ġcommunities":5348,"ĠFBI":5349,"ita":5350,"Ġbon":5351,"Ġstrateg":5352,"Ġinterests":5353,"lock":5354,"gers":5355,"mas":5356,"ĠAND":5357,"Ġconflict":5358,"Ġrequirements":5359,"Ġsac":5360,"Ġoperating":5361,"ini":5362,"related":5363,"Ġcommitted":5364,"Ġrelatively":5365,"Ġsouth":5366,"¯¯":5367,"Ġafford":5368,"Ġidentity":5369,"Ġdecisions":5370,"Ġaccused":5371,"place":5372,"Ġvictory":5373,"och":5374,"iat":5375,"Name":5376,"Com":5377,"tion":5378,"eds":5379,"Ġseek":5380,"Ġtight":5381,"ĠImages":5382,"Ġiniti":5383,"Ġhumans":5384,"Ġfamiliar":5385,"Ġaudience":5386,"Ġinternal":5387,"venture":5388,"Ġsides":5389,"ĠTO":5390,"Ġdim":5391,"Ġconclud":5392,"Ġappoint":5393,"Ġenforcement":5394,"ĠJim":5395,"ĠAssociation":5396,"Ġcircumst":5397,"ĠCanadian":5398,"Ġjoined":5399,"Ġdifferences":5400,"ĠLos":5401,"Ġprotest":5402,"Ġtwice":5403,"win":5404,"Ġglass":5405,"arsh":5406,"ĠArmy":5407,"Ġexpression":5408,"Ġdecide":5409,"Ġplanning":5410,"ania":5411,"Ġhandle":5412,"ĠMicrosoft":5413,"ĠNor":5414,"Ġmaximum":5415,"ĠRev":5416,"Ġsea":5417,"Ġeval":5418,"Ġhelps":5419,"ref":5420,"Ġbound":5421,"Ġmouth":5422,"Ġstandards":5423,"Ġclim":5424,"ĠCamp":5425,"ĠFox":5426,"cles":5427,"Ġarmy":5428,"ĠTechn":5429,"acking":5430,"xy":5431,"SS":5432,"Ġ42":5433,"Ġbug":5434,"ĠUkrain":5435,"ĠMax":5436,"ĠJones":5437,"ĠShow":5438,"lo":5439,"Ġplanet":5440,"Ġ75":5441,"Ġwinning":5442,"Ġfaster":5443,"Ġspect":5444,"Ġbroken":5445,"TR":5446,"Ġdefined":5447,"Ġhealthy":5448,"Ġcompetition":5449,"https":5450,"ĠIsland":5451,"ĠFe":5452,"Ġannounce":5453,"ĠCup":5454,"ĠInstead":5455,"Ġclient":5456,"Ġpossibly":5457,"section":5458,"ocket":5459,"look":5460,"Ġfinish":5461,"Ġcrew":5462,"Ġreserv":5463,"Ġeditor":5464,"Ġhate":5465,"Ġsale":5466,"Ġcontrovers":5467,"Ġpages":5468,"wing":5469,"Ġnumer":5470,"Ġopposition":5471,"Ġ2004":5472,"Ġrefuge":5473,"Ġflight":5474,"Ġapart":5475,"ĠLat":5476,"Americ":5477,"ĠAfrica":5478,"Ġapplications":5479,"ĠPalest":5480,"ĠBur":5481,"Ġgar":5482,"ĠSocial":5483,"Ġupgr":5484,"Ġshape":5485,"Ġspeaking":5486,"ansion":5487,"ao":5488,"ĠSn":5489,"Ġworry":5490,"ĠBritain":5491,"Please":5492,"roud":5493,"Ġhun":5494,"Ġintroduced":5495,"Ġdiet":5496,"Ind":5497,"ĠSecond":5498,"Ġfunctions":5499,"uts":5500,"ĠEach":5501,"ĠJeff":5502,"Ġstress":5503,"Ġaccounts":5504,"Ġguarant":5505,"ĠAnn":5506,"edia":5507,"Ġhonest":5508,"Ġtree":5509,"ĠAfrican":5510,"ĠBush":5511,"},":5512,"Ġsch":5513,"ĠOnly":5514,"Ġfif":5515,"igan":5516,"Ġexercise":5517,"ĠExp":5518,"Ġscientists":5519,"Ġlegislation":5520,"ĠWork":5521,"ĠSpr":5522,"ÃĤ":5523,"ĠHuman":5524,"Ġè":5525,"Ġsurvey":5526,"Ġrich":5527,"rip":5528,"Ġmaintain":5529,"Ġflo":5530,"Ġleadership":5531,"stream":5532,"ĠIslamic":5533,"Ġ01":5534,"ĠCollege":5535,"Ġmagic":5536,"ĠPrime":5537,"Ġfigures":5538,"2017":5539,"inder":5540,"xual":5541,"ĠDead":5542,"Ġabsolutely":5543,"Ġfourth":5544,"Ġpresented":5545,"respond":5546,"rible":5547,"Ġalcohol":5548,"ato":5549,"ĠDE":5550,"porary":5551,"Ġgrab":5552,"Ġvari":5553,"Ġquant":5554,"ĠPhoto":5555,"Ġplus":5556,"rick":5557,"arks":5558,"Ġalternative":5559,"Ġpil":5560,"Ġapprox":5561,"that":5562,"Ġobjects":5563,"ĠRo":5564,"ĠAndroid":5565,"Ġsignificantly":5566,"ĠRoad":5567,"kay":5568,"Read":5569,"avor":5570,"Ġacknow":5571,"ĠHD":5572,"ĠSing":5573,"Or":5574,"ĠMont":5575,"Ġuns":5576,"prof":5577,"Ġnegoti":5578,"ĠArch":5579,"iki":5580,"Ġtelevision":5581,"ĠJewish":5582,"Ġcommittee":5583,"Ġmotor":5584,"Ġappearance":5585,"Ġsitting":5586,"Ġstrike":5587,"ĠDown":5588,"comp":5589,"ĠHist":5590,"Ġfold":5591,"acement":5592,"ĠLouis":5593,"Ġbelong":5594,"ĠâĢ¢":5595,"Ġmort":5596,"Ġprepared":5597,"Ġ64":5598,"ĠMaster":5599,"Ġindeed":5600,"ĠDen":5601,"Ġrent":5602,"TA":5603,"ourney":5604,"arc":5605,"Su":5606,"97":5607,"Ġadvice":5608,"Ġchanging":5609,"Ġlisted":5610,"Ġlaunched":5611,"isation":5612,"ĠPeter":5613,"ishes":5614,"Ġlived":5615,"ĠMel":5616,"ĠSupreme":5617,"ĠFederal":5618,"Ġ);":5619,"ructure":5620,"Ġsets":5621,"Ġphilos":5622,"uous":5623,"ĠÂł":5624,"Ġapplied":5625,"ĠNOT":5626,"Ġhousing":5627,"ĠMount":5628,"Ġodd":5629,"Ġsust":5630,"DA":5631,"fficient":5632,"Ġ?":5633,"olved":5634,"Ġpowers":5635,"Ġthr":5636,"Ġremaining":5637,"ĠWater":5638,"LC":5639,"Ġcauses":5640,"ãģ®":5641,"Ġmanner":5642,"ads":5643,"Ġsuggests":5644,"Ġends":5645,"standing":5646,"fig":5647,"ĠDun":5648,"idth":5649,"Ġgay":5650,"Ġtermin":5651,"ĠAngeles":5652,"MS":5653,"Ġscientific":5654,"Ġcoal":5655,"apers":5656,"bar":5657,"ĠThomas":5658,"Ġsym":5659,"ĠRun":5660,"this":5661,"PC":5662,"igrants":5663,"Ġminute":5664,"ĠDistrict":5665,"cellent":5666,"Ġleaves":5667,"Ġcompleted":5668,"amin":5669,"Ġfocused":5670,"Ġmonitor":5671,"Ġvehicles":5672,"MA":5673,"ĠMass":5674,"ĠGrand":5675,"Ġaffected":5676,"itutional":5677,"Ġconstruct":5678,"Ġfollows":5679,"Ġton":5680,"reens":5681,"Ġhomes":5682,"ĠExt":5683,"ĠLevel":5684,"rast":5685,"ĠIr":5686,"Ġelim":5687,"Ġlargely":5688,"ĠJoe":5689,"Ġvotes":5690,"alls":5691,"Ġbusinesses":5692,"ĠFoundation":5693,"ĠCentral":5694,"Ġyards":5695,"Ġmaterials":5696,"ulner":5697,"Ġguide":5698,"Ġcloser":5699,"ums":5700,"Ġsports":5701,"eder":5702,"Just":5703,"Ġtaxes":5704,"84":5705,"ĠOld":5706,"Ġdecade":5707,"ola":5708,"Ġvir":5709,"Ġdropped":5710,"Ġdelay":5711,"itect":5712,"Ġsecure":5713,"stein":5714,"level":5715,"Ġtreated":5716,"Ġfiled":5717,"aine":5718,"Ġvan":5719,"Ġmir":5720,"Ġcolumn":5721,"icted":5722,"eper":5723,"Ġrot":5724,"Ġconsult":5725,"Ġentry":5726,"Ġmarijuana":5727,"ĠDou":5728,"Ġapparently":5729,"oking":5730,"clusive":5731,"Ġincreases":5732,"ano":5733,"Ġspecifically":5734,"Ġtele":5735,"ensions":5736,"Ġreligion":5737,"abilities":5738,"Ġframe":5739,"ĠNote":5740,"ĠLee":5741,"Ġhelping":5742,"Ġedge":5743,"oston":5744,"Ġorganizations":5745,"Ãĥ":5746,"ĠBoth":5747,"hips":5748,"Ġbigger":5749,"Ġboost":5750,"ĠStand":5751,"Ġrow":5752,"uls":5753,"abase":5754,"Ġrid":5755,"Let":5756,"aren":5757,"rave":5758,"Ġstret":5759,"PD":5760,"Ġvision":5761,"Ġwearing":5762,"Ġappreci":5763,"Ġaward":5764,"ĠUse":5765,"Ġfactor":5766,"war":5767,"ulations":5768,")(":5769,"Ġgod":5770,"Ġterrit":5771,"Ġparam":5772,"asts":5773,"87":5774,"Ġenemies":5775,"ĠGames":5776,"FF":5777,"Ġaccident":5778,"Well":5779,"ĠMartin":5780,"TER":5781,"Ġath":5782,"ĠHell":5783,"Ġforg":5784,"Ġveter":5785,"ĠMedic":5786,"free":5787,"Ġstars":5788,"Ġexpensive":5789,"Ġacad":5790,"rawn":5791,"ĠWhe":5792,"Ġlock":5793,"Ġformat":5794,"Ġsoldiers":5795,"sm":5796,"Ġagent":5797,"Ġresponsibility":5798,"ora":5799,"ĠScience":5800,"Ġrapid":5801,"Ġtough":5802,"ĠJesus":5803,"Ġbelieves":5804,"ML":5805,"Ġwear":5806,"lete":5807,"ÃĥÃĤ":5808,"ĠDri":5809,"Ġcommission":5810,"ĠBob":5811,"Oh":5812,"aped":5813,"Ġwarm":5814,"ÃĥÃĤÃĥÃĤ":5815,"Ġ2003":5816,"ortion":5817,"Ġhasn":5818,"uster":5819,"Ġunivers":5820,"ĠIll":5821,"Ġking":5822,"ologies":5823,"94":5824,"ĠTem":5825,"ĠMos":5826,"Ġpatient":5827,"ĠMexico":5828,"cean":5829,"ĠDeath":5830,"ĠSanders":5831,"you":5832,"ĠCast":5833,"ĠCompany":5834,"pty":5835,"Ġhappening":5836,"FP":5837,"ĠBattle":5838,"Ġbought":5839,"Am":5840,"Mod":5841,"Us":5842,"uters":5843,"ĠCre":5844,"ĠThose":5845,"Ġ44":5846,"iser":5847,"Ġsoul":5848,"ĠTop":5849,"ĠHarry":5850,"ĠAw":5851,"Ġseat":5852,"ffee":5853,"Ġrevolution":5854,"Ġ(\"":5855,"ĠDuring":5856,"ette":5857,"Ġring":5858,"Ġoffensive":5859,"Ġreturns":5860,"Ġvideos":5861,"Ġdiscl":5862,"Ġfamous":5863,"enced":5864,"ĠSign":5865,"ĠRiver":5866,"Ġ300":5867,"PM":5868,"ĠBus":5869,"ĠCH":5870,"Ġcandidates":5871,"arden":5872,"Ġpercentage":5873,"Ġvisual":5874,"Ġthank":5875,"Ġtrouble":5876,"nergy":5877,"Ġ2001":5878,"Ġprove":5879,"ashion":5880,"Ġenh":5881,"ĠLong":5882,"UM":5883,"Ġconnected":5884,"Ġpossibility":5885,"Over":5886,"Ġexpert":5887,"Ġlibrary":5888,"arts":5889,"ĠDirector":5890,"Ġfellow":5891,"92":5892,"irty":5893,"Ġdry":5894,"Ġsigns":5895,"ĠLove":5896,"Ġquiet":5897,"foot":5898,"Ġpure":5899,"ĠHun":5900,"Ġfilled":5901,"phas":5902,"ĠElect":5903,"endment":5904,"ĠExpl":5905,"Ġunable":5906,"ns":5907,"mo":5908,"Ġvast":5909,"obe":5910,"Ġidentify":5911,"apping":5912,"ĠCarolina":5913,"gress":5914,"Ġprote":5915,"Ġfish":5916,"Ġcircumstances":5917,"razy":5918,"ĠPhot":5919,"Ġbodies":5920,"ĠMur":5921,"Ġdeveloping":5922,"ĠAR":5923,"Ġexperienced":5924,"Ġsubstant":5925,"ĠBoard":5926,"esome":5927,"Ġdomestic":5928,"Ġcombined":5929,"ĠPut":5930,"Ġchemical":5931,"ĠChild":5932,"Ġpool":5933,"ĠCy":5934,"Ġegg":5935,"cons":5936,"sters":5937,"Ġhurt":5938,"Ġmarkets":5939,"Ġconservative":5940,"Ġsupporters":5941,"Ġagencies":5942,"idel":5943,"Ob":5944,"urb":5945,"Ġ43":5946,"ĠDefense":5947,"ye":5948,"ĠAp":5949,"dule":5950,"Ġtemperature":5951,"Ġconducted":5952,"ĠChief":5953,"Ġpulled":5954,"Ġfol":5955,"Last":5956,"onto":5957,"osis":5958,"VER":5959,"Des":5960,"ĠPan":5961,"First":5962,"Ġadvance":5963,"Ġlicense":5964,"rors":5965,"ĠJon":5966,"Ġimagine":5967,"Ġhell":5968,"Ġfixed":5969,"Ġincor":5970,"osite":5971,"ĠLog":5972,"icken":5973,"]:":5974,"Ġsurprise":5975,"hab":5976,"Ġcraft":5977,"olt":5978,"ĠJul":5979,"Ġdial":5980,"Ġrelevant":5981,"Ġentered":5982,"Ġleads":5983,"ĠAD":5984,"ĠClean":5985,"Ġpictures":5986,"essor":5987,"Ġalt":5988,"Ġpaying":5989,"Per":5990,"ĠMarket":5991,"Ġupdates":5992,"amily":5993,"ĠType":5994,"ĠHome":5995,"Ġ55":5996,"sembly":5997,"rome":5998,"83":5999,"Ġgreatest":6000,"Ġheight":6001,"Ġheav":6002,"aints":6003,"Ġlisten":6004,"aser":6005,"ĠSH":6006,"Ġcapable":6007,"acle":6008,"Ġperspect":6009,"inating":6010,"Ġoffering":6011,"rypt":6012,"ĠDevelop":6013,"abin":6014,"rc":6015,"Ġbright":6016,"alty":6017,"arrow":6018,"Ġsuppl":6019,"inding":6020,"acked":6021,"gypt":6022,"ĠAnother":6023,"pg":6024,"ĠVirginia":6025,"ĠLu":6026,"Ġplanned":6027,"Ġpit":6028,"Ġsweet":6029,"Type":6030,"ĠDi":6031,"Ġtypically":6032,"ĠFrancisco":6033,"Ġprospect":6034,"ĠDan":6035,"Ġteen":6036,"rees":6037,"Ġsched":6038,"Ġhol":6039,"Ġscr":6040,"Ġlots":6041,"life":6042,"Ġnewsp":6043,"Ġforget":6044,"ĠNone":6045,"ĠMiddle":6046,"ĠRyan":6047,"edd":6048,"Ġsevere":6049,"Ġsuit":6050,"ller":6051,"93":6052,"Ġcorrespond":6053,"Ġexplos":6054,"uations":6055,"Ġflag":6056,"game":6057,"rid":6058,"Ġprin":6059,"ĠData":6060,"Ġdeploy":6061,"ĠEnter":6062,"suit":6063,"ghan":6064,"ĠMen":6065,"Ġthoughts":6066,"Ġmatters":6067,"Ġadapt":6068,"ĠAri":6069,"Ġfill":6070,"Ġforth":6071,"Ġsam":6072,"Ġ41":6073,"Ġpayment":6074,"ĠHor":6075,"Ġspring":6076,"duc":6077,"Ġlosing":6078,"Ġbringing":6079,"FO":6080,"ala":6081,"Ġdistribution":6082,"hered":6083,"bour":6084,"ĠIsraeli":6085,"oma":6086,"Ġcombination":6087,"Ġplenty":6088,"VE":6089,"Can":6090,"ĠHaw":6091,"Ġperman":6092,"ĠSpecial":6093,"Ġtow":6094,"Ġseeking":6095,"Ġexamples":6096,"Ġclasses":6097,"cr":6098,"Ġbeer":6099,"Ġmoves":6100,"ĠIP":6101,"ĠKn":6102,"Ġpanel":6103,"Even":6104,"Ġproperly":6105,"Ġris":6106,"Ġplug":6107,"Ġestimated":6108,"Every":6109,"Ġdefensive":6110,"agraph":6111,"Ġpregn":6112,"Ġinstit":6113,"ĠVict":6114,"Ġvolume":6115,"Ġpositions":6116,"Ġlinks":6117,"ĠProgram":6118,"ĠWeek":6119,"agues":6120,"Ġtransform":6121,"ker":6122,"ĠCEO":6123,"Ġcas":6124,"Ġopponent":6125,"Ġtweet":6126,"ĠCode":6127,"Ġshop":6128,"Ġfly":6129,"Ġtalks":6130,"Ġbag":6131,"Phone":6132,"Ġaid":6133,"Ġplants":6134,"Ġ65":6135,"Ġattorney":6136,"arters":6137,"quest":6138,"ĠMagic":6139,"Ġbegins":6140,"Ġmyster":6141,"Ġenvironmental":6142,"Ġstorage":6143,"NN":6144,"Ġmarg":6145,"Ġske":6146,"Ġmetal":6147,"elly":6148,"Ġordered":6149,"Ġremained":6150,"Ġloved":6151,"Ġprompt":6152,"Ġupdated":6153,"Ġexperts":6154,"Ġwalking":6155,"Ġancient":6156,"Ġperformed":6157,"ATE":6158,"Ġneither":6159,"iency":6160,"Ġmanufacture":6161,"ĠPak":6162,"Ġselected":6163,"Ġmine":6164,"Ġultimately":6165,"Ġexplan":6166,"Ġlabel":6167,"ĠServices":6168,"ributed":6169,"Trump":6170,"Ġsyn":6171,"ĠUlt":6172,"SC":6173,"Ġmeat":6174,"Ġgiant":6175,"ĠWars":6176,"ĠON":6177,"Ġadm":6178,"Ġinterpret":6179,"Ġevening":6180,"Ġevil":6181,"ĠBoston":6182,"ĠWild":6183,"ĠÃ":6184,"ĠBitcoin":6185,"ĠAmazon":6186,"Dr":6187,"ĠInformation":6188,"Ġobviously":6189,"Ġadvanced":6190,"Photo":6191,"olar":6192,"Ġweather":6193,"Ġsymbol":6194,"Ġsole":6195,"Ġpotentially":6196,"oster":6197,"Ġoriginally":6198,"mun":6199,"300":6200,"aze":6201,"essions":6202,"Ġdeck":6203,"Ġstood":6204,"Ġyouth":6205,"ĠBern":6206,"Rep":6207,"ĠTest":6208,"Ġbasically":6209,"otic":6210,"Ġinvolve":6211,"olit":6212,"lyn":6213,"See":6214,"Ġaircraft":6215,"Ġconfirm":6216,"EW":6217,"Ġmessages":6218,"ĠRichard":6219,"Ġkit":6220,"Ġprohib":6221,"Ġvulner":6222,"isters":6223,"Ġexistence":6224,"Ġturning":6225,"ĠSP":6226,"Ġdesire":6227,"Ġflat":6228,"Ġment":6229,"season":6230,"anges":6231,"Ġneighborhood":6232,"ĠLake":6233,"ATION":6234,"Ġpointed":6235,"bur":6236,"Ġinnov":6237,"ucks":6238,"UL":6239,"Ġprofessor":6240,"Ġexpressed":6241,"AB":6242,"icious":6243,"Ġ2002":6244,"ĠDev":6245,"Ġsession":6246,"Ġbare":6247,"sen":6248,"Ġdiss":6249,"ĠCath":6250,"ĠPass":6251,"ĠPoint":6252,"Ġdoctor":6253,"orrow":6254,"ailed":6255,"ĠRub":6256,"ĠDC":6257,"ĠCharl":6258,"person":6259,"Ġwriter":6260,"ighters":6261,"ureau":6262,"Ġoblig":6263,"Ġrecorded":6264,"Ġbroke":6265,"Ġorders":6266,"ilty":6267,"Ġmotion":6268,"inity":6269,"law":6270,"adium":6271,"Ġimmigration":6272,"Ġcontrast":6273,"Ġbatt":6274,"Ġexcellent":6275,"Ġtechnical":6276,"ami":6277,"Ġtun":6278,"Ġcloud":6279,"ĠYear":6280,"geon":6281,"Ġcreation":6282,"Ġstrange":6283,"Ġauth":6284,"Ġfort":6285,"born":6286,"Ġextent":6287,"ĠToday":6288,"ĠClub":6289,"Ġrain":6290,"Ġsample":6291,"Ġaccepted":6292,"Ġtact":6293,"Ġfired":6294,"ĠSon":6295,"Ġstands":6296,"Ġboot":6297,"Ġ47":6298,"Ġstatements":6299,"Ġversions":6300,"Ġselling":6301,"ounded":6302,"Ġ1990":6303,"Ġweren":6304,"ĠWatch":6305,"Ġexperiment":6306,"Post":6307,"Ġretail":6308,"uled":6309,"Inst":6310,"unte":6311,"ãĥ¼":6312,"Ġdepart":6313,"Ġbond":6314,"ivery":6315,"ompl":6316,"Ġreaction":6317,"ĠSyrian":6318,"ĠPac":6319,"apped":6320,"aniel":6321,"DP":6322,"Ġresolution":6323,"Ġreact":6324,"Ġapproved":6325,"onom":6326,"mond":6327,"ĠOffic":6328,"---":6329,"Ġreplace":6330,"Ġtack":6331,"Ġsport":6332,"Ġchain":6333,"Ġemergency":6334,"rad":6335,"ĠPalestin":6336,"Ġ46":6337,"Ġautomatically":6338,"Ġroute":6339,"Ġpal":6340,"Ġbanks":6341,"ĠParis":6342,"ĠMedia":6343,"road":6344,"icing":6345,"ixt":6346,"isted":6347,"Ġgrew":6348,"Ġcoord":6349,"ĠWhere":6350,"omin":6351,"Ġsubs":6352,"��":6353,"Ġ±":6354,"Ġcorporate":6355,"Ġselection":6356,"noon":6357,"ĠReport":6358,"cs":6359,"cluding":6360,"orders":6361,"anche":6362,"ĠIts":6363,"Ġslowly":6364,"ĠEgypt":6365,"ĠAcc":6366,"Ġcolle":6367,"iques":6368,"EX":6369,"Ġattempts":6370,"url":6371,"ĠCross":6372,"Ġfindings":6373,"ĠSC":6374,"ĠOR":6375,"Ġindex":6376,"ensity":6377,"ĠWay":6378,"ĠLand":6379,"Ġshock":6380,"dis":6381,"Ġdynam":6382,"Ġcart":6383,"mosp":6384,"Since":6385,"iest":6386,"ĠBoy":6387,"Ġstorm":6388,"ĠContin":6389,"2013":6390,"hew":6391,"ilit":6392,"Ġessential":6393,"iquid":6394,"Other":6395,"ivered":6396,"Ġreasonable":6397,"Act":6398,"Ġsubsequ":6399,"ĠPack":6400,"ĠFort":6401,"Ġconsidering":6402,"Ġuniversity":6403,"log":6404,"Ġmarried":6405,"Ġillust":6406,"ĠTrue":6407,"£ı":6408,"Ġnumerous":6409,"rastructure":6410,"Ġseriously":6411,"Ġreferred":6412,"ua":6413,"Ġconsistent":6414,"onna":6415,"ĠReal":6416,"ruption":6417,"ciples":6418,"Ġfacts":6419,"91":6420,"otes":6421,"erg":6422,"Then":6423,"Ġaccompl":6424,"Note":6425,"Ġrevenue":6426,"Ġpassing":6427,"Ġmal":6428,"een":6429,"ĠYet":6430,"Ġgather":6431,"terday":6432,"ework":6433,"ĠAuthor":6434,"Pe":6435,"Ġoptim":6436,"Ġrub":6437,"Ġè£ı":6438,"Ġunknown":6439,"stone":6440,"Ġunion":6441,"olve":6442,"Ġopportunities":6443,"Ġbrowser":6444,"ĠWal":6445,"ĠCost":6446,"Ġreporting":6447,"sts":6448,"pet":6449,"Ġsand":6450,"Ġsuddenly":6451,"Ġsurprising":6452,"ĠVR":6453,"Ġsomewhat":6454,"ĠBas":6455,"ulture":6456,"izz":6457,"ĠCD":6458,"Ġchallenges":6459,"Ġsettings":6460,"Ġexperiences":6461,"ĠFull":6462,"Ġcann":6463,"Ġreceiving":6464,"EST":6465,"Ġjoint":6466,"Ġcultural":6467,"Ġast":6468,"82":6469,"astern":6470,"ceived":6471,"ĠCru":6472,"Ġbull":6473,"pired":6474,"amm":6475,"Ġfacing":6476,"power":6477,"Ġboss":6478,"ĠHol":6479,"Ġinstr":6480,"Ġincreasingly":6481,"Ġshift":6482,"Ġstreets":6483,"ĠWilliams":6484,"abb":6485,"Ġlie":6486,"Ġlaugh":6487,"ĠCa":6488,"PL":6489,"Ġadults":6490,"Ġcustomer":6491,"Ġobtained":6492,"Ġsupporting":6493,"html":6494,"fire":6495,"Ġdetailed":6496,"Ġpicked":6497,"ĠRight":6498,"lder":6499,"EE":6500,"stood":6501,"ĠKim":6502,"Ġwire":6503,"Ġsight":6504,"Ġdevelopers":6505,"Ġpersons":6506,"Ġsad":6507,"Ġcup":6508,"Ġwarning":6509,"Ġboys":6510,"long":6511,"Ġbird":6512,"fo":6513,"Ġwal":6514,"Ġobserved":6515,"Ġzone":6516,"iveness":6517,"Ġchannel":6518,"cript":6519,"Ġrefused":6520,"ĠAgain":6521,"Ġsuc":6522,"Ġspokesman":6523,"ĠRef":6524,"rite":6525,"ouston":6526,"ãĥ³":6527,"ĠSher":6528,"Ġacts":6529,"ĠName":6530,"Ġstruggle":6531,"arry":6532,"ometimes":6533,"Ġdiscrim":6534,"HT":6535,"Ġcategory":6536,"Ġrealize":6537,"Ġemployee":6538,"ĠAfghan":6539,"enger":6540,"Ġguns":6541,"ĠSteve":6542,"ĠMot":6543,"ĠOl":6544,"oked":6545,"Ġthick":6546,"Ġfairly":6547,"illy":6548,"Ġsurve":6549,"ĠMat":6550,"weight":6551,"âĶ":6552,"Ġtroops":6553,"Ġagents":6554,"Ġbattery":6555,"Ġmotiv":6556,"á":6557,"Sec":6558,"den":6559,"overy":6560,"LS":6561,"Ġflu":6562,"Ġconfident":6563,"ĠOper":6564,"Ġempty":6565,"Ġphen":6566,"Ġsector":6567,"Ġexcited":6568,"Ġremote":6569,"aph":6570,"oen":6571,"Ġdestroyed":6572,"Ġmoral":6573,"ĠHP":6574,"ĠRon":6575,"Ġdress":6576,"ĠBat":6577,"Ġlit":6578,"ĠMS":6579,"Ġaf":6580,"HL":6581,"rum":6582,"isms":6583,"Ġshouldn":6584,"Ġsympt":6585,"ĠToronto":6586,"hetic":6587,"Ġcarbon":6588,"Ġinstalled":6589,"Ġviolent":6590,"Ġsolar":6591,"ja":6592,"Ġpractices":6593,"Ġride":6594,"ĠPenn":6595,"Ġimproved":6596,"Ġaudio":6597,"Ġbehavi":6598,"ĠPS":6599,"Ġeating":6600,"Data":6601,"ĠReview":6602,"pass":6603,"claim":6604,"uated":6605,"angers":6606,"chen":6607,"Ġproperties":6608,"Ġanywhere":6609,"Another":6610,"Ġblow":6611,"ĠJackson":6612,"Ġproud":6613,"Ġplane":6614,"lines":6615,"Ġsquare":6616,"Ġproof":6617,"ansas":6618,"Ġtalked":6619,"makers":6620,"Ġsister":6621,"Ġholds":6622,"Ġresident":6623,"Ġ==":6624,"Ġresistance":6625,"Ġsplit":6626,"Ġprosecut":6627,"Ġconfidence":6628,"resents":6629,"Ġcuts":6630,"Ġexception":6631,"Ġzero":6632,"Getty":6633,"Ġcopyright":6634,"Ġtotally":6635,"ormal":6636,"ifications":6637,"ĠAustralian":6638,"Ġsick":6639,"Ġ150":6640,"Ġhousehold":6641,"Ġfees":6642,"Ġdrivers":6643,"ogen":6644,"ĠNY":6645,"Ġnecessarily":6646,"Ġregulations":6647,"earing":6648,"sl":6649,"Ġperspective":6650,"care":6651,"icial":6652,"His":6653,"Ġescape":6654,"Ġsurprised":6655,"ĠVan":6656,"urrent":6657,"Ġvac":6658,"81":6659,"ĠThus":6660,"Ġemphas":6661,"ĠChampions":6662,"ĠIce":6663,"Ġnarr":6664,"Ġheads":6665,"Ġcausing":6666,"bel":6667,"fortunately":6668,"ĠMa":6669,"Ġtargets":6670,"cipl":6671,"Ġafternoon":6672,"Ġadds":6673,"ĠMaybe":6674,"ĠFour":6675,"essed":6676,"plete":6677,"Ġusual":6678,"cho":6679,"ingu":6680,"Ġwithd":6681,"ĠEnergy":6682,"ĠEconom":6683,"OO":6684,"Ġarticles":6685,"Ġinjured":6686,"Ġmanage":6687,"Ġexplains":6688,"Ġdiagn":6689,"Rec":6690,"atures":6691,"Ġlinked":6692,"Ġdiscussed":6693,"Ġexplo":6694,"Ġoccasion":6695,"athan":6696,"Ġopposite":6697,"Ġfaces":6698,"Ġdenied":6699,"ĠKnight":6700,"Ġnut":6701,"Ġapproximately":6702,"Ġdisappoint":6703,"onymous":6704,"ĠBest":6705,"ĠLo":6706,"ĠHy":6707,"ĠAff":6708,"Ġvoting":6709,"anwhile":6710,"ĠIII":6711,"Ġinstitutions":6712,"agram":6713,"ĠDaily":6714,"Ġdrag":6715,"Ġnearby":6716,"Ġguilty":6717,"Ġconver":6718,"Pre":6719,"ship":6720,"Ġreward":6721,"Ġphilosoph":6722,"ĠSS":6723,"ugh":6724,"Ġapps":6725,"friend":6726,"Ġupper":6727,"Ġadvert":6728,"Ġsnow":6729,"Ġfrust":6730,"Ġourselves":6731,"Fr":6732,"ĠDie":6733,"ampion":6734,"Ġdismiss":6735,"Ġcere":6736,"Ġsignal":6737,"from":6738,"Ġ).":6739,"Ġ52":6740,"Ġcrimes":6741,"itors":6742,"estival":6743,"useum":6744,"Ġcouncil":6745,"ĠSaud":6746,"May":6747,"ĠGun":6748,"ician":6749,"ether":6750,"Ġsufficient":6751,"ĠHen":6752,"sole":6753,"Ġhistorical":6754,"ĠFar":6755,"ĠTurn":6756,"Ġpin":6757,"Ġsucceed":6758,"mat":6759,"lymp":6760,"Ġtradition":6761,"ĠOk":6762,"Ġcro":6763,"Ġdescription":6764,"alle":6765,"Ġsky":6766,"Te":6767,"Ġwidely":6768,"Ġwave":6769,"Ġdefinition":6770,"ĠJews":6771,"Ġcycle":6772,"Ġrefere":6773,"Ġbrings":6774,"usal":6775,"Ġalive":6776,"Ġfrequently":6777,"Ġintention":6778,"ĠControl":6779,"lv":6780,"ystem":6781,"Ġprivacy":6782,"gent":6783,"rence":6784,"ĠQuest":6785,"ĠChristmas":6786,"Ġrail":6787,"Ġcooper":6788,"Ġtested":6789,"ĠCapt":6790,"asks":6791,"Ġcomfortable":6792,"Ġdelivered":6793,"scape":6794,"Ġdepth":6795,"ĠGOP":6796,"Ġwrites":6797,"Ġassets":6798,"Ġsav":6799,"iments":6800,"Ġtransition":6801,"Ġartist":6802,"ĠLook":6803,"Ġlob":6804,"Ġcomponents":6805,"arity":6806,"Ġwalked":6807,"Ġroot":6808,"Ġparticipants":6809,"Ġnoticed":6810,"Ġresc":6811,"Ġnav":6812,"ĠAdminist":6813,"da":6814,"utral":6815,"plate":6816,"Ġimportance":6817,"Ġassert":6818,"iously":6819,"cription":6820,"Ġinjuries":6821,"ĠCheck":6822,"Ġregistered":6823,"Ġintent":6824,"Ġmissed":6825,"ographic":6826,"Ġsentence":6827,"ounter":6828,"Ġassistance":6829,"evin":6830,"Ġdatabase":6831,"Ġbuildings":6832,"Ġclassic":6833,"Ġthinks":6834,"ĠOhio":6835,"Pr":6836,"ugg":6837,"Ġfee":6838,"pan":6839,"Ġeffectively":6840,"Ġfacility":6841,"Ġbear":6842,"Ġchapter":6843,"Ġdogs":6844,"ĠColumb":6845,"Ġlatter":6846,"itial":6847,"Ġadmitted":6848,"TV":6849,"ĠGeorg":6850,"Ġposts":6851,"\\\\":6852,"Ġlawyer":6853,"Ġequival":6854,"Ġmand":6855,"Ġcontrolled":6856,"ĠWalk":6857,"ĠAndrew":6858,"Ġmenu":6859,"amental":6860,"Ġprotected":6861,"va":6862,"Ġadministr":6863,"oral":6864,"Ġrein":6865,"ĠSar":6866,"Ġamounts":6867,"Ġnative":6868,"ĠMoon":6869,"Ġrepresents":6870,"Ġabandon":6871,"Ġcarrying":6872,"Ġtank":6873,"mary":6874,"Ġdeclared":6875,"Tube":6876,"Ġhat":6877,"Ġpunish":6878,"ellect":6879,"mes":6880,"Ġuniverse":6881,"ĠRod":6882,"phy":6883,"Ġinfrastructure":6884,"Ġ51":6885,"Ġopposed":6886,"ownt":6887,"ca":6888,"ĠMake":6889,"Ġhardware":6890,"Ġcoffee":6891,"Rel":6892,"bal":6893,"world":6894,"ĠSaf":6895,"ĠSea":6896,"inals":6897,"Ġowned":6898,"Ġhall":6899,"ersion":6900,"Ġdescribe":6901,"ĠPot":6902,"Ġportion":6903,"Ġatmosp":6904,"Ġgovernments":6905,"Ġdepending":6906,"Ġoffense":6907,"Ġtrick":6908,"awa":6909,"ĠLine":6910,"ĠVis":6911,"ĠHard":6912,"ĠOrig":6913,"ĠClick":6914,"Ġdesk":6915,"ĠValley":6916,"ĠSov":6917,"Ġmovies":6918,"Ġremark":6919,"Ġmail":6920,"Ġconscious":6921,"Ġruling":6922,"ĠRights":6923,"Ġmedic":6924,"hent":6925,"ĠWomen":6926,"><":6927,"Ġreplaced":6928,"ĠPrem":6929,"ĠThanks":6930,"Ġrenew":6931,"ĠBall":6932,"iform":6933,"Ġshots":6934,"Comm":6935,"Ġarmed":6936,"Ġconstant":6937,"Ġtaste":6938,"Ġrealized":6939,"Ġbuff":6940,"Ġmo":6941,"Ġefficient":6942,"Most":6943,"oration":6944,"ifies":6945,"Ġcommunication":6946,"Ġflood":6947,"Ġconsequences":6948,"Ġanyway":6949,"igg":6950,"ĠGM":6951,"ĠThank":6952,"Ġiron":6953,"Ġevolution":6954,"ĠCop":6955,"twitter":6956,"Ġ95":6957,"Ġrelationships":6958,"adel":6959,"ĠYoung":6960,"Ġproposal":6961,"ayers":6962,"uilding":6963,"ĠHot":6964,"ORE":6965,"cos":6966,"Ġcollabor":6967,"PG":6968,"axy":6969,"Ġknowing":6970,"Ġsupports":6971,"owed":6972,"Ġcontrols":6973,"Ġmerely":6974,"umer":6975,"Ġathlet":6976,"Ġfashion":6977,"path":6978,"Ġgift":6979,"Ġera":6980,"AND":6981,"Ġkinds":6982,"ĠKorean":6983,"Ġlegit":6984,"ulous":6985,"Ġessentially":6986,"Ġtherap":6987,"nic":6988,"Ġsuffered":6989,"Ġhur":6990,"Ġpromise":6991,"Ġexcess":6992,"Ġoverw":6993,"Ġprime":6994,"ĠHouston":6995,"erry":6996,"ĠMs":6997,"RS":6998,"2012":6999,"Ġstores":7000,"ĠOlymp":7001,"Ġjourney":7002,"Although":7003,"Sub":7004,"ĠEduc":7005,"ĠChapter":7006,"Ġrequests":7007,"Ġconsumers":7008,"Ġtiny":7009,"Ġisol":7010,"ĠFair":7011,"ba":7012,"ĠYOU":7013,"Ġcrash":7014,"celer":7015,"Ġemotional":7016,"Ġgoods":7017,"Ġelected":7018,"Ġmoder":7019,"ĠLinux":7020,"Ġblocks":7021,"Ġisland":7022,"ĠSociety":7023,"Ġelections":7024,"Ġbroadcast":7025,"Ġcheap":7026,"Ġnations":7027,"Ġseasons":7028,"400":7029,"Ġwaste":7030,"ĠSat":7031,"Ġfields":7032,"employ":7033,"Ġprofile":7034,"Ġauthors":7035,"ALL":7036,"ĠGra":7037,"west":7038,"ĠTy":7039,"Ġdeaths":7040,"Ġvacc":7041,"Ġformed":7042,"Ġdu":7043,"Ġongoing":7044,"ĠMuslims":7045,"elf":7046,"igure":7047,"Ġassume":7048,"ĠUkraine":7049,"water":7050,"Ġcoast":7051,"Ġvoted":7052,"gor":7053,"ĠAS":7054,"ĠMichigan":7055,"aza":7056,"ĠArm":7057,"iro":7058,"Ġflex":7059,"asters":7060,"''":7061,"Ġwelcome":7062,"arl":7063,"Ġlocations":7064,"igation":7065,"ĠFil":7066,"Ġbuying":7067,"Ġarchitect":7068,"Ġharder":7069,"ĠCub":7070,"Ġinterface":7071,"Ġrestaurant":7072,"Ġdiscover":7073,"Ġexceed":7074,"Ġfavour":7075,"gery":7076,"Ġduty":7077,"Ġpitch":7078,"ador":7079,"ĠMach":7080,"boy":7081,"Ġresponded":7082,"Ġextended":7083,"hers":7084,"Many":7085,"raid":7086,"ifer":7087,"ĠIns":7088,"Ser":7089,"Ġmedium":7090,"she":7091,"ĠSports":7092,"Ġmagazine":7093,"utation":7094,"Ġlimits":7095,"ĠGall":7096,"Ġexternal":7097,"razil":7098,"Ġyounger":7099,"tle":7100,"Ġremind":7101,"ĠCON":7102,"Ġimmediate":7103,"Ġhidden":7104,"Ġvolunte":7105,"Ġsimpl":7106,"odcast":7107,"Ġphase":7108,"dr":7109,"Ġplot":7110,"Ġexposure":7111,"RI":7112,"ograp":7113,"vin":7114,"anish":7115,"ĠAcad":7116,"ĠEngine":7117,"Ġexpansion":7118,"ĠPay":7119,"Your":7120,"Ġpushed":7121,"ĠEll":7122,"ĠHead":7123,"Ġmarketing":7124,"ĠAC":7125,"ket":7126,"Ġhits":7127,"Ġgro":7128,"ĠAge":7129,"ĠScot":7130,"][":7131,"Ġstim":7132,"ĠiPhone":7133,"ĪĴ":7134,"Ġnarrow":7135,"ĠGetty":7136,"ĠTurkey":7137,"Ġperfectly":7138,"Ġenable":7139,"utch":7140,"Ġprecise":7141,"Ġregime":7142,"Ġshif":7143,"Ġcompens":7144,"gun":7145,"div":7146,"Ġchosen":7147,"ĠKen":7148,"Any":7149,"Ġtrees":7150,"Ġrecommended":7151,"ĠRen":7152,"uable":7153,"ĠHT":7154,"Follow":7155,"EG":7156,"ĠHand":7157,"ĠKenn":7158,"Ġarguments":7159,"Ġexists":7160,"Ġbike":7161,"ĠConserv":7162,"Ġbreaking":7163,"ĠGar":7164,"Ġcrazy":7165,"Ġvirtual":7166,"aylor":7167,"ixel":7168,"Ġ1980":7169,"Ġpermission":7170,"ĠSeries":7171,"Ġconsumer":7172,"Ġclosely":7173,"called":7174,"Ġ54":7175,"Ġhopes":7176,"Ġarray":7177,"ĠWin":7178,"ĠLabour":7179,"Ġspons":7180,"ĠIre":7181,"Ġpow":7182,"Ġreaders":7183,"Ġemployment":7184,"Ġcreature":7185,"Ġresulting":7186,"Ġaccurate":7187,"Ġmoments":7188,"Ġargued":7189,"Ġped":7190,"During":7191,"Ġ53":7192,"ĠTal":7193,"Ġsought":7194,"Ġsuffering":7195,"Ġicon":7196,"lee":7197,"Ġ($":7198,"alian":7199,"°":7200,"Ġpra":7201,"Ġbonus":7202,"(\"":7203,"ko":7204,"Ġacting":7205,"DE":7206,"fall":7207,"Ġcomparison":7208,"Ġsmooth":7209,"ĠNAS":7210,"upp":7211,"ĠJoseph":7212,"eping":7213,"ĠTake":7214,"ĠMid":7215,"Ġsending":7216,"fast":7217,"ĠFall":7218,"Ġdealing":7219,"user":7220,"ĠOrgan":7221,"Co":7222,"Ġattached":7223,"Ġsees":7224,"%.":7225,"Ġtypical":7226,"ART":7227,"Ġfinds":7228,"ĠAsia":7229,"umin":7230,"ĠCore":7231,"ĠEnt":7232,"inent":7233,"uce":7234,"ĠBlood":7235,"ĠNever":7236,"Ġemails":7237,"Ġhighlight":7238,"Ġconfront":7239,"atus":7240,"uted":7241,"Ġunus":7242,"Ġtopic":7243,"ĠAdam":7244,"Ġble":7245,"ati":7246,"Ġunderstood":7247,"Set":7248,"struct":7249,"TP":7250,"Ġmob":7251,"aa":7252,"ĠStart":7253,"pected":7254,"sell":7255,"Ġdedicated":7256,"ĠCA":7257,"uan":7258,"Ġsongs":7259,"escription":7260,"Ġtech":7261,"Ġrape":7262,"Ġaside":7263,"Ġgrant":7264,"Ġ56":7265,"sub":7266,"Ġargue":7267,"Ġcontaining":7268,"Ġschedule":7269,"Ġliberal":7270,"Ġpublicly":7271,"Ġheavily":7272,"ĠUt":7273,"iner":7274,"ĠSection":7275,"ĠCare":7276,"weet":7277,"ls":7278,"Dis":7279,"âĶĢ":7280,"ĠFollow":7281,"Back":7282,"ĠIT":7283,"Ġbes":7284,"ji":7285,"ĠHit":7286,"ested":7287,"Ġeverybody":7288,"ĠSwed":7289,"Ġfemin":7290,"Ġfacilities":7291,"Ġconven":7292,"Comp":7293,"ĠOS":7294,"core":7295,"Ġanx":7296,"Ġdivision":7297,"ĠCam":7298,"ĠStan":7299,"mates":7300,"Ġexplore":7301,"plom":7302,"Ġshares":7303,"pload":7304,"anes":7305,"Ġideal":7306,"eters":7307,"ĠBase":7308,"Ġplastic":7309,"Ġdistinct":7310,"ĠNetwork":7311,"ĠSeattle":7312,"Ġtrading":7313,"ensus":7314,"intend":7315,"Ġexhib":7316,"Ġinitially":7317,"ĠFood":7318,"Ġthousand":7319,"ĠBusiness":7320,"acter":7321,"Ġparagraph":7322,"Ġroughly":7323,"Ġwww":7324,"Ġcreative":7325,"ĠConf":7326,"Ġconsumption":7327,"Ġfilms":7328,"agan":7329,"Ġobtain":7330,"Ġtall":7331,"Ġtor":7332,"Ġacknowled":7333,"Ġgrown":7334,"alo":7335,"KE":7336,"Ġ400":7337,"enders":7338,"taining":7339,"UG":7340,"Ġsuicide":7341,"Ġwatched":7342,"ĠList":7343,"ali":7344,"rehens":7345,"Ġsurrounding":7346,"Ġpip":7347,"Ġflying":7348,"ĠJava":7349,"ordan":7350,"Ġserving":7351,"inations":7352,"post":7353,"Ġsho":7354,"Av":7355,"Ġjail":7356,"zy":7357,"Ġ1999":7358,"Ġ</":7359,"Ġliterally":7360,"ĠSir":7361,"Ġexposed":7362,"Ġlies":7363,"star":7364,"Ġbat":7365,"Ġearned":7366,"ĠDig":7367,"Ġspecified":7368,"ĠSeason":7369,"Ġdegrees":7370,"Donald":7371,"Ġcentre":7372,"Ġsharing":7373,"Ġwinter":7374,"ĠCO":7375,"Che":7376,"ĠÎ":7377,"MP":7378,"Ġunw":7379,"Ġfewer":7380,"ĠMir":7381,"Ġsomewhere":7382,"ĠKey":7383,"Ġattacked":7384,"ĠKir":7385,"Ġdomain":7386,"Ġstronger":7387,"Ġ99":7388,"Ġpenalty":7389,"Id":7390,"Script":7391,"Ġdeclined":7392,"Ġneck":7393,"Ġfraud":7394,"Ġcurrency":7395,"Ġrising":7396,"RC":7397,"â̦â̦":7398,"Hz":7399,"Ġtab":7400,"Ġtalent":7401,"nam":7402,"ĠNBA":7403,"Ġvillage":7404,"Ġlegs":7405,"ĠNext":7406,"Ed":7407,"Ġacid":7408,"Ġhyd":7409,"800":7410,"Ġinvolving":7411,"ĠImage":7412,"ĠBefore":7413,"Fl":7414,"Ġyesterday":7415,"Source":7416,"Ġterrorist":7417,"Ġsup":7418,"Ġsynt":7419,"ĠSaudi":7420,"Ġwest":7421,"Ġru":7422,"burg":7423,"Ġvisible":7424,"Ġstruck":7425,"rison":7426,"Ġawesome":7427,"Ġdrawn":7428,"Ġanswers":7429,"ĠGirl":7430,"ĠRam":7431,"Ġthreats":7432,"Ġdefeat":7433,"osit":7434,"Ġvent":7435,"aturally":7436,"American":7437,"enda":7438,"ĠHoly":7439,"Ġrum":7440,"%,":7441,"case":7442,"ĠHistory":7443,"ĠYouTube":7444,"Ġsituations":7445,"ĠDNA":7446,"Ste":7447,"Ġsaved":7448,"Item":7449,"Ġrecip":7450,"ologist":7451,"Ġfaced":7452,"Ġelig":7453,"Once":7454,"ĠLi":7455,"uh":7456,"Ġmistake":7457,"ĠDivision":7458,"ĠBell":7459,"Ġsymptoms":7460,"®":7461,"Ġdomin":7462,"Ġfalling":7463,"Ġending":7464,"ashes":7465,"Ġmatches":7466,"ĠOnline":7467,"Ġexplanation":7468,"Def":7469,"redit":7470,"Ġanymore":7471,"ĠTotal":7472,"ĠFOR":7473,"ushed":7474,"Ġletters":7475,"Ġrisks":7476,"ĠOK":7477,"Ġreportedly":7478,":\\":7479,"Ġplate":7480,"Ġsubjects":7481,"Ġattempted":7482,"ifier":7483,"iana":7484,"Ġunlikely":7485,"ĠThough":7486,"uma":7487,"ĠInvest":7488,"ĠPrin":7489,"ican":7490,"ĠDar":7491,"ĠColorado":7492,"aug":7493,"Ġveget":7494,"aos":7495,"ria":7496,"Ġshel":7497,"Ġmarked":7498,"Ġ()":7499,"Ġspr":7500,"po":7501,"ĠLink":7502,"Ġdefe":7503,"ĠJr":7504,"Ġtheme":7505,"Ġpassion":7506,"ĠPen":7507,"Ġinfo":7508,"izer":7509,"Ġshit":7510,"ĠCivil":7511,"apse":7512,"cre":7513,"Ġpoly":7514,"Ġcomponent":7515,"ĠCharles":7516,"ĠIreland":7517,"ĠProv":7518,"Ġdoctors":7519,"Ġgranted":7520,"Ġpaint":7521,"Ġhonor":7522,"Ġsmoke":7523,"Ġpayments":7524,"Ġprimarily":7525,"ĠKingdom":7526,"rich":7527,"atell":7528,"Ġdeals":7529,"Ġscheduled":7530,"Ġfundamental":7531,"Ġprotein":7532,"Ġnewspaper":7533,"Ġclients":7534,"ython":7535,"ĠDate":7536,"hus":7537,"Ġfeedback":7538,"Ġstretch":7539,"Ġcock":7540,"Ġhotel":7541,"ĠQueen":7542,"Ġsugar":7543,"Ġju":7544,"Ġmilk":7545,"Ġapproval":7546,"ĠLive":7547,"Ġequivalent":7548,"efully":7549,"Ġinsert":7550,"zona":7551,"Ġextension":7552,"dri":7553,"John":7554,"Ġaccomp":7555,"Sm":7556,"ĠFund":7557,"Ġconstantly":7558,"Ġ``":7559,"Ġgenerated":7560,"ĠAction":7561,"ĠPsych":7562,"ĠTri":7563,"Ġrecognize":7564,"Ġvary":7565,"pha":7566,"ĠRa":7567,"df":7568,"etch":7569,"ĠSoviet":7570,"Two":7571,"Ġpatterns":7572,"Ġprofession":7573,"aning":7574,"Time":7575,"ĠLim":7576,"Ġcolors":7577,"ĠAz":7578,"ĠTR":7579,"Ġinfect":7580,"Ġphenomen":7581,"Ġshell":7582,"Also":7583,"Ġputs":7584,"Ġdelivery":7585,"Ġbrown":7586,"Ġprocessing":7587,"Ġlights":7588,"essage":7589,"ĠBrook":7590,"ĠAud":7591,"lation":7592,"Ġindustrial":7593,"Like":7594,"ĠBrazil":7595,"rous":7596,"ESS":7597,"ĠLuc":7598,"Ġsomehow":7599,"Ġ85":7600,"Ġproport":7601,"Ġpoliticians":7602,"Ġindicate":7603,"Ġhole":7604,"Ġtechniques":7605,"Ġcompetitive":7606,"Ġphr":7607,"Ġvo":7608,"istent":7609,"ĠDream":7610,"Ġcampus":7611,"Ġaspects":7612,"Ġhelpful":7613,"Ġshield":7614,"orse":7615,"Ġtrigger":7616,"mal":7617,"Ġ58":7618,"Ġtort":7619,"Ġpersonally":7620,"Ġtag":7621,"Ġkeeps":7622,"ĠVideo":7623,"Ġbench":7624,"Ġgap":7625,"aire":7626,"Ġeast":7627,"Ġrecovery":7628,"perial":7629,"Ġprofit":7630,"ĠMic":7631,"Ġ57":7632,"Ġcolon":7633,"Ġstrongly":7634,"style":7635,"Ġallegations":7636,"han":7637,"Ġreporters":7638,"jo":7639,"rine":7640,"arget":7641,"andal":7642,"Ġ03":7643,"Ġflash":7644,"trans":7645,"Ġstrict":7646,"Ġparking":7647,"ĠPakistan":7648,"Ġli":7649,"Ġweird":7650,"ĠEric":7651,"Ġregions":7652,"ĠJun":7653,"Ġintellect":7654,"ĠWH":7655,"oding":7656,"ributes":7657,"upid":7658,"ĠTit":7659,"Ġfinger":7660,"oria":7661,"Ġelev":7662,"ĠField":7663,"Ġconclusion":7664,";;":7665,"Ġfeelings":7666,"Ġextensive":7667,"Ġmixed":7668,"Ġneuro":7669,"vy":7670,"Ġharass":7671,"ĠCirc":7672,"ouch":7673,"Ġterritory":7674,"Ġsuccessfully":7675,"Mar":7676,"Ġingred":7677,"Ġoverwhel":7678,"Ġlayer":7679,"View":7680,"Ġallies":7681,"illance":7682,"ĠThree":7683,"Ġbunch":7684,"Ġnormally":7685,"Ġnetworks":7686,"Ġsacr":7687,"ĠCIA":7688,"bles":7689,"Ġchose":7690,"Ġopponents":7691,"Ġregardless":7692,"Ġfranch":7693,"Ġpref":7694,"ĠPo":7695,"Ġbridge":7696,"anna":7697,"ĠSilver":7698,"Ġwage":7699,"page":7700,"rior":7701,"Ġradical":7702,"ĠLittle":7703,"Ġmanip":7704,"Ġsecretary":7705,"Ġgang":7706,"DR":7707,"FA":7708,"Ġdecent":7709,"ĠSpirit":7710,"Ġuncle":7711,"ĠDevelopment":7712,"Ġinvestors":7713,"Ġwalls":7714,"Ġpublish":7715,"Ġgenerate":7716,"issions":7717,"car":7718,"Ġpromote":7719,"Ġcutting":7720,"Ġchest":7721,"Ġdrinking":7722,"Ġcollected":7723,"Ġ72":7724,"Ġhoping":7725,"Ġembr":7726,"gorith":7727,"Ġwarned":7728,"Ġinstructions":7729,"OG":7730,"ĠDid":7731,"ĠAgency":7732,"Ġgear":7733,"Ġcriticism":7734,"ĠFurther":7735,"Ġutil":7736,"anny":7737,"Red":7738,"Ġcounsel":7739,"ĠAsian":7740,"Ġreduction":7741,"pool":7742,"Ġteaching":7743,"Ġdeeply":7744,"iy":7745,"Ġestimates":7746,"Ġchoices":7747,"Ġpermanent":7748,"inem":7749,"kel":7750,"Ġfasc":7751,"pse":7752,"file":7753,"ĠLow":7754,"ĠPerson":7755,"Ġtournament":7756,"stal":7757,"Ġmel":7758,"UST":7759,"ĠRay":7760,"azi":7761,"Val":7762,"Ġcontained":7763,"ĠHolly":7764,"Ġwake":7765,"Ġreveal":7766,"Ġprocesses":7767,"ĠISIS":7768,"Ġ09":7769,"Ġblind":7770,"Ġsteel":7771,"ĠBad":7772,"Ġcarefully":7773,"appy":7774,"roit":7775,"Ġgaming":7776,"Ġhouses":7777,"ĠColl":7778,"Ġtruck":7779,"erm":7780,"Ġscored":7781,"Ġoccas":7782,"return":7783,"bound":7784,"var":7785,"Ġsharp":7786,"Ġafraid":7787,"ĠEX":7788,"amber":7789,"cific":7790,"Ġscheme":7791,"NC":7792,"ĠPolit":7793,"Ġdecline":7794,"Ġ1998":7795,"Ġpushing":7796,"Ġpossession":7797,"Ġprivile":7798,"Ġteachers":7799,"Ġyield":7800,"HA":7801,"ĠDavis":7802,"itled":7803,"########":7804,"Ġrig":7805,"ĠDaniel":7806,"acon":7807,"Ġhide":7808,"uten":7809,"Ġcolleagues":7810,"Ġprinciples":7811,"Ġloud":7812,"Ġsin":7813,"ĠDemon":7814,"Ġstone":7815,"Ġ02":7816,"Ġtaught":7817,"Ġterrible":7818,"Ġstuck":7819,"ĠPolicy":7820,"teen":7821,"Ġimplementation":7822,"ĠBBC":7823,"ĠAPI":7824,"Ġwheel":7825,"allas":7826,"Ġchampions":7827,"olars":7828,"player":7829,"Ġrepeatedly":7830,"ĠStill":7831,"Ġlikes":7832,"asty":7833,"ester":7834,"ĠCatholic":7835,"RL":7836,"Ġbath":7837,"Ġnoise":7838,"title":7839,"Ġnorthern":7840,"Part":7841,"Ġmagn":7842,"Ġfab":7843,"ĠAsh":7844,"Ġdispl":7845,"Ġticket":7846,"Ġmurd":7847,"Ġalongside":7848,"ĠMusic":7849,"Ġriver":7850,"ĠSteel":7851,"ĠCL":7852,"ĠPlayer":7853,"ĠMult":7854,"owing":7855,"rep":7856,"size":7857,"Ġtur":7858,"ĠGeorgia":7859,"iscal":7860,"raction":7861,"Ġcable":7862,"Ġ59":7863,"Ġwins":7864,"Ġupcoming":7865,"Ġsurvive":7866,"Ġinspired":7867,"ĠEducation":7868,"Ġstatistics":7869,"ĠFoot":7870,"iami":7871,"Ġyellow":7872,"ĠPage":7873,".-":7874,"ĠHas":7875,"Ġurban":7876,"Ġax":7877,"essel":7878,"\\\"":7879,"Ġquarterback":7880,"Ġregister":7881,"ĠLabor":7882,"Ġabilities":7883,"ĠFamily":7884,"Ġvariable":7885,"ĠPrice":7886,"Ġcontem":7887,"Ġthin":7888,"ĠEqu":7889,"data":7890,"Ġgotten":7891,"Ġconstit":7892,"Ġasks":7893,"Ġtail":7894,"Ġexciting":7895,"ĠEffect":7896,"ĠSpanish":7897,"Ġencourage":7898,"inson":7899,"ĠAh":7900,"Ġcommitment":7901,"CS":7902,"Ġrally":7903,"Ġ::":7904,"Ġsubsid":7905,"Ġspin":7906,"Ġcaptured":7907,"2018":7908,"Ġinnoc":7909,"Ġallegedly":7910,"ĠCome":7911,"Ġartists":7912,"ĠNumber":7913,"Ġelectronic":7914,"Ġregional":7915,"apes":7916,"Ġwra":7917,"Ġmyth":7918,"prise":7919,"ĠMiller":7920,"ĠCreat":7921,"ĠEpisode":7922,"bell":7923,"Ġdirected":7924,"Ġextract":7925,"Ġsorry":7926,"Ġvice":7927,"agger":7928,"ĠSupport":7929,"Ġ66":7930,"ĠIron":7931,"Ġwonderful":7932,"Ġgra":7933,"Net":7934,"ione":7935,"Eng":7936,"Ġships":7937,"ikes":7938,"ĠKevin":7939,"itar":7940,"Ġactivists":7941,"true":7942,"ĠArizona":7943,"enth":7944,"ĠDespite":7945,"ĠSE":7946,"Ġhabit":7947,"ernel":7948,"Ġinqu":7949,"Ġabortion":7950,"Ġvoid":7951,"Ġexplicit":7952,"Ġengaged":7953,"Ġangry":7954,"Ġrating":7955,"Ġfrag":7956,"bro":7957,"icking":7958,"dev":7959,"Ġworried":7960,"Ġobser":7961,"Ġapartment":7962,"ĠGT":7963,"Ġestate":7964,"ĠConstitution":7965,"emon":7966,"ĠSnow":7967,"Ġcounty":7968,"Ġdisag":7969,"ĠStephen":7970,"Ġimmigrants":7971,"wind":7972,"ĠNations":7973,"Ġfolks":7974,"Out":7975,"Ġgall":7976,"Ġtargeted":7977,"Ġstead":7978,"ĠBon":7979,"ĠLib":7980,"Ġinformed":7981,"Ġ120":7982,"chain":7983,"idelines":7984,"orough":7985,"Ġdriven":7986,"Ġregularly":7987,"Ġbasket":7988,"Ġprinciple":7989,"ocument":7990,"Ġstun":7991,"ibilities":7992,"ĠRoman":7993,"ĠAbout":7994,"Ġalert":7995,"Ġdemocracy":7996,"Ġrepresented":7997,"HS":7998,"cers":7999,"parent":8000,"Art":8001,"pack":8002,"Ġdiplom":8003,"rets":8004,"ĠNO":8005,"Ġcapture":8006,"ĠAdv":8007,"Ħ¢":8008,"Ġannouncement":8009,"ĠLear":8010,"Ġhook":8011,"Ġpurs":8012,"ĠSuch":8013,"ĠCamer":8014,"Ġrefugees":8015,"ĠVe":8016,"Pol":8017,"Ġrecognized":8018,"lib":8019,"Ġhadn":8020,"Ass":8021,"Ġpilot":8022,"ushing":8023,"Ġreturning":8024,"Ġtrail":8025,"ĠStone":8026,"Ġroutine":8027,"Ġcourts":8028,"Ġdesper":8029,"Ġfriendly":8030,"ĠItaly":8031,"Ġpled":8032,"Ġbreath":8033,"Ġstudio":8034,"NS":8035,"Ġimpressive":8036,"ĠAfghanistan":8037,"Ġfing":8038,"Ġdownt":8039,"inking":8040,"ĠRog":8041,"iary":8042,"color":8043,"sex":8044,"aron":8045,"Ġfault":8046,"ĠNick":8047,"Down":8048,"ĠRose":8049,"ĠSouthern":8050,"XX":8051,"isodes":8052,"List":8053,"600":8054,"Ġoutcome":8055,"err":8056,"Ġelsewhere":8057,"Ġretire":8058,"Ġpounds":8059,"ĠGlobal":8060,"People":8061,"Ġcommunications":8062,"Ġloan":8063,"Ġratio":8064,"ĠEmpire":8065,"Ġgonna":8066,"Ġinvent":8067,"DF":8068,"Ġ1970":8069,"ĠCommon":8070,"pat":8071,"Ġpromised":8072,"Ġdinner":8073,"ĠHom":8074,"Ġcreates":8075,"Ġoperate":8076,"verty":8077,"ĠJordan":8078,"etime":8079,"Ġsustain":8080,"Reg":8081,"Ġincredible":8082,"ima":8083,"Ġwarrant":8084,"Ġmm":8085,"Att":8086,"Ġlawsuit":8087,"Ġreviews":8088,"iture":8089,"ĠSource":8090,"lights":8091,"ĠFord":8092,"Ġ63":8093,"group":8094,"store":8095,"Ġfeatured":8096,"Ġforever":8097,"Ġpoverty":8098,"ĠPop":8099,"ĠCNN":8100,"azz":8101,"abis":8102,"aching":8103,"Ġlaid":8104,"ĠSupp":8105,"Ġfilter":8106,"ena":8107,"ĠCommunity":8108,"Ġcreatures":8109,"uction":8110,"ĠRoyal":8111,"Ġassociation":8112,"ĠConnect":8113,"ĠBrad":8114,"âĸĪ":8115,"lers":8116,"there":8117,"ĠGi":8118,"Ġvaluable":8119,"ACK":8120,"ĠTaylor":8121,"Ġliquid":8122,"ĠAttorney":8123,"ĠCarl":8124,"ĠFinal":8125,"aga":8126,"ĠWilson":8127,"Because":8128,"ĠProfessor":8129,"aka":8130,"Ġincredibly":8131,"rance":8132,"!)":8133,"Ref":8134,"sk":8135,"Ġsolutions":8136,"Ġatmosphere":8137,"Ġblame":8138,"umes":8139,"ĠNob":8140,"CA":8141,"umps":8142,"rical":8143,"ĠPutin":8144,"ĠDest":8145,"oric":8146,"ĠPA":8147,"Ġrespectively":8148,"wan":8149,"Ġfifth":8150,"âĦ¢":8151,"ĠCry":8152,"Ġgovernor":8153,"resident":8154,"Ġpurchased":8155,"Ġhack":8156,"Ġintense":8157,"obs":8158,"Ġorigin":8159,"Ġdefine":8160,"Ġcareful":8161,"***":8162,"Ġshoulder":8163,"Click":8164,"Ġtied":8165,"Ġdestruction":8166,"oured":8167,"Ġnobody":8168,"Ġho":8169,"ĠExper":8170,"Ġtip":8171,"\";":8172,"Ġtechnique":8173,"Ġjur":8174,"ĠPok":8175,"bow":8176,"Ġlegend":8177,"Ġaccord":8178,"Ġbusy":8179,"ĠIntel":8180,"Ġhang":8181,"aki":8182,".]":8183,"âĢĶâĢĶâĢĶâĢĶ":8184,"Ġsurgery":8185,"Ġreprodu":8186,"Ġuniform":8187,"Ġscenes":8188,"code":8189,"Ġ62":8190,"lisher":8191,"ĠHave":8192,"phia":8193,"Ġcrypt":8194,"Ġrecon":8195,"Ġscream":8196,"Ġadopted":8197,"Ġscores":8198,"Ne":8199,"ĠItalian":8200,"including":8201,"BO":8202,"Ġindicated":8203,"Ġentertain":8204,"Gu":8205,"Text":8206,"iel":8207,"Ġtwenty":8208,"Ġengage":8209,"offs":8210,"ĠPacific":8211,"Ġsmile":8212,"Ġpersonnel":8213,"Ġtoler":8214,"Ġdoors":8215,"Ġtone":8216,"Ġmachines":8217,"Ġentering":8218,"tenance":8219,"CO":8220,"ĠJersey":8221,"Ġforest":8222,"Ġhorse":8223,"Ġcomplaint":8224,"ĠSpring":8225,"yo":8226,"ĠPlus":8227,"eding":8228,"ĠReturn":8229,"quarters":8230,"ials":8231,"cow":8232,"Ġacademic":8233,"Ġfruit":8234,"Ġ1996":8235,"ogether":8236,"Ġwine":8237,"Ġpursu":8238,"ĠSteven":8239,"Ġlicens":8240,"Who":8241,"Ġclothes":8242,"rection":8243,"Ġsquad":8244,"Ġstable":8245,"Ġraw":8246,"zens":8247,"Star":8248,"uties":8249,"ancer":8250,"Ġkeys":8251,"ĠMu":8252,"Ġcomplicated":8253,"iger":8254,"ĠText":8255,"Ġabsor":8256,"Ġ68":8257,"Ġfunny":8258,"Ġrelief":8259,"ĠLew":8260,"ĠCook":8261,"Ġchart":8262,"Ġdrawing":8263,"GE":8264,"Ġmodule":8265,"ĠBull":8266,"ILL":8267,"Ġsalt":8268,"00000000":8269,"ille":8270,"Ġresource":8271,"away":8272,"adelphia":8273,"ĠBru":8274,"Ġ67":8275,"Ġsomebody":8276,"Ġparticipate":8277,"Ġrose":8278,"wered":8279,"Ġmuscle":8280,"Ġconsent":8281,"Ġcontinuing":8282,"ĠGuardian":8283,"ĠOrder":8284,"regon":8285,"Ġrear":8286,"Ġprovision":8287,"Ġliked":8288,"rient":8289,"Ġbra":8290,"Trans":8291,"Ġmeetings":8292,"Ġtox":8293,"Ġconvent":8294,"Ġauto":8295,"Ġrecording":8296,"ĠSoft":8297,"001":8298,"ĠRoll":8299,"Ġprogramming":8300,"Ġpic":8301,"Ġproved":8302,"Ġstab":8303,"ĠAst":8304,"Ġcaption":8305,"ulating":8306,"ĠAttack":8307,"Ġnewly":8308,"Ġ1997":8309,"fr":8310,"Ġdiscipl":8311,"ĠGreek":8312,"Ġedition":8313,"ĠDoes":8314,"ĠBox":8315,"ifle":8316,"acket":8317,"Ġpasses":8318,"Ġguest":8319,"Ġacceler":8320,"itals":8321,"UD":8322,"Ġauthent":8323,"ĠRest":8324,"oval":8325,"ta":8326,"uine":8327,"Ġarmor":8328,"ĠTown":8329,"Ġcompat":8330,"Ġinches":8331,"Despite":8332,"Ġassign":8333,"herent":8334,"Ġprepare":8335,"ĠMeg":8336,"ockey":8337,"Ġdepends":8338,"Ġtracks":8339,"watch":8340,"Ġlists":8341,"ĠNorthern":8342,"Ġalter":8343,"rec":8344,"ĠEastern":8345,"Ġcondem":8346,"Ġeverywhere":8347,"?'":8348,"Ġaffili":8349,"Ġfought":8350,"\":{\"":8351,"Ġmac":8352,"itarian":8353,"Ġscope":8354,"ĠAL":8355,"aws":8356,"arms":8357,"Ġque":8358,"Ġenjoyed":8359,"nesota":8360,"Ġaggressive":8361,"ĠStory":8362,"ĠIV":8363,"Ġrecipe":8364,"Ġrarely":8365,"ĠMedical":8366,"value":8367,"angel":8368,"aying":8369,"omething":8370,"Ġsubsection":8371,"Ġsouthern":8372,"Ġfrequency":8373,"rete":8374,"rolled":8375,"ults":8376,"ĠNic":8377,"Ġbehalf":8378,"Ġsequence":8379,"abet":8380,"Ġcontroversial":8381,"Ġcomprom":8382,"Ġworker":8383,"Ġmainly":8384,"Ġalgorith":8385,"ĠMajor":8386,"orce":8387,"gender":8388,"Ġorganized":8389,"Ġfake":8390,"Ġconcluded":8391,"ĠED":8392,"ĠExec":8393,"rage":8394,"Ġchances":8395,"berry":8396,"ĠTrad":8397,"Ġconfiguration":8398,"Ġwithdraw":8399,"Ġfro":8400,"udes":8401,"ĠBrother":8402,"ĠBrian":8403,"Ġtries":8404,"Ġsamples":8405,"Ġbid":8406,"ĠGolden":8407,"Ġphotograph":8408,"ifest":8409,"ĠDO":8410,"ĠParliament":8411,"****************":8412,"Rem":8413,"Ġcontest":8414,"Ġsigning":8415,"px":8416,"ĠZeal":8417,"âĶĢâĶĢ":8418,"Ear":8419,"Ġexit":8420,"Before":8421,"ĠCorpor":8422,"null":8423,"month":8424,"Ġracial":8425,"otted":8426,"ĠVeg":8427,"ĠReuters":8428,"Ġsword":8429,"pson":8430,"ĠRomney":8431,"aed":8432,"Ġtrib":8433,"Ġinner":8434,"Ġprotocol":8435,"ĠBi":8436,"ĠMiami":8437,"everal":8438,"press":8439,"Ġshipping":8440,"ĠAmendment":8441,"ĠHoward":8442,"connect":8443,"ĠDisc":8444,"ĠJac":8445,"iamond":8446,"ĠTherefore":8447,"ses":8448,"ĠPrincess":8449,"ĠUSB":8450,"ĠAnth":8451,"Ġsurveillance":8452,"Ġapolog":8453,"Ġ61":8454,"owa":8455,"Ġfulf":8456,"js":8457,"Ġluck":8458,"usted":8459,"Ġ§":8460,"ni":8461,"Ġanticip":8462,"eman":8463,"Ġwinner":8464,"Ġsilver":8465,"lla":8466,"icity":8467,"Ġunusual":8468,"Ġcrack":8469,"Ġties":8470,"ez":8471,"Ġpractical":8472,"Ġprovince":8473,"ĠPlace":8474,"Ġpriority":8475,"ICE":8476,"Ġdescribes":8477,"Ġbranch":8478,"Form":8479,"aska":8480,"missions":8481,"bi":8482,"Ġporn":8483,"ĠTurk":8484,"Ġenthus":8485,"Ġfighters":8486,"Ġ08":8487,"ĠDetroit":8488,"Ġfoundation":8489,"avid":8490,"Are":8491,"Ġjudgment":8492,"cling":8493,"Ġsolve":8494,"ĠDesign":8495,"Where":8496,"hesis":8497,"ĠTro":8498,"after":8499,"Ġneutral":8500,"ĠPalestinian":8501,"ĠHollywood":8502,"Ġadvis":8503,"ĠNon":8504,"yes":8505,"olis":8506,"Ġreputation":8507,"Ġsmell":8508,"Ġbread":8509,"ĠBul":8510,"ĠBeach":8511,"Ġclaiming":8512,"Ġgenetic":8513,"Ġtechnologies":8514,"Ġupgrade":8515,"rows":8516,"Ġdeveloper":8517,"ĠJosh":8518,"ĠDisney":8519,"erved":8520,"ipal":8521,"Ġunex":8522,"Ġbarely":8523,"then":8524,"ĠPub":8525,"Ġillness":8526,"etary":8527,"ĠBal":8528,"Ġpatch":8529,"Ġbutt":8530,"Ġstupid":8531,"ĠDog":8532,"ĠDallas":8533,"front":8534,"iece":8535,"Ġprotests":8536,"Ġchat":8537,"oenix":8538,"Ġwing":8539,"Ġparliament":8540,"Ġ77":8541,"osexual":8542,"Ġrender":8543,"ptions":8544,"ĠCoast":8545,"osa":8546,"ĠGreg":8547,"hop":8548,"ĠManagement":8549,"Ġbitcoin":8550,"Ġrecover":8551,"Ġincorpor":8552,"orne":8553,"ĠUsing":8554,"Ġpreced":8555,"Ġthreatened":8556,"Ġspiritual":8557,"ĠEvent":8558,"ĠFred":8559,"Ġadvertising":8560,"Ġimprovements":8561,"ĠCustom":8562,"Ġerrors":8563,"Ġsensitive":8564,"ĠNavy":8565,"Ġcream":8566,"Look":8567,"Ġexclusive":8568,"Ġcomprehens":8569,"Ġdeleg":8570,"Ġconce":8571,"Ġremem":8572,"Ġstructures":8573,"Ġstored":8574,"ND":8575,"Ġ1000":8576,"UP":8577,"ĠBudd":8578,"AF":8579,"woman":8580,"ĠAcademy":8581,"ðŁ":8582,"sea":8583,"Ġtemporary":8584,"About":8585,"esters":8586,"Ġtickets":8587,"Ġpossess":8588,"inch":8589,"oz":8590,"Ġla":8591,"Ġcontracts":8592,"Ġunp":8593,"Ġcig":8594,"ĠKat":8595,"ultural":8596,"asm":8597,"Ġmountain":8598,"ĠCaptain":8599,"Step":8600,"making":8601,"ĠSpain":8602,"Ġequally":8603,"Ġlands":8604,"aters":8605,"Ġrejected":8606,"era":8607,"imm":8608,"rix":8609,"CD":8610,"Ġtransaction":8611,"gener":8612,"lessly":8613,"Ġ||":8614,"Ġcos":8615,"ĠHenry":8616,"Ġprovisions":8617,"Ġgained":8618,"Ġdirectory":8619,"Ġraising":8620,"ĠSep":8621,"olen":8622,"onder":8623,"Ġconsole":8624,"inst":8625,"Ġbom":8626,"Ġuncertain":8627,"150":8628,"ocking":8629,"Ġmeasured":8630,"Ġplain":8631,"Ġseats":8632,"Ġdict":8633,"SL":8634,"afe":8635,"Ġestimate":8636,"izon":8637,"athered":8638,"Ġcontributed":8639,"Ġepisodes":8640,"ommod":8641,"Gr":8642,"ANT":8643,"Ġ69":8644,"Gener":8645,"Ġ250":8646,"viously":8647,"rogen":8648,"Ġterrorism":8649,"Ġmovements":8650,"entle":8651,"ounce":8652,"ĠSoul":8653,"Ġprev":8654,"ĠTable":8655,"acts":8656,"riors":8657,"tab":8658,"Ġsuffer":8659,"Ġnerv":8660,"Ġmainstream":8661,"ĠWolf":8662,"Ġfranchise":8663,"bat":8664,"Ġdemands":8665,"Ġagenda":8666,"Ġdozen":8667,"Ġclinical":8668,"izard":8669,"ĠOp":8670,"td":8671,"Ġvisited":8672,"ĠPerhaps":8673,"Ġactor":8674,"Ġdelic":8675,"Ġcontribute":8676,"Ġinject":8677,"ĠEs":8678,"acco":8679,"Ġlistening":8680,"Ġcongress":8681,"ependent":8682,"Ġpremium":8683,"Ġ76":8684,"ĠIrish":8685,"Ġassigned":8686,"ĠPhys":8687,"Ġworldwide":8688,"Ġnarrative":8689,"otype":8690,"mont":8691,"base":8692,"ĠBowl":8693,"ĠAdministration":8694,"Ġrelation":8695,"ĠEV":8696,"CP":8697,"Ġcovers":8698,"Ġ78":8699,"Ġcertific":8700,"Ġgrass":8701,"Ġ04":8702,"piracy":8703,"ira":8704,"Ġengineering":8705,"ĠMars":8706,"Ġunemploy":8707,"ĠForeign":8708,"stract":8709,"Ġven":8710,"Ġsteal":8711,"Ġreplied":8712,"Ġultimate":8713,"Ġtitles":8714,"dated":8715,"Ġjoy":8716,"aus":8717,"Ġhyper":8718,"aku":8719,"Ġofficially":8720,"ĠProduct":8721,"Ġdifficulty":8722,"peror":8723,"Ġresulted":8724,"ribed":8725,"link":8726,"who":8727,"~~~~":8728,"ĠSpeed":8729,"ĠViet":8730,"Wind":8731,"ĠBarack":8732,"Ġrestrictions":8733,"ĠShare":8734,"Ġ1995":8735,"itionally":8736,"Ġbeauty":8737,"opt":8738,"Ġmaps":8739,"ĠCR":8740,"ĠNation":8741,"ĠCruz":8742,"Will":8743,"Ġelectricity":8744,"Ġorg":8745,"Ġburd":8746,"Ġviolation":8747,"Ġusage":8748,"Ġpermit":8749,"ĠChron":8750,"ĠFant":8751,"Ġnaturally":8752,"Ġ07":8753,"Ġthrown":8754,"ĠAwoken":8755,"Ġalien":8756,"ĠHero":8757,"ĠKent":8758,"ĠRick":8759,"rike":8760,"Ġpace":8761,"},{\"":8762,"GL":8763,"Ġpoison":8764,"ĠTower":8765,"Ġformal":8766,"alysis":8767,"Ġgenuine":8768,"Ġkil":8769,"aver":8770,"Ġprocedure":8771,"ĠProp":8772,"intendo":8773,"ĠMain":8774,"asant":8775,"Ġtrained":8776,"Game":8777,"ĠLoad":8778,"ĠMA":8779,"Ġcrucial":8780,"Ġlets":8781,"ĠFR":8782,"Ġchampion":8783,"101":8784,"ĠConference":8785,"Ġwriters":8786,"Ġconnections":8787,"Ġokay":8788,"irms":8789,"ĠRand":8790,"Ġencounter":8791,"ĠBuff":8792,"Ġachieved":8793,"Ġchecks":8794,"iscons":8795,"Ġassistant":8796,"Ġwhenever":8797,"ĠAccess":8798,"ĠUr":8799,"bin":8800,"Ġclock":8801,"isp":8802,"opher":8803,"Ġborrow":8804,"Ġmad":8805,"Ġpersonality":8806,"only":8807,"IST":8808,"abama":8809,"Ġgains":8810,"Ġcommonly":8811,"Ġterr":8812,"Ġhypot":8813,"Ġrely":8814,"Ġtiss":8815,"isconsin":8816,"Ġridic":8817,"function":8818,"ĠOregon":8819,"Ġuncom":8820,"rating":8821,"eland":8822,"ĠNC":8823,"Ġmoon":8824,"annon":8825,"Ġvulnerable":8826,"utive":8827,"³³³³":8828,"ĠRadio":8829,"Ġwestern":8830,"sect":8831,"ĠTony":8832,"Ġoccurs":8833,"ĠOs":8834,"ĠHon":8835,"ÃŃ":8836,"Ġvessel":8837,"ĠScotland":8838,"Ġdiscrimination":8839,"Ġsubsequent":8840,"string":8841,"Ġfantasy":8842,"ĠShadow":8843,"Ġtestim":8844,"WE":8845,"iti":8846,"ras":8847,"Ġboat":8848,"Ġmarks":8849,"Ġordinary":8850,"Ġren":8851,"Ġrepresentative":8852,"Ġpetition":8853,"Ġ73":8854,"Ġadventure":8855,"Ġignore":8856,"ĠPhiladelphia":8857,"ĠSav":8858,"VP":8859,"Ġfactory":8860,"Ġtasks":8861,"Ġdepression":8862,"zed":8863,"................................":8864,"ĠStorm":8865,"Ġcogn":8866,"Ġeligible":8867,"Ġreducing":8868,"via":8869,"Ġ05":8870,"Ġstriking":8871,"Ġdollar":8872,"ho":8873,"OV":8874,"Ġinstrument":8875,"Ġphilosophy":8876,"ĠMoore":8877,"ĠAvenue":8878,"Ġruled":8879,"ĠFront":8880,"INE":8881,"ĠMah":8882,"Ġscenario":8883,"ĠNASA":8884,"Ġenorm":8885,"Ġdebut":8886,"Ġtea":8887,"Today":8888,"Ġabsence":8889,"Sim":8890,"Ġham":8891,"leep":8892,"Ġtables":8893,"ĠHeart":8894,"MI":8895,"Ke":8896,"requ":8897,"VD":8898,"map":8899,"Ġchairman":8900,"Ġpump":8901,"Ġrapidly":8902,"vi":8903,"Ġsubstantial":8904,"EP":8905,"des":8906,"chant":8907,"ilipp":8908,"ĠSanta":8909,"riers":8910,"anchester":8911,"Load":8912,"ĠCase":8913,"Ġsaving":8914,"Ġ74":8915,"ĠAFP":8916,"erning":8917,"ounced":8918,"ĠMinnesota":8919,"ĠWas":8920,"Ġrecru":8921,"Ġassessment":8922,"ĠBron":8923,"UE":8924,"Ġdynamic":8925,"Ġfurn":8926,"ulator":8927,"Ġpropag":8928,"high":8929,"Ġaccommod":8930,"Ġstack":8931,"ĠSus":8932,"writ":8933,"Ġreven":8934,"ĠGodd":8935,"ĠZealand":8936,"abs":8937,"Ġbrut":8938,"Ġperpet":8939,"hot":8940,"Ġhardly":8941,"ĠBurn":8942,"ãĤ¹":8943,"Ġsty":8944,"Ġtransactions":8945,"Ġgate":8946,"Ġscreens":8947,"Ġsubmitted":8948,"Ġ101":8949,"Ġlanguages":8950,"ught":8951,"emen":8952,"Ġfalls":8953,"Ġcoc":8954,"Ĥ¬":8955,"Ġstrikes":8956,"pa":8957,"Ġdeliber":8958,"ĠIM":8959,"Ġrelax":8960,"annels":8961,"ĠSenator":8962,"Ġextrem":8963,"Ġ},":8964,"ĠDeb":8965,"Ġbell":8966,"Ġdisorder":8967,"cut":8968,"ĠiOS":8969,"Ġlocked":8970,"Ġemissions":8971,"Ġshortly":8972,"\"]":8973,"ĠJudge":8974,"ĠSometimes":8975,"Ġrival":8976,"Ġdust":8977,"Ġreaching":8978,"File":8979,"¯¯¯¯":8980,"inois":8981,"ĠJason":8982,"Ġsatell":8983,"aret":8984,"Ġstations":8985,"Ġagric":8986,"ĠTechnology":8987,"comes":8988,"ĠUnfortunately":8989,"ĠChildren":8990,"Ġapplies":8991,"asted":8992,"Ġanger":8993,"ailability":8994,"ĠDamage":8995,"Ġcompare":8996,"ĠStandard":8997,"Ġaimed":8998,"ĠBa":8999,"anguage":9000,"Ġregulation":9001,"Ġjury":9002,"Ġairport":9003,"Ġsections":9004,"ĠPrince":9005,"emed":9006,"Ġmedicine":9007,"Ġhitting":9008,"Ġspark":9009,"olves":9010,"Ġads":9011,"State":9012,"Ġfoods":9013,"Ġreplacement":9014,"Ġchicken":9015,"Ġlowest":9016,"Ġminds":9017,"Ġinvolves":9018,"ui":9019,"Ġarrang":9020,"Ġprocedures":9021,"ĠWhich":9022,"iversary":9023,"Ġbills":9024,"Ġimprovement":9025,"Ġinev":9026,"Ġexpectations":9027,"Ġintellectual":9028,"Ġspaces":9029,"Ġmechanism":9030,"250":9031,"break":9032,"ĠZe":9033,"ĠTenn":9034,"ĠBalt":9035,"Ġbarrel":9036,"Ġstatic":9037,"mann":9038,"Police":9039,"Ġtips":9040,"Ġhandling":9041,"cus":9042,"oded":9043,"ilton":9044,"iry":9045,"Ġjournalists":9046,"ourse":9047,"Ġcomic":9048,"Ġnomine":9049,"ITY":9050,"Ġversus":9051,"Ġloop":9052,"Ġsurf":9053,"ĠIndust":9054,"ĠHunter":9055,"Ġbeliefs":9056,"isan":9057,"Ġsetup":9058,"Ġbrew":9059,"image":9060,"Ġcomputers":9061,"fol":9062,"},\"":9063,"ĠMedal":9064,"Ġtaxp":9065,"Ġdisplayed":9066,"Ġgrav":9067,"Ġfiscal":9068,"Mon":9069,"ĠMoscow":9070,"ĠKong":9071,"ĠCentre":9072,"Ġcameras":9073,"ĠMrs":9074,"ĠHay":9075,"Ġaver":9076,"ĠKelly":9077,"py":9078,"Ġrequirement":9079,"Ġentitled":9080,"ombie":9081,"Ġshadow":9082,"agic":9083,"ĠAk":9084,"Ġelite":9085,"Ġdivided":9086,"Ġheading":9087,"Ġcopies":9088,"Ġlosses":9089,"Ġvit":9090,"ked":9091,"ĠBry":9092,"Ġans":9093,"ĠSteam":9094,"Ġreporter":9095,"heim":9096,"ĠItem":9097,"Ġsuperior":9098,"don":9099,"erent":9100,"ö":9101,"Ġtherapy":9102,"Ġpeak":9103,"ĠModel":9104,"Ġlying":9105,"Ġgam":9106,"zer":9107,"ritten":9108,"Ġresponses":9109,"Ġconsideration":9110,"ĠBible":9111,"Ġloyal":9112,"Ġinstant":9113,"Ġpm":9114,"ĠForest":9115,"ü":9116,"Ġextend":9117,"Ġconvicted":9118,"Ġfounder":9119,"Ġconvin":9120,"ĠOak":9121,"check":9122,"Ġscholars":9123,"ped":9124,"Ġoverse":9125,"Top":9126,"count":9127,"ĠArk":9128,"·":9129,"Ġ06":9130,"ĠLA":9131,"md":9132,"ĠLatin":9133,"imental":9134,"ĠCPU":9135,"Ġsubstance":9136,"Ġminority":9137,"Ġmanufacturing":9138,"Er":9139,"ocolate":9140,"Ġattended":9141,"ĠManager":9142,"rations":9143,"Ġappreciate":9144,"omy":9145,"GBT":9146,"idency":9147,"BL":9148,"Ġguarantee":9149,"position":9150,"Ġocean":9151,"clude":9152,"Ġheaded":9153,"Ġtape":9154,"Ġloose":9155,"Ġlogic":9156,"Ġproven":9157,"Ġspir":9158,"Ġadmit":9159,"isa":9160,"Ġinvestigate":9161,"Ġ1994":9162,"sylv":9163,"ĠLost":9164,"cest":9165,"Ġ71":9166,"Ġrequested":9167,"Ġwindows":9168,"ĠPoké":9169,"ĠWithout":9170,"Met":9171,"Ġbehaviour":9172,"Ġreader":9173,"Ġhung":9174,"ĠKeep":9175,"Ġroles":9176,"Ġimplemented":9177,"Ġblank":9178,"Ġserves":9179,"ĠJay":9180,"Ġcited":9181,"ĠFriend":9182,"profit":9183,"apon":9184,"Ġrepair":9185,"item":9186,"arrass":9187,"Ġcritics":9188,"adi":9189,"ĠFather":9190,"Ġshout":9191,"Ġfool":9192,"Ġ88":9193,"Ġproducing":9194,"Ġlib":9195,"Ġrounds":9196,"Ġcircle":9197,"Ġprepar":9198,"Ġsubmit":9199,"Ġnic":9200,"morrow":9201,"ãĥ«":9202,"Under":9203,"Ġvital":9204,"atern":9205,"Ġpassword":9206,"Ġpublication":9207,"Ġprominent":9208,"Ġspeaks":9209,"Ġbars":9210,"Ġdeeper":9211,"ĠMill":9212,"ported":9213,"Ġwid":9214,"Ġbutter":9215,"Ġsmoking":9216,"Ġindicates":9217,"Key":9218,"ropri":9219,"ĠFile":9220,"alling":9221,"asting":9222,"ĠRus":9223,"Ġadj":9224,"Ġ79":9225,"aval":9226,"Ġpresum":9227,"burgh":9228,"onic":9229,"Ġfur":9230,"Ġpolls":9231,"ika":9232,"Ġsecondary":9233,"Ġmonster":9234,"igs":9235,"ĠCurrent":9236,"Event":9237,"Ġownership":9238,"endar":9239,"Ġarrive":9240,"ĠTax":9241,"Ġnull":9242,"ĠPriv":9243,"Ġthro":9244,"Ġkiss":9245,"cat":9246,"Ġupset":9247,"angle":9248,"itches":9249,"ector":9250,"ologists":9251,"ĠGalaxy":9252,"Ġcorruption":9253,"Ġhint":9254,"enter":9255,"ĠHospital":9256,"Ġgreatly":9257,"Ġbegun":9258,"esy":9259,"Ġsoil":9260,"ĠAnton":9261,"Ġmaintenance":9262,"ãĥ©":9263,"Ġdozens":9264,"Ġhumanity":9265,"ĠAlabama":9266,"Ġrom":9267,"worth":9268,"aping":9269,"sylvania":9270,"lah":9271,"Ġgathered":9272,"GA":9273,"Ġattacking":9274,"found":9275,"ĠSquare":9276,"Ġarbit":9277,"ictions":9278,"ĠWisconsin":9279,"Ġdance":9280,"ĠSaint":9281,"archy":9282,"Ġbaseball":9283,"Ġcontributions":9284,"Ġliterature":9285,"Ġexha":9286,"perty":9287,"test":9288,"Ġbab":9289,"Ġcontainer":9290,"letter":9291,"Ġfallen":9292,"Ġwebsites":9293,"Ġbottle":9294,"ĠSac":9295,"Ġbreast":9296,"ĠPL":9297,"Ġveteran":9298,"Ġinterviews":9299,"ĠAle":9300,"Ġbanned":9301,"engers":9302,"ĠRevolution":9303,"inth":9304,"Ġconcerning":9305,"IVE":9306,"Ġexpenses":9307,"ĠMatthew":9308,"ĠColumbia":9309,"ds":9310,"istance":9311,"Ġentity":9312,"...\"":9313,"Ġreliable":9314,"Ġparalle":9315,"ĠChristians":9316,"Ġopinions":9317,"Ġindu":9318,"low":9319,"Ġcompete":9320,"Ġthorough":9321,"Ġemployed":9322,"Ġestablishment":9323,"igen":9324,"ĠCro":9325,"Ġlawyers":9326,"ĠStation":9327,"TE":9328,"ĠLind":9329,"ĠPur":9330,"itary":9331,"Ġefficiency":9332,"âĢIJ":9333,"ĠLy":9334,"Ġmask":9335,"Ġdisaster":9336,"Ġages":9337,"ERE":9338,"esis":9339,"ĠHold":9340,"Ġcasual":9341,"bled":9342,"Ġenabled":9343,"ĠEnvironment":9344,"ĠIntelligence":9345,"iper":9346,"ĠMap":9347,"ĠBE":9348,"Ġemerged":9349,"isdom":9350,"Ġcabin":9351,"Ġregistration":9352,"Ġfingers":9353,"Ġroster":9354,"Ġframework":9355,"ĠDoctor":9356,"etts":9357,"Ġtransportation":9358,"Ġawareness":9359,"Her":9360,"Ġattempting":9361,"Off":9362,"ĠStore":9363,"ÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤ":9364,"ĠKnow":9365,"Ġdefence":9366,"Ġscan":9367,"ĠTen":9368,"ĠChair":9369,"ĠPH":9370,"ĠAtlanta":9371,"Ġfucking":9372,"Ġanswered":9373,"bn":9374,"ĠKar":9375,"Ġcategories":9376,"Ġrational":9377,"Ġcust":9378,"Ġrobot":9379,"Ġcorrectly":9380,"Ġgif":9381,"Ġgraphics":9382,"mic":9383,"Ġgrounds":9384,"ĠOpp":9385,"iate":9386,"Ġdistributed":9387,"Ġsanctions":9388,"Ġchallenging":9389,"uto":9390,"Ġingredients":9391,"Ġinvited":9392,"Ġfounded":9393,"ĠRequ":9394,"ded":9395,"Ġbowl":9396,"Ġbrothers":9397,"ĠHa":9398,"IO":9399,"Ġwages":9400,"imore":9401,"ocial":9402,"Ġseed":9403,"atively":9404,"Ġaddresses":9405,"ĠIowa":9406,"abeth":9407,"Ġattitude":9408,"isd":9409,"child":9410,"Ġmole":9411,"Ġdiscovery":9412,"yard":9413,"Br":9414,"Ġ82":9415,"Ġsupplies":9416,"elling":9417,"Ġdistingu":9418,"CR":9419,"Ġrecept":9420,"Ġvert":9421,"Ġswim":9422,"bec":9423,"door":9424,"ĠYeah":9425,"Ġgal":9426,"Ġinteract":9427,"ĠESP":9428,"ĠCS":9429,"amps":9430,"Ġconvinced":9431,"Ġobjective":9432,"Ġdish":9433,"ĠPhotos":9434,"lad":9435,"Ġdowntown":9436,"oil":9437,"inction":9438,"Ġtomorrow":9439,"ĠCOM":9440,"Ġsurvival":9441,"shot":9442,"Ġsettlement":9443,"Cons":9444,"ĠXbox":9445,"interest":9446,"ĠSM":9447,"argo":9448,"eness":9449,"Ġethnic":9450,"bered":9451,"Min":9452,"ĠTok":9453,"Ġincent":9454,"ĠCommand":9455,"Ġmaintained":9456,"Ġbreaks":9457,"bridge":9458,"atar":9459,"agg":9460,"ĠFinally":9461,"unicip":9462,"ĠOnt":9463,"left":9464,"Ġrecognition":9465,"Ġ*/":9466,"ĠPers":9467,"Ġwelf":9468,"Ġaddressed":9469,"ĠKansas":9470,"Ġvirus":9471,"Ġwhereas":9472,"Ġpapers":9473,"rams":9474,"ĠMinistry":9475,"Ġpleasure":9476,"Ġacquired":9477,"Ġduration":9478,"jpg":9479,"Ġcalm":9480,"ĠNHL":9481,"Ġburning":9482,"Ġfolder":9483,"icked":9484,"ĠPy":9485,"ĠIllinois":9486,"Class":9487,"ĠGoddess":9488,"Ġperforming":9489,"Ġwelfare":9490,"jar":9491,"Inter":9492,"Ġlin":9493,"Ġenhance":9494,"Ġnotion":9495,"fare":9496,"ypes":9497,"ĠArea":9498,"Ġcannabis":9499,"ĠDiego":9500,"fs":9501,"ĠManchester":9502,"comm":9503,"inite":9504,"Ġcovering":9505,"ĠSound":9506,"Ġ1960":9507,"Ġ84":9508,"elect":9509,"zing":9510,"Ġcitizen":9511,"Ġphones":9512,"Ġraid":9513,"Ġignored":9514,"ĠObject":9515,"Ġupload":9516,"card":9517,"Ġmodified":9518,"Ġrooms":9519,"iah":9520,"range":9521,"heast":9522,"achus":9523,"Ġsuggesting":9524,"âĢĭ":9525,"grade":9526,"El":9527,"Ġclothing":9528,"Ġrh":9529,"ĠHan":9530,"unity":9531,"encing":9532,"ĠAustin":9533,"secution":9534,"tra":9535,"dem":9536,"ĠQual":9537,"Ġheaven":9538,"Ġstages":9539,"Ġwedd":9540,"plus":9541,"ificial":9542,"ĠImm":9543,"ĠHo":9544,"ieties":9545,"Ġphrase":9546,"Ġbrill":9547,"actory":9548,"Ġproviders":9549,"Ġsilence":9550,"Ġaer":9551,"ĠAI":9552,"ĠAdventure":9553,"Ġplatforms":9554,"Ġdemonstrated":9555,"Ġinterf":9556,"ington":9557,"Ġraces":9558,"Ġgrade":9559,"ultane":9560,"ĠThrough":9561,"false":9562,"Ġbow":9563,"ĠAB":9564,"Ġflavor":9565,"Ġhistoric":9566,"gov":9567,"Ġcolour":9568,"Ġviewed":9569,"ĠEmail":9570,"elcome":9571,"Ġintervention":9572,"Ġdiversity":9573,"Ġperiods":9574,"Ġreverse":9575,"ĠVery":9576,"Ġquote":9577,"ĠLeft":9578,"through":9579,"Ġscrew":9580,"Ġlanding":9581,"Ġpill":9582,"Ġwet":9583,"Ġprotesters":9584,"Ġrepeat":9585,"aved":9586,"erk":9587,"Ġsalary":9588,"ĠPennsylvania":9589,"Still":9590,"Ġmayor":9591,"Ġkitchen":9592,"Ġfeaturing":9593,"ĠMuseum":9594,"ĠTournament":9595,"ĠFal":9596,"Ġservers":9597,"UC":9598,"Ġanybody":9599,"img":9600,"ĠTrade":9601,"ixture":9602,"theless":9603,"Ġfinance":9604,"Ġclosing":9605,"ĠPatri":9606,"iac":9607,"abel":9608,"Ġ>>":9609,"orous":9610,"Ġfirms":9611,"screen":9612,"una":9613,"Ġembarrass":9614,"ulse":9615,"Ġletting":9616,"Ġthrew":9617,"iley":9618,"Ġchannels":9619,"lan":9620,"ĠVegas":9621,"Ġsear":9622,"Ġfantastic":9623,"arre":9624,"uzzle":9625,"ĠDer":9626,"Those":9627,"Ġswing":9628,"Ġsheet":9629,"index":9630,"cover":9631,"ogan":9632,"Ġvariables":9633,"ĠTech":9634,"Ġspoken":9635,"achel":9636,"ĠDa":9637,"ĠMountain":9638,"Ġloaded":9639,"Ġfootage":9640,"version":9641,"Ġunl":9642,"ĠPhoenix":9643,"Ġthrowing":9644,"Ġfiring":9645,"Ġtracking":9646,"Ġwidth":9647,"Ġstruggling":9648,"rooms":9649,"otion":9650,"Ġmonthly":9651,"ĠServer":9652,"Ġeggs":9653,"open":9654,"MC":9655,"Ġ1993":9656,"Ġhired":9657,"Ġstayed":9658,"ĠAllen":9659,"Ġstro":9660,"Ġ98":9661,"step":9662,"ĠTurkish":9663,"Ġfabric":9664,"isting":9665,"ĠDom":9666,"Ġdates":9667,"Ġpron":9668,"Ġbasketball":9669,"Ġlucky":9670,"ĠArabia":9671,"Ġassumed":9672,"esty":9673,"Ġaffairs":9674,"Ġglad":9675,"ĠIndeed":9676,"ĠFA":9677,"ĠWord":9678,"Ġjoining":9679,"ifice":9680,"pread":9681,"irts":9682,"ĠSelect":9683,"Ġpopulations":9684,"aware":9685,"Ġnose":9686,"Ġcomplaints":9687,"start":9688,"Ġscoring":9689,"Thanks":9690,"Ġmining":9691,"Ġvisitors":9692,"SH":9693,"Ġdamaged":9694,"Ġcharacteristics":9695,"ĠPent":9696,"DC":9697,"Ġ83":9698,"ĠSix":9699,"rates":9700,"Ġflags":9701,"ĠBrew":9702,"dog":9703,"Mark":9704,"////":9705,"Ġexecution":9706,"Ġjoke":9707,"phones":9708,"Ġtestimony":9709,"Ġobst":9710,"QL":9711,"ĠCut":9712,"Ġstudied":9713,"ĠNintendo":9714,"icket":9715,"ĠNBC":9716,"Ġlad":9717,"ĠBra":9718,"ĠMoh":9719,"Ġkernel":9720,"Ġoverwhelming":9721,"Ġaged":9722,"Ġapplicable":9723,"ĠCond":9724,"Ġroads":9725,"ĠBlock":9726,"made":9727,"odge":9728,"Ġcommands":9729,"Ġoffices":9730,"veland":9731,"Ġtut":9732,"Ġreceiver":9733,"ĠFro":9734,"Ġshopping":9735,"ĠiP":9736,"ĠStre":9737,"ĠABC":9738,"Ġentertainment":9739,"ĠBow":9740,"orted":9741,"Mc":9742,"Ġreads":9743,"grad":9744,"ĠCollect":9745,"ĠâĪĴ":9746,"ĠCapital":9747,"ederation":9748,"Ġemployer":9749,"Ġinvolvement":9750,"Ġanxiety":9751,"alia":9752,"Ġroof":9753,"ĠAmong":9754,"ĠDemocrat":9755,"Ġstats":9756,"ĠVill":9757,"Ġconstitutional":9758,"Ġreferring":9759,"itty":9760,"Ġtackle":9761,"outube":9762,"Ġbacked":9763,"ĠHong":9764,"ĠBroad":9765,"Ġele":9766,"ĠOtt":9767,"Ġ1992":9768,"hour":9769,"achusetts":9770,"Cal":9771,"Ġdefeated":9772,"Ġ81":9773,"esp":9774,"Ġseemingly":9775,"was":9776,"ĠJenn":9777,"ĠKurd":9778,"Ġgene":9779,"Ġdiscount":9780,"Ret":9781,"ECT":9782,"();":9783,"Ġclubs":9784,"Ġsid":9785,"ĠMarsh":9786,"Check":9787,"Ġpp":9788,"ĠEag":9789,"idespread":9790,"Ġbeings":9791,"FT":9792,"Ġintroduction":9793,"ĠChange":9794,"ARD":9795,"Ġ110":9796,"adows":9797,"ierce":9798,"Ġmeal":9799,"author":9800,"ĠBang":9801,"lahoma":9802,"Ġranks":9803,"2011":9804,"????":9805,"max":9806,"Ġcollapse":9807,"Ġopens":9808,"Ġecho":9809,"Ġsoph":9810,"Ġracist":9811,"Ġenormous":9812,"Ġwaves":9813,"Ġtap":9814,"Ġcomprehensive":9815,".--":9816,"ĠRoy":9817,"Ġfarmers":9818,"Related":9819,"aired":9820,"rones":9821,"ĠCrim":9822,"Ġproportion":9823,"Ġdesigns":9824,"Ġnegotiations":9825,"Ġvirtually":9826,"ĠBatman":9827,"Ġwarn":9828,"Ġlegitimate":9829,"mate":9830,"Ġconvention":9831,",,":9832,"netic":9833,"ĠSD":9834,"Ġconsistently":9835,"Ġcompensation":9836,"Ġpunishment":9837,"Ġye":9838,"Ġtie":9839,"ĠBureau":9840,"irlf":9841,"ĠBu":9842,"ĠAren":9843,"ĠPhilipp":9844,"Ġknife":9845,"Ġmemories":9846,"ĠRoss":9847,"Ġangle":9848,"Ġ86":9849,"ĠThunder":9850,"Ġrend":9851,"ĠTour":9852,"Ġcounts":9853,"sung":9854,"ĠImp":9855,"Ġeducational":9856,"Ġaccessible":9857,"COM":9858,"Ġdrew":9859,"yer":9860,"Gl":9861,"amine":9862,"ORT":9863,"OB":9864,"IB":9865,"master":9866,"Ġtrials":9867,"ogy":9868,"har":9869,"ĠTrust":9870,"Ġpreferred":9871,"irlfriend":9872,"ĠNev":9873,"Ġbin":9874,"Ġcow":9875,"Page":9876,"Ġsignature":9877,"ĠBL":9878,"700":9879,"Ġretired":9880,"Ġbytes":9881,"Ġneighb":9882,"ĠLegend":9883,"Ġdevast":9884,"Ġsuspected":9885,"isons":9886,"ĠPokémon":9887,"scale":9888,"Ġcapabilities":9889,"Ġrevel":9890,"Ġcheese":9891,"dy":9892,"igrant":9893,"Ġfailing":9894,"bits":9895,"ĠHeroes":9896,"ĠGhost":9897,"ĠScient":9898,"Ġappointed":9899,"uri":9900,"Ġinstitution":9901,"Ġexpanded":9902,"greg":9903,"Ġmonitoring":9904,"Ġpodcast":9905,"Ġcoalition":9906,"Ġ96":9907,"Jo":9908,"Ġstolen":9909,"ĠSab":9910,"Ġstops":9911,"Ġholiday":9912,"Ġintr":9913,"Car":9914,"Black":9915,"ĠLGBT":9916,"Ġwarming":9917,"ĠAnderson":9918,"Ġ89":9919,"Ġproducer":9920,"Med":9921,"Ġaccuracy":9922,"ĠMarvel":9923,"izabeth":9924,"ĠPatrick":9925,"mony":9926,"Ġmini":9927,"acles":9928,"Ġovert":9929,"they":9930,"Ġmembership":9931,"ĠVen":9932,"Ġexch":9933,"Ġremoval":9934,"ĠDave":9935,"TY":9936,"mad":9937,"ĠFind":9938,"Ġadequ":9939,"Ġec":9940,"Ġteeth":9941,"Ġemotion":9942,"Ġperm":9943,"Ġsolely":9944,"db":9945,"Ġextraord":9946,"IGHT":9947,"cal":9948,"Ġguidelines":9949,"Ġdying":9950,"Ġsuspended":9951,"ĠPremier":9952,"ĠAnthony":9953,"elve":9954,"Ġdad":9955,"ĠEth":9956,"ĠFootball":9957,"Ġabandoned":9958,"Ġ<<":9959,"Ġmarch":9960,"Ġhorror":9961,"â̦\"":9962,"Ġchildhood":9963,"Ġcampaigns":9964,"Ġlunch":9965,"ĠAlbert":9966,"block":9967,"âĸĪâĸĪ":9968,"ounding":9969,"Ġbone":9970,"organ":9971,"aders":9972,"ĠFlash":9973,"ĠDrive":9974,"Ġtonight":9975,"Ġwars":9976,"ĠFL":9977,"Ġformation":9978,"const":9979,"News":9980,"Ġcompe":9981,"orious":9982,"ĠStaff":9983,"Ġdiscussions":9984,"ĠProtection":9985,"ĠJam":9986,"Ġcriteria":9987,"Ġinstallation":9988,"Ġaccomplish":9989,"izza":9990,"Ġpublisher":9991,"Ġrescue":9992,"ĠTry":9993,"ULL":9994,"ĠSom":9995,"ĠHop":9996,"oret":9997,"ths":9998,"ordon":9999,"Ġpocket":10000,"ĠInv":10001,"Download":10002,"ĠCrime":10003,"Ġbene":10004,"ĠGuide":10005,"ĠAssembly":10006,"Ġparameters":10007,"IE":10008,"ĠAlexander":10009,"Ġconcert":10010,"ĠSche":10011,"Ġshoes":10012,"Ġvisiting":10013,"Ġrecall":10014,"Ġbub":10015,"Ġrural":10016,"Ġconcrete":10017,"ĠRos":10018,"Next":10019,"Russ":10020,"Ġloans":10021,"ĠShield":10022,"Ġtrem":10023,"hemat":10024,"kg":10025,"ĠHarris":10026,"isition":10027,"ĠMove":10028,"ĠFC":10029,"Ġfate":10030,"ĠCho":10031,"Ġtired":10032,"Ġprincipal":10033,"hist":10034,"iences":10035,"athy":10036,"Ġsevent":10037,"Ġmood":10038,"Ġstrategic":10039,"Ġdiseases":10040,"Ġforum":10041,"Ġtempor":10042,"Ġheadquarters":10043,"Par":10044,"ige":10045,"flix":10046,"Ġguitar":10047,"Ġ94":10048,"Only":10049,"Ġreleases":10050,"roph":10051,"================================":10052,"Ġ600":10053,"ĠContinue":10054,"igate":10055,"ĠCrit":10056,"system":10057,"Ġdisabled":10058,"Ġunexpected":10059,"ithub":10060,"Ġunclear":10061,"ĠEst":10062,"Ġcontrad":10063,"Ġstrategies":10064,"ventures":10065,"Ġpassage":10066,"AME":10067,"Ġimproving":10068,"Ġreveals":10069,"Ġdecrease":10070,"ova":10071,"Ġannoy":10072,"ĠShort":10073,"ĠLibrary":10074,"Ġcyber":10075,"nell":10076,"ĠHur":10077,"ĠCB":10078,"Ġphotograp":10079,"UI":10080,"Ġsed":10081,"Ge":10082,"Ġ87":10083,"Ġdiverse":10084,"Ġencouraged":10085,"Ġconspiracy":10086,"Ġbirds":10087,"Ġoperator":10088,"Ġhandful":10089,"Ġclassified":10090,"?)":10091,"Ġdramatic":10092,"Ġinvestigators":10093,"ito":10094,"Ġwidespread":10095,"ĠRoom":10096,"----------------------------------------------------------------":10097,"Ġcollective":10098,"Ġjournalist":10099,"String":10100,"Ġtemperatures":10101,"ila":10102,"Ġguid":10103,"Ġinspect":10104,"Ġmissile":10105,"ĠMayor":10106,"Ġmanual":10107,"Ġsimultane":10108,"Ġratings":10109,"Ġsuck":10110,"Ġ97":10111,"Ġuniversal":10112,"Ġpharm":10113,"Ġdisrupt":10114,"iano":10115,"AV":10116,"Ġft":10117,"Ġstatist":10118,"olds":10119,"ĠWalker":10120,"php":10121,"Ġundert":10122,"ĠLas":10123,"ishop":10124,"ntil":10125,"reshold":10126,"ĠWhether":10127,"Ms":10128,"Ġdeny":10129,"ĠCloud":10130,"Ġprovider":10131,"Ġsurviv":10132,"ĠUpdate":10133,"has":10134,"Ġmistakes":10135,"charge":10136,"pled":10137,"rity":10138,"Ġnode":10139,"ĠMassachusetts":10140,"ools":10141,"lication":10142,"Ġfails":10143,"emale":10144,"ori":10145,"backs":10146,"Ġshirt":10147,"Ġ''":10148,"ĠNAT":10149,"Ġwaters":10150,"elson":10151,"Ġease":10152,"Ġscar":10153,"Ġcontents":10154,"mind":10155,"Ġcontribution":10156,"Ġshr":10157,"Ġhanded":10158,"Ġstability":10159,"Ġtrave":10160,"Em":10161,"Ġmirror":10162,"123":10163,"Ġweigh":10164,"Ġfiction":10165,"ouver":10166,"istant":10167,"rition":10168,"ĠFed":10169,"Ġphysically":10170,"Ġstake":10171,"ĠArticle":10172,"ĠArc":10173,"ĠLewis":10174,"ĠMind":10175,"Ġdemonstrate":10176,"Ġprofits":10177,"vision":10178,"omic":10179,"olid":10180,"Ġbattles":10181,"Ġdrives":10182,"Ġeastern":10183,"ĠSony":10184,"!!!":10185,"aration":10186,"vard":10187,"ĠGL":10188,"portation":10189,"Ġ92":10190,"Ġlawmakers":10191,"Ġprotecting":10192,"ĠEPA":10193,"Ġyeah":10194,"Ġshame":10195,"olph":10196,"even":10197,"xit":10198,"Ġattach":10199,"Ġrepresenting":10200,"Ġobs":10201,"ĠUtah":10202,"iffs":10203,"ĠFreedom":10204,"ó":10205,"AK":10206,"Ġincidents":10207,"itage":10208,"Ġviewers":10209,"cd":10210,"Ġmouse":10211,"Ġclar":10212,"Ġaccordance":10213,"Ġbot":10214,"cor":10215,"ĠSummer":10216,"held":10217,"Ġinnocent":10218,"Ġinitiative":10219,"ols":10220,"________________________________":10221,"Ġspots":10222,"pace":10223,"Ġconventional":10224,"Ġcorporations":10225,"Ġblocked":10226,"HD":10227,"attered":10228,"Ġrefers":10229,"Ġbuck":10230,"ĠDigital":10231,"120":10232,"Ġtopics":10233,"TF":10234,"Äģ":10235,"brid":10236,"reement":10237,"Ġunderlying":10238,"ĠMember":10239,"Ġinvestigating":10240,"Ġpregnancy":10241,"Ġtouchdown":10242,"ĠBand":10243,"ĠCaller":10244,"Ġinstances":10245,"PP":10246,"wa":10247,"Good":10248,"Ġ1991":10249,"ĠCold":10250,"Ġfears":10251,"Ġremarks":10252,"ĨĴ":10253,"atal":10254,"Ġmit":10255,"Ġexperiments":10256,"ipt":10257,"Color":10258,"indu":10259,"Update":10260,"Ġ93":10261,"Ag":10262,"Ġå":10263,"ancouver":10264,"Both":10265,"Ġjudges":10266,"Object":10267,"Ġstere":10268,"umbn":10269,"Ġparticipation":10270,"ĠStars":10271,"ĠJere":10272,"Ġweekly":10273,"ĠBan":10274,"Ġconversations":10275,"ĠPitt":10276,"uz":10277,"ĠIndiana":10278,"ĠKick":10279,"Ġinfection":10280,"Ġheroes":10281,"Ġsettled":10282,"Ġstrip":10283,"Ġhal":10284,"Ġdump":10285,"ĠSci":10286,"Ġles":10287,"Ġreferences":10288,"ĠURL":10289,"ĠBridge":10290,"Ġwanting":10291,"Force":10292,"Ġexclus":10293,"Meanwhile":10294,"mn":10295,"Ġgentle":10296,"maker":10297,"senal":10298,"ĠGro":10299,"ouri":10300,"ĠRain":10301,"ĠAlliance":10302,"Ġlift":10303,"ela":10304,"SD":10305,"ĠCleveland":10306,"Ġranked":10307,"Ġstadium":10308,"Ġdeadly":10309,"ä¸":10310,"Ġriding":10311,"aria":10312,"ĠArmor":10313,"Ġdocumentation":10314,"ĠGreece":10315,"reek":10316,"Ġlens":10317,"ĠSa":10318,"Ġgross":10319,"ĠEmer":10320,"agers":10321,"ĠDub":10322,"ĠRh":10323,"ĠAMD":10324,"Ġarrival":10325,"Ġdesert":10326,"Ġsupplement":10327,"ĠResp":10328,"Ġknee":10329,"Ġmargin":10330,"font":10331,"ogg":10332,"2010":10333,"ĠPir":10334,"ĠProm":10335,"ivals":10336,"Ġintake":10337,"Ġdifferently":10338,"ugs":10339,"Ġbits":10340,"cluded":10341,"Ġsearching":10342,"ĠDu":10343,"umble":10344,"Ġfunctional":10345,"ĠBaltimore":10346,"ĠCould":10347,"Ġdesired":10348,"Ġcircuit":10349,"ĠLyn":10350,"ĠGO":10351,"ĠFalse":10352,"repre":10353,"':":10354,"alties":10355,"Ġminim":10356,"Ġdrove":10357,"ĠShould":10358,"Ġhip":10359,"Ġpros":10360,"Ġutility":10361,"ĠNature":10362,"ĠMode":10363,"President":10364,"opp":10365,"rat":10366,"formance":10367,"Ġconcentration":10368,"Ġfont":10369,"ĠBud":10370,"Ġamid":10371,"Ġrevers":10372,"ĠML":10373,"Bar":10374,"Ġinteraction":10375,"Ġjurisd":10376,"Ġspells":10377,"dep":10378,"fil":10379,"Ġcivilians":10380,"utter":10381,"ĠCooper":10382,"ĠBelow":10383,"Ġentrance":10384,"Ġconvert":10385,"Ġcontroversy":10386,"owered":10387,"Ġcontrary":10388,"Ġarc":10389,"ĠExecutive":10390,"ĠOfficer":10391,"Ġpackages":10392,"Ġprogressive":10393,"width":10394,"Ġreserved":10395,"vol":10396,"ĠSamsung":10397,"Ġprinted":10398,"Ġcenters":10399,"Ġintroduce":10400,"ĠKennedy":10401,"Ġodds":10402,"Ġsurely":10403,"Ġindependence":10404,"Ġpassengers":10405,"reprene":10406,"ĠBeh":10407,"Ġloves":10408,"ĠESPN":10409,"Ġfacilit":10410,"Ġidentical":10411,"Ġdoct":10412,"Ġpartnership":10413,"conf":10414,"ĠHide":10415,"Ġconfused":10416,"ĠCow":10417,"Men":10418,"Ġwrest":10419,"ĠIraqi":10420,"Ġholes":10421,"ĠStudies":10422,"Ġpregnant":10423,"hard":10424,"Ġsignals":10425,"IX":10426,"Ġpulling":10427,"Ġgraduate":10428,"Ġnominee":10429,"Date":10430,"Ġpermitted":10431,"ĠâĤ¬":10432,"ĠOklahoma":10433,"Start":10434,"Ġauthorized":10435,"Ġalarm":10436,"ĠCos":10437,"van":10438,"Ġgenerations":10439,"cular":10440,"Ġdragon":10441,"ĠSoftware":10442,"ĠEdward":10443,"Ġcontroller":10444,"Sen":10445,"gered":10446,"ĠVik":10447,"Ġapproached":10448,"Thank":10449,"Ġcance":10450,"Ġformula":10451,"ĠSmall":10452,"Ġweakness":10453,"Ġramp":10454,"itudes":10455,"jud":10456,"Ġbrilliant":10457,"Ġaccus":10458,"source":10459,"Ġ800":10460,"ĠEvil":10461,"Sw":10462,"Ġhomeless":10463,"week":10464,"iens":10465,"rics":10466,"ĠThird":10467,"TO":10468,"Ġorganic":10469,"Ġpresentation":10470,"agh":10471,"ĠDownload":10472,"vation":10473,"Ġassembly":10474,"orable":10475,"holders":10476,"ĠBernie":10477,"ĠHelp":10478,"Ġtong":10479,"ĠFight":10480,"Ġbeach":10481,"Book":10482,"ĠLic":10483,"Ġrush":10484,"ĠRound":10485,"oup":10486,"ĠMarx":10487,"Ġcalculated":10488,"ĠDevil":10489,"ĠSarah":10490,"Ġoccasionally":10491,"Ġbullet":10492,"Available":10493,"gate":10494,"Ġ91":10495,"Ġhosp":10496,"Ġpromises":10497,"ĠHIV":10498,"ĠStadium":10499,"ĠStock":10500,"ĠCorporation":10501,"gage":10502,"NG":10503,"ĠCredit":10504,"Ġsne":10505,"ibl":10506,"Ġaccum":10507,"such":10508,"Ġterrorists":10509,"Ġconsciousness":10510,"ĠZh":10511,"Ġdrama":10512,"oola":10513,"piration":10514,"Ġlabour":10515,"ĠNin":10516,"Ġutter":10517,"Ġdemocratic":10518,"Ġassass":10519,"ilation":10520,"Ġgest":10521,"Ġabroad":10522,"Ġmetab":10523,"Ġsorts":10524,"Ġflav":10525,"UB":10526,"Ġmg":10527,"ĠNothing":10528,"ĠOd":10529,"Ġmusical":10530,"2009":10531,"Ġdrops":10532,"ocated":10533,"ateral":10534,"000000":10535,"Ġgre":10536,"Ġequality":10537,"Ġburden":10538,"Ġvig":10539,"ĠLeader":10540,"------------":10541,"Ġceremony":10542,"Ġfighter":10543,"Ġactors":10544,"Ġæ":10545,"aman":10546,"Fi":10547,"Ġalign":10548,"puter":10549,"Ġelder":10550,"ĠNSA":10551,"Ġrepresentation":10552,"ĠOntario":10553,"ITH":10554,"usalem":10555,"Ġharassment":10556,"itzer":10557,"Ġsymp":10558,"Ġboxes":10559,"ĠDR":10560,"Ġmanifest":10561,"atre":10562,"Ġ^":10563,"Ġdies":10564,"leton":10565,"Ġmissions":10566,"ethe":10567,"Ġresolve":10568,"Ġfollowers":10569,"Ġasc":10570,"Ġkm":10571,"lord":10572,"ammed":10573,"Ġsilent":10574,"ĠAssociated":10575,"Ġtiming":10576,"Ġprisoners":10577,"ĠKings":10578,"ĠFive":10579,"Ġtower":10580,"Ġapproaches":10581,"Ġprecisely":10582,"Ġbureau":10583,"ĠMother":10584,"ĠIss":10585,"Ġkeyboard":10586,"itual":10587,"Ġfunded":10588,"Ġstaying":10589,"Ġpsychological":10590,"Ġmile":10591,"ĠLeon":10592,"ĠBarb":10593,"will":10594,"Ġwider":10595,"ĠAtlantic":10596,"Ġtill":10597,"ĠRome":10598,"rot":10599,"Ġaccompan":10600,"Ġflour":10601,"aco":10602,"World":10603,"ĠExpress":10604,"ĠYu":10605,"Cor":10606,"Ġpleased":10607,"party":10608,"Ġpointing":10609,"Ġinflation":10610,"Ġroy":10611,"Ġ),":10612,"ainer":10613,"Ġwedding":10614,"ormon":10615,"Ġrequiring":10616,"Ġqualified":10617,"Ġsegment":10618,"END":10619,"Ġsizes":10620,"eals":10621,"Ġcorrupt":10622,"assador":10623,"Ġceleb":10624,"Ġdreams":10625,"ĠMess":10626,"Ġchecking":10627,"ĠVersion":10628,"Ġpreparing":10629,"Ġactively":10630,"ĠDiff":10631,"Ġlux":10632,"ĠWinter":10633,"acteria":10634,"ĠNE":10635,"Ġdeputy":10636,"Ġtransgender":10637,"Ġsummary":10638,"Ġinher":10639,"eries":10640,"char":10641,"ĠYan":10642,"Ġknock":10643,"ĠPath":10644,"Ġlip":10645,"roller":10646,"Ġimpression":10647,"Ġcelebrate":10648,"Ġslide":10649,"Ġguests":10650,"Ġclip":10651,"FS":10652,"Ġsavings":10653,"Ġcaptain":10654,"Ġlegacy":10655,"ĠDenver":10656,"Ġwounded":10657,"taboola":10658,"ACT":10659,"Ġpursue":10660,"Ġoxy":10661,"Ġq":10662,"Ġsemi":10663,"ĠNeed":10664,"ĠAffairs":10665,"Ġobsc":10666,"Ġchecked":10667,"Ġdual":10668,"Code":10669,"ĠMD":10670,"lem":10671,"ulty":10672,"Ġ©":10673,"ĠElizabeth":10674,"Ġcenturies":10675,"arded":10676,"src":10677,"Ġevident":10678,"ennis":10679,"atin":10680,"Ġunemployment":10681,"ĠMario":10682,"Ġintim":10683,"Christ":10684,"Ġbiological":10685,"Ġsoldier":10686,"ĠAdded":10687,"Ġmath":10688,"ĠGil":10689,"Ġbias":10690,"Ġdating":10691,"ĠOcean":10692,"Ġmice":10693,"Mus":10694,"hire":10695,"ĠTes":10696,"Server":10697,"limited":10698,"Size":10699,"Ġmeters":10700,"Ġrocket":10701,"essee":10702,"Ġcertificate":10703,"ĠIranian":10704,"ASS":10705,"Ġgrid":10706,"Dec":10707,"Ġrolling":10708,"commun":10709,"ĠSweden":10710,"bury":10711,"Ġtissue":10712,"Ġracism":10713,"ĠLocal":10714,"Ġmystery":10715,"Ġexamine":10716,"Ġstem":10717,"Ġsits":10718,"Ġhoped":10719,"oting":10720,"Ġdialogue":10721,"Ġpersu":10722,"Watch":10723,"lay":10724,"MAN":10725,"Ġchronic":10726,"ĠPortland":10727,"market":10728,"ĠSEC":10729,"Ġparallel":10730,"Ġscandal":10731,"Ġcarries":10732,"Ġphenomenon":10733,"human":10734,"acker":10735,"ĠOx":10736,"Ġretirement":10737,"tainment":10738,"ovie":10739,"ĠGear":10740,"Ġduties":10741,"Ġdose":10742,"Ġscroll":10743,"MB":10744,"inf":10745,"Ġsauce":10746,"Ġlandscape":10747,"reddit":10748,"ĠChampionship":10749,"ĠReddit":10750,"alid":10751,"Ġcoin":10752,"Ġovers":10753,"Ġposting":10754,"about":10755,"Ġfel":10756,"andy":10757,"Ġbold":10758,"Ġfocusing":10759,"effect":10760,"GR":10761,"Ġdeemed":10762,"Ġrecommendations":10763,"Ġstepped":10764,"Ġvoter":10765,"ĠDeep":10766,"ĠInstagram":10767,"Ġmoderate":10768,"ĠMaryland":10769,"Ġrestricted":10770,"ĠMB":10771,"ĠChall":10772,"Ġtob":10773,"Ġcir":10774,"ĠOcc":10775,"ĠEver":10776,"Ġcollaps":10777,"INFO":10778,"=-":10779,"ĠPict":10780,"ĠAccount":10781,"nc":10782,"Ġought":10783,"Ġexport":10784,"Ġdrunk":10785,"('":10786,"Ġwise":10787,"ĠMort":10788,"necess":10789,"Ġancest":10790,"ĠIncre":10791,"Ġfrequent":10792,"mir":10793,"Ġinterpretation":10794,"Ġdependent":10795,"Ġcoins":10796,"ĠBol":10797,"Video":10798,"ĠJustin":10799,"Ġfatal":10800,"Ġcooking":10801,"Ġconfusion":10802,"ipher":10803,"Ġcustody":10804,"ĠMorgan":10805,"omach":10806,"ĠGovernor":10807,"Ġrestaurants":10808,"eling":10809,"Ġacknowledged":10810,"Ġther":10811,"Ġgenes":10812,"ching":10813,"Hey":10814,"Ġtactics":10815,"ĠMexican":10816,"Ġvend":10817,"Ġhes":10818,"quer":10819,"Ġnoting":10820,"ĠCameron":10821,"Ġtargeting":10822,"rock":10823,"Ġcredits":10824,"Ġemotions":10825,"Ġrepresentatives":10826,"news":10827,"Ġlegislative":10828,"Ġremoving":10829,"Ġtweeted":10830,"ĠCarter":10831,"ĠFixed":10832,"Ġforcing":10833,"Ġspeaker":10834,"Ġmales":10835,"ĠVietnam":10836,"lined":10837,"Ġconcepts":10838,"Ġvoices":10839,"oir":10840,"ĠTrib":10841,"Whe":10842,"ĠJerusalem":10843,"ĠSant":10844,"Ġcul":10845,"Ġlady":10846,"ĠHawai":10847,"Ġarts":10848,"ĠInn":10849,"ĠMachine":10850,"ĠEmperor":10851,"Ġslot":10852,"gly":10853,"ĠProcess":10854,"III":10855,"Ġathletes":10856,"ĠTemple":10857,"ĠRepresent":10858,"Ġpresc":10859,"Ġtons":10860,"Ġgolden":10861,"Ġpunch":10862,"ĠGR":10863,"iverpool":10864,"Ġenact":10865,"Ġlobby":10866,"Ġmos":10867,"Ġpicking":10868,"Ġlifetime":10869,"Ġcognitive":10870,"Each":10871,"zo":10872,"Ġdub":10873,"Ġconsists":10874,"oln":10875,"Ġfestival":10876,"amous":10877,"Ġintellig":10878,"words":10879,"ĠSmart":10880,"Ġdele":10881,"Ġlapt":10882,"Ġmagical":10883,"ĠSin":10884,"bus":10885,"urities":10886,"ighth":10887,"ĠRuby":10888,"ĠSure":10889,"olving":10890,"Ġjun":10891,"OST":10892,"Ġimposed":10893,"Ġastron":10894,"Ġcorrel":10895,"ĠNS":10896,"ĠKit":10897,"ĠFuture":10898,"burn":10899,"Ġimmune":10900,"ocus":10901,"Ġcourses":10902,"ĠString":10903,"Ġlean":10904,"Ġghost":10905,"Ġoutcomes":10906,"Ġexpense":10907,"Ġeveryday":10908,"Ġacceptable":10909,"Ah":10910,"Ġequipped":10911,"Ġorange":10912,"FR":10913,"ĠDutch":10914,"Though":10915,"ĠRank":10916,"QU":10917,"ĠRoberts":10918,"what":10919,"rend":10920,"Ġdisappear":10921,"Ġspawn":10922,"ĠLam":10923,"ois":10924,"Ġdeserve":10925,"Ġminimal":10926,"Ġnervous":10927,"ĠWould":10928,"Ġrook":10929,"ĠVancouver":10930,"Ġresign":10931,"shire":10932,"ĠWorks":10933,"ĠBuild":10934,"Ġaffordable":10935,"ĠGary":10936,"ĠArena":10937,"Ġhanging":10938,"Ġimplications":10939,"ĠSong":10940,"Ġmaintaining":10941,"Ġguards":10942,"CON":10943,"Ġderived":10944,"Ġexecuted":10945,"Ġtheories":10946,"Ġquoted":10947,"ĠAndre":10948,"oga":10949,"seless":10950,"info":10951,"ĠBelg":10952,"Ġtears":10953,"ĠSurv":10954,"Ġbirthday":10955,"igious":10956,"immer":10957,"Ġspectrum":10958,"Ġarchitecture":10959,"Ġrecruit":10960,"arma":10961,"Table":10962,"Ġmonsters":10963,"ĠGov":10964,"Ġdestination":10965,"Ġattractive":10966,"Ġfoss":10967,"ĠMoreover":10968,"Ġpresents":10969,"THE":10970,"Ġreply":10971,"pton":10972,"Ġcum":10973,"Ġdelight":10974,"Ġaffects":10975,"Ġdonations":10976,"ĠToy":10977,"ĠHim":10978,"MENT":10979,"Ġovercome":10980,"itched":10981,"ĠFantasy":10982,"ĠHat":10983,"ĠBeast":10984,"bott":10985,"Ġinvestigations":10986,"Run":10987,"Ġhunting":10988,"di":10989,"fund":10990,"Ġsessions":10991,"estyle":10992,"Ġportray":10993,"oids":10994,"Yeah":10995,"Ġcommunicate":10996,"Ġcomedy":10997,"ĠYang":10998,"Ġbelt":10999,"ĠMarine":11000,"Ġpredicted":11001,"Play":11002,"Ġimportantly":11003,"Ġremarkable":11004,"Ġeliminate":11005,"David":11006,"Ġbind":11007,"VID":11008,"Ġadvocates":11009,"ĠGaza":11010,"imp":11011,"DB":11012,"ĠNa":11013,"ĠSimilar":11014,"IES":11015,"Ġcharity":11016,"vas":11017,"math":11018,"Ġâĸ":11019,"oker":11020,"ndum":11021,"Ġcaps":11022,"ĠHal":11023,"2000":11024,"ean":11025,"Ġfleet":11026,"Ġrecre":11027,"Right":11028,"Ġsleeping":11029,"ijing":11030,"kind":11031,"Ġdesignated":11032,"ä":11033,"Ġanimation":11034,"kee":11035,"ĠIntrodu":11036,"Ġ/>":11037,"Ġdelayed":11038,"Ġtremend":11039,"Ġcurious":11040,"Use":11041,"Ġlect":11042,"dam":11043,"Ġinnovation":11044,"ĠPoints":11045,"Ġloading":11046,"Ġdispute":11047,"ctic":11048,"irds":11049,"ĠBY":11050,"Ġnurs":11051,"ĠValue":11052,"IONS":11053,"ĠHum":11054,"Ġtemplate":11055,"mers":11056,"Ġappearances":11057,"ĠEntertainment":11058,"Ġtranslation":11059,"Ġsake":11060,"Ġbeneath":11061,"Ġinhib":11062,"Ġeuro":11063,"abetes":11064,"Ġstudying":11065,"ĠMas":11066,"Ġperceived":11067,"Ġexamined":11068,"Ġeager":11069,"Ġcoaches":11070,"Ġimper":11071,"chi":11072,"Ġproduces":11073,"\").":11074,"ĠEveryone":11075,"Ġmunicip":11076,"Ġgirlfriend":11077,"Ġhire":11078,"ĠVice":11079,"Ġsuitable":11080,"opy":11081,"Ġinequ":11082,"ĠDuke":11083,"fish":11084,"first":11085,"ĠObs":11086,"Ġinterior":11087,"ĠBruce":11088,"ĠRy":11089,"Ġanalys":11090,"Ġconsiderable":11091,"Ġforecast":11092,"Ġfert":11093,"orship":11094,"ĠDrug":11095,"ĠALL":11096,":\"":11097,"thur":11098,"ĠMail":11099,"Ġballot":11100,"Ġinstantly":11101,"ĠChannel":11102,"Ġpicks":11103,"Ġ1989":11104,"Ġtent":11105,"oli":11106,"Ġcivilian":11107,"bling":11108,"ello":11109,"bu":11110,"Ġinch":11111,"Ġlogo":11112,"Ġcooperation":11113,"Ġwalks":11114,"Ġinvestments":11115,"Ġimprison":11116,"ĠFestival":11117,"ĠKy":11118,"Ġlegally":11119,"Ġgri":11120,"charg":11121,"Sl":11122,"Ġthreatening":11123,"duction":11124,"flow":11125,"Ġdismissed":11126,"ibraries":11127,"cap":11128,"ele":11129,"ĠMcG":11130,"ĠHarvard":11131,"ĠConservative":11132,"ĠCBS":11133,"png":11134,"Ġroots":11135,"ĠHaving":11136,"umbled":11137,"ĠFun":11138,"\\/":11139,"ĠSearch":11140,"plex":11141,"Ġdiscussing":11142,"Ġcontinu":11143,"ĠTai":11144,"ĠWik":11145,"Free":11146,"fit":11147,"Ġrefuse":11148,"Ġmanaging":11149,"Ġsynd":11150,"ipedia":11151,"walk":11152,"Ġprofessionals":11153,"Ġguidance":11154,"Ġuniversities":11155,"Ġassemb":11156,"untu":11157,"Finally":11158,"ASE":11159,"ĠAuto":11160,"ĠHad":11161,"Ġanniversary":11162,"LD":11163,"ĠDur":11164,"ĠUltimate":11165,"ihad":11166,"product":11167,"Ġtransit":11168,"Ġrestore":11169,"Ġexplaining":11170,"Ġasset":11171,"Ġtransferred":11172,"Ġburst":11173,"apolis":11174,"ĠMagazine":11175,"ĠCra":11176,"ĠBR":11177,"gged":11178,"ĠHE":11179,"Mich":11180,"bet":11181,"ĠLady":11182,"ylum":11183,"erves":11184,"Ġmeets":11185,"white":11186,"Log":11187,"Ġcorresponding":11188,"Ġinsisted":11189,"GG":11190,"Ġsurrounded":11191,"Ġtens":11192,"Ġlane":11193,"Ġcoinc":11194,"home":11195,"Ġexisted":11196,"ected":11197,"ĠDouble":11198,"lamm":11199,"Ġskept":11200,"exp":11201,"Ġperception":11202,"iev":11203,"ĠBeing":11204,"oft":11205,"Ġadopt":11206,".:":11207,"];":11208,"Windows":11209,"Ġsatellite":11210,"ASH":11211,"Ġinfant":11212,"description":11213,"ĠMeanwhile":11214,"cm":11215,"oca":11216,"ĠTreat":11217,"actor":11218,"Ġtobacco":11219,"ĠNorm":11220,"emption":11221,"Ġflesh":11222,"Ġje":11223,"oop":11224,"ĠHeaven":11225,"Ġbeating":11226,"anim":11227,"Ġgathering":11228,"Ġcultiv":11229,"GO":11230,"abe":11231,"ĠJonathan":11232,"ĠSafety":11233,"Ġbadly":11234,"prot":11235,"Ġchoosing":11236,"Ġcontacted":11237,"Ġquit":11238,"Ġdistur":11239,"Ġstir":11240,"Ġtoken":11241,"Det":11242,"ĠPa":11243,"Ġfunctionality":11244,"003":11245,"some":11246,"Ġlimitations":11247,"Ġmeth":11248,"build":11249,"config":11250,"NT":11251,"rell":11252,"blem":11253,"ĠMom":11254,"Ġveterans":11255,"ĠHu":11256,"Ġtrends":11257,"arer":11258,"ĠGiven":11259,"ĠCaption":11260,"may":11261,"AST":11262,"Ġwondering":11263,"ĠClark":11264,"normal":11265,"Ġseparated":11266,"Ġdesp":11267,"stic":11268,"brew":11269,"Ġrelating":11270,"ĠNik":11271,"ĠFarm":11272,"Ġenthusi":11273,"good":11274,"deb":11275,"Ġactivist":11276,"Ġmart":11277,"Ġexplosion":11278,"ĠEconomic":11279,"Link":11280,"Ġinsight":11281,"Ġconvenient":11282,"Ġcounterpart":11283,"support":11284,"ĠVirt":11285,"agen":11286,"ĠTennessee":11287,"ĠSimon":11288,"ĠAward":11289,"OCK":11290,"ĠFigure":11291,"Ġoverseas":11292,"Ġpride":11293,"ĠCas":11294,"note":11295,"mg":11296,"Current":11297,"Ġdisplays":11298,"content":11299,"Ġtraveling":11300,"Ġhospitals":11301,"ĠFinancial":11302,"ĠPast":11303,"Ġdefendant":11304,"Ġstreaming":11305,"mble":11306,"ĠBerlin":11307,"uki":11308,"Ġdistribut":11309,"Ġantib":11310,"Ġchocolate":11311,"ĠCastle":11312,"Ġinterrupt":11313,"ĠRow":11314,"Ġconversion":11315,"Ġbugs":11316,"ĠRather":11317,"liest":11318,"LY":11319,"ĠJean":11320,"common":11321,"akh":11322,"Ġ130":11323,"otton":11324,"ĠDean":11325,"Ġamendment":11326,"Ġgameplay":11327,"ĠWarren":11328,"oda":11329,"Ġhighlights":11330,"Ġirre":11331,"ĠNATO":11332,"Ġballs":11333,"Ġdemanding":11334,"URE":11335,"ĠLuke":11336,"Figure":11337,"stop":11338,"onia":11339,"zone":11340,"izers":11341,"ĠWR":11342,"Ġawarded":11343,"Ġregulatory":11344,"ĠHart":11345,"ĠSN":11346,"pling":11347,"Ġsour":11348,"ĠPixel":11349,"usive":11350,"Ġfet":11351,"ĠSent":11352,"Ġautomatic":11353,"Ġfer":11354,"vernment":11355,"ĠKhan":11356,"TON":11357,"father":11358,"Ġextraordinary":11359,"throp":11360,"ĠPython":11361,"ĠGPU":11362,"Ġsexually":11363,"Ġdesktop":11364,"itivity":11365,"ĠAntonio":11366,"Ġorient":11367,"Ġears":11368,"obby":11369,"ouses":11370,"vertisements":11371,"Ġmanufacturers":11372,"icient":11373,"minute":11374,"Ġconviction":11375,"Ġgarden":11376,"public":11377,"Ġsatisfied":11378,"fold":11379,"OK":11380,"Ġinhab":11381,"ĠThink":11382,"Ġprogramme":11383,"Ġstomach":11384,"Ġcoordin":11385,"Ġholy":11386,"Ġthreshold":11387,"Ġrhet":11388,"Ġserial":11389,"Ġemployers":11390,"ĠEverything":11391,"rah":11392,"Ġbother":11393,"Ġbrands":11394,"Value":11395,"ĠTed":11396,"ĠPlanet":11397,"Ġpink":11398,"ĠFurthermore":11399,"sa":11400,"PE":11401,"reck":11402,"ĠUSD":11403,"otte":11404,"Ġ&&":11405,"Ġlanded":11406,"gets":11407,"Ġproducers":11408,"Ġhealthcare":11409,"Ġdominant":11410,"Ġdestro":11411,"Ġamended":11412,"chron":11413,"Ġfits":11414,"ĠSyd":11415,"ĠAuthority":11416,"ATCH":11417,"Ġfights":11418,"ĠLLC":11419,"Ġ---":11420,"ĠCorp":11421,"Ġtoxic":11422,"specific":11423,"ĠCorn":11424,"ĠChel":11425,"Ġtelephone":11426,"ĠPant":11427,"Ġmysterious":11428,"aunch":11429,"odox":11430,"media":11431,"Ġwitnesses":11432,"agu":11433,"Ġquestioned":11434,"ĠBrexit":11435,"ĠRemember":11436,"enez":11437,"Ġendorse":11438,"iatric":11439,"ĠIdent":11440,"Ġridiculous":11441,"110":11442,"Ġprayer":11443,"Ġscientist":11444,"Ġ1950":11445,"ĠAqu":11446,"Ġunderground":11447,"ĠUFC":11448,"mare":11449,"ĠLater":11450,"wich":11451,"Ġsubscrib":11452,"Ġhosts":11453,"Ġerr":11454,"Ġgrants":11455,"antom":11456,"Ġsummon":11457,"early":11458,"ĠClear":11459,"ĠPrim":11460,"Ġsuspension":11461,"Ġguaranteed":11462,"apper":11463,"Ġrice":11464,"ĠSean":11465,"ĠShin":11466,"Ġreferendum":11467,"Ġfled":11468,"rust":11469,"Ġ360":11470,"tery":11471,"Ġshocked":11472,"BR":11473,"ĠOil":11474,"ĠAllah":11475,"Ġpartly":11476,"Ġignor":11477,"Ġtransmission":11478,"Ġhomosexual":11479,"iversal":11480,"Ġhopefully":11481,"ãĤ¤":11482,"Ġlesson":11483,"Leg":11484,"Ġ..":11485,"Yet":11486,"table":11487,"appropri":11488,"rett":11489,"Ġboards":11490,"Ġincorrect":11491,"Ġbacteria":11492,"aru":11493,"amac":11494,"Ġsnap":11495,".'\"":11496,"Ġparad":11497,"tem":11498,"heart":11499,"Ġavailability":11500,"Ġwisdom":11501,"Ġ(+":11502,"Ġpriest":11503,"ĠÂłĠÂł":11504,"Open":11505,"Ġspan":11506,"Ġparameter":11507,"Ġconvince":11508,"Ġ(%)":11509,"rac":11510,"Ġfo":11511,"Ġsafely":11512,"Ġconverted":11513,"ĠOlympic":11514,"Ġreserve":11515,"Ġhealing":11516,"ĠMine":11517,"Max":11518,"Ġinherent":11519,"ĠGraham":11520,"Ġintegrated":11521,"Dem":11522,"Ġpipeline":11523,"Ġapplying":11524,"Ġembed":11525,"ĠCharlie":11526,"Ġcave":11527,"2008":11528,"Ġconsensus":11529,"Ġrewards":11530,"Pal":11531,"ĠHTML":11532,"Ġpopularity":11533,"looking":11534,"ĠSword":11535,"ĠArts":11536,"')":11537,"Ġelectron":11538,"clusions":11539,"Ġintegrity":11540,"Ġexclusively":11541,"Ġgrace":11542,"Ġtorture":11543,"Ġburned":11544,"two":11545,"Ġ180":11546,"Produ":11547,"Ġentreprene":11548,"raphics":11549,"Ġgym":11550,"ricane":11551,"ĠTam":11552,"Ġadministrative":11553,"Ġmanufacturer":11554,"Ġvel":11555,"ĠNi":11556,"Ġisolated":11557,"ĠMedicine":11558,"Ġbackup":11559,"Ġpromoting":11560,"Ġcommander":11561,"Ġflee":11562,"ĠRussell":11563,"Ġforgotten":11564,"ĠMissouri":11565,"Ġresidence":11566,"mons":11567,"Ġresemb":11568,"Ġwand":11569,"Ġmeaningful":11570,"PT":11571,"Ġbol":11572,"Ġhelic":11573,"Ġwealthy":11574,"Ġrifle":11575,"strong":11576,"rowing":11577,"plan":11578,"asury":11579,"â̦.":11580,"Ġexpanding":11581,"ĠHamilton":11582,"Ġreceives":11583,"SI":11584,"eatures":11585,"ĠAnim":11586,"REE":11587,"Put":11588,"Ġbriefly":11589,"rive":11590,"Ġstimul":11591,"Ġ``(":11592,"Ġ__":11593,"Ġchip":11594,"Ġhaz":11595,"Ġprize":11596,"ĠThings":11597,"ACE":11598,"ulin":11599,"dict":11600,"oku":11601,"Ġassociate":11602,"ockets":11603,"youtube":11604,"Story":11605,"ategory":11606,"Ġmild":11607,"ailing":11608,"ĠYe":11609,"Orig":11610,"ĠKa":11611,"orig":11612,"Ġpropaganda":11613,"Ġanonymous":11614,"Ġstruggled":11615,"Ġoutrage":11616,"ATED":11617,"ĠBeijing":11618,"rary":11619,"Ġleather":11620,"Ġworlds":11621,"Ġbroader":11622,"125":11623,"idal":11624,"ĠBetter":11625,"Ġtear":11626,"Ext":11627,"Ġproposals":11628,"Ġiter":11629,"ĠSquad":11630,"Ġvolunt":11631,"mi":11632,"Did":11633,"ĠPu":11634,"pin":11635,"Ġspeakers":11636,"Ġborders":11637,"Ġfigured":11638,"='":11639,"Ġsimultaneously":11640,"aeda":11641,"Ġcharging":11642,"Ġurged":11643,"Ġconj":11644,"256":11645,"ĠGordon":11646,"merce":11647,"Ġdocumentary":11648,"Share":11649,"itol":11650,"ONE":11651,"ĠGarden":11652,"hatt":11653,"ĠThompson":11654,"aneous":11655,"apore":11656,"Ġtanks":11657,"Ġlessons":11658,"track":11659,"Ġoutstanding":11660,"Ġvolunteers":11661,"Ġspray":11662,"Ġmanagers":11663,"large":11664,"Ġcamps":11665,"Ġartificial":11666,"ĠRu":11667,"Ġbags":11668,"thal":11669,"Ġcompatible":11670,"ĠBlade":11671,"Ġfed":11672,"Ġargues":11673,"FI":11674,"Ġunfair":11675,"Ġcorn":11676,"Ġoffset":11677,"Ġdirections":11678,"Ġdisappointed":11679,"ĠConvention":11680,"Ġviewing":11681,"ME":11682,"ocity":11683,"Ġtowns":11684,"Ġlayers":11685,"Ġrolled":11686,"Ġjumped":11687,"Ġattribute":11688,"Ġunnecess":11689,"incoln":11690,"Ġsuppose":11691,"ĠNether":11692,"cha":11693,"Ġburied":11694,"Ġsixth":11695,"Ben":11696,"ressing":11697,"OUR":11698,"Ġwound":11699,"Ġcycl":11700,"Ġmechanisms":11701,"Ġcongressional":11702,"ĠElement":11703,"Ġagreements":11704,"Ġdecor":11705,"Ġclosest":11706,"ĠMit":11707,"Google":11708,"}}":11709,"Ġmixture":11710,"Ġfluid":11711,"Sign":11712,"ĠScholar":11713,"Ġpist":11714,"asket":11715,"abling":11716,"Ġracing":11717,"hero":11718,"riel":11719,"assy":11720,"Ġcheaper":11721,"ben":11722,"Ġvertical":11723,"amacare":11724,"ĠReading":11725,"gments":11726,"Ġhelicop":11727,"Ġsacrifice":11728,"aya":11729,"paren":11730,"VA":11731,"ĠLes":11732,"ĠStudio":11733,"Ġviolations":11734,"ĠAnna":11735,"acer":11736,"é¾":11737,"ĠRat":11738,"ĠBeck":11739,"ĠDick":11740,"ĠACT":11741,"Ġcomposition":11742,"Ġtexture":11743,"ĠOwn":11744,"Ġsmartphone":11745,"ĠNA":11746,"Ġforb":11747,"import":11748,"Ġdefending":11749,"ilst":11750,"rer":11751,"Ġoh":11752,"ĠJeremy":11753,"Ġbanking":11754,"ceptions":11755,"Ġrespective":11756,"/.":11757,"Ġdrinks":11758,"ĠWi":11759,"Ġbands":11760,"ĠLiverpool":11761,"Ġgrip":11762,"ĠBuy":11763,"Ġopenly":11764,"Ġreviewed":11765,"pert":11766,"Ġverify":11767,"ĠCole":11768,"ĠWales":11769,"MO":11770,"Ġunpre":11771,"Ġshelter":11772,"ĠImperial":11773,"Ġgui":11774,"ĠDak":11775,"Ġsuggestions":11776,"Ġexplicitly":11777,"Ġslave":11778,"Ġblockchain":11779,"Ġcompeting":11780,"Ġpromising":11781,"SON":11782,"Ġsoccer":11783,"Ġconstitution":11784,"429":11785,"Ġdistract":11786,"ĠUser":11787,"esides":11788,"ĠMethod":11789,"ĠTokyo":11790,"Ġaccompanied":11791,"Client":11792,"sur":11793,"alog":11794,"Ġidentification":11795,"Ġinvasion":11796,"asma":11797,"Ġindustries":11798,"ppers":11799,"Ġsubtle":11800,"ĠUnit":11801,"natural":11802,"Ġsurvived":11803,"Ġflaw":11804,"ĺħ":11805,"ĠHoll":11806,"Ġdeficit":11807,"Ġtutorial":11808,"ĠChance":11809,"Ġarguing":11810,"Ġcontemporary":11811,"Ġintegration":11812,"forward":11813,"Ġtum":11814,"itis":11815,"Ġhiding":11816,"ĠDomin":11817,"ĠTan":11818,"ĠBuilding":11819,"ĠVin":11820,"Ġspokesperson":11821,"ĠNotes":11822,"Ġemerging":11823,"Ġpreparation":11824,"Ġprost":11825,"Ġsuspects":11826,"Ġautonom":11827,"Description":11828,"Ġdealt":11829,"ĠPear":11830,"Ġsteady":11831,"Ġdecreased":11832,"Ġsovere":11833,"ĠClin":11834,"Ġgradually":11835,"orses":11836,"ĠWAR":11837,"Serv":11838,"ãĤ¢":11839,"hr":11840,"Ġdirty":11841,"ĠBarn":11842,"ĠBC":11843,"Ġdil":11844,"Ġcalendar":11845,"Ġcompliance":11846,"Ġchamber":11847,"bb":11848,"Ġpassenger":11849,"ateful":11850,"ĠTitle":11851,"ĠSydney":11852,"ĠGot":11853,"Ġdarkness":11854,"Ġdefect":11855,"Ġpacked":11856,"assion":11857,"Ġgods":11858,"Ġharsh":11859,"ICK":11860,"leans":11861,"Ġalgorithm":11862,"Ġoxygen":11863,"Ġvisits":11864,"Ġblade":11865,"Ġkilomet":11866,"ĠKentucky":11867,"Ġkiller":11868,"Pack":11869,"enny":11870,"Ġdivine":11871,"Ġnomination":11872,"being":11873,"Ġengines":11874,"Ġcats":11875,"Ġbuffer":11876,"ĠPhill":11877,"Ġtraff":11878,"AGE":11879,"Ġtongue":11880,"Ġradiation":11881,"erer":11882,"mem":11883,"ĠExplicit":11884,"é¾į":11885,"Ġcouples":11886,"Ġphysics":11887,"ĠMcK":11888,"Ġpolitically":11889,"awks":11890,"ĠBloom":11891,"Ġworship":11892,"eger":11893,"uter":11894,"ĠFO":11895,"Ġmathemat":11896,"Ġsentenced":11897,"Ġdisk":11898,"ĠMarg":11899,"Ġ/*":11900,"PI":11901,"Ġoptional":11902,"Ġbabies":11903,"Ġseeds":11904,"ĠScottish":11905,"Ġthy":11906,"]]":11907,"ĠHitler":11908,"PH":11909,"ngth":11910,"Ġrecovered":11911,"inge":11912,"Ġpowder":11913,"Ġlips":11914,"Ġdesigner":11915,"Ġdisorders":11916,"Ġcourage":11917,"Ġchaos":11918,"\"},{\"":11919,"Ġcarrier":11920,"bably":11921,"High":11922,"ĠRT":11923,"esity":11924,"len":11925,"Ġroutes":11926,"uating":11927,"Fil":11928,"NOT":11929,"wall":11930,"sburgh":11931,"Ġengaging":11932,"ĠJavaScript":11933,"orer":11934,"lihood":11935,"Ġunions":11936,"ĠFederation":11937,"ĠTesla":11938,"Ġcompletion":11939,"ĠTa":11940,"Ġprivilege":11941,"ĠOrange":11942,"Ġneur":11943,"parency":11944,"Ġbones":11945,"Ġtitled":11946,"Ġprosecutors":11947,"ĠME":11948,"Ġengineer":11949,"ĠUniverse":11950,"ĠHig":11951,"nie":11952,"oard":11953,"Ġhearts":11954,"ĠGre":11955,"ussion":11956,"Ġministry":11957,"Ġpenet":11958,"ĠNut":11959,"ĠOw":11960,"ĠXP":11961,"instein":11962,"Ġbulk":11963,"System":11964,"icism":11965,"ĠMarketable":11966,"Ġpreval":11967,"Ġposter":11968,"Ġattending":11969,"urable":11970,"Ġlicensed":11971,"ĠGh":11972,"etry":11973,"ĠTradable":11974,"Ġblast":11975,"à¤":11976,"ĠTitan":11977,"elled":11978,"die":11979,"Have":11980,"ĠFlame":11981,"Ġprofound":11982,"Ġparticipating":11983,"Ġanime":11984,"ĠEss":11985,"Ġspecify":11986,"Ġregarded":11987,"ĠSpell":11988,"Ġsons":11989,"owned":11990,"Ġmerc":11991,"Ġexperimental":11992,"lando":11993,"hs":11994,"ĠDungeon":11995,"inos":11996,"Ġcomply":11997,"ĠSystems":11998,"arth":11999,"Ġseized":12000,"local":12001,"ĠGirls":12002,"udo":12003,"oned":12004,"ĠFle":12005,"Ġconstructed":12006,"Ġhosted":12007,"Ġscared":12008,"actic":12009,"ĠIslands":12010,"ĠMORE":12011,"Ġbless":12012,"Ġblocking":12013,"Ġchips":12014,"Ġevac":12015,"Ps":12016,"Ġcorporation":12017,"Ġox":12018,"Ġlighting":12019,"Ġneighbors":12020,"ĠUb":12021,"aro":12022,"Ġbeef":12023,"ĠUber":12024,"Facebook":12025,"armed":12026,"itate":12027,"ĠRating":12028,"ĠQuick":12029,"Ġoccupied":12030,"Ġaims":12031,"ĠAdditionally":12032,"ĠInterest":12033,"Ġdramatically":12034,"Ġheal":12035,"Ġpainting":12036,"Ġengineers":12037,"MM":12038,"ĠMust":12039,"Ġquantity":12040,"Paul":12041,"Ġearnings":12042,"ĠPosts":12043,"stra":12044,"ãĥ¼ãĥ":12045,"Ġstance":12046,"Ġdropping":12047,"script":12048,"Ġdressed":12049,"Make":12050,"Ġjustify":12051,"ĠLtd":12052,"Ġprompted":12053,"Ġscrut":12054,"Ġspeeds":12055,"ĠGiants":12056,"omer":12057,"ĠEditor":12058,"Ġdescribing":12059,"ĠLie":12060,"mented":12061,"Ġnowhere":12062,"ocaly":12063,"Ġinstruction":12064,"fortable":12065,"Ġentities":12066,"Ġcm":12067,"ĠNatural":12068,"Ġinquiry":12069,"Ġpressed":12070,"izont":12071,"forced":12072,"Ġraises":12073,"ĠNetflix":12074,"ĠSide":12075,"Ġouter":12076,"Ġamongst":12077,"ims":12078,"owski":12079,"Ġclimb":12080,"never":12081,"Ġcombine":12082,"ding":12083,"Ġcompr":12084,"Ġsignificance":12085,"Ġremembered":12086,"ĠNevada":12087,"ĠTel":12088,"ĠScar":12089,"ĠWarriors":12090,"ĠJane":12091,"Ġcoup":12092,"bas":12093,"Ġterminal":12094,",-":12095,"OH":12096,"Ġtension":12097,"Ġwings":12098,"ĠMyster":12099,"����":12100,"ĠUnlike":12101,"valid":12102,"vironments":12103,"ĠAli":12104,"Ġnaked":12105,"books":12106,"ĠMun":12107,"ĠGulf":12108,"Ġdensity":12109,"Ġdimin":12110,"Ġdesperate":12111,"Ġpresidency":12112,"Ġ1986":12113,"hy":12114,"IND":12115,"Ġunlock":12116,"imens":12117,"Ġhandled":12118,"ĠEb":12119,"Ġdisappeared":12120,"Ġgenre":12121,"Ġ1988":12122,"Ġdetermination":12123,"Stream":12124,"iko":12125,"apters":12126,"Ġacknowledge":12127,"Jan":12128,"Ġcapitalism":12129,"Pat":12130,"Ġ2020":12131,"Ġpainful":12132,"Ġcurve":12133,"Ġbombs":12134,"storm":12135,"ĠMetal":12136,"encer":12137,"ĠFig":12138,"ĠAaron":12139,"anches":12140,"Ġinspiration":12141,"Ġexhaust":12142,"tains":12143,"ashi":12144,"Ġdescript":12145,"Ġritual":12146,"ĠChelsea":12147,"Ġpromotion":12148,"ĠHung":12149,"ĠWard":12150,"iva":12151,"ĠET":12152,"Ġtoss":12153,"allow":12154,"ĠFrancis":12155,"Dep":12156,"Ġhappiness":12157,"ĠGlass":12158,"Ġbeta":12159,"Ġstrengthen":12160,"NE":12161,"oa":12162,"Ġbuttons":12163,"ĠMurray":12164,"Ġkicked":12165,"Quest":12166,"ĠTalk":12167,"ĠSeveral":12168,"ĠZero":12169,"Ġdrone":12170,"ulk":12171,"Ġcam":12172,"ĠMobile":12173,"Ġpreventing":12174,"Ġretro":12175,"ĠAx":12176,"Ġcruel":12177,"Ġfloat":12178,".),":12179,"Ġfiling":12180,"ĠGrant":12181,"ĠBor":12182,"Ġrib":12183,"Ġchampionship":12184,"ĠMerc":12185,"Ġstyles":12186,"Ġcake":12187,"Ġbuilds":12188,"ĠSelf":12189,"iox":12190,"Ġepic":12191,"oyd":12192,"Bel":12193,"ĠStew":12194,".(":12195,"ahu":12196,"ĠBeyond":12197,"Ġouts":12198,"Ġsolo":12199,"ĠTree":12200,"Ġpreserve":12201,"Ġtub":12202,"ARE":12203,"roc":12204,"ĠImpro":12205,"ĠWright":12206,"Ġbund":12207,"Ġtraged":12208,"Ġoccasional":12209,"bian":12210,"Second":12211,"rons":12212,"Ġinteractions":12213,"formed":12214,"sing":12215,"Ġowns":12216,"Ġhockey":12217,"General":12218,"Ġlogical":12219,"Ġexpend":12220,"Ġescal":12221,"ĠGriff":12222,"ĠCrown":12223,"ĠReserve":12224,"Ġstopping":12225,"Ġexcuse":12226,"second":12227,"Ġoperated":12228,"Ġreaches":12229,"ĠMalays":12230,"Ġpollution":12231,"ĠBrooklyn":12232,"Ġdelete":12233,"Ġhash":12234,"Block":12235,"aha":12236,"â̳":12237,"Ġshorter":12238,"piece":12239,"></":12240,"Ġhorm":12241,"ĠWat":12242,"ĠBreak":12243,"Ġprohibited":12244,"Ġintensity":12245,"ĠAlan":12246,"Ġliability":12247,"?!":12248,"anded":12249,"Ġneighbour":12250,"ĠCollection":12251,"Ġfires":12252,"Ġrevolutionary":12253,"fly":12254,"ĠOrleans":12255,"White":12256,"ĠWrit":12257,"ĠDawn":12258,"Ġsettle":12259,"Ġexecute":12260,"BM":12261,"Ġspokeswoman":12262,"Ġlifestyle":12263,"Ġclicking":12264,"ĠKill":12265,"ĠLiberal":12266,"ĠNazi":12267,"Ġtrailer":12268,"Ġmountains":12269,"Ġdamn":12270,"zes":12271,"pes":12272,"Ġpressing":12273,"Ġbail":12274,"ĠOrganization":12275,"Ġpir":12276,"Ġthirty":12277,"Ġelectrical":12278,"Ġ115":12279,"ĠPoly":12280,"ĠRap":12281,"ĠStrike":12282,"ĠCann":12283,"Ġdemanded":12284,"Ġbacking":12285,"default":12286,"speed":12287,"ĠLegisl":12288,"Ġmothers":12289,"ĠBody":12290,"Ġvariation":12291,"cedented":12292,"powered":12293,"leading":12294,"Never":12295,"Ġgrave":12296,"ĠAnti":12297,"AW":12298,"Ġinterviewed":12299,"ĠGab":12300,"ĠFat":12301,"Ġrookie":12302,"uu":12303,"Ġdepos":12304,"ixon":12305,"Ġampl":12306,"retion":12307,"ĠHeat":12308,"Ġpeaceful":12309,"SM":12310,"ieve":12311,"Ġdiver":12312,"ĠVictoria":12313,"Ġmic":12314,"pdf":12315,"Ġstating":12316,"Ġlung":12317,"Ġcriticized":12318,"Ġvaccine":12319,"ĠLoading":12320,"urse":12321,"Take":12322,"ĠFran":12323,"ĠSold":12324,"ĠRobin":12325,"Ġdetected":12326,"ĠScript":12327,"Ġadjusted":12328,"Ġsenator":12329,"Ġopposing":12330,"Error":12331,"Count":12332,"Ġconflicts":12333,"Ġow":12334,"ĠArgent":12335,"Ġmatching":12336,"hh":12337,"ĠTrek":12338,"starter":12339,"\"),":12340,"ĠAF":12341,"oder":12342,"xxxx":12343,"ĠAlt":12344,"acre":12345,"ĠPick":12346,"ĠSolar":12347,"ĠDal":12348,"Oct":12349,"ĠBatt":12350,"Ġsrc":12351,"Ġengagement":12352,"Ġexecutives":12353,"Ġliberty":12354,"java":12355,"Ġtalented":12356,"igenous":12357,"Ġconsecut":12358,".....":12359,"Info":12360,"Ġhorrible":12361,"Ġsurprisingly":12362,"feed":12363,"icating":12364,"ĠLED":12365,"Ġfemales":12366,"Station":12367,"eller":12368,"ĠOakland":12369,"Ġmechanical":12370,"iology":12371,"ĠVar":12372,"Ġrobust":12373,"ettings":12374,"otta":12375,"Ġtheoret":12376,"Ġretain":12377,"kward":12378,"Ġda":12379,"Ġdeployed":12380,"del":12381,"ĠAndy":12382,"Ġsubscribe":12383,"web":12384,"Ġna":12385,"ĠMichel":12386,"Ġpartially":12387,"ĠComey":12388,"Ġcrown":12389,"ĠMaj":12390,"ĠBlu":12391,"rator":12392,"Day":12393,"INT":12394,"Ġdocumented":12395,"ĠGDP":12396,"gi":12397,"chell":12398,"Ġbrutal":12399,"ĠBab":12400,"stration":12401,"Ġtheft":12402,"Ġtube":12403,"@@":12404,"Ġquery":12405,"ĠLincoln":12406,"Ġpublishing":12407,"Ġwore":12408,"orical":12409,"Ġric":12410,"Ġnotable":12411,"Ġsubsequently":12412,"nex":12413,"Ġobserve":12414,"ĠBoe":12415,"Ġcodes":12416,"main":12417,"WH":12418,"ĠSL":12419,"Ġresidential":12420,"avan":12421,"Ġmas":12422,"arest":12423,"adeon":12424,"OUT":12425,"Ġsophistic":12426,"ante":12427,"Ġcens":12428,"Ġ**":12429,"Ġmortality":12430,"Ġyours":12431,"Ġoccasions":12432,"Ġrecalled":12433,"ĠDriver":12434,"Ġvocal":12435,"Ġbathroom":12436,"Ġshops":12437,"Ġcollaboration":12438,"ĠObamacare":12439,"ĠCell":12440,"Char":12441,"Super":12442,"Cre":12443,"Ġtends":12444,"Ġtorn":12445,"Ġeconomics":12446,"avery":12447,"ĠRaid":12448,"ĠSem":12449,"Ġshoulders":12450,"Ġexpecting":12451,"Ġexamination":12452,"ename":12453,"ĠUI":12454,"iability":12455,"olas":12456,"ĠAmb":12457,"ĠDra":12458,"Ġmidfield":12459,"ĠIC":12460,"Ġlayout":12461,"Ġfloating":12462,"fi":12463,"itative":12464,"Ġtremendous":12465,"ĠÐ":12466,"Ġabund":12467,"Work":12468,"ĠLightning":12469,"Ġsimilarly":12470,"Ġconservatives":12471,"Ġpray":12472,"BE":12473,"izarre":12474,"Ġtempt":12475,"Ġemphasis":12476,"ĠMetro":12477,"Ġfishing":12478,"Ġmarry":12479,"neg":12480,"ĠStudy":12481,"Ġreck":12482,"Ġdispos":12483,"oning":12484,"bsite":12485,"Ġsuspic":12486,"Ġmerch":12487,"ĠGib":12488,"ĠDescription":12489,"ĠDVD":12490,"whe":12491,"ĠYemen":12492,"Ġenvironments":12493,"ooting":12494,"ĠModern":12495,"eu":12496,"Ġreflects":12497,"Ġhoney":12498,"Ġanalyst":12499,"Ġgut":12500,"dec":12501,"Action":12502,"Ġhouseholds":12503,"Ġster":12504,"Ġtemple":12505,"Ġreforms":12506,"Ġfavourite":12507,"Ġdeadline":12508,"ĠLE":12509,"Three":12510,"ĠWithin":12511,"Aug":12512,"Ġnights":12513,"elta":12514,"Ġinvalid":12515,"ĠExchange":12516,"ĠDelhi":12517,"when":12518,"income":12519,"ĠðŁ":12520,"Ġwireless":12521,"scribe":12522,"ista":12523,"Ġhostile":12524,"Ġally":12525,"Ġgig":12526,"Ġoutlets":12527,"ĠDor":12528,"EMENT":12529,"Ġash":12530,"Ġabstract":12531,"ORD":12532,"ĠMotor":12533,"Ġadviser":12534,"istle":12535,"Ġbases":12536,"Ġcourtesy":12537,"Ġcrossing":12538,"Ġcleared":12539,"Ġrefugee":12540,"cosystem":12541,"Ġthrows":12542,"fun":12543,"bourne":12544,"days":12545,"Ġdisagree":12546,"ĠNative":12547,"Ġreflected":12548,"ĠFast":12549,"ĠYellow":12550,"ĠSingapore":12551,"ĠRaven":12552,"Ġembrace":12553,"ĠKu":12554,"ĠChen":12555,"ĠEarly":12556,"Ġappointment":12557,"ĠMini":12558,"itement":12559,"Ġplacing":12560,"Ġbicy":12561,"SR":12562,"Ġwhis":12563,"SU":12564,"Ġinvestigated":12565,"Ġphotographs":12566,"github":12567,"ĠBeat":12568,"ĠRing":12569,"ighed":12570,"iar":12571,"Ġevolved":12572,"erald":12573,"Ġdun":12574,"Ġhub":12575,"IAL":12576,"Ġencouraging":12577,"ĠPrint":12578,"ĠDays":12579,"Ġprosecution":12580,"Ġpants":12581,"azy":12582,"live":12583,"Ġfossil":12584,"ĠJu":12585,"Ġrocks":12586,"udge":12587,"ĠRace":12588,"Ġgreet":12589,"bie":12590,"Ġfilling":12591,"ĠLen":12592,"Ġdiabetes":12593,"Ġfirearms":12594,"uming":12595,"enezuel":12596,"ĠBB":12597,"Ġaccepting":12598,"ATH":12599,"Ġresort":12600,"Ġhunt":12601,"rik":12602,"ucker":12603,"aments":12604,"Ġsustained":12605,"Ġcrossed":12606,"Ġbreakfast":12607,"Ġattributes":12608,"lected":12609,"atile":12610,"Ġvibr":12611,"ĠKal":12612,"arson":12613,"oples":12614,"Ġtouched":12615,"Ġdamages":12616,"Ġimpressed":12617,"rup":12618,"Ġanch":12619,"ĠAdams":12620,"Hel":12621,"ĠVictor":12622,"Ġmounted":12623,"ĠCC":12624,"Ġdelicious":12625,"span":12626,"ella":12627,"Ġelabor":12628,"amples":12629,"Ġdefic":12630,"Ġconstitu":12631,"uates":12632,"ĠMission":12633,"ĠTher":12634,"ĠMonster":12635,"bes":12636,"Reuters":12637,"ĠIndones":12638,"hill":12639,"munition":12640,"Ġconfirmation":12641,"ĠConsider":12642,"acent":12643,"Ġjet":12644,"ĠEmploy":12645,"ĠGTX":12646,"nan":12647,"ĠSpider":12648,"Ġprocessor":12649,"Ġpatri":12650,"ĠPentagon":12651,"ĠRobinson":12652,"Ġrealistic":12653,"ñ":12654,"Ġappearing":12655,"Ġpipe":12656,"omed":12657,"Ġfru":12658,"Ġawful":12659,"Ġevaluation":12660,"Ġintelligent":12661,"ĠCitiz":12662,"Ġfundra":12663,"odium":12664,"Ġtweets":12665,"Ġworn":12666,"pring":12667,"Ġkidn":12668,"Ġrebels":12669,"ĠKam":12670,"ĠNetherlands":12671,"ĠSW":12672,"Ġacquisition":12673,"ĠMale":12674,"ãĥª":12675,"ombies":12676,"Ġtradem":12677,"ĠStatus":12678,"Bre":12679,"ĠTHIS":12680,"Ġadverse":12681,"ĠNEW":12682,"sign":12683,"Ġorganisation":12684,"enc":12685,"ĠHarper":12686,"apor":12687,"ĠMembers":12688,"ĠPeace":12689,"ĠAirport":12690,"ĠOthers":12691,"Ġscratch":12692,"ĠPil":12693,"Ġsensor":12694,"Ġadoption":12695,"ĠHotel":12696,"ĠDrag":12697,"Ġhonestly":12698,"Ġyard":12699,"ĠForces":12700,"Ġpatent":12701,"Ġbass":12702,"Ġquietly":12703,"Ġbreathing":12704,"Ġpose":12705,"iors":12706,"ĠJess":12707,"static":12708,"ITE":12709,"Offic":12710,"Ġjew":12711,"wcs":12712,"Ġ140":12713,"Ġpreview":12714,"ippi":12715,"Ġunfortunately":12716,"okemon":12717,"Ġhorn":12718,"Ġreass":12719,"Ġpeer":12720,"ocker":12721,"Ġunto":12722,"ĠGray":12723,"Ġcleaning":12724,"Ġattracted":12725,"2007":12726,"Point":12727,"kill":12728,"ĠAgreement":12729,"urches":12730,"Ġhorr":12731,"ĠMississ":12732,"Ġworthy":12733,"Ġflowers":12734,"town":12735,"dll":12736,"Ġreactions":12737,"Ġdece":12738,"Ġindicating":12739,"MD":12740,"Ġpreference":12741,"ĠMVP":12742,"essional":12743,"ĠTarget":12744,"gence":12745,"ĠIndians":12746,"Ġmisc":12747,"Ġfreely":12748,"Ġmuscles":12749,"Ġlineup":12750,"Ġimpacts":12751,"ousing":12752,"omi":12753,"acular":12754,"Ġcontrolling":12755,"agine":12756,"cery":12757,"hell":12758,"Ġranking":12759,"ĠNich":12760,"ĠAve":12761,"128":12762,"Ġhighway":12763,"Ġincons":12764,"Ġbinding":12765,"Ġstruggles":12766,"ĠPittsburgh":12767,"Ġgray":12768,"rin":12769,"Ġcomics":12770,"ĠSport":12771,"Ġrelatives":12772,"Ġfright":12773,"Ġprobe":12774,"ĠPortug":12775,"Ġvoc":12776,"Ġtu":12777,"ĠCorps":12778,"Ġpossibilities":12779,"Ġqualify":12780,"wcsstore":12781,"Ġlibraries":12782,"Ġmigrants":12783,"Ġentries":12784,"Ġconsecutive":12785,"vals":12786,"ĠChairman":12787,"Ġhill":12788,"IME":12789,"ĠGard":12790,"Ġinequality":12791,"fox":12792,"ĠSave":12793,"Ġcort":12794,"claimed":12795,"Ġtraits":12796,"Ġpour":12797,"Ġmissiles":12798,"Ġessence":12799,"Ġsends":12800,"Ġalliance":12801,"Ġwishes":12802,"ĠChristopher":12803,"Big":12804,"NY":12805,"ĠJacob":12806,"san":12807,"urred":12808,"ĠSO":12809,"lly":12810,"Ġadvocate":12811,"ĠBond":12812,"Ġ\"/":12813,"Using":12814,"Ġdistricts":12815,"ĠGate":12816,"ĠBir":12817,"ridge":12818,"ĠNaz":12819,"ĠRs":12820,"boards":12821,"ĠGa":12822,"ĠReagan":12823,"Ġinfluenced":12824,"1000":12825,"apy":12826,"Ġchallenged":12827,"Ġbarg":12828,"Ġfaculty":12829,"ĠFif":12830,"Ġacquire":12831,"Ac":12832,"Ġinsect":12833,"Ġinstruments":12834,"Ġleaf":12835,"thodox":12836,"Message":12837,"Ġtale":12838,"Ġthereby":12839,"Ġtrap":12840,"Ġstrongest":12841,"ĠMilitary":12842,"isible":12843,"Ġ1984":12844,"etheless":12845,"Ġflexible":12846,"Ġkills":12847,"Ġfinishing":12848,"ĠSize":12849,"Ġreduces":12850,"Ġepid":12851,"Ġorientation":12852,"full":12853,"Ġtrace":12854,"Ġlaser":12855,"Ġoppose":12856,"Ġediting":12857,"Ġmomentum":12858,"äº":12859,"show":12860,"VI":12861,"ĠLad":12862,"Ġ1985":12863,"Ġmurdered":12864,"900":12865,"uther":12866,"Ġprobability":12867,"ĠPoll":12868,"Ġreluct":12869,"ĠChem":12870,"ĠMontreal":12871,"Ġadequate":12872,"ĠPoland":12873,"ĠSheriff":12874,"umph":12875,"Ġok":12876,"Ġ000":12877,"Ġ\"[":12878,"Ġoperators":12879,"ĠFer":12880,"Ġmodes":12881,"ĠEve":12882,"Ġdiscipline":12883,"NET":12884,"Hand":12885,"Ġoral":12886,"ĠWE":12887,"email":12888,"JP":12889,"ĠPalestinians":12890,"Ġhence":12891,"ĠLess":12892,"Ġoverl":12893,"dig":12894,"Ġintimid":12895,"ĠCoal":12896,"Ġranging":12897,"tha":12898,"Ġdistant":12899,"Ġfib":12900,"ĠIndex":12901,"ĠWonder":12902,"ĠPel":12903,"hattan":12904,"ĠHug":12905,"ÃĹ":12906,"rait":12907,"Ġwrapped":12908,"ĠRPG":12909,"Ġchemicals":12910,"ĠMoney":12911,"Ġfrozen":12912,"Ġindirect":12913,"ĠAgainst":12914,"End":12915,"Ġuncomfortable":12916,"ĠGallery":12917,"ĠPosted":12918,"ا":12919,"onduct":12920,"Ġconsequence":12921,"Ġbitter":12922,"Ġ1987":12923,"pop":12924,"Ġcountless":12925,"ĠAlaska":12926,"ffff":12927,"Ġdeparture":12928,"Ġrefund":12929,"ĠIan":12930,"iated":12931,"Ġseeks":12932,"Ġmechanics":12933,"Ġjurisdiction":12934,"lynn":12935,"Ġalike":12936,"ĠHunt":12937,"athon":12938,"Ġresolved":12939,"Ġcache":12940,"Ġdistinction":12941,"direct":12942,"Ġencount":12943,"oub":12944,"beat":12945,"ĠCountry":12946,"search":12947,"Ġcontinuous":12948,"Ġmodest":12949,"ĠRail":12950,"thood":12951,"130":12952,"BUG":12953,"Ġcriminals":12954,"Ġindication":12955,"Ġencountered":12956,"last":12957,"ĠWy":12958,"Ġideology":12959,"ĠPDF":12960,"security":12961,"])":12962,"ĠJimmy":12963,"ĠEN":12964,"Ġhiring":12965,"Tem":12966,"Ġpig":12967,"aunt":12968,"ĠCrystal":12969,"Ġpenalties":12970,"Ġcapability":12971,"Ġpy":12972,"Ġproductive":12973,"Ġbalanced":12974,"ĠGeForce":12975,"click":12976,"olitan":12977,"ods":12978,"Ġafterwards":12979,"Ġplayoffs":12980,"ĠGill":12981,"User":12982,"Ġbacks":12983,"pub":12984,"tag":12985,"Ġabsurd":12986,"piring":12987,"Ġciting":12988,"Ġtrillion":12989,"Ġobligation":12990,"Ġmaxim":12991,"ahoo":12992,"cf":12993,"umi":12994,"ĠAlpha":12995,"ĠNelson":12996,"Ġpursuant":12997,"initely":12998,"Ġfract":12999,"entry":13000,"bery":13001,"ĠThor":13002,"Added":13003,"ĠDJ":13004,"ĠGene":13005,"Ġawkward":13006,"Stud":13007,"Ġwallet":13008,"ĠDivine":13009,"arios":13010,"Ġreleasing":13011,"Ġedited":13012,"Ġaccomplished":13013,"Best":13014,"Ġedges":13015,"Ġplanes":13016,"Ġfeeding":13017,"\"},\"":13018,"Ġdisclosure":13019,"Ġgrain":13020,"airy":13021,"oons":13022,"ernand":13023,"VR":13024,"Ġreasonably":13025,"Ġdrum":13026,"Ġpartial":13027,"Ġgraphic":13028,"Ġunprecedented":13029,"Ġadvised":13030,"Micro":13031,"ĠAssad":13032,"points":13033,"scar":13034,"ĠZone":13035,"ttes":13036,"Ġ700":13037,"vo":13038,"ĠHamp":13039,"Ġfixes":13040,"Ġcaution":13041,"Ġstrings":13042,"Ġpanels":13043,"Ġleak":13044,"Ġpricing":13045,"rowth":13046,"ĠError":13047,"ĠSaints":13048,"fix":13049,"Ġobservations":13050,"ĠAbs":13051,"Ġsuggestion":13052,"ĠUkrainian":13053,"Ġbarrier":13054,"Ġpainted":13055,"Bet":13056,"imir":13057,"ĠSpect":13058,"pot":13059,"orneys":13060,"Ġcompound":13061,"Ġbears":13062,"ĠRush":13063,"Ġluxury":13064,"Sum":13065,"Ġorbit":13066,"ĠMarc":13067,"Ġexempt":13068,"ĠTrail":13069,"ĠMO":13070,"ĠHans":13071,"ĠWeapon":13072,"ocused":13073,"uminum":13074,"ĠJerry":13075,"Ġbust":13076,"ĠAG":13077,"ĠWiki":13078,"Ġendless":13079,"ĠVlad":13080,"ĠBah":13081,"ĠRadeon":13082,"keys":13083,"ĠSurvey":13084,"ĠViol":13085,"define":13086,"lean":13087,"Ġcommod":13088,"Ġrevenues":13089,"Åį":13090,"Ġfurniture":13091,"Ġcasting":13092,"Ġdiplomatic":13093,"ĠPlayers":13094,"ĠKilled":13095,"Ġmodify":13096,"Ġinnovative":13097,"ĠAbu":13098,"nor":13099,"Ġbonds":13100,"Ġcoaching":13101,"Mer":13102,"Ġmodules":13103,"ĠPatriots":13104,"Ġenhanced":13105,"Ġproceedings":13106,"Ġteammates":13107,"Ġ128":13108,"ardo":13109,"Ġcompromise":13110,"ĠMuch":13111,"Ġflew":13112,"ĠEdge":13113,"Ġunnecessary":13114,"Ġdoctrine":13115,"report":13116,"ĠOrlando":13117,"ĠProfile":13118,"Ġplayoff":13119,"friendly":13120,"Ġcomplain":13121,"ĠMC":13122,"ĠOpt":13123,"ĠGB":13124,"Ġbeaten":13125,"Ġgolf":13126,"Ġplacement":13127,"Bit":13128,"Ġnewsletter":13129,"Ġ2019":13130,"visor":13131,"rawl":13132,"ĠiPad":13133,"Ġacted":13134,"Ġjuice":13135,"Ġdecks":13136,"PN":13137,"success":13138,"ĠHalf":13139,"Ġdeleted":13140,"Ġsecrets":13141,"Ġasylum":13142,"Mart":13143,"ĠActiv":13144,"ĠGuy":13145,"ĠTs":13146,"Ġdys":13147,"Ġassuming":13148,"Ġmana":13149,"Ġsubur":13150,"Ġ125":13151,"Media":13152,"ARY":13153,"ride":13154,"cp":13155,"Ġdifficulties":13156,"Ġcollecting":13157,"Ġbankrupt":13158,"non":13159,"Ġcomposed":13160,"Ġvolt":13161,"Ġmilitants":13162,"Ġ>>>":13163,"ĠMormon":13164,"tor":13165,"Ġparticles":13166,"ĠBart":13167,"ryption":13168,"Ġadmin":13169,"Ġsquee":13170,"VIDIA":13171,"Ġcreator":13172,"iameter":13173,"icular":13174,"NBC":13175,"Ġgrabbed":13176,"Ġnodd":13177,"Ġrated":13178,"Ġrotation":13179,"Ġgrasp":13180,"Ġexcessive":13181,"ĠEC":13182,"ĠWhit":13183,"Ġinventory":13184,"aults":13185,"ĠFB":13186,"Ġecosystem":13187,"Ġbillions":13188,"Ġventure":13189,"named":13190,"Ġdefender":13191,"oute":13192,"Instead":13193,"irable":13194,"War":13195,"Ġassumption":13196,"Ġbite":13197,"Ġearthqu":13198,"tail":13199,"space":13200,"Ġgifts":13201,"boys":13202,"Ġinevitable":13203,"Ġstructural":13204,"Ġbeneficial":13205,"Ġcompelling":13206,"hole":13207,"ervation":13208,"Ġcoat":13209,"oj":13210,"incarn":13211,"ĠYears":13212,"Ġdetermining":13213,"Ġrhetoric":13214,"Ġboundaries":13215,"Ġwhites":13216,"Ant":13217,"addy":13218,")-":13219,"raham":13220,"etermin":13221,"Ġharvest":13222,"ĠConc":13223,"Ġlaptop":13224,"ĠMatch":13225,"Ġenjoying":13226,"cca":13227,"ollar":13228,"Ġtrips":13229,"Ġaddiction":13230,"ĠSak":13231,"Ġpowered":13232,"Ġcous":13233,"ĠRussians":13234,"iere":13235,"Ġretrie":13236,"quality":13237,"Ġdiffer":13238,"Ġkingdom":13239,"ĠLaur":13240,"ĠCapitol":13241,"Ġconclusions":13242,"ĠAltern":13243,"ĠNav":13244,"Ġtransparent":13245,"BER":13246,"Group":13247,"ĠComplete":13248,"Ġinfer":13249,"Ġintrig":13250,"Ġinsane":13251,"RO":13252,"ophob":13253,"isen":13254,"qual":13255,"Michael":13256,"Ġmuseum":13257,"ĠPope":13258,"Ġreset":13259,"rative":13260,"five":13261,"Ġaggreg":13262,"ittees":13263,"ository":13264,"Ġcarb":13265,"ĠRecord":13266,"Ġdecides":13267,"ĠFix":13268,"Ġexceptions":13269,"ĠCommissioner":13270,"uns":13271,"ĠEnvironmental":13272,"Ġlegendary":13273,"istence":13274,"Ġtunnel":13275,"km":13276,"Ġinsult":13277,"Ġtroll":13278,"Ġshake":13279,"Ġdetention":13280,"ques":13281,"ĠChrome":13282,"ĠFiles":13283,"Ġsubt":13284,"Ġprospects":13285,"Ġprol":13286,"render":13287,"proof":13288,"Ġperformances":13289,"Str":13290,"Ġhref":13291,"ername":13292,"Ġachievement":13293,"Ġfut":13294,"Full":13295,"ĠLeban":13296,"google":13297,"ãĥĪ":13298,"ampa":13299,"Maybe":13300,"Ġprojected":13301,"ĠEmb":13302,"Ġcolleg":13303,"Ġawards":13304,"ĠâĶ":13305,"Gold":13306,"ĠBlake":13307,"ĠRaj":13308,"ifting":13309,"Ġpending":13310,"Ġinstinct":13311,"Ġdevelopments":13312,"Connect":13313,"ĠMand":13314,"ĠWITH":13315,"ĠPhilippines":13316,"profile":13317,"Ġaltogether":13318,"ĠBund":13319,"ĠTD":13320,"oooo":13321,"amped":13322,"iph":13323,"Ġsteam":13324,"Ġoldest":13325,"Ġdetection":13326,"ulpt":13327,"Ġç":13328,"ĠWayne":13329,"2006":13330,"fa":13331,"Ġcircles":13332,"ĠFu":13333,"Ġdonors":13334,"appropriate":13335,"ĠDakota":13336,"jamin":13337,"Ġmotivated":13338,"Ġpurchases":13339,"ĠLouisiana":13340,"ĠSpl":13341,"Ġglobe":13342,"Ġ105":13343,"zip":13344,"call":13345,"Ġdepartments":13346,"Ġsustainable":13347,"105":13348,"ĠOP":13349,"ifiers":13350,"Ġprevented":13351,"Ġincomp":13352,"ĠCommander":13353,"Ġdominated":13354,"Ġ»":13355,"Ġinvested":13356,"Ġcomplexity":13357,"Ġincl":13358,"Ġensuring":13359,"Ġrealm":13360,"ync":13361,"ĠIndependent":13362,"rained":13363,"ĠJen":13364,"ĠFlight":13365,"Ġathe":13366,"Ġspeculation":13367,"ĠTE":13368,"ocate":13369,"tic":13370,"Ġplaint":13371,"herry":13372,"Ġtoy":13373,"Ġ111":13374,"Ġplates":13375,"status":13376,"ĠIsa":13377,"Ġdevoted":13378,"Cop":13379,"ĠES":13380,"255":13381,"urrency":13382,"Main":13383,"Ġslaves":13384,"Ġpepper":13385,"Ġquotes":13386,"Ġceiling":13387,"ĠFish":13388,"Ġtransformation":13389,"Ġfraction":13390,"Ġadvantages":13391,"Ġtoile":13392,"Ġstunning":13393,"Ġmoist":13394,"breaking":13395,"si":13396,"ĠLocation":13397,"ĠMedium":13398,"Ġtexts":13399,"Ġugly":13400,"Ġbio":13401,".âĢĶ":13402,"ĠBased":13403,"Ġtrains":13404,"ĠWing":13405,"ĠAncient":13406,"ĠRecords":13407,"ĠHope":13408,"Special":13409,"adesh":13410,"obi":13411,"[/":13412,"Ġtemporarily":13413,"Ver":13414,"hu":13415,"oser":13416,"Ġovernight":13417,"Ġmamm":13418,"ĠTreasury":13419,"ĠVenezuel":13420,"ĠMega":13421,"Ġtar":13422,"Ġexpects":13423,"black":13424,"orph":13425,"\\\\\\\\":13426,"Ġacceptance":13427,"Ġradar":13428,"sis":13429,"Ġjunior":13430,"Ġframes":13431,"Ġobservation":13432,"acies":13433,"Power":13434,"ĠAdvanced":13435,"Mag":13436,"ologically":13437,"ĠMechan":13438,"Ġsentences":13439,"Ġanalysts":13440,"aughters":13441,"forcement":13442,"Ġvague":13443,"Ġclause":13444,"Ġdirectors":13445,"Ġevaluate":13446,"Ġcabinet":13447,"Matt":13448,"ĠClassic":13449,"Ang":13450,"Ġcler":13451,"ĠBuck":13452,"Ġresearcher":13453,"Ġ160":13454,"Ġpoorly":13455,"Ġexperiencing":13456,"ĠPed":13457,"ĠManhattan":13458,"Ġfreed":13459,"Ġthemes":13460,"advant":13461,"Ġnin":13462,"Ġpraise":13463,"104":13464,"ĠLibya":13465,"best":13466,"Ġtrusted":13467,"Ġcease":13468,"Ġdign":13469,"Direct":13470,"Ġbombing":13471,"Ġmigration":13472,"ĠSciences":13473,"Ġmunicipal":13474,"ĠAverage":13475,"Ġglory":13476,"Ġrevealing":13477,"Ġarena":13478,"Ġuncertainty":13479,"Ġbattlefield":13480,"iao":13481,"God":13482,"Ġcinem":13483,"rape":13484,"elle":13485,"apons":13486,"Ġlisting":13487,"Ġwaited":13488,"Ġspotted":13489,"keley":13490,"ĠAudio":13491,"eor":13492,"arding":13493,"idding":13494,"igma":13495,"ĠNeg":13496,"Ġlone":13497,"Ġ----":13498,"exe":13499,"deg":13500,"Ġtransf":13501,"Ġwash":13502,"Ġslavery":13503,"Ġexploring":13504,"ĠWW":13505,"atson":13506,"Ġencl":13507,"lies":13508,"ĠCreek":13509,"Ġwooden":13510,"Manager":13511,"ĠBrand":13512,"ummy":13513,"ĠArthur":13514,"Ġbureaucr":13515,"Ġblend":13516,"arians":13517,"Further":13518,"Ġsupposedly":13519,"Ġwinds":13520,"Ġ1979":13521,"Ġgravity":13522,"Ġanalyses":13523,"ĠTravel":13524,"ĠVeter":13525,"Ġdumb":13526,"Ġalternate":13527,"gal":13528,"Ġconsumed":13529,"Ġeffectiveness":13530,".''":13531,"Ġpaths":13532,"onda":13533,"LA":13534,"ĠStrong":13535,"Ġenables":13536,"Ġescaped":13537,"Ġ\"\"":13538,"Ġ112":13539,"Ġ1983":13540,"Ġsmiled":13541,"Ġtendency":13542,"Fire":13543,"Ġpars":13544,"ĠRoc":13545,"Ġlake":13546,"Ġfitness":13547,"ĠAth":13548,"ĠHorn":13549,"Ġhier":13550,"Ġimpose":13551,"mother":13552,"Ġpension":13553,"icut":13554,"borne":13555,"iciary":13556,"._":13557,"ĠSU":13558,"Ġpolar":13559,"isy":13560,"engu":13561,"itialized":13562,"ATA":13563,"write":13564,"Ġexercises":13565,"ĠDiamond":13566,"otypes":13567,"Ġharmful":13568,"onz":13569,"Ġprinting":13570,"story":13571,"Ġexpertise":13572,"ĠGer":13573,"Ġtragedy":13574,"ĠFly":13575,"Ġdivid":13576,"ampire":13577,"stock":13578,"Mem":13579,"Ġreign":13580,"Ġunve":13581,"Ġamend":13582,"ĠProphet":13583,"Ġmutual":13584,"ĠFac":13585,"Ġreplacing":13586,"Har":13587,"ĠCircuit":13588,"Ġthroat":13589,"ĠShot":13590,"Ġbatteries":13591,"Ġtoll":13592,"Ġaddressing":13593,"ĠMedicaid":13594,"Ġpupp":13595,"ĠNar":13596,"olk":13597,"Ġequity":13598,"MR":13599,"ĠHispan":13600,"ĠLarge":13601,"mid":13602,"Dev":13603,"Ġexped":13604,"Ġdemo":13605,"ĠMarshall":13606,"ergus":13607,"Ġfiber":13608,"Ġdivorce":13609,"ĠCreate":13610,"Ġslower":13611,"ĠParker":13612,"ĠStudent":13613,"ĠTraining":13614,"Return":13615,"ĠTru":13616,"Ġcub":13617,"ĠReached":13618,"Ġpanic":13619,"Ġquarters":13620,"Ġrect":13621,"Ġtreating":13622,"Ġrats":13623,"ĠChristianity":13624,"oler":13625,"Ġsacred":13626,"Ġdeclare":13627,"ulative":13628,"eting":13629,"Ġdelivering":13630,"estone":13631,"Ġtel":13632,"ĠLarry":13633,"Ġmeta":13634,"accept":13635,"artz":13636,"ĠRoger":13637,"handed":13638,"Ġheader":13639,"Ġtrapped":13640,"ĠCentury":13641,"Ġknocked":13642,"ĠOxford":13643,"Ġsurvivors":13644,"bot":13645,"Ġdemonstration":13646,"Ġdirt":13647,"Ġassists":13648,"OME":13649,"ĠDraft":13650,"ortunate":13651,"folio":13652,"pered":13653,"usters":13654,"gt":13655,"ĠLock":13656,"Ġjudicial":13657,"verted":13658,"Ġsecured":13659,"outing":13660,"ĠBooks":13661,"Ġhosting":13662,"Ġlifted":13663,"length":13664,"Ġjer":13665,"Ġwheels":13666,"ĠRange":13667,"umbnails":13668,"Ġdiagnosis":13669,"tech":13670,"ĠStewart":13671,"ĠPract":13672,"Ġnationwide":13673,"Ġdear":13674,"Ġobligations":13675,"Ġgrows":13676,"Ġmandatory":13677,"Ġsuspicious":13678,"!'":13679,"Apr":13680,"Great":13681,"Ġmortgage":13682,"Ġprosecutor":13683,"Ġeditorial":13684,"ĠKr":13685,"Ġprocessed":13686,"ungle":13687,"Ġflexibility":13688,"Earlier":13689,"ĠCart":13690,"ĠSug":13691,"Ġfocuses":13692,"Ġstartup":13693,"Ġbreach":13694,"ĠTob":13695,"cycle":13696,"ãĢĮ":13697,"rose":13698,"Ġbizarre":13699,"ãĢį":13700,"Ġvegetables":13701,"$$":13702,"Ġretreat":13703,"oshi":13704,"ĠShop":13705,"ĠGround":13706,"ĠStop":13707,"ĠHawaii":13708,"ĠAy":13709,"Perhaps":13710,"ĠBeaut":13711,"uffer":13712,"enna":13713,"Ġproductivity":13714,"Fixed":13715,"control":13716,"Ġabsent":13717,"ĠCampaign":13718,"Green":13719,"Ġidentifying":13720,"Ġregret":13721,"Ġpromoted":13722,"ĠSeven":13723,"Ġeru":13724,"neath":13725,"aughed":13726,"ĠPin":13727,"ĠLiving":13728,"Cost":13729,"omatic":13730,"mega":13731,"ĠNig":13732,"ocy":13733,"Ġinbox":13734,"Ġempire":13735,"Ġhorizont":13736,"Ġbranches":13737,"Ġmetaph":13738,"Active":13739,"edi":13740,"ĠFilm":13741,"ĠSomething":13742,"Ġmods":13743,"incial":13744,"ĠOriginal":13745,"Gen":13746,"Ġspirits":13747,"Ġearning":13748,"Hist":13749,"Ġriders":13750,"Ġsacrific":13751,"MT":13752,"ĠVA":13753,"ĠSalt":13754,"Ġoccupation":13755,"ĠMi":13756,"Ġdisg":13757,"lict":13758,"Ġnit":13759,"Ġnodes":13760,"eem":13761,"ĠPier":13762,"Ġhatred":13763,"psy":13764,"ãĥī":13765,"Ġtheater":13766,"Ġsophisticated":13767,"Ġdefended":13768,"Ġbesides":13769,"Ġthoroughly":13770,"ĠMedicare":13771,"Ġblamed":13772,"arently":13773,"Ġcrying":13774,"FOR":13775,"priv":13776,"Ġsinging":13777,"ĠIl":13778,"Ġcute":13779,"oided":13780,"olitical":13781,"ĠNeuro":13782,"å¤":13783,"Ġdonation":13784,"ĠEagles":13785,"ĠGive":13786,"Tom":13787,"Ġsubstantially":13788,"ĠLicense":13789,"ĠJa":13790,"Ġgrey":13791,"ĠAnimal":13792,"ĠER":13793,"ĠUnd":13794,"Ġkeen":13795,"Ġconclude":13796,"ĠMississippi":13797,"Engine":13798,"ĠStudios":13799,"Press":13800,"overs":13801,"llers":13802,"Ġ350":13803,"ĠRangers":13804,"Ġrou":13805,"erto":13806,"Ep":13807,"issa":13808,"ivan":13809,"Ġseal":13810,"ĠRegist":13811,"display":13812,"Ġweaken":13813,"uum":13814,"ĠCommons":13815,"ĠSay":13816,"Ġcultures":13817,"Ġlaughed":13818,"Ġslip":13819,"Ġtreatments":13820,"izable":13821,"mart":13822,"ĠRice":13823,"Ġbeast":13824,"Ġobesity":13825,"ĠLaure":13826,"iga":13827,"Which":13828,"holder":13829,"Ġelderly":13830,"Ġpays":13831,"Ġcomplained":13832,"Ġcrop":13833,"Ġproc":13834,"Ġexplosive":13835,"ĠFan":13836,"ĠArsenal":13837,"Author":13838,"eful":13839,"Ġmeals":13840,"Ġ(-":13841,"idays":13842,"Ġimagination":13843,"Ġannually":13844,"Ġms":13845,"asures":13846,"Head":13847,"ikh":13848,"matic":13849,"Ġboyfriend":13850,"ĠComputer":13851,"Ġbump":13852,"Ġsurge":13853,"ĠCraig":13854,"ĠKirk":13855,"Del":13856,"mediate":13857,"Ġscenarios":13858,"ĠMut":13859,"ĠStream":13860,"Ġcompetitors":13861,"ÙĦ":13862,"ĠStanford":13863,"ĠResources":13864,"azed":13865,"bage":13866,"Ġorganis":13867,"ĠRelease":13868,"Ġseparately":13869,"Ġhabits":13870,"Ġmeasurements":13871,"ĠClose":13872,"Ġaccompany":13873,"Ġgly":13874,"Ġtang":13875,"ĠRou":13876,"Ġplugin":13877,"Ġconvey":13878,"ĠChallenge":13879,"oots":13880,"jan":13881,"Ġcurs":13882,"ĠRelations":13883,"keeper":13884,"Ġapproaching":13885,"ping":13886,"Speaking":13887,"Ġarrangement":13888,"ĠVI":13889,"arettes":13890,"Ġaffecting":13891,"Ġpermits":13892,"because":13893,"Ġuseless":13894,"ĠHus":13895,"!!!!":13896,"Ġdestroying":13897,"Unfortunately":13898,"Ġfascinating":13899,"Sem":13900,"Ġelectoral":13901,"Ġtransparency":13902,"ĠChaos":13903,"Ġvolunteer":13904,"Ġstatistical":13905,"Ġactivated":13906,"rox":13907,"Web":13908,"HE":13909,"ĠHampshire":13910,"isive":13911,"Map":13912,"Ġtrash":13913,"ĠLawrence":13914,"stick":13915,"Cr":13916,"Ġrings":13917,"EXT":13918,"Ġoperational":13919,"opes":13920,"Does":13921,"ĠEvans":13922,"Ġwitnessed":13923,"Port":13924,"Ġlaunching":13925,"econom":13926,"wear":13927,"ĠParticip":13928,"umm":13929,"cules":13930,"ĠRAM":13931,"ĠTun":13932,"Ġassured":13933,"Ġbinary":13934,"Ġbetray":13935,"Ġexploration":13936,"ĠFel":13937,"Ġadmission":13938,"itated":13939,"Sy":13940,"Ġavoided":13941,"ĠSimulator":13942,"Ġcelebrated":13943,"ĠElectric":13944,"¥ŀ":13945,"Ġcluster":13946,"itzerland":13947,"health":13948,"Line":13949,"ĠNash":13950,"aton":13951,"Ġspare":13952,"Ġenterprise":13953,"ĠDIS":13954,"cludes":13955,"Ġflights":13956,"Ġregards":13957,"ĠÃĹ":13958,"half":13959,"Ġtrucks":13960,"Ġcontacts":13961,"Ġuncons":13962,"ĠClimate":13963,"Ġimmense":13964,"NEW":13965,"occ":13966,"ective":13967,"Ġembod":13968,"Ġpatrol":13969,"Ġbeside":13970,"Ġviable":13971,"Ġcreep":13972,"Ġtriggered":13973,"verning":13974,"Ġcomparable":13975,"ql":13976,"Ġgaining":13977,"asses":13978,"Ġ();":13979,"ĠGrey":13980,"ĠMLS":13981,"sized":13982,"Ġprosper":13983,"\"?":13984,"Ġpolling":13985,"Ġshar":13986,"ĠRC":13987,"Ġfirearm":13988,"orient":13989,"Ġfence":13990,"Ġvariations":13991,"giving":13992,"ĠPi":13993,"ospel":13994,"Ġpledge":13995,"Ġcure":13996,"Ġspy":13997,"Ġviolated":13998,"Ġrushed":13999,"Ġstroke":14000,"ĠBlog":14001,"sels":14002,"ĠEc":14003,",''":14004,"Ġpale":14005,"ĠCollins":14006,"terror":14007,"ĠCanadians":14008,"Ġtune":14009,"Ġlaboratory":14010,"Ġnons":14011,"tarian":14012,"Ġdisability":14013,"ĠGam":14014,"Ġsinger":14015,"alg":14016,"ĠSenior":14017,"Ġtraded":14018,"ĠWarrior":14019,"Ġinfring":14020,"ĠFranklin":14021,"Ġstrain":14022,"ĠSwedish":14023,"Ġseventh":14024,"ĠBenn":14025,"ĠTell":14026,"Ġsyndrome":14027,"Ġwondered":14028,"iden":14029,"++++":14030,"igo":14031,"Ġpurple":14032,"Ġjournalism":14033,"Ġrebel":14034,"Ġfu":14035,"blog":14036,"Ġinvite":14037,"rencies":14038,"ĠContact":14039,"Israel":14040,"ĠContent":14041,"Ġcheer":14042,"Ġbedroom":14043,"ĠEngineering":14044,"ĠQueens":14045,"Ġdwell":14046,"ĠPlayStation":14047,"ĠDim":14048,"ĠColon":14049,"lr":14050,"Ġoperates":14051,"Ġmotivation":14052,"USA":14053,"astered":14054,"Core":14055,"ĠTruth":14056,"olo":14057,"OSE":14058,"ĠMemory":14059,"Ġpredec":14060,"Ġanarch":14061,"Ġ1920":14062,"ĠYam":14063,"è":14064,"bid":14065,"Ġgrateful":14066,"Ġexcitement":14067,"Ġtreasure":14068,"Ġlongest":14069,"ctive":14070,"Ġdeserves":14071,"Ġreserves":14072,"Ġcops":14073,"ĠOttawa":14074,"ĠEgyptian":14075,"anked":14076,"Ġartif":14077,"Ġhypothesis":14078,":/":14079,"Ġpurchasing":14080,"Ġlovely":14081,"HP":14082,"Ġdivide":14083,"Ġstrictly":14084,"Ġquestioning":14085,"Ġtaxpayers":14086,"ĠJoy":14087,"Ġrolls":14088,"ĠHeavy":14089,"Ġports":14090,"Ġmagnetic":14091,"Ġinflamm":14092,"Ġbrush":14093,"tics":14094,"âĪĴ":14095,"Ġbottles":14096,"ppy":14097,"Ġpadd":14098,"ãĤ¯":14099,"million":14100,"Ġdevastating":14101,"Ġcompiled":14102,"Ġmedication":14103,"Ġtwelve":14104,"ĠPerry":14105,"Space":14106,"imb":14107,"your":14108,"Ġleaked":14109,"ĠTar":14110,"Ġunity":14111,"Ġinfected":14112,"Ġtraveled":14113,"IDE":14114,"ĠMcDonald":14115,"txt":14116,"ĠPrinc":14117,"Ġinterven":14118,"ĠTaiwan":14119,"ĠPow":14120,"Ġbearing":14121,"ĠThread":14122,"Ġzones":14123,"izards":14124,"unks":14125,"Chapter":14126,"llor":14127,"Ġ·":14128,"Ġwounds":14129,"Ġdiscretion":14130,"Ġsucceeded":14131,"iking":14132,"Ġiconic":14133,"Call":14134,"Ġscreening":14135,"ĠMis":14136,"icts":14137,"Ġministers":14138,"Ġseparation":14139,"Player":14140,"Ġbip":14141,"Ġbeloved":14142,"Ġcounting":14143,"ĠEye":14144,"around":14145,"inging":14146,"Ġtablet":14147,"Ġoffence":14148,"inance":14149,"have":14150,"ĠInfo":14151,"ĠNinja":14152,"Ġprotective":14153,"ĠCass":14154,"Mac":14155,"ĠQuality":14156,"North":14157,"Ġic":14158,"ĠCuba":14159,"ĠChronicle":14160,"ĠProperty":14161,"Ġfastest":14162,"otos":14163,"ĠGerm":14164,"OWN":14165,"Ġboom":14166,"ĠStanley":14167,"erguson":14168,"Ġclever":14169,"Ġenters":14170,"mode":14171,"terior":14172,"ĠSens":14173,"Ġlinear":14174,"ARK":14175,"Ġcomparing":14176,"Ġpurely":14177,"Ġsafer":14178,"ĠPotter":14179,"Ġcups":14180,"RT":14181,"Ġgluc":14182,"Ġattributed":14183,"Ġdupl":14184,"ĠPap":14185,"Ġprecious":14186,"Ġpa":14187,"ictionary":14188,"ĠTig":14189,"ĠToo":14190,"olutions":14191,"stan":14192,"Ġrobots":14193,"Ġlobb":14194,"Ġstatute":14195,"Ġprevention":14196,"western":14197,"160":14198,"ĠActive":14199,"ĠMaria":14200,"hal":14201,"None":14202,"ellar":14203,"ĠKB":14204,"ĠPartners":14205,"ĠSingle":14206,"ĠFollowing":14207,"ango":14208,"acious":14209,"Ġthou":14210,"Ġkg":14211,"Ġinfluential":14212,"ĠFriends":14213,"Sur":14214,"ainted":14215,"Ġforums":14216,"Ġstarter":14217,"Ġcitizenship":14218,"ĠElection":14219,"onge":14220,"otation":14221,"osph":14222,";;;;":14223,"utical":14224,"pur":14225,"eren":14226,"Ġaccusations":14227,"bitious":14228,"abbit":14229,"ĠOrd":14230,"Posted":14231,"irk":14232,"Ġsensitivity":14233,"iche":14234,"ĠAmy":14235,"ĠFab":14236,"Ġsummit":14237,"Ġpedest":14238,"Ġrubber":14239,"Ġagricultural":14240,"Ġcancel":14241,"AE":14242,"Ġinaug":14243,"Ġcontam":14244,"Ġfirmly":14245,"iw":14246,"stage":14247,"ĠKan":14248,"Ġtier":14249,"Ġinvention":14250,"Ġtranslated":14251,"ĠRules":14252,"Box":14253,"Twitter":14254,"IDS":14255,"Ġpizza":14256,"Ġdebug":14257,"ĠDrop":14258,"vs":14259,"Ġhorses":14260,"big":14261,"Ġboring":14262,"Ġhood":14263,"ĠMcCain":14264,"atched":14265,"ĠBros":14266,"Ġskip":14267,"Ġessay":14268,"stat":14269,"ĠLegends":14270,"Ġammunition":14271,"auc":14272,"Ġshooter":14273,"Ġunh":14274,"Ġsupplied":14275,"Ġgeneric":14276,"ĠSK":14277,"iban":14278,"yrics":14279,"Ġ255":14280,"Ġclimbing":14281,"Former":14282,"Ġflip":14283,"Ġjumping":14284,"Ġfrustration":14285,"ĠTerry":14286,"Ġneighborhoods":14287,"Ġmedian":14288,"bean":14289,"Ġbrains":14290,"Following":14291,"Ġshaped":14292,"Ġdraws":14293,"Ġaltered":14294,"Jack":14295,"Ġrecipes":14296,"Ġskilled":14297,"wealth":14298,"achi":14299,"election":14300,"Ġbehaviors":14301,"deals":14302,"ĠUntil":14303,"Fe":14304,"Ġdeclaration":14305,"marks":14306,"ĠBetween":14307,"celona":14308,"Ġreson":14309,"Ġbubble":14310,"Among":14311,"Ġimperial":14312,"GS":14313,"Ġfeminist":14314,"2005":14315,"ĠKyle":14316,"Ġaccounting":14317,"ĠTele":14318,"ĠTyr":14319,"Ġconnecting":14320,"Ġrehab":14321,"ĠPred":14322,"sim":14323,"Ġmeantime":14324,"Ġphysician":14325,"MW":14326,"ĠCampbell":14327,"ĠBrandon":14328,"Ġcontributing":14329,"ĠRule":14330,"ĠWeight":14331,"ĠNap":14332,"Ġinteractive":14333,"Ġvag":14334,"Ġhelmet":14335,"ĠComb":14336,"four":14337,"Ġshipped":14338,"Ġcompleting":14339,"ĠPD":14340,"PDATE":14341,"Ġspreading":14342,"Ġscary":14343,"erving":14344,"ĠGas":14345,"Ġfrank":14346,"school":14347,"Ġromantic":14348,"Ġstabil":14349,"Rob":14350,"Ġaccurately":14351,"Ġacute":14352,"ĠHann":14353,"Ġsymbols":14354,"Ġcivilization":14355,"ĠAW":14356,"Ġlightning":14357,"Ġconsiders":14358,"Ġvenue":14359,"Ġ×":14360,"Ġoven":14361,"ĠSF":14362,"his":14363,"Ġnu":14364,"ĠLearn":14365,"Ġpeoples":14366,"Ġstd":14367,"Ġslee":14368,"Ġslic":14369,"ĠStatistics":14370,"Ġcorners":14371,"ĠBaker":14372,"Ġ:)":14373,"mentation":14374,"olver":14375,"Ġlaughing":14376,"ĠTodd":14377,"onde":14378,"ĠHills":14379,"Ġnuts":14380,"ĠWoman":14381,"plane":14382,"Ġliver":14383,"ĠInside":14384,"Sorry":14385,"Ġagrees":14386,"Ġfundament":14387,"ĠFisher":14388,"Ġauction":14389,"Ġthreads":14390,"glas":14391,"ĠBasic":14392,"ĠNat":14393,"Ġlacking":14394,"Ġcelebration":14395,"ju":14396,"Ġsilly":14397,"Euro":14398,"Ġtatt":14399,"ighty":14400,"controlled":14401,"Test":14402,"ĠSingh":14403,"Ġrage":14404,"Ġrhyth":14405,"offic":14406,"ĠPhantom":14407,"Ġheadlines":14408,"Ġresponding":14409,"ĠMorning":14410,"Ġvitamin":14411,"Ġboots":14412,"ĠSite":14413,"alin":14414,"pi":14415,"Ġviral":14416,"ĠUC":14417,"DER":14418,"ĠSex":14419,"Ġstocks":14420,"current":14421,"Ġchurches":14422,"ĠRare":14423,"ĠMurphy":14424,"Ġdenial":14425,"ĠGaming":14426,"Ġtoug":14427,"Ġnick":14428,"Ġmakers":14429,"ĠRonald":14430,"Ġgenerous":14431,"ĠDoc":14432,"ĠMorris":14433,"Ġtransformed":14434,"ĠNormal":14435,"Ġ104":14436,"ĠKickstarter":14437,"ĠUpon":14438,"Online":14439,"ĠIRS":14440,"Ġwrap":14441,"Ġloving":14442,"Ġarrives":14443,"ĠDue":14444,"Ġheter":14445,"ĠMade":14446,"Ġrental":14447,"Ġbelongs":14448,"Ġattorneys":14449,"Ġcrops":14450,"Ġmatched":14451,"ulum":14452,"oline":14453,"109":14454,"Ġdispar":14455,"Ġbuyers":14456,"ĠCambridge":14457,"Ġethics":14458,"roups":14459,"Ġjustified":14460,"Ġmarginal":14461,"Ġrespected":14462,"winning":14463,"Ġnodded":14464,"ĠSerge":14465,"ĠFormer":14466,"Craft":14467,"################":14468,"ĠWarner":14469,"Ġdash":14470,"ete":14471,"Ġentert":14472,"ĠEscape":14473,"outheast":14474,"Ġknees":14475,"ĠBomb":14476,"Ġrug":14477,"Pass":14478,"Ġattitudes":14479,"government":14480,"ĠPrior":14481,"Ġqualities":14482,"Ġnotification":14483,"ĠPhone":14484,"lie":14485,"Ġanticipated":14486,"ĠCombat":14487,"ĠBarry":14488,"Ġ1982":14489,"Users":14490,"oner":14491,"Ġcomputing":14492,"ĠConnecticut":14493,"Ġlesser":14494,"Ġpeers":14495,"ĠCu":14496,"Ġtechnically":14497,"Ġsubmission":14498,"ĠUniversal":14499,"Ġmanually":14500,"ourge":14501,"Ġrespondents":14502,"ĠBTC":14503,"ĠHost":14504,"Ġfare":14505,"ĠBird":14506,"Ġreceipt":14507,"also":14508,"Ġjack":14509,"Ġagriculture":14510,"Ġskull":14511,"Ġ!=":14512,"Ġpassive":14513,"ĠCI":14514,"Ġsocieties":14515,"Ġreminded":14516,"Ġinterference":14517,"Buy":14518,"Ġâľ":14519,"gon":14520,"Ġscrutiny":14521,"ĠWitch":14522,"Ġconducting":14523,"Ġãĥ":14524,"Ġexchanges":14525,"ĠMitchell":14526,"Ġinhabit":14527,"Ġtwist":14528,"BD":14529,"Ġwherever":14530,"groupon":14531,"Ġjokes":14532,"ĠBenjamin":14533,"ĠRandom":14534,"frame":14535,"ĠLions":14536,"Ġhighlighted":14537,"ĠArkansas":14538,"Ent":14539,"Ġpile":14540,"Ġprelim":14541,"gs":14542,"minded":14543,"Ġfelony":14544,"ĠGA":14545,"ĠLuck":14546,"Ġpractically":14547,"ĠBos":14548,"Ġactress":14549,"Dam":14550,"ĠBou":14551,"Ġvisa":14552,"Ġembedded":14553,"Ġhybrid":14554,"Ġearliest":14555,"Ġsooner":14556,"social":14557,"ĠHA":14558,"Ġsteep":14559,"Ġdisadvant":14560,"Ġexploit":14561,"ĠEgg":14562,"ĠUltra":14563,"Ġnecessity":14564,"Local":14565,"iege":14566,"Ġdated":14567,"Ġmasses":14568,"Ġsubscription":14569,"pless":14570,"Ġanonym":14571,"Ġpresumably":14572,"Blue":14573,"Their":14574,"asketball":14575,"ĠPhilip":14576,"Ġcomed":14577,"loaded":14578,"rane":14579,"Ġreflection":14580,"China":14581,"Ġextends":14582,"Ġforming":14583,"Ġunders":14584,"2001":14585,"Ġgrat":14586,"Ġconcentrations":14587,"Ġinsulin":14588,"Ġsecular":14589,"Ġwhilst":14590,"Ġwinners":14591,"Advertisements":14592,"Ġdeliberately":14593,"ĠWorking":14594,"Ġsink":14595,"etics":14596,"dale":14597,"Ġmandate":14598,"Ġgram":14599,"Ġvacation":14600,"Ġwarnings":14601,"ripp":14602,"ĠTHAT":14603,"Ġcommentary":14604,"Ġintu":14605,"Ġaest":14606,"Ġreasoning":14607,"Ġbreakdown":14608,"ĠZombie":14609,"Ġ-->":14610,"ĠPolitical":14611,"cott":14612,"Ġthrust":14613,"Ġtechnological":14614,"Ġdeciding":14615,"Ġtrafficking":14616,"Long":14617,"Welcome":14618,"prising":14619,"ĠCommunications":14620,"Ġendors":14621,"Ġswift":14622,"Ġmetabol":14623,"coins":14624,"resa":14625,"ĠHTTP":14626,"Ġenroll":14627,"ĠHappy":14628,"usr":14629,"intage":14630,"Ġ[\"":14631,"uably":14632,"ĠMaterial":14633,"Ġrepeal":14634,"Sept":14635,"kh":14636,"ĠModi":14637,"Ġunderneath":14638,"ĠIL":14639,"shore":14640,"Ġdiagnosed":14641,"aceutical":14642,"Ġshower":14643,"aux":14644,"ĠSwitch":14645,"ĠStrength":14646,"Ġjihad":14647,"national":14648,"Ġtrauma":14649,"ussy":14650,"oni":14651,"Ġconsolid":14652,"Ġcalories":14653,"ĠFlynn":14654,"agged":14655,"168":14656,"ĠPink":14657,"Ġfulfill":14658,"Ġchains":14659,"Ġnotably":14660,"ĠAV":14661,"Life":14662,"ĠChuck":14663,"mus":14664,"ĠUrban":14665,"ĠHend":14666,"Ġdeposit":14667,"ĠSad":14668,"Ġaffair":14669,"ORK":14670,"ieval":14671,"ĠFDA":14672,"Ġtrop":14673,"ĠOverall":14674,"Ġvirtue":14675,"Ġsatisfaction":14676,"aund":14677,"Ġlun":14678,"ĠSwitzerland":14679,"ĠOperation":14680,"process":14681,"Ġshook":14682,"Ġcounties":14683,"leased":14684,"ĠCharlotte":14685,"112":14686,"Ġtranscript":14687,"Ġredd":14688,"push":14689,"ĠHey":14690,"ĠAnalysis":14691,"[\"":14692,"Ġalternatives":14693,"ardless":14694,"Ġeleph":14695,"Ġprejud":14696,"ĠLeaf":14697,"Having":14698,"ĠHub":14699,"Ġexpressions":14700,"ĠVolume":14701,"Ġshocking":14702,"ĠReds":14703,"Ġreadily":14704,"Ġplanets":14705,"adata":14706,"Ġcollapsed":14707,"ĠMadrid":14708,"Ġirrit":14709,"ipper":14710,"ĠEnc":14711,"ĠWire":14712,"Ġbuzz":14713,"ĠGP":14714,"asha":14715,"Ġaccidentally":14716,"uru":14717,"Ġfrustrated":14718,"ĠSA":14719,"Ġhungry":14720,"ĠHuff":14721,"Ġlabels":14722,"anto":14723,"ĠEP":14724,"Ġbarriers":14725,")|":14726,"ĠBerkeley":14727,"ĠJets":14728,"Ġpairs":14729,"ĠLan":14730,"James":14731,"ĠBear":14732,"Ġhumor":14733,"ĠLiberty":14734,"Ġmagnitude":14735,"Ġaging":14736,"ĠMason":14737,"Ġfriendship":14738,"umbling":14739,"Ġemerge":14740,"Ġnewspapers":14741,"Ġambitious":14742,"ĠRichards":14743,"aternal":14744,"Ġ1981":14745,"Ġcookies":14746,"Ġsculpt":14747,"Ġpursuit":14748,"Location":14749,"Ġscripts":14750,"pc":14751,"Ġarrangements":14752,"Ġdiameter":14753,"Ġloses":14754,"amation":14755,"Ġliqu":14756,"ĠJake":14757,"arette":14758,"Ġunderstands":14759,"ĠZen":14760,"vm":14761,"Ġapprove":14762,"Ġwip":14763,"Ġultra":14764,"Ġintend":14765,"ĠDI":14766,"ascular":14767,"Ġstays":14768,"ĠKor":14769,"ĠKl":14770,"Ġinvesting":14771,"La":14772,"Ġbelieving":14773,"bad":14774,"mouth":14775,"Ġtaxpayer":14776,"ãĥĥ":14777,"ĠQuebec":14778,"Ġlap":14779,"ĠSwiss":14780,"drop":14781,"Ġdrain":14782,"iri":14783,"etc":14784,"ften":14785,"ĠNex":14786,"Ġstraw":14787,"Ġscreaming":14788,"Ġcounted":14789,"Ġdamaging":14790,"Ġambassador":14791,"century":14792,"Ġprox":14793,"Ġarrests":14794,"uv":14795,"ilateral":14796,"ĠCharg":14797,"Ġprescribed":14798,"Ġindependently":14799,"Ġfierce":14800,"ĠBaby":14801,"Ġbrave":14802,"Ġsuits":14803,"=>":14804,"Ġbaseline":14805,"ĠRate":14806,"Ġislands":14807,"Ġ((":14808,"green":14809,"ixels":14810,"Ġnamely":14811,"ĠVillage":14812,"than":14813,"amy":14814,"Version":14815,"gmail":14816,"entials":14817,"ĠSud":14818,"ĠMelbourne":14819,"Ġarriving":14820,"Ġquantum":14821,"eff":14822,"ropolitan":14823,"Tri":14824,"Ġfuneral":14825,"ĠIR":14826,"ÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤ":14827,"ĠCob":14828,"itably":14829,"Ġturb":14830,"Ġcombo":14831,"Review":14832,"Ġdeployment":14833,"uity":14834,"ĠBott":14835,"Ġinvisible":14836,"Ġrendering":14837,"Ġunlocked":14838,"Ġaqu":14839,"ĠVladimir":14840,"Ġpad":14841,"ĠBrain":14842,"ĠLegacy":14843,"dragon":14844,"ĠKurdish":14845,"Ġsounded":14846,"Ġdetained":14847,"ĠDM":14848,"gary":14849,"Ġdaughters":14850,"Ġdisturbing":14851,"uka":14852,"ĠParad":14853,"Ġtast":14854,"Ġunfortunate":14855,"Ġul":14856,"emin":14857,"Ġattendance":14858,"trl":14859,"Ġparks":14860,"ĠMemorial":14861,"ĠAlice":14862,"othy":14863,"guard":14864,"ĠDise":14865,"ĠShan":14866,"ĠForum":14867,"Rich":14868,"Ġshifted":14869,"uez":14870,"Ġlighter":14871,"ĠMagn":14872,"Ġcod":14873,"Sch":14874,"hammad":14875,"Pub":14876,"350":14877,"ĠPokemon":14878,"Ġprototype":14879,"Ġunre":14880,"Base":14881,"ĠStudents":14882,"ĠReply":14883,"ĠCommunist":14884,"Ġgau":14885,"ĠTyler":14886,"IZ":14887,"Ġparticipated":14888,"Ġsuprem":14889,"ĠDetails":14890,"Ġvessels":14891,"rod":14892,"Ġtribe":14893,"keep":14894,"Ġassumptions":14895,"Ġpound":14896,"Ġcrude":14897,"ĠAvailable":14898,"Ġswimming":14899,"Ġinclusion":14900,"Ġadvances":14901,"culation":14902,"Ġconservation":14903,"Ġoverd":14904,"ĠBuffalo":14905,"Article":14906,"edge":14907,"Ġawa":14908,"ĠMadison":14909,"Ġsidew":14910,"Ġcatast":14911,"ĠKrist":14912,"ucle":14913,"ĠHighway":14914,"ĠTerror":14915,"Ġactivation":14916,"Ġunconscious":14917,"ĠSatan":14918,"ĠSusan":14919,"illery":14920,"Ġarranged":14921,"iop":14922,"Ġrumors":14923,"urring":14924,"think":14925,"ĠKeith":14926,"ĠKind":14927,"Ġavoiding":14928,"byn":14929,"nut":14930,"ĠSpeaker":14931,"rus":14932,"names":14933,"Ġguilt":14934,"ĠOlympics":14935,"Ġsail":14936,"ĠMes":14937,"levant":14938,"ĠColumbus":14939,"aft":14940,"City":14941,"South":14942,"ĠHarvey":14943,"ĠPun":14944,"Several":14945,"Ġmentally":14946,"Ġimpress":14947,"mount":14948,"ĠUbuntu":14949,"âĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶ":14950,"ĠSuperman":14951,"ĠMPs":14952,"Ġintentions":14953,"ĠRacing":14954,"Ġlikelihood":14955,"Ġ240":14956,"Total":14957,"Ġtoys":14958,"ĠWatson":14959,"Ġurge":14960,"Lear":14961,"ĠPaper":14962,"Ġoccurring":14963,"ĠBeng":14964,"ĠCert":14965,"Ġstones":14966,"Tim":14967,"ĠTwin":14968,"zb":14969,"ĠDynam":14970,"Ġpolitician":14971,"kens":14972,"ĠEnterprise":14973,"UTERS":14974,"Ġabol":14975,"Ġrefresh":14976,"Ġarbitrary":14977,"pection":14978,"Ġtroubles":14979,"Ġ});":14980,"tv":14981,"Ġpilots":14982,"Ġdistribute":14983,"Ġaudit":14984,"Ġpause":14985,"original":14986,"Ġrivals":14987,"£":14988,"Fig":14989,"TL":14990,"abil":14991,"rying":14992,"Lin":14993,"ioned":14994,"lon":14995,"Ġfancy":14996,"Ġcrashed":14997,"Ġtract":14998,"Ġshed":14999,"Ġconsume":15000,"Based":15001,"download":15002,"init":15003,"Ġvoltage":15004,"Introdu":15005,"Ġcondemned":15006,"ĠFinance":15007,"respect":15008,"Ġexcluded":15009,"Ġestablishing":15010,"heric":15011,"Ġheritage":15012,"Ġspectacular":15013,"Ġunst":15014,"ĠSnowden":15015,"ĠLane":15016,"San":15017,"Ġprotections":15018,"struction":15019,"incinn":15020,"Ġmacro":15021,"Custom":15022,"iosity":15023,"Ġesp":15024,"Ġfunctioning":15025,"Ġmush":15026,"Ġpuzzle":15027,"Ġethical":15028,"Mal":15029,"Ġgoverning":15030,"ĠFerguson":15031,"Ġrestored":15032,"Ġstressed":15033,"ĠCounter":15034,"ĠKas":15035,"clip":15036,"ANS":15037,"Ġseiz":15038,"UK":15039,"byss":15040,"oldown":15041,"api":15042,"Ġpermanently":15043,"ounters":15044,"West":15045,"Through":15046,"Light":15047,"atoes":15048,"Ġneat":15049,"Ġcord":15050,"urer":15051,"Ġseverely":15052,"ĠAven":15053,"Ġinterrog":15054,"Ġtriple":15055,"Given":15056,"Number":15057,"Ġarise":15058,"Ġsher":15059,"plant":15060,"Ġflower":15061,"ĠCou":15062,"Ġate":15063,"Ġnewer":15064,"bul":15065,"Ġmeanwhile":15066,"ĠLair":15067,"Ġadjustment":15068,"ĠCopyright":15069,"Ġdivers":15070,"iological":15071,"Ġgamers":15072,"oat":15073,"Ġhistorically":15074,"Ġanalog":15075,"Ġlongtime":15076,"Ġprescription":15077,"ĠMist":15078,"ĠHyper":15079,"ĠMaine":15080,"ĠDeity":15081,"Ġmultipl":15082,"ĠReincarn":15083,"ĠHyd":15084,"ĠPic":15085,"Sil":15086,"rants":15087,"ĠCris":15088,".;":15089,"({":15090,"ependence":15091,"Ġrecy":15092,"ateur":15093,"Ġquad":15094,"Ġglob":15095,"Ġconced":15096,"team":15097,"Ġcapitalist":15098,"ĠLot":15099,"Ġroyal":15100,"ĠCyber":15101,"Ġblacks":15102,"metic":15103,"riv":15104,"ĠDanny":15105,"Ġspo":15106,"ĠRO":15107,"Ġanimated":15108,"rypted":15109,"ĠDeputy":15110,"Ġrendered":15111,"FE":15112,"Ġstreak":15113,"Ġclouds":15114,"ĠDoug":15115,"~~~~~~~~":15116,"Ġdiscour":15117,"ĠVeh":15118,"Ġpsychology":15119,"ĠJourney":15120,"Ġcrystal":15121,"ĠFrost":15122,"Ġsuspicion":15123,"Ġrelate":15124,"orus":15125,"ĠCrypt":15126,"ĠNVIDIA":15127,"comed":15128,"uting":15129,"incinnati":15130,"Ġvulnerability":15131,"ostic":15132,"Ġisolation":15133,"Ġcooling":15134,"ĠCoalition":15135,"Ġ119":15136,"Four":15137,"ĠDeal":15138,"Ġâī":15139,"semble":15140,"rament":15141,"ĠBarcelona":15142,"Ġ102":15143,"Ġcocaine":15144,"ocalypse":15145,"Feb":15146,"ogenic":15147,"Ġmutation":15148,"Ġcryptoc":15149,"ĠKel":15150,"ĠGit":15151,"ais":15152,"Ġsisters":15153,"ANK":15154,"Ġactivate":15155,"Ter":15156,"Ġdread":15157,"ylon":15158,"Ġpropri":15159,"Aust":15160,"ĠDefault":15161,"Ġoutdoor":15162,"Ġsheer":15163,"ceive":15164,"Ġgently":15165,"о":15166,"Program":15167,"ĠâĨĴ":15168,"Ġvegan":15169,"ĠCrus":15170,"Ġresponsibilities":15171,"ĠHR":15172,"OLD":15173,"Ġprevents":15174,"Ġstiff":15175,"ĠWere":15176,"Ġathletic":15177,"ĠScore":15178,"Ġ):":15179,"Ġcolumns":15180,"ĠLoc":15181,"available":15182,"ĠFram":15183,"ĠSessions":15184,"Ġcompanion":15185,"Ġpacks":15186,"140":15187,"ĠKnights":15188,"Ġfart":15189,"Ġstreams":15190,"Ġshore":15191,"Ġappeals":15192,"ĠPerformance":15193,"haul":15194,"ĠStra":15195,"ĠNag":15196,"103":15197,"ĠTransportation":15198,"BB":15199,"Ev":15200,"zan":15201,"Public":15202,"Ġtwin":15203,"ulsion":15204,"Mult":15205,"Ġelectro":15206,"Ġstatue":15207,"ationally":15208,"ĠNort":15209,"Ġinspection":15210,"/*":15211,"igue":15212,"Ġcompassion":15213,"ĠTales":15214,"ĠStein":15215,"ĠScreen":15216,"ĠBug":15217,"ĠLion":15218,"girl":15219,"Ġwithdrawal":15220,"Ġobjectives":15221,"Ġbloody":15222,"Ġpreliminary":15223,"Ġjacket":15224,"Ġdimensions":15225,"ĠCool":15226,"ĠOccup":15227,"Ġwreck":15228,"Ġdoubled":15229,"anking":15230,"Ġ1975":15231,"Ġglasses":15232,"ĠWang":15233,"prov":15234,"Path":15235,"connected":15236,"ĠMulti":15237,"ĠNorway":15238,"agonist":15239,"Ġfeared":15240,"Ġtouching":15241,"Ġarguably":15242,"¯¯¯¯¯¯¯¯":15243,"ĠNCAA":15244,"chem":15245,"Ġspat":15246,"ĠWWE":15247,"ĠCel":15248,"igger":15249,"Ġattacker":15250,"ĠJoin":15251,"object":15252,"etta":15253,"Ġeliminated":15254,"det":15255,"Ġdestruct":15256,"ĠLucas":15257,"ctuary":15258,"180":15259,"ĠBrady":15260,"ĠBlues":15261,"Bay":15262,"aukee":15263,"Ġtimeline":15264,"Ġdelegates":15265,"written":15266,"ufficient":15267,"Ġshapes":15268,"Copyright":15269,"ouble":15270,"service":15271,"Ġpione":15272,"Ġcolleges":15273,"Ġrows":15274,"Ġspite":15275,"Ġassessed":15276,"360":15277,"Ġlease":15278,"Ġconfidential":15279,"cker":15280,"ĠManning":15281,"ĠVoice":15282,"Ġsealed":15283,"Ġcalculate":15284,"NO":15285,"ĠAssistant":15286,"Ġteenager":15287,"ulent":15288,"atherine":15289,"Ġmock":15290,"Ġdiamond":15291,"Ġfest":15292,"Ġswitched":15293,"Ġresume":15294,"ĠPuerto":15295,"Ġlanes":15296,"iration":15297,"ĠSimilarly":15298,"Ġrod":15299,"ĠSel":15300,"ĠPalace":15301,"ĠLimited":15302,"eous":15303,"Ġvariant":15304,"Ġward":15305,"Ġ))":15306,"Show":15307,"OOK":15308,"Alex":15309,"ĠNep":15310,"bris":15311,"ĠWikipedia":15312,"Ġexceptional":15313,"Ġmanages":15314,"ĠDraw":15315,"Again":15316,"Ġcopper":15317,"utt":15318,"Ġexports":15319,"Ġportfolio":15320,"Ġelevated":15321,"Rated":15322,"ĠOtherwise":15323,"ĠTact":15324,"ĠShel":15325,"ĠTX":15326,"\"âĢĶ":15327,"Ġresur":15328,"ĠWa":15329,"venant":15330,"Ġmonetary":15331,"people":15332,"Email":15333,"Ġfifty":15334,"ĠSweet":15335,"ĠMalaysia":15336,"Ġconfusing":15337,"ĠRio":15338,"uda":15339,"utenant":15340,"\");":15341,"Ġpraised":15342,"Ġvolumes":15343,"turn":15344,"Ġmature":15345,"Ġnonprofit":15346,"Ġpassionate":15347,"ĠPrivate":15348,"Ġ103":15349,"Ġdescend":15350,"ç¥ŀ":15351,"uffy":15352,"headed":15353,"Whether":15354,"rien":15355,"zech":15356,"beit":15357,"Ġchrom":15358,"ĠMcM":15359,"Ġdancing":15360,"Ġeleg":15361,"ĠNoticed":15362,"115":15363,"Ġadvocacy":15364,"ENTS":15365,"ambling":15366,"ĠMinor":15367,"ĠFinn":15368,"Ġpriorities":15369,"Ġthereof":15370,"ĠStage":15371,"ĠRogers":15372,"Ġsubstitute":15373,"ĠJar":15374,"ĠJefferson":15375,"Ġlightly":15376,"102":15377,"ĠLisa":15378,"uits":15379,"ysical":15380,"Ġshifts":15381,"Ġdrones":15382,"Ġworkplace":15383,"Ġresid":15384,"ensed":15385,"ahn":15386,"Ġpreferences":15387,"server":15388,"Ġdebates":15389,"doc":15390,"ĠGods":15391,"Ġhelicopter":15392,"Ġhonour":15393,"Ġconsiderably":15394,"eded":15395,"ĠFemale":15396,"ĠAnne":15397,"Ġreun":15398,"ĠFace":15399,"ĠHallow":15400,"ĠBudget":15401,"Ġcondemn":15402,"Ġtender":15403,"Prof":15404,"ocratic":15405,"ĠTurner":15406,"ĠAgric":15407,"Ġ1976":15408,"Ġapt":15409,"disc":15410,"ĠFighter":15411,"ĠAur":15412,"Ġgarbage":15413,"input":15414,"ĠKarl":15415,"ĠOliver":15416,"ĠLanguage":15417,"kn":15418,"Non":15419,"ĠClar":15420,"Ġtraditions":15421,"Ġadvertisement":15422,"ĠSor":15423,"Ġarchive":15424,"Ġvillages":15425,"750":15426,"Ġimplementing":15427,"waukee":15428,"Ġdietary":15429,"Ġswitching":15430,"Republic":15431,"Ġvelocity":15432,"Ġcit":15433,"ĠAwards":15434,"Ġfinancing":15435,"Ġlasted":15436,")]":15437,"Ġreminder":15438,"Person":15439,"Ġprecision":15440,"Ġdesigners":15441,"ĠFried":15442,"ĠBorder":15443,"Ġtragic":15444,"Ġwield":15445,"Ġinitiatives":15446,"ĠTank":15447,"wer":15448,"Ġjoins":15449,"Ro":15450,"inery":15451,"Ġarrow":15452,"Ġgenerating":15453,"founder":15454,"Ġsearches":15455,"Ġrandomly":15456,"Access":15457,"Ġbatch":15458,"Ġposed":15459,"lat":15460,"Ġpursuing":15461,"asa":15462,"Ġtestified":15463,"forming":15464,"ĠShar":15465,"wiki":15466,"ĠEither":15467,"Sometimes":15468,"Ġsenators":15469,"ĠJohnny":15470,"ĠTaliban":15471,"ĠGPS":15472,"\":\"/":15473,"ãģ®å":15474,"Ġanalyzed":15475,"ĠRubio":15476,"ĠMovement":15477,"opard":15478,"iii":15479,"Stand":15480,"fight":15481,"Ġignoring":15482,"iang":15483,"ĠGN":15484,"soever":15485,"ĠSTAT":15486,"Ġrefusing":15487,"Ġsweat":15488,"Ġbay":15489,"PORT":15490,"irmed":15491,"aky":15492,"Ġdispro":15493,"Ġlabeled":15494,"Ġ108":15495,"Hello":15496,"Ġpleasant":15497,"aba":15498,"Ġtriumph":15499,"Ġaboard":15500,"Ġincom":15501,"ĠCrow":15502,"lett":15503,"Ġfolk":15504,"Ġchase":15505,"``":15506,"ĠBrus":15507,"Ġteens":15508,"cue":15509,"Ġterrain":15510,"hyd":15511,"ilight":15512,"ORY":15513,"Support":15514,"ews":15515,"lli":15516,"raints":15517,"ĠCand":15518,"Ġabused":15519,"achment":15520,"larg":15521,"Bas":15522,"ĠCancer":15523,"Ġ1978":15524,"Ġsupporter":15525,"access":15526,"ĠTermin":15527,"ĠTampa":15528,"ĠANY":15529,"Ġnewest":15530,"ĠCriminal":15531,"edu":15532,"Ġ1930":15533,"Ġadmits":15534,"Ġende":15535,"Ġfailures":15536,"urate":15537,"fulness":15538,"cycl":15539,"ĠSubject":15540,"Ġinfinite":15541,"three":15542,"WA":15543,"pit":15544,"ĠInstall":15545,"Rad":15546,"iliation":15547,"GM":15548,"Ġcontinent":15549,"Ġaccommodate":15550,"ĠClay":15551,"Ġpup":15552,"ĠFunction":15553,"Ġhammer":15554,"ĠAlberta":15555,"Ġrevised":15556,"Ġminorities":15557,"Ġmeasurement":15558,"Connell":15559,"Ġdisable":15560,"ĠMix":15561,"Incre":15562,"Ġfork":15563,"ĠRosen":15564,"Ġimplies":15565,"umblr":15566,"ANG":15567,"Ġproteins":15568,"Ġaggression":15569,"Ġfacilitate":15570,"SN":15571,"Ġillegally":15572,"uer":15573,"Ġacadem":15574,"Ġpuzz":15575,"ĠShift":15576,"pay":15577,"ollo":15578,"Ġaudiences":15579,"Build":15580,"Ġnoble":15581,"Ġsyntax":15582,"âĺħ":15583,"Ġbeam":15584,"ĠBed":15585,"ĠAld":15586,"Ġorigins":15587,"video":15588,"Ġ1977":15589,"ĠAssault":15590,"Ġgarage":15591,"Team":15592,"Ġverdict":15593,"Ġdwar":15594,"ĠVirtual":15595,"event":15596,"Keep":15597,"Ġsentiment":15598,"Ġwildlife":15599,"shirt":15600,"Ġburg":15601,"Ġrecommendation":15602,"represent":15603,"Ġgallery":15604,"owners":15605,"Ġscholar":15606,"Ġconvenience":15607,"ĠSwift":15608,"Ġconvinc":15609,"Cap":15610,"Ġwarfare":15611,"ĠVisual":15612,"Ġconstitute":15613,"Ġabort":15614,"ĠWeather":15615,"ĠLooking":15616,"ĠHem":15617,"Ġmartial":15618,"Ġincoming":15619,"etition":15620,"Ġtolerance":15621,"ĠCreated":15622,"Ġflows":15623,"ĠElder":15624,"Ġsouls":15625,"Ġfoul":15626,"ĠPain":15627,"ĠCAN":15628,"Ġ220":15629,"bc":15630,"hend":15631,"Ġgenius":15632,"Real":15633,"ĠWr":15634,"ometer":15635,"pad":15636,"Ġlimiting":15637,"ĠSi":15638,"ĠLore":15639,"ĠAdventures":15640,"Ġvaried":15641,"Disc":15642,"fin":15643,"ĠPersonal":15644,"Chris":15645,"Ġinvented":15646,"Ġdive":15647,"ĠRise":15648,"Ġoz":15649,"ĠComics":15650,"Ġexpose":15651,"ĠReb":15652,"letters":15653,"site":15654,"imated":15655,"Ġhacking":15656,"Ġeducated":15657,"ĠNobody":15658,"Ġdepri":15659,"Ġincentive":15660,"ãĤ·":15661,"Ġoversight":15662,"Ġtribes":15663,"ĠBelgium":15664,"Ġlicensing":15665,"ourt":15666,"Product":15667,"ahl":15668,"ĠGem":15669,"Ġspecialist":15670,"Ġcra":15671,"anners":15672,"ĠCorbyn":15673,"Ġ1973":15674,"READ":15675,"Ġsummar":15676,"Ġoverlook":15677,"ĠApplication":15678,"Ġinappropriate":15679,"Ġdownloaded":15680,"Que":15681,"ĠBears":15682,"Ġthumb":15683,"ĠCharacter":15684,"ĠReincarnated":15685,"ĠSid":15686,"Ġdemonstrates":15687,"sky":15688,"ĠBloomberg":15689,"ĠArray":15690,"ĠResults":15691,"ĠFourth":15692,"ĠEDT":15693,"ĠOscar":15694,"cend":15695,"Ġ106":15696,"ĠNULL":15697,"ĠHERE":15698,"match":15699,"ĠBrun":15700,"Ġglucose":15701,"ieg":15702,"egu":15703,"Ġcertified":15704,"Ġrelie":15705,"Ġhumanitarian":15706,"Ġprayers":15707,"King":15708,"Ġnan":15709,"hou":15710,"108":15711,"ulu":15712,"Ġrenewable":15713,"Ġdistinguish":15714,"Ġdense":15715,"ĠVent":15716,"ĠPackage":15717,"ĠBoss":15718,"Ġeditors":15719,"Ġmigr":15720,"Tra":15721,"ĠPeters":15722,"ĠArctic":15723,"2004":15724,"ĠCape":15725,"Ġlocally":15726,"Ġlasting":15727,"Ġhandy":15728,".).":15729,"Pan":15730,"ĠRES":15731,"Index":15732,"Ġtensions":15733,"Ġformerly":15734,"Ġideological":15735,"Ġsensors":15736,"Ġdealers":15737,"Ġdefines":15738,"Sk":15739,"Ġproceeds":15740,"Ġproxy":15741,"azines":15742,"ĠBash":15743,"ĠPad":15744,"ĠCraft":15745,"ealous":15746,"Ġsheets":15747,"ometry":15748,"June":15749,"clock":15750,"TT":15751,"ĠTheatre":15752,"ĠBuzz":15753,"Ġchapters":15754,"Ġmillenn":15755,"Ġdough":15756,"ĠCongressional":15757,"Ġimagined":15758,"avior":15759,"Ġclinic":15760,"Ġ1945":15761,"Ġholder":15762,"root":15763,"olester":15764,"Ġrestart":15765,"BN":15766,"ĠHamas":15767,"ĠJob":15768,"Ġorb":15769,"Ġram":15770,"Ġdisclose":15771,"Ġtranslate":15772,"Ġimmigrant":15773,"Ġannoying":15774,"Ġtreaty":15775,"anium":15776,"ĠTea":15777,"ĠLegion":15778,"Ġcrowds":15779,"ĠBec":15780,"ĠAer":15781,"ohyd":15782,"Bro":15783,"Looking":15784,"Ġlbs":15785,"Ġaggress":15786,"Ġseam":15787,"Ġintercept":15788,"ĠMI":15789,"mercial":15790,"activ":15791,"ĠCit":15792,"Ġdimension":15793,"Ġconsistency":15794,"Ġrushing":15795,"ĠDouglas":15796,"Ġtrim":15797,"Install":15798,"icker":15799,"Ġshy":15800,"106":15801,"Ġmentions":15802,"pelled":15803,"ĠTak":15804,"cost":15805,"Ġclassroom":15806,"Ġfortune":15807,"driven":15808,"Ġunle":15809,"ĠWheel":15810,"Ġinvestor":15811,"ĠMasters":15812,"kit":15813,"Ġassociations":15814,"ĠEvolution":15815,"oping":15816,"uscript":15817,"Ġprovincial":15818,"ĠWalter":15819,"avi":15820,"SO":15821,"Ġunlimited":15822,"English":15823,"ĠCards":15824,"ĠEbola":15825,"nered":15826,"Ġrevenge":15827,"Ġoutright":15828,"umper":15829,"Ġfitting":15830,"ĠSolid":15831,"Ġformally":15832,"Ġproblematic":15833,"Ġhazard":15834,"Ġencryption":15835,"Ġstraightforward":15836,"ĠAK":15837,"Ġpse":15838,"ĠOrb":15839,"ĠChamber":15840,"ĠMak":15841,"Contents":15842,"Ġloyalty":15843,"Ġlyrics":15844,"ĠSym":15845,"Ġwelcomed":15846,"Ġcooked":15847,"Ġmonop":15848,"Ġnurse":15849,"Ġmisleading":15850,"Ġeternal":15851,"Ġshifting":15852,"Ġ+=":15853,"Vis":15854,"Ġinstitutional":15855,"illary":15856,"Ġpant":15857,"VERT":15858,"ĠACC":15859,"ĠEnh":15860,"Ġincon":15861,"ĠREUTERS":15862,"Ġdonated":15863,"â̦â̦â̦â̦":15864,"Intern":15865,"Ġexhibit":15866,"Ġtire":15867,"ĠRic":15868,"ĠChampion":15869,"ĠMuhammad":15870,"NING":15871,"ĠSoccer":15872,"Ġmobility":15873,"Ġvarying":15874,"ĠMovie":15875,"Ġlord":15876,"oak":15877,"Field":15878,"Ġvector":15879,"usions":15880,"Ġscrap":15881,"Ġenabling":15882,"make":15883,"Tor":15884,".*":15885,"||":15886,"ĠWebsite":15887,"ĠNPC":15888,"Ġsocialist":15889,"ĠBilly":15890,"ĠAdditional":15891,"Ġcargo":15892,"Ġfarms":15893,"ĠSoon":15894,"ĠPrize":15895,"Ġmidnight":15896,"Ġ900":15897,"seen":15898,"ĠSpot":15899,"Ġsheep":15900,"Ġsponsored":15901,"ĠHi":15902,"ĠJump":15903,"Ġ1967":15904,"Microsoft":15905,"ĠAgent":15906,"Ġcharts":15907,"dir":15908,"Ġadjacent":15909,"Ġtricks":15910,"Ġmanga":15911,"Ġexagger":15912,"/>":15913,"football":15914,"ĠFCC":15915,"GC":15916,"ĠTier":15917,"andra":15918,"OUND":15919,"%),":15920,"Ġfruits":15921,"VC":15922,"ĠAA":15923,"Rober":15924,"Ġmidst":15925,"âĹ":15926,"anka":15927,"Ġlegislature":15928,"ĠNeil":15929,"Ġtourists":15930,"\"\"":15931,"ĠWarning":15932,"ĠNevertheless":15933,"ĠOfficial":15934,"ĠWhatever":15935,"Ġmold":15936,"Ġdrafted":15937,"Ġsubstances":15938,"Ġbreed":15939,"Ġtags":15940,"ĠTask":15941,"Ġverb":15942,"Ġmanufactured":15943,"comments":15944,"ĠPolish":15945,"Prov":15946,"Ġdetermines":15947,"Obama":15948,"kers":15949,"Ġutterly":15950,"Ġsect":15951,"sche":15952,"ĠGates":15953,"ĠChap":15954,"Ġaluminum":15955,"Ġzombie":15956,"ĠTouch":15957,"ĠUP":15958,"Ġsatisfy":15959,"Ġpredomin":15960,"ascript":15961,"Ġelaborate":15962,"Ġ1968":15963,"Ġmeasuring":15964,"ĠVari":15965,"anyahu":15966,"Ġsir":15967,"ulates":15968,"idges":15969,"ickets":15970,"ĠSpencer":15971,"TM":15972,"oubted":15973,"Ġprey":15974,"Ġinstalling":15975,"ĠCab":15976,"reed":15977,"reated":15978,"Supp":15979,"Ġwrist":15980,"ĠKerry":15981,"107":15982,"ĠKle":15983,"ĠRachel":15984,"Ġcotton":15985,"ĠARE":15986,"ĠEle":15987,"Control":15988,"Ġloads":15989,"ĠDod":15990,"anas":15991,"bone":15992,"Ġclassical":15993,"ĠRegional":15994,"ĠInteg":15995,"VM":15996,"Ġdesires":15997,"Ġautism":15998,"supported":15999,"ĠMessage":16000,"Ġcompact":16001,"writer":16002,"Ġ109":16003,"ĠHurricane":16004,"cision":16005,"Ġcycles":16006,"Ġdrill":16007,"Ġcolleague":16008,"Ġmaker":16009,"German":16010,"Ġmistaken":16011,"Sun":16012,"ĠGay":16013,"Ġwhatsoever":16014,"Ġsells":16015,"ĠAirl":16016,"liv":16017,"ĠOption":16018,"Ġsolved":16019,"Ġsectors":16020,"Ġhorizontal":16021,"Ġequation":16022,"ĠSkill":16023,"ĠBio":16024,"gement":16025,"ĠSnap":16026,"ĠLegal":16027,"Ġtrademark":16028,"Ġmakeup":16029,"Ġassembled":16030,"Ġsaves":16031,"ĠHalloween":16032,"ĠVermont":16033,"ĠFROM":16034,"Ġfarming":16035,"ĠPodcast":16036,"acceptable":16037,"ĠHigher":16038,"Ġasleep":16039,"ullivan":16040,"Ġreferen":16041,"ĠLev":16042,"Ġbullets":16043,"oko":16044,"HC":16045,"Ġstairs":16046,"Ġmaintains":16047,"ĠLower":16048,"ĠVi":16049,"Ġmarine":16050,"Ġacres":16051,"Ġcoordinator":16052,"ĠJoh":16053,"Ġcounterparts":16054,"ĠBrothers":16055,"Ġindict":16056,"bra":16057,"Ġchunk":16058,"Ġcents":16059,"Home":16060,"ĠMonth":16061,"Ġaccordingly":16062,"ifles":16063,"ĠGermans":16064,"ĠSyn":16065,"Hub":16066,"Ġeyeb":16067,"âĶĢâĶĢâĶĢâĶĢ":16068,"Ġranges":16069,"ĠHolland":16070,"ĠRobot":16071,"fc":16072,"Mike":16073,"Ġplasma":16074,"Ġswap":16075,"Ġathlete":16076,"ĠRams":16077,",'\"":16078,"Ġinfections":16079,"Ġcorrid":16080,"Ġvib":16081,"Ġpatches":16082,"Ġtraditionally":16083,"Ġrevelation":16084,"Ġsweep":16085,"Ġglance":16086,"Ġinex":16087,"2003":16088,"ĠRaw":16089,"working":16090,"osures":16091,"ĠDat":16092,"ĠLynch":16093,"Ġleverage":16094,"ĠReid":16095,"Ġcorrelation":16096,"iances":16097,"avascript":16098,"Ġrepository":16099,"retty":16100,"Ġ1972":16101,"240":16102,"Ġoun":16103,"pol":16104,"ĠReed":16105,"Ġtactical":16106,"isite":16107,"Apple":16108,"ĠQuinn":16109,"Ġraped":16110,"illo":16111,"Europe":16112,"Ġalgorithms":16113,"ĠRodrig":16114,"iu":16115,"Ġillum":16116,"Ġfame":16117,"Ġintroducing":16118,"Ġdelays":16119,"ĠRaiders":16120,"Ġwhistle":16121,"Ġnovels":16122,"ĠReally":16123,"Ġderiv":16124,"Ġpublications":16125,"ĠNeither":16126,"ĠCommerce":16127,"Ġaston":16128,"language":16129,"Notes":16130,"ĠRoth":16131,"ĠFear":16132,"Ġmate":16133,"Ġparade":16134,"ĠQB":16135,"Ġmaneu":16136,"ĠCincinnati":16137,"mitting":16138,"Ġwaist":16139,"ĠRew":16140,"Ġdiscont":16141,"а":16142,"Ġstaring":16143,"Ġalias":16144,"Ġsecurities":16145,"Ġtoilet":16146,"ĠJedi":16147,"Ġunlaw":16148,"vised":16149,"////////":16150,"](":16151,"ĠWeiss":16152,"Ġprest":16153,"ĠCompan":16154,"Ġmemo":16155,"ĠGrace":16156,"July":16157,"ĠElite":16158,"center":16159,"ĠStay":16160,"Ġgalaxy":16161,"Ġtooth":16162,"ĠSettings":16163,"Ġsubjected":16164,"ãĤ¦":16165,"Ġlineback":16166,"Ġretailers":16167,"ĠWant":16168,"Ġdangers":16169,"Air":16170,"Ġvoluntary":16171,"eway":16172,"Ġinterpreted":16173,"otine":16174,"ç":16175,"Ġpel":16176,"Service":16177,"ĠEventually":16178,"Ġcareers":16179,"Ġthreaten":16180,"Ġmemor":16181,"ĠBradley":16182,"ancies":16183,"sn":16184,"ĠUnknown":16185,"National":16186,"Ġshadows":16187,"ailand":16188,"ĠDash":16189,"Everyone":16190,"izzard":16191,"March":16192,"=(":16193,"Ġpulls":16194,"Ġstranger":16195,"Ġbackwards":16196,"ĠBernard":16197,"imensional":16198,"Ġchron":16199,"Ġtheoretical":16200,"ktop":16201,"Ġware":16202,"ĠInvestig":16203,"ĠIniti":16204,"ĠOperations":16205,"oven":16206,"ocide":16207,"*/":16208,"Ġflames":16209,"ĠCash":16210,"shit":16211,"Ġcab":16212,"ĠAnaly":16213,"ĠSeah":16214,"Ġdefining":16215,"Ġordering":16216,"Ġimmun":16217,"Ġpersistent":16218,"ACH":16219,"Russian":16220,"mans":16221,"Ġhind":16222,"Ġphotography":16223,"©":16224,"Ġhug":16225,"Ġ107":16226,"ĠHence":16227,"iots":16228,"udeau":16229,"Ġsubsidies":16230,"Ġroutinely":16231,"ĠDevice":16232,"itic":16233,"Ġdisgust":16234,"lander":16235,"Ġ1940":16236,"Ġassignment":16237,"ĠBesides":16238,"wick":16239,"ĠDust":16240,"usc":16241,"structed":16242,"111":16243,"develop":16244,"Ġfond":16245,"Ġintersection":16246,"Ġdignity":16247,"Ġcommissioner":16248,"Without":16249,"reach":16250,"Ġcartoon":16251,"Ġscales":16252,"ãĥŃ":16253,"FIG":16254,"Ġsurveys":16255,"ĠIndonesia":16256,"Ġartwork":16257,"Ġunch":16258,"Ġcycling":16259,"unct":16260,"auer":16261,"orate":16262,"ĠObviously":16263,"Ġcharacterized":16264,"feld":16265,"Ġaffirm":16266,"Ġinnings":16267,"Ġé":16268,"Ġaliens":16269,"Ġcloth":16270,"etooth":16271,"ĠCertain":16272,"§":16273,"Ġdigest":16274,"know":16275,"ĠXL":16276,"Ġpredictions":16277,"Ġdin":16278,"WAR":16279,"Ġaftermath":16280,"Example":16281,"ĠSuccess":16282,"ĠThr":16283,"IGN":16284,"Ġminer":16285,"Bus":16286,"Ġclarity":16287,"heimer":16288,"ĠOUT":16289,"ĠSend":16290,"ĠCircle":16291,"ĠDiet":16292,"Ġpronounced":16293,"Ġcreators":16294,"Ġearthquake":16295,"attery":16296,"geons":16297,"Ġod":16298,"Ġlaying":16299,"orp":16300,"Ult":16301,"project":16302,"Ġundermin":16303,"Ġsequel":16304,"Sam":16305,"ĠDarkness":16306,"Ġreception":16307,"bull":16308,"YS":16309,"ĠVir":16310,"Ġsequences":16311,"ĠCoin":16312,"Ġoutfit":16313,"ĠWait":16314,"119":16315,"Ġdelivers":16316,"......":16317,"Ġblown":16318,"ĠEsc":16319,"ĠMath":16320,"perm":16321,"ĠUl":16322,"Ġglim":16323,"Ġfacial":16324,"Ġgreenhouse":16325,"Ġtokens":16326,"/-":16327,"ĠAnnual":16328,"ĠONE":16329,"Ġteenage":16330,"ĠPhysical":16331,"ĠLang":16332,"ĠCelt":16333,"Ġsued":16334,"ividually":16335,"Ġpatience":16336,"chair":16337,"regular":16338,"Ġaug":16339,"inv":16340,"except":16341,"ĠLil":16342,"Ġnest":16343,"fd":16344,"sum":16345,"ĠChase":16346,"Russia":16347,"ĠJennifer":16348,"Ġoffseason":16349,"Overall":16350,"Fore":16351,"Ġriot":16352,"Aud":16353,"former":16354,"Ġdefenders":16355,"ĠCT":16356,"iotic":16357,"ribly":16358,"Ġautomated":16359,"Ġpenis":16360,"Ġinsist":16361,"Ġdiagram":16362,"ĠSQL":16363,"ĠGarc":16364,"Ġwitch":16365,"client":16366,"ierra":16367,"ambers":16368,"Ġrecount":16369,"far":16370,"Very":16371,"osterone":16372,"Ġappreciated":16373,"ĠPerfect":16374,"Section":16375,"Ġdoses":16376,"ocaust":16377,"Ġcostly":16378,"Ġgrams":16379,"ĠShi":16380,"Ġwrestling":16381,"Ġ1971":16382,"Ġtrophy":16383,"Ġnerve":16384,"ĠKaz":16385,"ĠExperience":16386,"Ġpledged":16387,"Ġplayback":16388,"Ġcreativity":16389,"bye":16390,"Ġattackers":16391,"Ġholders":16392,"ĠCoach":16393,"ĠPhD":16394,"Ġtransfers":16395,"Ġcolored":16396,"ĠHindu":16397,"Ġdrown":16398,"Ġlistened":16399,"ĠWA":16400,"iasm":16401,"PO":16402,"Ġappealing":16403,"Ġdisclosed":16404,"ĠChicken":16405,"agging":16406,"Ġpleaded":16407,"Ġnavigation":16408,"ĠReturns":16409,"Ġ[[":16410,"ROR":16411,"EA":16412,"Ġphotographer":16413,"ĠRider":16414,"ippers":16415,"Ġslice":16416,"Ġerect":16417,"Ġhed":16418,"issance":16419,"ĠVikings":16420,"urious":16421,"Ġappet":16422,"oubtedly":16423,"Child":16424,"Ġauthentic":16425,"oos":16426,"ĠMaking":16427,"Ġannouncing":16428,"Ġbod":16429,"Ġmeter":16430,"ĠNine":16431,"ĠRogue":16432,"Ġworkforce":16433,"Ġrenewed":16434,"Ġorganisations":16435,"acs":16436,"PLE":16437,"Short":16438,"Ġcompounds":16439,"ĠVisit":16440,"Ġenvelop":16441,"earth":16442,"Ġsupportive":16443,"ggle":16444,"ĠBrussels":16445,"ĠGuild":16446,"Create":16447,"REL":16448,"Ġaveraged":16449,"Ġ1969":16450,"riages":16451,"Ġlengthy":16452,"Ġforgot":16453,"Okay":16454,"ĠErd":16455,"Ġdealer":16456,"Ġrecession":16457,"DD":16458,"Ġdesperately":16459,"Ġhunger":16460,"Ġsticks":16461,"Ġmph":16462,"ĠFaith":16463,"Ġintentionally":16464,"Ġdemol":16465,"ueller":16466,"ĠSale":16467,"Ġdebris":16468,"spring":16469,"Ġleap":16470,">>>>":16471,"Ġcontainers":16472,"selling":16473,"ranean":16474,"attering":16475,"Ġcommented":16476,"ĠCM":16477,"onut":16478,"Ġwoods":16479,"especially":16480,"Ġorganize":16481,"ivic":16482,"ĠWoods":16483,"anga":16484,"squ":16485,"Ġmaj":16486,"amon":16487,"Ġaxis":16488,"Ġ1974":16489,"ĠDenmark":16490,"Ġwarrior":16491,"ĠPand":16492,"Ġoutlined":16493,"ĠBO":16494,"insula":16495,"zilla":16496,"ebook":16497,"Ġdare":16498,"Ġsearched":16499,"Ġnavigate":16500,"Sn":16501,"writing":16502,"Ġunited":16503,"Japan":16504,"ĠHebrew":16505,"Ġflame":16506,"Ġrelies":16507,"Ġcatching":16508,"ĠSho":16509,"Ġimprisonment":16510,"Ġpockets":16511,"Ġclosure":16512,"ĠFam":16513,"tim":16514,"adequ":16515,"Activity":16516,"Ġrecruiting":16517,"ĠWATCH":16518,"ĠArgentina":16519,"dest":16520,"Ġapologize":16521,"oro":16522,"Ġlacks":16523,"Ġtuned":16524,"ĠGriffin":16525,"Ġinfamous":16526,"Ġcelebrity":16527,"sson":16528,"Ġ----------------------------------------------------------------":16529,"ĠIsis":16530,"ĠDisplay":16531,"Ġcredibility":16532,"Ġeconomies":16533,"Ġheadline":16534,"ĠCowboys":16535,"Ġindef":16536,"Ġlately":16537,"Ġincentives":16538,"button":16539,"ĠMob":16540,"Aut":16541,"Ġresigned":16542,"ĠOm":16543,"camp":16544,"Ġprofiles":16545,"Ġschemes":16546,"olphins":16547,"ayed":16548,"Clinton":16549,"enh":16550,"ĠYahoo":16551,"Ġabst":16552,"Ġank":16553,"suits":16554,"Ġwished":16555,"ĠMarco":16556,"udden":16557,"Ġsphere":16558,"ĠBishop":16559,"Ġincorporated":16560,"ĠPlant":16561,"114":16562,"Ġhated":16563,"pic":16564,"Ġdonate":16565,"Ġlined":16566,"Ġbeans":16567,"Ġstealing":16568,"Ġcostume":16569,"Ġsheriff":16570,"Ġforty":16571,"Ġintact":16572,"Ġadapted":16573,"Ġtravelling":16574,"bart":16575,"Ġnicely":16576,"Ġdried":16577,"Ġscal":16578,"osity":16579,"NOTE":16580,"ĠBh":16581,"ĠBroncos":16582,"ĠIgn":16583,"Ġintimate":16584,"Ġchemistry":16585,"Ġoptimal":16586,"Deb":16587,"ĠGeneration":16588,"Ġ],":16589,"ichi":16590,"ĠWii":16591,"ĠYOUR":16592,"ventions":16593,"Write":16594,"Ġpopul":16595,"unning":16596,"ĠWor":16597,"Vol":16598,"Ġqueen":16599,"heads":16600,"KK":16601,"Ġanalyze":16602,"opic":16603,"earchers":16604,"Ġdot":16605,"legraph":16606,"astically":16607,"Ġupgrades":16608,"Ġcares":16609,"Ġextending":16610,"Ġfreeze":16611,"Ġinability":16612,"Ġorgans":16613,"Ġpretend":16614,"Ġoutlet":16615,"113":16616,"olan":16617,"ĠMall":16618,"uling":16619,"talk":16620,"Ġexpressing":16621,"ĠAlways":16622,"ĠBegin":16623,"files":16624,"Ġlicenses":16625,"%%":16626,"ĠMitt":16627,"Ġfilters":16628,"ĠMilwaukee":16629,"GN":16630,"Ġunfold":16631,"Mo":16632,"Ġnutrition":16633,"ppo":16634,"Bo":16635,"Ġfounding":16636,"Ġundermine":16637,"Ġeasiest":16638,"ĠCzech":16639,"ĠMack":16640,"Ġsexuality":16641,"ĠNixon":16642,"Win":16643,"ĠArn":16644,"ĠKin":16645,"ãĤ£":16646,"icer":16647,"Ġfortun":16648,"Ġsurfaces":16649,"aghd":16650,"Ġcarriers":16651,"ĠPART":16652,"ĠTib":16653,"Ġinterval":16654,"Ġfrustrating":16655,"ĠShip":16656,"ĠArmed":16657,"ffe":16658,"Ġboats":16659,"ĠAbraham":16660,"inis":16661,"Ġsuited":16662,"thread":16663,"iov":16664,"abul":16665,"ĠVenezuela":16666,"Ġtom":16667,"super":16668,"Ġcastle":16669,"although":16670,"ioxide":16671,"eches":16672,"Ġevolutionary":16673,"Ġnegotiate":16674,"Ġconfronted":16675,"Remember":16676,"Ġ170":16677,"Such":16678,"Ġ911":16679,"mult":16680,"ĠAbyss":16681,"urry":16682,"kees":16683,"spec":16684,"ĠBarbara":16685,"Ġbelonging":16686,"Ġvillain":16687,"istani":16688,"Ġaccountable":16689,"Ġportions":16690,"ĠDecl":16691,"Ur":16692,"ĠKate":16693,"gre":16694,"Ġmagazines":16695,"UCK":16696,"Ġregulate":16697,"omon":16698,"ĠAlmost":16699,"Ġoverview":16700,"Ġscram":16701,"Ġloot":16702,"ĠFitz":16703,"Ġcharacteristic":16704,"ĠSnake":16705,"say":16706,"ĠRico":16707,"Ġtrait":16708,"ĠJoined":16709,"aucus":16710,"Ġadaptation":16711,"ĠAirlines":16712,"Ġarchae":16713,"ĠIde":16714,"Ġbikes":16715,"Ġliterary":16716,"Ġinfluences":16717,"ĠUsed":16718,"Creat":16719,"Ġplea":16720,"ĠDefence":16721,"ĠAssass":16722,"Ġpond":16723,"ULT":16724,")\"":16725,"Ġevaluated":16726,"Ġobtaining":16727,"Ġdemographic":16728,"Ġvigil":16729,"aley":16730,"Ġspouse":16731,"ĠSeahawks":16732,"respons":16733,"ĠBelt":16734,"umatic":16735,"Ġrises":16736,"runner":16737,"ĠMichelle":16738,"Ġpotent":16739,"race":16740,"ĠPAC":16741,"Find":16742,"olesterol":16743,"ISS":16744,"ĠIntroduced":16745,"resses":16746,"ignment":16747,"Os":16748,"ĠTu":16749,"ĠDex":16750,"icides":16751,"Ġsparked":16752,"ĠLaura":16753,"ĠBryant":16754,"Ġsmiling":16755,"ĠNexus":16756,"Ġdefendants":16757,"ĠCatal":16758,"Ġdishes":16759,"shaped":16760,"Ġprolong":16761,"mt":16762,"($":16763,"ãĢĤ":16764,"Ġcalculations":16765,"ĠSame":16766,"Ġpiv":16767,"HH":16768,"Ġcancelled":16769,"Ġgrin":16770,"Ġterritories":16771,"istically":16772,"Come":16773,"ĠParent":16774,"Project":16775,"Ġneglig":16776,"ĠPrivacy":16777,"Ġammo":16778,"LECT":16779,"olutely":16780,"ĠEpic":16781,"Ġmisunder":16782,"wal":16783,"April":16784,"mos":16785,"pathy":16786,"ĠCarson":16787,"Ġalbums":16788,"ĠEasy":16789,"Ġpistol":16790,"<<":16791,"Ġ\\(":16792,"target":16793,"help":16794,"Ġinterpre":16795,"conscious":16796,"ĠHousing":16797,"ĠJoint":16798,"127":16799,"Ġbeers":16800,"science":16801,"ĠFirefox":16802,"effective":16803,"ĠCabin":16804,"ĠOkay":16805,"ĠApplic":16806,"Ġspacecraft":16807,"ĠSR":16808,"vet":16809,"ĠStrange":16810,"SB":16811,"Ġcorps":16812,"iberal":16813,"efficient":16814,"Ġprevalence":16815,"Ġeconomists":16816,"118":16817,"Thread":16818,"ordable":16819,"ODE":16820,"ĠCant":16821,"=-=-":16822,"ifiable":16823,"ĠAround":16824,"Ġpole":16825,"Ġwillingness":16826,"CLA":16827,"ĠKid":16828,"Ġcomplement":16829,"Ġscattered":16830,"Ġinmates":16831,"Ġbleeding":16832,"every":16833,"Ġqueue":16834,"ĠTrain":16835,"Ġhij":16836,"Ġmelee":16837,"pleted":16838,"Ġdigit":16839,"Ġgem":16840,"official":16841,"Ġlifting":16842,"е":16843,"Requ":16844,"itutes":16845,"Ġpackaging":16846,"ĠWorkers":16847,"hran":16848,"ĠLebanon":16849,"olesc":16850,"Ġpunished":16851,"ĠJuan":16852,"Ġjam":16853,"ĠDocument":16854,"Ġmapping":16855,"icates":16856,"Ġinevitably":16857,"Ġvanilla":16858,"ĠTon":16859,"Ġwatches":16860,"Ġleagues":16861,"Ġinitiated":16862,"degree":16863,"portion":16864,"Ġrecalls":16865,"Ġruin":16866,"Ġmelt":16867,"IAN":16868,"Ġhem":16869,"Exp":16870,"Ġbaking":16871,"ĠColomb":16872,"atible":16873,"Ġradius":16874,"plug":16875,"ĠIF":16876,"etically":16877,"Ġfict":16878,"HER":16879,"ĠTap":16880,"atinum":16881,"Ġink":16882,"Ġcoh":16883,"ĠWizard":16884,"both":16885,"tex":16886,"Ġspends":16887,"ĠCurrently":16888,"ĠPit":16889,"Ġneurons":16890,"ignt":16891,"Ġrall":16892,"Ġbuses":16893,"building":16894,"Ġadjustments":16895,"Ġcried":16896,"iblical":16897,"atted":16898,"ĠZion":16899,"ĠMatter":16900,"Ġmeditation":16901,"ĠDennis":16902,"Ġours":16903,"ĠTab":16904,"Ġrankings":16905,"ortal":16906,"Ġadvers":16907,"Ġsurrender":16908,"ĠGob":16909,"cium":16910,"omas":16911,"imeter":16912,"Ġmultiplayer":16913,"Ġheroin":16914,"Ġoptimistic":16915,"Ġindicator":16916,"ĠBrig":16917,"Ġgrocery":16918,"Ġapplicant":16919,"ĠRocket":16920,"vid":16921,"Exception":16922,"pent":16923,"Ġorganizing":16924,"Ġencounters":16925,"ĠTOD":16926,"Ġjewel":16927,"Save":16928,"ĠChristie":16929,"Ġheating":16930,"Ġlazy":16931,"ĠCP":16932,"Ġcousin":16933,"Config":16934,"Ġregener":16935,"Ġnearest":16936,"Ġachieving":16937,"ENS":16938,"throw":16939,"ĠRichmond":16940,"antle":16941,"2002":16942,"Ġanten":16943,"bird":16944,"133":16945,"Ġnarc":16946,"raint":16947,"unny":16948,"ĠHispanic":16949,"ournaments":16950,"Ġprophe":16951,"ĠThailand":16952,"ĠTi":16953,"Ġinjection":16954,"Ġinherit":16955,"ravis":16956,"Ġmedi":16957,"Ġwhoever":16958,"ĠDEBUG":16959,"GP":16960,"ĠHud":16961,"Card":16962,"prom":16963,"Ġpor":16964,"Ġoverhead":16965,"Law":16966,"Ġviolate":16967,"Ġheated":16968,"Ġdescriptions":16969,"Ġachievements":16970,"ĠBeer":16971,"ĠQuant":16972,"Was":16973,"Ġeighth":16974,"ĠIv":16975,"Ġspecialized":16976,"UPDATE":16977,"ĠDelta":16978,"Pop":16979,"Jul":16980,"ĠAsk":16981,"ophy":16982,"Ġnewsletters":16983,"ĠTool":16984,"Ġgard":16985,"ĠConfeder":16986,"ĠGMT":16987,"ĠAbbott":16988,"Ġimmunity":16989,"ĠVM":16990,"Islam":16991,"Ġimplicit":16992,"wd":16993,"Ġ1944":16994,"ravity":16995,"ometric":16996,"Ġsurviving":16997,"urai":16998,"ĠPrison":16999,"Ġrust":17000,"ĠSketch":17001,"Ġbees":17002,"ĠTheory":17003,"Ġmerit":17004,"Tex":17005,"chat":17006,"Ġmim":17007,"Ġpaste":17008,"ĠKoch":17009,"Ġignorance":17010,"ĠShoot":17011,"Ġbasement":17012,"United":17013,"ĠAdvis":17014,"height":17015,"Ġfoster":17016,"Ġdetain":17017,"information":17018,"Ġneural":17019,"';":17020,"Ġproves":17021,"allery":17022,"Ġinvitation":17023,"umbers":17024,"Ġcattle":17025,"Ġbicycle":17026,"zi":17027,"Ġconsultant":17028,"Ġapology":17029,"ĠTiger":17030,"Ġ123":17031,"999":17032,"Ġindividually":17033,"rt":17034,"igion":17035,"ĠBrazilian":17036,"Ġdisturb":17037,"Ġentrepreneurs":17038,"Ġforests":17039,"cerpt":17040,"plates":17041,"pher":17042,"clipse":17043,"Ġtwitter":17044,"Ġacids":17045,"ographical":17046,"hum":17047,"ĠBald":17048,"ifully":17049,"Ġcompiler":17050,"ĠDA":17051,"Ġdonor":17052,"asi":17053,"Ġtribal":17054,"lash":17055,"ĠConfig":17056,"Ġapplicants":17057,"Ġsalaries":17058,"135":17059,"Putin":17060,"ĠFocus":17061,"irs":17062,"Ġmisconduct":17063,"ĠHaz":17064,"Ġeaten":17065,"Mobile":17066,"Muslim":17067,"ĠMarcus":17068,"viol":17069,"Ġfavorable":17070,"Ġstub":17071,"adin":17072,"ĠHob":17073,"Ġfaithful":17074,"Ġelectronics":17075,"Ġvacuum":17076,"wait":17077,"backed":17078,"economic":17079,"dist":17080,"Ġtenure":17081,"Ġsincere":17082,"ĠTogether":17083,"ĠWave":17084,"Ġprogression":17085,"Ġdenying":17086,"Ġdistress":17087,"braska":17088,"third":17089,"Ġmixing":17090,"Ġcolonial":17091,"Ġprivately":17092,"Ġunrest":17093,"aternity":17094,"Ġpremises":17095,"anti":17096,"gregation":17097,"Ġlicence":17098,"ĠHind":17099,"ĠSamuel":17100,"Ġconvincing":17101,"ĠAce":17102,"ĠRust":17103,"ĠNetanyahu":17104,"Ġhandles":17105,"ĠPatch":17106,"oriented":17107,"aho":17108,"ĠGonz":17109,"Ġhackers":17110,"claimer":17111,"Ġcustoms":17112,"ĠGran":17113,"fighters":17114,"Ġluc":17115,"Ġmanuscript":17116,"arenthood":17117,"Ġdevil":17118,"Ġwarriors":17119,"Ġoffenders":17120,"William":17121,"Ġholidays":17122,"Ġnightmare":17123,"Ġlever":17124,"ifferent":17125,"Stat":17126,"Ġexhibition":17127,"puted":17128,"ĠPure":17129,"Ġalpha":17130,"Ġenthusiasm":17131,"ĠRepresentatives":17132,"EAR":17133,"ĠTyp":17134,"Ġwheat":17135,"ĠAlf":17136,"Ġcorrection":17137,"Ġevangel":17138,"ATT":17139,"Miss":17140,"Ġsoup":17141,"Ġimplied":17142,"param":17143,"Ġsexy":17144,"ĠLux":17145,"Ġrepublic":17146,"patch":17147,"ablish":17148,"Ġicons":17149,"Ġfathers":17150,"ĠGET":17151,"ĠCarib":17152,"Ġregulated":17153,"ĠCohen":17154,"ĠBobby":17155,"Ġner":17156,"Ġbent":17157,"ventory":17158,"ĠAlong":17159,"ĠEST":17160,"ĠWallace":17161,"Ġmurders":17162,"rise":17163,"kell":17164,"ĠCommonwealth":17165,"Ġnasty":17166,"eta":17167,"ĠMIT":17168,"Ġadministered":17169,"Ġgenuinely":17170,"Editor":17171,"nick":17172,"Ġhydro":17173,"********************************":17174,"ĠBle":17175,"Ġfines":17176,"Ġgorge":17177,"ausible":17178,"rh":17179,"Ġapple":17180,"mentioned":17181,"Ġrope":17182,"otyp":17183,"HR":17184,"Ġdisappointing":17185,"Ġcage":17186,"nik":17187,"Ġdoubts":17188,"ĠFREE":17189,"prints":17190,"ĠMUST":17191,"Ġvendors":17192,"ĠInqu":17193,"Ġliberals":17194,"Ġcontractor":17195,"Ġupside":17196,"children":17197,"Ġtricky":17198,"Ġregulators":17199,"charged":17200,"liter":17201,"Ġ***":17202,"Ġrebell":17203,"lang":17204,"Ġlocals":17205,"Ġphysicians":17206,"Ġhey":17207,"arse":17208,"tm":17209,"ĠLex":17210,"Ġbehavioral":17211,"successful":17212,"FX":17213,"Ġbrick":17214,"ovic":17215,"Ġconform":17216,"Ġreviewing":17217,"Ġinsights":17218,"Ġbiology":17219,"ĠRemove":17220,"ĠExtra":17221,"Ġcommitting":17222,"induced":17223,"ignty":17224,"igm":17225,"Ġatomic":17226,"Common":17227,"ĠEM":17228,"ĠPere":17229,"ĠItems":17230,"eh":17231,"Ġpreserved":17232,"ĠHood":17233,"Ġprisoner":17234,"Ġbankruptcy":17235,"Ġgren":17236,"ushes":17237,"Ġexploitation":17238,"Ġsignatures":17239,"Ġfinan":17240,"],\"":17241,"ĠMR":17242,"Ġmeg":17243,"remlin":17244,"Ġmusicians":17245,"Ġselecting":17246,"Ġexamining":17247,"INK":17248,"lated":17249,"Hi":17250,"Ġartic":17251,"Ġpets":17252,"Ġimpair":17253,"ĠMAN":17254,"Ġtablets":17255,"include":17256,"Range":17257,"Ġcaut":17258,"Ġlogs":17259,"Ġmounting":17260,"Ġunaware":17261,"Ġdynamics":17262,"ĠPalestine":17263,"ĠQuarter":17264,"ĠPurple":17265,"Ġma":17266,"ĠImport":17267,"Ġcollections":17268,"ciation":17269,"Ġsuccessor":17270,"Ġclone":17271,"Ġaiming":17272,"Ġpossessed":17273,"Ġsticking":17274,"Ġshaking":17275,"Ġlocate":17276,"ĠHockey":17277,"Turn":17278,"170":17279,"Ġfifteen":17280,"ĠHarrison":17281,"Ġcontinuously":17282,"ĠTC":17283,"ĠValent":17284,"ĠRescue":17285,"Ġbypass":17286,"amount":17287,"Ġmast":17288,"Ġprotects":17289,"Ġartistic":17290,"Ġsometime":17291,"Ġshoe":17292,"Ġshouted":17293,"ificant":17294,"etitive":17295,"ĠRegister":17296,"ĠJin":17297,"Ġconcentrated":17298,"lington":17299,"onies":17300,"Ġgenerator":17301,"yrim":17302,"ĠArmen":17303,"Ġclearing":17304,"ido":17305,"ĠTW":17306,"alph":17307,"Ġladies":17308,"Hard":17309,"Ġdialog":17310,"Ġinputs":17311,"æľ":17312,"Ġposes":17313,"Ġslots":17314,"ĠPremium":17315,"Ġleaks":17316,"Ġbosses":17317,"Ġ113":17318,"course":17319,"Acc":17320,"ĠNewton":17321,"ĠAustria":17322,"ĠMage":17323,"Ġteaches":17324,"abad":17325,"Ġwears":17326,"Ġcyl":17327,"Ġcurse":17328,"ĠSales":17329,"ĠWings":17330,"Ġpsy":17331,"Ġgaps":17332,"ĠIceland":17333,"ĠPinterest":17334,"Ġlandlord":17335,"Ġdefinitions":17336,"ĠKer":17337,"Ġsufficiently":17338,"ĠPence":17339,"ĠArchitect":17340,"Ġsurpass":17341,"Ġ114":17342,"Ġsuperhero":17343,"ĠDisease":17344,"Ġpriests":17345,"ĠCulture":17346,"Ġdefinitive":17347,"Ġsecretly":17348,"ĠDance":17349,"install":17350,"chief":17351,"ĠJessica":17352,"Would":17353,"Updated":17354,"Ġlocker":17355,"ĠKay":17356,"Ġmemorial":17357,"è¦":17358,"fat":17359,"Ġdisgu":17360,"Ġflavors":17361,"ĠBaseball":17362,"ĠResistance":17363,"Ġkicks":17364,"Ġenv":17365,"Ġteenagers":17366,"Dark":17367,"ĠCAR":17368,"Ġhalt":17369,"ĠLG":17370,"ĠGabriel":17371,"Ġfever":17372,"Ġsatur":17373,"Ġmall":17374,"Ġaffiliate":17375,"ĠSleep":17376,"ĠSpecific":17377,"ĠVel":17378,"Ġjar":17379,"ĠSacred":17380,"ĠEdwards":17381,"ĠACL":17382,"Ġretained":17383,"ĠGiant":17384,"Ġlimitation":17385,"inces":17386,"Ġrefusal":17387,"ĠTale":17388,"ĠButler":17389,"Ġaccidents":17390,"ĠCSS":17391,"Ġimported":17392,"ĠCopy":17393,"α":17394,"ERT":17395,"zel":17396,"Ġdivisions":17397,"hots":17398,"ĠAlb":17399,"ĠDS":17400,"Loader":17401,"Washington":17402,"atisf":17403,"ĠCreative":17404,"\\.":17405,"ĠAutom":17406,"redict":17407,"Ġreceptor":17408,"ĠCarlos":17409,"Method":17410,"oka":17411,"Ġmalicious":17412,"Ġstepping":17413,",[":17414,"ĠDad":17415,"Ġattraction":17416,"ĠEffects":17417,"ĠPirate":17418,"ĠCer":17419,"ĠIndustry":17420,"ĠRud":17421,"Ġcharter":17422,"Ġdining":17423,"Ġinsists":17424,"Ġconfigure":17425,"Ġ(#":17426,"ĠSimple":17427,"ĠScroll":17428,"UTC":17429,"175":17430,"ĠKon":17431,"Ġmarketplace":17432,"ĠãĤ":17433,"Ġrefres":17434,"Ġgates":17435,"erred":17436,"ĠPod":17437,"Ġbehave":17438,"Frank":17439,"node":17440,"Ġendorsed":17441,"hett":17442,"asive":17443,"ĠHomeland":17444,"Ġrides":17445,"ĠLeave":17446,"erness":17447,"Ġflooding":17448,"AFP":17449,"Ġrisen":17450,"Ġcontinually":17451,"Ġunanim":17452,"ĠContract":17453,"ĠPas":17454,"Ġguided":17455,"ĠChile":17456,"bd":17457,"Ġsucc":17458,"ptic":17459,"Ġcommittees":17460,"ĠLuther":17461,"ĠAnyone":17462,"Ġsab":17463,"124":17464,"Ġpixel":17465,"ĠBak":17466,"ĠTag":17467,"ĠBennett":17468,"Enter":17469,"small":17470,"ĠPresidential":17471,"Ġpul":17472,"Ġcontrace":17473,"archive":17474,"Ġcoastal":17475,"ĠKids":17476,"192":17477,"â̲":17478,"icky":17479,"INGTON":17480,"Ġwolf":17481,"ĠStalin":17482,"Tur":17483,"idget":17484,"amas":17485,"ĠUnless":17486,"Ġsponsor":17487,"Ġmorph":17488,"ĠChoose":17489,"Ġrunner":17490,"Ġunbel":17491,"Ġmud":17492,"ĠMana":17493,"Ġdubbed":17494,"Ġgodd":17495,"urers":17496,"window":17497,"Ġrelied":17498,"Ġcelebrating":17499,"osc":17500,"Ġ135":17501,"Ġlobbying":17502,"Ġincomplete":17503,"Ġrestriction":17504,"Ġincap":17505,"itus":17506,"Ġexpectation":17507,"ĠApollo":17508,"Ġintens":17509,"Ġsync":17510,"GH":17511,"Ġmanipulation":17512,"BY":17513,"Ġspear":17514,"Ġbreasts":17515,"Ġvolcan":17516,"ilia":17517,"Material":17518,"Ġformats":17519,"ĠBast":17520,"Ġparliamentary":17521,"Ġsnake":17522,"Ġservants":17523,"ĠTrudeau":17524,"ĠGrim":17525,"ĠArabic":17526,"ĠSCP":17527,"ĠBoys":17528,"station":17529,"Ġprospective":17530,"orde":17531,"initialized":17532,"Ġbored":17533,"ABLE":17534,"Ġaccessed":17535,"Ġtaxi":17536,"ĠShell":17537,"aiden":17538,"ursed":17539,"inates":17540,"ĠInsurance":17541,"ĠPete":17542,"September":17543,"650":17544,"Ġadventures":17545,"ĠCover":17546,"Ġtribute":17547,"Ġsketch":17548,"Ġempower":17549,"ĠØ":17550,"ĠGlenn":17551,"ĠDaw":17552,"=\\\"":17553,"ĠPolitics":17554,"Ġguides":17555,"Ġdioxide":17556,"ĠGore":17557,"ĠBright":17558,"ĠSierra":17559,"Ġvalued":17560,"cond":17561,"Ġpointer":17562,"Select":17563,"Ġrisky":17564,"Ġabsorb":17565,"images":17566,"Ġrefuses":17567,"Ġbonuses":17568,"___":17569,"Ġhilar":17570,"ĠFeatures":17571,"220":17572,"ĠCollector":17573,"Foot":17574,"Ġ1964":17575,"culus":17576,"Ġdawn":17577,"Ġworkout":17578,"ĠLO":17579,"Ġphilosophical":17580,"ĠSandy":17581,"ĠYouth":17582,"Ġliable":17583,"Af":17584,"blue":17585,"Ġoverturn":17586,"lessness":17587,"ĠTribune":17588,"ĠIng":17589,"Ġfactories":17590,"Ġcatches":17591,"Ġprone":17592,"Ġmatrix":17593,"Ġlogin":17594,"Ġinacc":17595,"Ġexert":17596,"sys":17597,"Ġneedle":17598,"ĠQur":17599,"Ġnotified":17600,"oulder":17601,"tx":17602,"Ġreminds":17603,"Ġpublishers":17604,"Ġnort":17605,"Ġgit":17606,"Ġflies":17607,"ĠEmily":17608,"Ġflowing":17609,"ĠAlien":17610,"ĠStrateg":17611,"Ġhardest":17612,"Ġmodification":17613,"API":17614,"ĠMY":17615,"Ġcrashes":17616,"stairs":17617,"number":17618,"Ġurging":17619,"channel":17620,"ĠFalcon":17621,"Ġinhabitants":17622,"Ġterrifying":17623,"Ġutilize":17624,"Ġbanner":17625,"Ġcigarettes":17626,"Ġsenses":17627,"ĠHolmes":17628,"Ġpractition":17629,"ĠPhillips":17630,"otto":17631,"Ġcompile":17632,"Model":17633,"ĠKo":17634,"Ġ[]":17635,"Americans":17636,"ĠTerms":17637,"Ġmedications":17638,"ĠAna":17639,"Ġfundamentally":17640,"ĠNotice":17641,"Ġweaker":17642,"Ġ0000":17643,"Ġgarlic":17644,"Ġoutbreak":17645,"Ġeconomist":17646,"ĠBirth":17647,"Ġobstacles":17648,"arcer":17649,"ĠOrthodox":17650,"Ġplacebo":17651,"ĠCrew":17652,"aspberry":17653,"ĠAngels":17654,"Ġdischarge":17655,"Ġdestructive":17656,"117":17657,"ĠRising":17658,"Ġdairy":17659,"late":17660,"Ġcollision":17661,"ĠTigers":17662,"eanor":17663,"ocumented":17664,"ĠInvalid":17665,"Ġdont":17666,"ĠLiter":17667,"ĠVa":17668,"Ġhydrogen":17669,"Ġvariants":17670,"ĠBrowns":17671,"Ġ1965":17672,"Ġindigenous":17673,"Ġtrades":17674,"Ġremainder":17675,"Ġswept":17676,"ĠImpact":17677,"Ġredist":17678,"Ġunint":17679,"graduate":17680,"ãĥķ":17681,"ĠWILL":17682,"ãģ®ç":17683,"ĠCritical":17684,"Ġfisher":17685,"Ġvicious":17686,"Ġreversed":17687,"Year":17688,"ĠSox":17689,"Ġshootings":17690,"Ġfilming":17691,"Ġtouchdowns":17692,"aires":17693,"mel":17694,"Ġgrandfather":17695,"Ġaffection":17696,"ingle":17697,"Ġoverly":17698,"Additional":17699,"Ġsupreme":17700,"ĠGrad":17701,"Ġsporting":17702,"Ġmercy":17703,"ĠBrooks":17704,"ounty":17705,"Ġperforms":17706,"Ġtightly":17707,"Ġdemons":17708,"Ġkillings":17709,"Ġfaction":17710,"ĠNova":17711,"auts":17712,"Ġundoubtedly":17713,"arin":17714,"Ġunderway":17715,"rak":17716,"Ġliv":17717,"ĠRegion":17718,"Ġbriefing":17719,"sers":17720,"cloud":17721,"ĠMik":17722,"usp":17723,"Ġprediction":17724,"azor":17725,"Ġportable":17726,"ĠGand":17727,"Ġpresenting":17728,"Ġ1080":17729,"»":17730,"ushi":17731,"ĠSpark":17732,"thereum":17733,"Ġjustification":17734,"ĠNy":17735,"Ġcontractors":17736,"mingham":17737,"ĠStyle":17738,"åħ":17739,"ĠChronicles":17740,"ĠPicture":17741,"Ġproving":17742,"Ġwives":17743,"sett":17744,"Ġmolecules":17745,"ĠFairy":17746,"Ġconsisting":17747,"Ġpier":17748,"alone":17749,"inition":17750,"Ġnucle":17751,"json":17752,"Ġgotta":17753,"Ġmobil":17754,"Ġverbal":17755,"arium":17756,"Ġmonument":17757,"ucked":17758,"Ġ256":17759,"Tech":17760,"minecraft":17761,"ĠTrack":17762,"Ġtile":17763,"Ġcompatibility":17764,"asis":17765,"Ġsadd":17766,"Ġinstructed":17767,"ĠMueller":17768,"Ġlethal":17769,"Ġhormone":17770,"Ġorche":17771,"else":17772,"Ġskelet":17773,"Ġentertaining":17774,"Ġminimize":17775,"again":17776,"Ġundergo":17777,"Ġconstraints":17778,"Ġcigarette":17779,"ĠIslamist":17780,"Ġtravels":17781,"ĠPanthers":17782,"lings":17783,"Care":17784,"Ġlawsuits":17785,"uras":17786,"Ġcryst":17787,"Ġlowered":17788,"Ġaerial":17789,"Ġcombinations":17790,"Ġhaun":17791,"Ġcha":17792,"Ġvine":17793,"Ġquantities":17794,"Ġlinking":17795,"bank":17796,"Ġsoy":17797,"Bill":17798,"ĠAngela":17799,"Ġrecipient":17800,"ĠProtest":17801,"Ġsocket":17802,"Ġsolidarity":17803,"ĠâĨ":17804,"mill":17805,"Ġvaries":17806,"ĠPakistani":17807,"Dragon":17808,"Ġune":17809,"Ġhorizon":17810,"³³³³³³³³":17811,"Ġprovinces":17812,"Ġfrankly":17813,"Ġenacted":17814,"notes":17815,"['":17816,"Ġ192":17817,"ocracy":17818,"Ġendorsement":17819,"Ġovertime":17820,"True":17821,"Lab":17822,"licted":17823,"ĠDNC":17824,"Ġbeats":17825,"ĠJamie":17826,"152":17827,"ĠINT":17828,"Contact":17829,"Ġaccounted":17830,"hash":17831,"ĠPackers":17832,"pires":17833,"Ġlesbian":17834,"Ġamendments":17835,"Ġhopeful":17836,"ĠFinland":17837,"Ġspotlight":17838,"Ġconfigured":17839,"Ġtroubled":17840,"Ġgaze":17841,"ĠCalgary":17842,"Ġreliability":17843,"Ġinsurg":17844,"swer":17845,"buy":17846,"ĠSkin":17847,"Ġpixels":17848,"Ġhandgun":17849,"Ġparas":17850,"Ġcategor":17851,"ĠEL":17852,"ĠRex":17853,"Indeed":17854,"Ġkinda":17855,"Ġconjunction":17856,"ĠBryan":17857,"ĠManufact":17858,"yang":17859,"Plus":17860,"SQL":17861,"ishment":17862,"Ġdominate":17863,"Ġnail":17864,"Ġoath":17865,"Ġerupt":17866,"ĠFine":17867,"itbart":17868,"ĠChip":17869,"ĠAbd":17870,"ĠNam":17871,"Ġbuyer":17872,"Ġdissent":17873,"Leaks":17874,"Contin":17875,"Ġrider":17876,"ĠSomeone":17877,"Ġillusion":17878,"cin":17879,"ĠBoeing":17880,"Ġinadequ":17881,"ovation":17882,"iants":17883,"Ġrebuild":17884,"450":17885,"ĠDestiny":17886,"SW":17887,"ĠTill":17888,"Hit":17889,"iaz":17890,"ĠBangl":17891,"achers":17892,"ĠReform":17893,"Ġsegments":17894,"Ġsystematic":17895,"dc":17896,"ĠConservatives":17897,"Ġportal":17898,"hor":17899,"ĠDragonbound":17900,"Ġdragged":17901,"omo":17902,"Ġthee":17903,"advert":17904,"ĠReports":17905,"ĠEt":17906,"Ġbarrels":17907,"August":17908,"Ġcomparisons":17909,"Ġhex":17910,"Ġanthrop":17911,"\"[":17912,"borough":17913,"abi":17914,"Ġpictured":17915,"playing":17916,"ĠAddress":17917,"ĠMirror":17918,"Smith":17919,"Ġtires":17920,"ĠNPR":17921,"AAAA":17922,"Ġclassification":17923,"ĠThan":17924,"ĠHarm":17925,"ĠRA":17926,"Ġrejection":17927,"mination":17928,"Ġranged":17929,"ĠFalls":17930,"DI":17931,"Host":17932,"ãĤ´":17933,"ĠExample":17934,"listed":17935,"thirds":17936,"Ġsafegu":17937,"brand":17938,"Ġprobable":17939,"Canada":17940,"ITION":17941,"ĠQaeda":17942,"Ġchick":17943,"Ġimports":17944,"hit":17945,"loc":17946,"WW":17947,"Ġblew":17948,"Ġanytime":17949,"Ġwholes":17950,"iked":17951,"Ġcalculation":17952,"create":17953,"ĠOri":17954,"Ġupgraded":17955,"Ġappar":17956,"utory":17957,"ĠMol":17958,"Brit":17959,"ĠJong":17960,"INAL":17961,"ĠStarting":17962,"Ġdice":17963,"urtle":17964,"Ġrelying":17965,"closure":17966,"Ġprofitable":17967,"Ġslaughter":17968,"ĠManual":17969,"caster":17970,"Ġ\"$":17971,"Ġfeather":17972,"ĠSimply":17973,"ieves":17974,"Ġdeterior":17975,"ĠPCI":17976,"Ġstamp":17977,"Ġflaws":17978,"Ġshade":17979,"hammer":17980,"Ġpassport":17981,"Ġconting":17982,"amel":17983,"Ġobservers":17984,"Ġneglect":17985,"ĠRB":17986,"ĠBrotherhood":17987,"Ġskeptical":17988,"family":17989,"usk":17990,"Ġemotionally":17991,"âĻ":17992,"ĠBeta":17993,"asonable":17994,"idity":17995,"ĠMul":17996,"Ġkicking":17997,"ĠCarm":17998,"ollah":17999,"VERTIS":18000,"ĠAthen":18001,"Ġladder":18002,"ĠBullet":18003,"å£":18004,"0001":18005,"ĠWildlife":18006,"ĠMask":18007,"ĠNan":18008,"Rev":18009,"Ġunacceptable":18010,"legal":18011,"Ġcrowded":18012,"agi":18013,"ĠCox":18014,"je":18015,"Ġmorality":18016,"Ġfuels":18017,"Ġcables":18018,"Ġmankind":18019,"ĠCaribbean":18020,"Ġanchor":18021,"Ġbyte":18022,"ĠOften":18023,"ĠOz":18024,"Ġcrafted":18025,"Ġhistorian":18026,"ĠWu":18027,"Ġtowers":18028,"ĠCitizens":18029,"Ġhelm":18030,"Ġcredentials":18031,"Ġsingular":18032,"ĠJesse":18033,"Ġtackles":18034,"Ġcontempt":18035,"Ġafore":18036,"ĠShadows":18037,"Ġnil":18038,"Ġurgent":18039,"apple":18040,"blood":18041,"Ġvon":18042,"Ġoffline":18043,"Ġbreathe":18044,"Ġjumps":18045,"Ġirrelevant":18046,"oxic":18047,"omal":18048,"important":18049,"Jim":18050,"Ġgloves":18051,"arming":18052,"depth":18053,"Ġtalents":18054,"ookie":18055,"ĠSB":18056,"Ġpalm":18057,"uffs":18058,"esta":18059,"IGH":18060,"Ġcanon":18061,"ĠVerizon":18062,"ĠPle":18063,"Ġcoupled":18064,"velt":18065,"Ġfundraising":18066,"ĠGetting":18067,"ĠDLC":18068,"Ġmathematical":18069,"ĠHS":18070,"ĠCardinals":18071,"telling":18072,"Ġsponsors":18073,"ĠÏ":18074,"ĠBulls":18075,"option":18076,"Ġpropose":18077,"Ġmemorable":18078,"Ġembraced":18079,"Ġdeclining":18080,"Health":18081,"eda":18082,"Ġ};":18083,"Ġspam":18084,"mile":18085,"Ġpitcher":18086,"ĠEight":18087,"Ġcaring":18088,"utic":18089,"role":18090,"Ġairline":18091,"ernandez":18092,"ĠAthlet":18093,"Ġcertification":18094,"uxe":18095,"riger":18096,"Ġempir":18097,"Ġsensation":18098,"Ġdism":18099,"Ġbolt":18100,"Ġevolve":18101,"House":18102,"Ġconsultation":18103,"ĠDuty":18104,"Ġtouches":18105,"ĠNathan":18106,"Ġfaint":18107,"had":18108,"\"(":18109,"ĠConsumer":18110,"ĠExtreme":18111,"Ġ127":18112,"ĠHerm":18113,"ĠSacrament":18114,"izoph":18115,"Ġanxious":18116,"ulously":18117,"Ġsocially":18118,"ĠUTC":18119,"Ġsolving":18120,"ĠLetter":18121,"History":18122,"educ":18123,"Price":18124,"));":18125,"Ġreload":18126,"amic":18127,"Ġpork":18128,"Ġdiscourse":18129,"Ġtournaments":18130,"airo":18131,"ĠKur":18132,"ĠCosta":18133,"Ġviolating":18134,"Ġinterfere":18135,"Ġrecreational":18136,"uffle":18137,"Ġspeeches":18138,"Ġneeding":18139,"Ġremembers":18140,"Ġcredited":18141,"nia":18142,"focused":18143,"amera":18144,"Ġbru":18145,"umbs":18146,"ĠCuban":18147,"Ġpreceding":18148,"Ġnonsense":18149,"acial":18150,"Ġsmartphones":18151,"ĠStories":18152,"Sports":18153,"ĠEmergency":18154,"ouncing":18155,"efined":18156,"Ġber":18157,"Ġconsulting":18158,"Ġmasters":18159,"heastern":18160,".\"[":18161,"ĠRunning":18162,"Ġsuscept":18163,"ĠFeng":18164,"America":18165,"prises":18166,"stitial":18167,"ĠWeekly":18168,"ĠGreater":18169,"modules":18170,"ifter":18171,"Graphics":18172,"uler":18173,"Ġwholly":18174,"Ġsuppress":18175,"Ġconcealed":18176,"Ġhappily":18177,"Ġaccepts":18178,"ĠEnjoy":18179,"Ġrivers":18180,"ĠExcept":18181,"225":18182,"ĠNHS":18183,"ĠMcConnell":18184,"Ġpussy":18185,"ferred":18186,"utable":18187,"Ġattain":18188,"Ġ>=":18189,"Ġdeposits":18190,"rophic":18191,"Ġnotorious":18192,"ĠShaw":18193,"ilitation":18194,"Ġepidemic":18195,"allic":18196,"Ġsmallest":18197,"ovich":18198,"Ġaccessories":18199,"perties":18200,"Ġsurplus":18201,"ĠMech":18202,"Ġambig":18203,"ĠImmigration":18204,"Ġchim":18205,"eval":18206,"Ġpracticing":18207,"ĠMystery":18208,"Ġdomains":18209,"ĠSilicon":18210,"apps":18211,"Ġkilometers":18212,"ea":18213,"ĠSmash":18214,"Ġwarranty":18215,"Ġnost":18216,"sil":18217,"rev":18218,"Jon":18219,"ĠDublin":18220,"Ġtastes":18221,"Ġbout":18222,"great":18223,"error":18224,"Ġswitches":18225,"ĠBapt":18226,"DO":18227,"oki":18228,"Ġsourced":18229,"produ":18230,"Ġattachment":18231,"ĠIssue":18232,"ĠQuestion":18233,"Join":18234,"Ġfitted":18235,"Ġunlawful":18236,"^^":18237,"erek":18238,"Ġauthentication":18239,"Ġstole":18240,"Ġaccountability":18241,"label":18242,"Search":18243,"Ġalbeit":18244,"atican":18245,"funded":18246,"ĠAdding":18247,"ĠIQ":18248,"Ġsubmar":18249,"lit":18250,"aque":18251,"ĠLearning":18252,"Ġinteger":18253,"Master":18254,"ĠChrom":18255,"Ġpremier":18256,"Op":18257,"ĠLiu":18258,"Ġblessed":18259,"ĠGlobe":18260,"ĠResponse":18261,"Ġlegitim":18262,"ĠMerkel":18263,"Ġdisposal":18264,"´":18265,"Ġgauge":18266,"peat":18267,"Ġinduced":18268,"Ġquestionable":18269,"arthy":18270,"ĠVit":18271,"ĠFeed":18272,"Until":18273,"Ut":18274,"worthy":18275,"RY":18276,"ĠHerald":18277,"ĠHammer":18278,"Ġmedal":18279,"ĠRivers":18280,"ĠHack":18281,"Ġclarify":18282,"Ġtracked":18283,"Ġautonomous":18284,"Ġtenant":18285,"ĠQatar":18286,"erie":18287,"Ġgrim":18288,"ĠMonitor":18289,"Ġresistant":18290,"ĠSpec":18291,"ĠWells":18292,"NAS":18293,"148":18294,"Ġminers":18295,"iotics":18296,"Ġmisses":18297,"116":18298,"gian":18299,"git":18300,"ĠEyes":18301,"pres":18302,"Ġgraduated":18303,"Ġangel":18304,"Ġsynchron":18305,"Ġefficiently":18306,"Ġtransmitted":18307,"Harry":18308,"Ġglobally":18309,"ENCE":18310,"ĠMontana":18311,"raged":18312,"ĠPrevention":18313,"Ġpiss":18314,"ĠLl":18315,"Ġshelf":18316,"ĠBJP":18317,"ĠTestament":18318,"ĠLate":18319,"iker":18320,"ĠHapp":18321,"ĠJulian":18322,"hall":18323,"Ġspont":18324,"Ġshutdown":18325,"Ġinconsistent":18326,"Ġsubscribers":18327,"Ġskeleton":18328,"ĠNebraska":18329,"Ġinspire":18330,"ĠVoid":18331,"Feed":18332,"Ġangles":18333,"ĠSprings":18334,"Ġbenchmark":18335,"Ġvaccines":18336,"izophren":18337,"sexual":18338,"uffed":18339,"Ġshine":18340,"ĠKath":18341,"Ġgesture":18342,"inea":18343,"Ġrip":18344,"Ġoppression":18345,"Ġconscience":18346,"bt":18347,"ĠLum":18348,"Ġincidence":18349,"ĠFa":18350,"wr":18351,"Ġmineral":18352,"ĠSpurs":18353,"alky":18354,"Ġthunder":18355,"Ġopio":18356,"Being":18357,"ĠPalm":18358,"Ġwasted":18359,"Ġlb":18360,"iaries":18361,"ĠInitiative":18362,"Ġcurric":18363,"Ġmarker":18364,"ĠMcL":18365,"Ġextensions":18366,"ĠPv":18367,"ĠArms":18368,"Ġofferings":18369,"Ġdefenses":18370,"Ġvendor":18371,"Ġcontradict":18372,"ĠColin":18373,"Ġreddit":18374,"Ġperipher":18375,"122":18376,"Ġsins":18377,"Edit":18378,"ICT":18379,"Soft":18380,"ĠShah":18381,"Ġadministrator":18382,"ĠTrip":18383,"Ġpornography":18384,"Ġtuition":18385,"inence":18386,"ĠProgress":18387,"Ġcatalog":18388,"Ġsuite":18389,"Ġhike":18390,"Ġreproductive":18391,"engine":18392,"Ġdrought":18393,"ĠNoah":18394,"Ġ230":18395,"Ġdude":18396,"Ġrelaxed":18397,"Ġpartition":18398,"Ġparticipant":18399,"Ġtelesc":18400,"Ġfeas":18401,"ĠFF":18402,"owner":18403,"Ġsweeping":18404,"Ġlenses":18405,"Ġmatchup":18406,"ĠRepl":18407,"ournals":18408,"Ġcredible":18409,"Ġgrandmother":18410,"Ġthermal":18411,"Ġsubscribing":18412,"Ġidentities":18413,"colm":18414,"UCT":18415,"Ġreluctant":18416,"users":18417,"ĠCort":18418,"Ġassisted":18419,"OSS":18420,"ATIONS":18421,"ISH":18422,"Ġpharmaceutical":18423,"icable":18424,"adian":18425,"ĠSonic":18426,"ĠFury":18427,"ĠMong":18428,"AH":18429,"ĠPsychology":18430,"Ġphosph":18431,"Ġtreats":18432,"ŃĶ":18433,"Ġsteadily":18434,"ĠHello":18435,"Ġrelates":18436,"Ġclue":18437,"Expl":18438,"auth":18439,"Ġrevision":18440,"Ġeld":18441,"osion":18442,"Ġbron":18443,"144":18444,"rikes":18445,"Ġmines":18446,"Ġblanket":18447,"ĠFail":18448,"eled":18449,"ĠImagine":18450,"ĠPlanned":18451,"aic":18452,"Request":18453,"Mad":18454,"ĠHorse":18455,"ĠEagle":18456,"Ġcapac":18457,"157":18458,"Ġling":18459,"ĠNice":18460,"ĠParenthood":18461,"minster":18462,"ogs":18463,"ensitive":18464,"Nothing":18465,"Ġcarn":18466,"Fin":18467,"ĠPE":18468,"Ġrifles":18469,"ĠLP":18470,"Sand":18471,"ĠguiActive":18472,"Ġtourist":18473,"CNN":18474,"Ġunveiled":18475,"Ġpredecessor":18476,"}{":18477,"uber":18478,"Ġoffshore":18479,"Ġoptical":18480,"ĠRot":18481,"ĠPearl":18482,"eton":18483,"Ġstared":18484,"Ġfarther":18485,"atility":18486,"contin":18487,"ĠGy":18488,"ĠFoster":18489,"ĠCoc":18490,"rients":18491,"Ġdesigning":18492,"ĠEconomy":18493,"ONG":18494,"Women":18495,"ĠNancy":18496,"erver":18497,"Ġmascul":18498,"Ġcasualties":18499,"Ġ225":18500,"ĠSullivan":18501,"ĠChoice":18502,"Ġaster":18503,"ws":18504,"Ġhotels":18505,"Ġconsiderations":18506,"Ġcouch":18507,"ĠStrip":18508,"ĠGn":18509,"Ġmanipulate":18510,"lied":18511,"Ġsynthetic":18512,"Ġassaulted":18513,"Ġoffenses":18514,"ĠDrake":18515,"Ġimpe":18516,"October":18517,"ĠHeritage":18518,"hl":18519,"ĠBlair":18520,"Unlike":18521,"Ġgrief":18522,"Ġ450":18523,"Ġopted":18524,"Ġresignation":18525,"ilo":18526,"Ġverse":18527,"ĠTomb":18528,"Ġupt":18529,"Ġaired":18530,"ĠHook":18531,"ĠMLB":18532,"Ġassumes":18533,"outed":18534,"ĠVers":18535,"Ġinferior":18536,"Ġbundle":18537,"ĠDNS":18538,"ographer":18539,"Ġmultip":18540,"ĠSouls":18541,"Ġillustrated":18542,"Ġtactic":18543,"Ġdressing":18544,"Ġduo":18545,"Conf":18546,"Ġrelent":18547,"Ġcant":18548,"Ġscarce":18549,"Ġcandy":18550,"ĠCF":18551,"Ġaffiliated":18552,"Ġsprint":18553,"ylan":18554,"ĠGarcia":18555,"Ġjunk":18556,"Print":18557,"exec":18558,"Crit":18559,"Ġportrait":18560,"iries":18561,"ĠOFF":18562,"Ġdisputes":18563,"WR":18564,"Love":18565,"ãģĦ":18566,"ĠReyn":18567,"Ġhipp":18568,"opath":18569,"Ġfloors":18570,"ĠFeel":18571,"Ġworries":18572,"Ġsettlements":18573,"ĠPos":18574,"Ġmosque":18575,"Ġfinals":18576,"Ġcrushed":18577,"ĠProbably":18578,"ĠBot":18579,"ĠMans":18580,"ĠPeriod":18581,"Ġsovereignty":18582,"Ġseller":18583,"Ġapost":18584,"Ġamateur":18585,"Ġdorm":18586,"Ġconsuming":18587,"Ġarmour":18588,"ĠRoose":18589,"Ġintensive":18590,"Ġeliminating":18591,"ĠSunni":18592,"ĠAleppo":18593,"jin":18594,"Ġadvise":18595,"pal":18596,"ĠHalo":18597,"Ġdescent":18598,"Ġsimpler":18599,"Ġbooth":18600,"STR":18601,"Later":18602,"ĠCave":18603,"===":18604,"Ġmol":18605,"Ġfist":18606,"Ġshotgun":18607,"supp":18608,"Ġrobbery":18609,"Effect":18610,"Ġobscure":18611,"ĠProfessional":18612,"Ġembassy":18613,"Ġmilitant":18614,"Ġincarcer":18615,"Ġgenerates":18616,"Ġlaunches":18617,"Ġadministrators":18618,"Ġshaft":18619,"Ġcircular":18620,"Ġfreshman":18621,"ĠWes":18622,"ĠJoel":18623,"ĠDrew":18624,"ĠDuncan":18625,"ĠApparently":18626,"sight":18627,"ĠInternal":18628,"ĠIndividual":18629,"ĠFE":18630,"Ġbore":18631,"ĠMt":18632,"Ġbroadly":18633,"ĠOptions":18634,"ountain":18635,"ipes":18636,"ĠVideos":18637,"204":18638,"Ġhills":18639,"Ġsimulation":18640,"Ġdisappointment":18641,"itan":18642,"ĠLaboratory":18643,"Ġupward":18644,"Ġboundary":18645,"Ġdarker":18646,"hart":18647,"Ġdominance":18648,"Cong":18649,"ĠOracle":18650,"ĠLords":18651,"Ġscholarship":18652,"ĠVincent":18653,"ede":18654,"ĠRah":18655,"Ġencourages":18656,"rov":18657,"Ġquo":18658,"Ġpremise":18659,"ĠCrisis":18660,"ĠHolocaust":18661,"Ġrhythm":18662,"Ġmetric":18663,"club":18664,"Ġtransported":18665,"Ġnod":18666,"ĠPist":18667,"Ġancestors":18668,"ĠFreder":18669,"thumbnails":18670,"ĠCE":18671,"OND":18672,"Phil":18673,"venge":18674,"ĠProducts":18675,"castle":18676,"Ġqualifying":18677,"ĠKaren":18678,"VERTISEMENT":18679,"Ġmighty":18680,"Ġexplanations":18681,"Ġfixing":18682,"Di":18683,"Ġdeclaring":18684,"Ġanonymity":18685,"Ġjuven":18686,"ĠNord":18687,"ĠDoom":18688,"ĠActually":18689,"Ok":18690,"phis":18691,"ĠDesert":18692,"Ġ116":18693,"IK":18694,"ĠFM":18695,"Ġincomes":18696,"VEL":18697,"okers":18698,"Ġpecul":18699,"Ġlightweight":18700,"gue":18701,"Ġaccent":18702,"Ġincrement":18703,"ĠChan":18704,"Ġcomplaining":18705,"ĠBaghd":18706,"Ġmidfielder":18707,"Ġoverhaul":18708,"Process":18709,"ĠHollow":18710,"ĠTitans":18711,"Small":18712,"manuel":18713,"ĠUnity":18714,"ĠEvents":18715,"Sty":18716,"Ġdisproportion":18717,"nesty":18718,"enes":18719,"ĠCod":18720,"Ġdemonstrations":18721,"ĠCrimson":18722,"ĠOH":18723,"Ġenrolled":18724,"Ġcel":18725,"ĠBrett":18726,"Ġaide":18727,"Ġheels":18728,"Ġbroadband":18729,"Ġmarking":18730,"Ġwizard":18731,"ĠNJ":18732,"ĠChiefs":18733,"Ġingredient":18734,"Ġdug":18735,"ĠShut":18736,"urchase":18737,"endor":18738,"Ġfarmer":18739,"ĠGoldman":18740,"129":18741,"155":18742,"Order":18743,"Ġlion":18744,"iably":18745,"Ġstain":18746,"array":18747,"ilitary":18748,"ĠFAQ":18749,"Ġexploded":18750,"ĠMcCarthy":18751,"ĠTweet":18752,"ĠGreens":18753,"eking":18754,"ln":18755,"ensen":18756,"Ġmotorcycle":18757,"Ġparticle":18758,"Ġcholesterol":18759,"Bron":18760,"Ġstair":18761,"Ġoxid":18762,"Ġdesirable":18763,"ibles":18764,"Ġtheor":18765,"forcing":18766,"Ġpromotional":18767,"ovo":18768,"boot":18769,"ĠBonus":18770,"rawling":18771,"Ġshortage":18772,"ĠPsy":18773,"Ġrecruited":18774,"Ġinfants":18775,"Ġtestosterone":18776,"Ġdeduct":18777,"Ġdistinctive":18778,"Ġfirmware":18779,"built":18780,"145":18781,"Ġexplored":18782,"Ġfactions":18783,"Ġvide":18784,"Ġtattoo":18785,"Ġfinancially":18786,"Ġfatigue":18787,"Ġproceeding":18788,"constitutional":18789,"Ġmiser":18790,"Ġchairs":18791,"gging":18792,"ipple":18793,"Ġdent":18794,"Ġdisreg":18795,"çĶ":18796,"stant":18797,"llo":18798,"bps":18799,"akening":18800,"Ġabnormal":18801,"ĠERA":18802,"士":18803,"ĠHBO":18804,"ĠMAR":18805,"Ġconcess":18806,"Ġservant":18807,"Ġaspir":18808,"lav":18809,"ĠPanel":18810,"amo":18811,"Ġprecip":18812,"Ġrecordings":18813,"Ġproceeded":18814,"Ġcolony":18815,"ĠTang":18816,"ablo":18817,"Ġstripped":18818,"Left":18819,"too":18820,"Ġpotatoes":18821,"Ġfinest":18822,"%).":18823,"Ġcrap":18824,"ĠZach":18825,"abases":18826,"ĠGoth":18827,"Ġbillionaire":18828,"wolf":18829,"Ġsanction":18830,"SK":18831,"Ġlogged":18832,"Po":18833,"eyed":18834,"unal":18835,"Ġcricket":18836,"Ġarmies":18837,"Ġuncovered":18838,"Cloud":18839,"ón":18840,"Ġrebounds":18841,"Ġmes":18842,"Oper":18843,"Pac":18844,"Ġnationally":18845,"Ġinserted":18846,"pict":18847,"Ġgovernance":18848,"и":18849,"Ġprivileges":18850,"GET":18851,"Ġfavorites":18852,"imity":18853,"Ġlover":18854,"them":18855,"empl":18856,"Ġgorgeous":18857,"Ann":18858,"Ġslipped":18859,"Ġveto":18860,"Bob":18861,"Ġslim":18862,"ucc":18863,"ĠFame":18864,"uddenly":18865,"Ġdenies":18866,"ĠMaur":18867,"Ġdistances":18868,"Ġwanna":18869,"tar":18870,"ĠSER":18871,"ĠâĪ":18872,"Ġlemon":18873,"athetic":18874,"Ġliteral":18875,"Ġdistinguished":18876,"Ġanswering":18877,"GI":18878,"Ġreligions":18879,"ĠPhilos":18880,"ĠLay":18881,"Ġcompos":18882,"irements":18883,"ĠKos":18884,"inez":18885,"rolling":18886,"Ġyoungest":18887,"andise":18888,"ĠBorn":18889,"Ġaltar":18890,"amina":18891,"ĠBoot":18892,"voc":18893,"Ġdigging":18894,"Ġpressures":18895,"Ġlen":18896,"264":18897,"Ġassassination":18898,"ĠBirmingham":18899,"ĠMyth":18900,"Ġsovereign":18901,"ĠArtist":18902,"ĠPhotograph":18903,"Ġdepicted":18904,"Ġdispens":18905,"orthy":18906,"Ġambul":18907,"integ":18908,"ĠCele":18909,"ĠTibet":18910,"Ġhierarchy":18911,"Ġcu":18912,"Ġpreseason":18913,"ĠPeterson":18914,"Ġcolours":18915,"Ġworrying":18916,"Ġbackers":18917,"ĠPalmer":18918,"Ġμ":18919,"Ġcontributor":18920,"Ġhearings":18921,"Ġurine":18922,"ĠÙ":18923,"ourgeois":18924,"Similar":18925,"ĠZimmer":18926,"something":18927,"ĠUSC":18928,"Ġstrengths":18929,"ĠFI":18930,"Ġlogging":18931,"Asked":18932,"ĠThai":18933,"inqu":18934,"ĠWalt":18935,"Ġcrews":18936,"itism":18937,"301":18938,"Ġsharply":18939,"umed":18940,"Ġredirect":18941,"rators":18942,"Inf":18943,"ĠWeapons":18944,"Ġteasp":18945,"1999":18946,"Live":18947,"ĠEspecially":18948,"ĠSter":18949,"ĠVeterans":18950,"Ġintro":18951,"otherapy":18952,"Ġmalware":18953,"Ġbreeding":18954,"Ġmolecular":18955,"ĠRoute":18956,"ĠComment":18957,"ochem":18958,"Ġain":18959,"Season":18960,"Ġlinebacker":18961,"Ä«":18962,"ĠEconomics":18963,"esar":18964,"ĠLives":18965,"ĠEmma":18966,"Ġkin":18967,"ĠTerrit":18968,"Ġplanted":18969,"oton":18970,"ĠButter":18971,"ĠSpons":18972,"PER":18973,"Ġdungeon":18974,"Ġsymbolic":18975,"Ġfilmed":18976,"Ġdiets":18977,"Ġconcludes":18978,"Ġcertainty":18979,"ĠFormat":18980,"Ġstrangers":18981,"format":18982,"ĠPhase":18983,"Ġcopied":18984,"Ġmetres":18985,"lda":18986,"ĠUsers":18987,"Ġdeliberate":18988,"Ġwashed":18989,"ĠLance":18990,"imation":18991,"Ġimproper":18992,"ĠGenesis":18993,"ickr":18994,"ĠKush":18995,"Ġrealise":18996,"Ġembarrassing":18997,"alking":18998,"bucks":18999,"Ġverified":19000,"Ġoutline":19001,"years":19002,"ĠIncome":19003,"202":19004,"Ġzombies":19005,"Final":19006,"ĠMillenn":19007,"Ġmodifications":19008,"ĠVision":19009,"ĠMoses":19010,"verb":19011,"iterranean":19012,"ĠJet":19013,"Ġnaval":19014,"ĠAgg":19015,"Ġurl":19016,"Ġvictories":19017,"Ġnonetheless":19018,"Ġinjust":19019,"ĠFact":19020,"çļ":19021,"Ġinsufficient":19022,"review":19023,"facebook":19024,"Ġnegotiating":19025,"Ġguarantees":19026,"imen":19027,"utenberg":19028,"Ġgambling":19029,"Ġcongr":19030,"Loading":19031,"Ġnevertheless":19032,"Ġpresidents":19033,"ĠIndustrial":19034,"Ġ118":19035,"Ġpoured":19036,"ĠTory":19037,"Ġ175":19038,"Ġ:=":19039,"Scott":19040,"angered":19041,"Tok":19042,"Ġorganizers":19043,"Mat":19044,"ĠGrowth":19045,"Ġadul":19046,"Ġensures":19047,"Ġ117":19048,"é¾įå":19049,"Ġmassacre":19050,"Ġgrades":19051,"before":19052,"ADVERTISEMENT":19053,"ĠSlow":19054,"ĠMMA":19055,"âĢĶ\"":19056,"ĠVatican":19057,"Qaeda":19058,"Ġowe":19059,"6666":19060,"ĠSorry":19061,"ĠGrass":19062,"Ġbackgrounds":19063,"Ġexhausted":19064,"Ġclan":19065,"Ġcompromised":19066,"ĠElf":19067,"ĠIsaac":19068,"enson":19069,"Invest":19070,"IFA":19071,"Ġinterrupted":19072,"ãĥīãĥ©":19073,"Ġtwisted":19074,"ĠDragons":19075,"Mode":19076,"ĠKremlin":19077,"Ġfertil":19078,"heres":19079,"phan":19080,"ĠNode":19081,"fed":19082,"ĠOrc":19083,"Ġunwilling":19084,"Cent":19085,"Ġpriorit":19086,"Ġgraduates":19087,"Ġsubjective":19088,"Ġissuing":19089,"ĠLt":19090,"Ġviewer":19091,"Ġwoke":19092,"Thus":19093,"brook":19094,"Ġdepressed":19095,"Ġbracket":19096,"ĠGor":19097,"ĠFighting":19098,"Ġstriker":19099,"Report":19100,"ĠPortugal":19101,"Ġneo":19102,"wed":19103,"199":19104,"Ġfleeing":19105,"shadow":19106,"identified":19107,"USE":19108,"Steam":19109,"Ġstretched":19110,"Ġrevelations":19111,"arted":19112,"ĠDw":19113,"Ġalignment":19114,"eston":19115,"ĠJared":19116,"Sep":19117,"Ġblogs":19118,"update":19119,"gom":19120,"risk":19121,"Ġclash":19122,"ĠHour":19123,"Ġruntime":19124,"Ġunwanted":19125,"Ġscam":19126,"Ġrack":19127,"Ġenlight":19128,"onest":19129,"ĠFerr":19130,"Ġconvictions":19131,"Ġpiano":19132,"Ġcirculation":19133,"ĠWelcome":19134,"Ġbacklash":19135,"ĠWade":19136,"Ġreceivers":19137,"otive":19138,"Jeff":19139,"Ġnetworking":19140,"ĠPrep":19141,"ĠExplorer":19142,"Ġlecture":19143,"Ġuploaded":19144,"ĠMeat":19145,"BLE":19146,"ĠNazis":19147,"ĠSynd":19148,"stud":19149,"roots":19150,"rians":19151,"Ġportrayed":19152,"Ġ??":19153,"ĠBuddha":19154,"sun":19155,"Robert":19156,"ĠComplex":19157,"Ġoversee":19158,"Ġstealth":19159,"Title":19160,"ĠJobs":19161,"ĠKum":19162,"Ġappreciation":19163,"ĠMOD":19164,"Ġbasics":19165,"Ġclips":19166,"Ġnursing":19167,"Ġproposition":19168,"Ġrealised":19169,"ĠNYC":19170,"Ġallocated":19171,"rium":19172,"aran":19173,"ĠProduction":19174,"ĠVote":19175,"Ġsmugg":19176,"Ġhunter":19177,"azer":19178,"ĠChanges":19179,"Ġfluct":19180,"yon":19181,"Array":19182,"Ġkits":19183,"Water":19184,"Ġuncommon":19185,"Ġresting":19186,"ells":19187,"would":19188,"Ġpursued":19189,"Ġassertion":19190,"ometown":19191,"ĠMosul":19192,"ĠPlatform":19193,"iolet":19194,"Ġshareholders":19195,"Ġtrails":19196,"Pay":19197,"ĠEnforcement":19198,"types":19199,"ĠAnonymous":19200,"Ġsatisfying":19201,"ilogy":19202,"Ġ('":19203,"wave":19204,"city":19205,"Steve":19206,"Ġconfrontation":19207,"ĠEld":19208,"Capt":19209,"ahan":19210,"htm":19211,"ĠCtrl":19212,"ONS":19213,"230":19214,"ifa":19215,"holding":19216,"Ġdelicate":19217,"Ġjaw":19218,"ĠGoing":19219,"orum":19220,"Sal":19221,"Ġdull":19222,"ĠBeth":19223,"Ġprisons":19224,"Ġego":19225,"ĠElsa":19226,"avorite":19227,"ĠGang":19228,"ĠNuclear":19229,"Ġspider":19230,"atsu":19231,"Ġsampling":19232,"Ġabsorbed":19233,"ĠPharm":19234,"ieth":19235,"Ġbucket":19236,"ĠRecomm":19237,"OF":19238,"ĠFactory":19239,"ANCE":19240,"Ġbacter":19241,"Has":19242,"ĠObserv":19243,"121":19244,"Ġpremiere":19245,"Develop":19246,"Ġcurrencies":19247,"Cast":19248,"Ġaccompanying":19249,"ĠNashville":19250,"Ġfatty":19251,"ĠBrend":19252,"Ġlocks":19253,"Ġcentered":19254,"ĠUT":19255,"aughs":19256,"orie":19257,"ĠAffordable":19258,"vance":19259,"DL":19260,"emet":19261,"Ġthrone":19262,"ĠBluetooth":19263,"Ġnaming":19264,"ifts":19265,"ADE":19266,"Ġcorrected":19267,"Ġpromptly":19268,"ĠSTR":19269,"Ġgenome":19270,"Ġcope":19271,"Ġvalley":19272,"Ġrounded":19273,"ĠKend":19274,"alion":19275,"pers":19276,"Ġtourism":19277,"Ġstark":19278,"vl":19279,"Ġblowing":19280,"ĠSchedule":19281,"std":19282,"Ġunhappy":19283,"Ġlitigation":19284,"cedes":19285,"Ġandroid":19286,"Ġintegral":19287,"erers":19288,"uded":19289,"tax":19290,"Ġreiter":19291,"ĠMotors":19292,"ociated":19293,"Ġwonders":19294,"ĠApost":19295,"ucking":19296,"ĠRoosevelt":19297,"fram":19298,"Ġyields":19299,"Ġconstitutes":19300,"awk":19301,"Interest":19302,"Ġinterim":19303,"Ġbreakthrough":19304,"ĠCher":19305,"Ġprosec":19306,"ĠDj":19307,"ĠMT":19308,"Resp":19309,"ĠPT":19310,"Ġsperm":19311,"edit":19312,"BT":19313,"Linux":19314,"country":19315,"league":19316,"Ġdick":19317,"Ġoct":19318,"Ġinserting":19319,"Ġscra":19320,"ĠBrewing":19321,"Ġ1966":19322,"Ġrunners":19323,"Ġplun":19324,"idy":19325,"ĠDian":19326,"Ġdysfunction":19327,"Ġexclusion":19328,"Ġdisgr":19329,"Ġincorporate":19330,"Ġreconc":19331,"Ġnominated":19332,"ĠArcher":19333,"draw":19334,"achelor":19335,"Ġwritings":19336,"Ġshallow":19337,"Ġhast":19338,"ĠBMW":19339,"ĠRS":19340,"Ġthigh":19341,"Ġ1963":19342,"Ġlamb":19343,"Ġfavored":19344,"agle":19345,"Ġcooler":19346,"ĠHours":19347,"ĠGU":19348,"ĠOrigin":19349,"Ġglimpse":19350,"--------------------":19351,"Lim":19352,"Ġcheek":19353,"Ġjealous":19354,"-'":19355,"Ġharness":19356,"ĠPoison":19357,"Ġdisabilities":19358,"neapolis":19359,"Ġoutlook":19360,"Ġnotify":19361,"ĠIndianapolis":19362,"Ġabrupt":19363,"nsic":19364,"Ġencrypted":19365,"Ġforfe":19366,"reath":19367,"Ġrabb":19368,"Ġfoundations":19369,"Ġcompliment":19370,"ĠInterview":19371,"ĠSwe":19372,"Ġadolesc":19373,"Ġmonitors":19374,"ĠSacramento":19375,"Ġtimely":19376,"Ġcontempl":19377,"Ġpositioned":19378,"Ġposters":19379,"phies":19380,"iovascular":19381,"void":19382,"ĠFifth":19383,"Ġinvestigative":19384,"OUN":19385,"Ġintegrate":19386,"ĠINC":19387,"isha":19388,"iblings":19389,"ĠRequest":19390,"ĠRodriguez":19391,"Ġslides":19392,"ĠDX":19393,"Ġfeminism":19394,"Ġdatas":19395,"Ġbend":19396,"irus":19397,"ĠNigeria":19398,"Fox":19399,"Change":19400,"Ġairplane":19401,"ĠLaden":19402,"Ġpublicity":19403,"ixty":19404,"Ġcommitments":19405,"Ġaggregate":19406,"Ġdisplaying":19407,"ĠArrow":19408,"Ġ122":19409,"Ġrespects":19410,"android":19411,"six":19412,"ĠSha":19413,"Ġrestoration":19414,")\\":19415,"WS":19416,"oys":19417,"Ġillustrate":19418,"without":19419,"126":19420,"ĠâĶĤ":19421,"Ġpickup":19422,"nels":19423,"Ġ....":19424,"food":19425,"ĠFen":19426,")?":19427,"Ġphenomena":19428,"Ġcompanions":19429,"ĠWrite":19430,"Ġspill":19431,"Ġbridges":19432,"ĠUpdated":19433,"ĠFo":19434,"Ġinsects":19435,"ASHINGTON":19436,"Ġscare":19437,"iltr":19438,"ĠZhang":19439,"Ġseverity":19440,"Ġindul":19441,"149":19442,"ĠCoffee":19443,"Ġnorms":19444,"Ġpulse":19445,"ĠFT":19446,"Ġhorrific":19447,"ĠDestroy":19448,"ĠJSON":19449,"Ġolive":19450,"Ġdiscusses":19451,"Rest":19452,"Elect":19453,"ĠWinn":19454,"ĠSurviv":19455,"ĠHait":19456,"Sure":19457,"oped":19458,"Ġrooted":19459,"ĠSke":19460,"ĠBronze":19461,"Ġlol":19462,"Default":19463,"Ġcommodity":19464,"redited":19465,"Ġlibertarian":19466,"Ġforbidden":19467,"Ġgran":19468,"à¨":19469,"Ġlag":19470,"enz":19471,"drive":19472,"Ġmathematics":19473,"Ġwires":19474,"Ġcritically":19475,"Ġcarbohyd":19476,"ĠChancellor":19477,"ĠEddie":19478,"Ġbanning":19479,"ĠFri":19480,"Ġcomplications":19481,"etric":19482,"ĠBangladesh":19483,"Ġbandwidth":19484,"Stop":19485,"ĠOriginally":19486,"Ġhalfway":19487,"ynasty":19488,"shine":19489,"Ġtales":19490,"rities":19491,"avier":19492,"Ġspinning":19493,"ĠWHO":19494,"Ġneighbourhood":19495,"bach":19496,"Ġcommerce":19497,"ĠSle":19498,"BU":19499,"Ġentrepreneur":19500,"Ġpeculiar":19501,"ĠComments":19502,"fre":19503,"320":19504,"ICS":19505,"Ġimagery":19506,"ĠCanon":19507,"ĠElectronic":19508,"short":19509,"((":19510,"Dig":19511,"Ġcommem":19512,"uced":19513,"Ġinclined":19514,"ĠSummon":19515,"Ġcliff":19516,"ĠMediterranean":19517,"Ġpoetry":19518,"Ġprosperity":19519,"ĠRece":19520,"Ġpills":19521,"member":19522,"Ġfinale":19523,"unc":19524,"ĠGig":19525,"ä½":19526,"Ġlod":19527,"Ġbackward":19528,"-+":19529,"ĠForward":19530,"Ġthri":19531,"sure":19532,"Ġsoap":19533,"ĠFX":19534,"RES":19535,"ĠSexual":19536,"oulos":19537,"Ġfoolish":19538,"Ġrighteous":19539,"Ġcoff":19540,"terrorism":19541,"ustain":19542,"oter":19543,"Ġabuses":19544,"next":19545,"Ġabusive":19546,"Ġthereafter":19547,"Ġprohibition":19548,"ĠSUP":19549,"Ġdip":19550,"Ġripped":19551,"Ġinherited":19552,"Ġbats":19553,"stru":19554,"GT":19555,"Ġflawed":19556,"phabet":19557,"Ġfog":19558,"doors":19559,"Ġimaging":19560,"Ġdigits":19561,"ĠHungary":19562,"Ġarrog":19563,"Ġteachings":19564,"Ġprotocols":19565,"ĠBanks":19566,"à¸":19567,"pound":19568,"ĠCurt":19569,".\")":19570,"./":19571,"Ġexemption":19572,"endix":19573,"ĠMull":19574,"Ġimproves":19575,"ĠGamer":19576,"dimensional":19577,"Icon":19578,"ĠMargaret":19579,"Status":19580,"dates":19581,"Ġintends":19582,"Ġdepict":19583,"Ġparked":19584,"Joe":19585,"ĠMarines":19586,"chnology":19587,"!).":19588,"Ġjudged":19589,"Ġweights":19590,"Ray":19591,"Ġapartments":19592,"hester":19593,"Ġreinforce":19594,"Ġoffender":19595,"occup":19596,"Ġsore":19597,"ept":19598,"ĠPHP":19599,"ĠBrow":19600,"Ġauthorization":19601,"ĠRisk":19602,"ĠDelaware":19603,"ĠQU":19604,"Ġnotifications":19605,"Ġsunlight":19606,"Ġexclude":19607,"dat":19608,"Ġmesh":19609,"ĠSudan":19610,"Ġbelonged":19611,"Ġsubway":19612,"Ġnoon":19613,"ĠInterior":19614,"olics":19615,"ĠLakers":19616,"Ġcoding":19617,"Disclaimer":19618,"Calif":19619,"Old":19620,"Ġdisl":19621,"?????":19622,"Ġconfirms":19623,"Ġrecruitment":19624,"Ġhomicide":19625,"Consider":19626,"ĠJeffrey":19627,"fty":19628,"};":19629,"Ġobjection":19630,"doing":19631,"ĠLeo":19632,"Want":19633,"Ġglow":19634,"ĠClarke":19635,"ĠNorman":19636,"Ġverification":19637,"Ġpacket":19638,"ĠFormula":19639,"Ġplag":19640,"esville":19641,"Ġshouting":19642,"Ġov":19643,"ĠREC":19644,"ĠBub":19645,"Ġninth":19646,"Ġenerg":19647,"Ġvalidity":19648,"Ġups":19649,"jack":19650,"Ġneighboring":19651,"ĠNec":19652,"eworks":19653,"ĠHab":19654,"arez":19655,"Ġspine":19656,"Ġeventual":19657,"ĠLeaders":19658,"ĠCarn":19659,"Ġprobation":19660,"Ġromance":19661,"msg":19662,"ĠMechanical":19663,"ERY":19664,"Rock":19665,"Ġpartisan":19666,"Node":19667,"assets":19668,"minent":19669,"Ġforeigners":19670,"Ġtestify":19671,"ĠUsually":19672,"lords":19673,"ĠGren":19674,"ĠPowell":19675,"BIL":19676,"Ġsr":19677,"Ġaddict":19678,"Ġshells":19679,"Ġsigh":19680,"ĠYale":19681,"ternity":19682,"Ġ750":19683,"EU":19684,"ĠRifle":19685,"Ġpatron":19686,"ema":19687,"ĠBannon":19688,"anity":19689,"Ġtropical":19690,"ĠVII":19691,"cross":19692,"Everything":19693,"ĠISO":19694,"Ġhumble":19695,"assing":19696,"ĠFIG":19697,"Ġupdating":19698,"yson":19699,"Ġcalcium":19700,"Ġcompetent":19701,"Ġsteering":19702,"Prot":19703,"ĠSY":19704,"ĠFinals":19705,"ĠRug":19706,"159":19707,"137":19708,"ĠGolf":19709,"Ġ126":19710,"Ġaccommodation":19711,"ĠHughes":19712,"Ġaesthetic":19713,"artisan":19714,"ĠTwilight":19715,"Ġprince":19716,"ĠAgriculture":19717,"ĠDisco":19718,"Ġprecedent":19719,"Ġtyping":19720,"authorized":19721,"Option":19722,"ĠAub":19723,"lishes":19724,"acht":19725,"mag":19726,"Peter":19727,"ĠUFO":19728,"monton":19729,"ĠLith":19730,"Ġarom":19731,"Ġsecuring":19732,"Ġconfined":19733,"private":19734,"Ġswords":19735,"Ġmarkers":19736,"Ġmetabolic":19737,"select":19738,"ĠCurse":19739,"ĠOt":19740,"gressive":19741,"Ġincumb":19742,"ĠSaga":19743,"Ġpriced":19744,"Ġclearance":19745,"Content":19746,"Ġdrilling":19747,"Ġnotices":19748,"Ġbourgeois":19749,"Ġvest":19750,"Ġcookie":19751,"ĠGuardians":19752,"rys":19753,"inyl":19754,"Ġ124":19755,"Ġplausible":19756,"ongh":19757,"ĠOdin":19758,"Ġconception":19759,"ĠYuk":19760,"ĠBaghdad":19761,"ĠFlag":19762,"Austral":19763,"ĠIBM":19764,"Ġinternationally":19765,"ĠWikiLeaks":19766,"IED":19767,"Ġcyn":19768,"Ġchooses":19769,"ĠPill":19770,"Ġcombining":19771,"Ġradi":19772,"ĠMohammed":19773,"defense":19774,"atching":19775,"Subject":19776,"iciency":19777,"Frame":19778,"Ġ{\"":19779,"Ġchess":19780,"Ġtimer":19781,"190":19782,"Ġtin":19783,"Ġordinance":19784,"emetery":19785,"Ġaccusing":19786,"Ġnoticeable":19787,"Ġcentres":19788,"Ġlid":19789,"ĠMills":19790,"imgur":19791,"Ġzoom":19792,"ergic":19793,"Ġcompression":19794,"prim":19795,"find":19796,"Ġsurg":19797,"Ġpand":19798,"ĠKee":19799,"ĠChad":19800,"cellence":19801,"oyle":19802,"Ġsocialism":19803,"ĠTravis":19804,"ĠMHz":19805,"Ġguild":19806,"ALLY":19807,"ĠSubscribe":19808,"ĠRelated":19809,"Ġoccurrence":19810,"itching":19811,"Ġfictional":19812,"Ġcrush":19813,"ĠEA":19814,"cod":19815,"mix":19816,"ĠTriple":19817,"Ġretrieve":19818,"Ġstimulus":19819,"Ġpsychiat":19820,"ĠDoor":19821,"Ġhomosexuality":19822,"Ġelementary":19823,"Ġcellular":19824,"idian":19825,"ĠLaun":19826,"Ġintriguing":19827,"Ġfoam":19828,"ĠBass":19829,"idi":19830,"itsu":19831,"Ġassure":19832,"Ġcongrat":19833,"Ġbusinessman":19834,"ĠBoost":19835,"close":19836,"Ġlied":19837,"Ġsciences":19838,"ĠOmega":19839,"ĠGraphics":19840,"Ġ<=":19841,"spoken":19842,"Ġconnectivity":19843,"Saturday":19844,"ĠAvengers":19845,"Ġtoggle":19846,"Ġankle":19847,"Ġnationalist":19848,"model":19849,"ĠPool":19850,"ophobia":19851,"Var":19852,"ĠMons":19853,"atories":19854,"Ġaggressively":19855,"Clear":19856,"Forge":19857,"acters":19858,"Ġhedge":19859,"Ġpipes":19860,"Ġblunt":19861,"Ġsq":19862,"Ġremotely":19863,"Wed":19864,"asers":19865,"Ġrefriger":19866,"Ġtiles":19867,"Ġrescued":19868,"Ġcomprised":19869,"insky":19870,"Ġmanif":19871,"avanaugh":19872,"Ġprolifer":19873,"Ġaligned":19874,"xml":19875,"Ġtriv":19876,"Ġcoordination":19877,"ĠPER":19878,"ĠQuote":19879,"134":19880,"bf":19881,"ĠSaw":19882,"Ġtermination":19883,"Ġ190":19884,"Ġadditions":19885,"Ġtrio":19886,"Ġprojections":19887,"Ġpositively":19888,"Ġinclusive":19889,"Ġmembr":19890,"1990":19891,"older":19892,"Ġpracticed":19893,"inkle":19894,"Arch":19895,"Ġstarters":19896,"arius":19897,"Ġintermediate":19898,"ĠBenef":19899,"ĠKiller":19900,"Ġinterventions":19901,"ĠKil":19902,"ĠFlying":19903,"Inv":19904,"Ġpremature":19905,"Ġpsychiatric":19906,"Ġindie":19907,"Ġcollar":19908,"ĠRainbow":19909,"afi":19910,"Ġdisruption":19911,"ĠFOX":19912,"casting":19913,"Ġmisdem":19914,"cro":19915,"Ġwipe":19916,"ardon":19917,"Ġbast":19918,"ĠTommy":19919,"ĠRepresentative":19920,"Ġbelly":19921,"ĠPO":19922,"ĠBreitbart":19923,"132":19924,"Ġmessaging":19925,"Should":19926,"References":19927,"ĠGRE":19928,"istical":19929,"LP":19930,"ĠCav":19931,"ĠCrazy":19932,"Ġintuitive":19933,"keeping":19934,"ĠMoss":19935,"Ġdiscontin":19936,"ĠModule":19937,"Ġunrelated":19938,"ĠPractice":19939,"ĠTransport":19940,"Ġstatistically":19941,"orns":19942,"Ġsized":19943,"pu":19944,"Ġcaf":19945,"ĠWorlds":19946,"ĠRodgers":19947,"ĠLun":19948,"ĠComic":19949,"living":19950,"Ġcared":19951,"Ġclimbed":19952,"){":19953,"Ġconsisted":19954,"Ġmedieval":19955,"folk":19956,"Ġhacked":19957,"Ġdire":19958,"ĠHermione":19959,"Ġtended":19960,"ceans":19961,"Daniel":19962,"went":19963,"Ġlegislators":19964,"Ġredes":19965,"games":19966,"Ġgn":19967,"amiliar":19968,"Ġ++":19969,"ggy":19970,"threat":19971,"Ġmagnet":19972,"Ġperceive":19973,"Ġzip":19974,"Ġindictment":19975,"Ġcritique":19976,"gard":19977,"ĠSafe":19978,"ĠCream":19979,"Ġadvent":19980,"oba":19981,"Ġvowed":19982,"ousands":19983,"Ġski":19984,"Ġabortions":19985,"uart":19986,"Ġstunned":19987,"Ġadvancing":19988,"Ġlacked":19989,"Ġ\\\"":19990,"Ġschizophren":19991,"Ġelegant":19992,"Ġconferences":19993,"Ġcanceled":19994,"ĠHudson":19995,"ĠHopefully":19996,"Ġtrump":19997,"Ġfrequencies":19998,"Ġmeteor":19999,"ĠJunior":20000,"ĠFleet":20001,"ĠMalcolm":20002,"ĠTools":20003,"Ġ........":20004,"Ġhobby":20005,"ĠEuropeans":20006,"Ġ1500":20007,"ĠInto":20008,"Ġsway":20009,"ĠAppro":20010,"ĠCompl":20011,"Community":20012,"Ġtide":20013,"ĠSummit":20014,"ä»":20015,"Ġintervals":20016,"ĠEther":20017,"Ġhabitat":20018,"ĠStevens":20019,"lishing":20020,"ĠDomain":20021,"Ġtriggers":20022,"Ġchasing":20023,"Ġcharm":20024,"ĠFlower":20025,"itored":20026,"Ġblessing":20027,"Ġtextures":20028,"Five":20029,"Ġliquor":20030,"RP":20031,"FIN":20032,"Ġ1962":20033,"CAR":20034,"Unknown":20035,"Ġresil":20036,"ĠLily":20037,"Ġabundance":20038,"Ġpredictable":20039,"rar":20040,"Ġbullshit":20041,"leen":20042,"chet":20043,"Mor":20044,"Much":20045,"ä¹":20046,"Ġemphasized":20047,"Ġcrust":20048,"Ġprimitive":20049,"Ġenjoyable":20050,"ĠPictures":20051,"Ġteammate":20052,"pler":20053,"ĠTol":20054,"ĠKane":20055,"Ġsummoned":20056,"thy":20057,"rama":20058,"ĠHonda":20059,"Ġrealizing":20060,"Ġquicker":20061,"Ġconcentrate":20062,"clear":20063,"Ġ210":20064,"ĠErdogan":20065,"aris":20066,"Ġresponds":20067,"ĠBI":20068,"Ġeligibility":20069,"Ġpushes":20070,"ĠIdaho":20071,"Ġaggrav":20072,"Ġruins":20073,"urations":20074,"Ġbans":20075,"Ġanat":20076,"share":20077,"Ġgrind":20078,"hin":20079,"umen":20080,"Ġutilities":20081,"ĠYankees":20082,"Ġdatabases":20083,"ĠDD":20084,"Ġdisplaced":20085,"Ġdependencies":20086,"Ġstimulation":20087,"hun":20088,"houses":20089,"ĠPretty":20090,"ĠRavens":20091,"ĠTODAY":20092,"Ġassociates":20093,"Ġtherape":20094,"cled":20095,"Ġdeer":20096,"Ġrepairs":20097,"rentice":20098,"Ġreceptors":20099,"Ġremed":20100,"ĠCe":20101,"Ġmarriages":20102,"Ġballots":20103,"ĠSoldier":20104,"Ġhilarious":20105,"opl":20106,"138":20107,"Ġinherently":20108,"Ġignorant":20109,"Ġbounce":20110,"ĠEaster":20111,"RELATED":20112,"ĠCurrency":20113,"EV":20114,"ãĥŀ":20115,"ĠLead":20116,"Ġdeceased":20117,"Brien":20118,"ĠMusk":20119,"JS":20120,"Ġmerge":20121,"hearted":20122,"creat":20123,"mitt":20124,"mund":20125,"ĠâĢĭ":20126,"ĠBag":20127,"Ġprojection":20128,"Ġjava":20129,"ĠStandards":20130,"ĠLeonard":20131,"Ġcoconut":20132,"ĠPopulation":20133,"Ġtraject":20134,"Ġimply":20135,"Ġcuriosity":20136,"ĠDB":20137,"ĠFresh":20138,"ĠPor":20139,"Ġheavier":20140,"neys":20141,"gomery":20142,"Ġdeserved":20143,"Ġphrases":20144,"ĠGC":20145,"Ġyeast":20146,"desc":20147,"Death":20148,"Ġreboot":20149,"Ġmetadata":20150,"ICAL":20151,"Ġrepay":20152,"ĠIndependence":20153,"Ġsuburban":20154,"icals":20155,"Ġatop":20156,"Ġallocation":20157,"generation":20158,"ĠGram":20159,"Ġmoisture":20160,"Ġpine":20161,"ĠLiberals":20162,"Ġaides":20163,"Ġunderest":20164,"ĠBerry":20165,"Ġceremon":20166,"370":20167,"astrous":20168,"ĠPirates":20169,"Ġtense":20170,"ĠIndustries":20171,"ĠAppeals":20172,"ĠNear":20173,"Ġè£ıç":20174,"Ġlovers":20175,"ĠCAP":20176,"ĠCraw":20177,"Ġgiants":20178,"Ġefficacy":20179,"Element":20180,"ĠBehavior":20181,"ĠToyota":20182,"Ġintest":20183,"Priv":20184,"AI":20185,"Ġmaneuver":20186,"Ġperfection":20187,"Ġbang":20188,"paper":20189,"rill":20190,"George":20191,"border":20192,"inters":20193,"ĠSeth":20194,"Ġclues":20195,"ĠLevi":20196,"ĠRevenue":20197,"147":20198,"Ġvapor":20199,"Ġfortunate":20200,"Ġthreatens":20201,"Ġvet":20202,"Ġdependency":20203,"ersed":20204,"article":20205,"ĠBlizzard":20206,"Ġchlor":20207,"Ġminus":20208,"ĠBills":20209,"Ġcryptocurrency":20210,"Ġmetabolism":20211,"tering":20212,"Ġpestic":20213,"steps":20214,"ĠTreasure":20215,"racted":20216,"ĠConstant":20217,"Ġtemp":20218,"139":20219,"ĠDetective":20220,"urally":20221,"Ġrecovering":20222,"Ġcortex":20223,"Ġ144":20224,"closed":20225,"Ġprejudice":20226,"aunted":20227,"Ġstorms":20228,"ĠNOW":20229,"Ġmachinery":20230,"Address":20231,"Ġcompelled":20232,"270":20233,"Ġdespair":20234,"bane":20235,"Ġvegetable":20236,"Ġbeds":20237,"Learn":20238,"Ġcolorful":20239,"Ġspike":20240,"Ġmargins":20241,"Ġsympathy":20242,"Ġworkshop":20243,"ĠCBC":20244,"Sat":20245,"Ġburns":20246,"ĠGender":20247,"Ġ129":20248,"ĠCable":20249,"Ġdebts":20250,"ĠTheresa":20251,"Ġreflecting":20252,"Ġairst":20253,"Ġrim":20254,"ramid":20255,"Ġweaknesses":20256,"Writ":20257,"oggle":20258,"ti":20259,"ĠCharge":20260,"Ġweighed":20261,"Ġ(.":20262,"Ġlaughter":20263,"Ġrouter":20264,"ĠDemocracy":20265,"Dear":20266,"Ġhasht":20267,"Ġdy":20268,"Ġhints":20269,"running":20270,"Ġfinishes":20271,"arus":20272,"Mass":20273,"result":20274,"ascus":20275,"Ġvintage":20276,"Ġconqu":20277,"Ġwildly":20278,"acist":20279,"Ġlingu":20280,"Ġprotagonist":20281,"strom":20282,"teenth":20283,"ĠSolo":20284,"mac":20285,"filled":20286,"Ġrenown":20287,"itives":20288,"Ġmotive":20289,"ĠAntar":20290,"ĠMann":20291,"ĠAdjust":20292,"Ġrockets":20293,"Ġtroubling":20294,"ei":20295,"Ġorganisms":20296,"assis":20297,"Christian":20298,"Ġ145":20299,"ĠHass":20300,"Ġswall":20301,"Ġwax":20302,"ĠSurvival":20303,"VS":20304,"ĠMurd":20305,"vd":20306,"standard":20307,"Ġdragons":20308,"Ġacceleration":20309,"rational":20310,"final":20311,"Ġpaired":20312,"ĠEthereum":20313,"Ġinterfaces":20314,"Ġresent":20315,"Ġartifacts":20316,"Å«":20317,"arel":20318,"Ġcompetitor":20319,"ĠNicholas":20320,"ĠSurface":20321,"cpp":20322,"ĠTot":20323,"Ġeconomically":20324,"Ġorganised":20325,"Ġenforced":20326,"inho":20327,"Ġvarieties":20328,"Ġabdom":20329,"ĠBailey":20330,"idav":20331,"ĠSalv":20332,"paid":20333,"Ġaltitude":20334,"essert":20335,"ĠGutenberg":20336,"area":20337,"opoulos":20338,"Ġprofessors":20339,"iggs":20340,"ĠFate":20341,"hey":20342,"Ġ3000":20343,"Dist":20344,"Ġtwins":20345,"cill":20346,"ĠMaps":20347,"Ġtraps":20348,"Ġweed":20349,"ĠKiss":20350,"Ġyoga":20351,"Ġrecipients":20352,"ĠWestminster":20353,"Ġpools":20354,"ĠWalmart":20355,"188":20356,"ĠSchools":20357,"attack":20358,"ĠARM":20359,"paragraph":20360,"Warning":20361,"jl":20362,"Ġselfish":20363,"anchez":20364,"ĠHeights":20365,"Fre":20366,"ĠSoph":20367,"Ġ--------------------------------":20368,"tml":20369,"333":20370,"Ġraids":20371,"Ġsatellites":20372,"KEY":20373,"Ġlasts":20374,"ÑĤ":20375,"Ins":20376,"ĠDame":20377,"Ġunpredict":20378,"///":20379,"ghai":20380,"Ġartillery":20381,"Ġcruise":20382,"Ġgel":20383,"ĠCabinet":20384,"Ġblows":20385,"ĠEsp":20386,"Ġproximity":20387,"othe":20388,"ĠSkills":20389,"ĠUpper":20390,"obo":20391,"ĠNDP":20392,"Ġenjoys":20393,"Ġrepeating":20394,"ĠConstruction":20395,"ĠQuestions":20396,"Hillary":20397,"Ġuint":20398,"Ġprocessors":20399,"ĠGibson":20400,"ĠMultiple":20401,"qa":20402,"ĠBom":20403,"ĠMiles":20404,"ventional":20405,"Ġhurts":20406,"skin":20407,"ĠAIDS":20408,"Ġadvisers":20409,"ĠRoot":20410,"Ġmethodology":20411,"ĠDale":20412,"Ġdeton":20413,"ĠKnowledge":20414,"sequently":20415,"Ġ121":20416,"Ġconnects":20417,"Cy":20418,"ĠDanger":20419,"Ġcontributors":20420,"ĠBent":20421,"Ġbrass":20422,"ĠGuns":20423,"into":20424,"ĠFortune":20425,"Ġbroker":20426,"balance":20427,"Ġlengths":20428,"Ġvic":20429,"Ġaveraging":20430,"Ġappropriately":20431,"ĠCamera":20432,"Ġsandwich":20433,"ĠCDC":20434,"Ġcoordinate":20435,"Ġnavig":20436,"Ġgoodness":20437,"laim":20438,"Ġbrake":20439,"Ġextremist":20440,"ĠWake":20441,"ĠMend":20442,"ĠTiny":20443,"ĠCOL":20444,"ĠRF":20445,"ĠDual":20446,"ĠWine":20447,"Case":20448,"Ġrefined":20449,"Ġlamp":20450,"Lead":20451,"Ġbapt":20452,"ĠCarb":20453,"ĠSadd":20454,"ĠMinneapolis":20455,"PDF":20456,"Early":20457,"ĠHidden":20458,"Its":20459,"ĠTIME":20460,"Ġpap":20461,"Ġcommissioned":20462,"ĠFew":20463,"ĠColts":20464,"ĠBren":20465,"Ġbothered":20466,"Ġlikewise":20467,"Exper":20468,"ĠSchw":20469,"cry":20470,"nn":20471,"ĠMitch":20472,"imon":20473,"MG":20474,"bm":20475,"UMP":20476,"rays":20477,"Ġregistry":20478,"Ġ270":20479,"achine":20480,"rella":20481,"anting":20482,"00000":20483,"Ġruined":20484,"spot":20485,"Ġta":20486,"Ġmaximize":20487,"Ġinconven":20488,"Dead":20489,"Human":20490,"Enabled":20491,"ĠMarie":20492,"Ġchill":20493,"ĠParadise":20494,"Ġstarring":20495,"ĠLatino":20496,"ĠProtocol":20497,"ĠEVER":20498,"Ġsuppliers":20499,"message":20500,"ĠBrock":20501,"Ġserum":20502,"âĸĪâĸĪâĸĪâĸĪ":20503,"Ġencomp":20504,"Ġambition":20505,"uese":20506,"Ġarrows":20507,"Andrew":20508,"Ġantenna":20509,"Ġ1961":20510,"ĠBark":20511,"Ġbool":20512,"ãĤª":20513,"ĠStorage":20514,"Ġrailway":20515,"Ġtougher":20516,"ĠCad":20517,"Ġwashing":20518,"Py":20519,"']":20520,"embed":20521,"ĠMemphis":20522,"ackle":20523,"Ġfamously":20524,"ĠFortunately":20525,"ovies":20526,"Ġmindset":20527,"Ġsneak":20528,"ĠDh":20529,"RAW":20530,"ĠSimpson":20531,"Ġlivest":20532,"Ġlandmark":20533,"Ġcement":20534,"Low":20535,"Ġthrilled":20536,"ĠCourse":20537,"inel":20538,"Ġchuck":20539,"idate":20540,"global":20541,"Ġwhit":20542,"Ġ�":20543,"adays":20544,"ski":20545,"ĠSV":20546,"Ġviruses":20547,"306":20548,"ĠRespons":20549,"Ġtheaters":20550,"ĠBranch":20551,"ĠGeneva":20552,"ĠMK":20553,"Ġunbeliev":20554,"Ġcommunist":20555,"Original":20556,"ĠReceived":20557,"ĠTransfer":20558,"ĠArg":20559,"Input":20560,"ĠStrategy":20561,"Ġpalace":20562,"thening":20563,"Dri":20564,"Ġsentencing":20565,"umbnail":20566,"Ġpins":20567,"recy":20568,"Ġsiblings":20569,"Getting":20570,"ĠBU":20571,"ĠNorthwest":20572,"Ġprolonged":20573,"ĠSakura":20574,"Comb":20575,"ĠBour":20576,"Ġinadequate":20577,"ĠKash":20578,"Ġusername":20579,"ĠImprove":20580,"Ġbattling":20581,"ĠMAC":20582,"Ġcurriculum":20583,"Ġsoda":20584,"ĠCannon":20585,"Ġsensible":20586,"spons":20587,"December":20588,"Ġwicked":20589,"ĠPengu":20590,"Ġdictators":20591,"ĠHearts":20592,"ogyn":20593,"Ġsimilarities":20594,"ĠStats":20595,"Ġhollow":20596,"itations":20597,"\":[":20598,"Ġhover":20599,"ĠListen":20600,"sch":20601,"Sund":20602,"Ġcad":20603,"ĠParks":20604,"Ġlur":20605,"Ġhype":20606,"ĠLem":20607,"NAME":20608,"isure":20609,"Friday":20610,"Ġshoots":20611,"Ġcloses":20612,"Ġdb":20613,"ĠRidge":20614,"ĠDifferent":20615,"Ġreplies":20616,"ĠBroadway":20617,"opers":20618,"Ġintoler":20619,"ĠZeus":20620,"akespe":20621,"Ġproprietary":20622,"Ġrequesting":20623,"Ġcontrollers":20624,"ĠMIN":20625,"imedia":20626,"becca":20627,"Ġexpans":20628,"Ġoils":20629,"Bot":20630,"ĠChand":20631,"Ġprinter":20632,"Ġtopped":20633,"ĠPOL":20634,"ĠEarlier":20635,"Social":20636,"avin":20637,"Ġdecreases":20638,"ĠSeb":20639,"Ġspecifications":20640,"ĠBlast":20641,"ĠKurt":20642,"Ġfreel":20643,"Brown":20644,"Ġdilig":20645,"roe":20646,"ĠProblem":20647,"ĠQuad":20648,"Ġdecentral":20649,"ĠVector":20650,"anut":20651,"Ġplugins":20652,"ĠGregory":20653,"Ġfucked":20654,"elines":20655,"ĠAmbassador":20656,"take":20657,"Ġcleans":20658,"ongyang":20659,"Anonymous":20660,"stro":20661,"\"}":20662,"aline":20663,"ĠOdd":20664,"ĠEug":20665,"216":20666,"Ġboil":20667,"ĠPowers":20668,"Ġnurses":20669,"Obviously":20670,"ĠTechnical":20671,"Ġexceeded":20672,"ORS":20673,"Ġextremists":20674,"Ġtraces":20675,"expl":20676,"Ġcomr":20677,"ĠSach":20678,")/":20679,"Ġmasks":20680,"Ġsci":20681,"Bon":20682,"Ġregression":20683,"wegian":20684,"Ġadvisor":20685,"itures":20686,"ĠVo":20687,"example":20688,"ĠInstruct":20689,"Ġsiege":20690,"Ġreductions":20691,"ptr":20692,"Ġstatutory":20693,"Ġremoves":20694,"Ġpuck":20695,"redits":20696,"Ġbee":20697,"Ġsalad":20698,"Ġpromotions":20699,"ĠJoshua":20700,"withstanding":20701,"ETH":20702,"ĠCha":20703,"imus":20704,"Ġexpenditure":20705,"aunting":20706,"Ġdelighted":20707,"Ġ155":20708,"beh":20709,"Ġcarpet":20710,"ĠSpart":20711,"Ġjungle":20712,"lists":20713,"Ġbullying":20714,"ĠNobel":20715,"ĠGlen":20716,"Ġreferenced":20717,"Ġintroduces":20718,"sein":20719,"Ġchopped":20720,"glass":20721,"ĠWrest":20722,"Ġneutrality":20723,"ĠâĻ":20724,"Ġinvestigator":20725,"Ġshelves":20726,"Ġunconstitutional":20727,"Ġreproduction":20728,"Ġmerchant":20729,"mia":20730,"Ġmetrics":20731,"Ġexplosives":20732,"ĠSonia":20733,"Ġbodily":20734,"Ġthickness":20735,"Ġpredominantly":20736,"ĠAbility":20737,"Ġmonitored":20738,"ICH":20739,"Ġ].":20740,"ĠMartinez":20741,"Ġvisibility":20742,"Ġqueries":20743,"Ġgenocide":20744,"ĠWarfare":20745,"Query":20746,"Ġstudios":20747,"Ġembry":20748,"Ġcorridor":20749,"Ġcleaned":20750,"complete":20751,"ĠMH":20752,"Ġenrollment":20753,"INGS":20754,"Ġimpacted":20755,"Ġdisastrous":20756,"ĠYun":20757,"ĠClaire":20758,"ĠBasically":20759,"yt":20760,"usterity":20761,"Ġindirectly":20762,"wik":20763,"Ġdod":20764,"ĠCarr":20765,"Ġamp":20766,"Ġprohibit":20767,"ĠInitial":20768,"ĠRd":20769,"iji":20770,"Ġeducate":20771,"corn":20772,"iott":20773,"ĠBeauty":20774,"Ġdetective":20775,"ĠConn":20776,"since":20777,"Ġstagger":20778,"Ġobese":20779,"Ġbree":20780,"ologic":20781,"isse":20782,"walker":20783,"Ġblades":20784,"Ġlawful":20785,"func":20786,"ĠBehind":20787,"Ġappetite":20788,"Ġ(*":20789,"Ġtennis":20790,"Ġoffspring":20791,"Ġjets":20792,"Ġstructured":20793,"Ġaforementioned":20794,"Nov":20795,"Ġscaling":20796,"fill":20797,"Ġstew":20798,"Ġcurb":20799,"ĠStephan":20800,"edIn":20801,"SF":20802,"obic":20803,"éŃĶ":20804,"oug":20805,"ĠMM":20806,"Ġgenetically":20807,"opez":20808,"136":20809,"Ġumb":20810,"ancers":20811,"Ġcohort":20812,"Ġmerchandise":20813,"Ġimposing":20814,"ĠLegislature":20815,"ĠArchive":20816,"ivia":20817,"ĠNaval":20818,"Ġoffences":20819,"Ġmiracle":20820,"Ġsnapped":20821,"Ġfoes":20822,"Ġextensively":20823,"ĠRaf":20824,"Ġcater":20825,"edience":20826,"Kit":20827,"ĠBin":20828,"Ġrecommends":20829,"ĠCities":20830,"Ġrigid":20831,"ĠREAD":20832,"ĠNoble":20833,"ĠTian":20834,"Ġcertificates":20835,"antis":20836,"oiler":20837,"ĠBuddhist":20838,"did":20839,"Ġsurveyed":20840,"Ġdownward":20841,"Ġprints":20842,"ĠMotion":20843,"ronics":20844,"ĠSans":20845,"ossibly":20846,"uctions":20847,"Ġcolonies":20848,"ĠDanish":20849,"unit":20850,"Ġspoil":20851,"Ġadvisory":20852,"berries":20853,"Plan":20854,"Ġspecification":20855,"ophers":20856,"ĠResource":20857,"Ġshirts":20858,"prisingly":20859,"communications":20860,"Ġtrivial":20861,"Ġmentioning":20862,"isexual":20863,"Ġsupplements":20864,"Ġsupervision":20865,"BP":20866,"vor":20867,"Ġwit":20868,"Ġcooldown":20869,"Ġplaintiff":20870,"ĠReviews":20871,"ĠSri":20872,"ĠMint":20873,"ĠSugar":20874,"Ġafterward":20875,"ĠPriest":20876,"ĠInvestment":20877,"ogene":20878,"ĠTaking":20879,"Ġstretching":20880,"Ġinflammation":20881,"ĠTehran":20882,"Ġlining":20883,"Ġfreezing":20884,"ĠEntity":20885,"Ġinspiring":20886,"special":20887,"price":20888,"Ġsue":20889,"ĠPorter":20890,"ounge":20891,"ETA":20892,"ĠDerek":20893,"ĠLuis":20894,"uo":20895,"ymph":20896,"Ġexterior":20897,"ihil":20898,"ĠAshley":20899,"inator":20900,"Ġnutrients":20901,"ĠThrones":20902,"Ġfinances":20903,"ĠInspect":20904,"Ġspecially":20905,"ĠRequired":20906,"ĠPTS":20907,"ĠViolence":20908,"ointed":20909,"shots":20910,"Ġexcerpt":20911,"coon":20912,"INS":20913,"ĠGri":20914,"Ġrecognised":20915,"Week":20916,"Young":20917,"Ġvom":20918,"isle":20919,"ĠCurry":20920,"ĠBuddh":20921,"Ġnotebook":20922,"Ġdurable":20923,"/?":20924,"ĠGad":20925,"ĠPupp":20926,"Ġforgive":20927,"park":20928,"Ġpersonalities":20929,"analysis":20930,"clamation":20931,"Ġelevator":20932,"Ġwarehouse":20933,"ĠRole":20934,"unn":20935,"Ġillustration":20936,"ĠScan":20937,"Ġatmospheric":20938,"Import":20939,"ANC":20940,"ricted":20941,"fu":20942,"010":20943,"Ġarche":20944,"Ġrewarded":20945,"akespeare":20946,"Ġinternally":20947,"ĠRBI":20948,"alker":20949,"Ġelephant":20950,"owitz":20951,"ĠPizza":20952,"Ġbipartisan":20953,"és":20954,"Ġslowed":20955,"ĠStark":20956,"Ġoverride":20957,"OUS":20958,"Ġ320":20959,"undreds":20960,"ĠDeck":20961,"ĠCensus":20962,"bee":20963,"146":20964,"otor":20965,"Ġip":20966,"Ġub":20967,"ocations":20968,"ĠButton":20969,"rice":20970,"Ġcripp":20971,"fff":20972,"Ġoriginated":20973,"Ġoverwhelmed":20974,"appa":20975,"Ġforemost":20976,"âĢij":20977,"ĠLEG":20978,"release":20979,"eatured":20980,"atches":20981,"Ġreps":20982,"Ġlending":20983,"ĠReference":20984,"ĠClient":20985,"165":20986,"venth":20987,"Complete":20988,"ĠPatrol":20989,"Ġsworn":20990,"cam":20991,"Ġshuttle":20992,"ĠRalph":20993,"Ġhometown":20994,"-,":20995,"onal":20996,"ĠBP":20997,"åı":20998,"Ġpersuade":20999,"ĠAlexand":21000,"Ġcombines":21001,"Ġvivid":21002,"ĠLag":21003,"Ġencoding":21004,"Ġsalvation":21005,"wen":21006,"ĠRecovery":21007,"iya":21008,"University":21009,"ĠBiden":21010,"Ġbudgets":21011,"ĠTexans":21012,"fits":21013,"Ġhonored":21014,"Ġpython":21015,"TD":21016,"###":21017,"clone":21018,"Ġblink":21019,"ĠLiquid":21020,"Ġunemployed":21021,"Ġclashes":21022,"ĠCounsel":21023,"Ġdirecting":21024,"Ġpunct":21025,"ĠFalcons":21026,"Ġshark":21027,"ĠDamascus":21028,"Ġjeans":21029,"Ġembark":21030,"Ġseize":21031,"Ġupwards":21032,"280":21033,"ĠEz":21034,"ĠAnything":21035,"Ġexotic":21036,"lower":21037,"ĠCreator":21038,"ĠUm":21039,"Ġsuburbs":21040,"berger":21041,"ĠWend":21042,"Ġmint":21043,"ĠXX":21044,"ĠDro":21045,"Ġsuffers":21046,"Ġherb":21047,"tree":21048,"Ġfragile":21049,"Ġflooded":21050,"ĠAlcohol":21051,"olean":21052,"nyder":21053,"ĠKO":21054,"Fram":21055,"Ġ136":21056,"Ġowed":21057,"ĠMelee":21058,"ĠHash":21059,"Ġwhisk":21060,"Ġsudo":21061,"rr":21062,"Quick":21063,"appro":21064,"Ġii":21065,"ĠExamples":21066,"hee":21067,"Ġpromotes":21068,"perature":21069,"kar":21070,"ĠHonor":21071,"Ġsodium":21072,"ĠLif":21073,"rosso":21074,"intendent":21075,"Ġcorrespondent":21076,"Found":21077,"secret":21078,"Ġidentifies":21079,"agne":21080,"Ġlou":21081,"ĠPP":21082,"Ġcoincidence":21083,"move":21084,"Ġmilitia":21085,"Ġinfiltr":21086,"ĠPrimary":21087,"Ġpitching":21088,"ĠIb":21089,"ĠGOOD":21090,"ãĤ¸":21091,"ĠWizards":21092,"iral":21093,"ĠVenus":21094,"RR":21095,"ĠâĢķ":21096,"ĠCasey":21097,"Ġsadly":21098,"Ġadmire":21099,"Ġembarrassed":21100,"cb":21101,"Mel":21102,"Ġtubes":21103,"Ġbeautifully":21104,"ĠQueensland":21105,"Below":21106,"rez":21107,"quet":21108,"pleasant":21109,"Ġ«":21110,"Camp":21111,"Ġdecisive":21112,"1998":21113,"ĠLamb":21114,"utton":21115,"hn":21116,"ĠJagu":21117,"aunder":21118,"ĠCord":21119,"Ġclerk":21120,"Ġcaffe":21121,"Ġwiped":21122,"Ġreim":21123,"ĠMountains":21124,"Ġimprisoned":21125,"Ġdevelops":21126,"ĠPra":21127,"Ġmodeling":21128,"Anyone":21129,"ancel":21130,"ĠSit":21131,"Ġshields":21132,"Ġlawn":21133,"Ġcardiovascular":21134,"Ġdemonstrating":21135,"Ġparse":21136,"ĠIsraelis":21137,"Ġeuros":21138,"143":21139,"Ġglorious":21140,"inski":21141,"ecd":21142,"Ġconditioning":21143,"Ġhelpless":21144,"Ġmicrosc":21145,"ĠHarbor":21146,"Ġstakes":21147,"Ġ260":21148,"Ġunequ":21149,"ĠFloyd":21150,"Ġdamp":21151,"Ġapparatus":21152,"ĠLaws":21153,"Ġcounters":21154,"Ġinduce":21155,"atable":21156,"ĠAhmed":21157,"Ġslam":21158,"November":21159,"Ġpersist":21160,"Ġimminent":21161,"án":21162,"Ġshred":21163,"Ġphases":21164,"ĠEdmonton":21165,"ĠArmstrong":21166,"ĠMeet":21167,"ĠKitty":21168,"ÑĢ":21169,"circ":21170,"ĠAdult":21171,"Ġarose":21172,"ĠXen":21173,"Dan":21174,"gow":21175,"Ġsuperf":21176,"ĠAdmir":21177,"Ġendure":21178,"Ġkeyword":21179,"yrus":21180,"Ġyarn":21181,"Ġpathway":21182,"ĠHopkins":21183,"midt":21184,"Ġcensorship":21185,"dependent":21186,"Ġinstructor":21187,"Sources":21188,"Ġtoe":21189,"Ġballoon":21190,"Nob":21191,"Ġswear":21192,"ĠCastro":21193,"Ġgloss":21194,"ĠKavanaugh":21195,"Ġremarkably":21196,"Photos":21197,"ĠNom":21198,"ĠSoutheast":21199,"yers":21200,"Ġvalidation":21201,"Ġcannon":21202,"ĠVictory":21203,"ĠPierre":21204,"Ġcautious":21205,"Audio":21206,"Ġfetch":21207,"ĠGift":21208,"ĠHyp":21209,"Ġremedy":21210,"ZE":21211,"Ġscent":21212,"Ġbeard":21213,"ĠRut":21214,"-\"":21215,"Ġpatents":21216,"Hy":21217,"Ġunjust":21218,"Ġpotato":21219,"Ġforthcoming":21220,"Ġchef":21221,"ĠRift":21222,"affe":21223,"ĠROM":21224,"ĠLaunch":21225,"Ġpads":21226,"ĠNeo":21227,"Ġonset":21228,"Ġsqueeze":21229,"safe":21230,"Ġprefix":21231,"ĠTM":21232,"ĠNearly":21233,"ĠClinical":21234,"ĠMental":21235,"otiation":21236,"ĠUnic":21237,"antry":21238,"ĠCir":21239,"Ġepit":21240,"æ":21241,"Ġextracted":21242,"versely":21243,"riad":21244,"Ġstrains":21245,"Ġtops":21246,"Ġpoem":21247,"ĠRandy":21248,"ĠMaple":21249,"THER":21250,"upiter":21251,"ĠSSD":21252,"ļé":21253,"Ġuncon":21254,"pering":21255,"Ġslept":21256,"iners":21257,"Ġunderwater":21258,"ĠEvidence":21259,"gone":21260,"205":21261,"Ġhistorians":21262,"Ġsynthesis":21263,"Ġfrog":21264,"basketball":21265,"Ġvibrant":21266,"Ġsubord":21267,"Ġ365":21268,"ĠDial":21269,"Ġcooperate":21270,"HAHA":21271,"Ġgreeted":21272,"158":21273,"Ġjazz":21274,"Ġintox":21275,"ĠWalking":21276,"Ġsupervisor":21277,"ĠFusion":21278,"ĠMercedes":21279,"send":21280,"Ham":21281,"sd":21282,"nl":21283,"Ġtours":21284,"ĠFIFA":21285,"Ġculp":21286,"gd":21287,"304":21288,"Ġpleas":21289,"Ġillustrates":21290,"ĠColombia":21291,"Ġhighlighting":21292,"ĠSummary":21293,"Ġexposing":21294,"ĠDru":21295,"Ġirony":21296,"ritional":21297,"ĠCarroll":21298,"ĠEllis":21299,"Pict":21300,"ĠRapt":21301,"Ġadapter":21302,"Ġunm":21303,"Ġcorpse":21304,"Ġcelebrities":21305,"Den":21306,"atum":21307,"ĠApocalypse":21308,"ĠWag":21309,"lining":21310,"Ġhormones":21311,"Rub":21312,"ĠXi":21313,"ĠVaults":21314,"208":21315,"alkyrie":21316,"inosaur":21317,"Ġfeeds":21318,"vity":21319,"Ġdefeating":21320,"Wait":21321,"Ġemphasize":21322,"ĠSteelers":21323,"yrinth":21324,"leys":21325,"ĠWhenever":21326,"Currently":21327,"ĠClock":21328,"Ġcollectively":21329,"anyon":21330,"ĠJP":21331,"Ġmentality":21332,"Ġdownloads":21333,"Ġsurroundings":21334,"ĠBarnes":21335,"Ġflagship":21336,"Ġindicators":21337,"Ġgrapp":21338,"January":21339,"ĠElemental":21340,"ĠAthena":21341,"ibal":21342,"Ġsights":21343,"Ġcapita":21344,"ĠTreaty":21345,"Ġvoiced":21346,"ĠGaz":21347,"lette":21348,"Ġya":21349,"Ġexpired":21350,"Legend":21351,"Hot":21352,"nature":21353,"Ġunstable":21354,"Ġ280":21355,"ú":21356,"Comment":21357,"ALE":21358,"Ġquests":21359,"Ġhandler":21360,"nis":21361,"Ġversatile":21362,"Ġconceal":21363,"engeance":21364,"ĠInteractive":21365,"Ġobsessed":21366,"ĠDogs":21367,"Ġcracked":21368,"Sound":21369,"sv":21370,"ĠDylan":21371,"roads":21372,"fx":21373,"ĠCatholics":21374,"ĠHag":21375,"Ġslammed":21376,"Ġglowing":21377,"sale":21378,"Ġtissues":21379,"ĠChi":21380,"nee":21381,"Ġcher":21382,"sic":21383,"urrection":21384,"Ġbacon":21385,"ulatory":21386,").\"":21387,"Ġirregular":21388,"FORM":21389,"assed":21390,"Ġintentional":21391,"Ġcompensate":21392,"ĠSpeaking":21393,"ĠSets":21394,"153":21395,"Ġconventions":21396,"bands":21397,"emade":21398,"Ġecc":21399,"ĠWinston":21400,"ĠAssassin":21401,"ĠBelgian":21402,"Ġdependence":21403,"Ġniche":21404,"Ġbark":21405,"ĠJazz":21406,"Ġdisadvantage":21407,"Ġgasoline":21408,"Ġ165":21409,"çļĦ":21410,"essa":21411,"module":21412,"angular":21413,"OY":21414,"ĠTreatment":21415,"itas":21416,"olation":21417,"ĠArnold":21418,"Ġfeud":21419,"ĠNest":21420,"Ġtheatre":21421,"ewater":21422,"Ġminors":21423,"olicy":21424,"ĠHaven":21425,"division":21426,"Ġtrunk":21427,"Far":21428,"ĠPull":21429,"Ġcapturing":21430,"Ġ1800":21431,"ĠTeen":21432,"Ġexempl":21433,"Ġclinics":21434,"ĠBurg":21435,"Ġsubstit":21436,"Ġpayload":21437,"ĠLav":21438,"ĠTroy":21439,"ĠWitness":21440,"Ġfragments":21441,"Ġpasswords":21442,"Ġgospel":21443,"ĠGin":21444,"Ġtenants":21445,"olith":21446,"Six":21447,"Previous":21448,"ĠAges":21449,"ĠDarwin":21450,"Ġblat":21451,"Ġempathy":21452,"smith":21453,"bag":21454,"ĠEcho":21455,"ĠCamb":21456,"ĠMadd":21457,"ĠBoo":21458,"Ġrede":21459,"ĠBurning":21460,"Ġsmoothly":21461,"ĠAdrian":21462,"ĠVampire":21463,"ĠMonsters":21464,"steam":21465,"Style":21466,"Ma":21467,"rea":21468,"ĠDwar":21469,"alyst":21470,"ursor":21471,"Ġelimination":21472,"Ġcrypto":21473,"cht":21474,"ĠEternal":21475,"â̦]":21476,"ĠSorce":21477,"Ill":21478,"NER":21479,"Ġuh":21480,"Conclusion":21481,"wage":21482,"Ġrespir":21483,"Ġreminis":21484,"hetical":21485,"Ġgy":21486,"Ġutilized":21487,"icidal":21488,"Ġ1900":21489,"Ġhunters":21490,"ĠSwan":21491,"ĠReact":21492,"Ġvisitor":21493,"ĠThanksgiving":21494,"308":21495,"Posts":21496,"Ġhips":21497,"1997":21498,"omers":21499,"Ġknocking":21500,"ĠVehicle":21501,"Ġtil":21502,"Ġ138":21503,"Ġmi":21504,"ĠInvestigation":21505,"ĠKenya":21506,"Ġcasino":21507,"Ġmotives":21508,"Ġregain":21509,"rex":21510,"Ġweekends":21511,"Ġstabbed":21512,"boro":21513,"Ġexploited":21514,"ĠHAVE":21515,"ĠTelevision":21516,"cock":21517,"Ġpreparations":21518,"Ġendeav":21519,"ĠRemote":21520,"ĠMaker":21521,"ĠProdu":21522,"ĠEvan":21523,"Ġinformational":21524,"ĠLouisville":21525,"154":21526,"ĠDreams":21527,"Ġplots":21528,"ĠRunner":21529,"Ġhurting":21530,"Ġacademy":21531,"ĠMontgomery":21532,"nm":21533,"ĠLanc":21534,"ĠAlz":21535,"210":21536,"elong":21537,"Ġretailer":21538,"Ġarising":21539,"Ġrebellion":21540,"Ġblonde":21541,"played":21542,"Ġinstrumental":21543,"Cross":21544,"Ġretention":21545,"Ġtherapeutic":21546,"Ġseas":21547,"Ġinfantry":21548,"ĠClint":21549,"Ġprompting":21550,"Ġbitch":21551,"Ġstems":21552,"ĠKra":21553,"Ġthesis":21554,"ĠBog":21555,"rued":21556,"Ġkings":21557,"Ġclay":21558,"ificent":21559,"ĠYES":21560,"ĠThing":21561,"ĠCubs":21562,"veyard":21563,"elsh":21564,"inarily":21565,"ĠEy":21566,"ĠRolling":21567,"Ġevolving":21568,"India":21569,"Ġrecognizes":21570,"Ġgraduation":21571,"isers":21572,"Ġfertility":21573,"ĠMilan":21574,"Command":21575,"Ġboxing":21576,"Ġ1943":21577,"Ġgluten":21578,"ĠEmir":21579,"Ġidol":21580,"Ġconceived":21581,"ĠCreation":21582,"Merit":21583,"uddy":21584,"ussions":21585,"ĠLieutenant":21586,"ietal":21587,"Ġunchanged":21588,"ĠScale":21589,"ĠCrimea":21590,"balls":21591,"atorial":21592,"Ġdepths":21593,"Ġempirical":21594,"Ġtransm":21595,"Ġunsafe":21596,"missible":21597,"comfort":21598,"156":21599,"Ġmechanic":21600,"002":21601,"lins":21602,"Ġsmoked":21603,"Pos":21604,"Ġslowing":21605,"Ġlav":21606,"Texas":21607,"Ġcheating":21608,"ĠMetropolitan":21609,"ethyl":21610,"Ġdiscovering":21611,"asse":21612,"Ġpencil":21613,"ĠPyongyang":21614,"Ġcloset":21615,"ĠSheet":21616,"ĠEntry":21617,"oustic":21618,"Ġmyst":21619,"erate":21620,"ariat":21621,"Ġminerals":21622,"Ġmusician":21623,"ĠPul":21624,"ĠMaz":21625,"249":21626,"Ġpermissions":21627,"Ġiv":21628,"enary":21629,"ickers":21630,"ĠBing":21631,"hea":21632,"enable":21633,"Ġgriev":21634,"Ġasserted":21635,"ĠColonel":21636,"Ġaffidav":21637,"wo":21638,"Ġseated":21639,"ĠRide":21640,"Ġpaintings":21641,"ĠPix":21642,"Ġ137":21643,"ishi":21644,"umbai":21645,"gotten":21646,"ĠEarl":21647,"Ġinning":21648,"Ġcensus":21649,"Ġtravelled":21650,"ĠConsult":21651,"185":21652,"bind":21653,"Ġsimplicity":21654,"Ġoverlooked":21655,"ĠHelpful":21656,"Ġmonkey":21657,"Ġoverwhelmingly":21658,"Blood":21659,"ĠFlint":21660,"ĠJama":21661,"ĠPresent":21662,"ĠRage":21663,"ĠTA":21664,"ptive":21665,"Ġturnout":21666,"wald":21667,"ĠDolphins":21668,"ĠVPN":21669,"Ġonion":21670,"Ġcrafting":21671,"mma":21672,"ĠMercury":21673,"Ġarrange":21674,"Ġalerts":21675,"ĠOT":21676,"zbollah":21677,"Ġgases":21678,"ĠRichardson":21679,"sal":21680,"lar":21681,"Ġfrost":21682,"Ġlowering":21683,"Ġacclaim":21684,"Ġstartups":21685,"ĠGain":21686,"essment":21687,"Ġguardian":21688,"人":21689,"ĠPie":21690,"ĠLinks":21691,"Ġmerits":21692,"Ġawake":21693,"Ġparental":21694,"Ġexceeds":21695,"Ġidle":21696,"ĠPilot":21697,"ĠeBay":21698,"ĠAccept":21699,"ipeg":21700,"Cam":21701,"ĠKot":21702,"Ġtraders":21703,"olitics":21704,"unker":21705,"ĠPale":21706,"osi":21707,"anmar":21708,"Ġ1947":21709,"ĠFell":21710,"estial":21711,"itating":21712,"GF":21713,"ĠSr":21714,"ifted":21715,"Ġconnector":21716,"ĠBone":21717,"illes":21718,"260":21719,"hma":21720,"Ġoverlap":21721,"ĠGitHub":21722,"Ġcleaner":21723,"ĠBaptist":21724,"ĠWAS":21725,"Ġlungs":21726,"Ñģ":21727,"ĠBUT":21728,"Ġcite":21729,"Ġpitched":21730,"reatment":21731,"Ġtrophies":21732,"ĠNu":21733,"386":21734,"ĠPride":21735,"Ġattendees":21736,"[]":21737,"179":21738,"Ġspatial":21739,"Ġprizes":21740,"ĠReligion":21741,"Ġshowcase":21742,"ĠCategory":21743,"vidia":21744,"Target":21745,"Property":21746,"?,":21747,"Ġfusion":21748,"pie":21749,"ĠUCLA":21750,"Ġsoundtrack":21751,"Ġprincess":21752,"ĠCaval":21753,"should":21754,"Ġlimbs":21755,"Background":21756,"Ġlonely":21757,"Ġcores":21758,"ĠTail":21759,"sheet":21760,"Ġ132":21761,"Ra":21762,"ãĤ«":21763,"ĠBolt":21764,"Ġbooked":21765,"Ġadminister":21766,"Ġequals":21767,"wy":21768,"Ġobserving":21769,"ĠBaron":21770,"ĠAdobe":21771,"Ġvirgin":21772,"ĠSocialist":21773,"Move":21774,"ghazi":21775,"ĠLinda":21776,"212":21777,"Ġbrewing":21778,"Ġmerchants":21779,"burse":21780,"Ġdivor":21781,"Ġmetals":21782,"ĠNer":21783,"Ġsums":21784,"ĠEnemy":21785,"Ġenvision":21786,"Ġgranting":21787,"ĠHoney":21788,"ĠSkyrim":21789,"Ġsocio":21790,"graded":21791,"Ġselective":21792,"WASHINGTON":21793,"Ġ1948":21794,"ĠSirius":21795,"ĠGross":21796,"activity":21797,"ĠIvan":21798,"Ġfurious":21799,"BSD":21800,"ĠPrevious":21801,"Ġresponsive":21802,"Ġcharitable":21803,"Ġleaning":21804,"ĠPew":21805,"Ġviolates":21806,"\\\\\\\\\\\\\\\\":21807,"ĠComing":21808,"wire":21809,"Ġpoet":21810,"Ġresolutions":21811,"command":21812,"ĠPortuguese":21813,"Ġnickname":21814,"Ġdeaf":21815,"February":21816,"Ġrecognise":21817,"Ġentirety":21818,"Ġseasonal":21819,"placed":21820,"ĠTelegraph":21821,"Ġmicrophone":21822,"ouring":21823,"Ġgrains":21824,"Ġgoverned":21825,"Ġpostp":21826,"ĠWaters":21827,"inement":21828,"Ġundocumented":21829,"ĠComcast":21830,"Ġfox":21831,"Ġassaults":21832,"reon":21833,"many":21834,"ĠJenkins":21835,"ĠAnyway":21836,"Ġassessments":21837,"Ġdowns":21838,"ĠMouse":21839,"Ġsuperb":21840,"kt":21841,"ĠDow":21842,"Ġtaxation":21843,"401":21844,"Ġsmiles":21845,"Ġundertaken":21846,"Ġexh":21847,"Ġenthusiastic":21848,"Ġtwent":21849,"Ġgovernmental":21850,"Ġautonomy":21851,"ĠTechnologies":21852,"ĠChain":21853,"Ġprevalent":21854,"fb":21855,"Ġnicotine":21856,"ogram":21857,"job":21858,"Ġawaiting":21859,"ĠMenu":21860,"Ġdeputies":21861,"kov":21862,"ishops":21863,"Button":21864,"ĠShanghai":21865,"Ġdiesel":21866,"ĠDuck":21867,"Ryan":21868,"ĠPCs":21869,"NF":21870,"jury":21871,"ente":21872,"Ġinaccurate":21873,"eddy":21874,"Whatever":21875,"Ġshowc":21876,"ĠNad":21877,"odus":21878,"etr":21879,"Ġplaintiffs":21880,"ĠWOR":21881,"ĠAssange":21882,"Ġprivat":21883,"Ġpremiums":21884,"Ġtam":21885,"URL":21886,"Ġelites":21887,"ĠRanger":21888,"ottenham":21889,"ĠHoff":21890,"ĠAthens":21891,"Ġdefinite":21892,"Ġsighed":21893,"Ġevenly":21894,"211":21895,"ĠAmber":21896,"akia":21897,"Ġmailing":21898,"Ġcrashing":21899,"ĠConfederate":21900,"rugged":21901,"Wal":21902,"ĠDepths":21903,"Ġjuvenile":21904,"Ġreactor":21905,"Introduction":21906,"ĠDeluxe":21907,"1995":21908,"ĠSanchez":21909,"ĠMead":21910,"ivable":21911,":-":21912,"ĠPlanning":21913,"ĠTrap":21914,"quin":21915,"ĠProtect":21916,"vered":21917,"Information":21918,"Ġkidney":21919,"innamon":21920,"las":21921,"Ġpolicing":21922,"Ġtolerate":21923,"ĠQi":21924,"Ġbiased":21925,"Fort":21926,"ĠKi":21927,"save":21928,"Ġprivileged":21929,"Ġbeasts":21930,"ĠGlas":21931,"ĠCinem":21932,"Ġcomeback":21933,"Sunday":21934,"Ġextinction":21935,"hops":21936,"Ġtransmit":21937,"Ġdoubles":21938,"ĠFlat":21939,"167":21940,"Ġdisputed":21941,"Ġinjustice":21942,"foo":21943,"Vict":21944,"roleum":21945,"ĠJulie":21946,"Context":21947,"ĠRarity":21948,"issue":21949,"Component":21950,"Ġcounseling":21951,"anne":21952,"dark":21953,"Ġobjections":21954,"uilt":21955,"Ġgast":21956,"Ġplac":21957,"Ġunused":21958,"ãĥĩ":21959,"ĠTrial":21960,"ĠJas":21961,"hedral":21962,"obb":21963,"Ġtemporal":21964,"ĠPRO":21965,"ĠNW":21966,"ĠAnniversary":21967,"Large":21968,"Ġtherm":21969,"Ġdavid":21970,"Ġsystemic":21971,"ĠShir":21972,"mut":21973,"ĠNept":21974,"address":21975,"Ġscanning":21976,"Ġunderstandable":21977,"Ġcanvas":21978,"Cat":21979,"ĠZoo":21980,"Ġangels":21981,"LO":21982,"ĠStatement":21983,"ĠSig":21984,"ovable":21985,"ĠAway":21986,"sharing":21987,"ocrats":21988,"stated":21989,"Ġweighing":21990,"Nor":21991,"wild":21992,"Bey":21993,"Ġastonishing":21994,"ĠReynolds":21995,"Ġopener":21996,"Ġtrainer":21997,"Ġsurgical":21998,"pn":21999,"Ġadjusting":22000,"wheel":22001,"Ġfrown":22002,"ervative":22003,"Ġsuspend":22004,"Within":22005,"tein":22006,"Ġobstacle":22007,"Ġliberties":22008,"ymes":22009,"Ġuranium":22010,"ansom":22011,"anol":22012,"uba":22013,"ĠLoss":22014,"Ġarous":22015,"ĠHenderson":22016,"Wow":22017,"spl":22018,"cur":22019,"ĠÂŃ":22020,"Ġtheirs":22021,"Damage":22022,"Ġdownloading":22023,"Ġdiscern":22024,"ĠSto":22025,"ĠFla":22026,"Ġhath":22027,"ĠAj":22028,"Ġunpleasant":22029,"European":22030,"expensive":22031,"Ġscreenshot":22032,"ĠUV":22033,"Ġallied":22034,"ĠPersian":22035,"Ġmonopoly":22036,"Ġatom":22037,"ĠRedskins":22038,"\"><":22039,"Ġcancell":22040,"Ġcinema":22041,"131":22042,"fair":22043,"ĠAlfred":22044,"Ġduck":22045,"args":22046,"223":22047,"ĠISI":22048,"Ġsignaling":22049,"inar":22050,"Ġlaughs":22051,"Ġforwards":22052,"Ġreckless":22053,"Ġlisteners":22054,"ativity":22055,"Ġvastly":22056,"nant":22057,"Less":22058,"ĠHunting":22059,"ĠScientific":22060,"ITED":22061,"Ġknight":22062,"ĠHTC":22063,"usa":22064,"tmp":22065,"Ġrude":22066,"ĠLegendary":22067,"Ġarises":22068,"Bad":22069,"ĠClaim":22070,"peg":22071,"Ġrealities":22072,"Think":22073,"Ġ°":22074,"Ġrode":22075,"Ġstrive":22076,"Ġanecd":22077,"Ġshorts":22078,"Ġhypothes":22079,"Ġcoordinated":22080,"ĠGandhi":22081,"ĠFPS":22082,"RED":22083,"Ġsusceptible":22084,"Ġshrink":22085,"ĠChart":22086,"Help":22087,"Ġion":22088,"deep":22089,"ribes":22090,"ĠKai":22091,"ĠCustomer":22092,"Summary":22093,"Ġcough":22094,"wife":22095,"Ġlend":22096,"Ġpositioning":22097,"Ġlottery":22098,"ĠCanyon":22099,"Ġfade":22100,"Ġbronze":22101,"ĠKenny":22102,"Ġboasts":22103,"ĠEnhanced":22104,"record":22105,"Ġemergence":22106,"Ġakin":22107,"ĠBert":22108,"itous":22109,"âĸij":22110,"Ġstip":22111,"Ġexchanged":22112,"omore":22113,"alsh":22114,"Ġreservoir":22115,"Ġstandpoint":22116,"WM":22117,"Ġinitiate":22118,"Ġdecay":22119,"Ġbrewery":22120,"Ġterribly":22121,"Ġmortal":22122,"levard":22123,"Ġrevis":22124,"NI":22125,"elo":22126,"Ġconfess":22127,"ĠMSNBC":22128,"Ġsubmissions":22129,"Controller":22130,"Ġ202":22131,"ĠRuth":22132,"});":22133,"ĠAzure":22134,"Ġ.\"":22135,"206":22136,"ĠMarketing":22137,"Ġlaund":22138,"iencies":22139,"Ġrenowned":22140,"ĠTrou":22141,"ĠNGO":22142,"blems":22143,"Ġterrified":22144,"Ġwarns":22145,"Ġpert":22146,"Ġunsure":22147,"480":22148,"alez":22149,"ultz":22150,"ĠOutside":22151,"Ġstyl":22152,"ĠUnderground":22153,"Ġpanc":22154,"Ġdictionary":22155,"Ġfoe":22156,"riminal":22157,"ĠNorwegian":22158,"Ġjailed":22159,"Ġmaternal":22160,"ée":22161,"ĠLucy":22162,"cop":22163,"Cho":22164,"Ġunsigned":22165,"ĠZelda":22166,"ĠInsider":22167,"ĠContinued":22168,"Ġ133":22169,"ĠNaruto":22170,"ĠMajority":22171,"169":22172,"ĠWo":22173,"ãĤĵ":22174,"Ġpastor":22175,"Ġinformal":22176,"н":22177,"anthrop":22178,"join":22179,"ãģĹ":22180,"itational":22181,"NP":22182,"ĠWriting":22183,"fn":22184,"ĠBever":22185,"195":22186,"Ġyelling":22187,"Ġdrastically":22188,"Ġeject":22189,"Ġneut":22190,"Ġthrive":22191,"ĠFrequ":22192,"oux":22193,"Ġpossesses":22194,"ĠSenators":22195,"ĠDES":22196,"ĠShakespeare":22197,"ĠFranco":22198,"ĠLB":22199,"uchi":22200,"Ġincarn":22201,"Ġfounders":22202,"Function":22203,"Ġbrightness":22204,"ĠBT":22205,"Ġwhale":22206,"ĠTheater":22207,"mass":22208,"ĠDoll":22209,"Something":22210,"Ġechoed":22211,"ĠHex":22212,"crit":22213,"afia":22214,"Ġgoddess":22215,"Ġeleven":22216,"ĠPreview":22217,"ĠAurora":22218,"Ġ401":22219,"ulsive":22220,"ĠLogan":22221,"inburgh":22222,"ĠCenters":22223,"ĠONLY":22224,"ĠAid":22225,"Ġparadox":22226,"Ġhurd":22227,"ĠLC":22228,"Due":22229,"court":22230,"Ġoffended":22231,"Ġevaluating":22232,"ĠMatthews":22233,"Ġtomb":22234,"Ġpayroll":22235,"Ġextraction":22236,"ĠHands":22237,"ifi":22238,"Ġsupernatural":22239,"ĠCOMM":22240,"]=":22241,"dogs":22242,"Ġ512":22243,"ĠMeeting":22244,"Richard":22245,"ĠMaximum":22246,"Ġideals":22247,"Things":22248,"mand":22249,"ĠRegardless":22250,"Ġhumili":22251,"buffer":22252,"Little":22253,"ĠDani":22254,"ĠNak":22255,"Ġliberation":22256,"ĠAbe":22257,"ĠOL":22258,"Ġstuffed":22259,"aca":22260,"inda":22261,"raphic":22262,"Ġmosqu":22263,"Ġcampaigning":22264,"Ġoccupy":22265,"Squ":22266,"rina":22267,"ĠWel":22268,"ĠVS":22269,"Ġphysic":22270,"Ġpuls":22271,"rint":22272,"oaded":22273,"ETF":22274,"ĠArchives":22275,"Ġvenues":22276,"hner":22277,"ĠTurbo":22278,"Ġlust":22279,"Ġappealed":22280,"quez":22281,"ilib":22282,"ĠTimothy":22283,"Ġomn":22284,"dro":22285,"Ġobsession":22286,"ĠSavage":22287,"1996":22288,"Global":22289,"Jes":22290,"214":22291,"Ġsliding":22292,"Ġdisappro":22293,"ĠMagical":22294,"Ġvoluntarily":22295,"gb":22296,"aney":22297,"Ġprophet":22298,"ĠRein":22299,"ĠJulia":22300,"ĠWorth":22301,"aurus":22302,"Ġbounds":22303,"ieu":22304,")))":22305,"Ġcrore":22306,"ĠCitizen":22307,"Sky":22308,"Ġcolumnist":22309,"Ġseekers":22310,"ondo":22311,"ISA":22312,"ĠLength":22313,"Ġnostalg":22314,"Ġnewcom":22315,"Ġdetrim":22316,"entric":22317,"375":22318,"ĠGE":22319,"Ġautop":22320,"Ġacademics":22321,"AppData":22322,"ĠShen":22323,"Ġidiot":22324,"ĠTransit":22325,"Ġteaspoon":22326,"Wil":22327,"KO":22328,"ĠComedy":22329,">,":22330,"Ġpopulated":22331,"WD":22332,"Ġpigs":22333,"ĠOculus":22334,"Ġsympathetic":22335,"Ġmarathon":22336,"198":22337,"Ġseizure":22338,"sided":22339,"Ġdop":22340,"irtual":22341,"Land":22342,"ĠFloor":22343,"osaurs":22344,"...]":22345,"Ġlos":22346,"Ġsubsidiary":22347,"EY":22348,"ĠParts":22349,"ĠStef":22350,"ĠJudiciary":22351,"Ġ134":22352,"Ġmirrors":22353,"Ġket":22354,"times":22355,"Ġneurolog":22356,"Ġcav":22357,"ĠGuest":22358,"Ġtumor":22359,"scill":22360,"ĠLloyd":22361,"Est":22362,"Ġclearer":22363,"Ġstereotypes":22364,"Ġdur":22365,"nothing":22366,"Reddit":22367,"Ġnegotiated":22368,"------------------------":22369,"235":22370,"Ġflown":22371,"ĠSeoul":22372,"ĠResident":22373,"ĠSCH":22374,"Ġdisappearance":22375,"ĠVince":22376,"grown":22377,"Ġgrabs":22378,"ril":22379,"ĠInfinite":22380,"ĠTwenty":22381,"Ġpedestrian":22382,"Ġjersey":22383,"ĠFur":22384,"ĠInfinity":22385,"ĠElliott":22386,"Ġmentor":22387,"Ġmorally":22388,"Ġobey":22389,"secure":22390,"iffe":22391,"Ġantibiotics":22392,"angled":22393,"ĠFreeman":22394,"ĠIntroduction":22395,"Jun":22396,"Ġmarsh":22397,"icans":22398,"ĠEVENTS":22399,"ochond":22400,"Wall":22401,"iculty":22402,"Ġmisdemeanor":22403,"Ġly":22404,"Thomas":22405,"ĠResolution":22406,"Ġanimations":22407,"ĠDry":22408,"Ġintercourse":22409,"ĠNewcastle":22410,"ĠHog":22411,"ĠEquipment":22412,"177":22413,"Ġterritorial":22414,"Ġarchives":22415,"203":22416,"Filter":22417,"ĠMunich":22418,"Ġcommanded":22419,"ĠWand":22420,"Ġpitches":22421,"ĠCroat":22422,"Ġratios":22423,"ĠMits":22424,"Ġaccumulated":22425,"ĠSpecifically":22426,"Ġgentleman":22427,"acerb":22428,"Ġpenn":22429,"Ġaka":22430,"ĠFuk":22431,"Ġintervene":22432,"ĠRefuge":22433,"ĠAlzheimer":22434,"Ġsuccession":22435,"ohan":22436,"does":22437,"Lord":22438,"Ġseparat":22439,"Ġcorrespondence":22440,"Ġshiny":22441,"Prior":22442,"Ġsulf":22443,"Ġmiserable":22444,"Ġdedication":22445,"().":22446,"Ġspecialists":22447,"Ġdefects":22448,"ĠCult":22449,"ĠXia":22450,"Ġjeopard":22451,"ĠOre":22452,"Ability":22453,"Ġlear":22454,"Ġambitions":22455,"ĠBMI":22456,"ĠArabs":22457,"Ġ1942":22458,"Ġpreservation":22459,"ificate":22460,"Ġashamed":22461,"loss":22462,"ĠRestaur":22463,"Ġresemble":22464,"Ġenrich":22465,"ĠKN":22466,"ĠClan":22467,"float":22468,"Ġplayable":22469,"ITT":22470,"Ġharmony":22471,"arrison":22472,"ĠWeinstein":22473,"were":22474,"Ġpoisoning":22475,"ĠComput":22476,"ĠWordPress":22477,"major":22478,"ĠValve":22479,"Fan":22480,"ĠThrow":22481,"ĠRomans":22482,"ĠDepression":22483,"ados":22484,"Ġtortured":22485,"Ġbalancing":22486,"bottom":22487,"Ġacquiring":22488,"ĠMonte":22489,"ardi":22490,"Ġaura":22491,"Ġ##":22492,"ĠStanding":22493,"ĠAtlas":22494,"CF":22495,"Ġintrins":22496,"ĠBenghazi":22497,"Ġcamping":22498,"Ġtapped":22499,"blade":22500,"strous":22501,"ĠRabb":22502,"ĠWritten":22503,"tip":22504,"ĠNeigh":22505,"sterdam":22506,"ĠAllow":22507,"ĠHealing":22508,"ĠRhod":22509,"num":22510,"Ġcaffeine":22511,"ĠPercent":22512,"Ġboo":22513,"Ġapples":22514,"305":22515,"Ġwelcoming":22516,"Ġapplaud":22517,"Ġausterity":22518,"±":22519,"ĠReality":22520,"efe":22521,"å®":22522,"Ġsucks":22523,"Ġtabs":22524,"ĠPayPal":22525,"Ġbackpack":22526,"Ġgifted":22527,"abulary":22528,"ĠScout":22529,"irteen":22530,"Ġchin":22531,"Ġomitted":22532,"Ġnegatively":22533,"Ġaccessing":22534,"ĠEarn":22535,"Ġambulance":22536,"Ġheadphones":22537,"Ġ205":22538,"ĠRefresh":22539,"president":22540,"ĠKitchen":22541,"ĠEntered":22542,"ĠSnyder":22543,"005":22544,"omical":22545,"Ġborrowed":22546,"ĠNem":22547,"Ġaviation":22548,"Ġstall":22549,"rimination":22550,"Ġuniforms":22551,"itime":22552,"ĠSimmons":22553,"energy":22554,"ablished":22555,"yy":22556,"qualified":22557,"Ġrallies":22558,"ĠStuart":22559,"flight":22560,"Ġgangs":22561,"rag":22562,"Ġvault":22563,"lux":22564,"ĠCompar":22565,"Ġdesignation":22566,"209":22567,"ĠJos":22568,"dollar":22569,"zero":22570,"Ġwells":22571,"303":22572,"Ġconstituents":22573,"Ġheck":22574,"Ġcows":22575,"Ġcommanders":22576,"Ġdifferential":22577,"ĠCatherine":22578,"299":22579,"Ġvalve":22580,"Ġbrace":22581,"Ġperspectives":22582,"cert":22583,"fact":22584,"icularly":22585,"ĠMcN":22586,"planes":22587,"Ġintric":22588,"Ġpeas":22589,"ovan":22590,"Ġtossed":22591,"retch":22592,"ĠLopez":22593,"Ġunfamiliar":22594,"death":22595,"ĠApart":22596,"ĠChang":22597,"Ġrelieved":22598,"rophe":22599,"Ġairports":22600,"Ġfreak":22601,"util":22602,"Mill":22603,"ĠChin":22604,"ĠOwen":22605,"male":22606,"ĠBroken":22607,"ĠWinds":22608,"rob":22609,"rising":22610,"Ġfirefighters":22611,"Ġauthoritarian":22612,"Ġ148":22613,"Bitcoin":22614,"external":22615,"Ġbrowsers":22616,"ichever":22617,"orian":22618,"Ġunb":22619,"Ġpoke":22620,"ĠZot":22621,"Mid":22622,"ĠPopular":22623,"Ġcovert":22624,"Ġcontributes":22625,"Ġ650":22626,"Ġcontention":22627,"Gate":22628,"Ġconsoles":22629,"Ġchromos":22630,"ĠIX":22631,"Ġvisually":22632,"ĠEisen":22633,"Ġjewelry":22634,"Ġdelegation":22635,"Ġaccelerate":22636,"ĠRiley":22637,"Ġslope":22638,"Ġindoor":22639,"itially":22640,"Ġhugely":22641,"Ġtunnels":22642,"Ġfined":22643,"Ġdirective":22644,"Ġforehead":22645,"ustomed":22646,"Ġskate":22647,"Music":22648,"gas":22649,"Ġrecognizing":22650,"ambo":22651,"Ġoverweight":22652,"ĠGrade":22653,"ÙĬ":22654,"Ġsounding":22655,"Ġlocking":22656,"ĠREM":22657,"Store":22658,"Ġexcav":22659,"ĠLikewise":22660,"ĠLights":22661,"Ġelbow":22662,"ĠSupply":22663,"wic":22664,"Ġhandsome":22665,"1994":22666,"Coll":22667,"Ġadequately":22668,"ĠAssociate":22669,"Ġstrips":22670,"Ġcrackdown":22671,"Ġmarvel":22672,"ĠKun":22673,"Ġpassages":22674,"@@@@":22675,"ĠTall":22676,"Ġthoughtful":22677,"namese":22678,"Ġprostitution":22679,"business":22680,"Ġballistic":22681,"personal":22682,"cig":22683,"izational":22684,"Round":22685,"ĠÂłĠÂłĠÂłĠÂł":22686,"ĠColeman":22687,"Ġadmitting":22688,"ĠPlug":22689,"Ġbitcoins":22690,"ĠSuz":22691,"Ġfairness":22692,"Ġsupplier":22693,"Ġcatastrophic":22694,"ĠHelen":22695,"oqu":22696,"Marc":22697,"ĠArticles":22698,"gie":22699,"Ġendangered":22700,"Ġdestiny":22701,"ĠVolt":22702,"olia":22703,"axis":22704,"Ġcheat":22705,"Ġunified":22706,"ICO":22707,"quote":22708,"302":22709,"ĠSed":22710,"Ġsuppression":22711,"Ġanalyzing":22712,"Ġsquat":22713,"Ġfiguring":22714,"Ġcoordinates":22715,"Ġchunks":22716,"Ġ1946":22717,"Ġsubp":22718,"Ġwiki":22719,"ĠForbes":22720,"ĠJupiter":22721,"ĠErik":22722,"imer":22723,"ĠCommercial":22724,"\\)":22725,"Ġlegitimacy":22726,"Ġdental":22727,"ĠMean":22728,"Ġdeficits":22729,"550":22730,"Originally":22731,"ĠHorror":22732,"Ġcontamination":22733,"llah":22734,"Ġconfisc":22735,"ĠClare":22736,"TB":22737,"ĠFailed":22738,"aned":22739,"Ġruler":22740,"ĠController":22741,"Ġfeminists":22742,"Fix":22743,"gay":22744,"207":22745,"Ġrabbit":22746,"Third":22747,"owntown":22748,"Ġglue":22749,"Ġvolatile":22750,"Ġshining":22751,"Ġfoll":22752,"Ġimpaired":22753,"Ġsupers":22754,"æĪ":22755,"Ġclutch":22756,"ļéĨĴ":22757,"Ġprolet":22758,"Ġ(!":22759,"Ġyelled":22760,"ĠKiev":22761,"ĠErn":22762,"ĠShock":22763,"KB":22764,"Ġsituated":22765,"query":22766,"ĠNas":22767,"Ġannex":22768,"character":22769,"ĠHoliday":22770,"Ġautomation":22771,"ĠJill":22772,"ĠRemastered":22773,"Ġlinem":22774,"Ġwilderness":22775,"ĠHorizon":22776,"ĠGuinea":22777,"AZ":22778,"Ġmainland":22779,"Ġsecrecy":22780,"LEASE":22781,"Ġpunk":22782,"ĠProvince":22783,"(),":22784,"Speed":22785,"Ġhanding":22786,"ĠSebast":22787,"Sir":22788,"rase":22789,"Ġjournals":22790,"Ġcongest":22791,"ĠTut":22792,"irrel":22793,"Ġschizophrenia":22794,"Ġmisogyn":22795,"healthy":22796,"Iron":22797,"Ġreacted":22798,"-$":22799,"252":22800,"Ġplural":22801,"Ġplum":22802,"Ġbargain":22803,"Ġgrounded":22804,"finder":22805,"Ġdisse":22806,"ĠLaz":22807,"OOD":22808,"Ġatroc":22809,"Factory":22810,"Ġminions":22811,"Ġori":22812,"ĠBrave":22813,"ĠPRE":22814,"ĠMyanmar":22815,"ĠHod":22816,"Ġexpedition":22817,"Ġexplode":22818,"ĠCoord":22819,"Ġextr":22820,"ĠBrief":22821,"ĠADHD":22822,"Ġhardcore":22823,"feeding":22824,"Ġdile":22825,"ĠFruit":22826,"Ġvaccination":22827,"ĠMao":22828,"osphere":22829,"Ġcontests":22830,"-|":22831,"Ġfren":22832,"isphere":22833,"Rom":22834,"ĠSharp":22835,"ĠTrend":22836,"Ġdisconnect":22837,"âĢ¢âĢ¢":22838,"Ġpersecution":22839,"Earth":22840,"Ġhealthier":22841,"384":22842,"Ġcob":22843,"ĠTrinity":22844,"OWS":22845,"ANN":22846,"Ġspecialty":22847,"Ġgru":22848,"Ġcooperative":22849,"why":22850,"Starting":22851,"ĠIssues":22852,"stre":22853,"ensor":22854,"Ġ185":22855,"Adv":22856,"!?":22857,"ĠRevel":22858,"emia":22859,"ĠHulk":22860,"Ġcelebrations":22861,"ĠSou":22862,"raud":22863,"ĠKlein":22864,"Ġunreal":22865,"context":22866,"Ġpartnerships":22867,"Ġadopting":22868,"tical":22869,"Ġsplash":22870,"ĠHezbollah":22871,"category":22872,"cyclop":22873,"xton":22874,"ĠDot":22875,"urdy":22876,"tz":22877,"Ġenvelope":22878,"ĠNL":22879,"âķ":22880,"Ġwherein":22881,"Spec":22882,"184":22883,"Ġtelev":22884,"aliation":22885,"Ġmyths":22886,"å°":22887,"Ġrigorous":22888,"Ġcommunicating":22889,"Ġobserver":22890,"Ġrehe":22891,"ĠWash":22892,"Ġapologized":22893,"ĠTin":22894,"Ġexpenditures":22895,"workers":22896,"document":22897,"Ġhesitate":22898,"ĠLenin":22899,"Ġunpredictable":22900,"Ġrenewal":22901,"cler":22902,"okia":22903,"ĠCONT":22904,"Ġpostseason":22905,"Tokens":22906,"Ġexacerb":22907,"Ġbetting":22908,"Ġ147":22909,"Ġelevation":22910,"Wood":22911,"ĠSolomon":22912,"194":22913,"004":22914,"output":22915,"Ġredund":22916,"ĠMumbai":22917,"ĠpH":22918,"Ġreproduce":22919,"ĠDuration":22920,"MAX":22921,"Ġbog":22922,"CBS":22923,"ĠBalance":22924,"ĠSgt":22925,"ĠRecent":22926,"Ġcd":22927,"Ġpopped":22928,"Ġincompet":22929,"prop":22930,"ayan":22931,"guy":22932,"Pacific":22933,"Ġtyr":22934,"Ġ{{":22935,"ĠMystic":22936,"ĠDana":22937,"Ġmasturb":22938,"Ġgeometry":22939,"â":22940,"ĠCorrect":22941,"Ġtrajectory":22942,"Ġdistracted":22943,"Ġfoo":22944,"ĠWelsh":22945,"Luc":22946,"mith":22947,"Ġrugby":22948,"Ġrespiratory":22949,"Ġtriangle":22950,"Ġ215":22951,"Ġundergraduate":22952,"ĠSuperior":22953,"changing":22954,"_-":22955,"Ġrightly":22956,"Ġreferee":22957,"Ġlucrative":22958,"Ġunauthorized":22959,"Ġresembles":22960,"ĠGNU":22961,"ĠDerby":22962,"Ġpathways":22963,"ĠLed":22964,"Ġendurance":22965,"Ġstint":22966,"Ġcollector":22967,"Fast":22968,"Ġdots":22969,"Ġnationals":22970,"ĠSecurities":22971,"Ġwhip":22972,"Param":22973,"Ġlearns":22974,"Magic":22975,"Ġdetailing":22976,"moon":22977,"Ġbroadcasting":22978,"Ġbaked":22979,"265":22980,"holm":22981,"ĠSah":22982,"ĠHussein":22983,"ĠCourtesy":22984,"174":22985,"Ġ146":22986,"Ġgeographic":22987,"peace":22988,"Ġjudging":22989,"ĠStern":22990,"Bur":22991,"Ġstoryline":22992,"Gun":22993,"ĠStick":22994,"245":22995,"307":22996,"ãĤ´ãĥ³":22997,"ĠAdministrator":22998,"Ġburnt":22999,"Ġpave":23000,"choes":23001,"Exec":23002,"Ġcampuses":23003,"Result":23004,"Ġmutations":23005,"ĠCharter":23006,"Ġcaptures":23007,"Ġcompares":23008,"Ġbadge":23009,"Scient":23010,"Ġerad":23011,"iery":23012,"oi":23013,"ettes":23014,"ĠEstate":23015,"Ġstrap":23016,"Ġproudly":23017,"Ġfried":23018,"Ġwithdrawn":23019,"ĠVoy":23020,"phony":23021,"Items":23022,"ĠPierce":23023,"bard":23024,"Ġannotation":23025,"anton":23026,"illon":23027,"Impro":23028,"...)":23029,"Ġhappier":23030,"------":23031,"adjust":23032,"Ġstaffers":23033,"Ġactivism":23034,"Ġperf":23035,"Ġalright":23036,"Need":23037,"Ġcommence":23038,"Ġopioid":23039,"ĠAmanda":23040,"Es":23041,"ĠPars":23042,"ĠKaw":23043,"Works":23044,"248":23045,"Ġindo":23046,"tc":23047,"endant":23048,"ĠMoto":23049,"Ġlegalization":23050,"OTE":23051,"Ġtasked":23052,"Ġtsp":23053,"ĠACTIONS":23054,"166":23055,"Ġrefreshing":23056,"ĠNR":23057,"ĠPerez":23058,"Ġinfringement":23059,"SY":23060,"Listen":23061,"inning":23062,"ku":23063,"Ġrotate":23064,"program":23065,"arah":23066,"Design":23067,"Ġ(£":23068,"Ġstoring":23069,"Ġwarrants":23070,"Ġjudgement":23071,"ĠBrist":23072,"usually":23073,"photo":23074,"ĠRan":23075,"ĠPine":23076,"Ġoutrageous":23077,"ĠValentine":23078,"luence":23079,"ĠEverybody":23080,"Altern":23081,"Ġrelevance":23082,"Ġterminated":23083,"Ġdessert":23084,"Ġfulfilled":23085,"Ġprosecuted":23086,"ĠWords":23087,"Ġmigrant":23088,"Ġcultivation":23089,"ÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤ":23090,"idelity":23091,"ĠVern":23092,"ĠLogin":23093,"Ġmetaphor":23094,"ĠTip":23095,"Ġrecruits":23096,"ĠPig":23097,"ribing":23098,"Ġenthusiasts":23099,"exper":23100,"Ġfrightening":23101,"ĠHair":23102,"anson":23103,"strate":23104,"Ġhi":23105,"Height":23106,"Ġowning":23107,"none":23108,"Ġdislike":23109,"Ġknives":23110,"pherd":23111,"Ġloudly":23112,"ĠAPIs":23113,"Display":23114,"ĠLac":23115,"ĠUSS":23116,"abl":23117,"verages":23118,"Jew":23119,"Ġ172":23120,"ĠHistorical":23121,"atoon":23122,"ĠPhysics":23123,"intern":23124,"Ġwarmth":23125,"Ġtopp":23126,"DM":23127,"Ġgunman":23128,"Ġemperor":23129,"odi":23130,"ãĥ£":23131,"inatory":23132,"ĠRib":23133,"Ġ131":23134,"ĠSaturn":23135,"ĠShining":23136,"Ġwaking":23137,"Quotes":23138,"Ġcomedian":23139,"enberg":23140,"½":23141,"Ġbelievers":23142,"Ġpaperwork":23143,"custom":23144,"Ġlev":23145,"Ġlament":23146,"Ġpouring":23147,"222":23148,"political":23149,"ĠSupplement":23150,"maid":23151,"Ġcruelty":23152,"Ġtread":23153,"ysics":23154,"Aw":23155,"rites":23156,"Ġmodifier":23157,"ĠPosition":23158,"Adam":23159,"lb":23160,"ubs":23161,"Ġimperfect":23162,"Ġclusters":23163,"ĠEngineer":23164,"ĠCherry":23165,"Ġinauguration":23166,"ĠSau":23167,"Ġembodiment":23168,"ĠUncle":23169,"Ġoverr":23170,"Ġexplosions":23171,"cule":23172,"ĠPrinceton":23173,"ĠAndrea":23174,"Ġincorrectly":23175,"Ġearnest":23176,"Ġpilgr":23177,"ĠSprint":23178,"Ġsleeve":23179,"Ġhears":23180,"ĠAmazing":23181,"Ġbrowsing":23182,"agin":23183,"Ġhomeland":23184,"Ġhaw":23185,"Ġdiving":23186,"istered":23187,"178":23188,"Ġbargaining":23189,"ĠArcade":23190,"Ġdelegate":23191,"terson":23192,"................................................................":23193,"ĠJacksonville":23194,"275":23195,"Ġstagn":23196,"Ġadam":23197,"ĠSherman":23198,"CB":23199,"Ġsuburb":23200,"ĠFoods":23201,"Ġconverting":23202,"ĠArist":23203,"Ġchambers":23204,"love":23205,"Ġamino":23206,"ĠGan":23207,"Ġmadness":23208,"mc":23209,"ĠUSE":23210,"defined":23211,"Ġultr":23212,"indust":23213,"Ġwolves":23214,"lance":23215,"Additionally":23216,"Ġcracks":23217,"asia":23218,"ĠReason":23219,"ĠPump":23220,"Ġaccidental":23221,"ĠLaser":23222,"ĠRid":23223,"Ġinitialized":23224,"elli":23225,"Ġunnamed":23226,"Ġnoun":23227,"ĠPassed":23228,"Ġhostage":23229,"ĠEthiop":23230,"shirts":23231,"Ġunrel":23232,"ĠEmbassy":23233,"Ġ1941":23234,"Ġatoms":23235,"Ġpurported":23236,"164":23237,"ĠFi":23238,"Ġgallons":23239,"ĠMonica":23240,"Ġpg":23241,"enment":23242,"Ġsorted":23243,"ĠGospel":23244,"Ġheights":23245,"Ġtraced":23246,"Ġundergoing":23247,"Shell":23248,"Ġsacks":23249,"Ġproportions":23250,"Ġhalluc":23251,"Font":23252,"acet":23253,"Ġwarmer":23254,"ĠINTER":23255,"Ġgrabbing":23256,"Plug":23257,"Ġrealization":23258,"ĠBurke":23259,"Ġenchant":23260,"ATER":23261,"ĠSeed":23262,"Ġabundant":23263,"FM":23264,"Ġcivic":23265,"Vs":23266,"isi":23267,"Ġvow":23268,"Ġreper":23269,"ĠPartnership":23270,"Ġpenetration":23271,"Ġaxe":23272,"Ġshattered":23273,"ĠZombies":23274,"Ġvinyl":23275,"ĠAlert":23276,"eon":23277,"Ġobliged":23278,"ĠIllust":23279,"ĠPlaza":23280,"ĠFrontier":23281,"Ġdavidjl":23282,"ĠSerial":23283,"ĠHav":23284,"ĠNutrition":23285,"Bi":23286,"ĠâĸĪ":23287,"ĠJays":23288,"linux":23289,"Ġhurry":23290,"Ġvoy":23291,"Ġhopeless":23292,"ĠStealth":23293,"Ġãģ":23294,"essors":23295,"ttle":23296,"borg":23297,"ĠSafari":23298,"fell":23299,"Ġwary":23300,"due":23301,"ĠAbove":23302,"Ha":23303,"ELL":23304,"Ġnotor":23305,"ĠWon":23306,"Too":23307,"Ġoccupations":23308,"Ġpossessions":23309,"Ġinviting":23310,"Ġpredators":23311,"Ġaccelerated":23312,"Ġ157":23313,"uterte":23314,"ĠCube":23315,"east":23316,"account":23317,"Give":23318,"Ġtransplant":23319,"redients":23320,"idable":23321,"Ġscreenshots":23322,"ĠGund":23323,"ĠFS":23324,"Ġtravelers":23325,"Ġsensory":23326,"ĠFiat":23327,"ĠRockets":23328,"İĭ":23329,"_{":23330,"Friend":23331,"Ġcharming":23332,"ALS":23333,"Ġenjoyment":23334,"mph":23335,"Ġ5000":23336,"ĠREG":23337,"ÙĨ":23338,"bia":23339,"Ġcompilation":23340,"rost":23341,"ĠVP":23342,"ĠSchne":23343,"2019":23344,"Ġcopying":23345,"MORE":23346,"ĠFlore":23347,"falls":23348,"215":23349,"total":23350,"Ġdisciples":23351,"double":23352,"Ġexceeding":23353,"Ġsmashed":23354,"Ġconceptual":23355,"ĠRomania":23356,"ĠBrent":23357,"ĠICE":23358,"ĠTou":23359,"Ġgrap":23360,"Ġnails":23361,"189":23362,"ãĥĺ":23363,"Ġprocure":23364,"eur":23365,"Ġconfirming":23366,"ĠCec":23367,"awi":23368,"ĠEden":23369,"Ġng":23370,"Ġengineered":23371,"atics":23372,"Ġhooked":23373,"Ġdisgusting":23374,"ĠMurder":23375,"ãĤ¿":23376,"Library":23377,"Ġ168":23378,"Almost":23379,"hematic":23380,"Menu":23381,"ĠNotre":23382,"ĠJur":23383,"Ġkidnapped":23384,"Ġhacker":23385,"ĠJade":23386,"Ġcreepy":23387,"Ġdrawings":23388,"ĠSponsor":23389,"Ġcyclists":23390,"ĠGoblin":23391,"Ġoptimized":23392,"Ġstaged":23393,"ĠMcD":23394,"between":23395,"Age":23396,"eno":23397,"Sex":23398,"ĠWide":23399,"nings":23400,"avis":23401,"Ġincapable":23402,"ĠKob":23403,"Ġrewarding":23404,"ĠLone":23405,"olescent":23406,"Ġcontracted":23407,"Ġsticky":23408,"Jose":23409,"Ball":23410,"fest":23411,"ĠInput":23412,"ĠRecently":23413,"Ġtomat":23414,"square":23415,"Application":23416,"Ġnitrogen":23417,"Ġduplicate":23418,"ĠRecon":23419,"ĠDear":23420,"London":23421,"Ġintra":23422,"Ġdock":23423,"Ġoutreach":23424,"ĠMillion":23425,"Ġmammals":23426,"ampton":23427,"VAL":23428,"Ġsnaps":23429,"Ġdos":23430,"ĠWhole":23431,"ĠReady":23432,"Try":23433,"ĠWinnipeg":23434,"earance":23435,"Ġincurred":23436,"renched":23437,"ĠNSW":23438,"ilot":23439,"raine":23440,"Ġcube":23441,"got":23442,"Ġrunway":23443,"etermined":23444,"ĠHawks":23445,"Ġsurvivor":23446,"ĠWish":23447,"ĠDin":23448,"ĠDEF":23449,"ĠVault":23450,"187":23451,"Ġmushrooms":23452,"Ġcrisp":23453,"bey":23454,"ĠDiscovery":23455,"Ġdevelopmental":23456,"Ġparadigm":23457,"Ġchaotic":23458,"ĠTsu":23459,"Ġ333":23460,"bons":23461,"Ġbacterial":23462,"Ġcommits":23463,"Ġcosmic":23464,"Ġmega":23465,"ocative":23466,"ĠPaint":23467,"ophobic":23468,"Ġvain":23469,"Ġcarved":23470,"ĠThief":23471,"ĠGul":23472,"owship":23473,"Ġcites":23474,"ĠEdinburgh":23475,"Ġdiminished":23476,"Ġacknowledges":23477,"ĠKills":23478,"Ġmicrow":23479,"ĠHera":23480,"Ġseniors":23481,"Ġwhereby":23482,"Hop":23483,"atron":23484,"Ġunavailable":23485,"ĠNate":23486,"Ġ480":23487,"Ġslated":23488,"ĠRebecca":23489,"ĠBattery":23490,"Ġgrammar":23491,"Ġheadset":23492,"Ġcursor":23493,"Ġexcluding":23494,"anye":23495,"aundering":23496,"ebin":23497,"Ġfeasible":23498,"ĠPublishing":23499,"ĠLabs":23500,"ĠCliff":23501,"ĠFerrari":23502,"Ġpac":23503,"visible":23504,"marked":23505,"pell":23506,"Ġpolite":23507,"Ġstaggering":23508,"ĠGalactic":23509,"Ġsuperst":23510,"Ġparan":23511,"ĠOfficers":23512,"ãĢģ":23513,"Ġspecifics":23514,"ulus":23515,"239":23516,"ĠPaste":23517,"AMP":23518,"ĠPanama":23519,"ĠDelete":23520,"anguard":23521,"restrial":23522,"Ġheroic":23523,"ĠDy":23524,"اÙĦ":23525,"Ġincumbent":23526,"Ġcrunch":23527,"tro":23528,"Ġscoop":23529,"Ġblogger":23530,"Ġsellers":23531,"uren":23532,"Ġmedicines":23533,"ĠCaps":23534,"ĠAnimation":23535,"oxy":23536,"Ġoutward":23537,"Ġinquiries":23538,"229":23539,"Ġpsychologist":23540,"ĠSask":23541,"evil":23542,"Ġcontaminated":23543,"ãĤ¨":23544,"herence":23545,"Ġbranded":23546,"ĠAbdul":23547,"zh":23548,"Ġparagraphs":23549,"Ġmins":23550,"Ġcorrelated":23551,"erb":23552,"Ġimpart":23553,"Ġmilestone":23554,"ĠSolutions":23555,"otle":23556,"Ġundercover":23557,"Ġmarched":23558,"ĠChargers":23559,"fax":23560,"ĠSecrets":23561,"Ġruth":23562,"weather":23563,"Ġfeminine":23564,"Ġsham":23565,"Ġprestigious":23566,"iggins":23567,"Ġsung":23568,"history":23569,"ettle":23570,"ggie":23571,"Ġoutdated":23572,"oland":23573,"Ġperceptions":23574,"ĠSession":23575,"ĠDodgers":23576,"uj":23577,"ĠEND":23578,"Doc":23579,"Ġdeficiency":23580,"Grand":23581,"ĠJoker":23582,"Ġretrospect":23583,"Ġdiagnostic":23584,"Ġharmless":23585,"Ġrogue":23586,"ĠAval":23587,"Equ":23588,"Ġtransc":23589,"ĠRobertson":23590,"ĠDepending":23591,"ĠBurns":23592,"ivo":23593,"Ġhostility":23594,"Features":23595,"ĵĺ":23596,"Ġdiscomfort":23597,"ĠLCD":23598,"specified":23599,"ĠExpect":23600,"340":23601,"Ġimperative":23602,"ĠRegular":23603,"Chinese":23604,"Ġstatewide":23605,"Ġsymm":23606,"Ġloops":23607,"Ġautumn":23608,"Nick":23609,"Ġshaping":23610,"Ġquot":23611,"Ġcherry":23612,"ĠCrossref":23613,"è¦ļéĨĴ":23614,"Standard":23615,"heed":23616,"ĠDell":23617,"ĠVietnamese":23618,"Ġost":23619,"ĠValkyrie":23620,"OA":23621,"Assad":23622,"Ġrebound":23623,"ĠTraffic":23624,"places":23625,"æĺ":23626,"ĠBuc":23627,"172":23628,"Ġshelters":23629,"Ġinsisting":23630,"ĠCertainly":23631,"ĠKenneth":23632,"ĠTCP":23633,"Ġpenal":23634,"ĠReplay":23635,"heard":23636,"Ġdialect":23637,"iza":23638,"ĠFY":23639,"itcher":23640,"ĠDL":23641,"Ġspiral":23642,"Ġquarterbacks":23643,"Ġhull":23644,"Ġgoogle":23645,"Ġtodd":23646,"ĠSterling":23647,"ĠPlate":23648,"Ġspying":23649,"mbol":23650,"ĠRealm":23651,"ĠProced":23652,"ĠCrash":23653,"Ġterminate":23654,"Ġprotesting":23655,"Center":23656,"guided":23657,"Ġuncover":23658,"Ġboycott":23659,"Ġrealizes":23660,"sound":23661,"Ġpretending":23662,"ĠVas":23663,"1980":23664,"Ġframed":23665,"Ġ139":23666,"Ġdescended":23667,"Ġrehabilitation":23668,"Ġborrowing":23669,"ĠBuch":23670,"Ġblur":23671,"Ron":23672,"ĠFrozen":23673,"enza":23674,"Chief":23675,"ĠPoor":23676,"Ġtranslates":23677,"MIN":23678,"Ġ212":23679,"JECT":23680,"Ġerupted":23681,"Ġsuccesses":23682,"SEC":23683,"Ġplague":23684,"Ġgems":23685,"doms":23686,"Ġstretches":23687,"ĠSpy":23688,"Ġstorytelling":23689,"Credit":23690,"ĠPush":23691,"Ġtraction":23692,"Ġineffective":23693,"ĠLuna":23694,"Ġtapes":23695,"Ġanalytics":23696,"ercise":23697,"Ġprogrammes":23698,"ĠCarbon":23699,"Ġbehold":23700,"heavy":23701,"ĠConservation":23702,"ĠFIR":23703,"Ġsack":23704,"termin":23705,"ricks":23706,"Ġhoused":23707,"Ġunusually":23708,"Ice":23709,"Ġexecuting":23710,"ĠMoroc":23711,"eday":23712,"Ġeditions":23713,"Ġsmarter":23714,"ĠBA":23715,"Ġoutlaw":23716,"Ġvanished":23717,"iba":23718,"ALSE":23719,"ĠSilva":23720,"238":23721,"Could":23722,"Ġphilosopher":23723,"Ġevacuated":23724,"Secret":23725,"142":23726,"Ġvisas":23727,"ãĤ¬":23728,"ĠMalt":23729,"ĠClearly":23730,"ĠNiger":23731,"ĠCairo":23732,"ĠFist":23733,"380":23734,"ĠXML":23735,"auto":23736,"itant":23737,"Ġreinforced":23738,"Record":23739,"ĠSurvivor":23740,"GHz":23741,"Ġscrews":23742,"parents":23743,"Ġoceans":23744,"mares":23745,"Ġbrakes":23746,"vasive":23747,"Ġhello":23748,"ĠSIM":23749,"rimp":23750,"Ġore":23751,"ĠArmour":23752,"247":23753,"Ġterrific":23754,"Ġtones":23755,"141":23756,"ĠMinutes":23757,"Episode":23758,"Ġcurves":23759,"Ġinflammatory":23760,"Ġbatting":23761,"ĠBeautiful":23762,"Lay":23763,"Ġunpop":23764,"vable":23765,"Ġriots":23766,"ĠTactics":23767,"baugh":23768,"ĠCock":23769,"Ġorgasm":23770,"ĠSas":23771,"Ġconstructor":23772,"etz":23773,"Gov":23774,"Ġantagon":23775,"Ġtheat":23776,"Ġdeeds":23777,"hao":23778,"cuts":23779,"ĠMcCl":23780,"Ġum":23781,"ĠScientists":23782,"Ġgrassroots":23783,"yssey":23784,"\"]=>":23785,"Ġsurfaced":23786,"Ġshades":23787,"Ġneighbours":23788,"Ġadvertis":23789,"oya":23790,"Ġmerged":23791,"Upon":23792,"Ġgad":23793,"Ġanticipate":23794,"Anyway":23795,"Ġslogan":23796,"Ġdisrespect":23797,"Iran":23798,"ĠTB":23799,"acted":23800,"Ġsubpoen":23801,"mediately":23802,"OOOO":23803,"Ġwaiver":23804,"Ġvulnerabilities":23805,"ottesville":23806,"ĠHuffington":23807,"Josh":23808,"ĠDH":23809,"Monday":23810,"ĠEllen":23811,"Know":23812,"xon":23813,"items":23814,"228":23815,"Ġfills":23816,"ĠNike":23817,"Ġcumulative":23818,"andals":23819,"Ir":23820,"Ġì":23821,"Ġfriction":23822,"igator":23823,"Ġscans":23824,"ĠVienna":23825,"ldom":23826,"Ġperformers":23827,"Prim":23828,"Ġbidding":23829,"Mur":23830,"Ġleaned":23831,"ĠPrix":23832,"alks":23833,"Ġ[â̦]":23834,"ĠTwitch":23835,"ĠDeveloper":23836,"ĠGir":23837,"Ġcallback":23838,"Abstract":23839,"Ġaccustomed":23840,"Ġfreedoms":23841,"ĠPG":23842,"uracy":23843,"Ġlump":23844,"isman":23845,",,,,":23846,"1992":23847,"ĠRED":23848,"Ġworm":23849,"Match":23850,"ĠPlatinum":23851,"IJ":23852,"ĠOwner":23853,"Trivia":23854,"compl":23855,"Ġnewborn":23856,"Ġfantas":23857,"Own":23858,"Ġ1959":23859,"Ġsympath":23860,"Ġubiqu":23861,"Ġoutputs":23862,"Ġallev":23863,"Ġprag":23864,"Kevin":23865,"Ġfavors":23866,"Ġburial":23867,"Ġnurt":23868,"solete":23869,"cache":23870,"Ġ156":23871,"Ġunlocks":23872,"techn":23873,"Making":23874,"Ġconquer":23875,"adic":23876,"æĸ":23877,"Ġelf":23878,"Ġelectorate":23879,"ĠKurds":23880,"ĠStack":23881,"ĠSamurai":23882,"Ġâĺħ":23883,"Ġ{}":23884,"ĠSaid":23885,"ĠFallout":23886,"Ġkindness":23887,"ĠCustoms":23888,"ĠBoulevard":23889,"Ġhelicopters":23890,"otics":23891,"ĠVeget":23892,"comment":23893,"Ġcriticised":23894,"Ġpolished":23895,"ĠRemix":23896,"ĠCultural":23897,"Ġrecons":23898,"Ġdoi":23899,"atem":23900,"Screen":23901,"Ġbarred":23902,"Comments":23903,"ĠGenerally":23904,"Ġslap":23905,"720":23906,"Vari":23907,"pine":23908,"Ġempt":23909,"Ġhats":23910,"ĠPlaying":23911,"lab":23912,"average":23913,"forms":23914,"ĠCotton":23915,"Ġcans":23916,"ĠDON":23917,"ĠSomalia":23918,"Crypt":23919,"ĠIncreases":23920,"Ever":23921,"modern":23922,"Ġsurgeon":23923,"3000":23924,"Ġrandomized":23925,"================================================================":23926,"Bern":23927,"impl":23928,"ĠCOR":23929,"Ġproclaim":23930,"thouse":23931,"Ġtoes":23932,"Ġample":23933,"Ġpreserving":23934,"Ġdisbel":23935,"grand":23936,"Besides":23937,"Ġsilk":23938,"ĠPattern":23939,"hm":23940,"Ġenterprises":23941,"Ġaffidavit":23942,"ĠAdvisory":23943,"Ġadvertised":23944,"ĠReligious":23945,"sections":23946,"psych":23947,"ĠFields":23948,"aways":23949,"Ġhashtag":23950,"ĠNightmare":23951,"Ġvampire":23952,"Ġforensic":23953,"rossover":23954,"nar":23955,"Ġnavy":23956,"Ġvacant":23957,"ĠDuel":23958,"Ġhallway":23959,"Ġfacebook":23960,"identally":23961,"ĠNRA":23962,"Ġmatt":23963,"Ġhurricane":23964,"ĠKirby":23965,"ĠPuzzle":23966,"Ġskirt":23967,"oust":23968,"dullah":23969,"Ġanalogy":23970,"inion":23971,"Ġtomatoes":23972,"ĠNV":23973,"ĠPeak":23974,"ĠMeyer":23975,"Ġappointments":23976,"Ġmasc":23977,"Ġalley":23978,"rehend":23979,"Ġcharities":23980,"Ġundo":23981,"Ġdestinations":23982,"ĠTesting":23983,"\"></":23984,"Ġdestined":23985,"Ġimplements":23986,"ĠHarold":23987,"RECT":23988,"Ġoptimization":23989,"Ġkilometres":23990,"Ġcmd":23991,"Ġimpairment":23992,"Ġunsuccessful":23993,"Ġswiftly":23994,"ĠGlasgow":23995,"arten":23996,"ĠShares":23997,"ĠAnswer":23998,"ĠAlbum":23999,"Ġnutritional":24000,"ãĥĸ":24001,"ĠFut":24002,"Ġbloc":24003,"ĠNFC":24004,"Ġwholesale":24005,"ĠCW":24006,"Ġneglected":24007,"Ġlauncher":24008,"Ġannouncements":24009,"OULD":24010,"comb":24011,"Ġrotating":24012,"Ġrests":24013,"ĠTicket":24014,"chedel":24015,"Lou":24016,"ĠVic":24017,"Ġ\"'":24018,"Ġtemplates":24019,"Ġreplaces":24020,"Arc":24021,"::::":24022,"ĠGilbert":24023,"Ġillnesses":24024,"Ġschedules":24025,"Ġheterosexual":24026,"LINE":24027,"Ġherein":24028,"Ġcoerc":24029,"Ġdecreasing":24030,"Ġdeportation":24031,"sudo":24032,"ĠIndigenous":24033,"Ġweighs":24034,"Along":24035,"');":24036,"ĠBengals":24037,"707":24038,"Ġjoints":24039,"verts":24040,"Ġ149":24041,"naire":24042,"Ġsimplest":24043,"Ġlore":24044,"1080":24045,"fiction":24046,"ĠDatabase":24047,"Ġreservation":24048,"Ġsou":24049,"Ġsanctuary":24050,"audio":24051,"aple":24052,"Ġvegetarian":24053,"Ġanticipation":24054,"micro":24055,"Ġenduring":24056,"Ġdeparted":24057,"Ġsidewalk":24058,"Ġprohibits":24059,"ĠFont":24060,"Ġcompute":24061,"ĠSect":24062,"Ġ158":24063,"Battle":24064,"Ġbomber":24065,"Ġdistraction":24066,"Ġendured":24067,"Ġpractitioners":24068,"Ġdisturbed":24069,"Ġdrank":24070,"ordered":24071,"Ġsurprises":24072,"seat":24073,"Security":24074,"ĠWisdom":24075,"ogo":24076,"Ġsubparagraph":24077,"ĠPeninsula":24078,"ĠOrigins":24079,"iren":24080,"ĠPav":24081,"iggle":24082,"Ġgratitude":24083,"ĠGravity":24084,"overty":24085,"iman":24086,"ctr":24087,"ĠCaesar":24088,"could":24089,"gem":24090,"Ġskies":24091,"Ġchamp":24092,"Ġagreeing":24093,"Family":24094,"Div":24095,"176":24096,"Ġmessy":24097,"umption":24098,"Federal":24099,"erno":24100,"ĠChat":24101,"Beyond":24102,"Ġdevote":24103,"ĠWalsh":24104,"Ġdumped":24105,"Ġaccumulation":24106,"stad":24107,"hibition":24108,"Ġsmokers":24109,"Ġinspector":24110,"French":24111,"issan":24112,"ĠVita":24113,"Ġresearching":24114,"RAM":24115,"ĠCeltics":24116,"Ġcloak":24117,"ĠTerra":24118,"Mary":24119,"sold":24120,"ĠDOM":24121,"mods":24122,"Intel":24123,"Ġmultitude":24124,"ĠImproved":24125,"Ġreliance":24126,"Ġartifact":24127,"Ġalarming":24128,"Prom":24129,"hon":24130,"TION":24131,"medium":24132,"Ġreflex":24133,"ĠExcel":24134,"Ġweakened":24135,"163":24136,"224":24137,"Ġcostumes":24138,"Ġuniquely":24139,"Ġsorrow":24140,"Ġmansion":24141,"wp":24142,"Ġsalv":24143,"ĠGrove":24144,"bsp":24145,"ĠSniper":24146,"ĠShipping":24147,"ĠPOW":24148,"Ġundis":24149,"Ġbranding":24150,"Girl":24151,"ĠAhmad":24152,"ĠLakes":24153,"ĠCorey":24154,"Ġinheritance":24155,"enery":24156,"Ġpacking":24157,"ĠPrest":24158,"Dest":24159,"FW":24160,"Ġregulator":24161,"locked":24162,"Ġcontested":24163,"ĠMelissa":24164,"ĠDuc":24165,"Ġunpopular":24166,"Ġstacked":24167,"Ġ1917":24168,"Ġyearly":24169,"Ġstare":24170,"Ġassessing":24171,"ø":24172,"Ġbeverages":24173,"Ġcompetitions":24174,"Ġstrengthening":24175,"along":24176,"ĠLud":24177,"Ġmelted":24178,"stanbul":24179,"Ġbounty":24180,"ENC":24181,"ĠLands":24182,"Ġdeclares":24183,"Ġcustomize":24184,"Ġcomposite":24185,"ãĥ¬":24186,"CM":24187,"ographics":24188,"ĠTemp":24189,"Ġcontender":24190,"Ġinsign":24191,"ĠLAN":24192,"Ġdisasters":24193,"inspired":24194,"Ġjudgments":24195,"ustainable":24196,"ursion":24197,"Ġvariance":24198,"ĠUltimately":24199,"Ġ--------":24200,"uador":24201,"ĠRX":24202,"Ġmelting":24203,"ĠExtended":24204,"ĠTwe":24205,"Major":24206,"ĠBil":24207,"Ġsyrup":24208,"quick":24209,"ĠHolder":24210,"Ġinnocence":24211,"ULE":24212,"ĠMight":24213,"9999":24214,"Ġfal":24215,"Ġcontinuity":24216,"Ġ1953":24217,"ĠBS":24218,"still":24219,"Lat":24220,"ĠAbuse":24221,"Ġunsupported":24222,"xxxxxxxx":24223,"Ġinstitute":24224,"Ġfragment":24225,"ĠPep":24226,"Western":24227,"ĠCause":24228,"ĠFrag":24229,"ĠArs":24230,"à¥":24231,"astics":24232,"Ġbishop":24233,"Ġcrosses":24234,"Ġ154":24235,"ĠUpgrade":24236,"Ġmitigate":24237,"ĠRaymond":24238,"Mods":24239,"Ġtomato":24240,"Ġstumbled":24241,"Ġdiffers":24242,"Initial":24243,"ĠRaspberry":24244,"Ġignores":24245,"Ġtant":24246,"Ãł":24247,"Ġrelay":24248,"Ġbisexual":24249,"Ġconfession":24250,"Ġdement":24251,"inas":24252,"ĠHeather":24253,"platform":24254,"driving":24255,"bourg":24256,"ĠMush":24257,"Ġhyster":24258,"Details":24259,"Ġdrift":24260,"ĠWald":24261,"ĠLuckily":24262,"orf":24263,"Ġexpire":24264,"ĠPunch":24265,"zyme":24266,"gold":24267,"Ġunpaid":24268,"ĠTrent":24269,"Ġunarmed":24270,"Ġillicit":24271,"ĠTottenham":24272,"Ġsmash":24273,"International":24274,"inker":24275,"Ġsting":24276,"ĠSaddam":24277,"ĠART":24278,"Ġtruths":24279,"birth":24280,"Ġsober":24281,"ĠNit":24282,"Ġib":24283,"Ġusable":24284,"Ġstacks":24285,"ĠSylv":24286,"Ġnortheast":24287,"Ġdomination":24288,"ĠMour":24289,"ENSE":24290,"ĠMeasure":24291,"Ġprogrammer":24292,"Ġ<-":24293,"182":24294,"ĠCondition":24295,"Ġbackyard":24296,"irling":24297,"ĠJeb":24298,"ĠCreed":24299,"ĠHang":24300,"ĠCOMP":24301,"FER":24302,"ĠIsh":24303,"Ġdetectives":24304,"---------------":24305,"ĠMessenger":24306,"Ġlooph":24307,"Ġgateway":24308,"151":24309,"ĠMaterials":24310,"ĠDT":24311,"Ġdoomed":24312,"odo":24313,"Ġslices":24314,"Ġemailed":24315,"ĠPerl":24316,"Ġrenov":24317,"UTH":24318,"odynam":24319,"ĠSouthwest":24320,"getic":24321,"ĠTPP":24322,"Ġoptimism":24323,"ĠTow":24324,"ulators":24325,"protected":24326,"yles":24327,"«":24328,"Ġexile":24329,"env":24330,"Prop":24331,"ĠZimmerman":24332,"Ùİ":24333,"Ca":24334,"omaly":24335,"ãĥĨ":24336,"Ġrailroad":24337,"Lee":24338,"232":24339,"Ġreplicate":24340,"Ġcomfortably":24341,"actly":24342,"Ġrav":24343,"Ġtelescope":24344,"Ġhonesty":24345,"ĠPepper":24346,"ĠBring":24347,"Ġrichest":24348,"Ġoutdoors":24349,"Ġhalls":24350,"Ġcontend":24351,"ISE":24352,"Ġsubmitting":24353,"Ġnaive":24354,"arations":24355,"Ġ143":24356,"Ġpoised":24357,"responsible":24358,"Ġsocks":24359,"ĠSkull":24360,"Question":24361,"Ġdiscoveries":24362,"Joined":24363,"ĠEnemies":24364,"ĠWireless":24365,"ĠRevenge":24366,"Ġpuzzles":24367,"Ġceased":24368,"290":24369,"criptions":24370,"ĠConsole":24371,"Ġboiling":24372,"Ġdiscrep":24373,"Ġdeduction":24374,"Ġarsenal":24375,"XXXX":24376,"ĠAmsterdam":24377,"roximately":24378,"ĠShane":24379,"Ġposing":24380,"ĠACLU":24381,"ĠCompanies":24382,"Ġtheology":24383,"ĠUg":24384,"quarter":24385,"ĠHank":24386,"Coin":24387,"ĠLv":24388,"Ġallegation":24389,"ĠAvoid":24390,"Ġindefinitely":24391,"Ġcommodities":24392,"Ġbrig":24393,"ĠManit":24394,"Ġtenth":24395,"method":24396,"ĠKnicks":24397,"ĠâĢİ":24398,"Ġinvoked":24399,"Dial":24400,"ARA":24401,"Ġcaucus":24402,"227":24403,"ĠJab":24404,"Ġounces":24405,"bay":24406,"Ġbuddy":24407,"fan":24408,"234":24409,"ĠHil":24410,"adh":24411,"ĠTY":24412,"ĠIND":24413,"Ġ1939":24414,"Ġiteration":24415,"ĠGonzalez":24416,"ĠVert":24417,"ĠIO":24418,"emb":24419,"rera":24420,"ench":24421,"ĠRequirements":24422,"ĠWins":24423,"Ġlivestock":24424,"hours":24425,"\"â̦":24426,"bral":24427,"Marg":24428,"ĠDone":24429,"Ġwasting":24430,"inged":24431,"groups":24432,"Ġwishing":24433,"ĠTumblr":24434,"Ġtapping":24435,"Ġnationalism":24436,"ĠByr":24437,"Ġsquares":24438,"ĠActions":24439,"ãĥ¥":24440,"Inside":24441,"debug":24442,"Ġappend":24443,"Ġstubborn":24444,"ĠCind":24445,"Tell":24446,"Ġtearing":24447,"ĠRey":24448,"orc":24449,"ĠDayton":24450,"ĠNH":24451,"ĠMadness":24452,"Charl":24453,"ĠMorrison":24454,"filter":24455,"Ġaccuse":24456,"Ġ./":24457,"Ġtorrent":24458,"Ġdeclines":24459,"gallery":24460,"Mine":24461,"Ġnegotiation":24462,"ĠBashar":24463,"opia":24464,"1993":24465,"emort":24466,"ĠNovel":24467,"ĠFang":24468,"ersive":24469,"ĠInstant":24470,"Ġroller":24471,"Around":24472,"ĠElections":24473,"Games":24474,"Ġinexpensive":24475,"Ġwors":24476,"Ġvul":24477,"ĠHole":24478,"Ġunbelievable":24479,"Ġnause":24480,"Ġentr":24481,"boat":24482,"ĠSTE":24483,"Ġbush":24484,"ĠHassan":24485,"Ġwo":24486,"Ġpaused":24487,"ĠMig":24488,"lived":24489,"Ġscout":24490,"Ġlith":24491,"Published":24492,"duino":24493,"cool":24494,"Ġcirculating":24495,"idas":24496,"ĠPam":24497,"violent":24498,"ĠCrawford":24499,"uddle":24500,"ĠLetters":24501,"Guard":24502,"morph":24503,"Ġwandering":24504,"Ġsophomore":24505,"Ġqueer":24506,"ĠBlind":24507,"rue":24508,"ĠMarriage":24509,"Dom":24510,"Ġpadding":24511,"Ġfolders":24512,"Ġmeaningless":24513,"Ġcandidacy":24514,"afort":24515,"Ġwhistlebl":24516,"ĠIdentified":24517,"Ġcigar":24518,"Ġhid":24519,"ĠDubai":24520,"Ġposture":24521,"Ġhiking":24522,"ĠTerminal":24523,"Legendary":24524,"ĠTP":24525,"ĠATK":24526,"ĠStarbucks":24527,"ĠRiot":24528,"1991":24529,"ĠBottom":24530,"effic":24531,"ĠEugene":24532,"ĠWyoming":24533,"ĠRocky":24534,"Ġsalmon":24535,"Ġmetro":24536,"Ġbilateral":24537,"Ġcelebrates":24538,"Length":24539,"billion":24540,"Bat":24541,"Ġreleg":24542,"Ġpseudo":24543,"DT":24544,"ĠRhode":24545,"Parent":24546,"pletion":24547,"Ġattribut":24548,"Ġtuning":24549,"ĠNOTE":24550,"ĠRebel":24551,"icus":24552,"Fund":24553,"Ġcocktail":24554,"Ġ501":24555,"Ġspoon":24556,"Ġbrutality":24557,"Ġunite":24558,"Ġmicrobi":24559,"ĠReich":24560,"positive":24561,"Ġamazed":24562,"ĠNT":24563,"Desc":24564,"ECTION":24565,"Ġfalsely":24566,"ĠHighlander":24567,"ĠCrist":24568,"ĠVictorian":24569,"Ġdistributions":24570,"their":24571,"ĠEinstein":24572,"Ġpod":24573,"Ġepidem":24574,"Ġheap":24575,"ĠRanch":24576,"Ġanthem":24577,"Ġreapp":24578,"ĠAuburn":24579,"Ġconcurrent":24580,"ĠThroughout":24581,"ĠPOST":24582,"âĺ":24583,"Ġhomemade":24584,"kick":24585,"Beg":24586,"Ġchassis":24587,"counter":24588,"Ġmerger":24589,"Ġlaps":24590,"217":24591,"union":24592,"ĠTrigger":24593,"Ġdebated":24594,"Ġsilently":24595,"Ġrestraint":24596,"Bal":24597,"0000000":24598,"Ġformidable":24599,"ĠFilip":24600,"Ġsacrifices":24601,"Food":24602,"Ġdwarf":24603,"ĠSequ":24604,"inian":24605,"Moreover":24606,"Ġtangible":24607,"opsis":24608,"ĠMinecraft":24609,"ĠRegistration":24610,"oan":24611,"Ġrepresentations":24612,"Ġthirst":24613,"Ġcorp":24614,"irement":24615,"Made":24616,"loe":24617,">\"":24618,"cats":24619,"*.":24620,"Ġgestures":24621,"general":24622,"League":24623,"Ġpackets":24624,"ĠInspector":24625,"ĠBerg":24626,"Ġfraudulent":24627,"Ġcriticize":24628,"Fun":24629,"Ġblaming":24630,"ndra":24631,"Ġslash":24632,"ĠEston":24633,"Ġproposing":24634,"Ġwhales":24635,"Ġtherapist":24636,"Ġsubset":24637,"Ġleisure":24638,"ELD":24639,"ĠCVE":24640,"ĠActivity":24641,"Ġculmin":24642,"shop":24643,"ĠDAY":24644,"ischer":24645,"ĠAdmiral":24646,"ĠAttacks":24647,"Ġ1958":24648,"Ġmemoir":24649,"Ġfolded":24650,"Ġsexist":24651,"Ġ153":24652,"ĠLI":24653,"Ġreadings":24654,"Ġembarrassment":24655,"ĠEmployment":24656,"wart":24657,"chin":24658,"Ġcontinuation":24659,"lia":24660,"Recently":24661,"Ġduel":24662,"Ġevacuation":24663,"ĠKashmir":24664,"Ġdisposition":24665,"ĠRig":24666,"Ġbolts":24667,"Ġinsurers":24668,"467":24669,"Mex":24670,"Ġretaliation":24671,"Ġmisery":24672,"Ġunreasonable":24673,"raining":24674,"Imm":24675,"ĠPU":24676,"emer":24677,"Ġgenital":24678,"ãĤ³":24679,"ĠCandy":24680,"Ġonions":24681,"ĠPatt":24682,"liner":24683,"Ġconceded":24684,"Ġfa":24685,"Ġforc":24686,"ĠHernandez":24687,"ĠGeoff":24688,"debian":24689,"ĠTeams":24690,"Ġcries":24691,"Ġhomeowners":24692,"237":24693,"ABC":24694,"Ġstitch":24695,"Ġstatistic":24696,"Ġheaders":24697,"ĠBiology":24698,"Ġmotors":24699,"ĠGEN":24700,"ĠLip":24701,"Ġhates":24702,"Ġheel":24703,"Self":24704,"ipl":24705,"EDIT":24706,"orting":24707,"Ġannot":24708,"ĠSpeech":24709,"oldemort":24710,"ĠJavascript":24711,"ĠLeBron":24712,"Ġfootprint":24713,"Ġfn":24714,"Ġseizures":24715,"nas":24716,"hide":24717,"Ġ1954":24718,"ĠBee":24719,"ĠDeclaration":24720,"ĠKatie":24721,"Ġreservations":24722,"NR":24723,"female":24724,"Ġsaturated":24725,"Ġbiblical":24726,"Ġtrolls":24727,"Device":24728,"photos":24729,"Ġdrums":24730,"ãĥīãĥ©ãĤ´ãĥ³":24731,"Night":24732,"fighter":24733,"ĠHak":24734,"riber":24735,"Ġcush":24736,"Ġdisciplinary":24737,"baum":24738,"ĠGH":24739,"ĠSchmidt":24740,"ilibrium":24741,"Ġsixty":24742,"ĠKushner":24743,"rots":24744,"Ġpund":24745,"ĠRac":24746,"Ġsprings":24747,"Ġconve":24748,"Business":24749,"Fall":24750,"Ġqualifications":24751,"Ġverses":24752,"Ġnarciss":24753,"ĠKoh":24754,"ĠWow":24755,"ĠCharlottesville":24756,"edo":24757,"Ġinterrogation":24758,"ĠWool":24759,"365":24760,"Brian":24761,"Ġâľĵ":24762,"Ġalleges":24763,"onds":24764,"idation":24765,"ĠJackie":24766,"yu":24767,"Ġlakes":24768,"Ġworthwhile":24769,"Ġcrystals":24770,"ĠJuda":24771,"Ġcomprehend":24772,"Ġflush":24773,"Ġabsorption":24774,"ĠOC":24775,"Ġfrightened":24776,"ĠChocolate":24777,"Martin":24778,"Ġbuys":24779,"Ġbucks":24780,"Ġappell":24781,"ĠChampionships":24782,"Ġlistener":24783,"ĠDefensive":24784,"Ġcz":24785,"uds":24786,"ĠMate":24787,"Ġreplay":24788,"Ġdecorated":24789,"Ġsunk":24790,"ĠVIP":24791,"ĠAnk":24792,"Ġ195":24793,"aaaa":24794,"Nobody":24795,"ĠMilk":24796,"ĠGur":24797,"ĠMk":24798,"ĠSara":24799,"Ġseating":24800,"ĠWid":24801,"Track":24802,"Ġemploys":24803,"Ġgigantic":24804,"APP":24805,"ãĤ§":24806,"inventory":24807,"Ġtowel":24808,"atche":24809,"lasting":24810,"ĠTL":24811,"Ġlatency":24812,"Ġkne":24813,"Ber":24814,"meaning":24815,"Ġupheld":24816,"Ġplayground":24817,"Ġmant":24818,"Side":24819,"Ġstereo":24820,"Ġnorthwest":24821,"Ġexceptionally":24822,"Ġrays":24823,"Ġrecurring":24824,"Drive":24825,"Ġupright":24826,"Ġabduct":24827,"ĠMarathon":24828,"Ġgoodbye":24829,"Ġalphabet":24830,"hp":24831,"Ġcourtroom":24832,"rington":24833,"othing":24834,"Tag":24835,"Ġdiplomats":24836,"Ġbarbar":24837,"ĠAqua":24838,"183":24839,"3333":24840,"Ġmaturity":24841,"Ġinstability":24842,"ĠApache":24843,"Ġ===":24844,"Ġfasting":24845,"ĠGrid":24846,"ModLoader":24847,"Ġ152":24848,"Abs":24849,"ĠOperating":24850,"etti":24851,"Ġacquaint":24852,"Donnell":24853,"ĠKem":24854,"ĠForge":24855,"Ġarmored":24856,"Mil":24857,"Ġphilosophers":24858,"invest":24859,"Players":24860,"âĪ":24861,"Ġmyriad":24862,"Ġcomrades":24863,"Rot":24864,"Ġremembering":24865,"Ġcorresponds":24866,"Ġprogrammers":24867,"ĠLynn":24868,"Ġolig":24869,"Ġcoherent":24870,"ynchron":24871,"ĠChemical":24872,"Ġjugg":24873,"pair":24874,"posts":24875,"Eye":24876,"ĠInner":24877,"Ġsemester":24878,"ottest":24879,"ĠEmirates":24880,"ricanes":24881,"orously":24882,"mits":24883,"ĠWis":24884,"Ġdodge":24885,"location":24886,"Ġfaded":24887,"Amazon":24888,"ĠProceed":24889,"ĠINFO":24890,"journal":24891,"ĠTruck":24892,"Ten":24893,"Ġ217":24894,"Ġstatutes":24895,"mobile":24896,"ĠTypes":24897,"Recomm":24898,"buster":24899,"pex":24900,"Ġlegends":24901,"Ġheadache":24902,"faced":24903,"ĠWiFi":24904,"ifty":24905,"ĠHER":24906,"Ġcircuits":24907,"ERROR":24908,"226":24909,"olin":24910,"Ġcylinder":24911,"ospace":24912,"ikers":24913,"Prem":24914,"Quant":24915,"Ġconflicting":24916,"Ġslightest":24917,"Ġforged":24918,"ionage":24919,"Stephen":24920,"ĠKub":24921,"ĠOpportun":24922,"ĠHeal":24923,"Ġblo":24924,"Ġrulers":24925,"Ġhuh":24926,"Ġsubmarine":24927,"fy":24928,"asser":24929,"Ġallowance":24930,"ĠKasich":24931,"ĠTas":24932,"ĠAustralians":24933,"ForgeModLoader":24934,"ĠâĨij":24935,"ĠMatrix":24936,"amins":24937,"Ġ1200":24938,"ĠAcqu":24939,"236":24940,"Document":24941,"ĠBreaking":24942,"193":24943,"ĠSubst":24944,"ĠRoller":24945,"ĠProperties":24946,"ĠNI":24947,"tier":24948,"Ġcrushing":24949,"Ġadvocating":24950,"Furthermore":24951,"keepers":24952,"Ġsexism":24953,"xd":24954,"Ġcaller":24955,"ĠSense":24956,"chieve":24957,"ĠTF":24958,"Ġfueled":24959,"Ġreminiscent":24960,"Ġobsess":24961,"urst":24962,"Ġuphold":24963,"ĠFans":24964,"hetics":24965,"ĠâĹ":24966,"ĠBath":24967,"Ġbeverage":24968,"Ġoscill":24969,"254":24970,"Ġpoles":24971,"Ġgradual":24972,"Ġexting":24973,"ĠSuff":24974,"ĠSuddenly":24975,"Ġliking":24976,"Ġ1949":24977,"unciation":24978,"amination":24979,"ĠOmar":24980,"ĠLV":24981,"ĠConsequently":24982,"Ġsynthes":24983,"ĠGIF":24984,"Ġpains":24985,"Ġinteracting":24986,"uously":24987,"incre":24988,"Ġrumor":24989,"ĠScientology":24990,"197":24991,"ĠZig":24992,"Ġspelling":24993,"ĠASS":24994,"Ġextingu":24995,"mson":24996,"Ġgh":24997,"Ġremarked":24998,"ĠStrategic":24999,"ĠMON":25000,"å¥":25001,"gae":25002,"ĠWHAT":25003,"Eric":25004,"ĠCampus":25005,"Ġmethane":25006,"Ġimagin":25007,"JUST":25008,"ĠAlm":25009,"XT":25010,"iq":25011,"ĠRSS":25012,"Ġwrongdoing":25013,"atta":25014,"Ġbigot":25015,"Ġdemonstrators":25016,"ĠCalvin":25017,"ĠVilla":25018,"Ġmembrane":25019,"ĠAwesome":25020,"Ġbenefic":25021,"268":25022,"Ġmagnificent":25023,"ĠLots":25024,"Greg":25025,"ĠBoris":25026,"Ġdetainees":25027,"ĠHerman":25028,"Ġwhispered":25029,"Ġawe":25030,"Professor":25031,"funding":25032,"Ġphysiological":25033,"ĠDestruction":25034,"Ġlimb":25035,"Ġmanipulated":25036,"Ġbubbles":25037,"Ġpseud":25038,"Ġhydra":25039,"ĠBristol":25040,"Ġstellar":25041,"ĠExpansion":25042,"ĠKell":25043,"ĠInterestingly":25044,"Ġmans":25045,"Ġdragging":25046,"Ġecological":25047,"ĠFit":25048,"Ġgent":25049,"Ġbenefited":25050,"ĠHaiti":25051,"Ġpolyg":25052,"ãĥİ":25053,"Ġ2030":25054,"Ġprow":25055,"Ġreconstruction":25056,"Ġwast":25057,"Ġpsychic":25058,"ĠGreeks":25059,"Handler":25060,"162":25061,"ĠPulse":25062,"Ġsolicit":25063,"Ġsys":25064,"Ġinflux":25065,"ĠGentle":25066,"percent":25067,"Ġproliferation":25068,"Ġtaxable":25069,"Ġdisregard":25070,"Ġescaping":25071,"Ġginger":25072,"Ġwithstand":25073,"Ġdevastated":25074,"ĠDew":25075,"series":25076,"Ġinjected":25077,"elaide":25078,"Ġturnover":25079,"heat":25080,"ĻĤ":25081,"Happy":25082,"ĠSilent":25083,"ãĤŃ":25084,"ivism":25085,"Ġirrational":25086,"AMA":25087,"Ġreef":25088,"rub":25089,"Ġ162":25090,"Ġbankers":25091,"ĠEthics":25092,"vv":25093,"Ġcriticisms":25094,"Kn":25095,"186":25096,"Movie":25097,"ĠTories":25098,"Ġnood":25099,"Ġdistortion":25100,"False":25101,"odore":25102,"Ġtasty":25103,"Research":25104,"ĠUID":25105,"-)":25106,"Ġdivorced":25107,"ĠMU":25108,"ĠHayes":25109,"ĠIsn":25110,"iani":25111,"ĠHQ":25112,"Ġ\"#":25113,"ignant":25114,"Ġtraumatic":25115,"ĠLing":25116,"Hun":25117,"Ġsabot":25118,"online":25119,"random":25120,"Ġrenamed":25121,"rared":25122,"KA":25123,"dead":25124,"ét":25125,"ĠAssistance":25126,"Ġseaf":25127,"++++++++":25128,"Ġseldom":25129,"ĠWebb":25130,"Ġboolean":25131,"ulet":25132,"Ġrefrain":25133,"ĠDIY":25134,"rule":25135,"Ġshutting":25136,"Ġutilizing":25137,"loading":25138,"ĠParam":25139,"coal":25140,"ooter":25141,"Ġattracting":25142,"ĠDol":25143,"Ġhers":25144,"agnetic":25145,"ĠReach":25146,"imo":25147,"Ġdiscarded":25148,"ĠPip":25149,"015":25150,"ür":25151,"Ġmug":25152,"Imagine":25153,"COL":25154,"Ġcursed":25155,"ĠShows":25156,"ĠCurtis":25157,"ĠSachs":25158,"speaking":25159,"ĠVista":25160,"ĠFramework":25161,"ongo":25162,"Ġsubreddit":25163,"Ġcrus":25164,"ĠOval":25165,"Row":25166,"growing":25167,"Ġinstallment":25168,"Ġglac":25169,"ĠAdvance":25170,"ECK":25171,"ĠLGBTQ":25172,"LEY":25173,"Ġacet":25174,"Ġsuccessive":25175,"ĠNicole":25176,"Ġ1957":25177,"Quote":25178,"Ġcircumstance":25179,"ackets":25180,"Ġ142":25181,"ortium":25182,"Ġguessed":25183,"ĠFrame":25184,"Ġperpetrators":25185,"ĠAviation":25186,"ĠBench":25187,"Ġhandc":25188,"Ap":25189,"Ġ1956":25190,"259":25191,"rand":25192,"NetMessage":25193,"din":25194,"urtles":25195,"hig":25196,"ĠVIII":25197,"ffiti":25198,"ĠSwords":25199,"bial":25200,"Ġkidnapping":25201,"device":25202,"Ġbarn":25203,"ĠEli":25204,"aucas":25205,"Send":25206,"Constructed":25207,"Ġ½":25208,"Ġneedles":25209,"Ġadvertisements":25210,"Ġvou":25211,"Ġexhibited":25212,"ĠFortress":25213,"Ask":25214,"Berry":25215,"TYPE":25216,"Ġcancers":25217,"umping":25218,"ĠTerritory":25219,"Ġprud":25220,"Ġnas":25221,"Ġatheist":25222,"Ġbalances":25223,"ãģŁ":25224,"ĠShawn":25225,"&&":25226,"Ġlandsc":25227,"ĠRGB":25228,"Ġpetty":25229,"Ġexcellence":25230,"Ġtranslations":25231,"Ġparcel":25232,"ĠChev":25233,"East":25234,"ĠOutput":25235,"imi":25236,"Ġambient":25237,"ĠThreat":25238,"Ġvillains":25239,"Ġ550":25240,"ICA":25241,"Ġtaller":25242,"Ġleaking":25243,"cup":25244,"Ġpolish":25245,"Ġinfectious":25246,"ĠKC":25247,"Ġ@@":25248,"background":25249,"Ġbureaucracy":25250,"ĠSai":25251,"unless":25252,"itious":25253,"ĠSkype":25254,"Atl":25255,"IDENT":25256,"008":25257,"Ġhypocr":25258,"Ġpitchers":25259,"Ġguessing":25260,"ĠFINAL":25261,"Between":25262,"Ġvillagers":25263,"Ġ252":25264,"fashion":25265,"ĠTunis":25266,"Beh":25267,"ĠExc":25268,"ĠMID":25269,"288":25270,"ĠHaskell":25271,"196":25272,"ĠNOR":25273,"Ġspecs":25274,"Ġinvari":25275,"Ġglut":25276,"ĠCars":25277,"Ġimpulse":25278,"Ġhonors":25279,"gel":25280,"Ġjurisdictions":25281,"ĠBundle":25282,"ulas":25283,"California":25284,"ĠIncrease":25285,"Ġpear":25286,"Ġsingles":25287,"Ġcues":25288,"Ġunderwent":25289,"ĠWS":25290,"Ġexaggerated":25291,"Ġdubious":25292,"Ġflashing":25293,"LOG":25294,")].":25295,"Journal":25296,"tg":25297,"Van":25298,"ĠIstanbul":25299,"ĠInsp":25300,"ĠFranken":25301,"Draw":25302,"Ġsadness":25303,"Ġironic":25304,"ĠFry":25305,"xc":25306,"Ġ164":25307,"isch":25308,"Way":25309,"ĠProtestant":25310,"horn":25311,"Ġunaff":25312,"ĠViv":25313,"illas":25314,"ĠProductions":25315,"ĠHogan":25316,"Ġperimeter":25317,"ĠSisters":25318,"Ġspontaneous":25319,"Ġdownside":25320,"Ġdescendants":25321,"Ġorn":25322,"worm":25323,"Japanese":25324,"Ġ1955":25325,"Ġ151":25326,"ĠDoing":25327,"elsen":25328,"umbles":25329,"Ġradically":25330,"ĠDrum":25331,"ĠBach":25332,"Ġliabilities":25333,"ĠOB":25334,"ĠElementary":25335,"Ġmeme":25336,"ynes":25337,"Ġfingerprint":25338,"ĠGrab":25339,"Ġundertake":25340,"Members":25341,"ĠReader":25342,"ĠSims":25343,"god":25344,"Ġhypothetical":25345,"scient":25346,"ĠAJ":25347,"Ġcharism":25348,"Ġadmissions":25349,"ĠMissile":25350,"trade":25351,"Ġexercising":25352,"ĠBackground":25353,"Written":25354,"Ġvocals":25355,"whether":25356,"Ġvi":25357,"ĠWinner":25358,"Ġlitter":25359,"ĠShooting":25360,"STEM":25361,"ãĤ¡":25362,"ĠAFL":25363,"Ġvariability":25364,"Ġeats":25365,"ĠDPS":25366,"brow":25367,"Ġelephants":25368,"Ġstrat":25369,"ĠÅ":25370,"Ġsettlers":25371,"Matthew":25372,"Ġinadvert":25373,"HI":25374,"ĠIMF":25375,"ĠGoal":25376,"Ġnerves":25377,"Johnson":25378,"eye":25379,"ablishment":25380,"Thursday":25381,"BILITY":25382,"Had":25383,"amoto":25384,"hetamine":25385,"eps":25386,"Ġmitochond":25387,"Ġcompressed":25388,"ĠTrevor":25389,"ĠAnimals":25390,"Tool":25391,"Lock":25392,"Ġtweak":25393,"Ġpinch":25394,"Ġcancellation":25395,"Pot":25396,"Ġfocal":25397,"ĠAstron":25398,"173":25399,"ĠASC":25400,"ĠOTHER":25401,"umni":25402,"Ġdemise":25403,"dl":25404,"Ùħ":25405,"Semitism":25406,"Ġcracking":25407,"Ġcollaborative":25408,"Ġexplores":25409,"sql":25410,"Ġherbs":25411,"Ġconfigurations":25412,"mis":25413,"ĠResult":25414,"acey":25415,"ĠSmoke":25416,"Ġsanct":25417,"elia":25418,"Ġdegener":25419,"Ġdeepest":25420,"Ġscreamed":25421,"Ġnap":25422,"Software":25423,"ĠSTAR":25424,"EF":25425,"ĠXin":25426,"sponsored":25427,"manship":25428,"233":25429,"Ġprimaries":25430,"Ġfiltering":25431,"Ġassemble":25432,"mil":25433,"ĠMyers":25434,"bows":25435,"Ġpunched":25436,"Mic":25437,"Ġinnovations":25438,"Ġfunc":25439,"ando":25440,"Ġfracking":25441,"ĠVul":25442,"оÐ":25443,"oshop":25444,"ĠImmun":25445,"Ġsettling":25446,"Ġadolescents":25447,"Ġrebuilding":25448,"Ġtransforming":25449,"Ġparole":25450,"Ġharbor":25451,"Ġbooking":25452,"otional":25453,"ongevity":25454,"ĠYo":25455,"bug":25456,"Ġemerges":25457,"ĠMethods":25458,"ĠChu":25459,"Pres":25460,"ĠDungeons":25461,"Ġtrailing":25462,"ĠRum":25463,"ĠHugh":25464,"天":25465,"ĠEra":25466,"ĠBattles":25467,"Results":25468,"ĠTrading":25469,"Ġversa":25470,"css":25471,"axies":25472,"heet":25473,"Ġgreed":25474,"1989":25475,"Ġgardens":25476,"Ġcontingent":25477,"Park":25478,"ĠLeafs":25479,"hook":25480,"robe":25481,"Ġdiplomacy":25482,"ĠFuel":25483,"ĠInvasion":25484,"Ġupgrading":25485,"Male":25486,"Ġelic":25487,"Ġrelentless":25488,"ĠCovenant":25489,"apesh":25490,"ĠTrop":25491,"Ty":25492,"production":25493,"arty":25494,"Ġpunches":25495,"ako":25496,"cyclopedia":25497,"ĠRabbit":25498,"ĠHDMI":25499,"Ġ141":25500,"Ġfoil":25501,"ItemImage":25502,"ĠFG":25503,"Ġimplementations":25504,"ĠPom":25505,"ixtures":25506,"Ġawait":25507,"Ġ330":25508,"amus":25509,"Ġumbrella":25510,"Ġforesee":25511,"separ":25512,"Ġcircumcision":25513,"Ġperipheral":25514,"Say":25515,"ĠExpert":25516,"Inc":25517,"Ġwithdrew":25518,"ĠAnders":25519,"fried":25520,"Ġradioactive":25521,"ĠOpening":25522,"Ġboarding":25523,"ĠND":25524,"Ġoverthrow":25525,"Activ":25526,"WP":25527,"ĠActs":25528,"×Ļ":25529,"Ġmotions":25530,"vic":25531,"ĠMighty":25532,"ĠDefender":25533,"aer":25534,"Ġthankful":25535,"ĠKilling":25536,"ĠBris":25537,"moil":25538,"Ġpredicting":25539,"266":25540,"choice":25541,"Ġkillers":25542,"Ġincub":25543,"ĠChest":25544,"athering":25545,"Ġproclaimed":25546,"flower":25547,"ossom":25548,"umbledore":25549,"ĠCycling":25550,"ĠOccupy":25551,"AGES":25552,"Pen":25553,"ĠYug":25554,"Ġpackaged":25555,"Ġheightened":25556,"cot":25557,"stack":25558,"Cond":25559,"Ġstamps":25560,"mage":25561,"Ġpersuaded":25562,"Ġensl":25563,"ĠCardinal":25564,"Ġsolitary":25565,"Ġpossessing":25566,"ĠCork":25567,"Ġevid":25568,"ĠTay":25569,"Ġblues":25570,"Ġextremism":25571,"Ġlunar":25572,"Ġclown":25573,"Techn":25574,"Ġfestivals":25575,"ĠPvP":25576,"ĠLar":25577,"Ġconsequently":25578,"present":25579,"Ġsomeday":25580,"çİĭ":25581,"ĠMeteor":25582,"Ġtouring":25583,"culture":25584,"Ġbeaches":25585,"Ship":25586,"cause":25587,"ĠFlood":25588,"ãĥ¯":25589,"Ġpurity":25590,"those":25591,"Ġemission":25592,"bolt":25593,"Ġchord":25594,"ĠScripture":25595,"Lu":25596,"Ġ${":25597,"created":25598,"Others":25599,"258":25600,"Ġelemental":25601,"Ġannoyed":25602,"ĠAE":25603,"dan":25604,"ĠSag":25605,"Researchers":25606,"Ġfairy":25607,"âĢĵâĢĵ":25608,"============":25609,"Smart":25610,"GGGG":25611,"Ġskeletons":25612,"Ġpupils":25613,"linked":25614,"Ġurgency":25615,"enabled":25616,"ĠFuck":25617,"Ġcouncill":25618,"rab":25619,"UAL":25620,"TI":25621,"Ġlifes":25622,"Ġconfessed":25623,"Bug":25624,"Ġharmon":25625,"ĠCONFIG":25626,"ĠNeutral":25627,"Double":25628,"Ġstaple":25629,"ĠSHA":25630,"British":25631,"ĠSNP":25632,"ATOR":25633,"oco":25634,"Ġswinging":25635,"gex":25636,"oleon":25637,"plain":25638,"ĠMissing":25639,"ĠTrophy":25640,"vari":25641,"ranch":25642,"Ġ301":25643,"440":25644,"0000000000000000":25645,"Ġrestoring":25646,"Ġhaul":25647,"ucing":25648,"nerg":25649,"Ġfutures":25650,"Ġstrategist":25651,"question":25652,"Ġlateral":25653,"ĠBard":25654,"Ġsor":25655,"ĠRhodes":25656,"ĠDowntown":25657,"?????-":25658,"ĠLit":25659,"ĠBened":25660,"Ġcoil":25661,"street":25662,"ĠPortal":25663,"FILE":25664,"ĠGru":25665,"*,":25666,"231":25667,"neum":25668,"Ġsucked":25669,"Ġrapper":25670,"Ġtendencies":25671,"ĠLauren":25672,"cellaneous":25673,"267":25674,"Ġbrowse":25675,"Ġoverc":25676,"header":25677,"oise":25678,"Ġbeet":25679,"ĠGle":25680,"Stay":25681,"Ġmum":25682,"Ġtyped":25683,"Ġdiscounts":25684,"Talk":25685,"ĠOg":25686,"existing":25687,"ĠSell":25688,"uph":25689,"CI":25690,"ĠAustrian":25691,"ĠWarm":25692,"Ġdismissal":25693,"Ġaverages":25694,"camera":25695,"Ġallegiance":25696,"LAN":25697,"=\"#":25698,"Ġcommentators":25699,"ĠSetting":25700,"ĠMidwest":25701,"Ġpharmac":25702,"ĠEXP":25703,"Ġstainless":25704,"Chicago":25705,"Ġtan":25706,"244":25707,"Ġcountryside":25708,"ĠVac":25709,"295":25710,"Ġpinned":25711,"Ġcrises":25712,"Ġstandardized":25713,"Task":25714,"ĠJail":25715,"ĠDocker":25716,"colored":25717,"forth":25718,"\"},":25719,"Ġpatrons":25720,"Ġspice":25721,"Ġmourn":25722,"ĠMood":25723,"Ġlaundry":25724,"Ġequip":25725,"ĠMole":25726,"yll":25727,"ĠTHC":25728,"nation":25729,"ĠSherlock":25730,"Ġissu":25731,"ĠKre":25732,"ĠAmericas":25733,"ĠAAA":25734,"Ġsystematically":25735,"Ġcontra":25736,"ĠSally":25737,"Ġrationale":25738,"Ġcarriage":25739,"Ġpeaks":25740,"Ġcontradiction":25741,"ensation":25742,"ĠFailure":25743,"Ġprops":25744,"Ġnamespace":25745,"Ġcove":25746,"fields":25747,"ãĤĭ":25748,"Ġwool":25749,"ĠCatch":25750,"Ġpresumed":25751,"ĠDiana":25752,"ragon":25753,"igi":25754,"Ġhamm":25755,"Ġstunt":25756,"ĠGUI":25757,"ĠObservatory":25758,"ĠShore":25759,"Ġsmells":25760,"annah":25761,"Ġcockpit":25762,"ĠDuterte":25763,"850":25764,"Ġoppressed":25765,"breaker":25766,"ĠContribut":25767,"ĠPeru":25768,"ĠMonsanto":25769,"ĠAttempt":25770,"Ġcommanding":25771,"Ġfridge":25772,"ĠRin":25773,"ĠChess":25774,"uality":25775,"Ġol":25776,"Republican":25777,"ĠGlory":25778,"ĠWIN":25779,".......":25780,"agent":25781,"reading":25782,"Ġinh":25783,"Jones":25784,"Ġclicks":25785,"alan":25786,"Ġ[];":25787,"ĠMajesty":25788,"ĠCed":25789,"opus":25790,"atel":25791,"ê":25792,"ARC":25793,"ĠEcuador":25794,"ãĥł":25795,"ĠKuro":25796,"Ġrituals":25797,"Ġcaptive":25798,"Ġounce":25799,"Ġdisagreement":25800,"Ġslog":25801,"fuel":25802,"Pet":25803,"Mail":25804,"Ġexercised":25805,"Ġsolic":25806,"Ġrainfall":25807,"Ġdevotion":25808,"ĠAssessment":25809,"Ġrobotic":25810,"options":25811,"ĠRP":25812,"ĠFamilies":25813,"ĠFlames":25814,"Ġassignments":25815,"007":25816,"akedown":25817,"Ġvocabulary":25818,"Reilly":25819,"Ġcaval":25820,"gars":25821,"Ġsuppressed":25822,"ĠSET":25823,"ĠJohns":25824,"Ġwarp":25825,"broken":25826,"Ġstatues":25827,"Ġadvocated":25828,"Ġ275":25829,"Ġperil":25830,"omorph":25831,"ĠFemin":25832,"perfect":25833,"Ġhatch":25834,"Lib":25835,"512":25836,"Ġlifelong":25837,"313":25838,"Ġcheeks":25839,"Ġnumbered":25840,"ĠMug":25841,"Body":25842,"ravel":25843,"Weight":25844,"ĠJak":25845,"ĠHeath":25846,"Ġkissing":25847,"ĠJUST":25848,"Ġwaving":25849,"upload":25850,"Ġinsider":25851,"ĠProgressive":25852,"ĠFilter":25853,"tta":25854,"ĠBeam":25855,"Ġviolently":25856,"ipation":25857,"Ġskepticism":25858,"Ġ1918":25859,"ĠAnnie":25860,"ĠSI":25861,"Ġgenetics":25862,"Ġonboard":25863,"atl":25864,"ĠFriedman":25865,"ĠBri":25866,"ceptive":25867,"Ġpirate":25868,"ĠReporter":25869,"278":25870,"Ġmythology":25871,"Ġeclipse":25872,"Ġskins":25873,"Ġglyph":25874,"ingham":25875,"Files":25876,"Cour":25877,"women":25878,"Ġregimes":25879,"Ġphotographed":25880,"Kat":25881,"ĠMAX":25882,"Officials":25883,"Ġunexpectedly":25884,"Ġimpressions":25885,"Front":25886,";;;;;;;;":25887,"Ġsupremacy":25888,"Ġsang":25889,"Ġaggravated":25890,"Ġabruptly":25891,"ĠSector":25892,"Ġexcuses":25893,"Ġcosting":25894,"idepress":25895,"Stack":25896,"ĠRNA":25897,"obil":25898,"Ġghosts":25899,"ldon":25900,"atibility":25901,"Topics":25902,"Ġreimburse":25903,"ĠHM":25904,"ĠDeg":25905,"Ġthief":25906,"yet":25907,"ogenesis":25908,"leaning":25909,"ĠKol":25910,"ĠBasketball":25911,"Ġfi":25912,"ĠSeeing":25913,"Ġrecycling":25914,"Ġ[-":25915,"Congress":25916,"Ġlectures":25917,"Psy":25918,"Ġnep":25919,"Ġmaid":25920,"Ġoriented":25921,"AX":25922,"Ġrespectful":25923,"rene":25924,"flush":25925,"ĠUnloaded":25926,"request":25927,"grid":25928,"ĠAlternatively":25929,"ĠHugo":25930,"Ġdecree":25931,"ĠBuddhism":25932,"andum":25933,"Android":25934,"ĠCongo":25935,"ĠJoyce":25936,"Ġacknowledging":25937,"hesive":25938,"ĠTomorrow":25939,"ĠHiro":25940,"thren":25941,"ĠMaced":25942,"Ġhoax":25943,"ĠIncreased":25944,"ĠPradesh":25945,"Wild":25946,"______":25947,"161":25948,"Ġaunt":25949,"Ġdistributing":25950,"ĠTucker":25951,"ĠSSL":25952,"ĠWolves":25953,"Building":25954,"oult":25955,"ĠLuo":25956,"ĠYas":25957,"ĠSpir":25958,"ĠShape":25959,"ĠCambod":25960,"ĠIPv":25961,"Ġml":25962,"Ġextrad":25963,"390":25964,"ĠPenny":25965,"dream":25966,"Ġstationed":25967,"optional":25968,"eworthy":25969,".</":25970,"Ġundertaking":25971,"Ġchickens":25972,"Ġstimuli":25973,"ĠElse":25974,"igators":25975,"ĠBeginning":25976,"ctory":25977,"Ġprepares":25978,"Ġdelta":25979,"Ġvicinity":25980,"tool":25981,"Ġworkshops":25982,"MHz":25983,"Ġaccusation":25984,"Ġhistories":25985,"ropolis":25986,"ĠChurchill":25987,"Ġneon":25988,"Ġbaff":25989,"dies":25990,"maybe":25991,"Ġè£ıè¦ļéĨĴ":25992,"Ġsymptom":25993,"ECH":25994,"ĠManuel":25995,"Ġbanana":25996,"ĠHB":25997,"Ġ****":25998,"ĠKoreans":25999,"coll":26000,"FB":26001,"Ġpraying":26002,"ĠCannot":26003,"ĠMile":26004,"Ġembracing":26005,"ĠSilk":26006,"393":26007,"oters":26008,"FD":26009,"Ġdaylight":26010,"alias":26011,"ĠBrigade":26012,"ĠHannah":26013,"Ġclergy":26014,"Ġsoutheast":26015,"Ġalcoholic":26016,"Ġproposes":26017,"livion":26018,"Ġcalculating":26019,"Ġstimulate":26020,"Ġsplitting":26021,"eight":26022,"ĠIndy":26023,"plays":26024,"ĠPik":26025,"Ġdomest":26026,"Ġforgiveness":26027,"ĠRings":26028,"patient":26029,"kinson":26030,"Mont":26031,"igible":26032,";\"":26033,"Ġperiodically":26034,"ammad":26035,"ĠBritt":26036,"pard":26037,"Ġarbitration":26038,"ĠSchneider":26039,"ĠCorporate":26040,"ĠMaya":26041,"Ġsnakes":26042,"aum":26043,"Ġblasted":26044,"Ġmysteries":26045,"Ġrevive":26046,"ocamp":26047,"ĠDodge":26048,"ĠOpera":26049,"279":26050,"Ġorphan":26051,"Ġspecifies":26052,"ĠMets":26053,"Duration":26054,"Hen":26055,"Ġfireworks":26056,"Ġprosecute":26057,"ĠTillerson":26058,"dp":26059,"usage":26060,"liness":26061,"ĠDebian":26062,"Ġ224":26063,"rises":26064,"ĠInfect":26065,"atra":26066,"ĠRR":26067,"ĠLor":26068,"diff":26069,"ĠCharleston":26070,"Ġacoustic":26071,"Ġamuse":26072,"330":26073,"Ġcer":26074,"ĠTac":26075,"Ġ[+":26076,"Ġcardiac":26077,"ĠRestaurant":26078,"ergy":26079,"Ġfuzz":26080,"Ġbites":26081,"Ġhazardous":26082,"Ġbrighter":26083,"rans":26084,"ĠStephanie":26085,"extra":26086,"RET":26087,"ĠChristine":26088,"ĠSue":26089,"statement":26090,"Ġbolster":26091,"Ġantit":26092,"Radio":26093,"BIT":26094,"ãĤ°":26095,"Ġvisions":26096,"ĠConcept":26097,"Ġinline":26098,"ĠPhilosophy":26099,"isans":26100,"ĠIrving":26101,"ã":26102,"taking":26103,"Ġinconsist":26104,"ĠKumar":26105,"Ġlig":26106,"ĠSchumer":26107,"ĠRegulations":26108,"ĠHz":26109,"thro":26110,"ĠVoldemort":26111,"ĠMED":26112,"ĠFrederick":26113,"Pad":26114,"221":26115,"Ġalleging":26116,"ĠCommunication":26117,"Ġ167":26118,"Ġforecasts":26119,"Ġspiders":26120,"Organ":26121,"ĠParticipants":26122,"ĠOps":26123,"design":26124,"Close":26125,"Ġfacto":26126,"Ġbombers":26127,"resistant":26128,"ategories":26129,"School":26130,"Ġhomework":26131,"Ġcorro":26132,"Tuesday":26133,"ĠBrendan":26134,"ĠMX":26135,"ĠTS":26136,"ĠStri":26137,"Ġstakeholders":26138,"ĠMillennium":26139,"Ġtransferring":26140,"Jud":26141,"Ġtac":26142,"Ġ1600":26143,"ĠSDK":26144,"rb":26145,"Ġinterpretations":26146,"ĠSG":26147,"Ġupstairs":26148,"ĠHarvest":26149,"Ġvagina":26150,"Ġingest":26151,"xf":26152,"ĠOrion":26153,"ĠJoey":26154,"Ġsandwic":26155,"Ġimmortal":26156,"Ġflipped":26157,"ortex":26158,"threatening":26159,"Ġsniper":26160,"Ġconverts":26161,"Ġinstallations":26162,"ĠBulgar":26163,"orsche":26164,"mails":26165,"Ġlure":26166,"Ġnarrowly":26167,"Ġgrenade":26168,"ĠGing":26169,"Ġunderwear":26170,"--------------":26171,"Ġchased":26172,"ĠVAL":26173,"Ġparenting":26174,"ĠHamb":26175,"ĠBlaz":26176,"Ġanarchist":26177,"ĠMedian":26178,"ĠPrograms":26179,"ν":26180,"Ġobj":26181,"ĠNokia":26182,"orman":26183,"anqu":26184,"atism":26185,"opa":26186,"Ġfulfilling":26187,"Ġpuppy":26188,"Ġentit":26189,"ĠSebastian":26190,"Ġshooters":26191,"Ġricher":26192,"è¡":26193,"Ġtempted":26194,"ĠATT":26195,"ĠCV":26196,"Ġtore":26197,"Resource":26198,"ĠDevils":26199,"408":26200,"inational":26201,"Ġassurance":26202,"ĠDarren":26203,"Ġwhichever":26204,"posure":26205,"Ġfury":26206,"Stock":26207,"Ġuniversally":26208,"response":26209,"Ġoak":26210,"Ġworkload":26211,"ĠCorner":26212,"eele":26213,"\"...":26214,"Ġdeprived":26215,"kowski":26216,"Ġcasts":26217,"Ġaffiliation":26218,"ĠAch":26219,"ĠAsked":26220,"athe":26221,"Ġlact":26222,"ĠThu":26223,"rm":26224,"Ġairlines":26225,"Ġnotions":26226,"Format":26227,"ĠFAA":26228,"ãĥĬ":26229,"driver":26230,"Ġtranscend":26231,"Settings":26232,"ĠProsecut":26233,"Ġspinal":26234,"Ġdefaults":26235,"FK":26236,"Ġprefers":26237,"rendered":26238,"thus":26239,"film":26240,"Ġtiger":26241,"ĠSpicer":26242,"recogn":26243,"ĠRugby":26244,"Network":26245,"Ġpity":26246,"Ġcompartment":26247,"casters":26248,"ĠMonroe":26249,"Ġ720":26250,"Ġcorrections":26251,"Ġdopamine":26252,"ĠAZ":26253,"Cut":26254,"Ġroomm":26255,"Ġspeculate":26256,"Hash":26257,"Ġrestrictive":26258,"1111":26259,"redible":26260,"onel":26261,"Ġrampant":26262,"reported":26263,"ĠSuite":26264,"ĠMinimum":26265,"alys":26266,"azard":26267,"loop":26268,"Ġlent":26269,"sha":26270,"Ġvandal":26271,"menu":26272,"ĠBoehner":26273,"Ġnarratives":26274,"Ġauthenticity":26275,"269":26276,"anic":26277,"duty":26278,"285":26279,"Ġthanked":26280,"Ġbetrayed":26281,"lift":26282,"Ġsouthwest":26283,"ĠDexter":26284,"ĠBod":26285,"Ġkeywords":26286,"Average":26287,"DIS":26288,"Ġethnicity":26289,"!),":26290,"ĠNationals":26291,"á¹":26292,"ĠTah":26293,"ioxid":26294,"Ġwidget":26295,"Ġpasta":26296,"Ġbilling":26297,"Ġtrilogy":26298,"ĠLines":26299,"Ġsniff":26300,"Ġnephew":26301,"Late":26302,"Ġprincip":26303,"ĠLoop":26304,"ĠMarxist":26305,"Ġdissolved":26306,"Ġcontexts":26307,"ĠAmount":26308,"ĠSpike":26309,"Ġtotals":26310,"Ġorganizer":26311,"Ġuprising":26312,"ships":26313,"YY":26314,"ĠNortheast":26315,"money":26316,"gradation":26317,"Ġgoalkeeper":26318,"ĠHear":26319,"Ġsteak":26320,"ĠBuzzFeed":26321,"Ġsolemn":26322,"ĠScand":26323,"Ġpopping":26324,"Ġadhere":26325,"ĠAlleg":26326,"byte":26327,"ĠWolver":26328,"Ġunin":26329,"Ġrecol":26330,"itud":26331,"Ġmimic":26332,"ibus":26333,"Ġpredicts":26334,"ĠKeeper":26335,"iating":26336,"Ġdeception":26337,"Ġlearnt":26338,"Ġdiary":26339,"Ġconditional":26340,"Ġrelic":26341,"Ġinvoke":26342,"ienced":26343,"åĪ":26344,"ĠPont":26345,"Ġcellphone":26346,"Ġspeeding":26347,"Ġtackling":26348,"Ġnude":26349,"opened":26350,"ĠManafort":26351,"Ġ1952":26352,"Ġmajors":26353,"ĠSilence":26354,"Ġlogistics":26355,"Ġweighted":26356,"ĠPsychiat":26357,"\":[\"":26358,"Ġsickness":26359,"Ġdividends":26360,"zon":26361,"Release":26362,"ĠKeys":26363,"ĠIch":26364,"Ġenz":26365,"ĠFernand":26366,"Ġα":26367,"Ġmeanings":26368,"Ġpenny":26369,"Ġstern":26370,"Ġlar":26371,"ĠPublished":26372,"Ġbackdrop":26373,"Kim":26374,"ĠSynt":26375,"Ġdebuted":26376,"wm":26377,"ĠIsle":26378,"Ġregulating":26379,"otti":26380,"ĠScholars":26381,"icester":26382,"ĠChef":26383,"Ġpops":26384,"ĠLauncher":26385,"ĠVarious":26386,"Ġcommenting":26387,"oslav":26388,"enzie":26389,"Ġrivalry":26390,"âĤ¬":26391,"Really":26392,"Ġorc":26393,"Ġbean":26394,"ĠJudy":26395,"Notice":26396,"ĠBike":26397,"?]":26398,"Ġrented":26399,"sten":26400,"Ġforefront":26401,"ĠBaldwin":26402,"Ġyielded":26403,"tails":26404,"Prime":26405,"ĠSources":26406,"icator":26407,"Sean":26408,"Ġmarching":26409,"Output":26410,"ĠJungle":26411,"Ġreside":26412,"zzle":26413,"ĠAndrews":26414,"Ġtorque":26415,"Basic":26416,"Actually":26417,"strap":26418,"penter":26419,"Ġexams":26420,"ĠYa":26421,"Ġ159":26422,"ĠDecision":26423,"Ġransom":26424,"eteenth":26425,"ensing":26426,"213":26427,"Ġsunset":26428,"404":26429,"ĠRapid":26430,"ĠHein":26431,"ĠAboriginal":26432,"Ġorganism":26433,"ĠSever":26434,"Ġcla":26435,"aji":26436,"Simple":26437,"ĠFlavor":26438,"ĠEval":26439,"prus":26440,"Ġchorus":26441,"DAY":26442,"Ġdenounced":26443,"Ġbiography":26444,"ĠTurnbull":26445,"Recent":26446,"Normal":26447,"lections":26448,"Word":26449,"Ġferry":26450,"ĠWagner":26451,"hom":26452,"Unit":26453,"Ġsupermarket":26454,"ĠSith":26455,"Ġnominees":26456,"Ġdictatorship":26457,"iddler":26458,"Ġannounces":26459,"ĠThem":26460,"ĠNeptune":26461,"Ġdeity":26462,"ĠYi":26463,"Ġmonarch":26464,"ARR":26465,"Ġinvaded":26466,"ĠHok":26467,"untary":26468,"Certain":26469,"ega":26470,"Ġkidding":26471,"ĠRegulation":26472,"Ġtray":26473,"Ġphotographers":26474,"ĠArcane":26475,"Ġdischarged":26476,"Ġevangelical":26477,"Ġinterchange":26478,"Ġfilmmaker":26479,"ĠEndless":26480,"Ġ290":26481,"ĠSalvador":26482,"ASY":26483,"ĠSignal":26484,"Ġwrath":26485,"âľ":26486,"lot":26487,"'/":26488,"Ġprojectile":26489,"Ġemploying":26490,"ĠInterface":26491,"191":26492,"atellite":26493,"ĠRath":26494,"package":26495,"Ġindications":26496,"Jason":26497,"Ġargs":26498,"ĠGHz":26499,"Ġtilt":26500,"nants":26501,"won":26502,"ãĤµ":26503,"redd":26504,"rescent":26505,"ĠCalendar":26506,"Ġmodular":26507,"Ġassisting":26508,"Ġredeem":26509,"ĠBean":26510,"Ġworsh":26511,"Ġdecentralized":26512,")...":26513,"377":26514,"Ġarrays":26515,"Ġaccomplishments":26516,"ο":26517,"dot":26518,"Ġmutually":26519,"Ġobstruct":26520,"Ġmisrepresent":26521,"orest":26522,"ionic":26523,"ruce":26524,"%;":26525,"Ġknowingly":26526,"porting":26527,"inently":26528,"Ari":26529,"ĠSchultz":26530,"Da":26531,"ĠCere":26532,"Ġobsolete":26533,"ħĭ":26534,"give":26535,"Ġbait":26536,"Ġenlarg":26537,"Neill":26538,"Ġ1933":26539,"Ġreconsider":26540,"ĠSergeant":26541,"ĠDiane":26542,"ĠCogn":26543,"ĠIcon":26544,"Position":26545,"Ġfost":26546,"Ġstirring":26547,"seven":26548,"ĠSpaceX":26549,"uggets":26550,"Ġmedd":26551,"Gal":26552,"ĠSister":26553,"Boy":26554,"Ġtriggering":26555,"Taking":26556,"Ġscreams":26557,"Ġcausal":26558,"Ġawaken":26559,"Arm":26560,"297":26561,"Ġdispatched":26562,"ĠFALSE":26563,"Ġorganizational":26564,"ĠTong":26565,"Ġdilemma":26566,"demon":26567,"Spl":26568,"Ġhooks":26569,"uding":26570,"Ġvalidate":26571,"Ġpotion":26572,"Ġclaw":26573,"Ġburgl":26574,"Ġquir":26575,"ACA":26576,"ĠBrennan":26577,"Ġdurability":26578,"Ġbombings":26579,"ĠWindow":26580,"Ġculprit":26581,"325":26582,"Therefore":26583,"umbered":26584,"performance":26585,"warts":26586,"Ġenforcing":26587,"ĠBlow":26588,"Ġreprint":26589,"ifax":26590,"alpha":26591,"Ġsinister":26592,"Ġburger":26593,"fighting":26594,"Score":26595,"ĠStones":26596,"iem":26597,"405":26598,"chemy":26599,"Ġvinegar":26600,"nom":26601,"Ġprevailing":26602,"ĠLatest":26603,"¶":26604,"Ġba":26605,"ĠWriter":26606,"Ġ177":26607,"ĠConway":26608,"Ġcollects":26609,"Ġquantitative":26610,"Ġhorrors":26611,"ogens":26612,"ĠSlov":26613,"Ġlays":26614,"haw":26615,"ĠSlash":26616,"Ġnightclub":26617,"ĠDavies":26618,"Ġbride":26619,"ĠScarlet":26620,"ymm":26621,"ĠApplications":26622,"velength":26623,"Ġrevival":26624,"Ġsoftly":26625,"Ġzoo":26626,"itaire":26627,"Cur":26628,"Ġelectrom":26629,"Ġplanting":26630,"OTO":26631,"ĠElements":26632,"Ġswallow":26633,"porter":26634,"Ġlaptops":26635,"Ġpeanut":26636,"Ġlobbyists":26637,"β":26638,"Panel":26639,"ĠJoan":26640,"imil":26641,"tnc":26642,"Ġresisted":26643,"Ġoutwe":26644,"Ġretaining":26645,"atri":26646,"Ġpoorer":26647,"ĠSyrians":26648,"ĠHammond":26649,"Ġweld":26650,"uder":26651,"topic":26652,"ĠTT":26653,"ricia":26654,"Ġthieves":26655,"Lic":26656,"ĠGust":26657,"ĠWays":26658,"areth":26659,"243":26660,"Ġbroadcaster":26661,"shield":26662,"assium":26663,"uble":26664,"Ġairstrikes":26665,"onso":26666,"Ġpedal":26667,"Ġcollectors":26668,"ĠVander":26669,"ĠMesa":26670,"Ġdictator":26671,"Ġdir":26672,"enton":26673,"cart":26674,"score":26675,"adder":26676,"Cry":26677,"Ġssh":26678,"gger":26679,"Ġdrunken":26680,"ĠGS":26681,"ĠSeat":26682,"Ġcornerback":26683,"Ġskipped":26684,"ĠResearchers":26685,"ĠAudi":26686,"Reference":26687,"Ġhaunted":26688,"ë":26689,"ĠClinic":26690,"cz":26691,"Ġps":26692,"ĠPaladin":26693,"ĠRecipe":26694,"Ġstigma":26695,"oppy":26696,"Ġmonkeys":26697,"ĠHawk":26698,"Sad":26699,"\"/>":26700,"ĠWorkshop":26701,"ĠRetail":26702,"ĠAvatar":26703,"625":26704,"Na":26705,"ĠVC":26706,"ĠSecure":26707,"MY":26708,"1988":26709,"ossip":26710,"Ġprostate":26711,"Ġunden":26712,"Ġgamer":26713,"ĠContents":26714,"ĠWarhammer":26715,"ĠSentinel":26716,"310":26717,"Ġsegregation":26718,"ĠFlex":26719,"ĠMAY":26720,"Ġdrills":26721,"ĠDrugs":26722,"Islamic":26723,"Ġspur":26724,"Ġcafe":26725,"Ġimaginary":26726,"Ġguiding":26727,"Ġswings":26728,"ĠTheme":26729,"oby":26730,"Ġnud":26731,"Ġbegging":26732,"Ġstrongh":26733,"Ġrejecting":26734,"Ġpedestrians":26735,"ĠProspect":26736,"Rare":26737,"sle":26738,"Ġconcessions":26739,"ĠConstitutional":26740,"Ġbeams":26741,"Ġfibers":26742,"poon":26743,"Ġinstincts":26744,"property":26745,"ĠBIG":26746,"Sanders":26747,"imates":26748,"Ġcoating":26749,"Ġcorpses":26750,"ĠTRUE":26751,"checked":26752,"Ġ166":26753,"Ash":26754,"ĠJS":26755,"ĠFiction":26756,"Ġcommunal":26757,"Ġenergetic":26758,"oooooooo":26759,"Ġnowadays":26760,"ILD":26761,"ibo":26762,"ĠSUV":26763,"Ren":26764,"Ġdwelling":26765,"Silver":26766,"Ġtally":26767,"ĠMoving":26768,"Ġcoward":26769,"Ġgenerals":26770,"Ġhorns":26771,"Ġcirculated":26772,"Ġrobbed":26773,"ĠUnlimited":26774,"Ġharassed":26775,"Ġinhibit":26776,"Ġcomposer":26777,"ĠSpotify":26778,"Ġspreads":26779,"364":26780,"Ġsuicidal":26781,"Ġnoises":26782,"ĠStur":26783,"Ġsaga":26784,"ĠKag":26785,"iso":26786,"Ġtheoretically":26787,"Money":26788,"Ġsimilarity":26789,"Ġsliced":26790,"utils":26791,"inges":26792,"\"-":26793,"Ġanth":26794,"Ġimped":26795,"Module":26796,"Throughout":26797,"Ġmenus":26798,"committee":26799,"andi":26800,"obj":26801,"inav":26802,"fired":26803,"ĠAbdullah":26804,"Ġundead":26805,"Ġfonts":26806,"Hold":26807,"ENG":26808,"Ġsustainability":26809,"Ġflick":26810,"Ġrazor":26811,"ĠFest":26812,"ĠCharacters":26813,"Ġwording":26814,"Ġpopulist":26815,"Ġcriticizing":26816,"Ġmuse":26817,"vine":26818,"Ġcardboard":26819,"Ġkindly":26820,"Ġfringe":26821,"ĠTheft":26822,"icultural":26823,"Ġgovernors":26824,"Ġ����":26825,"Ġ163":26826,"Ġtimeout":26827,"ĠAuth":26828,"Children":26829,"AU":26830,"Ġredemption":26831,"ĠAlger":26832,"Ġ1914":26833,"Ġwaved":26834,"Ġastronauts":26835,"ograms":26836,"Ġswamp":26837,"ĠFinnish":26838,"Ġcandle":26839,"Ġtonnes":26840,"utm":26841,"Ġray":26842,"Ġspun":26843,"Ġfearful":26844,"articles":26845,"Ġcaus":26846,"orically":26847,"ĠRequires":26848,"ĠGol":26849,"Ġpope":26850,"Ġinaugural":26851,"Ġgle":26852,"ADA":26853,"ĠISIL":26854,"ĠOffensive":26855,"Ġwatchdog":26856,"Ġbalcon":26857,"entity":26858,"ĠHoo":26859,"Ġgallon":26860,"ACC":26861,"Ġdoubling":26862,"Ġimplication":26863,"ĠSight":26864,"Ġdoctr":26865,"-------":26866,"Ġ\\\\":26867,"Ġmalt":26868,"Roll":26869,"Ġâī¥":26870,"Ġrecap":26871,"adding":26872,"uces":26873,"ĠBend":26874,"figure":26875,"Ġturkey":26876,"Ġsocietal":26877,"ĠTickets":26878,"Ġcommercially":26879,"Ġspicy":26880,"Ġ216":26881,"ĠRamp":26882,"Ġsuperiority":26883,"ï":26884,"ĠTracker":26885,"Carl":26886,"ĠCoy":26887,"ĠPatriot":26888,"Ġconsulted":26889,"Ġlistings":26890,"Ġslew":26891,"reenshot":26892,"ĠGone":26893,"Ġ[...]":26894,"309":26895,"Ġhottest":26896,"ر":26897,"Ġrocky":26898,"ĠDiaz":26899,"Ġmassage":26900,"Ġparaly":26901,"Ġpony":26902,"Az":26903,"Ġcartridge":26904,"ĠNZ":26905,"Ġsnack":26906,"ĠLamar":26907,"plement":26908,"ĠLeslie":26909,"Ġmater":26910,"Ġsnipp":26911,"246":26912,"Ġjointly":26913,"ĠBrisbane":26914,"ĠiPod":26915,"Ġpumping":26916,"Ġgoat":26917,"ĠSharon":26918,"ealing":26919,"Ġcoron":26920,"Ġanomal":26921,"rahim":26922,"ĠConnection":26923,"Ġsculpture":26924,"Ġscheduling":26925,"ĠDaddy":26926,"athing":26927,"Ġeyebrows":26928,"Ġcurved":26929,"Ġsentiments":26930,"Ġdrafting":26931,"Drop":26932,"([":26933,"Ġnominal":26934,"ĠLeadership":26935,"ĠGrow":26936,"Ġ176":26937,"Ġconstructive":26938,"ivation":26939,"Ġcorrupted":26940,"gerald":26941,"ĠCros":26942,"ĠChester":26943,"ĠLap":26944,"ãģª":26945,"OTH":26946,"DATA":26947,"Ġalmond":26948,"probably":26949,"Imp":26950,"Ġfeast":26951,"ĠWarcraft":26952,"Flor":26953,"Ġcheckpoint":26954,"Ġtranscription":26955,"Ġ204":26956,"Ġtweaks":26957,"Ġrelieve":26958,"Science":26959,"Ġperformer":26960,"Zone":26961,"Ġturmoil":26962,"igated":26963,"hibit":26964,"ĠCafe":26965,"themed":26966,"Ġfluor":26967,"bench":26968,"Ġdecom":26969,"ĠUnt":26970,"ĠBarrett":26971,"ĠFacts":26972,"Ġtasting":26973,"ĠPTSD":26974,"ĠSeal":26975,"ĠJudaism":26976,"ĠDynamic":26977,"ĠCors":26978,"Ve":26979,"ĠMing":26980,"ĠTransform":26981,"von":26982,"ĠDefenders":26983,"ĠTactical":26984,"ĠVon":26985,"ĠUnivers":26986,"Ġdistorted":26987,"ĠBreath":26988,"?'\"":26989,"Ġagon":26990,"ĠDeadly":26991,"Ġlan":26992,"ĠCycle":26993,"orned":26994,"Ġreliably":26995,"Ġglor":26996,"ĠMonkey":26997,"ãĥ¡":26998,"Ġadren":26999,"Ġmicrowave":27000,"ĠAlban":27001,"ircraft":27002,"digit":27003,"smart":27004,"ĠDread":27005,"¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯¯":27006,"{{":27007,"ĠRochester":27008,"Ġsimplified":27009,"Ġinflicted":27010,"Ġtakeover":27011,"Ġyourselves":27012,"aditional":27013,"Ġmuscular":27014,"KS":27015,"Ġingen":27016,"Tax":27017,"ĠFeature":27018,"277":27019,"Ġcruc":27020,"Ġcrate":27021,"Ġunidentified":27022,"Ġacclaimed":27023,"ĠManga":27024,"ĠFrances":27025,"ĠNepal":27026,"ĠGerald":27027,"ĠKuwait":27028,"Ġslain":27029,"ĠHeb":27030,"ĠGoku":27031,"ã쮿":27032,"286":27033,"Mrs":27034,"ĠCody":27035,"ĠSanctuary":27036,"016":27037,"Ġdismant":27038,"Ġdataset":27039,"ĠHond":27040,"buck":27041,"ĠPatterson":27042,"Ġpalette":27043,"ĠGD":27044,"icol":27045,"ĠLodge":27046,"Ġplanetary":27047,"akin":27048,"ĠRegistered":27049,"abwe":27050,"ĠPetersburg":27051,"Ġhailed":27052,"ĠPiece":27053,"Sche":27054,"ĠDOJ":27055,"Ġenumer":27056,"181":27057,"ĠObserver":27058,"ĠBold":27059,"founded":27060,"commerce":27061,"Ġexploits":27062,"ĠFinding":27063,"URN":27064,"ĠSne":27065,"ĠAcid":27066,"ayette":27067,"ĠValues":27068,"Ġdrastic":27069,"Ġarchitectural":27070,"Ġ\".":27071,"×ķ":27072,"umped":27073,"Ġwrapping":27074,"Ġwidow":27075,"ĠSlayer":27076,"lace":27077,"once":27078,"Germany":27079,"avoid":27080,"Ġtemples":27081,"PAR":27082,"ô":27083,"ĠLucifer":27084,"ĠFlickr":27085,"lov":27086,"forces":27087,"Ġscouting":27088,"Ġlouder":27089,"tesy":27090,"Ġbeforehand":27091,"Äĵ":27092,"ĠNeon":27093,"ĠWol":27094,"ĠTypically":27095,"ĠPolitico":27096,"-+-+":27097,"Ġbuilder":27098,"Ġderive":27099,"Kill":27100,"Ġpoker":27101,"Ġambiguous":27102,"Ġlifts":27103,"Ġcyt":27104,"Ġribs":27105,"oodle":27106,"ĠSounds":27107,"hair":27108,"ĠSyndrome":27109,"tf":27110,"Ġproportional":27111,"uid":27112,"Ġpertaining":27113,"ĠKindle":27114,"ĠNegro":27115,"Ġreiterated":27116,"ĠTonight":27117,"oths":27118,"ĠCornell":27119,"Ġowing":27120,"Ġ208":27121,"elfare":27122,"ocating":27123,"ĠBirds":27124,"Subscribe":27125,"Ġessays":27126,"Ġburdens":27127,"Ġillustrations":27128,"arious":27129,"ERAL":27130,"ĠCalcul":27131,"Ġxen":27132,"ĠLinkedIn":27133,"ĠJung":27134,"Ġredesign":27135,"Connor":27136,"296":27137,"Ġreversal":27138,"ĠAdelaide":27139,"ĠLL":27140,"Ġsinking":27141,"Ġgum":27142,"USH":27143,"capt":27144,"ĠGrimm":27145,"Ġfootsteps":27146,"ĠCBD":27147,"ispers":27148,"Ġprose":27149,"Wednesday":27150,"ĠMovies":27151,"edin":27152,"Ġoverturned":27153,"Ġcontentious":27154,"USB":27155,"~~~~~~~~~~~~~~~~":27156,"ĠCopper":27157,"Ġpointless":27158,"NV":27159,"values":27160,"olphin":27161,"dain":27162,"Ġdeposited":27163,"ĠGW":27164,"Ġpreceded":27165,"ĠCla":27166,"ĠGolem":27167,"ĠNim":27168,"Ġβ":27169,"ĠEngineers":27170,"middle":27171,"Ġflatt":27172,"operative":27173,"Ġcouncils":27174,"imbabwe":27175,"elin":27176,"Ġstressful":27177,"ĠLD":27178,"Ġresh":27179,"lake":27180,"Ġwheelchair":27181,"ĠAlternative":27182,"Ġoptimize":27183,"operation":27184,"Ġpeek":27185,"Ġoneself":27186,"igil":27187,"Ġtransitions":27188,"opathy":27189,"blank":27190,"Ġ169":27191,"171":27192,"________________________________________________________________":27193,"Ġlaundering":27194,"Enc":27195,"ĠDEC":27196,"Ġworkouts":27197,"Ġspikes":27198,"Ġdinosaurs":27199,"Ġdiscriminatory":27200,"Pool":27201,"Rather":27202,"385":27203,"RNA":27204,"testers":27205,"eto":27206,"ĠIdentity":27207,"Ġvein":27208,"ĠBurton":27209,"Ġarcade":27210,"420":27211,"Ultimately":27212,"ĠSadly":27213,"ð":27214,"pill":27215,"Ġcubic":27216,"ĠSpectrum":27217,"these":27218,"states":27219,"Ġunofficial":27220,"hawks":27221,"ĠEVERY":27222,"Ġrainbow":27223,"Ġincarceration":27224,"anding":27225,"Ġsyll":27226,"ĠEverton":27227,"Ġ179":27228,"ĠSerbia":27229,"Ġ189":27230,"meter":27231,"ĠMickey":27232,"Ġantiqu":27233,"Ġfactual":27234,"neck":27235,"ĠNare":27236,"norm":27237,"must":27238,"Ġhighways":27239,"Ġglam":27240,"Ġdividing":27241,"ĠSquadron":27242,"ĠMartha":27243,"Ġbirths":27244,"Cover":27245,"////////////////":27246,"ĠWong":27247,"Phot":27248,"ĠALS":27249,"rio":27250,"ĠNonetheless":27251,"ĠLemon":27252,"Ġ206":27253,"ĠEE":27254,"Ġderivative":27255,"ĠWWII":27256,"vote":27257,"Ġtherein":27258,"Ġseparating":27259,"446":27260,"sync":27261,"ĠStreets":27262,"Ġratt":27263,"Ġmunicipality":27264,"ĠShortly":27265,"Ġmonk":27266,"),\"":27267,"Ġscrub":27268,"Ġoperatives":27269,"Neither":27270,"Place":27271,"ĠLimit":27272,"Female":27273,"ĠActor":27274,"Character":27275,"Ġconstituted":27276,"357":27277,"Ġprotested":27278,"ĠStraw":27279,"ĠHeight":27280,"ilda":27281,"ĠTyph":27282,"Ġfloods":27283,"Ġcosmetic":27284,"WAY":27285,"perture":27286,"upon":27287,"tons":27288,"essing":27289,"ĠPocket":27290,"Ġrooft":27291,"ĠCaucas":27292,"Ġantidepress":27293,"Ġincompatible":27294,"ECD":27295,"Ġopera":27296,"ĠContest":27297,"Ġgenerators":27298,"lime":27299,"Defense":27300,"1987":27301,"forum":27302,"Ġsavage":27303,"ĠHungarian":27304,"nz":27305,"Ġmetallic":27306,"Ġexpelled":27307,"Ġresidency":27308,"Ġdresses":27309,"666":27310,"ĠClement":27311,"fires":27312,"Category":27313,"Ġgeek":27314,"alis":27315,"Ġcemetery":27316,"educated":27317,"Ġcrawl":27318,"ĠUnable":27319,"ĠTyson":27320,"akis":27321,"Ġpardon":27322,"ĠWra":27323,"Ġstrengthened":27324,"ĠFors":27325,"335":27326,"ĠHC":27327,"ĠMond":27328,"Ġvisuals":27329,"ĠBeatles":27330,"ettlement":27331,"Ġï":27332,"gro":27333,"Ġbash":27334,"Ġpoorest":27335,"Ġexcel":27336,"Ġaspirations":27337,"ĠMunicip":27338,"ensible":27339,"Ġceremonies":27340,"Ġintimidation":27341,"ĠCONTR":27342,"beck":27343,"ĠKap":27344,"asu":27345,"Ġtrademarks":27346,"ĠSew":27347,"ĠCompetition":27348,"network":27349,"ĠArri":27350,"ĠTet":27351,"Roaming":27352,"WC":27353,"Dat":27354,"Ġsob":27355,"Ġpairing":27356,"Ġoverdose":27357,"SAY":27358,"aber":27359,"Ġrevolt":27360,"ĠFah":27361,"acting":27362,"eq":27363,"estation":27364,"Fight":27365,"ĠMarks":27366,"273":27367,"Ġ178":27368,"Raw":27369,"ãģĭ":27370,"349":27371,"blocks":27372,"Ġverge":27373,"estine":27374,"ĠPodesta":27375,"Ġinvasive":27376,"Ġprofoundly":27377,"ĠAo":27378,"each":27379,"Ġlest":27380,"interpret":27381,"Ġshrinking":27382,"Ġerrone":27383,"Ġchees":27384,"lys":27385,"ĠIvy":27386,"ĠDirectory":27387,"Ġhinted":27388,"VICE":27389,"Ġcontacting":27390,"ĠGent":27391,"hei":27392,"Ġlabeling":27393,"Ġmercury":27394,"ĠLite":27395,"Ġexpires":27396,"Ġdestabil":27397,"ritis":27398,"cu":27399,"Ġfeathers":27400,"Ġsteer":27401,"Ġprogrammed":27402,"ĠVader":27403,"Going":27404,"ĠElim":27405,"Ġyo":27406,"ĠMiche":27407,"Ġ203":27408,"Ġsleeves":27409,"Ġbully":27410,"ĠHumans":27411,"368":27412,"Ġcompress":27413,"ĠBanner":27414,"ARS":27415,"Ġawhile":27416,"Ġcalib":27417,"Ġsponsorship":27418,"ĠDifficulty":27419,"ĠPapers":27420,"Ġidentifier":27421,"}.":27422,"Ġyog":27423,"ĠShia":27424,"Ġcleanup":27425,"Ġvibe":27426,"introdu":27427,"imming":27428,"Australia":27429,"Ġoutlines":27430,"ĠYoutube":27431,"train":27432,"ĠMakes":27433,"Ġdeported":27434,"Ġcentr":27435,"ĠDug":27436,"ĠBoulder":27437,"ĠBuffy":27438,"Ġinjunction":27439,"ĠHarley":27440,"ĠGroups":27441,"ĠDumbledore":27442,"ĠClara":27443,"Ġ\"-":27444,"Ġsacrificed":27445,"eph":27446,"Shadow":27447,"ibling":27448,"Ġfreelance":27449,"Ġevidently":27450,"phal":27451,"Ġretains":27452,"Mir":27453,"Ġfinite":27454,"dar":27455,"ĠCous":27456,"Ġrepaired":27457,"Ġperiodic":27458,"Ġchampionships":27459,"Ġasteroid":27460,"blind":27461,"Ġexpressly":27462,"ĠAstros":27463,"Ġscaled":27464,"Ġgeographical":27465,"ĠRapids":27466,"Enjoy":27467,"Ġelastic":27468,"ĠMohamed":27469,"Market":27470,"begin":27471,"Ġdiscovers":27472,"Ġtelecommunications":27473,"Ġscanner":27474,"Ġenlarge":27475,"Ġsharks":27476,"Ġpsychedel":27477,"ĠRouge":27478,"Ġsnapshot":27479,"isine":27480,"XP":27481,"Ġpesticides":27482,"ĠLSD":27483,"ĠDistribution":27484,"really":27485,"Ġdegradation":27486,"Ġdisguise":27487,"Ġbiom":27488,"ĠEXT":27489,"Ġequations":27490,"Ġhazards":27491,"ĠCompared":27492,")*":27493,"Ġvirtues":27494,"Ġelders":27495,"Ġenhancing":27496,"ĠAcross":27497,"eros":27498,"angling":27499,"Ġcombust":27500,"ucci":27501,"Ġconcussion":27502,"Ġcontraception":27503,"ĠKang":27504,"Ġexpresses":27505,"Ġaux":27506,"ĠPione":27507,"Ġexhibits":27508,"Debug":27509,"OTAL":27510,"ĠAlready":27511,"ĠWheeler":27512,"Ġexpands":27513,"?:":27514,"Ġreconciliation":27515,"Ġpirates":27516,"Ġpurse":27517,"Ġdiscourage":27518,"Ġspectacle":27519,"Rank":27520,"Ġwraps":27521,"ĠThought":27522,"Ġimpending":27523,"Opp":27524,"ĠAnglo":27525,"ĠEUR":27526,"Ġscrewed":27527,"retched":27528,"Ġencouragement":27529,"models":27530,"Ġconfuse":27531,"mmm":27532,"ĠVitamin":27533,"âĸijâĸij":27534,"Cru":27535,"Ġknights":27536,"Ġdiscard":27537,"Ġbishops":27538,"ĠWear":27539,"ĠGarrett":27540,"kan":27541,"ãĥŁ":27542,"Ġmasculine":27543,"capital":27544,"ĠAus":27545,"Ġfatally":27546,"thanks":27547,"ĠAU":27548,"ĠGut":27549,"1200":27550,"Ġ00000000":27551,"Ġsurrog":27552,"ĠBIOS":27553,"raits":27554,"ĠWatts":27555,"Ġresurrection":27556,"ĠElectoral":27557,"ĠTips":27558,"4000":27559,"Ġnutrient":27560,"Ġdepicting":27561,"Ġsprink":27562,"Ġmuff":27563,"ĠLIM":27564,"ĠSample":27565,"psc":27566,"ibi":27567,"generated":27568,"Ġspecimens":27569,"Ġdissatisf":27570,"Ġtailored":27571,"Ġholdings":27572,"ĠMonthly":27573,"ĠEat":27574,"poons":27575,"Ġnec":27576,"ĠCage":27577,"ĠLotus":27578,"ĠLantern":27579,"Ġfrontier":27580,"Ġpensions":27581,"Ġjoked":27582,"ĠHardy":27583,"=-=-=-=-":27584,"rade":27585,"UID":27586,"Ġrails":27587,"Ġemit":27588,"Ġslate":27589,"Ġsmug":27590,"Ġspit":27591,"ĠCalls":27592,"ĠJacobs":27593,"feat":27594,"ĠUE":27595,"Ġrestruct":27596,"Ġregeneration":27597,"Ġenergies":27598,"ĠConnor":27599,"OHN":27600,"ĠCheese":27601,"Ġger":27602,"Ġresurrect":27603,"management":27604,"NW":27605,"Ġpresently":27606,"ĠBruins":27607,"Member":27608,"ĠMang":27609,"idan":27610,"Ġboosting":27611,"wyn":27612,"+.":27613,"requisite":27614,"ĠNYPD":27615,"ĠMegan":27616,"ĠConditions":27617,"Ġpics":27618,"nesium":27619,"ĠRash":27620,"Ġ174":27621,"ĠDucks":27622,"Ġembro":27623,"zu":27624,"onian":27625,"religious":27626,"Ġcraz":27627,"ĠACA":27628,"ĠZucker":27629,"EMA":27630,"ĠPros":27631,"Weapon":27632,"ĠKnox":27633,"ĠArduino":27634,"Ġstove":27635,"Ġheavens":27636,"ĠPurchase":27637,"Ġherd":27638,"Ġfundraiser":27639,"Digital":27640,"5000":27641,"Ġproponents":27642,"/âĢĭ":27643,"Ġjelly":27644,"ĠVisa":27645,"Ġmonks":27646,"Ġadvancement":27647,"ĠWer":27648,"Ġ187":27649,"eus":27650,"ertility":27651,"Ġfetal":27652,"Ġ1936":27653,"Lo":27654,"Ġoutfits":27655,"Ġstaircase":27656,"bomb":27657,"Ġcustomized":27658,"clair":27659,"Tree":27660,"Ġmapped":27661,"ĠConsidering":27662,"ĠTorres":27663,"Ġmethyl":27664,"Ġapproximate":27665,"Ġdoom":27666,"ĠHansen":27667,"Ġcrossover":27668,"Ġstandalone":27669,"ä¼":27670,"Ġinvites":27671,"Ġgraveyard":27672,"Ġhp":27673,"DonaldTrump":27674,"Ġescort":27675,"Gar":27676,"Ġpredecessors":27677,"Ġhay":27678,"Ġenzyme":27679,"ĠStraight":27680,"visors":27681,"Ing":27682,"aneously":27683,"ĠApplied":27684,"Ġfec":27685,"ĠDurant":27686,"Ġoutspoken":27687,"orb":27688,"Ġzeal":27689,"Ġdisgrace":27690,"').":27691,"ĠCheng":27692,"289":27693,"ĠRena":27694,"ĠSuicide":27695,"294":27696,"Ġoutraged":27697,"ĠNewman":27698,"ĠNvidia":27699,"ĠAber":27700,"ĠBers":27701,"Ġrecreation":27702,"Window":27703,"ĠDP":27704,"xe":27705,"Ġpedoph":27706,"Ġfallout":27707,"amboo":27708,"Ġpresentations":27709,"ĠApps":27710,"Ġhtml":27711,"345":27712,"ĠXXX":27713,"Ġrubbing":27714,"ĠLeather":27715,"Ġhumidity":27716,"seys":27717,"established":27718,"ĠUnits":27719,"646":27720,"Ġrespectable":27721,"Auto":27722,"Ġthriving":27723,"ĠInnovation":27724,"angs":27725,"Extra":27726,"regulation":27727,"298":27728,"pick":27729,"Examples":27730,"ĠCJ":27731,"Attack":27732,"Ġdracon":27733,"LT":27734,"Ġsticker":27735,"rers":27736,"Ġsunny":27737,"Iss":27738,"regulated":27739,"dim":27740,"ĠAbstract":27741,"Ġhusbands":27742,"Office":27743,"omination":27744,"itars":27745,"ANGE":27746,"ascal":27747,"ĠKris":27748,"ĠInfantry":27749,"Ġmalf":27750,"ĠAthe":27751,"ĠRally":27752,"balanced":27753,"........................":27754,"OUP":27755,"Ġmolecule":27756,"metics":27757,"ĠSplit":27758,"ĠInstructions":27759,"ĠNights":27760,"cards":27761,"Ġtug":27762,"Ġcone":27763,"åŃ":27764,"Ġtx":27765,"ĠDiscussion":27766,"Ġcatastrophe":27767,"ppe":27768,"gio":27769,"Ġcommunism":27770,"Ġhalted":27771,"ĠGuant":27772,"clean":27773,"ĠSched":27774,"ĠKanye":27775,"Ġwander":27776,"ĠSeriously":27777,"Ġ188":27778,"ennial":27779,"follow":27780,"productive":27781,"ĠFlow":27782,"ĠSail":27783,"Ġcraw":27784,"Ġsimulations":27785,"oru":27786,"angles":27787,"ĠNolan":27788,"Ġmenstru":27789,"470":27790,"Ġ207":27791,"aja":27792,"Ġcasually":27793,"boarding":27794,"Ġ222":27795,"ovy":27796,"ĠNumbers":27797,"umat":27798,"OE":27799,"287":27800,"ĠClemson":27801,"Ġcerts":27802,"Ġslid":27803,"ĠTribe":27804,"Ġtoast":27805,"Ġfortunes":27806,"Ġfals":27807,"ĠCommittees":27808,"Ġgp":27809,"Ġfiery":27810,"ĠNets":27811,"ĠAnime":27812,"Package":27813,"ĠCompare":27814,"laughter":27815,"infect":27816,"Ġatrocities":27817,"Ġjustices":27818,"Ġinsults":27819,"ĠVernon":27820,"Ġshaken":27821,"Ġpersona":27822,"estamp":27823,"367":27824,"brain":27825,"Ġexperimenting":27826,"Ken":27827,"ĠElectronics":27828,"Ġ161":27829,"domain":27830,"Ġgraphical":27831,"bishop":27832,"Ġwhopping":27833,"ĠEvangel":27834,"Ġadvertisers":27835,"ĠSpear":27836,"Ġbids":27837,"Ġdestroys":27838,"utz":27839,"Ġundersc":27840,"ĠADD":27841,"Ġants":27842,"ĠCum":27843,"ipples":27844,"ĠFill":27845,"Ġglanced":27846,"Ġindicted":27847,"ĠEff":27848,"Ġmiscon":27849,"ĠDesktop":27850,"Ġabide":27851,"ãĥĢ":27852,"ĠIo":27853,"ĠCoul":27854,"Ġcapsule":27855,"ĠChrys":27856,"MON":27857,"Ġundes":27858,"ĠIRA":27859,"Ġcitation":27860,"Ġdictate":27861,"ĠNetworks":27862,"ĠConflict":27863,"ĠStuff":27864,"xa":27865,"isec":27866,"ĠChemistry":27867,"Ġquarterly":27868,"Williams":27869,"anan":27870,"Opt":27871,"ĠAlexandria":27872,"outheastern":27873,"ĠSpringfield":27874,"ĠBlacks":27875,"Ġgeography":27876,"242":27877,"Ġutmost":27878,"ĠExxon":27879,"abouts":27880,"EVA":27881,"ĠEnable":27882,"ĠBarr":27883,"Ġdisagreed":27884,"ĠCyprus":27885,"Ġdementia":27886,"Ġlabs":27887,"Ġubiquitous":27888,"ĠLOVE":27889,"Ġconsolidated":27890,"sr":27891,"Ġcreamy":27892,"ĠTimber":27893,"Regardless":27894,"ĠCertificate":27895,"Ġ\"...":27896,"ogenous":27897,"Captain":27898,"Ġinsulting":27899,"ĠSoros":27900,"ĠInstr":27901,"ĠBulgaria":27902,"better":27903,"Ġsucking":27904,"ĠDavidson":27905,"atz":27906,"Ġcollateral":27907,"gif":27908,"Ġplagued":27909,"ĠCancel":27910,"ĠGardner":27911,"RB":27912,"Ġsixteen":27913,"Remove":27914,"uristic":27915,"cook":27916,"Rod":27917,"Ġcomprising":27918,"fle":27919,")âĢĶ":27920,"ĠViking":27921,"growth":27922,"agonal":27923,"Ġsrf":27924,"afety":27925,"mot":27926,"Nearly":27927,"stown":27928,"ĠFactor":27929,"Ġautomobile":27930,"Ġprocedural":27931,"mask":27932,"ampires":27933,"Ġdisappears":27934,"jab":27935,"315":27936,"Ġ1951":27937,"needed":27938,"Ġdaring":27939,"leader":27940,"Ġpodium":27941,"Ġunhealthy":27942,"Ġmund":27943,"Ġpyramid":27944,"ocre":27945,"Ġkissed":27946,"Ġdreamed":27947,"ĠFantastic":27948,"ĠGly":27949,"åĬ":27950,"Ġgreatness":27951,"Ġspices":27952,"Ġmetropolitan":27953,"Ġcompuls":27954,"iets":27955,"1016":27956,"ĠSham":27957,"ĠPyr":27958,"flies":27959,"ĠMidnight":27960,"Ġswallowed":27961,"Ġgenres":27962,"ĠLucky":27963,"ĠRewards":27964,"Ġdispatch":27965,"ĠIPA":27966,"ĠApply":27967,"Ġaven":27968,"alities":27969,"312":27970,"things":27971,"Ġ().":27972,"Ġmates":27973,"ĠSz":27974,"ĠCOP":27975,"olate":27976,"OFF":27977,"Ġrecharge":27978,"caps":27979,"ĠYorker":27980,"icone":27981,"Ġgalaxies":27982,"ileaks":27983,"Dave":27984,"ĠPuzz":27985,"ĠCeltic":27986,"ĠAFC":27987,"276":27988,"ĠSons":27989,"Ġaffirmative":27990,"Hor":27991,"Ġtutorials":27992,"ĠCITY":27993,"ĠRosa":27994,"ĠExtension":27995,"Series":27996,"Ġfats":27997,"Ġrab":27998,"lis":27999,"Ġunic":28000,"Ġeve":28001,"ĠSpin":28002,"Ġadulthood":28003,"typ":28004,"Ġsectarian":28005,"Ġcheckout":28006,"ĠCycl":28007,"Single":28008,"Ġmartyr":28009,"Ġchilling":28010,"888":28011,"oufl":28012,"Ġ];":28013,"Ġcongestion":28014,"mk":28015,"ĠWhereas":28016,"Ġ1938":28017,"urrencies":28018,"erion":28019,"Ġboast":28020,"ĠPatients":28021,"Ġchap":28022,"ĠBD":28023,"realDonaldTrump":28024,"Ġexamines":28025,"hov":28026,"Ġstartling":28027,"ĠBabylon":28028,"wid":28029,"omew":28030,"brance":28031,"ĠOdyssey":28032,"wig":28033,"Ġtorch":28034,"ĠVox":28035,"ĠMoz":28036,"ĠTroll":28037,"ĠAns":28038,"Similarly":28039,"ĠFul":28040,"006":28041,"Unless":28042,"ĠAlone":28043,"stead":28044,"ĠPublisher":28045,"rights":28046,"tu":28047,"ĠDoesn":28048,"Ġprofessionally":28049,"Ġclo":28050,"icz":28051,"Ġsteals":28052,"Ġá":28053,"1986":28054,"Ġsturdy":28055,"ĠJohann":28056,"Ġmedals":28057,"Ġfilings":28058,"ĠFraser":28059,"done":28060,"Ġmultinational":28061,"Ġfeder":28062,"Ġworthless":28063,"Ġpest":28064,"Yesterday":28065,"ankind":28066,"Ġgays":28067,"Ġborne":28068,"ĠPOS":28069,"Picture":28070,"Ġpercentages":28071,"251":28072,"rame":28073,"Ġpotions":28074,"AMD":28075,"ĠLebanese":28076,"Ġrang":28077,"ĠLSU":28078,"ongs":28079,"Ġpeninsula":28080,"ĠClause":28081,"ALK":28082,"oha":28083,"ĠMacBook":28084,"Ġunanimous":28085,"Ġlenders":28086,"Ġhangs":28087,"Ġfranchises":28088,"orers":28089,"ĠUpdates":28090,"Ġisolate":28091,"andro":28092,"Soon":28093,"Ġdisruptive":28094,"ĠSurve":28095,"Ġstitches":28096,"ĠScorp":28097,"ĠDominion":28098,"Ġsupplying":28099,"Arg":28100,"Ġturret":28101,"ĠLuk":28102,"Ġbrackets":28103,"*)":28104,"ĠRevolutionary":28105,"ĠHonest":28106,"Ġnoticing":28107,"ĠShannon":28108,"Ġafforded":28109,"Ġtha":28110,"ĠJanet":28111,"!--":28112,"ĠNarendra":28113,"ĠPlot":28114,"Hol":28115,"sever":28116,"eenth":28117,"Ġobstruction":28118,"Ġ1024":28119,"staff":28120,"jas":28121,"orget":28122,"scenes":28123,"laughs":28124,"ĠFargo":28125,"crime":28126,"Ġorchestr":28127,"Ġdelet":28128,"iliary":28129,"rieved":28130,"Ġmilitar":28131,"ĠGreene":28132,"âĹı":28133,"ãģ¦":28134,"ĠGuards":28135,"Ġunleashed":28136,"ĠWeber":28137,"Ġadjustable":28138,"Ġcaliber":28139,"Ġmotivations":28140,"ĠÃł":28141,"mAh":28142,"ĠLanka":28143,"handle":28144,"Ġpent":28145,"ĠRav":28146,"ĠAngular":28147,"ĠKau":28148,"umbing":28149,"Ġphilanthrop":28150,"Ġdehyd":28151,"Ġtoxicity":28152,"eer":28153,"ĠYORK":28154,"witz":28155,"å¼":28156,"ĠIE":28157,"community":28158,"ĠAH":28159,"Ġretali":28160,"Ġmassively":28161,"ĠDaniels":28162,"ĠDEL":28163,"Ġcarcin":28164,"Url":28165,"Ġrouting":28166,"ĠNPCs":28167,"ĠRAF":28168,"ryce":28169,"Ġwaived":28170,"ĠGuatem":28171,"Everybody":28172,"Ġcovenant":28173,"Ġ173":28174,"Ġrelaxing":28175,"Ġquart":28176,"almost":28177,"Ġguarded":28178,"ĠSoldiers":28179,"ĠPLAY":28180,"Ġoutgoing":28181,"LAND":28182,"Ġrewrite":28183,"ĠMOV":28184,"ĠImper":28185,"ĠSolution":28186,"Ġphenomenal":28187,"Ġlongevity":28188,"Ġimpat":28189,"ĠNissan":28190,"irie":28191,"Ġodor":28192,"ĠZar":28193,"oks":28194,"Ġmilitias":28195,"ĠSPEC":28196,"Ġtolerated":28197,"arser":28198,"ĠBradford":28199,"+,":28200,"Ġsurreal":28201,"sf":28202,"Canadian":28203,"Ġresemblance":28204,"Ġcarbohydrate":28205,"VIEW":28206,"Ġaccessory":28207,"meal":28208,"largest":28209,"iegel":28210,"Someone":28211,"Ġtoughest":28212,"oso":28213,"Ġfunnel":28214,"Ġcondemnation":28215,"luent":28216,"Ġwired":28217,"ĠSunset":28218,"Jesus":28219,"ĠPST":28220,"ĠPages":28221,"ĠTycoon":28222,"ĠPF":28223,"Ġselections":28224,"Ġà¤":28225,"partisan":28226,"Ġhighs":28227,"ĠRune":28228,"Ġcrafts":28229,"lead":28230,"ĠParents":28231,"Ġreclaim":28232,"eker":28233,"ĠAllied":28234,"aeper":28235,"Ġlooming":28236,"Ġbeneficiaries":28237,"ĠHull":28238,"Students":28239,"Jewish":28240,"dj":28241,"Ġpact":28242,"template":28243,"ĠOfficials":28244,"ĠBaylor":28245,"Ġhemp":28246,"Ġyouths":28247,"ĠLevels":28248,"ĠXiao":28249,"ĠChes":28250,"Ġendeavor":28251,"ĠRemoved":28252,"Ġhippocamp":28253,"Hell":28254,"ãĤĬ":28255,"805":28256,"Ġdinosaur":28257,"ĠWrath":28258,"ĠIndonesian":28259,"Ġcalculator":28260,"ĠDictionary":28261,"Ġ420":28262,"ĠMAG":28263,"(_":28264,"!,":28265,"tarians":28266,"Ġrestricting":28267,"racuse":28268,"Ġweekday":28269,"OUNT":28270,"Ġshrugged":28271,"leground":28272,"Ġbald":28273,"ĠDoctors":28274,"Ġtouted":28275,"ĠMaxwell":28276,"Ġ214":28277,"Ġdiplomat":28278,"Ġrepression":28279,"Ġconstituency":28280,"vice":28281,"ranked":28282,"ĠNapoleon":28283,"gang":28284,"ĠForever":28285,"tun":28286,"Ġbulb":28287,"ĠPDT":28288,"ĠCisco":28289,"VEN":28290,"Ġresumed":28291,"Steven":28292,"ĠManitoba":28293,"Ġfabulous":28294,"ĠAgents":28295,"1984":28296,"Ġamusing":28297,"ĠMysteries":28298,"Ġorthodox":28299,"floor":28300,"Ġquestionnaire":28301,"Ġpenetrate":28302,"Ġfilmmakers":28303,"ĠUnc":28304,"Ġstamped":28305,"Ġthirteen":28306,"Ġoutfield":28307,"Ġforwarded":28308,"Ġappra":28309,"Ġaided":28310,"try":28311,"Ġunfocused":28312,"ĠLiz":28313,"ĠWendy":28314,"ĠScene":28315,"Charg":28316,"Ġrejects":28317,"Ġleftist":28318,"ĠProvidence":28319,"ĠBrid":28320,"regn":28321,"Ġprophecy":28322,"ĠLIVE":28323,"499":28324,"Ġforge":28325,"ĠFML":28326,"Ġintrinsic":28327,"ĠFrog":28328,"Ġwont":28329,"ĠHolt":28330,"Ġfamed":28331,"CLUS":28332,"aepernick":28333,"ĠHate":28334,"ĠCay":28335,"Ġregistering":28336,"ortality":28337,"ropy":28338,"ocalyptic":28339,"aan":28340,"nav":28341,"Ġfascist":28342,"IFIED":28343,"Ġimplicated":28344,"ĠResort":28345,"ĠChandler":28346,"ĠBrick":28347,"Pin":28348,"ysc":28349,"Usage":28350,"ĠHelm":28351,"usra":28352,"âĺħâĺħ":28353,"ĠAbbas":28354,"Ġunanimously":28355,"Ġkeeper":28356,"Ġaddicted":28357,"???":28358,"Ġhelmets":28359,"Ġantioxid":28360,"apsed":28361,"808":28362,"giene":28363,"Ġwaits":28364,"Ġminion":28365,"raved":28366,"ĠPorsche":28367,"Ġdreaming":28368,"Ġ171":28369,"ĠCain":28370,"Ġunfor":28371,"asso":28372,"ĠConfiguration":28373,"kun":28374,"hardt":28375,"Ġnested":28376,"ĠLDS":28377,"LES":28378,"Ġtying":28379,"enos":28380,"Ġcue":28381,"ĠMarqu":28382,"skirts":28383,"Ġclicked":28384,"Ġexpiration":28385,"ĠAccordingly":28386,"ĠWC":28387,"Ġblessings":28388,"Ġaddictive":28389,"ĠNarr":28390,"yx":28391,"ĠJaguars":28392,"Ġrents":28393,"ĠSiber":28394,"Ġtipped":28395,"ousse":28396,"ĠFitzgerald":28397,"Ġhierarch":28398,"outine":28399,"Ġwavelength":28400,">.":28401,"chid":28402,"ĠProcessing":28403,"/+":28404,"ranking":28405,"Easy":28406,"ĠConstruct":28407,"Ġtet":28408,"insured":28409,"HUD":28410,"Ġquoting":28411,"Ġcommunicated":28412,"inx":28413,"Ġinmate":28414,"Ġerected":28415,"ĠAbsolutely":28416,"ĠSurely":28417,"Ġunim":28418,"ĠThrone":28419,"heid":28420,"Ġclaws":28421,"Ġsuperstar":28422,"ĠLenn":28423,"ĠWhis":28424,"Uk":28425,"abol":28426,"Ġsket":28427,"ĠNiet":28428,"Ġperks":28429,"Ġaffinity":28430,"Ġopenings":28431,"phasis":28432,"Ġdiscriminate":28433,"Tip":28434,"vc":28435,"Ġgrinding":28436,"ĠJenny":28437,"Ġasthma":28438,"holes":28439,"ĠHomer":28440,"Ġregisters":28441,"ĠGlad":28442,"Ġcreations":28443,"Ġlithium":28444,"Ġapplause":28445,"until":28446,"Justice":28447,"ĠTurks":28448,"Ġscandals":28449,"Ġbake":28450,"tank":28451,"Mech":28452,"ĠMeans":28453,"ĠMaid":28454,"Republicans":28455,"isal":28456,"windows":28457,"ĠSantos":28458,"Ġvegetation":28459,"338":28460,"tri":28461,"Ġflux":28462,"insert":28463,"Ġclarified":28464,"Ġmortg":28465,"ĠChim":28466,"ĠTort":28467,"Ġdisclaim":28468,"metal":28469,"ĠAside":28470,"Ġinduction":28471,"Ġinfl":28472,"Ġatheists":28473,"amph":28474,"Ġether":28475,"ĠVital":28476,"ĠBuilt":28477,"Mind":28478,"Ġweaponry":28479,"SET":28480,"Ġ186":28481,"admin":28482,"gam":28483,"contract":28484,"afa":28485,"Ġderivatives":28486,"Ġsnacks":28487,"Ġchurn":28488,"Econom":28489,"Ġcapped":28490,"ĠUnderstanding":28491,"ĠHers":28492,"ĠIz":28493,"Ġduct":28494,"IENT":28495,"aughty":28496,"ĠâľĶ":28497,"ĠNP":28498,"Ġsailing":28499,"Initialized":28500,"Ġted":28501,"Ġreactors":28502,"ĠLomb":28503,"Ġchoke":28504,"ĠWorm":28505,"Ġadmiration":28506,"Ġswung":28507,"ensibly":28508,"Ġrash":28509,"ĠGoals":28510,"ĠImportant":28511,"Shot":28512,"ĠRas":28513,"Ġtrainers":28514,"ĠBun":28515,"Working":28516,"Ġharmed":28517,"ĠPandora":28518,"ĠLTE":28519,"Ġmushroom":28520,"ĠCHAR":28521,"ĠFee":28522,"ĠMoy":28523,"Born":28524,"oliberal":28525,"ĠMartial":28526,"Ġgentlemen":28527,"Ġlingering":28528,"Official":28529,"Ġgraffiti":28530,"ĠNames":28531,"Der":28532,"Ġquint":28533,"istrate":28534,"azeera":28535,"ĠNOTICE":28536,"ĠFlorence":28537,"Ġpayable":28538,"Ġdepicts":28539,"ĠSpecies":28540,"Heart":28541,"âĶĢâĶĢâĶĢâĶĢâĶĢâĶĢâĶĢâĶĢ":28542,"Ġenclosed":28543,"Increases":28544,"Daily":28545,"ĠLis":28546,"Ġenactment":28547,"ĠBacon":28548,"ĠSteele":28549,"demand":28550,"Ġ183":28551,"Ġmouths":28552,"Ġstranded":28553,"Ġenhancement":28554,"011":28555,"ĠWhats":28556,"Ġhealed":28557,"eny":28558,"ĠRab":28559,"Ġ340":28560,"ĠLabyrinth":28561,"roach":28562,"ĠYosh":28563,"ĠClippers":28564,"Ġconcerts":28565,"Internet":28566,"355":28567,"Ġstickers":28568,"Ġtermed":28569,"ĠAxe":28570,"Ġgrandparents":28571,"France":28572,"ĠClim":28573,"ĠUh":28574,"ulic":28575,"Ġthrill":28576,"centric":28577,"ĠOverview":28578,"ĠConduct":28579,"Ġsubstantive":28580,"Ġ182":28581,"mur":28582,"Ġstray":28583,"ĠCoff":28584,"Ġrepetitive":28585,"ĠForgotten":28586,"Ġqualification":28587,"ewitness":28588,"ĠZimbabwe":28589,"Ġsimulated":28590,"ĠJD":28591,"253":28592,"ĠWare":28593,"Ġunsc":28594,"Times":28595,"Ġsummons":28596,"Ġdisconnected":28597,"Ġ184":28598,"cius":28599,"ĠGujar":28600,"odka":28601,"Ġerase":28602,"ĠTobacco":28603,"elected":28604,"Ġuncont":28605,"ĠShepard":28606,"ĠLamp":28607,"Ġalerted":28608,"Ġoperative":28609,"arna":28610,"uint":28611,"Ġnegligence":28612,"acements":28613,"Ġsupra":28614,"Ġprevail":28615,"ĠShark":28616,"Ġbelts":28617,"ãģ«":28618,"Ġtighter":28619,"Engineers":28620,"Ġinactive":28621,"Ġexponent":28622,"ĠWillie":28623,"aples":28624,"Ġheir":28625,"ĠHits":28626,"iann":28627,"ĠSays":28628,"Ġcurrents":28629,"ĠBengal":28630,"Ġarist":28631,"Buffer":28632,"Ġbreeze":28633,"ĠWesley":28634,"Cola":28635,"Ġpronoun":28636,"Ġdeed":28637,"ĠKling":28638,"Ġoft":28639,"Ġinflict":28640,"Ġpunishing":28641,"Ġnm":28642,"iku":28643,"ODUCT":28644,"014":28645,"Ġsubsidy":28646,"ĠDEA":28647,"ĠHerbert":28648,"ĠJal":28649,"Bank":28650,"Ġdeferred":28651,"Ġshipment":28652,"Bott":28653,"Ġalle":28654,"bearing":28655,"HTML":28656,"Offline":28657,"Ġ213":28658,"Ġscrolling":28659,"Ġscanned":28660,"ĠLibyan":28661,"ĠTOP":28662,"chrom":28663,"dt":28664,"column":28665,"PsyNetMessage":28666,"Zero":28667,"Ġtorso":28668,"050":28669,"âķIJ":28670,"Ġimperson":28671,"ĠSchwartz":28672,"udic":28673,"Ġpissed":28674,"ĠSapp":28675,"257":28676,"ĠISPs":28677,"ogl":28678,"Ġsupervised":28679,"Ġadolescent":28680,"Ġattained":28681,"ĠDelivery":28682,"ĠBunny":28683,"Ġ1937":28684,"Ġminiature":28685,"Ġos":28686,"Ġ370":28687,"608":28688,"ĠMourinho":28689,"Ġinnate":28690,"Ġtempo":28691,"ĠNM":28692,"ĠFallen":28693,"009":28694,"Ġprovocative":28695,"Streamer":28696,"ĠBenedict":28697,"ĠBolshe":28698,"Ġturtle":28699,"ĠPCB":28700,"ĠEqual":28701,"Director":28702,"ĠRend":28703,"Ġfluids":28704,"Authorities":28705,"Ġcousins":28706,"requency":28707,"ĠNeighbor":28708,"sets":28709,"shared":28710,"Charles":28711,"password":28712,"Ġgears":28713,"Ġ211":28714,"ĠHardware":28715,"rika":28716,"Ġupstream":28717,"Hom":28718,"Ġdisproportionately":28719,"ivities":28720,"Ġundefined":28721,"Ġelectrons":28722,"Ġcommemor":28723,"Eventually":28724,"Ġ><":28725,"Ġirresponsible":28726,"218":28727,"ĠReleased":28728,"ĠOVER":28729,"ĠIGN":28730,"ĠBread":28731,"stellar":28732,"ĠSage":28733,"tted":28734,"damage":28735,"edition":28736,"ĠPrec":28737,"Ġlime":28738,"Ġconfinement":28739,"Ġcalorie":28740,"weapon":28741,"Ġdiffering":28742,"ĠSina":28743,"mys":28744,"amd":28745,"Ġintricate":28746,"kk":28747,"ĠPAT":28748,"ão":28749,"stones":28750,"links":28751,"Ġranch":28752,"Semitic":28753,"Ġdifferentiate":28754,"ĠSinger":28755,"occupied":28756,"Ġfortress":28757,"cmd":28758,"Ġinterception":28759,"ĠAnkara":28760,"Ġrept":28761,"ĠSolitaire":28762,"Ġremake":28763,"pred":28764,"Ġdared":28765,"autions":28766,"ĠBACK":28767,"Running":28768,"Ġdebugging":28769,"Ġgraphs":28770,"399":28771,"ĠNigel":28772,"Ġbun":28773,"Ġpillow":28774,"Ġprogressed":28775,"fashioned":28776,"Ġobedience":28777,"ERN":28778,"Ġrehears":28779,"Cell":28780,"tl":28781,"Sher":28782,"Ġherald":28783,"ĠPayment":28784,"ĠCory":28785,"ĠDept":28786,"Ġrepent":28787,"ĠWeak":28788,"uckland":28789,"Ġpleasing":28790,"Ġshortages":28791,"Ġjurors":28792,"ĠKab":28793,"qqa":28794,"Anti":28795,"Ġwow":28796,"ĠRCMP":28797,"Ġtsun":28798,"ĠSic":28799,"Ġcomprises":28800,"Ġspies":28801,"Ġprecinct":28802,"nu":28803,"Ġurges":28804,"Ġtimed":28805,"Ġstripes":28806,"ĠBoots":28807,"Ġyen":28808,"Advanced":28809,"Ġdiscrete":28810,"ĠArchangel":28811,"employment":28812,"Diff":28813,"Ġmonuments":28814,"Ġ209":28815,"worker":28816,"Ġ196":28817,"ĠIg":28818,"utterstock":28819,"TPS":28820,"Jac":28821,"Ġhomelessness":28822,"Ġcommentator":28823,"Ġracially":28824,"fing":28825,"seed":28826,"Ele":28827,"ellation":28828,"Ġethanol":28829,"Ġparish":28830,"ĠDong":28831,"ĠAwakening":28832,"Ġdeviation":28833,"ĠBearing":28834,"ĠTsuk":28835,"Ġrecess":28836,"Ġlymph":28837,"ĠCannabis":28838,"åľ":28839,"ĠNEWS":28840,"Ġdra":28841,"ĠStefan":28842,"ĠWrong":28843,"ĠSAM":28844,"Ġloosely":28845,"Ġinterpreter":28846,"ĠPlain":28847,"Government":28848,"Ġbigotry":28849,"Ġgrenades":28850,"avez":28851,"pictured":28852,"Ġmandated":28853,"ĠMonk":28854,"ĠPedro":28855,"Ġlava":28856,"274":28857,"Ġcynical":28858,"ĠScrolls":28859,"locks":28860,"Mp":28861,"Ġcongregation":28862,"ornings":28863,"phil":28864,"ĠIbid":28865,"Ġferv":28866,"Ġdisappearing":28867,"Ġarrogant":28868,"syn":28869,"ĠMaver":28870,"ĠSuit":28871,"241":28872,"Ġabbre":28873,"ackers":28874,"Pa":28875,"ĠYel":28876,"Whenever":28877,"Ġ235":28878,"ĠVine":28879,"ĠAnat":28880,"Ġextinct":28881,"LET":28882,"Ġexecutable":28883,"VERS":28884,"oxide":28885,"DNA":28886,"ĠPrel":28887,"Ġresentment":28888,"Ġcomprise":28889,"ĠAviv":28890,"Ġinterceptions":28891,"Ġprolific":28892,"INA":28893,"ĠErin":28894,"thought":28895,"219":28896,"ĠPsychiatry":28897,"unky":28898,"chemist":28899,"Ho":28900,"ĠMcCoy":28901,"Ġbricks":28902,"Los":28903,"rily":28904,"ĠUSSR":28905,"Ġrud":28906,"Ġlaud":28907,"ĠWise":28908,"ĠEmerald":28909,"Ġrevived":28910,"Ġdamned":28911,"ĠRepair":28912,"idem":28913,"ctica":28914,"Ġpatriarch":28915,"ĠNurs":28916,"meg":28917,"Ġcheapest":28918,"reements":28919,"empty":28920,"ĠCelebr":28921,"Ġdeprivation":28922,"chanted":28923,"ĠThumbnails":28924,"Energy":28925,"ĠEthan":28926,"ĠQing":28927,"Ġopposes":28928,"WIND":28929,"vik":28930,"ĠMau":28931,"ĠSUB":28932,"667":28933,"GRE":28934,"ĠVolunte":28935,"nton":28936,"Cook":28937,"åIJ":28938,"esque":28939,"Ġplummet":28940,"Ġsuing":28941,"Ġpronounce":28942,"Ġresisting":28943,"ĠFishing":28944,"ĠTrials":28945,"Ġyell":28946,"Ġ310":28947,"Ġinduct":28948,"Ġpersonalized":28949,"often":28950,"Reb":28951,"EMBER":28952,"Ġviewpoint":28953,"Ġexistential":28954,"())":28955,"remove":28956,"MENTS":28957,"lasses":28958,"Ġevapor":28959,"Ġaisle":28960,"meta":28961,"Ġreflective":28962,"Ġentitlement":28963,"Ġdevised":28964,"music":28965,"ascade":28966,"Ġwinding":28967,"offset":28968,"Ġaccessibility":28969,"kered":28970,"Better":28971,"ĠJohnston":28972,"thinking":28973,"Snow":28974,"ĠCroatia":28975,"ĠAtomic":28976,"271":28977,"348":28978,"Ġtextbook":28979,"ĠSixth":28980,"ĠاÙĦ":28981,"Ġslider":28982,"ĠBurger":28983,"bol":28984,"Sync":28985,"Ġgrandchildren":28986,"Ġcerv":28987,"+)":28988,"Ġeternity":28989,"Ġtweeting":28990,"Ġspeculative":28991,"Ġpivotal":28992,"ĠWP":28993,"ĠTER":28994,"ynamic":28995,"Ġupl":28996,"ĠCats":28997,"perhaps":28998,"Ġclassmates":28999,"Ġblatant":29000,"'-":29001,"Ġlakh":29002,"antine":29003,"ĠBorg":29004,"iom":29005,"/(":29006,"ĠAthletic":29007,"Ġsar":29008,"OTA":29009,"ĠHoffman":29010,"Nevertheless":29011,"Ġadorable":29012,"Ġspawned":29013,"Associated":29014,"ĠDomestic":29015,"Ġimplant":29016,"ĠLuxem":29017,"ĠKens":29018,"Ġpumps":29019,"ĠSAT":29020,"Attributes":29021,"509":29022,"avour":29023,"Ġcentralized":29024,"ĠTN":29025,"Ġfreshly":29026,"ĠAchieve":29027,"Ġoutsiders":29028,"herty":29029,"ĠRee":29030,"ĠTowers":29031,"ĠDart":29032,"akable":29033,"Ġmp":29034,"ĠHeavenly":29035,"Ġripe":29036,"ĠCaroline":29037,"ryan":29038,"Ġclassics":29039,"Ġretiring":29040,"Ġ228":29041,"Ġah":29042,"Ġdealings":29043,"Ġpunching":29044,"ĠChapman":29045,"Options":29046,"maxwell":29047,"volume":29048,"Ġstal":29049,"Ġexported":29050,"ĠQuite":29051,"Ġnumerical":29052,"Burn":29053,"Fact":29054,"ĠKeystone":29055,"Ġtrending":29056,"Ġaltering":29057,"ĠAfricans":29058,"478":29059,"ĠMN":29060,"ĠKnock":29061,"Ġtemptation":29062,"Ġprestige":29063,"Overview":29064,"ĠTraditional":29065,"ĠBahrain":29066,"Private":29067,"ĠHOU":29068,"Ġbarr":29069,"ĠTat":29070,"Cube":29071,"USD":29072,"ĠGrande":29073,"ĠGat":29074,"ĠFlo":29075,"Ġresides":29076,"Ġindec":29077,"volent":29078,"Ġperpetual":29079,"ubes":29080,"Ġworldview":29081,"ĠQuantum":29082,"Ġfiltered":29083,"Ġensu":29084,"orgetown":29085,"ERSON":29086,"ĠMild":29087,"379":29088,"OTT":29089,"Ã¥":29090,"Ġvitamins":29091,"Ġribbon":29092,"Ġsincerely":29093,"ĠHin":29094,"Ġeighteen":29095,"Ġcontradictory":29096,"Ġglaring":29097,"Ġexpectancy":29098,"Ġconspir":29099,"Ġmonstrous":29100,"Ġ380":29101,"reci":29102,"Ġhandic":29103,"Ġpumped":29104,"Ġindicative":29105,"Ġrapp":29106,"Ġavail":29107,"ĠLEGO":29108,"ĠMarijuana":29109,"1985":29110,"erton":29111,"Ġtwentieth":29112,"################################":29113,"ĠSwamp":29114,"Ġvaluation":29115,"Ġaffiliates":29116,"adjusted":29117,"ĠFacility":29118,"262":29119,"Ġenzymes":29120,"itudinal":29121,"Ġimprint":29122,"Site":29123,"Ġinstaller":29124,"ĠTRA":29125,"mology":29126,"linear":29127,"ĠCollective":29128,"igating":29129,"ĠToken":29130,"Ġspeculated":29131,"KN":29132,"ĠCly":29133,"ority":29134,"Ġdefer":29135,"Ġinspectors":29136,"approved":29137,"RM":29138,"ĠSuns":29139,"Ġinforming":29140,"ĠSyracuse":29141,"ibli":29142,"765":29143,"Ġglove":29144,"Ġauthorize":29145,"â̦â̦â̦â̦â̦â̦â̦â̦":29146,"ĠCruise":29147,"Ġcontracting":29148,"shell":29149,"IFE":29150,"ĠJewel":29151,"pract":29152,"ĠPhotoshop":29153,"ĠKnowing":29154,"harm":29155,"Ġattractions":29156,"adan":29157,"etus":29158,"018":29159,"wagen":29160,"Alt":29161,"Ġmultiply":29162,"Ġequilibrium":29163,":{":29164,"ĠFighters":29165,"ĠEdgar":29166,"Ġfourteen":29167,"Govern":29168,"Ġmisuse":29169,"Ġabusing":29170,"Ġancestry":29171,"ramer":29172,"644":29173,"Ġworms":29174,"Ġthicker":29175,"ĠCombine":29176,"Ġpeasants":29177,"Ġvind":29178,"Ġconquest":29179,"Ġmocked":29180,"Ġcinnamon":29181,"ĠCald":29182,"ĠGallup":29183,"Ġavoidance":29184,"Ġincarnation":29185,"ĠStrat":29186,"Ġtasted":29187,"enta":29188,"ĠNeal":29189,"pared":29190,"Ġterminology":29191,"jection":29192,"Scientists":29193,"ĠINS":29194,"ĠDee":29195,"Ġdirectories":29196,"Road":29197,"ĠShap":29198,"bright":29199,"ĠDirectors":29200,"ĠColumn":29201,"Ġbob":29202,"Ġpreferably":29203,"Ġglitch":29204,"furt":29205,"Ġeg":29206,"idis":29207,"CBC":29208,"Ġsurrendered":29209,"Ġtestament":29210,"336":29211,"uggest":29212,"ĠNil":29213,"another":29214,"Ġpathetic":29215,"ĠDonna":29216,"Ġ218":29217,"ĠAvery":29218,"Ġwhiskey":29219,"Ġfixture":29220,"ĠConquest":29221,"Ġbets":29222,"Occ":29223,"ĠLeicester":29224,"].\"":29225,"Ġ));":29226,"Ġflashes":29227,"456":29228,"Ġmasked":29229,"gebra":29230,"Ġcomputed":29231,"chel":29232,"auder":29233,"Ġdefeats":29234,"ĠLiberation":29235,"ĠOsama":29236,"ĠVive":29237,"Changes":29238,"Channel":29239,"Ġtariffs":29240,"Ġmage":29241,"ĠSax":29242,"Ġinadvertently":29243,"ĠCRE":29244,"ĠReaper":29245,"inky":29246,"grading":29247,"Ġstereotyp":29248,"Ġcurl":29249,"ĠFANT":29250,"Ġframeworks":29251,"Mom":29252,"ĠAnch":29253,"Ġflavour":29254,"carbon":29255,"Ġpermitting":29256,"letcher":29257,"ĠMozilla":29258,"ĠParking":29259,"ĠChamp":29260,"Scroll":29261,"Ġmurderer":29262,"Ġrested":29263,"Ġowes":29264,"ĠPoss":29265,"ADD":29266,"IFF":29267,"resolution":29268,"ĠMining":29269,"Ġcomparative":29270,"Dim":29271,"Ġneighbouring":29272,"ĠAST":29273,"ĠToxic":29274,"Ġbiases":29275,"Ġgunfire":29276,"urous":29277,"ĠMoment":29278,"1983":29279,"Ġpervasive":29280,"ttp":29281,"ĠNormally":29282,"rir":29283,"Sarah":29284,"ĠAlbany":29285,"Ġunsett":29286,"ĠSMS":29287,"ipers":29288,"layer":29289,"ĠWhites":29290,"uple":29291,"Ġturbo":29292,"ĠLeeds":29293,"Ġthats":29294,"ĠMiner":29295,"MER":29296,"ĠReign":29297,"Ġperme":29298,"ĠBlitz":29299,"Ġ1934":29300,"Ġintimidating":29301,"tube":29302,"Ġeccentric":29303,"abolic":29304,"boxes":29305,"ĠAssociates":29306,"votes":29307,"Ġsimulate":29308,"umbo":29309,"astery":29310,"Ġshipments":29311,"FFFF":29312,"anth":29313,"Ġseasoned":29314,"Ġexperimentation":29315,"âĸł":29316,"laws":29317,"Meet":29318,"iddles":29319,"antics":29320,"Rating":29321,"ISIS":29322,"hift":29323,"Ġfronts":29324,"buf":29325,"017":29326,"Ġunatt":29327,"ĠDil":29328,"leases":29329,"ĠGardens":29330,"777":29331,"touch":29332,"vell":29333,"458":29334,"Ġ=====":29335,"saving":29336,"Ġerosion":29337,"ĠQuin":29338,"Ġearns":29339,"Ġaccomplishment":29340,"ĠWei":29341,"Ġ<[":29342,"_____":29343,"Ġirrig":29344,"ĠTeddy":29345,"Ġconquered":29346,"ĠArmored":29347,"Ġasserts":29348,"Ġmanipulating":29349,"ré":29350,"Ġtranscripts":29351,"Gallery":29352,"Ġplotting":29353,"Neil":29354,"Ġbetrayal":29355,"loader":29356,"ĠSul":29357,"Ġdisplacement":29358,"Ġroyalty":29359,"ĠWI":29360,"heit":29361,"ĠDevices":29362,"allel":29363,"Ġmunicipalities":29364,"Ġcanal":29365,"Stars":29366,"ĠUAE":29367,"Ġ\"â̦":29368,"ĠCU":29369,"above":29370,"Ġresonance":29371,"ĠguiActiveUn":29372,"added":29373,"ĠBraves":29374,"ĠIbn":29375,"Ġhereby":29376,"ĠBRE":29377,"Ġshareholder":29378,"ĠHir":29379,"ĠJi":29380,"Ġstrangely":29381,"Ġadmired":29382,"Ġplight":29383,"Ġbachelor":29384,"ĠPole":29385,"ciplinary":29386,"Tony":29387,"ĠArmenian":29388,"Ġunman":29389,"ĠZionist":29390,"Stage":29391,"iscover":29392,"Ġautomotive":29393,"Ġsidelines":29394,"Ġslick":29395,"ĠRenaissance":29396,"ĠFUN":29397,"Images":29398,"ĠHaj":29399,"Ġping":29400,"Ġshortcut":29401,"ĠBlvd":29402,"ĠLooks":29403,"Ġbursts":29404,"Ġclamp":29405,"Ġmish":29406,"Ġsorting":29407,"Ġpatriot":29408,"Ġcorrectness":29409,"ĠScandinav":29410,"ĠCavaliers":29411,"python":29412,"azar":29413,"Ġ375":29414,"ĠJaune":29415,"409":29416,"Ġdetrimental":29417,"Ġstabbing":29418,"Ġpoisoned":29419,"Ġfountain":29420,"ocent":29421,"orst":29422,"ĠMari":29423,"Ġrains":29424,"ĠOvers":29425,"ĠInstitution":29426,"udget":29427,"AMY":29428,"tale":29429,"ĠKR":29430,"ĠPrices":29431,"Ġheadaches":29432,"Ġlandsl":29433,"ĠAura":29434,"Bonus":29435,"ĠZhao":29436,"ĠHip":29437,"Ġhops":29438,"ĠKurdistan":29439,"Ġexploiting":29440,"ryn":29441,"Ġhypocrisy":29442,"opening":29443,"Ġgunshot":29444,"Ġwed":29445,"interstitial":29446,"Interstitial":29447,"Ġamen":29448,"Breaking":29449,"Ġmarketed":29450,"Wire":29451,"ĠCrowd":29452,"Continue":29453,"ĠKnown":29454,"ĠEffective":29455,"orean":29456,"izons":29457,"Joseph":29458,"Ġescalation":29459,"username":29460,"Ġcurtain":29461,"ATES":29462,"ĠPAR":29463,"ĠMiy":29464,"Ġcounterfe":29465,"lene":29466,"Ġcontenders":29467,"daily":29468,"ĠAsc":29469,"ĠPhillip":29470,"mostly":29471,"Ġfilename":29472,"hene":29473,"Ġresembling":29474,"Ġstaging":29475,"ĠChloe":29476,"Ġwiring":29477,"Hon":29478,"ĠRenew":29479,"ottage":29480,"ĠHybrid":29481,"much":29482,"Ġstrokes":29483,"Ġpolicymakers":29484,"APTER":29485,"ĠArkham":29486,"plot":29487,"Ġassistants":29488,"Ġdeport":29489,"ĠSega":29490,"Ġinfluenza":29491,"ĠCursed":29492,"ĠKobe":29493,"Ġskinny":29494,"Provider":29495,"ĠRip":29496,"Ġincremental":29497,"products":29498,"BF":29499,"Ġdome":29500,"ĠCredits":29501,"Ġlosers":29502,"ints":29503,"ĠBetty":29504,"ĠTalent":29505,"ĠDAM":29506,"Lv":29507,"Ess":29508,"Ġdens":29509,"temp":29510,"Judge":29511,"odic":29512,"Ġ'(":29513,"URES":29514,"etsk":29515,"VO":29516,"Ġretrieved":29517,"Ġarchitects":29518,"Ùĩ":29519,"Ġethic":29520,"ĠSecondary":29521,"stocks":29522,"adia":29523,"Ġ325":29524,"ĠOpinion":29525,"Ġsimultaneous":29526,"Ġdizz":29527,"ulp":29528,"Ġsmuggling":29529,"ippery":29530,"Random":29531,"facing":29532,"ĠDas":29533,"Ġstockp":29534,"Ġdisclosures":29535,"pointer":29536,"Ġcoral":29537,"ĠSelection":29538,"ĠPike":29539,"ivalent":29540,"Ġruthless":29541,"ĠRim":29542,"Ġensuing":29543,"ĠExperiment":29544,"Ġcongressman":29545,"Ġbeliever":29546,"Ġunspecified":29547,"ĠMord":29548,"Ġknowledgeable":29549,"ĠVERY":29550,"TX":29551,"Ġstraps":29552,"Ġturf":29553,"apeshifter":29554,"Ġmarital":29555,"Ġflock":29556,"ãģĨ":29557,"263":29558,"AMES":29559,"ĠOpposition":29560,"Ġtreasures":29561,"ĠGOD":29562,"Ġmodeled":29563,"ĠWORLD":29564,"Ġ([":29565,"ĠUsage":29566,"HF":29567,"Ġ$(":29568,"ussed":29569,"Ġpioneer":29570,"Eight":29571,"parse":29572,"bread":29573,"ritz":29574,"ĠMiranda":29575,"ĠKant":29576,"++)":29577,"oren":29578,"Ġprovoked":29579,"Ġbreeds":29580,"ĠIncludes":29581,"ĠPastebin":29582,"ĠFlip":29583,"Java":29584,"Ġbrink":29585,"Ġrumored":29586,"Ġunseen":29587,"Ġgarnered":29588,"ĠDefin":29589,"alted":29590,"Ġtattoos":29591,"Ġhesitation":29592,"isitions":29593,"ĠWeaver":29594,"ĠReporting":29595,"Ġtherapies":29596,"Ġconsultants":29597,"Ġresidual":29598,"ĠMali":29599,"ĠRoma":29600,"iago":29601,"ĠResidents":29602,"ubi":29603,"Ġremedies":29604,"Ġadaptive":29605,"ĠAlive":29606,"ĠBarcl":29607,"Ġwallets":29608,"crypt":29609,"etermination":29610,"ĠPelosi":29611,"Ġslipping":29612,"otonin":29613,"Ġalliances":29614,"patrick":29615,"iris":29616,"Ġorth":29617,"ĠPerkins":29618,"ĠDeV":29619,"ĠGets":29620,"Ġdrying":29621,"gee":29622,"forest":29623,"ĠForget":29624,"orem":29625,"339":29626,"Ġvaguely":29627,"ĠDion":29628,"ĠPorn":29629,"ĠHOW":29630,"Ġpneum":29631,"Ġrubble":29632,"ĠTaste":29633,"encia":29634,"ĠGel":29635,"Ġdst":29636,"Ġ245":29637,"ĠMorocco":29638,"inflamm":29639,"ĠTwins":29640,"Ġbots":29641,"daughter":29642,"ĠBalk":29643,"Ġbrethren":29644,"Ġlogos":29645,"Ġgobl":29646,"fps":29647,"Ġsubdivision":29648,"Ġpawn":29649,"Ġsqueezed":29650,"Ġmorale":29651,"ĠDW":29652,"'\"":29653,"Ġknot":29654,"ooky":29655,"Ġdivisive":29656,"Ġboosted":29657,"chy":29658,"ãĥIJ":29659,"ifact":29660,"Ġnewcomers":29661,"ĠWrestling":29662,"Ġscouts":29663,"wolves":29664,"Rat":29665,"Ġnineteenth":29666,"ĠOsborne":29667,"Stats":29668,"Ġempowered":29669,"Ġpsychopath":29670,"ĠOEM":29671,"uggage":29672,"ĠPK":29673,"ĠMohammad":29674,"Pak":29675,"Ġanarchists":29676,"ĠExtract":29677,"esthes":29678,"ĠStockholm":29679,"loo":29680,"ĠGraph":29681,"Ġdeploying":29682,"ĠStranger":29683,"ĠMold":29684,"Ġstaffer":29685,"Ġdiscounted":29686,"uckle":29687,"please":29688,"ĠLanding":29689,"ÃŃa":29690,"Ġ193":29691,"Ġante":29692,"Ġrepetition":29693,"Ġ+/-":29694,"Ġparody":29695,"Ġlively":29696,"AAA":29697,"ĠHorus":29698,"Ġpits":29699,"inders":29700,"LOC":29701,"ĠVenice":29702,"406":29703,"ĠDiscover":29704,"âĨ":29705,"ellectual":29706,"Ġpens":29707,"Ġeyel":29708,"iguous":29709,"Impl":29710,"Ġjoking":29711,"Ġinval":29712,"ĠBelfast":29713,"Ġcreditors":29714,"ĠSkywalker":29715,"ovsky":29716,"Ġceasefire":29717,"Ġseals":29718,"isoft":29719,")).":29720,"ĠFelix":29721,"ITS":29722,"Ġtresp":29723,"ĠBlockchain":29724,"eware":29725,"ĠSchwar":29726,"enne":29727,"mounted":29728,"ĠBeacon":29729,"lesh":29730,"Ġimmensely":29731,"Ġcheering":29732,"Employ":29733,"scene":29734,"ishly":29735,"atchewan":29736,"ĠNicolas":29737,"Ġdrained":29738,"ĠExit":29739,"ĠAzerb":29740,"jun":29741,"Ġfloated":29742,"uania":29743,"Deep":29744,"Ġsuperv":29745,"Ġmystical":29746,"ĠDollar":29747,"ĠApostle":29748,"ĠREL":29749,"ĠProvided":29750,"ĠBucks":29751,"ãĥ´":29752,"cutting":29753,"Ġenhancements":29754,"ĠPenguins":29755,"ĠIsaiah":29756,"Ġjerk":29757,"ĠWyn":29758,"Ġstalled":29759,"Ġcryptocurrencies":29760,"ĠRoland":29761,"single":29762,"Ġlumin":29763,"ĠFellow":29764,"ĠCapacity":29765,"ĠKazakh":29766,"WN":29767,"Ġfinanced":29768,"389":29769,"Ġtid":29770,"Ġcollusion":29771,"ĠMyr":29772,"îĢ":29773,"Senator":29774,"Ġpediatric":29775,"Ġneatly":29776,"Ġsandwiches":29777,"ĠArchitecture":29778,"Ġtucked":29779,"Ġbalcony":29780,"Ġearthquakes":29781,"quire":29782,"Future":29783,"Ġhefty":29784,"éĹ":29785,"Ġspecializes":29786,"Ġstresses":29787,"Ġsender":29788,"Ġmisunderstanding":29789,"Ġepile":29790,"Ġprovoke":29791,"ĠColors":29792,"Ġdismay":29793,"uko":29794,"[_":29795,"586":29796,"neutral":29797,"Ġdonating":29798,"ĠRandall":29799,"Multi":29800,"Ġconveniently":29801,"ĠSung":29802,"ĠCoca":29803,"Ġtents":29804,"ĠAcceler":29805,"Ġpartnered":29806,"272":29807,"irming":29808,"ĠBAS":29809,"sometimes":29810,"Ġobjected":29811,"ubric":29812,"posed":29813,"LCS":29814,"grass":29815,"Ġattributable":29816,"VIS":29817,"Israeli":29818,"Ġrepeats":29819,"ĠRM":29820,"vag":29821,"uta":29822,"inous":29823,"Ġinert":29824,"ĠMiguel":29825,"æŃ":29826,"ĠHawaiian":29827,"Board":29828,"Ġartific":29829,"ĠAzerbai":29830,"asio":29831,"ĠRent":29832,"AIN":29833,"Ġappliances":29834,"Ġnationality":29835,"Ġasshole":29836,"ĠNeb":29837,"Ġnotch":29838,"hani":29839,"ĠBride":29840,"Availability":29841,"Ġintercepted":29842,"Ġcontinental":29843,"Ġswelling":29844,"ĠPerspect":29845,"bies":29846,".<":29847,"ithmetic":29848,"ĠLara":29849,"Ġtempting":29850,"addr":29851,"Ġoverseeing":29852,"clad":29853,"ĠDV":29854,"ĠGingrich":29855,"Ġmun":29856,"ĠAppropri":29857,"Ġalterations":29858,"ĠPatreon":29859,"Ġhavoc":29860,"Ġdisciplines":29861,"Ġnotoriously":29862,"akuya":29863,"ieri":29864,"?).":29865,"ĠWent":29866,"Ġsilicon":29867,"Ġtremb":29868,"Container":29869,"Known":29870,"Ġmortar":29871,"este":29872,"icka":29873,"Arthur":29874,"ĠPreviously":29875,"ĠMarty":29876,"Ġsparse":29877,"gins":29878,"Ġinward":29879,"ĠParticipant":29880,"Copy":29881,"ĠMisc":29882,"Ġantibiotic":29883,"ĠRetro":29884,"Ġelusive":29885,"Ġassail":29886,"ĠBattalion":29887,"ĠBought":29888,"Ġdiminish":29889,"ĠEuropa":29890,"session":29891,"ĠDangerous":29892,"iesel":29893,"Ġdisbelief":29894,"Ġblasts":29895,"extreme":29896,"ĠBoyd":29897,"ĠProjects":29898,"ĠGuys":29899,"Ġundergone":29900,"Ġgrill":29901,"ĠDwight":29902,"Ġ197":29903,"USER":29904,"Ġfilesystem":29905,"Ġclocks":29906,"Taylor":29907,"Ġwrapper":29908,"Ġfolding":29909,"ousand":29910,"ĠPhilippine":29911,"ATIONAL":29912,"ĠPerth":29913,"Ġashes":29914,"Ġaccumulate":29915,"ĠGateway":29916,"Shop":29917,"orkshire":29918,"Han":29919,"ĠBarrel":29920,"ĠLeh":29921,"ĠXV":29922,"Ġwhim":29923,"Ġrepo":29924,"ĠCG":29925,"ĠMam":29926,"Ġincorporating":29927,"Ġbailout":29928,"Ġlinguistic":29929,"Ġdisinteg":29930,"CLE":29931,"Ġcinematic":29932,"ĠFiber":29933,"Syn":29934,"ilion":29935,"ĠCompos":29936,"chens":29937,"Ġneoc":29938,"Ġboiled":29939,"FINE":29940,"ono":29941,"uncle":29942,"iken":29943,"ĠBM":29944,"ι":29945,"Ġreceipts":29946,"Ġdisposed":29947,"ĠThirty":29948,"ĠRough":29949,"ĠABS":29950,"Ġnotwithstanding":29951,"ollen":29952,"#$":29953,"Ġunreliable":29954,"Ġbloom":29955,"Ġmediocre":29956,"Ġtram":29957,"ĠTasman":29958,"Ġshakes":29959,"Ġmanifesto":29960,"ĠMW":29961,"Ġsatisfactory":29962,"Ġshores":29963,"Ġcomputation":29964,"Ġassertions":29965,"ormons":29966,"arag":29967,"abit":29968,"Democrats":29969,"ĠLoot":29970,"ĠVolks":29971,"haired":29972,"Ġgravitational":29973,"Sing":29974,"ĠMiz":29975,"Ġthrottle":29976,"Ġtyranny":29977,"ĠViews":29978,"Ġrobber":29979,"ĠMinority":29980,"Ġshrine":29981,"scope":29982,"purpose":29983,"Ġnucleus":29984,"ourcing":29985,"ĠUSDA":29986,"ĠDHS":29987,"wra":29988,"ĠBowie":29989,"Scale":29990,"ĠBEL":29991,"xi":29992,"Iter":29993,"Ġ(),":29994,"wright":29995,"Ġsailors":29996,"oused":29997,"NASA":29998,"ĠProof":29999,"ĠMineral":30000,"token":30001,"ĠFD":30002,"Rew":30003,"Ġell":30004,"630":30005,"Ġchancellor":30006,"ĠGos":30007,"Ġamounted":30008,"ĠRecre":30009,"omez":30010,"ĠOptim":30011,"ĠOlive":30012,"Ġtracker":30013,"owler":30014,"ĠUnique":30015,"Root":30016,"Ġmaritime":30017,"ĠQuran":30018,"ĠAdapt":30019,"Ġecosystems":30020,"ĠRepeat":30021,"ĠSoy":30022,"ĠIMP":30023,"Ġgraduating":30024,"andem":30025,"Pur":30026,"ĠReset":30027,"ĠTrick":30028,"ĠPhilly":30029,"ĠTue":30030,"ĠMalaysian":30031,"Ġclimax":30032,"Ġbury":30033,"Ġconspic":30034,"ĠSouthampton":30035,"ĠFlowers":30036,"Ġescorted":30037,"ĠEducational":30038,"ĠIRC":30039,"Ġbrutally":30040,"eating":30041,"Ġpillar":30042,"ĠSang":30043,"ĠJude":30044,"arling":30045,"ĠAmnesty":30046,"Ġreminding":30047,"ĠAdministrative":30048,"hesda":30049,"Ġflashed":30050,"ĠPBS":30051,"perate":30052,"feature":30053,"Ġswipe":30054,"Ġgraves":30055,"oultry":30056,"261":30057,"breaks":30058,"ĠGuer":30059,"Ġshrimp":30060,"ĠVoting":30061,"quist":30062,"Ġanalytical":30063,"Ġtablespoons":30064,"ĠSOU":30065,"Ġresearched":30066,"Ġdisrupted":30067,"Ġjour":30068,"Ġreplica":30069,"Ġcartoons":30070,"bians":30071,"})":30072,"copy":30073,"Got":30074,"ouched":30075,"PUT":30076,"Ġswarm":30077,"notations":30078,"said":30079,"Ġrebuilt":30080,"Ġcollaborate":30081,"Ġraging":30082,"Ġnar":30083,"Ġdemographics":30084,"ĠDDR":30085,"Ġdistrust":30086,"ossier":30087,"ĠKro":30088,"Ġpumpkin":30089,"Ġregrets":30090,"Ġfatalities":30091,"ĠLens":30092,"ĠOle":30093,"pd":30094,"Ġpuppet":30095,"ĠOutlook":30096,"ĠStam":30097,"Ol":30098,"Fair":30099,"UU":30100,"Ġrewritten":30101,"ı":30102,"Ġfascinated":30103,"Ġvectors":30104,"Ġtribunal":30105,"uay":30106,"ĠMats":30107,"ĠCoins":30108,"[[":30109,"Ġ181":30110,"Ġrenders":30111,"ĠKaepernick":30112,"Ġespionage":30113,"Ġsumm":30114,"Ġditch":30115,"Account":30116,"Ġspreadsheet":30117,"Ġmutant":30118,"past":30119,"407":30120,"Ġdye":30121,"Ġinitiation":30122,"Ġ4000":30123,"Ġpunishable":30124,"Ġthinner":30125,"ĠKhal":30126,"Ġintermedi":30127,"Dun":30128,"ĠGotham":30129,"Ġeagerly":30130,"Ġvaginal":30131,"powers":30132,"VW":30133,"ĠWATCHED":30134,"Ġpredator":30135,"amsung":30136,"Ġdisparity":30137,"Ġ[*":30138,"Ġamph":30139,"Ġoutskirts":30140,"ĠSpirits":30141,"Ġskeletal":30142,"л":30143,"ĠRear":30144,"Ġissuance":30145,"ĠLogic":30146,"released":30147,"ZZ":30148,"ĠBound":30149,"Entry":30150,"Ġexits":30151,"isol":30152,"ĠFounder":30153,"Ġwre":30154,"ĠGreenland":30155,"ĠMMO":30156,"taker":30157,"INC":30158,"ãģ¾":30159,"Ġhourly":30160,"henko":30161,"Ġfantasies":30162,"Ġdisob":30163,"Ġdemolition":30164,"ãĥĭ":30165,"Ġenlisted":30166,"ratulations":30167,"Ġmisguided":30168,"Ġensured":30169,"Ġdiscouraged":30170,"mort":30171,"Ġflank":30172,"Ġcess":30173,"Ġreacts":30174,"ĠSere":30175,"sensitive":30176,"ĠSerpent":30177,"assad":30178,"Ġ247":30179,"Ġcalmly":30180,"busters":30181,"Ġbleed":30182,"ĠStro":30183,"Ġamusement":30184,"ĠAntarctica":30185,"Ġscept":30186,"ĠGaw":30187,"aq":30188,"asonic":30189,"Ġsprawling":30190,"native":30191,"aturated":30192,"ĠBattlefield":30193,"IVERS":30194,"EB":30195,"ĠGems":30196,"ĠNorthwestern":30197,"ĠFilms":30198,"ĠAutomatic":30199,"Ġapprehend":30200,"ãģ¨":30201,"ĠguiName":30202,"Ġbackend":30203,"Ġevidenced":30204,"geant":30205,"012":30206,"ĠSiege":30207,"ĠexternalTo":30208,"ĠunfocusedRange":30209,"ĠguiActiveUnfocused":30210,"ĠguiIcon":30211,"ĠexternalToEVA":30212,"ĠexternalToEVAOnly":30213,"Fri":30214,"chard":30215,"enaries":30216,"Ġchiefs":30217,"Ġcf":30218,"ĠHUD":30219,"Ġcorrobor":30220,"ĠdB":30221,"ĠTaken":30222,"ĠPatricia":30223,"rail":30224,"ĠCharm":30225,"ĠLibertarian":30226,"rieve":30227,"Personal":30228,"ĠOUR":30229,"geries":30230,"Ġdumping":30231,"Ġneurological":30232,"itimate":30233,"ĠClintons":30234,"rafted":30235,"ĠMolly":30236,"Ġterminals":30237,"register":30238,"Ġflare":30239,"Ġencoded":30240,"Ġautopsy":30241,"pel":30242,"machine":30243,"Ġexemptions":30244,"ĠRoyals":30245,"distance":30246,"Ġdrafts":30247,"Ġlame":30248,"ĠCunning":30249,"Ġspouses":30250,"ĠMarkets":30251,"ĠCarrier":30252,"Ġimplying":30253,"ĠYak":30254,"sid":30255,"Ġloser":30256,"Ġvigilant":30257,"Ġimpeachment":30258,"Ġaugmented":30259,"ĠEmployees":30260,"Ġunintended":30261,"ternally":30262,"ĠWatt":30263,"Ġrecognizable":30264,"essim":30265,"æĿ":30266,"Ġcoated":30267,"rha":30268,"Ġlieutenant":30269,"ĠLegislation":30270,"published":30271,"444":30272,"013":30273,"Ġideally":30274,"ĠPassword":30275,"Ġsimplify":30276,"ĠMeta":30277,"ĠMRI":30278,"Ġpleading":30279,"organized":30280,"handler":30281,"Ġunravel":30282,"correct":30283,"Ġicy":30284,"Ġparanoid":30285,"Ġpasser":30286,"Ġinspections":30287,"ofer":30288,"ĠHealthcare":30289,"283":30290,"ĠBrut":30291,"iola":30292,"forge":30293,"ĠMedieval":30294,"MSN":30295,"ievers":30296,"ĠProgramming":30297,"åī":30298,"Ġ223":30299,"mu":30300,"ĠCLE":30301,"uga":30302,"Ġshoppers":30303,"Ġinformative":30304,"ĠPlans":30305,"Ġsupplementation":30306,"ĠTests":30307,"tyard":30308,"ocytes":30309,"ĠVega":30310,"ĠGujarat":30311,"ermanent":30312,"Except":30313,"ĠLOT":30314,"alla":30315,"ĠCumm":30316,"ĠOsw":30317,"Ġvenom":30318,"ĠDebt":30319,"ĠDOWN":30320,"Ġreunion":30321,"Ġmuc":30322,"ĠRelief":30323,"Ġgeop":30324,"ĠðŁĺ":30325,"alogue":30326,"Anth":30327,"echo":30328,"Ġcorros":30329,"Ġreplication":30330,"ĠBlazing":30331,"ĠDaughter":30332,"Ġinflic":30333,"ĠLindsey":30334,"ÙĪ":30335,"284":30336,"Exit":30337,"Ġgloom":30338,"TAIN":30339,"Ġundermining":30340,"Ġadvising":30341,"hidden":30342,"Ġoverflow":30343,"Ġgor":30344,"urdue":30345,"Ġechoes":30346,"enhagen":30347,"Ġimpuls":30348,"drug":30349,"cash":30350,"Ġasync":30351,"Ġmirac":30352,"atts":30353,"punk":30354,"Ġpivot":30355,"ĠLegislative":30356,"Ġbloggers":30357,"ĠClaw":30358,"sburg":30359,"dyl":30360,"ĠRecommend":30361,"Ġverte":30362,"Ġprohibiting":30363,"ĠPanther":30364,"Jonathan":30365,"Ġomin":30366,"Ġhateful":30367,"281":30368,"ĠOrche":30369,"ĠMurdoch":30370,"downs":30371,"Ġasymm":30372,"GER":30373,"Always":30374,"Ġinforms":30375,"ĠWM":30376,"ĠPony":30377,"ĠAppendix":30378,"ĠArlington":30379,"Jam":30380,"Ġmedicinal":30381,"ĠSlam":30382,"ITIES":30383,"Ġreaff":30384,"ĠRi":30385,"FG":30386,"Spring":30387,"bool":30388,"Ġthighs":30389,"Ġmarkings":30390,"ĠRaqqa":30391,"ĠLak":30392,"poll":30393,"tsky":30394,"ĠMorty":30395,"ĠDefinition":30396,"Ġdebunk":30397,"endered":30398,"ĠLeone":30399,"avers":30400,"Ġmortgages":30401,"Apparently":30402,"Nic":30403,"haus":30404,"ĠThousands":30405,"auld":30406,"Ġmash":30407,"shoot":30408,"Ġdiarr":30409,"Ġconsciously":30410,"Hero":30411,"eas":30412,"ĠNaturally":30413,"ĠDestroyer":30414,"Ġdashboard":30415,"services":30416,"Rog":30417,"Ġmillennials":30418,"Ġinvade":30419,"-(":30420,"Ġcommissions":30421,"ĠAuckland":30422,"Ġbroadcasts":30423,"Ġfrontal":30424,"Ġcrank":30425,"ĠHistoric":30426,"Ġrumours":30427,"CTV":30428,"Ġsteril":30429,"Ġbooster":30430,"rocket":30431,"ãĤ¼":30432,"utsche":30433,"ĠPI":30434,"Ġ233":30435,"ĠProducer":30436,"ĠAnalytics":30437,"Ġinvaluable":30438,"Ġunintention":30439,"ĠCY":30440,"Ġscrutin":30441,"Ġgigg":30442,"Ġengulf":30443,"Ġproletariat":30444,"Ġhacks":30445,"ĠHew":30446,"arak":30447,"ĠSlime":30448,"ielding":30449,"agher":30450,"ĠElliot":30451,"Ġtelecom":30452,"Ġ219":30453,"ultan":30454,"ĠArbor":30455,"ĠScouts":30456,"Ban":30457,"Ġlifespan":30458,"Ġblasp":30459,"388":30460,"Ġjudiciary":30461,"ĠContinental":30462,"asking":30463,"McC":30464,"LED":30465,"Ġbaggage":30466,"ĠSorcerer":30467,"Ġremnants":30468,"ĠGriffith":30469,"etsu":30470,"ĠSubaru":30471,"ĠPersonality":30472,"designed":30473,"ushima":30474,"agnar":30475,"Ġrecoil":30476,"Ġpassions":30477,"\\\":":30478,"Ġtee":30479,"Ġabolition":30480,"ĠCreating":30481,"jac":30482,"Ġ194":30483,"019":30484,"Ġpillars":30485,"riched":30486,"/\"":30487,"tk":30488,"Ġlivelihood":30489,"Ġroasted":30490,"ahon":30491,"ĠHutch":30492,"assert":30493,"Ġdividend":30494,"Ġknit":30495,"Ġdaunting":30496,"Ġdisturbance":30497,"Ġshale":30498,"Ġcultivated":30499,"Ġrefrigerator":30500,"LB":30501,"ĠNET":30502,"Ġcommercials":30503,"Ġthinkers":30504,"455":30505,"Ġchop":30506,"Broad":30507,"Ġsuspicions":30508,"Ġtagged":30509,"lifting":30510,"Ġstylish":30511,"ĠShields":30512,"Shortly":30513,"Ġtails":30514,"Auth":30515,"STE":30516,"ĠGAME":30517,"Ġseism":30518,"ĠKis":30519,"ologne":30520,"Ġcowork":30521,"Ġforcibly":30522,"Ġthyroid":30523,"ĠPB":30524,"ANE":30525,"married":30526,"horse":30527,"Ġpolymer":30528,"ĠChal":30529,"odor":30530,"DEBUG":30531,"ĠContext":30532,"Ġbliss":30533,"Ġpinpoint":30534,"ĠMathemat":30535,"legram":30536,"ĠWeekend":30537,"Ġlabelled":30538,"Ġbart":30539,"itles":30540,"Ġestrogen":30541,"âĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶâĢĶ":30542,"\"'":30543,"Ġvisibly":30544,"Ġoutsider":30545,"aida":30546,"Area":30547,"Ġdissemin":30548,"Ġdishonest":30549,"ĠClosed":30550,"ĠBulletin":30551,"ĠRamsey":30552,"sword":30553,"ĠXI":30554,"ourced":30555,"Same":30556,"346":30557,"ĠRepe":30558,"ĠKou":30559,"cake":30560,"emis":30561,"Cache":30562,"ĠMeaning":30563,"ĠEnlight":30564,"onomy":30565,"Ġmanifestation":30566,"sworth":30567,"Jay":30568,"Ġchore":30569,"ör":30570,"Dream":30571,"Ġsanctioned":30572,"Ġculturally":30573,"ĠAra":30574,"Nav":30575,"Ġtheological":30576,"Ġstrut":30577,"ĠVO":30578,"ĠHandbook":30579,"Ġconstructing":30580,"Ġ¶":30581,"ĠBenefits":30582,"ĠPsychological":30583,"sac":30584,"å¸":30585,"policy":30586,"ĠMatters":30587,"ĠReported":30588,"ĠByte":30589,"Ġvitro":30590,"ĠMaiden":30591,"Ġlam":30592,"ĠJennings":30593,"Ġgarment":30594,"ĠRutgers":30595,"ĠStafford":30596,"ĠWellington":30597,"Ġintermitt":30598,"Ġnpm":30599,"Ġordeal":30600,"Ġplugged":30601,"ooming":30602,"inished":30603,"framework":30604,"Ġtimber":30605,"Ġcass":30606,"Ġ850":30607,"iless":30608,"ĠRedux":30609,"768":30610,"Stre":30611,"Ġsurpassed":30612,"whel":30613,"Ġparallels":30614,"Ġveil":30615,"ĠGI":30616,"ĠREST":30617,"Ġreadiness":30618,"sort":30619,"Ġmodifying":30620,"ĠSlate":30621,"ruff":30622,"Ġmarble":30623,"Ġinfrared":30624,"Ġauditor":30625,"ĠFANTASY":30626,"ĠPoverty":30627,"ĠSPD":30628,"Ġ\"(":30629,"Ky":30630,"RAY":30631,"Ġexecutions":30632,"ĠBeverly":30633,"ĠMarxism":30634,"ĠBurst":30635,"ĠKali":30636,"estones":30637,"Clearly":30638,"Ell":30639,"ãģ§":30640,"ĠProceedings":30641,"Token":30642,"IFIC":30643,"ña":30644,"Central":30645,"ĠHaley":30646,"ĠDrama":30647,"Ġformations":30648,"ORN":30649,"Books":30650,"Ġdominating":30651,"ĠFlyers":30652,"ĠCompanion":30653,"Ġdisciplined":30654,"ĠYugoslav":30655,"ĠSpells":30656,"Ġvengeance":30657,"Ġlandlords":30658,"Len":30659,"ĠOgre":30660,"anoia":30661,"Ġpiercing":30662,"Ġcongreg":30663,"Ġscorer":30664,"obia":30665,"Ġnickel":30666,"ĠLearns":30667,"Ġrejo":30668,"Ġmasterpiece":30669,"Flash":30670,"Ġinhabited":30671,"ĠOpenGL":30672,"ĠDud":30673,"ĠICO":30674,"Ġarter":30675,"Ġplur":30676,"Ġmastery":30677,"Ġlongstanding":30678,"sted":30679,"Ġwines":30680,"Ġtelevised":30681,"ĠShrine":30682,"ĠBayern":30683,"Ġâĵĺ":30684,"Ġenclosure":30685,"john":30686,"Ġprophets":30687,"ĠResurrection":30688,"ĠOrders":30689,"Ġuneven":30690,"rals":30691,"Ġdwind":30692,"ĠLah":30693,"ĠSloven":30694,"378":30695,"Ġinsistence":30696,"affle":30697,"ĠClone":30698,"Ġhardship":30699,"ĠCongressman":30700,"Ġplead":30701,"Ġreviewers":30702,"Ġcured":30703,"Ġ1935":30704,"asley":30705,"fake":30706,"ĠThinking":30707,"ydia":30708,"PART":30709,"ĠDota":30710,"oit":30711,"Ġwhipped":30712,"Ġbouncing":30713,"ĠHispanics":30714,"comings":30715,"Ġcannabin":30716,"ĠChambers":30717,"ĠZack":30718,"Optional":30719,"Ġcoats":30720,"Ġprowess":30721,"ĠNorton":30722,"Ġplainly":30723,"Ġfreight":30724,"Ġinhibition":30725,"Ġclam":30726,"Ġ303":30727,"kef":30728,"aleigh":30729,"Luke":30730,"Ġpsycho":30731,"atorium":30732,"MED":30733,"Ġtreaties":30734,"Ġindisc":30735,"Ġdc":30736,"OPS":30737,"Ġresilient":30738,"ĠInterstate":30739,"Ġslack":30740,"Ġmundane":30741,"Ġestablishes":30742,"359":30743,"Ġstrained":30744,"Ġnond":30745,"Sus":30746,"Ġcaste":30747,"arate":30748,"ieving":30749,"Ġunfairly":30750,"Ġparser":30751,"onial":30752,"ursive":30753,"Via":30754,"ĠOtto":30755,"ĠAuthorities":30756,"stroke":30757,"KR":30758,"ĠMercy":30759,"Ġfurnished":30760,"Ġoutset":30761,"Ġmetic":30762,"1982":30763,"olithic":30764,"ĠTent":30765,"ogical":30766,"ĠAircraft":30767,"Ġhides":30768,"ĠBecame":30769,"Ġeducators":30770,"reaching":30771,"Ġvolatility":30772,"Ġtoddler":30773,"ĠNASCAR":30774,"ĠTwelve":30775,"ĠHighlights":30776,"Ġgrape":30777,"Ġsplits":30778,"Ġpeasant":30779,"Ġreneg":30780,"ĠMSI":30781,"Temp":30782,"stars":30783,"Ġtrek":30784,"ĠHyde":30785,"binding":30786,"Ġrealism":30787,"Ġoxide":30788,"ĠHos":30789,"Ġmounts":30790,"Ġbiting":30791,"Ġcollapsing":30792,"Ġpostal":30793,"Ġmuseums":30794,"Ġdetached":30795,"Ġrespecting":30796,"Ġmonopol":30797,"Ġworkflow":30798,"ĠCake":30799,"Template":30800,"ĠOrganisation":30801,"Ġpersistence":30802,"369":30803,"Coming":30804,"Brad":30805,"Ġredundant":30806,"ĠGTA":30807,"Ġbending":30808,"Ġrevoked":30809,"Ġoffending":30810,"Ġframing":30811,"Ġprintf":30812,"Commun":30813,"members":30814,"Outside":30815,"Ġconstrued":30816,"Ġcoded":30817,"FORE":30818,"Ġchast":30819,"Chat":30820,"Indian":30821,"ĠYard":30822,"?!\"":30823,"ĠPorts":30824,"ĠXavier":30825,"ĠRET":30826,"'.\"":30827,"ĠBoat":30828,"ivated":30829,"icht":30830,"umerable":30831,"Ds":30832,"ĠDunn":30833,"Ġcoffin":30834,"Ġsecurely":30835,"ĠRaptors":30836,"ĠBes":30837,"Installation":30838,"Ġinception":30839,"ĠHealthy":30840,"endants":30841,"Ġpsychologists":30842,"ĠSheikh":30843,"cultural":30844,"ĠBlackBerry":30845,"shift":30846,"Fred":30847,"oche":30848,"Ġcakes":30849,"ĠSEO":30850,"ĠGian":30851,"ĠAsians":30852,"ogging":30853,"element":30854,"Ġpundits":30855,"ĠVaugh":30856,"ĠGavin":30857,"Ġhitter":30858,"Ġdrowned":30859,"Ġchalk":30860,"ĠZika":30861,"Ġmeasles":30862,"802":30863,"â̦..":30864,"ĠAWS":30865,"]\"":30866,"Ġdistort":30867,"ĠMast":30868,"Ġantibodies":30869,"ĠMash":30870,"Memory":30871,"ĠUganda":30872,"ĠProb":30873,"Ġvomiting":30874,"ĠTurns":30875,"Ġoccupying":30876,"Ġevasion":30877,"ĠTherapy":30878,"Ġpromo":30879,"Ġelectr":30880,"Ġblueprint":30881,"ĠDre":30882,"priced":30883,"ĠDepot":30884,"Ġalleviate":30885,"ĠSomali":30886,"marg":30887,"nine":30888,"Ġnostalgia":30889,"ĠShepherd":30890,"Ġcavalry":30891,"Ġtorped":30892,"ĠBloody":30893,"xb":30894,"Ġsank":30895,"Ġgoalt":30896,"reportprint":30897,"embedreportprint":30898,"cloneembedreportprint":30899,"ĠInitially":30900,"ĠFischer":30901,"Ġnoteworthy":30902,"cern":30903,"Ġinefficient":30904,"rawdownload":30905,"rawdownloadcloneembedreportprint":30906,"cation":30907,"ĠDynasty":30908,"lag":30909,"DES":30910,"Ġdistinctly":30911,"ĠEstonia":30912,"Ġopenness":30913,"Ġgossip":30914,"ruck":30915,"Width":30916,"ĠIbrahim":30917,"Ġpetroleum":30918,"Ġavatar":30919,"ĠHed":30920,"atha":30921,"ĠHogwarts":30922,"Ġcaves":30923,"678":30924,"Ġsafeguard":30925,"ĠMog":30926,"isson":30927,"ĠDurham":30928,"slaught":30929,"ĠGraduate":30930,"Ġsubconscious":30931,"ĠExcellent":30932,"ĠDum":30933,"-----":30934,"Ġpiles":30935,"ĠWORK":30936,"ĠGarn":30937,"ĠFol":30938,"ĠATM":30939,"Ġavoids":30940,"ĠTul":30941,"Ġbleak":30942,"ELY":30943,"ivist":30944,"lightly":30945,"Pers":30946,"ĠDob":30947,"ĠLS":30948,"Ġinsanity":30949,"ε":30950,"atalie":30951,"Enlarge":30952,"Ġtwists":30953,"Ġfaulty":30954,"Ġpiracy":30955,"Ġimpover":30956,"Ġrugged":30957,"ĠFashion":30958,"Ġsands":30959,"'?":30960,"swick":30961,"Ġnatives":30962,"Ġhen":30963,"ĠNoise":30964,"ãĥĹ":30965,"Ġgreens":30966,"Ġfreezer":30967,"Ġdynasty":30968,"ĠFathers":30969,"ĠNewark":30970,"Ġarchaeological":30971,"Ġot":30972,"obar":30973,"Ġblockade":30974,"Ġallerg":30975,"LV":30976,"Ġdebit":30977,"ĠRFC":30978,"ĠMilton":30979,"ĠPressure":30980,"Ġwillingly":30981,"Ġdisproportionate":30982,"Ġoppressive":30983,"Ġdiamonds":30984,"Ġbelongings":30985,"1970":30986,"Ġbells":30987,"Ġimperialism":30988,"Ġ227":30989,"Ġexploding":30990,"ĠEclipse":30991,"Ġ1919":30992,"Ġrant":30993,"Ġnominations":30994,"347":30995,"Ġpeacefully":30996,"rica":30997,"ĠFUCK":30998,"Ġvibration":30999,"malink":31000,"Ġropes":31001,"ĠIvanka":31002,"ĠBrewery":31003,"ĠBooker":31004,"ĠOwens":31005,"goers":31006,"Services":31007,"ĠSnape":31008,"Ġ191":31009,"395":31010,"Ġ299":31011,"justice":31012,"Ġbri":31013,"Ġdiscs":31014,"Ġprominently":31015,"Ġvulgar":31016,"Ġskipping":31017,"lves":31018,"Ġtsunami":31019,"374":31020,"ĠUrug":31021,"ĠEid":31022,"recated":31023,"phen":31024,"Ġfaults":31025,"ĠStarted":31026,"950":31027,"Ġpi":31028,"Ġdetector":31029,"Ġbastard":31030,"Ġvalidated":31031,"SpaceEngineers":31032,"OURCE":31033,"Ġ(~":31034,"Ġunsur":31035,"Ġaffirmed":31036,"Ġfascism":31037,"Ġresolving":31038,"ĠChavez":31039,"ĠCyn":31040,"Ġdetract":31041,"Lost":31042,"Ġrigged":31043,"Ġhomage":31044,"ĠBruno":31045,"555":31046,"eca":31047,"Ġpresses":31048,"Ġhumour":31049,"Ġspacing":31050,"Ġ'/":31051,"olkien":31052,"Coun":31053,"OPER":31054,"Tre":31055,"Son":31056,"ĠCambodia":31057,"ierre":31058,"mong":31059,"ozy":31060,"Ġliquidity":31061,"ĠSoviets":31062,"ĠFernando":31063,"Ġ229":31064,"Ġslug":31065,"ĠCatalan":31066,"electric":31067,"Ġscenery":31068,"ĠHearth":31069,"Ġconstrained":31070,"Ġgoalie":31071,"ĠGuidelines":31072,"ĠAmmo":31073,"ĠPearson":31074,"Ġtaxed":31075,"Ġfetus":31076,"Response":31077,"ĠAlexis":31078,"thia":31079,"Guy":31080,"Ġreconstruct":31081,"Ġextremes":31082,"Ġconcluding":31083,"ĠPeg":31084,"ooks":31085,"Ġdeductions":31086,"Rose":31087,"Ġgroundbreaking":31088,"ĠTarg":31089,"ãĥģ":31090,"ĠReve":31091,"resource":31092,"Ġmoons":31093,"Ġelectromagnetic":31094,"Ġamidst":31095,"ĠViktor":31096,"NESS":31097,"BACK":31098,"Ġcommute":31099,"ĠAnaheim":31100,"Ġfluctuations":31101,"640":31102,"Ġnoodles":31103,"ĠCopenhagen":31104,"ĠTide":31105,"ĠGrizz":31106,"ĠSEE":31107,"Ġpipelines":31108,"Ġscars":31109,"endo":31110,"agus":31111,"ĠETF":31112,"/#":31113,"ĠBecome":31114,"448":31115,"Ġvisc":31116,"ĠRecommended":31117,"Ġjumper":31118,"Ġcognition":31119,"Ġassassin":31120,"Ġwitnessing":31121,"ĠSetup":31122,"Ġlac":31123,"vim":31124,"ISM":31125,"pages":31126,"SSL":31127,"358":31128,"Ġadject":31129,"industrial":31130,"lore":31131,"chery":31132,"Ġglitter":31133,"Ġcalf":31134,"Florida":31135,"Ġspoilers":31136,"Ġsucceeds":31137,"Ġchanting":31138,"Ġslogans":31139,"ĠTracy":31140,"Visit":31141,"rology":31142,"Ġmornings":31143,"Ġlineage":31144,"Ġsip":31145,"Ġintensely":31146,"Ġflourish":31147,"ĠSleeping":31148,"ĠFem":31149,"orpor":31150,"ĠKlan":31151,"ĠDarth":31152,"hack":31153,"ĠNielsen":31154,"Ġtumors":31155,"Ġprocurement":31156,"ĠYorkshire":31157,"Ġraided":31158,"KY":31159,"Anna":31160,"Ġ//[":31161,"ĠDisorder":31162,"ĠMustang":31163,"ĠWen":31164,"ĠTrying":31165,"sq":31166,"Ġdeliveries":31167,"Ġshutter":31168,"Ġcerebral":31169,"Ġbipolar":31170,"ĠCN":31171,"lass":31172,"jet":31173,"Ġdebating":31174,">:":31175,"Ġeagle":31176,"grades":31177,"ĠDixon":31178,"UGC":31179,"MAS":31180,"ĠDraco":31181,"ĠMachines":31182,"affer":31183,"Ġeman":31184,"²":31185,"pron":31186,"ĠGym":31187,"Ġcomparatively":31188,"ĠTribunal":31189,"PRO":31190,"Ġlex":31191,"Ġfertile":31192,"Ġdepressing":31193,"Ġsuperficial":31194,"essential":31195,"ĠHunters":31196,"gp":31197,"Ġprominence":31198,"Liber":31199,"ĠAncest":31200,"otechnology":31201,"Ġmocking":31202,"ĠTraff":31203,"ĸļ":31204,"Medium":31205,"Iraq":31206,"Ġpsychiatrist":31207,"Quantity":31208,"ĠLect":31209,"Ġnoisy":31210,"520":31211,"GY":31212,"Ġslapped":31213,"ĠMTV":31214,"Ġpara":31215,"pull":31216,"Multiple":31217,"asher":31218,"Ġnour":31219,"ĠSeg":31220,"Spell":31221,"vous":31222,"ordial":31223,"Senior":31224,"ĠGoldberg":31225,"ĠPlasma":31226,"need":31227,"Ġmessenger":31228,"eret":31229,"Ġteamed":31230,"Ġliteracy":31231,"ĠLeah":31232,"ĠDoyle":31233,"Ġemitted":31234,"UX":31235,"Ġevade":31236,"Ġmaze":31237,"Ġwrongly":31238,"ĠLars":31239,"Ġstereotype":31240,"Ġpledges":31241,"Ġaroma":31242,"ĠMET":31243,"Ġacre":31244,"ĠOD":31245,"Ġff":31246,"Ġbreweries":31247,"ĠHilton":31248,"undle":31249,"ĠKak":31250,"ĠThankfully":31251,"ĠCanucks":31252,"inctions":31253,"ĠAppears":31254,"Ġcoer":31255,"Ġundermined":31256,"rovers":31257,"Andre":31258,"Ġblaze":31259,"umers":31260,"Ġfamine":31261,"amphetamine":31262,"ulkan":31263,"Amount":31264,"Ġdesperation":31265,"wikipedia":31266,"development":31267,"ĠCorinth":31268,"ussia":31269,"Jackson":31270,"LI":31271,"Native":31272,"Rs":31273,"Ohio":31274,"ĠKathleen":31275,"Fortunately":31276,"Ġattendant":31277,"ĠPreferred":31278,"ĠDidn":31279,"ĠVs":31280,"Mis":31281,"Ġrespondent":31282,"Ġboun":31283,"stable":31284,"Ġpaved":31285,"Ġunexpl":31286,"ĠCheney":31287,"LM":31288,"ĠCull":31289,"blown":31290,"Ġconfronting":31291,"ocese":31292,"serving":31293,"Wi":31294,"ĠLithuania":31295,"anni":31296,"Ġstalk":31297,"hd":31298,"Ġvener":31299,"APH":31300,"ynchronous":31301,"URR":31302,"umably":31303,"historic":31304,"Half":31305,"Hay":31306,"Ġresilience":31307,"spection":31308,"Ġabandoning":31309,"Obs":31310,"ĠDebbie":31311,"Ġgradient":31312,"ĠPlaint":31313,"ĠCanal":31314,"ARCH":31315,"Ġexpansive":31316,"Ġfung":31317,"Ġbounced":31318,"Und":31319,"Ġprecautions":31320,"Ġclarification":31321,"Ġdagger":31322,"Ġgrips":31323,"Ġµ":31324,"ĠRivera":31325,"ĠUndead":31326,"isites":31327,"ĠFIRST":31328,"ño":31329,"audi":31330,"Ġhostages":31331,"Ġcompliant":31332,"Ġalumni":31333,"Seven":31334,"Ġcybersecurity":31335,"either":31336,"Collect":31337,"Ġinvariably":31338,"ĠSoci":31339,"Ġlawmaker":31340,"Ġale":31341,"ĠPersonally":31342,"Nazi":31343,"Ġcustomization":31344,"ĠProc":31345,"ĠSaskatchewan":31346,"eaturing":31347,"Ġspared":31348,"Ġdiscontinued":31349,"Ġcomputational":31350,"ĠMotorola":31351,"Ġsupremacist":31352,"governmental":31353,"Ġparadise":31354,"ĠDowning":31355,"ĠNikon":31356,"Ġcatalyst":31357,"berra":31358,"Toronto":31359,"875":31360,"beta":31361,"ĠMacron":31362,"Ġunrealistic":31363,"vector":31364,"ĠVehicles":31365,"itiveness":31366,"ĠRV":31367,"ĠColbert":31368,"sin":31369,"oji":31370,"entin":31371,"ĠKrish":31372,"hello":31373,"ffield":31374,"oky":31375,"ĠTate":31376,"Ġmaple":31377,"Ġaids":31378,"chemical":31379,"334":31380,"nuts":31381,"ĠWarp":31382,"Ġxx":31383,"ĠRobb":31384,"umerous":31385,"_-_":31386,"ftime":31387,"ĠVW":31388,"Ġwinger":31389,"ĠDome":31390,"tools":31391,"ĠPV":31392,"ĠGeorgetown":31393,"Ġgeared":31394,"Ġjihadists":31395,"Ġcp":31396,"Ġsteroids":31397,"Mother":31398,"clerosis":31399,"ĠDRM":31400,"nesia":31401,"Ġlinger":31402,"Ġimmersive":31403,"ĠCOUN":31404,"Ġoutweigh":31405,"ensual":31406,"Band":31407,"Ġtransforms":31408,"matched":31409,"psons":31410,"ĠJudicial":31411,"factor":31412,"Ġreferral":31413,"Ġoddly":31414,"ĠWenger":31415,"Bring":31416,"ĠBows":31417,"602":31418,"ICLE":31419,"Ġlions":31420,"ĠAcademic":31421,"ĠThorn":31422,"ĠRaider":31423,"kefeller":31424,"Storage":31425,"Lower":31426,"ĠOrt":31427,"ĠEquality":31428,"ALT":31429,"ĠSOC":31430,"Types":31431,"Ġlyn":31432,"ĠAsset":31433,"coat":31434,"TPP":31435,"CVE":31436,"ĠPioneer":31437,"application":31438,"Modern":31439,"ĠHK":31440,"Environment":31441,"Alright":31442,"Rain":31443,"IPP":31444,"ĠShiite":31445,"Ġmound":31446,"ĠAbilities":31447,"condition":31448,"Staff":31449,"Ġcompetence":31450,"ĠMoor":31451,"ĠDiablo":31452,"Ġwithheld":31453,"Ġostensibly":31454,"ĠBrom":31455,"Ġmsg":31456,"Ġdenomin":31457,"ĠReferences":31458,"ĠFP":31459,"Ġplunged":31460,"Ġpamph":31461,"moving":31462,"central":31463,"Ġdownright":31464,"Ġfading":31465,"Tal":31466,"Typ":31467,"ĠThy":31468,"ukes":31469,"ithe":31470,"Ġove":31471,"Ġbattled":31472,"Ġseafood":31473,"Ġfigur":31474,"ĠRD":31475,"crop":31476,"Ġsquads":31477,"{\\":31478,"à¹":31479,"ĠEh":31480,"Ġinterviewing":31481,"ĠQin":31482,"Ġaspiring":31483,"PLIC":31484,"Ġclauses":31485,"ĠGast":31486,"ĠNir":31487,"Ġluggage":31488,"Ġhose":31489,"Ġsystemd":31490,"Ġdescending":31491,"ĠRevised":31492,"ĠRails":31493,"align":31494,"709":31495,"337":31496,"Ġfug":31497,"charging":31498,"tags":31499,"Ġuter":31500,"kish":31501,"WARNING":31502,"490":31503,"profits":31504,"Ġvoyage":31505,"Ġace":31506,"ĠVanguard":31507,"ĠTanks":31508,"ĠMuk":31509,"Ġ226":31510,"Safe":31511,"Armor":31512,"Ġvolcanic":31513,"Ġwomb":31514,"ĠMIL":31515,"Ġbeginner":31516,"ĠRecogn":31517,"ĠAAP":31518,"PLAY":31519,")!":31520,"Ġdetecting":31521,"cn":31522,"Ġbreaches":31523,"Basically":31524,"ĠPag":31525,"ĠMunicipal":31526,"ĠIndie":31527,"ĠLaf":31528,"ĠDisable":31529,"ĠOlson":31530,"Ġrestrained":31531,"Ġrulings":31532,"Ġhumane":31533,"events":31534,"ĠCinema":31535,"displayText":31536,"ĠHatch":31537,"actionDate":31538,"onnaissance":31539,"Ġassaulting":31540,"ĠLug":31541,"CHAT":31542,"Ġvigorous":31543,"ĠPerse":31544,"Ġintolerance":31545,"ĠSnapchat":31546,"ĠSharks":31547,"Ġdummy":31548,"ĠDiagn":31549,"ĠGuitar":31550,"imeters":31551,"403":31552,"REG":31553,"Ax":31554,"Ġseparates":31555,"ĠMahm":31556,"Ġtv":31557,"jah":31558,"OOL":31559,"Circ":31560,"ĠWindsor":31561,"ussian":31562,"Ġintuition":31563,"Ġdisdain":31564,"ĠDonovan":31565,"Ġ221":31566,"Emb":31567,"Ġcondemning":31568,"Ġgenerosity":31569,"zzy":31570,"Ġpanties":31571,"ĠPrevent":31572,"ActionCode":31573,"ANA":31574,"342":31575,"externalActionCode":31576,"Ġspecifying":31577,"Ġcrystall":31578,"Jere":31579,"Ġrupt":31580,"ĠApprentice":31581,"Ġprofiling":31582,"к":31583,"Strike":31584,"Ġsideline":31585,"Ġobligated":31586,"Ġoccult":31587,"Ġbureaucratic":31588,"antically":31589,"rupted":31590,"negative":31591,"ĠEthiopia":31592,"ĠCivic":31593,"Ġinsiders":31594,"eligible":31595,"ĠTVs":31596,"ĠBAR":31597,"ĠTI":31598,"iologist":31599,"ĠAIR":31600,"Ġsubstituted":31601,"Arab":31602,"ĠSaul":31603,"ĠYog":31604,"prem":31605,"Ġbuilders":31606,"Ġstationary":31607,"Ġdoubtful":31608,"Ġvigorously":31609,"Ġthrilling":31610,"Physical":31611,"ĠCarey":31612,"ĠHydra":31613,"geoning":31614,"ĠSly":31615,"yton":31616,"Ġborrowers":31617,"ĠParkinson":31618,"Ġë":31619,"ĠJamaica":31620,"Ġsatir":31621,"Ġinsurgents":31622,"ĠFirm":31623,"Ġisot":31624,"ĠKarn":31625,"ourning":31626,"akens":31627,"docs":31628,"little":31629,"ĠMonaco":31630,"CLASS":31631,"Turkey":31632,"Ly":31633,"ĠConan":31634,"assic":31635,"Ġstarred":31636,"ĠPacers":31637,"eties":31638,"Ġtipping":31639,"Moon":31640,"ĠRw":31641,"same":31642,"Ġcavity":31643,"Ġgoof":31644,"ĠZo":31645,"Shock":31646,"ummer":31647,"Ġemphasizes":31648,"Ġregrett":31649,"Ġnovelty":31650,"Ġenvy":31651,"ĠPassive":31652,"rw":31653,"505":31654,"Ġindifferent":31655,"ĠRica":31656,"ĠHimself":31657,"ĠFreddie":31658,"Ġadip":31659,"ä¸Ģ":31660,"Ġbreakout":31661,"Ġhurried":31662,"ĠHuang":31663,"ĠDisk":31664,"Ġroaming":31665,"?????-?????-":31666,"UV":31667,"ĠRicky":31668,"ĠSigma":31669,"Ġmarginalized":31670,"Ġedits":31671,"Ġ304":31672,"memory":31673,"Ġspecimen":31674,"293":31675,"ãģ¯":31676,"Ġvertically":31677,"Ġaudition":31678,"ĠHeck":31679,"Ġcaster":31680,"ĠHoldings":31681,"adal":31682,"ĠCron":31683,"ĠLiam":31684,"Ġdeflect":31685,"Pick":31686,"ĠDebug":31687,"REF":31688,"Ġversatility":31689,"othes":31690,"classified":31691,"ĠMahar":31692,"ĠHort":31693,"Counter":31694,"stasy":31695,"noticed":31696,"331":31697,"ĠShim":31698,"fuck":31699,"ĠBie":31700,"Ġairing":31701,"ĠProtein":31702,"ĠHolding":31703,"Ġspectators":31704,"iliated":31705,"ĠThatcher":31706,"nosis":31707,"ãĥ¼ãĥ³":31708,"Tele":31709,"Boston":31710,"ĠTempl":31711,"stay":31712,"Ġdeclarations":31713,"479":31714,"Volume":31715,"ĠDesigner":31716,"ĠOverwatch":31717,"idae":31718,"Ġonwards":31719,"Ġnets":31720,"ĠManila":31721,"particularly":31722,"Ġpolitic":31723,"oother":31724,"Ġportraits":31725,"Ġpavement":31726,"cffff":31727,"Ġsaints":31728,"Ġbeginners":31729,"ESPN":31730,"Ġshortcomings":31731,"âķIJâķIJ":31732,"Ġcomet":31733,"ĠOrganic":31734,"quel":31735,"Ġhospitalized":31736,"Break":31737,"Ġpeel":31738,"dylib":31739,"aspx":31740,"urances":31741,"ĠTIM":31742,"Pg":31743,"Ġreadable":31744,"ĠMalik":31745,"Ġmuzzle":31746,"Ġbenchmarks":31747,"dal":31748,"ĠVacc":31749,"ĠHicks":31750,"609":31751,"ĠBiblical":31752,"heng":31753,"Ġoverload":31754,"ĠCivilization":31755,"Ġimmoral":31756,"Ġfries":31757,"ãĤĴ":31758,"Ġreproduced":31759,"Ġformulation":31760,"jug":31761,"irez":31762,"gear":31763,"Ġcoached":31764,"MpServer":31765,"ĠSJ":31766,"ĠKw":31767,"Init":31768,"deal":31769,"ĠOro":31770,"ĠLoki":31771,"ĠSongs":31772,"Ġ232":31773,"ĠLouise":31774,"asionally":31775,"Ġuncond":31776,"ollywood":31777,"Ġprogressives":31778,"ĠEnough":31779,"ĠDoe":31780,"Ġwreckage":31781,"Ġbrushed":31782,"ĠBaseType":31783,"Ġzoning":31784,"ishable":31785,"hetically":31786,"ĠCaucus":31787,"ĠHue":31788,"Ġkarma":31789,"ĠSporting":31790,"Ġtrader":31791,"Ġseeming":31792,"ĠCapture":31793,"430":31794,"bish":31795,"Ġtunes":31796,"Ġindoors":31797,"ĠSphere":31798,"ĠDancing":31799,"TERN":31800,"Ġnob":31801,"ĠGST":31802,"maps":31803,"Ġpeppers":31804,"Fit":31805,"Ġoversees":31806,"ĠRabbi":31807,"ĠRuler":31808,"vertising":31809,"office":31810,"xxx":31811,"Ġraft":31812,"Changed":31813,"Ġtextbooks":31814,"Links":31815,"ĠOmn":31816,"ãĢij":31817,"Ġinconvenience":31818,"ĠDonetsk":31819,"=~":31820,"Ġimplicitly":31821,"Ġboosts":31822,"ĠBones":31823,"ĠBoom":31824,"Courtesy":31825,"Ġsensational":31826,"ANY":31827,"Ġgreedy":31828,"eden":31829,"Ġinexper":31830,"ĠLer":31831,"ĠVale":31832,"Ġtighten":31833,"ĠEAR":31834,"ĠNum":31835,"Ġancestor":31836,"Sent":31837,"ĠHorde":31838,"urgical":31839,"allah":31840,"Ġsap":31841,"amba":31842,"ĠSpread":31843,"twitch":31844,"Ġgrandson":31845,"Ġfracture":31846,"Ġmoderator":31847,"ĠSeventh":31848,"ĠReverse":31849,"Ġestimation":31850,"Choose":31851,"Ġparach":31852,"Ġbarric":31853,"ãĢIJ":31854,"Ġcompass":31855,"Ġallergic":31856,"âĢķ":31857,"OTHER":31858,"errilla":31859,"Ġwagon":31860,"Ġzinc":31861,"Ġrubbed":31862,"ĠFuller":31863,"ĠLuxembourg":31864,"ĠHoover":31865,"Ġliar":31866,"ĠEvening":31867,"ĠCobb":31868,"esteem":31869,"Ġselector":31870,"ĠBrawl":31871,"isance":31872,"ĠEk":31873,"Ġtroop":31874,"Ġguts":31875,"ĠAppeal":31876,"ĠTibetan":31877,"Ġroutines":31878,"ĠMent":31879,"Ġsummarized":31880,"steamapps":31881,"Ġtranqu":31882,"Ġ1929":31883,"oran":31884,"ĠAuthent":31885,"Ġgmaxwell":31886,"Ġapprehens":31887,"Ġpoems":31888,"Ġsausage":31889,"ĠWebster":31890,"urus":31891,"Ġthemed":31892,"Ġlounge":31893,"Ġcharger":31894,"Spoiler":31895,"Ġspilled":31896,"hog":31897,"ĠSunder":31898,"ĠAin":31899,"ĠAngry":31900,"Ġdisqual":31901,"ĠFrequency":31902,"ĠEthernet":31903,"Ġhelper":31904,"Percent":31905,"Ġhorrifying":31906,"Ġail":31907,"ĠAllan":31908,"EEE":31909,"ĠCrossing":31910,"449":31911,"Ġholog":31912,"ĠPuzzles":31913,"ĠGoes":31914,"erenn":31915,"604":31916,"ãģı":31917,"ĠRafael":31918,"Ġatten":31919,"ĠEmanuel":31920,"Ġupro":31921,"ĠSusp":31922,"Psych":31923,"ĠTrainer":31924,"ĠNES":31925,"ĠHunts":31926,"becue":31927,"Ġcounselor":31928,"Rule":31929,"Ġtoxins":31930,"Ġbanners":31931,"rifice":31932,"Ġgreeting":31933,"Ġfrenzy":31934,"Ġallocate":31935,"Ġ*)":31936,"expr":31937,"503":31938,"ĠChick":31939,"ĠTorn":31940,"Ġconsolidation":31941,"ĠFletcher":31942,"switch":31943,"frac":31944,"clips":31945,"ĠMcKin":31946,"ĠLunar":31947,"Month":31948,"ITCH":31949,"Ġscholarly":31950,"raped":31951,"398":31952,"Ġ1910":31953,"Ġegreg":31954,"Ġinsecure":31955,"Ġvictorious":31956,"cffffcc":31957,"Ġsingled":31958,"Ġelves":31959,"ĠWond":31960,"burst":31961,"Ġcamoufl":31962,"ĠBLACK":31963,"Ġconditioned":31964,"çī":31965,"answered":31966,"Ġcompulsory":31967,"ascist":31968,"Ġpodcasts":31969,"ĠFrankfurt":31970,"bnb":31971,"Ġneoliberal":31972,"ĠKeyboard":31973,"ĠBelle":31974,"warm":31975,"Ġtrusts":31976,"Ġinsured":31977,"ĠBucc":31978,"usable":31979,"607":31980,"ĠPlains":31981,"Ġ1890":31982,"Ġsabotage":31983,"Ġlodged":31984,"felt":31985,"Ġga":31986,"ĠNarc":31987,"ĠSalem":31988,"Ġseventy":31989,"ĠBlank":31990,"pocket":31991,"Ġwhisper":31992,"Ġmating":31993,"omics":31994,"ĠSalman":31995,"ĠKad":31996,"Ġangered":31997,"Ġcollisions":31998,"Ġextraordinarily":31999,"Ġcoercion":32000,"Ghost":32001,"birds":32002,"èĢ":32003,"kok":32004,"Ġpermissible":32005,"avorable":32006,"Ġpointers":32007,"Ġdissip":32008,"aci":32009,"Ġtheatrical":32010,"ĠCosmic":32011,"Ġforgetting":32012,"Ġfinalized":32013,"大":32014,"yout":32015,"library":32016,"Ġbooming":32017,"ĠBelieve":32018,"ĠTeacher":32019,"ĠLiv":32020,"ĠGOODMAN":32021,"ĠDominican":32022,"ORED":32023,"ĠParties":32024,"Ġprecipitation":32025,"ĠSlot":32026,"Roy":32027,"ĠCombined":32028,"Ġintegrating":32029,"Ġchrome":32030,"Ġintestinal":32031,"ĠRebell":32032,"Ġmatchups":32033,"Ġblockbuster":32034,"ĠLoren":32035,"ĠLevy":32036,"Ġpreaching":32037,"ĠSending":32038,"ĠPurpose":32039,"rax":32040,"fif":32041,"Ġauthoritative":32042,"ĠPET":32043,"astical":32044,"Ġdishon":32045,"Ġchatting":32046,"Ġ\"$:/":32047,"Connection":32048,"Ġrecreate":32049,"Ġdelinqu":32050,"Ġbroth":32051,"ĠDirty":32052,"ĠAdmin":32053,"zman":32054,"Ġscholarships":32055,"Ġ253":32056,"contact":32057,"alsa":32058,"767":32059,"creen":32060,"abbage":32061,"Ġ1915":32062,"Ġblended":32063,"Ġalarmed":32064,"Language":32065,"356":32066,"Ġblends":32067,"ĠChanged":32068,"Wolf":32069,"Ġhepat":32070,"Creating":32071,"Ġpersecut":32072,"Ġsweetness":32073,"arte":32074,"Ġforfeiture":32075,"ĠRoberto":32076,"impro":32077,"NFL":32078,"ĠMagnet":32079,"Detailed":32080,"Ġinsignificant":32081,"ĠPOLIT":32082,"ĠBBQ":32083,"ĠCPS":32084,"Ġseaw":32085,"aminer":32086,"mL":32087,"endif":32088,"finals":32089,"Ġ265":32090,"uish":32091,"Ġ})":32092,"ĠProblems":32093,"Ġemblem":32094,"Ġseriousness":32095,"Ġparsing":32096,"Ġsubstitution":32097,"Ġpressured":32098,"Ġrecycled":32099,"aleb":32100,"Ruby":32101,"Ġproficiency":32102,"Driver":32103,"ĠWester":32104,":'":32105,"AFTA":32106,"Ġmantle":32107,"ĠClayton":32108,"flag":32109,"Ġpractitioner":32110,"covered":32111,"ĠStruct":32112,"addafi":32113,"425":32114,"ĠTownship":32115,"ĠHydro":32116,"Louis":32117,"343":32118,"Ġcondo":32119,"ĠTao":32120,"Ġutilization":32121,"Ġnausea":32122,"ĠDems":32123,"ridges":32124,"pause":32125,"Ġformulas":32126,"Ġchallenger":32127,"376":32128,"Ġdefective":32129,"ĠRailway":32130,"ĠPubMed":32131,"Ġyogurt":32132,"lbs":32133,"ĠNorfolk":32134,"OPE":32135,"ĠMoody":32136,"Ġdistributor":32137,"Ġscrolls":32138,"Ġextracts":32139,"Stan":32140,"Ġviability":32141,"Ġexposes":32142,"Ġstarvation":32143,"ĠSteps":32144,"ĠDodd":32145,"few":32146,"STD":32147,"332":32148,"Ġclosures":32149,"Ġcomplementary":32150,"ĠSasha":32151,"umpy":32152,"Ġmonet":32153,"Ġarticulate":32154,"ĠDoct":32155,"killer":32156,"Ġscrim":32157,"Ġ264":32158,"Ġprostitutes":32159,"Ġsevered":32160,"Ġattachments":32161,"Ġcooled":32162,"Lev":32163,"ĠFalk":32164,"fail":32165,"Ġpoliceman":32166,"ĠDag":32167,"Ġprayed":32168,"ĠKernel":32169,"Ġclut":32170,"Ġcath":32171,"Ġanomaly":32172,"Storm":32173,"emaker":32174,"ĠBreakfast":32175,"uli":32176,"oire":32177,"JJ":32178,"hz":32179,"Operation":32180,"ĠSick":32181,"354":32182,"ĠGuatemala":32183,"Rate":32184,"Ġexposures":32185,"faces":32186,"ĠArchae":32187,"raf":32188,"ĠMia":32189,"Ġ2025":32190,"Ġopaque":32191,"Ġdisguised":32192,"ĠHeadquarters":32193,"Sah":32194,"Ġpots":32195,"978":32196,"ĠMalf":32197,"Ġfrowned":32198,"Ġpoisonous":32199,"ĠConvers":32200,"eeks":32201,"Ġcrab":32202,".\"\"":32203,"Ġtreason":32204,"Ġranc":32205,"Ġescalating":32206,"Ġwarr":32207,"Ġmobs":32208,"Ġlamps":32209,"ĠSunshine":32210,"ĠBrunswick":32211,"Phones":32212,"Ġspelled":32213,"ĠSkip":32214,"Ġ2050":32215,"Ġ1911":32216,"ĠPluto":32217,"ĠAmend":32218,"Ġmeats":32219,"387":32220,"Ġstomp":32221,"ĠZhou":32222,"ĠLeviathan":32223,"ĠHazard":32224,"adv":32225,"ĠOrwell":32226,"Ġaloud":32227,"Ġbumper":32228,"ĠAnarch":32229,"ubuntu":32230,"ĠSerious":32231,"fitting":32232,"ĠOptional":32233,"ĠCecil":32234,"REAM":32235,"Ġserotonin":32236,"Ġcultivate":32237,"agogue":32238,"}\\":32239,"Ġmosques":32240,"ĠSunny":32241,"Ġreactive":32242,"revolution":32243,"ĠLup":32244,"ĠFedora":32245,"Ġdefenseman":32246,"ĠVID":32247,"istine":32248,"Ġdrowning":32249,"ĠBroadcasting":32250,"Ġthriller":32251,"ĠScy":32252,"Ġaccelerating":32253,"Ġdirects":32254,"odied":32255,"bike":32256,"duration":32257,"Ġpainfully":32258,"Redd":32259,"Ġproductions":32260,"Ġgag":32261,"Ġwhist":32262,"Ġsock":32263,"Ġinfinitely":32264,"ĠConcern":32265,"ĠCitadel":32266,"Ġlieu":32267,"Ġcandles":32268,"ogeneous":32269,"arger":32270,"Ġheavenly":32271,"inflammatory":32272,"Performance":32273,"Cs":32274,"ructose":32275,"azaki":32276,"Ġpessim":32277,"Ġinference":32278,"Ġpowd":32279,"ĠZoe":32280,"Ġpaints":32281,"Ġdazz":32282,"pta":32283,"-----------":32284,"Ġinspir":32285,"ĠExperimental":32286,"ĠKnife":32287,"regor":32288,"bors":32289,"Ġshowers":32290,"romeda":32291,"Ġsaint":32292,"Ġbenign":32293,"ĠJiang":32294,"Ġenvisioned":32295,"Ġshroud":32296,"IFT":32297,"HO":32298,"Ġshuff":32299,"ĠICC":32300,"Ġsegreg":32301,"Ġrevisit":32302,"ighthouse":32303,"Li":32304,"Ġsubstrate":32305,"ĠSeas":32306,"ĠReward":32307,"ĠHep":32308,"ĠBrass":32309,"sbm":32310,"Ġeliminates":32311,"Ġstamina":32312,"ĠVAT":32313,"ĠLoan":32314,"Ġconstraint":32315,"Ġappropriated":32316,"Ġpes":32317,"ĠALE":32318,"ranging":32319,"Ġ404":32320,"392":32321,"Ġintellectuals":32322,"achu":32323,"Ġrestructuring":32324,"ĠLevin":32325,"Ġrunes":32326,"Ġdelightful":32327,"Ġcarbohydrates":32328,"ĠModels":32329,"ĠExpo":32330,"Ġtransporting":32331,"alloc":32332,"Ġringing":32333,"Samsung":32334,"Ġscarcely":32335,"ĠURLs":32336,"ĠMAS":32337,"Ġprototypes":32338,"Ġnarrator":32339,"ĠCPUs":32340,"cdn":32341,"ĠBarton":32342,"Ġdecidedly":32343,"ĠShu":32344,"ixir":32345,"ocious":32346,"ĠMyst":32347,"Nintendo":32348,"Ġreuse":32349,"Ġforgiven":32350,"Few":32351,"inical":32352,"nat":32353,"Ġseamless":32354,"ĠEva":32355,"ĠEVE":32356,"ĠJO":32357,"landers":32358,"Ġsofter":32359,"negie":32360,"Ġtransient":32361,"Ġorbital":32362,"Ġfulfil":32363,"ĠKom":32364,"Hopefully":32365,"Ġdynamically":32366,"ĠHunger":32367,"åĽ":32368,"ĠArmenia":32369,"elman":32370,"berto":32371,"Ġpige":32372,"ĠIDs":32373,"limit":32374,"Ġveins":32375,"Ġsoaring":32376,"packs":32377,"Golden":32378,"ĠCrab":32379,"istor":32380,"ĠRPM":32381,"Ġ$$":32382,"gression":32383,"Ġjihadist":32384,"Ġgamble":32385,"Ġcareg":32386,"Ġinflated":32387,"Face":32388,"ĠFirearms":32389,"ĠEmmanuel":32390,"âĿ":32391,"Ġshocks":32392,"grab":32393,"Ġsplend":32394,"ĠHPV":32395,"abortion":32396,"Above":32397,"Entity":32398,"players":32399,"Ġcommenced":32400,"ulence":32401,"Ġfulfillment":32402,"Ġembodiments":32403,"ĠWelfare":32404,"Ġhail":32405,"Ġ<@":32406,"tten":32407,"Ġcatcher":32408,"ĠJazeera":32409,"Ġvolcano":32410,"Ġstabilize":32411,"ĠHandler":32412,"Ġintensified":32413,"ĠAbrams":32414,"Ġhumiliation":32415,"paced":32416,"605":32417,"ĠCentOS":32418,"Specific":32419,"Ġheed":32420,"ĠCAM":32421,"ĠGalile":32422,"Die":32423,"Ġabolished":32424,"ĠThomson":32425,"ĠTeachers":32426,"ĠWass":32427,"jong":32428,"ĠISBN":32429,"ĠAllies":32430,"shake":32431,"å·":32432,"vict":32433,"Howard":32434,"Ġdeem":32435,"Ġexceedingly":32436,"ĠSmartstocks":32437,"ibe":32438,"Ġdoorway":32439,"Ġcompeted":32440,"igmat":32441,"Ġnationalists":32442,"Ġgroom":32443,"ĠKeen":32444,"Ġdisposable":32445,"decl":32446,"ĠTolkien":32447,"ĠScheme":32448,"Ġbiod":32449,"Ġavid":32450,"ĠElon":32451,"agar":32452,"ĠTSA":32453,"Roman":32454,"Ġartificially":32455,"Ġadvisors":32456,"XL":32457,"ĠInferno":32458,"366":32459,"Ġtedious":32460,"ĠPhotography":32461,"ĠCarrie":32462,"Ġtrope":32463,"ĠSandra":32464,"Ġdecimal":32465,"Queen":32466,"ĠGundam":32467,"ĠOM":32468,"otech":32469,"NBA":32470,"Ġ1932":32471,"Ġentrenched":32472,"ĠMarion":32473,"Ġfraternity":32474,"Labour":32475,"Henry":32476,"Ġlatitude":32477,"Either":32478,"Ġenhances":32479,"ĠPotential":32480,"Ġshines":32481,"idad":32482,"Ġbreadth":32483,"Ġcapacities":32484,"ĠðŁĻĤ":32485,"ĠBronx":32486,"Ġsexes":32487,"Ġdifferentiation":32488,"Ġheavyweight":32489,"ĠTaj":32490,"dra":32491,"Ġmigrate":32492,"Ġexhaustion":32493,"ĠRUN":32494,"elsius":32495,"ĠCuomo":32496,"Ġguitars":32497,"Ġclones":32498,"ĠSomew":32499,"ĠPry":32500,"-------------":32501,"Ġwarranted":32502,"cycles":32503,"Ġsalvage":32504,"Ġdisks":32505,"RANT":32506,"ĠNGOs":32507,"ĠMartian":32508,"\":[{\"":32509,"Ġaddicts":32510,"ojure":32511,"illet":32512,"Ġamazingly":32513,"artments":32514,"pixel":32515,"ĠGPUs":32516,"Layout":32517,"è£":32518,"ĠTamil":32519,"ĠBasil":32520,"Ġimpartial":32521,"ĠStructure":32522,"fork":32523,"bryce":32524,"Ġridge":32525,"ĠHamburg":32526,"rious":32527,"Ġblitz":32528,"cigarettes":32529,"Ġcanned":32530,"402":32531,"Ġironically":32532,"Ġcompassionate":32533,"ĠHawkins":32534,".#":32535,"ĠCathedral":32536,"Ġrallied":32537,"internal":32538,"Ġquota":32539,"stakes":32540,"TEXT":32541,"mom":32542,"Ġcompletes":32543,"Ġ238":32544,"Ġshrug":32545,"ãĥij":32546,"ĠNinth":32547,"Ġrevise":32548,"ĠProvider":32549,"Ġtreacher":32550,"Ġquasi":32551,"ĠPRES":32552,"Ġdeposition":32553,"Ġconfidentiality":32554,"issors":32555,"Ġimbalance":32556,"Ġspanning":32557,"Ġangular":32558,"ĠCul":32559,"communication":32560,"ĠNora":32561,"ĠGenius":32562,"opter":32563,"Ġsacked":32564,"Spot":32565,"Ġfinely":32566,"ĠCHR":32567,"282":32568,"waves":32569,"Palest":32570,"ĠRohing":32571,"NL":32572,"è¿":32573,"Ġshitty":32574,"ĠScalia":32575,"475":32576,"Progress":32577,"Ġreferencing":32578,"Ġclassrooms":32579,"abee":32580,"Ġsod":32581,"hesion":32582,"708":32583,"ĠZuckerberg":32584,"ĠFinish":32585,"ĠScotia":32586,"ĠSavior":32587,"ĠInstallation":32588,"antha":32589,"(-":32590,"Ġ302":32591,"ĠPunk":32592,"Ġcrater":32593,"youtu":32594,"Ġroast":32595,"Ġinfluencing":32596,"Ġdup":32597,"ĠJR":32598,"ĠGrav":32599,"Ġstature":32600,"Ġbathrooms":32601,"Aside":32602,"Wiki":32603,"mean":32604,"ĠZak":32605,"ĠOnes":32606,"ĠNath":32607,"Ġhypert":32608,"Ġcommencement":32609,"Civil":32610,"Ġmoderately":32611,"Ġdistributors":32612,"Ġbreastfeeding":32613,"Ġ980":32614,"ĠSik":32615,"ĠCig":32616,"ĠAMER":32617,"RIP":32618,"ĠCareer":32619,"usting":32620,"Ġmessed":32621,"Ġeh":32622,"ĠJensen":32623,"/$":32624,"Ġblackmail":32625,"Ġconversions":32626,"Ġscientifically":32627,"Ġmantra":32628,"paying":32629,"Ġivory":32630,"ĠCourts":32631,"OUGH":32632,"auntlet":32633,"Serial":32634,"Brow":32635,"ĠHundreds":32636,"323":32637,"Ġpee":32638,"Ġlinux":32639,"Ġsubmer":32640,"ĠPrincipal":32641,"485":32642,"ĠDSL":32643,"ĠCousins":32644,"Ġdoctrines":32645,"ĠAthletics":32646,"Ġ315":32647,"ĠKarma":32648,"Ġattent":32649,"urger":32650,"Ġprescribe":32651,"Ġencaps":32652,"ĠCame":32653,"Ġsecretive":32654,"ĠCrimes":32655,"dn":32656,"Clean":32657,"ĠEgyptians":32658,"ĠCarpenter":32659,"Ġll":32660,"Hum":32661,"ĠMilo":32662,"Ġcapitalists":32663,"Ġbriefed":32664,"Twe":32665,"ĠBasin":32666,"elvet":32667,"Mos":32668,"Ġplunge":32669,"ĠKaiser":32670,"ĠFuj":32671,"illin":32672,"Ġsafeguards":32673,"Ġoste":32674,"ĠOpportunity":32675,"ĠMafia":32676,"ĠCalling":32677,"apa":32678,"urban":32679,"brush":32680,"illard":32681,"cé":32682,"intelligence":32683,"ĠLob":32684,"ĠDruid":32685,"Ġsmoother":32686,"Ġfooting":32687,"Ġmotorists":32688,"arcity":32689,"Ġmasculinity":32690,"Ġmism":32691,"Ġabdominal":32692,"ĠTavern":32693,"ĠRoh":32694,"Ġescapes":32695,"signed":32696,"Anthony":32697,"Ġsacrificing":32698,"Ġintimacy":32699,"Ġanterior":32700,"ĠKod":32701,"Ġmotif":32702,"Ġgraz":32703,"Ġvisualization":32704,"Ġguitarist":32705,"ĠTrotsky":32706,"magic":32707,"Dar":32708,"ĠMori":32709,"Ġwards":32710,"Ġtoilets":32711,"lest":32712,"Ġteleport":32713,"ĠSundays":32714,"ĠPlat":32715,"ETS":32716,"ĠeSports":32717,"Patrick":32718,"ĠKatherine":32719,"enko":32720,"Ġhassle":32721,"ĠMick":32722,"ggles":32723,"Ġhob":32724,"aintain":32725,"Ġairborne":32726,"Ġspans":32727,"Ġchili":32728,"Ġaperture":32729,"Ġvolunteered":32730,"ĠIncident":32731,"ĠFres":32732,"ĠVeteran":32733,"aughtered":32734,"ingo":32735,"Ġuninsured":32736,"CLOSE":32737,"Ġfuse":32738,"Ġerotic":32739,"Ġadvertise":32740,"raising":32741,"Texture":32742,"Ġattends":32743,"ĠREAL":32744,"uddled":32745,"Ġsmoot":32746,"Ġ305":32747,"ĠWillis":32748,"Ġblond":32749,"Analysis":32750,"ĠVT":32751,"onica":32752,"Ġstronghold":32753,"RF":32754,"NM":32755,".>>":32756,"Ġprosperous":32757,"Ġboasted":32758,"292":32759,"ĠManufacturing":32760,"PRESS":32761,"gren":32762,"Ġpharmacy":32763,"ĠRockefeller":32764,"kai":32765,"Ġthumbs":32766,"ĠHut":32767,"Ġmotherboard":32768,"Ġguardians":32769,"ĠAlter":32770,"llular":32771,"Ġshack":32772,"Ġwisely":32773,"Ġbackbone":32774,"erva":32775,"Ġsuicides":32776,"ĠMcGregor":32777,"ijah":32778,"Emer":32779,"ĠBrav":32780,"Ġdesignate":32781,"POST":32782,"produced":32783,"Ġcleansing":32784,"irlwind":32785,"existent":32786,"ĠHumph":32787,"ĠPayne":32788,"Ġvested":32789,"Å¡":32790,"Ġstringent":32791,"iona":32792,"Ġunsub":32793,"Ġsummed":32794,"ĠHercules":32795,"subject":32796,"ĠRagnar":32797,"ĠNos":32798,"Ġcharacterization":32799,"Ġsavvy":32800,"ĠDawson":32801,"ĠCasino":32802,"Ġfri":32803,"ĠBarrier":32804,"Ġmisinformation":32805,"Ġinsulation":32806,"Ġcorridors":32807,"Ġairplanes":32808,"ĠNoct":32809,"ahi":32810,"Ġ1916":32811,"kb":32812,"armac":32813,"Ġshun":32814,"Ġschema":32815,"Ġhorrified":32816,"Ġ239":32817,"aunders":32818,"NB":32819,"iates":32820,"erity":32821,"ĠShard":32822,"Ġrarity":32823,"Ġgrouped":32824,"ĠGhana":32825,"against":32826,"ĠBiological":32827,"ĠAware":32828,"owell":32829,"ÏĦ":32830,"ĠBeau":32831,"shaw":32832,"Hack":32833,"ĠJulius":32834,"USS":32835,"olson":32836,"auna":32837,"cru":32838,"ĠMaurice":32839,"ĠIk":32840,"Ġsequencing":32841,"Ġradicals":32842,"Ġ(?,":32843,"virtual":32844,"Ġanyways":32845,"Ġreperc":32846,"Ġhandlers":32847,"Ġhesitant":32848,"éĥ":32849,"ĠMF":32850,"plementation":32851,"associated":32852,"Ġcampaigned":32853,"ĠYue":32854,"utations":32855,"ĠYoga":32856,"Ġsimmer":32857,"Ġrods":32858,"Ġmelody":32859,"Ġconvoy":32860,"videos":32861,"Ġscreened":32862,"Neg":32863,"ochemical":32864,"Ġ())":32865,"Ġultras":32866,"Ġantip":32867,"ĠIslanders":32868,"704":32869,"Ġfetish":32870,"Ġridiculously":32871,"ĠKart":32872,"Ġmitochondrial":32873,"Ġinterfering":32874,"Builder":32875,"Ġoverfl":32876,"Ġacne":32877,"ĠMud":32878,"ĠKerr":32879,"flex":32880,"ĠPostal":32881,"ĠBaltic":32882,"477":32883,"ĠPersons":32884,"ourage":32885,"HB":32886,"ĠMuse":32887,"ĠImmortal":32888,"ĠDriving":32889,"Ġpetitions":32890,"Ġsubscript":32891,"Ġsorce":32892,"ĠProcessor":32893,"uton":32894,"Sony":32895,"Ġphon":32896,"Ġraced":32897,"ĠAnthrop":32898,"Ġdaytime":32899,"ĠExercise":32900,"Adding":32901,"Ġengages":32902,"ĠQualcomm":32903,"Ġmiracles":32904,"Ġmemes":32905,"ĠDrink":32906,"ĠOrioles":32907,"Ġhairs":32908,"ĠPolar":32909,"athom":32910,"Ġslippery":32911,"ĠRemy":32912,"Ġcaramel":32913,"ĠYEAR":32914,"Ġalk":32915,"Ign":32916,"aution":32917,"ĠMerlin":32918,"ĠCran":32919,"Ġapologies":32920,"Ġ410":32921,"Ġouting":32922,"ĠMemories":32923,"appointed":32924,"Ġcountered":32925,"uld":32926,"posing":32927,"Ġfirewall":32928,"ĠWast":32929,"ĠWet":32930,"worked":32931,"seller":32932,"Ġrepealed":32933,"ereo":32934,"assuming":32935,"BLIC":32936,"mite":32937,"ĠCEOs":32938,"ĠChapel":32939,"elligent":32940,"________________________":32941,"Dog":32942,"Ġwart":32943,"Ġsubscriber":32944,"sports":32945,"Ġbegged":32946,"ĠMV":32947,"Ġsemif":32948,"ethical":32949,"Ġpreach":32950,"Ġrevital":32951,"Ġpunitive":32952,"Ġshortcuts":32953,"Ġinstituted":32954,"ĠWarsaw":32955,"Ġabdomen":32956,"ĠKING":32957,"Ġsuperintendent":32958,"Ġfry":32959,"ĠGeo":32960,"TOR":32961,"Ġcontradictions":32962,"aptic":32963,"Ġlandscapes":32964,"bugs":32965,"Ġclust":32966,"Ġvolley":32967,"cribed":32968,"Ġtandem":32969,"Ġrobes":32970,"WHAT":32971,"Ġpromoter":32972,"Ġeloqu":32973,"reviewed":32974,"ĠDK":32975,"ĠPlato":32976,"Ġfps":32977,"Tank":32978,"ĠDerrick":32979,"Ġprioritize":32980,"asper":32981,"ĠHonduras":32982,"ĠCompleted":32983,"nec":32984,"Ġmog":32985,"nir":32986,"ĠMayo":32987,"DEF":32988,"stall":32989,"inness":32990,"ĠVolkswagen":32991,"Ġprecaution":32992,"ĠMell":32993,"iak":32994,"istries":32995,"Ġ248":32996,"Ġoverlapping":32997,"Senate":32998,"ĠEnhance":32999,"resy":33000,"racial":33001,"ORTS":33002,"ĠMormons":33003,"Strong":33004,"ĠCoch":33005,"Mexico":33006,"ĠMaduro":33007,"Ġjars":33008,"Ġcane":33009,"Wik":33010,"olla":33011,"ifference":33012,"Ġphysicist":33013,"ĠMaggie":33014,"Ġ285":33015,"Ġdepiction":33016,"ĠMcLaren":33017,"Ju":33018,"Ġslows":33019,"Ġcommissioners":33020,"ĠWillow":33021,"ĠExplos":33022,"hovah":33023,"Ġtechnician":33024,"Ġhomicides":33025,"ĠFlav":33026,"ĠTruman":33027,"Ġ10000":33028,"uctor":33029,"Ġshader":33030,"Newsletter":33031,"457":33032,"Ġrever":33033,"Ġhardened":33034,"Ġwhereabouts":33035,"Ġredevelop":33036,"Ġcarbs":33037,"Ġtravers":33038,"Ġsquirrel":33039,"Ġfollower":33040,"Ġsings":33041,"508":33042,"Ġrabbits":33043,"emonium":33044,"Ġdocumenting":33045,"Ġmisunderstood":33046,")'":33047,"Rick":33048,"ggies":33049,"Ġpremie":33050,"Ġskating":33051,"Ġpassports":33052,"Ġfists":33053,"ageddon":33054,"Haw":33055,"ACP":33056,"080":33057,"ĠThoughts":33058,"ĠCarlson":33059,"Ġpriesthood":33060,"hua":33061,"Ġdungeons":33062,"ĠLoans":33063,"Ġantis":33064,"Ġfamiliarity":33065,"ĠSabb":33066,"opal":33067,"ĠInk":33068,"strike":33069,"Ġcram":33070,"Ġlegalized":33071,"Ġcuisine":33072,"Ġfibre":33073,"Travel":33074,"ĠMonument":33075,"ODY":33076,"ethy":33077,"Ġinterstate":33078,"ĠPUR":33079,"emporary":33080,"ĠArabian":33081,"developed":33082,"Ġsaddle":33083,"Ġgithub":33084,"ĠOffer":33085,"ĠISP":33086,"rolet":33087,"ĠSUPER":33088,"ĠDenis":33089,"Ġmultiplier":33090,"Ġstirred":33091,"Interestingly":33092,"Ġcustomary":33093,"Ġbilled":33094,"hex":33095,"Ġmultiplied":33096,"Ġflipping":33097,"ĠCrosby":33098,"Ġfundamentals":33099,"iae":33100,"ĠPlayed":33101,"ĠAtom":33102,"amazon":33103,"ĠFlam":33104,"eez":33105,"activated":33106,"Ġtablespoon":33107,"Ġliberalism":33108,"ĠPalin":33109,"ĠPatel":33110,"Num":33111,"ĠTAM":33112,"Ġsurn":33113,"ĠReloaded":33114,"Ġcoined":33115,"\"],":33116,"ĠClash":33117,"ĠAgu":33118,"Ġpragmatic":33119,"ĠActivate":33120,"Ġ802":33121,"Ġtrailers":33122,"Ġsilhou":33123,"Ġprobes":33124,"Ġcircus":33125,"ĠBain":33126,"ĠLindsay":33127,"ĠAbbey":33128,"Delivery":33129,"Ġconcession":33130,"Ġgastro":33131,"ĠSprite":33132,"ÄŁ":33133,"andel":33134,"Ġgimm":33135,"Ġautobi":33136,"ĠTurtle":33137,"Ġwonderfully":33138,"ĠHaram":33139,"ĠWorldwide":33140,"ĠHandle":33141,"Ġtheorists":33142,"Ġsleek":33143,"ĠZhu":33144,"ographically":33145,"EGA":33146,"ĠOwners":33147,"aths":33148,"ĠAntarctic":33149,"natal":33150,"=\"\"":33151,"flags":33152,"````":33153,"Ġsul":33154,"Kh":33155,"Ġpotassium":33156,"Ġlineman":33157,"Ġcereal":33158,"ĠSeasons":33159,"Ġ2022":33160,"Ġmathematic":33161,"Ġastronomers":33162,"professional":33163,"Ġfares":33164,"cknowled":33165,"Ġchi":33166,"Ġyoungsters":33167,"Ġmistakenly":33168,"Ġhemisphere":33169,"ĠDivinity":33170,"rone":33171,"Ġ\",":33172,"rings":33173,"Ġattracts":33174,"vana":33175,"å¹":33176,"CAP":33177,"Ġplaylist":33178,"Ġporch":33179,"ãģ£":33180,"Ġincorporates":33181,"Ġsoak":33182,"Ġasserting":33183,"ĠTerrorism":33184,"ĠPablo":33185,"Ja":33186,"cester":33187,"Ġfearing":33188,"ĠPrayer":33189,"Ġescalated":33190,"GW":33191,"Ġrobe":33192,"ĠBrighton":33193,"acists":33194,"ĠSymphony":33195,"ĠDwarf":33196,"ĠParade":33197,"ĠLego":33198,"Ġinexpl":33199,"Ġlords":33200,"leaf":33201,"RAG":33202,"liber":33203,"Ġcigars":33204,"ĠJehovah":33205,"606":33206,"WINDOWS":33207,"ĠLiberia":33208,"ebus":33209,"Heavy":33210,"Ġlubric":33211,"ĠRW":33212,"anguages":33213,"Ġnarrowed":33214,"computer":33215,"ĠEmber":33216,"Ġmurdering":33217,"Ġdownstream":33218,"ĠTuls":33219,"ĠTables":33220,"Topic":33221,"ĠAccuracy":33222,"=/":33223,"lost":33224,"ĠRei":33225,"Ġprogresses":33226,"bear":33227,"Ġestablishments":33228,"Justin":33229,"ĠPeach":33230,"ĠGomez":33231,"å¿":33232,"ĠTriangle":33233,"Ident":33234,"ĠHive":33235,"Resources":33236,"Ġmixes":33237,"ĠAssuming":33238,"Mu":33239,"Ġhypoc":33240,"Ġsane":33241,"ĠWan":33242,"idious":33243,"Success":33244,"Ġio":33245,"Angel":33246,"Ġdangerously":33247,"ĠCreature":33248,"WORK":33249,":[":33250,"ĠKatrina":33251,"Listener":33252,"Miller":33253,"ĠIdlib":33254,"hang":33255,"Ġcircumvent":33256,"href":33257,"Ġcelestial":33258,"ĠWeeks":33259,"ĠPug":33260,"ĠDalton":33261,"Ġsubpoena":33262,"uku":33263,"Ġpersisted":33264,"pei":33265,"olding":33266,"ĠDocuments":33267,"ĠHast":33268,"ĠCENT":33269,"Ġprimer":33270,"Ġsynonymous":33271,"Ġnib":33272,"ombs":33273,"Ġnotation":33274,"ĠDish":33275,"ĠAtmosp":33276,"Ġforbid":33277,"ĠANG":33278,"pattern":33279,"los":33280,"Ġprojectiles":33281,"brown":33282,".\",":33283,"ĠVenom":33284,"Ġfiercely":33285,"ublished":33286,"ĠUran":33287,"ĠNicarag":33288,"410":33289,"ĠCAL":33290,"OTOS":33291,"ĠMiracle":33292,"ĠEnchant":33293,"Ġguarding":33294,"append":33295,"Attach":33296,"Ġleveled":33297,"Ġcondoms":33298,"ihilation":33299,"649":33300,"Ġnightmares":33301,"ĠTHEY":33302,"ĠSTART":33303,"ĠKinn":33304,"Ġroommate":33305,"Ġhygiene":33306,"opping":33307,"Job":33308,"Ġlvl":33309,"ĠVER":33310,"ĠKeeping":33311,"abetic":33312,"Ġformatting":33313,"erala":33314,"Ġrevisions":33315,"Ġresurg":33316,"Tel":33317,"ĠGoodman":33318,"353":33319,"pod":33320,"Ġindisp":33321,"ĠTranslation":33322,"Ġgown":33323,"ĠMund":33324,"Ġcis":33325,"Ġbystand":33326,"collect":33327,"ĠPunjab":33328,"actively":33329,"ĠGamb":33330,"tell":33331,"Ġimporting":33332,"gencies":33333,"Ġlocom":33334,"ĠBrill":33335,"Holy":33336,"ĠBerger":33337,"Ġshowdown":33338,"Ġresponders":33339,"ILY":33340,"Ġtakedown":33341,"leted":33342,"Ġmattered":33343,"Ġpredictive":33344,"Ġoverlay":33345,"GPU":33346,"ĠVick":33347,"Ġconveyed":33348,"Tab":33349,"peer":33350,"Scan":33351,"Ġdefensively":33352,"vae":33353,"Ġapproving":33354,"Ġtiers":33355,"ĠVia":33356,"querade":33357,"ĠSaudis":33358,"Ġdemolished":33359,"ĠProphe":33360,"Ġmono":33361,"Ġhospitality":33362,"HAM":33363,"ĠAriel":33364,"MOD":33365,"ĠTorah":33366,"Ġblah":33367,"ĠBelarus":33368,"erential":33369,"ĠTuc":33370,"Ġbanker":33371,"397":33372,"Ġmosquit":33373,"ĠScientist":33374,"ĠMusical":33375,"Ġhust":33376,"Shift":33377,"Ġtorment":33378,"Ġstandoff":33379,"Educ":33380,"ĠFog":33381,"Ġamplifier":33382,"Shape":33383,"Instance":33384,"ĠCritics":33385,"Ġdaemon":33386,"Houston":33387,"Ġmattress":33388,"ĠIDF":33389,"Ġobscene":33390,"ĠAmer":33391,"hetti":33392,"Ġcompiling":33393,"352":33394,"verett":33395,"ĠReduction":33396,"istration":33397,"ĠBlessed":33398,"ĠBachelor":33399,"316":33400,"Ġprank":33401,"ĠVulcan":33402,"dding":33403,"Ġmourning":33404,"ĠQuint":33405,"ĠBlaster":33406,"testing":33407,"Ġsediment":33408,">>>":33409,"ĠEternity":33410,"ĠWHERE":33411,"ĠMaze":33412,"Ġreacting":33413,"ĠAlv":33414,"omsday":33415,"ĠCRA":33416,"Ġtranslator":33417,"Ġbogus":33418,"atu":33419,"Website":33420,"olls":33421,"Ġbaptism":33422,"Ġsibling":33423,"ĠAutumn":33424,"vez":33425,"ãģ®é":33426,"guards":33427,"Georg":33428,"assadors":33429,"ĠFreud":33430,"Ġcontinents":33431,"ĠRegistry":33432,"Bernie":33433,"ĸļ士":33434,"Ġtolerant":33435,"ĠUW":33436,"Ġhorribly":33437,"995":33438,"ĠMIDI":33439,"Ġimpatient":33440,"ocado":33441,"eri":33442,"ĠWorst":33443,"ĠNorris":33444,"ĠTalking":33445,"Ġdefends":33446,"ensable":33447,"Ġ2021":33448,"Ġanatomy":33449,"Lew":33450,"Ġdrawer":33451,"ĠCanberra":33452,"Ġpatriotic":33453,"é¾įåĸļ士":33454,"ĠAvg":33455,"ARM":33456,"Ġundisclosed":33457,"Ġfarewell":33458,"459":33459,"bable":33460,"ĠAllison":33461,"OLOG":33462,"Ġconco":33463,"tight":33464,"ĠACPI":33465,"ĠMines":33466,"lich":33467,"ĠâĶľ":33468,"represented":33469,"200000":33470,"Ġenthusiast":33471,"OTS":33472,"bil":33473,"ĠIngredients":33474,"Ġinventor":33475,"ĠMySQL":33476,"³³³":33477,"ĠABOUT":33478,"within":33479,"Ġmk":33480,"Bul":33481,"ĠFake":33482,"Ġdraconian":33483,"Wa":33484,"helm":33485,"ĠTerran":33486,"erville":33487,"Ġcommonplace":33488,"SIZE":33489,"Ġ\"<":33490,"replace":33491,"ographs":33492,"ĠSELECT":33493,"incible":33494,"ĠMostly":33495,"ĠSheffield":33496,"ĠIDE":33497,"uggle":33498,"Ġcitations":33499,"hurst":33500,"ĠUnix":33501,"Ġunleash":33502,"ĠPiper":33503,"ĠNano":33504,"Ġsuccumb":33505,"Ġreluctance":33506,"Ġ2500":33507,"ĠMerchant":33508,"Ġwiret":33509,"Ġcombos":33510,"ĠBirthday":33511,"Ġcharcoal":33512,"ĠUPS":33513,"ĠFairfax":33514,"Ġdriveway":33515,"ĠTek":33516,"ĠPitch":33517,"overe":33518,"Ġtechnicians":33519,"ĠActual":33520,"flation":33521,"ĠFiscal":33522,"ĠEmpty":33523,"anamo":33524,"Ġmagnesium":33525,"Ġslut":33526,"Ġgrowers":33527,"Investigators":33528,"():":33529,"ĠSatellite":33530,"ĠKeynes":33531,"missive":33532,"lane":33533,"Ġborough":33534,"344":33535,"ĠTEAM":33536,"ĠBethesda":33537,"CV":33538,"hower":33539,"ĠRAD":33540,"Ġchant":33541,"ĠRiy":33542,"Ġcompositions":33543,"Ġmildly":33544,"Ġmeddling":33545,"Ġagility":33546,"aneers":33547,"501":33548,"Ġsynth":33549,"linger":33550,"291":33551,"Ġexclaimed":33552,"Party":33553,"Ġcontamin":33554,"ĠManor":33555,"ĠRespond":33556,"Ġpraising":33557,"Ġmanners":33558,"fleet":33559,"Summer":33560,"ĠLynd":33561,"ĠDefinitely":33562,"grim":33563,"Ġbowling":33564,"stri":33565,"çĽ":33566,"ynt":33567,"Ġmandates":33568,"DIV":33569,"Ġreconcile":33570,"views":33571,"ĠDamon":33572,"vette":33573,"Flo":33574,"ĠGreatest":33575,"ilon":33576,"icia":33577,"Ġportrayal":33578,"Ġcushion":33579,"504":33580,"1979":33581,"ossal":33582,"Applic":33583,"scription":33584,"Ġmitigation":33585,"ATS":33586,"pac":33587,"Ġerased":33588,"Ġdeficiencies":33589,"ĠHollande":33590,"ĠXu":33591,"Ġbred":33592,"Ġpregnancies":33593,"femin":33594,"Ġemph":33595,"Ġplanners":33596,"Ġoutper":33597,"uttering":33598,"Ġperpetrator":33599,"Ġmotto":33600,"ĠEllison":33601,"ĠNEVER":33602,"Ġadmittedly":33603,"ARI":33604,"ĠAzerbaijan":33605,"Ġmillisec":33606,"Ġcombustion":33607,"ĠBottle":33608,"ĠLund":33609,"ĠPs":33610,"ĠDress":33611,"Ġfabricated":33612,"Ġbattered":33613,"Ġsidel":33614,"ĠNotting":33615,"Foreign":33616,"ĠJerome":33617,"020":33618,"ĠArbit":33619,"Ġknots":33620,"ĠRIGHT":33621,"Moving":33622,"ãģĻ":33623,"Ġsurgeries":33624,"Ġcourthouse":33625,"Ġmastered":33626,"Ġhovering":33627,"ĠBran":33628,"ĠAlison":33629,"Ġsafest":33630,"military":33631,"Ġbullied":33632,"Ġbarrage":33633,"Reader":33634,"ESE":33635,"ĠGeographic":33636,"Tools":33637,"314":33638,"ĠGeek":33639,"roth":33640,"glers":33641,"ĠFIN":33642,"Ïģ":33643,"ĠAston":33644,"altern":33645,"488":33646,"Ġveterin":33647,"Gamer":33648,"Ġintel":33649,"renches":33650,"Shield":33651,"Ġamnesty":33652,"ĠBhar":33653,"Ġpiled":33654,"Ġhonorable":33655,"ĠInstitutes":33656,"Ġsoaked":33657,"Ġcoma":33658,"ĠEFF":33659,"341":33660,"bytes":33661,"ĠGmail":33662,"lein":33663,"ĠCanadiens":33664,"material":33665,"Il":33666,"Ġinstructors":33667,"ĠKY":33668,"Ġconceive":33669,"ubb":33670,"ĠPossible":33671,"Ġeasing":33672,"ĠChristina":33673,"Ġcaric":33674,"ĠHDR":33675,"ROM":33676,"Ġshovel":33677,"delete":33678,"Ġpuff":33679,"ĠChanging":33680,"Ġseamlessly":33681,"Attribute":33682,"Ġacquisitions":33683,"akery":33684,"ĠEF":33685,"Ġautistic":33686,"ĠTakes":33687,"ĠPowder":33688,"ĠStir":33689,"510":33690,"ĠBubble":33691,"settings":33692,"ĠFowler":33693,"Ġmustard":33694,"Ġmoreover":33695,"Ġcopyrighted":33696,"ĠLEDs":33697,"1500":33698,"æī":33699,"ĠHIS":33700,"enf":33701,"Ġcustod":33702,"ĠHuck":33703,"Gi":33704,"Ġimg":33705,"Answer":33706,"Ct":33707,"jay":33708,"ĠInfrastructure":33709,"Ġfederally":33710,"Loc":33711,"Ġmicrobes":33712,"Ġoverrun":33713,"dds":33714,"otent":33715,"adiator":33716,">>>>>>>>":33717,"Ġtornado":33718,"Ġadjud":33719,"Ġintrigued":33720,"Ġsi":33721,"ĠRevelation":33722,"progress":33723,"Ġburglary":33724,"ĠSaiyan":33725,"ĠKathy":33726,"Ġserpent":33727,"ĠAndreas":33728,"Ġcompel":33729,"essler":33730,"ĠPlastic":33731,"ĠAdvent":33732,"ĠPositive":33733,"ĠQt":33734,"ĠHindus":33735,"registered":33736,"ularity":33737,"Ġrighteousness":33738,"Ġdemonic":33739,"uitive":33740,"ĠBDS":33741,"ĠGregg":33742,"cia":33743,"ĠCrusade":33744,"ĠSinai":33745,"WARE":33746,"+(":33747,"Ġmell":33748,"Ġderail":33749,"yards":33750,"Ast":33751,"Ġnoticeably":33752,"ĠOber":33753,"Ram":33754,"Ġunnoticed":33755,"Ġseq":33756,"avage":33757,"Ts":33758,"Ġ640":33759,"Ġconcede":33760,"Ġ])":33761,"Fill":33762,"Ġcaptivity":33763,"ĠImprovement":33764,"ĠCrusader":33765,"araoh":33766,"MAP":33767,"æĹ":33768,"Ġstride":33769,"always":33770,"Fly":33771,"Nit":33772,"Ġalgae":33773,"ĠCooking":33774,"ĠDoors":33775,"Malley":33776,"Ġpolicemen":33777,"ãģį":33778,"Ġastronaut":33779,"accessible":33780,"495":33781,"ĠRAW":33782,"cliffe":33783,"udicrous":33784,"Ġdepended":33785,"alach":33786,"Ġventures":33787,"rake":33788,"Ġtits":33789,"ĠHou":33790,"Ġcondom":33791,"ormonal":33792,"Ġindent":33793,"Ġuploading":33794,"Footnote":33795,"Important":33796,"Ġ271":33797,"Ġmindful":33798,"Ġcontends":33799,"Cra":33800,"Ġcalibr":33801,"ĠOECD":33802,"plugin":33803,"Fat":33804,"ĠISS":33805,"ĠDynamics":33806,"ansen":33807,"686":33808,"'),":33809,"Ġsprite":33810,"Ġhandheld":33811,"ĠHipp":33812,"=~=~":33813,"Trust":33814,"Ġsemantics":33815,"ĠBundes":33816,"ĠReno":33817,"ĠLiterature":33818,"sense":33819,"Gary":33820,"ĠAeg":33821,"ĠTrin":33822,"EEK":33823,"Ġcleric":33824,"ĠSSH":33825,"Ġchrist":33826,"Ġinvading":33827,"ibu":33828,"Ġenum":33829,"aura":33830,"Ġallege":33831,"ĠIncredible":33832,"BBC":33833,"Ġthru":33834,"Ġsailed":33835,"Ġemulate":33836,"Ġinsecurity":33837,"Ġcrou":33838,"Ġaccommodations":33839,"Ġincompetent":33840,"Ġslips":33841,"ĠEarthqu":33842,"sama":33843,"ILLE":33844,"ĠiPhones":33845,"asaki":33846,"Ġbye":33847,"Ġard":33848,"Ġextras":33849,"Ġslaughtered":33850,"Ġcrowdfunding":33851,"resso":33852,"Ġfilib":33853,"ĠERROR":33854,"ĠTLS":33855,"egg":33856,"ĠItal":33857,"Ġenlist":33858,"ĠCatalonia":33859,"ĠScots":33860,"Ġsergeant":33861,"Ġdissolve":33862,"NH":33863,"Ġstandings":33864,"rique":33865,"IQ":33866,"Ġbeneficiary":33867,"Ġaquarium":33868,"YouTube":33869,"ĠPowerShell":33870,"Ġbrightest":33871,"ĠWarrant":33872,"Sold":33873,"Writing":33874,"Ġbeginnings":33875,"ĠReserved":33876,"ĠLatinos":33877,"heading":33878,"Ġ440":33879,"Ġrooftop":33880,"ATING":33881,"Ġ390":33882,"VPN":33883,"Gs":33884,"kernel":33885,"turned":33886,"Ġpreferable":33887,"Ġturnovers":33888,"ĠHels":33889,"Sa":33890,"ĠShinji":33891,"veh":33892,"ĠMODULE":33893,"Viol":33894,"Ġexiting":33895,"Ġjab":33896,"ĠVanilla":33897,"Ġacron":33898,"ĠGap":33899,"bern":33900,"Ak":33901,"ĠMcGu":33902,"Ġendlessly":33903,"ĠFarage":33904,"ĠNoel":33905,"Va":33906,"MK":33907,"Ġbrute":33908,"ĠKru":33909,"ĠESV":33910,"ĠOlivia":33911,"âĢł":33912,"ĠKaf":33913,"Ġtrusting":33914,"Ġhots":33915,"324":33916,"Ġmalaria":33917,"Ġjson":33918,"Ġpounding":33919,"ortment":33920,"Country":33921,"Ġpostponed":33922,"Ġunequiv":33923,"?),":33924,"ĠRooney":33925,"udding":33926,"ĠLeap":33927,"urrence":33928,"shapeshifter":33929,"ĠHAS":33930,"osate":33931,"Ġcavern":33932,"Ġconservatism":33933,"ĠBAD":33934,"Ġmileage":33935,"Ġarresting":33936,"Vaults":33937,"Ġmixer":33938,"Democratic":33939,"ĠBenson":33940,"Ġauthored":33941,"8000":33942,"Ġproactive":33943,"ĠSpiritual":33944,"tre":33945,"Ġincarcerated":33946,"ĠSort":33947,"Ġpeaked":33948,"Ġwielding":33949,"reciation":33950,"×Ļ×":33951,"Patch":33952,"ĠEmmy":33953,"Ġexqu":33954,"tto":33955,"ĠRatio":33956,"ĠPicks":33957,"ĠGry":33958,"phant":33959,"Ġfret":33960,"Ġethn":33961,"Ġarchived":33962,"%-":33963,"cases":33964,"ĠBlaze":33965,"Ġimb":33966,"cv":33967,"yss":33968,"imony":33969,"Ġcountdown":33970,"Ġawakening":33971,"ĠTunisia":33972,"ĠRefer":33973,"ĠMJ":33974,"Ġunnatural":33975,"ĠCarnegie":33976,"izen":33977,"ĠNuggets":33978,"hess":33979,"Ġevils":33980,"647":33981,"Ġintroductory":33982,"loving":33983,"ĠMcMahon":33984,"Ġambiguity":33985,"Label":33986,"ĠAlmighty":33987,"Ġcoloring":33988,"ĠClaus":33989,"setting":33990,"NULL":33991,"ĠFavorite":33992,"ĠSIG":33993,">(":33994,"ĠShiva":33995,"ĠMayer":33996,"Ġstormed":33997,"ĠCoverage":33998,"weapons":33999,"igham":34000,"Ġunanswered":34001,"Ġleve":34002,"Ġcoy":34003,"cas":34004,"bags":34005,"asured":34006,"Seattle":34007,"ĠSantorum":34008,"serious":34009,"Ġcourageous":34010,"ĠSoup":34011,"Ġconfiscated":34012,"Ġ///":34013,"Ġunconventional":34014,"Ġmoms":34015,"ĠRohingya":34016,"ĠOrchestra":34017,"ĠPotion":34018,"Ġdiscredit":34019,"ĠFIL":34020,"fixed":34021,"ĠDeer":34022,"doi":34023,"ĠDimension":34024,"Ġbureaucrats":34025,"eteen":34026,"ĠactionGroup":34027,"ohm":34028,"Ġbumps":34029,"ĠUtility":34030,"Ġsubmarines":34031,"renheit":34032,"research":34033,"ĠShapiro":34034,"Ġsketches":34035,"Ġdeceptive":34036,"ĠVil":34037,"esame":34038,"ĠEssentially":34039,"Ġrampage":34040,"isky":34041,"Ġmuttered":34042,"thritis":34043,"Ġ236":34044,"fet":34045,"bars":34046,"Ġpupil":34047,"ĠThou":34048,"oS":34049,"song":34050,"Ġfractured":34051,"Ġrevert":34052,"picture":34053,"Ġcriterion":34054,"usher":34055,"Ġrepercussions":34056,"ĠVintage":34057,"ĠSuperintendent":34058,"Officers":34059,"Ġflagged":34060,"Ġblames":34061,"Ġinverse":34062,"ographers":34063,"Ġmakeshift":34064,"Ġdevoid":34065,"Ġfossils":34066,"ĠAristotle":34067,"ĠFunds":34068,"Ġdepleted":34069,"ĠFlu":34070,"ĠYuan":34071,"Ġwoes":34072,"Ġlipid":34073,"Ġsitu":34074,"requisites":34075,"Ġfurnish":34076,"ĠSamar":34077,"Ġshameful":34078,"Ġadversely":34079,"Ġadept":34080,"Ġremorse":34081,"Ġmurderous":34082,"uckles":34083,"ĠESL":34084,"Ġ314":34085,"sent":34086,"Ġredef":34087,"ĠCache":34088,"ĠPurs":34089,"igans":34090,"Ġ460":34091,"Ġprescriptions":34092,"Ġfres":34093,"Fuck":34094,"ocrates":34095,"Twenty":34096,"ĠWeird":34097,"ĠToggle":34098,"ĠCalled":34099,"itizens":34100,"Ġpoultry":34101,"Ġharvesting":34102,"ãĤ¦ãĤ¹":34103,"Bottom":34104,"Ġcautioned":34105,"tn":34106,"396":34107,"ĠNikki":34108,"Ġevaluations":34109,"Ġharassing":34110,"Ġbindings":34111,"ĠMonetary":34112,"Ġhitters":34113,"Ġadversary":34114,"unts":34115,"Ġsetback":34116,"Ġencrypt":34117,"ĠCait":34118,"Ġlows":34119,"enges":34120,"ĠNorn":34121,"Ġbulbs":34122,"Ġbottled":34123,"ĠVoyager":34124,"317":34125,"Ġspheres":34126,"politics":34127,"Ġsubtract":34128,"Ġsensations":34129,"Ġappalling":34130,"Ġ316":34131,"Ġenvironmentally":34132,"ĠSTEM":34133,"Ġpublishes":34134,"560":34135,"Ġdiligence":34136,"484":34137,"Ġadvises":34138,"Ġpetrol":34139,"Ġimagining":34140,"Ġpatrols":34141,"ĠInteger":34142,"ĠAshes":34143,"actus":34144,"ĠRadiant":34145,"ĠLT":34146,"itability":34147,"htaking":34148,"Setting":34149,"Ġnuanced":34150,"ĠReef":34151,"ĠDevelopers":34152,"Ni":34153,"pieces":34154,"990":34155,"License":34156,"Ġlowers":34157,"ĠOttoman":34158,"327":34159,"ooo":34160,"Ġquitting":34161,"markets":34162,"Behind":34163,"Ġbasin":34164,"Ġdocs":34165,"anie":34166,"flash":34167,"ctl":34168,"Ġcivilized":34169,"ĠFukushima":34170,"\"],\"":34171,"ĠKS":34172,"ĠHonestly":34173,"arat":34174,"Ġconstructs":34175,"ĠLans":34176,"ĠDire":34177,"ĠLIKE":34178,"ĠTrouble":34179,"Ġwithholding":34180,"ĠOblivion":34181,"Ġsanity":34182,"anya":34183,"Const":34184,"Ġgrocer":34185,"ĠCelsius":34186,"Ġrecounted":34187,"ĠWife":34188,"Border":34189,"atered":34190,"happy":34191,"Ġspoiler":34192,"Ġlogically":34193,"Hall":34194,"Ġsucceeding":34195,"Ġpolymorph":34196,"Ġaxes":34197,"ĠShotgun":34198,"ĠSlim":34199,"ĠPrinciples":34200,"ĠLeth":34201,"arta":34202,"Ġscor":34203,"Screenshot":34204,"Ġrelaxation":34205,"#$#$":34206,"Ġdeterrent":34207,"iddy":34208,"Ġpowerless":34209,"Ġlesbians":34210,"Ġchords":34211,"ĠEdited":34212,"selected":34213,"Ġseparatists":34214,"0002":34215,"Ġairspace":34216,"Ġturnaround":34217,"Ġcunning":34218,"PATH":34219,"Poly":34220,"Ġbombed":34221,"Ġtion":34222,"xs":34223,"Ġwithhold":34224,"Ġwaged":34225,"ĠLiberties":34226,"Flag":34227,"Ġcomforting":34228,"454":34229,"ĠIris":34230,"arers":34231,"Ġrag":34232,"Ġrelocated":34233,"ĠGuarant":34234,"Ġstrategically":34235,"Ġgamma":34236,"uberty":34237,"ĠLockheed":34238,"gres":34239,"Ġgrilled":34240,"ĠLowe":34241,"stats":34242,"ĠRocks":34243,"Ġsensing":34244,"Ġrenting":34245,"ĠGeological":34246,"اØ":34247,"otrop":34248,"Ġsew":34249,"Ġimproperly":34250,"486":34251,"Ġâĸł":34252,"Ġstarving":34253,"ĠBj":34254,"Discussion":34255,"328":34256,"ĠCombo":34257,"ĠFixes":34258,"NAT":34259,"Ġstriving":34260,"thora":34261,"Ġharvested":34262,"ĠPing":34263,"Ġplayful":34264,"Ġavenues":34265,"Ġoccupational":34266,"Ġwakes":34267,"ĠCourier":34268,"Ġdrummer":34269,"ĠBrowser":34270,"ĠHouth":34271,"itu":34272,"Ġapparel":34273,"paste":34274,"Ġhunted":34275,"ĠSecondly":34276,"lain":34277,"XY":34278,"ĠPIN":34279,"icons":34280,"Ġcocktails":34281,"Ġsizable":34282,"Ġhurdles":34283,"estinal":34284,"ĠRecreation":34285,"Ġeco":34286,"648":34287,"ĠDied":34288,"mint":34289,"Ġfingerprints":34290,"Ġdispose":34291,"ĠBosnia":34292,"tsy":34293,"2200":34294,"Ġinspected":34295,"ĠFou":34296,"Ġfuss":34297,"Ġambush":34298,"ĠRak":34299,"Ġmanifested":34300,"Prosecut":34301,"Ġsuffice":34302,"rences":34303,"Ġcompensated":34304,"ĠCyrus":34305,"Ġgenus":34306,"ĠWolverine":34307,"ĠTrends":34308,"Ġhikes":34309,"ĠSeen":34310,"Ġenrol":34311,"Cold":34312,"Ġpolitely":34313,"ĠSlav":34314,"ĠRupert":34315,"Ġeyewitness":34316,"ĠAlto":34317,"Ġuncomp":34318,"Ġposterior":34319,"Must":34320,"ĠHerz":34321,"Ġprogressively":34322,"Ġ234":34323,"Ġindifference":34324,"ĠCunningham":34325,"Ġacademia":34326,"Ġsewer":34327,"Ġastounding":34328,"ĠAES":34329,"rather":34330,"Ġeldest":34331,"Ġclimbs":34332,"ĠAdds":34333,"Ġoutcry":34334,"Ġcontag":34335,"ĠHouses":34336,"Ġpept":34337,"ĠMelania":34338,"interested":34339,"ĠUCH":34340,"ĠRoots":34341,"ĠHubbard":34342,"ĠTBD":34343,"ĠRomanian":34344,"filename":34345,"Stone":34346,"ĠImpl":34347,"Ġchromosome":34348,"Cle":34349,"dx":34350,"Ġscrambled":34351,"ĠPt":34352,"Ġ242":34353,"OPLE":34354,"Ġtremendously":34355,"Street":34356,"Ġcraving":34357,"Ġbundled":34358,"ĠRG":34359,"pipe":34360,"Ġinjuring":34361,"Ġarcane":34362,"Particip":34363,"ĠHeroic":34364,"sty":34365,"Ġtopping":34366,"ĠTempest":34367,"rentices":34368,"bh":34369,"Ġparanoia":34370,"ĠUnicode":34371,"Ġegregious":34372,"Ġ\\'":34373,"ĠOswald":34374,"Ġgravel":34375,"ĠSimpsons":34376,"Ġbland":34377,"ĠGuantanamo":34378,"Writer":34379,"liners":34380,"ĠDice":34381,"JC":34382,"Ġparity":34383,"Ġsided":34384,"Ġ237":34385,"ĠPyrrha":34386,"atters":34387,"dk":34388,"Fine":34389,"compan":34390,"Ġformulated":34391,"ĠIdol":34392,"ilers":34393,"hemoth":34394,"ĠFav":34395,"Ġintrusion":34396,"Ġcarrots":34397,"ĠLayer":34398,"ĠHacker":34399,"Ġ----------------":34400,"Ġmoderation":34401,"éģ":34402,"ococ":34403,"Ġcharacterize":34404,"ĠTeresa":34405,"Ġsocioeconomic":34406,"Ġperk":34407,"ĠParticipation":34408,"training":34409,"ĠPaulo":34410,"phys":34411,"Ġtrustworthy":34412,"Ġembodied":34413,"ĠMerch":34414,"currency":34415,"ĠPriority":34416,"Ġteasing":34417,"Ġabsorbing":34418,"Ġunfinished":34419,"ĠComparison":34420,"Ġdisple":34421,"writers":34422,"Ġprofessions":34423,"ĠPenguin":34424,"Ġangrily":34425,"ĠLINK":34426,"688":34427,"ĠCorrespond":34428,"Ġprevailed":34429,"Ġcartel":34430,"lp":34431,"asms":34432,"ĠRedemption":34433,"ĠIslamists":34434,"effects":34435,"dose":34436,"ĠLatter":34437,"ĠHalifax":34438,"Ġvas":34439,"ĠTopics":34440,"ĠNamed":34441,"advertising":34442,"zza":34443,"ICES":34444,"Ġretarded":34445,"achable":34446,"ĠPuppet":34447,"ĠItemLevel":34448,"Ġretract":34449,"Ġidentifiable":34450,"Aaron":34451,"ĠBuster":34452,"sol":34453,"helle":34454,"assemb":34455,"Hope":34456,"ranged":34457,"Ba":34458,"ĠPurch":34459,"éĢ":34460,"ĠSiri":34461,"Ġarrivals":34462,"Ġ1912":34463,"Ġshortened":34464,"Ġ312":34465,"Ġdiscrepancy":34466,"ĠTemperature":34467,"ĠWalton":34468,"Ġkinderg":34469,"polit":34470,"Ġremix":34471,"Ġconnectors":34472,"ãĥĺãĥ©":34473,"ĠKazakhstan":34474,"dominated":34475,"Ġsugars":34476,"imble":34477,"ĠPanic":34478,"ĠDemand":34479,"ĠColony":34480,"onen":34481,"ĠMER":34482,"775":34483,"uria":34484,"azaar":34485,"ĠDegree":34486,"Pri":34487,"Ġsunshine":34488,"Ġ251":34489,"Ġpsychedelic":34490,"Ġdigitally":34491,"ĠBraun":34492,"Ġshimmer":34493,"Ġshave":34494,"ĠTelesc":34495,"ĠAstral":34496,"ĠVenezuelan":34497,"ĠOG":34498,"Ġcrawling":34499,"Integ":34500,"ĠFeather":34501,"Ġunfolding":34502,"Ġappropriation":34503,"Ġè£ıè":34504,"ĠMobility":34505,"ĠNey":34506,"-.":34507,"bilt":34508,"LIN":34509,"ĠTube":34510,"ĠConversely":34511,"Ġkeyboards":34512,"ĠCao":34513,"Ġoverth":34514,"Ġlaure":34515,">>\\":34516,"ĠViper":34517,"acha":34518,"Offset":34519,"ĠRaleigh":34520,"ĠJae":34521,"Jordan":34522,"jp":34523,"Ġtotalitarian":34524,"Connector":34525,"Ġobserves":34526,"ĠSpartan":34527,"ĠImmediately":34528,"ĠScal":34529,"Cool":34530,"Ġtaps":34531,"Ġroar":34532,"Past":34533,"Ġchars":34534,"ĠBender":34535,"ĠSheldon":34536,"Ġpainter":34537,"Ġbeacon":34538,"ĠCreatures":34539,"Ġdownturn":34540,"Ġhinder":34541,"ĠAndromeda":34542,"ÃĽ":34543,"ccoli":34544,"ĠFitness":34545,"etrical":34546,"Ġutilizes":34547,"Ġsenate":34548,"Ġensemble":34549,"Ġcheers":34550,"TW":34551,"Ġaffluent":34552,"kil":34553,"rylic":34554,"ordering":34555,"Computer":34556,"Ġgruesome":34557,"ostics":34558,"ĠUbisoft":34559,"ĠKelley":34560,"Ġwrench":34561,"Ġbourgeoisie":34562,"IBLE":34563,"ĠPreston":34564,"worn":34565,"arist":34566,"reating":34567,"Ġstained":34568,"arine":34569,"Ġslime":34570,"ENN":34571,"Ġchests":34572,"Ġgroundwater":34573,"annot":34574,"ĠTray":34575,"ĠLocke":34576,"ĠCTR":34577,"Ġdudes":34578,"ĠExternal":34579,"ĠDecoder":34580,"Ġparamed":34581,"ĠMedline":34582,"809":34583,"ĠDinner":34584,"rupal":34585,"gz":34586,"ĠGum":34587,"ĠDemo":34588,"jee":34589,"Ġdh":34590,"berman":34591,"archs":34592,"Ġenqu":34593,"ĠEpstein":34594,"Ġdevastation":34595,"Ġfriendships":34596,"ĠArd":34597,"Ġ231":34598,"ĠRubin":34599,"ĠDistance":34600,"Ġspurred":34601,"Ġdossier":34602,"Ġoverlooking":34603,"\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\":34604,"Forest":34605,"ĠComes":34606,"\\\",":34607,"ĠIranians":34608,"Ġfixtures":34609,"Laughs":34610,"Ġcurry":34611,"ĠKingston":34612,"Ġsquash":34613,"Ġcatalogue":34614,"Ġabnormalities":34615,"Ġdigestive":34616,".........":34617,"Ġsubordinate":34618,"ogly":34619,"Ġ249":34620,"Middle":34621,"Ġmassac":34622,"Ġburgers":34623,"Ġdownstairs":34624,"Ġ1931":34625,"394":34626,"ĠVG":34627,"Ġlasers":34628,"ĠSikh":34629,"ĠAlexa":34630,"derived":34631,"Ġcyclist":34632,"ãģ®éŃĶ":34633,"oneliness":34634,"!!!!!!!!":34635,"Ġbuffs":34636,"legate":34637,"Ġraping":34638,"Ġrecommending":34639,"rored":34640,"Ġmulticultural":34641,"unique":34642,"Ġbusinessmen":34643,"Ġuneasy":34644,"ĠMAP":34645,"Ġdispersed":34646,"cipline":34647,"Jess":34648,"ĠKerala":34649,"å§":34650,"Ġabstraction":34651,"Surv":34652,"Uh":34653,"Ġprinters":34654,"ija":34655,"owder":34656,"Ġanalogous":34657,"ĠASP":34658,"afer":34659,"Ġunfolded":34660,"Ġleveling":34661,"Ġbreached":34662,"ĠHearing":34663,"Ġnat":34664,"Ġtranslating":34665,"critical":34666,"Ġantagonist":34667,"ĠYesterday":34668,"Ġfuzzy":34669,"wash":34670,"mere":34671,"Ġbewild":34672,"ĠMae":34673,"Virgin":34674,"phrase":34675,"Ġsignaled":34676,"ĠHIGH":34677,"Ġprotester":34678,"Ġgarner":34679,"unknown":34680,"Ġkay":34681,"Ġabducted":34682,"Ġstalking":34683,"amn":34684,"Ġdeserving":34685,"ĠRiv":34686,"ĠJorge":34687,"Ġscratching":34688,"ĠSaving":34689,"iping":34690,"Ġtease":34691,"Ġmissionary":34692,"ĠMorrow":34693,"TIME":34694,"Present":34695,"Ġchemotherapy":34696,"terness":34697,"ĠHomes":34698,"ĠPurdue":34699,"Ġstaunch":34700,"ĠWhitney":34701,"ĠTHERE":34702,"μ":34703,"iatus":34704,"ĠErnest":34705,"ĠDeploy":34706,"Ġcoveted":34707,"FML":34708,"ĠDialogue":34709,"Ġexited":34710,"fruit":34711,"Ġnerd":34712,"\":\"\",\"":34713,"Ġvivo":34714,"ruly":34715,"460":34716,"ĠAmen":34717,"rehensible":34718,"Ġâĺ":34719,"DIR":34720,"Ġadherence":34721,"Ġchew":34722,"ĠCoke":34723,"ĠSergei":34724,"digital":34725,"ĠNeck":34726,"gently":34727,"enthal":34728,"/)":34729,"Ġweary":34730,"Ġguise":34731,"ĠConcord":34732,"ĠOnion":34733,"atcher":34734,"Ġbinge":34735,"ĠDirective":34736,"Ġmanned":34737,"ansk":34738,"Ġillusions":34739,"Ġbillionaires":34740,"383":34741,"olyn":34742,"odynamic":34743,"ĠWheat":34744,"ĠAlic":34745,"Ġcoloured":34746,"ĠNAFTA":34747,"abo":34748,"Ġmacros":34749,"independent":34750,"sweet":34751,"Ġspac":34752,"ĠKabul":34753,"ĠÄ":34754,"eme":34755,"Ġdictated":34756,"Ġshouts":34757,"={":34758,"Ġripping":34759,"ĠShay":34760,"ĠCricket":34761,"directed":34762,"Ġanalysed":34763,"ĠWARRANT":34764,"agons":34765,"ĠBlazers":34766,"Ġcheered":34767,"Ġarithmetic":34768,"ĠTanz":34769,"373":34770,"ĠFlags":34771,"Ġ295":34772,"Ġwitches":34773,"ĠIncluded":34774,"ĠGained":34775,"ĠBlades":34776,"Gam":34777,"ĠSamantha":34778,"ĠAtlantis":34779,"ĠPratt":34780,"Ġspoiled":34781,"ĠIB":34782,"ĠRamirez":34783,"Probably":34784,"rero":34785,"ĠNg":34786,"ĠWarlock":34787,"tp":34788,"Ġoverhe":34789,"Ġadministrations":34790,"Ġtint":34791,"Ġregiment":34792,"Ġpistols":34793,"Ġblankets":34794,"Ġepist":34795,"Ġbowls":34796,"Ġhydraulic":34797,"Ġdean":34798,"Ġjung":34799,"Ġascend":34800,"705":34801,"ĠSantiago":34802,"î":34803,"Ġunavoid":34804,"ĠShaman":34805,"reb":34806,"Ġstemming":34807,"998":34808,"ĠMG":34809,"sticks":34810,"esthesia":34811,"ERO":34812,"Ġmorbid":34813,"ĠGrill":34814,"ĠPoe":34815,"anyl":34816,"Ġdeleting":34817,"ĠSurveillance":34818,"Ġdirectives":34819,"Ġiterations":34820,"ĠRox":34821,"ĠMilky":34822,"Father":34823,"Ġpatented":34824,"447":34825,"Ġprecursor":34826,"Ġmaiden":34827,"ĠPhen":34828,"ĠVegan":34829,"ĠPatent":34830,"Kelly":34831,"Redditor":34832,"Ġnods":34833,"Ġventilation":34834,"ĠSchwarz":34835,"Ġwizards":34836,"Ġominous":34837,"ĠHeads":34838,"ĠBG":34839,"Ġlumber":34840,"ĠSpiel":34841,"ĠisEnabled":34842,"Ġancestral":34843,"ĠShips":34844,"Ġwrestler":34845,"phi":34846,"Ġyuan":34847,"ĠRebellion":34848,"Ġiceberg":34849,"Ġmagically":34850,"Ġdiversion":34851,"arro":34852,"ythm":34853,"ĠRiders":34854,"ĠRobbie":34855,"ĠKara":34856,"ĠMaintenance":34857,"ĠHerb":34858,"Ġharms":34859,"packed":34860,"ĠFeinstein":34861,"Ġmarrying":34862,"Ġblending":34863,"ĠRates":34864,"Ġ1880":34865,"Ġwrink":34866,"ĠUnch":34867,"ĠTorch":34868,"described":34869,"Ġhumanoid":34870,"ilitating":34871,"ĠConv":34872,"ĠFeld":34873,"IGHTS":34874,"Ġwhistleblower":34875,"ortmund":34876,"etsy":34877,"arrett":34878,"ĠMono":34879,"ĠIke":34880,"ĠCNBC":34881,"ĠWAY":34882,"ĠMDMA":34883,"ĠIndividuals":34884,"Ġsupplemental":34885,"Ġpowerhouse":34886,"ĠStru":34887,"Focus":34888,"aphael":34889,"ĠColleg":34890,"atti":34891,"ZA":34892,"Ġperenn":34893,"ĠSignature":34894,"ĠRodney":34895,"Ġcubes":34896,"iddled":34897,"ĠDante":34898,"ĠINV":34899,"ilingual":34900,"ĠCth":34901,"Ġsofa":34902,"Ġintimidate":34903,"ĠRoe":34904,"ĠDiplom":34905,"ĠCountries":34906,"ayson":34907,"Ġextradition":34908,"Ġdisabling":34909,"ĠCardiff":34910,"Ġmemorandum":34911,"ĠTrace":34912,"Ġ???":34913,"sector":34914,"ĠRouhani":34915,"ĠYates":34916,"ĠFreeze":34917,"Ġbladder":34918,"Motor":34919,"ĠPromise":34920,"antasy":34921,"Ġforeseeable":34922,"ĠCologne":34923,"container":34924,"ĠTrees":34925,"ĠGors":34926,"ĠSinclair":34927,"Ġbarring":34928,"keye":34929,"Ġslashed":34930,"ĠStatistical":34931,"éĩ":34932,"Ġâĸº":34933,"Allows":34934,"Ġhumility":34935,"Ġdrilled":34936,"ĠFurn":34937,"443":34938,"Ġsewage":34939,"Ġhomepage":34940,"Ġcourtyard":34941,"Ġvile":34942,"Ġsubsidiaries":34943,"ajo":34944,"directory":34945,"Ġammon":34946,"Vers":34947,"charges":34948,"Ġ}}":34949,"ĠChains":34950,"Ġ246":34951,"nob":34952,"Ġpercept":34953,"Ġgrit":34954,"Ġfishermen":34955,"ĠIraqis":34956,"ĠDISTR":34957,"ĠFULL":34958,"ĠEvaluation":34959,"graph":34960,"atial":34961,"Ġcooperating":34962,"Ġmelan":34963,"Ġenlightened":34964,"Ġali":34965,"tailed":34966,"Ġsalute":34967,"Ġweakest":34968,"ĠBulldogs":34969,"UA":34970,"ĠAlloy":34971,"Ġsemen":34972,"ocene":34973,"ĠWilliamson":34974,"spr":34975,",âĢĶ":34976,"ĠGF":34977,"ittens":34978,"Beat":34979,"ĠJunk":34980,"iphate":34981,"ĠFarmers":34982,"ĠBitcoins":34983,"igers":34984,"dh":34985,"ĠLoyal":34986,"payer":34987,"Ġentertained":34988,"Ġpenned":34989,"Ġcoupon":34990,"Queue":34991,"Ġweakening":34992,"carry":34993,"Ġunderestimate":34994,"Ġshootout":34995,"Ġcharismatic":34996,"ĠProcedure":34997,"Ġprudent":34998,"inances":34999,"Ġriches":35000,"Ġcortical":35001,"Ġstrides":35002,"Ġdrib":35003,"ĠOilers":35004,"540":35005,"ĠPerform":35006,"ĠBangkok":35007,"Ġeuth":35008,"SER":35009,"Ġsimplistic":35010,"tops":35011,"campaign":35012,"Quality":35013,"Ġimpoverished":35014,"ĠEisenhower":35015,"Ġaugment":35016,"ĠHarden":35017,"Ġintervened":35018,"Ġlistens":35019,"ĠKok":35020,"Ġsage":35021,"Ġrubbish":35022,"ĠDed":35023,"Ġmull":35024,"pelling":35025,"Ġvideot":35026,"Production":35027,"DJ":35028,"miah":35029,"Ġadaptations":35030,"Ġmedically":35031,"Ġboarded":35032,"Ġarrogance":35033,"Ġscrapped":35034,"Ġoppress":35035,"FORMATION":35036,"Ġjunction":35037,"415":35038,"EEEE":35039,"Skill":35040,"Ġsubdu":35041,"ĠSuggest":35042,"ĠPett":35043,"Ġlett":35044,"ĠManip":35045,"ĠCaf":35046,"ĠCooperation":35047,"Ther":35048,"Ġregained":35049,"¶æ":35050,"reflect":35051,"Ġthugs":35052,"ĠShelby":35053,"Ġdictates":35054,"ĠWeiner":35055,"ĠHale":35056,"Ġbattleground":35057,"schild":35058,"Ġcondol":35059,"hunt":35060,"ositories":35061,"Ġaccuses":35062,"Filename":35063,"Ġshri":35064,"Ġmotivate":35065,"Ġreflections":35066,"Null":35067,"ĠLobby":35068,"¥µ":35069,"ĠSATA":35070,"ĠBackup":35071,"Ñĥ":35072,"nin":35073,"ĠCorrection":35074,"Ġjuicy":35075,"utra":35076,"ĠPric":35077,"Ġrestraining":35078,"ĠAirbnb":35079,"ĠArrest":35080,"Ġappropriations":35081,"Ġslopes":35082,"Ġmanslaughter":35083,"Ġworkings":35084,"ĠHuss":35085,"ĠFrey":35086,"Leave":35087,"ĠHarmony":35088,"ĠFeder":35089,"Ġ430":35090,"Ġtrench":35091,"Ġgladly":35092,"Ġbullpen":35093,"ĠGau":35094,"bones":35095,"Ġgroove":35096,"Ġpretext":35097,"ãħĭ":35098,"Ġtransmitter":35099,"ĠComponent":35100,"Ġunderage":35101,"ĠEmpires":35102,"Tile":35103,"Ġoy":35104,"ĠMarvin":35105,"ĠCAS":35106,"Ġbloss":35107,"Ġreplicated":35108,"ĠMariners":35109,"Marcus":35110,"ĠBlocks":35111,"Ġliberated":35112,"Ġbutterfly":35113,"Feel":35114,"Ġfermentation":35115,"Ġyoutube":35116,"Ġoffend":35117,"ĠTerm":35118,"resist":35119,"Ġcessation":35120,"Ġinsurgency":35121,"Ġbir":35122,"ĠRaise":35123,"595":35124,"Ġhypotheses":35125,"502":35126,"Ġplaque":35127,"ocrat":35128,"Ġjackets":35129,"ĠHuffPost":35130,"among":35131,"Ġconfer":35132,"487":35133,"ĠLilly":35134,"Ġadapting":35135,"ĠFay":35136,"Ġshoved":35137,"vec":35138,"Ġrefine":35139,"Ġgon":35140,"Ġgunmen":35141,"zai":35142,"ĠShuttle":35143,"ĠIzan":35144,"Ġ1913":35145,"Ġplethora":35146,"··":35147,"Ġ510":35148,"Ġpuberty":35149,"Ġ241":35150,"ĠWealth":35151,"ĠAlma":35152,"ĠMEM":35153,"ĠAdults":35154,"Cas":35155,"prison":35156,"Race":35157,"Ġwaterproof":35158,"Ġathleticism":35159,"Ġcapitalize":35160,"ĠJuice":35161,"Ġilluminated":35162,"ĠPascal":35163,"Ġirritation":35164,"ĠWitnesses":35165,"adle":35166,"ĠAstro":35167,"Ġfax":35168,"ĠElvis":35169,"Primary":35170,"ĠLich":35171,"ĠElves":35172,"Ġresiding":35173,"Ġstumble":35174,"319":35175,"ĠPKK":35176,"Ġadversaries":35177,"DOS":35178,"ĠRitual":35179,"Ġsmear":35180,"Ġarson":35181,"idental":35182,"Ġscant":35183,"Ġmonarchy":35184,"Ġhalftime":35185,"Ġresidue":35186,"Ġindign":35187,"ĠShaun":35188,"ĠElm":35189,"auri":35190,"Aff":35191,"WATCH":35192,"ĠLyon":35193,"helps":35194,"361":35195,"Ġlobbyist":35196,"Ġdiminishing":35197,"Ġoutbreaks":35198,"Ġgoats":35199,"favorite":35200,"ĠNah":35201,"sonian":35202,"ĠBooster":35203,"Ġsandbox":35204,"ĠFare":35205,"ĠMalta":35206,"ĠattRot":35207,"ĠMOR":35208,"lde":35209,"Ġnavigating":35210,"Touch":35211,"Ġuntrue":35212,"ĠDisaster":35213,"Ġludicrous":35214,"Password":35215,"ĠJFK":35216,"blogspot":35217,"416":35218,"ĠUNDER":35219,"ernal":35220,"Ġdelaying":35221,"TOP":35222,"Ġimplants":35223,"ĠAVG":35224,"ĠHuge":35225,"attr":35226,"Ġjournalistic":35227,"ĠPeyton":35228,"ĠIA":35229,"Rap":35230,"goal":35231,"ĠProgramme":35232,"Ġsmashing":35233,"wives":35234,"println":35235,"ĠPlague":35236,"inus":35237,"EEP":35238,"Ġcruiser":35239,"ĠParish":35240,"uminium":35241,"Ġoccupants":35242,"ĠJihad":35243,"mop":35244,"Ġpint":35245,"Ġhect":35246,"ĠMecca":35247,"director":35248,"ĠFunding":35249,"ĠMixed":35250,"Ġstag":35251,"Tier":35252,"Ġgust":35253,"Ġbrightly":35254,"orsi":35255,"Ġuphill":35256,"RD":35257,"Ġlesions":35258,"ĠBundy":35259,"livious":35260,"Ġbiologist":35261,"ĠFaculty":35262,"ĠAuthorization":35263,"Ġ244":35264,"Allow":35265,"ï¸":35266,"ĠGiul":35267,"Ġpertinent":35268,"otaur":35269,"esse":35270,"ĠRoof":35271,"Ġunmanned":35272,"351":35273,"ĠShak":35274,"ĠOrient":35275,"Ġendanger":35276,"Dir":35277,"Ġreplen":35278,"edient":35279,"Ġtailor":35280,"Ġgadgets":35281,"Ġaudible":35282,"âĺĨ":35283,"Nice":35284,"Ġbombard":35285,"ĠRape":35286,"Ġdefiance":35287,"ĠTWO":35288,"ĠFilipino":35289,"Ġunaffected":35290,"ervatives":35291,"Ġsoared":35292,"ĠBolton":35293,"Ġcompromising":35294,"ĠBrewers":35295,"RAL":35296,"ĠAHL":35297,"icycle":35298,"Ġvampires":35299,"Ġdipped":35300,"oyer":35301,"ĠXIII":35302,"Ġsideways":35303,"ĠWaste":35304,"ĠDiss":35305,"ĠâĶľâĶĢâĶĢ":35306,"$.":35307,"Ġhabitats":35308,"ĠBeef":35309,"truth":35310,"trained":35311,"split":35312,"Rus":35313,"Andy":35314,"ĠBram":35315,"REP":35316,"pid":35317,"è£ħ":35318,"ĠMutant":35319,"Anim":35320,"ĠMarina":35321,"Ġfutile":35322,"highest":35323,"frequency":35324,"Ġepilepsy":35325,"Ġcoping":35326,"Ġconcise":35327,"Ġtracing":35328,"ĠSUN":35329,"panel":35330,"ĠSophie":35331,"ĠCrowley":35332,"ĠAdolf":35333,"ĠShooter":35334,"Ġshaky":35335,"ĠIG":35336,"ĠLies":35337,"ĠBarber":35338,"pkg":35339,"Ġuptake":35340,"Ġpredatory":35341,"ULTS":35342,"/**":35343,"Ġintoxicated":35344,"ĠWestbrook":35345,"odder":35346,"hement":35347,"Ġbaseman":35348,"APD":35349,"storage":35350,"ĠFifty":35351,"editor":35352,"GEN":35353,"UTION":35354,"irting":35355,"Ġsewing":35356,"rift":35357,"Ġagony":35358,"ĠSands":35359,"Ġ254":35360,"Cash":35361,"Ġlodge":35362,"Ġpunt":35363,"Natural":35364,"ĠIdeas":35365,"Ġerroneous":35366,"ĠSensor":35367,"ĠHannity":35368,"Ġ1921":35369,"Ġmould":35370,"ĠGon":35371,"kaya":35372,"Ġanonymously":35373,"ĠKEY":35374,"Ġsimulator":35375,"Winter":35376,"Ġstreamed":35377,"507":35378,"?\",":35379,"Ġteased":35380,"Ġcoefficient":35381,"Ġwartime":35382,"ĠTHR":35383,"''.":35384,"ĠBanking":35385,"mpire":35386,"Ġfandom":35387,"Ġlia":35388,"Ga":35389,"Ġdownhill":35390,"Ġinterpreting":35391,"Individual":35392,"Norm":35393,"Ġjealousy":35394,"bitcoin":35395,"Ġpleasures":35396,"ĠToys":35397,"ĠChevrolet":35398,"ĠAdvisor":35399,"IZE":35400,"Ġreceptions":35401,"706":35402,"Cro":35403,"Ġ262":35404,"Ġcitrus":35405,"iru":35406,"Reviewer":35407,"jected":35408,"UES":35409,"anz":35410,"1981":35411,"ĠWorker":35412,"Ġcomplied":35413,"orescent":35414,"continental":35415,"Ton":35416,"ĠPrism":35417,"ĠSheep":35418,"Ġ288":35419,"nox":35420,"ĠVog":35421,"Ord":35422,"Ġrealms":35423,"tek":35424,"Ġirrigation":35425,"Ġbicycles":35426,"Ġelectronically":35427,"poly":35428,"tall":35429,"());":35430,"Ġaesthetics":35431,"ĠIntegrated":35432,"Explore":35433,"Ġdunk":35434,"476":35435,"pain":35436,"ĠJacques":35437,"ĠDmit":35438,"Frames":35439,"Ġreunited":35440,"Ġhumid":35441,"Dro":35442,"Political":35443,"Ġyouthful":35444,"Ġentails":35445,"Ġmosquito":35446,"363":35447,"species":35448,"Ġcoordinating":35449,"ĠMayhem":35450,"ĠMagnus":35451,"Mount":35452,"Improved":35453,"ĠSTATE":35454,"ATTLE":35455,"Ġflowed":35456,"Ġtackled":35457,"Ġfashioned":35458,"Ġreorgan":35459,"ivari":35460,"finger":35461,"Ġreluctantly":35462,"etting":35463,"ĠVand":35464,"young":35465,"ĠGarland":35466,"Ġpresumption":35467,"Ġamenities":35468,"ĠPleasant":35469,"onential":35470,"ĠOxy":35471,"Ġmorals":35472,"ĠYah":35473,"Ready":35474,"Simon":35475,"Enh":35476,"Demon":35477,"Ġclich":35478,"Monitor":35479,"ĠDU":35480,"Ġwelcomes":35481,"Ġstandout":35482,"Ġdreadful":35483,"Ġbananas":35484,"Ġballoons":35485,"hooting":35486,"basic":35487,"Ġsuffix":35488,"Ġduly":35489,"cano":35490,"Chain":35491,"atos":35492,"Ġgeopolitical":35493,"Ġ(&":35494,"ĠGemini":35495,"ÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤÃĥÃĤ":35496,"Ġacquitted":35497,"Luck":35498,"protect":35499,"1024":35500,"Ġscarcity":35501,"Ġmindfulness":35502,"ecided":35503,"DN":35504,"prime":35505,"ĠPresidents":35506,"ĠVIDEO":35507,"Ġ(âĪĴ":35508,"addock":35509,"NOR":35510,"ĠPru":35511,"pun":35512,"ĠLOL":35513,"))))":35514,"ĠLiqu":35515,"ĠSAS":35516,"Ġstyling":35517,"Ġpunishments":35518,"Ġnumb":35519,"Ġascertain":35520,"ĠRockies":35521,"flu":35522,"Thumbnail":35523,"Ġperpetrated":35524,"ĠSemi":35525,"Ġdisarm":35526,"ĠOlder":35527,"ĠException":35528,"Ġexponentially":35529,"ĠCommunities":35530,"Ġabolish":35531,"ĠPartner":35532,"ptoms":35533,"Ġ777":35534,"ĠFoley":35535,"ĠCases":35536,"Ġgrease":35537,"ĠRebirth":35538,"Ground":35539,"Ġ;)":35540,"ĠDoctrine":35541,"ikini":35542,"Ye":35543,"ĠBlossom":35544,"Ġpersists":35545,"bill":35546,"Ġinfusion":35547,"Ġbuddies":35548,"911":35549,"ĠPatient":35550,"Ġdemos":35551,"Ġacquaintance":35552,"ĠPaw":35553,"atari":35554,"Ġxml":35555,"Ġfascination":35556,"ĠServe":35557,"ÏĤ":35558,"branded":35559,"Ġaz":35560,"Returns":35561,"Ġovershadow":35562,"Ġroam":35563,"Ġspeedy":35564,"numbered":35565,"helial":35566,"Ġdisciple":35567,"Ġassurances":35568,"given":35569,"pecting":35570,"ĠNatalie":35571,"çͰ":35572,"Ġmosquitoes":35573,"rotein":35574,"Ġnumeric":35575,"Ġindependents":35576,"Ġtransitional":35577,"Ġreactionary":35578,"ĠMechdragon":35579,"doctor":35580,"Ġshortest":35581,"Ġsequential":35582,"ĠBac":35583,"ĠAccounts":35584,"ãģĮ":35585,"achy":35586,"ractive":35587,"ĠRegiment":35588,"Ġbreathtaking":35589,"fficiency":35590,"ĠBates":35591,"Ġ311":35592,"Ġwardrobe":35593,"fts":35594,"ĠBerk":35595,"Simply":35596,"ĠRiverside":35597,"ivering":35598,"idential":35599,"lucent":35600,"Ġenriched":35601,"ĠConver":35602,"ĠGiving":35603,"ãĥĻ":35604,"Ġlegalize":35605,"ĠFTC":35606,"Ġfreaking":35607,"Mix":35608,"Ġterrestrial":35609,"esian":35610,"cients":35611,"Wing":35612,"LOAD":35613,"Ġledge":35614,"ĠViolent":35615,"ĠMetall":35616,"Ġ308":35617,"Ġsoutheastern":35618,"hetto":35619,"Meat":35620,"Ġslowdown":35621,"Ġretreated":35622,"Jeremy":35623,"endas":35624,"*****":35625,"eric":35626,"Ġreins":35627,"oppable":35628,"ĠHumanity":35629,"earances":35630,"rigan":35631,"Camera":35632,"Ġwaivers":35633,"soc":35634,"Ġalteration":35635,"transform":35636,"ĠCemetery":35637,"506":35638,"Ġindefinite":35639,"Ġstimulating":35640,"yg":35641,"603":35642,"ĠSop":35643,"Ġdescriptive":35644,"Phase":35645,"ĠEdmund":35646,"Ġpneumonia":35647,"ventus":35648,"Amb":35649,"Ġlaboratories":35650,"ĠExclusive":35651,"ugar":35652,"Were":35653,"Ġmalfunction":35654,"Ġhomosexuals":35655,"Ġ-------":35656,"uni":35657,"Ġturbines":35658,"ĠEquity":35659,"Du":35660,"Ġminded":35661,"ĠRH":35662,"ĠBlackhawks":35663,"Ġfeats":35664,"Ġ1700":35665,"repl":35666,"362":35667,"laden":35668,"Ġindispensable":35669,"lyss":35670,"tti":35671,"Ġreel":35672,"Ġdiverted":35673,"Ġlikeness":35674,"Ġsubscriptions":35675,"Ġfingert":35676,"Ġfilthy":35677,"destruct":35678,"draft":35679,"ĠBernardino":35680,"launch":35681,"Ġperplex":35682,"ĠSUM":35683,"carb":35684,"Ġsweater":35685,"ĠVenture":35686,"ĠJag":35687,"ĠCeleb":35688,"ĠVoters":35689,"Ġsteadfast":35690,"Ġathletics":35691,"ĠHanson":35692,"ĠDrac":35693,"Tracker":35694,"Ġcommend":35695,"ĠPresidency":35696,"ĠDID":35697,"informed":35698,"Ġwebpage":35699,"Pretty":35700,"Ġforcefully":35701,"ãĥĥãĤ¯":35702,"Ġrelocation":35703,"Ġsatire":35704,"âī":35705,"ĠSunderland":35706,"æĦ":35707,"Voice":35708,"????????":35709,"Ġinformant":35710,"Ġbowel":35711,"ĠUniform":35712,"Ġ...\"":35713,"Ġpurge":35714,"Ġpicnic":35715,"ĠUmb":35716,"ĠUPDATE":35717,"ĠSapphire":35718,"ĠStall":35719,"learn":35720,"Ġobjectively":35721,"Ġobliter":35722,"Ġloophole":35723,"Ġjourneys":35724,"Ġomission":35725,"Pros":35726,"ĠSidney":35727,"ploma":35728,"Ġsprayed":35729,"Ġguru":35730,"Ġtraitor":35731,"Ġtimet":35732,"Ġsnapping":35733,"ĠSevent":35734,"urnal":35735,"ĠUkip":35736,"Ġbowed":35737,"poral":35738,"liberal":35739,"Ros":35740,"Questions":35741,"iOS":35742,"Ġsummarize":35743,"STAT":35744,"Ġ1850":35745,"apest":35746,"Ġlender":35747,"ĠVariable":35748,"bringing":35749,"ĠLORD":35750,",)":35751,"Ġcollapses":35752,"xiety":35753,"ĠNed":35754,"YD":35755,"ĠScha":35756,"Ġantibody":35757,"Ġdisband":35758,"yre":35759,"illusion":35760,"Ġrover":35761,"shed":35762,"ĠHirosh":35763,"cci":35764,"Ġcalam":35765,"ĠMorton":35766,"Pinterest":35767,"Ġ1928":35768,"ĠEuras":35769,"ordes":35770,"Ġfences":35771,"ĠInventory":35772,"ĠValencia":35773,"ĠUd":35774,"ĠTiff":35775,"Ġsque":35776,"Ġquotation":35777,"Ġtroublesome":35778,"erker":35779,"QUEST":35780,"ĠKingdoms":35781,"south":35782,"Ġlevy":35783,"Prince":35784,"ĠSting":35785,"Ġnicknamed":35786,"Ġappe":35787,"Ġphotographic":35788,"Ġcorpus":35789,"reference":35790,"ĠTrog":35791,"Unt":35792,")=(":35793,"ĠLatvia":35794,"Ġactivating":35795,"Ġlicensee":35796,"Ġdisparities":35797,"ĠNewsletter":35798,"ãĥĥãĥĪ":35799,"Ġfreeing":35800,"ĠJeep":35801,"ĠPerception":35802,"insk":35803,"Ġsilicone":35804,"ĠHayden":35805,"Lean":35806,"ĠSuzuki":35807,"ibrarian":35808,"668":35809,"Ġspor":35810,"Ġcorrelations":35811,"aghetti":35812,"Ġtuber":35813,"ĠIPCC":35814,"ilus":35815,"ĠVu":35816,"Ġwealthiest":35817,"ĠCarbuncle":35818,"anza":35819,"Ġfooled":35820,"ĠZur":35821,"Ġdaddy":35822,"rano":35823,"ilian":35824,"Ġknockout":35825,"fman":35826,"required":35827,"ĠWikileaks":35828,"ĠDuffy":35829,"ONT":35830,"Ġinsol":35831,"ĠObjects":35832,"Ġbou":35833,"ĠNordic":35834,"ĠInsert":35835,"scan":35836,"Ġdancers":35837,"Ġidiots":35838,"majority":35839,"ĠNeville":35840,"ĠFreeBSD":35841,"Ġtart":35842,"panic":35843,"690":35844,"Ġcocoa":35845,"Ġsampled":35846,"Ġlookup":35847,"Indust":35848,"Ġinjections":35849,"genre":35850,"Ġau":35851,"Ġroadway":35852,"Ġgenitals":35853,"Kind":35854,"ĠExaminer":35855,"ĠYaz":35856,"Fresh":35857,"Ġparalysis":35858,"ĠAluminum":35859,"Ġreap":35860,"oké":35861,"Ġsloppy":35862,"ĠTunnel":35863,"posium":35864,"nery":35865,"enic":35866,"Ġherbal":35867,"ĠOuter":35868,"ĠBuilder":35869,"Ġincur":35870,"Ġideologies":35871,"Ġbackups":35872,"consuming":35873,"ĠDetect":35874,"deck":35875,"ĠKNOW":35876,"ĠGret":35877,"ĠMIC":35878,"Ġtoughness":35879,"ĠExhibit":35880,"Ġhive":35881,"Les":35882,"ĠSCHOOL":35883,"ĠAtari":35884,"alde":35885,"ĠNull":35886,"andestine":35887,"mouse":35888,"Ġbrigade":35889,"489":35890,"Ġrevol":35891,"ĠLawson":35892,"ĠWah":35893,"opoly":35894,"ebted":35895,"ĠSaunders":35896,"Ġ313":35897,"ĠWinc":35898,"Ġtaboo":35899,"ĠHelmet":35900,"Ġwedge":35901,"chip":35902,"ĠTina":35903,"bg":35904,"Ġinfuri":35905,"rn":35906,"Ġanomalies":35907,"ĠSync":35908,"ĠExam":35909,"ĠCommit":35910,"ĠDiary":35911,"ĠALSO":35912,"ĠDebor":35913,"omedical":35914,"Ġcomprehension":35915,"655":35916,"Ġempowering":35917,"Ġire":35918,"Ġjuices":35919,"ĠETH":35920,"ĠBoxing":35921,"=\"/":35922,"Ġfacilitated":35923,"poke":35924,"ĠParsons":35925,"ĠModer":35926,"travel":35927,"Ġcivilizations":35928,"Ġlibertarians":35929,"Ġrune":35930,"ĠClarks":35931,"athed":35932,"Ġcampaigners":35933,"ĠDispatch":35934,"ĠFahrenheit":35935,"ĠCapcom":35936,"----------":35937,"Ġlace":35938,"Ġdraining":35939,"Ġliner":35940,"ĠArtificial":35941,"én":35942,"task":35943,"]).":35944,"ĠGMO":35945,"ĠOperator":35946,"ordinary":35947,"ĠInfluence":35948,"ĠUps":35949,"Ġpotency":35950,"ussen":35951,"ospons":35952,"ĠSwim":35953,"ĠDeadline":35954,"Unity":35955,"Ġculinary":35956,"Ġenlightenment":35957,"Ġwearer":35958,"Ġmined":35959,"Ġply":35960,"Ġincest":35961,"ĠDVDs":35962,"Walk":35963,"BTC":35964,"Trade":35965,"Ġdeval":35966,"iband":35967,"ĠOversight":35968,"Palestinian":35969,"Ġdart":35970,"Ġmul":35971,"LR":35972,"Ġremovable":35973,"ĠRealms":35974,"ìĿ":35975,"Ġmiscar":35976,"ĠVulkan":35977,"685":35978,"ère":35979,"ĠSap":35980,"Ġmerging":35981,"ĠCarly":35982,"chester":35983,"Ġbrisk":35984,"Ġluxurious":35985,"ĠGenerator":35986,"Ġbitterness":35987,"Ġedible":35988,"Ġ243":35989,"TG":35990,"Ġrectangle":35991,"WithNo":35992,"below":35993,"Jenn":35994,"Ġdarkest":35995,"Ġhitch":35996,"Ġdosage":35997,"Ġscaven":35998,"ĠKeller":35999,"ĠIllustrated":36000,"Certainly":36001,"ĠMavericks":36002,"Marginal":36003,"Ġdiarrhea":36004,"Ġenormously":36005,"Ġ999":36006,"shr":36007,"quart":36008,"Ġadamant":36009,"ĠMew":36010,"Ġrenovation":36011,"Ġcervical":36012,"ĠPercentage":36013,"eners":36014,"ĠKimber":36015,"Ġfloats":36016,"Ġdex":36017,"ĠWitcher":36018,"ĠSwansea":36019,"dm":36020,"Ġsalty":36021,"yellow":36022,"Ġcape":36023,"ĠDrain":36024,"ĠPaula":36025,"ĠToledo":36026,"lesi":36027,"Magazine":36028,"ĠWick":36029,"ĠMn":36030,"ĠAck":36031,"ĠRiding":36032,"ASON":36033,"Ġhomophobic":36034,"ARP":36035,"Ġwandered":36036,"CPU":36037,"oodoo":36038,"ĠPipe":36039,"Ġtightening":36040,"ĠButt":36041,"318":36042,"Ġdeserted":36043,"Session":36044,"Ġfacilitating":36045,"Jump":36046,"Ġemergencies":36047,"OWER":36048,"Ġexhaustive":36049,"ĠAFTER":36050,"Ġheartbeat":36051,"ĠLabel":36052,"acky":36053,"ĠCertified":36054,"iltration":36055,"Ze":36056,"ĠUtt":36057,"Ġ1300":36058,"Ġpresume":36059,"ĠDisp":36060,"Ġsurged":36061,"Ġdolls":36062,"Columb":36063,"Ġchimpan":36064,"ĠRazor":36065,"Ġticks":36066,"Ġcouncillor":36067,"Ġpilgrimage":36068,"ĠRebels":36069,"ĠQC":36070,"ĠAuction":36071,"xia":36072,"ikk":36073,"bred":36074,"Ġinsertion":36075,"Ġcoarse":36076,"dB":36077,"SEE":36078,"ĠZap":36079,"ĠFoo":36080,"Ġcontempor":36081,"ĠQuarterly":36082,"otions":36083,"ĠAlchemist":36084,"ĠTrey":36085,"ĠDuo":36086,"Sweet":36087,"804":36088,"ĠGiov":36089,"Ġfunn":36090,"Nin":36091,"hoff":36092,"Ġramifications":36093,"Ġ1922":36094,"ĠExperts":36095,"azes":36096,"Ġgarments":36097,"arial":36098,"ĠNab":36099,"Ġ257":36100,"ĠVed":36101,"Ġhumorous":36102,"ĠPompe":36103,"Ġnylon":36104,"Ġlurking":36105,"ĠSergey":36106,"ĠMattis":36107,"Ġmisogyny":36108,"ĠComponents":36109,"ĠWatching":36110,"ĠFolk":36111,"ractical":36112,"Bush":36113,"Ġtaped":36114,"Ġgrouping":36115,"Ġbeads":36116,"Ġ2048":36117,"Ġcondu":36118,"querque":36119,"Reading":36120,"Ġgrievances":36121,"Ultra":36122,"Ġendpoint":36123,"Hig":36124,"ĠStatic":36125,"ĠScarborough":36126,"Lua":36127,"ĠMessi":36128,"aqu":36129,"ĠPsyNet":36130,"ĠRudd":36131,"Ġavenue":36132,"vp":36133,"Jer":36134,"Ġshady":36135,"ĠResist":36136,"ĠArtemis":36137,"Ġcareless":36138,"Ġbrokers":36139,"Ġtemperament":36140,"Ġ520":36141,"Tags":36142,"ĠTurning":36143,"Ġuttered":36144,"Ġpedd":36145,"Ġimprovised":36146,"Ġ:(":36147,"Ġtabl":36148,"Ġplains":36149,"1600":36150,"pressure":36151,"ĠEssence":36152,"margin":36153,"friends":36154,"ĠRestoration":36155,"Ġpollut":36156,"ĠPoker":36157,"ĠAugustine":36158,"ĠCIS":36159,"ĠSEAL":36160,"orama":36161,"Ġthwart":36162,"seek":36163,"Ġpagan":36164,"º":36165,"cpu":36166,"Ġgarn":36167,"Ġassortment":36168,"ĠILCS":36169,"tower":36170,"Recommended":36171,"Ġunborn":36172,"ĠRandomRedditor":36173,"ĠRandomRedditorWithNo":36174,"Ġparalyzed":36175,"Ġeruption":36176,"Ġintersect":36177,"ĠStoke":36178,"ĠSco":36179,"Bind":36180,"å¾":36181,"ĠPNG":36182,"ĠNegative":36183,"ĠNOAA":36184,"Leon":36185,"Ġalloy":36186,"ĠLama":36187,"ĠDiversity":36188,"575":36189,"Ġunderestimated":36190,"ĠScor":36191,"Ġmural":36192,"Ġbusted":36193,"soon":36194,"lif":36195,"Ġnonex":36196,"Ġallergy":36197,"ĠUnderworld":36198,"ĠRays":36199,"ĠBlasio":36200,"Ġhrs":36201,"ĠDir":36202,"Ġ327":36203,"byter":36204,"Ġreplacements":36205,"Ġactivates":36206,"rived":36207,"MH":36208,"Ġpans":36209,"ĠHI":36210,"Ġlongitudinal":36211,"Ġnuisance":36212,"aler":36213,"Ġswell":36214,"ĠSigned":36215,"sci":36216,"ĠIsles":36217,"ĠAGA":36218,"Ġdefiant":36219,"Ġsonic":36220,"ocon":36221,"KC":36222,"ĠAim":36223,"tie":36224,"ahah":36225,"ĠmL":36226,"DX":36227,"Ġbisc":36228,"ĠBillboard":36229,"ĠSYSTEM":36230,"NEY":36231,"gaard":36232,"Ġdistressed":36233,"formerly":36234,"Alan":36235,"Ġchefs":36236,"Ġoptics":36237,"ĠComet":36238,"ĠAMC":36239,"Ġredesigned":36240,"irmation":36241,"Ġsightings":36242,"382":36243,"311":36244,"ĠWB":36245,"Ġcontraction":36246,"ĠTOTAL":36247,"Dual":36248,"Ġstartled":36249,"Ġunderstandably":36250,"Ġsunglasses":36251,"ETHOD":36252,"Ġdocker":36253,"Ġsurfing":36254,"ĠHEL":36255,"ĠSlack":36256,"tones":36257,"Ġshalt":36258,"Visual":36259,"498":36260,"Department":36261,"cussion":36262,"Ġunrestricted":36263,"Ġtad":36264,"Ġrename":36265,"employed":36266,"Ġeducating":36267,"Ġgrinned":36268,"bedroom":36269,"ĠActivities":36270,"ĠVelvet":36271,"ĠSWAT":36272,"Ġshuffle":36273,"igor":36274,"Ġsaturation":36275,"Finding":36276,"cream":36277,"icter":36278,"Ġvodka":36279,"tracking":36280,"tec":36281,"Ġforeground":36282,"iesta":36283,"Ġvehement":36284,"ĠECB":36285,"ĠTie":36286,"Ey":36287,"Ġturtles":36288,"ĠRailroad":36289,"ĠKatz":36290,"ĠFrames":36291,"Ġmenace":36292,"ĠFellowship":36293,"ĠEssential":36294,"uggish":36295,"Ġdrip":36296,"chwitz":36297,"ĠKyoto":36298,"sb":36299,"ĠNina":36300,"Parameter":36301,"Ġalarms":36302,"ĠClaud":36303,"Ġpioneering":36304,"Ġchiefly":36305,"ĠScream":36306,"Collection":36307,"Ġthankfully":36308,"ĠRonaldo":36309,"åŃIJ":36310,"strip":36311,"ĠDisneyland":36312,"commercial":36313,"Seeing":36314,"Soul":36315,"Ġevacuate":36316,"Ġciv":36317,"ĠAshe":36318,"Ġdivides":36319,"ĠDagger":36320,"rehensive":36321,"Ġberries":36322,"ĠDF":36323,"Ġsushi":36324,"Ġplurality":36325,"WI":36326,"Ġdisadvantaged":36327,"Ġbattalion":36328,"obiles":36329,"451":36330,"Ġcling":36331,"Ġundeniable":36332,"ĠLounge":36333,"Ġhaunt":36334,"phe":36335,"Ġquantify":36336,"Ġdiffered":36337,"Ġ[*]":36338,"ĠViz":36339,"cum":36340,"slave":36341,"Ġvideog":36342,"Ġquar":36343,"Ġbundles":36344,"ĠAlonso":36345,"tackle":36346,"Ġneuronal":36347,"Ġlandslide":36348,"confirmed":36349,"ĠDepth":36350,"Ġrenewables":36351,"Bear":36352,"ĠMacedonia":36353,"Ġjerseys":36354,"Ġbunk":36355,"ĠSpawn":36356,"ĠControls":36357,"ĠBuchanan":36358,"Ġrobotics":36359,"Ġemphasizing":36360,"ĠTutorial":36361,"hyp":36362,"iston":36363,"Ġmonumental":36364,"æ°":36365,"ĠCarry":36366,"Ġtbsp":36367,"enance":36368,"Hill":36369,"arthed":36370,"Ġrotten":36371,"Dean":36372,"Ġtwisting":36373,"Ġgoodwill":36374,"Ġimmersion":36375,"Living":36376,"Ġbrushes":36377,"ĠCGI":36378,"ĠAtk":36379,"traditional":36380,"Ġphantom":36381,"ĠStamina":36382,"Ġexpansions":36383,"ĠMarin":36384,"Ġembarked":36385,"ĠEg":36386,"intestinal":36387,"ĠPEOPLE":36388,"ĠBooth":36389,"ĠAppalach":36390,"Ġrelegated":36391,"VT":36392,"MIT":36393,"Ġmuster":36394,"Ġwithdrawing":36395,"Ġmicroscope":36396,"ĠGathering":36397,"ĠCrescent":36398,"ĠArgentine":36399,"ĠDecre":36400,"ĠDominic":36401,"Ġbuds":36402,"antage":36403,"ĠIon":36404,"Ġwidened":36405,"ONSORED":36406,"ĠGloves":36407,"iannopoulos":36408,"razen":36409,"feel":36410,"Ġrepayment":36411,"Ġhindsight":36412,"ĠREALLY":36413,"ĠPistol":36414,"ĠBrah":36415,"Ġwatts":36416,"Ġsurvives":36417,"Ġflurry":36418,"issy":36419,"Alert":36420,"ĠUruguay":36421,"Phoenix":36422,"Slow":36423,"ĠGrave":36424,"ĠFir":36425,"Ġmanageable":36426,"Ġtariff":36427,"ĠUDP":36428,"ĠPistons":36429,"ĠNigerian":36430,"Ġstrikeouts":36431,"Ġcosmetics":36432,"whelming":36433,"fab":36434,"cape":36435,"proxy":36436,"Ġrethink":36437,"Ġovercoming":36438,"simple":36439,"Ġwoo":36440,"Ġdistracting":36441,"ĠStanton":36442,"ĠTulsa":36443,"ĠDock":36444,"659":36445,"Ġdiscord":36446,"ĠEmacs":36447,"ĠVes":36448,"ĠROB":36449,"Ġreassuring":36450,"Ġconsortium":36451,"Muslims":36452,"321":36453,"Ġprompts":36454,"sei":36455,"ĠHitch":36456,"imposed":36457,"ĠFool":36458,"Ġindiscrim":36459,"wrong":36460,"buquerque":36461,"Davis":36462,"!]":36463,"Ġtimeless":36464,"ĠNEED":36465,"Ġpesticide":36466,"Ġrallying":36467,"ĠCalder":36468,"Ġå¤":36469,"Ġxp":36470,"ĠUnle":36471,"ĠExport":36472,"luaj":36473,"Buff":36474,")</":36475,"Boot":36476,"ĠChrysler":36477,"orative":36478,"Mess":36479,"Ġnegligible":36480,"ertodd":36481,"ĠMushroom":36482,"ĠGale":36483,"gc":36484,"ĠCosby":36485,"ĠRural":36486,"ritical":36487,"Bell":36488,"Ġturbine":36489,"00200000":36490,"Ġlegitimately":36491,"ĠAnimated":36492,"TED":36493,"ĠTheodore":36494,"conduct":36495,"ĠHier":36496,"Ġcounterfeit":36497,"ĠAlgeria":36498,"Ġunbeat":36499,"controller":36500,"Ġunres":36501,"Ġscrambling":36502,"ĠFallon":36503,"Tes":36504,"Ġamber":36505,"Ġroyalties":36506,"ĠShelter":36507,"ĠLester":36508,"Ġclassify":36509,"Remote":36510,"Ġunheard":36511,"Ġcontroversies":36512,"Ġenrichment":36513,"ĠYankee":36514,"gamer":36515,"Ġplatinum":36516,"Ġecology":36517,"ĠSark":36518,"Ġuntouched":36519,"Ġsupervisors":36520,"Ġ\"%":36521,"Ġfooth":36522,"Ġcommons":36523,"Ġnarcotics":36524,"Ġindices":36525,"ĠPly":36526,"Ġadditionally":36527,"ĠGawker":36528,"ĠEQ":36529,"Playing":36530,"Ġcaveat":36531,"ĠAbsolute":36532,"ossus":36533,"Baby":36534,"Ġration":36535,"Ġresin":36536,"Ġcalibration":36537,"ĠNewport":36538,"Ġknocks":36539,"vt":36540,"Ġcompost":36541,"Scene":36542,"Ġsarcast":36543,"Ġkisses":36544,"Ġns":36545,"alli":36546,"ĠMarcel":36547,"ĠPiet":36548,"iatrics":36549,"Ġsurrounds":36550,"ĠReprodu":36551,"ĠPhillies":36552,"Ġuncertainties":36553,"ĠEur":36554,"ĠRomance":36555,"ĠHath":36556,"ĠNeeds":36557,"ĠCloak":36558,"Ġcrem":36559,"queue":36560,"Ġ355":36561,"Ġupfront":36562,"]);":36563,"Ġreciproc":36564,"Ġ1927":36565,"Ġ1100":36566,"utsu":36567,"Ġdepressive":36568,"owment":36569,"Fans":36570,"Ġmech":36571,"Ġannihil":36572,"Ġcounterterrorism":36573,"ĠFigures":36574,"bold":36575,"ĠMoines":36576,"ĠDrivers":36577,"Ġmanuscripts":36578,"ĠCrypto":36579,"Ġhypnot":36580,"reddits":36581,"Ġprosecutions":36582,"Ġdivert":36583,"CRIP":36584,"ĠBene":36585,"ĠReggie":36586,"Ġtaxing":36587,"ĠMorales":36588,"enting":36589,"tur":36590,"significant":36591,"ĠPROV":36592,"Ġstrands":36593,"Ġpouch":36594,"ĠRookie":36595,"»Ĵ":36596,"Ġnicer":36597,"hemy":36598,"hw":36599,"ECA":36600,"Ġintimidated":36601,"Ġstricter":36602,"Ġmicrobial":36603,"details":36604,"Ġvows":36605,"Ġquake":36606,"hhhh":36607,"Ġreinvent":36608,"Ub":36609,"Ġrelinqu":36610,"ĠBuffett":36611,"licensed":36612,"ittered":36613,"ĠPicard":36614,"Ġchewing":36615,"ucl":36616,"organic":36617,"Ġlocalized":36618,"ĠEconomist":36619,"Ġacquainted":36620,"Definition":36621,"sed":36622,"Critics":36623,"Ġcc":36624,"453":36625,"381":36626,"Ġfellows":36627,"Ġcheckpoints":36628,"025":36629,"Ġreelection":36630,"Ġmediated":36631,"ĠKDE":36632,"Ġhurdle":36633,"Ġtexting":36634,"Perfect":36635,"Ġtrustees":36636,"fecture":36637,"Ġdich":36638,"monary":36639,"Ġdistinctions":36640,"Ġ1400":36641,"Ġusher":36642,"Ġparasites":36643,"ĠSharing":36644,"ĠVim":36645,"Ġbarbecue":36646,"ĠMinisters":36647,"erella":36648,"Ġeb":36649,"Ġmc":36650,"ĠSomehow":36651,"ĠInsect":36652,"changes":36653,"broad":36654,"ĠByz":36655,"Ġgrapes":36656,"669":36657,"Ġ=================":36658,"Ġassimil":36659,"Ġhaunting":36660,"Ġfirepower":36661,"Ġdefamation":36662,"emphasis":36663,"Ġcompose":36664,"Ġallergies":36665,"Ġstrang":36666,"rollers":36667,"bang":36668,"Ġbrewers":36669,"rongh":36670,"riot":36671,"poor":36672,"cold":36673,"Sample":36674,"Ġbuoy":36675,"040":36676,"ĠCourtney":36677,"Ġ268":36678,"ĠWedding":36679,"702":36680,"Ġobsessive":36681,"Ġbraking":36682,"ĠLal":36683,"anical":36684,"å¦":36685,"aten":36686,"Construction":36687,"Ġclinically":36688,"iership":36689,"Names":36690,"ĠDiscuss":36691,"ĠRamos":36692,"Ġlocale":36693,"ĠAgricultural":36694,"Enable":36695,"Ġhorsepower":36696,"enture":36697,"Pref":36698,"Court":36699,"Ġstaffing":36700,"Ġfuturistic":36701,"drivers":36702,"ĠMarketplace":36703,"æĪ¦":36704,"Friends":36705,"Ġdamning":36706,"ĠCustomers":36707,"Ġweeds":36708,"ĠMai":36709,"Ġagile":36710,"ĠTatt":36711,"icent":36712,"Ranked":36713,"croft":36714,"ĠKaty":36715,"Extreme":36716,"Ġcarve":36717,"ĠRover":36718,"ĠByron":36719,"372":36720,"Ġconducts":36721,"ratch":36722,"itia":36723,"ĠPumpkin":36724,"Sadly":36725,"Reloaded":36726,"Policy":36727,"Ġlick":36728,"peak":36729,"isks":36730,"ĠCDs":36731,"ĠEncyclopedia":36732,"initial":36733,"Cos":36734,"ĠAwareness":36735,"ĠDram":36736,"$$$$":36737,"Ġriff":36738,"Ġscripture":36739,"runners":36740,"Ġboiler":36741,"onson":36742,"oin":36743,"Ġhamstring":36744,"Ġcataly":36745,"ĠArchbishop":36746,"chall":36747,"Ġfaux":36748,"okin":36749,"localhost":36750,"ĠNAME":36751,"adobe":36752,"SAN":36753,"amate":36754,"Ġscramble":36755,"Ġcarc":36756,"ĠManifest":36757,"ĠCedar":36758,"ĠSergio":36759,"later":36760,"ffer":36761,"Ġgrappling":36762,"ĠDeutsche":36763,"agonists":36764,"ĠNewsp":36765,"Ġpretended":36766,"archment":36767,"Ġcurated":36768,"Ġheadphone":36769,"ĠUncommon":36770,"ĠSIGN":36771,"Agent":36772,"Ġdeadlines":36773,"Ġhorizontally":36774,"ĠMAT":36775,"ĠSummers":36776,"Ġordained":36777,"ĠLastly":36778,"ĠKendall":36779,"Ġfrig":36780,"ĠMachina":36781,"ĠWaterloo":36782,"ĠMexicans":36783,"Ġprotector":36784,"Ġglare":36785,"}\"":36786,"Premium":36787,"Ġrift":36788,"ĠTelescope":36789,"Metal":36790,"Ġrecapt":36791,"Ġ;;":36792,"Ġinclination":36793,"Ġimposes":36794,"ingen":36795,"^{":36796,"Ġhaste":36797,"Ġdolphins":36798,"Ġcommuters":36799,"planned":36800,"cong":36801,"mx":36802,"ĠUpload":36803,"Ġextrap":36804,"ĠTucson":36805,"ĠExploration":36806,"efeated":36807,"Ġslender":36808,"703":36809,"ĠBuk":36810,"isel":36811,"Ġcompetitiveness":36812,"chlor":36813,"ĠPermanent":36814,"ĠEverett":36815,"ĠSpecialist":36816,"ĠSOL":36817,"Ġcyan":36818,"ĠExactly":36819,"UF":36820,"ĠLIFE":36821,"aryl":36822,"onet":36823,"ĠEmployee":36824,"awed":36825,"ĠRatings":36826,"Ġextravag":36827,"ulhu":36828,"ĠPlane":36829,"Ġelevate":36830,"ĠCoordinator":36831,"ĠWatkins":36832,"Ġexcludes":36833,"Ġsentient":36834,"Ġepoch":36835,"Ġalloc":36836,"Previously":36837,"ĠShy":36838,"ĠSlovakia":36839,"LOCK":36840,"Ġmarkedly":36841,"Ġknob":36842,"Ġadventurers":36843,"ĠBeen":36844,"ĠCosts":36845,"ammers":36846,"Ġonslaught":36847,"ĠSupported":36848,"ĠTau":36849,"ikarp":36850,"ĠSovere":36851,"ĠHampton":36852,"ãĤī":36853,"Prev":36854,"ĠWorse":36855,"Ġcottage":36856,"ĠHades":36857,"lez":36858,"bowl":36859,"Ġfragrance":36860,"ĠLok":36861,"EMOTE":36862,"ĠPetro":36863,"Ġ1925":36864,"ĠPend":36865,"producing":36866,"Ġrelocate":36867,"vati":36868,"pole":36869,"Ġsemin":36870,"ĠNUM":36871,"Ġrocked":36872,"buff":36873,"bly":36874,"Reply":36875,"ĠHai":36876,"Ġarticulated":36877,"ĠIslamabad":36878,"665":36879,"ĠClaims":36880,"Desktop":36881,"Ġtrustee":36882,"Ġscripting":36883,"ĠSob":36884,"ĠAsylum":36885,"STDOUT":36886,"ĠClown":36887,"ĠDortmund":36888,"ĠDevon":36889,"lite":36890,"ĠMarble":36891,"Ġbunker":36892,"Ġcrest":36893,"Ġarousal":36894,"ĠSears":36895,"ĠBuddy":36896,"eredith":36897,"ĠPolly":36898,"Ġdecode":36899,"ĠVish":36900,"ĠReflect":36901,"anon":36902,"Ġrefunds":36903,"immers":36904,"HM":36905,"Ġwiping":36906,"Ġpuzzled":36907,"Ġmatte":36908,"uno":36909,"Pierre":36910,")),":36911,"Ġtainted":36912,"Ġsymbolism":36913,"ĠFraz":36914,"Ġprotestors":36915,"etheus":36916,"%%%%":36917,"Wra":36918,"Ġlax":36919,"adem":36920,"aturation":36921,"ãĥĵ":36922,"ĠTrailer":36923,"ĠENG":36924,"ĠBowser":36925,"Ġattm":36926,"Dur":36927,"807":36928,"Ġsidx":36929,"Ġcider":36930,"ĠAffect":36931,"Ġwoven":36932,"ĠBarker":36933,"benef":36934,"Ġdstg":36935,"ĠRyu":36936,">[":36937,"Ġsqor":36938,"Saudi":36939,"Ġistg":36940,"Ġindulge":36941,"proc":36942,"Ġdisgusted":36943,"Ġcompounded":36944,"Ġnem":36945,"Ġschooling":36946,"ĠCure":36947,"processing":36948,"Sol":36949,"Ġproverb":36950,"itized":36951,"ĠAlvarez":36952,"Ġscarf":36953,"Ġrectangular":36954,"reve":36955,"Ġhormonal":36956,"ĠStress":36957,"itizen":36958,"Ġ425":36959,"girls":36960,"ĠNoir":36961,"ĠRapp":36962,"Ġmarches":36963,"church":36964,"ĠUses":36965,"Ġ405":36966,"ĠBerm":36967,"Ġordinances":36968,"ĠJudgment":36969,"Charges":36970,"ĠZin":36971,"Ġdusty":36972,"Ġstrawberries":36973,"Ġperce":36974,"ĠThur":36975,"ĠDeborah":36976,"netflix":36977,"ĠLambert":36978,"Ġamused":36979,"ĠGuang":36980,"YOU":36981,"RGB":36982,"ĠCCTV":36983,"Ġfiat":36984,"rang":36985,"Ġfederation":36986,"ĠMant":36987,"ĠBust":36988,"ĠMare":36989,"respective":36990,"ĠMigration":36991,"ĠBIT":36992,"590":36993,"Ġpatriotism":36994,"Ġoutlining":36995,"region":36996,"ĠJosé":36997,"Ġblasting":36998,"ĠEzra":36999,"Bs":37000,"Ġundermines":37001,"ĠSmooth":37002,"Ġclashed":37003,"radio":37004,"Ġtransitioning":37005,"ĠBuccaneers":37006,"ĠOwl":37007,"Ġplugs":37008,"Ġhiatus":37009,"ĠPinball":37010,"Ġmig":37011,"ĠNutr":37012,"ĠWolfe":37013,"Ġintegers":37014,"Ġorbits":37015,"ĠEdwin":37016,"ĠDirectX":37017,"bite":37018,"Ġblazing":37019,"vr":37020,"Edge":37021,"ĠPID":37022,"exit":37023,"ĠComed":37024,"ĠPathfinder":37025,"ĠGuid":37026,"ĠSigns":37027,"ĠZer":37028,"ĠAgenda":37029,"Ġreimbursement":37030,"Mesh":37031,"iPhone":37032,"ĠMarcos":37033,"ĠSites":37034,"hate":37035,"enburg":37036,"Ġsockets":37037,"pend":37038,"Batman":37039,"vir":37040,"ĠSHOW":37041,"Ġprovisional":37042,"conn":37043,"ĠDeaths":37044,"ATIVE":37045,"Profile":37046,"sym":37047,"JA":37048,"Ġninja":37049,"installed":37050,"idates":37051,"ebra":37052,"ĠOmaha":37053,"Ġseizing":37054,"ĠBeasts":37055,"Ġsalts":37056,"Mission":37057,"Generally":37058,"ĠTrilogy":37059,"heon":37060,"legates":37061,"Ġdime":37062,"Ġfaire":37063,"parable":37064,"Graph":37065,"Ġtotaling":37066,"Ġdiagrams":37067,"ĠYanuk":37068,"plet":37069,"ĠMeh":37070,"Ġmythical":37071,"ĠStephens":37072,"autical":37073,"ochemistry":37074,"Ġkilograms":37075,"Ġelbows":37076,"ancock":37077,"ĠBCE":37078,"ĠPrague":37079,"Ġimprov":37080,"ĠDevin":37081,"Ġ\"\\":37082,"paralle":37083,"Ġsupremacists":37084,"ĠBillion":37085,"Ġregimen":37086,"innacle":37087,"Ġrequisite":37088,"angan":37089,"ĠBurlington":37090,"ainment":37091,"ĠObjective":37092,"omsky":37093,"GV":37094,"Ġunilateral":37095,"Ġtc":37096,"Ġhires":37097,"mental":37098,"Ġinvoluntary":37099,"Ġtranspl":37100,"ĠASCII":37101,"¨":37102,"Events":37103,"Ġdoubted":37104,"ĠKaplan":37105,"ĠCourage":37106,"igon":37107,"ĠManaging":37108,"ĠTart":37109,"Ġfalsehood":37110,"ĠViolet":37111,"Ġairs":37112,"Ġfertilizer":37113,"Britain":37114,"Ġaquatic":37115,"ouf":37116,"Words":37117,"ĠHartford":37118,"Ġevenings":37119,"ĠVengeance":37120,"quite":37121,"Gall":37122,"ĠPret":37123,"Ġpdf":37124,"ĠLM":37125,"ĠSochi":37126,"ĠIntercept":37127,"920":37128,"Ġprofitability":37129,"ĠIdle":37130,"ĠMacDonald":37131,"ĠEstablishment":37132,"umsy":37133,"Ġgatherings":37134,"ĠNaj":37135,"Charlie":37136,"Ġascent":37137,"ĠProtector":37138,"Ġalgebra":37139,"Ġbios":37140,"forums":37141,"ELS":37142,"Introduced":37143,"Ġ335":37144,"Ġastronomy":37145,"Contribut":37146,"ĠPolic":37147,"Platform":37148,"Ġcontainment":37149,"wrap":37150,"Ġcoronary":37151,"ĠJelly":37152,"manager":37153,"Ġheartbreaking":37154,"cair":37155,"ĠChero":37156,"cgi":37157,"Medical":37158,"ĠAccountability":37159,"!!\"":37160,"ophile":37161,"Ġpsychotic":37162,"ĠRestrict":37163,"Ġequitable":37164,"issues":37165,"Ġ1905":37166,"ĠNek":37167,"cised":37168,"ĠTracking":37169,"Ġozone":37170,"Ġcooker":37171,"rosis":37172,"Ġreopen":37173,"Ġinfinity":37174,"ĠPharmaceutical":37175,"ensional":37176,"Attempt":37177,"ĠRory":37178,"Marco":37179,"Ġawaits":37180,"HOW":37181,"treated":37182,"Ġbolst":37183,"Ġrevered":37184,"Ġpods":37185,"oppers":37186,"0010":37187,"Ġamplitude":37188,"rican":37189,"SPONSORED":37190,"Ġtrousers":37191,"Ġhalves":37192,"ĠKaine":37193,"ĠCutler":37194,"ĠAUTH":37195,"Ġsplendid":37196,"Ġpreventive":37197,"ĠDudley":37198,"ifacts":37199,"uminati":37200,"ĠYin":37201,"Ġadmon":37202,"ĠVag":37203,"Ġinverted":37204,"Ġhastily":37205,"ĠHague":37206,"Lyn":37207,"Ġledger":37208,"Ġastronomical":37209,"getting":37210,"Ġcirca":37211,"ĠCic":37212,"ĠTennis":37213,"Limited":37214,"Ġdru":37215,"ĠBYU":37216,"Ġtravellers":37217,"Ġpane":37218,"ĠIntro":37219,"Ġpatiently":37220,"Ġaiding":37221,"Ġloos":37222,"ĠTough":37223,"Ġ293":37224,"Ġconsumes":37225,"SourceFile":37226,"Ġ\"\"\"":37227,"Ġbonding":37228,"Ġtilted":37229,"Ġmenstrual":37230,"ĠCelestial":37231,"ULAR":37232,"Plugin":37233,"Ġrisking":37234,"Naz":37235,"ĠRiyadh":37236,"Ġaccredited":37237,"Ġskirm":37238,"éĽ":37239,"Ġexaminer":37240,"Ġmessing":37241,"Ġnearing":37242,"ĠChern":37243,"ĠBeckham":37244,"Ġswapped":37245,"Ġgoose":37246,"Kay":37247,"Ġlofty":37248,"ĠWallet":37249,"Ġ['":37250,"Ġapocalypse":37251,"Ġbamboo":37252,"ĠSPACE":37253,"ĠElena":37254,"Ġ306":37255,"acons":37256,"Ġtightened":37257,"Ġadolescence":37258,"Ġrainy":37259,"Ġvandalism":37260,"ĠNewtown":37261,"Ġconject":37262,"cakes":37263,"Ġcheated":37264,"Ġmoderators":37265,"params":37266,"EFF":37267,"Ġdeceit":37268,"ĠSTL":37269,"ĠTanzania":37270,"ĠRI":37271,"Ġ1923":37272,"ĠExile":37273,"thel":37274,"Ġtheolog":37275,"Ġquirky":37276,"ĠIrvine":37277,"Ġneedy":37278,"oris":37279,"Um":37280,"Ka":37281,"Ġmailbox":37282,"322":37283,"Ġbos":37284,"ĠPetra":37285,"KING":37286,"Ġenlarged":37287,"Often":37288,"Ġbadass":37289,"Ġ343":37290,"ĠPlaces":37291,"ĠCAD":37292,"Ġpristine":37293,"Ġintervening":37294,"direction":37295,"Ġlaz":37296,"ĠDSM":37297,"Ġprojecting":37298,"ĠFunk":37299,"agog":37300,"payment":37301,"nov":37302,"Ġchatter":37303,"ARB":37304,"Ġexaminations":37305,"ĠHousehold":37306,"ĠGus":37307,"Ford":37308,"414":37309,"Boss":37310,"Ġmystic":37311,"Ġleaps":37312,"ĠBav":37313,"ulz":37314,"budget":37315,"Football":37316,"Ġsubsidized":37317,"Ġfirsthand":37318,"Ġcoincide":37319,"ocular":37320,"Conn":37321,"ĠCollabor":37322,"Ġfools":37323,"amura":37324,"ahar":37325,"rists":37326,"Ġswollen":37327,"Ġexpended":37328,"ĠPau":37329,"sup":37330,"Ġspar":37331,"Ġkeynote":37332,"suff":37333,"Ġunequal":37334,"Ġprogressing":37335,"strings":37336,"ĠGamergate":37337,"Disney":37338,"ĠEleven":37339,"omnia":37340,"Ġscripted":37341,"Ġearners":37342,"brother":37343,"ĠEnabled":37344,"æ³":37345,"Ġlarvae":37346,"ĠLOC":37347,"mess":37348,"Wilson":37349,"ĠTemplate":37350,"successfully":37351,"Ġparamount":37352,"Ġcamouflage":37353,"Ġbinds":37354,"ĠQuiet":37355,"ĠShutterstock":37356,"rush":37357,"Ġmascot":37358,"fortune":37359,"ĠColt":37360,"ĠBeyon":37361,"habi":37362,"Ġhairc":37363,"Ġ267":37364,"ĠDeus":37365,"Ġtwitch":37366,"Ġconcentrating":37367,"Ġnipples":37368,"cible":37369,"Ġgir":37370,"NZ":37371,"Math":37372,"nih":37373,"Required":37374,"Ġponder":37375,"ĠSAN":37376,"Ġweddings":37377,"Ġloneliness":37378,"NES":37379,"ĠMahjong":37380,"695":37381,"addle":37382,"ĠGarner":37383,"ĠCOUR":37384,"Bridge":37385,"Ġspree":37386,"ĠCaldwell":37387,"Ġbribery":37388,"Ġ��������":37389,"plugins":37390,"Ġracket":37391,"Ġchampagne":37392,"versible":37393,"Vote":37394,"Ġmodifiers":37395,"Mayor":37396,"680":37397,"Ġassemblies":37398,"ĠSultan":37399,"ĠNing":37400,"ĠLadies":37401,"Ġsulfur":37402,"Ġorbs":37403,"Ġ-----":37404,"_______":37405,"ĠJournalism":37406,"Ġesports":37407,"Ġlush":37408,"Ġhue":37409,"Ġspectral":37410,"Honest":37411,"ãĥı":37412,"Ġbushes":37413,"Ġreinforcement":37414,"Ġreopened":37415,"ĠWheels":37416,"ĠMorg":37417,"rieving":37418,"Ġauxiliary":37419,"ĠjQuery":37420,"ĠBAT":37421,"tesque":37422,"Ġvertex":37423,"pure":37424,"frey":37425,"ãĤº":37426,"dos":37427,"Ġtyph":37428,"Ġcull":37429,"Ġeq":37430,"Ġdecon":37431,"Ġtossing":37432,"Ġdisparate":37433,"ĠBrigham":37434,"printf":37435,"ledged":37436,"Ġsund":37437,"Ġcozy":37438,"Ġhepatitis":37439,"performing":37440,"Ġaval":37441,"ĠGG":37442,"future":37443,"Ġpetertodd":37444,"ĠKosovo":37445,"Ġmagnets":37446,"Already":37447,"ĠEdison":37448,"ĠCeres":37449,"ĠRAID":37450,"Ġbrilliance":37451,"576":37452,"Ġderives":37453,"Ġhypertension":37454,"ĠÎĶ":37455,"Ġlambda":37456,"Ġflair":37457,"Ġmissionaries":37458,"Ġrapes":37459,"ĠStarter":37460,"ĠMonths":37461,"Ġdefy":37462,"Ġseismic":37463,"ĠRaphael":37464,"Ġeurozone":37465,"656":37466,"zsche":37467,"Ġscratched":37468,"Ġbows":37469,"ĠLennon":37470,"ĠGaia":37471,"Ġdripping":37472,"facts":37473,"Ale":37474,"Ġfrogs":37475,"ĠBreast":37476,"ogeneity":37477,"ĠProsecutor":37478,"Ġamplified":37479,"ĠHodg":37480,"ĠFn":37481,"Thousands":37482,"ĠNIH":37483,"ĠMonitoring":37484,"FTWARE":37485,"ĠPriebus":37486,"ĠGrowing":37487,"hunter":37488,"Ġdiagnose":37489,"ĠMald":37490,"ĠLR":37491,"Ġcrowned":37492,"Ġbursting":37493,"Ġdissolution":37494,"javascript":37495,"Ġusefulness":37496,"ĠExecution":37497,":(":37498,"ĠIvory":37499,"aah":37500,"Ġpersecuted":37501,"violence":37502,"istas":37503,"ĠCrate":37504,"Ġimpulses":37505,"ĠSpani":37506,"edes":37507,"Handle":37508,"ĠZerg":37509,"thinkable":37510,"Lastly":37511,"Ġspontaneously":37512,"Ġinconvenient":37513,"Ġdismissing":37514,"Ġplotted":37515,"Ġeighty":37516,"Ġ737":37517,"rish":37518,"ĠThornton":37519,"atham":37520,"Ġsitcom":37521,"Ven":37522,"Recipe":37523,"tel":37524,"lund":37525,"Ġclears":37526,"ĠSasuke":37527,"Ġ258":37528,"Ġopting":37529,"Ġenraged":37530,"esthetic":37531,"ĠAe":37532,"uchs":37533,"Prep":37534,"Flow":37535,"Ġrunoff":37536,"ĠEating":37537,"ĠGiles":37538,"ĠActing":37539,"resources":37540,"ibaba":37541,"Ġrpm":37542,"Ġskewed":37543,"ĠBlanc":37544,"ĠSakuya":37545,"Ġhotter":37546,"Ġ1924":37547,"opian":37548,"cko":37549,"Ġcrumbling":37550,"Ġcaptains":37551,"ĠAppropriations":37552,"leaders":37553,"dropping":37554,"anuts":37555,"Ġreversing":37556,"ĠPose":37557,"ĠSek":37558,"Scot":37559,"ĠIdea":37560,"cise":37561,"ĠSlovenia":37562,"Ġ317":37563,"Doctor":37564,"Ġcrocod":37565,"aldi":37566,"Sea":37567,"ĠFarrell":37568,"Ġmercenaries":37569,"ĠRNC":37570,"ĠGuess":37571,"Ġpacing":37572,"Machine":37573,"StreamerBot":37574,"ĠCharity":37575,"Ġ298":37576,"Ġcannons":37577,"ĠToby":37578,"TPPStreamerBot":37579,"ĠPassion":37580,"cfg":37581,"Thom":37582,"Ġbadges":37583,"ĠBernstein":37584,".âĢĵ":37585,"ĠPOP":37586,"ĠConj":37587,"Ġinitialization":37588,"Ġbiodiversity":37589,"Dub":37590,"Ġfeudal":37591,"Ġdisclaimer":37592,"Ġcrow":37593,"Ġignition":37594,"arf":37595,"SHA":37596,"ĠkHz":37597,"hazard":37598,"ĠArtists":37599,"oeuv":37600,"679":37601,"ĠRudy":37602,"Nine":37603,"ĠRamadan":37604,"å½":37605,"itto":37606,"Ġadrenaline":37607,"Cert":37608,"Ġsmelled":37609,"Ġimpunity":37610,"Ġagendas":37611,"ĠReborn":37612,"ĠConcent":37613,"ĠSeems":37614,"Ġomega":37615,"ĠDustin":37616,"Ġbacker":37617,"ĠSauce":37618,"ĠBoyle":37619,"WIN":37620,"Ġspins":37621,"Ġpauses":37622,"upt":37623,"Ġshredded":37624,"Ġstrapped":37625,"ĠCorruption":37626,"Ġscratches":37627,"Ġni":37628,"Ġattire":37629,"ĠSAF":37630,"FactoryReloaded":37631,"ĠIPS":37632,"Ġ(%":37633,"Ġseminar":37634,"focus":37635,"civil":37636,"Ġ1860":37637,"intosh":37638,"Ġcontinual":37639,"Ġabbrevi":37640,"ĠSok":37641,"ocobo":37642,"XM":37643,"Ġfrantic":37644,"Ġunavoidable":37645,"Ġartery":37646,"Ġannotations":37647,"bath":37648,"Climate":37649,"Ġdors":37650,"ĠSlide":37651,"coord":37652,"ĠReload":37653,"ĠLDL":37654,"ĠLovecraft":37655,"Ġunimagin":37656,"Ġresembled":37657,"Ġbarracks":37658,"np":37659,"Ġsurrogate":37660,"Ġcategorized":37661,"ãĤ©":37662,"Ġvaccinated":37663,"Ġdrainage":37664,"Ġindist":37665,"ĠWhatsApp":37666,"Ġ1870":37667,"olerance":37668,"invoke":37669,"amorph":37670,"Ġreconnect":37671,"Ġemanc":37672,"Ġblindness":37673,"Ġ1280":37674,"internet":37675,"collar":37676,"Ġaltru":37677,"Ġabyss":37678,"ĠTRI":37679,"657":37680,"Ġinfused":37681,"HEAD":37682,"Ġforestry":37683,"ĠWoody":37684,"ĠCi":37685,"wi":37686,"sam":37687,"784":37688,"holiday":37689,"Ġmogul":37690,"ĠFees":37691,"ĠDEN":37692,"Internal":37693,"urbed":37694,"fusc":37695,"atom":37696,"ĠIllusion":37697,"Ġpolled":37698,"Ġflap":37699,"Ġcoax":37700,"LGBT":37701,"Analy":37702,"ĠSections":37703,"ĠCaliforn":37704,"emn":37705,"Ġhither":37706,"ĠNIGHT":37707,"Ġnailed":37708,"ĠPipeline":37709,"391":37710,"oof":37711,"ĠPrimal":37712,"verend":37713,"Ġslashing":37714,"Ġretri":37715,"aviour":37716,"Ġdeparting":37717,"gil":37718,"ISC":37719,"Ġmidway":37720,"Ġultrasound":37721,"Ġbehaving":37722,"ĠTara":37723,"classes":37724,"Virtual":37725,"ĠColonial":37726,"Ġstripping":37727,"Ġorchestrated":37728,"ĠGraves":37729,"452":37730,"ĠIronically":37731,"ĠWriters":37732,"Ġlends":37733,"ĠManz":37734,"Ġraven":37735,"Ġoxidative":37736,"Ġ266":37737,"ELF":37738,"actually":37739,"ascar":37740,"Draft":37741,"Ġfavourable":37742,"Ġhumiliating":37743,"Ġfidelity":37744,"ĠHof":37745,"ĠXuan":37746,"496":37747,"Ġlayered":37748,"atis":37749,"790":37750,"Ġpaycheck":37751,"iton":37752,"Kar":37753,"ĠVMware":37754,"ĠFarmer":37755,"Ġservic":37756,"glomer":37757,"Ġslump":37758,"ĠFabric":37759,"ĠDOC":37760,"esting":37761,"Ġreassure":37762,"Ġphyl":37763,"volt":37764,"itory":37765,"Rules":37766,"Ġoxidation":37767,"Ġprized":37768,"Ġmistress":37769,"ĠDjango":37770,"WARN":37771,"åij":37772,"Ġencode":37773,"ĠFeedback":37774,"Ġstupidity":37775,"Ian":37776,"ĠYugoslavia":37777,"ר":37778,"acl":37779,"UTE":37780,"1977":37781,"Ġqualifies":37782,"Ġpulses":37783,"pretty":37784,"Ġfroze":37785,"Ġss":37786,"Iterator":37787,"Ġurgently":37788,"Ġmailed":37789,"ĠCham":37790,"Ġsustaining":37791,"Ġbasil":37792,"Ġpuppies":37793,"ilant":37794,"ĠPLEASE":37795,"lap":37796,"aceous":37797,"Fear":37798,"ĠMastery":37799,"automatic":37800,"ĠTAG":37801,"Ġantim":37802,"agles":37803,"473":37804,"frames":37805,"Ġwhispers":37806,"ĠWhoever":37807,"Ġbravery":37808,"ĠUKIP":37809,"ractions":37810,"\"\"\"":37811,"Ġtame":37812,"Ġparted":37813,"everything":37814,"CONT":37815,"Ġindebted":37816,"Ġaddr":37817,"rek":37818,"IRED":37819,"Ġeminent":37820,"clinton":37821,"Ġousted":37822,"Ġreviewer":37823,"Ġmeltdown":37824,"Ġrearr":37825,"ĠYao":37826,"thereal":37827,"abyte":37828,"Ġstumbling":37829,"Ġbatches":37830,"Ġ259":37831,"Ġcontraceptive":37832,"Ġprostitute":37833,"ensis":37834,"Decl":37835,"ĠStrikes":37836,"Military":37837,"ĠOath":37838,"vacc":37839,"ppings":37840,"052":37841,"ĠpartName":37842,"amping":37843,"Reports":37844,"KI":37845,"CHR":37846,"Ġsubtly":37847,"swers":37848,"Blake":37849,"usual":37850,"Ġcontestants":37851,"Ġcartridges":37852,"ĠGREAT":37853,"Ġblush":37854,"ĠâĢº":37855,"472":37856,"Ġreasoned":37857,"ãĥ¤":37858,"paralleled":37859,"Ġdyn":37860,"agate":37861,"Ġnightly":37862,"åĨ":37863,"556":37864,"Ġsemantic":37865,"ĠAdvoc":37866,"Ġ!!":37867,"Ġdisagrees":37868,"ĠBW":37869,"Veh":37870,"Ġharming":37871,"Ġembraces":37872,"Ġstrives":37873,"Ġinland":37874,"ĠKard":37875,"Ġheats":37876,"ĠGinny":37877,"utan":37878,"ernaut":37879,"ylene":37880,"ĠElev":37881,"JD":37882,"Ġhars":37883,"ĠStarr":37884,"Ġskysc":37885,"Ġcollaborators":37886,"Usually":37887,"Ġrevolutions":37888,"ĠSTATS":37889,"Ġdismantle":37890,"Ġconfidently":37891,"Ġkinetic":37892,"Ali":37893,"Ġpercentile":37894,"Ġextracting":37895,"illian":37896,"estead":37897,"Ġphysicists":37898,"ĠMarshal":37899,"Ġfellowship":37900,"Ġdashed":37901,"ĠUR":37902,"ĠSioux":37903,"ĠCompact":37904,"amide":37905,"Python":37906,"ĠLeigh":37907,"ĠPharmac":37908,"istrates":37909,"herical":37910,"Ġfue":37911,"ĠEmin":37912,"Ġ({":37913,"ĠNeighborhood":37914,"Ġdisrupting":37915,"ĠDup":37916,"Ġgland":37917,"ĠSev":37918,"ĠMarian":37919,"argon":37920,"ĠDund":37921,"Ġ<!--":37922,"Ġstrand":37923,"Ġstadiums":37924,"zos":37925,"Ġpsychosis":37926,"ĠRack":37927,"Ġbrilliantly":37928,"ï¸ı":37929,"Ġsubmerged":37930,"ĠInstit":37931,"ĠChow":37932,"Ġcages":37933,"ĠHats":37934,"ĠUrs":37935,"Ġdiluted":37936,"usat":37937,"ienne":37938,"ĠMembership":37939,"ĠBurk":37940,"Ġie":37941,"Ġarchetype":37942,"Drug":37943,"ulton":37944,"ĠSpock":37945,"ĠMcKay":37946,"ĠDepend":37947,"Featured":37948,"Soc":37949,"1978":37950,"ĠBere":37951,"Ġrelentlessly":37952,"Ġcrippling":37953,"Ġarthritis":37954,"çĶŁ":37955,"ĠTropical":37956,"ĠBulg":37957,"ĠCheryl":37958,"Ġadmirable":37959,"Ġsubtitle":37960,"Override":37961,"Ġoriginating":37962,"ĠCCP":37963,"Ġswore":37964,"ĠSole":37965,"ĠDisorders":37966,"329":37967,"Ġprocession":37968,"Ġrefurb":37969,"Ġimmersed":37970,"requently":37971,"Ġskeptics":37972,"Ġceramic":37973,"mitter":37974,"enstein":37975,"belt":37976,"ĠTIT":37977,"bidden":37978,"Ġfir":37979,"mist":37980,">]":37981,"Ġweave":37982,"ĠParadox":37983,"Ġentrusted":37984,"ĠBarclays":37985,"Ġnovelist":37986,"ogie":37987,"806":37988,"Ġninety":37989,"Ġdisagreements":37990,"@@@@@@@@":37991,"ĠAuschwitz":37992,"cars":37993,"ĠLET":37994,"tub":37995,"arantine":37996,"POS":37997,"Ġbackstory":37998,"Ġcheerful":37999,"ĠRag":38000,"eka":38001,"biased":38002,"Ġinexperienced":38003,"akra":38004,"ĠWitt":38005,"tan":38006,"Ġrapist":38007,"Ġplateau":38008,"chal":38009,"ĠInquis":38010,"expression":38011,"Ġcipher":38012,"Ġshaving":38013,"adden":38014,"rely":38015,"(\\":38016,"isma":38017,"ĠRegulatory":38018,"CHAR":38019,"ilyn":38020,"NVIDIA":38021,"GU":38022,"Ġmurm":38023,"laus":38024,"Christopher":38025,"Ġcontractual":38026,"ĠProxy":38027,"ĠJaime":38028,"ĠMethodist":38029,"Ġstewards":38030,"sta":38031,"peria":38032,"Ġphysiology":38033,"Ġbumped":38034,"Ġfructose":38035,"Australian":38036,"ĠMetallic":38037,"ĠMasquerade":38038,"arb":38039,"Ġpromul":38040,"Ġdownfall":38041,"Ġbutcher":38042,"Ġbour":38043,"ĠINFORMATION":38044,"ĠBis":38045,"pects":38046,"adena":38047,"Ġcontemplating":38048,"aroo":38049,"centered":38050,"ĠPeaks":38051,"Used":38052,"Ġmodem":38053,"Ġgenders":38054,"Ġ8000":38055,"371":38056,"Ġmaternity":38057,"ĠRaz":38058,"Ġrocking":38059,"Ġhandguns":38060,"ĠDACA":38061,"Autom":38062,"ĠNile":38063,"Ġtumult":38064,"ĠBenefit":38065,"ĠApproach":38066,"workshop":38067,"ĠLeaving":38068,"Ger":38069,"instead":38070,"Ġvibrations":38071,"Ġrepositories":38072,"497":38073,"ĠAunt":38074,"ĠJub":38075,"ĠExpedition":38076,"Alpha":38077,"Ġsans":38078,"Ġoverdue":38079,"Ġovercrowd":38080,"Ġlegislatures":38081,"Ġpaternal":38082,"ĠLeonardo":38083,"Ġexpressive":38084,"Ġdistractions":38085,"Ġsilenced":38086,"trust":38087,"Ġbiking":38088,"Ġ560":38089,"Ġpropriet":38090,"Ġimposition":38091,"Ġconglomer":38092,"Ġ=================================================================":38093,"ĠTeaching":38094,"ĠYose":38095,"intensive":38096,"Town":38097,"Ġtrolling":38098,"ĠGrac":38099,"ĠASUS":38100,"Yo":38101,"Ġspecials":38102,"ĠNeph":38103,"ĠGodzilla":38104,"Database":38105,"ĠHegel":38106,"Ġ272":38107,"1976":38108,"ĠGloria":38109,"Ġdisemb":38110,"ĠInvestigations":38111,"ĠBane":38112,"agements":38113,"Strange":38114,"Ġtreasury":38115,"ĠPlays":38116,"Ġundesirable":38117,"Ġwidening":38118,"Ġverbally":38119,"Ġinfancy":38120,"Ġcutter":38121,"fml":38122,"Ġ2100":38123,"prototype":38124,"fine":38125,"Ġdecriminal":38126,"Ġdysfunctional":38127,"Ġbesie":38128,"ĠErnst":38129,"zeb":38130,"Ġnortheastern":38131,"Ġaust":38132,"porate":38133,"ĠMarlins":38134,"Ġsegregated":38135,"eworld":38136,"ĠMaher":38137,"Ġtraverse":38138,"Ġmonastery":38139,"urgy":38140,"Gear":38141,"sand":38142,"Compl":38143,"ĠEMP":38144,"Ġplent":38145,"ĠMercer":38146,"Ġ276":38147,"TABLE":38148,"Configuration":38149,"Hundreds":38150,"Ġpric":38151,"Ġcollaborating":38152,"ĠParamount":38153,"ĠCummings":38154,"Ġ(<":38155,"Ġrecorder":38156,"Ġflats":38157,"Ġ416":38158,"whose":38159,"FontSize":38160,"ĠOrbit":38161,"YR":38162,"Ġwrists":38163,"Ġbakery":38164,")}":38165,"ĠBounty":38166,"ĠLancaster":38167,"Ġendings":38168,"according":38169,"ĠSalam":38170,"easy":38171,"755":38172,"ĠBurr":38173,"ĠBarnett":38174,"onomous":38175,"Union":38176,"Ġprecedence":38177,"ĠScholarship":38178,"ĠUX":38179,"Ġrollout":38180,"Ġboon":38181,"alm":38182,"ĠCanter":38183,"æµ":38184,"Ġrounding":38185,"Ġclad":38186,"Ġvap":38187,"ĠFeatured":38188,"isations":38189,"Ġ540":38190,"police":38191,"Ġunsettling":38192,"Ġdrifting":38193,"ĠLumia":38194,"ĠObamaCare":38195,"ĠFavor":38196,"Hyper":38197,"ĠRothschild":38198,"ĠMiliband":38199,"analy":38200,"ĠJuliet":38201,"Hu":38202,"Ġrecalling":38203,"ahead":38204,"696":38205,"Ġunfavorable":38206,"Ġdances":38207,"Ox":38208,"Ġlegality":38209,"Ġ403":38210,"romancer":38211,"Ġinquire":38212,"ĠMoves":38213,"\\\">":38214,"ĠVariant":38215,"ĠMessiah":38216,"ĠLCS":38217,"ĠBahá":38218,"756":38219,"Ġeyebrow":38220,"ĠÂ¥":38221,"ĠMcF":38222,"ĠForty":38223,"Mas":38224,"Ġpanicked":38225,"Ġtransformations":38226,"qq":38227,"Ġrevolves":38228,"ringe":38229,"ĠAi":38230,"axe":38231,"Ġonward":38232,"ĠCFR":38233,"ĠBare":38234,"login":38235,"Ġliquids":38236,"Ġdecomp":38237,"secondary":38238,"ilan":38239,"ĠConvert":38240,"amiya":38241,"Ġprosecuting":38242,"Ġâī¡":38243,"ĠYorkers":38244,"ĠByrne":38245,"slow":38246,"awei":38247,"Jean":38248,"Ġ269":38249,"ĠSkydragon":38250,"Ġé":38251,"ĠNicaragua":38252,"ĠHuckabee":38253,"ĠHighly":38254,"Ġamphib":38255,"ĠPastor":38256,"ĠLets":38257,"Ġblurred":38258,"Ġvisceral":38259,"ĠCBO":38260,"Ġcollaborated":38261,"zig":38262,"Legal":38263,"Ġapartheid":38264,"Ġbrid":38265,"Ġpreset":38266,"ĠDET":38267,"ĠAMA":38268,"×Ķ":38269,"arching":38270,"aucuses":38271,"builder":38272,"Ġpoetic":38273,"Ġemulator":38274,"ĠMolecular":38275,"Ġhonoring":38276,"iseum":38277,"Ġtractor":38278,"ĠCluster":38279,"ĠCalm":38280,"aredevil":38281,"Ġsidewalks":38282,"Ġviolin":38283,"Ġgeneralized":38284,"ĠAlec":38285,"Ġembargo":38286,"Ġfastball":38287,"ĠHTTPS":38288,"ĠLack":38289,"ĠChill":38290,"river":38291,"Chel":38292,"ĠSwarm":38293,"ĠLevine":38294,"roying":38295,"Launch":38296,"Ġkicker":38297,"Ġadditive":38298,"ĠDeals":38299,"Widget":38300,"containing":38301,"Ġescalate":38302,"ĠOPEN":38303,"Ġtweaked":38304,"Ġstash":38305,"Ġsparks":38306,"ĠEssex":38307,"ĠEcc":38308,"Ġconvict":38309,"Ġblogging":38310,"IER":38311,"ĠHL":38312,"Ġmurderers":38313,"759":38314,"ĠHib":38315,"Ġdepl":38316,"ĠJord":38317,"Sac":38318,"Ġdissect":38319,"ĠHowe":38320,"osher":38321,"Ġcustomizable":38322,"ĠFranz":38323,"Ġatro":38324,"Äĩ":38325,"Ġ0004":38326,"Ġoutpost":38327,"Ross":38328,"Ġglyphosate":38329,"ĠHastings":38330,"ĠBEFORE":38331,"Ġshove":38332,"opped":38333,"ĠScala":38334,"Ġamulet":38335,"anian":38336,"Ġexacerbated":38337,"Ġeater":38338,"471":38339,"UME":38340,"Ġpulp":38341,"izontal":38342,"ĠZam":38343,"ĠATI":38344,"immune":38345,"abytes":38346,"Ġunnecessarily":38347,"ĠCAT":38348,"ĠAxis":38349,"Ġvisualize":38350,"Ãī":38351,"ĠRadical":38352,"fm":38353,"Documents":38354,"ĠForrest":38355,"Ġcontextual":38356,"ĠSymbol":38357,"Ġtentative":38358,"ĠDOES":38359,"ĠGoods":38360,"Ġintermittent":38361,"}:":38362,"mediated":38363,"Ġridicule":38364,"Ġatheism":38365,"Ġpathogens":38366,"ĠMum":38367,"Ġreintrodu":38368,"Ġ307":38369,"iHUD":38370,"Ġflashlight":38371,"Ġswearing":38372,"Ġpengu":38373,"Bu":38374,"Ġrotated":38375,"ĠCrane":38376,"Ġ());":38377,"Ġfashionable":38378,"Ġendorsing":38379,"463":38380,")[":38381,"Ġingestion":38382,"Ġcooks":38383,"Ġ950":38384,"otomy":38385,"ĠImam":38386,"Ġka":38387,"Ġteaser":38388,"ĠGhosts":38389,"ĠãĤµ":38390,"1969":38391,"Ïĥ":38392,"ubby":38393,"Ġconverter":38394,"zanne":38395,"ende":38396,"ĠPrepar":38397,"ĠNickel":38398,"ĠChimera":38399,"him":38400,"ĠTyrann":38401,"ĠSabbath":38402,"ĠNichols":38403,"Ġrapt":38404,"ihar":38405,"Ġshelling":38406,"Ġilluminate":38407,"Ġdentist":38408,"utor":38409,"ĠIntegration":38410,"Ġwhims":38411,"ĠLiterary":38412,"Beaut":38413,"Ġparchment":38414,"agara":38415,"Brand":38416,"Ġderog":38417,"â̦)":38418,"ĠNorse":38419,"Ġunwitting":38420,"Ġcuc":38421,"Ġborderline":38422,"Ġupsetting":38423,"Ġrecourse":38424,"Ġdraped":38425,"ĠRadar":38426,"Ġcolder":38427,"ĠPepsi":38428,"iminary":38429,"],[":38430,"658":38431,"Vi":38432,"ĠFrem":38433,"ĠPes":38434,"Ġveterinary":38435,"ĠTED":38436,"ĠEpidem":38437,"nova":38438,"kid":38439,"Ġdevout":38440,"oct":38441,"jad":38442,"Moh":38443,"ĠPAY":38444,"Ġgeometric":38445,"Ġ323":38446,"Ġcircumference":38447,"ichick":38448,"1975":38449,"ĠYuri":38450,"ĠShall":38451,"ĠHover":38452,"unin":38453,"Spr":38454,"Ġgraft":38455,"ĠHappiness":38456,"Ġdisadvantages":38457,"attacks":38458,"Ġhubs":38459,"ĠStarCraft":38460,"éĸ":38461,"Ġgalleries":38462,"ĠKorra":38463,"Ġgroceries":38464,"ĠGorsuch":38465,"Ġrapists":38466,"Ġfungi":38467,"ĠTyphoon":38468,"Vector":38469,"ĠEmpress":38470,"battle":38471,"468":38472,"Ġparasite":38473,"ĠBomber":38474,"SG":38475,"exist":38476,"ĠPf":38477,"Ġunse":38478,"Ġsurgeons":38479,"Birth":38480,"ĠUnsure":38481,"ĠPrinted":38482,"ĠBehavioral":38483,"ĠAster":38484,"Pakistan":38485,"Ġunethical":38486,"Ġsv":38487,"ĠIoT":38488,"Ġlayouts":38489,"Pain":38490,"Ġconstants":38491,"ĠLW":38492,"ĠBake":38493,"Ġtowels":38494,"Ġdeterioration":38495,"ĠBolivia":38496,"Ġblinded":38497,"ĠWarden":38498,"ĠMistress":38499,"Ġonstage":38500,"Ġclans":38501,"ĠBEST":38502,"1960":38503,"Ġantique":38504,"Ġrhetorical":38505,"ĠPercy":38506,"ĠRwanda":38507,",.":38508,"Bruce":38509,"Ġtraumat":38510,"ĠParliamentary":38511,"Ġfootnote":38512,"idia":38513,"ĠLearned":38514,"seeking":38515,"genic":38516,"Ġdimensional":38517,"Hide":38518,"èĢħ":38519,"Ġintrigue":38520,"inse":38521,"Ġleases":38522,"Ġapprentices":38523,"washing":38524,"Ġ1926":38525,"VILLE":38526,"Ġswoop":38527,"scl":38528,"Ġbedrooms":38529,"onics":38530,"ĠCrunch":38531,"compatible":38532,"Ġincapac":38533,"ĠYemeni":38534,"ashtra":38535,"zhou":38536,"danger":38537,"Ġmanifestations":38538,"ĠDemons":38539,"AAF":38540,"Secretary":38541,"ACTED":38542,"LOD":38543,"Ġamy":38544,"raper":38545,"ethnic":38546,"417":38547,"Ġpositives":38548,"Ġ273":38549,"ĠRefugees":38550,"Ġusb":38551,"ĠVald":38552,"oddy":38553,"ĠMahmoud":38554,"Asia":38555,"Ġskulls":38556,"ĠExodus":38557,"ĠCompet":38558,"ĠLIC":38559,"ĠMansion":38560,"ĠAme":38561,"Ġconsolidate":38562,"storms":38563,"ontent":38564,"996":38565,"Ġclen":38566,"Ġmummy":38567,"flat":38568,"758":38569,"ĠVOL":38570,"oteric":38571,"nen":38572,"ĠMinute":38573,"Sov":38574,"Ġfiner":38575,"Rh":38576,"lycer":38577,"Ġreinforcements":38578,"ĠJohannes":38579,"ĠGallagher":38580,"Ġgymn":38581,"Suddenly":38582,"Ġextortion":38583,"kr":38584,"iator":38585,"Ta":38586,"Ġhippocampus":38587,"NPR":38588,"ĠComputing":38589,"Ġsquarely":38590,"Ġmodelling":38591,"ĠForums":38592,"ĠLisp":38593,"ĠKrishna":38594,"Ġ324":38595,"Ġrushes":38596,"Ġensued":38597,"Ġcreeping":38598,"onte":38599,"nai":38600,"ilater":38601,"ĠHornets":38602,"Ġoblivious":38603,"INST":38604,"559":38605,"Ġjeopardy":38606,"Ġdistinguishing":38607,"jured":38608,"Ġbegs":38609,"similar":38610,"phot":38611,"530":38612,"ĠParkway":38613,"Ġsinks":38614,"ĠHearthstone":38615,"ibur":38616,"ĠBaton":38617,"Avoid":38618,"Ġdancer":38619,"Ġmagistrate":38620,"aryn":38621,"Ġdisturbances":38622,"ĠRomero":38623,"Ġparaph":38624,"Ġmischief":38625,"âĸĵ":38626,"ĠSharia":38627,"Ġurinary":38628,"route":38629,"ivas":38630,"fitted":38631,"Ġejected":38632,"ĠAlbuquerque":38633,"Ġ470":38634,"Ġirritated":38635,"ĠZip":38636,"ĠBiol":38637,"Ãį":38638,"Ġdenounce":38639,"Ġbinaries":38640,"ĠVerse":38641,"Ġoppos":38642,"ĠKendrick":38643,"ĠGPL":38644,"Ġspew":38645,"ĠElijah":38646,"ĠEas":38647,"Ġdrifted":38648,"sofar":38649,"Ġannoyance":38650,"ĠBET":38651,"474":38652,"ĠStrongh":38653,"itates":38654,"ĠCognitive":38655,"ophone":38656,"ĠIdentification":38657,"ocrine":38658,"connection":38659,"Ġboxer":38660,"ĠASD":38661,"ĠAreas":38662,"Yang":38663,"tch":38664,"ullah":38665,"Ġdeceive":38666,"Combat":38667,"episode":38668,"crete":38669,"Witness":38670,"Ġcondolences":38671,"htar":38672,"Ġheals":38673,"Ġbuckets":38674,"ĠLAW":38675,"Blu":38676,"Ġslab":38677,"ĠORDER":38678,"ocl":38679,"atton":38680,"ĠStevenson":38681,"ĠGinger":38682,"ĠFriendly":38683,"ĠVanderbilt":38684,"spirit":38685,"igl":38686,"ĠRegarding":38687,"ĠPROG":38688,"Ġsealing":38689,"starting":38690,"Ġcardinal":38691,"ĠVec":38692,"ĠBeir":38693,"Ġmilliseconds":38694,"weak":38695,"perse":38696,"Ġsterile":38697,"ĠContemporary":38698,"ĠPhant":38699,"ĠClo":38700,"Ġoutp":38701,"Ġexiled":38702,"Ġ277":38703,"Ġselfie":38704,"Ġmanic":38705,"Ġnano":38706,"terms":38707,"Alexander":38708,"Ġresolves":38709,"Ġmillennia":38710,"Ġexplodes":38711,"Ġconstellation":38712,"Ġadultery":38713,"motion":38714,"DOC":38715,"Ġbroadcasters":38716,"Ġkindergarten":38717,"ĠMayweather":38718,"ĠEco":38719,"icho":38720,"Ġ287":38721,"laun":38722,"Ġmute":38723,"Ġdiscreet":38724,"Ġpreschool":38725,"Ġpreempt":38726,"Delete":38727,"ĠFreed":38728,"Pi":38729,"HK":38730,"Ġblocker":38731,"ĠCumber":38732,"Ġwrought":38733,"dating":38734,"Ġinsurer":38735,"Ġquotas":38736,"Ġpreached":38737,"Ġeviction":38738,"ĠRegina":38739,"ĠPens":38740,"Ġseventeen":38741,"ĠNass":38742,"Dick":38743,"Ġfolds":38744,"Ġdotted":38745,"ĠAad":38746,"Universal":38747,"Ġpizz":38748,"ĠGuru":38749,"Ġsoils":38750,"Ġnovice":38751,"ĠNeander":38752,"Ġstool":38753,"Ġdetonated":38754,"ĠPikachu":38755,"ĠMassive":38756,"IVER":38757,"ĠAbdel":38758,"Ġsubdued":38759,"Ġtallest":38760,"Ġprecarious":38761,"Ġay":38762,"rification":38763,"ĠObj":38764,"cale":38765,"Ġunquestion":38766,"culosis":38767,"adas":38768,"igrated":38769,"Days":38770,"Ġqueens":38771,"ĠGazette":38772,"ĠColour":38773,"ĠBowman":38774,"ĠJJ":38775,"ïve":38776,"Ġdominates":38777,"Student":38778,"Ġmu":38779,"Ġbacklog":38780,"ĠElectro":38781,"Truth":38782,"483":38783,"Ġcondensed":38784,"rules":38785,"ĠConspiracy":38786,"Ġacronym":38787,"handled":38788,"ĠMatte":38789,"jri":38790,"ĠImpossible":38791,"lude":38792,"creation":38793,"Ġwarmed":38794,"ĠSlave":38795,"Ġmisled":38796,"Ġferment":38797,"ĠKah":38798,"inki":38799,"keleton":38800,"cyl":38801,"ĠKarin":38802,"Hunter":38803,"Register":38804,"ĠSurrey":38805,"Ġstares":38806,"ĠWidth":38807,"ĠNay":38808,"ĠSki":38809,"Ġblacklist":38810,"ucket":38811,"Ġexpulsion":38812,"imet":38813,"Ġretweet":38814,"vantage":38815,"Feature":38816,"Ġtroopers":38817,"Ġhomers":38818,"969":38819,"Ġcontingency":38820,"ĠWTC":38821,"ĠBrewer":38822,"foreign":38823,"Ware":38824,"Solar":38825,"Ġundue":38826,"REC":38827,"ulnerable":38828,"pathic":38829,"ĠBoise":38830,"Ġ322":38831,"Ġaroused":38832,"ĠYing":38833,"ä¸į":38834,"ueless":38835,"Ġpas":38836,"Ġmorp":38837,"Ġfloral":38838,"Express":38839,"udging":38840,"kB":38841,"ĠGranted":38842,"د":38843,"ĠMicha":38844,"ĠGothic":38845,"ĠSPECIAL":38846,"ĠRicardo":38847,"Fran":38848,"Ġadministering":38849,"620":38850,"pora":38851,"Ġ®":38852,"Ġcompromises":38853,"Ġbitten":38854,"Accept":38855,"Thirty":38856,"в":38857,"Ġmaterially":38858,"ĠTerr":38859,"igmatic":38860,"chains":38861,"Ġdove":38862,"stadt":38863,"Marvel":38864,"FAULT":38865,"Ġwindshield":38866,"Ġ336":38867,"adier":38868,"Ġswapping":38869,"Ġflawless":38870,"ĠPredator":38871,"ĠMichele":38872,"Ġpropulsion":38873,"ĠPsychic":38874,"Ġassigning":38875,"Ġfabrication":38876,"Ġbarley":38877,"lust":38878,"Ġtowering":38879,"Ġaltercation":38880,"ĠBentley":38881,"Sphere":38882,"Ġtuna":38883,"ĠClasses":38884,"Freedom":38885,"uner":38886,"Lady":38887,"voice":38888,"Ġcoolest":38889,"orr":38890,"Ġpalp":38891,"${":38892,"Ġhysteria":38893,"ĠMetatron":38894,"pants":38895,"Ġspawning":38896,"Experts":38897,"ĠInvestors":38898,"ĠAnarchy":38899,"Ġshrunk":38900,"ĠVictim":38901,"Ġ289":38902,"Ġecstasy":38903,"ĠBinding":38904,"585":38905,"ĠMelody":38906,"578":38907,"otally":38908,"ĠEtsy":38909,"liga":38910,"Ġapplauded":38911,"Ġsweating":38912,"Ġredistributed":38913,"Ġpopcorn":38914,"Ġseminal":38915,"fur":38916,"ĠNeuroscience":38917,"Rand":38918,"ĠOst":38919,"ĠMadden":38920,"ĠIncreasing":38921,"ĠDawkins":38922,"ĠSubway":38923,"Ġarsen":38924,"conserv":38925,"BUR":38926,"Ġspiked":38927,"ĠLyft":38928,"ĠImperium":38929,"ĠDropbox":38930,"Ġfavoured":38931,"Ġencompasses":38932,"ghost":38933,"Ġinspires":38934,"Ġburgeoning":38935,"ĠYoshi":38936,"ĠVertical":38937,"ĠAuditor":38938,"Ġintending":38939,"Ġfilibuster":38940,"Bloom":38941,"fac":38942,"ĠCavs":38943,"igning":38944,"Ġcoworkers":38945,"ĠBarbarian":38946,"remember":38947,"FLAG":38948,"Ġauditory":38949,"asonry":38950,"College":38951,"Ġmuted":38952,"gemony":38953,"obin":38954,"ĠPsycho":38955,"968":38956,"Ġlavish":38957,"Ġhierarchical":38958,"ĠDrone":38959,"ouk":38960,"Ġcrippled":38961,"ĠMaxim":38962,"Slot":38963,"Ġquiz":38964,"ĠVid":38965,"ifling":38966,"Ġarchaeologists":38967,"Ġabandonment":38968,"dial":38969,"leon":38970,"ĠFas":38971,"Ted":38972,"Ġraspberry":38973,"Ġmaneuvers":38974,"Ġbehaviours":38975,"Ġinsure":38976,"Ġremod":38977,"Switch":38978,"hoe":38979,"Ġspaced":38980,"Ġaffordability":38981,"ĠFern":38982,"notation":38983,"ĠBalanced":38984,"Ġoccupies":38985,"environment":38986,"Ġnecklace":38987,"Ġsedan":38988,"FU":38989,"ĠBravo":38990,"Ġabusers":38991,"ĠAnita":38992,"metadata":38993,"ĠGithub":38994,"aito":38995,"ĠFaster":38996,"ĠWasserman":38997,"ĠFlesh":38998,"Ġthorn":38999,"rarily":39000,"ĠMerry":39001,"wine":39002,"Ġpopulace":39003,"ĠLann":39004,"Ġrepairing":39005,"Ġpsyche":39006,"Ġmodulation":39007,"awaru":39008,"âĢĭâĢĭ":39009,"arij":39010,"Ġdecorations":39011,"Ġapologise":39012,"ĠGarg":39013,"apply":39014,"Ġgiveaway":39015,"ĠFlan":39016,"ĠWyatt":39017,"Uber":39018,"Ġauthorised":39019,"ĠMoral":39020,"HAHAHAHA":39021,"activate":39022,"Ġtorpedo":39023,"ĠFAR":39024,"Ġamassed":39025,"ĠAram":39026,"arkin":39027,"ĠVictims":39028,"stab":39029,"Ġom":39030,"ĠECO":39031,"Ġopioids":39032,"Ġpurposely":39033,"ĠVest":39034,"Ġerg":39035,"atan":39036,"ĠSurgery":39037,"Ġcorrecting":39038,"ĠOrtiz":39039,"ĠBeet":39040,"Ġrevoke":39041,"Ġfreeway":39042,"ĠHiggins":39043,"Fail":39044,"ĠFarms":39045,"ĠATP":39046,"hound":39047,"Ġpoking":39048,"ĠCommunists":39049,"monster":39050,"imentary":39051,"Ġunlocking":39052,"Ġunfit":39053,"weed":39054,"enario":39055,"atical":39056,"ĠEnlightenment":39057,"ĠNG":39058,"ĠCompensation":39059,"deen":39060,"ĠWidow":39061,"ĠCindy":39062,"ĠAfterwards":39063,"Ġ6000":39064,"ikhail":39065,"agically":39066,"Ġratified":39067,"Ġcasualty":39068,"HOME":39069,"psey":39070,"fee":39071,"Ġsparkling":39072,"Ġdé":39073,"Ġconcerted":39074,"Catal":39075,"Ġcomplying":39076,"ĠAres":39077,"ĠDent":39078,"Shut":39079,"Ġskim":39080,"administ":39081,"Ġhostilities":39082,"ĠGins":39083,"Ġ608":39084,"Ġmuddy":39085,"ĠMcInt":39086,"ĠDecay":39087,"525":39088,"Ġconspicuous":39089,"ĠExposure":39090,"Ġrescind":39091,"Ġwearable":39092,"Ġ328":39093,"ourmet":39094,"ahs":39095,"ĠRobots":39096,"Ġeclips":39097,"instance":39098,"ĠREPORT":39099,"ĠAppl":39100,"030":39101,"ĠSkies":39102,"0100":39103,"Ġfallacy":39104,"Socket":39105,"ĠReceiver":39106,"Ġsolves":39107,"ĠButterfly":39108,"ĠShopping":39109,"ĠFIRE":39110,"654":39111,"Medic":39112,"Ġsingers":39113,"ĠNeedless":39114,"''''":39115,"ishers":39116,"ĠDive":39117,"588":39118,"Ġselectively":39119,"Ġclumsy":39120,"889":39121,"Ġpurchaser":39122,"earned":39123,"ardy":39124,"Ġbenefiting":39125,"english":39126,"Ġyielding":39127,"ĠPour":39128,"Ġspinach":39129,"Ġdelve":39130,"ĠCrom":39131,"610":39132,"Ġexporting":39133,"ĠMAKE":39134,"Ġ263":39135,"Ġgrop":39136,"Ġenvoy":39137,"ĠInquiry":39138,"ĠLuigi":39139,"dry":39140,"ĠTuring":39141,"ThumbnailImage":39142,"ĠVariety":39143,"Ġfacet":39144,"Ġfluffy":39145,"Ġexcerpts":39146,"Ġshorth":39147,"ĠOlsen":39148,"CLUD":39149,"Ġreliant":39150,"ĠUNC":39151,"Tour":39152,"Ġbathing":39153,"Company":39154,"Ġglobalization":39155,"Pred":39156,"ĠMalfoy":39157,"Ġhoc":39158,"jam":39159,"crafted":39160,"ĠBonds":39161,"ĠKissinger":39162,"England":39163,"Ġorderly":39164,"catentry":39165,"Ġ261":39166,"Ġexchanging":39167,"ĠIntent":39168,"ĠAmendments":39169,"DOM":39170,"Ġstout":39171,"³³³³³³³³³³³³³³³³":39172,"ĠAirbus":39173,"Ġ278":39174,"hyde":39175,"Poll":39176,"ItemThumbnailImage":39177,"Ġloopholes":39178,"ĠPillar":39179,"Ġexplor":39180,"Stretch":39181,"Apart":39182,"Ġunmarried":39183,"Limit":39184,"ĠTransformers":39185,"Ġintellectually":39186,"uncture":39187,"1800":39188,"Ġdarn":39189,"Brazil":39190,"Ġleftover":39191,"berus":39192,"fred":39193,"Minecraft":39194,"326":39195,"ĠForms":39196,"Ġproofs":39197,"ĠDesigned":39198,"Ġindexes":39199,"ĠSuppose":39200,"EMS":39201,"ĠLoving":39202,"ĠBonnie":39203,"imating":39204,"OTUS":39205,"Ġconductor":39206,"Ġbehaved":39207,"ĠFren":39208,"Ġsynerg":39209,"Ġmillennium":39210,"Ġcatering":39211,"ĠLauder":39212,"Wr":39213,"ĠYiannopoulos":39214,"ĠATF":39215,"Ġenslaved":39216,"Ġawakened":39217,"DVD":39218,"ĠEDITION":39219,"ĠConcert":39220,"ĠChallenger":39221,"ĠHaku":39222,"umeric":39223,"Ġdeprecated":39224,"ĠSHAR":39225,"412":39226,"Ġdystop":39227,"Ġtrembling":39228,"Ġdreaded":39229,"ĠSpac":39230,"padding":39231,"Repl":39232,"ĠGarrison":39233,"Mini":39234,"Ġunparalleled":39235,"amar":39236,"URRENT":39237,"wreck":39238,"certain":39239,"tal":39240,"ĠCLS":39241,"appings":39242,"Ġsensed":39243,"Ġfencing":39244,"ĠPaso":39245,"ĠDesk":39246,"Ġscoff":39247,"Ġcontemplate":39248,"ĠLiga":39249,"liquid":39250,"757":39251,"Ġapprentice":39252,"ĠUCHIJ":39253,"570":39254,"ĠThousand":39255,"ĠIllum":39256,"Ġchampioned":39257,"ãĤĮ":39258,"Ġelectors":39259,"Ġ398":39260,"ĠHancock":39261,"rounded":39262,"ĠJOHN":39263,"Ġunsatisf":39264,"Ġqualifier":39265,"ĠGadget":39266,"ENE":39267,"Ġdeadliest":39268,"ĠPlants":39269,"Ġions":39270,"Ġaccents":39271,"Ġtweaking":39272,"Ġshaved":39273,"FREE":39274,"ĠChaser":39275,"Against":39276,"960":39277,"Ġmethamphetamine":39278,"Ġnormalized":39279,"Ġ$\\":39280,"ĠPrecision":39281,"ĠGuam":39282,"Ġchoked":39283,"ĠXII":39284,"ĠCasting":39285,"Torrent":39286,"Ġscalp":39287,"ĠJaguar":39288,"wit":39289,"Ġsemic":39290,"ixie":39291,"ĠGould":39292,"Ġconfines":39293,"Nusra":39294,"ĠLon":39295,"ĠJugg":39296,"ycle":39297,"ĠCodec":39298,"Egypt":39299,"Ġrestrain":39300,"ĠAliens":39301,"Ġchoking":39302,"ĠDunk":39303,"ĠBella":39304,"abc":39305,"Ġslang":39306,"Ġneurotrans":39307,"sav":39308,"Ġempowerment":39309,"âĨĴ":39310,"Ġclimbers":39311,"ĠMim":39312,"ĠFra":39313,"rosse":39314,"Capital":39315,"ĠCthulhu":39316,"Interface":39317,"Ġproficient":39318,"ĠINTO":39319,"Ġ318":39320,"rontal":39321,"580":39322,"ĠDespair":39323,"Kenn":39324,"Ġscrimmage":39325,"ĠCoat":39326,"asions":39327,"Ġwallpaper":39328,"ĠJol":39329,"Ġresurgence":39330,"Ġantiv":39331,"ĠBalls":39332,"²¾":39333,"Ġbuffers":39334,"Ġsubsystem":39335,"ĠStellar":39336,"ĠLung":39337,"AIDS":39338,"Ġeradicate":39339,"Ġblatantly":39340,"Ġbehaves":39341,"ĠNun":39342,"Ġantics":39343,"export":39344,"DEV":39345,"wb":39346,"Ġphp":39347,"ĠIntegrity":39348,"Ġexplorer":39349,"Ġrevolving":39350,"authored":39351,"gans":39352,"Ġbask":39353,"Ġasynchronous":39354,"åį":39355,"THING":39356,"698":39357,"Gene":39358,"ĠRacer":39359,"ĠNico":39360,"issued":39361,"Ġsermon":39362,"possibly":39363,"Ġsizeof":39364,"Ġentrepreneurial":39365,"oxin":39366,"ĠMinerva":39367,"Ġplatoon":39368,"nos":39369,"riks":39370,"AUT":39371,"ĠAvalanche":39372,"ĠDesc":39373,"ij士":39374,"ĠPoc":39375,"Ġconferred":39376,"λ":39377,"Ġpatched":39378,"FBI":39379,"662":39380,"Ġfractures":39381,"Ġdetects":39382,"Ġdedicate":39383,"Ġconstituent":39384,"Ġcosmos":39385,"WT":39386,"Ġsweats":39387,"Ġsprung":39388,"bara":39389,"solid":39390,"Ġunsus":39391,"Ġbulky":39392,"ĠPhilippe":39393,"ĠFenrir":39394,"Ġtherapists":39395,"oreal":39396,"^^^^":39397,"Ġtotaled":39398,"Ġbooze":39399,"ĠRPC":39400,"Prosecutors":39401,"Ġdiseng":39402,"ĠShared":39403,"Ġmotorcycles":39404,"Ġinventions":39405,"Ġlettuce":39406,"ĠMerge":39407,"ĠJC":39408,"Ġspirituality":39409,"ĠWARNING":39410,"Ġunlucky":39411,"ĠTess":39412,"Ġtongues":39413,"ĠDUI":39414,"Tumblr":39415,"Ġleans":39416,"Ġinvaders":39417,"Ġcanopy":39418,"ĠHurricanes":39419,"ĠBret":39420,"ĠAPPLIC":39421,"idine":39422,"ickle":39423,"Regarding":39424,"Ġveggies":39425,"Ġejac":39426,"juven":39427,"Fish":39428,"DEM":39429,"ĠDino":39430,"Throw":39431,"ĠChecking":39432,"beard":39433,"(&":39434,"Ġjails":39435,"Ġhr":39436,"transfer":39437,"ivating":39438,"Ġfleets":39439,"ĠImag":39440,"ĠMcDonnell":39441,"Ġsnippet":39442,"Isa":39443,"ĠChatt":39444,"ĠStain":39445,"ĠSetFontSize":39446,"ĠOy":39447,"ĠMathematics":39448,"494":39449,"Ġelectroly":39450,"ĠGott":39451,"ĠBras":39452,"BOOK":39453,"ĠFinger":39454,"dump":39455,"Ġmutants":39456,"Ġrentals":39457,"Ġintertw":39458,"Ġcreek":39459,"aila":39460,"Brother":39461,"ĠDiscord":39462,"pee":39463,"rawler":39464,"Ġcarp":39465,"Ġ279":39466,"ãĤ·ãĥ£":39467,"relations":39468,"Ġcontrasts":39469,"Column":39470,"Ġreconnaissance":39471,"Ġunknow":39472,"Ġlooting":39473,"Ġregulates":39474,"Ġoptimum":39475,"ĠCherokee":39476,"ĠAry":39477,"Latest":39478,"Ġroadside":39479,"Ġdanced":39480,"ĠUnicorn":39481,"Acknowled":39482,"Ġuncontroll":39483,"ĠMUS":39484,"atio":39485,"chance":39486,"haven":39487,"VALUE":39488,"Ġfavourites":39489,"Ġceremonial":39490,"binary":39491,"peed":39492,"woods":39493,"EMP":39494,"Ġvascular":39495,"Ġcontemplated":39496,"Ġbarren":39497,"ĠLIST":39498,"Yellow":39499,"osponsors":39500,"Ġwhisky":39501,"ĠMamm":39502,"ĠDeVos":39503,"minimum":39504,"Hung":39505,"442":39506,"Pic":39507,"ĠSnapdragon":39508,"776":39509,"Ġcarving":39510,"Ġundecided":39511,"Ġadvantageous":39512,"Ġpalms":39513,"ĠAQ":39514,"Ġstarch":39515,"Loop":39516,"Ġpaddle":39517,"Ġflaming":39518,"ĠHorizons":39519,"Animation":39520,"boost":39521,"Ġprobabilities":39522,"ĠMish":39523,"Ġexodus":39524,"ĠEditorial":39525,"Ġfungus":39526,"Ġdissenting":39527,"ĠDelicious":39528,"rogram":39529,"ĠDyn":39530,"disk":39531,"tom":39532,"Ġfabrics":39533,"ĠCove":39534,"ĠBans":39535,"Ġsoften":39536,"ĠCONS":39537,"Ġineligible":39538,"Ġestimating":39539,"ĠLexington":39540,"practice":39541,"ofi":39542,"Ġshedding":39543,"ĠNope":39544,"Ġbreathed":39545,"ĠCorinthians":39546,"yne":39547,"eki":39548,"Bull":39549,"Ġattaching":39550,"reenshots":39551,"Ġanalyse":39552,"ĠKappa":39553,"Ġunsustainable":39554,"Ġinterpol":39555,"anky":39556,"hemer":39557,"Ġprotagonists":39558,"Ġformatted":39559,"ĠBryce":39560,"ĠAchilles":39561,"ĠAbedin":39562,"shock":39563,"Ġbum":39564,"bos":39565,"qua":39566,"ĠWarn":39567,"qt":39568,"ĠDiabetes":39569,"864":39570,"ĠInvisible":39571,"Ġvanish":39572,"Ġtransmitting":39573,"Ġmurky":39574,"ĠFei":39575,"Ġawaited":39576,"ĠJurassic":39577,"ummies":39578,"Ġmenacing":39579,"gall":39580,"Cath":39581,"Built":39582,"ildo":39583,"ĠVotes":39584,"Ġont":39585,"Ġmunitions":39586,"ĠFreem":39587,"ÃŃn":39588,"Ġdecency":39589,"lopp":39590,"ieved":39591,"ĠGord":39592,"Ġunthinkable":39593,"ĠNewsweek":39594,"Ġ321":39595,"Heat":39596,"Ġpresenter":39597,"jiang":39598,"Ġplank":39599,"ĠAvalon":39600,"Ġbenz":39601,"ĠRout":39602,"Ġslamming":39603,"ĠDai":39604,"outer":39605,"ĠCookie":39606,"ĠAlicia":39607,"gey":39608,"Ġvanity":39609,"Ġowl":39610,"áµ":39611,"tested":39612,"ĠAwakens":39613,"Ġcanv":39614,"Ġblindly":39615,"ĠRidley":39616,"ĠEmails":39617,"Requires":39618,"ĠSerbian":39619,"ographed":39620,"iframe":39621,"eteria":39622,"Ġalternating":39623,"quiet":39624,"Ġsociology":39625,"ĠUnlock":39626,"ĠCommunism":39627,"Ġops":39628,"Ġattribution":39629,"Ġabduction":39630,"ĠAbram":39631,"Ġsidelined":39632,"ĠBOOK":39633,"Ġrefining":39634,"ĠFeeling":39635,"ĠOslo":39636,"ĠPruitt":39637,"rack":39638,"angible":39639,"Ġcautiously":39640,"ĠMARK":39641,"eeds":39642,"Mouse":39643,"ĠSteph":39644,"ĠPair":39645,"Sab":39646,"997":39647,"ĠBaal":39648,"Bec":39649,"Ġcomma":39650,"ĠPall":39651,"ĠGael":39652,"Ġmisunderstand":39653,"ĠPesh":39654,"Orderable":39655,"Ġdismal":39656,"ĠShiny":39657,"%\"":39658,"Ġrealistically":39659,"Ġpatio":39660,"ĠGw":39661,"ĠVirtue":39662,"Ġexhausting":39663,"whatever":39664,"ophys":39665,"yip":39666,"418":39667,"Adjust":39668,"ĠWaiting":39669,"esson":39670,"ĠMazda":39671,"ĠDozens":39672,"Ġstreamlined":39673,"Ġincompetence":39674,"ĠMeth":39675,"Ġethos":39676,"ONES":39677,"Ġincentiv":39678,"Ġgritty":39679,"ĠButcher":39680,"Header":39681,"Ġexponential":39682,"ÃŁ":39683,"Ġcorrelate":39684,"Ġconsensual":39685,"sounding":39686,"Ring":39687,"Origin":39688,"Ġconclusive":39689,"feet":39690,"acly":39691,"ĠFernandez":39692,"Buyable":39693,"Ġducks":39694,"auntlets":39695,"Ġelong":39696,"Ġ286":39697,"Ġsimul":39698,"Gas":39699,"ĠKirst":39700,"Ġprotr":39701,"ĠRobo":39702,"ĠAoE":39703,"opol":39704,"Ġpsychologically":39705,"spin":39706,"ilaterally":39707,"ĠConrad":39708,"Wave":39709,"441":39710,"ĠAdvertisement":39711,"ĠHarmon":39712,"ĠOriental":39713,"isSpecial":39714,"Ġpresumptive":39715,"Ġwil":39716,"ĠKier":39717,"nea":39718,"Ġppm":39719,"Ġharbour":39720,"ĠWired":39721,"company":39722,"Ġcoroner":39723,"aturdays":39724,"ĠProud":39725,"ĠNEXT":39726,"ĠFlake":39727,"valued":39728,"ceiver":39729,"Ġfraught":39730,"Ġcasing":39731,"Ġrunaway":39732,"Ġgin":39733,"ĠLaurent":39734,"ĠHarlem":39735,"ĠCuriosity":39736,"quished":39737,"Ġneuroscience":39738,"ĠHulu":39739,"Ġborrower":39740,"Ġpetitioner":39741,"ĠCooldown":39742,"WARD":39743,"Ġinvoking":39744,"confidence":39745,"Forward":39746,"Ġsts":39747,"population":39748,"DeliveryDate":39749,"Film":39750,"ĠCov":39751,"quickShip":39752,"quickShipAvailable":39753,"primary":39754,"isSpecialOrderable":39755,"inventoryQuantity":39756,"channelAvailability":39757,"BOX":39758,"ĠMultiplayer":39759,"ĠJenner":39760,"778":39761,"ĠMd":39762,"Ġ~/.":39763,"MN":39764,"Ġchildish":39765,"Ġantioxidant":39766,"ĠChromebook":39767,"Ġ274":39768,"Ġscreenplay":39769,"Ġadventurous":39770,"ĠRelationship":39771,"responsive":39772,"mington":39773,"Ġcornerstone":39774,"ĠFey":39775,"FIR":39776,"Ġrookies":39777,"ĠFeaturing":39778,"Ġoriginate":39779,"Ġelectrodes":39780,"antes":39781,"Ġscriptures":39782,"Ġglued":39783,"Ġdiscontent":39784,"Ġafflicted":39785,"layout":39786,"Brave":39787,"Ġmosa":39788,"ĠQuantity":39789,"ĠHik":39790,"winner":39791,"Hours":39792,"Ġentail":39793,"ĠCells":39794,"ologue":39795,"Ġvil":39796,"Ġpreacher":39797,"Ġdecorative":39798,"different":39799,"Ġprejudices":39800,"ĠSmoking":39801,"ĠNottingham":39802,"soType":39803,"Ġrhythms":39804,"ĠAlph":39805,"blast":39806,"Steel":39807,"ĠDanielle":39808,"Ġstrife":39809,"Ġrematch":39810,"soDeliveryDate":39811,"ĠFork":39812,"trip":39813,"olulu":39814,"heses":39815,"CG":39816,"ĠPOLITICO":39817,"osta":39818,"ĠDrift":39819,"é¾įå¥":39820,"é¾įå¥ij士":39821,"Ġvetting":39822,"ĠJinping":39823,"ĠRecession":39824,"Minor":39825,"ĠFraud":39826,"enfranch":39827,"Ġconvened":39828,"ĠNAACP":39829,"ĠMillions":39830,"ĠFarming":39831,"ĠWoo":39832,"ĠFlare":39833,"rito":39834,"immigrant":39835,"Ġvacancy":39836,"ĠHEAD":39837,"ĠVaj":39838,"egal":39839,"ĠVigil":39840,"Study":39841,"Ġruining":39842,"Ġracks":39843,"Ġheater":39844,"ĠRandolph":39845,"ĠBrush":39846,"ĠTir":39847,"ب":39848,"Ġcov":39849,"%]":39850,"Ġrecounts":39851,"ĠOPT":39852,"ĠMelt":39853,"Ġtruce":39854,"Ġcasinos":39855,"Ġcrusade":39856,"Ġcarnage":39857,"Ġstripe":39858,"ĠKyl":39859,"Textures":39860,"Ġ698":39861,"Ġproclamation":39862,"Ġgoodies":39863,"Ġ..........":39864,"proclaimed":39865,"Polit":39866,"Ġtopical":39867,"Ġspecialize":39868,"ĠAmin":39869,"gm":39870,"Ġanchored":39871,"Ġbearings":39872,"sample":39873,"ĠHighland":39874,"ĠAutism":39875,"Ġmercenary":39876,"Ġinterviewer":39877,"LER":39878,"ĠSomers":39879,"Ġembryo":39880,"ĠAssy":39881,"Ġ281":39882,"ĠEditing":39883,"ĠChosen":39884,"660":39885,"Ġpci":39886,"ĠThunderbolt":39887,"BILL":39888,"Ġchuckled":39889,"jriwal":39890,"hof":39891,"Ġearthly":39892,"(){":39893,"independence":39894,"Ġdispers":39895,"ĠVendor":39896,"ĠGareth":39897,"Ġpals":39898,"Penn":39899,"ĠSubmit":39900,"icum":39901,"Thu":39902,"Ġclandestine":39903,"Ġcannibal":39904,"ĠClerk":39905,"EStream":39906,"galitarian":39907,"âĻ¥":39908,"gew":39909,"Ġhorrend":39910,"ĠLov":39911,"ĠReaction":39912,"ocrin":39913,"Classic":39914,"Ġechoing":39915,"Ġdisclosing":39916,"ĠInsight":39917,"ogun":39918,"ĠIncarn":39919,"uploads":39920,"pperc":39921,"guyen":39922,"Ġ1901":39923,"ĠBars":39924,"687":39925,"Ġbribes":39926,"ĠFresno":39927,"urat":39928,"ĠReese":39929,"Ġintrusive":39930,"Ġgripping":39931,"ĠBlueprint":39932,"ĠRasm":39933,"unia":39934,"managed":39935,"ĠHebdo":39936,"Ġ345":39937,"Ġdecoding":39938,"Ġpoets":39939,"Ġjaws":39940,"ĠFIGHT":39941,"ameless":39942,"ĠMeadows":39943,"ĠHarbaugh":39944,"Interview":39945,"ĠHosp":39946,"ĠBRA":39947,"Ġdeletion":39948,"mob":39949,"Walker":39950,"ĠMoonlight":39951,"ĠJed":39952,"ĠSophia":39953,"Ġusur":39954,"Ġfortunately":39955,"ĠPutting":39956,"ĠFold":39957,"Ġsanitation":39958,"Ġpartisans":39959,"ISON":39960,"Bow":39961,"ĠCONC":39962,"ĠReduced":39963,"ĠSutton":39964,"Ġtouchscreen":39965,"Ġembryos":39966,"âĢ¢âĢ¢âĢ¢âĢ¢":39967,"ĠKrug":39968,"combat":39969,"ĠPetroleum":39970,"Ġamd":39971,"ĠCosmos":39972,"Ġprescribing":39973,"Ġconformity":39974,"ourses":39975,"Ġplentiful":39976,"Ġdisillusion":39977,"ĠEcology":39978,"ittal":39979,"Ġfanc":39980,"Ġassassinated":39981,"regnancy":39982,"Ġperennial":39983,"ĠBullets":39984,"Ġstale":39985,"Ġcached":39986,"ĠJudith":39987,"ĠDiseases":39988,"Allen":39989,"Ġlas":39990,"Ġshards":39991,"ĠSuarez":39992,"ĠFriendship":39993,"interface":39994,"ĠSupporters":39995,"addons":39996,"462":39997,"ĠImran":39998,"ĠWim":39999,"Ġnewfound":40000,"ĠMb":40001,"Animal":40002,"Ġdarling":40003,"ande":40004,"Ġrhy":40005,"ĠTwisted":40006,"posal":40007,"ynski":40008,"Various":40009,"׾":40010,"ĠKiw":40011,"uyomi":40012,"Ġwellbeing":40013,"ĠLau":40014,"anos":40015,"Ġunmist":40016,"ĠmacOS":40017,"Ġrestroom":40018,"ĠOliv":40019,"ĠAirways":40020,"Ġtimetable":40021,"980":40022,"Ġradios":40023,"voy":40024,"iasco":40025,"Ġcloudy":40026,"ĠDrawing":40027,"Anything":40028,"Syria":40029,"ĠHert":40030,"staking":40031,"Ġunchecked":40032,"Ġbrazen":40033,"ĠNRS":40034,"697":40035,"onomic":40036,"establish":40037,"Ġleng":40038,"Ġdiagonal":40039,"ĠFior":40040,"Lair":40041,"ĠStard":40042,"Ġdeficient":40043,"joining":40044,"beam":40045,"Ġomnip":40046,"Ġblender":40047,"Ġsunrise":40048,"Moore":40049,"ĠFault":40050,"ĠCostume":40051,"ĠMub":40052,"Flags":40053,"anse":40054,"Ġpayout":40055,"ĠGovernors":40056,"ĠDillon":40057,"ĠBanana":40058,"Nar":40059,"Ġtrailed":40060,"Ġimperialist":40061,"umann":40062,"atsuki":40063,"435":40064,"ĠRoads":40065,"Ġslur":40066,"ĠIdeally":40067,"Ġtrenches":40068,"Ctrl":40069,"Ġmirrored":40070,"ĠZel":40071,"ĠCrest":40072,"Compat":40073,"ĠRolls":40074,"scrib":40075,"ĠTrails":40076,"ometers":40077,"winter":40078,"Ġimmortality":40079,"ilated":40080,"Ġcontradicts":40081,"universal":40082,"illions":40083,"ĠMama":40084,"optim":40085,"ATURE":40086,"Ġgeo":40087,"etter":40088,"ĠCarlo":40089,"424":40090,"Ġcanonical":40091,"ĠStronghold":40092,"near":40093,"Ġperfume":40094,"Ġorchestra":40095,"odiac":40096,"Ġuphe":40097,"Ġreigning":40098,"versive":40099,"Ġcaucuses":40100,"ĠDEM":40101,"Ġinsulted":40102,"Ġ------":40103,"ĠCrush":40104,"Ġrooting":40105,"ĠWraith":40106,"Ġwhore":40107,"Ġtofu":40108,"Cmd":40109,"ĠBree":40110,"Ġ$_":40111,"Ġrive":40112,"ĠAdvertising":40113,"Ġwatt":40114,"ĠHO":40115,"Ġpersuasive":40116,"ĠParameters":40117,"Ġobservational":40118,"ĠNCT":40119,"ĠMoj":40120,"ĠSalon":40121,"Ġtrunc":40122,"Ġexquisite":40123,"ĠMara":40124,"Ġpoop":40125,"ĠANN":40126,"Exc":40127,"ĠWonderful":40128,"ĠTaco":40129,"Ġhomeowner":40130,"ĠSmithsonian":40131,"orporated":40132,"mmmm":40133,"Ġloaf":40134,"ĠYamato":40135,"ĠIndo":40136,"Ġclinging":40137,"ás":40138,"Ġimmutable":40139,"hub":40140,"Orange":40141,"Ġfingertips":40142,"ĠWooden":40143,"ĠKidd":40144,"ĠJPM":40145,"ĠDamn":40146,"Cow":40147,"codes":40148,"482":40149,"Ġinitiating":40150,"ĠElk":40151,"ĠCutting":40152,"Ġabsentee":40153,"ĠVance":40154,"ĠLilith":40155,"GUI":40156,"Ġobscured":40157,"Ġdwarves":40158,"ĠChop":40159,"ĠBoko":40160,"Values":40161,"Ġmultimedia":40162,"Ġbrewed":40163,"Regular":40164,"CRIPTION":40165,"ĠMortal":40166,"Ġapex":40167,"Ġtraveler":40168,"Ġboils":40169,"Ġspraying":40170,"Represent":40171,"ĠStarship":40172,"428":40173,"Ġdisapproval":40174,"Ġshadowy":40175,"Ġlamented":40176,"ĠReplace":40177,"ĠFranç":40178,"677":40179,"dor":40180,"Ġunstoppable":40181,"Ġcohorts":40182,"gyn":40183,"ĠClassics":40184,"ĠAmph":40185,"Ġsluggish":40186,"ĠAddiction":40187,"ĠPadres":40188,"Ġinscription":40189,"Ġinhuman":40190,"minus":40191,"ĠJeremiah":40192,"atars":40193,"Terror":40194,"ĠTos":40195,"ĠSharma":40196,"asta":40197,"catch":40198,"Ġplumbing":40199,"ĠTimbers":40200,"Shar":40201,"Hal":40202,"ĠOsc":40203,"Ġcoupling":40204,"humans":40205,"Ġsponge":40206,"Ġidols":40207,"ĠSpa":40208,"ĠAdvocate":40209,"ĠBeats":40210,"lua":40211,"Ġticking":40212,"Ġloader":40213,"ĠGron":40214,"810":40215,"Ġstimulated":40216,"Ġsidebar":40217,"ĠManufacturer":40218,"oreAnd":40219,"1973":40220,"Ġpraises":40221,"ĠFlores":40222,"disable":40223,"ĠElectrical":40224,"raise":40225,"Eth":40226,"Ġmigrated":40227,"Ġlecturer":40228,"Kids":40229,"ĠCavern":40230,"Ġkettle":40231,"Ġglyc":40232,"ĠMandela":40233,"ĠFully":40234,"å§«":40235,"FINEST":40236,"Ġsqueezing":40237,"ĠRyder":40238,"ampoo":40239,"oreAndOnline":40240,"InstoreAndOnline":40241,"BuyableInstoreAndOnline":40242,"Ġcommemorate":40243,"ĠRampage":40244,"Austin":40245,"ĠShroud":40246,"ĠRuins":40247,"915":40248,"ĠKH":40249,"Ġwaterfront":40250,"ĠESC":40251,"baby":40252,"ĠCout":40253,"ĠEmblem":40254,"Ġequivalents":40255,"492":40256,"Unique":40257,"ĠNietzsche":40258,"browser":40259,"Ġimitation":40260,"ĠWerewolf":40261,"ĠKirin":40262,"acas":40263,"',\"":40264,"Ġþ":40265,"Reviewed":40266,"Ġcunt":40267,"Ġvoic":40268,"ĠLenovo":40269,"Ġbonded":40270,"481":40271,"Ġinhibitors":40272,"Ġendeavors":40273,"ĠHavana":40274,"ĠStout":40275,"ĠJolly":40276,"Actor":40277,"*/(":40278,"Ġoccurrences":40279,"ĠTens":40280,"Increased":40281,"ĠACTION":40282,"ĠãĢĮ":40283,"ĠRankings":40284,"ĠBreat":40285,"Ġ309":40286,"Dou":40287,"Ġimpacting":40288,"ĠDuchess":40289,"prefix":40290,"QB":40291,"Ġsummoning":40292,"Ġbestowed":40293,"ĠKepler":40294,"ĠPOWER":40295,"cube":40296,"ĠKits":40297,"ĠGrip":40298,"Ġopium":40299,"Ġreputable":40300,"toc":40301,"ichael":40302,"ĠRipple":40303,"Ġcafé":40304,"ĠZoom":40305,"ĠBurma":40306,"Ġwaive":40307,"Ġstalls":40308,"Ġdemeanor":40309,"incerity":40310,"Ġfluoride":40311,"ĠSHOULD":40312,"Paris":40313,"Ġlonging":40314,"Ġplat":40315,"Ġgrossly":40316,"Ġbulls":40317,"Ġshowcasing":40318,"expected":40319,"ĠGaddafi":40320,"engineering":40321,"Repeat":40322,"ĠKut":40323,"Ġconceivable":40324,"Ġtrimmed":40325,"oscope":40326,"ĠCandidate":40327,"ĠTears":40328,"rolog":40329,"Lewis":40330,"SUP":40331,"Ġroadmap":40332,"Ġsaliva":40333,"Ġtrumpet":40334,"Jimmy":40335,"Ġmiraculous":40336,"Ġcolonization":40337,"Ġamput":40338,"ĠGNOME":40339,"atech":40340,"Different":40341,"ĠELE":40342,"ĠGovernments":40343,"ĠAhead":40344,"ãħĭãħĭ":40345,"wordpress":40346,"LIB":40347,"ĠInclude":40348,"ĠDorothy":40349,"045":40350,"ĠColombian":40351,"Ġleased":40352,"884":40353,"Ġdegrading":40354,"ĠDaisy":40355,"iations":40356,"Ġbaptized":40357,"Ġsurname":40358,"cox":40359,"Ġblinked":40360,"ãĥ¢":40361,"Ġpollen":40362,"Ġdermat":40363,"Ġregex":40364,"ĠNicholson":40365,"ĠEater":40366,"çľ":40367,"rador":40368,"Ġnarrower":40369,"Ġhurricanes":40370,"Ġhallucinations":40371,"ridden":40372,"ISSION":40373,"ĠFirefly":40374,"Ġattainment":40375,"Ġnominate":40376,"Ġavocado":40377,"ĠMeredith":40378,"Ġts":40379,"Ġreverence":40380,"Ġeuph":40381,"Ġcrates":40382,"ĠTEXT":40383,"Ġ443":40384,"Ġ319":40385,"JSON":40386,"iquette":40387,"Ġshortstop":40388,"ickey":40389,"Ġpropelled":40390,"Ġapi":40391,"ĠThieves":40392,"779":40393,"Ġoversaw":40394,"Ġcoli":40395,"ĠNicola":40396,"Ġovercl":40397,"ikawa":40398,"ĠCyr":40399,"Ġ384":40400,"789":40401,"ĠAllows":40402,"1027":40403,"Detroit":40404,"TRY":40405,"setup":40406,"ĠSocialism":40407,"Soviet":40408,"susp":40409,"ĠAPR":40410,"ĠShutdown":40411,"Ġaluminium":40412,"zbek":40413,"ĠLover":40414,"GGGGGGGG":40415,"Ġdemocracies":40416,"Ġ1908":40417,"ĠMerrill":40418,"ĠFrancois":40419,"gdala":40420,"Ġtraffickers":40421,"ĠTil":40422,"ĠGoat":40423,"Ġsped":40424,"ĠReserv":40425,"Ġprod":40426,"552":40427,"Ġcac":40428,"ĠUniv":40429,"ĠSchwe":40430,"Ġswirling":40431,"ĠWilderness":40432,"ĠEggs":40433,"Ġsaddened":40434,"Ġarchaic":40435,"Hyd":40436,"Ġexcessively":40437,"BRE":40438,"Ġaerospace":40439,"ĠVoices":40440,"Craig":40441,"Ġignited":40442,"Initially":40443,"ĠMcA":40444,"Ġhandset":40445,"Ġreforming":40446,"Ġfrustrations":40447,"ĠDeadpool":40448,"ĠBelichick":40449,"ractor":40450,"ĠRagnarok":40451,"ĠDrupal":40452,"ĠApproximately":40453,"1920":40454,"ĠHubble":40455,"armor":40456,"ĠSaras":40457,"ĠJonas":40458,"Ġnostalgic":40459,"Ġfeasibility":40460,"Saharan":40461,"Ġorbiting":40462,"Ġ970":40463,"Ru":40464,"Ġshin":40465,"ĠInvestigators":40466,"Ġinconsistencies":40467,"ĠPAN":40468,"BG":40469,"Ġgrazing":40470,"Ġdetectors":40471,"ĠStartup":40472,"ĠFunny":40473,"ĠNaomi":40474,"Considering":40475,"Ġhog":40476,"utf":40477,"cemic":40478,"Ġfortified":40479,"ĠFunctions":40480,"Ġcodec":40481,"nutrition":40482,"Hat":40483,"\"!":40484,"microsoft":40485,"558":40486,"ĠThin":40487,"ĠACE":40488,"Alias":40489,"ĠOPS":40490,"papers":40491,"PK":40492,"ãĢİ":40493,"Ġimprobable":40494,"Northern":40495,"equal":40496,"Ġlookout":40497,"Ġtyres":40498,"ĠModified":40499,"ĠKop":40500,"Absolutely":40501,"Ġbuildup":40502,"silver":40503,"Ġaudi":40504,"Ġgrotesque":40505,"ĠSaber":40506,"ĠPresbyter":40507,"ONY":40508,"Ġglaciers":40509,"ĠShoals":40510,"ĠKass":40511,"ĠHRC":40512,"ĠNicol":40513,"ĠLunch":40514,"ĠFoss":40515,"âĸĴ":40516,"ADRA":40517,"ĠOnePlus":40518,"oing":40519,"grounds":40520,"Ġincidental":40521,"Ġdatasets":40522,"689":40523,"ĠClarkson":40524,"Ġassembling":40525,"ĠCorrections":40526,"Ġdrinkers":40527,"Ġqualifiers":40528,"Ġleash":40529,"Ġunfounded":40530,"ĠHundred":40531,"Ġkickoff":40532,"Ti":40533,"Ġreconcil":40534,"ĠGrants":40535,"ĠCompliance":40536,"ĠDexterity":40537,"Ġ1906":40538,"warn":40539,"Dallas":40540,"Maximum":40541,"nard":40542,"avia":40543,"beaut":40544,"ensitivity":40545,"trace":40546,"Ġpioneers":40547,"ĠFract":40548,"ãĢı":40549,"Ġprecept":40550,"Ġglossy":40551,"ĠIEEE":40552,"Across":40553,"Ġ680":40554,"Sleep":40555,"cheon":40556,"Ġsatirical":40557,"ĠMinotaur":40558,"ĠClaude":40559,"Ġré":40560,"apego":40561,"Ġcarrot":40562,"ĠSemin":40563,"inoa":40564,"Ġzo":40565,"Independent":40566,"Ġdiagnoses":40567,"ĠCue":40568,"MAR":40569,"Ġrendition":40570,"ĠKik":40571,"Ġpathology":40572,"Ġselects":40573,"LinkedIn":40574,"Ġassay":40575,"ĠDres":40576,"Ġtextual":40577,"posted":40578,"ITAL":40579,"ĠMaul":40580,"Neal":40581,"Ġinterconnected":40582,"Ġerratic":40583,"ĠVirus":40584,"Ġ530":40585,"Ġenvironmentalists":40586,"ĠPhelps":40587,"Ġengagements":40588,"ĠINST":40589,"Ġeconomical":40590,"noxious":40591,"Ġgearing":40592,"izzy":40593,"Ġfavorably":40594,"ĠMcGill":40595,"Term":40596,"Ġhanged":40597,"Ġballpark":40598,"ĠReyes":40599,"Ġbeware":40600,"ĠPsal":40601,"ĠMassacre":40602,"qi":40603,"Ġinaccessible":40604,"aclysm":40605,"Ġfray":40606,"illac":40607,"Ġbitterly":40608,"ĠCertification":40609,"Michigan":40610,"Ġirrespective":40611,"alore":40612,"Empty":40613,"Ġendorsements":40614,"Ġundet":40615,"fg":40616,"equipped":40617,"Ġmerciless":40618,"ĠCust":40619,"Ġimmature":40620,"Ġvoucher":40621,"ĠBlackwell":40622,"Ñı":40623,"hawk":40624,"disciplinary":40625,"ilee":40626,"ĠMakoto":40627,"ĠDude":40628,"ãĥĩãĤ£":40629,"Years":40630,"Ġinver":40631,"Ġshaman":40632,"ĠYong":40633,"ipel":40634,"ellen":40635,"ĠCathy":40636,"brids":40637,"Ġsarc":40638,"651":40639,"Near":40640,"Ġgroundwork":40641,"Ġamaz":40642,"Ġ415":40643,"ĠHuntington":40644,"hews":40645,"ĠBung":40646,"Ġarbitrarily":40647,"ĠWit":40648,"ĠAlberto":40649,"Ġdisqualified":40650,"bestos":40651,"461":40652,"Ġpc":40653,"Ġ284":40654,"robat":40655,"Robin":40656,"Ġhugs":40657,"ĠTransition":40658,"ĠOccasionally":40659,"Ġ326":40660,"ĠWhilst":40661,"ĠLey":40662,"Ġspaceship":40663,"csv":40664,"Ġunsuccessfully":40665,"ĠAu":40666,"leck":40667,"ĠWinged":40668,"ĠGrizzlies":40669,".�":40670,"Ġnearer":40671,"ĠSorceress":40672,"ĠIndigo":40673,"Else":40674,"840":40675,"letes":40676,"Coach":40677,"Ġupbringing":40678,"ĠKes":40679,"Ġseparatist":40680,"Ġracists":40681,"Ġchained":40682,"Ġabstinence":40683,"learning":40684,"Ġreinstated":40685,"Ġsymmetry":40686,"Ġreminders":40687,"ĠChevy":40688,"Ġmont":40689,"Ġexemplary":40690,"ĠTOR":40691,"ZX":40692,"Ġqualitative":40693,"ĠStamp":40694,"ĠSavannah":40695,"ĠRossi":40696,"Ġpaed":40697,"Ġdispensaries":40698,"ĠWalls":40699,"ĠChronic":40700,"Ġcomplimentary":40701,"ĠBeirut":40702,"Ġ+---":40703,"igslist":40704,"Ġcryptographic":40705,"masters":40706,"ĠCapitals":40707,"Ġmaximal":40708,"Ġentropy":40709,"Points":40710,"Ġcombatants":40711,"lip":40712,"ĠGlob":40713,"ĠBMC":40714,"phase":40715,"thank":40716,"HTTP":40717,"Ġcommuter":40718,"Ġ\\(\\":40719,"../":40720,"ĠRegener":40721,"ĠDOI":40722,"ĠActivision":40723,"Ġslit":40724,"osal":40725,"REM":40726,"Ġchants":40727,"Yu":40728,"Keys":40729,"Brexit":40730,"ĠForced":40731,"Arizona":40732,"Ġsquadron":40733,"ISO":40734,"ĠMalone":40735,"Ġ338":40736,"Ġcontrasting":40737,"Ġtidal":40738,"Ġlibel":40739,"Ġimplanted":40740,"Ġuproar":40741,"ĠCater":40742,"Ġpropositions":40743,"Manchester":40744,"ĠEuros":40745,"itamin":40746,"Gil":40747,"ĠElven":40748,"ĠSeek":40749,"ĠBai":40750,"Ġredevelopment":40751,"ĠTowns":40752,"ĠLub":40753,"!\",":40754,"alon":40755,"Krist":40756,"Ġmeasurable":40757,"Ġimaginable":40758,"Ġapostles":40759,"YN":40760,"760":40761,"Ġsteroid":40762,"Ġspecificity":40763,"ĠLocated":40764,"ĠBecker":40765,"ĠEdu":40766,"ĠDietary":40767,"utsch":40768,"ĠMarilyn":40769,"Ġblister":40770,"ĠMEP":40771,"ĠKoz":40772,"ĠCMS":40773,"yahoo":40774,"ĠCarney":40775,"Ġboasting":40776,"ĠCaleb":40777,"Byte":40778,"reads":40779,"aden":40780,"Problem":40781,"ĠWoodward":40782,"Swe":40783,"Sup":40784,"ĠKGB":40785,"Setup":40786,"Ġtacit":40787,"Ġretribution":40788,"Ġdues":40789,"ĠMü":40790,".?":40791,"ä¸Ń":40792,"pots":40793,"Ġcameo":40794,"ĠPAL":40795,"education":40796,"Amy":40797,"likely":40798,"gling":40799,"Ġconstitutionally":40800,"ĠHamm":40801,"ĠSpeak":40802,"Ġwidgets":40803,"brate":40804,"Ġcrappy":40805,"ĠIter":40806,"Ġanticipating":40807,"ĠBout":40808,"Pixel":40809,"ĠYep":40810,"ĠLaurie":40811,"Ġhut":40812,"Ġbulletin":40813,"ĠSalvation":40814,"Ġchats":40815,"earable":40816,"Honestly":40817,"ALTH":40818,"onsequ":40819,"cult":40820,"iscovery":40821,"ovych":40822,"Ġselves":40823,"ĠSatoshi":40824,"Sounds":40825,"Ġconvergence":40826,"ĠRosenberg":40827,"1974":40828,"Ġnasal":40829,"Ġfullest":40830,"Ġferocious":40831,"xus":40832,"iste":40833,"AMS":40834,"Ġlobbied":40835,"Ġsoothing":40836,"ĠGunn":40837,"today":40838,"024":40839,"Ġinspirational":40840,"ĠNBN":40841,"pb":40842,"gewater":40843,"orah":40844,"allowed":40845,"ĠColiseum":40846,"Ġspecializing":40847,"Ġinsanely":40848,"ĠTape":40849,"delay":40850,"Ġtarn":40851,"ĠPound":40852,"Ġmelanch":40853,"Ġdeployments":40854,"iland":40855,"Ġlessen":40856,"Ġfurry":40857,"ĠUEFA":40858,"Ġbloodshed":40859,"ĠMeier":40860,"ithering":40861,"Ġheirs":40862,"ĠJaw":40863,"axter":40864,"ĠPublications":40865,"Ġalters":40866,"intention":40867,"ĠWinchester":40868,"determination":40869,"ĠLifetime":40870,"thin":40871,"Monster":40872,"780":40873,"Ġapproximation":40874,"Ġsupermarkets":40875,"ĠSeconds":40876,"oros":40877,"huge":40878,"Ġbribe":40879,"ĠLIMITED":40880,"uned":40881,"Ġmisinterpret":40882,"ĠInjury":40883,"Ġ367":40884,"Ġthresholds":40885,"ĠCarnival":40886,"Ġgastrointestinal":40887,"Ġguideline":40888,"Ġdeceived":40889,"features":40890,"Ġpurportedly":40891,"ĠRonnie":40892,"ĠNewt":40893,"Ġspacious":40894,"asus":40895,"Ġsuperheroes":40896,"ĠCynthia":40897,"legged":40898,"kamp":40899,"chio":40900,"Ġthumbnail":40901,"ĠShirley":40902,"illation":40903,"Ġsheds":40904,"ĠZy":40905,"EPA":40906,"Ġdams":40907,"Ġyawn":40908,"nah":40909,"ĠPeggy":40910,"ĠErie":40911,"ĠJuventus":40912,"ĠFountain":40913,"rx":40914,"donald":40915,"album":40916,"ĠComprehensive":40917,"Ġcaching":40918,"ĠUz":40919,"ulnerability":40920,"ĠPrinciple":40921,"ĠJian":40922,"ingers":40923,"casts":40924,"ĠOsiris":40925,"chart":40926,"tile":40927,"ĠTiffany":40928,"ĠPatton":40929,"ĠWhip":40930,"Ġoversized":40931,"Je":40932,"ĠCinderella":40933,"ĠBorders":40934,"ĠDaesh":40935,"Mah":40936,"Ġdogma":40937,"Ġcommunists":40938,"vu":40939,"Council":40940,"Ġfreshwater":40941,"Ġwounding":40942,"Ġdebacle":40943,"Ġyoungster":40944,"Ġthreaded":40945,"ĠBots":40946,"ĠSavings":40947,"ãģĤ":40948,"oling":40949,"oho":40950,"Ġillumination":40951,"MRI":40952,"Ġloosen":40953,"trump":40954,"agency":40955,"urion":40956,"Ġmomentarily":40957,"ĠChun":40958,"ĠBudapest":40959,"ĠAlley":40960,"Disk":40961,"Ġastonished":40962,"ĠConquer":40963,"ĠAccounting":40964,"having":40965,"ĠWein":40966,"ĠAlright":40967,"Ġrevolver":40968,"Ġdelusion":40969,"Ġrelics":40970,"Ġadherent":40971,"quant":40972,"Ġhandmade":40973,"orio":40974,"Ġcombating":40975,"coded":40976,"Ġquadru":40977,"reth":40978,"Nik":40979,"ĠTribal":40980,"ĠMysterious":40981,"Ġinhal":40982,"ĠWinning":40983,"ĠClassification":40984,"changed":40985,"Ġunab":40986,"Ġscorn":40987,"icipated":40988,"wl":40989,"onductor":40990,"Ġreinforcing":40991,"ĠChildhood":40992,"anova":40993,"Ġadventurer":40994,"Ġdoctoral":40995,"ĠStrategies":40996,"Ġengulfed":40997,"ĠEncounter":40998,"Ġlashes":40999,"Critical":41000,"ricular":41001,"ĠUTF":41002,"ociation":41003,"checking":41004,"ĠConsulting":41005,"Runtime":41006,"period":41007,"ĠAsgard":41008,"Ġdistilled":41009,"ĠPasadena":41010,"ĠDying":41011,"ĠCOUNTY":41012,"Ġgranite":41013,"Ġsmack":41014,"Ġparachute":41015,"ĠSUR":41016,"Virginia":41017,"ĠFurious":41018,"787":41019,"ĠOkin":41020,"Ġcamel":41021,"ĠMbps":41022,"1972":41023,"ĠChao":41024,"ĠCyan":41025,"joice":41026,"efer":41027,"ĠWrap":41028,"ĠDebate":41029,"Seg":41030,"Ġforearm":41031,"ĠIgnore":41032,"Ġtimestamp":41033,"Ġprobing":41034,"ĠNoon":41035,"ĠGrail":41036,"fen":41037,"Ġdormant":41038,"ĠFirstly":41039,"ĠEighth":41040,"ĠHUN":41041,"ĠDesire":41042,"oras":41043,"Girls":41044,"ĠDesmond":41045,"zar":41046,"amines":41047,"OAD":41048,"execute":41049,"Ġboobs":41050,"ĠATL":41051,"_(":41052,"Chelsea":41053,"Ġmasturbation":41054,"ĠCoC":41055,"Ġdestroyer":41056,"ĠChomsky":41057,"Ġscatter":41058,"ĠAssets":41059,"796":41060,"ĠCargo":41061,"Ġreceptive":41062,"ĠScope":41063,"Ġmarketers":41064,"Ġlaunchers":41065,"Ġaxle":41066,"ĠSEA":41067,"seq":41068,"ĠMoff":41069,"finding":41070,"ĠGibbs":41071,"Georgia":41072,"extremely":41073,"NJ":41074,"Ġlaborers":41075,"stals":41076,"Ġmediation":41077,"ĠHedge":41078,"atown":41079,"Ġiod":41080,"despite":41081,"vill":41082,"Jane":41083,"existence":41084,"Ġcoincided":41085,"ĠUtilities":41086,"ĠCheap":41087,"Ġlogistical":41088,"Ġculmination":41089,"ĠNicotine":41090,"pak":41091,"Folder":41092,"Ġrodents":41093,"stuff":41094,"Ġlawfully":41095,"Ġreperto":41096,"ioch":41097,"jj":41098,"Dialogue":41099,"HHHH":41100,"liction":41101,"Looks":41102,"Ġ297":41103,"Ġturrets":41104,"ĠAbandon":41105,"Ġincess":41106,"ĠTrafford":41107,"Ġcurled":41108,"Ġpreferring":41109,"Ġprivatization":41110,"Ġirresist":41111,"ĠPanda":41112,"ĠShake":41113,"ĠMcGr":41114,"ãĥĦ":41115,"unders":41116,"Ġdiscriminated":41117,"Ġbartender":41118,"ILE":41119,"Atlantic":41120,"Ġpropensity":41121,"ĠWiz":41122,"ĠGim":41123,"conference":41124,"Ġreinforces":41125,"Gh":41126,"wagon":41127,"Ġeerie":41128,"Fal":41129,"Ġhugged":41130,"racist":41131,"RIC":41132,"Fu":41133,"Ġfiller":41134,"ĠStub":41135,"Ġengraved":41136,"ĠWrestle":41137,"Ġimaginative":41138,"ĠPeer":41139,"ĠFactors":41140,"anus":41141,"ĠDracula":41142,"monitor":41143,"Ġrouters":41144,"ibia":41145,"ĠBoolean":41146,"endale":41147,"ĠSlaughter":41148,"ĠShack":41149,"RFC":41150,"ĠSpielberg":41151,"Sax":41152,"ĠPHOTO":41153,"ĠClover":41154,"ĠRae":41155,"Depending":41156,"ĠMemor":41157,"aram":41158,"Ġpierced":41159,"Ġcurtains":41160,"vale":41161,"ĠInquisition":41162,"ĠPoke":41163,"Ġforecasting":41164,"Ġcomplains":41165,"Sense":41166,"ĠHermes":41167,"iscovered":41168,"Ġbible":41169,"ĠMorph":41170,"Ġgerm":41171,"785":41172,"DON":41173,"Ġcongen":41174,"Ġcrane":41175,"ĠDPR":41176,"Ġrespectfully":41177,"Room":41178,"ĠNaw":41179,"ĠDalai":41180,"reason":41181,"ĠAngus":41182,"Education":41183,"ĠTitanic":41184,"Ëľ":41185,"Ġoval":41186,"united":41187,"Ġthirds":41188,"Ġmoistur":41189,"ĠCPC":41190,"Miami":41191,"Ġtentacles":41192,"ĠPolaris":41193,"exc":41194,"exclusive":41195,"ĠPrairie":41196,"Ġcolossal":41197,"ĠBlend":41198,"surprisingly":41199,"ÃŃs":41200,"Ġindoctr":41201,"Ġbasal":41202,"ĠMPEG":41203,"undo":41204,"Split":41205,"Development":41206,"Ġlantern":41207,"1971":41208,"Ġprovocation":41209,"Ġanguish":41210,"ĠBind":41211,"ĠLeia":41212,"ducers":41213,"ippy":41214,"conservancy":41215,"Ġinitialize":41216,"ĠTwice":41217,"ĠSuk":41218,"Ġpredic":41219,"Ġdiploma":41220,"Ġsociop":41221,"Ingredients":41222,"Ġhammered":41223,"ĠIrma":41224,"Qaida":41225,"Ġglimps":41226,"ĠBian":41227,"Ġstacking":41228,"Ġfend":41229,"govtrack":41230,"Ġunn":41231,"democratic":41232,"igree":41233,"Ġ580":41234,"Ġ294":41235,"Ġstrawberry":41236,"IDER":41237,"Ġcherished":41238,"ĠHots":41239,"Ġinferred":41240,"Ġ808":41241,"ĠSocrates":41242,"Oregon":41243,"ĠRoses":41244,"ĠFOIA":41245,"Ġinsensitive":41246,"Ġ408":41247,"Recommend":41248,"ĠShine":41249,"Ġpainstaking":41250,"UGE":41251,"ĠHeller":41252,"ĠEnterprises":41253,"IOR":41254,"adj":41255,"NRS":41256,"LG":41257,"Ġalienated":41258,"Ġacknowledgement":41259,"ĠAUD":41260,"ĠReneg":41261,"Ġvouchers":41262,"Ġ960":41263,"Ġmoot":41264,"ĠDimensions":41265,"Ġcabbage":41266,"Bright":41267,"gat":41268,"ĠKlu":41269,"Ġlatent":41270,"Ġze":41271,"ĠMeng":41272,"Ġdisperse":41273,"Ġpandemonium":41274,"HQ":41275,"Ġvirtuous":41276,"ĠLocations":41277,"eeper":41278,"provided":41279,"Ġseams":41280,"ĠWT":41281,"izo":41282,"PROV":41283,"Ġtitanium":41284,"Ġrecollection":41285,"Ġcran":41286,"Ġ780":41287,"ĠNF":41288,"491":41289,"642":41290,"packing":41291,"598":41292,"texture":41293,"Spider":41294,"freedom":41295,"cipled":41296,"ĠTAMADRA":41297,"âϦ":41298,"authent":41299,"ĠWANT":41300,"rified":41301,"Ġrites":41302,"Ġuterus":41303,"kiss":41304,"Ġâī¤":41305,"Ġskillet":41306,"Ġdisenfranch":41307,"ĠGaal":41308,"Compan":41309,"Ġageing":41310,"guide":41311,"Balt":41312,"Ġiterator":41313,"Ġdiscretionary":41314,"tips":41315,"Ġprimates":41316,"ĠTechnique":41317,"ĠPayments":41318,"azel":41319,"ĠROCK":41320,"stantial":41321,"060":41322,"Ġdmg":41323,"ĠJackets":41324,"ĠPlayoff":41325,"Ġnursery":41326,"ĠSymb":41327,"arton":41328,"Ġannexation":41329,"Colorado":41330,"Ġcoils":41331,"ĠShoes":41332,"âĦ¢:":41333,"ĠRoz":41334,"COMPLE":41335,"ĠEverest":41336,"ĠTriumph":41337,"Joy":41338,"Grid":41339,"à¼":41340,"processor":41341,"ĠProsper":41342,"ĠSeverus":41343,"ĠSelected":41344,"rg":41345,"ĠTayyip":41346,"Stra":41347,"Ġskiing":41348,"Ġ?)":41349,"Ġpeg":41350,"Tesla":41351,"Ġtimeframe":41352,"Ġmastermind":41353,"ĠNB":41354,"scientific":41355,"ĠShit":41356,"generic":41357,"INTER":41358,"NUM":41359,"Ġstroll":41360,"ĠEnix":41361,"ĠMMR":41362,"ĠEMS":41363,"movie":41364,"Ĥª":41365,"Ġminimizing":41366,"iddling":41367,"Ġillegitimate":41368,"Ġprototyp":41369,"Ġprematurely":41370,"Ġmanuals":41371,"obbies":41372,"ĠCassidy":41373,"DEC":41374,"desktop":41375,"Ġaeros":41376,"Ġscreenings":41377,"Ġdebilitating":41378,"ĠGrind":41379,"natureconservancy":41380,"Ġfades":41381,"termination":41382,"assetsadobe":41383,"Factor":41384,"Ġdefinitively":41385,"Poké":41386,"apult":41387,"ĠLafayette":41388,"Corn":41389,"ĠCoral":41390,"Ġstagnant":41391,"Tue":41392,"Ġdissatisfaction":41393,"Gender":41394,"Ġkidneys":41395,"ĠGow":41396,"ĠDefeat":41397,"ĠAshton":41398,"Ġcartels":41399,"Ġforeclosure":41400,"ĠExplore":41401,"strength":41402,"otin":41403,"Ġveterinarian":41404,"Ġfumble":41405,"Ġparap":41406,"ĠStrait":41407,"rils":41408,"Ġprick":41409,"ĠBermuda":41410,"ĠAmmunition":41411,"skinned":41412,"Ġabound":41413,"ĠBraz":41414,"Ġsharper":41415,"ĠAscension":41416,"Ġ978":41417,"Ġpreviews":41418,"Ġcommunion":41419,"ĠXY":41420,"Ġphony":41421,"Ġnewcomer":41422,"Ġ332":41423,".\",\"":41424,"Ġredistribution":41425,"Protect":41426,"ĠSof":41427,"Kal":41428,"Ġlipstick":41429,"worst":41430,"Ġtangled":41431,"Ġretrospective":41432,"integer":41433,"Ġvolunteering":41434,"Ġ1907":41435,"Ġ--------------------":41436,"ichen":41437,"Ġunveiling":41438,"Ġsenseless":41439,"Ġfisheries":41440,"\\-":41441,"Ġhinges":41442,"Ġcalculus":41443,"Myth":41444,"Ġundefeated":41445,"Ġoptimizations":41446,"Ġdepress":41447,"Ġbillboard":41448,"ĠYad":41449,"ĠPyramid":41450,"Isn":41451,"Ide":41452,"Ġlegion":41453,"ĠKramer":41454,"entanyl":41455,"Ġpenetrating":41456,"ĠHawth":41457,"ĠPRODUCT":41458,"ĠGerard":41459,"ĠPact":41460,"ĠIncluding":41461,"ĠElias":41462,"ĠElaine":41463,"visual":41464,"Ġhumming":41465,"Ġcondesc":41466,"ĠFasc":41467,"ä¸Ĭ":41468,"Ġegalitarian":41469,"Ġdevs":41470,"ĠDahl":41471,"Ops":41472,"DH":41473,"ĠBounce":41474,"idated":41475,"aldo":41476,"Ġrepublican":41477,"Ġhamb":41478,"ĠSett":41479,"ographies":41480,"CHAPTER":41481,"Ġtranssexual":41482,"Ġskyrocket":41483,"answer":41484,"Ġmarkup":41485,"ت":41486,"Ġheroine":41487,"Compare":41488,"ĠTav":41489,"Beast":41490,"Ġsuccessors":41491,"Ġnaïve":41492,"ĠBuckley":41493,"stress":41494,"meat":41495,"Ġdownloadable":41496,"Ġindexed":41497,"Ġscaff":41498,"ĠLump":41499,"ĠHomo":41500,"Studio":41501,"Insp":41502,"Ġracked":41503,"farious":41504,"ĠPetty":41505,"External":41506,"Ġ1909":41507,"Wars":41508,"commit":41509,"puters":41510,"Ġunob":41511,"ĠErr":41512,"ĠEG":41513,"ĠAlam":41514,"ĠSiberia":41515,"ĠAtmospheric":41516,"ISTER":41517,"ĠSatanic":41518,"translation":41519,"ĠLoud":41520,"traumatic":41521,"lique":41522,"Ġresonate":41523,"ĠWelch":41524,"Ġsparking":41525,"ĠTOM":41526,"tone":41527,"Ġoutl":41528,"Ġhandcuffed":41529,"ĠSerie":41530,"801":41531,"Ġlandmarks":41532,"ĠReeves":41533,"Ġsoftened":41534,"Ġdazzling":41535,"ĠWanted":41536,"months":41537,"Magikarp":41538,"Ġuntreated":41539,"ĠBedford":41540,"Mi":41541,"ĠDynamo":41542,"Ore":41543,"795":41544,"Ġwrongful":41545,"Ġlured":41546,"Ġcortisol":41547,"Ġvex":41548,"drawn":41549,"ilet":41550,"Downloadha":41551,"ĠFaction":41552,"Ġlabyrinth":41553,"Ġhijacked":41554,"waters":41555,"erick":41556,"Ġsuperiors":41557,"ĠRowling":41558,"ĠGuinness":41559,"Ġtd":41560,"992":41561,"Ġunearthed":41562,"Ġcentrif":41563,"Ġshameless":41564,"Pod":41565,"ĠFib":41566,"Ġicing":41567,"Ġpredictor":41568,"Ġ292":41569,"forestation":41570,"construct":41571,"Cand":41572,"@#":41573,"Ġagitated":41574,"Ġrepr":41575,"OVA":41576,"Ġknitting":41577,"ĠLima":41578,"Ġfodder":41579,"684":41580,"ĠPersona":41581,"kl":41582,"701":41583,"Ġbreakup":41584,"á¸":41585,"Ġappalled":41586,"Ġantidepressants":41587,"ĠSussex":41588,"Harris":41589,"ĠThermal":41590,"eeee":41591,"Upload":41592,"Ġgulf":41593,"Ġdoorstep":41594,"ĠShank":41595,"LU":41596,"ĠMEN":41597,"ĠPond":41598,"sorry":41599,"Ġmisfortune":41600,"nance":41601,"Ġbona":41602,"Mut":41603,"Ġdegraded":41604,"ĠLOG":41605,"ĠNess":41606,"animal":41607,"Ġaversion":41608,"undown":41609,"Ġsupplemented":41610,"ĠCups":41611,"Ġ504":41612,"Ġdeprive":41613,"ĠSparkle":41614,"ÅĤ":41615,"ĠMeditation":41616,"authors":41617,"ĠSaban":41618,"ĠNaked":41619,"aird":41620,"ĠMandarin":41621,"ĠScriptures":41622,"ĠPersonnel":41623,"ĠMaharashtra":41624,"Ġ1903":41625,"ĠPai":41626,"ĠMirage":41627,"ombat":41628,"Accessory":41629,"Ġfragmented":41630,"Together":41631,"Ġbelievable":41632,"ĠGladiator":41633,"aligned":41634,"ĠSlug":41635,"MAT":41636,"Ġconvertible":41637,"ĠBourbon":41638,"ameron":41639,"ĠRehab":41640,"ntax":41641,"Ġpowdered":41642,"pillar":41643,"Ġsmoker":41644,"ĠManson":41645,"ĠBF":41646,"511":41647,"ĠGoodell":41648,"ĠDAR":41649,"mud":41650,"gart":41651,"Ġobedient":41652,"ĠTransmission":41653,"ĠDonation":41654,"880":41655,"Ġbothering":41656,"Materials":41657,"ãĤ±":41658,"destroy":41659,"Ġforegoing":41660,"Ġanarchism":41661,"ĠKry":41662,"iceps":41663,"Ġlittered":41664,"ĠSchiff":41665,"Ġanecdotal":41666,"units":41667,"Ġfian":41668,"ĠStim":41669,"ĠSOME":41670,"ĠInvaders":41671,"Ġbehavioural":41672,"ĠVentures":41673,"Ġsublime":41674,"Ġfruition":41675,"ĠPenalty":41676,"Ġcorrosion":41677,"¶ħ":41678,"Ġlikened":41679,"Ġbesieged":41680,"weeney":41681,"ĠCreep":41682,"Ġlinemen":41683,"multi":41684,"icably":41685,"udder":41686,"Ġvitality":41687,"Ġshortfall":41688,"ĠPants":41689,"apist":41690,"Hidden":41691,"ĠDrops":41692,"medical":41693,"Ġpronunciation":41694,"ĠNRL":41695,"Ġinsightful":41696,"JV":41697,"ĠBeard":41698,"ĠChou":41699,"Ġcharms":41700,"Ġbins":41701,"Ġambassadors":41702,"ĠSaturdays":41703,"Ġinhibitor":41704,"ĠFranch":41705,"601":41706,"','":41707,"ĠConor":41708,"artney":41709,"ĠXperia":41710,"grave":41711,"bees":41712,"ĠProtestants":41713,"Ġsoaking":41714,"ĠMandal":41715,"Ġphased":41716,"Ġ660":41717,"Ġscams":41718,"Ġbuzzing":41719,"ĠItalians":41720,"ĠLorenzo":41721,"ĠJA":41722,"Ġhesitated":41723,"Ġcliffs":41724,"ĠGOT":41725,"inguishable":41726,"Ġko":41727,"Ġinterruption":41728,"Zip":41729,"Learning":41730,"Ġunderscores":41731,"ĠBlink":41732,"Ku":41733,"579":41734,"ĠAutob":41735,"IRE":41736,"Ġwatering":41737,"Ġpastry":41738,"820":41739,"Ġvisionary":41740,"ĠTemplar":41741,"awaited":41742,"Ġpiston":41743,"Ġantid":41744,"currently":41745,"Ġpard":41746,"Ġwaging":41747,"Ġnobility":41748,"ĠYus":41749,"Ġinjecting":41750,"faith":41751,"ĠPASS":41752,"åº":41753,"Ġretake":41754,"ĠPROC":41755,"Ġcathedral":41756,"bash":41757,"Ġwrestlers":41758,"Ġpartnering":41759,"Ġnoses":41760,"Ġ358":41761,"Transform":41762,"amen":41763,"Ġbouts":41764,"ĠIdeal":41765,"ĠConstantin":41766,"Ġsep":41767,"ĠMonarch":41768,"atten":41769,"ĠPeoples":41770,"modified":41771,"Ġmoratorium":41772,"Ġpenchant":41773,"Ġoffensively":41774,"Ġproxies":41775,"okane":41776,"ĠTaiwanese":41777,"ĠPoo":41778,"ĠHOME":41779,"usional":41780,"Ġverbs":41781,"ĠOman":41782,"visory":41783,"Ġpersuasion":41784,"Ġmultit":41785,"Ġscissors":41786,"Gay":41787,"oway":41788,"ophysical":41789,"lus":41790,"gnu":41791,"Ġapocalyptic":41792,"Ġabsurdity":41793,"Ġplaybook":41794,"Ġautobiography":41795,"IUM":41796,"Ġsneaking":41797,"ĠSimulation":41798,"pps":41799,"ellery":41800,"Planet":41801,"Ġrightfully":41802,"Ġniece":41803,"ĠNEC":41804,"ĠIPO":41805,"ĠDisclosure":41806,"leanor":41807,"ousy":41808,"STER":41809,"Ġ282":41810,"Cruz":41811,"Chall":41812,"643":41813,"ĠSurvive":41814,"ĠFatal":41815,"ĠAmid":41816,"apo":41817,"Weapons":41818,"DEN":41819,"770":41820,"ĠGreenwald":41821,"Ġlinen":41822,"alos":41823,"Ġpollutants":41824,"ĠPCIe":41825,"kat":41826,"Ġpaw":41827,"ĠKraft":41828,"Chem":41829,"ĠTerminator":41830,"Ġreincarn":41831,"Ġ][":41832,"ĠSeeds":41833,"Ġsilhouette":41834,"ĠStores":41835,"Ġgrooming":41836,"ĠDirection":41837,"ĠIsabel":41838,"ĠBridges":41839,"ðŁij":41840,"EED":41841,"ĠMorsi":41842,"Ġvalves":41843,"ĠRanked":41844,"ĠPharma":41845,"ĠOrganizations":41846,"Ġpenetrated":41847,"ĠRodham":41848,"ĠProtoss":41849,"Ġoverest":41850,"Ġexasper":41851,"ĠTJ":41852,"Ġ000000":41853,"Ġtrickle":41854,"Ġbourbon":41855,"WHO":41856,"Ġwretched":41857,"Ġmicroscopic":41858,"Ġchecklist":41859,"Ġadorned":41860,"Royal":41861,"Administ":41862,"ĠRetirement":41863,"ĠHighest":41864,"Weather":41865,"ilege":41866,"Ġincrements":41867,"ĠCosponsors":41868,"Ġmasse":41869,"ĠSinn":41870,"rf":41871,"Ġhordes":41872,"assembly":41873,"754":41874,"ĠNatasha":41875,"ĠTYPE":41876,"ĠGENERAL":41877,"Ġarranging":41878,"Ġ407":41879,"lator":41880,"Ġglean":41881,"Ġdiscredited":41882,"Ġclinicians":41883,"UNE":41884,"Ġachieves":41885,"ĠEmerson":41886,"complex":41887,"=[":41888,"Ġprincipally":41889,"Ġfrail":41890,"picked":41891,"Ġthanking":41892,"Ġrecl":41893,"ĠLAST":41894,"Ġsuppressing":41895,"ilic":41896,"Ġantidepressant":41897,"ĠLisbon":41898,"Ġthor":41899,"Ġspa":41900,"Ġkingdoms":41901,"ĠPearce":41902,"emo":41903,"Ġplung":41904,"Ġdivest":41905,"Ġ********************************":41906,"bis":41907,"ospels":41908,"adr":41909,"Spirit":41910,"halla":41911,"Pink":41912,"endez":41913,"Ġresurrected":41914,"escape":41915,"ĠRosenstein":41916,"Ġgeological":41917,"Ġnecessities":41918,"Ġcarniv":41919,"ĠElys":41920,"ĠBarney":41921,"Ġ296":41922,"digy":41923,"STON":41924,"DOWN":41925,"Ġmilestones":41926,"Ġker":41927,"Ġdismantling":41928,"Ġreprim":41929,"Ġcrossings":41930,"1945":41931,"Ġpatriarchy":41932,"Ġblasphemy":41933,"Ġ359":41934,"metry":41935,"ĠObesity":41936,"ĠDifferences":41937,"blocking":41938,"ãĥķãĤ¡":41939,"ichita":41940,"ĠSabha":41941,"phalt":41942,"ĠColo":41943,"uala":41944,"efficients":41945,"ĠMedina":41946,"console":41947,"557":41948,"ĠHannibal":41949,"ĠHabit":41950,"ĠFever":41951,"Ġthence":41952,"Ġsynagogue":41953,"Ġessentials":41954,"Ġwink":41955,"ĠTrader":41956,"IDA":41957,"ĠSpoiler":41958,"ĠIcelandic":41959,"ĠHayward":41960,"Ġpeac":41961,"Ġmalice":41962,"Ġflashback":41963,"Ġthw":41964,"Ġlayoffs":41965,"Liquid":41966,"Ġtrooper":41967,"Ġhinge":41968,"ĠReaders":41969,"Phill":41970,"ĠBauer":41971,"Created":41972,"Ġaudits":41973,"accompan":41974,"Ġunsuspecting":41975,"iera":41976,"66666666":41977,"Ġbroch":41978,"Ġapprehended":41979,"ĠMalk":41980,"cerning":41981,"ĠCodex":41982,"OVER":41983,"Marsh":41984,"ĠDeng":41985,"ĠExpression":41986,"Ġdisrespectful":41987,"Ġascending":41988,"tests":41989,"ĠPlaintiff":41990,"stery":41991,"ĠAlibaba":41992,"dinand":41993,"ĠDempsey":41994,"Applications":41995,"moral":41996,"Ġthroughput":41997,"Ġquarrel":41998,"Ġmills":41999,"Ġhemor":42000,"ĠCASE":42001,"terrorist":42002,"stim":42003,"ifestyle":42004,"rozen":42005,"CEPT":42006,"Ark":42007,"uci":42008,"lectic":42009,"Ġirritating":42010,"sheets":42011,"Ay":42012,"Ġredeemed":42013,"Ġhorny":42014,"ĠTeach":42015,"ĠSear":42016,"democracy":42017,"465":42018,"ĠRestore":42019,"Ġstandby":42020,"ĠPis":42021,"iffin":42022,"Ġsleepy":42023,"Ġextrater":42024,"Ġcompliments":42025,"Frameworks":42026,"Ġinstalls":42027,"Ġbanging":42028,"surface":42029,"foundland":42030,"Ġmetaphysical":42031,"Ġ283":42032,"ouls":42033,"devices":42034,"Args":42035,"ĠSacrifice":42036,"ĠMcCorm":42037,"eson":42038,"Conservative":42039,"ĠMikhail":42040,"seeing":42041,"isively":42042,"ĠRooms":42043,"ĠGeneric":42044,"Ġenthusiastically":42045,"Ġgripped":42046,"Ġcomedic":42047,"ĠElectricity":42048,"Ġguerrilla":42049,"Ġdecoration":42050,"ĠPerspective":42051,"Ġconsultations":42052,"Ġunamb":42053,"Ġplagiar":42054,"Ġmagician":42055,"Ġerection":42056,"ĠTourism":42057,"oried":42058,"roxy":42059,"1100":42060,"Tam":42061,"Īè":42062,"γ":42063,"ת":42064,"ĠPredators":42065,"Nitrome":42066,"Ġtelescopes":42067,"projects":42068,"Ġunprotected":42069,"Ġstocked":42070,"ĠEntreprene":42071,"nexpected":42072,"Ġwastewater":42073,"Vill":42074,"Ġintimately":42075,"ĠiCloud":42076,"ĠConstable":42077,"Ġspoof":42078,"Ġnefarious":42079,"Ġfins":42080,"Ġcensor":42081,"ĠModes":42082,"ĠEsper":42083,"arbon":42084,"Ġintersections":42085,"Ġlauded":42086,"Ġphysi":42087,"Ġgenerously":42088,"ĠTheNitrome":42089,"ĠTheNitromeFan":42090,"Ġarisen":42091,"ĠÙĪ":42092,"Ġglands":42093,"ĠPavilion":42094,"ĠGupta":42095,"Ġuniformly":42096,"Ġramps":42097,"riet":42098,"ĠWHEN":42099,"ĠVanessa":42100,"Ġrouted":42101,"Ġlimp":42102,"ĠCPI":42103,"pter":42104,"intuitive":42105,"Ġvaping":42106,"Ġexperimented":42107,"ĠOlympus":42108,"ĠAmon":42109,"Ġsighting":42110,"Ġinfiltrate":42111,"ĠGentleman":42112,"Ġsignings":42113,"ĠMeow":42114,"ĠNavigation":42115,"checks":42116,"433":42117,"Ġelapsed":42118,"ĠBulgarian":42119,"espie":42120,"ĠSOM":42121,"during":42122,"Ġspills":42123,"anca":42124,"ĠPlymouth":42125,"MAL":42126,"Ġdomestically":42127,"ĠWatergate":42128,"ĠFAM":42129,"killed":42130,"edited":42131,"ĠYourself":42132,"Ġsynchronization":42133,"ĠPractices":42134,"STEP":42135,"Ġgenomes":42136,"ĠQR":42137,"notice":42138,"Ġlocating":42139,"zin":42140,"Ġ329":42141,"alcohol":42142,"Ġkitten":42143,"Vo":42144,"Ġrinse":42145,"Ġgrapple":42146,"ĠScrew":42147,"ĠDul":42148,"AIR":42149,"Ġleasing":42150,"ĠCafé":42151,"Ġroses":42152,"ĠRespect":42153,"Ġmislead":42154,"Ġperfected":42155,"Ġnudity":42156,"Ġnonpartisan":42157,"ĠConsumption":42158,"Reporting":42159,"Ġnuances":42160,"Ġdeductible":42161,"ĠShots":42162,"Ġ377":42163,"Ġæľ":42164,"anooga":42165,"Benef":42166,"ĠBam":42167,"ĠSamp":42168,"ifix":42169,"Ġgalvan":42170,"ĠMedals":42171,"radius":42172,"Ġnobles":42173,"Ġeaves":42174,"igrate":42175,"KT":42176,"ĠHarbour":42177,"uers":42178,"Ġrisked":42179,"req":42180,"Ġneurot":42181,"gettable":42182,"aina":42183,"Romney":42184,"Ġunderpin":42185,"Ġloft":42186,"ĠSubcommittee":42187,"ĠMongol":42188,"biz":42189,"Ġmanifests":42190,"assisted":42191,"ĠGaga":42192,"Ġsynergy":42193,"Ġreligiously":42194,"ĠPref":42195,"ĠGerry":42196,"TAG":42197,"ĠChoi":42198,"466":42199,"behind":42200,"ĠOu":42201,"GoldMagikarp":42202,"Ġhemorrh":42203,"River":42204,"Ġtendon":42205,"Ġinjure":42206,"ĠFiona":42207,"Ġpag":42208,"Ġagitation":42209,"||||":42210,"uran":42211,"ĠESA":42212,"Ġesteem":42213,"Ġdodging":42214,"Ġ412":42215,"rss":42216,"Ġceases":42217,"excluding":42218,"Ġintakes":42219,"Ġinserts":42220,"Ġembold":42221,"ĠOral":42222,"upuncture":42223,"411":42224,"ĠUnified":42225,"ĠDele":42226,"Ġfurnace":42227,"ĠCoyotes":42228,"ĠBrach":42229,"Labor":42230,"Ġhandshake":42231,"Ġbruises":42232,"Grade":42233,"éĹĺ":42234,"ĠGrammy":42235,"ileen":42236,"States":42237,"ĠScandinavian":42238,"ĠKardash":42239,"866":42240,"Ġeffortlessly":42241,"ĠDIRECT":42242,"ĠTHEN":42243,"ĠMei":42244,"ertation":42245,"1968":42246,"Ġgroin":42247,"witch":42248,"Requirements":42249,"985":42250,"Ġroofs":42251,"Ġestates":42252,"ĠHF":42253,"Ġhaha":42254,"Ġdensely":42255,"ĠOCT":42256,"Ġplastics":42257,"Ġincidentally":42258,"ĠTracks":42259,"ĠTaxes":42260,"Ġchanted":42261,"Ġforceful":42262,"ĠBieber":42263,"ĠKahn":42264,"Kent":42265,"ĠCot":42266,"licts":42267,"Fed":42268,"Ġhideous":42269,"ĠVerd":42270,"ĠSyndicate":42271,"ĠIllegal":42272,"Jet":42273,"ĠDAV":42274,"reasonable":42275,"crew":42276,"Ġfundamentalist":42277,"Ġtruthful":42278,"ĠJing":42279,"Ġlil":42280,"Ġdowned":42281,"Ġenchanted":42282,"ĠPolicies":42283,"ĠMcMaster":42284,"ĠHare":42285,"ideshow":42286,"Ġparams":42287,"encers":42288,"gorithm":42289,"Ġallowances":42290,"Ġturbulent":42291,"Ġcomplexities":42292,"ĠKT":42293,"Ġ337":42294,"ĠGenetic":42295,"FUN":42296,"Doug":42297,"tick":42298,"Ġgigs":42299,"umenthal":42300,"Ġpatriarchal":42301,"Ġcalc":42302,",...":42303,"Ġcout":42304,"ĠGuan":42305,"Ġpathological":42306,"ĠRivals":42307,"Ġunderrated":42308,"Ġfluorescent":42309,"ĠJiu":42310,"arnaev":42311,"ĠQuan":42312,"Ġ429":42313,"Ġà¨":42314,"Mario":42315,"Construct":42316,"ĠCitation":42317,"ĠRacial":42318,"ĠRSA":42319,"ĠFidel":42320,"Ġ395":42321,"Personally":42322,"Cause":42323,"û":42324,"radical":42325,"inen":42326,"Ġvehemently":42327,"ĠPapa":42328,"Ġinternship":42329,"Ġflakes":42330,"ĠReck":42331,"Luckily":42332,"Bra":42333,"2020":42334,"ravings":42335,"RN":42336,"Wonder":42337,"Seriously":42338,"Ġreusable":42339,"Ġpolluted":42340,"ĠPeng":42341,"leigh":42342,"indle":42343,"Ġcircuitry":42344,"ĠMadonna":42345,"ĠBART":42346,"Residents":42347,"attribute":42348,"Philadelphia":42349,"Club":42350,"Ġplanner":42351,"Ġfrantically":42352,"Ġfaithfully":42353,"ĠTerritories":42354,"ĠLAT":42355,"ĠAndersen":42356,"anu":42357,"ĠPARK":42358,"ĠSora":42359,"iage":42360,"ĠPlayoffs":42361,"ĠGCC":42362,"427":42363,"Ġabnorm":42364,"ĠLever":42365,"Ġdisobedience":42366,"Async":42367,"ĠShea":42368,"Vert":42369,"Ġskirts":42370,"ĠSawyer":42371,"xp":42372,"Ġworsening":42373,"Ġscapego":42374,"ĠAngle":42375,"othal":42376,"Ġtrove":42377,"ĠSty":42378,"ĠNguyen":42379,"marine":42380,"ideon":42381,"Depths":42382,"Blog":42383,"ĠIlluminati":42384,"Ġtracts":42385,"Ġorganise":42386,"Ġostr":42387,"Fs":42388,"Ġleveraging":42389,"ĠDaredevil":42390,"asar":42391,"Ġlang":42392,"Ġextermin":42393,"ursions":42394,"ĠRomo":42395,"ãĤ¤ãĥĪ":42396,"Ġcontended":42397,"Ġencountering":42398,"ĠTablet":42399,"ĠAlternate":42400,"skill":42401,"Ġsweets":42402,"Ġcohesive":42403,"capacity":42404,"Ġrepud":42405,"Ġlizard":42406,"roo":42407,"Ġpilgrims":42408,"ĠRuff":42409,"ĠInstrument":42410,"ĠLogo":42411,"uitous":42412,"EH":42413,"Ġsalesman":42414,"Ġankles":42415,"Led":42416,"ĠPatty":42417,"udos":42418,"Owner":42419,"Ġdiscrepancies":42420,"kj":42421,"MU":42422,"Ġunconditional":42423,"DragonMagazine":42424,"iard":42425,"Oak":42426,"ĠConversation":42427,"beer":42428,"ĠOsaka":42429,"Delta":42430,"usky":42431,"Ġsecretion":42432,"Ġplaza":42433,"Ġming":42434,"Ġdepletion":42435,"ĠMous":42436,"ĠITS":42437,"ĠHimal":42438,"ĠFleming":42439,"Ġcytok":42440,"ĠHick":42441,"Ġbatters":42442,"ĠIntellectual":42443,"675":42444,"ér":42445,"ISION":42446,"ĠQuentin":42447,"ĠChapters":42448,"ihadi":42449,"Ġcoaster":42450,"WAYS":42451,"ĠLizard":42452,"ĠYor":42453,"andering":42454,"Skin":42455,"haust":42456,"abby":42457,"Ġportraying":42458,"Ġwielded":42459,"dash":42460,"Ġproponent":42461,"Ġripple":42462,"Ġgraphene":42463,"Ġflyer":42464,"Ġrecurrent":42465,"Ġdevils":42466,"Ġwaterfall":42467,"æĺ¯":42468,"goo":42469,"TextColor":42470,"Ġtampering":42471,"IVES":42472,"TRUMP":42473,"ĠAbel":42474,"ĠSAL":42475,"ĠHendricks":42476,"ĠLucius":42477,"bots":42478,"Ġ4096":42479,"ISTORY":42480,"Guest":42481,"ĠNX":42482,"inant":42483,"Benz":42484,"ĠLoaded":42485,"ĠClever":42486,"treatment":42487,"Ġtavern":42488,"Ġ339":42489,"ĠTNT":42490,"ificantly":42491,"Temperature":42492,"Fel":42493,"Ġunderworld":42494,"ĠJudges":42495,"Ġ<+":42496,"Ġstump":42497,"Ġoccupancy":42498,"Ġaber":42499,"ĠFinder":42500,")\",":42501,"ĠNunes":42502,"reset":42503,"inet":42504,"ectomy":42505,"Ġwellness":42506,"ĠPeb":42507,"quartered":42508,"andan":42509,"Ġnegatives":42510,"ĠThiel":42511,"ĠClip":42512,"ĠLTD":42513,"Ġblight":42514,"Ġrepertoire":42515,"Kyle":42516,"Ġquer":42517,"ĠCes":42518,"Ġhapl":42519,"989":42520,"ĠThames":42521,"iscopal":42522,"Desk":42523,"ivariate":42524,"ĠExcellence":42525,"foundation":42526,"Ġâĩ":42527,"Xi":42528,"Ġmysteriously":42529,"estyles":42530,"Ġperish":42531,"ĠEngels":42532,"ĠDEAD":42533,"090":42534,"}}}":42535,"ĠUnreal":42536,"Ġrestless":42537,"IDES":42538,"orthodox":42539,"ĠIntermediate":42540,"Ġdinners":42541,"ĠTrout":42542,"ĠSeym":42543,"ĠHalls":42544,"ogged":42545,"Ġtragedies":42546,"Ġdidnt":42547,"676":42548,"Ġailments":42549,"Ġobservable":42550,"ĠVide":42551,"adapt":42552,"ĠDusk":42553,"Ġprofessionalism":42554,"ĠPrescott":42555,"ĠIndies":42556,"pox":42557,"ĠMehran":42558,"Wide":42559,"Ġendemic":42560,"ĠParan":42561,"Bird":42562,"Ġpedals":42563,"ĠIU":42564,"ĠAdamant":42565,"ĠHurt":42566,"Ġcorrelates":42567,"urden":42568,"Ġsponsoring":42569,"climate":42570,"ĠUniversities":42571,"ĠKnot":42572,"ennes":42573,"ĠDamian":42574,"ĠAxel":42575,"Sport":42576,"Ġbarb":42577,"ĠSno":42578,"shown":42579,"steen":42580,"udence":42581,"Ġnonviolent":42582,"Ġhomophobia":42583,"Ġbiomass":42584,"ĠDetail":42585,"ĠsrfN":42586,"ĠTune":42587,"accompanied":42588,"IENCE":42589,"Albert":42590,"ĠMongo":42591,"zx":42592,"ĠCerberus":42593,"orbit":42594,"cens":42595,"Ġslay":42596,"SHARE":42597,"HY":42598,"Ġbrawl":42599,"ĠProbe":42600,"Ġnonexistent":42601,"ĠClarence":42602,"ĠBlackburn":42603,"Ġportals":42604,"ĠRita":42605,"ĠRemain":42606,"ĠLevant":42607,"Ġtricked":42608,"ĠFerry":42609,"avering":42610,"ĠStrawberry":42611,"ĠAnswers":42612,"Ġhorrendous":42613,"ĠAman":42614,"Supplement":42615,"ĠToad":42616,"Ġpeeled":42617,"Ġmanoeuv":42618,"ĠUzbek":42619,"monds":42620,"ĠHector":42621,"Ġ402":42622,"pees":42623,"fixes":42624,"Ġdj":42625,"Ġresumes":42626,"Ġaccountant":42627,"Ġadversity":42628,"Ġhampered":42629,"ĠLarson":42630,"Ġdoping":42631,"parts":42632,"Hur":42633,"Ġbearded":42634,"Ġyr":42635,"ĠPlugin":42636,"女":42637,"Ġ/**":42638,"rolley":42639,"Ġwatershed":42640,"ĠSubmission":42641,"iflower":42642,"ASC":42643,"Ġchoir":42644,"Ġsculptures":42645,"mA":42646,"increasing":42647,"aii":42648,"Ġsneakers":42649,"Ġconfronts":42650,"ĠElephant":42651,"ĠElixir":42652,"Ġrecal":42653,"ĠTTL":42654,"widget":42655,"ĠWax":42656,"ĠGrayson":42657,"Ġhairst":42658,"Ġhumiliated":42659,"ĠWARN":42660,"appiness":42661,"ĠTTC":42662,"Fuel":42663,"Ġpolio":42664,"Ġcomplexes":42665,"Ġbabe":42666,"ĠXIV":42667,"PF":42668,").[":42669,"Parts":42670,"Ġ435":42671,"Meg":42672,"ĠYards":42673,"ĠALP":42674,"Ġyells":42675,"Ġprinces":42676,"Ġbullies":42677,"ĠCapitalism":42678,"exempt":42679,"FAQ":42680,"ĠSponge":42681,"ĠAla":42682,"Ġpleasantly":42683,"Ġbuf":42684,"Ġdenote":42685,"Ġunpublished":42686,"Ġkneeling":42687,"asca":42688,"Ġlapse":42689,"alien":42690,"994":42691,"Ġreferees":42692,"ĠLawyers":42693,"Santa":42694,"Ġpuzzling":42695,"ĠPrometheus":42696,"ĠPharaoh":42697,"ĠDelay":42698,"Ġfacilitates":42699,"ĠCES":42700,"Ġjewels":42701,"Ġbooklet":42702,"onding":42703,"Ġpolarization":42704,"ĠMoran":42705,"ĠSalad":42706,"ĠSOS":42707,"ĠAdvice":42708,"PHOTOS":42709,"ICAN":42710,"iatures":42711,"express":42712,"ĠWonderland":42713,"ĠCODE":42714,"ĠCLASS":42715,"975":42716,"Ġgrep":42717,"ĠDiesel":42718,"ĠGlac":42719,"!?\"":42720,"Ġrm":42721,"oine":42722,"discrimination":42723,"ĠNurse":42724,"mallow":42725,"Ġvortex":42726,"ĠConsortium":42727,"ĠlargeDownload":42728,"straight":42729,"aughlin":42730,"Grad":42731,"Ġpublicized":42732,"ĠWaves":42733,"ĠRedd":42734,"Ġfestivities":42735,"ĠMane":42736,"arov":42737,"Ġfleeting":42738,"ĠDrunk":42739,"ugen":42740,"Cele":42741,"Ġchromosomes":42742,"ĠDOT":42743,"-+-+-+-+":42744,"Ġbusiest":42745,"ĠBeaver":42746,"Syrian":42747,"ĠKyr":42748,"kas":42749,"ĠCrossRef":42750,"1950":42751,"7601":42752,"Ġrepealing":42753,"ĠWinners":42754,"ĠMacro":42755,"ĠDOD":42756,"blance":42757,"Sort":42758,"641":42759,"Ġmetre":42760,"ĠDirk":42761,"Ġgoggles":42762,"Ġdrawbacks":42763,"Ġcomplainant":42764,"Ġauthorizing":42765,"Ġantitrust":42766,"operated":42767,"Ġmah":42768,"Ġexaggeration":42769,"Amazing":42770,"ĠSeraph":42771,"Ġhaze":42772,"wow":42773,"Ġextinguished":42774,"Ġcanyon":42775,"ĠBosh":42776,"Ġvents":42777,"Ġscrape":42778,"Correct":42779,"426":42780,"Ġavg":42781,"Demand":42782,"Ġâμ":42783,"Ġmicrobiota":42784,"\"}],\"":42785,"ĠStev":42786,"Bio":42787,"ĠPlanes":42788,"Ġsuggestive":42789,"Ġdecipher":42790,"ĠRefugee":42791,"ĠKejriwal":42792,"ĠGreenpeace":42793,"Ġdeclass":42794,"ĠSounders":42795,"Ġtho":42796,"Ġdecrypt":42797,"Ġbrushing":42798,"ĠJaneiro":42799,"ipop":42800,"Si":42801,"877":42802,"ĠGeoffrey":42803,"Ġcpu":42804,"ĠHazel":42805,"Ġviewpoints":42806,"Ġcrispy":42807,"ĠNotification":42808,"Ġsolder":42809,"ĠModest":42810,"ĠHemisphere":42811,"Ġcassette":42812,"includes":42813,"Ġidentifiers":42814,"ĠCALL":42815,"incent":42816,"Todd":42817,"ĠSweep":42818,"Ġ334":42819,"boss":42820,"Ġsmir":42821,"ginx":42822,"Ġtownship":42823,"Ġgrieving":42824,"ĠMosque":42825,"Netflix":42826,"ASED":42827,"ĠMillennials":42828,"ocom":42829,"1967":42830,"Ġboldly":42831,"sleep":42832,"Ġesche":42833,"arijuana":42834,"Ġswirl":42835,"ĠPenal":42836,"Ġnegligent":42837,"ĠStephenson":42838,"KER":42839,"ĠZoro":42840,"risis":42841,"Ġlocalization":42842,"ĠSeymour":42843,"ĠAnglic":42844,"reditation":42845,"protection":42846,"ĠPaige":42847,"Ġomit":42848,"ĠRousse":42849,"ĠTub":42850,"Ġinvitations":42851,"tty":42852,"Ġmoss":42853,"physical":42854,"Credits":42855,"Ġanarchy":42856,"Ġchildcare":42857,"Ġlull":42858,"ĠMek":42859,"ĠLanguages":42860,"latest":42861,"ĠSanford":42862,"Ġusability":42863,"Ġdiffuse":42864,"ĠDATA":42865,"Ġsprites":42866,"ĠVegeta":42867,"ĠPromotion":42868,"ãĥ¼ãĤ¯":42869,"ricting":42870,"zee":42871,"Turkish":42872,"ĠTDs":42873,"proven":42874,"571":42875,"Ġsmugglers":42876,"70710":42877,"Ġreformed":42878,"ĠLois":42879,"Ġunfl":42880,"ĠWITHOUT":42881,"ĠReturning":42882,"annie":42883,"ĠTomas":42884,"Franc":42885,"ĠProfit":42886,"ĠSERV":42887,"ĠRumble":42888,"ikuman":42889,"esan":42890,"Ġtesters":42891,"Ġgadget":42892,"Ġbracelet":42893,"ĠFSA":42894,"component":42895,"Ġparamedics":42896,"Ġjan":42897,"ĠRemem":42898,"ĠSkinner":42899,"Ġlov":42900,"ĠQuake":42901,"roma":42902,"Ġflask":42903,"Princ":42904,"Ġoverpower":42905,"Ġlodging":42906,"ĠKKK":42907,"rette":42908,"Ġabsorbs":42909,"wrote":42910,"Ġ,\"":42911,"Kings":42912,"ĠHail":42913,"ĠFalling":42914,"xtap":42915,"ĠHelena":42916,"irens":42917,"Larry":42918,"Ġpamphlet":42919,"ĠCPR":42920,"Gro":42921,"ĠHiroshima":42922,"Ġholistic":42923,"\".[":42924,"Ġdetachment":42925,"Ġaspire":42926,"Ġcomplicit":42927,"ĠGreenwood":42928,"Ġrespawn":42929,"ĠStupid":42930,"ĠFinished":42931,"fal":42932,"bass":42933,"Ġabhor":42934,"Ġmockery":42935,"ĠFeast":42936,"VIDEO":42937,"Ġconsec":42938,"ĠHungry":42939,"Pull":42940,"ĠHust":42941,"itance":42942,"?ãĢį":42943,")--":42944,"ĠParallel":42945,"conv":42946,"469":42947,"haar":42948,"want":42949,"Paper":42950,"mins":42951,"ĠToro":42952,"ĠTRUMP":42953,"ĠRai":42954,"DW":42955,"ĠWicked":42956,"ĠLep":42957,"Ġfunky":42958,"Ġdetriment":42959,"iosis":42960,"achev":42961,"Ġdegrade":42962,"imilation":42963,"Ġretard":42964,"Ġfragmentation":42965,"Ġcowboy":42966,"ĠYPG":42967,"ĠHAL":42968,"Parents":42969,"ĠSieg":42970,"ĠStrauss":42971,"ĠRubber":42972,"×IJ":42973,"Frag":42974,"Ġpt":42975,"Ġoptionally":42976,"ĠZIP":42977,"ĠTranscript":42978,"ĠDwell":42979,"882":42980,"Merc":42981,"ĠMOT":42982,"ãĥ¯ãĥ³":42983,"Ġhunts":42984,"Ġexecutes":42985,"Includes":42986,"Ġacidic":42987,"ĠResponsibility":42988,"ĠDumb":42989,"wei":42990,"Anderson":42991,"ĠJasper":42992,"ighton":42993,"absolutely":42994,"Adult":42995,"Ġplunder":42996,"Morning":42997,"ĠTours":42998,"ĠDane":42999,"κ":43000,"ĠTEST":43001,"ĠGina":43002,"Ġcanine":43003,"awan":43004,"Ġsocialists":43005,"ĠSoda":43006,"Ġimpetus":43007,"ĠSupplementary":43008,"oliath":43009,"ĠKinnikuman":43010,"mittedly":43011,"seconds":43012,"Ġorganisers":43013,"Ġdocumentaries":43014,"Variable":43015,"GREEN":43016,"Ġresorts":43017,"Ġbragging":43018,"Ġ368":43019,"Artist":43020,"wk":43021,"blers":43022,"Uncommon":43023,"ĠRetrieved":43024,"Ġhectares":43025,"Ġtoxin":43026,"rank":43027,"Ġfaiths":43028,"ĠGraphic":43029,"Ġvec":43030,"ĠLIA":43031,"African":43032,"Ġardent":43033,"endiary":43034,"Lake":43035,"ĠDOS":43036,"cientious":43037,"ĠOkawaru":43038,"ĠAlly":43039,"ĠTimeline":43040,"Dash":43041,"ĠIc":43042,"continue":43043,"Ġtidy":43044,"Ġinstinctively":43045,"ĠPossibly":43046,"ĠOutdoor":43047,"ĠWouldn":43048,"Ġlich":43049,"ĠBray":43050,"ĠAX":43051,"ĠÃī":43052,"Ġ+#":43053,"\\'":43054,"Directory":43055,"abiding":43056,"Ġferal":43057,"icative":43058,"butt":43059,"Ġperverse":43060,"Salt":43061,"Ġwarped":43062,"Ġnineteen":43063,"Ġcabinets":43064,"ĠsrfAttach":43065,"ĠSloan":43066,"Ġpowering":43067,"regation":43068,"Flight":43069,"severe":43070,"Ġstren":43071,"Ġcog":43072,"apache":43073,"ĠâĿ":43074,"Ġcafeteria":43075,"paces":43076,"ĠGrimoire":43077,"utonium":43078,"Ġraining":43079,"Ġcircling":43080,"Ġlinebackers":43081,"credit":43082,"Ġrepatri":43083,"ĠCamden":43084,"license":43085,"Ġlyric":43086,"Ġdescriptor":43087,"Ġvalleys":43088,"Ġreq":43089,"Ġbackstage":43090,"ĠProhibition":43091,"ĠKet":43092,"Opening":43093,"Sym":43094,"æĸ¹":43095,"Ġservings":43096,"Ġoverseen":43097,"Ġasteroids":43098,"ĠMods":43099,"ĠSpringer":43100,"ĠContainer":43101,"è»":43102,"ĠMens":43103,"Ġmultim":43104,"Ġfirefighter":43105,"pec":43106,"Ġchlorine":43107,"м":43108,"endi":43109,"Ġsparing":43110,"Ġpolygamy":43111,"ĠRN":43112,"ĠPell":43113,"Ġtigers":43114,"Ġflashy":43115,"ĠMadame":43116,"Sword":43117,"Ġprefrontal":43118,"Ġprerequisite":43119,"uca":43120,"Ġwifi":43121,"Ġmisconception":43122,"Ġharshly":43123,"ĠStreaming":43124,"otom":43125,"ĠGiuliani":43126,"footed":43127,"Ġtubing":43128,"individual":43129,"zek":43130,"nuclear":43131,"mol":43132,"Ġrightful":43133,"493":43134,"Ġspecialization":43135,"Ġpassionately":43136,"ĠVelocity":43137,"ĠAvailability":43138,"Tenn":43139,"Ġlatch":43140,"ĠSomebody":43141,"Ġhelium":43142,"claw":43143,"Ġdipping":43144,"XXX":43145,"Ġinterpersonal":43146,"710":43147,"Ġsubter":43148,"Ġbiologists":43149,"ĠLighting":43150,"Ġoptic":43151,"Ġdenim":43152,"endon":43153,"ĠCorm":43154,"Ġ341":43155,"ĠCoup":43156,"Ġfearless":43157,"Ġalot":43158,"ĠClifford":43159,"ĠRuntime":43160,"ĠProvision":43161,"updated":43162,"leneck":43163,"Ġneuron":43164,"Ġgrading":43165,"ĠCt":43166,"sequence":43167,"inia":43168,"concept":43169,"Ġroaring":43170,"rival":43171,"ĠCaucasian":43172,"Ġmonog":43173,"keyes":43174,"Ġappellate":43175,"Ġliaison":43176,"EStreamFrame":43177,"ĠPlum":43178,"!.":43179,"Ġspherical":43180,"Ġperished":43181,"Ġblot":43182,"Ġbenches":43183,"Ġ411":43184,"Ġpioneered":43185,"Ġhurled":43186,"Jennifer":43187,"ĠYosemite":43188,"Chair":43189,"Ġreefs":43190,"Ġelector":43191,"ĠAnthem":43192,"652":43193,"Ġuninstall":43194,"Ġimpede":43195,"Ġblinking":43196,"Ġgoto":43197,"Decre":43198,"Aren":43199,"Ġstabilization":43200,"ĠDisabled":43201,"ĠYanukovych":43202,"Ġoutlawed":43203,"ĠVentura":43204,"teness":43205,"Ġplantation":43206,"Ġyacht":43207,"ĠHuawei":43208,"Ġsolvent":43209,"Ġgracious":43210,"Ġcuriously":43211,"Ġcapacitor":43212,"Ġcx":43213,"ĠReflex":43214,"Phys":43215,"ĠCf":43216,"ptin":43217,"conservative":43218,"Ġinvocation":43219,"cour":43220,"FN":43221,"ĠNewly":43222,"Hour":43223,"Asian":43224,"ĠLeading":43225,"ĠAerospace":43226,"Anne":43227,"Ġprenatal":43228,"Ġdeteriorating":43229,"HCR":43230,"ĠNormandy":43231,"olini":43232,"ĠAmbro":43233,"910":43234,"Ġsetbacks":43235,"ĠTRE":43236,"Ġsig":43237,"ĠScourge":43238,"597":43239,"798":43240,"Gameplay":43241,"Ġmsec":43242,"MX":43243,"Ġpricey":43244,"ĠLLP":43245,"akeru":43246,"Ġoverarching":43247,"ĠBale":43248,"Ġworldly":43249,"Clark":43250,"Ġscenic":43251,"Ġdisliked":43252,"ĠControlled":43253,"Tickets":43254,"ĠEW":43255,"abies":43256,"ĠPlenty":43257,"Nonetheless":43258,"Ġartisan":43259,"Transfer":43260,"ĠFamous":43261,"Ġinfield":43262,"bley":43263,"Ġunresolved":43264,"ĠMLA":43265,"ãĤĤ":43266,"Correction":43267,"Ġdemocrat":43268,"ĠMoreno":43269,"rocal":43270,"ilings":43271,"Ġsailor":43272,"Ġrife":43273,"hung":43274,"Ġtropes":43275,"Ġsnatched":43276,"ĠLIN":43277,"ĠBib":43278,"ESA":43279,"ĠPrev":43280,"ĠCamel":43281,"runtime":43282,"Ġobnoxious":43283,"437":43284,"Ġsummers":43285,"Ġunexplained":43286,"ĠWalters":43287,"caliber":43288,"Ġgull":43289,"ĠEndurance":43290,"ä½ľ":43291,"Ġ347":43292,"Irish":43293,"Ġaerobic":43294,"Ġcramped":43295,"ĠHonolulu":43296,"à©":43297,"userc":43298,"ecast":43299,"ACY":43300,"ĠQuery":43301,"ãĤ¹ãĥĪ":43302,"Beta":43303,"Ġsusceptibility":43304,"ĠShiv":43305,"ĠLimbaugh":43306,"ĠÃĸ":43307,"ĠNXT":43308,"ĠMuss":43309,"ĠBritons":43310,"ESCO":43311,"EGIN":43312,"Ġ%%":43313,"Ġsecession":43314,"ĠPatron":43315,"ĠLua":43316,"naires":43317,"ĠJPMorgan":43318,"usb":43319,"ocyte":43320,"Ġcouncillors":43321,"ĠLiang":43322,"farm":43323,"Ġnervously":43324,"Ġattractiveness":43325,"ĠKov":43326,"jump":43327,"Plot":43328,"Ġstains":43329,"ĠStatue":43330,"ĠApostles":43331,"heter":43332,"ĠSUPPORT":43333,"Ġoverwhelm":43334,"YES":43335,"Ġ291":43336,"density":43337,"Ġtrapping":43338,"Mit":43339,"Ġfide":43340,"ĠPamela":43341,"atlantic":43342,"Damn":43343,"Ġpts":43344,"OPA":43345,"Ġservicing":43346,"Ġoverflowing":43347,"ulo":43348,"ĠErit":43349,"ticket":43350,"lighting":43351,"ĠHmm":43352,"ãĥ¼ãĥ«":43353,"imoto":43354,"Ġchuckle":43355,"423":43356,"ãģķ":43357,"shape":43358,"Ġqueues":43359,"Ġanchors":43360,"ãĤ¼ãĤ¦ãĤ¹":43361,"Fer":43362,"Ġawoke":43363,"Ġ666":43364,"hands":43365,"Ġdivergence":43366,"Ġ505":43367,"Tips":43368,"Ġdepot":43369,"Ġskew":43370,"ĠDeliver":43371,"opot":43372,"Ġdivul":43373,"ĠEB":43374,"unsigned":43375,"ĠUni":43376,"Xbox":43377,"Ġforks":43378,"Ġ702":43379,"å¯":43380,"Ġpromoters":43381,"ĠVapor":43382,"Ġlevied":43383,"slot":43384,"Ġpigment":43385,"Ġcylinders":43386,"CRE":43387,"Ġsnatch":43388,"Ġperpetually":43389,"Ġlicking":43390,"ĠFeet":43391,"ĠKraken":43392,"ĠHolden":43393,"ĠCLSID":43394,"mr":43395,"Ġprojector":43396,"Ġdenotes":43397,"Ġchapel":43398,"ĠTorrent":43399,"bler":43400,"Route":43401,"ĠDefendant":43402,"ĠPublishers":43403,"ĠMales":43404,"ĠInnov":43405,"ĠAgility":43406,"riter":43407,"tymology":43408,"stores":43409,"Lind":43410,"Ġfolly":43411,"ĠZurich":43412,"Ble":43413,"Ġnurture":43414,"Ġcoastline":43415,"uchin":43416,"Domin":43417,"Ġfrivol":43418,"ĠConsolid":43419,"results":43420,"MJ":43421,"Ġphylogen":43422,"Ġhauled":43423,"ĠWiley":43424,"ĠJessie":43425,"ĠPrepare":43426,"ĠEps":43427,"Ġtreasurer":43428,"IAS":43429,"Ġcolonists":43430,"Ġinund":43431,"ĠWWF":43432,"ĠConverted":43433,"6000":43434,"outside":43435,"ĠAppearance":43436,"ĠRelic":43437,"ĠMister":43438,"saw":43439,"Ġresultant":43440,"Ġadjective":43441,"ĠLaurel":43442,"ĠHindi":43443,"bda":43444,"Peace":43445,"Ġrebirth":43446,"Ġmembranes":43447,"Ġforwarding":43448,"Ġcollided":43449,"ĠCarolyn":43450,"Kansas":43451,"599":43452,"ĠSolidGoldMagikarp":43453,"Beck":43454,"Ġstressing":43455,"ĠGoo":43456,"ĠCooperative":43457,"Ġfs":43458,"ĠArchie":43459,"Liter":43460,"ĠKlopp":43461,"Jerry":43462,"Ġfootwear":43463,"Warren":43464,"Ġscree":43465,"hare":43466,"Understanding":43467,"Ped":43468,"Ġanthology":43469,"ĠAnnounce":43470,"Mega":43471,"Ġfluent":43472,"Ġbondage":43473,"ĠDiscount":43474,"ilial":43475,"Cart":43476,"ĠNightmares":43477,"Sham":43478,"ĠBoll":43479,"ussie":43480,"Http":43481,"Atlanta":43482,"Ġunrecogn":43483,"ĠBid":43484,"Ġundergrad":43485,"Ġforgiving":43486,"ĠGlover":43487,"AAAAAAAA":43488,"445":43489,"VG":43490,"paio":43491,"killers":43492,"Ġresponsibly":43493,"Ġmobilize":43494,"Ġeffected":43495,"ĠLumin":43496,"Ġkale":43497,"Ġinfringing":43498,"announced":43499,"Ġfitt":43500,"batch":43501,"ĠTackle":43502,"ĠLime":43503,"ĠAPP":43504,"ukemia":43505,"Ġruby":43506,"Ġexoner":43507,"ĠCasual":43508,"070":43509,"Ġpelvic":43510,"Ġautomate":43511,"ĠKear":43512,"ĠCoastal":43513,"Ġcreed":43514,"Ġboredom":43515,"ĠStun":43516,"riott":43517,"Ĥİ":43518,"Ġregenerate":43519,"Ġcomedians":43520,"ĠOPER":43521,"Spons":43522,"idium":43523,"onis":43524,"Located":43525,"057":43526,"Ġsuspense":43527,"ĠDating":43528,"Cass":43529,"Ġneocons":43530,"ĠShinzo":43531,"Ġawoken":43532,"christ":43533,"ĠMessages":43534,"attled":43535,"ĠSpray":43536,"ĠSpice":43537,"CW":43538,"Ġshielding":43539,"ĠGaul":43540,"Amid":43541,"Ġparamilitary":43542,"Ġmultif":43543,"ĠTanner":43544,"ilk":43545,"Ġgoddamn":43546,"gements":43547,"Ġbefriend":43548,"mobi":43549,"Ġ388":43550,"folder":43551,"acca":43552,"Ġinsin":43553,"gap":43554,"Nev":43555,"fifth":43556,"Ġpsychiatry":43557,"banks":43558,"THIS":43559,"Ġharb":43560,"acqu":43561,"Ġfacade":43562,"ĠPowerPoint":43563,"803":43564,"Ġbluff":43565,"Shares":43566,"Ġfavoring":43567,"Elizabeth":43568,"ÃįÃį":43569,"Ġranger":43570,"772":43571,"ĠArche":43572,"hak":43573,"ĠGenetics":43574,"ĠFEMA":43575,"Ġevolves":43576,"Ġeste":43577,"ĠPets":43578,"ĠMé":43579,"ĠInteresting":43580,"ĠCanterbury":43581,"chapter":43582,"ĠStarfleet":43583,"Spanish":43584,"Ġdrawback":43585,"ĠNorwich":43586,"970":43587,"north":43588,"aganda":43589,"Ġtransformative":43590,"ramids":43591,"biology":43592,"aday":43593,"Ġpropagation":43594,"ĠGamma":43595,"ĠDenise":43596,"ĠCalculator":43597,"entimes":43598,"ĠBett":43599,"Ġappendix":43600,"ĠHDD":43601,"AKING":43602,"Ġstigmat":43603,"Ġholster":43604,"Ġordinarily":43605,"Chance":43606,"ĠContrary":43607,"Ġadhesive":43608,"Ġgathers":43609,"612":43610,"reau":43611,"onyms":43612,"eways":43613,"Ġinduces":43614,"Ġinterchangeable":43615,"sem":43616,"Whit":43617,"Ġtrance":43618,"Ġincorporation":43619,"ĠExtras":43620,"Financial":43621,"Ġawkwardly":43622,"ĠSturgeon":43623,"ĠHY":43624,"Normally":43625,"ĠEnding":43626,"ĠAssist":43627,"encrypted":43628,"Ġsubjug":43629,"Ġnos":43630,"Ġfanatic":43631,"Cub":43632,"CU":43633,"?\".":43634,"Ġirreversible":43635,"åĤ":43636,"031":43637,"ĠHAR":43638,"spread":43639,"ulia":43640,"=$":43641,"Scope":43642,"Lots":43643,"Ġlifestyles":43644,"olon":43645,"Ġfeds":43646,"Ġcongratulate":43647,"webkit":43648,"Ġindistinguishable":43649,"ĠSwing":43650,"Ġcommandments":43651,"quila":43652,"abella":43653,"methyl":43654,"annabin":43655,"Ġovere":43656,"Ġlobster":43657,"ĠQUEST":43658,"ĠCONTIN":43659,"bernatorial":43660,"::::::::":43661,"ĠTrave":43662,"ĠSamoa":43663,"ANI":43664,"752":43665,"д":43666,"usercontent":43667,"ĠModerate":43668,"yeah":43669,"ĠKitt":43670,"Ġwee":43671,"Ġstuffing":43672,"ĠIntervention":43673,"ĠDign":43674,"Ġwarehouses":43675,"ĠFiji":43676,"Ġpellets":43677,"Ġtakeaway":43678,"ĠTABLE":43679,"ĠClassical":43680,"collection":43681,"Ġlandfall":43682,"ĠMuscle":43683,"Ġsettles":43684,"ĠADV":43685,"Ġ344":43686,"Laura":43687,"Ġfared":43688,"ĠPartial":43689,"436":43690,"ossibility":43691,"ĠDaly":43692,"ĠTarant":43693,"ĠFuji":43694,"aml":43695,"cence":43696,"551":43697,"ĠProcedures":43698,"ĠOCD":43699,"ĠUD":43700,"tin":43701,"QUI":43702,"acho":43703,"438":43704,"Ġglitches":43705,"Ġenchantment":43706,"Ġcalculates":43707,"IRO":43708,"ĠHua":43709,"alyses":43710,"ĠLift":43711,"umo":43712,"Ġleapt":43713,"Ġhypothesized":43714,"ĠGustav":43715,"itans":43716,"VERSION":43717,"æł":43718,"Roger":43719,"Ġrand":43720,"ĠAdapter":43721,"Ġ331":43722,"ĠPetition":43723,"kies":43724,"Mars":43725,"Ġundercut":43726,"zees":43727,"ĠLyons":43728,"ĠDHCP":43729,"Missing":43730,"Ġretirees":43731,"Ġinsidious":43732,"eli":43733,">)":43734,".ãĢį":43735,"Ġfinalists":43736,"ĠAure":43737,"Ġaccuser":43738,"Ġwastes":43739,"ĠYs":43740,"ĠLori":43741,"Ġconstituencies":43742,"Ġsupper":43743,"Ġmayhem":43744,"orange":43745,"Ġmisplaced":43746,"Ġmanagerial":43747,"Ġexce":43748,"ĠCLI":43749,"Ġprimal":43750,"ĠLent":43751,"Crystal":43752,"hover":43753,"ĠNTS":43754,"endum":43755,"Ġdw":43756,"ĠAlc":43757,"nostic":43758,"Ġpreserves":43759,"ĠTsarnaev":43760,"Ġtripled":43761,"relative":43762,"Arcade":43763,"killing":43764,"ĠWEEK":43765,"ĠHanna":43766,"Dust":43767,"Completed":43768,"ģ«":43769,"Ġapproves":43770,"ĠSurf":43771,"ĠLutheran":43772,"venants":43773,"Ġrobberies":43774,"weights":43775,"software":43776,"atana":43777,"ugal":43778,"Ġgravy":43779,"ĠCance":43780,"OLOGY":43781,"lyak":43782,"Tonight":43783,"Ġunveil":43784,"Ġ1904":43785,"ĠMinion":43786,"entious":43787,"stice":43788,"packages":43789,"ĠGEAR":43790,"Ġgol":43791,"ĠHutchinson":43792,"ĠProfession":43793,"ĠGUN":43794,"ĠDifference":43795,"ĠTsukuyomi":43796,"ĠLesbian":43797,"670":43798,"Ġfugitive":43799,"ĠPlanetary":43800,"--------------------------------------------------------":43801,"Ġaccrued":43802,"Ġchicks":43803,"Ġstopp":43804,"Ġblockers":43805,"Cod":43806,"Ġcommenters":43807,"ĠSomewhere":43808,"ĠPhotographer":43809,"theme":43810,"Ġmayoral":43811,"wu":43812,"Ġantennas":43813,"Ġrevamped":43814,"ĠSubjects":43815,"ité":43816,"imura":43817,"Ġentrances":43818,"literally":43819,"Ġtenets":43820,"ĠOMG":43821,"ĠMPH":43822,"ĠDonkey":43823,"ĠOffense":43824,"Ġ\"+":43825,"Snap":43826,"ĠAFB":43827,"Ġanimate":43828,"ĠSod":43829,"Hispanic":43830,"Ġinconsistency":43831,"Db":43832,"FY":43833,"Export":43834,"Ġape":43835,"Ġpearl":43836,"ibel":43837,"ĠPACs":43838,"Ġ{\\":43839,"Ġactu":43840,"ĠHSBC":43841,"campus":43842,"Ġpayoff":43843,"Ġdeities":43844,"ĠNato":43845,"ouple":43846,"Ġcensored":43847,"ĠClojure":43848,"Ġconfounding":43849,"eni":43850,"Ġreckon":43851,"ophe":43852,"Ġspotting":43853,"Ġsignifies":43854,"Ġpropel":43855,"Ġfestive":43856,"Suggest":43857,"Ġpledging":43858,"ĠBerman":43859,"Ġrebellious":43860,"Ġovershadowed":43861,"Ġinfiltrated":43862,"jobs":43863,"672":43864,"Ġscalable":43865,"Ġdominion":43866,"ĠNewfoundland":43867,"ĠMeadow":43868,"Ġpartitions":43869,"AMI":43870,"Ġsupplementary":43871,"strument":43872,"Ġhairy":43873,"Ġperpetuate":43874,"Ġnutshell":43875,"ĠPotato":43876,"ĠHobbit":43877,"Ġcurses":43878,"Float":43879,"Ġquieter":43880,"Ġfueling":43881,"Ġcapsules":43882,"ĠLust":43883,"ĠHaunted":43884,"Executive":43885,"Ġchildbirth":43886,"Gre":43887,"Ġradiant":43888,"åİ":43889,"Ġmalls":43890,"Ġinept":43891,"ĠWarranty":43892,"Ġspectator":43893,"Eh":43894,"thens":43895,"Ġculminating":43896,"æ©":43897,"arya":43898,"ãĤ®":43899,"ilitarian":43900,"ĠORIG":43901,"ĠSpending":43902,"ptives":43903,"ĠSiren":43904,"ĠRecording":43905,"ayne":43906,"Ġvim":43907,"Ġsprang":43908,"Tang":43909,"ĠMFT":43910,"morning":43911,"ĠWeed":43912,"mpeg":43913,"cession":43914,"ĠChung":43915,"730":43916,"warning":43917,"562":43918,"handedly":43919,"Poor":43920,"Politics":43921,":#":43922,"Ġpian":43923,"Ġfeces":43924,"ĠDocumentation":43925,"Ġbanished":43926,"Ġ399":43927,"ĠARC":43928,"Ġheinous":43929,"Jake":43930,"ĠAmir":43931,"wayne":43932,"vre":43933,"oshenko":43934,"Ġnotebooks":43935,"Ġfoundational":43936,"Ġmarvelous":43937,"ixtape":43938,"Ġwithdrawals":43939,"Ġhorde":43940,"ĠDhabi":43941,"isable":43942,"ĠKD":43943,"Ġcontagious":43944,"ĠDip":43945,"ĠArrows":43946,"Ġpronouns":43947,"Ġmorphine":43948,"ĠBUS":43949,"682":43950,"Ġkosher":43951,"finished":43952,"ĠInstruments":43953,"Ġfused":43954,"yden":43955,"ĠSalmon":43956,"Fab":43957,"affected":43958,"KEN":43959,"CENT":43960,"Domain":43961,"Ġpokemon":43962,"ĠDrinking":43963,"Growing":43964,"ĠInvestigative":43965,"ĠAether":43966,"emi":43967,"Ġtabloid":43968,"Ġrepro":43969,"ĠNotwithstanding":43970,"ĠBerserker":43971,"Ġdramas":43972,"Ġcliché":43973,"Ġbung":43974,"ĠURI":43975,"ĠDos":43976,"044":43977,"Ġpastors":43978,"Ġls":43979,"Ġacrylic":43980,"aunts":43981,"Edward":43982,"Ġmajorities":43983,"Bang":43984,"Ġfielding":43985,"ĠReplacement":43986,"ĠAlchemy":43987,"ppard":43988,"ĠRomeo":43989,"ĠSanct":43990,"ĠLavrov":43991,"ibble":43992,"Instruct":43993,"Ġimpractical":43994,"ĠPlayboy":43995,"cephal":43996,"Ġswaps":43997,"Ġkan":43998,"ĠTheo":43999,"Ġillustrating":44000,"Ġdismantled":44001,"ĠTransgender":44002,"ĠGuth":44003,"UGH":44004,"Ġtriumphant":44005,"Ġencompass":44006,"Ġbookmark":44007,"uddin":44008,"jer":44009,"Ġpredicate":44010,"ESH":44011,"Ġwhence":44012,"ĠABE":44013,"Ġnonprofits":44014,"Sequ":44015,"Ġdiabetic":44016,"Ġpend":44017,"Ġheartfelt":44018,"shi":44019,"Ġinteracts":44020,"ĠTelecom":44021,"Ġbombardment":44022,"depending":44023,"ĠLowry":44024,"ĠAdmission":44025,"ĠBlooming":44026,"ustration":44027,"enegger":44028,"Brew":44029,"Ġmolten":44030,"ĠNerd":44031,"PIN":44032,"âĸĢ":44033,"avement":44034,"Ġtoured":44035,"Ġcoefficients":44036,"ĠTrayvon":44037,"ansson":44038,"Ġsandy":44039,"told":44040,"flows":44041,"Ġpopulous":44042,"ĠTinder":44043,"ĠBliss":44044,"Rachel":44045,"Minimum":44046,"Ġcontestant":44047,"ĠReduce":44048,"ĠMorse":44049,"ĠGrassley":44050,"ĠClicker":44051,"Ġexpr":44052,"Ġsincerity":44053,"Ġmarqu":44054,"Ġelicit":44055,"ĠProposition":44056,"ĠDemonic":44057,"Ġtacos":44058,"Greek":44059,"Ġpostwar":44060,"Ġinsofar":44061,"ĠPork":44062,"Ġ352":44063,"doctoral":44064,"walking":44065,"Ġmidterm":44066,"ĠSammy":44067,"sighted":44068,"ĠTRANS":44069,"ici":44070,"ALD":44071,"ĠUSL":44072,"ĠFISA":44073,"ĠAmpl":44074,"ĠAlexandra":44075,"inelli":44076,"Train":44077,"Ġsignify":44078,"ĠVersus":44079,"Ġobfusc":44080,"Ġkh":44081,"Ġaggro":44082,"ĠRenault":44083,"Ġ348":44084,"518":44085,"oxicity":44086,"022":44087,"ĠTwist":44088,"Ġgoofy":44089,"Dynamic":44090,"Ġbriefings":44091,"might":44092,"899":44093,"Ġderogatory":44094,"Tro":44095,"Ġforging":44096,"ĠKoran":44097,"ĠMarried":44098,"ĠBucs":44099,"Ġpalate":44100,"ĠConversion":44101,"mable":44102,"413":44103,"Ġ(_":44104,"Ġsiph":44105,"ĠNEO":44106,"college":44107,"Ġmarginally":44108,"Ġflirt":44109,"ĠTraps":44110,"ĠPace":44111,"é»Ĵ":44112,"Ġgoaltender":44113,"Ġforbids":44114,"Ġclerks":44115,"ĠTant":44116,"ĠRobbins":44117,"ĠPrinting":44118,"Ġpremiered":44119,"Ġmagnification":44120,"ĠTG":44121,"ĠRouse":44122,"ĠMock":44123,"odynamics":44124,"Ġpreclude":44125,"ismo":44126,"ĠPulitzer":44127,"Ġavalanche":44128,"ĠKodi":44129,"ribune":44130,"ĠLena":44131,"Electric":44132,"Ġrefinery":44133,"Ġendowed":44134,"Ġcounselors":44135,"Ġdolphin":44136,"ĠMith":44137,"Ġarmoured":44138,"hibited":44139,"Begin":44140,"ĠPW":44141,"Oil":44142,"ĠVor":44143,"ĠSharif":44144,"ĠFrazier":44145,"estate":44146,"Ġjams":44147,"Proxy":44148,"Ġbandits":44149,"ĠPresbyterian":44150,"ĠPremiere":44151,"tiny":44152,"ĠCruel":44153,"Testing":44154,"Ġhomer":44155,"ĠVERS":44156,"ĠProl":44157,"ĠDeposit":44158,"ĠCoffin":44159,"Ġseminars":44160,"Ġsql":44161,"ĠDefendants":44162,"Alternatively":44163,"ĠRats":44164,"ç«":44165,"ethyst":44166,"'>":44167,"Ġissuer":44168,"589":44169,"Ġchaired":44170,"ĠAccessories":44171,"manent":44172,"Ġmarrow":44173,"ĠPrimordial":44174,"CN":44175,"Ġlimitless":44176,"ĠCarnage":44177,"Ġundrafted":44178,"qv":44179,"INESS":44180,"onew":44181,"Ġcohesion":44182,"987":44183,"Ġnecks":44184,"Ġfootballer":44185,"ĠGER":44186,"Ġdetectable":44187,"ĠSupporting":44188,"ĠCSV":44189,"ocally":44190,"kHz":44191,"Ġunde":44192,"Ġshone":44193,"Ġbudding":44194,"trak":44195,"Standing":44196,"ĠStarcraft":44197,"ĠKemp":44198,"Bench":44199,"Ġthwarted":44200,"ĠGrounds":44201,"athi":44202,"Lisa":44203,"Dialog":44204,"ĠSX":44205,"Vision":44206,"Ġingenious":44207,"ÙIJ":44208,"Ġfostering":44209,"ĠZa":44210,"ĠIngram":44211,"Ġ\"@":44212,"Naturally":44213,"616":44214,"035":44215,"ĠFAC":44216,"Hmm":44217,"554":44218,"Ġaccelerator":44219,"ĠVend":44220,"Ġsunscreen":44221,"Ġtuberculosis":44222,"raviolet":44223,"ĠFunctional":44224,"ĠErrors":44225,"edar":44226,"1966":44227,"ĠSpectre":44228,"ĠRecipes":44229,"885":44230,"ĠMankind":44231,"Liverpool":44232,"Ġ|--":44233,"Ġsubstitutes":44234,"ĠXT":44235,"wired":44236,"Ġinco":44237,"ĠAfgh":44238,"Eva":44239,"icc":44240,"Song":44241,"Knight":44242,"Ġdiligently":44243,"ĠBroadcast":44244,"Aid":44245,"Ġafar":44246,"ĠHMS":44247,"atonin":44248,"ĠGrateful":44249,"Ġfireplace":44250,"ĠOmni":44251,"euro":44252,"ĠFRE":44253,"ĠShib":44254,"ĠDigest":44255,"toggle":44256,"Ġheadsets":44257,"Ġdiffusion":44258,"ĠSquirrel":44259,"ĠFN":44260,"Ġdarkened":44261,"outher":44262,"Ġsleeps":44263,"ĠXer":44264,"guns":44265,"Ġsetups":44266,"Ġparsed":44267,"Ġmammoth":44268,"ĠCurious":44269,"gob":44270,"ĠFitzpatrick":44271,"ĠEmil":44272,"imov":44273,".............":44274,"ĠBenny":44275,"Secondly":44276,"Ġhearty":44277,"Ġconson":44278,"stained":44279,"Ġgalactic":44280,"clave":44281,"Ġplummeted":44282,"Ġpests":44283,"Ġswat":44284,"Ġreferrals":44285,"ĠLionel":44286,"holy":44287,"Ġunderdog":44288,"ĠSlater":44289,"ĠProvide":44290,"ĠAmar":44291,"ressor":44292,"åĮ":44293,"onga":44294,"Ġtimid":44295,"Ġpiety":44296,"ĠDek":44297,"Ġsurging":44298,"azo":44299,"Ġ610":44300,"Ġdesks":44301,"ĠSpokane":44302,"ĠAnfield":44303,"Ġwarships":44304,"ĠCobra":44305,"Ġarming":44306,"clusively":44307,"ĠBadge":44308,"agascar":44309,"ĠPRESS":44310,"ĠMcKenzie":44311,"ĠFerdinand":44312,"burning":44313,"Afee":44314,"Ġtyrann":44315,"ĠIw":44316,"ĠBoone":44317,"1007":44318,"ĠRept":44319,"ĊÂł":44320,"Ġcaravan":44321,"ĠDill":44322,"ĠBundesliga":44323,"Chuck":44324,"Ġhealer":44325,"ãĥ¼ãĥĨ":44326,"ĠHobby":44327,"Ġnegate":44328,"Ġcritiques":44329,"sectional":44330,"mopolitan":44331,"Ġdx":44332,"Ġoutsourcing":44333,"ĠCipher":44334,"tap":44335,"Sharp":44336,"Ġupbeat":44337,"Ġhangar":44338,"Ġcruising":44339,"ĠNiagara":44340,"Ġ342":44341,"illus":44342,"ĠSv":44343,"Ġsubtitles":44344,"Ġsquared":44345,"Ġbookstore":44346,"Ġrevolutionaries":44347,"ĠCarlton":44348,"abal":44349,"Utah":44350,"Ġdespise":44351,"ĠUM":44352,"consider":44353,"aido":44354,"Ġcarts":44355,"ĠTurtles":44356,"Training":44357,"Ġhonorary":44358,"¢":44359,"Ġtriangles":44360,"422":44361,"Ġreprinted":44362,"Ġgraceful":44363,"ĠMongolia":44364,"Ġdisruptions":44365,"ĠBoh":44366,"Ġ349":44367,"Ġdrains":44368,"Ġconsulate":44369,"Ġbends":44370,"Ġmafia":44371,"uron":44372,"ĠFulton":44373,"misc":44374,"Ġrenal":44375,"Ġinaction":44376,"cking":44377,"Ġphotons":44378,"Ġbruised":44379,"ĠCodes":44380,"ogi":44381,"Ġnests":44382,"ĠLovely":44383,"ĠLibre":44384,"ĠDaryl":44385,"Ġ###":44386,"Sys":44387,".,\"":44388,"Ġfreezes":44389,"establishment":44390,"andowski":44391,"Ġcumbers":44392,"ĠStarg":44393,"ĠBombs":44394,"Ġlegions":44395,"Ġhandwriting":44396,"Ġgrun":44397,"ĠCah":44398,"sequent":44399,"Ġmoth":44400,"ĠMSM":44401,"Insert":44402,"Fif":44403,"Ġmotel":44404,"Ġdexter":44405,"ĠBild":44406,"heartedly":44407,"Ġprope":44408,"ĠTexture":44409,"ĠJunction":44410,"ynthesis":44411,"ocard":44412,"ĠVera":44413,"ĠBarth":44414,"Ġμg":44415,"Ġlashed":44416,"Ġ351":44417,"ĠZamb":44418,"ĠStaples":44419,"ĠCortex":44420,"ĠCorker":44421,"Ġcontinuum":44422,"ĠWRITE":44423,"unta":44424,"ridor":44425,"Ġdeems":44426,"033":44427,"ĠGOLD":44428,"pas":44429,"Ġrepressive":44430,"ãĥĨãĤ£":44431,"Ġbaffled":44432,"Scar":44433,"Ġcrave":44434,"Ġ______":44435,"Ġentrepreneurship":44436,"ĠDirectorate":44437,"Ġ'[":44438,"Ġvines":44439,"Ġascended":44440,"ĠGROUP":44441,"ĠGoodbye":44442,"Ġdogged":44443,"ãĥ´ãĤ¡":44444,"Manufact":44445,"Ġunimaginable":44446,"riots":44447,"ierrez":44448,"Ġrelativity":44449,"ĠCrafting":44450,"raught":44451,"uden":44452,"cookie":44453,"Ġassassins":44454,"Ġdissatisfied":44455,"acci":44456,"Ġconduit":44457,"Spread":44458,"ĠRican":44459,"nice":44460,"izzle":44461,"Ġscares":44462,"ĠWHY":44463,"phans":44464,"535":44465,"Ġprotracted":44466,"ĠKristen":44467,"536":44468,"ĠScrib":44469,"ĠNeh":44470,"Ġtwenties":44471,"Ġpredicament":44472,"Ġhandcuffs":44473,"Ġfruitful":44474,"ĠUL":44475,"ĠLudwig":44476,"Ġattest":44477,"ĠBreaker":44478,"Ġbiologically":44479,"ĠDealer":44480,"Ġrenovations":44481,"fw":44482,"essen":44483,"Alice":44484,"ĠHenri":44485,"Ġunilaterally":44486,"ĠSidd":44487,"hai":44488,"ĠStretch":44489,"Sales":44490,"Ġcumbersome":44491,"ĠJavier":44492,"Ġtrendy":44493,"Ġrotting":44494,"ĠChallenges":44495,"Ġscraps":44496,"Ġfacets":44497,"ĠVeronica":44498,"ĠVerge":44499,"ĠSana":44500,"Alien":44501,"ĠRih":44502,"Ġradial":44503,"ectar":44504,"Ġ630":44505,"cli":44506,"Marie":44507,"Ġwildfire":44508,"ĠCato":44509,"hander":44510,"Ġwaitress":44511,"Ġchops":44512,"ĠSECTION":44513,"Ġbluntly":44514,"ĠCatalog":44515,"nian":44516,"study":44517,"Ġpatrolling":44518,"ĠTenth":44519,"nexus":44520,"ĠNON":44521,"opsy":44522,"Ġscathing":44523,"sie":44524,"Ġdeteriorated":44525,"VB":44526,"Nazis":44527,"Ġdepictions":44528,"Ġauthenticated":44529,"ĠConce":44530,"krit":44531,"Ġpromulg":44532,"ĠLONG":44533,"UFC":44534,"ĠVisitors":44535,"ĠRecall":44536,"Ġrehabilit":44537,"ĠSLI":44538,"Ġglacier":44539,"ĠBite":44540,"Ġ503":44541,"Ġvomit":44542,"Ġfermented":44543,"ĠKhalid":44544,"Ġgraded":44545,"ĠMagicka":44546,"ĠIchigo":44547,"powerful":44548,"icators":44549,"753":44550,"Ġshrew":44551,"Ġ356":44552,"Ġlegalizing":44553,"Ġallotted":44554,"ĠArchdemon":44555,"ithing":44556,"iggurat":44557,"VOL":44558,"Leod":44559,"Ġoily":44560,"Ġinducing":44561,"Ġamygdala":44562,"Ġadmins":44563,"ĠAcquisition":44564,"CAN":44565,"Ġschematic":44566,"Ġmoan":44567,"ĠCameroon":44568,"Ġtink":44569,"Ġmerry":44570,"Ġbutterflies":44571,"ĠGoff":44572,"Ġworkspace":44573,"ĠCorona":44574,"Ġjavascript":44575,"ĠDolphin":44576,"ĠCantor":44577,"464":44578,"toe":44579,"APS":44580,"ĠAging":44581,"Ġpadded":44582,"ĠZheng":44583,"ĠHeld":44584,"Ġestranged":44585,"Ġ770":44586,".}":44587,"ĠDunham":44588,"Ġsmokes":44589,"Ġcapitals":44590,"undai":44591,"Shin":44592,"ĠFounding":44593,"Ġentitle":44594,"Ġcenterpiece":44595,"Discover":44596,"Ġthereto":44597,"alert":44598,"ĠNou":44599,"ĠAnalyst":44600,"lc":44601,"FH":44602,"FIELD":44603,"ĠPOV":44604,"gray":44605,"Ġarcs":44606,"ĠHOT":44607,"Ġrs":44608,"Ġobligatory":44609,"ĠArchitects":44610,"ĠSven":44611,"ĠFEC":44612,"0200":44613,"Christmas":44614,"ĠAlbania":44615,"ratom":44616,"587":44617,"Ġhardships":44618,"Ġautos":44619,"ĠCharges":44620,"Ġapes":44621,"Ġ376":44622,"wallet":44623,"Ġintoxication":44624,"Ġgoblin":44625,"Ġ570":44626,"++++++++++++++++":44627,"ĠYelp":44628,"ĠMagnetic":44629,"ĠBriggs":44630,"Rail":44631,"Ġspawns":44632,"ĠWiggins":44633,"Ġshowcased":44634,"Ġresorted":44635,"uben":44636,"Ġwhipping":44637,"Ġimitate":44638,"Ġdigestion":44639,"ĠUSPS":44640,"ĠGest":44641,"Ġyea":44642,"ĠTight":44643,"indal":44644,"icas":44645,"`.":44646,"CAST":44647,"'';":44648,"ĠFet":44649,"opathic":44650,"Invalid":44651,"Ġregretted":44652,"Ġbroccoli":44653,"ĠScores":44654,"eve":44655,"Ġpostings":44656,"Ġaccumulating":44657,"Ġneedless":44658,"elfth":44659,"Ġmayors":44660,"Ġscrib":44661,"Ġanecdotes":44662,"Ġbotched":44663,"ĠRibbon":44664,"ĠConstantine":44665,"iuses":44666,"esses":44667,"Ġdevise":44668,"Compared":44669,"Ġpudding":44670,"Ġgarg":44671,"Ġevoke":44672,"797":44673,"Ġdetox":44674,"909":44675,"ĠPieces":44676,"ĠMcCartney":44677,"Ġmetast":44678,"ĠKrypt":44679,"POR":44680,"Ġtending":44681,"ĠMerchants":44682,"Proof":44683,"ĠVarg":44684,"ĠPortable":44685,"ãĥ¼ãĥĨãĤ£":44686,"Brain":44687,"2500":44688,"Ġfoliage":44689,"ع":44690,"Ġmentors":44691,"ĠAires":44692,"Ġminimalist":44693,"Ġingested":44694,"ĠTrojan":44695,"ĠQian":44696,"involved":44697,"027":44698,"Ġeroded":44699,"RAFT":44700,"Ġblurry":44701,"Mob":44702,"Ġbuffet":44703,"ĠFnatic":44704,"aea":44705,"KNOWN":44706,"ĠInit":44707,"safety":44708,"enum":44709,"ACTION":44710,"ĠCrusher":44711,"ĠDates":44712,"Ġ................":44713,"calling":44714,"akov":44715,"Ġventured":44716,"Ġ555":44717,"auga":44718,"Hart":44719,"ĠAero":44720,"MAC":44721,"Ġthinly":44722,"Ġarra":44723,"STATE":44724,"ilde":44725,"ĠJacqu":44726,"ĠFemales":44727,"Ġtheorem":44728,"Ġ346":44729,"Ġsmartest":44730,"ĠPUBLIC":44731,"ĠKron":44732,"ĠBits":44733,"ĠVessel":44734,"ĠTelephone":44735,"Ġdecap":44736,"Ġadjunct":44737,"ĠSEN":44738,"merga":44739,"Ġredacted":44740,"Ġprehistoric":44741,"Ġexplanatory":44742,"ĠRuns":44743,"ĠUttar":44744,"ĠManny":44745,"ĠAUTHOR":44746,"ĠUnleashed":44747,"ĠBowling":44748,"beans":44749,"793":44750,"Ġuniverses":44751,"Ġsensit":44752,"ĠKung":44753,"repeat":44754,"ctrl":44755,"Ġpaced":44756,"Ġfuller":44757,"Clock":44758,"Ġrecomb":44759,"ĠFaul":44760,"ĠBunker":44761,"Ġpooled":44762,"Ġana":44763,"ĠMouth":44764,"LLOW":44765,"humane":44766,"Ġbulldo":44767,"ĠMichaels":44768,"fam":44769,"Ġwrecked":44770,"Ġportrays":44771,"ĠWhale":44772,"ĠHes":44773,"Ġguesses":44774,"ĠBrowse":44775,"ĠLAPD":44776,"Ġconsequential":44777,"ĠInnocent":44778,"ĠDRAG":44779,"Ġtransgress":44780,"ĠOaks":44781,"Ġtrivia":44782,"ĠReson":44783,"ĠADS":44784,"--+":44785,"ĠToll":44786,"Ġgrasping":44787,"ĠTHEM":44788,"ĠTags":44789,"ĠConclusion":44790,"Ġpracticable":44791,"Ġhoop":44792,"Ġunintentionally":44793,"Ġignite":44794,"ĠMov":44795,"urized":44796,"lehem":44797,"Termin":44798,"Ġcolourful":44799,"ĠLinear":44800,"ĠEllie":44801,"Gy":44802,"Ġmanpower":44803,"Ġjs":44804,"Ġemoji":44805,"ĠSHARES":44806,"_.":44807,"00007":44808,"Ġsophistication":44809,"Ġunderscore":44810,"Ġpractise":44811,"Ġblob":44812,"opens":44813,"Ukraine":44814,"Keeping":44815,"YC":44816,"JR":44817,"ultimate":44818,"Claim":44819,"Ġautomobiles":44820,"993":44821,"steel":44822,"Ġparting":44823,"ĠLank":44824,"...?":44825,"Ġ385":44826,"Ġremembrance":44827,"Ġeased":44828,"Ġcovari":44829,"ĠSind":44830,"Effective":44831,"Ġdissemination":44832,"ĠMoose":44833,"ĠClapper":44834,"brates":44835,"Apply":44836,"Ġinvis":44837,"Ġworsened":44838,"âĢĶ-":44839,"Ġlegislator":44840,"ĠLol":44841,"ĠRowe":44842,"Ġdealership":44843,"umar":44844,"idences":44845,"Ġinvestigates":44846,"Ġcascade":44847,"Ġbidder":44848,"ĠBEN":44849,"Ironically":44850,"Ġpresiding":44851,"Ġding":44852,"Ġcontradicted":44853,"Ġshuts":44854,"ĠFIX":44855,"Ġ366":44856,"District":44857,"Ġsinful":44858,"ĠCharisma":44859,"oops":44860,"Ġtotality":44861,"Ġrestitution":44862,"ĠOptimus":44863,"ĠDah":44864,"Ġclueless":44865,"urned":44866,"Ġnutrit":44867,"Ġlandowners":44868,"Ġflushed":44869,"Ġbroaden":44870,"mie":44871,"Ġprintln":44872,"Ġnig":44873,"ĠCorpus":44874,"Jen":44875,"Ġproto":44876,"ĠWikimedia":44877,"ĠPalo":44878,"COR":44879,"Ġstorylines":44880,"Ġevangelicals":44881,"ĠDarrell":44882,"Ġrotor":44883,"ĠHW":44884,"skilled":44885,"eryl":44886,"Ġbegg":44887,"ĠBlumenthal":44888,"Ġweaving":44889,"Ġdownwards":44890,"ĠJacket":44891,"ĠANGEL":44892,"Technology":44893,"Ġesoteric":44894,"aldehyde":44895,"Ġfuriously":44896,"Ġforeigner":44897,"Weak":44898,"CHO":44899,"ĠHound":44900,"Experience":44901,"ĠPlaystation":44902,"ĠMIA":44903,"ĠUng":44904,"cloth":44905,"agall":44906,"Ġcalming":44907,"izens":44908,"Struct":44909,"ĠWitches":44910,"ĠCelebration":44911,"Ġ..............":44912,"ptroller":44913,"ĠTCU":44914,"Ġbunny":44915,"ãĥį":44916,"utorial":44917,"Ġupscale":44918,"ĠSta":44919,"ĠColossus":44920,"Ġchloride":44921,"ĠZac":44922,"ĠReasons":44923,"ĠBrookings":44924,"ĠWHITE":44925,"][/":44926,"ĠLose":44927,"905":44928,"Ġunderside":44929,"ernels":44930,"Ġvape":44931,"dozen":44932,"uppet":44933,"ĠSTOP":44934,"matical":44935,"ĠStatements":44936,"heddar":44937,"PAC":44938,"Customer":44939,"Ġmemos":44940,"ĠPJ":44941,"endars":44942,"ĠLimits":44943,"laugh":44944,"Ġstabilized":44945,"ĠALEC":44946,"YA":44947,"Upgrade":44948,"alam":44949,"Ġtechno":44950,"Ġanew":44951,"foreseen":44952,"Ġcollegiate":44953,"ĠPyro":44954,"ĠDism":44955,"Ġfrontline":44956,"Ġammonia":44957,"IU":44958,"Quite":44959,"Johnny":44960,"assin":44961,"GOP":44962,"ĠStyles":44963,"ĠSovereign":44964,"acterial":44965,"549":44966,"ĠRIP":44967,"ĠLists":44968,"Ġ364":44969,"ĠRecep":44970,"socket":44971,"ĠByrd":44972,"ĠCandle":44973,"Ancient":44974,"Ġappellant":44975,"enforcement":44976,"acea":44977,"anski":44978,"Ġolds":44979,"886":44980,"Ġslurs":44981,"Ġempires":44982,"Ġbuckle":44983,"Ġalienation":44984,"ĠAberdeen":44985,"Ġunicorn":44986,"Ġoverriding":44987,"ĠLX":44988,"ppa":44989,"Ġdespised":44990,"ĠBugs":44991,"ĠBST":44992,"Southern":44993,"533":44994,"Ġhallmark":44995,"ĠPoster":44996,"Ġstemmed":44997,"Ġprincipals":44998,"ĠTECH":44999,"ĠSandwich":45000,"Italy":45001,"Ġcheesy":45002,"ĠSetTextColor":45003,"ĠProtective":45004,"ĠCohn":45005,"JO":45006,"aptop":45007,"Reason":45008,"Leader":45009,"ĠUnderstand":45010,"ĠFridays":45011,"ĠContinuous":45012,"Ġclipping":45013,"ĠRye":45014,"Ġberth":45015,"timer":45016,"annis":45017,"react":45018,"Ġbuffalo":45019,"ĠParas":45020,"Ġ655":45021,"Ġpresided":45022,"ĠSunrise":45023,"Ġvets":45024,"Ġcloves":45025,"ĠMcCull":45026,"Strength":45027,"GAN":45028,"Ġilliter":45029,"ĠPricing":45030,"lé":45031,"Ġresistor":45032,"Ġbrun":45033,"ĠSuffolk":45034,"Ñĭ":45035,"ĠLiver":45036,"Released":45037,"Ġwhats":45038,"860":45039,"ĠMeasures":45040,"Ġdenouncing":45041,"ĠRyzen":45042,"Ġsouven":45043,"Ġcaregivers":45044,"chini":45045,"ĠScarlett":45046,"Ġtrough":45047,"Congratulations":45048,"Ġtaxis":45049,"ĠTradition":45050,"jit":45051,"Ġtabletop":45052,"Ġhitherto":45053,"Ġdisinformation":45054,"offensive":45055,"hra":45056,"ĠDISTRICT":45057,"Ġcomplicate":45058,"chenko":45059,"ĠReconstruction":45060,"Ġpalpable":45061,"Ġausp":45062,"Ġ428":45063,"Ġshowcases":45064,"ĠPublication":45065,"knowledge":45066,"innon":45067,"419":45068,"Ġretrieval":45069,"anders":45070,"Ġrefute":45071,"Ġinquired":45072,"gur":45073,"Ġnegativity":45074,"Ġconserve":45075,"Ġafterlife":45076,"Ġpresupp":45077,"ĠGillespie":45078,"Ġmt":45079,"ĠDN":45080,"Tap":45081,"Ġperpend":45082,"ĠSmy":45083,"doesn":45084,"Ġspilling":45085,"Ġhypers":45086,"Kate":45087,"®,":45088,"kept":45089,"ĠPowered":45090,"Ġja":45091,"ĠKlux":45092,"arde":45093,"aban":45094,"Ġ444":45095,"Ġflattened":45096,"ĠImprovements":45097,"urga":45098,"ĠKund":45099,"Ġinscribed":45100,"Ġfacult":45101,"Ġunprepared":45102,"ĠConsumers":45103,"Ġsatisfies":45104,"Ġpulmonary":45105,"Ġinfiltration":45106,"Ġexternally":45107,"Ġcongratulations":45108,"aghan":45109,"Ġairliner":45110,"Ġflung":45111,"Ġflyers":45112,"GD":45113,"Ġsnippets":45114,"Ġrecursive":45115,"Ġmastering":45116,"Lex":45117,"Ġovertly":45118,"vg":45119,"Ġluckily":45120,"Ġencro":45121,"ĠLancet":45122,"ĠAbyssal":45123,"functional":45124,"Ġsow":45125,"Ġsquid":45126,"Ġnarration":45127,"Ġnaughty":45128,"ĠHonour":45129,"ĠSpartans":45130,"Ġshatter":45131,"ĠTacoma":45132,"ĠCalories":45133,"ĠRaces":45134,"Submit":45135,"Ġpurposefully":45136,"wav":45137,"ĠYok":45138,"Fest":45139,"ĠGerr":45140,"Metro":45141,"Ġitiner":45142,"famous":45143,"Ġ\"{":45144,"inline":45145,"washer":45146,"Issue":45147,"ĠCLIENT":45148,"ozo":45149,"Versions":45150,"725":45151,"ĠGlock":45152,"Ġshielded":45153,"ĠPCR":45154,"ENCY":45155,"ĠWeld":45156,"ĠSimpl":45157,"Ġredirected":45158,"ĠKham":45159,"Ġ(>":45160,"Ġlabou":45161,"Ġdiapers":45162,"ssl":45163,"Ġcellar":45164,"organisms":45165,"oresc":45166,"ĠBerks":45167,"didn":45168,"Shipping":45169,"Chest":45170,"Ġundone":45171,"Ġmillionaire":45172,"Ġcords":45173,"ĠYounger":45174,"appropriately":45175,"Ġsequels":45176,"uve":45177,"anticipated":45178,"Ġlewd":45179,"ĠShirt":45180,"ĠDmitry":45181,"Veter":45182,"Ġslaying":45183,"ĠYar":45184,"Ġcomplication":45185,"Iowa":45186,"ĠErica":45187,"ĠBLM":45188,"girlfriend":45189,"bodied":45190,"626":45191,"1963":45192,"Ġintermediary":45193,"Ġconsolation":45194,"Mask":45195,"ĠSiem":45196,"owan":45197,"Beginning":45198,"Ġfixme":45199,"Ġculminated":45200,"Ġconduc":45201,"ĠVolunteer":45202,"Ġpositional":45203,"Ġgreets":45204,"ĠDefinitions":45205,"Ġthinker":45206,"Ġingenuity":45207,"Ġfreshmen":45208,"ĠMoments":45209,"Ġ357":45210,"ateurs":45211,"ĠFedEx":45212,"sg":45213,"694":45214,"Ġdwindling":45215,"ĠBOX":45216,"selage":45217,"Ġtmp":45218,"Ġsten":45219,"ĠSut":45220,"Ġneighbourhoods":45221,"Ġclassmate":45222,"fledged":45223,"Ġleftists":45224,"Ġclimates":45225,"ATHER":45226,"ĠScythe":45227,"uliffe":45228,"Ġsag":45229,"Ġhopped":45230,"ĠFt":45231,"ĠEck":45232,"ĠCK":45233,"ĠDoomsday":45234,"kids":45235,"Ġgasped":45236,"Ġmoniker":45237,"ĠLod":45238,"ĠCFL":45239,"tions":45240,"rums":45241,"folios":45242,"Ġmd":45243,"Ġuncanny":45244,"Ġtransports":45245,"ĠLabrador":45246,"Ġrailways":45247,"Ġappliance":45248,"ĠCTRL":45249,"æĢ":45250,"Population":45251,"ĠConfederacy":45252,"Ġunbearable":45253,"Ġdorsal":45254,"ĠInform":45255,"opted":45256,"ĠKILL":45257,"Marx":45258,"Ġhypocritical":45259,"qus":45260,"ĠNumerous":45261,"ĠGeorgian":45262,"ĠAmbrose":45263,"ĠLoch":45264,"Ġgubernatorial":45265,"ĠXeon":45266,"ĠSupports":45267,"enser":45268,"eely":45269,"ĠAvenger":45270,"1965":45271,"Army":45272,"Ġjuxtap":45273,"Ġchopping":45274,"ĠSplash":45275,"ĠSustainable":45276,"ĠFinch":45277,"Ġ1861":45278,"ictive":45279,"atmeal":45280,"ĠGohan":45281,"Ġlightsaber":45282,"ĠGPA":45283,"ugu":45284,"ĠREPL":45285,"variable":45286,"Ġherpes":45287,"Ġdeserts":45288,"aciously":45289,"Ġsituational":45290,"weekly":45291,"obl":45292,"Ġtextile":45293,"ĠCornwall":45294,"Ġcontraceptives":45295,"ĠAke":45296,"]-":45297,"ä¹ĭ":45298,":,":45299,"ĠWem":45300,"ĠBihar":45301,"Ġ'.":45302,"Ġbere":45303,"Ġanalogue":45304,"ĠCookies":45305,"Ġtakeoff":45306,"Wheel":45307,"Ġmajestic":45308,"Ġcommuting":45309,"023":45310,"ĠCorpse":45311,"assment":45312,"mini":45313,"Ġgorilla":45314,"ĠAlas":45315,"eree":45316,"Ġacquaintances":45317,"ĠAdvantage":45318,"Ġspiritually":45319,"Ġeyed":45320,"pmwiki":45321,"ĠEnder":45322,"Ġtranslucent":45323,"Ġnighttime":45324,"ĠIMAGES":45325,"545":45326,"ĠKamp":45327,"ĠFreak":45328,"Ġig":45329,"Portland":45330,"432":45331,"ĠMata":45332,"Ġmarines":45333,"Ġhors":45334,"aterasu":45335,"ĠAttribution":45336,"Ġ---------":45337,"Ġkins":45338,"ĠBELOW":45339,"+++":45340,"Ġreeling":45341,"oled":45342,"Ġclutter":45343,"ĠRelative":45344,"Ġ427":45345,"BUS":45346,"Ġavert":45347,"ĠCheong":45348,"ĠAble":45349,"ĠPryor":45350,"Developer":45351,"Ġencyclopedia":45352,"ĠUSAF":45353,"ĠGarry":45354,"Spain":45355,"Blocks":45356,"Ġexposition":45357,"ĠGamerGate":45358,"WOR":45359,"Ġstockpile":45360,"Ġclothed":45361,"ĠTone":45362,"ĠRue":45363,"tumblr":45364,"Ġtreacherous":45365,"Ġfrying":45366,"ÑĮ":45367,"ĠSph":45368,"Ġrestraints":45369,"Ġembodies":45370,"ĠGes":45371,"Safety":45372,"Ġnegotiators":45373,"mining":45374,"ĠAppalachian":45375,"LOS":45376,"ĠJenna":45377,"Ġpassers":45378,"çĭ":45379,"snap":45380,"Ġshorten":45381,"creator":45382,"Ġinnumerable":45383,"utherland":45384,"674":45385,"ĠWOM":45386,"ĠAscend":45387,"ĠArmory":45388,"ĠTransaction":45389,"Kick":45390,"Ġsuitcase":45391,"dayName":45392,"Ġwasteful":45393,"marriage":45394,"ĠMcCabe":45395,"itech":45396,"ĠOss":45397,"Closure":45398,"ĠTreasurer":45399,"Ġindecent":45400,"ĠDull":45401,"Ġresidences":45402,"1959":45403,"ĠSettlement":45404,"Hamilton":45405,"Ġselfies":45406,"ĠRanking":45407,"ĠBarkley":45408,"ĠBore":45409,"ĠWCS":45410,"ĠMaritime":45411,"ĠHuh":45412,"ĠForestry":45413,"Ġcultivating":45414,"ĠBallard":45415,"Ġgarrison":45416,"ĠSDL":45417,"930":45418,"Ġnascent":45419,"Ġirresistible":45420,"Ġawfully":45421,"\\/\\/":45422,"Ġequate":45423,"Ġanthropology":45424,"ĠSylvia":45425,"Ġintestine":45426,"Ġinnocuous":45427,"cessive":45428,"agra":45429,"ĠMetroid":45430,"Grant":45431,"855":45432,"ģĸ":45433,"Ġ\"_":45434,"ãĥĥãĥī":45435,"Ġappraisal":45436,"ĠFreddy":45437,"046":45438,"Ġ406":45439,"Ġ1830":45440,"Ġdocking":45441,"Static":45442,"Ġpont":45443,"ĠVoltage":45444,"ĠStead":45445,"ĠMortgage":45446,"ĠJonah":45447,"YL":45448,"CLASSIFIED":45449,"Ġasbestos":45450,"nikov":45451,"Ġcollagen":45452,"ĠOrbital":45453,"Pocket":45454,"799":45455,"Ġhybrids":45456,"inches":45457,"Ġinvoice":45458,"undy":45459,"Ġinequalities":45460,"Trend":45461,"washed":45462,"BALL":45463,"Ġlucid":45464,"ĠCommentary":45465,"Ġwitty":45466,"Brandon":45467,"Ġbruising":45468,"Ġ620":45469,"escent":45470,"boxing":45471,"POL":45472,"Ġ378":45473,"Rect":45474,"Ġlicences":45475,"ĠMcGee":45476,"pressed":45477,"Danny":45478,"Ġjammed":45479,"ordinate":45480,"Ġleth":45481,"Ġdistinguishes":45482,"ĠYamaha":45483,"ILS":45484,"ĠHume":45485,"ĠCategories":45486,"Roberts":45487,"Chart":45488,"Ġbeetle":45489,"ĠGraveyard":45490,"Ġ($)":45491,"oÄŁ":45492,"Ġtwilight":45493,"arella":45494,"á½":45495,"Ġbooths":45496,"ĠHHS":45497,"ĠFeldman":45498,"Ġexcavation":45499,"Ġphilosophies":45500,"atography":45501,"ĠGarage":45502,"technology":45503,"Ġunforgettable":45504,"Ġverifying":45505,"Ġsubordinates":45506,"Els":45507,"Ġneb":45508,"Gaming":45509,"ENA":45510,"ĠAchievement":45511,"itters":45512,"ĠGabe":45513,"Ġdumps":45514,"forcer":45515,"Ġpoignant":45516,"ĠMBA":45517,"ĠHeidi":45518,"imei":45519,"Ġmages":45520,"Ġliberate":45521,"Ġcircumcised":45522,"ĠMermaid":45523,"ĠMatth":45524,"together":45525,"ĠWichita":45526,"Ġstorefront":45527,"ĠAdin":45528,"VII":45529,"Fourth":45530,"Ġexplorers":45531,"WER":45532,"Notable":45533,"Brook":45534,"mens":45535,"Faith":45536,"---------":45537,"ĠJou":45538,"¬¼":45539,"Ġpineapple":45540,"Ġamalg":45541,"eln":45542,"arkable":45543,"ĠãĤµãĥ¼ãĥĨãĤ£":45544,"ĠãĤµãĥ¼ãĥĨãĤ£ãĥ¯ãĥ³":45545,"Ġovarian":45546,"ĠEchoes":45547,"Ġhaircut":45548,"Ġpav":45549,"Ġchilled":45550,"anasia":45551,"Ġstyled":45552,"Ġdab":45553,"niper":45554,"Ġministerial":45555,"ĠDUP":45556,"Tan":45557,"Ġsulph":45558,"ĠDeter":45559,"ĠBohem":45560,"odan":45561,"Ġeducator":45562,"âĵĺ":45563,"spir":45564,"Chicken":45565,"ĠEleanor":45566,"Ġqui":45567,"Ġheaviest":45568,"Ġgrasped":45569,"URA":45570,"Ġcrooked":45571,"Jessica":45572,"problem":45573,"Ġpredetermined":45574,"Ġmaniac":45575,"Ġbreaths":45576,"ĠLauderdale":45577,"Ġhobbies":45578,"yz":45579,"Crime":45580,"Ġcharisma":45581,"dL":45582,"Ġleaping":45583,"Ġkittens":45584,"Angelo":45585,"ĠJACK":45586,"ĠSuzanne":45587,"Ġhalting":45588,"ENTION":45589,"Ġswallowing":45590,"ĠEarthquake":45591,"Ġeighteenth":45592,"ĠNIC":45593,"ĠINF":45594,"ĠConscious":45595,"Ġparticulars":45596,"circle":45597,"740":45598,"Ġbenevolent":45599,"Ġ747":45600,"Ġ490":45601,"Ġrundown":45602,"ĠValerie":45603,"ĠBUR":45604,"Ġcivilisation":45605,"ĠSchn":45606,"WB":45607,"otide":45608,"international":45609,"Ġjohn":45610,"Ġ1902":45611,"Ġpeanuts":45612,"Ġflavored":45613,"kus":45614,"Ġroared":45615,"Ġcutoff":45616,"é£":45617,"Ġornament":45618,"Ġarchitectures":45619,"Ġ369":45620,"olor":45621,"ĠWilde":45622,"ĠCRC":45623,"ĠAdjusted":45624,"Ġprovoking":45625,"landish":45626,"Ġrationality":45627,"Ġjustifies":45628,"Ġdispel":45629,"Ġameric":45630,"ĠPoles":45631,"Ø©":45632,"Ġenvis":45633,"ĠDoodle":45634,"使":45635,"igsaw":45636,"auldron":45637,"Technical":45638,"Teen":45639,"uphem":45640,"ĠXiang":45641,"Ġdetractors":45642,"ĠZi":45643,"ĠJournalists":45644,"Ġconducive":45645,"ĠVolunteers":45646,"Ġsd":45647,"Knowing":45648,"Ġtransmissions":45649,"ĠPLAN":45650,"ĠLIB":45651,"Ġalluded":45652,"Ġobe":45653,"Ġdope":45654,"ĠGoldstein":45655,"Ġwavelengths":45656,"ĠDestination":45657,"nda":45658,"ugi":45659,"Ġattentive":45660,"ĠLean":45661,"raltar":45662,"Ġmang":45663,"mbuds":45664,"akings":45665,"bender":45666,"Ġaccol":45667,"Ġcrawled":45668,"NOW":45669,"Minnesota":45670,"Ġflourished":45671,"ĠZup":45672,"ĠSupervisor":45673,"ĠOlivier":45674,"Excellent":45675,"Ġwiden":45676,"Done":45677,"Ġwig":45678,"Ġmisconceptions":45679,"Corp":45680,"Wan":45681,"Ġvenerable":45682,"ĠNotably":45683,"ĠKlingon":45684,"animate":45685,"Boost":45686,"ĠSAY":45687,"missing":45688,"ibliography":45689,"melon":45690,"Ġpayday":45691,"س":45692,"bole":45693,"Ġveiled":45694,"ĠAlphabet":45695,"Italian":45696,"Ġeverlasting":45697,"ĠRIS":45698,"ĠCree":45699,"rompt":45700,"Ġhating":45701,"Ġgrinning":45702,"Ġgeographically":45703,"OSH":45704,"Ġweeping":45705,"ĠÂłĠÂłĠÂłĠÂłĠÂłĠÂłĠÂłĠÂł":45706,"Ġimpecc":45707,"Letter":45708,"Ġbloated":45709,"PLA":45710,"ĠFein":45711,"Ġpersever":45712,"Thunder":45713,"Ġaur":45714,"ĠRL":45715,"Ġpitfalls":45716,"âĸº":45717,"Ġpredominant":45718,"Ġ525":45719,"718":45720,"APE":45721,"714":45722,"Ġfarmland":45723,"ĠQiao":45724,"Ġviolet":45725,"ĠBahamas":45726,"Ġinflicting":45727,"ĠEfficiency":45728,"Ġhomebrew":45729,"Ġundertook":45730,"Ġcurly":45731,"ĠHarding":45732,"mania":45733,"596":45734,"Ġtempered":45735,"Ġharrowing":45736,"ĠPledge":45737,"ĠFrankenstein":45738,"èª":45739,"Motion":45740,"Ġpredictably":45741,"ĠExplosion":45742,"ocusing":45743,"erd":45744,"colo":45745,"FFER":45746,"Ġbackfield":45747,"ĠVIDE":45748,"uebl":45749,"Narr":45750,"ĠArgument":45751,"Ġgenomic":45752,"Ġboutique":45753,"Ġbatted":45754,"ĠBinary":45755,"Ġgamb":45756,"ĠRhythm":45757,"673":45758,"Ġafloat":45759,"ĠOlympia":45760,"YING":45761,"Ġendif":45762,"isin":45763,"Ġwinters":45764,"Ġscattering":45765,"Iv":45766,"Distance":45767,"Ġtru":45768,"ĠComfort":45769,"Ġnexus":45770,"Ġairflow":45771,"ĠByzantine":45772,"payers":45773,"coni":45774,"ĠBetsy":45775,"Deal":45776,"ĠNug":45777,"ĠContinent":45778,"redibly":45779,"Ġoptimizing":45780,"albeit":45781,"Ġecstatic":45782,"ĠProto":45783,"ç·":45784,"ivot":45785,"âĸĦ":45786,"emp":45787,"rounder":45788,"Ġclout":45789,"ĠIST":45790,"663":45791,"ĠDollars":45792,"ĠDAC":45793,"Ġsubscribed":45794,"Ġrehearsal":45795,"Ġamps":45796,"ĠShang":45797,"esm":45798,"Ġsprinkle":45799,"Ġassailant":45800,"ĠOo":45801,"ĠCoinbase":45802,"Tact":45803,"Ġretina":45804,"Ġnuns":45805,"RON":45806,"atto":45807,"Ġjug":45808,"ĠSVG":45809,"Ġbikini":45810,"ĠFILE":45811,"ĠFounders":45812,"eport":45813,"ĠKP":45814,"Ġrestores":45815,"ĠThick":45816,"Ġashore":45817,"Ġapprovals":45818,"Render":45819,"MAG":45820,"Graham":45821,"ĠCortana":45822,"ãĥ³ãĤ¸":45823,"ssh":45824,"orians":45825,"arsity":45826,"ĠInspired":45827,"upper":45828,"Ġsignalling":45829,"Ġrebuke":45830,"Ġflares":45831,"Ġdowntime":45832,"Studies":45833,"Ġstagnation":45834,"ĠSequence":45835,"Ġgrunt":45836,"Ġassures":45837,"ĠPLA":45838,"592":45839,"Ġintraven":45840,"depend":45841,"Susan":45842,"ĠManziel":45843,"Mania":45844,"Contract":45845,"Ġslams":45846,"Ġcultured":45847,"Ġcreditor":45848,"LIST":45849,"ĠHUM":45850,"ĠChattanooga":45851,"served":45852,"Ġcloaked":45853,"ĠFTP":45854,"powder":45855,"ĠStella":45856,"uctive":45857,"Ġcheaply":45858,"ĠMUCH":45859,"ĠGalileo":45860,"Ġsuites":45861,"speech":45862,"Ġdeliberations":45863,"ĠChips":45864,"«ĺ":45865,"Balance":45866,"ĠWynne":45867,"ĠAkron":45868,"Asset":45869,"Ġhonoured":45870,"Ġedged":45871,"Likewise":45872,"animous":45873,"ĠWage":45874,"ĠEzek":45875,"advertisement":45876,"ĠRTX":45877,"ĠMAD":45878,"Ġmigrating":45879,"ĠSQU":45880,"Ġ475":45881,"Edited":45882,"Ġshorthand":45883,"ĠBasics":45884,"Ġcrotch":45885,"ĠEVEN":45886,"Ġvm":45887,"efficiency":45888,"Ġcalves":45889,"ĠFrie":45890,"ĠBrilliant":45891,"Ġstrikers":45892,"Ġrepentance":45893,"Ġarteries":45894,"rl":45895,"Bed":45896,"hap":45897,"Ġcryptography":45898,"ĠSabres":45899,"Ġ414":45900,"viks":45901,"ihara":45902,"apses":45903,"Talking":45904,"Ġintertwined":45905,"Ġdocks":45906,"Ġallele":45907,"ĠArtifact":45908,"ĠHIM":45909,"torn":45910,"çķ":45911,"Ġopacity":45912,"ĠEly":45913,"osuke":45914,"Ġnipple":45915,"Ġhandwritten":45916,"ĠVK":45917,"ĠChamberlain":45918,"ĠLaos":45919,"igraph":45920,"grow":45921,"Ġtrillions":45922,"Ġdescendant":45923,"ĠSailor":45924,"asuring":45925,"Ġceilings":45926,"ĠWarehouse":45927,"flying":45928,"ĠGlow":45929,"Ġnont":45930,"Ġmiscarriage":45931,"Ġrigs":45932,"Ġministries":45933,"Ġelaborated":45934,"Ġdelusional":45935,"ĠHumane":45936,"Ġ379":45937,"nets":45938,"Ġblackout":45939,"adders":45940,"Ġnp":45941,"ĠTire":45942,"rosc":45943,"Ġsubdiv":45944,"Ġlinkage":45945,"Ġchronological":45946,"ĠHERO":45947,"Ġresettlement":45948,"ĠVinyl":45949,"Ġpastoral":45950,"ĠMobil":45951,"ĠBarbar":45952,"Cooldown":45953,"ĠFritz":45954,"criminal":45955,"repe":45956,"Ġbellig":45957,"ĠBreed":45958,"Ġ418":45959,"Ġsemblance":45960,"ijk":45961,"Ġcurtail":45962,"Ġclinch":45963,"contained":45964,"ĠPrompt":45965,"aston":45966,"Ġwi":45967,"Ġpursuits":45968,"515":45969,"ĠGloss":45970,"Ġflips":45971,"Ġcoupons":45972,"Ġcloning":45973,"ĠLikely":45974,"Removed":45975,"ĠQuartz":45976,"rices":45977,"ĠSpears":45978,"Ġpious":45979,"Ġdepreciation":45980,"ĠDare":45981,"ounces":45982,"amaz":45983,"Ont":45984,"Ġpinnacle":45985,"docker":45986,"026":45987,"ĠWyr":45988,"ĠProper":45989,"ËĪ":45990,"nil":45991,"Bytes":45992,"Ġseeker":45993,"trial":45994,"Ġunfolds":45995,"ĠMarse":45996,"Ġextravagant":45997,"ĠSurvivors":45998,"REDACTED":45999,"ĠSpeedway":46000,"ĠCraigslist":46001,"submit":46002,"ĠGenerations":46003,"Ġupholding":46004,"Ġbloodstream":46005,"ĠMissions":46006,"ĠLawn":46007,"Ġlimbo":46008,"enei":46009,"Huh":46010,"ĠWildcats":46011,"prep":46012,"ĠMarkus":46013,"ĠForbidden":46014,"ritic":46015,"INO":46016,"Ġexhibiting":46017,"requent":46018,"chuk":46019,"Ġhabitual":46020,"ĠCompatibility":46021,"Drag":46022,"RIPT":46023,"ujah":46024,"GROUND":46025,"Ġdelinquent":46026,"Ġburner":46027,"Ġcontemporaries":46028,"Ġgimmick":46029,"loads":46030,"Ġnozzle":46031,"podcast":46032,"ĠWak":46033,"ĠStaten":46034,"ĠKuh":46035,"ãģĵ":46036,"interrupted":46037,"Ġinvincible":46038,"ĠBurnett":46039,"cigarette":46040,"ĠPebble":46041,"ĠTemporary":46042,"ĠMarino":46043,"582":46044,"Ġwasteland":46045,"idently":46046,"Tx":46047,"Ġrite":46048,"ĠPanasonic":46049,"ĠMiddles":46050,"ĠHorton":46051,"aeus":46052,"Ġcuring":46053,"Ġmats":46054,"Ġadjourn":46055,"Ġfearsome":46056,"pez":46057,"boats":46058,"Ġpropell":46059,"Ġconflicted":46060,"ĠAnger":46061,"Ġinsurgent":46062,"Karl":46063,"Ġcoales":46064,"Ġsouthwestern":46065,"Ġdissu":46066,"ĠOvert":46067,"************":46068,"Ġboxed":46069,"ĠBrune":46070,"aaa":46071,"Ġgardening":46072,"ĠEngel":46073,"tracks":46074,"Ġpurified":46075,"Ġplaceholder":46076,"ĠLikes":46077,"Ġdan":46078,"Gab":46079,"Ġect":46080,"ĠFaw":46081,"ĠEliot":46082,"Ġ',":46083,"otropic":46084,"ĠRuin":46085,"hedon":46086,"Ġcaul":46087,"Ġaft":46088,"ĠCadillac":46089,"gha":46090,"assian":46091,"udeb":46092,"ĠTick":46093,"Ġadjusts":46094,"ARGET":46095,"537":46096,"ische":46097,"anty":46098,"ĠFriedrich":46099,"ĠBlizz":46100,"ĠAOL":46101,"Campaign":46102,"Ġmammal":46103,"ĠVeil":46104,"ĠKev":46105,"ĠMaurit":46106,"ĠDamien":46107,"Nation":46108,"Eastern":46109,"Ġ{:":46110,"Ġ=================================":46111,"Ġstereotypical":46112,"Ġattic":46113,"ĠCyborg":46114,"require":46115,"Ġawarding":46116,"ĠPapua":46117,"btn":46118,"bent":46119,"Boo":46120,"Ġ(=":46121,"ĠXander":46122,"ĠSomerset":46123,"Ġcatchy":46124,"Ġcertify":46125,"STRUCT":46126,"Ġital":46127,"Ġtides":46128,"ĠBrands":46129,"Gray":46130,"competitive":46131,"Ġcurator":46132,"ĠDG":46133,"ominium":46134,"ĠGMOs":46135,"ciating":46136,"ĠCarmen":46137,"oward":46138,"Baltimore":46139,"Ġrgb":46140,"Cu":46141,"Ġwipes":46142,"spell":46143,"ITNESS":46144,"Ġsummarizes":46145,"ĠRevis":46146,"Ġwhistleblowers":46147,"ĠBreach":46148,"Ġcrochet":46149,"kos":46150,"ewski":46151,"Ġrepet":46152,"Ġcrimson":46153,"ĠKarachi":46154,"readable":46155,"dimension":46156,"ĠIgor":46157,"ilded":46158,"ĠZed":46159,"ĠKeane":46160,"ĠCosmetic":46161,"DEP":46162,"Ġretreating":46163,"ĠUA":46164,"ensical":46165,"Ġdusk":46166,"ĠDickens":46167,"Ġarenas":46168,"ĠPassage":46169,"levels":46170,"Ġcurv":46171,"Pope":46172,"Ġchores":46173,"ĠElise":46174,"ĠCompass":46175,"bub":46176,"Ġmammalian":46177,"ĠSanskrit":46178,"ĠANC":46179,"ĠCrack":46180,"Qual":46181,"Laun":46182,"ampunk":46183,"Ġlearners":46184,"Ġglamorous":46185,"Ġfurthe":46186,"ermott":46187,"cand":46188,"Generic":46189,"Ġnarrated":46190,"Ġdisorderly":46191,"ĠTransactions":46192,"ĠDetention":46193,"ĠRoku":46194,"Äį":46195,"Ġunderstatement":46196,"ĠSaur":46197,"ĠRodrigo":46198,"ĠASAP":46199,"Sin":46200,"Ġrejoice":46201,"Methods":46202,"Ġelectrode":46203,"Ġworshipped":46204,"Ġidi":46205,"ĠPhysicians":46206,"Ġpopup":46207,"Ġdeft":46208,"ĠRemoval":46209,"ĠBuenos":46210,"verbs":46211,"Ġfunk":46212,"usha":46213,"riction":46214,"orea":46215,"ĠBangalore":46216,"ĠKenobi":46217,"zzi":46218,"Ġnormative":46219,"Ġgoblins":46220,"Ġcafes":46221,"ĠUNCLASSIFIED":46222,"ĠFired":46223,"SIGN":46224,"Ġsclerosis":46225,"ĠVoter":46226,"ĠSonny":46227,"ĠExtend":46228,"ĠEVs":46229,"Arsenal":46230,"Ġpsi":46231,"Ġwidest":46232,"ĠTus":46233,"Ġlooms":46234,"Ġjustifying":46235,"ĠGranger":46236,"è¯":46237,"Refer":46238,"583":46239,"Ġflourishing":46240,"abre":46241,"Ġrave":46242,"ĠContra":46243,"Ġ1898":46244,"Adds":46245,"Ġful":46246,"ĠCooke":46247,"someone":46248,"=#":46249,"671":46250,"Ġyak":46251,"Ġarte":46252,"ĠMiscellaneous":46253,"ĠDetection":46254,"ĠClancy":46255,"âģ":46256,"assies":46257,"Ġvaliant":46258,"ĠFeminist":46259,"corruption":46260,"Vel":46261,"Pear":46262,"Ġsuccinct":46263,"Ġquickest":46264,"kw":46265,"Ġspitting":46266,"ĠLibraries":46267,"åħī":46268,"antz":46269,"Dad":46270,"ĠSpecifications":46271,"rupulous":46272,"andr":46273,"RESULTS":46274,"Ġsnowball":46275,"Ġpredis":46276,"ĠBaxter":46277,"ĠNursing":46278,"ĠChaff":46279,"swe":46280,"Ġoutage":46281,"Ġnesting":46282,"Ġnotoriety":46283,"trigger":46284,"onite":46285,"jon":46286,"Ġfou":46287,"ooked":46288,"ĠCelebrity":46289,"reality":46290,"Ġfatig":46291,"Ġhugging":46292,"Ġbothers":46293,"ĠPanzer":46294,"ĠChandra":46295,"figured":46296,"Ġvolts":46297,"ĠClouds":46298,"Ġfeeble":46299,"ĠCurve":46300,"ĠAsus":46301,"786":46302,"absor":46303,"ĠVICE":46304,"ĠHess":46305,"Ġmanufactures":46306,"Ġgrizz":46307,"ĠPowerful":46308,"acid":46309,"Ġsubsections":46310,"ĠKrugman":46311,"ĠAlps":46312,"isu":46313,"Ġsequest":46314,"ĠUltron":46315,"ĠTinker":46316,"ĠGoose":46317,"Ġmismatch":46318,"Attorney":46319,"Ġmorphology":46320,"ĠSixers":46321,"uttered":46322,"ĠELECT":46323,"gran":46324,"Russell":46325,"ĠGSL":46326,"Ġfortnight":46327,"Ġ.)":46328,"Ġapostle":46329,"prone":46330,"elist":46331,"Untitled":46332,"ĠImplementation":46333,"istors":46334,"Ġtanker":46335,"Ġplush":46336,"Ġattendants":46337,"ĠTik":46338,"ĠGreenwich":46339,"ĠYon":46340,"ĠSPL":46341,"cells":46342,"untled":46343,"Solution":46344,"ĠQué":46345,"Ġvacated":46346,"Ġuptick":46347,"ĠMeridian":46348,"æĥ":46349,"ĠDrill":46350,"925":46351,"584":46352,"Ġrenovated":46353,"ĠKubrick":46354,"zyk":46355,"Ġlousy":46356,"ppel":46357,"ohydrate":46358,"ĠIzzy":46359,"lesiastical":46360,"CCC":46361,"ĠAjax":46362,"Ġadapters":46363,"ĠPetraeus":46364,"Ġaffirmation":46365,"ĠSTOR":46366,"lems":46367,"adoes":46368,"ĠConstantinople":46369,"Ġponies":46370,"Ġlighthouse":46371,"Ġadherents":46372,"ĠBrees":46373,"omorphic":46374,"Fighting":46375,"Ġplaster":46376,"ĠPVC":46377,"ĠObst":46378,"Ġdearly":46379,"ĠTooth":46380,"ickson":46381,"Ġshaming":46382,"Plex":46383,"Agg":46384,"Ġâ̦\"":46385,"Ġsubreddits":46386,"Ġpigeon":46387,"ĠResidential":46388,"ĠPassing":46389,"Ġlum":46390,"ĠPension":46391,"Ġpessimistic":46392,"Ġ432":46393,"zinski":46394,"cade":46395,"075":46396,"Ġapologised":46397,"iyah":46398,"Putting":46399,"Ġgloomy":46400,"ĠLyme":46401,"=-=-=-=-=-=-=-=-":46402,"ĠTome":46403,"ĠPsychiatric":46404,"ĠHIT":46405,"cms":46406,"apolog":46407,"Ġbreaker":46408,"Ġdeepen":46409,"Ġtheorist":46410,"ĠHighlands":46411,"Ġbaker":46412,"Ġstaples":46413,"Ġinterfered":46414,"ĠAbortion":46415,"joined":46416,"chu":46417,"Ġformulate":46418,"Ġvaccinations":46419,"Ġbanter":46420,"pheus":46421,"Ġoutfielder":46422,"ĠMeter":46423,"Ġ#####":46424,"Ġ1895":46425,"Ġnarrowing":46426,"ĠSTORY":46427,"fp":46428,"ĠCST":46429,"ignore":46430,"Ġproclaiming":46431,"ĠRU":46432,"ĠBALL":46433,"yna":46434,"653":46435,"Ġposit":46436,"PRE":46437,"594":46438,"ĠRegistrar":46439,"ĠPilgrim":46440,"icio":46441,"Ġprett":46442,"Ġlifeless":46443,"Ġ___":46444,"Neigh":46445,"ĠChurches":46446,"orno":46447,"Ġorcs":46448,"Ġkindred":46449,"ĠAudit":46450,"Ġmillennial":46451,"ĠPersia":46452,"gravity":46453,"ĠDisability":46454,"ĠDARK":46455,"Ws":46456,"odon":46457,"Ġgranddaughter":46458,"ĠBrooke":46459,"ĠADA":46460,"ERA":46461,"Ġpickups":46462,"ĠWilkinson":46463,"ĠShards":46464,"ĠNK":46465,"Ġexpel":46466,"ĠKislyak":46467,"Ġjargon":46468,"Ġpolarized":46469,"iane":46470,"Publisher":46471,"Ġrebutt":46472,"Ġapprehension":46473,"ĠKessler":46474,"Ġprism":46475,"FUL":46476,"1964":46477,"ĠLoll":46478,"ä¿":46479,"lethal":46480,"ÅŁ":46481,"Ġghetto":46482,"Ġboulder":46483,"ĠSlowly":46484,"ĠOscars":46485,"ĠInstruction":46486,"ĠUltr":46487,"ĠMoe":46488,"Nich":46489,"ĠPATH":46490,"(*":46491,"ĠRELEASE":46492,"uning":46493,"rouse":46494,"eneg":46495,"Ġreimb":46496,"ĠDetected":46497,"DoS":46498,"Ġsterling":46499,"Ġaggregation":46500,"ĠLonely":46501,"ĠAttend":46502,"higher":46503,"Ġairstrike":46504,"kson":46505,"SELECT":46506,"Ġdeflation":46507,"ĠHerrera":46508,"Cole":46509,"ritch":46510,"Ġadvisable":46511,"Fax":46512,"Ġworkaround":46513,"Ġpid":46514,"mortem":46515,"ersen":46516,"Ġtypo":46517,"Ġalum":46518,"782":46519,"ĠJamal":46520,"scripts":46521,"Ġcaptives":46522,"ĠPresence":46523,"ĠLieberman":46524,"angelo":46525,"Ġalcoholism":46526,"assi":46527,"Ġrecite":46528,"Ġgaping":46529,"Ġbaskets":46530,"ĠGou":46531,"Browser":46532,"neau":46533,"Ġcorrective":46534,"unda":46535,"scoring":46536,"ĠXD":46537,"Ġfilament":46538,"Ġdeepening":46539,"ĠStainless":46540,"Integer":46541,"Ġbuggy":46542,"Ġtenancy":46543,"ĠMubarak":46544,"Ġtuple":46545,"ĠDroid":46546,"ĠSitting":46547,"Ġforfeit":46548,"ĠRasmussen":46549,"ixties":46550,"esi":46551,"ĠKimmel":46552,"Ġmeticulously":46553,"Ġapopt":46554,"ĠSeller":46555,"088":46556,"ecake":46557,"hematically":46558,"TN":46559,"Ġmindless":46560,"Ġdigs":46561,"ĠAccord":46562,"onsense":46563,"eming":46564,"brace":46565,"ĠeBook":46566,"ĠDistribut":46567,"ĠInvestments":46568,"wt":46569,"]),":46570,"behavior":46571,"563":46572,"Ġblinding":46573,"ĠProtesters":46574,"topia":46575,"Ġreborn":46576,"ĠKelvin":46577,"ĠDover":46578,"ĠDairy":46579,"ĠOuts":46580,"Ġ[/":46581,"ÏĢ":46582,"bp":46583,"ĠVanity":46584,"ĠRecap":46585,"ĠHOUSE":46586,"ĠFACE":46587,"Ġ422":46588,"692":46589,"ĠAntioch":46590,"cooked":46591,"Ġcollide":46592,"Ġapr":46593,"Ġsleeper":46594,"ĠJarvis":46595,"Ġalternatively":46596,"ĠLeaves":46597,"ĠMaw":46598,"Ġantiquity":46599,"ĠAdinida":46600,"Ġabuser":46601,"Pokémon":46602,"Ġassorted":46603,"ĠRevision":46604,"ĠPiano":46605,"ĠGideon":46606,"Ocean":46607,"Ġsalon":46608,"Ġbustling":46609,"ognitive":46610,"ĠRahman":46611,"Ġwaiter":46612,"Ġpresets":46613,"ĠOsh":46614,"ĠGHC":46615,"operator":46616,"Ġreptiles":46617,"Ġ413":46618,"ĠGarr":46619,"ĠChak":46620,"Ġhashes":46621,"Ġfailings":46622,"Ġfolklore":46623,"Ġabl":46624,"ĠCena":46625,"ĠMacArthur":46626,"ĠCOURT":46627,"Ġperiphery":46628,"appers":46629,"Ġreckoned":46630,"ĠInflu":46631,"ĠCET":46632,"Ġ372":46633,"ĠDefinitive":46634,"assault":46635,"421":46636,"Ġreservoirs":46637,"Ġdives":46638,"ĠCoil":46639,"DAQ":46640,"Ġvividly":46641,"ĠRJ":46642,"ĠBellev":46643,"Ġeclectic":46644,"ĠShowdown":46645,"ĠKM":46646,"iped":46647,"reetings":46648,"ĠAsuka":46649,"Liberal":46650,"ĠÏĦ":46651,"Ġbystanders":46652,"ĠGoodwin":46653,"ukong":46654,"Sit":46655,"ĠTrem":46656,"Ġcriminally":46657,"ĠCircus":46658,"chrome":46659,"887":46660,"Ġnanop":46661,"ĠObi":46662,"ĠLOW":46663,"ogh":46664,"ĠAuthors":46665,"obyl":46666,"Urban":46667,"Ġti":46668,"ĠWeir":46669,"trap":46670,"agy":46671,"Ġparentheses":46672,"Ġoutnumbered":46673,"Ġcounterproductive":46674,"ĠTobias":46675,"ubis":46676,"Parser":46677,"STAR":46678,"Ġsynaptic":46679,"ĠGears":46680,"Ġhiber":46681,"Ġdebunked":46682,"Ġexalted":46683,"awatts":46684,"HOU":46685,"Church":46686,"ĠPixie":46687,"ĠUri":46688,"ĠFormation":46689,"ĠPrediction":46690,"CEO":46691,"Ġthrott":46692,"ĠBritann":46693,"ĠMadagascar":46694,"ëĭ":46695,"Ġbillboards":46696,"ĠRPGs":46697,"ĠBees":46698,"completely":46699,"FIL":46700,"Ġdoesnt":46701,"ĠGreenberg":46702,"reys":46703,"Ġsling":46704,"Ġemptied":46705,"ĠPixar":46706,"ĠDharma":46707,"luck":46708,"inguished":46709,"Ġendot":46710,"Ġbabys":46711,"059":46712,"chest":46713,"rats":46714,"Ġridden":46715,"Ġbeetles":46716,"Ġilluminating":46717,"Ġfictitious":46718,"ĠProvincial":46719,"Ġ768":46720,"Ġshepherd":46721,"ĠRender":46722,"Ġ1896":46723,"Crew":46724,"Ġmolded":46725,"ĠXiaomi":46726,"ĠSpiral":46727,"Ġdelim":46728,"Ġorganising":46729,"Ġhoops":46730,"ĠBei":46731,"zhen":46732,"Ġfuckin":46733,"Ġdecad":46734,"Ġunbiased":46735,"ammy":46736,"swing":46737,"Ġsmuggled":46738,"Ġkios":46739,"ĠPERSON":46740,"ĠInquisitor":46741,"Ġsnowy":46742,"Ġscraping":46743,"ĠBurgess":46744,"Ptr":46745,"agame":46746,"RW":46747,"Ġdroid":46748,"ĠLys":46749,"ĠCassandra":46750,"Jacob":46751,"Ġ354":46752,"Ġpasture":46753,"Ġfranc":46754,"ĠScotch":46755,"ĠEnds":46756,"ĠIGF":46757,"definition":46758,"Ġhysterical":46759,"ĠBrowne":46760,"771":46761,"Ġmobilization":46762,"æķ":46763,"iqueness":46764,"Thor":46765,"Ġspearheaded":46766,"Ġembroiled":46767,"Ġconjecture":46768,"judicial":46769,"Choice":46770,"Ġpaperback":46771,"Pir":46772,"Ġrecovers":46773,"ĠSurge":46774,"ĠShogun":46775,"ĠPediatrics":46776,"ãģł":46777,"Ġsweeps":46778,"ĠLaboratories":46779,"ĠPacks":46780,"alus":46781,"addin":46782,"Ġheadlights":46783,"gra":46784,"Evidence":46785,"COLOR":46786,"Admin":46787,"Ĭ±":46788,"Ġconcoct":46789,"sufficient":46790,"Ġunmarked":46791,"Ġrichness":46792,"Ġdissertation":46793,"Ġseasoning":46794,"Ġgib":46795,"ĠMages":46796,"unctions":46797,"ĠNid":46798,"cheat":46799,"ĠTMZ":46800,"citizens":46801,"ĠCatholicism":46802,"nb":46803,"Ġdisembark":46804,"ĠPROGRAM":46805,"aques":46806,"Tyler":46807,"Org":46808,"ĠSlay":46809,"ĠNero":46810,"ĠTownsend":46811,"INTON":46812,"tele":46813,"Ġmesmer":46814,"901":46815,"Ġfireball":46816,"evidence":46817,"affiliated":46818,"ĠFrenchman":46819,"ĠAugusta":46820,"021":46821,"Ġsled":46822,"Ġreused":46823,"ĠImmunity":46824,"Ġwrestle":46825,"assembled":46826,"Maria":46827,"Ġgunshots":46828,"ĠBarbie":46829,"Ġcannabinoids":46830,"ĠToast":46831,"ĠKinder":46832,"IRD":46833,"Ġrejuven":46834,"Ġgore":46835,"Ġrupture":46836,"Ġbreaching":46837,"ĠCartoon":46838,"Ġ455":46839,"ĠPaleo":46840,"614":46841,"Ġspears":46842,"ĠAmes":46843,"abus":46844,"Madison":46845,"GROUP":46846,"Ġaborted":46847,"yah":46848,"Ġfelon":46849,"Ġcausation":46850,"Ġprepaid":46851,"Ġpitted":46852,"oplan":46853,"ĠShelley":46854,"ĠRusso":46855,"ĠPagan":46856,"Ġwillfully":46857,"ĠCanaver":46858,"undrum":46859,"ĠSalary":46860,"ĠArpaio":46861,"reader":46862,"ĠRational":46863,"ĠOverse":46864,"ĠCauses":46865,"Ġ*.":46866,"Ġwob":46867,"Keith":46868,"ĠConsent":46869,"manac":46870,"773":46871,"623":46872,"Ġfateful":46873,"etimes":46874,"Ġspirited":46875,"ĠDys":46876,"Ġhegemony":46877,"Ġboycot":46878,"ĠEnrique":46879,"emouth":46880,"Ġtimelines":46881,"ĠSahara":46882,"ĠRelax":46883,"ĠQuincy":46884,"ĠLessons":46885,"ĠEQU":46886,"SEA":46887,"NK":46888,"ĠCostco":46889,"Increase":46890,"Ġmotivating":46891,"ĠChong":46892,"amaru":46893,"ĠDivide":46894,"Ġpedigree":46895,"ĠTasmania":46896,"ĠPrelude":46897,"Las":46898,"940":46899,"574":46900,"Ġchau":46901,"ĠSpiegel":46902,"unic":46903,"-->":46904,"ĠPhilips":46905,"ĠKafka":46906,"Ġupheaval":46907,"Ġsentimental":46908,"Ġsax":46909,"ĠAkira":46910,"serial":46911,"Matrix":46912,"Ġelecting":46913,"Ġcommenter":46914,"ĠNebula":46915,"plets":46916,"ĠNadu":46917,"ĠAdren":46918,"Ġenshr":46919,"ĠRAND":46920,"financial":46921,"ĠClyde":46922,"utherford":46923,"Ġsignage":46924,"Ġdeline":46925,"Ġphosphate":46926,"roversial":46927,"fascist":46928,"ĠVall":46929,"ĠBethlehem":46930,"Ġfors":46931,"Ġenglish":46932,"Solid":46933,"Nature":46934,"Ġva":46935,"ĠGuests":46936,"Ġtantal":46937,"Ġautoimmune":46938,";;;;;;;;;;;;":46939,"ĠTotally":46940,"ĠOv":46941,"Ġdefences":46942,"ĠCoconut":46943,"Ġtranquil":46944,"Ġploy":46945,"Ġflavours":46946,"ĠFlask":46947,"ãĤ¨ãĥ«":46948,"ĠWeston":46949,"ĠVolvo":46950,"870":46951,"Ġmicrophones":46952,"verbal":46953,"RPG":46954,"Ġiii":46955,";}":46956,"028":46957,"Ġheadlined":46958,"Ġprimed":46959,"Ġhoard":46960,"ĠShad":46961,"ĠENTER":46962,"Ġtriangular":46963,"Ġcapit":46964,"lik":46965,"ĠAncients":46966,"Ġlash":46967,"Ġconvol":46968,"Ġcolonel":46969,"enemy":46970,"Gra":46971,"Ġpubs":46972,"utters":46973,"Ġassigns":46974,"ĠPenet":46975,"ĠMonstrous":46976,"ĠBowen":46977,"ilver":46978,"Haunted":46979,"ĠDing":46980,"started":46981,"plin":46982,"Ġcontaminants":46983,"ĠDOE":46984,"ffen":46985,"ĠTechnician":46986,"Ry":46987,"Ġrobbers":46988,"Ġhotline":46989,"ĠGuardiola":46990,"ĠKaufman":46991,"rower":46992,"ĠDresden":46993,"ĠAlpine":46994,"Elf":46995,"Ġfmt":46996,"ĠSard":46997,"urses":46998,"gpu":46999,"Unix":47000,"Ġunequivocally":47001,"ĠCitizenship":47002,"quad":47003,"mire":47004,"ĠSweeney":47005,"Battery":47006,"615":47007,"Ġpancakes":47008,"Ġoats":47009,"Maps":47010,"ĠContrast":47011,"mbudsman":47012,"ĠEPS":47013,"Ġsubcommittee":47014,"Ġsourcing":47015,"Ġsizing":47016,"ĠBuffer":47017,"ĠMandatory":47018,"Ġmoderates":47019,"ĠPatterns":47020,"ĠChocobo":47021,"ĠZan":47022,"ĠSTATES":47023,"ĠJudging":47024,"ĠInher":47025,"*:":47026,"Ġbil":47027,"ĠYen":47028,"Ġexhilar":47029,"ollower":47030,"zers":47031,"Ġsnug":47032,"maximum":47033,"Ġdespicable":47034,"ĠPACK":47035,"ĠAnnex":47036,"Ġsarcastic":47037,"Ġlatex":47038,"Ġtamp":47039,"ĠSao":47040,"bah":47041,"ĠReverend":47042,"ĠChinatown":47043,"ĠAUT":47044,"documented":47045,"ĠGABA":47046,"ĠCanaan":47047,"ĠÙħ":47048,"Ġgoverns":47049,"prev":47050,"Esc":47051,"ĠEstimates":47052,"OSP":47053,"Ġendeavour":47054,"ĠClosing":47055,"ometime":47056,"everyone":47057,"Ġworsen":47058,"Ġscanners":47059,"Ġdeviations":47060,"ĠRobotics":47061,"ĠCompton":47062,"Ġsorcerer":47063,"Ġendogenous":47064,"Ġemulation":47065,"ĠPiercing":47066,"ĠAph":47067,"ĠSocket":47068,"Ġbould":47069,"ĠOU":47070,"ĠBorderlands":47071,"Ġ1863":47072,"Gordon":47073,"ĠWTO":47074,"Ġrestricts":47075,"Ġmosaic":47076,"Ġmelodies":47077,"çĦ":47078,"Tar":47079,"Ġdisson":47080,"ĠProvides":47081,"Ġ......":47082,"bek":47083,"FIX":47084,"Ġbroom":47085,"anship":47086,"Doctors":47087,"Ġnerds":47088,"ĠRegions":47089,"naissance":47090,"Ġmete":47091,"Ġcrept":47092,"plings":47093,"Ġgirlfriends":47094,"knit":47095,"igent":47096,"owe":47097,"Ġushered":47098,"ĠBaz":47099,"Mobil":47100,"434":47101,"ĠPresents":47102,"origin":47103,"Ġinsomnia":47104,"ĠAux":47105,"439":47106,"ĠChili":47107,"irsch":47108,"GAME":47109,"Ġgestation":47110,"algia":47111,"romising":47112,"$,":47113,"crow":47114,"ĠInspection":47115,"atomic":47116,"Relations":47117,"JOHN":47118,"roman":47119,"ĠClockwork":47120,"ĠBakr":47121,"mone":47122,"MET":47123,"Ġthirsty":47124,"Ġbc":47125,"Ġfaculties":47126,"Rum":47127,"Ġnuance":47128,"ĠDarius":47129,"pleting":47130,"fters":47131,"etchup":47132,"Registration":47133,"ĠKE":47134,"Rah":47135,"Ġpreferential":47136,"ĠLash":47137,"ĠHH":47138,"Valid":47139,"ĠNAV":47140,"Ġstarve":47141,"ĠGong":47142,"zynski":47143,"ĠActress":47144,"Ġwik":47145,"Ġunaccompanied":47146,"lvl":47147,"Bride":47148,"ADS":47149,"ĠCommando":47150,"ĠVaughn":47151,"Wallet":47152,"Ġhopping":47153,"ĠVie":47154,"Ġcaveats":47155,"Ġalas":47156,"ifled":47157,"abuse":47158,"661":47159,"Ġibn":47160,"Ġgul":47161,"Ġrobbing":47162,"til":47163,"ILA":47164,"Ġmitigating":47165,"Ġaptly":47166,"Ġtyrant":47167,"Ġmidday":47168,"ĠGilmore":47169,"ĠDecker":47170,"Ġ§§":47171,"partial":47172,"Exactly":47173,"Ġphenotype":47174,"Ġ[+]":47175,"ĠPlex":47176,"ĠIps":47177,"versions":47178,"Ġebook":47179,"Ġchic":47180,"gross":47181,"\":\"\"},{\"":47182,"ĠSurprisingly":47183,"Morgan":47184,"Ġresidues":47185,"ĠConfederation":47186,"infeld":47187,"Ġlyr":47188,"moderate":47189,"Ġperpendicular":47190,"VK":47191,"Ġsynchronized":47192,"Ġrefreshed":47193,"Ġadore":47194,"ĠTorment":47195,"olina":47196,"Ġ2600":47197,"ItemTracker":47198,"Ġpies":47199,"ĠFAT":47200,"ĠRHP":47201,"048":47202,"ĠRESP":47203,"ĠBJ":47204,"allows":47205,"Pand":47206,"Ġunwelcome":47207,"ĠVoc":47208,"ĠBastard":47209,"ĠOW":47210,"ĠLAR":47211,"ĠHealer":47212,"Environmental":47213,"ĠKenyan":47214,"ĠTrance":47215,"ĠPats":47216,"Ġaliases":47217,"ĠGarfield":47218,"Ġcampaigner":47219,"Ġadvancements":47220,"ĠOkinawa":47221,"ĠCoh":47222,"owsky":47223,"Ġstarved":47224,"Ġsizeable":47225,"Ġ:-)":47226,"ĠmRNA":47227,"Ġsuspensions":47228,"istar":47229,"Scotland":47230,"Prin":47231,"------------------------------------------------":47232,"Ġ502":47233,"Ġteaspoons":47234,"Ġ1050":47235,"Ġcoercive":47236,"ĠMasonic":47237,"edded":47238,"ĠPassenger":47239,"Ġlatt":47240,"Ġbraces":47241,"ĠSteal":47242,"ĠNYT":47243,"ĠKats":47244,"ĠCelest":47245,"aez":47246,"Tu":47247,"ĠCoulter":47248,"ðŁĺ":47249,"Flickr":47250,"ĠWilmington":47251,"iths":47252,"++;":47253,"Ġvending":47254,"Ġnegro":47255,"ĠPhi":47256,"ĠYellowstone":47257,"Callback":47258,"Ġshampoo":47259,"ĠShades":47260,"wat":47261,"Ġsuperhuman":47262,"Ġridiculed":47263,"Ġholiest":47264,"ombo":47265,"Ġinterns":47266,"Ġhone":47267,"ĠParagu":47268,"URI":47269,"Ġdangling":47270,"ãĤ»":47271,"sov":47272,"ictional":47273,"availability":47274,"Ġrevocation":47275,"Ġdow":47276,"inic":47277,"ĠTHEIR":47278,"Ġiso":47279,"Ġoutings":47280,"ĠLethal":47281,"Ġ)))":47282,"Ġinaccur":47283,"Ġoutlandish":47284,"Ġanus":47285,"letico":47286,"idon":47287,"lol":47288,"Ġunregulated":47289,"Ġsuccumbed":47290,"Ġcuff":47291,"ĠWasteland":47292,"letal":47293,"Ġsubstr":47294,"Ġcoffers":47295,"Ġautomakers":47296,"ovi":47297,"ĠXue":47298,"ĠDaytona":47299,"Ġjarring":47300,"Ġfumes":47301,"Ġdisbanded":47302,"zik":47303,"itton":47304,"Ġstrikingly":47305,"Ġspores":47306,"Adapter":47307,".):":47308,"ĠLyndon":47309,"ivalry":47310,"Ġorally":47311,"Ġtumultuous":47312,"Ġdispleasure":47313,"Ġcones":47314,"orrect":47315,"Ġappease":47316,"Ġderby":47317,"ĠTripoli":47318,"ĠAless":47319,"Ġpoked":47320,"ĠGuilty":47321,"vP":47322,"Enough":47323,"Ġoriginals":47324,"699":47325,"Ġrabbi":47326,"Ġproverbial":47327,"Ġpostpone":47328,"elope":47329,"ĠMisty":47330,"Ġstaffed":47331,"ĠUnemployment":47332,"reditary":47333,"Ġdiligent":47334,"recomm":47335,"measures":47336,"asin":47337,"825":47338,"Ġponds":47339,"Ġmmol":47340,"ĠSAR":47341,"ĠCARE":47342,"Ġ371":47343,"Ġclenched":47344,"ĠCorsair":47345,"Ġcaricature":47346,"zn":47347,"attach":47348,"ĠSchro":47349,"speak":47350,"painted":47351,"ĠSuc":47352,"ĠENT":47353,"Ġcellul":47354,"ĠPaid":47355,"diagn":47356,"WHERE":47357,"Ġtexted":47358,"Barn":47359,"Ġretracted":47360,"ĠReferred":47361,"Sav":47362,"Ġupkeep":47363,"Ġworkplaces":47364,"ĠTokens":47365,"Ġamplify":47366,"clinical":47367,"Ġmultic":47368,"mberg":47369,"Ġconvoluted":47370,"Region":47371,"565":47372,"ĠTopic":47373,"Ġsnail":47374,"Ġsaline":47375,"Ġinsurrection":47376,"ĠPetr":47377,"forts":47378,"BAT":47379,"ĠNavajo":47380,"Ġrudimentary":47381,"ĠLaksh":47382,"ONDON":47383,"Measure":47384,"Ġtransformer":47385,"ĠGoddard":47386,"Ġcoincides":47387,"irin":47388,"Rex":47389,"ĠBok":47390,"quit":47391,"Ġshotguns":47392,"Ġproletarian":47393,"Ġscorp":47394,"ĠAda":47395,"514":47396,"Ġslander":47397,"recorded":47398,"Ġembell":47399,"risome":47400,"Ġapologizing":47401,"ĠMulcair":47402,"ĠGibraltar":47403,"Cla":47404,"Ġallot":47405,"ĠAttention":47406,"Ġ433":47407,"leave":47408,"Ġwhine":47409,"ĠIssa":47410,"ĠFaust":47411,"ĠBarron":47412,"heny":47413,"Ġvictimized":47414,"Jews":47415,"Ġnurturing":47416,"ettel":47417,"Winged":47418,"ĠSubtle":47419,"Ġflavorful":47420,"ĠReps":47421,"enged":47422,"callback":47423,"Ġdirectional":47424,"Ġclasp":47425,"ĠDirections":47426,"planet":47427,"iculture":47428,"Helper":47429,"icion":47430,"acia":47431,"Ġç¥ŀ":47432,"Ġsurges":47433,"Ġcanoe":47434,"ĠPremiership":47435,"been":47436,"Ġdefied":47437,"ĠTrooper":47438,"Ġtripod":47439,"Ġgasp":47440,"ĠEuph":47441,"ĠAds":47442,"vernight":47443,"highly":47444,"Role":47445,"Ġentangled":47446,"ĠZeit":47447,"618":47448,"ĠRusty":47449,"Ġhavens":47450,"ĠVaughan":47451,"HAEL":47452,"ĠSERVICE":47453,"/,":47454,"Ġstricken":47455,"Ġdelusions":47456,"Ġbis":47457,"ĠHaf":47458,"Ġgratification":47459,"Ġenticing":47460,"UNCH":47461,"Adams":47462,"ĠOLED":47463,"ĠBeetle":47464,"Ġ1899":47465,"ĠSOFTWARE":47466,"ategor":47467,"VL":47468,"ĠTotem":47469,"ĠGators":47470,"ATURES":47471,"Ġimpedance":47472,"Registered":47473,"ĠCary":47474,"ĠAerial":47475,"onne":47476,"enium":47477,"Ġdred":47478,"ĠBeg":47479,"Ġconcurrently":47480,"Ġsuperpower":47481,"ĠXan":47482,"jew":47483,"imester":47484,"ĠDickinson":47485,"âĶģ":47486,"Fla":47487,"Ġpree":47488,"ĠRollins":47489,"©¶æ":47490,"Ġdenomination":47491,"ĠLana":47492,"516":47493,"Ġinciting":47494,"scribed":47495,"juries":47496,"ĠWonders":47497,"approximately":47498,"Ġsuspending":47499,"Ġmountainous":47500,"ĠLaugh":47501,"oidal":47502,"Ns":47503,"Detect":47504,")=":47505,"ĠLuthor":47506,"ĠSchwarzenegger":47507,"ĠMuller":47508,"ĠDevi":47509,"ecycle":47510,"Jar":47511,"613":47512,"ĠLongh":47513,"Bah":47514,"ĠSPORTS":47515,"nw":47516,"Ġrefinement":47517,"Ġwaterways":47518,"Ġdiner":47519,"Blade":47520,"683":47521,"Fac":47522,"Ġinitials":47523,"Ġrog":47524,"Ġparanormal":47525,"BUT":47526,"Ġ[(":47527,"ĠSwanson":47528,"ĠMesh":47529,"âĸ¬":47530,"Improve":47531,"ĠRadiation":47532,"ĠEsther":47533,"ĠEsk":47534,"ĠAly":47535,"iky":47536,"Ġirrad":47537,"ĠBuckingham":47538,"Ġrefill":47539,"Ġ._":47540,"Repe":47541,"CONCLUS":47542,"Ġdifferentiated":47543,"Ġchirop":47544,"ĠAtkins":47545,"Pattern":47546,"Ġexcise":47547,"Ġcabal":47548,"NSA":47549,"ĠSTA":47550,"ĠSIL":47551,"ĠParaly":47552,"Ġrye":47553,"ĠHowell":47554,"ĠCountdown":47555,"nesses":47556,"alysed":47557,"Ġresize":47558,"ãĤ½":47559,"Ġbudgetary":47560,"ĠStras":47561,"wang":47562,"Ġapiece":47563,"Ġprecincts":47564,"Ġpeach":47565,"Ġskyline":47566,"Ġ353":47567,"popular":47568,"Appearances":47569,"ĠMechanics":47570,"ĠDevOnline":47571,"Sullivan":47572,"Zen":47573,"Ġpu":47574,"opolis":47575,"544":47576,"Ġdeform":47577,"Ġcounteract":47578,"ĠLange":47579,"Ġ417":47580,"Console":47581,"774":47582,"Ġnodding":47583,"Ġpopulism":47584,"Ġhep":47585,"Ġcounselling":47586,"compliance":47587,"UFF":47588,"Ġundeniably":47589,"Ġrailing":47590,"ĠHorowitz":47591,"ĠSimone":47592,"ĠBungie":47593,"Ġak":47594,"ĠTalks":47595,"xff":47596,"flake":47597,"Crash":47598,"Ġsweaty":47599,"Ġbanquet":47600,"ĠOFFIC":47601,"Ġinventive":47602,"Ġastronomer":47603,"ĠStamford":47604,"ĠScare":47605,"ĠGREEN":47606,"olicited":47607,"Ġrusher":47608,"Ġcentrist":47609,"ighting":47610,"Ġsubclass":47611,"Ġdisav":47612,"Ġdefund":47613,"ĠNanto":47614,"ociate":47615,"mast":47616,"Ġpacif":47617,"Ġmend":47618,"eers":47619,"immigration":47620,"ESSION":47621,"Ġnumbering":47622,"Ġlaughable":47623,"ĠEnded":47624,"viation":47625,"emark":47626,"Pitt":47627,"Ġmeticulous":47628,"ĠLF":47629,"Ġcongratulated":47630,"ĠBirch":47631,"Ġswayed":47632,"Ġsemifinals":47633,"Ġhumankind":47634,"matter":47635,"ĠEquip":47636,"opausal":47637,"Said":47638,"ĠLayout":47639,"Ġvoicing":47640,"Ġthug":47641,"Ġpornographic":47642,"IPS":47643,"Ġmoaning":47644,"Ġgrievance":47645,"Ġconfessions":47646,"escal":47647,"TEXTURE":47648,"Authent":47649,"osaurus":47650,"Purchase":47651,"Ġrelegation":47652,"alter":47653,"Ġ³³":47654,"Ġriddled":47655,"Ġogre":47656,"ĠLowell":47657,"Occup":47658,"Eat":47659,"ĠHyder":47660,"ĠAdviser":47661,"Commerce":47662,"Hunt":47663,"ĠOrth":47664,"ĠCompetitive":47665,"ĠCLA":47666,"CDC":47667,"Ġsalads":47668,"Fle":47669,"Ġindustrialized":47670,"`,":47671,"ĠOWN":47672,"Ġbeck":47673,"ĠParticularly":47674,"oubt":47675,"ĠmM":47676,"ĠHussain":47677,"ĠChennai":47678,"Ġ920":47679,"Ġappointing":47680,"ĠCullen":47681,",,,,,,,,":47682,"Ġpores":47683,"verified":47684,"Ġbiochemical":47685,"emate":47686,"Ġcowardly":47687,"ĠHelsinki":47688,"ĠEthiopian":47689,"SOURCE":47690,"ERC":47691,"estro":47692,"Ġbiotech":47693,"ĠSour":47694,"Ġbrewer":47695,"Bloomberg":47696,"Ġintensify":47697,"Glass":47698,"anco":47699,"ĠFDR":47700,"greSQL":47701,"ĠFires":47702,"©¶æ¥µ":47703,"eco":47704,"1001":47705,"ĠHomeless":47706,"Ġinstantaneous":47707,"ĠHaste":47708,"igel":47709,"Diamond":47710,"Ġpaving":47711,"Ġlandfill":47712,"Ġdads":47713,"houn":47714,":]":47715,"Ġincendiary":47716,"ĠLivingston":47717,"ĠHilbert":47718,"ĠChecks":47719,"styles":47720,"inators":47721,"ĠClive":47722,"phrine":47723,"Ġchimpanzees":47724,"Ġpall":47725,"ĠJM":47726,"ĠAadhaar":47727,"ðĿ":47728,"Ġachievable":47729,"disabled":47730,"PET":47731,"OOOOOOOO":47732,"Mot":47733,"Ġintangible":47734,"Ġballet":47735,"ĠWebs":47736,"ĠEstimated":47737,"Effects":47738,"Ġbailed":47739,"Joshua":47740,"Ġturbulence":47741,"Ġoccupant":47742,"ĠDaylight":47743,"Ġ361":47744,"meet":47745,"Ġstatically":47746,"Ġonlook":47747,"Ġki":47748,"illegal":47749,"Ġvelvet":47750,"Ġdehydration":47751,"Ġacquies":47752,"ĠRez":47753,"akura":47754,"ĠUpton":47755,"atro":47756,"Ġincomprehensible":47757,"Ġbackdoor":47758,"ĠRhino":47759,"727":47760,"Ġmaths":47761,")+":47762,"Ġheresy":47763,"Ġdf":47764,"ĠRoche":47765,"ĠLydia":47766,"Ġpancreat":47767,"reply":47768,"arrell":47769,"Ġsolicitation":47770,"Ġcircadian":47771,"BIP":47772,"Ġforay":47773,"Ġcryptic":47774,"izu":47775,"imeo":47776,"ĠTomato":47777,"ĠHoms":47778,"examination":47779,"Ġquarry":47780,"ĠValiant":47781,"ĠJericho":47782,"ĠINCLUD":47783,"Ġ1840":47784,"519":47785,"Ġresists":47786,"Ġsnapshots":47787,"ĠSpur":47788,"ĠAntiqu":47789,"Login":47790,"Ġbestselling":47791,"Ġantic":47792,"ĠSutherland":47793,"ãĤ¢ãĥ«":47794,"Ġ~/":47795,"ĠParm":47796,"èĥ":47797,"Pages":47798,"intensity":47799,"Ġimmobil":47800,"Ġ1865":47801,"zzo":47802,"Ġnifty":47803,"Ġfentanyl":47804,"ĠPreservation":47805,"ophen":47806,"Ġdarts":47807,"ĠDinosaur":47808,"pointers":47809,"ĠRite":47810,"suggest":47811,"awareness":47812,"ĠSheridan":47813,"Ġstances":47814,"Ġsorcery":47815,"Ġperjury":47816,"ĠNikola":47817,"iever":47818,"Ġfiance":47819,"ĠJordanian":47820,"ĠBalloon":47821,"Ġnab":47822,"Ġkb":47823,"Ġhumanities":47824,"ĠTanaka":47825,"hillary":47826,"Ġconsultancy":47827,"ĠZub":47828,"Ġremission":47829,"Ġconfid":47830,"CHQ":47831,"ĠFug":47832,"Ġimprovis":47833,"Yep":47834,"/_":47835,"Ġunwillingness":47836,"Ġportfolios":47837,"055":47838,"ĠInstructor":47839,"aiman":47840,"Ġclaimants":47841,"Mbps":47842,"ĠBye":47843,"received":47844,"Tweet":47845,"Ġindemn":47846,"riz":47847,"amara":47848,"Nat":47849,"Ġevaluates":47850,"ĠLur":47851,"epad":47852,"FOX":47853,"ĠThro":47854,"Ġrusty":47855,"Ġbedrock":47856,"ĠOprah":47857,"JB":47858,"Ġmanipulative":47859,"Ġwillful":47860,"Ġrelapse":47861,"Ġextant":47862,"Theme":47863,"Sensor":47864,"ĠStability":47865,"govern":47866,"Ġpoppy":47867,"Ġknack":47868,"Ġinsulated":47869,"ĠTile":47870,"ĠExtrem":47871,"Ġuntold":47872,"Ġconverge":47873,"Ġrefuel":47874,"igroup":47875,"Ġdistortions":47876,"Ġravaged":47877,"Ġmechanically":47878,"ĠReilly":47879,"ĠNose":47880,"ĠIncarnation":47881,"ĠBecky":47882,"abbling":47883,"Ġtaco":47884,"Ġrake":47885,"Ġmelancholy":47886,"Ġillustrious":47887,"ĠDartmouth":47888,"Guide":47889,"ĠRazer":47890,"ĠBenz":47891,"Ultimate":47892,"ĠSurprise":47893,"Ġpageant":47894,"offer":47895,"Whoever":47896,"Ġwiser":47897,"Ġchemist":47898,"ĠHELL":47899,"ĠBulk":47900,"Ġplutonium":47901,"ĠCOVER":47902,"Ö¼":47903,"failed":47904,"Ġtirelessly":47905,"Ġinfertility":47906,"ĠTrident":47907,"ĠShowtime":47908,"ĠCiv":47909,"Vice":47910,"requires":47911,"ittance":47912,"Ġuncontrolled":47913,"interesting":47914,"561":47915,"Ġinnovate":47916,"ategic":47917,"Lie":47918,"ĠSelling":47919,"Ul":47920,"Ġsavior":47921,"ĠTosh":47922,"Ġswast":47923,"PASS":47924,"Ġrink":47925,"Ġcardio":47926,"ĠIro":47927,"udi":47928,"Ġvantage":47929,"Ġvans":47930,"ĠNiño":47931,"+=":47932,"Ġpropagate":47933,"<?":47934,"Ġmethodological":47935,"20439":47936,"Ġtriglycer":47937,"Ġingrained":47938,"ĠAnnotations":47939,"arranted":47940,"617":47941,"ĠSodium":47942,"ĠAAC":47943,"technical":47944,"multipl":47945,"Ġ373":47946,"åĭ":47947,"Ġdecisively":47948,"Ġboosters":47949,"Ġdesserts":47950,"ĠGrenade":47951,"Ġtestifying":47952,"ĠScully":47953,"IDs":47954,"Ġlockdown":47955,"ĠScher":47956,"ĠRé":47957,"ĠWhitman":47958,"ĠRamsay":47959,"remote":47960,"Ġhikers":47961,"ĠHyundai":47962,"Ġconscientious":47963,"Ġclerics":47964,"ĠSiberian":47965,"uti":47966,"isbury":47967,"Ġrelayed":47968,"Ġquartz":47969,"ĠCBI":47970,"seekers":47971,"ulla":47972,"Ġwelding":47973,"ĠShal":47974,"bleacher":47975,"Tai":47976,"ĠSamson":47977,"Ġtumble":47978,"ĠInvestor":47979,"Ġsubcontract":47980,"ĠShinra":47981,"owicz":47982,"jandro":47983,"dad":47984,"Ġterminating":47985,"ĠNeural":47986,"代":47987,"Ġleakage":47988,"ĠMidlands":47989,"ĠCaucasus":47990,"íķ":47991,"cit":47992,"llan":47993,"ivably":47994,"ĠAlbion":47995,"Ġ457":47996,"Ġregistrations":47997,"Ġcomrade":47998,"Ġclipboard":47999,"047":48000,"Ġdiscouraging":48001,"ĠOops":48002,"Adapt":48003,"Ġempath":48004,"nv":48005,"ĠPROT":48006,"ĠDonn":48007,"ĠPax":48008,"ĠBayer":48009,"tis":48010,"Square":48011,"Ġfootprints":48012,"particip":48013,"ĠChilean":48014,"Brend":48015,"inducing":48016,"Magn":48017,"Ġclubhouse":48018,"ĠMagnum":48019,"Ġencamp":48020,"ĠEthnic":48021,"ucha":48022,"erey":48023,"Ġwatered":48024,"ĠCalais":48025,"Ġcomplexion":48026,"Ġsects":48027,"Ġrenters":48028,"Ġbras":48029,"oÄŁan":48030,"Timeout":48031,"Management":48032,"Ġinfographic":48033,"Pokemon":48034,"Clar":48035,"Ġlocality":48036,"Ġflora":48037,"asel":48038,"Pont":48039,"Ġpopulate":48040,"ĠOng":48041,"Ġsubsistence":48042,"Ġauctions":48043,"ĠMcAuliffe":48044,"ĠLOOK":48045,"bringer":48046,"Ġtitan":48047,"Ġmanifold":48048,"ĠâĹı":48049,"Ġcalibrated":48050,"Ġcaliphate":48051,"ĠSHE":48052,"ĠCommissioners":48053,"ceivable":48054,"jc":48055,"Winner":48056,"524":48057,"Ġcondone":48058,"Otherwise":48059,"Ġpiling":48060,"Ġembody":48061,"ĠCrimean":48062,"utics":48063,"ĠExhibition":48064,"Ġ426":48065,"eering":48066,"Ġvying":48067,"ĠHUGE":48068,"*=-":48069,"Ġprincipled":48070,"à¦":48071,"Ġquirks":48072,"ĠEditors":48073,"puting":48074,"GES":48075,"ĠFTA":48076,"ा":48077,"addon":48078,"ĠHAM":48079,"ĠFrieza":48080,"Woman":48081,".$":48082,"Ġcrib":48083,"ĠHerod":48084,"Ġtimers":48085,"ĠSpaces":48086,"ĠMacintosh":48087,"ataka":48088,"Ġglide":48089,"Ġsmelling":48090,"ĠBAL":48091,"Ġunsu":48092,"Ġcondos":48093,"Ġbicycl":48094,"ĠRevival":48095,"553":48096,"Ġjuggling":48097,"Hug":48098,"ĠKardashian":48099,"ĠBalkans":48100,"multiple":48101,"Ġnutritious":48102,"ocry":48103,"1900":48104,"Ġintegrates":48105,"Ġadjoining":48106,"ĠFolder":48107,"rollment":48108,"venient":48109,"Ġuber":48110,"yi":48111,"Ġwhiff":48112,"ĠJuven":48113,"ĠBorough":48114,"nette":48115,"Ġbilingual":48116,"ĠSparks":48117,"phthal":48118,"manufact":48119,"Ġtouting":48120,"ĠPHI":48121,"Keefe":48122,"Reward":48123,"Ġinfall":48124,"ĠTemper":48125,"typically":48126,"ĠNikol":48127,"Ġregulars":48128,"Ġpseudonym":48129,"Ġexhibitions":48130,"Ġblaster":48131,"Ġ409":48132,"warming":48133,"Ġreverber":48134,"Ġreciprocal":48135,"Ġ670":48136,"ipient":48137,"bett":48138,"ĠBegins":48139,"Ġitching":48140,"ĠPhar":48141,"Assuming":48142,"Ġemitting":48143,"ĠMLG":48144,"Ġbirthplace":48145,"Ġtaunt":48146,"ĠLuffy":48147,"ĠAmit":48148,"Ġcircled":48149,"ĠNost":48150,"ennett":48151,"Ġdeforestation":48152,"ĠHistorically":48153,"ĠEveryday":48154,"Ġovertake":48155,"792":48156,"Ġnun":48157,"ĠLucia":48158,"Ġaccompanies":48159,"ĠSeeking":48160,"ĠTrash":48161,"anism":48162,"Rogue":48163,"Ġnorthwestern":48164,"ĠSupplemental":48165,"ĠNYU":48166,"ĠFRI":48167,"ĠSatisf":48168,"xes":48169,"517":48170,"Ġreassured":48171,"Ġsporadic":48172,"Ġ701":48173,"Ġmedial":48174,"Ġcannabinoid":48175,"Ġbarbaric":48176,"Ġepis":48177,"ĠExplosive":48178,"ĠDough":48179,"Ġunsolved":48180,"Supported":48181,"Ġacknowledgment":48182,"spawn":48183,"Ġkitchens":48184,"Ġ-=":48185,"talking":48186,"icist":48187,"ĠPegasus":48188,"ĠPSU":48189,"Ġphoton":48190,"ĠAuthentication":48191,"RG":48192,"@#&":48193,"762":48194,"ĠClair":48195,"Ġdiaper":48196,"Ġbrist":48197,"ĠProsecutors":48198,"ĠJem":48199,"628":48200,"ĠEverywhere":48201,"ĠJeanne":48202,"equality":48203,"ãĥ©ãĥ³":48204,"objects":48205,"ĠPelicans":48206,"Ġ392":48207,"Ġblu":48208,"bys":48209,"ĠAgo":48210,"Ġinstructional":48211,"Ġdiscriminating":48212,"ĠTRAN":48213,"ĠCornel":48214,"agos":48215,"Ġtyre":48216,"Ġaspiration":48217,"ĠBridgewater":48218,"\":-":48219,"!\".":48220,"ĠEns":48221,"ĠCoco":48222,"Pie":48223,"Ġdetach":48224,"ĠCouch":48225,"Ġphysique":48226,"ĠOccupations":48227,"oscopic":48228,"enough":48229,"Buzz":48230,"Appearance":48231,"YP":48232,"Ġracer":48233,"Ġcomplicity":48234,"rpm":48235,"Toy":48236,"Ġinterrupts":48237,"ĠCatalyst":48238,"Ġutilitarian":48239,"impact":48240,"Ġspaghetti":48241,"Ġporous":48242,"Ġesteemed":48243,"Ġinciner":48244,"ĠIOC":48245,"748":48246,"Ġespresso":48247,"ĠSmile":48248,"abilia":48249,"635":48250,"Ġmathematician":48251,"Ġ424":48252,"ĠKL":48253,"ĠHIP":48254,"Ġoverheard":48255,"ĠTud":48256,"ĠTec":48257,"Ġquizz":48258,"Ġflattering":48259,"Ġconn":48260,"âĢİ":48261,"Ġattaches":48262,"ĠROS":48263,"ĠACS":48264,"Ġtcp":48265,"ĠShame":48266,"skip":48267,"respected":48268,"ĠTrinidad":48269,"grain":48270,"Ġfoothold":48271,"ĠUncharted":48272,"ĠJulio":48273,"zl":48274,"avored":48275,"ĠAnxiety":48276,"errors":48277,"ĠCentauri":48278,"itsch":48279,"Daddy":48280,"Ġclutching":48281,"ĠImplement":48282,"ĠGutierrez":48283,"Ġ760":48284,"Ġteleportation":48285,"endra":48286,"Ġreversible":48287,"stros":48288,"Adventure":48289,"083":48290,"Ġliberating":48291,"Ġasphalt":48292,"ĠSpend":48293,"ARDS":48294,"imsy":48295,"PRES":48296,"ĠEmerging":48297,"Ġwildfires":48298,"Ġtechnologically":48299,"Ġemits":48300,"ĠARTICLE":48301,"Ġirregularities":48302,"Ġcherish":48303,"çīĪ":48304,"Ġstink":48305,"ĠRost":48306,"Economic":48307,"Ġcoughing":48308,"ĠMcCann":48309,"properties":48310,"ilantro":48311,"Ġrenegoti":48312,"Translation":48313,"Ġinquest":48314,"ĠGrape":48315,"ooters":48316,"gui":48317,"ĠSwordsman":48318,"aceae":48319,"hitting":48320,"Ġrc":48321,"Ġexerted":48322,"ĠSAP":48323,"itent":48324,"Ġperilous":48325,"Ġobscurity":48326,"Ġassassinate":48327,"Ġaboriginal":48328,"Ġrescuing":48329,"ĠShattered":48330,"locking":48331,"allion":48332,"Changing":48333,"ĠHarrington":48334,"ĠBord":48335,"ĠAfghans":48336,"Jamie":48337,"aretz":48338,"ĠAugustus":48339,"Ġ386":48340,"830":48341,"Ġjog":48342,"okingly":48343,"Trigger":48344,"ĠHOR":48345,"Statistics":48346,"Ġviewership":48347,"Ġadditives":48348,"hur":48349,"Ġmaximizing":48350,"ĠRove":48351,"ĠLouie":48352,"ĠBucket":48353,"ĠCHRIST":48354,"ousel":48355,"Ġstreaks":48356,"irted":48357,"Ġtert":48358,"Ġcolonialism":48359,"Ġburying":48360,"yk":48361,"Condition":48362,"ĠDPRK":48363,"ById":48364,"751":48365,"âĹ¼":48366,"Ġworrisome":48367,"Ġvocational":48368,"slice":48369,"Ġsails":48370,"ĠCorrectional":48371,"954":48372,"Ġtul":48373,"Kid":48374,"luster":48375,"Ġfamilial":48376,"ĠSpit":48377,"ĠEpiscopal":48378,"Specifically":48379,"ĠVolcano":48380,"runs":48381,"qs":48382,"Ġvetted":48383,"Ġcrammed":48384,"trop":48385,"herer":48386,"Thankfully":48387,"Ġpercussion":48388,"Ġoranges":48389,"Ġroundup":48390,"Ġ499":48391,"xious":48392,"Characters":48393,"ĠZionism":48394,"ĠRao":48395,"ÃĽÃĽ":48396,"WF":48397,"Ġunintentional":48398,"ONEY":48399,"Grab":48400,"Commercial":48401,"Ġglutamate":48402,"ĠMcKenna":48403,"ruciating":48404,"nington":48405,"ihu":48406,"Chan":48407,"ĠSwap":48408,"Ġleaflets":48409,"Ġfunctionally":48410,"erous":48411,"Farm":48412,"Ġcaloric":48413,"ĠLiterally":48414,"concert":48415,"Ġshenan":48416,"Ġrepaid":48417,"eyes":48418,"Ġbashing":48419,"ĠGorge":48420,"Ġcollaborations":48421,"Ġunaccount":48422,"itchie":48423,"Ġteamwork":48424,"ppelin":48425,"Ġpiping":48426,"Ġminced":48427,"Ġdiam":48428,"rieg":48429,"Ġmascara":48430,"Ġsucker":48431,"ĠMoons":48432,"Apps":48433,"ĠPeck":48434,"Ġperv":48435,"ĠFloat":48436,"oley":48437,"ĠNish":48438,"imize":48439,"Ġaromatic":48440,"uin":48441,"endish":48442,"!/":48443,"ĠBicycle":48444,"ĠASIC":48445,"ileged":48446,"ĠQuadro":48447,"iosyn":48448,"Ġlockout":48449,"ĠWink":48450,"SPEC":48451,"Attempts":48452,"Ġseeded":48453,"redo":48454,"iasis":48455,"Ġsnag":48456,"ãĥķãĤ©":48457,"ãĤ¶":48458,"Ġgrounding":48459,"Ġreliever":48460,"Ġfrivolous":48461,"ĠGifts":48462,"ĠFaces":48463,"Especially":48464,"Ġmicrobiome":48465,"imag":48466,"ĠSchl":48467,"ĠPles":48468,"ĠBleach":48469,"ĠIrwin":48470,"ĠEaton":48471,"ĠDisciple":48472,"Ġmultiplication":48473,"Ġcoerced":48474,"Ġ419":48475,"sth":48476,"Evil":48477,"Bomb":48478,"Ġexorc":48479,"Ġstaggered":48480,"LESS":48481,"Ġinertia":48482,"ĠEDIT":48483,"Ġgob":48484,"Traditional":48485,"Ġclassy":48486,"Leary":48487,"ĠPAGE":48488,"yrs":48489,"Ġtransporter":48490,"Ġmatured":48491,"Ġhijab":48492,"Ġbiome":48493,"Whereas":48494,"Ġextermination":48495,"ĠTues":48496,"ĠTakeru":48497,"ĠAudrey":48498,"erial":48499,"ĠAden":48500,"affles":48501,"Ġnarcissistic":48502,"ĠBaird":48503,"UTF":48504,"Ire":48505,"ĠConnie":48506,"Champ":48507,"Ġwhispering":48508,"ĠHatt":48509,"DK":48510,"Ġdisinfect":48511,"Ġdeducted":48512,"Ġpartake":48513,"Ġdowngrade":48514,"ĠEsports":48515,"ĠContinuing":48516,"Ġdemocratically":48517,"icrobial":48518,"itta":48519,"Ġlimestone":48520,"Ġexempted":48521,"ĠFrenzy":48522,"Herm":48523,"728":48524,"Ġfledgling":48525,"Meta":48526,"76561":48527,"693":48528,"%:":48529,"wake":48530,"526":48531,"ĠDiscipline":48532,"Ġvirginity":48533,"ĠLegions":48534,"ĠFrankie":48535,"intent":48536,"Ġrestrooms":48537,"ĠRouter":48538,"daq":48539,"Ġobjectionable":48540,"âĨij":48541,"wark":48542,"ĠRahul":48543,"gain":48544,"activation":48545,"absolute":48546,"ĠAccessed":48547,"Ġ2400":48548,"oggles":48549,"Ġsecondly":48550,"ĠDEFENSE":48551,"Ġpostage":48552,"wrapper":48553,"sharp":48554,"729":48555,"Ġcommunicates":48556,"Ġaddon":48557,"ĠMilitia":48558,"Hong":48559,"Ġslumped":48560,"ĠJPEG":48561,"ĠIcar":48562,"adish":48563,"681":48564,"Ġmajesty":48565,"ĠWolfgang":48566,"ĠElastic":48567,"uper":48568,"Ġviz":48569,"Ġunconsciously":48570,"ĠSTD":48571,"ĠSass":48572,"Ġflowering":48573,"ĠHelic":48574,"ĠDraper":48575,"ĠAmateur":48576,"Ġmanure":48577,"Ġdisingen":48578,"ĠLei":48579,"bring":48580,"949":48581,"Ġinhibited":48582,"Ġheadquartered":48583,"Ġenigmatic":48584,"���":48585,"Ġredress":48586,"RH":48587,"Ġrattled":48588,"Ġdiction":48589,"lio":48590,"ĠTBA":48591,"ĠSNAP":48592,"Calling":48593,"Ġfascists":48594,"ĠDove":48595,"iewicz":48596,"036":48597,"Ġcoasts":48598,"ĠRect":48599,"Ġ)]":48600,"Lot":48601,"629":48602,"ĠSEM":48603,"ĠPetersen":48604,"ĠExplain":48605,"ĠBoards":48606,"ĠBezos":48607,"ĠJournals":48608,"Ġ2024":48609,"parser":48610,"Ġmistrust":48611,"Ġgrate":48612,"ĠLocked":48613,"boa":48614,"Saint":48615,"gaming":48616,"Ġvowel":48617,"inately":48618,"blow":48619,"Allah":48620,"Ġunmatched":48621,"Ġbordering":48622,"ĠExpend":48623,"nr":48624,"Oracle":48625,"rouch":48626,"Ġcontiguous":48627,"acus":48628,"Ġdistraught":48629,"581":48630,"Ġanatomical":48631,"OX":48632,"apixel":48633,"833":48634,"ĠPLUS":48635,"Ġresusc":48636,"Ġabiding":48637,"573":48638,"Ġvacancies":48639,"Emily":48640,"Ġhypothal":48641,"ĠWerner":48642,"ĠWee":48643,"ĠDJs":48644,"513":48645,"Ġwitchcraft":48646,"Ġacupuncture":48647,"entary":48648,"benefit":48649,"Products":48650,"ĠPSP":48651,"ĠMPG":48652,"ĠJinn":48653,"ĠJarrett":48654,"Ġ445":48655,"ĠImaging":48656,"ĠPyth":48657,"Finish":48658,"Ġtex":48659,"Ġjuveniles":48660,"Ġheroism":48661,"Ġdoubtless":48662,"ĠAki":48663,"ĠTend":48664,"ĠPatriarch":48665,"Ġbitters":48666,"ĠTelecommunications":48667,"itatively":48668,"agna":48669,"Ġrg":48670,"ĠSOLD":48671,"Ġcompulsion":48672,"ĠNasa":48673,"ĠKathryn":48674,"Ġmillionaires":48675,"Ġintrinsically":48676,"Ġbolstered":48677,"timeout":48678,"flo":48679,"Ġtutor":48680,"pour":48681,"Statement":48682,"Ġ{*":48683,"ĠRudolph":48684,"ĠKimberly":48685,"rogens":48686,"adiq":48687,"]+":48688,"Ġindignation":48689,"Ġfracturing":48690,"ĠReleases":48691,"ĠGrain":48692,"protein":48693,"Lago":48694,"Ġvacations":48695,"Ġbooted":48696,"ĠTHREE":48697,"ĠHG":48698,"orescence":48699,"Ġtf":48700,"Ġsoar":48701,"iosyncr":48702,"Ġglances":48703,"ĠSpoon":48704,"ĠJury":48705,"ĠCowboy":48706,"Ġcreatively":48707,"Higher":48708,"Ġsolicitor":48709,"Ġhawk":48710,"acio":48711,"896":48712,"Ġsuperflu":48713,"Ġbombshell":48714,"cture":48715,"Ġbrokerage":48716,"Ġraiding":48717,"Ġfrench":48718,"Ġangled":48719,"Transaction":48720,"ĠGenocide":48721,"upe":48722,"ĠHaitian":48723,"572":48724,"!:":48725,"Ġunwittingly":48726,"iterator":48727,"scroll":48728,"Ġtallied":48729,"Ġbiomedical":48730,"ĠCARD":48731,"Ġeuphem":48732,"Ġbrainstorm":48733,"aquin":48734,"Ko":48735,"Michelle":48736,"ĠRunes":48737,"ĠBallistic":48738,"uders":48739,"Ġmodesty":48740,"ĠiPads":48741,"ĠEzekiel":48742,"YE":48743,"Ġstarship":48744,"Ġpowerfully":48745,"Ġperl":48746,"ĠShade":48747,"ĠQuart":48748,"ĠEEG":48749,"Ġfisherman":48750,"OSED":48751,"ĠTypical":48752,"dfx":48753,"Ġmeshes":48754,"Ġetched":48755,"worthiness":48756,"Ġtoppled":48757,"Ġ396":48758,"orius":48759,"Weiss":48760,"Ġmysql":48761,"ĠValhalla":48762,"ÙĴ":48763,"leasing":48764,"Ġrecomp":48765,"rapnel":48766,"Sel":48767,"043":48768,"Ġderailed":48769,"ĠGuides":48770,"IRT":48771,"Ġdehuman":48772,"ĠBrittany":48773,"\"))":48774,"Ġexclaim":48775,"Ġbalk":48776,"Ġ840":48777,"CLAIM":48778,"intel":48779,"LAB":48780,"Ġpegged":48781,"Ġastroph":48782,"smoking":48783,"Ġrigging":48784,"Ġfixation":48785,"Ġcatapult":48786,"inside":48787,"ĠCascade":48788,"ĠBolshevik":48789,"Gaza":48790,"Depth":48791,"Ġloudspe":48792,"Ġalmonds":48793,"meyer":48794,"leness":48795,"jen":48796,"fresh":48797,"Ġunbeaten":48798,"ĠSquid":48799,"ĠPresumably":48800,"Timer":48801,"BW":48802,"Ġrosters":48803,"Ġellipt":48804,"ĠHarriet":48805,"database":48806,"ĠMutual":48807,"ĠCommodore":48808,"uked":48809,"knife":48810,"ĠCOMMUN":48811,"hya":48812,"Ġmelts":48813,"archives":48814,"Ġratification":48815,"Ġmultiplying":48816,"Ġinteroper":48817,"Ġascert":48818,"wings":48819,"verting":48820,"ĠScorpion":48821,"aye":48822,"ĠPortsmouth":48823,"ĠMTA":48824,"nit":48825,"iazep":48826,"Ġquarantine":48827,"Ġslideshow":48828,"Ġcentimeters":48829,"Ġsynopsis":48830,"Ġspate":48831,"thirst":48832,"Ġnominating":48833,"ĠMelvin":48834,"Preview":48835,"Ġthrob":48836,"Ġgenerational":48837,"ĠRadius":48838,"restling":48839,"putable":48840,"awar":48841,"NECT":48842,"Ġunlawfully":48843,"ĠRevelations":48844,"Wikipedia":48845,"surv":48846,"Ġeyeing":48847,"ijn":48848,"ĠFW":48849,"Ġbrunt":48850,"Ġinterstellar":48851,"Ġclitor":48852,"ĠCroatian":48853,"ĠChic":48854,"eva":48855,"ĠDisapp":48856,"ĠAkin":48857,"ineries":48858,"dust":48859,"Interested":48860,"Ġgenesis":48861,"ĠEucl":48862,"ön":48863,"picking":48864,"Ġmutated":48865,"Ġdisapprove":48866,"ĠHDL":48867,"Ġ625":48868,"̶":48869,"cancer":48870,"Ġsquats":48871,"Ġlevers":48872,"Discuss":48873,"=]":48874,"Dex":48875,"ĠVIDEOS":48876,"AUD":48877,"Ġtransact":48878,"ĠKinect":48879,"ĠKuala":48880,"ĠCyp":48881,"747":48882,"Ġshattering":48883,"Ġarsenic":48884,"ĠIntake":48885,"ĠAngelo":48886,"ĠQuit":48887,"ĠKhe":48888,"Ġ1893":48889,"Maker":48890,"029":48891,"ĠPainting":48892,"Disable":48893,"916":48894,"Ġanalges":48895,"Ġtactile":48896,"Ġprophes":48897,"Ġdiced":48898,"ĠTravels":48899,"ĠHeader":48900,"ĠClubs":48901,"Assistant":48902,"Ġincrim":48903,"Ġdips":48904,"Ġcrucifix":48905,"ĠShanahan":48906,"ĠInterpret":48907,"Ġ4090":48908,"alogy":48909,"abba":48910,"Ġsimulac":48911,"husband":48912,"SIM":48913,"Ġrecycle":48914,"ucer":48915,"edged":48916,"Ġrenaissance":48917,"ĠBombay":48918,"Catholic":48919,"ĠLINE":48920,"ĠClothing":48921,"reports":48922,"Ġplaus":48923,"Ġdag":48924,"ĠMace":48925,"ZI":48926,"Ġintruder":48927,"ĠVeterinary":48928,"gru":48929,"Ġsneaky":48930,"ĠSie":48931,"ĠCinnamon":48932,"POSE":48933,"Ġcourier":48934,"ĠCNS":48935,"Ġemancipation":48936,"sit":48937,"Ġplaythrough":48938,"ĠFacilities":48939,"virt":48940,"ĠGauntlet":48941,"Thompson":48942,"Ġunbelievably":48943,"Parameters":48944,"Ġstitching":48945,"igne":48946,"ĠTHESE":48947,"Privacy":48948,"Ġshenanigans":48949,"Ġvitri":48950,"ĠValid":48951,"591":48952,"Ń·":48953,"ĠPrototype":48954,"inka":48955,"SCP":48956,"ĠTid":48957,"èĪ":48958,"olded":48959,"Ġindividuality":48960,"Ġbarking":48961,"Ġmars":48962,"ĠWD":48963,"Ġ820":48964,"Ġtir":48965,"Ġslapping":48966,"Ġdisgruntled":48967,"ĠAngola":48968,"rius":48969,"ĠTornado":48970,"ĠThurs":48971,"Ġcaptcha":48972,"Ġangst":48973,"ĠPog":48974,"ĠAssassins":48975,"ĠAdidas":48976,"Ġjoyful":48977,"Ġwhining":48978,"Emergency":48979,"Ġphosphorus":48980,"Ġattrition":48981,"ophon":48982,"ĠTimberwolves":48983,"ĠJah":48984,"ĠBringing":48985,"ĠWad":48986,"ĠEnsure":48987,"ohl":48988,"ĠXie":48989,"ommel":48990,"cmp":48991,"Ġzipper":48992,"Ġrelat":48993,"ĠCorridor":48994,"milo":48995,"TING":48996,"Avg":48997,"Ġcropped":48998,"]}":48999,"Ġraged":49000,"ĠLumpur":49001,"ĠGuerrero":49002,"ourke":49003,"Nut":49004,"Ġoffsets":49005,"oglu":49006,"drm":49007,"Ġmortals":49008,"latable":49009,"Ġdismissive":49010,"ä¸ī":49011,"Ġthroats":49012,"Ġchipset":49013,"ĠSpotlight":49014,"Catalog":49015,"artist":49016,"Gb":49017,"Ġchilly":49018,"Ġstoked":49019,"Ġ374":49020,"Ward":49021,"Latin":49022,"Ġfiasco":49023,"Ġbleach":49024,"Ġbrav":49025,"Enhanced":49026,"Ġinoc":49027,"ĠFiorina":49028,"_>":49029,"Ġleukemia":49030,"Ġeluc":49031,"Ġannouncer":49032,"ĠLithuan":49033,"ĠArmageddon":49034,"åĩ":49035,"Lenin":49036,"ĠRuk":49037,"Ġpepp":49038,"ĠRomantic":49039,"ĠPIT":49040,"ĠInterstellar":49041,"ĠAtkinson":49042,"Raid":49043,"Js":49044,"Goal":49045,"Course":49046,"Ġvanishing":49047,"esley":49048,"ĠRounds":49049,"Elsa":49050,"593":49051,"Ġredundancy":49052,"ĠSTAND":49053,"Ġprophetic":49054,"Ġhabitable":49055,"ryu":49056,"Ġfaintly":49057,"MODE":49058,"Ġflanked":49059,"IRC":49060,"Awesome":49061,"Ġspurious":49062,"ĠZah":49063,"ĠMSG":49064,"Ġshading":49065,"Ġmotivational":49066,"ĠSantana":49067,"ĠSPR":49068,"Ġexcruciating":49069,"omial":49070,"ĠMiko":49071,"ĠLeopard":49072,"Abyss":49073,"Ġ[|":49074,"dirty":49075,"Ġbaths":49076,"Ġdemoral":49077,"andre":49078,"PB":49079,"Ġunification":49080,"Ġsacrament":49081,"Ġ[&":49082,"Ġpriceless":49083,"Ġgelatin":49084,"Ġemanating":49085,"ĠAllaah":49086,"986":49087,"Ġoutburst":49088,"Ġeras":49089,"ĠXVI":49090,"ĠSPI":49091,"Ott":49092,"ĠLazarus":49093,"PLIED":49094,"Flying":49095,"blogs":49096,"Wisconsin":49097,"Raven":49098,"Ġrebate":49099,"Ġcreeps":49100,"ĠSpan":49101,"ĠPainter":49102,"ĠKira":49103,"ĠAmos":49104,"ĠCorvette":49105,"Consumer":49106,"ĠRecover":49107,"cki":49108,"Ġpesky":49109,"ĠInvention":49110,"Companies":49111,"Ġchallengers":49112,"ademic":49113,"ĠUkrainians":49114,"ĠNeurolog":49115,"ĠForsaken":49116,"Ġentrants":49117,"Ġembattled":49118,"Ġdefunct":49119,"ĠGlacier":49120,"Ġpoisons":49121,"ĠHorses":49122,"makes":49123,"ĠDirt":49124,"Ġ423":49125,"hhh":49126,"ĠTransformation":49127,"QUIRE":49128,"..................":49129,"Ġtraveller":49130,"ĠSexy":49131,"ĠKern":49132,"ipolar":49133,"Ġransomware":49134,"oooooooooooooooo":49135,"Ec":49136,"ruby":49137,"Professional":49138,"ĠOutbreak":49139,"argument":49140,"Grey":49141,"ĠFifa":49142,"ĠCHO":49143,"ĠFORM":49144,"ĠAmtrak":49145,"-[":49146,"Ġcradle":49147,"Ġantioxidants":49148,"ãģ®å®":49149,"736":49150,"ĠNASL":49151,"ĠContributions":49152,"Indiana":49153,"ĠSTEP":49154,"CSS":49155,"Ġsalient":49156,"Ġallocations":49157,"yrights":49158,"Ġmashed":49159,"ĠCutter":49160,"Sexual":49161,"Ġpounded":49162,"Ġfanbase":49163,"Ġcasc":49164,"ĠTransparency":49165,"Ġanalytic":49166,"ĠSummoner":49167,"×ŀ":49168,"ĠADC":49169,"detail":49170,"Ġvanquished":49171,"Ġcrabs":49172,"arie":49173,"Destroy":49174,"ĠSack":49175,"Ġtransistor":49176,"Alabama":49177,"ĠKoen":49178,"ĠFisheries":49179,"cone":49180,"Ġannexed":49181,"ĠMGM":49182,"esa":49183,"Ġfaked":49184,"ĠCongratulations":49185,"Ġhindered":49186,"Ġcorrectional":49187,"ĠITV":49188,"leeve":49189,"Ġinappropriately":49190,"licks":49191,"Ġtrespass":49192,"Ġpaws":49193,"Ġnegotiator":49194,"ĠChristensen":49195,"limits":49196,"ĠDianne":49197,"Ġelegance":49198,"ĠContracts":49199,"anke":49200,"Obj":49201,"Ġvigilance":49202,"Ġcastles":49203,"ĠNAD":49204,"ĠHolo":49205,"Ġemphatically":49206,"ĠTitus":49207,"ĠServing":49208,"ĠRichie":49209,"ĠPigs":49210,"568":49211,"Ġanimosity":49212,"ĠAttributes":49213,"ĠUriel":49214,"MQ":49215,"myra":49216,"ĠApplicant":49217,"Ġpsychiatrists":49218,"ĠVij":49219,"ĠAbby":49220,"agree":49221,"Push":49222,"ĠkWh":49223,"hiba":49224,"Ġincite":49225,"ĠWeasley":49226,"ĠTaxi":49227,"ministic":49228,"hyper":49229,"ĠFarn":49230,"Ġ601":49231,"ĠNationwide":49232,"Fake":49233,"952":49234,"Ġmaize":49235,"Ġinteracted":49236,"Ġtransitioned":49237,"Ġparasitic":49238,"Ġharmonic":49239,"Ġdecaying":49240,"Ġbaseless":49241,"nsics":49242,"Ġtranspired":49243,"Ġabundantly":49244,"ĠForensic":49245,"Ġtreadmill":49246,"ĠJav":49247,"aband":49248,"Ġsshd":49249,"Ġfrontman":49250,"ĠJakarta":49251,"oller":49252,"drops":49253,"ĠSERVICES":49254,"romptu":49255,"ophical":49256,"hospital":49257,"bledon":49258,"645":49259,"Ġmidrange":49260,"ĠEVENT":49261,"culated":49262,"rawled":49263,"Ġperched":49264,"Ġoverboard":49265,"ĠPeel":49266,"ĠPwr":49267,"ĠCarth":49268,"ĠCOMPLE":49269,"coe":49270,"shall":49271,"Ġdeterrence":49272,"METHOD":49273,"ĠAbsent":49274,"MEN":49275,"Ġsill":49276,"ĠLEVEL":49277,"York":49278,"Ġsinners":49279,"ĠOPEC":49280,"ĠNur":49281,"ĠDesigns":49282,"selection":49283,"Ġunworthy":49284,"CHA":49285,"Ġstrengthens":49286,"883":49287,"edly":49288,"Ġslicing":49289,"Ġmalnutrition":49290,"Ġfilmmaking":49291,"ĠPolk":49292,"urated":49293,"Ġ421":49294,"breakers":49295,"!'\"":49296,"Ġwetlands":49297,"ĠDiscrimination":49298,"Ġallowable":49299,"Ġsteered":49300,"ĠSicily":49301,"SAM":49302,"Ġmustache":49303,"Ġmids":49304,"Ġclipped":49305,"Ġcirculate":49306,"Ġbrittle":49307,"ĠBuildings":49308,"raised":49309,"ĠRoundup":49310,"Ġwealthier":49311,"Ġoverwrite":49312,"Ġoverpowered":49313,"ĠGerrard":49314,"sites":49315,"PDATED":49316,"Ġacutely":49317,"ĠGamble":49318,"Ġpim":49319,"ĠKus":49320,"Typically":49321,"Deploy":49322,"ĠMoroccan":49323,"potion":49324,"combe":49325,"Ġvigilante":49326,"Ġ363":49327,"Stew":49328,"ĠBagg":49329,"Ġresided":49330,"ĠSpo":49331,"Ġremnant":49332,"Ġemptiness":49333,"brainer":49334,"Ġoutpatient":49335,"priority":49336,"Ġleptin":49337,"ĠPayton":49338,"ĠGleaming":49339,"ĠShed":49340,"ĠPolo":49341,"ĠMormonism":49342,"restricted":49343,"arlane":49344,"wx":49345,"Ġcreatine":49346,"ĠAnon":49347,"ĠSTUD":49348,"ĠJUL":49349,"ĠTee":49350,"528":49351,"089":49352,"Ġhatched":49353,"Dispatch":49354,"ĠComposite":49355,"Ġ451":49356,"puff":49357,"ĠXCOM":49358,"ĠOrn":49359,"ĠTHANK":49360,"ENDED":49361,"ĠAsheville":49362,"ĠÃľ":49363,"Ġmango":49364,"ĠSlightly":49365,"worldly":49366,"ĠWander":49367,"ĠExpand":49368,"ĠChr":49369,"Mist":49370,"Ġorthodoxy":49371,"ĠUNESCO":49372,"regate":49373,"Elsewhere":49374,"kie":49375,"irled":49376,"Ġtopple":49377,"Ġadoptive":49378,"ĠLegs":49379,"dress":49380,"ĠSagan":49381,"bare":49382,"ĠGlou":49383,"Crunch":49384,"Ġhelpers":49385,"Ġchronically":49386,"ĠHuma":49387,"10000":49388,"Ġaccommodating":49389,"äºĶ":49390,"Ġwrinkles":49391,"Ġdodged":49392,"fourth":49393,"Ġprecon":49394,"Ġcompressor":49395,"ĠKare":49396,"Ġevict":49397,"ĠWarwick":49398,"imar":49399,"Ġmodernization":49400,"Ġbandwagon":49401,"Ġrefuted":49402,"Ġnetted":49403,"ĠNaples":49404,"ĠGenie":49405,"perors":49406,"Ġfielded":49407,"Ġdere":49408,"ĠParables":49409,"lees":49410,"Ġtrout":49411,"aspers":49412,"Ġnihil":49413,"Ġhappiest":49414,"Ġfloppy":49415,"ĠLoft":49416,"ĠHeard":49417,"Ġunison":49418,"Ġlug":49419,"ĠRedmond":49420,"classic":49421,"Supporters":49422,"SHIP":49423,"GMT":49424,"Ġfuelled":49425,"çIJ":49426,"Ġdd":49427,"ĠEminem":49428,"Ġ1897":49429,"NYSE":49430,"Ġsecretaries":49431,"ĠFIA":49432,"ĠCanaveral":49433,"Favorite":49434,"Ġpomp":49435,"Ġdetainee":49436,"ership":49437,"aimon":49438,"iour":49439,"ĠApex":49440,"Ġplantations":49441,"amia":49442,"acion":49443,"Rust":49444,"Ġtowed":49445,"ĠTruly":49446,"577":49447,"Ġsheltered":49448,"rider":49449,"Wo":49450,"Ġlair":49451,"ĠIntelligent":49452,"improve":49453,"matically":49454,"Ġetiquette":49455,"adra":49456,"allo":49457,"ĠJuno":49458,"anything":49459,"ĠStruggle":49460,"ĠPredict":49461,"ĠGrimes":49462,"ĠAMERICA":49463,"ctx":49464,"ĠSituation":49465,"WOOD":49466,"Ġsoluble":49467,"meier":49468,"Ġintolerable":49469,"angering":49470,"Ġuninterrupted":49471,"Ġtooltip":49472,"Ġinterrogated":49473,"Ġgunned":49474,"ĠSneak":49475,"æŃ¦":49476,"Ġtether":49477,"Ġcrumble":49478,"Lens":49479,"Ġclustered":49480,"ĠSyl":49481,"ĠHasan":49482,"Ġdystopian":49483,"wana":49484,"Ġjoystick":49485,"ĠThib":49486,"ammu":49487,"Tomorrow":49488,"546":49489,"Ġovercame":49490,"Ġminimized":49491,"ceptor":49492,"Runner":49493,"ENGTH":49494,"ĠBrenda":49495,"ĠAchievements":49496,"Ġtorches":49497,"Ġrapport":49498,"ĠInvestigator":49499,"ĠHandling":49500,"relation":49501,"grey":49502,"815":49503,"Ġkcal":49504,"ĠCommands":49505,"dq":49506,"Ġcurls":49507,"Ġbearer":49508,"Ġcynicism":49509,"itri":49510,"ĠUseful":49511,"Bee":49512,"DCS":49513,"Ġabras":49514,"Pract":49515,"BILITIES":49516,"712":49517,"Ġdebugger":49518,"Ġdebtor":49519,"ĠLia":49520,"ĠKers":49521,"Ġexacerbate":49522,"ĠStacy":49523,"ĠBland":49524,"ĠScenes":49525,"Ġbranching":49526,"âĸĪâĸĪâĸĪâĸĪâĸĪâĸĪâĸĪâĸĪ":49527,"apeake":49528,"Ġsalsa":49529,"Ġmishand":49530,"ĠKonami":49531,"ĠNib":49532,"Ġanecdote":49533,"Ġagreeable":49534,"Ïī":49535,"ĠNathaniel":49536,"ĠHeisman":49537,"ĠBeware":49538,"Ġ1886":49539,"spective":49540,"691":49541,"522":49542,"Ġinhibits":49543,"Ġhashing":49544,"Ġ1889":49545,"å°Ĩ":49546,"vich":49547,"Pure":49548,"Ġsolidly":49549,"Ġaspirin":49550,"imaru":49551,"Ġstreetcar":49552,"ĠUCS":49553,"ĠJudd":49554,"Ġflashbacks":49555,"pins":49556,"Ġ1440":49557,"ĠUNHCR":49558,"ĠSymptoms":49559,"TIT":49560,"538":49561,"Fra":49562,"%);":49563,"Ġooz":49564,"Ġcurfew":49565,"Ġcalmed":49566,"Ġparticipates":49567,"TeX":49568,"Ġnonsensical":49569,"Ġfullback":49570,"ĠDeL":49571,"monkey":49572,"hari":49573,"Ġmetabolites":49574,"Ġlooted":49575,"ĠALWAYS":49576,"ĠBCC":49577,"Lt":49578,"ochet":49579,"Bone":49580,"Ġvetoed":49581,"Ġgcc":49582,"ĠCLICK":49583,"Ġ1888":49584,"saf":49585,"Ġstiffness":49586,"Ġlowly":49587,"ĠGeh":49588,"verson":49589,"orset":49590,"Ġunforeseen":49591,"Ġanesthesia":49592,"ĠOptical":49593,"Ġreconstructed":49594,"ĠTup":49595,"shows":49596,"NEWS":49597,"ĠNewspaper":49598,"ĠASA":49599,"tera":49600,"Numbers":49601,"Ġinexplicable":49602,"×ij":49603,"Ġhardness":49604,"untarily":49605,"ĠAcer":49606,"gradient":49607,"ARDIS":49608,"Ġwoodland":49609,"Ġmetaphors":49610,"ĠWembley":49611,"ĠPavel":49612,"philis":49613,"Ġrewriting":49614,"Ġperceptual":49615,"Ġ1070":49616,"worms":49617,"ĠDowns":49618,"Ġunsurprisingly":49619,"Ġtagging":49620,"flame":49621,"Ġlitres":49622,"Ġbounces":49623,"ĠBabe":49624,"shut":49625,"Ġoverdoses":49626,"ĠSheila":49627,"ĠChau":49628,"ĠBless":49629,"Capture":49630,"ĠSignificant":49631,"ĠScion":49632,"Ġ389":49633,"ĠMcH":49634,"ĠTitanium":49635,"ĠMeal":49636,"ameda":49637,"agents":49638,"aggressive":49639,"Billy":49640,"763":49641,"ĠSaying":49642,"DERR":49643,"itone":49644,"Collins":49645,"Bound":49646,"Ġbolted":49647,"ĠDMCA":49648,"953":49649,"Ġuniqueness":49650,"Ġepigen":49651,"unci":49652,"antam":49653,"Ġreckoning":49654,"chairs":49655,"OGR":49656,"ĠSenegal":49657,"Ġ1862":49658,"relevant":49659,"Ġ¯":49660,"Ġpharmacies":49661,"ĠGeral":49662,"vier":49663,"Yan":49664,"ORPG":49665,"Ġrabid":49666,"bending":49667,"ĠUNITED":49668,"Ġ465":49669,"Assembly":49670,"Ġweep":49671,"Ġbehest":49672,"ĠMothers":49673,"ĠJace":49674,"hid":49675,"Ġwhirlwind":49676,"ĠUNIVERS":49677,"Ġutopian":49678,"Ġkidnap":49679,"Philipp":49680,"Kin":49681,"893":49682,"Ġlivestream":49683,"ĠMISS":49684,"Ġsubversive":49685,"ĠTechniques":49686,"ĠJUSTICE":49687,"ĠBASE":49688,"Ġ387":49689,"Ġassailants":49690,"ĠHardcore":49691,"Ġsprinkled":49692,"ĠPse":49693,"éļ":49694,"printed":49695,"ĠHau":49696,"ORGE":49697,"ĠTOUR":49698,"Ġlaced":49699,"Ġitch":49700,"Giving":49701,"Ġported":49702,"781":49703,"////////////////////////////////":49704,"breeding":49705,"Ġlogger":49706,"ĠHOL":49707,"innie":49708,"Firstly":49709,"Ġembryonic":49710,"Ġdelegated":49711,"pai":49712,"OIL":49713,"Ġcentrally":49714,"ĠRx":49715,"ĠScouting":49716,"Dutch":49717,"Ġhereditary":49718,"ĠCruiser":49719,"sat":49720,"529":49721,"ĠMarriott":49722,"othermal":49723,"Ġprohibitions":49724,"Earn":49725,"ĠStab":49726,"ĠColleges":49727,"ĠBelief":49728,"stretched":49729,"ĠLH":49730,"ĠEntityItem":49731,"CIA":49732,"Ġunrem":49733,"Ġlaureate":49734,"Ġdenominations":49735,"summary":49736,"hler":49737,"Spect":49738,"ĠKlaus":49739,"ĠBeans":49740,"Ġinsur":49741,"ĠPAX":49742,"Ġfielder":49743,"ĠVet":49744,"ĠSparrow":49745,"zie":49746,"ĠSQ":49747,"ĠMondays":49748,"ĠOffline":49749,"ĠLerner":49750,"ĠExtensions":49751,"Ireland":49752,"Ġpatronage":49753,"Ġcontrasted":49754,"ĠMania":49755,"hirt":49756,"Moscow":49757,"Ġcondemns":49758,"ĠAnge":49759,"Ġcomposing":49760,"ĠPepe":49761,"ĠPaddock":49762,"Ġheterogeneity":49763,"Ġideologically":49764,"Ġfishes":49765,"Ġcursing":49766,"ĠRutherford":49767,"ĠFloating":49768,"ĠAmelia":49769,"Tea":49770,"Synopsis":49771,"Ġstunts":49772,"Ġbead":49773,"Ġstocking":49774,"ĠMILL":49775,"obook":49776,"massive":49777,"\\<":49778,"Ġhump":49779,"ĠPreferences":49780,"EngineDebug":49781,"geist":49782,"ĠNieto":49783,"omever":49784,"ishy":49785,"evaluate":49786,"colonial":49787,"Alternative":49788,"ĠGoPro":49789,"ĠVortex":49790,"ĠNETWORK":49791,"ansky":49792,"Secure":49793,"ĠThrust":49794,"Snake":49795,"Ġparcels":49796,"Ġsamurai":49797,"Ġactresses":49798,"Nap":49799,"MF":49800,"iferation":49801,"Beer":49802,"523":49803,"ĠIly":49804,"ointment":49805,"Ping":49806,"Ġstriped":49807,"ĠMellon":49808,"ossession":49809,"Ġneutron":49810,"endium":49811,"Ġaph":49812,"ĠFlavoring":49813,"Ġ383":49814,"Ġresponsiveness":49815,"ĠJindal":49816,"ĠHitchcock":49817,"Denver":49818,"ĠDRAGON":49819,"smanship":49820,"ĠDupl":49821,"Ġsly":49822,"Ġwebcam":49823,"ĠTwain":49824,"ĠDarling":49825,"iliate":49826,"consumer":49827,"DIT":49828,"Ġnamesake":49829,"Ġunorthodox":49830,"Ġfuner":49831,"ĠPLoS":49832,"ĠCONTROL":49833,"ozyg":49834,"oglobin":49835,"FACE":49836,"ERG":49837,"ĠDia":49838,"ĠFiesta":49839,"cele":49840,"034":49841,"Ġenclave":49842,"âĸ¬âĸ¬":49843,"onement":49844,"alist":49845,"Mand":49846,"Ġhomegrown":49847,"ĠFancy":49848,"Ġconceptions":49849,"ĠContains":49850,"ureen":49851,"Ġreiterate":49852,"Ġmeager":49853,"Ġinstallments":49854,"Spawn":49855,"627":49856,"Ġphotoc":49857,"ĠCabrera":49858,"ĠRosenthal":49859,"ĠLansing":49860,"isner":49861,"Ġinvests":49862,"ĠUFOs":49863,"EXP":49864,"Hardware":49865,"Ġtragically":49866,"Ġconcedes":49867,"ieft":49868,"cham":49869,"borgh":49870,"ĠSchr":49871,"ĠMelanie":49872,"ĠHoy":49873,"Ġvisitation":49874,"Ġidiosyncr":49875,"Ġfractions":49876,"Ġforeskin":49877,"obos":49878,"Ġpoaching":49879,"ĠVIEW":49880,"Ġstimulates":49881,"ĠGork":49882,"canon":49883,"MIC":49884,"ĠNemesis":49885,"ĠIndra":49886,"ĠDMV":49887,"Ġ529":49888,"Ġinspecting":49889,"Ġgrandma":49890,"ĠWhedon":49891,"ĠShant":49892,"ĠPurg":49893,"ikan":49894,"ĠTeg":49895,"ĠCLR":49896,"zac":49897,"Victoria":49898,"ĠVerify":49899,"ionics":49900,"Ġpartying":49901,"ĠMou":49902,"colour":49903,"Ġtestimonies":49904,"lations":49905,"Ġpressuring":49906,"hiro":49907,"acers":49908,"Ġfid":49909,"angler":49910,"ĠCSI":49911,"Ġhereafter":49912,"Ġdissidents":49913,"reporting":49914,"iphany":49915,"chev":49916,"Ġsolitude":49917,"Ġlobe":49918,"Ġindis":49919,"Ġcredential":49920,"recent":49921,"adult":49922,"ĠNirvana":49923,"ĠFranchise":49924,"Layer":49925,"Hyp":49926,"ĠBerkshire":49927,"Ġwills":49928,"tif":49929,"Ġtotem":49930,"ĠJudah":49931,"repair":49932,"Instant":49933,"548":49934,"Ġembassies":49935,"Ġbottleneck":49936,"Ġbount":49937,"Ġtypew":49938,"ĠAlvin":49939,"jing":49940,"imilar":49941,"Rush":49942,"Ġbrim":49943,"ĠHELP":49944,"Aim":49945,"]'":49946,"Ġpassively":49947,"Ġbounded":49948,"ĠRated":49949,"Ġcriminality":49950,"Ġbiomark":49951,"Ġdispatcher":49952,"ĠTowards":49953,"Ġ+++":49954,"righteous":49955,"frog":49956,"ĠPanc":49957,"Carter":49958,"032":49959,"æ©Ł":49960,"Ġultraviolet":49961,"ĠLicensed":49962,"ĠTata":49963,"ĠBlessing":49964,"ĠGAM":49965,"Ġchemically":49966,"ĠSeaf":49967,"ĠRELE":49968,"ĠMercenary":49969,"capitalist":49970,"Ġformulations":49971,"Ġannihilation":49972,"ĠVerb":49973,"ĠArgon":49974,"Ġunloaded":49975,"Ġmorphed":49976,"Ġconquering":49977,"backer":49978,"IELD":49979,"Ġthefts":49980,"Ġfrontrunner":49981,"ĠRoyale":49982,"ĠFundamental":49983,"elight":49984,"Chip":49985,"necessary":49986,"ayn":49987,"ĠSlip":49988,"Ġ448":49989,"cerned":49990,"Pause":49991,"Ġshockingly":49992,"ĠABV":49993,"Ġcomposure":49994,"733":49995,"ĠMotorsport":49996,"ahime":49997,"Murray":49998,"Mach":49999,"Ġgrids":50000,"Ġdebian":50001,"Ġfurthermore":50002,"Ġdexterity":50003,"ĠCollections":50004,"oslov":50005,"ilage":50006,"bj":50007,"ĠMonteneg":50008,"ĠstrutConnector":50009,"Ġmassacres":50010,"Ġbriefs":50011,"fetched":50012,"uvian":50013,"olition":50014,"Failure":50015,"emonic":50016,"Ġflared":50017,"Ġclaimant":50018,"Ġcures":50019,"Ġgiveaways":50020,"ĠSubstance":50021,"alions":50022,"Ġcringe":50023,"ĠKul":50024,"Ġaristocracy":50025,"ĠUlster":50026,"olated":50027,"housing":50028,"ĠMIS":50029,"Ġglared":50030,"ĠWilhelm":50031,"needs":50032,"lambda":50033,"builders":50034,"ĠVIS":50035,"Ġradiator":50036,"ĠGhostbusters":50037,"Ġ436":50038,"actual":50039,"Ġherds":50040,"ça":50041,"watching":50042,"Ġcountering":50043,"Charge":50044,"Ġcharred":50045,"Ġwarheads":50046,"Ġiodine":50047,"ĠMacy":50048,"041":50049,"Ġdepartures":50050,"ĠSins":50051,"Ġdyed":50052,"ĠConcepts":50053,"gado":50054,"713":50055,"Ġquotations":50056,"Ġgist":50057,"ĠChristy":50058,"Ġantigen":50059,"ĠHemp":50060,"ĠDrawn":50061,"ĠBarg":50062,"ezvous":50063,"Ġpaternity":50064,"Ġardu":50065,"ĠAnchorage":50066,"ĠRik":50067,"Ġoverloaded":50068,"ĠUsername":50069,"ĠTammy":50070,"ĠNau":50071,"ĠCellular":50072,"Ġwaning":50073,"Ġrodent":50074,"ĠWorcester":50075,"ilts":50076,"ĠTad":50077,"Ġdwellings":50078,"Ġbullish":50079,"431":50080,"Ġretaliate":50081,"Ġmigraine":50082,"ĠChevron":50083,"CHECK":50084,"Ġdonkey":50085,"crim":50086,"SPA":50087,"ĠAnalog":50088,"Ġmarquee":50089,"ĠHaas":50090,"Bir":50091,"ĠGDDR":50092,"ĠDownloads":50093,"Ġwillpower":50094,"ĠForth":50095,"ĠRecorded":50096,"Ġimpossibility":50097,"ĠLogged":50098,"ĠFranks":50099,"ĠRatt":50100,"initions":50101,"Ġcleaners":50102,"Ġsorely":50103,"Ġflickering":50104,"ĠExamination":50105,"catching":50106,"alloween":50107,"Msg":50108,"Ġdunno":50109,"Fa":50110,"Ġdysph":50111,"crazy":50112,".''.":50113,"Ġmainline":50114,"Ġcs":50115,"Ġptr":50116,"ĠWally":50117,"igun":50118,"951":50119,"ĠBigfoot":50120,"fights":50121,"Ġretrieving":50122,"Jr":50123,"Ġduplication":50124,"ĠExplan":50125,"Ġrelational":50126,"Ġquaint":50127,"Ġbiscuits":50128,"Ġado":50129,"Ġshudder":50130,"Ġantidote":50131,"blooded":50132,"ksh":50133,"Ġsauces":50134,"Ġreinvest":50135,"Ġdispensary":50136,"ĠDiver":50137,"Ġ9000":50138,"student":50139,"Ġinsepar":50140,"escap":50141,"Ġtoddlers":50142,"ĠGPIO":50143,"ĠAssignment":50144,"headers":50145,"Ġlackluster":50146,"Ġaback":50147,"956":50148,"Ġtoolbar":50149,"745":50150,"Ġoust":50151,"Ġcontemplation":50152,"ĠPRESIDENT":50153,"Ġ458":50154,"======":50155,"Ġguaranteeing":50156,"ĠHeist":50157,"ĠCannes":50158,"Ͻ":50159,"Ġcollaborator":50160,"ĠAmp":50161,"Ġgou":50162,"ĠSHALL":50163,"stories":50164,"783":50165,"Ġmobilized":50166,"Ġbrood":50167,"ĠLU":50168,"ĠðŁij":50169,"Ġrefin":50170,"ĠAnthropology":50171,"vind":50172,"illi":50173,"Ġwarranties":50174,"ĠBabel":50175,"Ġswath":50176,"Ġcaches":50177,"Ġantagonists":50178,"artifacts":50179,"Ġhotly":50180,"ĠStarts":50181,"ĠGö":50182,"zag":50183,"!!!!!":50184,"Ġscourge":50185,"Ġconspiring":50186,"ruits":50187,"reverse":50188,"ĠSheen":50189,"ĠJesuit":50190,"ĠGiovanni":50191,"adies":50192,"Ġbuttocks":50193,"earcher":50194,"acan":50195,"Ġvolleyball":50196,"Ġshrouded":50197,"Ġscoreboard":50198,"bats":50199,"ĠIPM":50200,"Ġasses":50201,"Ġderegulation":50202,"ĠTelegram":50203,"ĠReboot":50204,"Ġ7000":50205,"ĠCanary":50206,"Ġkernels":50207,"ĠFrançois":50208,"ĠDuff":50209,"ĠPon":50210,"ĠLeica":50211,"ĠGarmin":50212,"Ġorphans":50213,"ĠClaudia":50214,"Ġcalendars":50215,"ĠLeilan":50216,"ento":50217,"Rocket":50218,"Ġbrunch":50219,"ĠHawking":50220,"ainers":50221,"Ġsensibilities":50222,"ĠkW":50223,"ĠKand":50224,"Ġreclaimed":50225,"Ġinterestingly":50226,"ש":50227,"romy":50228,"JM":50229,"ĠEnhancement":50230,"bush":50231,"Skip":50232,"Ġrappers":50233,"Ġgazing":50234,"pedia":50235,"athlon":50236,"Revolution":50237,"Ġsnipers":50238,"Ġreverted":50239,"Ġconglomerate":50240,"Terry":50241,"794":50242,"Ġharsher":50243,"Ġdesolate":50244,"ĠHitman":50245,"Commission":50246,"Ġ(/":50247,"â̦.\"":50248,"Compar":50249,"Ġamplification":50250,"ominated":50251,"Ġregress":50252,"ĠCollider":50253,"Ġinformants":50254,"Ġgazed":50255,"<|endoftext|>":50256} \ No newline at end of file diff --git a/whisper/assets/mel_filters.npz b/whisper/assets/mel_filters.npz new file mode 100644 index 0000000000000000000000000000000000000000..9793b084efeb26bf24cb2ba1f30d0989b2c94aa1 --- /dev/null +++ b/whisper/assets/mel_filters.npz @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:dd2cc75e70e36fcbdd8ffbc2499062f30094093e6bf2cbafa9859f59972b420b +size 2048 diff --git a/whisper/assets/multilingual/added_tokens.json b/whisper/assets/multilingual/added_tokens.json new file mode 100644 index 0000000000000000000000000000000000000000..5c509999d68b2eb119d8a4d1218376910beac339 --- /dev/null +++ b/whisper/assets/multilingual/added_tokens.json @@ -0,0 +1 @@ +{"<|endoftext|>": 50257} diff --git a/whisper/assets/multilingual/merges.txt b/whisper/assets/multilingual/merges.txt new file mode 100644 index 0000000000000000000000000000000000000000..afde9f4fa8bcf6d4762c5780977825666dc49eac --- /dev/null +++ b/whisper/assets/multilingual/merges.txt @@ -0,0 +1,50000 @@ +Ġ t +Ġ a +Ġt h +i n +e r +Ġ w +Ġ s +o u +Ġth e +r e +o n +a t +e n +Ġ c +i t +i s +Ġ b +n d +Ġ d +Ġ m +Ġ h +Ġ o +in g +e s +Ġ p +Ġt o +a n +Ġ f +o r +l l +Ġ I +Ġ l +Ġ y +a r +Ġ g +Ġy ou +e d +Ġa nd +Ġ in +Ġo f +a s +Ġ n +o m +i c +Ġth at +u s +e t +v e +a l +o w +l e +Ġ is +Ġ e +Ġ it +o t +' s +Ġb e +i on +Ġ T +Ġw h +Ġ A +en t +Ġ S +Ġ re +a y +Ġw e +Ġ on +er e +Ġh a +u t +a c +i d +i g +o s +k e +v er +i m +Ġ Ð +ĠT h +a m +a ll +Ġf or +e l +c h +r o +Ġth is +Ġs t +Ġ W +Ġ u +a d +ou t +i r +l d +c t +Ġ k +i f +Ġg o +. . +Ð ¾ +it h +l y +h t +q u +Ġ - +Ġd o +Ġ j +Ġha ve +Ġ B +Ġa n +Ġw ith +Ġa re +Ġ r +Ġd e +Ġs e +Ġs o +Ġ v +s t +i ll +u r +Ġl i +Ġ M +es t +o d +all y +' t +us t +Ġa s +Ġ C +c e +Ġm e +Ð ° +Ð µ +i l +Ġ H +Ġw as +t er +t h +Ġc an +an t +Ġc om +ou r +ig ht +Ġ Y +at ion +ĠA nd +o l +Ġs h +Ñ Ĥ +o p +s e +Ġn ot +ĠS o +Ġn e +u n +Ġa b +Ġli ke +Ġa t +Ġ D +i e +Ġh e +Ġc on +Ġc h +o re +Ġa l +Ġo r +Ġ qu +Ġ O +om e +r a +u l +Ġ N +p p +Ġyou r +ou ld +Ġ P +Ġf r +g e +er s +' re +Ð ¸ +Ġthe y +Ġwh at +us e +Ġa ll +ĠTh e +Ġ L +es s +e m +Ġk n +Ġj ust +ar t +Ġp ro +ver y +u m +Ġl o +Ġ ì +Ġm y +o k +Ġe x +a b +Ġth ere +Ġb ut +Ġkn ow +Ġs u +Ġ G +Ñ ģ +Ġ E +Ġm a +о Ð +Ġ en +Ġab out +ĠI t +is t +Ġw or +r i +in d +Ġon e +at e +a nd +in k +Ġl e +or t +' m +Ġ F +ic h +Ñ Ģ +id e +Ġg et +Ġ out +.. . +Ġw ill +ã ģ +i ve +Ð ½ +Ġfr om +a in +ĠW e +Ġu p +p e +re s +c a +Ġ R +Ġ if +Ġp l +Ġd on +ac k +Ġ 1 +Ġ " +Ġt r +Ġ us +ĠW h +it y +Ġ J +ĠY ou +Ġh ere +h er +Ġs ome +ou g +a k +ar d +Ġgo ing +Ġu n +m ent +Ġth ink +Ġp e +en d +Ġ ( +ca use +Ġt im +as t +à © +Ġ our +Ġw ant +am e +i es +Ġ ë +u d +in e +Ġre ally +Ġt e +Ġse e +c i +Ġb y +s o +u re +os e +Ġ [ +a re +Ġm ore +a h +on e +c k +op le +а Ð +Ġthe n +Ġth ing +Ġthe m +v en +ou nd +os t +on g +e ct +Ġr ight +a g +Ġin t +Ġpe ople +Ġwh en +ou s +p l +Ġtim e +Ġ im +Ġwh o +Ġ 2 +a p +Ġbe cause +h ing +Ġn o +ic e +Ġlo ok +Ġh as +Ġw ould +Ġh ow +ac t +Ġf e +n t +oug h +Ġp r +ĠB ut +Ġs ay +Ñ ĥ +Ġn ow +Ġm an +Ġ very +Ġwor k +i z +Ġ K +i v +it t +Ġa r +e p +Ġc l +Ġwh ich +Ġc o +an s +' ve +Ġs a +f f +' ll +Ġan y +Ġa ct +Ġy e +b er +ac h +a ge +p er +Ġal so +f er +Ġthe se +Ġa d +е Ð +th er +ac e +ic k +a ke +re at +i re +u e +Ġa g +Ġ U +u ch +ion s +r y +0 0 +n a +Ġd id +Ġqu e +Ġha d +Ġe very +ĠH e +Ġl a +Ġw ay +Ġs p +b le +ĠTh is +as s +Ġthe ir +it e +Ġne ed +Ġp art +Ġw ere +Ġb ack +i p +ow n +om et +b e +as e +Ġma ke +ir st +i a +en ce +an g +an k +Ġg ot +Ġp re +Ġcon t +Ġo ther +p t +ĠTh at +o g +Ġgo od +Ġint o +al k +Ġbe en +Ġa m +Ġo ver +u ally +Ġ â +ì Ŀ +Ġu nd +h e +w ay +Ġg r +Ñ Į +Ġd if +Ġp er +Ñ ı +ĠI n +Ġt w +on d +ar s +in t +or m +Ġl ot +Ġwh ere +Ġ à +Ġ V +Ġs omet +Ð » +en s +Ġg u +Ġa c +u g +Ñ ĭ +Ä ± +Ġf irst +re e +Ġh is +itt le +Ġim p +Ġm o +a v +Ġl ittle +ĠWh at +Ġm uch +Ġ z +Ġ ê +ab le +ĠÐ ¿ +Ġp o +Ġcom p +n e +Ġd is +Ġl et +an ce +Ġh er +Ġthing s +Ġst art +ul t +Ġa pp +Ġre s +Ġf o +Ġc ould +Ġin ter +Ġth ose +Ġd es +Ġwe ll +Ġtw o +Ġk ind +x t +res s +el y +à ¤ +Ġb r +Ġth r +ĠÐ ² +Ġ i +is h +Ġdif fer +Ġ ro +ĠS t +Ġsomet hing +Ġt ake +Ġb o +y s +Ġsh e +Ġt alk +l o +Ñ ĩ +Ġe ven +Ð º +ã Ģ +ĠÐ ½ +Ġb u +ĠI f +Ġd own +ĠC h +ad e +ation s +Ġ use +or d +Ġof f +Ġact ually +Ġs pe +d u +at ed +at er +os s +n ing +à ¼ +Ġdo es +Ġ Ñģ +Ġne w +Ġb et +ve l +c ess +p le +Ġha pp +t ing +on na +Ġ es +Ġd ay +Ġon ly +ig n +k ay +s el +ent s +ou nt +i ld +i le +Ġs c +Ġh im +Ġag ain +v ing +Ġg onna +Ġcom m +Ġh el +ot her +Ġ ke +ic al +Ġ 3 +Ġe l +Ġthr ough +Ġcom e +ar k +d ay +i er +à ³ +Ġth an +ĠThe y +Ġm ay +Ġs er +í ķ +Ġc all +Ġdiffer ent +Ġsh ould +ĠTh ere +ar y +ĠN ow +ã Ĥ +th ing +w e +or y +f ter +Ġp ut +or s +i al +ë ĭ +Ġund er +Ġin c +ĠY e +u b +f orm +Ġv ide +à ¸ +ver s +Ġfe el +à ¡ +od y +f t +f ore +Ġe m +g et +Ġsa id +it ion +Ġre c +i ous +at ch +Ġtr y +Ġhel p +Ġsh ow +Ð ´ +Ġb it +u ll +Ð ² +ÑĤ о +g r +Ġpl ay +if e +a il +ĠYe ah +Ġqu est +Ġman y +Ġp ers +Ġg reat +Ã Ń +Ġ est +n g +Ġâ Ļ +t y +l a +ĠO h +Ġ × +à ® +ĠB e +ad y +Ġm ost +ct ion +ĠN o +Ġdo ing +Ġbe ing +Ġto o +c es +Ġb l +. " +Ġre m +is s +on s +> > +r u +w n +on t +i b +e ll +Ġs m +ot h +u al +Ġ >> +Ġp h +l es +o c +f ul +Ġse c +is e +Ġad d +ig h +er t +Ġs ame +â Ģ +Ġme an +Ġf ind +e k +Ġen d +- - +Ð ¼ +Ġst ill +a z +Ġ ' +Ġm in +Ġye ars +ur n +Ġar ound +sel f +Ġw r +b s +oug ht +ĠâĻ ª +Ġf l +an ge +Ġa fter +Ġpo int +m er +v ed +Ġl ong +o y +ä ¸ +Ġc r +way s +Ġs y +Ġt ra +Ġ2 0 +a ve +Ġch e +Ġ ent +Ġbe fore +p h +Ġat t +i an +i ly +Ġpers on +Ġb ig +Ġs ch +Ġre al +Ġne xt +Ġlo ve +Ġvide o +ĠL et +Ġf in +Ġma k +i ble +Ġto day +er m +ĠA l +ow er +an n +i x +Ġp ar +Ġst ud +à ¶ +Ġimp ort +t e +Ġg ive +v es +Ġd ie +Ġde c +Ġte ll +ĠÐ º +Ñģ ÑĤ +Ġwh y +ic ally +ic t +re d +Ġb as +Ġsu re +Ġbe l +at ing +Ġt ak +Ġs et +Ġl ife +Ġdid n +Ø § +o b +u nd +at h +Ġo p +ĠÐ ¾ +a it +Ġwor ld +Ġsu pp +i o +Ġc our +ĠÐ ¸ +w ard +е н +Ġal ways +u p +Ġha nd +ĠH ow +ci al +Ġcon s +Ġ Ñ +Ġin d +Ġ 4 +ĠA s +Ġf un +j ect +Ġimport ant +Ġs ur +e w +at es +Ġ 5 +Ġd i +Ġm ade +Ġin s +Ġas k +Ġ et +Ġn um +Ġc ar +ĠO kay +Ġs im +i k +Ġl ast +ĠG o +Ġm us +Ġre l +ul ar +´ ì +ĠWe ll +pe ct +ĠTh ank +Ġth ree +à £ +ã ĥ +Ġin v +Ġg en +l ic +Ġhapp en +ë Ĭ +i en +e ver +оР² +Ġst r +ĠA ll +Ġin st +Ġâ Ģ +Ġde f +Ġs l +Ġm ight +un g +Ġye ar +Ġo wn +Ġke ep +b ody +d er +Ġ ÑĤ +ĠÐ ´ +Ġan other +Ġm od +Ġe v +Ġgu ys +Ġab le +ã o +qu e +id ent +ĠY es +Ġit s +Ġpl ace +Ġpro du +ar n +ĠÐ ¼ +Ġre p +Ġex per +Ġf am +it ies +if ic +Ġh igh +i ed +o ol +ie w +е ÑĤ +re n +Ġdon e +Ġ ... +ëĬ Ķ +st em +ĠS e +Ġbet ter +c ome +Ġd el +Ġt y +Ġu m +Ġh o +ĠA n +Ġm on +ing s +Ġs k +Ġo b +c om +ble m +op e +st and +' d +ment s +Ġe le +ĠI s +Ġd a +Ġre g +le ase +i ke +al s +iz e +ê ° +Ġc are +Ġne ver +ìĿ ´ +es e +Ġm et +ol og +ĠWh en +u ck +е ÑĢ +Ġ é +Ġd at +à § +Ġex am +il ity +Ġd et +c ri +Ġus ed +ĠD o +Ġtr ans +e g +t en +Ñ İ +c us +Ġsec ond +Ġb est +Ġh ard +Ġ ide +Ġpro blem +ê ³ +ĠU n +Ñ ħ +Ġ Î +Ġw atch +ĠS h +at ter +Ġpre t +Ġd er +Ġcour se +Å Ł +at ive +ic s +Ġquest ion +ut e +ì Ĺ +ĠF or +at her +Ġc ol +i end +Ġ í +Ġ Z +Ġdoes n +ar ch +Ġinter est +Ġp ol +Ġc or +i ence +Ġp res +Ġe ach +Ġsy stem +Ġf act +i el +ab ly +Ġ er +Ġr un +Ġì Ŀ +Ġto p +n er +Ġth ought +Ġe as +i ent +Ġc re +Ñ Ī +Ġcomm un +y e +re ady +ll ow +Ġevery thing +om m +Ġm ed +ļ Ķ +Ġc ount +it s +Ġcom pl +h ip +Ù Ħ +o ok +Ġto get +Ġtoget her +am p +Ġg ame +Ġal ready +аР» +Ġcall ed +al e +Å Ĥ +ĠM y +Ġunder stand +Ġd r +Ġm om +it ed +оР» +Ġus ing +z y +Ġnum ber +ãĢ ģ +c ed +Ġc le +н о +ëĭ ¤ +in ce +Ġlook ing +Ġpret ty +Ġpro b +ĠS he +Ġ ve +Ġget ting +Ġwe ek +Ġe ff +u ff +a ir +u es +er n +Ġ Q +ou p +ent ion +Ġs ide +оР¼ +Ġfor m +Ġb us +Ġas s +Ġ ed +as on +we en +âĢ ¦ +Ġt urn +Ġc ur +Ġco ll +Ġd ire +ĠG od +Ġ1 0 +Ġe qu +ĠÐ ± +Ġop en +Ġsu ch +ir d +аРº +Ġe ar +Ä Ļ +g an +Ġpart ic +Ġfr iend +Ġex p +Ġex t +Ġh ome +Ġw ater +ĠO n +ÑĤ ÑĮ +or k +Ġп ÑĢ +Ġmo ve +n ess +en se +h o +Ġch ar +c o +in s +Ġb oth +Ġ1 9 +Ġg ra +Ġbet ween +á » +Ġì ķ +as h +ĠR e +a i +al th +u res +em ber +Ġa v +Ġ ver +à ª +one y +Ġth ank +Ġmay be +u c +im e +ê³ ł +Ġa way +Ġn ame +ou se +Ġac c +Ġmus ic +Ġch ange +Ġp ass +g er +Ġbu ild +Ġv al +in ess +an y +Ġfe w +´ ë +t a +Ġl ist +à ¥ +Ġo ld +Ġì ŀ +Ġs ort +Ġme m +Ġc a +ce pt +Ġgen er +Ġye ah +Ġwh ile +Ġany thing +r ic +gr am +Ġe in +c y +ur ing +ĠD e +Ġp ower +Ġcom ing +Ġwor d +Ġ- - +Ġbel ie +Ġf ound +t o +Ð ¿ +Ġme ans +Ġin form +Ġ Ø +Ġ Ñĩ +Ġsm all +00 0 +Ġc ame +Ġ íķ +w h +Ġwork ing +Ġexam ple +Ġp os +Ġde p +ê ² +ä º +ot e +Ġde m +ì § +t s +Ġv ar +a ut +Ġt ri +ch n +Ġhe ad +Ġwho le +× Ļ +z e +Ġtry ing +Ġt em +Ġc ou +et s +Ġ 6 +Ġf il +vel op +Ġc ase +à ¯ +Ġprob ably +Ġo kay +Ġpl an +Ġs it +Ġsch ool +ĠTh en +¸ ë +m e +Ġpro cess +Ġf ar +Ġre ad +Ġp oss +Ġb re +Ġso l +ic ht +Ġsupp ort +ĠT o +ert ain +Ġstart ed +Ġc ap +Ġle ft +Ġdat a +Ġtim es +еР» +Ġwant ed +а н +Ġtalk ing +Ġis t +Ġha ving +um p +Ġcont in +Ġsu b +ĠÐ · +p r +ëĭ Ī +in a +Å ¼ +Ġc reat +od e +× ķ +æ ĺ +! ! +Ġt erm +is m +оР´ +ĠBe cause +Ġw ent +id er +Ġpro v +Ġch ild +Ġd en +Ġl ight +b r +³ о +o h +Ġbo ok +Ġ Ù +ut ion +ĠJ ust +en e +Ġf our +Ġv is +ê° Ģ +Ġh ope +Ġmak ing +ĠL e +ì ķ +Ġo pp +a u +Ġm oney +Ġpro gram +à ¨ +Ġst and +I N +Ġs ign +Ġle arn +à ł +ĠD on +Ġte am +Ġн а +l ud +Ġre st +ic es +æ ľ +Ġ ÑĢ +Ġa ut +Ġle ad +ation al +d e +g y +Ġn ice +Ġd as +Ġd ist +Ġh um +ĠO ne +æ Ī +Ġcom es +Ġj o +Ġc ent +Ġex pl +Ġm ark +re en +l ed +g in +ì ļĶ +Ġle vel +Ġcon f +us h +Ġde velop +Ġt est +en g +v ious +at ure +еР¼ +re t +Ġj e +Ġst uff +Ġcl ass +ow s +Ġê · +Ġs i +Ġl es +ro p +ç ļ +Ġp or +Ġw ar +ìĹ IJ +Ġevery one +Ġg e +Ġche ck +ot t +Ġs ing +Ġar t +Ġfo llow +Ġ20 1 +ĠF r +a is +ì ĸ +Î ± +å ° +Ġà ł +im es +Ġre t +Ġch ang +Ġp ub +Ġin f +Ġte chn +ad a +iv es +Ġbe h +æĺ ¯ +Ġlook s +ãĢ Ĥ +Ð · +ĠWh y +çļ Ħ +Ġen ough +Ġb ra +it ch +ä » +Ġad v +Ð ± +Ġwith out +w er +mer ic +d en +Ġcompl et +Ġide a +ter s +o ck +Ġdef in +Ġe ver +Ġg l +Ġon ce +Ġbr ing +Ġsay ing +Ġan s +Ġhe ar +n ect +Ġl ess +g o +re am +ad o +ì ŀ +Ġm ind +ent e +Ġf ull +Ġb ad +Ġw om +Ġsome one +Ġd u +Ġw on +Ġcont ro +ort un +Ġhe alth +Ġch o +ĠA r +Ġcon c +Ġinform ation +Ġst op +at t +at ely +ä ½ +Ġgr oup +Ġ Ñĥ +Ġqu ite +Ġres p +E R +ug ht +ê ¸ +m an +iz ed +ĠB r +Ġrem ember +Ġfam ily +Ġbus iness +a w +Ġspe c +Ġa u +ĠO r +Ä ħ +Ġse en +Ġl ar +Ġ 7 +g g +b ers +Ġd ra +Ġmon th +Ġsay s +Ġis s +Ġli ve +Ġl ine +Ġmom ent +Ġex c +el s +Ġs ound +Ġco ol +Ġlo c +Ġc ertain +Ġd ri +о ÑĤ +am es +Ġm ust +n y +и ÑĤ +Ġk id +Ġinc lud +ìĿ Ħ +at or +Ä Ł +h a +are d +Ġse em +Ð ¹ +ì Ħ +Ġel se +Ġì ł +ir l +Ġ 8 +Ġv o +Ġquest ions +in es +e e +æĪ ij +ü r +ĠA meric +Ġst ory +Ġser v +ver n +ag es +l and +ĠâĢ ĵ +er a +ĠC an +Ġp op +et her +Ġn a +Ġor der +Ġmak es +Ġs ince +c on +ct or +Ġth ough +Ġprodu ct +л и +Ġle g +Ġme et +al f +Ñģ Ñı +un ch +it er +o ve +×ķ × +i et +аР¼ +it al +Ġsu per +l ing +Ġp ay +Ġpar a +Ġj ob +ĠH ere +Ġs w +k s +pt ion +m a +Ġbelie ve +¬ ë +Ġw ait +оР¹ +Ġun t +Ġqu ick +h r +ĠÑ į +ĠP ro +Ġm en +à ¹ +Ġday s +Ġgo es +Ġspe ak +ĠA t +em ent +Ġm iss +Ġa w +Ġdes ign +Ġpro ject +о ÑĢ +i j +ant s +at s +ĠCh r +Ġ 9 +Ġc ut +Ġre qu +Ġн е +ĠN ot +as ter +Ġm ill +Ġpartic ular +Ġp ie +Ġstud ents +Ġf ive +ou n +ĠN e +Ġg i +Ġp as +Ġf ree +ĠS p +l ich +Ġpro f +Ġen g +Ġpr ot +ĠL ike +os ed +Ġcon nect +a pp +Ġë § +it ing +Ġb lo +Ġl os +ist s +Ġexper ience +re nt +Ġst ay +Ġfo od +t on +ru ct +Ġh ist +v iew +in ing +m ost +i vers +b o +ãģ Ħ +ĠT r +g en +Ġp lease +Ġcommun ity +Ġc e +A N +n o +Ġb ody +Ġh our +Ġ vers +á º +c er +Ġê ° +Ġre ason +ĠR ight +Ġl ater +Ï Ħ +Ġh ouse +Ġ X +оР½ +Ġst ate +f ic +å ¤ +Å Ľ +iel d +Ġp ri +Ġp ast +Ġw alk +olog y +er ing +an na +Ġt er +Ġho ld +Ġor gan +b en +Î ¿ +ó n +Ġeff ect +Ġyour self +Ġpl us +a j +and o +ur al +Ġro om +le ct +ê² Į +? " +s ide +Ġbe come +Ñ Ĩ +Ġ  +o od +Ġcon st +Ġn ight +ut es +Ð ¶ +Ġbre ak +Ġp ain +Ġst ep +ire d +Ġnot hing +Ġunt il +Ñ ĸ +аР² +Ù Ĭ +Ġd uring +ì§ Ģ +l ess +o ll +н Ñĭ +Î ¹ +f ect +i ver +ı Ħ +ith er +y ing +Ġbe gin +×Ļ × +iv id +Ġà § +Ġs al +Ġt a +Ġp ot +Ġ $ +Ġm ar +Ġcle ar +Ġf ace +Ġgr ow +Ġ * +Ġins ide +Ġfriend s +Ġle ave +en n +Ġeas y +Ġare a +al ity +ou d +Ġe at +Ù Ĩ +Ġp ur +or n +Ġsa w +Ġans wer +Ġfr ont +Ġbe aut +¼ ë +Ġm atter +Ġs on +ĠN ew +Ġres ult +id es +ch e +Ġf ut +p s +Ġfo cus +Ġinterest ing +å ¥ +Ġa p +" . +Ġcre ate +о Ñģ +Ġp ress +r oss +Ġp ick +l ine +Ġto ok +ĠM ay +r ow +Ġ ich +ĺ ë +Ġre f +Ġm or +r act +are nt +A R +Ġex act +Ġsp ace +w ork +н и +Ġb ir +Ġde v +Ð ³ +Ġto ld +Ġpub lic +ci ally +Ġv iew +ĠHe y +m ed +ll o +c c +Ġf ac +Ġcou ple +Ġhe art +l er +Ġre ady +Ġal most +ar ing +Ġh alf +ĠM e +av or +i que +Ġchar ac +Ġpr act +O N +an e +Ġ il +н а +Ġv i +l ish +he ad +Ġle ast +Ġbas ically +as ed +r ight +Ġy et +Ġtak ing +Ġcount ry +Ġw in +Ġis n +Ġposs ible +Ġc am +Ġinc re +Ġp at +Ġw anna +Ġcons ider +Ġab s +Ġwith in +Ġhum an +Ġthink ing +Ġo h +¡ ľ +Ġqu i +as es +Ġ 0 +it ely +ä¸ į +Ġk ill +Ġm il +Ġinv est +is ter +Ġsu c +ion al +el f +Ġwh ether +Ġcontro l +Ġagain st +ot s +ëĭĪ ëĭ¤ +i or +Ġpres ent +Ġ ا +Ġwatch ing +u be +er v +Ġn icht +Ġgo vern +ĠTh ese +Ġ : +u it +ug h +Ġwork s +o o +Ġw ir +Ġa ir +ĠT e +аР· +is ion +wh ere +Ġto t +j oy +ì ĭ +Ġv ol +ĠÐ µ +Ġcl ose +ĠA d +Ñ ī +in ed +Ġun a +Ġê· ¸ë +° ë +or ry +Ġb ro +Ġfil m +if t +2 0 +Ġty pe +Ġhappen ed +ĠA m +Ġg irl +ĠA re +ward s +Ġp our +Ġcol or +el t +а Ñģ +Ġs ense +le x +ĠW ith +us s +ri b +Ġre se +Ġn orm +Ġfut ure +Ġde al +end ing +e y +Ġ x +er o +ĠC l +u k +Ġwhat ever +sel ves +Ġyou ng +ì Ĭ +ĠM ar +ĠChr ist +Ġgu ess +Ġper form +Ġen er +r on +Ġh it +Ġw ond +Ġdire ct +ĠE very +Ġof ten +Ġf a +Ġal ong +Ġcl ick +ĠL ook +Ġsit u +Ġhapp y +e ad +Ġag o +Ġen c +Ġmy self +Ġco ver +оР± +Ġm id +Ġc ost +Ġt en +ĠS ch +Ġex pect +Ġwas n +Ġstr ong +if ul +Ġopp ortun +in al +y le +Ġsh are +Ġtr ue +Ġapp ro +Ġch all +Ġmin utes +Ġch ann +Ġë Ĥ +Î µ +l i +Ġm ess +or ies +pe cially +Ġwr ong +Ġy es +Ġì Ĺ +ir on +Ġall ow +Ġsu bs +Ġf ore +Ġf ight +Ġso cial +Ġc ra +an a +Ġa ff +Ġ ess +Ġway s +Ġsh ort +Ġf all +Ġla w +ĠWh o +Ġen joy +Ġc al +Ġac cess +f e +Ġn on +Ġac ross +er y +vious ly +ĠE x +id ed +Ġl ink +ĠP r +Ġterm s +ac es +Ġl and +az ing +Ġ1 5 +Ġm ult +Ġspe cial +å Ģ +iv ing +ìĿ Ģ +Ġty p +Ġst e +Ġ Ä +Ġfor ward +å ı +Ġf re +å¥ ½ +Ġrese arch +௠į +а ÑĤ +Ġma in +Ġrec ord +Ġh u +Ġdefin itely +Ġe ither +Ġlist en +Ġke y +Ġmark et +ĠÑĩ ÑĤо +iz ation +Ġvide os +Ġgu y +Ġf ig +Ġst ra +ĠP l +ull y +am os +Ġm ention +Ġs ong +Ġinter n +r al +ur s +Ġh on +Ġval ue +Ġb ar +c le +оР¶ +Ä ĩ +ľ ë +Ġz u +и м +ä½ ł +Ġsing le +Ġa uch +cus s +Ġget s +Ġsomet imes +å ¾ +am b +m m +c ing +Ġper fect +ĠB l +out h +ì ł +Ġs ci +p ar +Ġre d +Ġp ost +Ġm ot +Ġele ct +ĠE u +it ive +ĠS ome +Ġdes cri +Ġcur rent +é s +Ġt re +ĠE n +Ġm it +E N +Ī ë +i um +Ġhe ard +Ġsim ple +l ar +Ġevery body +il ar +Ġneed s +Ġdif fic +ĠGo od +um ent +c ent +Ġo per +а ÑĤÑĮ +et y +Ġbl ack +Ġgi ven +on es +Ġwe l +é Ģ +Ġìķ Ħ +Ġ3 0 +A T +Ġst at +ou ch +ĠM r +а ÑĢ +Ġsh o +Ġcon d +× Ķ +m y +Ġchild ren +Ġe u +еР´ +ìķ Ħ +ter n +Ġu h +Ġh ar +Ġpr om +Ġp ull +re w +Ġcomp any +Ġbeaut iful +ust om +íķ ĺ +к и +Ġst re +Ġam azing +ri es +Ġsuc cess +Ġm ach +n ot +Ġdis cuss +Ġn at +¦ ¬ +Ġun e +Ġdiffic ult +Ġr is +Î ½ +Ġc amp +Ġbu y +ä¸ Ģ +Ġma g +p o +ĠY our +Ġbeh ind +ic a +ı n +ĠO K +Ġl ang +Ġwom en +Ġen v +Ġre ce +Ġchann el +i ally +u le +Ġ1 2 +th ers +Ġb ott +Ġrep ort +ent ly +f ully +T he +Ġs ent +Ġev ent +Ġener gy +l t +Ġword s +ar r +d le +Ġa head +ard s +Ø ± +äº Ĩ +Ġto ol +con om +е Ñģ +Ġexact ly +Ġf avor +Ġl ow +Ġpro per +Ġìŀ Ī +Ġ ! +Ġrel ations +Ġm as +Ġkid s +Ġent ire +ud e +Ù ħ +ĠWh ere +Ġon es +Ġc ity +ol ut +Ġs ix +ab ility +ö r +il i +ĠE s +Ġhapp ens +ain s +Ġmod el +Ġp ict +Ġes pecially +Ġ1 00 +k t +Ġso on +b y +ro du +Ġan n +Ġsubs cri +ĠQ u +Ġav ail +im ent +Ġv oc +k a +Ġ2 00 +ap er +ĠI nd +Ġì § +h or +į ° +j or +и л +Ġs qu +A U +ar ning +ĠÐ ³ +I S +ĠÐ » +еР¹ +y es +å ħ +ĠÐ Ĵ +Ġor ig +оР³Ð¾ +Ġask ed +il t +оР³ +Ġcontin ue +Ġì ĺ +r am +Ġo thers +E S +oh n +Ġl ay +Ġbas ed +Ġp u +Ġapp e +Ġl im +Ġpro p +Ģ ë +m in +Ġh ot +ĠL a +Ġf ast +Ġprot ect +Ġam ount +Ġa qu +Ġf und +Ġc ustom +Ġc ult +Ġhand s +Ġha ven +Ġa ud +Ġout side +ĠA fter +ap s +Ġan im +pl oy +Ġh at +ĠF irst +Ġt reat +Ġe p +Ġm ater +Ġbuild ing +Ġë ° +å IJ +ìĦ ľ +z a +ught er +ĠP e +ne y +et er +at ic +Ġed uc +ê¸ ° +Ġmo v +ĵ ¤ +am a +r ation +Ġs n +Ù Ī +Ġs um +Ġph ot +ĠÐ Ŀ +Ġ . +æľ ī +Ġfin ish +itt ing +å ® +Ġlar ge +Ġì ĸ +Ġwh ite +ar a +Ġma is +ĠH i +Ġd am +Ġا ÙĦ +Ġbo x +ĠHe llo +Ġs le +Ġo pt +ri ed +¥ ¼ +Ġact iv +Ġn ão +ĠC om +Ġplay ing +T h +Ġavail able +Ġp ort +å Ī +ĠA h +Ġl as +Ġear ly +Ġwond er +± ° +Ġ1 8 +c ul +Ġfun ction +Ġmor ning +ll e +i ents +u x +Ġc ir +it ions +Ġde ep +Ġpol it +y or +m p +ak ing +Į ë +ĠM an +Ġmill ion +Ġ / +Ġind ivid +Ġp an +Ġgovern ment +Ġwr ite +ĠT od +am ent +Ġ Ï +Ġw ind +ĠE ng +ch en +W h +ì ľ +Ġ ident +ãģ § +v ent +ur ch +Ġh y +Ġy a +Ġtr ad +Ġrelations hip +à º +Ġd ou +O R +Ġs we +Ġne g +in ation +Ġte xt +i pp +Ġf ine +á s +ĠD r +ĠC ome +Ġmonth s +, " +ен и +Ġhour s +Ġp od +ir t +Ġinv ol +Ġcoll ect +Ġau f +Ġp a +Ġhist ory +m b +if y +Ġ ? +Ġbel ow +as ure +ab y +Ġlang u +Ġan t +Ġcom b +at o +Ġex ist +Ġë ĭ +Ġtak es +Ġcharac ter +a ff +Ġf ield +Ġe conom +ie f +Ġpie ce +å ľ +Ġre ach +Ġê ² +on y +Ġmater ial +Ġd ig +Ġph ys +Ġimp ro +Ġsim ilar +I C +Ġn et +y n +Ġpos ition +Ã Ł +Ġb ene +re ad +Ġle arning +um e +Ġcle an +ÑĤо ÑĢ +Ġco ok +Ġseem s +Ġo l +ĠU S +ĠJ es +Ġ à® +ent ial +ivers ity +ac y +Ġ Ñı +olut ely +re ct +ĠP lease +Ġrep res +Ġt ouch +m en +ĠÐ ° +i ón +ĠThank s +Ġan g +Ġma jor +Ġit self +ill s +" , +i ans +Ġsc reen +Ġh or +Ġknow n +Ġenv iron +Ġfin al +Ġfig ure +ĠT w +Ġe yes +Ġim ag +Ġsee ing +Ġha ir +re m +Ġapp lic +end s +p ut +Ġnew s +Ġcomplet ely +ugh s +Ġkn ew +if ied +ĠJ e +ĠD id +Ġsitu ation +Ġf lo +m s +Ġph one +Ġb all +d o +Ġp arent +Ġs orry +ur y +и н +ip s +аР´ +Ġinst ead +Ġhu ge +Ġt u +Ġ ãģ +ĠG r +Ġdet ail +ĠÐ Ł +Ġindivid ual +Ġf ire +Ġcl os +Ġw er +un e +Ġrun ning +Ġcon vers +Ġrec omm +Ġcom o +Ġsome body +ĠJ ohn +ĠìĿ ´ +ĠO ur +pl es +ĠP h +Ġan al +Ġ5 0 +Ġof fer +Ġ < +ition al +g est +Ġv ous +l et +ic y +Ġfeel ing +L E +r os +Ġth ird +оРº +Ġser ies +ĠAn y +is ed +o ld +Ġdra w +Ġserv ice +Ġcan not +b al +ãģ Ĩ +Ġli ving +ı m +Ġdiffer ence +Ġopportun ity +Ġne ar +or th +k en +Ġloc al +Ø ª +ĠC on +Ġob ject +Ġd ass +ãģ Ļ +IJ × +Ġquick ly +ra ph +Ġiss ues +éĢ Ļ +ĠAmeric an +Ġpre p +en ces +Ġprof ess +ll ing +o f +Ġfo ot +b re +Ġus ually +Ġgener al +d a +an ces +Ġd est +Ġo cc +Ġmem bers +Ġd ans +Ġequ al +z t +Ġbe com +Ġmo ving +Ġspec ific +ÃŃ a +Ġf ur +Ġne cess +Ġcomm on +Ġatt ack +ĠÑį ÑĤо +ĠTod ay +Ġun s +ĠG u +i od +Ġacc ount +Ġgra nd +Ġs elf +ĠE l +Ġt ast +Ġcont ent +Ġc u +Ħ ë +ĠMay be +ĠJes us +ore s +p ort +© ´ +Ġg ives +Ġnorm al +ÑĢ Ñĥ +Ġimp act +ä r +Ġd ies +Ġl ab +s h +i os +ĠP res +ĠU nd +ĠO f +Ġfin ally +Ġdo ll +Ġvoc ê +p ly +ĠA g +Ġtak en +Ġgr ound +f ort +Ġg ave +ĠIn st +Ġl ost +Ġwork ed +Ġl iter +Ġiss ue +Ġind ust +Ġret urn +Ġhappen ing +Ġwant s +и в +Ġproblem s +ĠC ar +Ŀ ¼ +ĠAl so +Ġs ize +Ġob viously +ĠS u +ĠS c +Ġrecomm end +our ces +ast ic +.. .. +Ġm i +l ier +ĠE ven +ci a +Ġh ur +v a +Ġm ass +Ġwould n +un t +ck s +Ġf elt +os p +l ight +ол ÑĮ +n ie +Ġbott om +Ġб Ñĭ +ore d +is on +Ġgr ad +Ġum a +Ġv a +Ġì Ĥ +ress ion +ul ation +I D +id ence +Ġb ur +Ġg one +l u +ìĸ ´ì +Ġre du +Ġj a +ìĿ ĺ +it a +Ġso ft +Ġç a +ic o +er al +à ± +a f +Ġpoint s +g u +Ġd é +ap t +a x +ĠAl right +Ġcam era +Ġa ch +Ġп о +Ġse ver +5 0 +Ġs ie +Ï ģ +Ġm al +Ġcomp ut +Ġmid dle +Ġcould n +m ing +Ġì ĭ +ĠH is +Ġg ames +Ġint rodu +Ġc ell +p or +Ġsle ep +Ġë ³ +id ing +Ġ ou +Ġde g +Ġdr ink +Ġenviron ment +ĠUn ited +Ġtalk ed +Ġcho ose +Ġj our +e ge +ĠM in +Ġint e +Ġr ather +Ġoff ic +к а +ac hing +Ġmention ed +Ġf ill +Ġtr ack +Ġn ie +Ġ ut +Ġв Ñĭ +ib ility +Ġv ac +Ġr ad +Ġp ack +Ġs end +ĠD as +ĠA b +Ġeng ine +ãģ Ĺ +Ġcomp et +à ´ +Ġв Ñģ +Ġdo or +Ġlong er +å° į +Ġlangu age +Ġext ra +pl ay +Ġwe bs +um b +ro om +ç ľ +Ġbegin ning +Ġre fer +A M +n en +ig her +f ace +er c +Ġfor get +Ġcom ment +еРº +л Ñı +r or +ż e +ĠG e +Ġd ark +Ġany one +ant e +g es +ìĬ µ +Ñ ij +b ed +j e +ruct ure +Ġpr im +id a +è ¦ +ãģ ¾ +Ġm ix +Ġstart ing +ĠìĿ ´ë +Ġprov ide +act ion +Ġm other +Ġper iod +Ġst ick +ĠYou T +Ġtechn ology +ê ¹ +Ġb ed +Ġg iving +Ġexpl ain +z en +im ate +Ġrepres ent +lo ad +ĠHow ever +Ġli ves +ut h +ir it +og n +Ġli k +Ġresp ons +Ġpri v +Ġto m +ç ão +i am +Ġexc ited +Ġc ard +gr ound +Ġ× Ķ +Ġs ens +Ġte ach +id o +h od +Ġep is +Ġwel come +Ġw all +ä ¹ +Ġch ance +h en +ĠÐ ¡ +ĠÄ ij +Ġsim ply +ĠÑĤ ак +r ing +j a +b ook +Ġsever al +st e +Ġcreat ed +Ġо ÑĤ +Ġp ush += = +Ġh igher +u f +our ce +o ke +Ġon line +Ġre le +Ġt on +ens ive +Ġfavor ite +Ñĥ д +Ġlook ed +Ġv on +âĢ Ķ +Ġf ür +Ġbut ton +Ġb ill +Ġchang es +! " +Ġsl ow +ab les +Ġde ath +and s +ate g +Ġthem selves +ãģ £ +Ġc op +ãģ ® +Ġperson al +ug hing +Ġ1 1 +g ar +ad es +Ġneed ed +Ġstud y +ag ed +ÑģÑĤ в +in o +Ġdis c +k i +Ġadd ress +× ¨ +itt en +es ome +ĠÐ ¶ +¤ ë +ur a +Ġm u +Ġcontin u +f or +Ġm atch +ãģ ¦ +Ġstra ight +IJ ë +n ers +Ġdo g +Ġde b +ĠC O +Ġo s +g ed +c ame +Ġcor rect +et te +ĠSe e +Ġinclud ing +ĠEu ro +est er +Ġj ump +ĠWh ich +Ġк ак +s on +y a +IN G +Ġe ine +os h +en cy +Ġmed ia +Ġsubscri be +é Ĥ +Ġpr in +Ġha b +ĠP er +ĠW as +Ġp age +it or +Ġto wards +Ġtri ed +en ge +art ment +Ġvar i +Ġp aper +Ġpict ure +Ġvers ion +Ġbr ought +w are +ĠSt ates +Ġs ich +led ge +Ġper cent +Ġgo d +e c +ĠC omm +Ġdec ided +Ġse lect +íķ ľ +) . +ur ity +Ġfur ther +Ġcom ments +le ment +Ġd ream +Ġcent er +m i +Ġc as +Ġwom an +Ġro ad +Ġf ail +Ġbe came +l us +il ities +ãģ ¯ +ĠC o +Ġman age +Ġrec ogn +Ġact ion +Ġbene f +Ġear lier +× ľ +Ġspe ed +Ġm ent +Ġso ci +Ġsho ot +u i +Ġà ¤ +Ġapp ly +v o +x im +Ġca use +Ġsur pr +Ġha ben +D I +Ġf ather +ĠNe xt +ĠYouT ube +Ġc ode +Ġro le +g ress +Ġg reen +et t +Ġbu ilt +Ġfl ow +Ġb ase +Ġtra ining +Ġr ound +ĠW ill +Ġp ath +ĠR o +Ġinterest ed +ìĸ ´ +Ġres pect +Ġchang ed +iss ion +Ġstud ent +og raph +Ġappro ach +Ġshow s +å° ± +Ġt ar +Ġcr it +Ġg lo +ìĬµ ëĭĪëĭ¤ +Ġde ad +ĠPres ident +Ġth ous +Ġb al +st er +e x +Ġabs olutely +Ġm ic +Ġpract ice +Ġqu ality +Ġl ower +og le +Ġse par +b all +med i +Ġre view +ĠA pp +Ġo k +âĢ ĭ +Ġexper ien +Ġconc ern +ent ially +m ore +ĠJ o +ap an +ĠI ch +ist ic +Ġf air +Ġwebs ite +i res +ĠB y +Ġtra vel +Ġris k +Ġm ir +Ġbo ard +Ġs en +Ġparent s +ĠW ow +Ġfe ed +Ġsa ve +Ġser ious +Ġin it +E L +und red +A S +Ġv an +or row +Ġwor th +Ġse arch +Ġ1 6 +Ġpart s +ÑģÑĤ ÑĮ +Ġcomp an +Ġmov ie +Ġmet hod +Ġ ill +Ġw ish +d y +Ġit em +Ġmin us +ang er +Ġvo ice +Ġsk in +Ġare as +Ġe ight +Ġo bs +Ġ , +аР¹ +Ġo il +Ġc y +Ġb aby +s y +Ġem ploy +ĠK e +Ġpl aces +Ġf ix +Ġest á +ãģ ¨ +iv ed +Ġlot s +Ġse ason +un k +al t +Ġt able +ĠÐ ¢ +à ¢ +Ġatt ention +ãģ ª +ĠH er +Ġa ge +Ġp ra +b ack +c il +Ġnet work +r it +Ġdo c +Ġare n +ig en +Ġë Ħ +Ø ¯ +end er +Ġtot al +Ġpr ice +Ġcra zy +ì ļ +i qu +th ough +Y ou +Ù ĩ +ãĤ ĵ +Ï ħ +Ġs at +Ġb i +ĠD ie +Ġsh a +Ġthank s +u h +Ġst age +аР¶ +ĠF l +Ġle av +Ġbo y +Ġa f +ö n +ĠG et +Ġac cept +Ġent er +Ġt ur +Ġsi ÄĻ +Ġhon est +ãĢ Į +Ġs am +Ġre pl +g ing +Ġdevelop ment +ĠA ct +or a +ãĢ į +ä ¾ +Ġknow s +Ġim age +ĠL ord +и ÑĤÑĮ +Ġweek s +Ġse x +Ķ ë +Ġh undred +Ġsound s +Ġlearn ed +Ġb ud +ĠÑģ ÑĤ +Ġinc red +â Ļ +Ġn os +Ġd rop +Ġb en +ĠÐ ĺ +Ġsa fe +at a +Ġf uck +so ci +Ġd an +Ġcr oss +1 0 +m o +ver t +Ġ1 7 +z ie +å ķ +Ġd om +ĠB o +Ġset ting +Ġinvol ved +ar ily +Ġs ind +Ġs us +Ġwor ry +et h +ê¹ Į +Ġs un +Ġh ier +Ġcertain ly +ou l +ort s +ĠE r +ĠU m +Ġca us +Ġnat ural +Ġà ¼ +Ġc ry +ĠSe c +Ġs om +æ ² +Ġeduc ation +а еÑĤ +Ġmult ip +Ġal one +Ġe ye +Ġr ate +ĠEuro pe +è ¿ +m on +Ġf it +iz ing +pp ed +Ġpress ure +th e +и Ñģ +it es +ĠA f +re ci +att le +Ġserv ices +ĠGo ogle +é ģ +Ġc ases +Ġdri ve +Ġchall eng +u z +ĠM o +ìľ ¼ë +v al +åĢ ĭ +Ġf ol +Ġì ¢ +ff ic +Ġr a +Ġs in +Ġbl ue +Ġaff ect +Ġm is +Ġsh ot +Ġо б +as ing +Ġsign ific +ĠC he +Ġê ³ +Ġpos itive +ì £ +Ġw ie +Ġ4 0 +ord ing +ĠFr om +ê µ +Ġbra nd +Ġtr ust +Ġp le +Ġcommun ic +Ġwe ight +Ġask ing +Ġta x +ĠJ apan +ãģ Ł +Ġíķ ĺ +op s +Ï Ĥ +Ġput ting +Ġro ll +ĠAmeric a +re g +ŀ × +at ures +ens ion +ĠS omet +Ġorig inal +p ing +Ġ ÅŁ +Ġproduct s +ãĥ ¼ +Ġcont act +ol ution +Ġgo al +Ġp ow +Ġperform ance +Ġblo od +at ors +ĠM ich +Ġtem per +ĠD an +Ġsu gg +ÑĤ и +Ġim m +Ġoff ice +Ġar ri +Ġcom fort +ĠÐ Ķ +Ġsugg est +Ġpl at +Ĥ ĺ +1 9 +Ġo m +Ġse ven +ĠC ent +ill e +Ġcon cept +Ġb ag +ü n +ive ly +Ġd iv +m os +æ ī +Ġfeel s +Ġ ir +ak es +le y +Ġpartic ip +ĠÐ ļ +f l +j ust +Ġs il +ĠP a +A L +Ġgot ta +Ġf an +Ġchall enge +Ġcompan ies +ĠPe ople +< / +оР· +Ġp en +is ing +Ġa us +em ic +am ente +Ġmeet ing +Ġvis it +Ġsupp osed +ĠOn ce +д а +or ld +3 0 +U S +Ġvi ol +Ġnot ice +ĠÐ IJ +h an +p ed +ì ĺ +h h +Ġtr ou +Ġmin ute +ĠP ar +r ay +Ġt it +Ġup d +Ġblo ck +Ġd ue +a ur +Ġfor ce +Ġcou n +ĠâĢ Ķ +Ġtyp es +ë § +Ġl ate +Ġimpro ve +Ġì Ī +Ġa ve +ul es +c l +am ed +Ġaw esome +ĠO k +Ġv ot +Ġmach ine +Ġfollow ing +Ġme asure +ac ión +u el +ch an +Ġab ility +Ġt out +Ġide as +Ġincre ase +Ġen s +ĠÑ ħ +Ġë ª +Ġj est +ĠÐ ľ +Ġtr uth +h y +Ġsp end +Ġsci ence +et e +Ġ1 4 +Ġepis ode +Ġal g +end ed +ãģ ĵ +ar i +ll a +Ġf ish +Ġthr ow +m it +å ¹ +Ġcir c +ĠC al +Ġt our +Ġdire ction +Ġno ch +еР² +é n +Ġcount ries +Ġindust ry +in y +ic le +Ġfe et +I t +Ġlead ers +et zt +Ġst aff +ç Ķ +Ġpur p +it o +? ! +ĠJ a +Ġst ore +et ic +ĠCh ina +Ġë IJ +ĠUn iversity +Ġ # +Ġdec ision +Ġach ie +Ġact ual +u ly +Ġse ction +Ġresult s +Ġst ar +Ġm ist +ib ly +Ġd ad +Ġnum bers +om b +è ª +ĠS pe +Ġm er +Ġ2 5 +Ġaut om +Ġco ld +Ø ¨ +Ħ ľ +ag er +ĠT V +ĠS ie +ĠH ave +Ġ że +ug g +ain ed +Ġup on +Ġlo g +Ġcomplet e +Ġbra in +ag ing +ĠM us +o ver +Ġeas ier +Ġinte gr +Ġm ás +Ġturn ed +Ġst ri +iv al +Ġhe av +ĠT H +Ġwr iting +ÑĢ Ð° +åľ ¨ +å¤ § +Ġcl a +d ing +Ġtell ing +и д +ic ated +ä» ¥ +ac ht +ãģ Ĥ +h aps +ĠSt e +Ġres ources +Ġd ann +Ġpart y +Ġ ÏĦ +Ġsa f +is es +t re +o int +Ġknow ledge +Ġany more +Ġf ly +Ġma int +и к +å ij +Ġse ll +la ughs +ĠY ork +Ġb ien +Ġo d +Ġeas ily +Ġr ange +Ġo ption +Ø ¹ +Ġapp reci +oc r +Ġdet erm +Ñ Ħ +Ġmean ing +Ġs ite +Ġdis co +ver age +Ġl ose +Ġinst all +Ġem ot +ant ly +ä t +Ġt amb +ĠW ar +ĠH o +ĠG en +em y +еР· +ĠP ol +Ġmess age +Ġnot e +Į Ģ +Ġh et +Ġim medi +Ġav o +Ġbook s +Ġbecom es +res h +è s +as ons +Ġhim self +ut s +Ġj u +Ġaw are +Ġrequ ire +Ġsystem s +ĠH ar +Ġam ong +Ġh om +Ġb reat +Ġwe ird +Ġë ¶ +Î » +Ø © +if f +or ing +Ġplat form +ĠT ake +Ġhelp s +ut ions +Ġfor g +Ġl uck +ĠEng lish +Ġwe b +Ġneg ative +Ġt ut +Ġab ove +ng th +Ġê ±° +Ġst ories +Ġlo ad +Ġback ground +Ġsw itch +g a +Ġprin ci +Ġfin an +Ġvar ious +Ġl Ãł +Ġkind s +ain ing +Ġn ature +ĠÐ ŀ +c z +Ġpr ay +Ġg ar +ir m +Ġ & +Ġì ĥ +n s +ĠR ep +ĠF e +Ġre v +ra nd +Ġlike ly +Ġunderstand ing +ı r +ãģ ĭ +Ġf al +Ġ1 3 +ÑĨ и +Ġsu d +Ġbr other +Ġpl ant +Ġthrough out +w ise +p re +Ġcult ure +ĠÙ ħ +Ġwonder ful +Ġa h +pp er +Ġso ld +Ġstart s +Ġwr itten +Î ¯ +n i +Ġ×Ķ × +ĠD av +Ġu lt +Ġar m +Ġro ck +Ġwe ar +ë į° +an o +ra g +Ġsqu are +ан и +c ast +le br +Ġliter ally +Ġplay ed +Ġhe at +on se +r ict +Ġins p +id s +Ġpop ular +ë ıĦ +Ġc atch +Ġm ount +Ġj ud +Wh at +еР± +R A +a ud +к о +Ġsur face +Ġcon v +Ġpie ces +O h +æ Ģ +Ġst yle +pp ing +Ġread ing +Ġconvers ation +оР¿ +ä¾ Ĩ +ĠAg ain +Ġb ank +t ime +Ñĥ ÑĤ +er ve +ĠG reat +Ġcap t +аР± +ay s +ĠF in +ific ation +Ġä r +а Ñİ +Ġe gg +ĠW el +Ġtar get +ul a +ch es +an i +O O +ic ious +n ow +Ï ĥ +bo ard +Ġg ente +Ġd ro +ĠE t +Ġd in +Ġc os +Ġaut hor +Ø ³ +Ġo ch +Ġem ail +Ġsp irit +Ġs itting +m as +Ġstre ngth +Ġbig ger +ĠW ait +Ġm at +Ġpol ice +ress ed +Ġwait ing +is hing +Ġdoll ars +ho od +s s +Ġimag ine +in i +Ġm es +Ġdis e +id ge +ab or +Ġp et +Ġh op +ĠK ing +Ġcomput er +Ġgo ld +Ġn u +Ġf ing +) , +Ġsec urity +ru ction +Ġsol ution +e xt +Ġp atter +ick en +ure d +Ġstand ard +ìĭ ľ +Ġdou ble +Î · +Ġw ife +is a +Ġdirect ly +ac ed +Ġb unch +Ġ ¿ +ал ÑĮ +Ġreg ard +Ġswe et +Ġun ique +ĠâĻ « +Ġtra in +ĠG erm +Î ¬ +R E +Ġbeh av +Ġpre d +ì ĥ +s et +Ġdescri ption +é e +Ġc at +å ĵ +Ġcoll ege +ì Ľ +Ġapplic ation +ĠS en +as k +Ġc red +ub lic +Ġmultip le +Ġn i +Ġpres ident +Ġadd ed +Ġro b +Ġaqu i +Ġh osp +Ġtool s +Ġg un +Ġbas ic +Ġl ines +Ġst ructure +ĠR uss +Ġtot ally +Ġbig gest +Ġe en +Ġar g +Ġ× ľ +Ġp ark +ĠD es +Ġce lebr +Ġf ait +ен ÑĮ +Ġsu ff +Ġreg ular +¨ ë +Ġm ine +ĠK ore +Ġpre vious +Ġp i +Ġse g +Ġpol icy +Ġк о +ĠTr ump +Ġvac c +ó w +ĠS y +и Ñĩ +it ter +Ġpolit ical +r as +Ġal s +ел ÑĮ +Ġsha pe +an z +Ġon to +Ġar ch +Ġam b +ag ram +ĠS m +ct ions +Ġjo in +b or +å Ľ +Ġfr ame +ł ĩ +Ġcho ice +௠ģ +Ñĥ Ñİ +ĠC or +ĠS w +I T +Ġt end +ĠE ar +Ġto r +Ġev ents +Ġcla im +ĠD a +ĠM ark +Ġgroup s +Ġe ating +ĠW orld +Ġrec ently +Ġtast e +Ġsur v +à ¤ +Ġsk ills +Ġи з +itt ed +Ġsh op +ìĿ ´ì +Ġest ab +ĠëĤ ĺ +Ġsecond s +ĠTh ose +ĠE nt +Ġì Ħ +ers on +Ġto wn +Ġc and +Ġopt ions +Ġ ing +V ID +Ġenc our +Ġr é +âĻ ª +Ġent re +Ġmove ment +ĠB en +Ġbir th +Ġwh e +Ġh ang +ĠE m +ig e +ro ll +Ġun f +ì Ĥ +Ġr id +Ġsp read +Ġh ost +al d +ĠE d +Ġcons um +U N +Ġop in +it ar +ĠM ed +Ġsub ject +Ġp al +Ġcar ry +Ġag ree +ĠWh ile +Ġcare er +Ġsci ent +Ġsud den +Ġf ile +z i +Ġex cept +é º +Ġpot ential +ĠAn other +Ġcomp lex +ĠS im +end o +Ġr ais +Ġphys ical +Ġd ate +ak er +ĠC ol +Ġpower ful +Ġmem ber +ra p +Ġsp ot +Ġs ource +Ġf em +é m +Ġem p +j i +iet y +Ġinf lu +Ġd ry +Ġlo ck +Ġz ero +ĠU h +Ġr out +Ġpor que +Ġ2 4 +Ġt al +Ġfol ks +Ġla unch +Ġcomp on +ĠWel come +Ġk ann +ä n +ĠÑį ÑĤ +e es +ĠÙ Ī +Ġany way +Ġaud ience +äº º +Ġsl ight +on a +Ġu r +Ġrel ig +Ġext rem +ı z +ĠM a +Î ¼ +Ġà ¶ +Ġall ows +Ġf at +ĠF ace +Ġn ational +Ġinter view +ĠM c +é t +Ġc ute +el a +Ġsec ret +ĠW est +ĠD ep +Ġex erc +Ġhist or +Ġpri or +Ġ6 0 +av a +ac her +y ond +ĠH a +Ġest e +in ary +ĠN orth +on st +Ġsm art +am s +ал и +Ġd ar +er ed +Ġfun ny +ĠO b +ĠBl ack +Ġrel ated +ĠB u +Ġsome where +ĠR em +n es +ment e +ĠRe ally +Ġcreat ing +Ġfam il +Ġsoci ety +Ġg el +Ġtrans form +Ä ĥ +Ġinclud e +Ġh ol +l ike +k o +air s +Ġп од +Ġpers pect +Ġb es +Ġparticular ly +Ġshow ing +ĠP art +Ġqu al +lo ck +Ġreal ity +ho ld +ict ion +o on +Ġv ir +ãģ « +it ary +Ġdr ug +Ġfe ature +Ġre asons +Ġ× © +Ġwr ote +Ġf ant +Ġb and +Ù ĥ +en a +ke y +Ġear th +d om +Ġfe atures +Ġflo or +Ġspeak ing +Ġt ip +ĠA ust +Ġst ock +Ġch urch +Ġr ac +ìľ¼ë ¡ľ +à¸ Ļ +ãĤ Į +k y +Ġresp onse +Û Į +ul ations +Ġsl ide +Ġgrad u +ci ous +Ġme ant +Ġ == +Ġ× IJ× +ã ħ +Ġkind a +Ġsc ene +Ġm uit +Ġê° Ģ +r ast +re st +Ġplay ers +w a +Ġbro ad +Ġtom orrow +oc ol +ĠÑģ в +ĠB ar +ı k +Ġse a +Ġrem ove +Ġrem ind +ом Ñĥ +ĠS ince +Ġave c +ce ll +и Ñħ +Ġdoc ument +Ġê·¸ë Ł +Ġne igh +be at +Ġp Ã¥ +Ġas pect +Ġd ed +lish ed +il s +Ġour selves +u ce +Ġhe y +ĠпÑĢ Ð¾ +ent y +Ġas soci +ad os +um ber +Ġ ] +éĤ £ +no v +Ġì Ļ +Ñĥ Ñĩ +Ġcond ition +ëĬĶ ëį° +Ġval ues +Ġsc en +min ist +Ġc ast +Ġgrow ing +Ġus er +Ġresp ond +l im +é r +y m +çľ ĭ +os es +sy ch +ĠÑĢ Ð°Ð· +Ġappe ar +Ġpro gress +eng th +Ġj ak +ĠD is +Ġpat ients +ĠS er +Ġg as +è re +ìĸ´ì ļĶ +Ġre ci +ìĿ ¸ +Ġs ca +ep end +Ñģ к +аР¿ +Ġb atter +Ġve h +ð Ł +Ġac com +Ġbe at +Ġpain t +Ġcont rib +Ġs ad +Æ ° +al es +Ġt ree +b a +Ġb orn +ic ed +à® ķ +b and +Ġme chan +ĠD et +Ġcap ital +Ġdel iver +Ġfe ar +ŀ ĺ +ĠS outh +Ġb ought +Ġst ress +Ġv or +? ? +i h +ìķ ¼ +Ġer a +ìĿ´ ë +а Ñı +is ions +iv ity +Ġhelp ed +Ġass ist +Ġplay er +r an +Ġimmedi ately +Ġmo ved +c ie +ê ± +Ġann oun +å ¿ +ìŀ IJ +Ġprodu ction +Ġsum mer +Ġt un +Ġprogram s +G H +al ing +ir a +el ess +. ) +Ġa verage +è¦ ģ +Ġgl ass +om an +if ically +Ġëĭ ¤ +ĠC ong +ĠV er +Ġtr ick +Ġbe gan +Ġv ill +ê ±° +h ow +æ Ń +Ġt ill +Ġ9 0 +ber t +Ġê ¸ +Ġtemper ature +à ² +à¹ Ī +Ġgra ph +Ġê· ¸ +Ġr ot +Ġmo b +A Y +a el +Ġre pe +Ġdev ice +Ġ19 9 +Ġte le +Ġke pt +p a +æ ĸ +ver se +Ġst ream +е Ñĩ +ess ion +Ġstr ugg +z z +Ġdeg ree +Ġhelp ing +Ġsm ell +Ġper haps +p ro +Ġcont ext +Ġi k +Ġп еÑĢ +Ġcal cul +éº ¼ +b ing +Ġreal ize +l am +ĠCh ar +y t +ĠìĿ ´ì +Ġd anger +ĠI m +a a +Ġlo ved +Ġpurp ose +Ġfinish ed +Ġpe ace +Ġo t +Ġglo bal +Ï Ģ +Ġab er +ĸ Ī +Ġcharac ters +Ġn ur +Ġdam age +Ġem er +Ġpre c +ĠW ir +Ġinst it +ij × +Ġallow ed +b on +Ġto d +еР³Ð¾ +Ġj etzt +Ġmed ic +Ġsmall er +ce ed +Ġlevel s +Ġint ell +W e +Ġse m +Ġcurrent ly +Ġmod ern +Ġcont ract +Ġdetail s +ortun ately +O S +Ġst ates +Ġad just +ant age +e z +ĠV ery +Ġsc ale +Ġre lease +Ġf az +Ġ ic +it ude +A C +ĠP at +id en +Ń IJ +Ġpre fer +olog ical +ĠFace book +Ġê° Ļ +Ġ .. +ĠM ake +Ġко ÑĤоÑĢ +ĠDav id +ĠAf ric +Ġmod e +ĠC ity +Ġsh all +ĠÑ Ħ +im in +Ġз а +r om +u a +Ġbe yond +Ġdist rib +к Ñĥ +ĠDo es +Ġv ict +r ate +Ġv ai +Ġsuccess ful +Ġh ous +ah a +est s +ĠE st +Ġdisco ver +Ġthere fore +ch a +Ġc up +Ġpop ulation +ĠI l +s c +Ġsp ent +re l +Ġuse ful +Ġt ab +æ Ŀ +Ġ Å +Ġìł ľ +Ġcon se +Ġqu ant +ay a +Ġb on +åı ¯ +ĠCh in +Ġê² ĥ +ound s +е ÑĪ +ell e +Ġ ice +2 1 +Ġk ick +ä¸ ĭ +Ġstep s +Ġton ight +нÑĭ й +ren ch +. ' +Ġgra b +Ġimp lement +ĠìĪ ĺ +Ġmiss ion +Ġclear ly +Ġappreci ate +è Ģ +Ġf resh +ar m +ĠTw o +Ġex ec +Ġproject s +Ġcommun ities +ri ble +Ġreg ion +Ġfre qu +ro y +Ġhow ever +Ġpart ners +an c +Ġmin im +Ġl at +Ġfamil ies +Ġev idence +Ġp un +ra ft +Ġl oss +Ġma p +Ġany body +Ġchang ing +Ġr ules +Ġorgan ization +Ġess entially +ĠR ed +Ġele ment +æ Ĺ +Ġv irt +r at +Ġpr int +and er +are n +em os +ο Ïħ +Ġcond itions +ab e +Ġd ance +и ÑĢ +Ġd os +о Ñĩ +ĠQ ue +Ġwalk ing +Ġt ro +Ġ id +Ġadd itional +Ġfull y +Ġf ans +Ġadd ition +Ġlik ed +Ġü ber +Ġb ow +d i +Ġm aster +o ff +) : +m ber +Ġë ¬ +å ¯ +åĪ ° +la use +Ġo der +Ġsaf ety +Ġre act +à® ¿ +b t +Ġdis app +Ġgirl s +S t +ĠA ng +Ġfa ith +Ġturn s +Ġt ight +Ġm outh +am i +z er +Ġwe ap +Ġб Ñĥд +Ġhosp ital +ra id +Ġmic ro +ĠSt ate +ĠM ost +ag n +Ġdec ide +Ġpat ient +Ġcor ner +Ġdi ed +N o +ĠSt ud +re nd +em pt +Ġli e +Ġl if +ĠBe fore +t ó +ĠSu per +Ġbe ll +6 0 +Ġpriv ate +ĠPa ul +Ġg ib +Ġag re +´ì Ħľ +Ġs ig +Ġinvest ig +Ñı ÑĤ +en ing +Ġdist ance +Ġwar m +Ġdig ital +å¾ Ī +in er +Ġp and +ĠCO VID +Ð ³Ð¾ +g n +Ġr ace +Ġpr oud +Ġte aching +Ġ ÑĤо +ìŀ ¥ +ĠAll ah +I n +Ġw ood +Ġcol ors +Ġw ird +u j +id ad +Ġcustom ers +Ġconnect ed +Ġlay er +Ġachie ve +Ġperspect ive +ĠC oll +Ù Ĥ +Ġcl oud +!! ! +Ġend ed +łĩ ê²Į +Ġmanage ment +Ġr ich +Ġsub st +Ġrem o +Ġser ve +Ġres ist +Ġthought s +Ġgrow th +ili ar +Ġright s +Ġchar ge +Ġcons ist +Ġwer den +Ġem b +and om +Ġhur t +Ġk an +i as +л о +Ġsh it +Ġbe g +Ġrece ived +it ation +Ġme at +Ġis so +ff ee +Ġfam ous +Ġcomfort able +I L +ĠB ye +èª ª +åĢ ij +oth es +Ġmed ical +Ġenjoy ed +Ġhealth y +Ġw y +c ies +Ġeff ort +Ġdo ctor +Ġmil itary +L AU +Ġg ro +Ġb attle +Ġf ed +Ġcap ac +Ġaf raid +iv il +ĠвÑģ е +Ġl ength +ys is +Ġbe i +¤ í +Ġorgan iz +or g +in c +Ġinter act +ĠChin ese +Ġacc ording +Ġincred ible +Ġkill ed +Ġda ughter +ĠÏ Ģ +Ñĭ в +Ġschool s +Ġ « +ll er +Ġshould n +n al +Ġcr is +Ġch icken +Ġf aster +Ġextrem ely +Ġopp os +Ġn ous +Ġ + +ri a +Ġfinan cial +Ġexc iting +Ġjour ney +×Ļ× Ŀ +ł ë +Ġdis play +Ġmem ory +Ġheav y +н е +Ġpass ed +ÑĢ Ð¸ +il es +Ġp sych +Ġspec ifically +Ġeng age +Ġl ed +or ge +ĠD em +ord er +Ġ8 0 +Ġcre am +ester day +Ġed ge +Ġп ол +Ġbu ll +Ġind ic +Ġk tó +Ġhope fully +um ents +ag en +н ого +Ġh ate +ch t +8 0 +Ġeff ic +Ġì§ Ģ +Ġintern et +Ġbud get +Ġproper ty +id ay +Ġì ļ +Ġм ож +ol a +Ġshow ed +ĠM on +Ġthous and +A P +Ġpo or +us ed +ĠJ ack +Ġs Ã¥ +ĥ ½ +Ġes c +Ġsoft ware +Ġqu ar +ĠØ ¨ +Ġnecess arily +om en +i y +Ġevent ually +ish ed +Ġbr ight +E D +Ġs pl +Ġdem and +Ġth reat +Ġs ir +Ġrele ased +ck et +ĠâĢ « +Ġrequ ired +Ġv ote +ì ¹ +à® ¤ +Ġdevelop ed +ĠìĤ ¬ +at ory +Ġd ir +ca pe +Ġslight ly +à ¬ +๠ī +re et +Ġdise ase +Ġcour t +Ġitem s +ĠEar th +ÑģÑĤ и +ж е +ì ² +Ġchalleng es +ĠBr it +Ġdesign ed +1 2 +Ġhear ing +Ġlisten ing +z o +ĠÑģ л +ãģ§ ãģĻ +Ġper o +Ġwe aring +pl ic +Ġch em +Ġbal ance +Ġb a +Ġrece ive +im a +Ġsignific ant +Ġм Ñĭ +an ch +ĠC r +ĠC oun +ê¸ Ī +Ġjo bs +Ġoffic ial +Ġper m +om s +Ġopportun ities +Ġover all +Ġh us +od es +Ġn ation +ĠR eg +Ġor d +Ġrest aur +Ġì Ĩ +Ġm el +v in +Ġw enn +Ġk ön +æ ĥ +Ġopin ion +ãĤ Ĥ +è ¬ +ĠSomet imes +ç Ĥ +Ñī е +as c +O U +Ġ20 20 +Ġdel icious +ig er +Ġìķ Ī +o le +Ġhand le +Ġc it +Ġíķ ľ +Ġf ör +o oth +Ġnecess ary +Ġind epend +æ Ħ +ist en +h am +Ġé t +ãĥ ³ +Ġmult i +Ï Į +? ) +Ġcamp us +Ġtop ic +Ġr ain +Ġpan el +ĠS am +Ġlar ger +aud ience +Ġpa id +Ġeconom ic +ol t +Ġstre et +ĠC ont +Ġdri ving +Ġìł Ģ +Ġh ay +Ġprofess ional +ĠIn tern +å ¸ +Ġin put +Ġc ateg +Ġc ro +Ġ ll +E T +Ñĭ й +* * +ĠZ e +B LE +Ġì ¤ +re es +ĠÐ ¯ +ed e +ier t +Ġfo ld +Ġd ur +ĠN ational +Ġìĸ ´ë +an ced +Ġfa ire +ut ed +Ġk ing +Ġw ild +o i +up beat +Ġpre vent +i us +Ġà ¨ +Ġw ide +Ġr ing +Ġtit le +Ġstand ing +Ġal though +Ġh i +Ġsa uce +Ġs ides +Ġanim als +il ing +at ives +ìĹIJ ìĦľ +ĠO ver +Ġdes p +Ġconsider ed +ar ies +i ers +Ġein en +Ġs ister +Ġë ķ +ĠS ure +ãĤ ĭ +ri end +a ign +Ġsh own +Ġs ac +Ġs ont +Ġcent ury +Ġt ien +ĠÎ º +ĠS T +åķ Ĭ +Ġold er +ie m +Ġtr uly +ĠS i +Ġwind ow +iqu es +ar io +æ² Ĵ +Ġloc ation +Î º +Ġì ľ +v i +ag ue +ĠS orry +Ġdis p +Ġhe ll +Ġà ī +Ġtr ade +Ġcrit ical +Ġê ± +Ġn amed +Ġprep ared +ĠH ouse +al u +Ġt ough +Ġtri p +Ġs and +c el +ü z +ĠP ut +Ġap art +is f +v is +Ġli br +a ven +Ġv ie +Ġeffect ive +ภ² +Ġmag n +Ġmuit o +Ġê µ +h al +Ġlim it +Ġn ine +Ġwill ing +ı ÅŁ +s p +еР³ +h i +Ġal t +ĠJ an +Ġorig in +ĠU s +Ġele ments +Ġus es +Ġhelp ful +Ġfl at +Ġfam iliar +ĠP ark +Ġc ore +Ġclos er +Ġact ive +Ġad minist +C E +нÑĭ е +ç Ħ +Ġrel ative +Ġment al +Ġr andom +Ġpart ner +Ġut il +ph one +Ġr ule +w w +Ġìł ķ +Ġsch on +Ġco ffee +H A +Ġconnect ion +Ġun it +la ughing +l og +Ġapp l +л а +us ic +ĠB ra +Ġany where +AU DI +Ġsepar ate +bo x +Ġd ivid +Ġtest ing +Ġs ick +Ġwer en +ä» ĸ +Ġ׾ × +Ġadv antage +Ġtrans fer +' . +Ġë ¹ +Ġfind ing +н ой +Ġì¢ ĭ +Ġfor t +Ġeconom y +Ġl ack +Ġleav ing +Ġd im +å İ +ĠR es +Ø Ń +Ġdiscuss ion +еР¿ +Ġg es +du ct +Ġch ain +Ġus ers +e ch +ÅĤ a +Ġdis h +Ġcare ful +Ġte acher +Ġopt im +Ġfl u +at ically +Ġref lect +Ġtreat ment +e ed +i ÄĻ +à ¹ +à® ¾ +Ġequ ip +Ġplan ning +Ġsol ve +ãģ Ŀ +ĠT om +Ġavo id +Ġp ou +Ġgreat er +l in +O L +ĠL u +ĠM ore +Ġatt ract +ê n +un a +Ġphot o +er ation +Ġplan et +Ġcop y +Ġvis ual +ir ing +Ġintern ational +Ġla ughing +Ġth ick +Ġhold ing +Ġbring ing +Ġlet ter +Ġb urn +Ġeffect s +it é +our s +O T +ê me +ĠSch ool +×ķ× ª +rop ri +l ig +α ι +Ġad ult +Ġsu gar +Ġr ide +Ġhigh light +Ġno body +Ġ2 1 +Ġch at +ĠпÑĢ Ð¸ +Ġin nov +ung en +Ġatt ach +ed om +å Ĭ +y l +Ġleg al +Ġr ice +Ġcoll abor +k ing +d own +æ Ļ +ãĤ Ĭ +Ġi h +ĠA c +ous ly +Ġr ap +Ġsol id +Ġgener ally +Ġpatter n +al i +à¸ Ń +Ġtrans l +in ter +a ult +Ġë ¨ +Ġexp ress +Ġexam ples +Ġch ose +Ġtell s +ÃŃ s +ain t +ĠT ell +ĠMich ael +æ ¨ +ĠN umber +Ġt ap +Ġexper iment +Ġbenef it +Ġì ° +Ġse qu +Ġexp ensive +Ġgener ation +ĠM any +Ġadd ing +Ġk il +Ġcamp aign +ĠA nt +ra w +omm en +Ġs oul +j o +ĠAct ually +am m +ê² ł +Ġma xim +Ġsal t +Ġc ru +Ġcall ing +ãģ Į +Ġbas is +b an +Ġkeep ing +ĠM or +ed s +ì Ĩ +Ġto do +ам и +н Ñı +Ġli ved +ĠD u +ãĤ ī +å® ¶ +for ce +å¹ ´ +fer ence +al a +Ġocc ur +s k +Ġrec ent +Ġc ars +Ġtrad itional +ent le +² Ī +Ġhel d +Ġn ach +ĠCent er +er en +Ġb in +Ù ģ +Ġcomm e +Ġre ve +Ġìĺ ¤ +Ġexpect ed +ab il +Ġfocus ed +o v +Ġi P +or ial +i ro +Ġet c +am ing +ĠS on +Ġy esterday +Ġstr ate +ĠÑ Ĩ +Ġë ı +p es +Ġactiv ity +Ġadv ice +Ġopen ing +f in +Ġre la +é ĸ +Ġinst ance +ĠEvery one +b l +p en +Ġvis ion +ĠA lex +if orn +Ġt ick +H e +Ġstrate gy +Ġk om +P E +ĠG l +Ġelect ric +1 5 +Ġda ily +Ġhus band +Ġst ation +Ġanal ysis +yn am +Ġatt empt +Ġbill ion +v ant +Ġfor th +Ġm ath +al y +Ġbehav ior +ĠM as +k an +ĠD ay +Ġbl ess +Ġg ut +ĠH igh +o x +Ġd ress +Ġj ed +è ¯ +å ĸ +Ġexperien ces +ist a +Ġfight ing +å · +ĠÑģ к +Ġmost ly +a use +Ġpict ures +ен ÑĤ +Ġm ad +Ġmod els +ÑĪ Ðµ +ĠC ount +Å Ħ +ÅĤ o +ep t +O M +ĠA N +Ġtrou ble +4 0 +Ġb ird +ul ate +Ġm ur +Ġprodu ce +Ġmar ried +b it +Ġthe ory +í ĺ +Ġlead er +ĠL ast +A A +è µ +Ġim ages +Ġexp and +ĠP or +Ġpur ch +ĠS an +ĠChrist mas +ĠAust ral +Ġw id +ĠM iss +Ġknow ing +Ġz e +s hip +k u +Ñħ од +ĠInst agram +ĠInd ia +Ġest a +ĠCal iforn +Ġ7 0 +Ġdra g +Ġbr ush +Ġn ames +A nd +Ġy o +ill a +Ġsch ed +Ġdest roy +ye ar +Ġv amos +Ġ ÙĦ +ç a +Ġforg ot +и е +Ġra ise +re me +íķ ´ +ĠG ive +Ġcont ain +ra b +Ġg ift +ĠÑģ п +Ġrequ est +Ġsh ut +Ġdeg rees +Ġbenef its +Ñĭ е +Ġstud ies +Ġend s +Ġevery where +Ġher o +op h +er ry +Ġmaterial s +en ed +N A +å į +Ġmu y +Ġwor se +ä» Ģ +ĠM ad +Ġdec isions +ion e +Ġfore ign +la ughter +i ber +ени Ñı +ãħ ĭ +Ġreal ized +Ġ ign +Ġwe ak +ĠÎ ¼ +Ġsca red +Ġass um +A K +ï ¿ +ï¿ ½ +Ġcover ed +ĠS at +Ġо н +Ġindividual s +Ġcomp ared +1 1 +ĠAd d +ic les +Ġc ert +r ar +Ġbr ief +Ġactiv ities +Ġf ab +b ar +Ġa st +ĠO ther +Ġclass es +Ġo g +Ġmiss ing +ãģ ł +é Ŀ +w ers +× © +Ġintrodu ce +Ġequ ation +ãģ¾ ãģĻ +Ġn om +Ġpain ting +us hing +ĠA P +Ġencour age +Ġsh ip +itt ee +iver se +ot a +n am +ãĥ » +Ġexerc ise +ĠÐ Ń +Ġn as +Ġthous ands +ĠCaliforn ia +Ġs es +Ġr ow +ŀ Ī +Ġpand emic +Ġsk ill +b el +Ġdire ctor +Ġmil k +Ġn ut +Ġmot ion +Ġcl osed +è ¨ +Ġcred it +ah r +Ġche ese +Ġal tern +im ately +Ġs ust +ĠT ra +Ġgl ad +Ġhigh ly +Ġw a +Ġredu ce +Ġb le +ad or +in ated +ion es +ci ent +Ġdep ending +Ġsh aring +Ġca ught +ra el +Ġme hr +Ġpass ion +ç Ľ +Ġr u +Ġfar m +T I +av es +ĠR ob +ĠB ro +Ġmot iv +ret ch +ru pt +ĠB ig +Ġall e +Ġet t +ub s +ĠJapan ese +ĠH all +и ли +AUDI BLE +ç ¬ +Ġcell s +ik a +el ine +il er +Ġì £ +Ġsk y +IN AUDIBLE +end e +ap ter +Ġp in +Ġg ather +h ol +le ction +Ġsy n +Ġpl ug +r ound +Ġun iversity +h ib +Ġfant astic +k n +Ġho le +ĠRem ember +in ct +ak s +C H +Ġbro ken +Ġstr ateg +Ġal ive +Ġt ank +Ġc art +r ated +r ie +ĠSt ep +ĠEvery thing +Ġb ound +Ġso bre +Ġcustom er +¡ Į +ur g +ĠB ill +L a +wh at +Ġre action +Ġs ession +Ġpl ans +ĠìĿ´ë łĩê²Į +Ġdown load +ì Ļ +u er +Ġc ab +Ġinst r +if ying +ĠN ice +Ġteam s +ı l +Ġgo als +is ch +Ġtrans port +Ġanim al +Ġcost s +Ġcall s +Ġse hr +ì Ī +ri an +Ġd ial +Ġwe ather +à¹ Ģ +Ġв оÑĤ +ĠPl ay +Ġsh ared +Ġsm ooth +ab a +Ġleav es +à® © +Ġconc ent +Ġsh ift +ĠëIJ ĺ +ĠGo vern +Ġdem onst +Ġbut ter +ĠìĹ ¬ +Ġsat isf +Īë ¬ +Ġrecogn ize +ĠF rench +Ġvol ume +ä nd +Ñĥ м +Ġì§ Ħ +ĠKe ep +ow a +ipp ed +ÑģÑĤ ÑĢ +Ġdet ect +ĠÏ ĥ +Ġl ift +Ġcl othes +ĠSt op +à µ +m et +Ġcl in +Ġar r +f riend +Ġst uck +Y e +h and +um a +Ġsc ri +Ġfuck ing +ct ors +× ª +Ġjo ining +Ġc ette +ĠØ £ +ĠWh ite +Ġi hr +Î Ń +ãģ Ń +Ġinclud ed +ess o +Ġac ad +b um +Ġs ab +Ġд лÑı +è¿ Ļ +uf act +ĠRep ublic +r im +Ġye llow +Ġlim ited +T ER +ĠT y +Ġnot es +v est +и з +al ed +Ġph ase +and a +ĠM om +R I +Ġim mer +m al +Ġin j +Ġy ang +ud ible +аР³ +Ġset t +Ġmag ic +Ġens ure +Ġsp ring +Ġsh ock +Ġwhe el +ог да +ãĤ Ī +Ġcan cer +Ġro ot +Ð IJ +gen cy +Ġë į +i i +Ġout put +Ġcomm it +Ġwork ers +ìķĦ ìļĶ +ĠÑģ ам +ve y +Ġpe u +Ġc ivil +is c +Ġbr ings +ÑĢ Ð°Ð² +an ia +Ä ģ +c raft +mb ol +Ġintell ig +b i +ac ing +y ou +Ġbecom ing +ĠD er +em a +å°± æĺ¯ +Ġing red +Ġcomm and +Ġupd ate +Ġpre m +Ġopen ed +Ħ ¤ +ени е +Ġg ard +Ġstat ement +Ġsc rew +Ġpr ote +Ġc ards +Ġt ask +Ġeven ing +Ġst itch +in en +ĠB er +m ark +ĠD ad +Ġе ÑģÑĤÑĮ +Ġ× ŀ× +ìĹ Ī +Ġb an +Ġcl im +Ġfre edom +Ġnorm ally +еÑģ ÑĮ +å ¦ +Ġprov ided +Ġìŀ IJ +ĠìķĦ ëĭĪ +ĠK im +ied er +ìĿ Į +Ġcit iz +Ġb ike +Ġb ak +Ġno ise +Ġcl imate +iz es +å¾ Į +Ġincre asing +ĠTH E +Ġli qu +Ġperson ally +e f +res p +Ġleg s +ind er +Ġp ed +Ġë§ İ +Ġdep end +Ġvar iety +ĠIs rael +Ġwas h +å Ĩ +Ġqu iet +ĠJ ames +ĠJ ew +Ġfore ver +ĠI nt +Ġcoun ter +ur ance +ĠAny way +ca re +ĠOn ly +ci ón +ad i +ĠE v +ëĭĪ ê¹Į +ĠÎ ± +Ġslow ly +Ġо д +Ġnot iced +ier en +Ġfe ll +ĠÐ ij +Ġm ême +Ġwhen ever +! ) +ĠH y +å ¼ +ord s +us ion +ĠSt ar +Ġí ĺ +ĠM ac +ä¸ Ĭ +i ven +Ġìĭ ľ +ĠìĹ Ĩ +ĠT ur +Ġg er +r is +Ġve z +Ġл Ñİ +Ġvers us +ا Ø +ocol ate +Ġplan e +Ġz o +Ġsu it +Th is +Ġn erv +ĠA cc +Ñĥ ж +ìĤ ¬ +n h +em e +Ġa uss +Ġme as +Ġtr ès +Ï ī +Ñģ ли +ĠAr t +ĠSec ond +олÑĮ ко +ch o +it ect +е ÑģÑĤ +Ġb oss +Ġinc ome +ł ¤ +Ġsh ad +Ġapp ropri +ĠM al +op t +Ġart ist +Ġplay s +oth ers +ĠIn ter +Ġvir us +Ġh ung +Ġconst ant +Ġscri pt +Ġsn ow +ul f +k et +Ġdev ices +Ġmet al +ight s +ìĦ ¸ +Ġsal es +Ġve get +Ġcollect ion +Ġv ia +k er +Ġgot ten +O W +i én +Ġacc ur +Ġw ave +ult y +ĠA ir +Ġlead ing +ic ing +Ġcent ral +ĠChrist ian +f r +ĠAl though +Ġsong s +Ġf if +нÑĭ Ñħ +Ġbel ong +oss ible +ì ° +Ġphot os +is l +Ġrela x +s a +US IC +ê · +Ġman ufact +ĠTw itter +Ġdanger ous +Ġhy d +le ar +i ant +ĠâĢ ¦ +Ġsudden ly +Ġla ugh +Ġang le +ĠG ot +Ġwor ried +о е +Ġp ap +ĠM art +en o +Ġbatter y +Ġп оÑģ +Ġlight s +Ġar ms +ĠA bs +m es +âĢ ĵ +use um +Ġte a +ĠM ic +Ġfor mer +ograph y +Ġapplic ations +ĠD ire +çĦ ¶ +Ġfeed back +itch en +yor um +u ed +ig t +ư á» +os ition +ĠD el +Ġíķ ĺë +ĠB ack +ad s +Ġpr ime +ì£ ¼ +ì£ ł +× ij +Ġm ut +] . +ĠÐ Ĺ +lo c +k in +Ġexper t +Ġal right +ung s +Ġsupp ly +Ġleaders hip +ĠF ra +Ġtyp ically +Ġs el +Ġtre es +Ġ2 2 +h ar +Ġwor st +Ġbus y +ant o +ĠU p +ĠB as +Ġpresent ation +Ġstr ange +Ġth in +ÑĤ е +Ġveh icle +Ġд о +cell ent +7 0 +Ġt ired +Ġcris is +Ġt iny +as y +Ġr an +é ĩ +Ġfor ces +Ġо Ñĩ +Ġident ify +Ġass ess +иÑĤ е +S E +Ġcreat ive +ç Ł +Ġdep artment +Ġinit ial +æĪij åĢij +ĠD am +ak t +v ere +Ġinf ect +Ġp ump +Ạ¡ +Ġv iel +Ġr are +Ġd ot +ash ion +em pl +Ġf lex +Ġk on +Ġtr uck +Ġle ct +Ġpl astic +la w +Ġlik es +Ġr ough +ĠM AT +í ŀĪ +Ġcomm er +Ġas se +Ġc ake +Ġact ions +Ġad m +Ġother wise +ĠHe alth +Ġcoll e +à¹Ģ ภ+Ġr ub +å¾ Ĺ +æ Ķ +Ġsc r +Ġz um +ĠH im +Ġch amp +Ġconcern ed +Ġ5 00 +Ġpl ate +ĠO ut +Ġdon c +Ġequip ment +Ġta ught +ll ed +Ġí Ļ +iv a +Ġmot or + » +Ġgu ide +å ī +Ġstop ped +Ġr at +Ġlab or +Ġa im +Ġprep are +ĠÑ Ī +Ġshoot ing +ann ed +cri pt +Ġen emy +Ġdep ends +Ġn av +Ġb er +Ġland s +Ġun ivers +i u +Ġfact or +ok ing +Ġcar bon +b ut +ĠL ove +el d +ĠÎ µ +Ġg a +Ġé s +Ġbre ad +Ġvol t +í Ĭ +Ġwas te +Ġkeep s +æī Ģ +Ġst or +Ġhon or +Ġun less +Ġcol um +Ġë ĮĢ +Ġpl ants +Ye ah +Ġinclud es +ä¸ Ń +Ġo x +Ġpe ut +ë§ Į +ìĥ ģ +ist ry +ภ± +ĠDep artment +ant a +Ġfing er +Ġst retch +Ġsy mbol +Ġneigh bor +æ ¬ +ê° Ħ +~ ~ +ĠÑĤ Ñĭ +ĠA ber +k es +Ġmass ive +ĠC H +ĠS al +× ł +ãĤ Ĵ +Ġd ynam +ach e +ĠP re +Ġmon itor +ent ed +E O +Ġrais ed +ist ics +Ú © +Ġv ou +it en +¡ ° +Ġbusiness es +Ġe arn +Ġmob ile +id ade +Ġha be +y r +l ict +Ġcon duct +Ġfed eral +Ġw o +b u +Ġn one +Ġteach ers +ĠاÙĦ Ø +éģ ĵ +id ents +ا ÙĦ +Ġtre nd +еР¶ +Ġal bum +Ġm ich +b ased +ภµ +Ġtrans ition +Ġн о +õ es +h ost +ed y +ĠPro f +p an +ij n +Ġcapac ity +und o +Ġ× ij× +Ġbreat h +Ġм ен +Ġm ü +í Ļ +ĠA ut +hing ton +Ġn or +Ġg ain +po int +Y es +ĠØ ª +ĠN a +Ã¥ r +Ġi ç +ĠM ary +Ġsp in +Ġant i +åIJ § +Ġsome how +Ġlaw s +Ġmom ents +Ġg re +Ġmo ves +ĠW ould +Ġpred ict +Ġv ra +Ġ201 9 +¶ Ħ +Ġfund ament +2 5 +Ġp ure +Ġw ow +Ġis land +Ġinvest ment +Ġb ath +ĠY a +Ġhard er +Ġt ips +å Ĺ +Ġelect ron +ĠB ob +Ġb ond +od ies +ĠA ug +Ġgib t +Ġch air +Ġtw ice +w ood +Ġcl ar +Ġmas k +Ġhonest ly +Ġ201 8 +t ies +' , +Ġp ens +Ġsurpr ised +Ġcommunic ation +ãģ£ ãģ¦ +Ġsp r +Ġwh ose +Ġst ars +× IJ× +ĠâĢ ĭ +Ġproper ly +Ġg rew +os ing +Ġdi vers +A D +Ġem pt +Ġexp ression +Ạ¿ +ĠP al +ãģ Ĭ +Ġjust ice +Ġp air +w o +Ġse at +or ter +Ġlink s +ĠM er +Ġre nd +но е +up id +ĠH el +ĠM arch +ĠL o +Ñģ ÑĮ +Ġhas n +Ġev alu +ãģ ı +å¤ © +il os +Ġfund ing +Ġv en +u an +ĠM aster +ĠO l +ĠF re +Ġy ap +ĠS ir +s ch +Ġmist ake +am an +Ġdin ner +ĠWas hington +Ġorganiz ations +Ġж е +av ing +Ġv ÃŃ +Ġbirth day +Ġbe ar +ĠÙ ģ +Ġaff ord +Ġre ven +Ġrelationship s +r ough +ĠT ime +Ġt ag +ĠS un +u ary +ĠP o +c ar +ab ilities +Ġpr ison +Ġl ic +ìł ķ +id den +Ġspec ies +é » +Ġf irm +Ġsc ore +Ġd it +Ġspe ct +Ġp el +Ġcompl icated +æ¨ £ +Ġr ank +Ġoppos ite +Ġpick ed +Ġк он +el er +Ġm ig +ĠS l +ĠN et +Ġne ck +ĠFr ance +Ġtechn ical +ภ¡ +Ġmil es +Ġprim ary +Ġse in +s es +Ġla ughs +b ra +ÅĽ ci +ri age +Ġn ic +et ers +Ġà ª +olog ies +ĠI S +r ad +ud o +ı nd +m ar +Ġex ch +Ġcompet ition +Ġauss i +ĠS erv +Ġre nt +Ġch ocolate +Ġw ieder +Ġnear ly +Ġspe ech +Ġun c +Ġpar am +ĠBrit ish +Ġrem ain +ภģ +ur t +ĠØ ¹ +Ġcr ack +ail s +Ġprom ise +Ġpay ing +i ÃŁ +Ġad apt +ал а +Ġmov ies +Ġw ire +Ł ¬ +æľ ĥ +Ġter rible +Ġs ó +Ġperfect ly +åij ¢ +ord in +Ġj á +Ġimp ossible +ĠTh ree +Ġn h +Ġtur ning +r um +ĠB el +ig g +Ġrespons ible +и й +Ġincred ibly +w i +ian o +Ġhum ans +Ġà ĩ +Ġsetting s +Ġj oy +o ot +Ġdeal ing +ill ed +Ġsur round +Ġfollow ed +Ġposs ibly +Ġinit i +st en +Ġpr os +Ġcand id +Ġass ign +Ġviol ence +W ell +Ġr ise +P S +Ġtamb ém +Ġë ĵ¤ +i ance +y an +Ġaud io +ĠB et +ĠAmeric ans +ĠAs s +is chen +ìŀ ħ +Ġult imately +Ġpol ic +Ġmajor ity +éĢĻ åĢĭ +ĠFin ally +er ap +Ġgu ard +ĠMAT T +Ġbr own +м и +Ġch a +ĠHo ly +Ġnerv ous +ipp ing +ÄĻ d +ĠS a +ĵ ľë +¶ Ģ +l ie +çľ Ł +Ġn uc +ĠA pr +é Ľ +ĠKore a +eg o +ĠCan ada +Ġkön nen +Ġcomp ar +Ġg anz +ĠM ais +Ġthem e +Ġk i +Ġdraw ing +az on +ĠO ff +t t +ĠW ind +Ġtod os +Ġob vious +на Ñı +I M +ĠÐ ł +we ll +Ġbl ow +Ġho ok +Ġcir cle +Ġë³ ´ +Ġarch itect +ĠK r +Ġc ó +Ġprotect ion +eg a +å ĩ +Ġwatch ed +Ġans wers +Ġdi et +iv o +Ġpow der +Ġyour s +Ġhigh est +çĤ º +F F +å º +Ġbo ys +ö yle +Ġl unch +è¬ Ŀ +ĠI I +Ġset s +Ġmo le +Û ģ +Ġwin ter +Ġluck y +Ġrespons ibility +Ġsign al +Ġwond ering +Ġa x +Ġcook ing +ов оÑĢ +le g +Ġп оÑĤ +Ġsurpr ise +Ġdem ocr +Ġlo op +Ġj ag +Ġcur ious +Ġmarket ing +Ð Ŀ +ar on +ĠApp le +Ġvirt ual +Ġ19 8 +no on +ĠM et +оÑģ ÑĤо +об Ñĭ +it u +ĠA w +Ġbu ying +Ġrestaur ant +ĠB ud +Ġdou bt +Ġgr ant +Ġver d +Ġc ash +Ġfac ulty +Th at +ĠE in +å¤ ļ +Ġw ed +it ness +ĠM ag +n el +Ġn arr +Ġacc ident +Ġmed ium +em ents +Ġcr ow +n ight +ìĿ ¼ +ä¹ Ł +Ġlibr ary +аÑİ ÑĤ +Ġtamb ién +Ġrefer ence +Ġfour th +h ouse +v ention +Ġfill ed +ĠC our +ib r +Ġn g +Ġdevelop ing +Ġprov ides +Ġpo ll +Ġtra ffic +arent ly +à® Ł +Ġform s +Ġcl ient +Ġg entle +Ġmus s +ĠCong ress +ĠInd ian +ce an +Ġp il +Ġc zy +st ood +ut y +Ġn ä +Ġsp ending +Ġconst ruction +ina udible +Ġë§ Ī +Īë¬ ´ +Ġìĥ Ŀ +om a +os en +ag o +Ġlar gest +ãħĭ ãħĭ +Ġun iverse +b es +os a +Ġе го +Ġd ude +ĠM AR +Ġind eed +ε ι +Ġman aged +ĠSh ould +S o +Ġappl ied +Ġfair ly +ĠD en +Ġanal y +Ġconst antly +Ñģ п +H ow +ĠS ay +en cies +ĠP C +Ġegg s +à® ° +Ġet h +ĠEnt ão +in ar +i ot +Ġc z +ĠEurope an +ãģ Ī +ĠA M +Ġc á +Ġrad io +§ Į +Ġh ide +ä» Ĭ +ĠSt art +Ġcl ub +ĠH ope +Ġeff orts +lus ion +Ġc ities +h one +Ġreach ed +Ġgu id +ro id +Ġhar m +Ġcut ting +Ġb ul +1 8 +i est +ĠMe x +Ġ iron +çŁ ¥ +Ġafter noon +Ġha ll +Ġpr zy +Ġg osh +Ġinflu ence +Ġв ид +Ġincre ased +ĠMin ister +Ġdis ci +ĠP eter +Ġver t +Ġmen u +Ġse lling +ur ally +Ġqu ote +Ġ ¡ +Ġcontin ues +mp re +ĠÅŁ ey +it ution +Ġна Ñģ +c les +ĠGerm an +c zy +ĠÐ £ +B e +Ġk itchen +ĠT ry +i pe +Ġic on +ar p +Ġprov iding +ĠTr ans +Ġtechn ique +Ġh är +Ġinf rast +Ġsus p +ü ck +ic ip +ĠÐ ķ +Ġc in +ìĸ ´ë +Ġpr z +Ġcompon ent +Ġby e +ĠB ible +iz er +C h +Ġsol utions +Ġaccom pl +Ġ201 6 +I E +ĠT a +Ġass ume +Ġliqu id +Ġë¨ ¹ +Ġquar ter +Ġfem ale +ĠTh ink +Ġstat us +it ute +Ġco ach +Ġre in +Ġcomb ination +è · +ĠT er +Ġobject s +Ġdist rict +Ġmake up +Ġmur der +w as +f en +Ġbow l +Ġpub lished +Ġsp orts +ãģ ¡ +Ġident ity +Ġseem ed +Ġact ing +л Ñİ +ri x +Ġup load +Ġh ast +Ġbo at +ĠM od +ri o +Ġ = +Ġcy cle +¯ ¸ +Ġl oud +ust ed +com ing +Ġ201 7 +Ġon t +Ġleg isl +Ġst ruct +ĠSomet hing +Ġconf lict +Ġu pper +Ġman ager +Ġm ort +Ġf ra +ĠÄ ° +ĠM ike +ĠW ork +Ġn ó +ph ere +ĠìĤ ¬ë +ĠL and +Ġfil ter +Ġprom ot +æ ° +æĻ Ĥ +ķ ¼ +Ġrecord ing +× Ŀ +Ġassoci ated +Ġf uel +und er +Ġele ction +Ġemploy ees +ĠCom p +ÑĢÑĥ г +ĠW o +ro l +Ġsa ved +ĠH on +ĠV i +åĪ Ĩ +ac a +p ret +Ġw et +Ġst upid +Ġl ad +Ġf est +Ġw ake +Ġи н +Ġgreat est +ĠJ im +Ġserious ly +Ġì ¹ +Ġfeel ings +Ġ3 00 +i ation +Ġbeaut y +Ġìŀ ĺ +Ġs an +ĵ ł +Ġ- ( +Ġcons cious +Ġд ел +b ye +ç Ļ +M an +Ġlet s +Ġsho es +y d +ä¹ Ī +Ġdisapp e +ĠCount y +ĠSc ott +Ġbut t +Ġaqu ÃŃ +Ġconf ig +resp ond +LAU GH +© ëĭĪëĭ¤ +Ġdivid ed +Ġac qu +Ġz one +Ġk omm +a ção +ì§ ľ +c ut +Ġ2 3 +Ġmaxim um +ro g +Ġrun s +Ġcompon ents +Ġarri ved +Ġconf ident +ÑĢ Ð¾Ð² +Ġhe ight +Ġpro ced +E M +ĠÐŃ ÑĤо +ĠM en +Ġtalk s +Ġconf idence +ĠChr is +Ġlead s +Ġn ose +f all +b b +ĠNot hing +is er +Ġindepend ent +Ġmin or +Ġsy m +l en +ci ence +Ġf ashion +Ġsex ual +Ġb un +h ere +Ġso il +Ġdies e +Ġsh ap +Ġempt y +Ġjour nal +ag on +ĠThe ir +Ġweek end +ÃŃ t +Ġer ror +Ġn ar +à ¸ +è © +an cy +Ġìķ Ĭ +Ġfore st +Ġha cer +Ġmiss ed +ãģ ķ +åı¯ 以 +Ġev il +Ġstor age +Ġsing ing +in ha +Ġkn ock +Ġimp ress +ĠоÑĩ енÑĮ +ĠGo ld +ĠS ur +ĠP ort +åİ » +ĠL ond +Ġfaz er +ot y +ot o +Ġan x +ĠWill iam +Ġexist ing +pl ace +ĠC D +Î ³ +ĠColl ege +l or +ĠE ast +s en +f ach +o ft +Ġexperien ced +Ġlo ves +im m +Ġpo ly +Ġes se +ì ¤ +ĠG rand +è § +ch er +Ġvict im +ĠG es +л ÑĮ +v ision +Ġt all +Ġl ens +Ġз на +ĠB oth +Ġì ² +Ġsust ain +Ġarg ument +Ġfact ors +Ġautom atically +Ġfr uit +Ġli ber +Ġa le +ĠP ress +ĠB a +ĠÐ ³Ð¾ +Ġhundred s +th at +ĠR ich +Ġreci pe +ĠI T +è ĩ +Ạ¥ +Ġdescri be +Ġdri ver +ĠO ct +ĠM at +д е +Ġme al +Ġlat est +Ġth erap +Ġcomp are +ĠAm azon +Ġì¢ Ģ +ĠRuss ia +Ġstr ing +Ġk a +ĠComm un +Ġd ia +I s +Ġmill ions +Ġcor por +Ġcor respond +Ġfix ed +ĠJo e +Ù İ +Ġview s +Ġr iver +Ġstud io +ig ger +Ġfl avor +Ġpres ence +Ġun its +Ġsa ving +av our +Ġp esso +or ith +Ġh ers +ĠN at +as ion +ĠFr ank +о ÑĪ +ÅĤ y +í Ħ +Ġein em +Ġfun ctions +um an +Ġn orth +Ġìł Ħ +Ġhor se +v id +Ġple asure +а ÑĪ +é es +ind a +Ġt ail +Ġexpl ore +S T +Ġcommer cial +ĠD uring +ar l +] : +f it +Ġr ates +æ ³ +M USIC +Ġhous ing +Ġein er +Ġsitu ations +æ ĭ +Ġdec re +Ġappropri ate +ен но +% . +Ġb ac +Ġw at +ens ity +ä h +kn own +it z +Ġemot ional +erv ation +Ġbl ind +1 6 +í ĥ +大 å®¶ +Ġjo ined +Ġloc ated +ĠÑģ м +ad as +ber g +Ġd ess +Ġde ar +ed en +c os +Ġad opt +1 00 +ow e +ĠChe ck +ism o +Ġsim pl +Ġang ry +Ġмен Ñı +ĠC am +Ġp ad +Ġatt end +Ġsam ple +æĹ ¥ +Ġì Ľ +ĠI N +ul ous +ĠS ar +ĠSh ow +Ġinfrast ructure +ĠAug ust +Ġless on +Ġn iet +æ İ +Ġfo i +Ġbro ke +t r +ç ķ +Ġ4 5 +Ġg ew +Ñĥ п +at i +Ġmaint ain +Ġart ists +ing er +æĿ ¥ +er ved +I A +Ġequ als +Ġoper ation +ill y +ĠëĤ ´ +Ġcrow d +Ġintern al +Ġtest s +ĠR ock +ĠC ons +ĠëĦ Ī무 +w ar +Ġs ou +Ġch art +ĠJ une +ĠApr il +g ent +Ġv ent +Ġqu and +ĠKore an +im o +ç ī +id ers +Ġmount ain +ÑģÑĤ ав +æľ Ī +ij k +Ġdiscover ed +ĠS und +ĠS il +Ġso lo + ´ +Ġsch ol +ĠE ach +ç µ +Ġb are +Ġí Į +ĠvÃŃ de +Ġingred ients +ĠIt s +Ŀ¼ ê³ł +Ġì Ĭ +Ï į +ĠLe e +Ġsc ary +Ġprinci p +Ġspirit ual +ì ħ +ĠH old +æ²Ĵ æľī +Ġdef ine +ĠL es +ĠN or +ĠE nd +Ġbl og +ĠG reen +аеÑĤ ÑģÑı +p art +el es +äº ĭ +ĠUnd er +Ġpart e +Ġ3 5 +Ġse ctor +ĠS ept +Ġaut h +à® ® +om in +Ġcl ients +Ġc i +ĠFr iday +er as +Ġtw e +ul ated +Ġcult ural +ĠÑģв о +Ġëį Ķ +Ġà º +Ġpar ce +à® ² +Ġtrad ition +Ġjud ge +ĠGen eral +Ġdeterm ine +ĠIs n +ĠP L +ne ath +Ġmatter s +íķ ´ì +! ] +а Ñħ +Ġpo ol +Ġvari able +Ġvacc ine +Ġcaus ed +Ġw est +ĠY ep +f ast +Ġph ilos +hor a +Ġcontinu ed +Ġunf ortunately +ãģ į +æ ķ +Ġfl ight +Ġw rap +Ġhu h +ĠAbs olutely +Ġp ink +Ġrem ains +Ġn é +Ġf le +ĠS ol +Ġlos ing +Ġalg orith +Ġrequ ires +Ġfound ation +ĠB ur +Ġprofess ion +ĠM id +Ġë ŃIJ +c an +ĠM il +Ġyoung er +Ġappe ars +ter m +íķĺ ê³ł +ac le +ĠLond on +Ġengine ering +ภ¢ +Ġadv ent +ìĦ¸ ìļĶ +Ġê¸ ° +ĠM aj +ÑĢ ÐµÐ¼ +ing u +ĠU K +u ro +s pe +Ġt ent +Ġreport ed +ĠA L +H ey +Ġë§ IJ +Ġd ent +ĠAustral ia +ĠJan uary +³ ´ +ag ues +ars h +r ig +Ġtien e +ภ£ +Î ® +Ġmach en +un te +Ñĥ Ñģ +Ġelect r +Ġtut orial +Ġpl aced +ĠìĿ´ ê±° +ĠCoun cil +í ĸĪ +°ë ¦¬ +ah ren +Ġê·¸ë ŀĺ +Ġpro ve +f ol +Ġqu er +Ġche ap +ĠF ather +ĠP ower +ĵ ľ +Ġpur s +Ġes p +ĠB re +ê¸ °ë +om as +æĥ ³ +ил ÑĮ +Ġge ht +os ter +ê³ ¼ +Ġfil es +ĠÐ § +be ll +Ġwh om +Ġë ĺ +Ġex cellent +Ġdat ab +Ġg ö +Ġì§Ħ ì§ľ +Ġbelie f +j et +Ġj ack +Ġsw im +ri al +um in +a uc +Ġso ll +Ġess ential +íķĺ ëĬĶ +Ġev ol +cha ft +ain e +th let +Ġinc or +Ġreport s +Ġdefin ition +ke l +Ġcirc um +Ġprodu ced +Ġ× Ľ +ant ic +n et +Ġa ward +Ġd urch +Ġtrans p +Ġm ale +¦ ¬ë +Ġmo on +ĠGe orge +Ġfly ing +i ó +Ġs ources +Ġpl enty +ĠDem ocr +R O +Ġ 00 +Ġsec ure +ĠB ir +ra in +Ġz ur +Ġeffic ient +Ġrepe at +Ġmethod s +Ġcal m +Ġdiscuss ed +ĠìŀĪ ëĬĶ +Ġser ver +an ie +ĠInst ead +Ġide al +Ġcon ven +Ġhop ing +ĠT or +Ġdep th +Ġhe aven +EN CE +Ġhab it +gr ad +Ġfl ag +Ġin e +Ġk h +ĠL I +Ġfac ing +ĠA U +ĠT im +Ġg em +ĠJ ul +Ġel a +iz za +Ġfe llow +Ġqu el +Ġsp oke +Ġcitiz ens +u ge +é ĥ½ +Ġp ages +Ġf asc +Ġrelig ious +at en +Ġch apter +ĠV al +Ġcons ult +ĠM ill +g l +op er +Ġinf in +Ġmar riage +Ġmedic ine +Ġд в +Ġdog s +Ġinstr ument +ĠEx act +á n +Ġ20 21 +Ġf er +Ġwe alth +Ġgr ade +Ñĭ Ñħ +Ġcr ime +Ġth read +Ġess a +Ġw ine +co hol +ph a +ภĩ +og ue +Ġins urance +arr ator +ĠSept ember +Ġv id +ĠSp irit +Ġg est +ĠRuss ian +Ġproper ties +Ġart icle +Ġunder neath +y er +Ġjo int +Ġrelative ly +Ġin ch +Ġdesp ite +ĠG ree +Ġclass ic +Ġsupport ing +Ġinst ruct +lus ive +Ġdi agn +æ Ĭ +Ġadminist ration +аб оÑĤ +ĠO pen +æīĢ ä»¥ +Ġп ок +Ġdoll ar +Ġconse qu +o ber +ĠGerm any +Ġter r +ĠQ U +ĠÐ ĵ +ç ¾ +Ġstrong er +É Ļ +ĠÙ Ĭ +ĠiP hone +Ġfab ric +ü h +Ġen em +æ ¯ +Ġsub t +E E +ond e +Ġcre w +Ġremo ved +Ġl ady +Ġpot entially +ĠÐĿ о +y al +Ġsym pt +Ġar my +Ġintrodu ced +t es +Ġaspect s +1 4 +ĠL ou +Ġ ) +Ġde ploy +p et +Ġh an +ĠW atch +Ġweap ons +Ġph en +Ġreg ister +Ġein fach +Ġsp ort +Ġbr idge +Ġin ner +Ġminim um +Ġw itness +Ġes o +Ġvill age +Ġown er +¦¬ ê³ł +Ġsc ream +il ed +Ġp itch +b ru +Ġadv ance +ä¸į æĺ¯ +Ġsupp ose +ĠAt t +еÑĤ ÑģÑı +Ġdiffer ences +ak ed +Ġinter pret +à ¦ +iend o +Ġabs ol +ĠбÑĥд еÑĤ +Ġë ² +Ġtri al +Ġthink s +ly ing +cept ion +ĠAfric an +Ġchem ical +Ġta pe +Ġconvers ations +Ġdistrib ution +t i +ĠA I +Ġfl ash +Ġunder stood +ĠGovern ment +å° ı +! ? +ĠS k +ê± °ë +ri er +T S +ĠAcc ording +Ñİ ÑĤ +Ġsp ons +ÑĤ обÑĭ +Ġval u +ere m +icht ig +Ġresist ance +ĠG al +ger y +Ġbeg ins +Ġadv anced +Ġrele vant +Ġpolit ics +ĠF am +Ġç ok +ĠN ever +ill ing +Ġfoot ball +и и +ĠI D +ĠAfric a +Ġfing ers +Ġб олÑĮ +Ġà ¡ +Ġcl ip +ĠL at +ãĤ Ħ +Ġì§Ģ ê¸Ī +es se +Ġvo or +Ġas ide +æ ŀ +Ġto ward +Ġb at +Ġval id +ĠM ens +Ġcomplet ed +ı ÄŁ +Ġpod cast +ĠB on +Û Ĵ +ĠJ uly +il a +Ġpack age +Ġpull ed +ch ar +ĠM el +o is +Ġs outh +Ġë Ķ +Ġimport ance +Ġp ushing +Ġis ol +Ġstand s +c ill +ä ¼ +Ġ ðŁ +or i +ê° ģ +Ġhom es +Ġconcern s +Ġb iz +å ½ +b ie +Ġb is +Ġge ar +ĠM S +Ġh un +ĠM att +Ạ£ +se y +ĠSec ret +Ġod d +ĠM ax +oll y +f ord +ĠS H +Ġrepl ace +Ġnav ig +Ġin i +и Ñı +Ġgi ant +Ġma nd +ĠH app +TI ON +g un +iam o +ìŀħ ëĭĪëĭ¤ +Ġg ap +Ġê tre +Ġclass room +Ġhy p +ak i +è ® +is ters +ack s +ĠÑģ о +Ġb ug +Ġgra v +am in +Ġevery day +Ġì ¡° +Ġgard en +ce mber +Ġest o +åĹ İ +Ø ¬ +Ł ° +å ģ +Ġr om +Ġìłľ ê°Ģ +Ġfall ing +Ġfa ult +ell y +Ġch est +Ġл и +Ġpot ato +Ġbuild ings +Ġoper ating +Ġp are +w r +D on +ĠF our +Ġv ul +Ġl á +Ġfr ust +ĠD ann +ol es +ny a +Ġì ¶ +ĠÑĢ Ð°Ñģ +× Ľ +Ġa ÃŃ +w ord +Ġweap on +Ġob t +ĠF all +ĠSte ve +Ġmix ed +Ġp ode +ĠA S +ĠL eg +Ġdes c +Ġspl it +Ġemer gency +ĠS ing +Ġprof it +Ġtyp ical +ĠDon c +Ġannoun ce +ĠTe x +Ġsac r +tern al +Ġcomm ittee +ig o +Ġdi am +ph as +Ġdef e +ĠProf ess +Ġdec l +Ñĥ ÑĢ +2 2 +ol f +ĠM ond +u y +Ġa y +Ġl em +Ġlove ly +ĠC ould +Ġgu ar +H H +Ġcare fully +ĠL isten +Ġк ÑĢ +Ġyou th +ĠThere fore +Ġdream s +ĠJe ff +? ] +Ġë Ī +D A +Ġb odies +au x +Ġtechn iques +Ġmechan ism +× ĵ +Ġо ни +Ġdes ire +à ® +ĠV o +qu es +ĠÑĥ же +ĠWho a +ĠG ame +Ġh al +an ish +Ġpract ices +5 00 +Ġsort s +up s +ate ful +Ġhers elf +Ġgu itar +Ġprop os +Ġsit es +Ġbe ach +Ġ× ¢ +ç¬ ¬ +н Ñĥ +Ġdr am +ĠNo ve +V E +r ant +Ġpl ot +ĠìŬ 기 +ĠC a +Ġestab lished +Ġ201 5 +Ġinsp ired +Ġannoun ced +ä¸ ª +ĠÑĤ ÑĢ +Ġ2 6 +Ġv oy +Ġte ch +ìł ģ +Ġprocess es +ont o +ĠP an +Ġrap id +ist an +Ġ19 7 +Ġrelig ion +Ġ2 8 +Ġsm ile +Ġb ab +Ġ Ú© +ĠV ir +Ġsched ule +Ġexec ut +Ġpr on +Ñ į +ĠÐĿ Ñĥ +m usic +ìĽ IJ +Ġg an +ìĭ ł +Ġdef ault +Ġbe m +Ù ī +Ġfor ced +ĠOb viously +Ġst one +Ġt ie +Ġdrink ing +Ġser ved +C ause +Ġcon ference +ĠExact ly +ãĥ Ī +ł ľ +ìĻ Ģ +ĠR a +Ġf ake +Ġdif f +ãģ © +Ġchalleng ing +Ġì¤ ij +Ï ĩ +ä»Ģ 麼 +Ġintellig ence +re te +Ġstud ying +Ġapp oint +Ġt an +Ġи м +Ġcur ve +ĠTe am +ĠA z +Ġз д +ĠMus ic +f ield +ir ation +Ġfail ed +Ġno vel +Ġdifferent ly +Ġes cape +ĠY o +ĠOct ober +ı yor +Ġdescri bed +Ġcon vert +ac ement +Ġhot el +is ation +Ġsu is +ãģ ij +å ŃIJ +æĢ İ +Ġwalk ed +2 00 +Ġneighbor hood +is p +ĠL os +Ġh idden +Ġ2 7 +л е +Ġph r +ĠIs land +ĠSt reet +end a +hip s +os ure +Ġdefin ed +ภ§ +Ġv ida +Ġlab el +ĠEvery body +Ġjo ke +ia o +ا ÙĨ +Ġa thlet +... " +ĠF ire +D o +Ġdef ense +Ġent ertain +á t +Ġpolic ies +Ġal cohol +ĠEng ine +Ġg al +ĠJ ud +Ġvol unte +ick s +et a +ag t +Ġ× ķ +Ġm ö +1 3 +Ġenc oun +Ġe h +Ġor ange +Ġabs or +Ġsp aces +ĠNove mber +êµ ¬ +i at +Ġt am +ck now +Ġst orm +ĠDire ctor +Ġpre gn +ĠìĿ ¼ +Ġо п +Ġres ource +Ġb ard +ne w +ĠDe cember +u its +Ġwe il +Ġconst ruct +s i +n ic +Ġfl our +Ġrest rict +ü t +Ġentire ly +Ġbreak ing +ent lich +Ġtw enty +Ġcaus es +Ġele v +ĠS pr +ĠIntern et +Ġk iss +Ġoper ations +s zy +Ġë Ĭ +Ġscient ists +Ġgr own +Ġown ers +out s +Ġcour ses +Ġus ual +Ġin n +Ġtrans m +ñ o +Ġnu est +к ов +Ġcateg ory +ĠL ife +ĠPl us +Ġat mos +wh ile +Ġrecord s +Ġde ÄŁ +ëĭ¤ ê³ł +ĠìĤ¬ë ŀ +Ġrequire ments +in n +Ġimm ig +Ġdeep er +ç ´ +Ġapp s +Ġcolle agues +ż y +Ġoff ers +Ġt á +Ġcolum n +la ud +I R +ĠM s +Ġexch ange +l as +ĠL aw +ĠJ on +is se +ro gen +Ġmo i +× Ĺ +Ġs ending +Ġhe llo +е е +ÅĽ Äĩ +Ġsuc ceed +Ġsuff ering +Ġad vert +Ġì£ ¼ +çŁ¥ éģĵ +Ġrec o +ın ı +Ġк ом +all ey +Ġfail ure +ie j +Ġëķ Į +Ġdrug s +Ġcu ando +Ġìĸ´ë ĸ +ĠAb out +Ġqu ando +9 0 +ĠF ed +1 7 +S h +in ho +ĠSund ay +ĠPh il +Ġacad emic +ĠIn c +Ġmaint en +åĩ º +Ġre ward +er d +Ġcomm itted +ìĬ ¤ +г ÑĢ +Ġstand ards +Ġk al +Ġint ention +ĠZ h +Ġa cknow +ä ¿ +Ġ== = +og y +å § +Ġfilm s +is k +Ġte eth +Ġstrugg le +r d +u en +Ġdis s +ĠD ar +am y +Ġenem ies +Ġve loc +ĠC all +um bs +иÑĤ елÑĮ +Ġo cean +é d +ìļ ° +Ġtre m +ient o +еÑĪ ÑĮ +ffic ient +Ġbott le +Ġinstit ution +est y +ĠH an +h ab +ëĬ ĺ +Ġar rest +éĤ Ħ +Ġlet ters +oun ce +í Į +A n +Ġcreat es +Ġcl ock +Ġdeb t +Ġan cient +ific ations +g i +B ut +ĠT u +k l +Ġb order +Ġo ok +ĠB ay +est a +Ġë³ ´ì +Ġw ra +pre ne +Ġê² Į +ang le +Ġbelie ved +ien cy +ak a +Ġcrit ic +Ġb omb +Ġha m +ĠÐ Ľ +êµ Ń +ĠGu ys +ros oft +Ġcr im +et ch +AR R +Ġs ight +и на +Ġa in +á» ij +is che +Ġau x +Ġnum er +Ġsurv ive +A ll +B C +Ġs z +Ł ¬ë +Ġj am +ĠCour t +Ġall es +Ġtr igger +Ð ŀ +Ġform at +Ġdec ades +Ġc es +Ġsign s +Ġrob ot +ĠCh urch +Ġa z +Ġs oup +ĠTex as +ut en +ĠÑĩ ÑĤобÑĭ +Ġneigh b +ĸ ×Ķ +Ġcommunic ate +Å ¡ +Ġel imin +Ġfrequ ency +her n +id os +Ġem phas +Ġmess ages +Ġg ender +ĠW enn +Ġв о +Ġpr ices +ol o +Ġп он +w ing +ĠF il +а ем +ĠC ur +Ġfal se +Ġfield s +Ġs é +2 4 +Ġm ac +u ÅŁ +Ġlay ers +Ġadv oc +w an +Ġk ar +ĠÅ ŀ +Ġdec or +Ġwall s +o e +iss ions +Ġres ol +× ¢ +ĠCar ol +ĠV ide +le ep +ĠY OU +Ġfl ip +Ġsur gery +Ġch op +U R +. , +Ġag ency +Ġwant ing +Ġsol ar +Ġhor iz +ĠAd am +Ġstay ing +ol ic +Ġgr ateful +Ġrem ark +Ġtechn ologies +Ġprote in +å¿ ĥ +д ел +ĠM ont +Ġshould er +Ġz a +re y +ĠO oh +Ġst y +ic ar +оÑĤ ÑĢ +Ġrout e +ĠT urn +Ġb om +Ġdeb ate +Ġposs ibility +Ġíķ ´ì +ap a +Ġinv ent +ür lich +Ġprof ile +Ġsen ior +pp y +v as +Ġm undo +ate ver +Ġapp arently +en er +× IJ +ç Ń +Ġprec is +Ġal ign +Ġkn ife +ĠRo bert +å ĭ +Ġfo ol +Ġinv ite +us ing +Ġcircum st +Ġcapt ure +Ġd ough +ĠS and +Ġse u +ĠNew s +Ġb ite +Ġne ut +w ide +Ġlect ure +Ġëĺ IJ +Ġorigin ally +Ġcho ices +ĠG ar +Ġver se +Ġl it +Ġ19 6 +íķ ł +Ġmeas ures +ç ões +w ater +ri ve +Ġz ijn +í ģ +ĠB us +Ġhe b +е Ñħ +ĠK ar +ĠN ão +Ġkill ing +à® ª +Ġmir ror +m od +Ġm ol +Ġcre ation +Ġest im +Ġatmos phere +Ġg am +Ġt ables +is i +ĠL ittle +Ġt as +ĠE le +é l +Ġscen es +Ġt one +Ġaffect ed +ĠAU DI +ĠBr own +I f +ĠÙ ĩ +ĠDan iel +羣 çļĦ +qu er +ch i +íķ ĺë +Ġmist akes +Ġs la +ãĤ ¤ +Ġent r +Ġе Ñģли +Ġsh out +Ġport ion +Ñ Ĺ +Ġpre viously +á» Ļ +ĠпÑĢ ÐµÐ´ +оÑģ ÑĮ +Ġhead s +ç İ +å Ń +åľ ĭ +Ġgr ass +ภ° +cri be +Ġqu é +ĠSp anish +Ġoffer ed +ĠбÑĭ ло +ĠCl oud +Ġve ctor +ĠH uh +Ġk ad +if ts +ĠÎ ½ +Ġhung ry +Ð ¡ +Ġpar all +AN D +ĠvÃŃde o +iz z +Ġocc up +Ġí Ķ +Ġsee k +h es +Ġdo ors +Ġhous es +Ġconsider ing +Ġgradu ate +Ġf ulf +è ¡Į +è £ +Ġext reme +Ġflow ers +it ate +ĠP ri +Ġfundament al +Ñĩ аÑģ +è¯ ´ +Ġtext ure +į ĺ +ĠAN D +à® ± +ĠT em +Ġn ada +ì§ Ħ +Ġcelebr ate +um s +Ġp ill +Ġи ли +go ing +Ġh ip +Ġsupport ed +Ġper man +Ġagre ement +Ġty m +Ġë ij +ĵ¤ ìĿ´ +Ġpurch ase +í Ķ +ĠPl an +eg en +Ġrec over +P U +ĠMic rosoft +du c +Ġhol es +Ġdro pped +Ġp ig +Ġend ing +Ġattack s +be c +Ġre n +Ġr app +Ġìļ °ë¦¬ +Ġter ror +Ġ× Ļ +Ġed it +Ġa o +. </ +Ġ2 000 +ĠUn ion +Ġscient ific +Ġp unch +ort ion +Ġput s +ĠMond ay +ĠJ er +E C +Ġmat rix +Ġinstit utions +Ġm ont +Ġex hib +Ġspeak er +Ġmet ers +. ] +Ġser ving +Ġdatab ase +ĠL AU +Ġdam n +Ġpod er +!! !! +Ġí ĸĪ +ĠAUDI ENCE +Ġj un +ĠA C +ĠIt al +se c +ĠYou ng +ru ck +ou ve +ภĦ +ç Ī +Ġë§ Įë +ad ing +ur ation +ĠP S +Ð ļ +ĠUn f +è ģ +or ia +Ġman if +Ġsent ence +Ġsign ed +B S +Ġpro of +ĠMus lim +Ġnuc lear +Ġг овоÑĢ +Ġw oll +Ġf avour +ĠW H +Ġvul ner +Ġclos ely +Ġind ex +ÑĤ еÑĢ +ach el +Ġcap able +ĠB es +Ġcro ch +ek t +Ġshe et +Ġse es +Ġnat urally +ĠEng land +Ġparticip ate +Ġex ists +Ġsh arp +p y +Ġbreak fast +b ow +Ġtw ist +ç § +in ating +ot i +ĠF ound +Ġde ux +Ġselect ed +ìł Ħ +os is +Ġpresent ed +Ġline ar +Ġê ´ +Ġk un +é» ŀ +ô ng +Ġb ÄĻd +Ġtem por +Ġc able +ĠпÑĢ Ð¾ÑģÑĤо +к е +ĠÑĤ ам +Ġwin ning +è ĥ½ +ĺë ıĦ +Ġ201 4 +ĠìĹ ¬ë +ĠU N +ĠCl ick +Ġpre par +ĠT O +Ġsu a +ĠH am +Ġl ä +Ġabsol ute +Ġeng aged +å¦ Ĥ +ĠH mm +Ġd ash +T A +ñ os +Ġsp o +çĶ Ł +) ] +Ġtest ed +Ġbl ank +Ġre ject +Ġass im +Ġre ar +ĠSt r +Ġcr ash +Ġна ÑĪ +иÑĤ ÑģÑı +Ġcol on +ĠU nt +ĠC e +Ġac id +é Ĺ +Ġk it +ib ilities +ut o +Ġvalu able +l ist +Ġpart ies +ĠM m +Ġcol our +Ġch am +Ġste el +ĠI mp +Ġfund s +ĠD NA +ĠK en +ind e +íķ ´ìĦľ +ãĥ ĥ +ĠHapp y +ĠU se +ĠL ight +Ġli p +Ġauthor ity +ĠL ong +ĠI ran +Ġe ll +Ġco ordin +Ġsub m +Ġrecord ed +Ñĥ ÑĪ +Ġdel ta +Ġre form +ĠSt ill +Ġopp on +Ġallow ing +Ġpatter ns +Ġlet ting +Ġsleep ing +O kay +Ġp izza +Ġ ÅĽ +Ġд ол +Ġtal ent +ens ions +Ġenvironment al +Ġprofess or +Ġsh ots +Ġcont ains +ug ar +y o +ı Ļ +Ġsequ ence +ι α +ad er +é ł +а Ñĩ +ÙĨ ا +ĠI k +Ġt ous +ur ies +Ġp ounds +Ġex ternal +im ents +Ġvra iment +ìĭ ¤ +Ġhapp iness +Ġpr ze +est ic +Ġestab lish +ĠFl or +Ġr ig +Ġh oney +Ġp ul +Ġsympt oms +Ġbr ows +ел и +ĠÏĦ ο +Ġsh irt +ĠTe chn +ĠPro gram +ем Ñĥ +Ġup set +Ġgu est +b urg +Ġun like +Ġsome what +Ġhang ing +a e +Ġr um +Ġphot ograph +ĠL i +åĽ ŀ +Ġst able +Ġvolt age +ĠE ll +Ġentre prene +us es +ass en +¬ ¸ +Ġë§İ ìĿ´ +Ġg host +Ġs agen +Ġcomb at +Ġg ör +ĠC ap +Ġs ão +ĠK at +Ġform a +Ġsum m +Ġm arch +Ġv ast +ü k +Ġcommit ment +im os +L et +Ġded icated +ist e +l ay +éĢĻ æ¨£ +Ġtop ics +Ġmach ines +ĠPar is +ĠìĿ´ë ٰ +Ġmin i +Ġmark ets +Ġk o +Î ´ +v ille +Ġgood ness +Ġframe work +ult ure +Ġbas ket +ess a +а ÑĨи +ust er +Ġê ¹ +ä½ Ĩ +Ġext ent +ĠMens chen +Ġconsist ent +Ġaut o +ri p +Ġm ere +à¯ Ī +Ñ Ķ +Ġe lle +Į Ģë +ok en +Ġpull ing +Ġc ow +out hern +Ġmeet ings +Ġc ada +нÑĭ м +ient e +Ġb ast +an ing +Ġfocus ing +ro ad +Ġro of +ĠProfess or +ĠS P +ÑĢ Ð°Ð· +Ġno od +Ġ4 00 +ĠìĿ´ì łľ +ìŀ Ī +ĠM ount +ей ÑĩаÑģ +Ġ× IJ +Wh y +× ŀ +ınd a +Ġpos itions +è me +ç ı +Ġд ÑĢÑĥг +i yor +Ġpass ing +Ġasse mb +Ġsm oke +Ġt il +Ġm useum +Ð Ķ +ĠP erson +ни м +le ich +Ġint ent +Ġs que +Ġcra ft +ìĪ ĺ +ors un +Ġ15 0 +Ġbr others +v or +ĠSpe aker +ic ians +Ġoffic er +Ġiç in +ĠÑĤ еб +Ġscr atch +Ġgener ate +y i +Ġemot ions +a us +ì¹ ĺ +4 5 +ĠL ink +ĠRe al +Ġat e +Ġн ад +Ġn ative +á» ĩ +ı y +Ġen orm +Ġblo cks +Ġfac es +ac c +iven ess +Ġin ches +u is +he it +Ġstre ets +Ġprob ability +as i +Ġim pl +Ġ ठ+ur day +Ġf aut +om y +Ġp ip +Ġill ust +à® ¯ +ĠJ un +Ġl ying +9 9 +Ġmem ories +Ġpract ical +ian a +on ces +Ġview ers +ĠTh omas +æ Į +ĠG irl +ĠWh ether +Ġinnov ation +Ġdisapp oint +M y +Ġwin ner +Ġ ig +Ġrat io +ĠBl ue +ĠS ub +Ġdoc uments +Ġform ula +Ġë © +Ñ Ĭ +Ġappe ared +v ar +and on +Ġsp ray +m ak +ĠQU ES +K E +Ġwed ding +R e +аÑĤÑĮ ÑģÑı +Ġun o +Ġg all +íĦ ° +ci o +c ers +Ġм не +Ġpe pper +ãģĹ ãģŁ +ĠFe bru +Ġaltern ative +Ġf u +ĠBas ically +ĠSm ith +Ġg ate +ĠT am +ĠWh atever +Ġappro xim +Ġconc ert +Ġju ice +ĠEs pecially +Ġdynam ic +Q u +ond er +i very +Ġb ang +Ġr ul +ĠPart y +Ġschol ars +Ġcry ing +j Äħ +Ð ¢ +ĠQUES TION +r id +Ġaccur ate +ç o +ĠC ool +co in +Ġìĥ ģ +ĠF o +Ġpr ó +ĠR oman +ĠÐŁ ÑĢ +Ġcheck ing +? ' +Ġattach ed +ĠIs lam +Ġexper ts +× § +ĠCon st +ÑĢ Ð°Ð½ +Ġshad ow +Ġdel ay +Ð Ĵ +Ġor ient +ë Ĥ +ell en +Ġas ÃŃ +ки й +Ġhistor ical +Ġun com +om p +h m +Ġb il +Ġpl anned +ĠUnf ortunately +ĠWind ows +Ø ´ +Ġencoun ter +ĠìĥĿ ê°ģ +Ġregard ing +arr ass +Ġreco very +ĠH ur +ĠE mp +Ġs ÃŃ +íķĺ ê²Į +Ġdef end +Ġc et +as se +ëĭ ¨ +ok es +Ġrem ote +ĠØ ³ +Ġar ts +is co +auc oup +ĠMex ico +Ġп ом +Ġch osen +em at +od ing +Ġfl ower +stand ing +ĠAs soci +um my +IL L +Ġcam eras +åĨ į +Ġ æĪij +ĠA rab +ĠS um +Ġte go +Ġcrim inal +if orm +Ġst ack +ìĦ ± +ĠDon ald +ĠO ld +Ġd ust +ĠJ ose +Ġhe m +Ġincre ases +ost a +Ġd ying +ĠR iver +Ġmo ist +ÑĤ ов +a res +Ġdisci pl +ra it +ĠH as +y gen +ĠT re +Ġë ´ +Ġlangu ages +ĠH en +Ġ3 6 +ĠDis ney +int s +Ġal go +Ġfood s +Ġset up +l an +Ġeffect ively +Ġwhere ver +æľ Ģ +Ġun ter +form ation +Ġh its +Ġprinci ple +Ġtast es +§ Ī +Ġtreat ed +Ġres olution +Ġpriv ile +ĠI P +ë ° +Ġter rit +Ġpow ers +Ġí ĥ +ĠV ict +Ġb other +ĠCh air +Ġmus cle +Ġs ale +Ġdec ent +Ġc oup +ĠS qu +Ġco ast +Ġro d +ĠFr anc +Ġbath room +Ġshop ping +Ġмож еÑĤ +Ġi ÅŁ +ĠSt ay +gr ade +Ġform ed +Ġba ÅŁ +Ġbr ill +j our +í ĸ +åĽ ł +w ie +ic ate +ĠâĢĭ âĢĭ +ĠN orm +à ¥ +Ġmain ly +ĠSp ace +Ġtrem end +it i +à® µ +U T +M usic +ĠFebru ary +Ġcont rast +å¯ ¹ +est ing +ĠÎ ´ +ing ing +ĠÙ Ĩ +ss en +ĠH ome +Ġshe ll +ĠH ay +Ġall er +ĠA p +ĠWest ern +ĠW ord +ĠPL AY +Ġë ħ +ĠA qu +Ġent ry +Ġlaunch ed +ĠM em +ĠP our +Ġz we +ĠSome one +ing e +ĠPro b +m ble +ĠR el +ur u +Ġr hy +Ġg ig +Ġengage ment +ü ÅŁ +ãĤ ĩ +Ġoffer ing +wh el +Ġact or +Ġ å°į +AP P +w est +ĠR oy +Ġreturn ed +Ġsil ver +r ating +Ġest ar +Ġs ke +Ġt i +ic ation +Ġann oy +Ġdeep ly +ìļ © +Ġnat ürlich +EL L +ĠC ath +Ġr ail +н ов +Ġpray er +c ol +G B +ĠТ ак +Ġg la +ĠW ater +Ñı ÑĤÑĮ +ĠN on +ô t +ag ers +Ġh ug +Ġdo ctors +an cing +ĠT alk +z ing +Ġhad n +Ġl ui +Ġat é +Ġê·¸ë ¦¬ê³ł +ê¹Į ì§Ģ +ic i +Ġincor por +ĠD i +z il +any a +ª ħ +Ġ » +3 5 +Ġbe er +Ġbe aucoup +ĠM C +Ġe ars +og en +ĠQu est +ed a +æľ ¬ +ĠSat urday +Ġfall s +st on +b les +Ġth us +ĠëĦ ¤ +๠Ħ +Ġth erm +Ġdivers ity +Ġso y +az u +im p +Ġtele vision +éģ İ +Ġש ׾ +Ġw ur +Ġed ges +Ġless ons +ĠA ud +ãģĹ ãģ¦ +vo ir +ament o +Ġexplain ed +Ġо на +Ġtem ps +Ï İ +The y +Ġsurpr ising +ани Ñı +ĠD rag +éĿ ¢ +ĠC le +Ġn am +ĠлÑİ Ð´ +Ġhard ware +Ġth umbs +Ġκ αι +ĠT op +Ġà ¥ +é Ļ +×ķ× ¨ +Ġê·¸ëŀĺ ìĦľ +ĠBud d +ther n +Ġinterest s +Ø ° +Ġdevelop ers +Ġh itting +Ġopp osed +Ġheart s +ĠAnd roid +ĠH and +Ġrepres ents +g lich +íĬ ¸ +Ġ3 2 +Ġdom in +ĠAn n +ä¸Ģ ä¸ĭ +Ġét é +Ġzo om +Ġktó re +Ġadult s +Ġorder ed +Ġpick ing +ĠH ong +Ġfilm ing +æĢ Ŀ +Ġse ed +ĠA T +Ġcalcul ate +Ġк огда +ĠO s +ic it +Ġrem aining +Ġse gu +à » +Ġìĺ¤ ëĬĺ +Ġarri ve +Ġcon gr +Ġgrand e +Ġhealth care +Ġмож но +S A +est e +Ġaware ness +Ġsqu ared +xt ure +ĠBe ing +Ġsold iers +Ñĥ б +Ġrev olution +Ġtra ined +end en +è ° +Ġdan cing +Ġinstall ed +pr ise +Ġv eter +Ġmen os +ne ll +ĠBr other +Ġn un +Ġimportant ly +all ed +ia ÅĤ +ab led +ĠSy stem +ĠV ol +Ġe ld +Ġemot ion +ic an +ĠB ank +ik es +Ġv log +Ġв оз +Ġpu ede +ìĺ ¤ +Ġte en +Ġse vere +% , +Ġclean ing +z Äħ +Ĺ IJ +ĠTh rough +ĠS et +E P +" ? +ĠM other +Ġfigure d +Ġm ud +ĠÑ ĸ +ĠOff ice +Ġr aw +Ġdestroy ed +ent a +Ġag gress +Ġо Ñģ +Ġëª ¨ë +ä ä +ĠA R +Ġcorrect ly +åī į +Ġst ir +Ġext ract +Ġveh icles +éĸ ĭ +ĠR un +Ġв ÑĢем +Ġparall el +Ġl ag +j u +Ġd are +ĠM ot +on o +Ġbeing s +Ġst ro +Ġexc use +Ġal pha +Ġask s +Ġpo cket +... ? +Ġk ita +ü m +Ġappear ance +ord an +Ġins ert +Ġна Ñĩ +Ľ i +Ġtem po +Ġfac ility +Ġvis ible +å Ĵ +ĠS cience +ur os +ĠÙģ ÙĬ +ĠV an +Ġt ension +Ġíķ ł +Ġdel ivery +Ġst im +Ġsur vey +ĠG ra +Ġb ol +æ ł +Ġwe iter +ÃŁ en +ä¸Ģ åĢĭ +Ġpro ceed +Ġimpress ive +ĠV oc +ious ly +Ġд а +h ale +o ch +Ġgl ue +ph et +c ont +Ġf its +Ġbox es +Ġcontrol s +ĠCh ild +Ġscen ario +Ġt rop +Ġprocess ing +ĠÑĤ олÑĮко +Ġbird s +ĠCh ic +Ġн ап +Ġ201 3 +Ġmü ssen +ĠJ ag +Ġs Äħ +Ġper ce +re h +ĠF ore +Ġconf used +a ire +Ġaccompl ish +Ġcas a +c lock +Ġinflu en +ĠR O +Ġb one +ic ian +ĠS C +Ġstrateg ies +g h +д Ñĥ +Ġit u +Ġperson ality +Ġbard zo +Ġaccept ed +Ġst om +ie v +ĠH ist +ĠA us +Ġë° Ķë +AT OR +æĦ ı +o ir +Ġmag az +Ġexpl an +Ġcor n +Ġil s +Ġcirc uit +Ġg ay +h op +ãĤ ĥ +Ġequ ival +Ġdies er +er ves +com es +k lich +Ġëķ Įë +ab et +Ġex ha +Ġman ner +ĠâĻª âĻª +é c +ä l +Ġconf irm +Ġenter ed +empl o +ĠF ar +Ġo ù +ess ions +Ġn urs +Ġent ão +Ġab andon +l ife +Ġw is +N arrator +Ġìĸ ´ +Th ere +ĠR am +ast e +Ġatt rib +ĠA y +Ġmes mo +Ġν α +é « +ens es +Ġc rop +Ġзд еÑģÑĮ +ĠUnt il +ste in +Ġo ven +Ġsus pect +h et +Ġpu is +Ġcar ried +é g +ĠDe v +em s +re ens +ber ry +Ġtem pl +ĠB it +Ġvari ables +Ġover whel +μ ε +Ġinit ially +ìķ ĺ +ot hing +еÑĤ ÑĮ +ĠH ill +Ġdep art +Ġmy st +az z +Ġflu id +ĠD C +Ġclin ical +ĠR yan +ĠFlor ida +ĠT ak +Ġanx iety +b ro +Ġcircumst ances +ĠÙ ĥ +Ġexist ence +Ġt ong +Ġ201 2 +ĠSecret ary +Ġsp icy +Ġ[ ( +ĠWith out +Ġfact s +Ġt ons +A pp +ĠSt and +Ġli es +ĠA D +w in +ÏĦ ε +app lause +I P +st a +ĠS up +ph ones +ŀ ij +p ie +ĠP ot +ĠN O +èµ · +Ġ× ŀ +ĠÐĶ Ð° +ic as +ĠI r +Ġpush ed +Ġun cle +ĠÙħ ÙĨ +Ġl on +Ġprinci ples +ĠIntern ational +Ġà ĸ +Å ¾ +Ġsay a +Ġê³ ł +Ġr ib +Ġpast e +Ġwar ning +Ġmus ical +Ġagre ed +оÑĢ Ð¼ +Ġgar lic +Ġox ygen +ìĺ Ī +A l +Ġë§ ŀ +el ines +LAU SE +ç¾ İ +gy pt +G E +ck er +t u +Ġsh el +Ġstay ed +Ġг од +Ġl apt +ĠMart in +Ġinv ited +Ġconf ir +Ġemb arrass +ac iones +ĠC amp +Ġhold s +ax y +Ġd ive +uck les +Ġbo ost +Ġw ür +st al +ĠÑĢ Ð°Ð±Ð¾ÑĤ +Ġdé c +Ġoffic ers +ĠìķĦ ë +olog ist +× ŀ× +Ġse eds +Ġbu ff +Ġupd ates +ãĤ ı +d ed +Ġfriend ly +Ġcoun cil +ĠProb ably +Ġp iano +Ġredu ced +ÏĦ α +Ġauth ent +Ġexpl os +p ass +ĠH it +j ud +ĠN av +om i +Ġcomm ission +Ġg ym +Ð Ł +Ġp on +ÑĢ Ð¾Ñģ +Ġinter face +Ġstruct ures +ĠJ en +Ġy ok +Ġme u +ì§Ģ ë§Į +n ed +ĠW ie +Ġident ified +Ġchann els +ı na +Ġphilos op +ke it +Ġbit s +ent es +Ġf rag +ĠK ind +Ġdo ch +Ġs ne +ind ing +ĠJew ish +оÑĢ Ð¾ÑĪ +Ġf ue +æĸ ¹ +Ġí ı +Ġm ı +Ġke ine +Ġloc ations +çĶ ¨ +Ġmet er +Ġbe ef +ãģ ĺ +Ġman ip +Ġson o +zz le +ç ¶ +Ġp es +Ġhor rible +ĠS n +Ġfact ory +Ġfif th +Ġcook ed +Ġmo od +Ġveloc ity +Ġob lig +Ġconnect ions +ÄŁ im +Ġê³ µ +Ġdom ain +Ġapply ing +Ġrid ic +Ġc el +Ġchild hood +ĠT est +rat ulations +ĠVir gin +ĠC EO +Ġп л +Ġalgorith m +Ġinter action +ag a +Ġkid ding +Ġtom ato +Ġcontinu ing +l ad +st ream +ож е +Ġìĺ ģ +ел ов +B A +Ġn ap +ĠNo body +Ġth umb +ĠO N +Ġr ush +D R +Ġstri ke +Ġev olution +ich e +Ġì » +Ġê·¸ëŁ ° +ا ت +Ġa k +Ġwind ows +Ġex cess +ãģª ãģĦ +Ġconc lud +Ġepis odes +Ġstrugg ling +ĠD at +Ŀ ¼ë +Ġke ys +Ġk le +æŀ ľ +Ġveget ables +y stem +ên cia +r ick +Ġreven ue +ĠH aw +Ġl an +ant es +in iz +ãģĵ ãĤĮ +и ÑģÑĤ +Ġsu p +© ´ìĦľ +Ġmoment o +ist o +ãģ ¤ +ĠE ric +i ors +b aj +Ġintrodu ction +ir ty +Ġde ck +re al +ĠMar io +Ġlo ving +à¸ Ķ +Ġsupport s +иÑĩ еÑģ +Ġinc ident +ut ch +u v +Ġbo om +еÑĢ ÑĮ +Ġн Ñĥж +Ġcomb ined +ĠL in +2 3 +or ation +nt e +Ġs or +Ġdir ty +if er +ĠAP I +Ġcollabor ation +i able +Ġprior ity +ĠA le +ĠPr in +ĠEx c +Ġv ais +Ġgr an +Ġst ood +Ġrec ru +ĠM ur +es is +as p +Ġlock ed +ĠP ero +ĠHar ry +Ġt udo +ĠT en +Ø µ +force ment +) ) +ol i +ĠìĿ ¸ +Ġsupp l +Ġcroch et +Ġphen omen +l os +ath an +ĠSu pp +Ġem br +Ġbe k +ĠZe it +g end +Ġroom s +ª ½ +V ER +ny ch +Ġdon t +Ġcab in +Ġaccount s +ĠE aster +×ķ× ľ +ãĥ « +Ġfac ilities +be it +Ġlink ed +ĠG er +Ġprogram ming +ot ic +Ġdr ama +Ġ2 9 +Ġí ģ +Ġinstruct ions +Ġimportant e +Ġw aves +Ġa id +C K +ê²ł ìĬµëĭĪëĭ¤ +ĠM ir +Ġt id +ĠH ot +Ġarr ange +ĠB aby +Ġt ack +ĠÑ ī +í Ŀ +Ġvert ical +Ġhe el +ĠC ut +Ġnar row +ĠA ri +Ġkn ee +ĠBra zil +ĠF ive +Ġpost ed +U D +Ġro lling +Î ¸ +Ġclaim s +ĠIn s +O K +ãģĦ ãģĨ +u in +ĠInst itute +Ġint ense +i ar +ĠN ick +Ġse lection +Ġleg end +Ġun iform +ú n +Ġstud ied +å¤ ª +ĠÐ ¥ +Ġìķ Į +g ers +Ġd ow +ĠC S +Ġag ent +ĠA uf +è¦ º +Ġj og +Ġair craft +ëĭ ĺ +Ġv it +ul s +Ġseg ment +Ġord ers +ĠCl ass +Ġap olog +Ġplatform s +Ġmy th +аж е +ĠB ook +Ġsens itive +Ġпол ÑĥÑĩ +Ġdam it +ĠC apt +so le +Ġarchitect ure +ĠW il +Ġin her +ca p +ĠB oy +æ¬ ¡ +Ġbur ning +ĠP ublic +Ġbeh alf +Ġìľ Ħ +Ġtherap y +ubs cribe +Ġinvol ve +Ġexp osed +i ÅŁ +ä» ¬ +ê tre +Ġto il +Ġs ink +p ir +å ĥ +I I +Ġag encies +Ġ q +ĠD own +au f +Ġë§ Ľ +ãĥ» ãĥ» +Ġpro c +ok ed +Ġst ores +p ower +ĠTh ings +Ġaccess ible +Ġte ż +ĠE duc +Ġspeak ers +ĠSar ah +Ķ Ķ +Ġdi verse +ìŀ ĸ +ĠU lt +Ãł y +ĠChic ago +S he +ath y +Ġen able +Ġtrad ing +Ġmus cles +æ Ľ +ĠC are +ĠU r +ĠSc ot +Ġphr ase +EN T +Ġê² ½ +ĠJ ac +p ack +Ġdeterm ined +ü nd +Ġneg oti +Ġvid é +Ġro z +ĠS us +Ġr iding +h men +ĠDe f +ĠC re +ãĤ ¹ +ĠW all +ig an +Ġse mpre +Ñĸ д +Ġdri ven +Ġfoot age +Ġf ond +ĠW ay +ä m +ĠOb ama +ĠServ ice +Ġ7 5 +ĠD ark +Ġê· ¼ë +ĠC at +Ø · +é Į +Ġj ug +Ġet was +Ġbreat hing +á» ĥ +åħ ¶ +ĠWe b +ä¹ ĭ +èµ ° +Ġfo is +Ġlight ing +ĠD A +Ġob st +Ġle ur +çı ¾ +ĠE gypt +ĠAr my +ic ide +аÑĤ и +Ġëĭ ¤ë +Ġap artment +Ġch ief +ĠW ed +Ġnetwork s +Ġb att +æ ¸ +ĠL uc +Ġnic ely +Ġver b +ภ´ +ì ¶ +os it +Ġreve aled +Ġt at +Ġt ied +á» ģ +Ġanim ation +Ġro les +ìĬ ¤í +Ġvers ions +Ñĩ иÑĤ +Ġtas ks +¯ ¼ +Ġres c +s he +Ġlo ose +Ġc á» +Ġco isa +Ġal ert +Ġn in +ĠS AM +Ġtra baj +ir us +T H +Æ ¡ +og ether +ĠT ai +Ġfig ures +Ġ×IJ× ª +Ġcre ep +Ġinvestig ation +Ġrecommend ed +ĠA k +Ġres idents +ÑģÑĤв о +se ct +ани е +Ġmind s +u ing +å ± +ow ing +Ġno g +Ġr az +ا ر +Ġqu ot +Ġи Ñħ +Ġs ed +Ġapp laud +Ġcover age +v ol +ĠRe c +Ä Ľ +ĠвÑģ Ñij +Ġexpect ing +Ġoper ate +Ġcon ver +ĠS uch +ĠR ad +ĠPr ime +Ġpur ple +Ġ201 0 +Ġìķ Īë +Ġex em +Ġcompar ison +Ġlands cape +Ġne ither +ĠE h +ë ħ +Ġstom ach +Ġcas o +â n +Ġpercent age +w ich +it an +Ġk l +Ġexp ans +ĠاÙĦ Ùħ +Ġocc asion +re ts +ig ning +Ġkil omet +è· Ł +Ġg ust +c ze +Ġur ban +Ġag ric +Ġassist ance +Ġsur f +im eter +Ġpet it +Ġassess ment +Ġman ual +Ġimpro ved +b st +Ġpil ot +ĠM ars +Ġvie le +ĠCong ratulations +Ġarg ue +Ġwir klich +Ġclick ing +R IS +Ġlo go +Ġout come +ĠCent ral +ĠJ i +Ġg aming +Ġcons erv +Ġult imate +ĠV e +ĠW al +ar o +æĦ Ł +st ar +Ġconsum er +Ġtravel ing +im er +Ġ1 000 +ни к +Ġprincip al +Ġsa ke +Ñĸ в +Ġm ouse +ar ios +Ġrel ation +èĩ ª +Ġmor al +åķ ¦ +Ġthe ta +w y +Ġk am +Ġe ig +Ġgold en +× ¤ +Ġam pl +Ġv u +st r +r ors +Ġwhere as +iz ar +Ġadminist r +Ġnó s +ĠP ret +ĠAc ad +ang ing +b age +ét ait +ur i +Ġhe aling +Ġtip o +Ġmar ry +Ñĥ в +Ġest ate +u u +ì Ķ +ĠB est +Ġsuff er +Ġ19 4 +Ġbac ter +ĠÐĴ оÑĤ +ĠO m +Ġd z +è ¶ +ì ¦ +Ġold u +Ġphys ically +ĠLou is +et ime +c ase +Ġp ier +ìł ľ +v an +Ġass ets +Ġë ģ +v et +и б +Ġprom ote +Ġcongr at +ues day +Ġd uty +ĠVide o +Ø ® +ĠJohn son +kt ion +ĠVoc ê +ãĢ ĭ +Ġa i +Ġann ual +ĠJ osh +itt e +ĠJ O +Ġsl ides +Ġan c +¹ Ħ +te en +Ġcarry ing +ly mp +ed ing +Ġf ro +Ġad mit +r er +Ġofficial s +pt ions +g al +Ġhe ute +Ġvo ices +Ġball s +Ġgu ests +ann er +ãĢ Ĭ +is her +ĠM R +ĠRich ard +Ġrough ly +l ı +Ġvict ory +Ġalg un +ĠMr s +ÅĽ cie +ĠU k +Ġe y +ĠW ars +Ġbr anch +ast y +ĠPr ince +ек ÑĤ +Ġrecogn ized +Ġmuch o +ĠLe ave +con nect +Ġspe ll +Ġtouch ed +Ġag enda +è ¾ +ar ia +ĠK ong +og a +Ġparam eters +ëĭ¤ ë +Ġinst ant +Ġreg ul +C on +Ġed itor +ĠD ist +Ġun known +Ġpun ish +Ġexpect ations +Ġcry pt +Ġdiv ide +ak en +ĠM ess +Ġhy per +ĠPro ject +ik i +Ġag ora +Ġab use +Ġcaus ing +Ġconv in +ĠL A +Ġconcent ration +Ġbreak s +ur er +Ġconc rete +Ġform al +Ġbet a +it ors +ĠCh amp +Ġhead ing +ĠB lo +Ġpre nd +ĠSen ate +Ġadvent ure +os o +Ġop ens +ĠPLAY ING +ĠS U +ure n +ik t +ĠлÑİ Ð± +ĠFo llow +ĠB iden +el n +ĠS ky +et ing +ĠE xt +н ÑĥÑİ +ĠìĻ ľ +Ġsh r +ell a +ĠD iv +Ġtransform ation +Ġhouse hold +et ry +è ¡ +ĠDes p +Ġcour age +Ġpark ing +Ġett ä +c al +ly n +Ġla id +Ġtri es +ir ts +ig a +Ġrec all +if ier +Ïģ α +Ġa an +Ġbutt ons +Ġre aching +Ġê·¼ë į° +Ġsp ark +ĠSo cial +Ġе Ñīе +Ġcan al +Ġcr iter +Ġktó ry +Ġten emos +Ĥ ¬ +Ġн еÑĤ +Ġt ube +ac les +и ÑĪ +ĠdeÄŁ il +Ġst amp +Ġinf l +Ġa hora +Ġtra il +Ġmi xture +ĠR oll +Ġrout ine +Ġcount y +Ġenjoy ing +но ÑģÑĤÑĮ +er es +Ġpurp oses +ĠS anta +Ġbre ast +ä ng +Ġwr iter +å Į +ÑĢ Ð¾ +Ġne m +ic os +а ÑģÑĤ +Ġdetail ed +Ġre verse +ĠRe ady +Ġdist ract +ĠAl ors +ut ter +Ġdes erve +ĠR on +н ом +Ġobs erv +Ġlog ic +ĠP y +ĠKe vin +ãģĿ ãģĨ +¥ ´ +ÙĬ ÙĨ +Ġsk a +Ġt act +Ġhol iday +Ġb ump +Ġм ог +Ġde ix +í ħ +Ġwor ship +C l +Ġsu ck +ĠÑģ еб +Ġapp lause +ĠE p +Ġм о +Ġp atch +áº Ń +Ġlad ies +Ġbroad cast +Ġill eg +Ġnarr ative +oss a +ARR ATOR +Ġs ang +Ġmove ments +Ġpartners hip +Ġorgan ized +Ġn ode +est yle +ĠM eg +Ġindust rial +Ġgo l +Ġb oring +åĬ ł +ãģ Ķ +Ġcut s +Ġrec on +as a +Ġimp ression +ìļ ´ +g ie +M A +Ĩ µ +Ġed iting +r ont +Ġfollow s +ĠItal ian +ÑĢ Ð¾Ð´ +Ġê°Ļ ìĿĢ +Ġë° © +Ġpartic les +ĠBo ard +×Ļ× ª +j un +ron ic +Ġe j +ĠÏĦ η +×ķ× ĵ +c ion +itt y +ĠT uesday +um es +ĠPr ot +ed er +Ġpesso as +Ġн ов +Ġsk ip +Ġobject ive +ÃŃ as +Ġdes k +ĠLook s +und en +Ġprim arily +iment o +Ġreport ing +Ġha ce +Ġcheck ed +é ĺ +Ġë³ ´ë +Ġsmell s +Ġact ors +ĠAs ia +il Ãł +Ġrece iving +Ġtax es +Ġgr ace +Ġcompet itive +Ġdiv ision +Ġes per +Ġwhe els +Ġkomm t +Ġtremend ous +Ġes pe +... ) +Ġìŀ ħ +Ġlist ed +ä ll +Ġun us +ĠH olly +Ġguid ance +Ġc ub +Ġintell ect +ĠбÑĭ л +Ġregard less +ĠSt an +æ² ¡ +Ġconc lusion +aca ÄŁ +Ġl ol +ĠB at +Ġmanif est +ĠCh ief +Ġsh ame +Ġout comes +Ġma il +Ġk ur +ι κ +et z +Ġprep aring +2 7 +ĠQue en +à® ³ +Ġë¹ Ħ +Ġt iss +Ġconscious ness +Ġp ants +Ġm elt +uch t +in h +ìĽ Į +Ġvot re +Ġmod ule +ow y +Ġmon ster +Ġë Ĩ +Ġelectron ic +Ġcent re +Ġstop s +Ġt ou +Ġë Ń +Ġl amb +Ġconsequ ences +Ġstra w +Ġim per +Ġext end +ãģ£ ãģŁ +Ġanswer ed +ĠM ah +ĠLAU RA +if ting +u ate +åħ Ī +ĠUS B +ĠAnd rew +ãĤ « +ĠF red +ĠD E +ĠGe org +ç » +ì nh +Ġdra wn +Ġli ps +b ir +Ġmay or +im i +Ġenc ore +åIJ ĥ +fort able +urs day +ĠF orm +Ġbl ame +Ġshow er +Ġcontain er +st ers +ud es +ĠT ay +ภ¥ +Ġìĺ Ī +Ġv om +Ġb ass +ĠL ab +iss a +Ġdim ension +Ġexecut ive +ĠR om +ê²Į ìļĶ +ĠDo ctor +Ġdeliver ed +Ġg ang +Ġc er +Ġp it +el i +Ġextra ord +j ar +Ġder iv +Ġill ness +Ġgun s +Ġ201 1 +Ġair port +Ð ķ +Ġatt itude +Ġgr at +ĠW r +ĠN ARRATOR +Ġì ļĶ +Ġre new +Ġcos a +Ġcontro lled +omm y +ond s +Ġes e +ä ch +Ġv end +d am +Ġar gu +Ġacc eler +Ġn ail +ien e +ìĥ Ŀ +Ġenc ont +ese arch +é ¡ +Ġgood s +Ġf ishing +APP LAUSE +ĠN AS +ect ion +Ġtem ple +lich e +Ġkey board +çŃ ī +Ġdes de +Ġeduc ational +ĠN ight +3 3 +Ġbreat he +lich en +th m +i ère +ภļ +lar ı +Ġal i +Ġcomp os +Ġsens or +Ġë¶ Ģë +Ġnews p +ĠB und +ĠM i +Ġperform ing +Ġdr um +B E +Ġp ork +Ġco al +en ger +Ġr am +Ġë ²Ī +çĦ¶ å¾Į +иÑĢ Ð¾Ð² +ĠP op +Ġph ones +Ġfac il +Ġtrack s +ont e +Ġorgan ic +Ġdial ogue +ĠH aving +ĠP ost +Ġpay ment +Ġar ray +Ġint ended +ú s +Ġb ars +Ġreview s +land s +Ġking dom +Ġst ages +Ġmount ains +Ġd un +Ġdec ir +Ä į +Ġbank s +Ġthrow ing +Ġëª » +Ġan ger +ĠÑģ ейÑĩаÑģ +Ġdist ur +Ġhuman ity +Ġel es +Ġshould ers +ĠPer fect +Ġfan cy +Ġbrill iant +Ġinsp iration +h mm +å¿ « +Ġl id +U L +Ġm Ã¥ +ind i +è Ī +Ġsh ield +Ġìĺ ¤ë +C T +ag ine +u ber +ĠB R +Ġquest o +Ġз ак +ĠK now +Ġt ang +íķ ©ëĭĪëĭ¤ +Ġbare ly +ĠS E +Ġmar gin +re i +аÑĤ елÑĮ +Ġcont r +Ġv Ãł +Ġleg it +Ð ĺ +k ins +ÑĢ ÐµÐ´ +ĠAs h +Ġadv is +ĠGree k +Ñĥ к +Ġsh ake +id ades +аÑģ ÑĮ +Ġconv ention +Ġcont est +M S +ĠYe ar +Ġrepresent ation +ind en +end ar +Ġpr ost +ĠH uman +ĠC y +ang ed +P A +Ġax is +Ġthe ore +at z +Ġíķĺ ê³ł +Ġel s +ĠR esearch +Ġbene fic +Ġd ensity +ind o +ìľ ¼ +im di +Ġresearch ers +ê±°ë ĵł +igh s +d an +Ġd ice +Ġma ar +Ġsub mit +Ġd umb +Ġb ij +a way +ĠP ass +Ġext ension +Ġcr ush +Ġcover ing +ed i +b orn +in ations +ĠÑģ дел +в еÑĢ +ĠOther wise +ist ant +ай ÑĤе +Ġt anto +Ġperform ed +Ġз ап +al o +ĠFound ation +Ġprot ocol +ĠZ o +m ay +Ġha ck +Ġbud dy +m ade +Ġad s +Ġfasc inating +Ġequival ent +g el +Ġar c +ĠÑĩ елов +Ġprop osed +Ġnot re +ang es +Ġcoun sel +all a +Ġ3 1 +we et +È Ļ +Ġelectric ity +Ġto x +ÅĤ ad +Ġì ´ +Ġdifficult y +ł ×Ļ +nes day +иÑģ ÑĮ +Ġall eg +ĠG O +Ġqu it +ĠHer r +Ġestá n +Ġgirl friend +Ġt eng +ific ial +ĠJ am +Ġcan cel +Ġfrequ ently +I V +å¯ ¦ +Ġclos ing +Ġdec ade +Ġrepresent ed +ĠCan ad +ĠкоÑĤоÑĢ Ñĭе +Ġest amos +ĠTh ursday +ĠG a +ĠL ive +le m +b ble +S ON +Ġ200 8 +Ġd ich +ĠAw esome +Ġconcept s +PE AK +Ġliter ature +ĠO lymp +л ад +Ġn ost +v it +ĠEn ter +ord ers +ick ing +nie j +Ġe uch +ĠTh ough +Ġbag s +Ġlim its +Ġst ake +ĥ ¥ +Ġo c +ĠV is +Ġ1 20 +Ġn ue +Ġcon ce +Ġdis ag +ç ¨ +Ġant icip +ł Ī +s l +Ġvot ing +Ġexp osure +ĠCommun ity +ĠJust ice +or ney +szy st +Ġfr ied +ìĭ ľë +ĠW in +Ġ @ +ĠHope fully +es z +Ġm onde +Ġcomb ine +g ment +Ġrecommend ations +Ġpregn ant +ìĭ Ŀ +ra f +Ġl u +èĢ ģ +ä»Ģ ä¹Ī +do or +аз Ñĭв +ue go +Ġimprove ment +Ġtr im +Ġe igen +Ġapproxim ately +Ġв ам +aw a +ĠÑģ об +Ġcor on +Ġon going +Ġh es +Ġinj ury +Ġfr ank +Ġkad ar +ren cy +ĠCol or +ĠG ru +Ġd ip +ÑĢ Ñĭ +Ġte ars +g t +ĠP D +Ġpa use +os c +Ġ usted +ĠW oo +Ġw iÄĻ +è¦ ĭ +Ġden n +ĠP et +Ġover come +ĠëĤ´ ê°Ģ +ĠM ove +Ġlic ense +Ġrepe ated +௠ĩ +Ġcateg ories +Ġnood les +Ġflo od +ĠM ass +Ġn uts +ĠJ ess +ĠI h +Ġch ances +IJ ĺ +Ġdon de +I G +Ġand ere +Ġb ones +ìŀ ij +Ġeffic iency +Ġmod er +ro at +ĠìĿ´ ê²Į +ill er +Ġom ega +Ġп ов +ĠGr oup +Ġprodu cing +am o +Ġparticip ants +u pp +if ice +Ġfort un +iet nam +ac ak +ĠK o +mi ÅŁ +Ġj ail +ĠJ ones +ÅĽ my +ĠDe uts +Ġbrief ly +ĠT al +ĠPer haps +ĠR ub +ĠK n +ëĭ¤ ëĬĶ +r é +Ġvocê s +ĠChar les +еÑĤ е +ri ers +Ġhe al +ant ee +Ġdemocr acy +Ġlo an +Ġche f +Ñı м +Ġuncom fortable +Ġet ern +app ing +Ġrep air +r ot +ĠT ar +Ġco vers +om ing +ĠE th +ĠÎ Ń +Ñĩ но +Ġafter wards +Ġв еÑĢ +Ġd aha +Ġkn ees +Ġord inary +ü l +g as +Ġtick et +ĠìłĢ ëĬĶ +ĠìŀĪ ìĬµëĭĪëĭ¤ +ch te +M r +Ġs ist +h ui +ê· ¸ë +ìĹ ¬ +Ġv ary +Ġmem or +Ġcontro ller +ĠbÄĻd zie +Ġmin ister +× Ĵ +fl ow +A H +Ġto wer +ç IJ +Ġsc ar +æĥ ħ +ĠP en +Ġpa ÃŃs +× ĺ +ìĿ ¸ë +Ġener g +Ġsw ord +Ġpap ers +ил а +ĠWed nesday +ĠFor ce +Ġextraord inary +ĠL ake +Ġê° Ģë +ĠBe aut +Ġreason able +Ġcontrib ute +Ġple ased +Ġupd ated +Ġpi ù +el o +Ġsignific antly +Ġb ot +Ġgener ations +Ġprotect ed +åĵ Ī +Ġh iding +ĠI ll +Ġneut ral +] , +ÏĦ ο +Ġtong ue +Th ank +Ġê³ Ħ +Ġpay s +ί ν +Ġapp le +0 1 +er k +ier a +Ġj eg +ĠS ubscribe +Ġthe ater +Ġstrong ly +ĠìĨ Į +ĠпÑĢ Ð°Ð² +uck y +ĠJ in +k ward +ê± ´ +Ġoppon ent +ĠS O +Ġho ly +Ġfill ing +: ] +Ġh ij +Ð ľ +Ġb iss +Ġbl end +Ġim plic +Ġì ½ +lle icht +ÙĬ Ø© +as ant +ert e +ĠS ame +Ġinter ior +S e +Ġben ch +Ġpo co +Ġmark s +Ġw ins +åĸ Ķ +ĠÎ ³ +Ġdist inct +ĠAs ian +Ġmole c +ĠJack son +Ġe ast +Ġphys ics +im al +Ġpe ak +ar ian +ep s +Ġne at +Ġв аÑģ +ur ning +Ġsyn th +Ġreve al +Å º +g on +n is +at iv +ĠL as +Ġp y +ĠMaj esty +ĠV alley +Ġen f +Ġg ens +Ġro ots +e ze +b et +Ġact s +é ļ +è IJ +Ġphilosop hy +Ġmatch es +Ŀ i +Ġju ż +Ġdes per +ĠEduc ation +Ġsp ots +Ġreg ions +A r +ĠN am +e en +Ġdi agram +Ġre ly +Ġt ens +Ġd ating +Ġco at +ĠH or +Ġacknow ledge +ĠPret ty +Ġп оп +Ġvo ir +Ġfavour ite +Ġmo ż +Ġk m +ĠD O +Ġf ert +Ġë ıĦ +ĠP ac +Ġf ont +Ġfind s +ĠIt aly +Ġк ол +Ġcomp ass +ë ³ +li ament +Ġnot ion +Ġin ject +Ġwis dom +Ġà ľ +ĠMo on +ĠBus iness +r ics +ĠY out +Ġforg ive +Ġfin ance +il o +Ø £ +ah l +Ġdem o +Ġclim b +Ġexp ort +å ł +Ġsuccess fully +ĠF er +pect ed +d em +Ġret ire +Ġlapt op +Ġsp ir +ĠAssoci ation +Ġг л +ĠS el +Ġíķ ľë +Ġemploy ee +Ġm olt +R L +Ð ¯ +Ġcont ra +Ġu g +ĠB all +ĠJ ava +ér ie +Ġproced ure +Ġgr id +ĠëĬ IJë +Ġbel t +ĠÑįÑĤ ого +ur d +Ġcomp reh +Ġdevelop er +ĠÑįÑĤ ом +å ĺ +c r +Ġë ĵ +Ġsp oken +ren ce +Ġterm in +Ġaggress ive +Ġbiss chen +Ġhast a +ĠB rian +ĠComm ission +ĠY u +Ġprom ised +Ġequ ity +ik o +ver ty +Ġrepl aced +ĠHel p +Ġp ose +ĠMid dle +Ġk im +Ġme in +ĠCoun cill +ĠÐĴ Ñģ +or o +ĠB ern +Ġbe z +Ġanal yt +ang en +Ġìĭ ¶ +ĠG lo +Ġqu ad +ÑĤ а +Ġspeak s +ìĺĪ ìļĶ +ĠìĹ¬ë Ł¬ë +f ree +н Ñĸ +r ich +Ġë ¯¸ +ĠD ies +ab b +¥ ¸ +Ġdep ression +Ġret ail +Ħë ĵ¤ +ĠV ous +ĠLat in +á ¹ +Ġì¢ĭ ìķĦ +Ġtor t +Ġcomput ers +Ġsearch ing +Ġt ub +ate ll +Ġm erc +Ġglass es +p erson +Ġdish es +Ġguar antee +Ġme g +s m +ĠW alk +ìľ¼ë ©´ +Ġfold er +ĠM it +Ġtim ing +Ġab st +ĠL og +ãĤ ¯ +Ġappro ved +ĠUS A +в еÑĤ +Ġw ise +ess ed +Ġdou b +Ġres ident +Ġgener ated +Ġstay s +Ġexplan ation +Ġpo ison +at re +Ġins ane +Ġrefer red +ai res +ĠT RA +Ġse i +Ġinn oc +A h +Ġm ant +h us +Ġout er +ge b +o ice +Ġdiscuss ing +Ġconven ient +_ _ +Ġavo ir +Ġshap es +Ġgr ay +Ġdent ro +Ġm acht +Ġ19 5 +Ù ı +Ġadd s +ut ing +Ġcap abilities +Ġse ctions +Ġt une +ĠC ause +ard e +ĠÑģк аз +av irus +ĠR E +Ġtun ed +Ġle af +ter ior +ĠCapt ain +ĠØ ¬ +Ġcho osing +h in +gg ing +v iet +Ġreg ret +2 6 +ond ern +Ġbon us +ĠR ay +A s +Ġtor n +ĠH ier +ĠE U +Ġris ks +Ġam a +ĠY et +Ġcharacter istics +Ġê° IJ +ĠSen ator +ĠV amos +Ġr ose +Ġcorpor ate +g han +Ġcent ers +st airs +Ġn it +Ġunus ual +ĠT ony +ĠG R +ĠW ild +ĠSim ilar +Ġtod as +åģ ļ +Ġhoriz ont +m el +Ġstr ict +Ġc ual +Ġwr it +Ġext ended +Ġíķĺ ëĬĶ +Ġrel ief +Ġon ion +Ġbab ies +Ġdif er +Ġintegr ated +üz ik +ep ing +-- -- +Ġm ens +Ġstrateg ic +fin itely +Ġeig entlich +W ho +åľ ° +Ġ { +Ġ ä½ł +ĠT ri +Ġpoint ed +ð Ŀ +n ament +е ÑĨ +Ġpr ide +ĠRepublic an +Ġsam ples +Ġdom estic +L Y +ve z +Ġweb inar +ا Ùħ +Ġen h +Ġsuggest ed +Ġme ine +Ġpu ed +ore n +r ir +Ġheav ily +Ġinst ruction +Ġmicro phone +Ġig ual +ĠI ra +Ġvulner able +ĠVirgin ia +Ġcontinu ous +Ġpo verty +Ġbl ade +ä¸ ī +Ġrel ate +Ġcar a +ĠGo ing +Ġreg ional +ĠF uck +Ġto w +ĠM useum +r ants +Ġб ез +la im +Ġchamp ion +t le +ÃŃ n +en cia +Ġdies em +ĠD ig +m ates +Ġinvest ing +ĠJ ordan +Ġintegr ation +Ġí İ +ภ« +ens us +ĠAr ch +Ġpen cil +алÑĮ но +iss en +ĠK a +Ġro cks +Ġr ating +Ġref uge +Ġa pr +et ed +Ġassist ant +Ġmeaning ful +Ġperman ent +Ġh ill +Ġw szyst +Ġw ound +ĠAt l +Ġl ake +ĠF ort +è¬Ŀ è¬Ŀ +Ġredu ction +Ġv iv +Ġs our +Ġe cos +Ġha z +Ġste al +Ġmy ster +ĠÐļ ак +ĠÑį ÑĤи +ĠV ietnam +Ġant es +Ġconnect ing +éĸ ĵ +ĠD ave +Ġb öyle +ĠC ast +L e +Ġc ul +Ġgen re +ë§ IJ +Ġcompl ain +Ġhur ry +art e +g reg +Ġmonitor ing +Ġdes ert +ĠÑģ ов +el ing +ĠSup reme +Ġgib i +Ġlar g +Ġn ations +ĠT ok +Ġneed le +æ µ +Ġas leep +Ġcom un +ĠJew s +Ġachie ved +Ġex it +Ġdise ases +l ines +ãģĭ ãĤī +ri ends +Ġre ct +Ġsc an +ãģ¯ ãģĦ +Ġhur ts +z ÄĻ +ĠLook ing +ãĤ · +í Ĵ +ult ural +á» ĵ +in ent +Ġp ues +Ġche ering +§ Ģ +ag ger +Ġad a +La ughter +ĠW omen +è£ ¡ +è « +Ġoccur red +Ġse ats +èĢ Į +Ġemp ower +un u +ell ing +B ER +ens ional +Ġcon sole +as hing +Ġein mal +f are +Ġëı ¼ +Ġs essions +Ù IJ +Ġridic ulous +ÃŃ an +ĠHen ry +ĠH ol +Ġcollect ed +Ġdiscuss ions +D e +Ġdis ability +Ġí Ľ +Ġsubscri bers +Ġir gend +Ġf el +Ġdirect ions +Ġmanufact uring +ĠR od +Ġìĸ ĺ +à¸ Ĺ +æĺ İ +Ġcriter ia +Ġmo ld +è© ± +Ġent ering +ri j +is en +ĠP ara +ie ve +Ġchar ged +Ġj ou +Ġc ats +л ед +ad ays +ан ов +j ÄĻ +v ation +Ġast ron +it als +ĠB rand +ĠK an +Ġpl ain +Ġand eren +and e +Ñı з +Ġto ler +ÅĤ em +Ġpr é +м оÑĤÑĢ +age ment +u ct +ch é +ĠE ner +aj Äħ +Ġíķ ´ë +Ġst a +Ġr ings +Ġtoil et +ĠC ra +Ġexperien cing +Ġsl ip +Ġsand wich +ĠUs ing +Ġspect rum +ĠR os +ap se +ĠJ ay +м Ñĥ +æ³ ķ +E x +Ġrecogn ition +ĠDid n +ud a +aj e +est ly +Ġfem in +it ure +ÑĢ Ð°ÑĤ +Ġh ire +Ġnow here +ä½ į +Ạ§ +Ġw ing +Ġsa v +ĠSec urity +Ġr ural +ĠF un +ay er +Ġac cus +Ġm m +ĠJose ph +Ġsc reens +Ġb orrow +Ġsw ing +Ġ4 8 +Ġtouch ing +th is +int endo +é ĥ +Ð ł +ĠScot land +ĠJ ason +ĠV en +Ġexcept ion +Ġnear by +Ġbrows er +ang ers +ĠS in +ÏĢ Î¿ +ä½Ĩ æĺ¯ +osp el +Ġwur de +Ġdr unk +í ļ +ìĨ į +ãĥ ī +ĠìĬ ¤í +ĠL ie +oc o +ĠLe ague +Ġign ore +Ġ: ) +Ġland ing +Ġع ÙĦ +ĠT ag +2 8 +Ġdra ft +Ġa er +Ġê·¸ë ĥ¥ +Ġp ense +Ġд аже +Ġbed room +Ġna j +ì§Ģ ê³ł +igen ous +Ġde als +ell o +äº Į +Ġpos it +ê » +Ġvis ited +if ies +Ġpre mi +Ġcan t +ĠR ick +Ġrais ing +Ġperm ission +Ġpub l +un ci +Ġb end +Ġchamp ions +d ie +Ġaw ful +Ġjump ing +Ġll eg +Ġsustain able +ĠT ot +Ġcand y +åĢ Ļ +Ġsatisf ied +Ġpi pe +Ġco ck +Ø ¶ +st one +Ġmoment um +ĠÐĿ а +Ġal ors +Ġreturn s +am men +ç ® +Ñĭ м +a wn +ott ed +Ġwoll en +ict ed +Ġcandid ates +ĠL ady +Ġy ield +Ġmainten ance +ff ect +Ġexpans ion +ĠL ED +Ġdark ness +Ġout fit +ìķ Ī +Ġи Ñģп +ru ption +ãģĦ ãģ¾ãģĻ +Ġeng aging +Ġins ight +ĠAl ways +Ġge f +ra k +Ġp ix +覺 å¾Ĺ +Ġquant ity +Ġin k +ĠKing dom +Ġc ort +å¸ ¸ +Ġgovern ments +Ġprot est +po on +ĠÑĤ ого +å® ĥ +uch en +qu ality +ĠPor que +ĠCl ub +Ġr it +Ġart icles +B I +ig ible +Ġdis aster +и г +Ġн ик +Ùĩ ا +ë ¥¼ +are t +Ġun able +Ġà ® +Ġer st +Ġ× ł +v ard +Ġannoy ing +ĠK ir +еÑĢ Ð¶ +enn is +Ġunc ertain +3 6 +ö s +Ġecos ystem +z ed +j Ãł +s un +ìĸ´ì Ħľ +Ġże by +Ġma ps +ë Ĥĺ +åħ ¨ +ĠJust in +Ġtr ash +Ġenorm ous +Ġst ated +Ġbrand s +Ġyou t +ĠÑĩелов ек +ĠMat th +Ġtransport ation +Ġlegisl ation +Ġprov iders +ĠØ Ń +Ġmagaz ine +Ġse hen +ĠDesp ite +Ġpass es +æĪ IJ +Ġal ter +ad an +Ġfarm ers +è° ¢ +Ġconfir med +Ġes a +it os +Ġroad s +V IS +Ġwork er +Ġdesign s +ĠSo viet +br id +Ġpract icing +Ġë¶ Ģ +ĠSe a +ãĥ © +ĠпÑĢ Ð¾Ð´ +Ġch ill +Ġlem on +ìĹIJ ëĬĶ +Ġflex ible +ĠExc use +Ġterrit ory +åķ ı +ãģ ¿ +Ġl ux +Ġlif etime +Ġdist ingu +ĠT imes +Ġgr oss +en z +Ġsc roll +m Ä±ÅŁ +ci p +£ ¼ +D P +Ġpub lish +Ġe ben +Ġreg ist +Ġed ition +ĠL E +Ġchar ging +ut ation +yr ics +id as +ĠÎ ¿ +Ġк оÑĢ +ĠT on +çĽ ® +Ġwho ever +ĠF ox +æī ĭ +ê±°ëĵł ìļĶ +Ġf ought +Ġdr ill +ĠAf ghan +~ ! +ĠTo o +Ġsecond ary +r ä +ĠHe ad +in nen +Ġy arn +Ġн ам +Ġwid th +Ġengine er +i Äħ +Ġw ings +ĠëķĮë ¬¸ +Ġtra uma +Ġrep rodu +Ġch ip +Ġpassion ate +Ġaw kward +Ġí Ĭ +аж д +ĠBit coin +Ġkh ông +Ġr ó +re ction +Ġг де +ĠUs ually +Ġimplement ation +Ġgame play +Ġmyst ery +Ġо к +Ġa ños +and y +им и +Ġpriv acy +ac o +ãĤ ģ +Ġd ump +ĠP ay +Ġdi pl +Ġf urn +Ġsh ips +L A +ĠÑħ оÑĢоÑĪ +Ġe c +Ġdrop s +ch l +3 2 +Ġobs erve +ĠDe velop +M üzik +ann el +owa Äĩ +Ġfac ed +á l +Ġvictim s +Ġg ifts +Ġbo ot +ÃŁ e +ro d +Ġ200 9 +ör t +Ġunivers al +Ġn ouve +Ġboy friend +Ġcet era +ÑģÑĤ а +' S +Ġn ive +Ġcru cial +Ġsur ve +Ġco in +Ġs ondern +Ġsh ade +Ġl ugar +Ġsure ly +Ġma x +Ġimpro ving +åĽł çĤº +Ġw en +Ġ× ij +Ġìĸ ´ì +Ġen forcement +ib l +Ġli v +ler i +Ġme jor +ĠCarol ina +Ġv as +Ġcomp rom +Ġd irt +Ġup grade +ĠBe ll +Ġrestaur ants +Ġtra p +Ġte as +b lic +ĠG reg +s an +Ġo w +u est +Ġpropos al +ĠR et +fr ont +Ġpass age +Ġsurround ing +Ġú lt +Ġup coming +Ġhor ror +Ġcl othing +Ġìķ ½ +Ġd il +r ome +ĠI d +ĠRo ad +ĠÑįÑĤо ÑĤ +ch ain +ĠбÑĭ ÑĤÑĮ +ĠO ffic +ĠÐĿ е +Ġins an +Ġdecre ase +ĠÑħ оÑĤ +bu ild +ĠDrag on +å Ĥ +Ġinvest ors +ant i +Ġsacr ifice +Ġtro ops +ĠB ad +Ġpass word +Ġconst ra +ภķ +ĠÃĩ a +ad ow +th rough +ÑĨ а +C an +Ġcandid ate +Ġant ib +ìĹ ħ +Ġtast y +ÙĪ ÙĨ +ĠIn f +ĠB ang +iÃŁ t +in ity +f ather +Ġcontro vers +ĠP ak +il ty +êµ ¬ë +Ġlight er +Ġfall en +Ġz us +ĠGu ard +Ġc ott +ĠF ree +Ġiniti ative +al ous +Ġnot ification +ĠMed ic +ĠComm ittee +ìĹ ° +ĠW ood +Ġmus h +ul um +è ² +ah ah +Ġsu fficient +Ġsing er +к ой +AL I +ät t +ĠP R +ĠL ar +cul es +iem po +Ġune x +Ġintegr al +Ġtransm ission +Ġic i +Ñĥ Ñħ +g ic +ĠN intendo +ĠC op +ĠTr ust +en as +Ġab ilities +Ġch ips +p at +Ġan che +л ен +Ġapproach es +Ġth or +Ġs isters +Ġdri vers +Ġall a +0 3 +Ġrub ber +Ġn Ã¥ +AC K +Ġdisappe ar +ê° ľ +Ġcomp ens +Ġv ibr +ç¬ ij +G O +Ġs izes +Ġtrack ing +íĻ Ķ +ĠìĦ ¸ +Ġimpact s +ib il +f ish +B R +Ġar row +Ġlarg ely +ann y +Ġlaw yer +æĢİ éº¼ +j ours +Ú º +v ia +Ġde lla +Ġmath emat +ĠM ine +ĠK oll +Ø ² +ĠC ross +Ġ6 5 +Ġgr ac +Ġinvol ves +Ġdel ight +ĠHolly wood +Ġimmedi ate +on ic +Ġl ado +Ġbull et +ĠN ote +Ġun lock +Ġdisc ount +Ġris ing +p ress +Ġp ace +Ġsh orter +Ġten er +ge on +Ġman aging +Ġc ere +C hr +Wh en +ach en +Ġì ĵ +ĠH un +Ġof t +Ġ2 50 +ier ung +Ġst abil +ĠCon nect +Ġy ani +Ġdow nt +μ α +Ġvoc al +ν α +Ġle an +Ġvidé o +ĠFam ily +res ent +Ġamount s +ì§ ģ +cl ass +Ġv ib +ĠA v +ar se +Ġgentle men +Ġsee king +Ġun ion +Ġregular ly +æ ı +ĠJ ahr +ĠF ood +ĠPro blem +Ġart ificial +ĠS ix +Ġimp ressed +Ġto oth +ĠK h +Ġy ard +Ġíķ ´ +Ġown ed +Ġëı Ļ +ì² Ń +Ġto da +Ġport fol +ĠëĤ ¨ +orge ous +Ġd ates +олÑĮ з +еÑĩ но +Ġconfig uration +Ġrequire ment +Ġbell y +Ġpain ful +Ġdemonst rate +Ġg leich +Ġvis iting +ĠCon f +Ġd al +Ù ij +Ġam end +ĠF ur +æ¯ Ķ +Ġv ital +á» ĭ +Ġm ate +ĠO u +Ġleg acy +ust ing +Ġaccom mod +Ġqu oi +au en +Ġlif estyle +C C +ä än +art en +Ġmin ha +r ó +Ġëª ¨ +Ġform ation +Ġtra iler +per or +Ġг ÑĢ +Ġu d +z u +Ġk ommen +Ġc ave +ĠCouncill or +Ġthr own +Ġtrick s +LAUGH TER +ĠAcad emy +row d +¡ Ŀ +ìł Ģ +ĠIm agine +Ġinform ed +ro ph +Ġl ig +Ġsk ull +ab eth +Ġfunction al +ere k +O n +é ¦ +Ġanc est +Ġsaf ely +ĠH T +ëĭ ¹ +Ġd av +Ġdri ves +A meric +Ġt ire +Ġsa is +á ri +av ors +Ġcorrespond ing +Ġpr és +ch est +Ġbacter ia +Ġinfect ion +us al +Ġave z +Ġbasket ball +Ġsuppl ies +Ġexpert ise +ł ¥ +f a +Ġt iempo +ĠW ant +Ġs illy +Ġu pp +Ġelect ed +Ġf ired +ĠØ ¯ +Ġunivers ities +all e +Ġjack et + ° +Ġtra v +l s +Ġdefe at +Ġco gn +Ġequ ations +uk i +ĠS her +Ġth irty +Ġstream ing +ot ros +ĠPro du +ne j +Ġdesign er +ĠëĬIJë Ĥ +Ġpaint ed +ra ine +m ail +Ġv ess +Ġrhy thm +les h +Ġ9 9 +Ġa inda +ch ied +Ġét ait +Ġaffect s +é £ +ĠF ind +Ġé l +Ġpotato es +Ġp ag +Ġп аÑĢ +art s +ĠN ach +Ġ3 3 +ĠH ard +ĠIra q +Ġopin ions +w ith +erm an +à ½ +è Ń +ĠS PEAK +¬ ¼ +Ġst ability +ĠH E +Ġcapt ured +Ġbu cks +Ġmas ks +Ġcompet e +Ġforgot ten +лÑİ Ñĩ +se qu +ĠìĦ ł +ill ion +Ġgraph ics +Ġh ub +ĠìĹ ° +em por +Ġcr own +Ġw ider +Ġocc urs +D S +æ ģ +ĠB attle +ãģª ãĤĵ +Ġd ual +Ġ6 00 +ath ers +๠ģ +Ġsett le +Ġav ait +Ġdo is +ки Ñħ +ad ores +Ġà ³ +ne go +ĠGeorg ia +ĠR og +Ġdiv or +ĠS ong +ĠSpe cial +Ġm un +Ġpret end +M AN +Ġviol ent +Ġbes ides +v y +ĠN az +2 9 +Ġswe at +Ġz w +ĠH u +ĠBu ild +Ġh orm +ĠC ard +Ġìľ ł +Ġrecommend ation +c alled +st ick +ĠPol ice +Ġconsum ers +Ġgro cer +Ġst un +ĠÐĴ Ñĭ +Ð £ +ĠD ata +Ġsubst ant +ire ct +à ² +Ġв з +ĠAr m +Ġsem ester +ĠBr ad +Ġour s +ĠкоÑĤоÑĢ Ñĭй +§ a +Ġgr ams +Ġexerc ises +7 5 +Ġswe ar +Ġinc ent +Ïģ ο +Ġilleg al +ä½ ķ +ĠDam n +Ġn ú +Ġne ces +Ġcu z +ic on +Ġh ors +ĠCom o +ä½ ľ +Ġëij IJ +Ġover se +Ġhar vest +Ġth rew +ĠпоÑĤ омÑĥ +×Ļ× Ķ +Ġot ro +ĠпеÑĢ Ð² +Ġsc ope +Ġgl ory +ĠMich igan +Ġassum ing +ĠÑĥ д +Ġbo ld +g ue +m other +Ġw aren +è¬ Ľ +ĠØ ¥ +ĠK am +isp iel +Ġtou jours +Ġconst itution +Ġ ~ +Ġfrank ly +ol en +ons cious +Ġwür de +th on +ĠO F +ìŀ IJë +und a +Ġ æĺ¯ +Ġп оÑĢ +Ġemploy ment +Ñij ÑĤ +ãģ ģ +Ġste am +ĠD I +Ġprofession als +Ġengine ers +ĠX ia +ç « +ìĺ ģ +ĠD un +Ġbit ch +ĠF ord +ä¸į è¦ģ +se ction +Ġv ice +ĠL ater +ost on +Ñį ÑĤ +× ¦ +ĠAz ure +pl ing +Ġ18 0 +ĠC reat +IS HA +Ġbu eno +íĿ ¬ +ru p +l ers +ĠY ang +ĠH A +b at +ĠCath olic +Ġacc ent +Ġmix ing +ck ets +Ġenh ance +ü hr +ê s +Ġí ĸ +Ġswim ming +Ġcá» §a +ĠEl iz +Ġimm une +Ġб ол +Ġf are +ĠG ab +× ¡ +Ġs atell +ĠAny thing +Ġass et +Ġsched ul +Ġrad ical +Ġzwe i +ĠM E +rel ated +Ġsepar ated +ĠL ibr +Ġg rip +Ġà® ª +è¨ Ģ +Ġbe ans +ĠO p +ìĨ Į +Ġp ound +Ġentr ance +Ï Ĩ +ĠN ie +ĠRepublic ans +Ġat om +Ġperson as +ĠAl i +ä hr +å¤ ĸ +Ġdram atic +ĠF ine +Ġremind s +è Ļ +Ġdé jÃł +Ġafford able +Ġbr an +ier o +al ar +c u +Ġ \ +Ġmo że +Ġbl ast +Ġrec y +f ire +Ġ lle +ĠвÑĢем Ñı +ĠW W +Ġv s +ĠD ude +ĠR ome +Ġg reet +ĠH et +ci as +Ġëĭ ¹ +less ly +Ġprem ium +Ġexper iments +at ar +é ri +Ġoffic ially +Ġfe e +๠ĩ +ĠÑĩ ем +re a +Ġto y +O P +ĠTay lor +ĠMc C +ile y +ĠB ak +Ġal um +ĠUn ter +Ġmag ical +Ġtra bal +Ġvot es +it age +Ġmechan ical +h n +ãģ¾ ãģĹãģŁ +Ġин ÑĤеÑĢ +ä»Ĭ 天 +Ġh int +Ġauthor ities +ĠNAS A +ivers ary +Ġпо Ñĩ +r ac +ĠSPEAK ER +ö t +Ġfr ames +Ġgood bye +Ġch er +h u +Ġne ur +å® ļ +ĠM ach +ĠHe ll +Ġfest ival +ëħ Ħ +ut a +Ġmush room +Ġt ant +Ġtat to +Ġdel ete +Ġd iz +Ġv ä +Ġse vent +ĠQu ick +Ġb aking +Ġassemb ly +G o +ĠD ream +ĠL ad +éĿ ŀ +â y +ag s +Ġgrav ity +Ġì§ ij +em ploy +Ġdies es +Ġdisco very +ÑģÑĤв а +Ġheb ben +Ġger ade +ĠD R +Ġ' ' +Ġtechn ically +ĠÐŁ о +Ġprivile ge +ĠE ver +ĠServ ices +ur an +Ġconsum ption +ĠRe v +ĠSh all +ass er +¶Ģ íĦ° +Ġrac ial +ĠYout ube +ĠP ra +ÑģÑĤв ен +ce k +æ ´ +ash a +Ġ Ûģ +ij ľ +ah n +IC K +Ġdrink s +Ġcar b +ãĤ ¿ +Ġ6 4 +ĠM mm +Ġelectr ical +Ġfr uits +ĠH D +ñ a +ĠDe finitely +Ġë° Ľ +Ġf ais +r ations +Ġco e +ah u +ĠF air +Ġeat en +Ġf ir +ĠA u +Ñĥ н +ul ating +ing ly +Ġvacc ines +Ġdrag on +Ġpoint ing +Ġpel o +or ters +Ġwork out +им еÑĢ +m ond +ĠN ope +Ġ× ĸ×Ķ +ĠÙ Ĥ +Ġadopt ed +b ul +Ġs ans +Ġposs ibilities +Ġp end +Ġz aman +h ou +Ġsha res +Ġcal endar +Ġperson a +Ġse al +Ġgen e +Ġst ored +Ġп оз +Ġl yrics +Ġinstr uments +ĠM A +Ģ ìĿ´ +Ġcloud s +h ot +Ạ¯ +Ġê°Ļ ìķĦìļĶ +ĠEmp ire +Ġb io +w ind +ie go +ĠEu rop +Ġ 好 +ed ge +Ġback wards +Ġì§ Ģë +Ġque en +Ġsh ine +Ġç ık +Ġc ad +ĠO d +ĠìĤ¬ëŀ Į +Ġbu bble +ô i +z es +Ġreact ions +Ġjud gment +ĠDemocr ats +Ġcos as +ash ed +Ġдол ж +ÅĽ nie +ê ´ +Ġexem ple +M P +á» ¯ +ĠV ers +Ġres il +Ġm á +ÅĦ st +bel iev +ĠV or +Ġsch eme +ond a +Ġpod emos +Ġchar ges +Ġdest ination +ĠK y +ĠS S +Ġsil ence +Ġem bed +n at +á» Ľi +AN T +gg ed +Ġredu cing +Ġug ly +Ġm im +Ñĥд а +3 4 +Ġc ord +ĠÑĤ оже +ĠL isa +Ġö n +ĠChrist ians +umb les +olog ists +az a +Ġt ends +ĠC ook +Ġges agt +Ġíķĺë Ĥĺ +ĠT es +erem ony +ĠнÑĥж но +ĠMAR ISHA +Ġen roll +ĠC ry +ES S +ĠS ad +Ġimplement ed +Ġd ÃŃa +à ľ +Ġp ist +D r +Ġs abe +ĠSo ci +ä re +Ġк ÑĤо +ĠFranc isco +Ġìŀ ¥ +Ġcreat ures +aw s +Ġearn ed +Ġche aper +Ġd la +Ġw arn +s che +Ġbl ah +Ġnut r +è ¼ +Ġg orgeous +ĠAng eles +Ġgem acht +Ġhom eless +ograph ic +ĠTai wan +ĠS om +ĠH ad +act ions +Ġpost s +Ġout ra +ĠMe an +k ar +Ġc ous +Ġbr ack +иÑĤÑĮ ÑģÑı +Ġbelie ves +Ġsu icide +Ġequ ally +Ġca res +ож но +Ġst em +ĠM uch +Ġprodu cer +×ķ× IJ +Ġprotect ing +ĠTRA VIS +Ġinterview s +Ġal ien +ĠAs k +Ġso le +C O +ĠS ud +Ġsurv iv +Ġsk etch +Ġw ÅĤa +Ġcol oc +Ġapolog ize +we ight +Ġ5 5 +Ġ > +Ġhero es +ĠB oston +Ġdepend ent +Ġmotiv ation +fl ix +Ġse am +ки е +Ġdra in +od ed +Ġgu ilty +ĠJ enn +ing en +Ġgrant ed +ĠK elly +ĠS av +ĠUn cle +ĠHon estly +EL I +Ġnavig ate +Ġbless ed +c ore +Ġear ning +Ġsign als +Ġdis k +ial s +Ġag es +æ ħ +Ġpartic le +ĠÑĩ еÑĢ +Ġcan n +Ġt ier +Ġstat ements +ê³ł ìļĶ +ĠëķĮ문 ìĹIJ +ĠCh o +Ġpol ar +an ç +ĠK enn +ĠN i +ĠF ight +or gan +é ķ +ĠCh a +ĠS ÃŃ +ãĥ ª +Ġs lic +Ġcert ific +Ġtempl ate +ĠFed eral +Ġconsider ation +Ġexpl o +ĠM ain +ĠN E +Ġalong side +Ġd ressed +ĠP oint +Ġenviron ments +Ġpró xim +Ġda ar +Ġprom pt +Ġpurs ue +Ġentertain ment +Ġth roat +Ġproblem a +Ġm art +ì ¼ +Ġprov ider +Ø Į +Ġ× Ĺ +int e +m aking +Ġstro ke +Ġtiss ue +U n +Ġpre cious +ĠAr ts +ink ing +ĠÐŀ н +Ġи Ñģ +n ah +ĠÐķ Ñģли +Ġcor ners +Ġtrick y +in ch +l ijk +Ġpress ing +le vel +AN G +Ġrad iation +ìĦ ł +Ġconf ront +Ġv et +Ġrepresent ative +Ġprop ag +Ġcra p +ĠDe c +Ġr amp +еп еÑĢÑĮ +u és +ess en +cri ption +Ġb ills +ĠMatth ew +Ġan ime +ấ t +Ġlow est +h as +sc reen +og rap +ал о +int on +ĠJ ah +èĢ ħ +it Ãł +Ġk ay +Ġrot ation +ĠW ere +abe i +Ġtri als +Ġle ver +ight y +Ġsp oon +Ġh unt +c ling +Ġdis m +ĠболÑĮ ÑĪ +Ġass ault +Ġíĺ ķ +Ġweek ly +Ġm ismo +Ġgen etic +ul pt +ĠStud ent +Ġreal istic +Ġauthent ic +æī ĵ +ast a +Ġarrest ed +Ġguid elines +Ġ×ľ× IJ +Ġд ав +ĠCom ing +f ür +Ġrequ ests +ĥ IJ +Ġanaly ze +Ġinter ess +Ġh alt +ĠO per +on om +Ġd uck +Ġwith d +s er +ĠÏ Į +ĠHist ory +Ġyout ube +ãĤ į +Ġsab er +w alk +f ont +Ġover view +3 9 +ü y +ett i +Ġfro zen +Ġf lesh +ÄŁ i +ĠP M +ĠìĻ Ģ +é ¢ +ÑĨи и +Ġê¸ °ë +íģ ¬ +Ġpr ose +oo oo +r ates +W S +Ġautom atic +Ġcollect ing +Å ij +Ġneighb ors +» . +ĠEx pl +Ġcir cul +co ver +we g +Ġstick s +Ġe ller +Ġw ww +Ġd orm +ĠEx per +Ġstat istics +Ġemail s +Ġgra ve +im iz +H S +Ġu it +, ' +Ġlas er +è ī +ĠÑĤ ем +Ñĭ ÑĪ +Ñī Ñij +Ġgen au +Ġtien en +Ġmed itation +ĠOr gan +Ġest imate +Ġë¬ ´ì +l ets +Ġn Ãły +Ġmind set +Ġres on +Ġm és +Ġnumer ous +Ġvie lleicht +ĠTh ird +u ous +ĠDe ad +ан д +H N +Ġrac ing +Ġag ents +ĠU t +Ġte ar +ĠH P +Ġchem istry +Ġsurv ival +æĸ ° +Ġconvin ced +Ġ ; +Ġreg ulations +ĠE S +åĴ Į +3 00 +Ġen se +Ġì µ +Ġd ict +G A +Ġah ÃŃ +åĭ ķ +Ġte j +Ġо ÑģÑĤ +ĠE lect +Ġintellect ual +Ġbi as +Ġbur den +çĤ ¹ +Ġìĸ´ëĸ » +Ġche er +Ġso ph +Ġportfol io +ub a +Ġest os +T V +F or +Ġas h +Ġkom mer +Ġcollect ive +Ġw rest +ĠJ etzt +ĠW at +re ich +Ġprim er +act ive +Ġm ie +ick ed +Ġhun ting +Ġtest im +Ġcompass ion +ĠØ ± +Ġbr ut +Ġsal ad +об Ñīе +Ġsol ving +Ġflo ating +ç · +Ġattract ive +ÙĪ ÙĦ +Ġper d +if fer +Ġsc ulpt +hh h +ĠWe ek +Ġent hus +Ġn ad +Ġmer ch +ĠíĻ ķ +Ġm ile +好 äºĨ +ĠÎ ¸ +ĠëĤ ĺë +éĩ į +3 8 +Ġch ains +ĠAl most +Ġtick ets +r in +ĠC C +Ġdistrib uted +abet es +Ġtemper atures +Ġg ained +Ġflex ibility +Ġscream ing +Ġab road +un o +Ġentreprene urs +ĠNet work +ĠCanad ian +Ġpre v +Ġs ö +ĠÑĤеб Ñı +ĠP oke +ĠP od +ĠTur key +çı¾ åľ¨ +Ġabst ract +Ġsn ake +ĠAm y +ĠëĬIJëĤ Į +Ġbra ve +ĠìŀĪ ìĸ´ìļĶ +ĠK al +Ġ200 7 +á rio +Ġmark ed +gin es +Ġall oc +ON G +Ġscient ist +Ġes ca +Ġrac ism +× ij× +ĠS ams +ĠP enn +Ġload s +Ġà® ¨ +ü ber +M e +ix ò +Ġper ò +an ne +Ġexp ressed +м еÑĢ +Ġmo et +Ġret urning +n ia +Ġexp on +P ro +Ġlo yal +M L +Ġl amp +Ġsh y +Ġcomp osition +ĠL y +Ġmagn etic +Ġprem ier +Ġmeasure d +Ġsumm ary +Ġattack ed +Ġfin ishing +Ð Ĺ +ç ¥ +Ġs its +Ġhyd rogen +Ġma i +ĠDeuts ch +as ı +Ġobt ain +v ie +Ġso it +Ġë° Ķ +Ġl ane +Ġconse gu +в о +Ġe ase +ak in +ĠF a +Ġunt uk +Ġbur st +Ġc um +al ım +ú blic +id i +ĠRoy al +ĠK on +Ġcommon ly +Ġremo ving +Ġj ur +il ib +Ġan ch +íĸ ī +ưỠ£ +ĠÐľ Ñĭ +ĠAn th +ĠS Ã¥ +Ġinter rupt +Ġst ere +ĠO S +ony m +ter y +ĠMar ia +ê² ĥ +Ġexpl oring +Ġtransp arent +Ġf ate +ĠJ ung +Ġgr up +Ġdark er +ĠD oug +Ġman e +æĶ ¾ +ạ i +d ri +lo ok +ĠDes ign +Ġtut aj +Ġhorizont al +re on +ort e +ĠCor rect +ĠSte ven +Ġv ine +0 2 +i Äĩ +Ġsie mpre +ĠK ey +åĥ ı +ĠG ames +Ġna ar +Ġshock ed +el ve +ĠR ose +ìĭ ¬ +Ġstop ping +oh l +ĠM ix +Ġsuff ered +Ġsig ma +Ġweak ness +ĠO w +ี à¹Ī +I F +Ġà® ħ +ad ed +ĠNet flix +an es +Ġrem ained +ir y +Ġr ip +ell t +Ġsil ent +Ġpro ven +Ġtox ic +Ġal umin +Ġmulti pl +al and +Ġ3 4 +0 6 +ĠB ru +Ġìłķ ë§IJ +J ust +b oy +Ġsho e +Ġcreat ure +Ġhead ed +ĠоÑĤ к +æ ± +Ġess ence +Ġremark able +Ġnú mer +Ġd rew +Ġpu zzle +ĠLibr ary +ĠF u +ash es +k k +ĠI st +¦ ° +ĠB ry +Ġc eremony +Ġà® İ +Ġc ri +e qu +ãĤ ¢ +Ġpri ze +Ġdim ensions +og ram +Ġle ather +Ġpop ulations +u um +Ġve gan +Ñı д +Ġcó mo +å Ħ +Ġstri p +å £ +Ġvac ation +ħ ķ +Ġme als +ili pp +Ġ ents +ar am +ric ht +Ġgra in +ĠSp ain +Ġche ek +ĠA ff +I ON +ĠBr ing +Ġ3 8 +iel en +ul u +ĠболÑĮ ÑĪе +Ġannounce ment +ĠÑĤ ÑĥÑĤ +ĠPro phet +ard o +3 7 +Ġw oke +Ġtransl ation +ĠN OT +ĠC L +Ġd Ã¼ÅŁ +ÑĨ Ñĸ +ac er +ĠL oc +Ġper ception +N O +Ġdies en +L ook +he art +av ed +Ġbound ary +Ġfl ows +Ñij м +Ġarg uments +Ġelect ions +ı s +Ġhe ck +Ġsuit able +Ġf iber +ĠSt ra +x y +ĠH um +Ġmonth ly +u per +Ġgol f +Ġl ately +ĠG ard +ĠR en +ĠA st +ĠF ant +аÑģ Ñģ +Ġobs er +ë ¡ľ +Ġeas iest +į Ķë +Ġwebs ites +p ol +Ġco con +Ġà® ĩ +ĠV eg +Ġwalk s +Ġint ro +Ġdirect ed +ĠAn na +Ġëĵ¤ ìĸ´ +ĠEaster n +ĠS aint +ĠB ow +Ġro ast +ĠU RL +Ġjed en +ur as +aj a +Ġse mi +Ġrapid ly +Ġtarget s +ĠCont rol +Ġb ah +Ġref lection +Ġcreat ivity +hold ers +Ġìĺ ¬ë +Ġamong st +Ġfeed ing +ÑįÑĤ омÑĥ +Ġвид е +Ġë§Įë ĵ¤ +ĠSm art +Ġrel iable +Ġvez es +Ġ× ¨ +ch uckles +az ione +ĠWilliam s +Ġa ç +Ġsle e +е Ñī +Ġtim eline +Ġthor ough +á» į +ĠO t +ạ n +Ġimag ination +Ġmechan ics +r ist +Ġclaim ed +ÏĦ η +ê te +ĠHur ry +ĠiP ad +Ġconst ru +ĠC la +ĠAl s +ä¼ ļ +ut z +Ġcult ures +Ġìĸ´ëĸ» ê²Į +Ġbelong s +Ġy er +ĠDoes n +Ġge omet +Ġb id +Ġfo am +Ġh ob +ĠBrit ain +Ġsubst ance +Ġann iversary +ĠëĦ Ī +Ġnot ed +Ġgovern or +Ġstock s +3 1 +Ġdi ye +ìĬ ¤ë +Ġre b +z el +Ġmultip ly +Ġoper ator +Ħ¤ ìļĶ +Ġwat ers +Ġd är +Ġuns er +ĠEliz abeth +é« ĺ +Ġincreasing ly +ĠG ro +Ġen gines +ir s +Ø « +Ġtre asure +P C +in ction +ir i +Ġacc um +Ġvari ation +Ġp om +Ġtit les +ĠF est +ó s +Ġeld er +ny m +r un +Ñı в +Ġinnov ative +Ġnom bre +Ġco inc +Ġfr anch +Ġent onces +Ġnicht s +Ġexc lusive +ĠChe ers +ĠB i +u je +æŃ ¡ +Ġp ok +ĠP rem +Ġrock et +ELI PE +Ġhosp itals +ri um +Ġjust e +Ġham mer +Ġquant um +Ġrespons es +ll y +end i +Ġact ively +Ġfr idge +i ate +l ong +Ġqu em +Ġdeath s +Ġsuper ior +ck en +ìĿ´ì ĹIJ +kt op +Ġgather ed +£ ¨ +Ġd azu +Ġreci pes +Ġbu zz +c en +Ġany time +ons ense +Ġcirc les +Ġsol ved +Ġìĭ ł +Ġcoron avirus +ĠLu ke +Ġbu bb +Ġcont empor +r zy +ĠJ ane +Ġд ом +Ġscrew s +Ġhy brid +Ġcas ual +Ġsel bst +be ing +ĠÄ IJ +ĠCol umb +ĠÑħ оÑĩ +Ġbu cket +Ġevalu ate +Ġid ol +Ġrep utation +ĠìĨ Įë +ÙĪ Ø± +Ġhe cho +Ġpo em +Ġsubject s +pl ant +ĠBe h +ĠSpe aking +Ġbatter ies +Ġfollow ers +ö l +Ġg ently +Ġsi xt +Ġparam eter +Ġik ke +ĠT our +ĠD J +ot te +ĠJ ahren +Ġprepar ation +Ġд Ñĥм +Ġ8 00 +c op +ik ing +Ġë¬ ¸ +Ġн Ñĥ +Ġл еÑĤ +åIJ Į +ĠI de +Ġì¡° ê¸Ī +Ġla ughter +Ġmole cules +ĠR est +Ġobs erved +d zie +Ġadvert ising +ert o +Ġmo ins +ĠM IT +Ġexc it +Ġt um +Ġty l +Ġinvest ed +Ġph arm +Ġunex pected +Ġph i +oty pe +we ise +Ġge ç +jour d +Ġhors es +n Äħ += " +ĠS M +Ġf ib +Ġcl ips +çķ ¶ +å¦Ĥ æŀľ +Ġreg ime +Ġrot ate +r ou +n ik +Ġarm or +ðŁ ĺ +еÑĢ Ð° +åº ¦ +ĠO ch +Ġr ichtig +üz el +ane ously +m ek +éĮ ¯ +ĠX iao +Ġexist ed +w orth +ãģ£ ãģ¨ +Ġna ught +Ġhe iÃŁt +ĠB al +Ġres id +iv ot +om atic +Ġh ired +Ġgrad ually +Ġon ions +Ġcomp at +Ġint im +Ġj ew +Ġcontrib ution +ĠI re +ac ji +Ġsl ice +Ġimm un +ĠR us +Ġgr ows +ĠSimilar ly +Ġhard est +Ġst ruck +Ġmeasure ment +... ] +th ey +Ġìł Ģë +Ġsne ak +Ġappl ies +Ġн ем +æ ĵ +×ij ר +ĠЧ ÑĤо +Ġout ro +Ġinnoc ent +Ġm og +ĠSams ung +Ġmer cy +Ġhand ling +Ġinter vention +id ays +g ot +Ġcur ric +Ġbound aries +Ġconf using +Ŀ¼ ëĬĶ +æ ĩ +Ġstitch es +ÃŃ vel +Ġtun nel +it ä +Ġg ost +im y +Ġcz as +Ġm é +Ġcat al +ĠSim on +ĠLI AM +m ic +ĠÐ ¤ +Ġey el +is as +ĠC PU +ĠD ou +Ġnä ch +Ġinfin ity +Ġr if +ĠPe ace +ĠC u +Ġminim al +Ġlisten ed +Ġpo le +hal b +Ġload ed +Ġste ady +ĠBes ides +ê m +Ġl ap +Ġco op +Ġfriends hip +w orld +Ġge h +Ġtyl ko +ĠLa ura +Ġsurround ed +ĠE vent +Ġch ap +ĠW onder +bre ak +Ġdro ve +Ġbroad er +Ġch i +F i +Ġge hen +Ġwest ern +Ġintellig ent +Ġpers ist +Ġfound ed +ãģĵ ãģ¨ +Ġhistor ic +Ġfr Ã¥ +cks Ã¥ +Ġhand y +Ġsy mp +Ġr ows +Ġnut ri +b ur +ĠLe on +Ġsist ema +Ġext ensive +ĠÑĥ в +í ı +Ġnight s +Ġcá c +Ġcount ing +ĠM ust +all ow +еÑģ Ñģ +M om +Ġнад о +Ġbar rel +ãĥ ŀ +AR D +Ġinstall ation +Ġin sect +Ġëħ ¸ë +uj Äħ +ĠÄij i +Ġpack ed +Ġf iction +N ow +ĠY ay +Ġper t +r ons +und e +ach es +Ġsty les +Ġapr ès +ok u +ĠV ice +ın ız +com m +Ġassign ed +Ġinteract ions +Ġac ab +F ELIPE +Ġresc ue +Ġindust ries +ĠAnd y +Ġpra ise +Ġfl ame +Ġsn ack +í Ĥ +ç ģ +Ġsw o +rend er +Ġbo ards +ĠÑĤ ом +en ne +Ġpast a +Ġdev il +ĠF el +Ġhat te +Ġcoll eg +e h +ì » +ãģĵ ãģ® +Ġproduct ive +for ward +и п +Ġsmart phone +Ġinv is +Ġb um +Ġwho a +ìŀ Ħ +Ġo cksÃ¥ +ĠL ang +ĠSy ria +Ġses i +ί α +Ġappro val +4 8 +Ġод ин +Ġë ĸ +ĠH arr +ĠAd minist +Ġ× ¤ +ĠDe an +f i +Ġcitiz en +Ġsh ark +0 5 +Ġbo il +Ġindic ate +å ¡ +A re +Ġlay out +Ġref r +ĠPac ific +AA AA +ĠAustral ian +g ression +V oice +ал ÑģÑı +Ġshel ter +T o +au pt +Ġevalu ation +ap or +Ġcur rency +Ġм ного +ig os +ãģ ° +Ġo ct +Ġro yal +è ³ +as il +ĠChild ren +Ġr ien +Ġë ĵľë +Ġbar rier +Ġej emplo +Ġe k +N D +es p +ен а +Ġp ic +Ġkill er +Ġintegr ate +Ġfew er +Ġdis abilities +Ġ .... +Ġtri angle +Ġfe es +Ġwid ely +em i +Ġoverwhel ming +Ġz omb +Ġb ere +Ġho od +ĠA ye +ĠHar vard +e v +ĠÏĦ οÏħ +Ġcup s +ĠA uch +z ona +Ġ199 0 +Ġwe iÃŁ +Ġcr unch +æ ¥ +Ġз ав +Ġmeas uring +Ġst ations +ĠStep hen +Ġshort ly +Ġsig ning +Ġcom edy +om o +Ġsuggest ions +Ġsign ature +ĠпÑĢ Ð¸Ð² +Ġdis order +as ka +Ġworld s +Ġprecis ely +n orm +ra v +ĠC ivil +In ter +ĠC ertain +Ġinj ured +Ġsuggest s +ĠGold en +Ġcy ber +ĠØ ´ +Ġtempor ary +Ġco oper +Ġvot ed +Ġ ought +ấ y +x ual +Ġpan els +Ġ9 5 +Ġhands ome +ĠпÑĢ Ð¾Ð² +Ġper mit +Ġke in +Ġbad ly +Ġnot ifications +iz a +ĠNot ice +Ġinc lusive +Ġanswer ing +Ġí Ĺ +u ld +íħ Į +Ġnow adays +Ġ3 7 +Ġb olt +Ġstat ic +ĠH op +Ġav ant +aj o +Ġë§Ľ ìŀĪ +Ġfif ty +ĠF inal +Ġsc ores +ĠT ap +Ġcy l +Ġconv ince +Ġany ways +od a +Ġìķ ¼ +Ġser ves +ĠÑĤак ой +ĠZo om +Ġsaving s +ul o +Ġs outhern +view er +Ġho je +Ġse ja +Ġrepresent ing +Īë įĺ +l ik +ĠSome body +Ġbe ast +Ġstick ing +Ġins ist +Ġtal ented +Ġexplain ing +Ġatt orney +éĥ ¨ +Ġst airs +ĠD og +í ĭ +Ġc ig +Ġshap ed +Ġs ons +Ïģ ι +ut t +Ġì Ķ +Ġpar ad +ìĿ¸ë į° +Ġh orn +ĠJ our +ann o +Ġworld wide +åĬ Ľ +Ġparticip ation +¦ Ħ +Ġm ów +Ġburn ed +Ġwrit ers +all ah +ĠF und +Ġcle ver +ĠLe ute +b in +Ġbe ating +f oot +ĠìĽ IJ +ĠStud io +Ġv ag +be y +r ze +Ġoppos ition +Ġж из +w ho +Ġê± ´ +Ġtr ace +Ġд енÑĮ +Ġep id +Ġges ch +ĠN ar +ĠB E +Ñĥ й +ĠS ign +ed ly +Ġcl ay +Ġinst antly +Ġgather ing +ĠGal axy +Ġb ored +ĠBudd h +c é +Ġm am +Ġsl ope +Ġëĭ¤ ìĿĮ +Ġsch ön +Ġp ir +ge f +am er +Ġh ö +Ġcolle ague +Ġpres ents +ad ium +Ġà® µ +Ġfal ar +be ep +Ġdri ed +ism s +Ġro pe +Ġworks hop +Ġest ud +Ġb ands +Ġthem es +åħ ¬ +ÙĬ ر +åIJ İ +Ġremind er +ÑĤ Ñĥ +ĠB h +Ġcocon ut +ĠÑģ ÑĤо +ĠCh annel +Ġimmig ration +ä s +.. ... +ä¸ » +çĻ ½ +st op +Ġк аÑĢ +Ġco ins +ĠÑĩ аÑģ +Ġdest ruction +l ined +Ġbar riers +ant ine +Ġprint ed +Ġcongrat ulations +ĠHe art +Ġin qu +th a +Ġhard ly +ĠA ven +Ġt inha +ĠS ony +ĠN F +Ġgradu ates +Ġsque eze +ere my +ÏĦ ι +Ġep ic +ĠJ u +Ġol m +ĠLa ughter +Ġbelief s +ĠC ru +ĠTr ue +ĠS oul +owe en +Ġrom antic +Ġз в +Ġan os +ĠY up +éĺ ¿ +d im +Ġin fer +Ġз ам +Ġso c +uk a +Ġprec ise +Ġdro pping +Ġcl ue +Ġer rors +char ge +ĠP u +omet er +Ġlamb da +ac ional +ĠD ong +Ġcham ber +Ġthank ful +ĠN u +ĠHaw ai +Ġinf o +Ġactiv ate +ĠQ ual +Ġqu ed +Ñĥ лÑĮ +Ġcl oth +åĸ ľ +Ġw ichtig +5 5 +Ġot ra +ograp her +Ġcur ios +Ġ19 80 +Ġemp res +d ess +e ur +Ġcl uster +ar ter +ob ile +ĠY an +ĠAd v +Ġdiscipl ine +Ġìłķ ëıĦ +ĠPl ace +ĠSe lect +T E +ĠбÑĭ ла +Ġwh is +Ġb ay +ĠD or +en cing +Ġrep et +Ġf icar +p ad +Ġf og +u yor +Ġsn ap +ib t +Ġso bie +Ġappoint ment +ĠR y +Ġce iling +our se +Ġwr ites +ĠAfghan istan +Ġm os +az e +Ġpen al +Ġcry stal +IC E +ê° IJ +é Ł +ĠTes la +Ġthe ories +Ġappe al +Ġnewsp aper +Ġcook ies +æ © +ĠاÙĦ ÙĦ +Ġma j +ĠGet ting +k ommen +ĠHe aven +ell s +Ġdiv ine +Ä « +Ġa kt +Ġhop es +ĠCh en +we gen +** * +ĠFra ge +Ġн и +ภ¹ +min ister +nes ota +wh ich +Ġexpl icit +Ġverd ad +Ġgradu ated +ĠPh ilipp +Q L +ĠM I +Ġdev ot +Ġc ure +Ġclos est +Ġà Ħ +Ġsex y +ãģ Ľ +ĠDe ath +ok o +ug u +ĠAn ne +itar ian +es a +ег од +ĠD ur +Ġ 000 +ze it +Ġtour nament +Ġmel hor +ภª +Ġin du +Ġf law +Ġw ars +ĠM ind +ĠI ron +ÑĤ ак +ĠV R +Ġs iz +ĠS outhern +Ġê·¸ëŁ ¬ë +Ġaw ak +Ġìķ ŀ +Ġc ube +believ able +if all +d is +Ġabandon ed +m ind +Ġpar l +Ġclass ical +è ĭ +á»Ļ t +ĠAut o +ĠB or +ç © +4 00 +ĠSoci ety +Ġsubt le +Ġmiss ions +Ġremember ed +ĠE ither +Ġda für +OR D +Ġint ensity +ES IN +ĠC up +Ġrare ly +Ġto ys +ĠChar lie +á» Ł +Ġgla ube +Ġround s +T IN +Ġcap ability +Ġderiv ative +Ġrefer ring +Ġd Ã¥ +ĠT ALI +Ġcott on +Ġcon fer +Ġcolum ns +Ġliber al +Ġnun ca +Ġμ ε +Ġind o +ib en +ĠBe ispiel +Ġê·¸ë łĩ +ĠÑĥ Ñĩ +Ġh oy +Ġfr y +ĠScott ish +è Ĭ +Ġc iv +Ġconserv ative +Ġair pl +Ġs ar +r us +Ġinvest ments +Ġinfin ite +Ġà® ķ +ĠTALI ESIN +ĠG ary +ue ll +Ġа к +ĠC ir +Ġrit ual +Ġ>> > +Ġtem pt +ĠTe ch +ĠPoke mon +Ġimprove ments +Ġsp are +Ġtransl ate +Ġson ra +ĠFil m +w ort +Ġм и +Ġperiod s +Ġje alous +ãģĦ ãģĦ +Ġt ir +M I +Ġconduct ed +ĠìķĪë ħķ +0 9 +ĠPol it +ĠWhere as +Ġmoist ure +Ġs ins +Ġk ap +ĠÑį к +Ġben im +Ġelimin ate +Ġathlet es +ĠMan ager +Ġfeature d +ap ore +äº Ľ +Ġë° ľ +Ġper f +ĠTh us +Ġdeb ut +об ÑĢ +Ġse ñ +Ġmyster ious +w ords +Ķ ê°Ģ +Ġcheck s +Ġvolunte er +Ġwas hing +ĠMar vel +ĠA B +iss ors +! ' +ĠF ull +ye on +Ġwe igh +ĠJO HN +Ġv os +Ġproced ures +Ġaddress ed +ĠBer lin +put er +ĠB an +Ġmedic ation +Ġdr one +ĠÑĥ б +ĠJe an +Ġcap s +Ġdisappoint ed +Ġw ore +Ġêµ Ń +Ġorgan ize +ĠHall oween +Ġfant asy +y ard +Ġnos otros +Ġjump ed +Ġphot ography +ĠN ame +re c +A B +Ġbless ing +ĠSh ut +Ġbit ter +p op +ãģĿ ãĤĮ +Ġde i +Ġfulf ill +çIJ Ĩ +Ġden gan +Ġbe lo +ĠMean while +Ġdep ois +Ġdi abetes +Ġbu nd +ĠZe aland +Ġdig est +Ġt ires +Ġdo d +ag ne +ế t +Ġpe el +Ġз аб +Ġn odes +Ġtrend s +ĠSw itch +ĠA ward +ĠOr ig +ĠH al +Ġest as +Ġ3 60 +Ġsim ult +Ġcom ic +Ġm Ãł +Ġbal anced +ĠPrin cess +Ġkilomet ers +á» © +Ġpart ir +ì¤ ij +so ft +ĠV iew +Ġbi ological +in st +4 4 +Ġman era +Ġcompreh ensive +ĠS ab +Ġcr imes +y ers +ĠComp any +ĠPh ot +Ġpou co +i ac +Ġbe im +in ate +Ġsub sequ +ĠMay or +Ġcent uries +è res +ìŀĸ ìķĦìļĶ +Ġê·¸ëŁ ¼ +ĠFra u +ĠO H +Ġëģ Ŀ +ĠN ah +ĠSer ies +Ġover night +íĴ Ī +ĠâĢ ¢ +Ġtra ve +atter ed +Ġwar ri +ĠGru nd +ĠInd ones +Ġsc ra +ob y +ĠBro ok +Ġcur s +Ġë ¸ +Ġexpl ains +ram atic +Ġparticip ating +Ġmin ut +Ġcontract s +Ġg egen +Ġdisappe ared +ĠS N +Ġrob ust +ap h +Ġsh rim +Ġdev ast +c ope +Ġme ets +Ġpeace ful +m ate +Ġwe ld +Ġ× ª +d on +Ñĥ ÑĤÑĮ +Ġregister ed +ĠN ik +j in +Ġc av +Ġe cht +io x +Ġflow ing +но ÑģÑĤи +Ġto e +Ġent ity +ов а +f its +ĠPat rick +ÑĤ ÑĢ +Ġle verage +Ġcor rel +i ah +Ġstr ings +ist inct +Ġg ue +arch y +Ġteng o +ım ız +Ġor bit +ä¸ º +Ġе ÑīÑij +ca ke +Ġ׾ ×Ķ +ĠMin nesota +Ġbra ke +ow ie +Ġcra w +ê¸°ë ¥¼ +Ġprogram me +ĠÑģл ÑĥÑĩ +åı ª +ien ces +ĠO ui +ĠP ers +im iento +ĠIn vest +Ġsl ower +æĻĤ åĢĻ +ĠB eth +Ġnur se +ĠSpr ing +S p +Ġun employ +д и +Ġgen ius +ĠA aron +Ġê·¸ëŁ ¬ +Ġe i +ãģĹ ãĤĩ +Ġtank s +Ġau jourd +Ġcomplex ity +ĠÑĢ ÐµÑĪ +Ġold est +Ġlet z +åħ ¥ +Ġphenomen on +pr int +ĠBund es +it at +ê» ĺ +Ġ4 2 +ĠW i +Ġinc om +Ġg ek +Ġembr ace +Ġt ies +out e +Ġd ose +ĠF riends +Ñĭ ÑĤ +егод нÑı +Ġor g +Ħë ¡ľ +ó g +Ġex ceed +Ġgod s +Ġê±° ìĺĪìļĶ +Ġsoci et +ĠUn ivers +it ät +Ġword en +Ġsm oking +Ġint ens +ab ul +em ia +è ij +4 7 +f ly +Ġ200 6 +ĠSer iously +Ġprze z +æ ¼ +c re +Ġn an +Ġmod es +ов аÑĤÑĮ +ĠH ang +em en +Ġbenefic ial +Ġvot ers +ĠBro ad +Ġb ent +W ow +Ġm ul +åĵ ¥ +ĠU C +Ġdam aged +ĠUk raine +Ġw ipe +Ġst ones +Ġman agers +Ġr ab +ÑģÑĤÑĢ Ð¾ +l at +Ġde ce +Ġgraph ic +Ġf oss +Ġdisag ree +ĠAm en +Ġsec rets +ho le +ink le +Ġfortun ate +Ġì ± +ìľ Ħ +èIJ ¬ +Ġhab its +Ġbur ied +Ġh in +Ġvirt ually +ol as +ĠR P +ĠT ab +l ow +Ġsacr ific +Ġestim ated +ol n +Ù ĭ +c ur +ĠFe el +Ġcast le +Ġus eless +Ġdis g +ĠJac ob +Ġga an +Ġup side +Ġpare ce +ãĥ³ ãĥ +Ġsh ipping +ĠC R +Ġdis rupt +ac ter +UN D +f u +å® Į +ĠP ick +ĠChar l +ĠB ull +Ġenter prise +Ġpunish ment +ack ing +Ġfr action +Ġtab let +Ġch ord +Ġsimilar ly +åħ¶ 實 +ĠTor onto +Ġcour ts +ÄŁ l +esz cze +Ġpron oun +ĠS ister +ĠM P +Ġgreat ly +ĠD ank +ic op +Ġgar bage +Ġresol ve +ĠS af +ĠG un +Ġcomp ound +Ġë° ° +ĠMus ik +âĻ « +Ġcha os +ĠWhen ever +Ġe uros +Ġor chest +Ġrefr iger +al an +ภ· +ĠAm azing +Ġp ud +ag an +Ġj eszcze +is y +Ġaccur acy +ĠA ma +is ode +ë ĮĢ +Ġinterpret ation +ĠL iber +æ · +c am +Ġevol ved +ĠK ay +ÑĨ Ñĭ +Ġcreat or +it as +Ġal arm +Ġcelebr ation +z ent +Ġfun cion +Ġo v +umb ling +Ġ % +à¸ Ī +Ġrestrict ions +Ġн ав +ĠK inder +Ġban ana +ÑĮ Ñı +Ġdiam eter +Ġnor thern +ur ers +ĠP as +æĪij çļĦ +Ġwork force +Ġj ung +Ġguar ante +Ġequ ilib +Ġsu ite +Ġeu ro +Ġdel iber +S te +Ġdownt own +Ġch in +Ġc odes +ed ia +Ġshe ep +res hold +wn ie +ó b +Ġunder lying +l ia +j er +ÏĢ ÏĮ +ç Ŀ +th rop +Ġz ap +Ġvac uum +ĠH ab +Ġwra pped +ì ¢ +Ġinvent ory +м а +Ġco ord +Ġpl ates +Ġsy mm +T e +ĠwÅĤa ÅĽnie +Ġreach es +Ġlon ely +S cript +le e +ess er +Ġê± ¸ +ĠGes ch +ĠMo ving +Ġré p +ĠV ill +åIJ Ī +ĠR achel +Ġtem os +ON E +Ġstra in +Ġang el +Ġf Ã¥ +T r +Ġach o +Ġhighlight s +ĠW er +ĠCar l +Ġbl ur +Ġreg ards + · +ил ÑģÑı +Ġrec re +ĠY ani +U CK +ł ¸ +Ġelectr ons +ĠSp iel +Ġv ed +Ú ¾ +Ġbe am +Ġid iot +ë ĵ¤ +на Ñĩ +id d +Ġsk i +it ative +Ġhyp othes +ãģ§ãģĻ ãģŃ +ent er +ĠìķĦëĭĪ ë +Ġih re +Ġpre view +ang el +Ġdem on +Ġd us +Ġd ic +ĠK om +LE Y +... ! +Ġsie ht +ĠSon ic +Ġten ho +an as +Ġdig it +ĠMa ar +Ġunder grad +oun cer +uff y +Ġconvers ion +Ġdis connect +Ġe cho +om er +Ġcurric ulum +Ġper ché +Ġw and +.. ? +Ġroll ed +Ġentreprene ur +Ġtheore t +ĠÑī о +Ġins ights +Ġzus ammen +o in +ret t +p rodu +Ġvisit ors +e ous +Ġgrand mother +Ġhum or +Ġн иÑħ +zen ia +ins on +Ġres et +Ġbase ball +Ġmatch ing +ëĭ¤ ê°Ģ +Ġpun to +ì ¡ +Ġre de +Ġaddress ing +Ġfore cast +ĠB ol +Ġcol ored +Ġdocument ation +Ġexpect ation +ĠNor thern +Ġcre o +Ġà® ļ +f on +Ġuns ere +U M +Ġcop ies +Ġexpand ed +Ġveter ans +ĠAl m +Ġво обÑīе +Ġpsych ological +Ġnos so +Ġpay ments +im eters +Ġ-- > +ĠJenn ifer +Ġvolunte ers +os se +or ious +ĠбÑĭ ли +è Ĥ +ĠEs s +w s +ĠB C +ĠI C +W oman +Ġv ont +Ġeth nic +EN N +им о +Ġlo b +Ġou i +c s +Ġre he +Ġìł ģ +Ġch ick +ús ica +Ġk ont +ĠDist rict +Ġp ile +Ġа в +ей ÑģÑĤв +Ġ £ +Ġiss ued +Ġком п +Ġpros per +Ġprof ound +ĠDe ar +Ġãģ ĵ +Ġfund ed +Ġb isa +ŀ ĺë +× Ł +ĠìĿ ĺ +Ġtw elve +ĠChamp ions +éĿŀ 常 +Ñģ л +Ġ200 5 +p m +Ġon de +Ġdiff é +ĠCh all +Ġdifficult ies +Ġgar age +Ġd á +ün k +Ġë¬ ¼ +Ġtr an +Ġsubm itted +z w +ÙĪ Ø§ +Ġar k +ĠìĦ ± +Ġgrocer y +он а +i ere +Ġa est +Ġexhib ition +Ġr és +Ġconsist ency +Ġcook ie +н ей +Ġrepl acement +æ² ¹ +ĠS em +ĠìĤ¬ ìļ© +8 00 +Ġgen es +Ġtrans action +ĠE L +Ġdur ante +ib les +ĠE at +t ail +iss ance +Ġto ss +Ġsurv ived +Ġoff ices +Ġsupport ive +Wh ere +Ġtout es +Ġë§ ī +Ġj okes +ier on +ap ers +Ġm ature +ĠM arsh +Ġs ido +k ind +Ġreal mente +ĠChe f +Ġquel que +Ġjud ges +e ft +ER S +Ġj et +Ġpers ons +è » +iz ations +ri k +Ġsh ops +ĠW y +Ġele g +qu è +qu oi +Ġjug a +Ġíķľë ²Ī +ĠQuest ion +ĠGlo bal +Ġìķ½ ê°Ħ +ĠSt ation +æİ ¥ +ĠOh io +Ġstick y +Ġst ressed +Ġg ün +Ġí Ŀ +ÑģÑĤ Ñĥп +é ¡Į +ĠPh D +im mer +Ġment or +Ġinv ented +Ġre un +Ġine vit +Ġpol ÃŃt +Ġexec ute +ĠSt ory +Ġout standing +Ġgu er +ĠR ain +Ġch oses +ĠT it +ĠÑģ еÑĢ +ĠSing apore +ĠN one +Ġch ronic +°ë į° +Ġe go +æł · +ES T +ãģĤ ãĤĬ +ĠW ang +ĠN AT +Ġa ug +Ġdes ktop +Ġetern al +ĠìĤ¬ ìĭ¤ +ĠConst itution +ìĤ ¬ë +×Ļ× ľ +p res +ĠТ Ñĭ +Ġinter f +Ġlist s +Ġfight s +ft en +ĠI owa +Ġmotiv ated +ĠH osp +Ġelse where +Ġpath s +Ġinst ances +B l +r ange +á» ± +ĠS it +man a +Ġìĭľ ìŀij +Ġm ình +ans as +Ġs na +Ġphilos oph +Ġpas se +ưỠĿi +ak h +ent al +Ġih n +ru ctor +Ġв аÑĪ +Ġgener ous +Ġp ivot +п ол +Ġjam ais +Ġcom ent +ĠL ew +od zi +ĠX box +Ġв од +Ġcons ent +ī ìŀ¥ +Ġdis par +l ass +ĠGovern or +Be ifall +Ġê° ľ +Ġbelo ved +׳ ×ķ +se ll +Ġhon ored +le h +Ġw äre +un ting +Ġfra ud +ĠR AM +ê± ¸ +Ġkill s +Ġeconom ics +0 4 +п еÑĢ +Ġco isas +Ġи гÑĢ +ÃŃ m +Ġmö chte +Ġìµ ľ +Ġstim ul +Ġfast est +l v +Ġg én +ĠS ounds +Ġ19 70 +Ġhome work +spe aking +Ġencour aging +Ġqu ery +Ġre vers +pro fit +Ġd y +Ġìŀ ij +ëĬĶëį° ìļĶ +Ġso ap +ĠG all +ĠC N +ĠAn s +Ġf ic +ank s +Ġdess ert +ĠìłĢ íĿ¬ +ĠM aking +Ġcome ç +ê³ Ħ +Ġassoci ation +D ad +he e +Ġh ogy +Ġap ro +Ġinvis ible +Americ an +í İ +Ġvi be +Ġem issions +Ġadvoc ate +Ġkick ed +Ġ vel +Ġsum mar +Ġfre aking +ch ron +Ġpin ch +Ġwszyst k +isc al +Ġpro ved +Ġmind ful +Ġt ä +Ġno ises +Ġisol ated +Ġcross ed +Ġê° ķ +Ġvo ilÃł +Ġch ore +ĠR A +C om +Ġrelax ed +at ro +Ġpre vention +Voice over +O D +ĠCo vid +Ġsepar ation +Ġ- [ +иÑĩ его +çĻ ¼ +ĠS D +ble ep +Ġindepend ence +Ġpart ial +Ġalgorith ms +ĠAny one +Ġassoci ate +h um +ic ular +Ġb ạn +Ġbatt les +G ood +App lause +Ġbast ante +Ġadv ant +ĠS weet +Ġref used +ãĤ ¸ +ĠÑĤеб е +pl et +Ġencour aged +åĵ ¦ +Ġmir acle +ĠB un +ĠV ar +rim ination +e lect +ĠM ult +Ġdeliver ing +e ing +Ġc m +ne hmen +ĠL ine +Ġë§ Į +en ced +ĠS ound +ĠCont in +ij d +UN G +k le +Ġth reshold +Ġcomp act +ad t +Ġto es +ĠP ur +own ed +ment ed +Ġdes igning +Ġvacc inated +Ġexha ust +Ġbas ics +Ġcons ists +ĠGu y +ac zy +Ġm ÃŃ +w on +å® ³ +Ġ8 5 +æ Ĥ +Ġm um +Ġign or +Ġprint ing +ac ular +p ow +Ġexpand ing +Ġg ir +ĠC ab +íĺ ¸ +ÑĤÑĮ ÑģÑı +ĠìĹ¬ëŁ¬ë ¶Ħ +Ġang les +Ġterm inal +ĠW on +ĠInter esting +Ġcross ing +Ġbond s +Ġpu eden +Ġor b +lar ın +Ġcreep y +Ġnutr ition +Ġall ies +Ġwire less +Ġdes ired +Ġcomp ute +ĠAri zona +ĠBeaut iful +Ġprodu ces +Ġnuest ro +t ed +Ġel igible +ĠÑģ оз +ic ial +ĠH ero +Ġcons ume +Ġrob ots +Ġpurch ased +c ción +Ġ iz +ượ c +ίν αι +ĠØ£ ÙĨ +Ġshad ows +ĠMed ia +Ġprin cess +Ġk lar +Ġwood en +Ġus ar +Ġg üzel +Ġsl ot +r ade +Ġë Ĵ +Ġhar mon +Ġingred ient +ors hip +ek i +Ġgrand father +Ġexcit ement +Ġpolit icians +.. ! +Ġout s +Ġsepar ately +ĠÑı к +ĠW elt +ĠP ow +j an +Ġorient ation +åı ĭ +L C +age m +ÛĮ Úº +åIJ Ĺ +Ġbran ches +ad en +rent e +ĠI hr +as m +Ġest ão +ĠN ic +Ġsla ve +Ġcomp ress +c rowd +Ġclim bing +ĠMan agement +ĠB ah +Ġpan ic +Ġk or +Ġcool ing +Ġb ind +Ġз ад +Ġr ack +Ġent it +Ġs ends +Ġyour selves +d es +ĠMuslim s +Ġí ļ +ism a +cy cle +un kt +ĠC ore +Ġinj uries +Ġident ical +ка Ñı +ĠDeutsch land +Ġе е +is an +Ġtr uc +let on +Ġback up +Ġult ra +Ġab und +ille urs +Ġby ÅĤo +åħ ĥ +ort ed +Ġearth qu +Ġк л +Ġobs ervation +Ġmainten ant +el en +Ġsett led +Ġp ela +ĠE conom +Ġ Õ +Ġste ering +ĠAL L +ĠC her +Ġpat ience +ĠS now +Ġb or +Ġworth y +Ġcá i +Ġ× § +Ġκ α +d og +ĠK aren +ill es +Î ² +Ġagric ulture +×ķ× Ł +ĠSe an +Ġsens ors +íķ ´ë +ag h +Ġpublic ly +Ġpe ux +ĠAlex ander +Ġprior it +Ġla zy +ard on +atter ing +Ġcost ume +س ت +è¿ ĺ +Ġun w +Ð Ľ +Ġthick ness +qu ito +g unt +ist as +ne ys +ĠëIJĺ ê²Į +ĠBr asil +Ġto ken +Ġaff ili +l on +Ġf Ã¥r +ĠBe ach +Ġw itch +ĠSe ven +Ġp ant +λ λ +Ġcapt ain +å Ŀ +Ġve ut +Ġpou voir +ac z +ĠBar b +Ġut ility +Ġcontempor ary +Ġobt ained +Ġpainting s +e ar +Ġpe an +ĠO g +Ġc ust +л ем +Ĥ ĺë +ĠIs so +Ġac onte +ĠTe le +ĠAss istant +à ī +íĸĪ ìĬµëĭĪëĭ¤ +Ġcount s +Ġbu ck +ĠDe ep +Ġtack le +Ġh arsh +Ġdec ides +éĹ ľ +. âĢĭ +éĤ Ĭ +ĠAng el +Ġlay ing +Ġcal ories +Ġcontro lling +Ġadvant ages +ĠÑįÑĤ ой +Ġappro aching +Ġthreat s +ak an +em atic +m ann +ê³ µ +m umbles +ac ió +Ġmaint aining +Ġfound er +l ah +f ight +Ġadm itted +â̦ . +ķ Į +ab ol +Ġus age +Ġn onsense +ĠPal est +Ġcont re +ĠDemocr atic +ĠE R +j ekt +Ġar bit +Ġг ол +ĠMich elle +ich er +es h +ĠP ho +к ом +4 9 +ĠEner gy +ο Ïį +Ġc ents +Ġref ers +Ġg ospel +ĠSh a +ĠSh are +×Ļ× ł +Ġclin ic +ĠëĦ £ +Ġequ ality +ug s +Ġsh ed +Ġplan es +Ġtout e +re ck +Ġstra nd +Ġbi ology +Ġle ague +ĠP ok +Ġnúmer o +ĠCo ast +Ġconsist ently +Ġnuc le +OO OO +Ġob jet +Ġch or +Ġg inger +Ġd abei +Ġcoop eration +à¯į . +nt en +ç ¤ +l Ãł +ìĸ ij +r ado +Ġpass ive +Ġglo ves +Ġunder ground +Ġlog ical +Ġk et +Ġfunction ality +¸ë ¦¬ +Ġport al +ell er +×Ļ× ¨ +ĠT ed +ĠG re +IJ ľ +Ġperson nel +Ġemer ging +ĠF ür +Ġmeant ime +usal em +ĠC lear +Ġtra pped +Ġìļ ° +Ġdis pl +Ġmet tre +Ġmun icip +Ġwithd raw +Ġsp at +un es +Ġaccess ibility +æĪij 们 +Ġap are +Ġpros pect +Ġн аз +Ġcop per +ĠP RO +Ïħ ÏĦ +Ġattack ing +ĠV in +ĠSt one +Ġinvestig ate +st yle +ĠÎ » +ë ¡Ŀ +ë§ Ī +Ġins pect +Ġli ver +ал иÑģÑĮ +Ġser a +hal ten +em an +Ġmin istry +' ' +Ġd ots +ãħĭãħĭ ãħĭãħĭ +Ñĥ ÑģÑĤ +ĠJ ak +AK E +Ġg aps +uck er +ĠинÑĤеÑĢ ÐµÑģ +ĠEm ily +Ġinter val +Ġt ender +ĠTechn ology +g ame +Ġtri b +ÙĦ ا +ĠDevelop ment +Ùħ ا +Ġwr ist +Ġf ires +Ġtarget ed +ìł IJ +Ġso d +íļ Į +Ġoldu ÄŁ +Ġse asons +vent ions +Ġн его +Ġsomet ime +ли в +n é +Ġt ú +ĠDe us +Ġexec ution +á p +ĠCh ange +ĠInd eed +Ġreg ulation +ĠH ung +é is +Ġwish es +Ġj azz +Ġstruct ural +Ġblow ing +Ġby Äĩ +Ġtherm al +ph ant +ÑĢÑĥ з +ан ÑĤ +ĠP ull +Ġconf usion +нÑĭ ми +Ġscen arios +ìłģ ìľ¼ë¡ľ +Ġд еÑĤ +Ġtatto o +Ġaut re +Ġhe ating +Ġtreat ing +Ġпон им +Ġexc lus +ĠL OL +we ar +ag le +Ġzur ück +Ġr ational +s u +Ġdet er +ĠN ative +à®ķ ள +ach ed +Ġ ãĥ +ĠEnt onces +Ġhor a +ìĿ´ìĹIJ ìļĶ +Ġl ite +à « +Ġsix th +Ġбол ее +act or +Ġpsych ology +çĽ ¸ +Ġdem ands +Ġpe er +Ġnew ly +ĠWW E +Don ald +ĠBo x +Ġp ine +Ġload ing +ĠN ico +Ġs ÅĤ +omm e +AR T +Ġrecru it +Ġbug s +arent s +ĠпÑĢ Ð¾Ð± +ĠIn side +ipp er +d ramatic +Ġplan ets +ord e +Ġy oga +ch ild +ĠMar ie +Ġãģ Ĥ +ĠB L +Ġfil med +Ġref resh +Ġtomato es +Ġf et +Qu é +Ġ !! +ĠëĤ ´ë +r ine +Ġinteract ive +s al +ann ah +pe z +ç¶ ĵ +Ġunderstand s +ĠTok yo +Ġlibr aries +Ġread er +ij IJ +o z +ĠEnd e +ĠF lo +Ġm ild +Ġpo etry +Ġж ив +æĦ Ľ +Ġbeh ave +Ġdo en +ĠSus an +p age +ra ham +Ġcommunic ations +Ġtun ing +Ġp ac +Ġanx ious +I O +M ark +Ġhi ç +book s +Ġp iss +Ġen abled +achel or +ĠF OR +Ġé c +ĠT R +il st +h at +ĠìĿ Į +Ġty ch +Ġj ar +Ġbuild s +ĠAr gent +Ġinter medi +Ġl ou +Ġa ra +Ġassign ment +Ġcabin et +Ġretire ment +ãģ » +Ġdis abled +ric a +Ġa wards +Ġbo ots +Ġacknow led +Ġth y +Ġêµ ¬ +Ġsy nd +ни й +il ton +Ġprob l +ĠF al +Ġverd ade +Ġ7 00 +ĠLe arning +oc us +Ġpal ace +N ot +t ain +c m +Ġmagn et +inc oln +Ġfig uring +ĠL yn +ĠB oss +ĠV O +Ġdiagn osis +Ġequ ipped +w atch +in os +ad ers +Ġsh elf +Ġorgan is +Ġn od +Ġk ız +pp ers +Ġrest ore +Ġart ic +ĠVo ice +ı yorum +ê² © +Ġspread ing +Ġh ips +Ġw ard +ure au +Ġinter section +6 6 +Ġ3 9 +ç ³ +Ġwait ed +ì ´ +hh hh +Ġd ys +ĠE N +Ġb atch +Ġca f +Ġmark er +大家 好 +or able +ó ria +Ġste pped +Ġcelebr ating +ан а +Ġwor n +ĠF ol +Ġpl a +Ġattempt s +Ġtwe et +Ġr ust +g ence +í Ĩµ +Ġre vel +Ġre cept +en ess +Ġ( ( +ãĥ¼ ãĥ +! âĢĭ +ĠìĨ IJ +Ġinfluen ced +и ж +Ġкон еÑĩно +Ġcolleg es +ion i +Ġs ag +An n +ol ar +Ġexpress ions +Ġsu its +Ġowners hip +el and +pie ce +æĢİ ä¹Ī +Ġdesp ués +Ġt el +Ġins ult +Ġêµ īìŀ¥ +ĠSm all +ĠF R +ok a +ber ries +ĠAnt on +ел Ñı +Ñı Ñģ +Ġval ve +act s +Ġwood s +à® £ +Ġcult iv +Ġf á +ãģ¨ ãģĦãģĨ +Ġche ers +Ġassum ption +Ġfit ness +ÃŃ cul +Ġpod r +Ġwe it +ĠH ind +Ġd ign +Ġз н +Ġsqu ad +Ġdest ro +c ere +sh irt +imm t +eng ers +Ġs ä +k ÅĤad +Ġ ÈĻ +Ġocc as +Ġì¤ Ħ +Ġprocess or +ĠD M +ĠDad dy +Ġsoon er +Ġstraight forward +Ġdepart ments +ĠChr ome +Ġwork place +ĠPy thon +Ġm eng +ĠD AN +ĠI ce +ĠëĪ Ī +ĠG i +Ġh iring +Ġland ed +Ġdemocr atic +ied z +ãģĺ ãĤĥ +Ġse v +ic ia +Ġespe cial +ĠN ous +Ġh ät +Ġb ou +per t +ies z +åij Ģ +Ġv il +ÅĽ li +Ġî n +Ġloss es +éķ · +Ġto ast +Ġreal m +ĠAust in +ĠIn formation +Ġres ume +Ġch ase +Ġsal ary +Ġë¶ Ħ +ли Ñĩ +ĠÑģл ед +ĠFur ther +Ġcar ing +Ġv ig +Ġval or +è¿Ļ 个 +ĠÑĩ а +Ġanalyt ics +Ġglo be +ĠM AN +Ġn el +ìĿ´ì ķ¼ +Ł ¼ +Ġo y +íķĺ ìĦ¸ìļĶ +j en +Ġtrou bles +ah aha +Ġchurch es +u et +Ġmeasure ments +b il +ì ½ +if ully +ин Ñĥ +ĠWil son +¦ ´ +ĠíĮ Į +Ġì° ¨ +Ġp úblic +ĠJer usalem +Ġn ails +Ġsp ine +Ġhe mos +Ġz n +qu is +ĠLe ben +Ġrefer ences +IT H +i per +ĠÑģеб Ñı +ì ģ +ĠW a +st ate +§ Ŀ +åħ ± +ĠGen er +Ġact ress +ĠEn joy +๠ĥ +Ġ× Ĵ +Ġinfect ed +Ġsh aking +Ġn ick +ภ¸ +Ġf ot +Ġaccompl ished +u ke +Ġshe ets +Ġf ence +Ġnurs ing +Ġintrodu cing +Ġfe at +O ne +T O +Ġcl ubs +ĠBru ce +on ge +ch ange +ĠBat man +åı ° +ĠOffic er +Ġhyd ro +Ġsupp lement +Ġc ela +Ġlong est +Ġcompet ing +Ġcon he +g iving +Ġbra ins +Ġlo ans +Ġw age +ĠCl inton +Ġs Äĥ +ane ous +Ġl ord +ÑĢÑĥ ж +Ġqu iz +Ġst iff +ĠL GB +s z +M E +m are +th ere +Ġn är +ĠM and +l ast +Ġd ag +Ġhalf way +ĠB and +Ġëĭ¤ ìĭľ +ĠA ren +Ġi le +P N +ent o +Ġalg um +Ġsoc cer +Ġblock ed +ĠJon athan +Ġse w +ĠTest ament +Ġv ale +Ġbehav i +å§ ĭ +Ġcon na +IC H +Ġaud iences +m l +amm ad +ĠìĤ ´ì +I GH +Ġr aces +em ed +Ġm á»Ļt +à ¯ +Ġover s +Ġdecl ared +Ġs ana +ĠU na +ĠÑĢ Ðµ +uck s +Ġp airs +Ġan ge +N e +Ġup s +av y +ø r +ree k +Ġbehav iors +Ġreflect ed +Ġprior ities +Ġcon du +Ġret reat +Ġexp enses +Ġë´ IJ +Ġtri ple +Ġêµīìŀ¥ íŀĪ +ä lt +Ġind igenous +Ġmin ing +Ġaccept able +Ġru in +C A +u ine +Ġpip eline +ct ic +ê t +ĠвÑģ его +Ġb oun +ĠDig ital +ĠBo om +ÑĨ е +Ġл ÑĥÑĩ +Ġas c +ĮĢë ¡ľ +ĠGood bye +Ġrend er +ene z +ar re +ĠTH AT +b our +ic ión +ãĤ Ń +E very +Ġw ires +ĠPar liament +n ung +ate ur +ĠS ave +ĠPh ys +Ġam or +ĠE ve +Ġfr ight +Ġgam ma +Ġmic ros +m itt +ĠC ode +ĠBe y +pl ed +ĠиÑģп олÑĮз +ç Ĺ +ìĥ ī +å¥ ¹ +Ġmon et +ĠJah re +Ġlux ury +Ġde af +Ġbet ray +Ġê² ° +и ки +Ġdefe ated +Ġunder t +Ġwe g +Ġcool er +ãģķ ãĤĵ +iam i +éĤĦ æľī +ĠJess ica +ĠJ oy +Ġsoph istic +ени и +ðĿ ĺ +Ġch ili +ĠTy pe +Ġprote ins +Ġpresent ing +al ia +ìļ ¸ +ĠMaj or +Ġmolec ule +um er +Ġcoll apse +ĠAny ways +ĠMount ain +ant ed +ãĢ IJ +Ġвиде о +æ° ´ +A ud +Ġcon qu +Ġvo ll +Ġkn it +Ġmem br +ĠMark et +Ġd ari +Ġcalcul ated +г и +Ġshrim p +ĠM u +ĠпÑĢ Ð¾ÑĤ +Ġìĺģ ìĥģ +Ġproduct ivity +Ġcogn itive +ĠHe b +ict ions +ê² ½ +Ġcr é +f ör +Ġpray ing +ash i +ĠT ik +ó r +w en +ÑĮ Ñİ +ix o +Ġ( " +ĠÑĤ ел +Ġìĸ´ëĸ ¤ +ĠпеÑĢ ÐµÐ´ +ĠD rive +ãĢ ij +ĠE qu +Ġequilib rium +Ġdescri bes +не е +4 2 +ĠCur rent +y y +Ġabsor b +Ġsold ier +d ers +Ġtestim ony +Ġdec line +ľë ¡ľ +g age +Ġinsp ire +la pping +Ġspin ning +Ġsla very +Ġfac ial +Ġtrad itions +ári os +ĠHosp ital +Ġn est +ĠëĪ Ħ +Ġto i +Ġfe ars +ìħ ¨ +ĠM uh +Ġgradu ation +Ġimpact ed +Ġa unt +ĠLet s +Ġalumin um +Ġdomin ant +ĠDav is +ĠNav y +Ġcom pt +op les +Ġest ava +è ¥ +Ġsc al +Ġpres erve +ĠO pp +Ġpract ically +Ġmagn itude +Ġf itting +Ġcoordin ate +Ġfurn iture +ĠFam il +Ġexplos ion +Ġdocument ary +ĠS cript +Ġport ray +m at +Ġschedul ed +Ġdynam ics +ph y +ak y +ĠU I +C he +Ġcontinu ously +ĠPro v +å° ij +Ñĥ з +ra h +Ġger ne +pro of +Ġsecret ary +ĠPat reon +sc ream +ĠK ids +á»ĵ i +Ġk g +Ġuncertain ty +Ġк ажд +Ġmit ig +Ġread s +å· ² +ĠR u +Ġpri est +Ġн ед +Ġlimit ations +Ġflo at +6 00 +ĠT oy +ĠJim my +Ġoff ensive +en i +ĠX i +Ġeye br +ĠTur k +Ġaccident ally +Ġoh ne +ĠS aud +9 5 +ĠD utch +ан Ñģ +ĠSe attle +Ġëĵ ± +che ck +k ÄĻ +Ġcontrib utions +Ġbes ide +Ġqu indi +Ġfle w +æĹ ¶ +ذ ا +ĠL O +Ġwa ist +ĠE V +Ġhol idays +j on +Ġmis under +Ñı н +Ġb out +Ġd imin +Ạ½ +ó l +ĠGr ace +Ġinput s +Ġden y +Ġform ing +ĠB ild +Ġad equ +Ġfol k +Ġreject ed +se mb +Ġfrust rated +op en +ĠBet ter +il on +Ġtow el +Ġdifferent ial +Ġsac red +Ġsa il +éĩ Į +ent imes +Ġgentle man +Ġicon ic +Ġcomp aring +Ġs agt +Ġtext s +Ġgrand ma +Ġroll s +Ġcont ents +ä¸į 好 +оÑģ Ñģ +Ġsusp ension +ro it +¦ ¼ +Ġasse z +Ġd ort +ĠM ath +ĠVict or +ĠJava Script +ä¸į å°į +Ġen han +Å Ļ +ĠB ush +Ġpromot ion +Ġk in +Ġmon sters +ĠColor ado +ĠÎ ² +íķ´ì ļĶ +æŃ £ +iffer ent +Ġn aked +Ġpro d +et ics +ĠW oman +Ġtreat ments +Ġest oy +v é +Ġlif ting +Ġy apt +ĠRo ber +Ġì¹ ľ +Ġsubst itute +ak u +r idge +Ġê± °ë +Ġrespond ed +Ġb é +ĠEngine er +Ġtransfer red +ë ² +Ġha ber +o op +ĠW E +Ġv est +Ġfor ty +ĠD S +Ġ200 4 +Ġco aching +n om +ĠB ab +Ġn ossa +ĠJ ake +Ġg y +Ġde leg +Ġìŀ ł +ĠкÑĢ Ð°Ñģ +Ġstand point +Ġdis ad +Ġart work +A d +ill o +ĠÄij ược +ĠPr om +ĠL ib +Ġcritic ism +Ġcontact s +ÑĢ Ð°Ð¼ +Ġachieve ment +ÐĶ Ð° +Ġdiss ol +ĠVeg as +Ġstream s +ĠK ent +ĠعÙĦ Ùī +Ġrad ius +Ġsu cks +ĠA ch +Ġf i +ou st +ĠлÑİд и +Ġpal ette +ĠH az +ĠAnth ony +Ġtem a +ĠC os +Ġsa fer +α ÏĤ +Ġcont rad +Ġma ior +Ġinfl ation +ĠSil ver +Ġatt ending +íķľ íħĮ +art o +Ġapplaud ing +Ġcomput ing +ĠH at +æ » +k now +mak ers +Ġcon oc +Ġeduc ated +Ġmod ified +Ġinc lusion +ment al +ŀ IJ +is ia +ĠÏĢ Î¿Ïħ +Ġa un +ĠIre land +Ġk ö +Ġcompl iance +Ġinsp iring +иÑĤелÑĮ но +Ġdisp os +ì° ¨ +Ġw ip +r ical +raw d +Ġt res +Ġmob il +olut ions +B O +Ġb ounce +Ġassum ed +ĠMed ical +Ġf iscal +Ġng ưá»Ŀi +ition ally +Ġst olen +ĠB M +Ġmechanism s +ε ί +Ġqual ified +Ġìŀ IJë +ught ers +ĠH IV +ĠL ots +Ġser vers +Ġcar r +ĠT ogether +Ġattract ed +Ġk r +æĪij æĺ¯ +th ur +in in +ĠH alf +È Ľ +ĠP ap +Ġremind ed +AL L +Ġhel met +Ġbott les +Ġprofess ors +Ġse ine +ÅĤ Äħ +ãĥ ı +Ġê±° ìķ¼ +Ġ×¢ ׾ +f un +ĠB ird +Ġfight er +ĠëĶ °ë +ĠT ool +Ġt in +ino is +ë ¶Ħ +×Ļ× Ł +ĠC AR +åIJ į +irst y +Ġout door +ĠN S +ãħ İ +ff en +Ġl ud +H ello +Ġroll er +ie le +ĠPol and +Ġap a +ex p +Ġcertific ate +ĠT own +аÑİÑĤ ÑģÑı +ild e +Ġdeterm in +P R +Ġfree ze +Ġmain stream +Ġobject ives +b lo +Ġtak ie +åĵĪ åĵĪ +Ġë°Ķë ¡ľ +el et +ĠI V +ĠF ast +Ġd ere +em p +ĠD ra +ĠìŀĪ ìĹĪ +Ġdisc rimination +Ġε ίναι +ne cess +æ ® +ıģ ı +Ġpost ing +wi ÅĽcie +Ġl ub +Ġol ive +Ġr im +Ġmodel ing +Ġa ño +ĠPak istan +Ġover l +Ġinf lam +N E +ìĹIJ ê²Į +Ġatt ended +Ġdeal t +ĠAl t +ĠL incoln +Ġaw ake +Ġfil ters +ĠWith in +czy wiÅĽcie +Ġs û +ĠJohn ny +Ġintegr ity +Ġisol ation +ĠE asy +ĠпÑĢ Ð¸Ð½ +ĠAl ice +Ġsm iling +en ix +, ... +Î ¶ +Ġbeg un +Ġjew el +Ġconvention al +Ġstat ist +Ġhand ed +Ġir re +Ġpro hib +Ġsatell ite +é¦ Ļ +ĠInd ust +Ġtra ged +Ġtra va +Ġih m +Ġcru el +ĠAg ora +ĠD oc +Ġz ones +Ġm all +Ġtr ay +×ķ× ł +Ġir rit +Ġk ans +ĠBe at +ud ge +ie lle +Ġtrust ed +Ġb ikes +ĠÑĥ п +ĠM ember +w ick +Ġcreat ors +Ġher itage +ind istinct +Ġres ur +enn en +C ome +Ġf iring +ĠBu eno +ĠТ о +ik an +ett es +Ġk es +Ġtri ps +Ġdivor ce +ĠK l +Ġcons ol +ke ep +기 ê°Ģ +ĠRep ort +Ġhost ing +Ġdiam ond +Ġcompl ic +Ġhel icop +Ġdep uis +d s +ĠCh an +Ñı л +Ġsc issors +il ation +Ġprop ortion +ER E +ĠÙĪ Ø§ÙĦ +int a +Ġmuch as +u ation +it is +æĬ Ĭ +Ñı Ñī +Ġni in +Ġemphas ize +uel a +Ġprodu cers +Ġr ze +änd er +ET H +æ º +Ġconst itu +åĽ ½ +Ġperform ances +ist le +go v +ĠL iter +Ġincorpor ate +Ġeduc ate +ĠN in +ì ª½ +Ùĩ Ùħ +el eration +×ķ× ij +Ġya ÅŁ +or ous +ĠC as +Ġgr ants +ëĬ ¥ +am el +Ġê·¸ë łĩê²Į +ĠE ste +Ñħод иÑĤ +ĠпоÑģ ле +Ġg ent +Ġfocus es +al ities +ĠR h +ë ³´ +æ° ij +ĠD ance +r r +Ġam er +Ġutil ize +Ġl ÃŃ +ĠAm ong +Ġpregn ancy +Ġlo ops +ал оÑģÑĮ +ĠM oh +Ġcatch ing +Ġglo b +Ġa jud +Ġ[ ? +ĠAn al +lo oking +Ġsurf aces +Ġprogress ive +Ġvir al +0 8 +Î ¾ +K A +Ġ ży +Ġpick s +ann on +Ġbul k +ĠR oss +Ġdescri bing +ĠG el +Ġloc ally +Ġend less +Ġmass age +Ġclean ed +Ġtravel ed +ен Ñĭ +Ġsent iment +ig ma +ĠN as +Ġchemical s +Ġright eous +ĠMag ic +Ġrel ates +Ġtruck s +Ġ19 60 +åĪ ¥ +Ġapp et +Ġsn acks +ĠSum mer +Ġy üz +Ġpr is +ĠMex ican +Ġtransp aren +Ġminor ity +Ġver te +Ġl assen +4 6 +л ек +é p +ĠÑĦ илÑĮ +Ġi yi +Ġsp an +íķĺ ì§Ģ +Ġind icated +qu ar +Ġscholars hip +ĠLGB T +Ġhistor ically +ó ÅĤ +Ġmin ist +Ġpen et +ĠR ap +Ġcons ervation +çĽ ´ +ĠH oney +ĠBe i +id el +Ġrespons ibilities +Ġmess y +ĠEx cept +OR E +Ġiniti atives +Ġjun ior +Ġdesign ers +Ġexpl oration +Ġspons or +Ġmob ility +Ġint eg +land o +Ġb ark +Ġindic ates +à ¶ +Ġemploy er +å® ī +Ġcous in +Ġbo iling +Ġch rom +Ġç al +Ġper pet +Ġcont ained +Ġpark s +Ð « +ĠEngine ering +P lease +ĠStart ing +her o +Ġlaw yers +è¥ ¿ +Ġz d +Ġfranch ise +ra ge +Ġint uit +ĠG L +re ach +ĠE lle +Ġnh ư +ĠN ord +Ġbe an +0 7 +Ġple asant +å½ ĵ +v iron +Ġgrad ient +z us +ĠE M +Ġess ay +ìĹIJ ìļĶ +ế n +n u +á» « +ĠÃī s +Ġden omin +ĠGirl s +Ġperson nes +ĠاÙĦØ £ +b ild +ĠSt at +Ġcompl iment +ĠK ate +Ġoptim al +Ġh id +د ÙĬ +Ġquick er +w all +E n +IN E +?? ? +ì² ´ +ĠA ction +å Ł +Ġpenal ty +ĠK az +' ? +Ġc ried +Ġcan vas +ft e +Ġexc lud +¸ë ¡ľ +Ġemphas is +Ġen zy +ĠH ou +Ġoverse as +ÃŃ amos +å¸ « +ö glich +Ġhead phones +c n +ĠA ge +Ġa kan +Ġcharacter istic +íķĺë ©´ +get s +Ġë¶ Ī +Ġr ival +Ġb orders +em ente +em ás +Ġy ol +Ġcom pe +end ers +ınd an +Ġmö glich +Ġbubb les +nat ural +Ġar med +Ġel abor +ĠìĿ´ë ²Ī +Ġwash ed +οÏħ με +è« ĭ +Ġfl avors +Ġexist e +Ġpre st +ĠThe ma +оп ÑĢоÑģ +er on +U E +er i +Ġconc er +Ġa ixò +åħ © +Ġprotect ive +Ġзна Ñİ +ĠëĤ ł +ĠII I +Ġme er +ĠSh op +ll i +ĠOr der +ĠM Y +ĠG host +ãĤĤ ãģĨ +ad el +Ġst ole +Ġrele asing +ĠCom ment +Ġtra ins +ë ªħ +Ġw issen +ens ed +Ġdesc end +Ġf ier +Ġrad i +Ġpers u +ç ¢ +Ġм н +ĠD est +Ġwor ries +it et +b as +Ġst ab +n ame +or ic +ĠCl ose +Ġalum ni +ĠS elf +ff e +it ating +ather ine +ĠRight s +Ġell os +Ġwar rant +Ġn erve +Ġveget able +ĠTe il +Ġê°Ļ ìĿ´ +R Y +Ġsustain ability +Ġste ht +Ġbr id +ada ÅŁ +Ġt v +Ġdur ation +Ġpesso a +Ġmet rics +Ġad am +c as +аÑĢ Ð¸ +Ġev ident +Ġdisplay ed +Ø§Ø ¦ +Ġre ck +ĠBudd ha +Ġde le +ĠDie go +os ph +Ġb la +ĠM ik +ul ator +Ġ200 1 +Ġpromot ing +y ch +ĠE X +Ġlast ly +Ġout line +Ġspir its +Ġve ux +Ġsubt ract +ĠÅŁ imdi +Ġp ins +Ġbur ger +Ġmol to +Ġhab ÃŃa +Ġë° ĺ +ig u +er st +Ġn en +Ġbac on +it ious +Ġcar ries +Ġprom ises +nd e +ĠLe ft +ĠL im +æ £ +Ġ4 4 +Ġcare ers +Ġì£ ¼ë +Ġspeed s +qu é +m ad +mark et +is me +Ġ200 3 +Ġre cess +ĠJ UD +Ġrac ist +ĠSch l +Ġpar ler +Ġot ros +ish es +Ġconvert ed +aa aa +ани и +ĠAr k +ĠCh ance +Ġelement ary +ε ν +ink s +Inter viewer +Ġfre ely +al ah +Ġëĭ¤ë ¥¸ +Ġrequest ed +Ġtor que +no ÅĽci +ou red +ĠSt aff +Ġst ain +ĠAl an +Ġv ere +ĠW inter +Ġdef ect +ied y +Ġbe ats +Ġh á +um n +o ons +it udes +Ġse it +o ly +Ġres erv +Ġext r +Ġphys ician +vis or +Ġhand ful +ĠN ations +Ġì¢ĭ ìĿĢ +uc cess +Ġup stairs +ĠSqu are +Ġhe in +ĠSe ason +ol is +Ġpr ince +Ġdef ensive +ç ½ +Ġм еÑģÑĤ +Ñĸ й +Ġا ÙĨ +um ble +ê¹Į ìļĶ +Ġass ass +Ġcirc ular +Ġqual ities +Ġh mm +Ġbl own +ĠL iz +ĠK ur +ĠS A +Ġfind ings +Ġcol ours +Ġde lle +ĠI R +ĠA th +ĠD ub +ĠO x +ĠØ ® +Ġpo ckets +Ġgr ill +Ġswitch ing +Ġprefer red +ĠW ales +Ġex emplo +Ġchop ped +Ġvacc ination +Ġne uro +Ġspec ify +iv os +Ġser á +Ġz ie +Ġà® ® +Ġresult ing +ĠU gh +Ġmess ed +C D +Ġpa ar +Ġcom er +Ġcou ch +ĠFest ival +Ġ4 9 +v ous +z ens +ç¨ ® +ĠKenn edy +ĠT s +Ġë³´ì Ĺ +Ġdemonst ration +Ġun to +Ġfrust rating +Ġlabor atory +Ġe gy +Ġbeaut ifully +Ġìŀ ¬ë +Ġal gu +Ġö yle +ä½ł çľĭ +ĠP H +Ġfort une +Ġclean er +ĠRob in +Ġsa us +ĠG eld +Ġk at +o bs +Ġol ur +Ġm att +Ġquest a +Ġsuggest ion +en cer +о ÑģÑĤ +Ġrad ar +Ġìŀ ¡ +ish a +à® ¨ +ãĤĵ ãģª +j es +Ġve el +ìĤ ° +Ġauth ors +ãĢ İ +pl an +Ġcollabor ative +Ġinst inct +Ġfar ming +au ge +E du +Ġmembers hip +Ġsimult aneously +Ġb ake +Ġk ä +Ġlect ures +Ñĩ еÑģ +Ġprend re +Ġcoll aps +ĠS aya +ĠF ut +Ġy og +ĠR ather +ر ÙĬ +Ġcamp s +ол од +Ġsim ulation +ĠM ak +La ughs +Ġgre y +Ġsent ences +y en +ĠUn less +J e +ĠSat an +ĠÑĤак же +ĠN A +Ġbr on +Ġ? ] +Ġsoul s +Ġlight ning +Ġimag ined +Ġczy li +ps ilon +et ta +Ġbelie ving +Ġstrong est +ĠC ON +Ġquel ques +Ġimmig rants +Ġwall et +éĢĻ æĺ¯ +ĠJer sey +Ġimplic ations +Ġfor b +ãĢ ı +Ġun believable +Ø§Ø ¡ +Ġoper ational +ü s +ĠG M +Ġê·¸ëŁ °ëį° +Ġgrac ias +Ġent end +ĠReg ard +ro b +ĠÑĤ еÑħ +è ı +ĠRev olution +Ġwa ar +ĠB iz +th eless +Ġspons ored +qu ier +ĠìĿ ¼ë +Ġte k +ĠëIJ ł +ig keit +ĠL uck +ĠCertain ly +Ġto ll +Ġн иÑĩего +ĠM oney +ĠÑģ ÑĤоÑĢ +ĠDou ble +ĠW olf +Ġch unk +ά ν +it és +on ing +M ar +Ġgrand es +Ġcollect ions +ĠEurop a +Ġа ÑĢ +ĠâĢĭâĢĭ âĢĭ +Ġê·¸ëŁ¬ë ©´ +Ġоб ÑĬ +Ġãģ ª +Ġìĭľ ê°Ħ +ĠC ustom +Ġì² ĺ +Ñĸ лÑĮ +Ġindivid ually +í Ĺ +Ġdo zen +Ġo we +ĠVict oria +åı¯ èĥ½ +Ġbe et +ur b +Ġanal og +i ção +Ĥ ľ +so ever +Ġmod o +Ġsubscri bed +ìŀ ¬ +Ġent ities +çī ĩ +Ġclos et +Ġrespond ing +Ġprin ter +ĠStep han +Ġby ÅĤ +ĠD om +ĠF ern +ĠP ier +ĠwiÄĻ c +Ġh ence +Ġmod ules +ãĥ ¬ +ĠëĶ ± +ĠDann y +ĠÑģеб е +Ġv ad +ĠìĹ Ħ +Ġs ous +Ġsp here +B Y +ĠP ed +ign ed +Ġwhe at +Ġund ers +Ġevol ve +Ġdec lar +Ġlight ly +Ġident ifying +æĦı æĢĿ +Ġlegend ary +Ġgen uine +Ġgr ind +ĠU ne +ge ben +Ġb icy +Ġjump s +Ġprov ince +zi ÄĻ +Ġ×IJ× ł×Ļ +Ġh oc +Ġб л +ĠGr ad +Ġreven ge +ĠاÙĦ ت +o oh +æĭ ľ +аÑĨи и +å¹ ³ +Ġelect ro +ĠëIJ IJ +ãģ§ ãģ¯ +Ġf als +ri el +ok er +ĠEx cellent +ĠMor gan +Ġbr ick +Ġsubstant ial +Ġpoll ution +ĠT ür +ĠEv et +Ġl ung +ãģ ĸ +×Ļ× © +omm es +Ġreal izing +Ġhum ble +ĠL ock +Ġb od +Ġìĸ ¸ +Ġpe ers +uz z +Ġembed ded +Ġclar o +Ġag greg +Ġemploy ers +ĠR aj +Ġãģ ¨ +ĠY i +Ġje u +at ers +Ġstri kes +n os +aut res +d r +op her +ĠApp arently +íĺ Ħ +Ġinf ant +ا ب +ÑĤ Ñĭ +í Ľ +Ú ¯ +Ġred es +acaÄŁ ım +ĠDA VID +ĠCh icken +Ġperspect ives +Ġview er +Ġsh ar +ĠпÑĢо из +lig t +er os +it able +ил оÑģÑĮ +Ġdif ÃŃ +´ë į° +Ġret ired +Ġthat s +zen ie +be iten +Ġmy cket +ĠR ab +Ġinflam m +ì° ® +Ġd um +Ġdad dy +æľ Ł +Ġimm ers +Ġplay list +௠Ĩ +Ġtra um +Ġref use +st ep +à® ļ +c up +Ġpop s +r imin +ay ım +Ġa ld +Ġun necess +Ġd ah +ĠIr ish +Ġcomp r +la ÅŁ +T P +Ġtransl ated +S c +ce ÄŁim +´ IJ +Ġd rei +ĠлÑİд ей +Ġqu iero +Ġhe le +z lich +Ġapp les +Ġdistrict s +Ġcred its +Ġas p +Ġëĭ ¨ +or al +å½ ± +Ġste pping +ĠV a +Ġg ains +6 5 +Ġnuest ra +ed ay +ass ador +ĠL ind +Ġcrop s +ci endo +ig ue +Ġb ana +A m +Ġp ent +Ġadd iction +Ġpack aging +ä d +ª ¨ +Ġper què +Ġcampaign s +Ġste ep +Ġne ue +Ġembarrass ed +Ġdist inction +it zer +åij Ĭ +Ġregist ration +Ġll am +ĠAlm ighty +li est +Ġu z +n ak +ç º +Ġter az +iam ente +Ġtrans actions +Ġc ôt +Ġswitch ed +Ġcom bo +Ġpray ers +Ġintern ship +Ġaddress es +Ġchar ity +ĠW OO +Ġb ait +è¿ ĩ +Ġ � +Ġf ica +ĠTy ler +ar u +Ġat oms +ĠLe vel +ĠпоÑĤ ом +Ġf ame +ul k +Ġteach es +Ġre build +ед ÑĮ +ĠIndones ia +ush i +ĠSh ort +Ġens uring +f s +e le +Ġmargin al +Ġconclud e +am t +Ġver ify +ĠMc Donald +Ġsk al +Ġrec onst +ĠM ann +Ġbas ement +Ġtransform ed +Ġoccasion ally +z one +ĠD ans +Ġкак ой +Ġdiagn osed +ĠÏĦ α +Ġcomm ands +Ġpresident ial +Ġab b +Ġbrack et +ĠL em +Ã¥ ng +Ġfavor ites +Ġrev ol +ĠíĬ ¹ +Ġhar ass +é ħ +Ġcle ans +st änd +Ġknock ed +Ġpe oples +Ġmusic ians +Ġmut ual +ĠC old +8 8 +ze j +at ie +ĠHon or +Ġobs essed +ĠM USIC +ĠBre ak +ú ng +Ġmod ify +Ġs öyle +Ġ×ŀ ×Ķ +ĠOn line +f o +ĠMill er +Ġlik ing +Ġin hab +Ġgrat itude +ĠJour nal +arn ess +J ohn +ĠG it +åī Ľ +Ġsin cere +ĠS ci +ĠE li +Ġsymbol s +Ġman ually +ε ÏĤ +Ġв Ñĸд +ĠF at +Ġlab els +Ġsophistic ated +ump s +Ġrele ases +Ġ4 7 +ĠO M +ê°Ģ ë +ĠB ien +ĠRe f +è¨ ĺ +ĠSt a +ĠE gg +Ġindic ator +ps on +Ġnas ıl +R ight +Ġcon vey +Ġkn ot +Ġconnect s +ul as +Ġpre ced +Ġine quality +am iento +Ġrep ly +O Y +Ġdism iss +ĠëIJ ľ +çĦ ¡ +ĠÑħоÑĢоÑĪ Ð¾ +Ġm éd +Ġrandom ly +ĠO nt +u ard +Ġpull s +ĠÑĤ епеÑĢÑĮ +ĠNe ed +ĠSo ft +Ġstrength s +Ġgo ed +um en +æŃ » +Ġíİ ¸ +Ġд об +Ġclar ity +ĠA i +Ġball oon +ĠP and +ĠìķĦ ëĭ +Ġsh iny +Ġsmall est +on ia +h ill +ot ing +Ġe ing +Ġmere ly +Ġse us +Ġн еп +Ġí Ĩµ +Ġgu ides +Ġspecial ist +Ġste ak +ãĤĪ ãģĨ +Ġmig ration +que le +Ġru ined +Ġpu pp +å¥ ³ +Ġk end +ang an +Ġpal m +Ġunf air +Ġz m +ĠD V +ch ester +и Ñİ +Ġo oh +er g +AT H +° © +åĵ ª +r ison +Ġinvol ving +Ġpart ly +anç ais +Ġv ow +Ġprom inent +Ġcry st +ib a +Ġdes erves +Ġover t +Ġsens it +ĠWh e +Ġtight en +Ġintim id +Ġal iment +w ill +Ġstrength en +ĠT an +åı Ī +ãģĹ ãģ¾ãģĻ +on i +ĠM un +Ġpro ph +Ġrehe ars +ĠK le +Ġve ces +Ġwonder ed +ok i +Ġsens es +´ì ĭ +ưỠĽ +ĠÈĻ i +Ġmuch os +Ġwatch es +ortun ate +ĠJ uan +ìŀĸ ìķĦ +ÑĢ Ðµ +e i +ion en +Ġexperiment al +Ġda ughters +ภĽ +Ġment ally +bec ca +aw are +ìĦ Ŀ +Ġwhat soever +Ġen ables +ĠL ow +o id +ภĬ +ó d +Ø º +Ġconstruct ed +ĠLad ies +Ġaccus ed +Ġа н +D an +Ġsp awn +Ġcontain ers +Ġart istic +ı p +Ġdisc l +Ġaut res +in as +ĠN ation +Ġn ag +be an +w he +ľë ıĦ +ĠSe oul +Ġíı ¬ +ĠN ich +Ġcomp lement +Ġinter ven +ĠMod el +ĠOr ange +nam on +Ġcalcul ation +se e +Ġusted es +Ġle b +Ġdo ct +Ñĸ н +Ġf oster +Ġel astic +ĠAh h +Ġa ce +ĠP ink +ĠJ eg +Ġde er +ãģĹ ãģĦ +s is +Ġjak o +ĠEm ma +ÑģÑĤв енно +Ġport rait +Ġmak er +Ġa ument +ÑĢ Ð¾Ð± +Ġairpl ane +Ġtransparen cy +Ġadjust ment +ĠCD C +ç on +Ġupload ed +Ġд ейÑģÑĤв +Ġго ÑĤов +Ġit er +Ġcur se +ô n +mer ce +ar an +Ġle ak +çµ IJ +Ġabs ence +Ñģ кий +Ġread ers +al er +Ġbene ath +ang o +h etic +Ġfin ns +Ġpo op +Ġdu plic +H i +ig s +olog ically +op p +Ġd izer +ĠAll en +Ġgl i +Ġacc eleration +Ġvit amin +ãĥ Ń +v ä +ĠAc cess +à® Ļ +r ás +Ġappreci ated +Ġn ah +Ġpos ter +Ġt ale +Ġhighlight ed +æĸ ĩ +ż eli +Ġblock chain +Ġmic row +Ġcin ema +ĠCh ang +ĠSe arch +ust ers +ĠZ ero +ĠDiv ision +ÑĢ Ð°Ñģ +Ġsca re +Ġj elly +ĠAdminist ration +S O +Ġl ined +Ġê° Ħ +Ġge ben +Ġso da +Ġwin ners +³ ¼ +Ù Ĵ +ĠAm b +åķı é¡Į +å Ķ +Ġpe g +å· ± +4 3 +Ġra us +Ġre wards +Ġinc lus +Ġhigh way +Ġha h +Ġmultipl ied +Ġs ẽ +Ġdisci ples +Ġn ing +Ġdress ing +Ġattrib utes +ĠM osc +ĠGree ce +Ġse k +ĠLe arn +Ġj us +rend re +Ġperson ne +pl ete +Ġpl acing +Ġl uego +ill ance +Ġоб Ñī +Ġprov ision +Ġl ion +t ra +bo ards +Ġbehavi our +he y +Ġsubscri ption +Ġprot agon +ãĥ £ +Ġvar a +ĠÅŁ u +Ġha ha +Ġteas poon +æ Ł +av oir +Ġcrypt o +ĠÑģÑĤ аÑĢ +ĠSt ore +ab s +ĠStud ents +Ġla und +int o +Ġapproach ed +° ľ +ÑĥÑİ Ñī +ĠL abor +ot es +iat ric +Ġgro ÃŁ +ut ive +Ġи д +ĠG ib +Ġpl acement +ĠdifÃŃ cil +Ġf rog +ĠвÑģе Ñħ +ĠJ r +az ed +Ñĥ Ñī +Ġê ¼ +fr ame +а еÑĪÑĮ +Ġlock down +åij ³ +Ġmed i +Ġ×Ķ× ŀ× +ени й +em ale +ì¢ ħ +ater al +Ġdist ant +Ġbe ars +Ġjournal ist +è§ £ +ĠMarsh all +ĠIh nen +uet ooth +b ag +ĠÄij ã +ĠHigh ness +Ġì° į +и ка +ĠW u +ĠFr an +Ġp eng +Ġf on +Ġhypothes is +ĠÑĢ Ñĥ +Ġl y +× ļ +ìĽ Ķ +ĠRad io +ภŀ +D av +Ġembarrass ing +ĠìŀĪ ìĸ´ +Ġcast ing +Ġc age +ĠP sych +ĠìĿ¼ ëĭ¨ +ĠÅ ¾ +im b +Ġdirect ors +S H +ĠÏĦη ν +á»ģ u +Ġkon uÅŁ +Ġoption al +quar ters +ik er +ĠS ant +Ġvers es +ë ¶Ģ +Ġo lar +ĠÏ ĩ +ãĥ ķ +Ġγ ια +ĠI mm +Ġcontrovers ial +Ġer sten +Ġreci p +ĠChristian ity +Ġê´ ľ +ord on +×ķ× © +Ġsl ash +ĠP f +Ñĥд ÑĮ +×ķ× Ŀ +ĠPer ry +Ġm amy +Ġbackground s +Ġà®İ ன +Ġpend ant +ĠColumb ia +Ġin verse +ĠÑĩеÑĢ ÐµÐ· +Ġs v +Ġdig ging +4 1 +ch em +Ġnavig ation +ĠSh in +ĠFr ont +P D +Ġbe aring +ĠW asser +Ġw ax +ĠCH RIS +ch ing +Ġpress ed +E l +ĠD al +ons in +Ġb inding +Ñģк ой +po ons +Ġmo ck +are st +к ÑĢа +M M +Ġcor rupt +st orm +Ġref res +ĠCo ach +ll ä +ĠTH IS +Ġpar ag +Ġìĵ ° +p ool +Ġbill ions +Ġê¹ Ģ +gr oup +Ġwel coming +cell ence +ĠDu ke +ê¸ ´ +Ġprim era +ìł ¸ +Ġp ond +Ġstat ue +Ġêµ ¬ë +Ġh atch +Ġinstrument al +Ġresident ial +ì» ¤ +Ġaccept ing +osh i +d ate +ĠìĶ ¨ +Ġplant ed +Ġj oking +Ġì Ħľ +Ġh ated +ĠÑĢаÑģ Ñģк +Ġsle pt +Ġpack ages +Ġisland s +es en +ÄŁ ı +Ġdi agon +ĠO sc +Ġmes h +Ġsc ales +ar ity +ĠDef ense +ãģ¡ ãĤĩ +ĠLew is +ĠÑģ егоднÑı +Ġfl ies +uin ely +ĠCons ider +Ġst ark +he w +ĠAs ÃŃ +³ ´ë +Ġprop ose +Ġíķĺë ©´ +od o +ĠNorm ally +Ġhe eft +ĠHarr is +g ro +ĠBlo od +b ase +Ġi OS +Ġtouch es +Ġinsp ir +Ġ× ĵ +Ġb inary +Ġì¶ Ķ +Ġser ial +Ġ ion +Ġunemploy ment +Ġodd s +ĠF ab +ĠF BI +BR UN +Ġweight s +ν ο +at ile +Ġnurs es +Ġinvolve ment +ĠíĶ ¼ +Ġgovern ance +Ġâ Ĥ¬ +ÑĢÑĥ п +ier ra +íĺ ķ +ĠJ erry +Ġbe ard +Ġsal vation +ĠAl ong +g entle +ĠK i +b ol +ĠPl at +Ġhas ht +è¿ ij +Ġw are +Ġpart ie +y cz +Ġint r +F ih +n ent +Ġche at +il en +Ġë ¯ +or ie +Ġfá cil +et ric +Ġaffect ing +unci ation +Ġaff airs +Ġbe e +Ġview ing +Ġor ang +ĠL an +ĠС ÑĤ +ä¸ ĸ +ĠM es +ĥ ģ +er ie +Ġes pa +Ġinter pre +Ġposs ess +Ġpure ly +rit o +f ound +as ma +ìłģ ìĿ¸ +Ġexam ine +ĠÑĥ м +Ġbes ch +ĠTom orrow +ĠB lock +Ġvari ant +Ġprefer ence +Ġcoach es +Ġmedic ations +Ġíĺ Ħ +Ġemp ire +ë Ħ¤ +ĠIll inois +Ġcris py +Ġth ì +Ġbe es +7 7 +Ġgl ow +è º +ĠStud ies +åIJ Ħ +ĠChall enge +Ġunlike ly +Ð § +ıy orsun +DI E +Ġminim ize +iz ard +Ġú n +Ġencont rar +ĠK ill +å » +Ġvan illa +ĠGr ant +ĠG T +se a +Ġs ought +в од +Ġnä m +ĠA unt +OW N +Ġpump kin +st ellen +Ġr ag +ег да +Ġstory t +Ġfor um +æ© Ł +Ġestab a +uch e +Ġcon gress +ĠRe y +Ġdram atically +ĠSp ort +ĠYe llow +Ġê³Ħ ìĨį +Ġdisg usting +ĠRe cent +Ġacqu ired +Ġc ables +çĶ ļ +d in +Ġv isto +Ġcommunic ating +ÑģÑĤав лÑı +еÑģ ÑĤо +ãĥ»ãĥ» ãĥ» +Ġré g +Ġso cks +Ġpro ces +be cause +Ġut ter +Ġcoloc ar +Ġnew est +Ġgr amm +è¡ ¨ +ä¸į çŁ¥éģĵ +Ġsh ifting +Ġcar rier +ĠÑģк оÑĢ +ĠSch w +Ġexec uted +Ġmaint ained +ĠÏ Ĩ +ĠM oses +Ġdis se +Ġhor r +ãĢ ľ +Ġr ally +Ġall em +ĠEvent ually +Ġdi yor +lv ania +Ġsch nell +Ġê³ ¼ +Ġë§ ¤ +Ġstrugg les +l ate +Ġclar ify +é ment +Ġmulti plic +иб о +Ġjour n +Ġfra gr +Ġsurprising ly +Ġdesper ate +5 2 +Ġs ul +ĠRe ad +ĠF ried +Ġm ond +w oo +Ġorgan izing +ãģĹãĤĩ ãģĨ +ĠSo on +Ġв опÑĢоÑģ +ĠN ur +ĠÐĹ Ð´ +Ġsp ider +е ÑģÑı +Ġtutorial s +Ġnutri ents +or er +Ġcoe fficient +Ġarrange ment +Ġpr icing +n an +y u +B L +Ġtri be +ĠHow ard +un ks +Ġnew er +Ġprov in +Ġpred iction +h os +Ġol sun +ĠAr ound +Ġv ier +ĠÑģÑĤоÑĢ Ð¾Ð½ +Ġv alley +ĠE la +if i +Ġgal axy +Ġtran qu +Ġad vers +ĠTem ple +iff s +ig ence +èĩª å·± +Ġkön nte +ĠÄij ó +D id +Ġphotograph s +ĠA WS +ÑĨи Ñı +Ġgu ards +Ġappoint ed +ĠG il +Ġм ом +Ġc od +ĠUn like +Ġeven ly +isc onsin +Ġest ou +Ġm nie +ĠEx ec +ĠM V +ĠE ine +ä¿ ¡ +ĠRog er +ĠF ac +ĠL ist +Ġf uer +аеÑĤ е +om ed +Ġattract ion +èī ² +Ġter rain +ĠD rop +Ġcorpor ations +Ġsci ences +Ġthr one +ãģĦ ãģŁ +Ġa j +ĠR ot +çī ¹ +Ġsupp orters +ĠB ere +H ere +Ġdifer entes +Ġsignific ance +Ïĥ η +æĪij 覺å¾Ĺ +Ġcl amp +Ġë ĮĢë +Ġfab ulous +re z +æĮ ģ +Ġassum ptions +ut her +w id +p ot +è¿ İ +Ġy an +ul in +ÑĢ Ñĭв +ĠSl ow +ĠPenn sy +Ġíķ ´ìĦľ +Ġme io +Ġwealth y +ĠE ight +Ġpul se +Ġfr iction +id ity +ĠH oll +i yorum +Ġsound ed +ĠC arr +Ġfor k +â ĺ +ĠP A +Ġcons pir +Ġc oding +r t +ĠTy p +Ġìĸ ij +Ġп ог +Ġmis er +ĠÑģм оÑĤÑĢ +ĠSw eden +Ġolar ak +ĠZh ang +ĠCh i +ĠT itan +Ġscreen ing +ĠSp ider +ĠÅŀ imdi +Ġobst acles +lar a +Ġchalleng ed +p se +T ON +á» ¥ +ĠP i +Ġlag i +ie urs +Ġhur ting +Ġneg lect +Ġgener ating +Ġyoung est +Ġaud it +ĠÑĢ ÐµÐ· +Ïģ ά +Ġdon ate +ĠPD F +Ġvis its +Ġcru ise +P P +as er +Ġw sp +back s +iv als +ãģĨ ãĤĵ +Ġde ve +Ġprop ort +Ġc ath +ĠE ffect +Ġwind s +ĠìĻ Ķ +Ġchart s +Ġs ama +Ġautom ation +Ġпок а +Ġol an +Ġbo ats +Ġca fe +Ġden ied +ĠM ama +Ġblock ing +ĠTh or +Ġphenomen al +Ġstake holders +Ġun os +Ñĥ еÑĤ +ĠAb raham +ãģ§ ãĤĤ +Ġdetect ion +Ġjur is +Ġpower ed +z ial +Ġwel fare +Ġup grad +Ġmoż na +ĠC ase +c ular +Ķ ìĿ´ +ãĥ ģ +ĠGu ess +Ġcy cles +ä¾ ĭ +çµ ¦ +ro ck +um i +Ġel ite +Ġqu è +åł ± +ÑĤ ом +Ġsh ore +gun ta +Ġk u +Ġfaith ful +ĠJ eremy +a id +à · +ug al +å°į åķĬ +ĠV el +Ġvra i +st ell +¨ ¸ +Ġk ol +è ½ +Ġquant o +Ġз аÑĢ +Ġ200 2 +es y +Ġres erve +Ġмом енÑĤ +Ġdeploy ed +Ġdefin ing +Ġsa u +Ġga at +" ) +Ġtrans mit +Ġpubl ishing +Ġrank ing +Ġoff ense +Ġ4 6 +p in +ĠT aking +Ġentit led +Ġgen uinely +Ġvari ations +Ġfind e +Ġt au +Ġunf ortunate +ĠR ah +port s +Ġc Å +Ġmon key +Ġbr ac +we i +l ung +Ġart if +Ġsy rup +ĠÐĶ Ð°Ð² +Ġlift ed +Ġche z +ĠAd vent +ĠSt ock +Ġdo l +м ен +иÑĪ ÑĮ +Ġy n +g io +d et +Ġdes se +Ġg ri +ĠChair man +ç ħ +Ġcu enta +an im +Ġcra b +Ġesc al +Ġpremi ère +ĠGe f +Ġd ining +Ġsevent h +Ġch asing +ĠT ower +Ġbrut al +Ġfundament ally +ãģ¨ ãģĨ +л ениÑı +st age +Ġacqu is +Ġcyl inder +Ġcomm ander +m em +ĠU V +ha ppy +Ġe psilon +Ġinv itation +Ġfar mer +ch air +Ġdest iny +Ġso vere +ĠHeb rew +Ġserv ant +Ġbe w +Ġg ast +ut ies +Ġadministr ative +ĠComm and +é ta +Ġnit rogen +ê· ¼ +Ġab i +Ġvill ain +Ġblank et +ĠS end +Ġbeat en +² Ħ +Ġvol unt +Ġschol ar +ĠEm peror +Ġ4 3 +v able +ĠD us +ĠG U +Ġtarget ing +ww w +Ġamend ment +ìĨ Įë +Ġt ing +Ġn asty +Ġg auge +ĠÑĢ Ð¾Ð´ +ĠH ans +Y our +α ν +Ġpro jet +ĠHawai i +Ġsusp icious +Ġsch w +Ġremo val +Ġint rig +ĠM U +Ġp onto +ठ¾ +Ġоб ÑĢаз +Ġguess ing +p ace +Ġm others +Ġmill imeter +л ение +没 æľī +Ġavail ability +ic z +æŃ ¤ +Ġfr act +Ġbas es +k m +ĠB TS +ĠF ield +Ġd zie +Ġseg undo +ĠëĤĺ ëĬĶ +Ġlegit imate +im as +Ġв н +Ġcor ruption +Ġsm ash +ĠVal ent +Ġalign ed +ĠPennsy lvania +Ġg ab +ĠE un +ent h +ĠMor ning +Ġcand le +Ġback pack +ĠIslam ic +a ções +Ġenc ry +Ġmushroom s +íĮ Į +d it +Ġtrans it +ĠW isconsin +Ġparticip ated +ĠIl s +Ġunf old +¶ Ģë +Ġprof its +Ġwar ming +ĠG ang +Ġnetwork ing +Ġme ga +Ġthorough ly +le ments +ĠH m +Ġdec iding +Ġemotion ally +Ġexha usted +ĠÐŁ оÑĤ +c ido +ĠHT ML +Ġcopy right +Ġmel ody +y im +Ġand ers +osh op +Ġë³ ¼ +Ġathlet e +ĠG E +Ġfrequ ent +Ġdes ires +Ġneed ing +ĠY un +Ġrif le +Ġlo ver +' T +Ġd ense +Ġt ão +Ġnot ified +Ġid i +ìĹ Ń +í Ĩ +Ġinteract ing +Ġrapp ort +еÑĢ Ð¸ +s ki +Ġb esser +Ġmanufact urer +ĠK yle +Ġaccount able +ĠS ak +ĠP il +ĠD omin +Ġpres um +ĠÐĴÑģ е +Ġvine gar +Ġguarante ed +çľĭ åΰ +Ġhand led +éŁ ³ +c at +Ġcivil ization +Ġaccom p +ĠV M +é mon +Ġde ze +Ġgrad es +Ġsoll te +Ġst aring +×IJ× ª +ar nt +Ġhoriz on +Ġtrav ail +h our +第 ä¸Ģ +ĠE D +ĠD ak +Ġn y +Ġcon ve +ĠCh am +Ġfir ms +ĠL iu +ĠÑģÑĤ ÑĢан +Ġli bert +Ġlens es +Ġint ake +ĠвÑĭ б +Ġmens en +h el +Ġpract ition +Ġ3 50 +ãĤ ³ +F O +Ġbed s +Ġancest ors +ĠìĹĦ ì²Ń +Ġdistur b +ĠLast ly +ĠSupp ort +ี à¹ī +ĠCor ona +Ġenthus i +Ġвоз м +ĠìĤ¬ëŀ Įë +Ġ5 2 +b ird +Ġredu ces +ĠìŀĪ ìĿĦ +ĠG ene +êµ IJ +ÄĻ p +ĠÃľ ber +Ġconcer ning +us er +Ġconcent rate +ĠWH AT +ish op +onym ous +no ld +Ġsuggest ing +© ° +ĠF ish +.... .... +Ġvess el +Ġtrabaj o +ãģ µ +ĠO cean +å§ IJ +y g +Ġtown s +d el +Ġterr ifying +Ġçal Ä±ÅŁ +Ġs ino +Ġe ats +Ġge z +Ġg eme +ĠìĻ Ħ +Ġcomp art +Ġimplement ing +ĠPot ter +ĠGerm ans +Ġg ÅĤ +Ġt ennis +Ġcar pet +au er +ĠSaud i +ye ong +Ġcur ry +ĠFore st +Ñĭ л +Ġfif teen +Ġbol ts +Ġ{ \ +¬ ´ +Ġsett lement +Ġl ange +Ġb am +G et +íķ Ļ +Ġsw ap +ĠK han +Ġcomm ence +Ġquar antine +Ġsc ored +ç ĸ +Ġ19 50 +Ġthick er +Ġsû r +åı £ +ĠLar ry +Ġall ez +ìĭľ ëĬĶ +Ġg ü +Ġspect acular +/ / +b oth +Ġst ats +å¦ ³ +ĠN ancy +Ġbun u +Ġcr ust +Ġactiv ated +Ġê·¸ë ŀ +out he +Ġport s +Ġne ural +Ġj aw +Ġobserv ations +Ġvo it +ab an +ả i +¦¬ë ¥¼ +om es +௠ĭ +qu i +Ġkind ness +Ð ij +Ġ4 1 +Ġmoder ate +Ġang els +ĠT amb +è t +Ġch lor +ĠBill y +ì² ĺë +ac on +Ġselect ing +ĠDel ta +Ġn ull +den ly +Ġci ud +Ġtend ency +Ġbreak down +Ġm int +ÑĦ оÑĢм +or ph +Ġda wn +s pr +ĠW ILL +äch lich +Ġpu ppy +7 00 +Ġà® ¤ +Ġfail s +ĠCon c +Ġrel atives +Ġinv iting +Ġaut onom +Ġcomp osed +Ġun ity +Ġdec is +Ġaccess ories +ĠC ass +Ġb ist +ĠT ip +ì§ ¸ +Ġp unt +Ġr áp +éĢ ² +AN K +ãģ ļ +ex ist +Ġcompat ible +Ġn er +Ġе мÑĥ +Ġa plic +Ġb apt +Ġfail ing +ĠTam am +Ġos cill +Ġletz ten +Ġrepeated ly +Ġjung le +ĠP ush +h ai +ĠÎ · +Ġdead ly +Ñı ж +wi Äħ +ĠComm on +ĠÎ ķ +Ġsk ate +T C +ĠMin i +Ġhob by +ầ n +Ġrout es +Ġam igos +Ġcon jun +Ġpartners hips +Ġno vo +Ġa ver +Ġpou vez +br idge +Ġpre oc +h im +Ġtur b +Ġso b +ĠSn ap +Ġì° ¸ +min ute +Ġtra ject +uj ÄĻ +Ġe ager +Ġregul atory +Ġbank ing +b ling +ÑĪ ÑĮ +a ż +Ġbiz arre +it ated +d ire +Ġthreat ened +Ġsh ining +Ġn esse +Ġcor ps +ĠÑģ Ñĥ +Ġt eles +Ġtem p +t em +Ġк ан +Ġfe ver +N ew +Ġheav ier +ĠS ah +b ud +Ġout ros +Ġì° ¾ +Ġëª ħ +arr ing +Ġê´ľ ì°® +ĠN ap +Ġse min +ĠTh an +if s +Ġdes en +ĠÑĤак ое +Ġlos es +ĠB alt +k on +Ġнап ÑĢ +Ġvo is +ĠMosc ow +Ġch airs +h is +Ġrefuge es +k g +Ġk ole +į ¨ +аÑģ ибо +¦ ½ +ĠUn iverse +ĠDire ct +Ġche ating +ĠC in +Ġpat ri +Ġadv ise +ĠN ether +Ġprime iro +Ġmention ing +n ut +5 6 +ar ı +Ġpet ite +b led +Ġpens ar +ic io +IN D +Ġveter an +Ġlad der +Ġconsequ ence +ож ал +ĠB urn +Ġr ug +ĠM ade +Ġg it +" ... +Ġcompet itors +Ġprz ed +Ġapp arent +ĠArgent ina +ĠWork ing +Ġcollabor ate +w oman +Ġret ain +Ġle urs +Ġdash board +×Ļ× ĵ +ĠEar ly +B M +Ġе Ñij +ол ог +Ġsatisf ying +Ġoft entimes +Ġma pping +ünk ü +ar th +f old +Ġlaunch ing +Ġa ura +Ġprec ision +work s +G od +Ġstra p +ĠIm per +Ġr ivers +Ġ | +Ġcu er +reg on +Ġarri val +ка Ñħ +ĠM iami +ан Ñĭ +Ġsurviv ors +ĠSen ior +Dav id +Ġest ado +Ġse ctors +Ġpop ping +Ġch im +ay ı +Ġkun nen +Ġgall ery +Ġsun light +ese hen +Ġye lling +ĠMe in +ĠPho enix +Ġman o +Ġhistor ia +Ġoccur ring +æ¬ ¸ +ì ¸ +ад и +å¾ ħ +Ġinstitution al +ĠT ut +ç ² +Ġsl aves +ãģ© ãģĨ +Ġforg iveness +Ġtw in +ĠHy un +н ÑĮ +ĠK omm +and ra +sh ot +ss ä +ĠÑĨ е +at ta +Ġexp ense +ĠG PU +ĠP ast +rib ly +ĠëŃIJ ìķ¼ +Ġгод а +Ġresp ir +æĿ ± +ĠQue ens +h ops +Ġs érie +Ġpre f +Ġcom ed +Ġpl ut +ĠOver all +Ġãģ Ŀ +Ġc ush +Ġring ing +Ġincor rect +ĠÑģÑĤ ÑĢ +Ġgeomet ry +Ġadvert is +ĠÐ ¨ +Ġreview ed +ãģĤ ãģĤ +Ġdo zens +Ġdeterm ination +ĠPh ill +Ġcontrib uted +ĠC it +Ġpass engers +Ġcôt é +Ġre ver +Ġtechn ological +Ġall en +Ġr aining +av i +Ġsal ty +Ġtyp ing +ĠÑĤ е +Ġt ilt +Ġì¹ ĺ +Ġо ÑĢ +ĠпÑĢ Ñıм +Ġr ou +Ġare na +ar at +åĪ « +HH HH +Ġmanufact urers +ĠEd ward +Ġt uck +Ġbl ows +ing o +ĠMar c +ìķĦ ìĦľ +M ich +ĠCle an +è ´ +est o +ĠP ack +Ġsha ft +BRUN O +Ġa ven +u ur +Ñģк олÑĮко +ê´ Ģ +Ġautom ated +Ġvent ure +Ġsurve illance +ĠG row +ĠE mer +Ġд оÑĢ +Ġinvest or +ĠY ok +Ġl atter +ĠN I +Ġfunction ing +ĠHam ilton +Ġ5 1 +Ġmurder ed +Ġanch or +Ġc uc +ĠSC P +ĠMad am +Ġconstra ints +Ġb arn +ank en +Ġë§İ ìĿĢ +ĠMot or +ĠDo ing +Ġam en +et ts +Ġinst ructor +eg t +ak o +Ġpost ure +iv ia +ĠPol ish +Ġдв а +Ġcolor ful +Ġel bow +Ġpar le +Ġpass er +Ġcond em +ort al +Ġfert il +ا د +ĠCol omb +Ġalign ment +Ġastron aut +ĠM ut +Ġsal mon +Ġstructure d +ŀ ר +Ġclick s +Ġm iej +æĶ ¿ +ãģĦ ãĤĦ +ĠR ound +Ġrain bow +ĠV A +ãģĶ ãģĸ +ì§ Ī +ot z +, </ +ĠNico le +l ishing +Ġwh ilst +Ġrep ublic +Ġtam am +vert ed +Ġrecogn izing +Ġгл ав +Ġd ub +ĠJ os +fall s +ich i +Ġcz ÄĻ +ĠÐ ¦ +ĠM itch +C R +cl ick +ãģĦ ãģ¦ +Ġstun ning +ĠJul ia +m ers +ĠPo ly +Ġdess a +Ġint é +Ġê³ łë +Ġdo ÄŁ +Ġdi ver +Ġstri king +ap hor +Ġap enas +ous es +Ġtraged y +ĠF an +ĠTurk ish +Ġpro phet +Ġdist ancing +ĠH em +Ġcart oon +K e +ant ing +ĠCl ark +ç ¿ +Ġdav on +Ġí ħ +Ġy ummy +Ġcomprom ise +Ġstart up +r itt +Ġcert ified +Ġpill ow +b ere +ì¤ Ģ +Ġsegu ir +Ġst adium +at ivo +Ġsimpl er +³ ¸ +Ġvis a +Ġpath way +Ġnue vo +Ġr ay +ãĥ IJ +é ľ +ö ÃŁ +Ġз ан +Ġcelebr ity +з а +Ġein es +ĠG iven +ĠA ra +ĠJ ob +Ġy ak +ĠAr beit +ress ing +á nd +Ġgrab bed +p end +Ġs ine +ir k +ĠÐŀ ÑĤ +ĠF le +ich en +ç ¦ +ĠNe il +èĻ Ł +Ġrepe ating +Ġdraw ings +r ise +Ġgl itter +f ive +Ġsur t +Ġsich er +Ġadjust ments +Ġ éĤ£ +ipp i +c ke +Ġrepresent atives +Ġmid st +Ġspo il +me ye +Ġtag s +Ġy ep +ĠStephan ie +Ġg ere +ĠR ud +ç ĭ +Ġgr os +Ġque ue +Ġacc ord +Ġorgan isation +end y +ĠTe xt +ü yor +ĠÃ Ń +Ġconc lus +Ġì¤ Ģë +Ġam p +ĠL ess +ĠëIJĺ ëĬĶ +c ano +ĠP ix +ap ed +Ġdar auf +u o +yn th +ab el +ĠD one +Ġd ick +ath on +Ġh ilar +ac co +ĠìĨ į +ĠO regon +ĠWe il +Ġmathemat ics +Ġal m +Ġpix els +ĠfrÃ¥ n +б о +F C +н Ñİ +he im +g os +ĠFor get +f end +ĠVo ilÃł +ĠG reet +Ġα ÏħÏĦ +Ġrec ur +æĶ ¶ +5 1 +ĠìŀĪ ê³ł +A t +Ġy ards +иÑĤ и +Ġoff set +ro lling +ĠÐŁ оÑģ +Ġen light +ĠP ad +lim ited +илÑĮ но +ĠS ara +ĠÑģдел аÑĤÑĮ +m art +ĠJ ump +Ġad orable +or se +che ering +Ġemp athy +ĠTon ight +or p +ĠHun ter +P oint +г а +Ġpass enger +ĠK night +Ġseem ingly +h uh +Ġthe atre +Ġto mb +Ġdep ressed +Ġsum mon +Ġsatisf action +do ors +ĠHou ston +аÑİ Ñī +ĠR io +г лÑı +Ġarr anged +Ġhand les +Ġtr illion +Ġnight mare +ĠQu ando +Ġo le +ĠGu ide +oo o +Ġb ile +Ġem pez +Ġ7 2 +cri bed +Ġpro gression +ĠLin ux +ë ¦¬ +Ġì²ĺ ìĿĮ +Ġfoss il +Ġqu ero +ìĨ ¡ +at iva +Ġpu zz +ĠZ us +ãĤ ª +Ġthr illed +ĠC B +Ġmin er +ÑĢа Ñī +ĠS AR +ĠN os +Ġго ÑĢод +Ġcam b +ĠÑĤ а +Ġresult ed +ĠD ick +ou ng +Ġcom ics +Ġabs olut +st an +dim ensional +Ġt ense +m us +ĠInt ell +ĠÑįÑĤ Ñĥ +Ġph ases +Ġvol ta +Ġv ão +b ound +ĠAnd erson +Ġcurios ity +Ġp ont +éĢĻ è£¡ +Ġdemonst rated +ol ine +ĠSpe ed +Ġm ama +Ġshock ing +Ġk iedy +Ġearthqu ake +Ġimpl ies +Ġent ers +ŀ Ģ +Ġelev ator +Ġdelight ed +ĠM itt +ĠB ased +ĠD ol +Ġk en +Ġworry ing +Ġfil ed +ail and +Ġм еÑĤ +Ġmas c +ĠÎ ij +ĠJul ie +Ġdim ensional +h uman +T ok +à ¿ +Ġun st +Ġse ule +Ġemb ar +Ġíķ ©ëĭĪëĭ¤ +ac ion +Ġì ī +Ġë¶Ģë ¶Ħ +Ġhe ated +â̦ â̦ +" ! +Ġreal ise +еÑĤ Ñĭ +ien ia +ie z +Ġf üh +ĠEs se +Ġp s +Ġd ó +as ters +Ġon s +P M +Ġret ro +m aker +wh en +Ġe lla +ĠL iving +ĠL am +Ġtr ong +Ġappro ve +Ġθ α +Ġs ung +ени Ñİ +ĠRem ove +è ne +ire n +Ġstr anger +и нÑĭ +Ġv æ +a fter +ot to +Ķë ¡ľ +ĠA hora +m ill +IS H +Ġgradu ating +k te +Ġre nov +Ġprocess ed +ke ys +ек о +Ġen rich +ĠÅŁ ek +Ġin sec +ĠN an +ca kes +Ġill usion +ĺë ¥¼ +Ġa irl +im s +Ġan ten +ữ ng +s n +Ġprec isa +기 ìŀIJ +ĠاÙĦ ع +Ġfore most +Ġparag raph +av ais +Ġв оÑģ +Ġman s +ÃŃ fic +b ot +Ġع ÙĨ +Ġbr oth +Ġaltern ate +ĠCh apter +Ġve ctors +es ar +Ġindic ation +ĠNe in +¶ ģ +Ġje ans +Y E +c ond +Ġun ited +ab i +ĠSer ge +Ġpart ially +Ġmac ro +æī į +å¼ µ +Ġeth ical +ru it +Ġshift ed +Ġc abe +Ġmathemat ical +Ġr ude +×Ļ ×ķת +ĠM erc +Ġgan ze +ic ion +Ġunc onscious +Ġbur nt +ĠÑĢ ÐµÐ± +íĬ ¸ë +Ġchar m +and al +ì² ľ +oth y +ĠH adi +Ġappreci ation +EN D +Ġré al +¶ Ħëĵ¤ +ĠN ag +ł¤ ê³ł +ĠLa uren +Ġv Ỽi +ĠBr idge +ĠU mm +ĠWe g +Ġcha que +ĠS oph +Ġg dzie +í ijľ +Ġst er +ĠB la +Ġreflect s +Ġbench mark +в аÑĤ +am ine +ãģ¡ ãĤĥ +Ġan h +Ġcontin ent +ĠF DA +ì ¡° +Ġê tes +×Ļ× IJ +å¼ Ģ +Ġblo ody +ĠN ine +iel t +em and +Ġë³´ ê³ł +Ġtid ak +ĠS cient +ple x +ost en +Ġanim ated +ass a +Ġder ived +ĠиÑģ ÑĤоÑĢ +ĠM ig +ìħ ĺ +Ġr os +pl us +os aur +Ġ ^ +Ġint ensive +Ġglob ally +Ġdif eren +ìĿ´ ê³ł +ä½ł çļĦ +Äħ d +Ġd és +Ġpresent ations +ĠC ro +Ġess es +ĠBet ween +P a +Ġn aw +à¸Ń à¸ĩ +Ġbre ed +icht e +ĠÐŀ ни +ĠBuild ing +Ġcon form +M O +ĠÐ ĸ +ĠK id +n as +ĠD ue +r és +Ġd iox +ĠB in +Ġtax i +Ġs ap +ĠH ub +çĤº ä»Ģ麼 +Ġcenter ed +Ġsur ge +Ġav ons +Ġle arnt +ĠY am +ĠDies e +ни ки +ĠBe ij +W ill +Ġattempt ed +Ġgr ief +ó j +Ġkid ney +Ġoppon ents +æĽ ´ +Ġn ome +5 7 +ÑıÑĤ но +Ġmid night +Ann ouncer +ac ity +on ed +Ġpued es +Ġproblem atic +Ġcop s +ĠP ete +r int +unt ed +Ġb ip +æ ¢ +ĠÃ Ģ +Ġc ens +ative ly +Ġ ä¸į +Ġur gent +Ġstrugg led +ach us +Ġmicrow ave +ĠS ide +ĠD enn +ĠÑı в +Ġur ge +Ġfor cing +w ang +ĠкоÑĤоÑĢ Ð°Ñı +Ġm amm +ĠðŁ İ +Ġtri bes +ĠSh adow +ĠS ang +ĠHit ler +Ġl un +Ġsc ent +ì§ ij +Ġoverwhel med +Ġbom bs +Ġcr imin +Ġconsol id +Ġmolec ular +×ķ× § +n or +Ġperce ived +Ġv é +Ġalt ogether +Ġor th +Ġve m +Ġz war +iz o +Å « +Ġmelt ed +ord en +ĠCharl otte +ĠEx cel +art a +ìľ ł +ĠG ew +Ġrom ance +ere mos +Ġcolon ial +Ġtradition ally +Ġqu an +ho o +Ġchampions hip +Ġarbit r +ìħ Ķ +Ġм ин +Ġself ish +Ġble w +ry ing +Ġoper ators +Ġjuris d +ı ħ +u ition +ĠE C +ĠAny body +v ate +iet ies +Ġanaly st +´ì ĹIJ +ĠвÑģ егда +ç ek +ĠK un +Ġag ing +Õ ¡ +ÑĢа ÑĦ +ĠMom ent +ĠH ua +è ĥ +th en +ел а +est one +Ġend e +Ġaward ed +Ġnäch sten +ĠSp ot +ĠN eg +Ġfair y +ä» £ +ĠCo ver +Ġdep osit +Ġstress ful +Ġj unk +Ġmet abol +J a +Ġê· Ģ +Ġundergrad uate +Ġcan cell +Ġcons ensus +Ġo so +éĩ ij +Ạ· +ÄŁ er +r ada +ĠPal ace +Ġped al +Ġex agger +Ġbehavior al +play er +ll es +Ġconnect or +Ġske pt +įĶë Ŀ¼ê³ł +Ġm itt +ĠH aha +Ġpe que +ĠG ott +f ang +à ° +j os +Ġkick ing +Ġmount ed +Ġrepl acing +v os +Ġquiet ly +Ġmil it +Ġown s +Ġnive au +Ġa ur +ĠBu y +Ġpredict ed +Ġc ows +Ġpon er +ĠD ri +Ġremark s +Ġrep orter +Ġark adaÅŁ +е ÑģÑĤи +Ġsa ves +Ġç oc +Ġmet aphor +ĠK el +st ation +semb ly +Ġadvis or +Ġworks hops +Ġaccount ing +Ġto k +n ier +in ner +Ġbur ada +ĠB B +ĠOlymp ic +ĠP ract +Chr ist +ĠÑģ Ñİ +Ġk as +Ġview ed +Ġmark ers +Ġf oto +get ic +ĠLuc as +Ġp ads +ĠJ oh +ĠCD U +aff en +are m +ĠBe ck +ĠG osh +sh it +ãģĮ ãģ¨ãģĨ +ĠM ater +abul ary +ĠRo om +ll en +ĠFollow ing +Ġdo it +ball s +ix a +Ġground s +ĠìŀĪ ëĬĶëį° +L S +Ġwild life +ĠS QL +Ġsh ifts +ä¸Ģ é»ŀ +B ook +Ġhost ed +ll or +Ġsn aps +Ġbes oin +Ġש ×Ķ +Ġpean ut +ä ft +¹ ł +ÅĽ l +Aud ience +ĠBarb ara +Ġadopt ion +Ġw olf +ĠоÑģ нов +ard a +Ġexp ose +Ġì ¦ +j as +Ä ĵ +Ġcount less +Ġì§ ģ +he alth +u ent +is o +ot ion +Ġhung er +Ġmo is +off s +Ġclaim ing +ĠÎ ļ +ĠBel g +Ġн ай +기ë ıĦ +Ġun pre +Ġg ed +ĠI o +ĠпоÑģ моÑĤÑĢ +Ġco ÅĽ +ĠN arrator +ĠÃĩ ok +íĻ © +à¸Ń ย +ci pl +Ġtim er +Ġdef ic +av in +Ġcateg or +Ġthr ows +ĠëĤ ľ +ĠпоÑģ лед +ĠTh ai +Ġmas cul +Ġbek ommen +Ġintern ation +ul se +Ġa ye +Ġpo i +Ġpix el +Chr is +Ġst ove +ο ι +Ġgener ator +Ġì» ¬ë +Ġacad em +Ġpract iced +Ġaqu est +Ġcontrib uting +ĠI g +Ġ ợ +Ġcont aining +Ġwrest ling +ĠÑĩ его +h aupt +Ġess as +vel ope +Ġexcept ional +Y U +ĠApp lause +ric ane +Ġconven ience +Ġдел аÑĤÑĮ +или ÑģÑĮ +ĠEn viron +8 5 +Ġc â +ĠìķĪëħķ íķĺìĦ¸ìļĶ +ĠM O +ĠP ope +Ġs ah +ob i +Ġmas ters +ain es +Ġbless ings +Ġo bey +Ġfl ux +Ġbr ow +Ġìĭ ¤ +Ġpopular ity +ĠL amb +ze ug +ìĻ Ķ +ıĦ ë¡Ŀ +itu ation +Ġaccom pan +Ġdial og +ĠJam ie +åĬł æ²¹ +Ġse wing +Ġble eding +Ġb ail +Ġthread s +od ge +ĠSh ang +Ġdeploy ment +ch ed +Ġsatisf y +Ġla z +Ġmiss ile +ĠLink ed +Ġmak ers +ci um +f re +Ġë¨ ¼ +Ġë¬ ´ë +ĠEd ge +Ġsociet ies +Ġag ua +Ġsyn chron +¡ ł +un ft +Ġun m +Ġtri ang +Ġin just +t op +Ġor al +k or +Ġíķ ¨ +ld igt +ce ÄŁ +qu et +ĠLe o +Ġsa voir +Ġeas tern +ie u +Ġexp ed +ĠС п +Ġunnecess ary +ĠPer form +ĠM ing +ĠÑĢ Ð°Ð² +Ġintent ions +Ġcomp ression +ĠS ac +ο λ +ars on +Ġtrou ve +ĠMuh ammad +ĠвÑĭ Ñģ +Ġfin ite +Ġна Ñħод +ug a +ÑĢаз Ñĥ +Ġcelebr ated +Ġconf ess +Ġsqu ares +ĠG ordon +ĠëĤĺ ìĺ +Ġsynd rome +Ġcomplet ion +Ġback ing +Ġdar f +ĠQ uran +Ġintermedi ate +Ġk er +Ġd ü +hes ive +Ġaccount ability +ĠRe becca +èij Ĺ +ĠS leep +Ġdiffé rent +ol s +ĠR ice +Ġë³ ¸ +Ġantib iot +ÏĦ ά +r z +amb ling +Ġsensit ivity +Ġch ron +all as +6 4 +Ġfle et +Ġoptim istic +Ñģк ого +Ġj adi +a illeurs +ĠEn ough +Ġsen in +Ġpack s +b n +ĠAre a +ĠT ro +¨ë ¦¬ +а ÑĶ +ĠTh om +Ġharm ony +ни ка +Ġsom eday +IS E +ĠBroad way +la res +ern ess +à¹Ħ ม +ĠT enn +ĠNAT O +ãĤĬ ãģ¾ãģĻ +Ġminut os +ĠK ansas +ĠM ong +Ġcompt e +åĽ Ľ +Ĭ ¤ +ĠìĹ Ń +Ġsuper hero +ĠGard en +ĠM os +Ġattach ment +Ġb ust +௠Ĭ +ĠTh ailand +st at +Ġsp ice +ĠLe b +Ġle ap +ze ch +G L +Ġver l +Ġfix ing +Ġë³´ë ©´ +Ġpor n +Ġb üy +ĠÙħ ا +ĠV irt +ĠT ommy +Ġcar go +ĠOl ha +Ġro ku +Ùĥ ÙĨ +Ġbak ed +Ġtact ics +Ġmarket place +Ġktó ra +ar lo +Ġswitch es +Ġc ache +ĠH R +ĠG an +ĠG PS +Ġdu as +her es +еÑĢ ÑĪ +tr ack +Ġl ungs +St ation +igg les +Ġcamp ing +åĵ ĩ +Ġcomplet ing +am as +Ġcy cl +Ġprot otype +ĠJud ge +oty pes +Ġinfect ions +ł ¤ë +еÑĢ Ð³ +ob a +ĠB od +ĠSecond ly +Ġap ost +Ġso gar +Ġre ass +ie k +æĸ ¼ +Ġash amed +Ġcur ves +Ġв аж +Ġense mble +at ur +Ġphot ographer +Ġeight h +Ġwas ted +ç® Ĺ +Ġd amp +Ġм ал +are na +Ġintern ally +Ġhe els +ĠS alt +Ġbl ir +Īë Ĥĺ +Ġcontr ary +Ġprim a +Ġos s +Ġrab bit +Ġaut or +Ġbroad ly +ÃŃ st +Ġback s +íĶ Ħ +et o +Ġj ury +è ± +Ġprost u +Ġbar a +Ġpar liament +or ient +ил аÑģÑĮ +Ġind irect +á m +ĠÃ¥ r +Ġtra its +Ġd ÃŃas +ÙĦ Ùħ +ĠC T +aly st +Ġli vest +Ġk os +M ay +ĠJ ing +Ġjournal ists +Ñĩ ик +ar ms +Ġê°IJ ìĤ¬ +Ġим е +Ġé gal +ĠNew ton +Ġrecover ed +Ġbra uchen +ĠBr on +а но +Ġp ale +pr ises +Ġhor as +ch ts +éĢ ļ +ÿ ÿ +ak ers +ĠAl aska +zie j +Ġsc oop +ìĿ´ ê°Ģ +ãģķ ãģĦ +c or +él é +Ġsur g +Ġv iene +ĠKr ist +5 4 +Ġb anned +Ġsmooth ly +Ġtreat s +Ġpron ounce +Ġfl ush +Ġcam bi +Ġmusic ian +ĠAsh ley +ĠSP D +ĠBob by +Ġgl oss +res pect +Ġreview ing +Ġgener ic +Æ°á»Ľ c +ats ächlich +Ġhealth ier +ub ers +Ġд ан +ĠMedic are +5 3 +Ġcomplain ts +j ac +Ġagric ultural +S pe +ĠJ ong +Ġdiox ide +ê² ¨ +el ijk +ĠSh it +ain ts +ĠI an +ĠSim ply +ĠSt re +æľ ĭ +ĠG DP +5 9 +as z +ĠKat ie +Ġб ÑĢ +Ġpe ek +owy ch +Ġres ort +Ġres idence +Ġsp ices +ci ó +Ġjed er +Ġem o +ar ium +Ġp uff +ë§ ī +ÑĥлÑĮ ÑĤ +Ġmet a +Ġìł Ħë +Ġoptim ization +g ang +Ġíķ Ħ +Ġefficient ly +Ġvis ually +Ġfr ost +ĠAr thur +Ġ ż +Ġachie ving +Ġrot ating +Ġl ining +Ġoccup ied +å¼ Ł +ment ation +Ġstretch ing +Ġst all +ost ic +ĠS ever +Ġgl uc +Ġró ż +Ġout reach +st ra +ik en +Ġìĸĺ 기 +ĠJo in +Ġim pe +Ġcompens ation +ĠT at +ĠCar los +ühr t +ĠFranc is +c ji +ye ah +Ġmembr ane +Ġex hale +Ġrel i +ĠO R +Ġrefriger ator +ĠV enez +L ike +Ġrais es +ott le +at ura +Ġrul er +Ġwe er +Ġgu ided +ĠM agn +ĠCor por +į Ķ +Ġattrib ute +ĠWo ah +Ġarr ows +Ġaw ait +ĠPr im +Ġdign ity +ĠOnt ario +isch er +Ġìĭ Ŀ +im en +ou ver +AS S +á»ĩ n +op y +achus etts +Ġelder ly +åİ Ł +F A +ĠDa ily +sh ine +Ġ5 6 +è ¢ +ier no +Ġskill ed +Ġgro ÃŁe +ĠO ak +第 äºĮ +igg le +ел ей +Ġbir az +Ġargu ing +Ġпо ÑįÑĤомÑĥ +Ġdr ift +Ġh arness +Ġdeix ar +aut re +ĠSee ing +Ġcapital ism +ĠE ld +z ione +ĠBe yond +Ġperfect ion +Ġho e +Ġdecl are +ал аÑģÑĮ +Ġpo ke +Ġ× ¡ +Ġfight ers +ê²ł ëĭ¤ +оÑĢ Ð¾Ð² +Ġaccording ly +ĠIs a +Ġoptim ize +ĠMin istry +Ġsa ge +ìĭľë ©´ +Ġben i +Ġdon ation +Ġcle ared +ĠLuck ily +Ġharm ful +µ 커 +Ġc ement +п иÑģ +Ġded i +ĠCra ig +Ġdem ons +Ġcustom ize +Ġign ored +ĠT ian +Ġhop ed +ĠB ureau +Ġr i +ĠY ah +Ġso cket +Ġfeat uring +Ġpar f +ĠT E +ĠTe acher +Ġcatal og +ê°Ģ ì§Ģê³ł +ĠSe ite +Ġcon e +ĠPalest in +Ġgew oon +Ġg aining +ĠØ ¢ +Ġcat ast +Ġneighb our +IS T +Ġste aling +Ġtro is +Ġint end +ĠSh oot +Ġp ione +ĠInt el +ĠL IN +Ġbright er +ĠY esterday +Ġs ow +s in +od s +Ġeth ics +Ġinterview ed +re ll +Ġrefres hing +s Ã¥ +Ġabs urd +Ġph osph +f il +Ġste hen +v als +Ġcare d +æĪ ĸ +Ġde ll +b one +Ġho ch +Ġp up +Ġi o +Ġaconte ce +ell es +ĠS pl +ig i +Ġt än +Ġele phant +Ġg ates +Ġslic es +Ġpr ank +ok rat +Ġhilar ious +ĠS id +ĠëĴ ¤ +Ġess ere +Ġtele phone +in ally +r ator +Ġhelicop ter +ĠiÅŁ te +Ġg id +Ġtour ist +Ġconflict s +аÑĤ а +Ġt é +Ġass ert +Ġlaund ry +ĠB om +Ġspecial ized +ĠMod ern +og raf +Ġan o +Ġret rie +ĠPut in +ĠH AR +Ġм аÑĪ +Ġα ÏĢÏĮ +Ġtut ti +Ġв ÑĤоÑĢ +ìĸ µ +ĠB ul +ëĭ¤ë ©´ +ÅĤ e +æľĭ åıĭ +ar in +Ġtherap ist +Ġg Ã¥r +ĠC zy +pp e +m ir +ĠT erm +ĠBe ar +l ace +ĠMore over +ĠDis c +Ġíĥ Ģ +Ġtit led +Ġstri ps +ĠF ahr +ĠR ing +rand o +af a +èº « +Ġshort s +Ġtr unk +Ġsent ido +Ïī ν +Ġac res +Ġover d +ĠOlymp ics +åı « +ĠMer ci +ĠëĤĺ ìĺ¤ +Ġg erm +am med +Ġpre gunt +ĠN ut +Ġ< / +Ġtravel s +Ġvoc abulary +et en +od er +Ġconsum ing +wr iting +è¶ ħ +Ġappe aring +Ġadjust ed +se m +Ġf rente +Ġmaxim ize +Ġzw ischen +Ġz am +c onscious +z ek +è°¢ è°¢ +ha o +ì²ĺë Ł¼ +ĠEp isode +Ġvis ibility +Ġm ijn +Ġviel en +ĠBr others +×Ļ× ij +Ġvä ldigt +Ġcrush ed +uf en +ä½ł åĢij +act ic +ĠB ed +ĠF A +iss ippi +Ġrem ot +Ġp ets +Ġth under +ĠM am +ìķ µì»¤ +p arents +Ġb ı +Ġsurt out +Ġseg ments +Ġne hmen +Ġutil iz +ĠRub y +Ġr á»ĵi +Ġhapp ily +Ġbus h +ult an +çİ © +Ø ¸ +ĠH il +Ġla wn +Ġeyebr ows +me z +ĠSy d +re p +in f +éł Ń +Ġover head +cz nie +Ġox id +ĠW ol +Ġdestro ying +ĠAdd itionally +umb led +d ep +Ġdep os +Ġcomm od +Ġc akes +Ġtal ents +Ġpour quoi +Ġcont empl +n els +о Ñī +ĠArab ic +ĠMary land +çİ ĭ +ow o +ĠP la +ÄŁl um +Ġprop he +ĠRep resent +op ol +acc ord +ĠMe aning +Ġjoint s +Ġbra kes +ck t +Ġ199 9 +Ġpublic ation +ĠRe view +ой д +Ġn iche +Ġsignific a +Ġde br +Ġoverl ap +Ġdemand ing +ĠS ó +Ġsubsequ ent +Ġquot es +ĠCurrent ly +Ġprevent ing +Ġ1 30 +ĠC el +on n +wnie ż +ìķ ½ +Ġкак ие +AC H +Ġg um +ĠIsrael i +ìľ¼ ëĭĪê¹Į +å ¨ +ru kt +Ġcl apping +ĠMass achusetts +Ġresil ience +Ġsubscri bing +Ġjewel ry +ge bra +Ġcor rection +bo o +Ø ¦ +l io +s am +Ġen velope +k al +ĠF arm +Ġc attle +Ġbr as +Ġrep ent +Ġt ones +os ion +pe ction +Ġden en +È Ľi +ĠMar g +Ġacqu ire +ibl ings +Ġas pir +Ġs ized +Ġal c +Ġvib ration +t il +em in +Ġcorrel ation +Ġsing ular +Ġпо Ñıв +re k +Ġchap ters +mb re +Ġaud ition +ç as +Ġv amp +Ġt es +ĠÑĢаз в +Ġrespect ed +c in +Ġfuck in +Ġüber haupt +Ġп об +Ġal ike +¶ Ī +ro bi +î t +ĠT ouch +an za +Ġfirm ly +ĠGreet ings +sc ale +d ad +ак ÑĤи +Ġback yard +ож д +G r +ĠST E +оÑĢ ÑĤ +Ġhät te +ĠFirst ly +ĠO ften +as ures +Ġdraw s +red it +AT E +P e +C P +Ġcompe lling +Ġsubs id +Ġneighborhood s +Ġdipl om +Ġent ender +per ing +a ug +ch at +ÐĿ Ñĥ +ĠDo ll +Ġìł IJ +Ġh ose +n ar +Ġreward ing +ĠSo ld +Ġtak i +Ġbl ades +ĠK ath +Ġjog o +Ġsens ation +u ana +p el +ĠRecent ly +Ġpoly mer +ĠU P +-- - +Ġho ver +Ġrul ed +æµ · +Ġ×Ķ× IJ× +Ġaffect ion +ĠÄij á»ĥ +Ġb ree +ç§ ģ +ĠL ay +ĠY ong +Ġrece iver +ľë ¥¼ +Ġdis so +ĠQ ing +Ġé v +Ġm úsica +Ġaest hetic +ĠB reat +ĠT A +Ġaccur ately +? âĢĭ +Ġw ages +rawd ÄĻ +Ġsw allow +Ġcompl aint +Ġli ed +bec ue +Ġrelax ing +ĠPok émon +Ġte cn +b ang +³ ´ì +Ġqu ien +н омÑĥ +Ġhabit at +.... .. +ab ling +ĠÑĤак ие +Ġbes ond +Ġemploy ed +Ġarri ves +Ġvess els +ĠA x +Ġdisplay s +1 50 +olog ie +ĠìĹ IJ +Ġcl o +Ġд ов +ĠÐŀ д +Ġv uel +èĬ ± +w end +Ġsl ipp +ur p +ĠL ot +Ġbull ets +Ġr age +Ġsk irt +ient es +Ġnh ững +ĠNat ural +Ġh ind +Ġwork load +m u +íĥ ľ +Ġsun set +в ол +p it +åį ģ +ĠAS H +Ġë¶ Ħëĵ¤ +Ġdown stairs +é Ń +Ġcount ed +Ġn az +×ķ× ¤ +ĠPhilipp ines +Ġ11 0 +ĠPark er +Ġg itu +Ġinter es +Ġum bre +ĠN ature +Ġj er +en os +Ġpanel ists +Ġco ating +Ġch erry +ĠP ent +ĠM ist +reg ation +Ġv ind +ĠCor ps +ĠM ission +Ġno ble +Ġfon ction +Ġwarri or +Ġprot ests +our i +Ġconstitution al +ÅĤ am +Ġemer ged +Ġd ye +ĠTry ing +ig m +ä¸Ģ 个 +é qu +L O +ĠV erm +er ving +ĠT IM +ĠC i +Ġfree zer +Ġgrup o +ĠSp orts +ĠпÑĢ Ð¾Ð³ +ĠÙĦ ا +other ap +iff any +b ian +Ġrank ed +Ġpropos als +ĠÄij ây +Ġfree zing +Ġinsect s +v il +Ġcomp ost +çİ ° +Ġse mana +Ġdistingu ish +Ġfacil itate +Ġplus ieurs +Ġver g +Ġalgun s +ĠTik Tok +ĠEx press +м енÑĤ +S U +Ġint imate +ĠAut hor +Ġwitness es +Ġkal au +Ġargu ed +Ġavo iding +ct ive +Ġpurs uing +Ġsy ll +á vel +ĠAtl anta +ĠUt ah +ĠT ill +Ġer f +Ġ20 22 +ä ter +Ġfun eral +ĠFl ash +ĠAtl antic +Ġge le +ì¦ Ī +Ġmort gage +ĠëĦ ĺ +lic ht +Ġamb itious +ĠBeij ing +Ġd iving +Ġun box +ill as +Ġot ras +Ġev ac +Ġmar ine +ĠÑģоз д +ĠCre ate +Ġg j +Ġfrequ encies +ing ton +ĠRom ans +Ġaim ing +ĠB uff +Ġem peror +ĠMo i +Ġprom ising +ãģ ľ +Ġalg uma +Ġpas a +Ġdis orders +S I +Ġsucceed ed +Ġcuer po +Ġsod ium +Ġst ub +he iro +Ġdelay ed +et era +t w +Ġsyn c +h d +Ġtour ists +Ġsy st +Ġm ét +Ġqual ify +ĠO thers +ll ers +аÑĤелÑĮ но +ĠÐŀ на +Ġperce ive +Ġê² Ģ +Ġê°Ģ ìŀ¥ +Ġи Ñģк +ĠM atter +ĠBl uetooth +Ġpe arl +Ġar ise +Ġmon ument +Ġим енно +ag i +ÙĦ ÙĬ +Ġr ho +Ġsm arter +Ġcon j +ок а +Ġke en +ĠT reat +к лÑİÑĩ +Ġpack et +els ius +ĠAl ab +и ни +Ġp si +Ġenjoy able +ĠEll en +Ġв м +Ġelimin ated +ĠR ow +Ġzomb ie +ĠK u +Ġphr ases +Ġg ren +ut er +Ġdire kt +× ĸ +en en +us a +ĠÑģл ов +Ä ° +ĠG h +Ġcor rid +Ġque er +ĠL inda +Ġon a +Ġoblig ation +d ar +ĠØ µ +em ment +ac ies +Ġscrew ed +Ġn ak +Ġay ud +ä¸ Ķ +á r +le z +Ġdr own +ĠMedic ine +Ġlab s +Ġjus qu +ĠG onna +Ġterror ist +qu est +Ġfar ther +Ġrepl ied +ĠS W +ĠMiss issippi +ish na +Ġhold er +Ġre ign +Ġaccept ance +Ġu l +¶ Į +ĠHot el +ĠCo oper +t an +ĠG rab +Ġv apor +Ġact ed +ĠK ang +f an +ĠìĿ´ì ĥģ +çĶļ 麼 +ut et +Ġword t +Ġfar ms +d at +Ġcou ples +Ġbe ads +ient os +Th en +ä¿ Ĥ +os ity +ĠStan ford +. - +W ait +Ġdat as +o ire +Ġhasht ag +im me +Ġencounter ed +Ġshout ing +Ġresist ant +ĠSe ung +Ġtra gic +ĠD raw +, , +Ġshow case +ĠA F +ĠSt ri +Ġback ed +ĠÑĥ г +ĠбÑĥд ÑĥÑĤ +ĠCo le +e urs +( ?) +Ġesca ped +AS T +ĠAs sembly +Ġstick er +Ġmie ux +Ġentertain ing +ĠD ON +ĠAm end +ĠK arl +Ġin hib +s st +ie g +~~ ~ +Ġhook ed +Ġliter al +Ġsun ny +st eps +Ġë° ľë +ĠMar ine +Ġsu e +Ġprison ers +ĠE b +5 8 +Ġdr ums +Ġgu ilt +al g +Ġhapp ier +ĠC M +ĠìķĦëĭĪ ìķ¼ +ĠÐŁ еÑĢ +Ñĥ лÑı +Ġkey word +ĠPar ce +ĠFore ign +ĠAm anda +ç¥ ŀ +Ġëª © +pl ess +Ī ¬ +ó mo +Ġqual quer +ìĿ´ë Ŀ¼ê³ł +Ġconspir acy +Ġstraw berry +Ġhat ten +E s +Ġsp os +Ġvill ages +Ġle v +ĠÑģ ÑĢед +Ġw aking +Ġcalcul ations +ĠÙħ ع +Ġpour ing +Ġleb ih +Ġpol ish +ĠT out +Ġfun ktion +м о +ĠT i +Ġwas ting +ist ically +Ġmanip ulate +Ġsimpl ify +Ġteam mates +Ġб о +Ġcont am +ĠQu ite +Ġkur z +ĠC and +ty pe +outhe ast +Ġfinan cially +ол н +els on +Ġfore head +u age +na udible +ĠBeh ind +Ġnegoti ations +Ġë§Ī ìĿĮ +Ġaltern atives +r ank +hold er +æĩ ī +Ġhe aled +ÑĤо Ñĩ +ĠSpe c +ä» ¶ +ä»ĸ åĢij +Ġexhib it +Ġshall ow +Ġgo b +Ġë ľ +Ġfrust ration +ÃŃ o +Ġmel ting +ĠSt orm +Ġpat ent +ĠBar cel +Ġped est +ÙĪ Ùħ +Ġt ai +ĠM ode +Ġw il +Ġëª¨ë ¥´ +Ġégal ement +éĤ£ 麼 +Ġ×IJ× Ĺ +ay an +Ġam azed +ì§Ģ ëĬĶ +Ġha ciendo +ĠìĿ´ì ķ¼ +λ α +ภĤ +еÑĤ а +Ġexam s +Ġtrave lling +P ress +и ÑĢÑĥ +Ġbas eline +Ġbus es +Ġrein for +ven ant +ĠTr uth +Ŀ ½ +o be +Ġye ll +Ġsaus age +T F +ĠEv il +Ġme iner +×Ļ× § +Ġhope ful +Ġró wnież +ĠPer ò +t wo +nd er +Ġм иÑĢ +Ġcons cience +ĠWar ren +ick y +Ġaim ed +Ġgö ra +X T +Ġpy ram +R ed +éĽ » +at u +ĠEst a +Ġearning s +Ġh ats +ĠSt adt +ick et +point s +in ander +Ġmotor cycle +Ġëı Į +Ġíķ´ì ķ¼ +k om +ĠD ing +æ Ĵ +Ġrec urs +Ġestim ates +Ġder ni +Ġvers ch +ãģĿ ãģ® +ĠM IC +ив аÑĤÑĮ +ĠпÑĢо ÑĪ +Ġd ost +Ġв ÑģÑĤÑĢ +Ġw iel +Ġs iblings +Ġд ев +Ġear liest +Ġfat igue +Ġn hi +Ġgust a +Ġbon ne +æľĢ å¾Į +f rom +ĠJen ny +Ġsupposed ly +int age +Ġcount ies +Ġun re +Ġplant ing +ĠGr ac +ĠGen esis +ĠAl pha +ys z +Ġt ile +Ġê²½ ìļ° +Ġ×Ļ ×© +qu el +Ġdistrib ute +de f +é ral +Ġcl utch +adel ph +ĠPlay Station +Ħ ¸ +Ġs j +bre aking +ĠëIJ ĺë +ĠC uba +ĠRuss ians +ĠMAR K +Ġper se +Ġrestrict ed +ig es +ĠTra vel +Ġelectron ics +Ġquar ters +ĠKe ith +s ized +Ġdead line +aren th +ĠvÃŃde os +Ġprotocol s +am ment +ĠTra ining +Ġà ¢ +Ġsequ el +н ак +Ġke inen +Ġmatt ress +lud ing +Ġclass ified +Ġreact or +ĠK ont +Ġpass ar +Ġhon our +or ig +IN A +ĠN athan +в а +ĠÑģказ аÑĤÑĮ +t ır +Ġexclus ively +Ġsh ades +ĠпÑĢо ÑĨ +Ġoccas ions +ij a +çļĦ æĻĤåĢĻ +åİ ² +æħ ¢ +f ig +Ġt us +Ġrem em +ĠChrist opher +Ġsl ime +Ġalg una +ĠF ortunately +Ġl ors +v oll +a ver +Ġout let +ĠLinked In +ĠExec utive +Ġorg ans +ĠBe gin +ĠíĻ Ķ +Ġtrans plant +ra gen +V O +ĠF ör +Ġب اÙĦ +ĠAnd re +is ine +Ġlast s +Ġhist ória +Ġl uz +Ġcoll ar +Ġkid na +Ġopt ical +io v +Ġto b +Ġex terior +Ġmet ric +ie ur +Ġt roll +ĠÑĢ Ð¾Ð· +æĺ Ł +Ġt ô +ĠìĺĪ ìģ +ĠGes etz +Ġе д +Ġdenomin ator +ì ³ +Ġlet t +åħ ī +Ġgr Ã¶ÃŁ +é¡ ĺ +ĠL uther +Ġrest e +Ġrese mb +Ġperm et +ks i +Ġf isher +ãģŁ ãģĦ +ĠV on +íĶ ¼ +ĠÏĥ ÏĦο +Ġlo cks +Ġsho ots +Ġkam u +ĠK er +ĠO bs +çĿ Ģ +Ġb ili +Ġë° ± +Ġtort ure +ass y +Ġи г +Ġlast ing +好 çļĦ +Ġtien es +Ġrece ives +ĠOsc ar +Ġremember ing +Ġproblem as +Ġ ia +åĺ Ľ +Ġmemor able +Ġjour s +Ġfa çon +am ic +Ġë´ ¤ +at ique +ĠëŃ Ķê°Ģ +Ġz ip +h alt +ĠðŁ ĺ +Ġfr ies +Ġfind en +g ra +ÑĢÑĥ д +im port +Ġëĭ ¬ë +Ġi ki +Ġcompl aining +Ġfaz endo +Ġgo ogle +Ġtab s +Ġëĵ¤ ìĸ´ì +ãĤ ¦ +ug o +ier to +au fen +Ġ먼 ìłĢ +Ġskull e +Ġsu iv +Ġsp y +ĠK ai +éĤ£ åĢĭ +Ġmart ial +Ġon der +èª ° +at ility +Ġirgend wie +Ġcl ap +int ell +Ġinstall ing +Ġun iqu +ĠCent re +ast s +u ar +Ġrev is +Ġthreat ening +ra is +Ġcu id +s ka +Ġresol ved +Ġr ides +Ġfail ures +Ġse mb +Ġmal es +U FF +å¾Ī å¤ļ +Ġtr ês +app ed +Ġnewsp apers +ri et +Ġapplaud s +Ð ĵ +Ġãģ ¯ +ĠN C +åį ĥ +æĻĤ éĸĵ +Ġh eter +Ġhaz ard +Ġr y +Ġstrict ly +Ġ5 4 +Ġëĵ¤ìĸ´ ê°Ģ +Ġsp ont +Ġt atsächlich +Ġë§IJ ìĶ +la ub +Ġabsor bed +acaÄŁ ız +Ġon u +ĠÐIJ н +Ġexplicit ly +Ġìŀ ¬ +ĠFut ure +acht en +Ãł o +y on +Ġser ia +ĠHer ren +ce j +ĠAl bert +ìĿ´ ëĬĶ +ect or +Ġpack ing +Ġvirt ue +Ġven ir +D D +Ġy az +Ġlog s +ĠPhot oshop +Ġs id +l ings +Ġremot ely +ĠD ifferent +Ġoper ated +light s +Ġdisc rimin +ist ance +ĠG RE +Ġpl ac +Ġsh irts +Ġjust ify +Ġtrabal ho +ut il +v oc +Ġqu art +ĠÎ ¤ +S C +ĠS R +Ġ- " +Ġhes itate +Ġp ak +èĩ ³ +gu a +J o +Ġsou vent +ĠAng ela +esse e +adelph ia +ark s +Ġwe ed +Ġkann st +åĤ Ļ +Ġê·¸ëŁ¬ ëĭĪê¹Į +Ġplut ôt +ĠComm ander +Ġsummar ize +à¯ Ģ +Ġ9 8 +ãģ ĩ +Ġdevelop ments +ĠC ost +Ġtheoret ical +Ġo re +Ġmet all +οÏħ ν +f ahr +Ðļ ÐIJ +Ġch uck +Ġadapt ed +ĠOk lah +ĠNether lands +Ġpo et +st o +k at +Ġwe ars +ç ¯ +Ġìĸ´ë ĶĶ +ĠEst o +Ġlaugh ed +Ġdon ner +Ġë į° +ĠìĽ IJë +oc ur +ĠK ick +ĠDet roit +Ġbicy cle +Ġlack ing +ph abet +ĠK end +A ss +Ġreve als +ĠÎ ł +ĠNo ah +¦¬ ëĬĶ +Ġsell s +ĠAlab ama +Ġterr ific +ĠE lement +Ġí Ĩ +Ġtur bo +ĠH om +Ġtheore m +Ġadvent ures +Ġpurch asing +ĠT á +Ġм аÑĤ +Ġve mos +Ġd uties +Ġwen ig +Ġbo oth +Ġent rar +V A +Ġge ars +ĠJa e +è n +Ġcal cium +ĠRober ts +ĠпÑĢоб лем +Ġrib bon +Ġн азÑĭв +Ġla v +Ġinter ventions +ĠUlt ra +Ġnam ely +Ġadequ ate +Ġre cap +Ġdo ck +f ting +Ġvo i +Ġconsult ation +ĠÑģ ем +Ġpod em +Ġposs ession +Ġcl ues +ĠRus sell +Ġrenew able +åݲ 害 +ĠÑĥ з +in formation +igg ers +W ith +wn o +Ġelabor ate +ctor al +ĠD ow +Ġram en +æı IJ +á» ķ +Ġer ste +ĠZ el +ãĥ Ĺ +Ġqu asi +Ġн ак +ç§ Ĵ +ĠSt ars +Ġtri bal +Ġse ated +Ġw ol +Ġch ol +äm ä +Ġout break +Ġc res +Ġunser er +Ġí ijľ +Ġunder water +Ġass ure +OO D +Ġnap rawdÄĻ +Ġestablish ment +Ġinc on +Ġdifer ente +Ġex cus +ĠD im +о Ñħ +ĠL ing +ro log +Ġãģ ¾ +Ġout doors +na j +Ġepid emic +Ġun ters +Ġ3 000 +ĠGab riel +ĠìĹĨ ëĬĶ +Ġenc l +ĠO der +ĠF oot +p as +ĠZ uk +åĵ ¡ +Ġwork flow +Ġun p +Ġall iance +ens chaft +Ġyog urt +ин е +Ġer u +Ġf iz +äº Ķ +Ġa ÅŁ +Ġap rend +Ġcual quier +Ġcarr ots +ın ın +af ood +Ġflo ors +Ġkey words +Ġsp otted +Ġdr ank +Ġpar as +Ġúlt imo +Ġhab lar +Ġprose cut +ìĹIJ ëıĦ +éĸĭ å§ĭ +Ġé p +Ġstick ers +Ġpush es +k h +Ġrest art +ĠTh under +á» Ŀi +Ġmuit a +Ġfo x +arde ÅŁ +ĠZ ach +ĠMine craft +ç ¸ +Ġ== == +Ġgö re +Ġst ance +ig ung +Ùİ Ùij +k ä +Ġteaching s +é Ĩ +Ġdec ay +Ġr ic +om ena +ĠвÑģ ем +ch ten +ĠV ert +Ġíķľ êµŃ +¬ ´ë +Ġco c +: ) +ke iten +ĠB A +eth eless +Ġhead quarters +Ġsp ike +ĠB ase +Ġ10 1 +Ġcoordin ates +Ġt ard +Ġbo iled +ĠMon ster +Ġnote book +Ġê´ Ģ +ĠW ake +ĠSet ting +ìĿ´ì Ĺ +ĠSyd ney +ĠFin n +Ġlob by +å¾ ŀ +Ġsen iors +ни Ñħ +av an +ĠJ E +Ġtra ff +th ink +Ġsl ap +ĠCast le +© ng +Ġalgun os +ĠPerson ally +ĠM ale +íĭ ° +ĠGener ally +ĠP el +Ġdi as +Ġevol ving +it ol +в оÑĢ +Ġple in +Ġfl ights +Ġele ven +owe j +á»ij ng +Ġa ku +Ġgl ance +Ġconnect ivity +Ġbal d +Ñĭ Ñĩ +Ġint est +á g +ĠGR Ãľ +ibl ical +ĠP apa +Ġp ity +Ġf aint +Ġwur den +Ġleg ally +Ġpre y +ĠSci ences +ĠпÑĢ Ð¾Ñģ +Ġtrain er +Ġprobl ème +Ġkil o +к ого +Ġbrid ges +8 9 +Ġlast ed +Ġeleg ant +b ows +Ġpal ab +Ġdirect ory +ä¸į æľĥ +Ġbul b +pe ople +I X +Ġge b +Ġ6 6 +ĠTenn essee +ah len +ie val +Ġca ut +ĠDam en +pl o +ian e +ал е +att an +ĠاÙĦ س +Ġrisk y +Ġslee ve +Ġinc idents +Ġë° ķ +C o +Ġapplic able +Ġimper ial +ĠPhil ip +ĠYe a +еÑĢ Ð¾ +Ġпок аз +ü ne +ìĺ Ģ +H ub +t or +Ġsig u +c end +Ġpolit ically +ĠìĤ ´ +Ġp ars +Ġou v +Ġprime ira +ĠSh ah +Ġsat ur +Ġcomb ust +Ġpromot ed +ì£ ¼ë +æĢ ķ +Ġtempl ates +Ġëĭ ¬ +Ġha ul +ĠÑĤ еÑĢ +Ġsl iding +ced ented +Ġãģ ® +child ren +M R +ĠWe i +Ġb ör +æĹ © +Ġpróxim o +ar ÃŃa +Ġsam pling +ел ен +es i +ĠDan ielle +ĠOklah oma +è ħ +çķ Į +еÑģ п +ĠDV D +ĠвÑĭ п +r ous +c ons +Ġenhan ced +éĽ £ +Ġpast or +ĠSud denly +è® ĵ +f ar +P ER +ĠN g +1 000 +Ġche w +Ġrum ors +ĠA na +Ġann ées +ĠÑĥ ÑģÑĤ +ĠPhil adelphia +åĹ ¯ +еж дÑĥ +Ġeffect iveness +è¿Ļ æł· +ét é +Ġd ing +Ġrelig ions +Ġag ed +zie Äĩ +ĠR ic +ĠK ap +ĠP age +Ġs ü +Ġnäm lich +Ġman kind +Ġrest ing +Ġinflu ences +ĠSch ul +Ġн ев +Ġman a +Ġconsum ed +ĠP om +ç¾İ åľĭ +Ġconsegu ir +ĠThanks giving +ĠHind u +la is +Ġth rive +Ġcont our +аÑĨи Ñı +Ġfal ando +ĠJ á +z an +иÑĤ Ñĥ +ip her +j amin +ĠHall o +Ġ16 0 +ĠоÑģ об +Ġmet e +Ġìķ Įë +ĠBarcel ona +let ter +ĠÐĿ еÑĤ +å Ļ +Ġad emás +Ġcoord ination +un ts +Ġsl op +ĠпÑĢ Ð¸Ð´ +ì§Ģ ë§ī +Ġquestion ing +Ġdies el +Ġde j +Ġaff irm +įĶëĿ¼ê³ł ìļĶ +ien ne +Ġcr ank +Ġpredict ions +Ġphys i +ch sel +Ġcomb inations +Ġex cellence +éĢĻ éº¼ +á» Ŀ +wid th +we ed +Ħë ¥¼ +Ħë §Ī +Ġal to +Ġda iry +ĠNorm al +pp en +Ġob en +Ġdevast ating +Ġpo z +ĠH us +m az +Ġwarn ed +Ġden k +ĠA uss +Ġtrad es +he ll +Ġprim ero +Ġm ia +в аÑĢ +ب ÙĬ +Ġkick s +Ġa ÄŁ +ĠM ü +Ġl uc +ени ем +ĠStand ard +r ice +ĠC ub +Ġg ou +ĠJo ão +Ñĥ Ñģк +Ġen qu +£ Į +ge w +Ġíģ ° +ow ania +ian i +Ġf akt +Ñı ни +Ġbe f +Ġthumb na +Ġce ux +æŃ¡ è¿İ +app le +N EN +Ġg ad +ap on +ĠFant astic +Ġconcent rated +g irl +l ene +ĠÐĶ Ð»Ñı +Ġé ta +a an +Ġout ta +Ġnar c +ĠB ody +br ush +Ġlegisl ative +ĠMeg an +Ġmist aken +ĠMiss ouri +Ġlabel ed +лÑı еÑĤÑģÑı +Ġreal ised +y orsun +ãģĤãĤĬ ãģĮãģ¨ãģĨ +ĠSaf ety +Ġacceler ate +Ġsan ctions +Ġpe e +Ġj uego +Ġpe ppers +Ġw al +ê¸ ī +ell ow +Ġж ен +Ġcin co +ĠÑģ иÑģÑĤ +co very +Ġgr am +Ġé po +ĠBM W +iv ol +ĠCh em +çļĦ 話 +use ment +ĠSupp ose +Ġê°Ģ ì§Ģê³ł +Ġmill enn +ĠT un +Ġmed al +Ġha cia +Ġstimul us +Ġbright ness +a ient +ĠH ands +in et +Ġcoal ition +åŃ ¸ +Ġris es +r ina +Ġsc oot +Ġ ãģ§ +Ġdef ending +Ġin vers +Ġh ills +Ġfulf illed +åΰ äºĨ +ll ie +Ġad oles +ĠCh ase +åĸľ æŃ¡ +ĠJ J +Ġne uen +ĠT ru +Ġinher it +Ġsixt y +ĠEx p +ĠCl ay +оÑģ об +ar na +ĠImper ial +ĠÑįÑĤ а +Ġso cially +at y +ody nam +Ġrib s +om ic +ĠT ol +ол ж +Ġ199 8 +Ġfr am +Ġrank s +ĠбÑĥд Ñĥ +ĠCol on +H z +Ġaccommod ate +Ġexpl ode +íĦ °ë +HA EL +ĠH art +Ġжиз ни +æ ¡ +Ġdel icate +ł ×Ĺ +Ġto fu +Ġachieve ments +ĠS or +Ġagre ements +Ġ5 7 +Ġt amp +Ġfr ançais +Ġher bs +c orn +Ġkon k +AN A +ĠQ i +Ġpró p +Ġt iger +Ġëij ĺ +Äĥ m +Ġapp rent +ah an +Ġrul ing +Ġt sp +Ġtw itter +Ġteen ager +b us +Ġí Ĵ +ĠAmend ment +Ġt apping +ĠAP Is +åł ´ +Ġmatch ed +ë ©´ +W A +ĠBeaut y +Ġinevit able +Ġg ases +ĠÙ ¾ +h igh +ĠO pt +Ġpred omin +Ïģ ÏĮ +Ġtub es +Ġìķ ł +ĠA a +Ġ æľī +omet own +ĠI M +Ġdes ar +ä ren +Ġм аÑģ +ĠM öglich +Ġrent al +Ġíķ¨ ê»ĺ +ĠD iana +Ġaut ism +ĠPu erto +ı ld +Ġfal an +Ġdream ing +Ġg ute +Ġк ам +Ġw reck +Ġstoryt elling +ĠLeg end +ĠUk rain +ĠпÑĢо иÑģ +ĠS K +Ġíĸ ī +ĠÅĽ wi +ĠBel ieve +Ġmost rar +ĠTod d +ĠN iger +ic ting +h ard +: // +ir able +ig ation +ĠMem bers +Ġìłľ íĴĪ +Ġdisc our +Ł ½ +ri ka +ĠD N +ĠF if +ĠCap ital +ÑĢ Ð¾Ð¼ +ĠS ans +y un +Ġpil ots +Ġtr at +Ġn yt +Ġë ¯¼ +Ġexpon ential +Ġemer ge +Ġtraject ory +ĠпоÑĩ емÑĥ +Ġse aled +att i +Ġw ides +Ġо гÑĢ +ian ces +Ġwitness ed +O r +os i +ĠJo el +on al +èģ ½ +ĠInt e +ced es +ĠGot ta +an ium +Ġfem ales +ĠLeb ens +Ġmoist ur +ĠSim ple +ĠDo ch +ar á +Ġg esehen +US T +Æ¡ i +Ġclass ification +Ġdiagon al +Ġperm ett +com p +ĠاÙĦ ØŃ +ĠMal ays +Ġgeh ört +Ġpop ped +Ġcontact ed +Ġ׼ ׾ +Ġ14 0 +Ġadapt ation +Ġman us +Ġtur key +Ġpre ach +br ight +Ġdown s +Ġunpre cedented +Ġmight y +Ġc ater +itt i +g s +ĠDep uty +wr ite +ĠB less +á c +Ġsum mit +Ġëı¼ ìļĶ +Ġthought ful +Ġsh red +s inging +ĠлÑĥÑĩ ÑĪе +Ġy en +Ġvibr ant +ĠWal ter +Ġhost s +Ġamb ul +Ġinv asion +og an +Ġreason ing +Ġsu cc +л екÑĤ +Ġfal a +Ġk ings +Ġgo in +Ġcal ib +ĠGRÃľ NEN +ot er +Ġein z +Ġins ulin +Ĭ ¨ +Ġsc aling +ĠC orn +hy d +Ġmat te +P L +Ġali ens +ĠSe g +è¯ Ŀ +est i +ast ics +Ġwar mer +Ġing en +ĠM L +Ġro de +ĠE ye +be its +ĠB arn +» , +ĠCh uck +Ġprofit able +ugu ese +ĠArab ia +Ġco co +Ġpued o +Ġinflamm ation +cl ip +Ġtables poons +Ġìł ij +ĠSw ed +Ġan at +ìĪ ł +Ġar rib +Ġdan cer +ĠCar ter +Ġmagn ific +st ore +éģ ¸ +Ġf ade +Ġaccomp any +Ġw ahr +Ġye ast +Ġmin eral +Ġlegisl ature +ä½ ı +ir os +Ġcrowd ed +ÑĢа ÑĪ +oc ado +ìĸ´ì ķ¼ +ĠíĽ Ħ +ĠBar ry +m aster +Ġnick name +Ġ" ... +ĠR s +ĠMo ore +Ġven ue +Ġб Ñĥ +ãĥ ¡ +li hood +ĠA gency +л ов +Ġk ah +ĠìĨĮë ¦¬ +Ġm arsh +Ġincorpor ated +ant wort +Ġkim chi +Ġw oo +Ġdistract ed +er ies +Ġinform ación +ĠCho ose +ĠJ adi +Ġanal ogy +s ay +uff le +b ok +Ġac ids +Ġacquis ition +Ġvari ants +èµ· ä¾Ĩ +Ġpass iert +ìĿ´ë Ĥĺ +ruct ive +br ig +Ġ ãĢĮ +ep her +Ġp H +ut lich +å· ® +Ġrel ie +u ite +Ġre ception +Ġco h +ĠPre p +Ġanticip ate +æĢ § +ke e +Ġdesign ated +Ñı ÑĤи +ĠK or +ĠAn im +üh l +ĠWh it +Ġun cover +ĠMay a +ĠÑĤ огда +° ķ +uten ant +Ġìĸ ¼ë +Ġforest s +Ġmem e +Ġdistingu ished +ĠMar x +ĠL ion +Ġserv ants +ĠD iam +çķ¶ çĦ¶ +ĠPol icy +į ¼ +Ġtrigger ed +abil ir +ĠìĿ ij +Ġnegoti ate +Ġfe z +Ġer w +Ġvar ies +Ġj emand +Ġdis charge +ÑģÑı Ñĩ +ĠP AR +ĠAff airs +Ġvot er +Ġat en +Ġcro is +ob il +ĠO ops +ĠAr c +ĠHe ather +ank a +Ġsim ples +ο ν +" > +Ġch ords +ĠSand ers +Ġë¶ Ħë +B en +Ġdar über +ili ans +Ġorder ing +ĠMan h +Ġkil ogram +Ġkar ÅŁ +Ġgr asp +Ġghost s +al en +ĠJ edi +Ġб ли +Ġdownload ed +Ġconduct ing +ĠH ak +Ġresearch er +il an +go od +ĠH annah +ĠdÃ¼ÅŁ ün +ĠMess iah +u ity +ion a +Ġprob able +ĠY E +Ġindepend ently +Ġbuff er +b urn +our d +ĠMc K +Ġl ingu +uj emy +еÑĢ ÑĤ +Ġintuit ive +Ġcrack s +app ropri +nt y +Ġge en +Ġl end +Ġcert ification +ID S +un ter +pe es +Ġtr ump +Ġbank rupt +Ġfe as +è Ĺ +Ġdu ż +æ¸ ħ +Ġvirus es +Ġ5 8 +g od +Ġж ел +Ġst alk +I nd +ach i +ĠC F +ĠC ond +Ġsan ct +Ġcont en +Ġfre ed +ĠR T +Ġment ors +ì¡ ± +Ġport able +ĠPaul o +r ane +HA HA +ĠS ection +ç Ĩ +hy un +ĠÎŃ Ïĩ +ĠP ub +ĠInd epend +Ġcomp ounds +ĠÑģ Ñĭ +Ġmess aging +Ġded ication +Ġnot icing +Ġdevot ed +ÑİÑĤ ÑģÑı +Ġsn akes +Ġbattle field +p ers +Ġdel a +9 2 +Ġha i +ill ä +ér er +e very +Ġrespons ive +×Ļ ×ķ +op f +é ī +Ĭ ¸ +Be cause +Ġtour ism +Ġê·¸ ê²Į +×ķ× ¦ +Ġcan s +st üt +Ġdon ne +ĠD ios +ĠU ber +act ory +Ġorient ed +ĠH erm +Ġpat ron +ur f +be i +Ġprogram a +ĠOh h +gen er +Ġf ist +ĠW endy +Ġand a +Ġguess ed +Ġfre ak +ä¸Ń åľĭ +ĠK ings +ch ool +Ġoff line +ĠIndian a +ĠAll iance +Ġ5 3 +Ġpartic ul +ĠF ocus +Ġinhab it +Ġê°ĻìĿĢ ëį° +ĠMc G +ows ki +ĠìĿ´ ê±´ +Ġpa ÅĦst +он и +itt a +Ġconfirm ation +ĠBrook lyn +Ġnood le +f und +it ud +Ġgrand parents +Ġbar becue +ει ÏĤ +Ġ á +Ġball ot +ĠV eter +Ġpip es +ig ious +ĠG raph +est ed +Ġë¸ Įë +ĠK E +ãģ¡ãĤĩ ãģ£ãģ¨ +Ġe ins +Ġhat red +ãģij ãģ© +Ġd ang +ee ee +Ġarch ae +ĠJes se +Ġdetect ed +Ġsen i +burg h +Ġdispl acement +Ġdo p +Ġcondition ing +Ġне ÑģколÑĮко +Ġdistur bing +P H +Ġthin ner +Ġwound ed +ĠCu ando +Ġcush ion +Ġwh ites +Ġprefer ences +Ġì¤Ģë ¹Ħ +Ġka ż +ĠG ate +ĠP ath +d les +à¸Ħ ร +im ore +Ġë³´ìĹ ¬ +Ġdiscipl ines +á» ı +Ġmes ma +Ġìĥ Īë +Ġìĭ ¬ +Ġg ing +Ġumbre lla +IGH T +Ġp ension +Ġcomb ining +S S +Ġrect angle +á»ĩ t +Ġpro xim +ĠC ow +¸ Į +Ġintention al +æķ Ļ +Ġdec id +ĠÑģк аж +ĠU ma +ias m +b uz +Ġdebr is +Ġc ass +ĠP rop +is ka +ë ł¥ +ester ol +uss ian +ìĿ´ë ŀij +Ġun limited +Ġadm ire +Ġtight ly +Ġgen ome +ĠJun ior +ven ir +g us +Ġc Äĥ +ĠV lad +Ġí Ĥ +Ġrel ativ +in ci +Ġaun que +ĠBo ys +ÑĨи он +ĠSw iss +Ġphys icians +Ġíı ī +ĠP ET +Ġw ounds +ab out +Ãł i +on z +ur ities +ĠÑĥв ид +å· ¦ +Ġment ality +Ġvari ance +Ġseg unda +Ġvol cano +al ie +ॠĩ +Ġt iles +ĠT erry +ĠاÙĦÙĦ Ùĩ +Ġcan on +Ġsc attered +pt on +Ġdefin itions +Ġal gebra +ot en +ab lo +ij uana +Ġwra pping +Ġses ame +ĠнаÑĩ ина +ĠAl f +ĠÐł оÑģÑģ +or no +Ġan kle +Ġspecial ty +Ġattempt ing +ili ation +Ġ19 20 +Ġphen omena +ĠPro duct +ĠB uck +ĠA ww +se en +Ġvo id +ĠFrank lin +Ġadvoc acy +ĠS ep +Ġcool est +ĠÑģ ÑĢазÑĥ +ĠQu and +Ġ9 00 +ĠTr ad +d ies +Ġhas h +æĪij å°± +ä¹Ł æĺ¯ +Ġpot s +Ġsad ly +Ġvi able +ĠT iger +ĠON E +Ġneur ons +ow anie +Ä Ĺ +ĠSh ar +ĠLand es +Ġconfer ences +è© ² +Ġcred ential +Ġl ime +ine e +x it +p ay +Ġinc ons +Ġ>> : +èª į +Ġí ŀĺë +Ġless er +Ġsp ill +Ġprem ise +Ġ36 5 +ĠH ost +Ġtom ar +×IJ× ľ +ë ²Ī +ĠWhat s +Ġlight weight +ĠM ap +f ia +ells chaft +Ġvend ors +uest o +ĠM ister +ĠÐŁ ÑĢи +åı ³ +h ma +Ġintention ally +ĠT ang +éĹ ® +Ġident ification +Ġetc etera +ĠN ee +ĠÑĤ ÑĢи +ê· ¸ +Ġcrypt ocur +Ġin hale +Ġadd ict +åIJĦ ä½į +Ġma u +ĠÑĤак аÑı +Ġë² Ħ +Ġcomp rar +ied zieÄĩ +ĠоÑĤ но +Ġbegin ner +Ġм Ñĥж +Ġobs c +Ġlim iting +asc ular +Ġins pection +ac i +Ġre jo +M us +Ġz aten +Ġsz cz +ĠMad rid +Ġvar ieties +Ġest Ãł +ĠSh akes +Ġk its +Ġad minister +Ġla va +Ġg Ã¥ +è© ¦ +ת ×Ļ +ĠWay ne +Ġinst agram +Ġr ated +p aper +Ġb ild +Ġpret ending +Ġobser ving +ĠÑģам ом +Ġtr or +Ġorgan isms +Ġfal ta +Ġh ometown +ç ± +Ġí ĭ +Ġche g +Ġì ¡ +Ġcomm a +is é +Ġlike lihood +av ored +Ġgel di +ни ков +Ġmed io +Ġjak ie +ĠJ up +Ġgreen house +Ġsp it +ко е +Ġк аж +ĠG ram +ĠCon ference +Ġdef icit +s ın +in se +u ÄŁ +Ġr icht +Ġcoinc idence +åı į +Ġeu rop +Ġbutter fly +p read +Ġìĸ ¼ +èĢ ¶ +Ġwa vel +ĠIn fin +ĠPlan et +Ġself ie +ient ras +Ġar rog +os er +id al +ł×Š׳×ķ +üt ün +Ġfresh man +ĠMach ine +Ïĥ ÏĦ +ĠD ia +ìĿ´ ëĭ¤ +ãģĵ ãģĨ +ne a +Ġlist ing +Ġconfig ure +ut or +U p +ts chaft +ri ère +Ġup wards +ĠÑħоÑĩ Ñĥ +Ġswe ep +B r +Ġexpress ing +Ġun happy +Ġmand atory +g ender +ĠA ÃŃ +Ġindic ators +Ġoil s +n ote +Ġseg ur +ож еÑĤ +yn asty +Ġdist ances +Ġmer ge +BER T +Ġsur render +Ġbu at +ĠA wards +Ġseñ or +od ox +Ġfl avour +Ġab dom +Ġconfig ur +8 6 +ĠDI Y +Ġrig id +° ĺ +Ġcorpor ation +Ġg room +j aw +ĠNe ar +ил о +Ġoper a +ĠIn nov +и ÑĢа +ĵ ± +Ġspec ified +Ġcos m +ĠFre edom +Ġcl own +ĠN em +Ġв ол +Ñij н +Ġchar ger +à¹ģ ล +Ġinflu ential +äs ident +é ¤ +ĠìĦ łë +Ġvol umes +æ IJ +Ġout ras +ĠTw itch +Ġfound ing +Ġa while +Ġco il +ê° Ļ +Ġc ả +ĠTh row +ĠH ence +omm t +ĠBen jamin +глÑı д +T ime +ob ic +Ġm our +Ġd read +ĠL Ãł +ĠCh ile +Ġpre val +Ġv ain +Ġart ık +Ġpres erved +ĠоÑĤ д +Ġware house +Ġbest e +ĠSever al +ĠS ituation +Ġcard board +T od +er na +Ġgar ant +Ġgest ure +Ġh en +Ġspe lling +ose xual +Ġan ne +Ġm ice +ĠMe ine +c ard +Ġre bell +Ġcert o +Ġìľ łë +Ġvers chied +ĠB os +Ġinv ention +Ġtr ze +Ġman ière +ĠCh ad +Ġsp re +Ġorganis ations +Ġpoor ly +Ġan terior +Ġst air +к ÑĢ +Ġatom ic +Ġsymp ath +Ġcontin ually +Ġkle ine +è te +и Ñī +ο ÏĤ +pe ut +Ġrep osit +Ġent ra +E m +Ġfinan cing +Ġмн ог +Ġthe sis +ĠCom puter +e au +ĠT ree +Ġbr ide +ons ieur +sh ire +w ic +D E +ĠìĪ ĺë +Ġac om +ĠP O +ers ch +Ġпом оÑī +ĠAr men +Ġì£ ½ +Ġz or +Ġprint s +ĠD ass +æ¸ ¯ +Ġdur able +ĠTrans port +ìŀIJ ê°Ģ +Ġл ег +Ġdé t +ô le +am ous +Y N +Ġcl iff +Ġgramm ar +ĠÐŁÐ¾ ÑįÑĤомÑĥ +ĠlÃł m +es ch +Ġmiser able +Ġvol ts +ĠC ad +uk an +ÑĤ ив +r ust +Ġìĺ¬ë Ŀ¼ +Ġver k +Ġchick ens +ĠY oo +Ġout fits +c ode +Ġhier archy +net es +Ġcounter part +Ġt ôi +Ġt ed +ĠB art +Ġë Ŀ¼ +ĠGen au +Ġinc oming +ĠA BC +ri que +ĠоÑĤ п +qu al +Ġincent ive +Ġih ren +׳ ×Ļ +lo e +Ġ19 30 +Ġbar g +Ġd iction +Ġön ce +IN S +Ġre h +isia j +m outh +Ġsc oring +l ık +ĠìķĦ 주 +OR IA +ĠEst ados +Ġcompan ion +Ġasse mble +Ġpun ished +Ġit al +Ġprev ents +ist es +ĠKent ucky +Ġloc ate +Ġfast ing +ãģ¨ æĢĿ +ĥ Ģ +ĠSe b +ĠCr own +op ia +Ġwh ip +us z +к ами +Ġdatab ases +åŃ Ĺ +Ġprose c +Ġ199 7 +ĠìĤ´ì §Ŀ +ĠSol ar +ĠP ues +ĠZ en +oll o +ĠG uru +Ġsque ez +ĠÐĹ Ð° +ĠÄ į +cept ions +c ca +iz able +m and +Ġbreak through +Ġtables poon +ĠS EC +ik h +ĠS ão +Ġп ло +am en +Ġpr ac +Ġdar ling +Ġtall er +Ġrend ering +Ġìļ°ë¦¬ ê°Ģ +ĠÏĦη ÏĤ +Ġm ã +Ġes os +uer do +ĠÑģ ÑĩиÑĤ +all er +ìĹĪ ìĸ´ìļĶ +Ġmill ones +ler in +Ġpe gar +on ne +Ġenroll ment +Ġli egt +Ġbo a +w iÄĻ +bs p +Ġcy cling +ĠBern ie +Ġ198 9 +Ġд алÑĮ +ĠDak ota +ĠÑģв Ñıз +ĠC P +Ġst are +íĤ ¤ +Ġprosper ity +Ġarrange ments +Ġarri ving +m ä +Ġkay ak +ip t +Ġp ardon +Ġrel at +Ġver ste +ĠF ig +Ġfo il +ĠTalk ing +pe are +Ġno i +ĠпÑĢи ÑĪ +Ġhoc key +Ġad o +ĠO UT +6 7 +Ġhorm ones +ĠAven ue +ĠSuper man +Ġpres cription +uber netes +C L +ot ive +N IS +ien en +Ġsad ness +ĠV it +T y +Ġstar ter +Ġbed e +Ġfound ations +Ġso re +åº Ĺ +Ñīе ÑģÑĤв +ìļ °ë +ĠÑĩ Ñĥв +l ink +Ġmane u +work ing +Ãł n +ĠAtt ack +ĠC art +ve is +ĠRes p +ens ing +Ġì¢ĭ ìķĦìļĶ +Ġesc uch +ĠR NA +Ĥ ´ +Ġad op +Ġb ending +ع د +Ġman ages +us p +Ġt art +Ġrout er +B o +Ġestab lishing +Ġbal ancing +Ġathlet ic +ĠS lo +Ġf ills +Ġн аб +Ġд ал +Ġpos so +ĠV ielen +Ġcrit ics +Ġlaws uit +ĠIsa ac +ĠÑĦилÑĮ м +Ġtr as +Ġpra w +ĠCra zy +Ġne u +Ġk ull +Ġtum or +ĠAP P +g ate +ĠA RE +9 8 +ĠSte am +Ġfuck ed +l age +ĠâĻ ¬ +ĠM D +f y +Ġshell s +ĠSe ems +iz ers +Ġr anges +ĠAnton io +AT ION +ĠB aba +Ġìĥ ī +k un +Ġpray ed +ÑĢ Ñı +ĠпÑĢоÑĤ ив +Ġse as +b ury +Ġ×Ķ× © +Ġtra it +ĠDep ending +Ġd re +Ġkön nt +ÑĨ Ñĥ +Ġlip stick +ee z +ĠпÑĢ Ð¸Ð¼ÐµÑĢ +Ġassign ments +B ob +Ġmet als +Ġspe cially +å°į ä¸įå°į +Ġìĺ Īë +ĠÅ ¡ +Ġv ista +ĠÎ ¬ +Ġtw ins +Ġnot able +ĠS au +Ġdé velop +Ġç ek +Ġpoly nom +av am +Ġtamb é +он ом +Ġpl asma +Ġe fect +Ġlä ng +Ġcas i +Ñģ а +ım ı +ãģĻ ãĤĭ +ĵ¤ ìĿĢ +Ġlab our +oss en +ĠP un +r if +Ġd oses +Ġoper ates +ил ли +Ġja ar +st aw +ĠìĤ¬ëŀ ij +Ġat m +Ġprotect s +Ġimp ed +H O +Ġc ima +Ġto ch +ab is +Ġsend o +la us +Ġcur l +ĠN um +Ġspons ors +Ġdé but +ĠAlex a +ĠB ür +ĠA mer +Ġc ope +Ġиз в +j al +Ġ199 5 +ap at +res se +ĠPri ze +ĠCla ire +ĠBrand on +Ġwszyst ko +Ġval ued +à¸Ļ ะ +Ġse ct +Ġsecret ly +Ġdiam onds +ĠEv an +ĠRP G +ãģ« ãģª +Īë ıĦ +ĠUnivers al +Ġdoub ts +ĠP in +wiÄħ z +ļ © +Ġal bo +Ġbra ucht +AU L +ĠM obile +gr ades +Ġsch em +wh y +ĠN icht +p i +g le +Ġchor us +Ġg ly +Ġrein force +Ġm uff +ĠSh en +ĠH ola +Ñĥ г +vid emment +v ial +ac ious +laim ed +ĠR ico +Ġve gg +Ġillust ration +ĠBut ter +ow ad +Ġeu x +Ġenf ants +ĠLe ader +ĠVill age +et ically +ÙĨ ÙĬ +Ġst ew +Ġsurpr ises +Ġc ue +ĠGrand ma +ĠC elsius +ĠR icht +en c +Ġpet ition +Ġher b +Ġw icked +Ġsch le +oc aly +Ġtrans f +Ġtok ens +ĠGr ay +ĠB BC +I K +Ġ15 00 +z n +ĠNe v +Ġk oy +Ġz ar +Ġbull shit +ĠColomb ia +ul ative +Ġwides pread +y ect +k it +Ġempres a +Ġn our +Ġburn s +at in +a ired +Ġrevolution ary +Ġгод Ñĥ +ĠLog an +Ġ199 6 +ĠGra ham +re b +ĠN HS +æľ Ľ +Ġcost umes +Ġnaw et +Ġlo vers +ĠLuc y +ĠInd igenous +íķĺ 기 +Ġimmun ity +¥ ´ë +uit o +Ġexcess ive +Ġdon ations +Ġ×Ķ ×¨ +Ġì² « +éī Ħ +Ġdry ing +mel on +Ġsurve ys +Ġ무ì Ĭ¨ +é¢ ¨ +aa a +Ġpro be +an cial +Ġlou der +Ġhot els +ü ÄŁ +ag ner +Ġorig ins +Ġë§Ī ì§Ģë§ī +Ġ* * +Ġstr angers +ĠHa us +com ed +Ġan throp +Ġus o +ĠìķĦ ì§ģ +ĠY uan +ĠíķĦ ìļĶ +pl er +ress ive +Ġsp raw +ĠSt ew +Ġ199 4 +Ġeld ers +Ġme inen +Ġj unt +Ġac oust +ĠW ohn +Ġban anas +Ġproject ion +ĠSt ick +leg t +spe ed +ĠcÅ ©ng +ĠW ort +ĠBalt imore +ĠÑĨ ел +Ġdun no +å¼ · +? , +ãĥī ãĥ³ +ĠLoc al +ost o +Ð Ń +од а +ĠPort uguese +Ġtheir s +Ġdé m +åı ¦ +Ġdra uf +ĠBuddh ist +ert a +G e +Ġcar rot +ĠWonder ful +Ġso ak +Ġchair man +gg i +IC A +f ried +Ġfl ick +ĠThrough out +Ġìļ °ë +Ġc ough +Ġfl uffy +sch ool +Ġr ipped +---- ---- +ĠZuk unft +Ġн еб +Ġst o +ĠB O +p ent +ĠLaw rence +Ïī ÏĤ +st icks +ĠE ins +ĠÑĢ Ñĭ +ĠStr ong +Ġcar amel +Ġsp ite +az ar +éĥ½ æĺ¯ +Ġcrit ically +Ġob ra +ow itz +ĠZ one +ĠÑĢ ÐµÐº +Ġsu g +ard ed +Ġg ì +ff entlich +an che +Ø Ł +ast ically +ìĿ ¼ë +л ав +Ġsimpl est +ĠF riend +Ġque llo +Ġamb ition +Ġabb iamo +åº ķ +ĠÑĦ оÑĢм +ĠEs sa +Ġeduc ators +Ġstatist ical +éĢĻ éĤĬ +Ġchang er +Ġat au +éta is +ĠShakes peare +ë IJĺ +Ġtr iggers +Ġreal iz +Ġcel ui +whe el +Ġloyal ty +Ġscream s +ke hr +ĠM ega +e ast +Ġtop s +ĠTot ally +ount ain +l ord +Ġviol ation +ĠG A +Ġnic er +ĠF resh +ĠMel issa +fun ction +Ġra pe +Ġexcept ions +Ġsil icon +Ġliber ty +Ġhousehold s +ãģį ãģ¾ãģĻ +ĠC A +ĠÐŀ б +Ġli b +ŀ Į +c ific +Ġtrop ical +Ġinvestig ating +H D +Ġad apter +ĠP itt +an cia +ĠShe ll +friend ly +Ġconclus ions +Ġtur tle +Ġdec omp +Ġanim ations +ĠÑģ ек +ins i +Ġret ention +k ie +Ġinject ion +ĠMad ison +ì° ° +Ġv ient +Ġvar ied +Ġviol in +ĠB il +Ġluck ily +Ġh tt +l ä +Ġr anch +çľĭ çľĭ +Ġsó lo +ìķ ħ +ĠD erek +ĠScript ure +оÑĢ Ð° +Ġclassroom s +av il +form ed +Ġbefore hand +ĠG em +pre ch +Ġl in +Ġgre ens +ÑĨ ев +ĠMer cedes +Ġdr ought +gas ps +Ġab ortion +Ġter ribly +Ġspos ób +Ġsec ured +Ġat rás +Ġwavel ength +Ġgra ins +ect ive +Ġspace craft +Ġtour s +Ġprof es +Ġsur geon +ĠP ie +Ġide ally +arn er +U P +op ard +s ce +Ġimm ense +ĠOr t +roll er +ĠD allas +ĠNich olas +Ġs ulf +ĠToy ota +Ġquant ities +ce ans +Ġcu i +an ça +ĠC AN +itzer land +åĦ ¿ +Ġz ou +ĠCy ber +le gen +ĠIn it +ed u +Ġa pert +Ġad jac +ou v +èĢĮ ä¸Ķ +r s +Ġcab bage +Ġwheel chair +iny l +ĠD ynam +ĠìķĦëĭĪë Ŀ¼ +Ġl ing +h l +Ġмог Ñĥ +Ġcris p +Ġm ij +Ġd ug +n in +Ġbl oss +Ġbelong ing +Ġloud ly +Ġminer als +Ġconclud ed +Ġsearch ed +9 6 +ĠMe et +ĠS EO +ĠС к +ĠH ob +ot ta +Ġpropag anda +Ġcin namon +Ġhun ter +Ġgeme ins +Ġsculpt ure +uls ion +Ġv äl +Ġmagaz ines +Ġcontrovers y +ä¸Ģ 樣 +Ġsequ ences +ãģĦ ãĤĭ +Ġíļ Į +Ġdel eted +ä½ ¿ +IJë ıĦ +Ġvary ing +ãĥ Ĩ +Ġmount ing +Ġaff air +Ġpath ways +æ ¦ +Ġdig o +äº ® +Ġд ок +A lex +Ġtob acco +ĠC V +Ġbother ed +Ġamb ient +ink y +ĠS L +Ġh ates +Ġje żeli +Ġcon greg +Ġel as +Ġde uts +ĠStud ios +ch ÄĻ +Ġdocument ed +ĠCru z +ĠL en +ĠDoug las +ĠPort ugal +ent i +Ġsp ouse +Ġanal ys +av ia +Ġed ited +Ġl ại +bu ilt +Ġv ille +ad ora +Ġbrac elet +Ġs ushi +Ġp m +Ġtra ils +Ġl ug +Ġö ver +Ġs orrow +Ġcol ony +ado x +Ġser ie +any ak +ĠØ · +ĠG ulf +æĺ¯ ä¸įæĺ¯ +ĠP V +ĠSam uel +ĠK it +ĠR al +ont in +ex pl +Ġent ries +Ġactiv ists +P s +Ġs ant +ĠÑĤо Ñĩ +ĠBr uno +ke ley +Ġtut to +é Ķ +Ġv intage +Ġterr ified +Ġпо Ñħ +us ive +ow ers +ай ÑĤ +ë ıĻ +Ġtwist ed +ĠTh ought +Ġt ah +Ġshr ink +Ġshe er +l it +Ġdal am +Ġd ib +Ġv ard +ow ane +Ġdo br +ĠR ena +ĠÑģво Ñİ +ĠpaÃŃs es +ĠE ra +ãģ® ãģ§ +ĠB UT +s ighs +Ġê·¸ ê±° +Ġgro ÃŁen +Ġë¹ ¨ë¦¬ +Ġn erves +Ġconst it +Ġpreoc up +ĠG ay +ĠX u +keep er +he ure +.. ) +ĠCal m +ĠUn idos +ĠìĿ´ ê²ĥ +ĠAqu i +Ġìłľ ìĿ¼ +d ır +ì¦ ĺ +y our +ĠÑįÑĤ им +20 20 +Ġr und +ĠH O +ĠC atherine +iel i +Ġf usion +Ġide ology +Ġfor am +sh aped +ĠíĽ Ħë +Ġw t +Ġret r +Ġpr éc +Ġê° ij +Ġopen ly +v ity +구 ìļĶ +Ġobst acle +Ġbo o +Ġse iner +ic orn +Ġeigen lijk +Ġhead er +are mos +Ġso fter +ĠÐŁ од +Ġpre jud +Ġdefin es +ier te +Ġbl ending +Ġbelie vers +ĠWo chen +Ġник ак +ĠÐļ огда +ĠTyp ically +Ġíģ ¬ +ç® ¡ +ci os +Ġmiss iles +Ġsp onge +ĠK itchen +Ġt ren +ning en +Ġsc rap +Ġser ait +´ì ł +ç ¹ +Ġë° ĺë +Ġrest ored +Ġprzy kÅĤad +ĠK ubernetes +Ġsa it +Ġu w +Ġen abling +Ġtra vers +amp s +åı Ĺ +ĠOM G +ens or +Ġz osta +Ġpronoun ced +A ng +norm al +Ġeconom ies +t in +ĠChamp ion +iz en +Ġar beiten +ĠG ospel +ĠZ u +ng a +Ġliter acy +ĠM ans +Ġcircul ation +Ġad ap +ĠTot al +Ġmere ka +Ġol acak +ÑģÑĤ аÑĤи +J ack +Ġm und +Ġth ief +b ies +Ġê² ģ +a que +ĠÚ© ÛĮ +ĠSc ar +å ² +Ġab ol +Ġdev ote +Ġ0 1 +Ġs itten +ĠVis ual +we ek +s ome +ing t +Ġjournal ism +ĠH ir +ĠB achelor +in ery +Ãľ ND +ãĥ Ł +ç» Ļ +Ġcolor ing +ĠCr ist +Ġcelebr ities +ĠÑĩ иÑģ +ĠC rit +Ġdifferent iate +ĠÐľ не +el im +Ġse afood +Ġalgum as +otherap y +æĪ ° +Ġgla ub +Ġarbitr ary +g ens +ĠбÑĥд ем +Ġt av +Ġcream y +ĠCount ry +a ñ +м еÑĤ +Ġh inter +Ġm ism +Ġillust rate +ÃľND NIS +Ġdecre asing +Ġwen iger +AK I +ix on +Ġн ей +Ġfat to +Ġn erd +ç ł +Ġb itte +P er +Ġt ane +Ġgö z +Ġfor te +ĠE y +Ġнав еÑĢ +è¢ « +ĠWord Press +ĠM is +Å ¯ +z äh +Ġinté ress +osa urs +ĠFall s +Ġn essa +9 7 +Ġmuseum s +Ġcorrespond s +Ġs ings +f our +Ġed er +ĠCommun ist +o a +ne k +ĠWH O +Ġcor po +Ġmess ing +ÏĦ αι +Ġbrush es +Ġb isc +ĠAr beits +ĠT ax +Ġse le +Ġflag s +ou pe +Ġanticip ated +ãĥ ij +ĠN ad +Ġpou red +Ġm l +Ġll ama +Ġvisual ize +Ġlisten ers +ÙĦ Ùĥ +al ten +Mich ael +Ġcos ì +Õ¡ Õ +op us +Ġíķ´ì £¼ +Ġh ike +ĠAtt orney +ĠHill ary +ud ed +Ġíķĺ ì§Ģë§Į +Ġdo ve +Ġstorm s +ак Ñģ +Ġdoct rine +Ġhe x +ik s +no ÅĽÄĩ +Ġscript s +Ġδ εν +ĠÑįÑĤи Ñħ +ĠÐ Ĩ +ab er +ĠV as +Ġcent imeters +×ŀ ×Ķ +ни б +Ġrid ers +ĠT rib +åĮ ħ +Ġtak że +Ġn oun +Ġic ons +Ġsole ly +mind ed +Ġdisp on +ĠSw itzerland +Ġcl usters +Ġqu eda +ail ing +Ġman ga +Ġ6 8 +Ħ Ī +Ġt et +g ins +ha us +ç© º +å· ¥ +ĠO P +ot ed +Ġnouve au +AL LY +ÙĪ Ø¯ +ò n +Ġmort ality +ĠGit Hub +d rop +Ġdis gu +Ġrec om +Ġloc als +Ġhome made +amb a +Ġpron unciation +Ġal phabet +ан ÑĮ +ow any +ir as +id ency +OM E +ĠÑĢаÑģ Ñģ +ar ak +v iamente +Ġnon profit +ĠYouT uber +Ġp arenth +ĠB oo +v at +ĠSt ir +Ġpre cip +Ġan ts +Ġall y +ĠMa ori +ĠëĮĢ íķľ +åı¯ æĺ¯ +og ene +ĠLab our +aret te +Ġrecy cling +ens a +Ġpurs uit +Ġs ak +ĠÐĹд еÑģÑĮ +Ġtoler ance +Ġsa at +Ġclick ed +âĻ ¥ +Ġface book +ĠInt o +Ġincent ives +기 ëĬĶ +ĠD ennis +ĠW ik +ges ch +à¹ĢภĽ +ĠÏĢ Î± +ĠWh oo +Ġround ed +Ġdo pe +Ġcapt uring +ĠWar ri +Ġcivil ian +Ġchar ming +Ġes as +Ġsust ained +Ġle aning +Ġabund ance +ÃŃ lia +алÑĮ нÑĭй +Ġph ải +ac ja +Ġê°Ļ ìķĦ +act iv +า ย +Ġ9 7 +Ġм ой +c ro +ĠJack ie +itt ees +br acht +ul ent +Ġìł ľë +Ġplug in +v antage +part y +Ġsu as +Ġan te +Ñĥ л +ÐĿ ÐIJ +æĤ ¨ +ĠÏĥ Ïħ +Ġmet h +Ġenthus iasm +ÑıÑĤ ÑģÑı +íĻ Ķë +Ġsynth etic +Ġseason ing +ĠL ost +on omy +ĠSp ark +Ġb ure +Ġass ured +Ġimag in +Ġcar ro +S ha +Äħ t +нÑĥ ÑĤÑĮ +át ica +T Y +Ġk ern +ĠBrazil ian +à ° +Ġsusp ended +ĠCar ib +Ġbiz im +ĠOl iver +ãģ ¶ +T om +Ġпл ан +Ġn ope +omet hing +Ġbe iden +ÑĨ ен +Ġflu ct +Ġμ οÏħ +Ġf athers +ĠBl ake +Ġup ward +ĠD ash +ĠL il +ĠìĪ ĺëıĦ +Ġrevel ation +Ġelev ated +ĠJi ang +LE D +ĠThom pson +Ġмог ÑĥÑĤ +ÑģÑĤ ÑĢÑĥ +if iers +Ġcome back +Ġbuy ers +ê² ° +ĠS ales +иÑĩ е +c iones +Ġwh istle +Ġd ull +LE X +Ġíķĺ ê²łìĬµëĭĪëĭ¤ +Ġcrimin als +Ġdes cent +ipp le +mas ı +Ġfool ish +ĠдÑĥм аÑİ +t ar +Ġman go +Ġchore ography +M att +Ġterr itor +Ġac aba +ĠEin stein +ĠI BM +ĠMet al +ĠCry stal +Ġr ah +Ġf oul +ĠIsland s +Ġint act +ĠR ail +. : +Ġac á +ĠпÑĢ Ð¾Ð¿ +еÑĢ Ðµ +ĠWr ite +he he +ĠF O +ĠÏĥ ÏĦη +Ġdo in +h eld +Ġappropri ately +Ġdeliber ately +Ġarch ive +Ġgive away +ãģĵ ãģĵ +Ġfin ale +л аÑģ +ен о +Æ¡ n +æ£ Ĵ +og o +çī © +ĠAud ience +ãħ ł +Ġsub ur +Ġhead ache +ан нÑı +ĠW itch +ĠSwed ish +ĠB I +Ġer ase +Ġk hi +Ġcomment ary +ĠS ultan +íĥ Ŀ +ĠLe ban +Ġë³´ì ĭ +ĠP am +pe kt +mon th +Ġground ed +ê ¾ +ĠÅŁek ilde +2 50 +ĠS CH +ios o +Ġin aug +he imer +Ġreflect ing +ĠR uth +ĠO il +Ġtrou ver +u ep +.. ] +Ġìŀ Īë +Ġol ha +Ġreason ably +Ġgl itch +U B +ĠGr an +Ġad alah +Ġl ent +ر ا +Ġtr action +Ġadjust ing +´ ¤ +ниб ÑĥдÑĮ +Ġд оп +Ġstretch ed +Ġor t +Ġcos ine +vi ol +Ġì ħ +c ir +Ġbast ard +ä¸ ĩ +ĠÑħ од +Ġqu ier +Ġpress ures +ĠAn h +å¹ ¾ +Ġell es +Ġд ÑĢÑĥз +ĠможеÑĤ е +Ġch á» +ĠM é +ö k +ầ u +ìł Ī +z in +Ġca ution +ib an +Ġjud ging +ÑĥÑİ ÑĤ +Ġb aj +ĠС ейÑĩаÑģ +ĠPo or +ĠNaz i +Ġup beat +y ang +Ġweek ends +ĠEss entially +Ġol uyor +Ġspat ial +ack er +Ġsell er +Ġ×IJ ×ķת +ij ׾ +Ġv ivid +ĠB ond +ê ¶Į +is kt +ãĤ µ +Ġgo at +dri ver +Ġm ug +ict ional +Ġall t +ĠIn iti +ĠR and +Ġfinish es +Ġê° Ī +Ġvit am +Ġteen agers +ĠMor ris +ì¤ Ħ +ĠO ri +i ya +Ġmy ös +St ep +ĠK re +è¾ ¦ +Ġdin osaur +Ġëª ĩ +aff e +ĠëIJ ©ëĭĪëĭ¤ +Ġz eg +åĪ ĩ +ĠManh attan +Ġsu jet +ue lle +st off +Ġd ür +Ġsub mar +es es +Ġa quele +Ġn ou +ĠFa ith +t z +ĠÑĤ омÑĥ +ace ut +li ers +Ġband width +ưỠĿ +Ġrespect ive +ĠA ve +Ġspread she +ĠS ent +ic amente +Ġinf ra +Ġlearn ers +Ġà® ī +ai ah +ren al +Ġmust ard +Ġhab t +ç ĥ +ĠQu é +Ġanaly zing +æ¯ ı +Ġso lic +Ġ×Ķ ×ķ×IJ +Ġcaus a +Ġwel comed +ĠS uccess +Ġfac ile +ĠÐŁÐ¾ÑĤ омÑĥ +sche in +Ġf etch +Ġstr at +ĠÑģÑĤо иÑĤ +ìĹIJìĦľ ëĬĶ +ĠÑģп оÑģоб +m am +Ġser ÃŃa +nam ents +wr iter +Ġconsult ing +íĺ Ģ +ĠBer keley +e u +as ive +U U +ĠAnal yt +Ġsubm ission +Ġmagnific ent +en za +Ġe con +Ġprof iles +Ġinc ar +A b +ĠN un +Ġh ic +scream ing +Ġresil ient +åĪ © +gr und +Ġconc ur +Ġbere its +L D +Ġnur t +ì ī +Ġfe ast +Ġenc uent +ĠMich el +Ġsup rem +" ] +Ġfeed s +ĠKoll egen +iss er +ĠF eng +ĠW en +m un +Ġten ÃŃa +ĠW rest +Ġìĺ¤ëĬĺ ìĿĢ +Ġst ead +Ġrest oration +Ġdon ated +Ġdel s +Ġc ensus +Ġdesper ately +worth y +H E +ĠSp a +ĠBry an +Ġh j +ĠR aw +ìķĦ ë +ĠCam era +Ġz ien +Ġst yl +ĠT W +ĠChe ese +bor ne +Ġob l +ĠAl ready +Ġunst able +Ġfl ames +p ost +H a +rom agn +ĠìĹ Ħë§Ī +d est +Ġkole j +Ġtempor arily +Ġdeterm ining +ĠGl ass +ÑĢ Ð¾Ð½ +ol an +Ġdom inated +åĮ ĸ +__ __ +ĠÙĩ ذا +ĠD ana +Ġdin heiro +a qu +ë ¯¼ +ĠÃł s +ĠJo ey +ĠGr iff +Ġatt ain +Ġtrans itions +ĠLiter ally +ен д +ĠHa ven +Ġgrab bing +Ġcryst als +ĠFour th +Ġcand les +ĠÑģлÑĥÑĩ а +ric o +Ġ5 000 +et to +Ġund o +Ġk to +Ġdi vert +Ġch ir +Ġper sec +Ġh iking +Ġannounce ments +çĶ ± +з Ñĭ +Ġa uc +Ġsystem ic +ĠR M +Ïĥ α +ĠÐĶ Ð¶ +Ġy ar +ĠW ard +Ġpiss ed +Ġcar n +Ġautonom ous +ãħİ ãħİ +so ver +æ²Ĵ éĮ¯ +å¾Ī 好 +Ġref lex +Ġgard ens +Ġd ated +ì ± +ami ÄĻ +Ġcontinu ity +Ġcitizens hip +Ġsch wer +Ġz ak +t able +ĠÑģ Ñĩ +è§ ģ +ĠÏĥ ε +Ġgener ates +구ë Ĥĺ +ö h +ó m +al am +ĠJUD Y +ĠB ug +Ġãģ ¦ +Ġdr ones +Ġá gua +ac aks +æ ļ +ĠÐļ он +× ĸ×Ķ +Ġstri ve +ĠAl tern +Ġne arest +Ġpro yect +ter a +ĠASH LEY +Ġwor m +Ġre play +Ġt ara +ĠInd ians +ãĤ ° +ica id +ĠìĪ ľ +Ġappe aling +ĠW es +Ġment ions +Ġдел е +Ġk w +Ġfrag ile +is z +k ów +h ang +col or +Ġpresident e +8 7 +е ÑĦ +çĪ ¸ +Ġдоб ав +ĠN elson +á fic +ĠMIC HAEL +Ġmechan ic +Ġmet res +Ġo czywiÅĽcie +ĠC ind +Ġog sÃ¥ +Ġlands ca +AC E +Ġhead lines +Ġcat alyst +ĠC atch +ink les +Ġp ills +ord o +Ġimmig rant +Ġexam ination +Ġacc idents +zÄħ d +Ġqui ere +Ġne lla +Ġ6 7 +Ġpass a +Ġsuper fic +ist or +Ġno v +ëĭ µ +Ġmand ate +is ons +ĠVirt ual +Ġsel ber +Ġcounsel ing +ĠN BA +Ġse pt +Ġbelie ver +Ġmar vel +ĠInte gr +Ġм Ñĸ +Ġor ph +Ġback ward +ĠGen eration +ĠP ict +ĠÑĤо ÑĤ +Ġtap i +pro chen +Ġhall way +ht e +ĠÛģ ÛĴ +ĠZ um +èĢģ 師 +ach ment +iqu er +fol g +ĠEd die +ĠK il +Ġwell ness +st ock +è¼ ĥ +Ġka ç +Ġterror ism +Ġpo inter +O f +her ic +ĠUlt imately +Ġmes es +ĠTr ade +Ġp int +Ġtu ition +Ġdisag re +Ġê²Į ìŀĦ +Ġmanus cript +Ġro omm +Ġoutput s +е ÑĨи +Ġr ies +Ġsal ud +otz dem +Ġmass es +Ġby ÅĤa +Ġclear ing +Ġdisc ourse +ats on +Ġfold ed +ĠJ ar +ÙĦ Ùī +9 00 +ĠÑĥ Ñģп +Ġprophe cy +Ġinterf ere +иÑħ од +๠Į +Ġth ri +Ġ×ŀ× © +Ġlaz ım +Ġ199 2 +Ġfut uro +Ġlock ing +Ġembar go +ĠNe ither +iv amente +ĠmÃ¥ ste +Ġm ik +Ġcollect or +еко ÑĤоÑĢ +ĠG and +Ġsent ir +ĠM ight +å¡ Ķ +Ġgan zen +U C +Ġrel ating +S D +Ġmos quito +G R +Ġho llow +âĺ ħ +ĠWalk er +Ġaffili ate +Ġduplic ate +н ем +Ġgra pe +ĠOrgan ization +Ġsy nt +J oe +Ġg eg +Ġreve aling +ĠEth an +out er +Ġy ay +é« Ķ +л аÑĢ +Ġreported ly +Ġihr er +Ġrecogn ise +Ġbum per +ĠR andy +ĠVen us +t les +Ġappet ite +Ġgluc ose +Ġch odzi +ĠFurther more +t ir +Ġcont a +Ġint uition +Ġalt itude +Ġch unks +ĠJosh ua +ıģ ım +ry lic +le ans +ĠíĶ ¼ë +L L +Q ue +Ġg or +Ġзна ÑĩиÑĤ +Ġpo ems +Ġexc el +Ġexpl ored +Ġpop ul +Ġinclus o +st ä +ĠG avin +all ing +ĠÏĦο ν +é © +ar beit +ĠG as +Ġgl orious +rie ben +Ġsp am +Ġindo or +Ġthr ust +ĠA ld +ĠPri or +Ġon board +ãģł ãģķãģĦ +o ca +AS H +£ ł +ĠChrist ine +Ġdra wer +Ġno on +Ġìŀ ĺë +Ġperman ently +æ· ± +ĠнапÑĢ Ð¸Ð¼ÐµÑĢ +Ġpodcast s +era peut +pr it +Ġstain less +ĠÚ© ÛĴ +Ġfamil ia +ĠÑĢаз ÑĢ +un to +ĠÑģÑĤ ол +Ġh ä +ĠH ai +ĠP B +iz on +Ġkon nte +Ġbüy ük +Ġutil izar +Ú Ĩ +Ġaqu esta +Ġmix er +ud ent +лек Ñģ +ÅĤ u +ĠÑģиÑģÑĤ ем +Ġн оÑĢм +Ġfat al +Ġconsider ations +Ġvalid ation +Ġo li +Ġk ardeÅŁ +ĠGL ORIA +Ġp all +еÑģÑĤ е +Ġrect ang +Ġmed ieval +allah i +ast i +ĠSy rian +Ġshe ar +Ġdeb ug +ĠM ai +Ġknock ing +ĠLe x +ard an +ro v +Ġmem orial +æ° £ +ook y +Ġstuff ed +Ġpass é +Ġw ig +Ĥ ł +Ġpróxim a +Ġ199 1 +Ġм еждÑĥ +Ġnuest ros +ĠBe ast +Ġsm o +atch ed +olog ia +Ġм од +Ġge e +Ġconcept ual +Ġà ´ +Ġdecre ases +Ġquer ies +олÑĮ ÑĪ +ĠA part +Ġex empl +å± ± +Ġfl ed +ĠO FF +gg ak +Ġbe ad +h ir +l ies +ĠClear ly +ı lar +Ġch ess +Ġwhich ever +Ġ9 6 +Ạ± +Ġrespect s +Ġм оÑĢ +Ġorgan ism +Ġgrand pa +ĠV ie +è·Ł ä½ł +Ġflo oding +Ġupgrad ed +Ñij ÑĢ +Ġcheek s +Ġcon quer +Ġstub born +Ġpuzz les +Ġau ction +Ġre lying +ĠPRO F +ĠEs per +ĠÐľ У +Ġhy pe +Ġposs ibil +Ġimp rison +ĠEr n +ìĹĪ ìĬµëĭĪëĭ¤ +Ġenv ie +Ġresur rection +ä¸į è¡Į +Ġs per +ĠVenez uela +s om +Ġìŀł ê¹ +Ġnouve lle +Ġclos es +Ġ19 40 +Ġqu a +ĠJ ared +ĠP ir +Ġind e +Ġscr ub +uk u +Ġrequ iring +Ġв ами +Ġconsider able +åIJ Ľ +il ia +Ġin ne +Ġmein em +Ġhard ship +Ġtra ps +ro c +ĠìĦ ¤ë +Ġresearch ing +ĠMarg aret +Ġpen ny +Ġbı rak +Ñij л +Ġw ool +Ġr het +Ġflat ten +ç ĩ +à¹Ģภ£ +Ġp ied +ĠCh ap +Ġunder m +Ġf ret +Ġcrash ed +ĠFra uen +ذ Ùĩ +iv an +Ġliter ary +late go +Ġsp äter +Ġsimilar ities +â Ĩ +ĠCor on +ĠC reek +Ġboss es +Ġaccompan ied +Ġdeb ates +Ġassemb led +Ġà ģ +ĠV ai +Ġtr act +Ġsimple ment +ĠAr in +Ġvulner ability +Ġhorm one +I EL +OO K +Ġrel ay +ĠAnd rea +r il +Ġnecess ity +aceut ical +Ñİ Ñī +ous ing +nah men +Ġfoot print +m ap +ĠT ier +ann ya +int end +åĸ ® +å ¢ +Ġdecor ate +Ġzomb ies +ĠHy d +ĠSu z +Ġcampus es +ĠE mb +Ġthr ottle +Ġad min +Ġop ortun +Ġmir rors +Ġident ities +ĠCl in +Ġë¹ Ħë +á¹ £ +ĠO tt +Ġbl ues +Ġimpress ions +- , +Ġv ague +a fe +Ġinfer ior +eral d +Ġmedic ines +Ġpre gunta +os ely +Ġt élé +ĠMon th +ĠLe aders +ĠEgypt ian +Ġr ation +k ers +he its +Ġre cht +P lay +Ġe g +Ġpoll s +ĠWOO DR +Ġsl ots +j am +B oth +ĠR at +ÑĢ Ð°Ð¶ +ĠBr ight +ä¸Ģ å®ļ +á»ij i +ur ious +Ġsing ers +Ġlo gin +Ġt êm +l ation +ĠM um +ưá»Ŀ ng +ĠEd itor +åIJ ij +Ġinnov ations +h ave +ĠS ek +Ġwe aker +ĠG ob +A fter +´ì §Ģ +Ġ문 ìłľ +ãĥ¼ ãĥ¼ +Ġdisad vantage +ç¢ º +Ġg aze +ĠM ack +Ïģ ί +ĠK iss +ĠH olo +ĠBir th +iz i +b ab +ä¿ Ŀ +ìĭľ ê³ł +д еÑĢж +Ġsqu at +кÑĥ Ñģ +un i +ĠComm e +ĠWOODR UFF +ĠChampions hip +Ġwel che +ĠY outh +z em +Ġod pow +Ġpersist ent +r ut +ìĶ © +íĸ ¥ +la ir +ik u +Ġvend or +Ġch úng +Ġfinan ci +Ġover ly +â u +Ġgl uten +Ġ18 00 +Ġdiv isions +Ġciud ad +Ġob ed +Ġwar um +Ġe her +Ġel im +ĠÐĴ о +Ġpeu vent +ĠW anna +Ġattend ance +Ġassess ments +ĠB og +Ġimag ery +Ġcollect ively +Ġinform al +ĠSch we +Ġde utlich +ĠCh el +ĠP E +ow ed +Ġb anner +Ġshel ves +ĠRet urn +æĭ ¿ +LAUGH S +Ġcongrat ulate +ĠNor way +Ġd well +ĠCarib bean +Ġnorm s +ĠAn imal +ĠValent ine +Ġext ending +ĠV ou +or r +ĠCh eng + ¡ +ĠдоÑĢ Ð¾Ð³ +Ġve g +Ġh Ã¥ +ĠX in +Ġì¹ ´ë +em et +Ġhyp oth +Ġinteress ante +ric es +I Z +ĠUS D +Ġrun ner +ĠB ag +Ġê ½ +Ġcomeç ar +Ġpig s +Ġweakness es +P h +ĠVi ol +ä¸į ç͍ +Ġdra gging +ĠAqu ÃŃ +ĠCS S +Ġmill imeters +Ġest ás +Ġac ute +Ġde jar +i ÄŁ +ob ra +L ove +Ġsil k +** ** +Ġjo ins +Ġpro l +Ġê°IJìĤ¬ íķ©ëĭĪëĭ¤ +æĶ ¯ +ØŃ د +agh etti +än ner +Ġstr ang +Ġdoub led +Ġdescri ptions +Ġst ellen +Ġpart i +ç« ĭ +² Ħë +Ġö ÄŁ +ig hing +Ġang ular +Ġnat uur +ĠSh el +ư Æ¡ +Ġr ays +Ġse per +st art +v ised +Ġrush ed +Ġinternation ally +Ġnive l +Ġbox ing +fall en +á»ij c +Ġse inen +plic ity +Ġcarb oh +ĠTra vis +us o +ĠPh ase +Ġactiv ation +Ġop io +· ¨ +Ġdecre ased +C ar +Ġbund le +Ġexp end +orm al +Ġadjac ent +Ġme e +ĠоÑĢ Ð³ +Ġtrans cript +ĠLang uage +G S +è§ ī +Ġse ul +Ãł nh +Ġn ya +ning s +Ġìĭ ľë +ĠëͰë Ŀ¼ +ĠA gr +ÃŃ d +çķ Ļ +Ġab y +ĠNe o +ıyor uz +ĠThink ing +a ime +Ġv ite +Ġtrav és +Ġ×ij× ¢ +Ġм ед +O ur +ho ot +Ġl iner +ĠP izza +Ġhy g +fl ies +ĠContin ue +Ġdent al +ĠT ib +Ġreg ulate +lie ÃŁ +AL K +ĠTa e +ê¸ ¸ +ĠBre xit +ĠG ut +Ġoccup ation +Ġz robi +â m +Ġwh isk +ä¸ĸ çķĮ +Ġkans ke +om on +ro be +Ġwar fare +Ġth á»ĥ +Ġjak i +Ġstro kes +Ġpe as +ĠDam it +H AN +Ġinter ference +Ġмин ÑĥÑĤ +N ER +out ing +Ġtext ures +Ł ī +ow i +Ġíķ Ļ +Ġd ens +Ġprotagon ist +än n +Ġgod dess +Ġwoll te +ij o +ĠWo che +ĠV PN +st ory +Ġkind erg +Ġfun nel +Ġdist ress +ноÑģÑĤÑĮ Ñİ +Ġno isy +ĠпÑĢод олж +Ġdar an +Ġenzy me +л ож +Ġm ute +Ġd war +Ġا س +Ġkom pl +Ġmer it +Ġf osse +ĠDr ink +Ġfor a +Ġw ohl +Ġbree ze +Ġsan it +Ġdr in +ĠìĿ´ê±° ëĬĶ +Ġ6 2 +Ġì° ¨ë +aby tes +Ġde eds +ĠÐ ¹ +i ème +igg ling +Ġ" ' +ĠÑĩа ÑģÑĤÑĮ +ĠAns wer +Ġev angel +Ġ10 80 +ĠVis it +ic ient +Ġreli ability +Ñİ ÑģÑĮ +ĠEar lier +Ġf id +çŃī ä¸Ģä¸ĭ +Ġslee ves +iy orsun +Ġb ib +ĠAcc ount +Ñı ли +cipl inary +z as +Ġб еÑĢ +Ġneck lace +Ġbl ender +ĠPhill ips +et i +ĠJup iter +Ġprov oc +ĠYe ars +ent re +ac io +Ġk ü +Ġanten na +Ġnovel s +Ġf art +ĠS ugar +ĠJud y +Ġcollaps ed +ç ° +rit is +Ġìĥģ íĻ© +ÐĹ Ð« +ĠVer f +rane an +ere um +ĠTar get +Ġ8 8 +ĠÐĺ з +ide o +Ġreg ression +ì¶ ľ +Ġmów i +Ġstud ios +i ens +ip h +Ġfr ying +Ġfasc inated +ĠW ah +b ucks +m aya +ĠSat urn +ĠM ommy +Ġrating s +Ġaut umn +ươ ng +Ġlos er +Ġcent ro +érie ur +ĠF old +Ġsuper visor +ĠNo bel +Ġunder est +ob ia +Ġв ÑģÑı +Ġver w +Ġfu els +Ġartif acts +Ġë¶ Ļ +ĠAut om +çļĦ æĺ¯ +Û Ķ +×ķ× ¡ +Ġih nen +Ġ5 9 +ound ing +еÑĢ Ñĭ +in ars +ch ant +Ġadd icted +Ġexplos ive +Ġdisp ers +â ĸĪ +ax is +AR Y +Ġl um +ĠÑĥ Ñģл +ĠØ Į +Ġru pees +ĠPe arl +c amp +t v +oy a +Ġconclud es +Ġcoll ision +Ġbuy er +Ġplay ground +Ġspr ings +Ġfemin ine +ĠR as +Ġincar cer +íĹ ĺ +Ġdial ect +Ġclos ure +Ġchat ting +Ġb abe +Ġspot light +Ġnot ation +è· ¯ +St ar +i ão +Ġt ête +Ġt ide +Ġjun to +Ġsen ator +Ð ¥ +Ġexcus es +Ġbl ink +Ġadm ission +ĠL ily +Ñĭ ми +Ġam igo +Ġl ust +ëĭ ¬ +Ġam ino +äºĭ æĥħ +Ġconsult ant +ĠElect ric +Ġëħ¸ë ŀĺ +uj ah +Ġshoot er +icht en +ĠUkrain ian +Ġaim s +ĠEnter tain +Ġmir acles +èŃ ° +Ġze igen +Ġl am +Ġres s +ĠJ ill +yl an +Ġro ok +Ġh aya +Ġpass port +ad ata +Ġju icy +con f +л ей +ĠS z +Ġinter cept +ãģĤãĤĬãģĮãģ¨ãģĨ ãģĶãģĸ +ĠTe ams +Ġmak en +ir rel +ĠLI KE +áºŃ y +êµ ° +Ġshort age +Ġparad igm +Ġpap el +Ġast ero +ãģ¾ ãģŁ +Ġsoll en +ĠMic key +ĠOr leans +Ġchol esterol +Ġgo ose +ÑĨи Ñİ +ãģĤ ãĤĭ +ĠF L +Ġгол ов +Ġtrib ute +ĠG am +Ġé videmment +Ñı Ñħ +å® ŀ +çĶ ° +Ġin appropri +uh an +Ġorganiz ational +ail ed +Ġend ure +Ġ7 6 +Ġshot gun +Ġliv re +Ġsu ited +Ġwarm th +ĠS IM +Ġenv ision +Ġde grad +î ne +La ughing +ĠWho ever +ĠBuddh ism +Ġspr inkle +ceÄŁ iz +Ġru ins +Ġst arch +ĠHer z +Ġinjust ice +Ġhum idity +ожал Ñĥй +ĠOb ject +ĠI gn +ĠEx am +ig ers +Ġth ou +ĠSo y +iv as +Ġpol es +m ath +Ġв ним +ING ING +ed ral +Ġexpl or +Ġroast ed +Ġcraw l +Ġco ff +Ġan om +Ġw ij +Ġimpro ves +Ġtreat y +Ġdiscover ing +Ġstat ute +Ġmerc ado +ĠÑģ ил +Ġint el +ĠChance llor +ĠMed icaid +ug i +Ġver bal +Ġd ön +Ġscript ure +Ġit eration +ek s +ĠOx ford +Ġw äh +ĠV ad +ĠA K +ĠìķĦ ìĿ´ë +Ġi ets +Ġneed les +Ùĥ Ùħ +Ġpas ado +Ġalbum s +Ġye a +et zen +Ħë ıĦ +Ġdeterm ines +Ġthe e +ĠPlay ing +är t +Ġ× ¦ +c led +Ġdown ward +al one +Ġsol u +Ġpart ition +Ġw z +d d +Ġpesso al +å ª½ +Ġfact ories +Ġble ibt +ม า +als a +ĠNF L +Ġfu era +Ġres erved +ĠE arn +Ġhel t +Ġshort cut +Ġconvin cing +sp ace +Ġen force +Ġc ores +Ġe fter +Ġrecess ion +x ico +Ġprop osition +ar ians +rop ol +Ġëª °ë +ĠÎ ľ +ĠìļĶ ì¦ĺ +Ġactiv ist +Ġconv iction +Ġz ab +Ġcancel ed +ÑĤо Ñĩно +ĠÎ ® +éĢĻæ¨£ åŃIJ +n ite +Ġfund ra +buz zer +ел о +ic ations +Ġz ona +Ġte ens +Ġmethod ology +Ġì¤ij ìļĶ +th an +ĠU l +ĠG rey +Ġh og +IN K +ĠS ung +ĠC laud +ĠCN N +Ġdel ivers +al in +ĠAd obe +ot he +ĠDes wegen +ภ³ +Ġwer de +Ġgre ase +Ġup grades +ĠFin land +ac cept +Ġinter rog +be e +Ġãģ « +Ġpre de +ĠN ep +ĠCam bridge +Ġgraph s +Ġha unted +Ñģ ем +æ § +åħ ĭ +S ome +ĠM all +Ġrehears al +ĠUr ban +ĠL ag +Ġn im +ê° ķ +Ġposition ed +Ġavo ided +EM A +Ġlleg ar +Ġráp ido +Ġgou vern +Ġh ing +Ġdeal er +Ġreform s +Ġfat ty +к ол +ĠA ce +Ġne p +Ġì² Ń +Ġcomput ation +ĠSt ream +bour ne +t ur +P or +Ġsleep y +Ġbang et +ãģĤ ãģ® +Ġwe ighs +Ġble iben +ĠG ren +Ġun ions +Ġêµ IJ +Ġap render +uit ar +ĠJ est +um ing +ĠPlay er +ĠExt rem +Ġinteg er +аÑĩ е +Ġconcert s +×ķ× Ľ +Ġtro chÄĻ +ĠRe pe +éĩį è¦ģ +๠Ĥ +ż en +Ġsound ing +Ġan onymous +Ġex ca +ĠIran ian +Ġener getic +Ġw ives +ĠÑĨ веÑĤ +Ġa is +ãģĭ ãģª +Ġsud ah +Ġunder wear +Ġcrunch y +ĠP ain +Ġger çek +red ict +Ġm isma +Ñĸ ÑĤ +Ġsurv iving +ÎŃ ÏĤ +Ġparticip ant +ĠH essen +ári as +Ġsub way +ist ä +Ġcor al +Ġmar ijuana +ĠMem orial +ÑĪ Ð¸Ð¹ +ri z +Ġsatell ites +Ġle ase +ĠCam eron +um ph +Ġclass mates +äh än +ÑģÑĤв е +Ġh ue +ĵ¤ ìĿĦ +Ġproport ional +Ġn oss +Ġl aps +r Ã¥ +Ġbit coin +ÐĹЫ ÐļÐIJ +Ġì¶ © +ĠÙĦ ÙĦ +ĠM ort +ĠEs p +arn os +ĠÑģказ ал +Ġä nd +åħ Ħ +×Ļ ×Ļ×Ŀ +ĠGe b +ge hen +I naudible +bor ough +ÑĦ ÑĦ +Ġfellow ship +ĠP aper +Ġcur ved +ĠGE OR +Ġcalcul ator +ĠCat al +ĠvÃł o +Ġby pass +л еÑĤ +à ³ +tr ans +ren cies +ì ¡Į +ig ent +Ġtast ed +Ġo ceans +u ft +erv ice +ĠÐľÐ£ ÐĹЫÐļÐIJ +ĠClass ic +Ġrespect ively +~ ) +î tre +ĠN ash +Ġz it +ĠìĽ ĥ +ĠëĨ Ĵ +qu ote +ĠUn s +Ġt ac +Ġpro ves +ĠPort land +b ly +Ġ ere +ì¶ Ķ +Ġépo ca +ĠÑĤÑĭ ÑģÑıÑĩ +7 6 +Ġhad e +ĠF ro +ĠpolÃŃt ica +t ag +Ġíķ Ń +Ġsch ö +are tt +Ġprov isions +Ġmot ors +Ġimag ing +Ġdo k +ul ously +Ġme ille +çݰ åľ¨ +ë IJ +ĠIS O +ĠST EM +ĠBow l +Ġto wers +ĠE e +ĠPerform ance +Ġlo in +cuss ion +Ġcoast al +ial e +com pass +Ġspell s +Ġdisappoint ing +Ġë²Ī 째 +E ER +Ġvers atile +as ury +Ġen fin +Ġdown side +Ġgu iding +ĠاÙĦ ÙĤ +Ġnin ety +char ged +ĠF ans +Ġphilosoph ical +Ġg arn +ĠmÃ¥ nga +Ġwilling ness +Ġport ions +ab en +Ġ ï + ¿ +ra ul +Ġspr int +if en +ıy la +Ġк Ñĥп +ãģı ãģłãģķãģĦ +Ġens uite +ĠCap itol +Ġ6 3 +ĠговоÑĢ Ð¸ÑĤ +Ġappoint ments +æī ¾ +omi ast +Ġcare g +Ġpubl isher +Ġher aus +Ġε ί +ĠV S +ãģĿ ãģĹãģ¦ +ä¸Ń åħ± +Ġsacrific es +th ird +Ġhuman itarian +ĠëĤ ´ì +im on +Ġine qu +Ġz ob +Ġcomfort ably +ĠD inge +Ġcancell ed +ĠPS AKI +ĠRob inson +Ġfin s +) ? +ĠHist or +ĠÑĩеловек а +Ġt bsp +te xt +k im +Ġupd ating +Ġgel d +f eld +ı ¼ +Ġm ä +Ġcaf é +Ö Ģ +ĠS ri +ĠReg ion +ĠH ahaha +Ġfin ances +ĠاÙĦØ ´ +Ġb unk +ru k +ha ft +Ġlater al +Ġext ensions +ĠìķĦ ìĿ´ +Ġdefin ite +ĠZ hao +ĠLu is +st y +Ġcas os +ĠK lim +Ġ199 3 +Ġreal ization +Ġhistor ian +Ġcrack ed +ëĤ ´ +Ġsyst ème +ĠC IA +ĠÑĤ во +osp heric +Ġfle e +Ġr ất +ĠRegard less +Ġrel uct +Ġtim ely +ĠJul ian +G M +é Ĵ +ad ura +é£ Ł +Ġdress es +çģ £ +ĠëĶ Ķ +Ġnom inated +Ġadvoc ates +ym ph +Ġrecord ings +Ġdev iation +Ġpriorit ize +Ġspir al +ĠYOU R +Ġtransp ose +amp oo +ĠìĽIJë ŀĺ +ĠV ision +Ġpol ite +Ġha mb +ĠPat ient +æ¯Ķ è¼ĥ +íģ ¬ë +Ġs ia +Ġê³ ³ +Ġž e +è§ Ģ +Ġsuper market +ë ¹ +ĠS ierra +Ġgr illed +ĠUp on +Ġabs ent +Ġme c +ĠAp ollo +Ġp unk +ĠPa ÅĦst +ĠÑģв ой +Ġê±° 기 +G irl +Ġskin ny +ĠPrem ier +Ġterrit ories +Ġli ability +Ġj erk +r atic +Ġdan cers +ĠÑĥ ÑĢов +Ġê´ Ģë +on ly +ĠSt u +Ġske leton +ĠëŃ IJë +Ġзак он +ı kt +ĠMI KE +Ġl ö +m ie +Ġre iter +ãģĵãĤĮ ãģ¯ +ĠKoll eg +ĠAd ams +lich er +Ġçoc uk +Ñı г +Ġbl ush +Ġsun shine +Ġe z +ĠDev il +Ġê¸ ¸ +Ġãģ Ĭ +ad d +Ġlic ensed +Ġv inyl +ĠC zech +im ag +Ġcrack ing +Ġì º +Ġud ah +Ġs ommes +Ġìĸ¼ êµ +wa Äĩ +Ġf res +åij ½ +ĠWal mart +ĠТ епеÑĢÑĮ +at isf +C I +l ang +Ġdiff usion +çĶ · +Ġsom os +ĠM akes +æĪij æĥ³ +ĠRick y +Ġmuch a +íķ ¨ +Ġhorse power +as ia +Ġfib ers +Ġ erm +Ñģ кие +Ġjest e +Ġfire fight +Ġcu isine +Ġbesond ers +d ig +Ġì¢ ħ +ĠÑĥ ж +Ġtr acing +Ġcertain s +ĠApp ly +Ñĭв аÑĤÑĮ +ç Į +Ġbr u +ĠY ES +ĠB ai +ĠD it +ĠB is +Ġun le +ÑģÑĤа ÑĤоÑĩно +ĠAw ak +.. " +Ġ12 5 +Ġroot ed +Ġcaut ious +con st +Ġorchest ra +çľ ¼ +Ġвн ÑĥÑĤ +Ġquel qu +ĠоÑĤ веÑĤ +ĠMet hod +ì¹ ľ +Ġμ αÏĤ +l ü +ĠìķĦ ê¹Į +Ġn aming +C har +ĠS icher +Ġprivile ged +ĠF ly +Ġãģ ĭ +áºŃ t +Ġadv ances +ĠZel da +Ġand ra +Ġgr inding +ĠEd ition +p f +Ġwarri ors +Ġh edge +Ġuns eren +ĠÑģÑİ Ð´Ð° +el iness +Ġpersonal ities +Ġf ö +' M +ĠÑĤо Ñĩно +Ġsh ipped +Ġmete or +Ġsurround ings +ĠF ill +u esta +ĠPerson al +ĠAll e +OR T +ä¹ ħ +ĠS che +V I +Ġcompar able +dam n +Ġd itch +Y AN +ism us +Ġpick up +Ġd ak +ĠE P +b est +ĠS ue +äll t +Ġpop corn +Ġfold ing +h ome +ив аеÑĤ +å·² ç¶ĵ +Ġan not +ch uck +Ġfier ce +Ġdam aging +Ġfl op +Ġpas ar +Ġre ef +ĠÑģво ей +Ġz oo +o vers +j ets +Ġpr ès +ĠSil icon +te ok +ĠS eth +at amente +Ġtransm itted +Ġrepl icate +Ġsl im +ĠC ream +æĦŁ ãģĺ +Ġside walk +ìĪ ĺë +Ġжиз нÑĮ +ĠMon ica +ä¾Ĩ äºĨ +Ġcop ied +ĠTer ra +ist ent +ç³ » +Ġо но +Ġwh ale +ĠW ITH +л ÑĥÑĪ +å½± çīĩ +ĠE en +ĠÑģво и +Ġord in +Ġpl ural +Ġsp okes +Ġdisp ute +Ġsens ible +Ġpre aching +Ġktó rzy +pt ed +av ier +Ġpist ol +ĠTap i +Ġ ÅĤ +ff ff +Ġac rylic +Ġignor ance +ĠZ iel +r ans +Ġweld ing +m id +æĪij ä¸į +Ġзан им +Ġlan es +Ġmin es +Ġmom s +×ķ× Ĺ +ĠCham ber +t ier +Ġmod est +ĠìĹ¬ê¸° ìĦľ +Ġun as +Ġw rench +hand ed +Ġsatur ated +ĠF ang +ĠCommission er +ठ° +Ġ× ĸ +ĠLouis iana +ĠM ask +Ġcub es +ìĶ ¨ +Ġvidé os +ĠnÃ¥ gon +Ġr ider +Ġì¶ ľ +Ġs ón +ĠLat ino +b ank +íķ´ì £¼ +ĠB rend +Ġsexual ity +... , +Ġforget ting +Ġ ÛĮ +ĠAven gers +ĠBon jour +cess or +кÑĢа ÑĹ +c ence +Ġge ograph +cul o +о ÑģÑĤÑĮ +Ġswe ating +íĥ Ģ +Ġsymm etry +ts Ã¥ +Ġj an +ĠFer r +é¦ ĸ +Ġamb assador +ziÄĻ k +Ġmus un +ĠÑĥ ÑĤ +ĠL G +iss ent +comm un +Ġcour s +Ġdevelop s +Ġbron ze +Ġsubst ances +dri ven +주 ìĦ¸ìļĶ +Ġa os +åĦ Ħ +ĠPROF ESS +h alf +Ġsort ed +ĠB omb +л аг +ĠMalays ia +ĠChrist ina +Ġteam mate +èģ ŀ +F T +Ġk ı +heart ed ++ + +ogen ic +Ġbell s +ĠOu ais +Ġspecial ists +б Ñĭ +dep th +lass es +g ies +ĠCo ffee +Ġmark ing +Ġfo ll +ul i +Ġad hesive +ĠB ot +ĠP unkt +e ye +ĠB ub +el ong +åĪ ¶ +ĠпÑĢ Ð¸Ðº +Ġdon or +8 4 +Ġen for +Ġcatch es +Ġbr icks +Ġkn itting +ĠKnow ing +ok s +H Y +r ide +ĠFant asy +im an +Ġp se +Ġìĺ ¨ +Ġв д +Ġrest ra +Ġevalu ated +ÑĢ ÐµÐ² +Ġfortun ately +Ġche gar +ر ب +Ġdom ains +ib i +ar ry +Ġshut ter +Ġfic ou +M ike +Ġinc lu +Ġdon ors +Ġa pl +ĠL ower +Ġimport ed +Ġacad emy +Ġfin als +Ġdisappe ars +ÙĬ ا +Ġadministr ator +j s +Ġcut ter +Ġr anging +ör per +Ġconstra int +ĠT able +ĠSh an +v ic +ĠF ix +ĠSw ift +oun ces +ĠWar um +Ġlett uce +app elle +Ġsh ave +Ġb ás +Ġ7 7 +ĠO oo +a o +ĠMc M +ĠD rew +Ġl ump +Ġl ashes +schein lich +R ep +in is +ĠC ette +Ġcompos ite +emet ery +Ġsort e +ĠFin ancial +он е +ron es +ĠV oy +Ġt éc +ł ¹ +ĠNin ja +ĠCor in +ен нÑı +ìĿ´ìĹ Ī +Ġn ich +Ġdetect ive +â̦ " +Ïĥ ε +Ŀ¼ë ıĦ +Ġë³ Ģ +Ġë¸ Ķë +Ġpro pe +ĠW right +Ġ×Ķ× ª +ĠSh i +Ġãģ Ł +Ġinvestig ations +éĤĦ æĺ¯ +ĠPower Point +ĠCh u +Ġìĺ ¤í +ĠìĻĦ ìłĦ +ĠFra gen +un ning +Ġpour rait +Ġtext book +м Ñĭ +Ġf ahren +Ġ ÑĤоÑĢ +Ġl akes +ünd e +I nt +ĠMet ro +Ġmans ion +Ġа б +ĠZh ou +Ġcorrid or +Ġesc ol +Ġindic ating +ia ÅĤa +Ġm ommy +Ġarch ives +Ġfound ers +eng ine +ĠDie u +Ġsick ness +Ġë³´ ëĭĪê¹Į +Ġar b +Ġn ed +ĠCh op +Ġco vid +Ġsl am +Ġpublic ations +D C +Ġsp ends +æ ¾ +Ġrefuge e +Ġd ile +Ġ×IJ× ĸ +ific ar +ĠS ach +G u +Ġre load +?? ?? +Ġje ÅĽli +ĠÑģ оÑģÑĤо +Ġsim plicity +Ġbull ying +Ġм ол +Ġreal idad +Ġuncle ar +app a +le vant +ĠIS IS +ĠW atson +Ġde in +ĠMic ro +íķ ľë +ü g +Ġdev am +Ġtwe eted +å° İ +Ġunderstand able +at an +Ġvers a +Ġpre ca +Ġv á»ģ +ĠCop y +ĠOr acle +Ġmindful ness +Ġdisc ret +ern en +ĠP le +H ave +Ġisol ate +Ġde u +Ġsevent y +ĠH ills +Ġarc ade +ĠÑģп еÑĨи +Ġsigu iente +ĠB ÃľNDNIS +lig a +ĠвÑģÑĤÑĢ ÐµÑĩ +ô m +Ġtwe ets +Ġsch auen +Ġcrit ique +ĠðŁİ µ +Ġst att +ĠÑģам ое +ân cia +Ġsuper natural +Ġplug ged +F l +yn ı +ĠTamb ién +Ġencourage ment +ĠSer ver +ëĤ ľ +up a +Ġast on +Ġhe ars +ÑĢа Ñħ +Ġsch e +Ġr ats +Ġrec uper +Ġun ten +ĠFight ing +Ġacadem ics +ç¤ º +ĠS ü +Ñģ киÑħ +Ġpa ired +Ģ ìĿĦ +Ġá rea +Ġsweet ness +åı Ĭ +Ġde fer +Ġmuit as +ĠAud io +Ġlock er +ÙĬ د +ĠÑģÑĤ ав +Ġbu ena +AN S +Ġdetect or +av o +be k +Ġα ν +íİ ¸ +Ġdra gged +Ġдолж ен +à ĸ +ر Ø© +ìĿ´ì §Ģ +Ġcell e +ck ing +ĠاÙĦØ ¬ +ĠCan vas +Ġespa ñ +Ġgl imp +Ġspread s +ong o +ĠM ason +ĠIn g +Ġê°Ģ ëĬ¥ +ÏĦ ικ +Ġsec ular +Ġb ater +Ġinqu iry +Ġenerg ies +Ġmanufact ured +Ġveget arian +Ġpine apple +ÑıÑĤ а +Ġpractition ers +2 000 +Ġíķ´ì ļĶ +ĠìĹ¬ëŁ¬ë ¶Ħëĵ¤ +Ġë¶ Īë +ĠJeff erson +ĠJo an +Ġtr am +å® ¹ +ch mal +ĠH ait +á¹ ĩ +Ġun real +Ġsymbol ic +Ġste alth +Ġspl ash +ĠEntertain ment +Ġmetall ic +?" . +è¶ Ĭ +ar ound +Ġdesp air +ĠNev ada +ĠFin ance +Ġk rie +ĠL ux +ĠSm ash +ke eping +Ġз аг +Ġnarc iss +Ġdz isiaj +Ġtoler ate +o ard +Ġlink ing +ĠEconom ic +Ġì ¼ +Ġmor ph +ĠN ak +ĠB aker +at on +r ings +ĠP eng +ĠAir port +ãģĭ ãģ£ãģŁ +íķĺ ëĭ¤ +§ ģ +pr ints +Ġhad i +Ġemp ir +ĠL ives +ann ers +Ġн им +ĠPROFESS OR +Ġpositive ly +ant om +Ġbad ge +ke lt +Ġinter fer +Ġfulf illing +Ġvisual ization +éĹľ ä¿Ĥ +ĠPr ice +� � +Ġscen ery +Ġpr one +Ġw izard +Ġb anyak +ver b +s ky +Ġwish ed +Ġrail way +Ġü zer +Ġalgu ien +ĠA W +Ġкол иÑĩе +Ġreact ing +ĠB uch +ภ¶ +Ġan th +Ġsi h +Ġh ust +ĠSc reen +il ant +ah o +Ġfragr ance +Ġelev ation +ĠMed iter +Ġë ¿ +Ġé qu +Ġwra ps +Ġin ert +Ġrecre ate +л аÑĤ +Ġbo leh +Ġharass ment +unk y +Ġglimp se +reg ierung +Ġfut ur +Ġreposit ory +Ġeng ra +Ġtraff icking +ass is +ĠTre k +Ġë² Į +Ġë§ Īë +ĠK ab +ani u +g ive +Ġdin osaurs +Ġfe ather +Ġatt itudes +Ġpl um +ĠR S +ĠAn fang +ill ery +ĠìĬ ¤ +M Y +Ġtrze ba +Ġsk ies +ĠA j +ur able +C U +ĠSh ane +Ġdepart ure +ĠT ON +iet en +r ats +æ° Ĺ +is u +Ġb ord +Ġinteresting ly +çĻ » +oug hing +Ġr ushing +Ġvol atility +Ġp yt +Ġform ats +Ġз аÑĤ +Ġê¼ Ń +Ġwhat not +Ġcomp ort +s w +ore an +ĠRel ax +Ġcl an +ĠA H +Ġpe w +Ġdiction ary +T ake +sh irts +ĠH ugh +ĠعÙĦ ÙĬ +ĠP ic +Ġenroll ed +Ġjed nak +Ġoffer ings +Ġcor az +L ife +Ġ !!! +Ġcl er +ĠVide os +ĠRod rig +ĠId ent +ĠP os +ĠSt age +ĠR ace +Ġen act +ãģĦ ãģ¾ãģĹãģŁ +ĠG y +ĠHis pan +Ġdef ence +ĠCamp bell +m atic +Ġrele v +Ġpe ach +Ħ¸ ìļĶ +Ġparad ise +Ġcere mon +Ġannoy ed +æĮ ĩ +la x +Ġexplo it +Ġcla use +ek er +ĠBlo om +n ant +ate urs +Ġhe ights +E ven +Ñģ он +Ġoutra ge +ĠVietnam ese +ãģ¯ ãģ¯ +T R +Ġe er +Ġcann on +ĠCom b +IJë §Į +è» Ĭ +Ġê²ĥ ëıĦ +Ġaccomplish ments +ĠAnalyt ics +Ġshap ing +re iben +Ġb achelor +Ġfing ert +ack ed +Ġpyram id +ĠStew art +á st +Ġsurviv or +Ġdu ct +Ġdeal ers +æ´ » +ع Ùħ +ли н +Ġed e +×ķ× ¢ +ĠÙĥ اÙĨ +ĠÏĦ ι +Ġcho oses +ĠO wn +го ÑĤов +h ire +алÑĮ нÑĭе +ĠÐĽ Ñİ +Ġо ÑģÑĤав +te ch +Ġdro it +Ġsubject ive +en es +Ġdiv is +ave z +Ġmaneu ver +à¹Ħ à¸Ķ +ade ce +ĠEn s +ac ial +ĠProt ection +ĸ ´ +Ġform ally +Ġwy d +ingu ém +Ġz iem +Ġrecru iting +×Ļ× ļ +n em +Ġforb idden +ĠB apt +×IJ× ł×Ļ +Ġsubs et +ĠMag az +n ement +Ġaqu ela +rag on +Ġcomm ittees +Ġéta ient +ud i +ĠDa wn +Ġb ore +Ġcompos er +ĠwiÄĻ cej +ang a +Ġdis like +ĠD ays +åŁ º +Ġpar al +Ġm ientras +Ġheaven s +ãģ Ĵ +he id +Ġtrad ers +on ce +Ġmasc ara +ĠÏĢ Ïģο +Ġwhis per +ĠMus k +éĽ Ĩ +ĠFamil ie +All ah +ĠOl ivia +ĠPr os +Ġol ika +il im +Ġrép ond +ĠP eters +Ġ å¾Ī +Ġbit es +Ġv ic +ĠN Y +em ption +Ġ4 50 +Ġvisual s +Ġlie u +ück en +ĠSte el +ĠG P +w ait +Ġnotice able +uch a +Ġreh abil +Ġreject ion +ĠÑģлед ÑĥÑİÑī +Ġsl ider +Ġregard ed +Ġgrav it +ĠRes erve +c ount +Ġbre eding +Ġlon ge +ale b +Ġkn ight +Ġв ой +Ġprés ent +Ĥĺ ìļĶ +ĠSpec ifically +Ġpos es +Ġve ure +ok ay +em as +Ġ ãģ§ãģĻ +Ġma jÄħ +Ġweb inars +Ġcann abis +Ġdam als +ĠNorth west +Ġp ada +Ġcrowd s +Ġfut ures +Ġä n +Ġciv ilians +ĠS achen +æ į +Ġtr aces +Ġ먹 ê³ł +Q U +é¡ĺ ãģĦ +ĠI F +an ın +ìĤ ´ +Ġb iblical +ĠV ed +Ġst oring +ÑĢав лÑı +æĩī 該 +Ġn ast +Ġd ö +ÑĢ Ð¾Ð¿ +el ia +Ġside ways +ĠUnder stand +ĠQ ur +Ġper pend +ĠMill ionen +Ġwater melon +ĠDiv ine +ult ur +ab ord +Ġsuccess es +Ġhom bre +Ġcar p +Ġsus cept +ung kin +Ġk ij +ul us +Ø§Ø ¬ +Ġnot ch +Ġpolynom ial +å¹ ² +å © +Ġún ico +Ġteles cope +Ġpolit ique +k iem +ĠÎŃ Î½Î± +Ġaggreg ate +ĠGe off +Ġtr il +ĠG RA +Ġsubscri ber +im et +Ġдол лаÑĢ +op ing +Ġth erapeut +ĠCan cer +Ġpar ade +Ġir rig +âĻª âĻª +Ġclear er +Ġb og +ĠM aur +า à¸ĩ +ĠShang hai +acht e +ĠK ol +el ujah +Ġha v +ĠCr ime +se k +Ġë ¡ľ +ien na +ĠG or +è Ľ +ĠпоÑĤ ÑĢ +Ġкаж еÑĤÑģÑı +ĠL ift +ĠS ort +ĠP sal +Ġp ing +ĵ Ŀ +ph is +ĠF UCK +ĠS yn +Ġbam boo +¬ ìĺģ +c uts +Ġm mm +Ġfunktion iert +Ġ _ +ÃŃ cio +St op +Ġimag inary +Ġnot amment +ĠIniti ative +ãĥ ¥ +ĠK urt +Ġlo osen +Ġbus car +çģ « +Ġz elf +Ġpro ps +åĽ ī +Ġmoet en +Ġmill i +Ġhall s +ĠM atch +Ġbrack ets +ĠC ou +æ¦ Ĥ +ĠÐľ аÑĢ +IS A +Ġcig arette +Ġcompet itions +ĠM IN +Ġbeh ö +vo or +Ġ ust +ĠZ i +ĠO cc +ul ates +Ġball oons +Ġpr onto +ĠM iy +ĠF ile +Ġкл аÑģÑģ +нÑĥ л +Ġcere al +Ġincre ment +Ġref ined +åı¦ å¤ĸ +pr ising +ĠR F +Ġrespect ful +Ġlo ot +ask et +Ġdeix a +ing le +Ġfuncion a +ĠRe vel +Ġso ber +Ġperform s +ĠG entle +ãĤ ¨ +Ġrecip ient +ĠHa use +Ġë ĥ +F rom +Ġmin isters +Ġpar adox +å°±æĺ¯ 說 +Ġtast ing +Ġ×Ķ× Ĺ +Ġre use +ĠL ane +ĠÑģов еÑĢÑĪ +Ġremem bers +Ġfemin ist +Ġcommit ments +Ġproject ed +Ġg az +iyor uz +Ġoblig ations +R o +z ar +Ġch w +ĠJ AM +ĠbÄĻd Äħ +asp berry +Ġм еÑģÑĤо +ë² ķ +Ġreg ulated +Ġw icht +ĠTre vor +Ġsecond ly +ĠIh re +els h +Ġrep orters +ÑĤоÑĢ Ð° +oy o +G I +Ġinter connect +é IJĺ +OS H +æŃ ² +Ġbr ass +Ġign oring +ä»Ĭ æĹ¥ +in fect +Ġpro jekt +ore t +ÏĦα ν +ĠÑĤ ип +Ġmut ta +Ġunbox ing +Ħ ° +å¡ Ĭ +Ġadv ised +ĠDen ver +Ġsevere ly +ĠM hm +Ġfl ipped +Ġp ien +Ġkomm un +ĠF RE +Ġà®ĩ à®° +aint ed +Ġkn ives +Ġhab l +Ġgew orden +arett es +C S +Ġмал енÑĮ +Ġgal ax +Ġnin ete +ê±°ë Ĥĺ +Ġs is +Ġadvis ory +Ġdr illing +ĠWould n +ün f +gest ellt +ĠHel en +Ġ×ŀ× IJ +ap olis +Ġrze czy +Ġter ra +Ġhe p +Ġalg ún +ik k +Ġastron om +ĠStar bucks +k Äħ +Ġpat rol +Ġì½ Ķ +Ġg on +Ġ ãĢIJ +Ġson st +Ġencoun ters +Ġret rou +Ġshark s +Ġd or +ĠR ever +Ġev apor +Ġreserv oir +Ġalleg ed +ul er +Ġver m +Ġcommer ce +Ġf itted +ge m +Ġtact ical +Ġl ith +éīĦ å¡Ķ +h ad +è® Ĭ +Ġcarboh yd +Ġlength s +ι ο +Ġdem ographic +R ob +ĠS kin +cc oli +Ġsimpl ified +Ġread ily +ĠC um +ades h +ĠD Ã¥ +us st +ig ne +et on +Ġmen or +q i +OO M +à¸Ń à¸Ļ +Ġpsych iat +Ġeight y +Ġм илли +ĠT ob +ed o +ç¶ ² +ĠÄij ến +Ġcirc uits +ĠLAU GH +ic ism +em or +Ġreg ener +eg ree +Ġbure auc +ĠAl ber +ä¹ĭ å¾Į +ĠW or +å¤ « +Ġres in +Ġby ÅĤy +ĠI G +à¯į , +Ġ7 8 +Ġwe eds +ĠMy th +9 3 +æ ¿ +ĠëĤĺ ìĻĶ +é v +á ½ +ö ren +ç ar +ĠP AUL +Ġdisad vant +Ġposition ing +Ġcock tail +Ġagre es +n n +ĠS ally +M s +Ġinher ent +Ġmonet ary +Ġnat ur +ĠN h +ĠImp ort +Ġle ben +Ġw i +uss y +Ġob es +Ġwand ering +Ġìĭ łë +Äħ da +etch up +Ġdispos al +ĠJ A +ĠC er +z illa +Ġvir gin +ĠSl ide +and el +Ġrighteous ness +ĠÎ £ +Ġide ia +ä½ł 好 +иÑĢов аÑĤÑĮ +ר ×IJ +Com ment +Ġpre lim +ĠV ale +Ġì§Ģë Ĥľ +ĠV anc +OM AN +Ġп Ñĸд +Ġy um +st re +ce m +Ġpo cz +Ġfrag ment +ĠÑģлÑĥÑĩа е +Ġunder go +ĠH ank +ce ks +ĠF PS +Ġoc ur +Ġdeter ior +æ³ ¨ +Ġempres as +Pa ul +Ġ) )) +ĠвÑĢем ени +Ġsc old +×Ļ× ¢ +Ġsuspect ed +Ġaccess ing +Ġsubst it +Ġhistor ians +ä» » +Ġдел о +Ġsoci ed +r one +Ġre den +Ġext ends +epher d +Ġbal con +ä¸į èµ· +ĠSol o +Ġpolit ician +олÑĮ но +Ġirgend w +Ġtraum atic +Ġrapp er +ĠRO BERT +Re ally +æģ ¯ +Ġline up +AS E +Ġcontract or +ĠCorpor ation +g or +ĠTod o +ÑģÑĤÑĢ Ð¾Ð¹ +F BE +Ġnews letter +Ġko ÅĦ +alt ies +ĠпÑĢ Ð¸Ñĩ +ĠHe avy +Ġsw ords +Ġmanip ulation +Ġfun k +Ġv Ã¥r +ĠTal iban +Ġë° ¥ +Ġac ne +ür ü +Ġdes wegen +ĠD ust +Ġsil ic +Ġhook s +Ġbl ij +Ġpet its +Ġfil me +ĠBere ich +ĠSa id +Ġimp osed +Ġdi ary +Ġго ÑĢ +ĠG ates +Ġal ta +å¸ Į +Ġch cia +ple asant +Ġë° Ŀ +Ġmoż emy +ĠAust ria +Ġbro ker +Ġsuck ed +èĢ ĥ +Ġcomp artment +Ġcl one +Ġ×Ķ× ¢ +ĠDan ke +Ġnoch mal +ез д +Ġad renal +Ġkle inen +ãģ¾ ãģĹãĤĩãģĨ +Ġsubsequ ently +Ġdecent ral +Ġgen etics +Ġê´ ij +Ġmon itors +ĠApp lic +ĠRep orter +w ert +Ġwie m +ĠMove ment +Ġinterview ing +Ġhair s +Ġpu ò +ĠChel sea +Ġco her +Ġc ot +Ġz as +Ġpatch es +Ġl ah +Ñĥн к +ĠRe agan +ĠMar co +c ity +Ġdef ender +Ġdecor ation +ij i +Ġl itter +Ð ¨ +Ġj ego +RE W +ĠP ik +ĠHe e +ĠI v +Ġи де +ĠThe ater +ĠÑĩаÑģ ÑĤо +Ġswe ater +Ġhighlight ing +Ġa insi +Ġdipl omatic +ĠNever theless +å ³ +AS ON +Ġpúblic o +Ġf erm +reat ed +c od +Ġë¬ ¼ë +Ġm ister +ĠVanc ouver +Ġrecogn izes +ec d +Ġcomplic ations +en cial +ãģĹ ãģı +Ġê°Ģ ì§Ģ +ĠUlt imate +Ġva ig +ĠM erry +×ķ× Ĵ +ĠMar cus +ç¸ ½ +ow ego +Ġm ente +S m +Ġa ja +ĠTa o +Ġjud icial +Ġentrepreneurs hip +Ġнем ного +Ġp is +Ġer g +Ġch rist +ĠC urt +ĠÑĢаÑģ п +λ ε +ens ch +ÃŃ re +Ġfo cal +ĠDiam ond +av ÃŃa +Ġh anno +ĠSqu ad +Ġassoci ations +ĠCreat ive +Ġmess enger +Ġbe gging +Ġdec imal +Ġd Ä±ÅŁ +Ġmet adata +sel s +Ġİ ÅŁ +ữ a +Ġdiffic ile +d ı +Ġs laughter +ĠVer g +Ġ×Ĵ ×Ŀ +ç° ¡ +æĮ ī +ĠTe a +ass es +O k +Ġsynth es +ot iation +Ġpain ter +Ġel bows +Ġarchitect ural +ĠÑĢ Ð°Ð´ +Ġgl or +im age +amp a +cul iar +ł ¨ +Ġte ve +ĠSt elle +ĠB am +Ġì´ Ī +as is +ip edia +ĠG I +ĠAct ive +çĦ¶ åIJİ +az i +ãĤĮ ãģ¦ +ĠL ucky +íķ © +ĠпÑĢ Ð¸Ñħод +Ġrun way +Ġauthent ication +Ġpos ible +Ġsupp lements +Ġsurg ical +G en +Ġfeas ible +D O +Ġout look +Ġinter vals +Ġan ecd +Ãł ng +Ġstra ps +ĠSh u +ud d +iss enschaft +Ġport e +Ġcomm itting +Ġall ey +Ġco venant +ĠPed ro +less ness +ĠSol id +ĠM olly +Ġн екоÑĤоÑĢ +Ġcooper ate +åĮ Ĺ +oll en +Ġtun a +Ġkinderg arten +ĠS iz +Ġduż o +ĠM BA +ĠGEOR GE +ĠF isher +å¿ ĺ +ĠCa esar +ĠкÑĢаÑģ ив +ĠDel hi +zy m +Ġexpl icar +ê°Ģ ì§Ģ +un s +gr ow +ĠпÑĢ Ð¸Ñģ +Ġ8 6 +Ġst ating +Ġmass a +ch ter +Ġì»¬ë Ł¬ +Ġdep uty +S M +n oc +Ġge ography +ĠEnter prise +ĠC ant +ö z +Ġun pack +ĠíĻ Ķë +Ġsearch es +Ġpres idency +Ġtri vial +Ġp ige +ou bt +ãĤ ļ +ì¼ ĢìĿ´ +Ġbudget s +Ġu b +Ġp ne +ĠY ale +ĠÅŁ öyle +reg ular +Ġimper fect +AR A +Ġfam ÃŃlia +ur m +ĠAdvent ure +ãĥ Ĭ +c is +em ark +Ġne go +Ġinappropri ate +ĠпÑĢи з +ĠÑĢ Ð¾Ð» +Ġdream ed +B ry +Ġshut tle +Ġpill ars +Ġb ik +in um +ĠÑĥ Ñģ +ĠNe br +Ġperpend icular +Ġbook ed +ber y +Ġv ikt +be ar +es us +Ġвозм ожно +¨ ¹ +Ġpresum ably +ĠMem phis +Ġambul ance +×ķ× ŀר +Ġthumbna il +Ġmod ification +éĩ ı +Ġinterpret ed +Ġprom o +Ġκ ά +Ġε ÏĢ +Ġacoust ic +ĠD B +åĵ İ +Ġnon etheless +ou le +Ġpe qu +Ġkn ob +ãĤ £ +ĠëıĮ ìķĦ +Ġpurch ases +ĠÃĩ ünkü +Ġdivid ing +per form +ract ion +health y +ĠTit le +Ġu k +Ġcer ca +Ġargu ably +Ġf ale +ë³ µ +Ġgam ers +Ġutil izing +Ġoff ended +Ġt ava +al ı +Ġmed ian +Ġinfect ious +ĠAn nie +Ġsmart phones +Ġpar ole +åĸ Ŀ +ĠEp ic +z za +Ġun ified +Ġê·¸ë ķĮ +Ġcur tain +ĠÄ ĥ +Ġsex ually +Ġuns erem +ĠCon vention +Ġalleg edly +Y a +ĠH oo +en ment +æĢ ª +íĽ Ħ +Ġgig antic +Ġnot ing +Ġre bo +ĠJ ama +ĠAl z +Ġborrow ed +ì¹ ¨ +Ġper ipher +оÑĤ а +ĠG B +ĠGe ar +Ġeconom ically +Ġtele fon +Ġqu eremos +ĠдалÑĮ ÑĪе +Ġr as +ĠTe ach +ic ios +at os +Ġpl edge +b au +ĠHim self +L ink +Ġesper o +Ġchrom os +ĠP ER +Ġer le +Ġpod ium +ç os +Ġnie u +Ġf en +ĠGO D +ĠCh ocolate +wer k +Ġt ừ +Ġsupp ress +λ η +Ġ24 0 +Ġsit ä +Ġhonest y +ĠB io +ĠB ard +ĠобÑī ем +Ġм Ñĥз +Ġmar ble +ĠÑĨ енÑĤ +Ġproc ure +Ġrot or +ber n +Ġtu h +Ġhead set +at em +Ġwarrant y +à® ´ +Ġfil ing +ι ά +Ġcomp rendre +Ġimp ulse +Ġsal v +wr itten +Ġinstit ute +K im +ĠLGBT Q +fic iente +H is +ĠαÏħÏĦ ÏĮ +Ġteen age +or us +ĠÑĢаз б +S ee +ĠCons erv +á»ģ n +ful ness +Ġstraw berries +ĠAb u +и он +Ġo lla +NO ISE +ĠEm ploy +Ġwip ed +ur ger +Ġmod ifications +Ġíķĺ ì§Ģ +Ġfoot steps +Ġhon ors +Ġad ul +Ġfl ipping +ĠH U +Z Y +Ġintegr ating +ب ر +ull a +Ġnatuur lijk +ĠíĹ Ī +ĠEth ereum +ÙĬ ÙĦ +w ed +Ġpe aks +ĠK es +Ġblo om +Ġcr ashing +Ġ9 11 +ĠоÑĤ лиÑĩ +Ġcontro llers +ĠD od +Ġвм еÑģÑĤе +Ġsort ir +å¥ ĩ +ĠStra ight +ĠGrac ias +Ġgro ove +Ġto gg +Ġìĭ¶ ìĿĢ +é ro +Ġout ward +ĠW A +ĠRock y +Ġsc am +Ġhay at +ig nty +â Ħ +pl ings +Ġantibiot ics +Ġ ä¸Ģ +Ġnever theless +j ang +com merce +Ġspo iler +Ġglo ve +Ġch atter +ĠB Y +~ ? +Ġíĺ ¸ +Ġdem ol +we chsel +im ir +Ġra id +еÑĢ Ñħ +ìŀIJ 기 +en f +Ġcomment ed +Ġoptim ized +Ġconv icted +Ġb ats +ĠS B +ĠA ur +ĠT ong +Ġimplic it +ĠJan et +Ġre ag +ãģ ² +ĠAdv anced +Ġimp ose +ש ×Ķ +Ġschem es +oug her +ab olic +Ġê±° ì£ł +Ġslow ing +Ġwt edy +Ġdest ructive +Ġоп ÑĢед +Ġland mark +Ġëı Ī +ĠWalk ing +Ạ¹ +Ġt ijd +ĠK N +ĠQu ant +ìĺ ¤ë +Ġк ÑĢÑĥ +Ġper der +Ġno ve +änd e +Ġãģ Ĺ +b ia +Ġcust ody +Ġb iod +æĿ± 西 +Ġdirect ing +... âĢĭ +Ġre loc +Ġdemand e +ãĤĵ ãģł +Ġo ÄŁlum +Ġод на +ĠMil k +åı · +ĠK ra +ĠH onda +Ġp ue +Ġele kt +Ġbegin ners +Ġspe ar +ÃŃ nh +ĠLu ft +Ġn ig +ĠSchool s +Ġfor ums +ĠQ in +pp o +Ġz ag +ĠÐ ® +Ġtooth p +ĠSt yle +ì´ Ī +Ġpun ct +Ġrep s +ĠA ly +Ġamend ments +Ġö z +Ġdig its +ur ai +Ġcha otic +ĠMas ters +e on +ĠC ash +ĠC uz +Ġbede utet +Ġscan ning +Ġж д +н еÑĤ +Ġcertain ty +j ek +Ġdi jo +ĠCl imate +Ġr inse +Ġk rij +vel and +Ġsound track +ĠSa fe +ĠNo va +9 4 +Ġa the +ĠVer b +ol er +ìĿ´ì £ł +Ġv in +Ġrespir atory +ĠStud y +ĠC AM +Ġav ocado +ĠZ hen +Ġlat ency +Ġfe athers +Ġcont ar +Ġв еÑī +Ġf ark +Ġbl ended +Ġexpl oded +ĠX X +ĠBen im +Ġalgu ém +isto ire +Ġconfident ial +Ġm ast +Ġì ¿ +ge h +Ġdis respect +ĠSystem s +ư a +E d +Ġw ys +Ġex otic +Ġgl owing +ù ng +oun ge +è Ħ +ани з +Ġpal av +ĠSw ord +Ġg im +ĠC row +Ġpot ent +b ish +Ġab used +ĠJ ed +Ġg ambling +ĠS pect +Ġinvestig ators +æĻ ļ +Ġr att +Ġdo b +ĠD ES +h og +ĠоÑĤк ÑĢÑĭ +íĮ ħ +ĠденÑĮ ги +Ġíĺ ¹ +Ġë¨ ¸ë¦¬ +Ġsat uration +Ġinher ited +ĠInnov ation +ìĹ Īëįĺ +Ġtang ible +Ġdep ri +h ed +Ġпом ог +Ġslic ed +ॠį +Ġth ế +Å ¥ +6 8 +Ġcor ona +Ġgift ed +Ġso ir +Ġhum ility +ĠìĿ´ 걸 +Ġflaw s +ĠпÑĢ Ð°ÐºÑĤи +Ġk ald +wa ż +y w +ãĤĵ ãģ§ãģĻ +ir teen +Ġcroch ets +¦¬ ê°Ģ +ĠìłĦ ìĹIJ +Ġdes e +æ¥ Ń +Ġм аг +Ġdz iaÅĤ +Ġl ég +ch anging +Ġlle v +ÅĦ sk +çĶ » +Ġ198 4 +orn s +ĠW elsh +Ġpharm aceutical +Ġpump ing +ĠSh aw +p unk +Ġva ult +Ġkin etic +Ġhur ricane +ĠInc luding +ứ c +ĠGrand pa +ans hip +é¦Ļ 港 +ĠвÑĭ Ñħод +н ож +ľ ł +ut ta +Ġê²ģ ëĭĪëĭ¤ +Ġb az +Ġпо ÑĪ +Ġpe culiar +zy Äĩ +ĠEll ie +Ġlearn s +ĠKr ishna +Ġconse cut +Ġemp ath +ĠD in +Ġtrad ed +ĠBor is +ugg age +oll a +Ġназ в +Ġetern ity +Ġв п +è mes +Ġgra pp +b é +ĠпÑĢед ÑģÑĤав +ĠF C +į ëĭĪëĭ¤ +e ven +ĠNebr aska +ortun e +Ġk arena +ĠAg ent +Ġst ing +ĠP I +Ġmunicip al +power ed +Ġconse gue +ĠMan chester +Ġrain y +Ġbl i +Ġk ost +Ġhal ten +ĠAh hh +ins ula +er ting +ĠاÙĦ Ùģ +Ġrel acion +Ġk omen +Ġd ome +Ġpri ests +ĠInt rodu +rop he +sh ore +vel t +clip se +ĠÑĢ ÑĥÑģ +×Ļ× ¡ +Ġsab emos +ĠHoll and +og i +ank i +ĠM ats +Ġsm oked +ull ie +Ġeuro pe +ĠдейÑģÑĤв иÑĤелÑĮно +Ġbard ziej +Ġtransform ing +ĠE z +op ath +Ġìĸ¸ ëĭĪ +ĠÑģÑĤ ан +ằ ng +ั à¹ī +ĠO uch +Ġclear ance +ust ain +Ġsolid arity +Ġpro ving +ĠÐĺ н +ĠÑģ ÑĬ +Ġpro long +ад но +Ġs os +ĠDe al +Ġ17 0 +m ons +Ġз ем +Ġlo gged +Ġlif elong +Ġsens ory +Ġbe hold +ĠF AR +èt ement +ĠFed eration +Ġdod ge +ĠSh ir +Ġdrag ons +ĠAr ctic +Äħ ż +Å į + º +Ġden ke +Ġpodr ÃŃa +co le +ÑĥлÑĮÑĤ аÑĤ +Ġsystem atic +ам а +ch os +Ġclin ics +ĠB S +Ġtal es +us ions +Ġí ά +Ġpres ervation +Ġl ore +ĠProt est +á» Ľ +å¸ Ĥ +Ġacknowled ged +ĠIs aiah +ĠëķĮ ëĬĶ +Ġ× ĺ +Ġcompet itor +Ġadv ancing +z ip +Ġtent h +ĠLa ure +Ġh ints +Ġexerc ising +ŀ ľë +ĠIntell igence +u ated +OU T +op ed +Ġaut onomy +Ġbrand ing +ĠMediter ranean +Ñĸ к +Ġscrew driver +Ġsu pre +Ġst ap +Ġjurisd iction +ĠSetting s +Ġfore front +ĠF emale +com fort +Ġmultiplic ation +ĠMur ray +Ġbo b +ĠT as +Ġt ahu +Ġon un +et ter +Ġproph ets +l ag +Ġreven ues +Ġpr á +Ġupload ing +Ġmach inery +asc al +ĠEst á +ĠG oth +ĠB ald +ĠS aw +Ġstri pes +ìł ij +Ġpow in +æĹ¥ æľ¬ +Ġhost ile +Ġdar um +Ġprevent ed +ожалÑĥй ÑģÑĤа +Ġalgun as +Ġhop eless +Ġz naj +Ġread ings +Ġcra ving +t at +ĠP ig +Ġli ar +çĪ ± +Ġmulti player +Ġd ale +ĠCour se +íģ ¼ +ĠK ita +Ġcustom s +Ġrespond s +end ra +è¦ ĸ +Ġmet ro +Ñģ ол +Ġmitig ate +Ġopp ression +Ġ æĪijåĢij +qu inho +Ġam mo +Ġen fer +Ġp ony +Ġ ounces +° Ķ +ĠìĪĺ ê°Ģ +Ġdich o +ĠDe b +Ġwond ers +ĠRo ose +Ġpri zes +ĠA LEX +Ġthank fully +Ġtiss ues +ĠÑĢав но +ĠL una +intell igible +ĠìĻ ¸ +ê° ij +ĠHe at +ĠÑģ ид +ĠQu i +Ġ ions +Ġaccommod ation +ä¾ ¿ +ĠK art +ien st +Ġt arde +Ġso aked +ĠCase y +Ġì´ Ŀ +ĠÑĢ Ñĥб +Ġdifferent i +Ġleft over +Ġexch anges +sec ond +Ġfirst ly +Ġbuild er +ri en +Ġd w +Ġboun cing +? </ +ĠëĮĢ íķ´ìĦľ +ĠÑģ е +ĠM iles +ien ie +Ġпод пиÑģ +Ġë¬ ´ +Ġar ises +Ġsub conscious +ĠSand y +Ġlot tery +âĢ ij +am iliar +Ġcoordin ator +è Į +Ġextra ordin +ĠRon ald +ĠM ON +g reen +Ġmanufact ure +ĠRec ord +ĠMark eting +и ÑĨ +Ġcredential s +Ġup right +ĠHer itage +Ġgör d +æľ į +exp ensive +áºŃ n +Ġì± Ħ +Ġout lined +ĠO ooh +orient ed +Ġw ired +Ġout lets +Ġhug ely +ĠíĸĪ ëĬĶëį° +аÑĢ ÑĤ +Ġlog istics +Ġseason al +Ġde be +Ġthe or +Ġpir ate +app y +Ġkn ots +Ġfem me +ĠSoft ware +g ende +ÑĤак и +Ġtem ples +Ġlim itation +Ġampl itude +Ġha cen +Ġaud i +Ġëĸ ¨ +ĠW ahl +Ġni h +Ġampl ifier +ari us +iz ado +ach a +Ġkull an +ĠTw in +ĠFor ces +Ġab rir +ĠE PA +ĠA ha +Ġê·¸ëŀ ĺëıĦ +Ġbi om +ĠТ ам +Ġsa iling +ĠJ oker +F irst +è¿Ļ æĺ¯ +~ ] +ors ch +Ġvæ re +Ġbeet je +ĠSpa ÃŁ +pol it +Ġtur bul +ĠìłĢíĿ¬ ê°Ģ +Ġc ic +ĠDra ke +ĠB RI +iz ação +ĠìŀĪ ëĭ¤ +ĠLyn n +Ġtrans gender +Ġres ign +Ġchar ter +ĠJ H +ĠHol mes +ĠL ip +d as +Ġped iatric +Ġmemor ize +Ġevalu ating +ĠðŁ IJ +ca k +Ġconjun ction +Ġres erves +Ġsh ampoo +Ġjud ged +Ġwid z +V IN +Ġab oard +ar is +ĠR oh +Ġcool ed +ÑģÑĤ е +ce p +r ost +h ots +ĠMel bourne +оÑĩ ÑĮ +Ġvent il +ин ов +Ġmot ions +ìĹĪ ëĬĶëį° +меÑĢ Ð¸Ðº +ĠCh at +Ġgouvern ement +ä¸Ģ 次 +ĠK ivol +ĠKivol owitz +Ġnó i +Ġк Ñĥда +Ġhyd raul +ĠBer g +yl um +ĠPr äsident +rop y +Ġsem ic +Ñı еÑĤ +ĠCa pe +Ġcan e +Ġbring en +Ġwir ing +un ya +Ġrep ay +ª © +Ġw ont +á nt +Ġgo ver +ĠLiber ty +Ġelect romagn +ĠSing h +Ġг ÑĢÑĥп +г ов +Īë¬ ´ë +ĠR ule +Ġunder way +ĠFred er +Ġturb ine +ish i +Ġf ÃŃs +ĠC ulture +ac re +Ġw ander +Ġguer ra +Ġsö y +ĠJ ur +a ways +Ġschw ier +gu ard +ĠAb d +u ction +ĠarkadaÅŁ lar +ĠH amb +? . +s ize +ĠOr th +Ġs way +ĠÎ Ķ +Ġabsor ption +ine es +Ġpat rons +Ġbeach es +G G +Ġcont amin +intend ent +Ġн ÑĢав +Ġд еÑĢж +Ġqu ilt +Ġevolution ary +ìĿ´ë Ŀ¼ +az ioni +Ġer kl +ĠBut ler +Ġdo o +Ġneg otiation +end um +Ġtermin ology +Ġk ul +ĠUnter nehmen +é ric +x i +b ad +Ġдолж нÑĭ +ĠMitch ell +th ree +å¼ ı +Ġsubst rate +ĠIn hale +ĠAgr ic +un ge +Ġз ÑĢ +Ġad verse +ĠìłĢë ıĦ +Ġpill ar +ĠMin uten +ĠM ate +ĠPl atz +Ġhel pless +Ġal ar +Ġf rench +Ġalloc ation +Ġst ems +Ġmar athon +ĠHAR F +iz ación +J ess +Ġзна Ñĩ +Ġdeclar ation +EER ING +ster dam +ass ium +Ġse iz +Ġpres idents +t ake +Ġwild erness +Ġcos mic +Ġ모ë ijIJ +st ro +Ġpow iedz +ĠMagaz ine +ĠV I +Ġд еÑĢ +Ġwür den +Ġtab lets +Ġpier ws +Ġmort al +Ġsuppl ied +ĠN ós +ĠPro per +Ġкажд Ñĭй +ol óg +ë° © +Ġmis con +Ġproxim ity +ĠAll es +Ġгл аз +Ġl ame +Ġvib es +Ġde emed +Ġur ine +Ġremind ing +Ġcircumst ance +ë ĵ¤ìĿ´ +Ġlapt ops + ² +íķ´ì ķ¼ +ĠOm ega +ãģªãĤĵ ãģĭ +N Y +Ġpump s +Ġr ails +Ġsur pass +ĠBr os +Ġnation ally +Ġgew esen +äº « +³´ ëĭ¤ +os hing +ê° Ī +ç¤ ¾ +Ġc rian +ĠìĤ¬ëŀĮ ìĿ´ +ca ust +æķ ´ +ÑĨи п +ĠO ber +ĠD AY +ĠCan on +z ung +Ġê° ĸ +Ġав ÑĤом +Ġdivor ced +×Ļ× ¤ +Ïģ ε +cel and +ci er +ÑĢ ÐµÐ· +Tod ay +Ġorb ital +Ġst ret +Ġsat u +Ġíģ ¬ë +z os +ĠS co +μ ÎŃ +ĠGuard ian +inter est +ĠV ER +ünd en +ĠÑħоÑĤ ел +t it +B y +Ġan lat +S how +Ġo ily +ç¯ Ģ +Ġleg ends +Ġspec ulation +ĠW ish +Ġmon k +G AN +Ġh á»į +Ġd angers +ĠB ene +iqu ement +ĠëĤĺ ìĻĢ +Ġа д +Ġdisc rete +à ĩ +Ġcond itional +ĠG ill +u ates +ĠÑģов Ñģем +Ġscreens hot +c ado +Ġ모ë ĵł +Ġfingert ips +ĠM AC +Ġd udes +c ost +Ġbump s +ond o +Ġdat os +Ġbe eps +ĠP ron +ĠK hal +z ego +ĠAb by +U h +Y o +ĠT el +Ġμ ÎŃ +K I +Ġstress es +Ġspreadshe et +ĠN OW +D B +Ġliber ation +Ġpredict able +ĠQuest ions +Ġsp acing +Ġinhabit ants +Ġz wiÄħz +ç± ³ +ĠS AP +Ġl uggage +Ġh ipp +è ĸ +Ġtang ent +Ġv Ã¥ +алÑĮ ной +se hen +Ġprocess ors +Ġfind et +Ġcart ridge +Ġadministr ators +Ġìĸ´ì ļ +Ġsupre me +ĠAnt i +ĠíĶ Ħë¡ľ +Ġinform ative +Ġkom t +æĪij ä¹Ł +×Ļ× ĺ +Ass istant +Ġlist a +ö ll +Ġdistinct ive +ĠH ud +Ġsal on +ä¸ĭ ä¾Ĩ +m ême +ĠMot ion +Ġseule ment +ĠMens ch +Ġpump ed +ü her +ib o +Ġwa ż +Ġquant itative +Ù ¾ +Ġ모 ìĬµ +Ġp ouch +ĠThe atre +ah i +Ġspin ach +Ġreal ities +Ġle y +ĠMar tha +Ġre cher +e ches +Ġperiod ic +oc ide +ĠInc red +Ġth ấy +ot on +ĠE so +Ġgén éral +il ight +Ġimag ining +he a +et ical +á» Ń +ĠDem okrat +Ġen jo +Ġadjust able +Ġr ains +iew aż +Ġjust ement +Ġjust ified +ĠSh ake +v iv +ìĤ¬ë ¥¼ +Ġmet t +ĠEnviron mental +Ġsol amente +Ġinter sect +Ġ198 8 +Ġsim ulate +J A +Ġз аÑģ +Ġcont ing +ĠT ek +Ġtor ch +ĠдÑĢÑĥг ой +Ġins cre +Ġmodel o +ĠG eg +ĠDemocr at +к в +ĠBud dy +Ġredu nd +Ġcraft s +ĠH ij +Ġj ue +ĠKir k +Ġk ab +á» £ +Ġaest het +ĠJ ON +Ġsuper com +ĠÑģ иÑĤÑĥ +ĠÏĮ ÏĦι +Ùħ ÙĨ +ĠE VER +ìķĺ ìĸ´ +o it +ĠCle veland +Ġsixt een +Ġwater fall +ï ¸ +in fl +Ġcounsel or +ĠP unk +Ġspre chen +æµ ģ +ex c +ĠSk ills +ro z +ad amente +Ġpan cakes +ê¸°ë ¡ľ +Ġpl ank +Ġsovere ignty +Ġf ui +Ġне об +ĠW ii +ĠSch ol +âĢ İ +ĠSpe ak +èĭ ± +c iliation +Ġth igh +Ġê±° ìĿĺ +Ġj ot +Ġì´ ¬ìĺģ +ĠÙħ ÛĮÚº +ĠCC P +Ġпо ÑģÑĤ +Ġobser ver +á b +Ġst igma +Ġprop riet +Ġc idade +ĠbaÅŁ ka +ع Ø© +k re +Ġpow iedzieÄĩ +Ġce ase +Ġsk ins +Ġvegg ies +Ġoppos ing +op oly +ĠJ ug +ĠY oon +ĠUn it +Ġ198 6 +Ġk ons +Ġdiagn ostic +Ġempower ed +Ġth o +Ġc en +é ration +ĠÑ Ĺ +Ġphys ic +ĠPract ice +å· Ŀ +ĠS outheast +ĠEs pa +è¯ · +ĠGe or +rop ortion +Ġspec s +Ġadapt ive +ĠUn ity +ĠWork s +ug en +ĠMont ana +Thank s +Ġwh ipped +Ġdun geon +Ġvitam ins +S P +Ġsc andal +Ġdin ero +o va +Ġemb ro +ĠE agle +Ġthe ology +ĠVan essa +ĠA IDS +ë IJľ +Ġfre el +ĠAlz heimer +ĠÅ ļ +H er +Ġtorn ado +ag ens +ĠìŀĪ ìĸ´ìĦľ +ĠTrans form +Ġprocess o +Ġmill ise +Ġprofession ally +Ġmem b +oc ation +Ġsty ling +Ġоб Ñıз +ĠOper ation +Ġwy gl +ĠR an +Ġ çļĦ +ĠK in +á»± c +ĠB AR +Ġpaper work +Ġt ule +Ġquer ia +Ġcomp ly +ĠH air +×Ļ× Ľ +ĠпÑĢо ÑģÑĤ +Ġmut ation +Ġrep rés +Ġoct opus +Ġimportant es +Ġdes erved +et r +Ġdis asters +l ında +iqu é +ĠDes halb +so o +oss ip +Ġrelie ved +ĠColl ins +Ġwater proof +ĠY uk +Ġcop ying +Ġb ütün +ĠHe ute +ĠEnt re +Ġresid ual +Ġcolon ies +Ġé norm +ĠEr in +Ġst an +Ġtremend ously +Ġcapt ures +ĠS ai +â ce +Ġm iaÅĤ +Ġ8 7 +Ġlo gging +Ġinsert ed +Ġinher ently +ìĿ ij +la ve +ни Ñĩ +Ġfem mes +Ġdé p +u ks +ac ia +ĠW ade +Ġj ij +ĠVin cent +ĠI celand +h em +Ġap ology +ĠP eg +Ġgl ued +Ġcompan ions +ĠL iver +Ġcritic ized +le ading +Ġsä ga +æ¼ Ĥ +Ġsqu id +Ġnarr atives +Ġtak a +ne z +we it +Ġtrip od +Ġexpl ic +Ġsp inal +Ġapproxim ation +Ġpag ar +ĠCal vin +Ġв едÑĮ +Ġl ac +Ġpro active +ĠTra in +or f +Ġst en +Ġgra pes +Ġme us +Ġautom at +Ġbi ased +Ġcha îne +co al +Ġren cont +ĠK um +Ġfest ivals +Ġstart ups +Ġa ka +ãģ ¹ +Ġcyl ind +s na +C RI +Ġresult ado +Ġmil estone +ĠÏ ħ +Ġtele port +zy ch +6 2 +åħ ³ +ĠFe ar +Ġnucle us +Ġsh ines +ho v +ĠPart ners +ĠK as +Ġnad ie +Ġalert s +ĠB ILL +str ong +ĠN ate +ĠDen mark +ĠC av +OS T +h ält +ĠìķĦëĭ Į +any on +Ġencour ages +Ġпо ÑģÑĤав +ĠHu ang +ãģĬ é¡ĺãģĦ +ST A +Ġpain ts +ãģĻ ãģĶ +Ġsched ules +Ġche ated +Ġappro x +Ġï · +Ġ» . +Ġsm iles +is ure +Ġn ered +ard en +Ġcur t +Ġë Į +ĠR oth +Ġpuis que +ĠG ET +ĠVe get +Ġprodu z +ĠBelg ium +ĠCamp us +ר ×Ļ×Ŀ +ic ut +ĠÑģ ним +Ġré uss +Ġslipp ery +ĠE w +Å ³ +ĠLeg ends +ĠT iffany +али з +ĠпеÑĢ ÐµÐ² +ĠогÑĢ Ð¾Ð¼ +Ġcr os +ĠC E +B u +Ġens ures +Ġgrand children +Ġac uerdo +Ġprison er +Ġth irsty +b ane +Ġë¹ ł +Ġúlt ima +ĠLa unch +n ity +Ġcombust ion +Ġun icorn +Ġfam ille +Ġlower ing +ĠY ing +build ing +Ġdu o +ĠMé xico +ast ian +Ġ먹 ìĿĦ +ĠRal ph +Ġre write +Ġgl am +if ique +E r +ĠRun ning +он ов +Ġmean ings +Ġche wy +ĠLes lie +Ġfin est +Ġhah aha +ĠST EP +Ġlon eliness +ri ans +Ġquestion ed +Ġes que +Ġsink ing +Ġpes o +ĠWr ong +asm ine +Ġdefin itive +Ġbu ys +Ġcru c +c ool +Ġë łĪ +Ġp ó +Ġutil ized +Ġworth while +ĠD ylan +ES E +Ġverte x +t ı +ĠF ir +Ġz aw +ĠG ed +ĠÐĿ ап +d z +Ġcurs or +Ġsw ipe +Ġinevit ably +Ġpos ters +Ġinc lined +Ġgreet ing +Ġdisappoint ment +ãģ¾ ãģ§ +Ġrela ção +T T +Ġr abb +ĠM aine +Ġanaly zed +F E +ĠÐŁ ол +ĠSand ra +Ġpl ague +AR E +Ġv är +ĠV iv +um ed +h ando +hou ette +ĠBa iley +ä¸į éģİ +ys on +Ġsem ua +Ġhard core +â Ĥ¬ +Ñĸ м +é ra +OT H +Ġforeign ers +ĠPalestin ian +Ġprop rio +ани й +Ġmyth s +W H +Ġnin th +ĠCreat or +л ом +ĠFl ip +Ġem an +Ġki ÅŁ +zie h +ĠEarn est +sy stem +ĸ ìĹIJ +Ġarm ies +ĠOut side +Ġhar us +æº ĸ +од аÑĢ +Ġvis itor +çŃ Ķ +Ġstrength ening +Ġ9 2 +v io +Ġë ¦¬ +Ġgre edy +Ġpo quito +ud er +ĠK opf +Ġëĭ¤ìĿĮ ìĹIJ +Ġse is +át ico +Ġtrust ing +ÃŃ p +ĠE mm +le en +ĠاÙĦ ÙĨ +Ġrecruit ment +ĠFil ip +ĠÙĥ ÙĦ +Cl int +Ġв еÑģ +au ft +Ġdomin ate +Ġrest o +Ġk ra +á i +ĠC ait +r ows +Ġcountry side +Ġ19 45 +аÑĨи Ñİ +Ġд и +Ġkern el +lo v +Ġcalcul ating +د ا +ĠW alt +Ġempower ing +Ġch assis +line ar +г Ñĥ +Ġno va +Ġu y +Ġ6 9 +Ġen compass +tr l +Ġcomput ational +Ġwor ms +Ġnhi á»ģu +Ġastronaut s +Ġv es +Ġsy tu +Ġdemand ed +Ġc s +ĠM ol +Ġ ` +Ġch ant +Ġthere by +Ġpen is +Ġem oc +w yn +Ñĥ же +Ġtre ad +ó le +Ġdeep est +Ġmach e +ĠV ent +ĠAm sterdam +ãĥ Ľ +Ġre bel +Ġ6 1 +Ġв кÑĥÑģ +uff s +ĠdoÄŁ ru +ĠNap ole +ή Ïĥ +Ġwork outs +ĠGl ad +н еÑģ +Ġt ensions +ĠSh ift +ĠGu er +íĮ IJ +Ġì¹ľ 구 +Ð ĸ +Ġimpl ant +ê u +ê¸ Ģ +Ġauthor ized +C ER +ĠR V +Ġh il +le v +c imento +ĠU FO +ìĥ Ī +è¨ Ĥ +w or +Ġd ances +ĠPix el +çľĭ ä¸Ģä¸ĭ +Ġtr otzdem +Ġob ten +ĠAlf red +Ġcost ly +ĠStan ley +Ġterror ists +ĠW id +ħ ëĭĪëĭ¤ +Ġle icht +ìĿ´ì Ĭ¤ +Ġdobr ze +Ġhes it +Ġer zäh +Ġein ige +Ġhe bt +Ñģ е +Ġunp redict +C ómo +rem os +ĠThank fully +Ġpur se +ch s +an cer +ul os +st ud +æľī æ²Ĵæľī +Ġneuro log +ĠAn cient +O ut +aws ze +Ġopp ose +Ġantib odies +ĠSome how +ropol itan +kt or +ĠÑģÑĤоÑĢон Ñĭ +Ġrock ets +Ġdis able +Ġcatast roph +´ì ŀ +Ġc yn +ĠдÑĢÑĥз ÑĮÑı +Ġinstruct ors +ema al +Ġet wa +Ġy uan +ĠGr ound +Ġpremi ere +Ñĩ ив +Ġs aint +y ba +Ġk ok +Ġcontract ors +Ġê° ģ +Ġ×IJ× ľ +Ġhead line +Ġcomplet amente +Ġin expensive +Ġvi u +ĠGrand e +Ġble ed +ë ¬¼ +Ġ7 3 +Ġtod avÃŃa +ĠR ush +ĠEld er +ê°Ģ ëĬĶ +ĠR ou +Ġжен Ñī +ĠM ira +Ġde ine +Ġkar ma +Ġum m +Ġents che +ĠHolo caust +Ġdiscover ies +am ents +Ġrais on +Ġbur gers +B ack +Ġg dy +ĠA G +ĠD aw +ìķ ł +head ed +ĠCl ar +In st +ĠLie utenant +ĠAf D +ĠC es +Ġpersonal ized +Ġinterf aces +à¸Ī ะ +ĠÑĢ ÐµÐ¶ +Ġsu ic +Ġstar ving +Ġox ide +Ġdecor ated +ĠD U +ĠìĺĪìģ ĺ +Ġqu o +Ġdist ortion +æ® µ +Ġ먹 ìĸ´ë +Ġst akes +æĺİ çϽ +Ġsynt ax +Ġbi ết +th y +ic ie +Ġbras ile +is is +R C +Ġsh ook +Ġdepth s +ĠC osta +Ġvoc als +Ġco aster +Ġfal ou +ett le +Ġk ennen +Ġder ive +Ġa ids +ĠÐĿ ик +Ġent wic +Ġvert ically +Ġ Í +ĠSU V +Ġfire works +Ġspecific s +äº ¤ +Ġinsist ed +Ġdes halb +ĠG onz +lo ve +ĠMil itary +ĠPier re +Ġâ Ī +ĠWh ose +Ġperf ume +ĠÏĢ Îµ +Ġlower ed +Ġcross es +Ġtransl ates +Ġarrib a +ÃŃ do +ĠLe v +åħ § +ĠC iao +Ġscholars hips +Ġgest ures +ĠÑĢез ÑĥлÑĮÑĤаÑĤ +Ġquest ão +ĠColon el +ĠB ott +ر Ùģ +N ING +ĠWatch ing +ĠPur ple +ÑģÑĤÑĢ Ð°Ð½ +Ġexecut ives +ĠK ris +or neys +ен нÑĭй +Ġco ated +Ä © +Ġpark ed +ĠÑģв еÑĤ +!! !!! +ĠFlo yd +ıs ı +zi Äĩ +Ġmotiv ate +ĠEl on +le an +Ĩ ĵ +Ġ ip +Ġni ż +ĠExper ience +ĠT ina +ĠKoll ege +ĠAmb assador +in ya +Ġthe ft +Ġhe ures +ĠMy st +Ġmais on +le b +Ġbowl s +ĠBür ger +ĠRoose velt +R P +ê°Ģ ìļĶ +ĠDel icious +erd ings +ĠAssoci ate +ous se +ĠC ort +ĠRepe at +ĠGl ory +Ġcont ag +à¹Ģภ¥ +ĠPar ad +ĠK erry +Ġê ¿ +ĠW ave +å¿ ħ +Ġgate way +çIJ ĥ +! ãĢį +Ġtrans cend +Ġdam ages +Ġt ails +Ġgravit ational +ĠSh ield +Ġprim itive +Ġcar riers +ĠHua wei +ÙĤ د +Ġfel iz +ĠM ia +åĥ ķ +ĠпÑĢÑıм о +ĠпÑĢоиÑģ ÑħодиÑĤ +ĠMur phy +ĠAct iv +ãĥĥ ãĤ¯ +Ġdis comfort +×ij ×Ķ +ĠK ell +ĠCent ury +Ġsp aghetti +ĠD urch +Ġc ierto +ĠEmp ress +Ġg uts +ne g +Ġдо ÑģÑĤаÑĤоÑĩно +Ġvolunt ary +å¤ ± +Ġsqu irrel +æ¬ ¢ +ãģ¡ ãĤī +ĠM az +´ìĭ ¬ +Ġв и +ãĤ § +ĠÑĤак иÑħ +ĠSh aron +Ġenthusi astic +ire ment +Ġíŀĺë ĵ¤ +Ġpot rze +Ġiniti ated +ãĥ § +ĠÅĽ rod +ĠìĿ´ë ¦Ħ +Ġrem ake +Ġcul min +Ġconf use +mi yor +ur ar +CT OR +Ġbun ny +Ġ 大 +ä¸į èĥ½ +el p +Ġvamp ire +Ġill umin +ĠH end +Ġк аÑĩе +ĠSal v +Ġкан ал +Ġport a +Ġass hole +Ġsupp orter +Ġskept ical +Ġkn ead +Ġìĺ ¬ +e za +Ġqu ê +ĠD H +Ġrod z +own ers +Ġpl ots +Ġdel ays +Ġbelong ed +Ġa hh +Ġcar ved +Ġris en +Ġor den +ph ony +iss y +!!!! !!!! +ĠolduÄŁ unu +Ġr oses +Ġintr ins +ĠAng st +Ġfinal ement +ì§ Ŀ +SO UND +Ġind ul +° Į +Ġ×ķ ×Ķ +ch y +акÑģ им +Ġn ggak +Ġli z +Ġele ctoral +ĠSh awn +ric ia +Ġar sen +ĠP ep +Ġ20 30 +Ġtro phy +Ġsmo other +Ġer re +Ġcrash es +Ġsch ne +Ġas i +ĠMa ÃŁ +Ñĥ ли +ÑĩеÑģ ки +ie ves +RE AM +Ġstir ring +ãĥ Ģ +ust a +Ġin ver +s ight +ord u +o or +ĠÄĥ n +Ġperm itted +ÑĢ ÑĮ +Ġch alk +ãĤĪ ãģĹ +Ġtatto os +ĠRel ations +ĠH oy +ks am +Ġdent ist +Ġ미 êµŃ +Ġso fa +ĠÑ Ķ +Ġform e +ÙĤ Ø© +Ġë² ł +Ġembr aced +m il +Ġsung lasses +Ġê° Ķ +Ġseam less +Ġbe ep +äch st +Ġswe ets +Ġsem aine +Ġirre levant +Ġdesen vol +Ïģ Ïī +ĠпÑĢоиз вод +ang s +Ġar oma +Ġpool s +Ġgi á»Ŀ +ĠU g +Ġclim bed +Ġtrend ing +Ġseper ti +ĠB arr +Ġp ÅĤ +ĠOrig inally +Ġ Ú¯ +ut to +Ĭ ¸ë +ĠкоÑĤоÑĢ ÑĭÑħ +Ġза Ñħ +Ġeigen en +Ġmurder er +ern ame +Å ŀ +Ġannoun cing +ĠPlat form +Ġexplan ations +Ġpres ente +ĠNas ıl +Ġorph an +ĠFort nite +ros pect +ered ith +ĠìĹĨ ìĸ´ +ĠNI H +w agen +Ġrem ed +§ Ģë +m ont +ĠJeff rey +pr om +Ġf ünf +Ġназ ад +Ġcuc umber +ĠSum mit +åĪ Ŀ +§ ¤ +ÐĿÐIJ Я +ĠJ et +Ġcamb io +Ñĥй ÑĤе +Ġcub ic +Ġdisp roportion +ere z +Ġmad ness +çĹ Ľ +Ġt int +Ġfuer on +Ġk y +Ġbip art +ãģ¾ ãģĽ +S am +Ġë ½ +Ġr iv +ĠT ank +ĠëĨ ĵ +Ġrend ered +ÅĽl ÄĻ +c onds +Ġdis ruption +Ġincon ven +Ġqu iser +Ġden ial +Ġgalax ies +Ġsovere ign +Ġpol sk +Ïģ Ïİ +Ġme x +Ġcar acter +ĠL ego +and en +.' " +ĠíĶ Įë +Ġcompress or +ĠMo vie +Ġapplic ants +zie hen +Ġveget ation +Ġbe lle +ĠG OOD +ĠB au +Ġres ent +se x +ament os +Ġ×Ķ× ĸ×Ķ +Ġover load +Ġsilic one +еÑģÑĤ но +Ġden ken +Ġdefin it +ĠWas n +Ġalter ed +ĠSo o +ĠW ing +ind re +ĠN PC +Ïģ ÎŃ +ĠTw enty +ĠLie be +Ġhomeless ness +ould er +ĠÐĺ ÑĤак +Ñģ каÑı +Ġcu atro +ĠHar vey +Ġph ilan +ĠBe et +Ġpol icing +ĠAlex and +Ġм олод +Ġmü s +Ġh izo +ë³´ ëĭ¤ +Ġпоз вол +Ġп ÑĭÑĤ +оÑĩ емÑĥ +Ġíĥ ľ +Ġcryptocur rency +Ġl oro +Ġsumm ation +Ġbak alım +Ġne uros +Ø ¥ +Ġмож ем +Ġü st +Ġprelim inary +Ġhorn s +ĠT I +Ùĥ ÙĦ +Y O +Ġh inge +Ġrep airs +Ġbond ing +Ġb ize +ĠÑĪ ÑĤ +Ġmot ive +ĠNiger ia +1 20 +b lock +Ġav iation +ĠKomm un +Ġок аз +Ġten ha +Ġeduc ating +Ġsta at +æ ¶Ī +ĠÑģк олÑĮко +Ġfright ened +Ġsee ks +ÑĢÑĥ ÑĪ +qu ent +ĠN ou +Ġpr at +ĠSh ot +W ork +kar ang +ĠLight ning +nold s +roll ed +gl ass +Ġcred ibility +IT Y +Ġatm ospheric +Ġha via +änd ern +che ers +Th ese +ĠC ell +Ġmag nes +ĠBra vo +se ason +ĠÅŁey ler +ðŁ İ +wh ite +ĠM B +Ġstack ed +Ġ7 4 +Ġдав ай +Ġp ave +Ġо Ñħ +Ġdatas et +Ġret our +Ġmat urity +Ġqu ase +Ġ9 3 +ĠSy m +Ġbrief ing +Ġcult urally +Ġì ·¨ +inh as +Ġmad am +Ġajud ar +ĠTib et +Ġle aks +ci le +Ġthe aters +ìĺ ¨ +ãĥ ĸ +7 2 +ĠW ash +ĠQual ity +ĠI van +ĠB ent +ig ator +ĠGesch ichte +Ġreact ive +Ġ19 00 +æ¡ Ī +Ġcontrad ict +Ġziem lich +Ġcoh ort +á» § +Ġp estic +Ġor az +Ġtell ement +é ¾ +ĠNow adays +c rew +Ste ve +Ġf ictional +Ġil k +ãģĤ ãģ£ +Ġgas oline +z am +Ġpan cake +èn cia +Ġmuit os +Ġb ury +Ġk op +ĠI Q +Ġres ervation +ĠUp date +Ġje j +ĠE yes +åı ij +Ġv ive +Ġch ce +ĠIn i +resp ons +Ġreflect ive +ĠW an +Ñĸ з +Ġen ca +Ġemb od +ĠBur ger +Ġacad emia +ĠCir c +ĠпÑĢ ÐµÐº +Ġan lam +Ġphilan throp +ĠBa ÅŁ +ĠAud i +Ġv ost +ä½ł çŁ¥éģĵ +Ġre per +P eter +Ġcons oles +Ġscr ut +ĠTurn er +ĠбÑĭ в +II I +è¨ ´ +ĠF light +ภĸ +ĠR aven +Ġcor ros +fer n +Ġprov a +ĠSe v +Ġreci pro +Ġ198 5 +Ġnue va +Ġd ab +ãĢģ ãĢĮ +Ġme z +ĠSt ark +pp ings +о ÑģÑĤи +ì¦ Ŀ +Ġfr aming +ĠÐł аз +Ġpost p +ĠSh annon +Ġк ÑĥÑĢ +Ġjak by +ien nent +ĠM aps +ĠRevel ation +ĠÑģÑĤ ал +ìļ ´ëį° +Ġdev ant +ĠG iving +ĠW AS +Ġк ого +Ġrem a +ĠR C +n ÃŃ +Ġsl ipped +ĠR ams +Ġwe et +Ġmascul ine +ĠE c +Ġre op +ĠPl ant +ĠM AY +Ġsp ikes +Ġno zzle +ĠWik ipedia +ĠC oh +IS SA +chl ossen +ì§Ģ 를 +Ġë¯ ¸ë +ĠN eder +J osh +ĠÐłÐ¾ÑģÑģ ии +Ġ198 7 +ĠThe ory +ek k +Ġut an +Ġдом а +ch u +ĠÑģ б +Ġapro ve +V EN +uep rint +Ġ8 4 +æ¼Ĥ 亮 +C or +Ġrich er +Ġsandwich es +ats u +ÑĪ Ð¸Ñħ +Ġl att +~~ ~~ +f riends +Ġderni ère +Ġstere o +ĠÑįк Ñģп +Ġprotect ions +Ġha ut +Every one +Ġenter prises +ĠMost ly +ĠSpot ify +ĠSe x +Ġun g +Įë ¥¼ +Ġactiv ism +ct ica +orig inal +ĠпÑĢог ÑĢам +Ġbro ccoli +à ¦ +ог ÑĢаÑĦ +Ġse karang +Ġcra fting +Ġб ан +ãģ» ãģ© +ĠR az +Ġna ive +Ġsc rolling +Ġnumer ical +Ġschedul ing +Ġapart ments +ç į +Ġstret ches +ace y +ĠH ER +ãĤ º +Ġz inc +Ġd arn +Ġc él +Ġward robe +Ġred irect +Ġj um +ĠStr ange +Ġn Ãło +Ġexperiment ing +ér é +Ġvou lez +Ġge be +ĠK ann +ĠÄij á»Ļ +ĠMax im +ĠK ön +ĠGl as +Ġpol ished +Ġnum a +I ch +Ġritual s +ĠS I +иÑĤ ели +Ġinf ilt +Ġscar f +op hy +Ġy ine +Ġciv ic +ĠM eng +än ge +Õ ¥ +h istoire +ĠO ke +Ġìĺ Ĩ +Ġsoll ten +Ġ8 2 +é ¦¬ +Ġpres cribed +ĠDub ai +ĠEl tern +Ġnation wide +Ġsk ating +i ary +Ġreward ed +Ġmor ality +ĠMag gie +ĠOh hh +ĠF ahren +ol ved +æĹ¶ åĢĻ +Ġdeux ième +te chn +ro le +Ġle ider +ĠJ AY +Ġин ÑĦоÑĢм +Ġca ffe +reich en +Ġk art +ĠC ute +ffect ive +Ġbull y +ag ar +Ġcommod ity +Ġob rig +OU R +Ġun pleasant +no x +J ul +ol ith +ÑĤо ÑıÑī +ĠBe lla +Ġdoll s +ĠHo ff +Ġadvis ors +Ġtransf ers +ĠG oku +Ġ12 00 +inh os +P al +Ġëĺ ij +Ġre pt +Ġaccomplish ment +Ġwe ave +Ġovers ight +Ġun healthy +Ġfil t +Ġpud ding +ĠMig uel +Ġch uckles +åı° çģ£ +vers ion +Ġconf ession +val ue +Ġtri umph +Ġsa ir +Ġëħ ¸ +Ġar te +ĠMater ial +ut i +Ġliqu or +ĠBay ern +ĠM ail +Ġíĸ ¥ +Ñģк ом +Ġcheap est +ĠÑĩа ÑģÑĤи +ĠJo bs +ĠC anyon +har ma +ale y +and ro +Ġappear ances +pro f +Ġо з +l agen +Ġ/ / +Ġли ÑĪÑĮ +Ġrecover ing +д ж +ps y +ãĥ ¢ +Ġsw ift +ĠSp in +å¸ Ī +Ġsein em +Ġdol ph +f ühr +â t +Ġalt ijd +ĠMart y +ĠHo ch +Ġpred ators +Ġvor her +ĠÐĶав ай +Ġfrag ments +Ġpast ry +Ġcomm en +ĠS ana +Ġê± ´ëį° +uss en +Ġt ela +ĠN ina +le k +Ġc ries +Ġth ighs +ĠF lex +ĠB uzz +ã Ħ +U s +Ġpas o +Ġdecl ined +ĠN y +bal ance +Ġmas a +Ġj os +ãģª ãĤĭ +ĠСп аÑģибо +ach u +l oud +Ġpen a +ĠW ald +Ġelim ination +Ġв еÑģÑĮ +or age +Ġmisunder standing +Ġend orse +Ġog óle +Ġgre ed +Ġkle in +׾ ×Ķ +RE Y +ĠE ating +Ġsemin ar +ĠBirth day +Ġque lle +ĠMult i +Ġtir ar +Ġper ch +Ġla vor +ĠJ ia +Ġmut ations +Ġcig arettes +ÙĪ Ø¬ +Ġcous ins +Ġcaps ule +Ġhorr ific +Ġst ur +Ġze igt +n uts +Ġmean while +ĠCol in +Ġgob ierno +Ġg w +Ġuh h +ĠJ ER +spe cific +Ġalleg ations +Ġë© ĭ +ĠE lla +ook ed +ĠF it +aff le +ĠApr ès +ĠD uck +Ġcell ular +c ów +ĠÑĩÑĥв ÑģÑĤв +gen ommen +ìĬ¤í Ĭ¸ +Ġl ain +is ol +Ġhold ers +Ġbo oster +ĠS asha +Ñĭв аеÑĤ +ģ ¼ +Ġsepar ating +Ġrein forcement +Ġод ной +ìĹ Ĩ +ID E +ĠO ption +ph on +Ġpl ais +ĠC amb +ĠíĻ ĺ +Ġuncom mon +" : +mi yorum +mo i +ac je +аж Ñĥ +Õ ¶ +Ġgem s +ü ler +ool s +Ġenzy mes +Ġkidna pped +Ġk etchup +t alk +Ġz ach +Ġwas her +ãĢĤ ãĢĤ +ĠArch itect +ven ue +ĠPlan ning +éĢ ģ +ĠSav ior +ĠгÑĢÑĥп п +íĬ ¼ +ary a +Ġproces o +Ġlim bs +Ġreal izes +i ander +F S +aj i +Ġun ite +ĠìĿ ĺë +Ġposs ÃŃvel +ra its +ĠAg re +ÛĮ Ú© +ìĦ ľëıĦ +æİ ī +Ġв ел +Ġм еÑģÑı +an or +P at +Ġder nier +Ïĥ ÏĦε +Ġкак аÑı +Ġlä sst +æİ ° +ĠMe h +Ġng h +Ġam ateur +è« ĸ +F e +Ġê ¶ģ +Ġsitu ación +Ġsed an +Ġcleans ing +last ing +Ġcommun ist +AN E +Ġir regular +Ġs out +ĠCar ney +Ġall emaal +Ġmuch ÃŃs +Ġli bro +ÐŃ ÑĤо +Ġа п +Ġcontinu ation +ĠL or +?" , +qu in +Ġcharacter ized +aj es +Ġs ights +ĠÑı зÑĭ +ĠU hh +è· ³ +bir th +d ong +Ġhab lando +Ġsympt om +çµ Ĥ +Ġcapac itor +Ġtransport ed +Ġignor ant +Ġник огда +Ġdri p +ĠE va +Ġad ject +Ġmass ively +ĠEth i +ĠCir cle +Ġrain fall +ĠM ouse +Ġref und +ĠZ w +asse mb +Ġ2 20 +ĠOr d +è§ Ĵ +Ġve ins +ĠG iant +Ġmã e +Ġv ap +Ġmiss es +οÏħ ÏĤ +M o +ĠEnt wick +IN T +ÙĨ ت +Ġtheoret ically +Ġte aring +Ġtrou bled +p rem +Ġrepet itive +Ġâ ĸ +Ġheaven ly +ĠAm ber +Ġпол ож +Ġíķ´ì ¤ +Ġvow el +ank ing +ĠWir tschaft +Ġir r +Ġco zy +Ġunf amiliar +ĠP ors +Ġë§ŀ ìķĦ +ĠTim othy +Ñģол ÑİÑĤ +pe x +ĠV IS +) ( +Ġsuper st +Ġimpro v +ĠB eng +Ġdisconnect ed +Ġa pt +ÑĢ ÐµÐ½ +ĠExt ra +Ġб ел +sh op +d ings +ĠConnect icut +ì° ¬ +ĠG C +åı ĸ +be h +J eremy +ĠB att +ãģ ¸ +ath a +ĠZus ammen +scream s +Ġgr as +aff t +ĠInit ially +ĠB rett +Ġspecific ations +Ġsea weed +Ġo ath +Ġf ountain +ĠкоÑĤоÑĢ Ð¾Ð¹ +ĠSte in +èģ ² +ĠCorin th +Ġconj ug +å·¦ åı³ +Ġcompens ate +ĠëĬIJëĤĮ ìĿ´ +Ġon ze +Ġskin care +B rian +Ġserv ir +} } +ĠV ik +Ġun int +Ġsupp liers +Ġbalcon y +Ġenerg ia +omet ric +з Ñı +Ġs igh +ĠT OM +ĠP ure +yt t +Ñĭ Ñģ +ĠRain bow +ĠPitt s +×Ļ× ŀ +Ġstat ues +head s +Ġcou pled +éĮ ¢ +Ġher d +ä½ ĵ +Ġexclud ed +Ġg ilt +ĠÑ İ +Ġswo je +ĠS ver +6 3 +iss ant +Ġdür fen +ł Īë +Ġkiss ing +oo f +以 ä¸Ĭ +Ġcurs ed +Ġshow ers +Ġsw inging +Ġreprodu ce +ãģ¨ãģĦãģĨ ãģĵãģ¨ +Ġs ätt +el come +Ġfundament als +Ġal mond +Ġp é +Ġwell being +Ġhun ters +å¾ Ģ +S ec +ĵľë ¦´ +Ġem ission +Ġpsych ologist +Ġbetray ed +ĠRey nolds +L ES +Ġpo lling +Ġnegative ly +Ġcomb ines +׾ ×IJ +аÑĢ Ð° +λλ ά +ĠTurn s +OT T +Ġ×Ķ ×Ļ +ais on +Ġairl ine +Ġrestrict ion +w al +Ġaur ait +ĠLeban on +ĠM OR +Ġmon keys +é ner +Ñĸ ÑĹ +Ġmother f +ĠÙĩ ذÙĩ +Ġfe u +üh ren +Ġhyg iene +ente en +D es +Ġdiss ip +E st +Ġs aints +Ġpot assium +Ġreck on +Clint us +Ġmanifest ation +ĠApp ro +ĠIns pect +Ġvent ilation +Ġhel m +Ġk ara +า à¸Ļ +Ġfavor able +ĠìķĬ ìķĺ +ĠHispan ic +ภľ +Ġ×Ķ× Ľ +Ġvalid ate +ĠRes ident +Ġcom enz +be iter +er er +ä¸Ģ èµ· +Ġd ado +atch ing +met ros +ĠH in +ĠD um +Ġhaz ır +ĠNat alie +Ġencry ption +оÑĩ ка +m ma +h ouses +Ġanalyt ical +ĠD ang +f irst +æŃ Į +çº Į +ĠEn c +c ando +Ġlud zi +w art +Ġstat istic +ĠìĤ ° +Ġcomment ing +Ġcoord inated +ĠHy per +å ļ +ĠB ert +çľ ¾ +ĠH ip +ke m +ün ü +Ġz al +Ġíķĺ ëĬĶëį° +ĠRob ot +éĸ ± +ra wn +Ġrhet oric +ull ah +ĠD iet +Ġtak ich +Ġposs essed +ĵľ ëĬĶ +Ġw akes +ĠR af +M art +Ġe cc +ĠF M +Ġdif ic +ĠAll ez +Ġc ured +åŃ ¦ +ĠQu ad +Ġbe le +Ġjourn als +Ġt ad +Ġsocial es +æĩ Ĥ +Ġwhat s +ĠB ass +Ġjest em +ĠSad ly +ĠS ource +Ġü ç +alt ung +ier ten +Ġj ullie +if a +ĠÐļ оÑĢ +ĠDo or +ĠÐĿ ад +Ġзд оÑĢов +Ġrum or +Ġp ies +ĠпеÑĢ Ðµ +ĠоÑĤ в +ен нÑĭе +H ost +ĠSoph ie +ant en +A ny +ĠAuf g +ç¨ ĭ +ĠH DR +ĠRock et +res so +Ġver de +Ġprés ident +Ġindo ors +Ġst agger +Ġstat o +ĠD ial +Ġbuzz ing +em er +ĠÐĴÑģ Ñij +ĠдеÑĢ ÐµÐ² +Ġpou v +Ġstrand s +Ġê²ĥ ìĿ´ +ĠPar l +ок ой +Ġs ip +Ġ( * +äng t +Ġde ber +ĠA in +Ġdr astically +ĠSlow ly +ĠBr ig +ĠTor ah +Ġa che +Ġ? ?? +ĠD ob +kan nt +M ary +Ġst am +ĠDem on +pl a +ĠFre und +ĠB enn +Ġhigh s +ĠÚ© ر +ĠPrep are +Ġpro xy +Ġcamp o +ĠAug en +£ ¨ë +ĠCh loe +icular ly +you ng +Ġãģ Į +© Ķë +Ġscratch ing +Ġgl ac +Ġgemeins am +an al +acaks ın +ĠFor um +enn ial +ĠRes ources +ã썿ĢĿ ãģĦãģ¾ãģĻ +Ġme isten +ĠF ell +Ġun anim +ĠT B +ĠSel bst +æ Ĩ +Ġintimid ating +ĠGef ühl +Ġì½ Ķë¡ľ +æĭ ī +id or +ic iones +ars a +] .. +az o +Ġk endi +ĠT age +ter min +ĠPro zent +May be +l é +Ġquest i +Ġmem es +Ġcor re +ĠV IP +ĠGall ery +Ġur gency +Ġno che +Ġkind ly +ĠM eredith +Ġv áºŃy +ĠاÙĦ ب +ĠEst ado +åĩº ä¾Ĩ +z ug +o que +Ġobes ity +O ff +ĠEurope ans +ö d +ì¹ ´ë +Ġho op +Ġenjo ys +ĠCh ip +pat ient +Ġmicros cope +Ġlegit im +ĠÑıв лÑıеÑĤÑģÑı +Ïĥ ι +ar gent +Ġsh am +Ġlic ensing +ol ia +S orry +ram a +Ġacceler ated +Ġw ym +Ġfair ness +ĠRe ading +Ġsl ack +ĠD ok +ziÄĻk ujÄĻ +Ġrub bing +аÑĤ Ñĥ +Ġalloc ated +j ung +Ġpain s +Ġwind ing +Ġgel iyor +ĠC U +m ot +co ck +ĠP osition +br os +Ġlivest ream +ĠBra in +ì° © +Ġprz ek +ĠE i +ĠC oco +б а +Ġsho vel +ãĥı ãĥı +e a +Ġch ocol +Ġrebell ion +Ġshow c +ĠH alo +Ġdivid end +m ission +Ġus ando +Ġ[ " +Ġfale i +æĽ ¸ +Bl ack +ĠSure ly +ĠÅ » +Ġphilosop her +ä½ł 们 +Ġover he +ĠB orn +Ġobjet ivo +Ġ12 8 +sche id +ĠNaz is +Ġsol che +l ift +ced e +ad ors +Ġmarsh m +ĠL ORD +ĶìĿ´ íģ¬ +Ġow ning +C ont +Ġlandsca pes +Ġl ending +ĠAuthor ity +ов ой +o qu +ĠS es +ĠFerr ari +Ġrespons abil +Ġv ários +Ġdel ic +Ġemb ark +Ġembro ider +Ġframework s +Ġsim mer +Ġn acional +Ġrema inder +ĠVie lleicht +Ġquier es +ìĹ Ķ +Ġtest oster +i hen +ĠO z +è le +Ġportray ed +κ ε +ĠPolit ik +Ġapert ure +Ġbl and +ind ust +Ġоб ÑĢаÑĤ +ĠTh ous +B ay +Ġd ando +Ġsh er +Ġadm issions +ĠC rew +ĠÑĸ н +S INGING +Ġ ounce +Ġi y +Ġbas il +Ġover time +Ġthreat en +Ġpartner ed +ĠC ann +av ana +Ġзна еÑĤе +éĢĻ äºĽ +ĠоÑĤ Ñģ +ĠT udo +ì½ Ķ +ĠëĨ Ģë +f el +Ġre arr +Ġin ward +ĠRog ers +à¹ĥ ห +Ġtwe ak +Ġdry er +cess ion +Ġrig orous +ĠDa ar +om ics +Ġf ats +v ad +Ġz ipper +accept able +Ġdemonst rating +ĠY um +Ġbe au +Ġr oster +Ġpredomin antly +еÑĢ Ñĥ +ning ar +Ġtriang les +Ġtext ing +Ġber ries +ĠìĤ¬ ì§Ħ +éĶ Ļ +ad der +Ġfait es +ĠIm age +l ere +Ġb ounds +ĠLa ur +ĠìķĦë ¬´ë +Ġm io +Ġus a +ĠØ ° +Ġto en +ĠJ ang +ž e +ch od +an an +ĠобÑĢаз ом +Ġperse ver +ĠS we +Ġaug ment +ä¸ ĥ +ugg ling +ière ment +ist les +ac jÄĻ +9 1 +Ġma h +ĠK IR +D ie +Ġdown hill +Ġ196 8 +оÑĢоÑĪ Ð¾ +å¹ ¹ +ograph ics +Ġtä ssä +ê²ł ì£ł +Ġл иÑĩ +AUDI O +Ġпло Ñħ +Ġprop osing +éł » +Ġtempt ed +Ġconver ting +ĠLe hr +Ġpers one +ĠFe eling +ìĸ´ì £¼ +omb res +Ġ׾ ×Ļ +Ġg uru +Ġde ment +ни з +иÑĤ елей +Ġcomp añ +æľ ª +å¸Į æľĽ +Ġred o +Ġcondu ctor +m ia +Ġidol s +ĠM ul +Ġin ex +Ġt ämä +Ġimpact ing +Ġday light +g il +Ġhel fen +Ġents prech +ĠwiÄĻ ks +Ġscript ures +Ġdismiss ed +ãĥ³ ãĥĪ +ĠPod cast +Ùħ ر +Ġann ually +Ġus able +Ġli bre +оз м +Ġrub bish +çļĦ 人 +Ġcontinu ar +Ġhum ili +Ġspe eches +ÑĢа Ñĩ +b ard +7 1 +> < +olog ÃŃa +we alth +Ġmed itate +ĵ¤ ìĿĺ +ĠC raft +è§ī å¾Ĺ +æĻ ® +ri v +ĠAgain st +Ġcer amic +esp ère +Ġcompet ent +ĠHop kins +Ġkil os +Ġgra vel +Ġpist on +Ġfriends hips +Ġesc re +Ġvo z +ĠGes ellschaft +Ġunter stüt +Ġmu j +Ġwarning s +p os +ĠProfess ional +w szy +od le +b ands +Ġteam work +stell ung +Ġd x +åį Ĭ +Ġatt orneys +Ġweit ere +ãħĭãħĭ ãħĭ +ĠOrig inal +×Ļ× Ĺ +Ġbroadcast ing +ĠпеÑĢв Ñĭй +uch i +Ġhe ure +Ġgra bs +ĠW OR +ĠPla id +M in +Ġp az +ĠP uis +um u +it ates +Ġco ats +Ġbu en +Ġhe ir +Ġpne um +ש ר +ens er +ĠJUD GE +Ġbl onde +á¹ Ľ +Ġg ak +Ġs ık +Ġquot ed +Ġequip o +Ġw ishing +ÃŃ cia +Ġver bs +çµ Ħ +ĠCanad ians +Ġgover ning +ĠEv ans +E uro +Ġgen res +Ġunters chied +ĠBeck y +³¼ ê²ĮìļĶ +Ġe inge +ĠRa ise +ol and +ĠStr ateg +Ġer es +ĠVeter ans +Ġbreak out +Ġsant é +Ġad el +Ġinvestig ated +Ġpe ur +Ġag ile +Ġrail road +ans ka +Ġе й +Ġexp os +ator ies +ĠCont ent +Ġtruth s +ĠTra il +Ġgu a +Ġp ores +Ġwrit ings +ĠU hr +ĠThat s +Ġic ing +O C +ĠProdu ction +Ġcar ne +IS S +Ġn inguém +n on +Ġv icious +×ķ× Ķ +Ġrecon nect +Ġcent res +ĠK em +Ġcre ase +ĠìĿ´ë ¯¸ +айÑĤ еÑģÑĮ +Ġб оÑĢ +ĠHay ır +ĠÑģ Ñĥд +Ġún ica +owa ÅĤ +Ġad her +h ua +Z Z +Ġprecis o +Ġcurrent s +Ġseason ed +ĠIo T +ĠB ishop +è¨ Ī +st ed +ĠBern ard +ì¤ ĺ +æ² » +ĠGl enn +Ġktóry m +ื à¹Ī +Ġast rolog +ĠK ot +å¤ ľ +Ġparf ois +Ġfor wards +ĠW iÄĻ +ĠÎ ĺ +Ġn ano +è» į +s ub +ĠBr ill +Ġgr it +Ġc ited +g ado +Ġmel ts +Ġfor cé +âĸĪ âĸĪ +Ġb ajo +Ġdiscret ion +° ° +at ivity +Ġsitu ated +ãĥ« ãĤ¯ +Ñīе е +åľ° æĸ¹ +ĠпÑĢин ÑĨип +am az +Ġaqu arium +Ġdissol ve +ĠGod s +S uper +Ġam id +z k +Ġ ãģĦ +éł IJ +amp f +Ġhel a +' ! +Ġdevelopment al +ĠD ise +ĠÑĢабоÑĤ аеÑĤ +Ġsnaps hot +好 好 +Õ ¸ +ĠY ue +ĠH ulk +ĠDo om +ĠFel ix +Ġré f +M ale +ç· Ĭ +ph ants +EN S +ĠMe chan +ĠG olf +åĨį è¦ĭ +Ġgener osity +ät ze +Ġunlock ed +Ġ ãĤĴ +íĥ ģ +ocaly pse +Al right +Ġê° ľë +Ġ×IJ× ij׾ +ĠKeep ing +Ġcollabor ating +ch ief +ĠFern ando +Ġchef s +ĠíĶ¼ë ¶Ģ +Ġsk ipped +Ġperson n +Ġax e +che z +Ġextract ion +ĠA V +ĠGib bs +Ġí ľ +Ġs ı +I AM +V iew +ĠGR ANT +Ġëª ¸ +Ġver ification +Ġdep icted +ĠMo z +ou x +Ġt ul +Ġsc anner +Ġcomed ian +ĠVol ks +ĠJE FF +è¨Ĥ éĸ± +§ Ħ +Ġdistract ion +r á +ĠIN TER +Ġsin cer +Ġ×ŀ× ª +Ġש ׳ +Ġconstruct ive +ar f +ĠëĪ Ħë +Ġe co +r amos +Ġrenew ed +in ement +ĠU b +ĠPe pper +ì§Ģ ê°Ģ +ĠDar win +Ġmerch and +Ġv árias +è ce +N G +ĠìľĦ íķ´ìĦľ +Ġак ÑĤив +ĠUn ters +ع ÙĦ +Ġint ric +omm a +ie ving +ĠCarol ine +åĵ ģ +ĠPR ES +Ġperform er +Ġaut our +ãģ¾ãģĽ ãĤĵ +Ġutter ly +Ġsynth esis +Ġles bian +Ġretrie ve +Ġmane ira +Ġimp air +Ġment oring +ĠSoul s +ĠGo Pro +ÑĢ Ð°ÑĤÑĮ +Ġc ose +ĠSS D +I RE +Ġup front +ĠA un +Ġgam er +Ġl itt +Ġag gression +ĠLike wise +ĠBet ty +ĠD art +ĠD LC +ish ment +ìŀ¥ ìĿĦ +Ġ 对 +ç» ı +c ream +ĠBaby lon +Ġn ug +br ar +Ġa ynı +am ily +b ike +ahah aha +lo yd +Ġmir a +Ġper me +ĠG aming +Ġfirm ware +M a +Ġassist ed +at ics +Ġìķŀ ìľ¼ë¡ľ +ĠM ental +niej s +ĠI z +ow Äħ +Ġt ougher +Ġde ed +èĭ ¦ +Ġsty lish +ĠTool s +ĠH amp +Ġsun screen +Ġartic ulate +i ye +и ÑĦ +ĠSp read +ĠHA VE +Ġsw irl +Ġspons oring +ä» ĭ +iov ascular +mes i +Ġrelax ation +ĠÑģво иÑħ +Ġmar gins +Ġsa ÄŁ +ĠPr ide +ĠÏĦοÏħ ÏĤ +и ÑĨи +en ci +Do es +Ġcor pse +Ġend urance +Ġí ŀĺ +ì¹ ´ +Ġhair cut +Ġinterrupt ed +Ġwind y +ĠC aleb +Ïģ Ïĩ +ĠPour quoi +Ġhol istic +uc lear +ĠWho le +å£ « +A ct +Ġgall on +c ade +ĠReg ional +ro ads +ĠSch ne +á ng +Ġиз мен +ãĤĪ ãģŃ +Ġmen us +Ġspl itting +Ġpr iced +ĠÎ ĵ +Ġus ername +ĠÐŀ Ñĩ +Ġcomp ressed +y in +Ġguard ian +Ġgo of +Ġcheck list +Ġinter change +Ġexped ition +Ġex tern +Ġinfra red +eng o +Ġden ying +Ġpack ets +on ent +B B +ĠInc re +Ġsin i +ÃŁ er +è g +ma al +gen eration +Ġminor ities +Ġlle var +Ġnom ination +Ġcons id +Ġ×ľ× ¢ +m uÅŁ +ĠEs c +Ġnumer ator +Ġka ik +Ġktóry ch +ies en +Ġv ê +ĠUS S +ĠPri vate +Ġод но +Ġal ém +ÃŃt ulo +Ġlim b +Ġforg iven +Ġdiscl osure +ÏĦ ί +Ġning ún +Ġtherapeut ic +Ġnegoti ating +ĠN ike +ense ful +Ġin cap +Ġflag ship +t own +â Ī +ĠÏĢ Î¿Î» +Ġwol ves +Ġviol ations +ĠAr nold +Ġinterven e +Ġhe ater +Ġrecurs os +Ġma id +ê² ¼ +Ġдав айÑĤе +ĠCe lebr +Ġca pe +ĠSt y +ain en +s ite +b ij +Ġп олÑĮз +Ġfr amed +Ġpublish ers +ĠÑĩ ÑĥÑĤÑĮ +Ġtempt ation +Ġcert eza +Ġex empt +ìĬ ¹ +se lling +ĠT ask +ho on +ĠC oc +ĠPark s +Ġrepet ition +ĠÑĤ Ñĥда +Ġens l +ĠdeÄŁ iÅŁ +ĠOr lando +ĠMain ten +æŃ ¢ +oc ument +ĠH C +Ġscoot er +Ġнап иÑģ +Ġtight er +Ġte ase +Ġremo ves +Ġkij ken +ĠÑģÑĥ ÑīеÑģÑĤв +Ġth é +ĠвÑĭ глÑıд +Ġrel ieve +Ġmit ä +Ġstation ary +ö ff +p able +Ġar ter +Ġdé f +r ative +Ġcon ect +Ġsad dle +ĠD iane +Ġcomm emor +fend im +S ÃŃ +Ġíģ ´ë +Ġman ge +at te +Ġarrog ant +Ġrobot ic +Ġgi Ãł +æĺ¯ çļĦ +Ġneighbour hood +iss on +Ġдв иж +ĠR I +ĠNorm an +b rand +am ation +Ġraz or +Ġmur ders +ĠÑĤ Ñĥ +Ġwszystk im +Ġut ilities +Ġmicros cop +ê ¿ +Ġda qui +oll ar +ĠÐĶав айÑĤе +Ġann ée +Ġkilomet res +Ġhom osexual +Ġarchitect s +ãģ¡ ãģ¯ +Ġni ye +L ER +Ġmicro phones +ĠSt unden +Ġconsecut ive +iend a +v änd +D ER +Ġlif ts +ĠMe at +Ġsave z +íĸ Īëįĺ +M en +Ġdism ant +ê±°ë ¥¼ +Ġins ulation +Ġsc all +Ġsp ooky +Ġpar c +Ġball et +ĠWhats App +Ġfr anc +Ġdeliber ate +Ġíħ Į +Ġm ars +ĠZ ur +P r +dis ciplinary +Ġobs ession +м е +Ġmarch ing +ĠEmer gency +ig uous +Ġs zy +ĠL ands +Ġboard ing +ĠпоÑĩ ÑĤи +Ġenv y +Ġcompassion ate +Ġmer ci +Ġdes irable +d ale +Ġcan ım +ĠAnt ar +tem ps +Ġconfig ured +ĠComp ared +ne h +ic ating +Ġnic kel +ÙĪ ÙĤ +Ùĥ ÙĪÙĨ +op es +Ġform ulas +ĠÐķ ÑģÑĤÑĮ +Ġpo bl +ĠP J +ĠL ud +ä»Ĭ åĽŀ +ĠBr id +ĠH og +ĠBr is +J en +Ġshad ing +ĠY as +Ġdistur bed +Ġrecomm ending +Ġc é +ĠH OW +ìĹĪ ìĸ´ +Ġrevers ed +ĠInteresting ly +iox id +åħ Ń +Ġìĺ¤ ì¼ĢìĿ´ +ế u +x x +Ġou ais +ĠYouT ubers +ĠR osa +ĠH aupt +j adi +Ġvlog s +Ġcult ura +ĠLeaders hip +ĠH ep +Ġill um +´ë ıĻ +Ġcustom ized +Ġmar ca +Ġqu atro +Ġн аг +ĠSpace X +ĠE igen +ast ing +ĠolduÄŁ u +Ġfor ts +ãģ ī +r iment +ien cia +Ġten ir +ro ffen +Ġ197 9 +Ġc ie +ĠëIJĺ ê³ł +Ġes cri +ÏĮ ÏĤ +íı ¬ +uz zy +C ong +ìĿ¸ ìĿ´ +G reat +s il +é ch +ãģ¨ ãģĭ +Ġmult ic +ĠDis k +² ķ +Ġfaz la +Ġle vant +Ġab ajo +ur ry +st ru +Ġ먹 ëĬĶ +Ġaccess ory +Ġдв иг +ĠR id +20 19 +Ġdown stream +æķ ¸ +Ġk az +ut an +Ġchar coal +Ġa fect +w u +Ġcontext s +Ġfe ared +ĠìĦ ¤ +Ġhist ories +Ġf as +ens ible +Ġcoco a +ill ar +ge ons +Ġspiritual ity +ĠP ew +Ġpharm acy +Ġpass ions +Ġb os +Ġall á +Ġthri ving +ĠRe act +Ġoccup y +Ġwithdraw al +Ġallow ance +ĠFra ktion +Ġbud dies +Ġid le +Ġdissol ved +Ġpreval ent +Ġmil itar +Ġsens ing +Ġpo jaw +Ġanc ora +Ġabund ant +Ġha irst +ãģĤ ãĤĮ +Ġtw ee +Ġnäch ste +ĠMöglich keit +Ġho o +uff icient +Ġfant ast +Ġed ible +Ġëĸ¨ ìĸ´ì +ìĽ ĥ +Ġve in +uc ci +Ġdevot ion +Ġconce aler +in come +Ġrecy cled +ĠìĬ¤í ĥĢ +Ġpont os +Ġdess us +Ġvé rit +Ġreflect ions +ĠA A +Ġtake away +b are +ĠCont act +e il +ĠHe ar +Ġmir ac +ĠGer ilim +ĠÑģам Ñĭй +Ġv ivo +Ġkilogram s +ĠCr im +û t +7 8 +Ġsincere ly +ra z +Ġë³ µ +Ġarri v +Ġconcept ion +ĠPers ian +Ġsj äl +Ġst arring +ĠìķĦë ¬´ +ĠFore ver +е ÑģÑĤÑĮ +Ġve il +Ġsubt it +od ka +ĠоÑĤно ÑĪ +Ġcook s +ен Ñı +K ay +Ġni ños +ĠPh one +Ġstitch ing +Ġfinger print +é¢ ĺ +λ ά +Ġded icate +ĠL ob +Ġblack s +ĠB le +b out +ĠÄij ang +Ġe ks +Ġsqu ash +ĠK ü +od i +Ġn Æ°á»Ľc +Ġvoy age +Ġplay ful +ĠØ¥ ÙĦÙī +an ic +Ġcondem n +ĠB öyle +ĠPol ize +ãĤ¿ ãĥ¼ +Ġay uda +Ġp am +à¹Ħ à¸Ľ +ĠK athy +ед ин +нов а +Ġbr ig +eg er +Ġe agle +Ġvis ions +ĠíķŃ ìĥģ +Ġsh itty +Ġh ott +ĠBr itt +ut ors +ENT E +æĽ ² +Ġph on +ĠB ing +Ġпод деÑĢж +spr ing +æĸ ¯ +et ten +Ġpil gr +Ġed iyor +енÑĤ Ñĭ +ag gio +Ġj ul +Ġcomp rend +te il +ĠØ ² +Ġperform ers +Ġinf amous +ĠM K +ç ª +æ³ ģ +ot le +e ff +ĠH ash +Ġcow ard +ĠB RA +ĠD D +Ġcom ida +Ġpl ata +Ġfl ap +ĠMe hr +rib ution +ĠY emen +Ġmyster ies +Ġİ yi +Ġst ell +Ġeyel iner +Ġdel es +Ġnail ed +Ġillness es +Ġst acks +Ġtrabaj ar +fl ower +ci u +Ġcr ude +Ġsubstant ially +Ġhome m +Ġnep hew +Ġstamp s +Ġcar bs +ÑĮ ÑĤе +mo oth +Ġtun nels +ac ie +æ³ ¢ +ĠSe ñ +ĠH era +ĠìķĦëĭĪ ìĹIJìļĶ +ĠWy oming +ĠHD MI +ĠL is +u ción +Ġste er +о Ñİ +иÑĤ а +N T +Ġìĸ¼êµ ´ +Ġpal ms +Ġne on +ов аниÑı +Ġfilter ing +Ġjou er +ĠH ö +Ġне Ñģ +ê²ł ìĸ´ìļĶ +Ġ8 1 +Ġstory line +Ġprz ep +Ġthank ing +ĠBo eing +Ġsoft ly +j em +алÑĮ нÑĭÑħ +Ġflash light +Ġп Ñĥ +ĠW OMAN +ắ c +ÃŃ ch +Ġlux urious +Ġw ün +Ġimpact ful +Ġcons on +re u +ir ring +if ter +Ġconstitu ents +èIJ ½ +Ġ9 4 +ĠT ou +g om +ĠìĥĿê°ģ ìĿĦ +Ġstere otypes +Ġmoż li +åĪĨ 享 +Ĥ ¨ +Ġpencil s +ĠÑģл ож +Ġih rem +ĠBes ch +ĠK oh +ĠEnt scheid +Ġle k +Ġför s +Ġtotal mente +Ġlive ly +Ġent ropy +Ġdisc ern +ĠÐĹ Ð½Ð° +Ġdo v +Ġmyth ology +è¨ĺ å¾Ĺ +apan ese +Ġapprox imate +аÑĤ ив +if iable +ĠSe o +åĢ Ĵ +´ìĭ¬ íŀĪ +Ġìĺ · +Ġtempor al +Ġi T +Ġest at +к им +Ġspr ink +Ġgr und +Ġinfant ry +Ġsch affen +ç´ Ħ +Ġan k +ri ages +ĠYe on +ĠMor oc +Ġinv asive +ģ Ķ +Ġparent ing +ĠR is +ib ile +Ġmod s +å½ ¢ +ĠпÑĢов еÑĢ +ĠTh ing +ĠWhere ver +Ġacknowled ging +Ġpa wn +um mer +or b +6 9 +Ġretr ouve +Ġrel ies +ĠHigh way +Ġa we +ãģ§ãģĻ ãģĭ +ita ire +Ġapplic ant +Ġais le +w orm +Ġpay load +Ġcar re +ĠB ach +æł ¼ +Ġì¹ľ 구ë +ни е +Ġit ÃŃs +onna ise +s ol +èı ¯ +alg ia +Ġrock ing +Ġbest en +rit es +^ ^ +ин ой +Ġba ixo +Ġ기 ìĸµ +оÑĤ ÑĢи +s im +Ġinc arn +ëĭ¤ ìĿĮ +Ġl ick +s ided +Ġ7 1 +f order +Ġreson ance +Ġte gen +Ġmet aph +ows er +Ġ×IJ× ł×Ĺ׳×ķ +? ãĢį +Ġsp ielen +Ġvoll ey +ĶìĿ´íģ¬ ìĹħ +lo oked +Ġsent enced +Ġmultip lying +Ġide als +Ġwahr scheinlich +Ġdepos its +bil ir +Ġeff et +ill on +Īë §Į +Ġtestim on +Ġz awsze +ĠпÑĢоÑĨ еÑģÑģ +ĠL av +ä¸į éĮ¯ +Ġtrava iller +Ġla isse +ĠMount ains +ĠÑĢ Ð¾Ð± +Ġexam ined +it us +W as +л Ñĭ +Ġattrib uted +ĠìĬ ¹ +ĠBar on +Ġg ep +Ġatt ent +ĠColl ection +Ġthe at +ĠC ai +Ġwell s +Ġhuman o +çĹ ħ +ĠH ast +ĠÑħоÑĤ Ñı +cz as +Ġperm its +Ġle gg +Ġe po +ĠF en +Ġth i +ĠF oi +Ġé lect +Ġ8 3 +Ġover th +Ġ è¬Ŀè¬Ŀ +Ġten ant +è² · +N ext +Ġpra ised +sec urity +ĠImp act +为 ä»Ģä¹Ī +Ġv ouch +Ġneg ó +Ġun ve +Ġcritic ize +ĠKen ya +Ġtact ic +Ġlo gr +Ġpo is +Ġpap a +spe aks +ðŁ ij +isp ers +Ġsur plus +Ġcold er +åį Ĺ +åIJ ¬ +pl ets +ĠV ienna +ĠLe ad +Ġaer ial +ĠT ah +енÑĤ ов +ĠGree ks +C am +Ġmá xim +Ġk uin +ch io +Ġdemonst rates +an os +ĠC ert +ĠÑį н +Ġblog s +ĠìĦľ ìļ¸ +Ġbe ams +ик ов +Ġprompt ed +Ġfright ening +ĠPors che +ãģĪ ãģ¦ +lar ını +Ġch illing +is phere +Ġfl ashing +ĠK ard +b read +Ġex h +Ġty cker +Ġec ological +ĠMa e +Ġ×ŀ×IJ ×ķ×ĵ +ĠëĤ ĺëıĦ +л он +ys s +Ġper gunt +Ġpri x +izz ard +Ġcan cers +Ġ9 1 +s usp +ĠIt em +ÅŁ a +Ġp est +Ġtak Äħ +Ġl ymph +ĠPat ri +f ill +Ġrec onna +Ġoptim ism +Ġmim ic +Ġì² ľ +ĠMad ame +oc y +l ining +åijĬ 訴 +erm e +Ġfold ers +Ġcz ÅĤ +uch ar +Ġcur so +Ġbre ach +ни ÑĤÑĮ +Ġp amiÄĻ +Ġel ig +Ġaut op +F low +Ġprogram med +ĠPro cess +Ġfig ur +ĠS F +ĠE les +Ġprogram mes +Ġdiz zy +ìĭľ ê°Ħ +Ġли бо +Ġsn iff +ĠSeb astian +ĠH ye +Ġ4 000 +Ġperm ite +æ¢ Ŀ +Ġза Ñī +Ġgu it +ĠD ais +Ġaccord ance +Ġmod ular +ogene ous +æĭ į +Ġpou quinho +Ġart illery +Ġlub ric +Ġvol can +ĠN H +ðŁ ¤ +Ġde an +R h +Ġminist re +åĿ IJ +ĠIn v +ĠBul gar +ĠD aten +è İ +I m +Ġorigin ated +ĠN ixon +inte gr +Ġlack s +ĠN acht +ìĸ´ë Ĥĺ +cam era +Ġrad ish +ki ye +Ġang es +Ġpré f +j uk +ĠBe e +ĠB U +ĠвоÑģ п +ĠB T +ê mes +ĠSt ück +ĠIn k +æĪĸ èĢħ +ĠSerge ant +ĠMult ip +Ġhiç bir +ĠС ам +ĠD é +ol ph +ìĸ ¸ +Ġimp at +ĠìķĬ ê³ł +ĠÑĤак ого +ĠнавеÑĢ Ð½Ð¾Ðµ +Ġunpredict able +Ġm end +ĠìĹĨ ìĸ´ìļĶ +Ġjakie ÅĽ +Ġann i +Ġdon né +ĠK irsty +Ġrectang ular +Ġempez ar +ĠEx change +ê° Ķ +Ġé conom +ãģĵ ãĤĵ +el in +re ibt +Ġ×Ķ× ¤ +Ġc emetery +Ġespañ ol +ol in +лÑİ Ð´ +Ġgr âce +all en +ĠPh ilos +ĠEr st +Ġìĥ Ī +ĠV id +G ive +O H +μ ο +ĠP are +Ġmetabol ism +Ġma ple +Ġax le +ĠD y +Ġkomm e +Ïİ Î½ +Ġgreat ness +Ġver ified +Ġsp é +ĠFahren heit +ĠB ren +ĠConf eder +Ġhist oire +Ġelimin ating +ĠAd ding +ĠAb i +æĿ İ +Ġhospital ity +t im +Ġbon ito +Ġpart es +ĠдÑĢÑĥг иÑħ +ĠSh ay +ĠS ed +Ġreg rets +Ñı ми +Ġten ants +éĢ Ł +ĠP TS +Ġdev i +ĠL ate +ue z +Ġsö yl +ãĤ » +Ġìŀ¬ë °Į +Ġtogg le +Ġmas king +алÑĮ ного +Ġpers ön +Ġamer ican +f ik +ĠR GB +ens on +ĠK A +ww ww +ĠÑĢ ÐµÐ³ +met ics +Ġeduc ator +ãĤ· ãĥ«ãĤ¯ +p ark +елÑĮ зÑı +ar us +ÑĢ ÐµÑĤ +Ġfe ito +Ġcho ir +Ġlar go +Ġe ens +Ġwat ts +ĠSing le +Ġsuscept ible +ic er +Ġв клÑİÑĩ +Ġp us +íĻ ĺ +E ng +Ġfant as +Ġspecific ation +Ġconfront ed +ĠColumb us +ив еÑĤ +ar ım +Ġcaffe ine +mun ition +Ġmig rants +l ide +it ations +ĠG eme +Ạ« +Ġpl anner +Ġstim ulate +Ġapro xim +ce u +ĠN om +Ġv og +ĠÑĢ Ð°ÑģÑĤ +Ġense ñ +Ġsell ers +Ġgut en +z d +C al +Ġdescri pt +Ġrecon ciliation +z inho +á¹ĩ a +ãģĺãĤĥ ãģĤ +acy j +ĠCO L +s aw +ĠíĻķ ìĿ¸ +Ġvar it +Ġpartner ing +Ġdet ention +Ġbomb ing +c lapping +ien cies +ond u +AM E +Ġê°Ļ ìĬµëĭĪëĭ¤ +c ÃŃa +ĠпоÑģ ÑĤо +ĠAS MR +Ġhome page +Ġsi è +an tha +ĠP oll +Ġ igen +cy ch +Ġê°ij ìŀIJ기 +Ġconsider ably +ä»ĸ çļĦ +ĠAr ist +Ġwith stand +Ġqual itative +ĠK raft +ĠÑį лекÑĤ +ĠBe ad +екÑĤ ив +Ġcr ushing +ì³ IJ +Ġnav y +ÙĪ Úº +s ho +Ġo ak +ipp ers +Ġso ils +Ġpig ment +Ġev itar +ãĥ ĩ +Ġf use +ĠD ale +: " +Ġcompl ètement +Ġke l +๠Ĩ +Ġqu atre +ĠU M +Ġë§ IJë +æł ¹ +ÃŃ r +Ġle isure +ĠH ousing +Ġfold s +est ion +AR S +Ġm ash +urp ose +Ġaccum ulated +ĠSt uff +èª ŀ +Ġtap es +ĠÑģ илÑĮно +ĠLO VE +Ġ198 2 +Ġsc ars +Ġcapital ist +ĠN ed +Ġsoft en +Ġnot ably +Ġforcé ment +ĠRa um +Ġнеоб Ñħод +Ġtrad emark +Ġfert ig +Ġ? ! +æĹ ł +Ġreinfor ced +Ġre charge +ĠPut ting +Ġvill ains +Ġhand ic +Ġadvertis ement +ت ÙĬ +ĠÑģ Ñĥм +ĠR iley +×ķ× ij× +äº ¬ +O s +Ø§Ø ² +B oy +Ġsqu ish +ock et +Ġtest ify +æ¼ Ķ +Ġ×ľ× ŀ× +Ġм аÑģÑģ +man uel +ĠArk ansas +if fe +Ġanalyst s +ĠDe af +Ġj ó +Ġgrocer ies +ĠWhe el +ĠÑĢ Ð¸Ñģ +Ġc òn +ĠC ob +Ġpris ons +è ve +ĠCab inet +Ġpos ed +Ġguer re +ĠL loyd +Ġcl erk +Ġcr ises +ĠSh o +ĠO re +ĠFoot ball +ĠAd vis +ĠZh eng +è į +ĠAM Y +Ġun for +Ġmon aster +Ġcomp ile +Ġimm ortal +at able +Ġpar ano +Ġt iver +ĠStep h +ĠFu ÃŁ +Ġdisc ontin +Ġr ipe +Ġhack ing +Ġs iendo +Ġsegu ro +alt res +Ġand eres +Ġë ¦¬ë +Ġexp orts +æŃ ¥ +Ġtab ii +Ġ기 ëĭ¤ë +Ġbother ing +Ġpick le +ĠBRI AN +Ġalt ar +ĠпÑĢи б +Ġtransfer ring +ĠV ors +ĠÙĩ ÙĪ +ĠZ a +ĠFr ances +Ġbrow se +em it +Ġche wing +ĠFred dy +Ġedit ors +ä lle +Ġí ĮĢ +ĠS que +ĠC ultural +aw k +ĠS ache +ĠCar bon +ắ t +F L +ĠN GO +pe ÅĤ +ĠS ou +Ġh vor +un intelligible +Ġë² ķ +Ġ ° +i in +Ġ×¢ ×Ŀ +Ġder rière +Ġczy m +ĠAp ost +Ġregard er +Ġag rade +ĠC andy +Ġma re +Ġintrodu ces +bird s +Ġuniqu ely +Ġm uk +Ġcook er +Ġcrew s +Ġje ito +ER T +¶ Ħë +n isse +Ġe f +Ġcart e +ĠY ak +ĠP AT +и но +bok ki +Ġm ates +Ġdist int +Ġì½Ķë¡ľ ëĤĺ +Ġy ıl +Ġκ άν +Ġconfigur ations +eng a +re cht +H appy +ãĤĦ ãģ£ãģ¦ +in vest +Ġreconst ruct +ĠÑįÑĤ омÑĥ +Ġmos que +ra um +Ġvoy ez +ĠN BC +ĠìŀIJ ìĭł +Ġstur dy +Ġк ап +Ġans ch +al id +Ġmas ih +ĠR EP +Ġì½ Ķë +Ġded uct +Ġsal ir +w urf +il ot +ĠM utter +old s +ĠF EMA +ĠB ib +Ġneighb oring +Ġbl iss +Ġíĺ ¼ +ли ÑģÑĮ +ĠÑĤÑĢ ÐµÐ± +Ġ å°±æĺ¯ +Ġgren ade +Ġe gal +Ġfin ely +Ġpet als +Ġke er +Ġch yba +Ġsk ipping +Ġth irteen +Ġgrav y +ĠS AT +6 1 +Ġн ог +Ġmin s +IT E +Ġso zial +íķĺë ©´ìĦľ +rukt ur +Ġвозм ож +Ġоп ÑıÑĤÑĮ +Ġar th +ĠCub an +Ġtre asures +Ġfertil izer +Ġawak ening +Ġë°± ìĭł +Ġr all +Ġdep ict +ĠP ablo +Ġninete en +Ġw att +Ġentire ty +K S +ĠWood s +S ch +ĠÚ© ÙĪ +ĠD ry +ãģ ŀ +u ve +Ġreconst ruction +Ġanat omy +Īë ¥¼ +Ġb aba +Ġlisten er +Ġshar pen +ĠPer u +ĠвÑĭ з +Ġrecre ation +Ġiniti ate +Ġcal or +ĠN aj +ge e +ĠFe els +ĠSnap chat +ĠT et +ĠN est +ĠD af +ĠFin ish +ĠÑĤак им +ú c +iz ens +Ġsp ins +Ġemb ry +Ġpass ages +Ġc ient +Ġjust ification +ä»ĸ 說 +Ġolm az +Ġflood ed +Ġemo ji +Ġembr acing +Ġdisc ard +ĠBas ic +ag og +ĠìľĦ íķ´ +Ġas ylum +er in +Ġf im +Ġnin ja +Ġautom ate +Ġaller gic +ÿÿ ÿÿ +am am +Ġм аÑĢ +ĠO i +ä us +Ġin duct +ĠB EN +Ġz ÅĤ +Ġkaż dy +ĠAM P +n ÄĽ +S ure +Ġqu il +Ġespe c +ro k +BS CRI +Ġlie be +p us +ach sen +Ġcr icket +ëĬ IJ +ĠFr ame +ekk ür +ar b +Ġp ÅĻ +иÑģ Ñģ +Ġzeg gen +Ġdou bles +ĠD re +t est +ins p +bo ys +Ġm ão +ĠVer se +Ġmus cular +ĠMA LE +Ġd ulu +Ġoccas ional +L o +conom ic +Ġv ak +Ġrem edy +å¤ ł +ĠâĻªâĻª âĻª +ve m +Ġön em +ĠkarÅŁ ı +ĠSh arp +h ur +Ġë°© ë²ķ +Ġgrand son +Ġakt iv +ĠTh rones +ĠìķĪ ìĹIJ +Ġto ts +Ġsub d +ĠPa ula +Ġgra ves +ĠB rent +Ġник ÑĤо +Ġsö z +Ġcre c +ĠVlad imir +çĸ « +Ġп ой +Ġ" - +Ġp sy +at ri +id an +Ġa ún +Ġstandard ized +ì¹ ĺë +Ġк ÑĢов +ĠZh u +s omething +Ġ7 50 +Ġmuj eres +Ġa it +éĹ ´ +ag u +Ġcorrect ed +ik ka +el ed +ĠCare er +ow ym +Ġroomm ate +Ġdescend ants +ĠNapole on +ĠÐĶ Ð¾ +íĸĪ ìĸ´ìļĶ +Ġbun un +ĠMich a +ç· ļ +Ġdesc ob +P I +Ġpalab ra +Ġtrack ed +Ġdepend ence +ĠBar ack +åģ ĩ +Ġfert ility +ĠSouth west +Ġincom plete +Ġcomun ic +Ġcomp ris +ĠRest aur +Ġac ron +κ α +Ġapprent ices +Ġmus st +ĠA br +Ġpent ru +ĠCons ort +ĠAve c +Ġdum plings +L R +Ġwszystk ie +Ġsw amp +н ев +ugg le +Ġwater color +Ġprot on +ĠEspa ña +ock ing +ов ал +Ġtak im +V ery +Ġdement ia +ĠÅŁey i +J ac +ĠMac Book +ĠL iv +ffic ients +ĠH unt +Ġover lay +æĦŁ è¦º +ĠSky pe +p unkt +Ġconf ined +ĠAd rian +ر Ùĥ +ĠJe ep +Ġenqu anto +Ġan est +оÑĤ веÑĤ +Ġм енÑĮ +Ġirrig ation +á»ij n +Ġeight een +ĠP on +Ġresc ued +Ġ198 3 +r ü +ja e +ĠJe ong +Ġamazing ly +ĠF DP +Ġback stage +c ue +ĠÏĥÏĦη ν +ĠاÙĦØ µ +Ġlivest ock +ĠW arner +Ġmaj ors +ãĥģ ãĥ£ +Ġcooper ative +ĠBr ady +ra ined +rie b +Ġ×ij× ŀ× +Ġдов олÑĮно +ĠF E +Ġle aked +ĠMerc ury +Ġpersu ade +Ġtransform er +ĠNor weg +ĠìĹ¬ë Ł¬ +Ġzrobi Äĩ +Ġcard iovascular +ĠCr ash +Ġg ossip +а ÑģÑĤÑĮ +Ġì ª½ +Ġsw ept +ĠH orn +ĠAt é +Ġbu kan +ĠK aw +K Y +ĠSt ories +G ary +Ġgard ening +ĠQuick ly +ĠFal con +Ġov at +c ı +ĠCom plet +ĠD ate +ĠпÑĢ Ð¸Ð¼ +Ġlä uft +ĠAud rey +ĠW ent +Ġpel ÃŃcul +Ġcar riage +Ġun acceptable +ny mi +ĠÑģл ÑĭÑĪ +Ġter re +uell ement +EE EE +Ġpharm ac +h ões +Ġz ich +Ġmig rate +ĠF ry +ñ ana +ĠM uito +EO VER +Ġfort ress +ĠCom pan +ĠJ SON +ord nung +Ġw arto +Ġun gef +ìħĶ ìĦľ +ĠÑĢ Ð¾Ðº +Ġpad dle +J ared +Ġsubm itting +Ġl atch +Ġf ug +Ġк оÑģ +ĠE f +Ġlaunch es +Ġf t +ote chn +Ġtrave lled +ا Ùģ +éģ ķ +Ġpro ch +Ġded im +8 3 +Ġreb ound +ĠL U +p ath +ĠÑģп ÑĢав +Ġö l +ĠíĤ ¤ +Ġpriv at +Ġtr actor +ĠAtt ention +S er +Ġcos es +á ria +p al +ĠìĿ Ģ +Ġsuccess or +Ġconnect ors +ĠÑĥÑģÑĤ анов +Ġgen ocide +Ġsufficient ly +ĠA ixò +Ġstabil ize +Ġcon gest +Ġcar ving +Ġz ost +ĠбÑĭ ÑģÑĤÑĢо +Ġshort est +Ġli vel +Ġ8 9 +éģ Ĭ +Ġer k +Ġport raits +à¥ Ģ +è ĺ +bo at +ll ah +AN C +Ġempir ical +ĠE cho +ĠNeder land +è¿Ļ ä¹Ī +N et +Ġcuid ado +ĠR oma +Ġc alf +Ġgi ants +ĠExpl orer +ĠColl ect +al ition +ĠDest iny +Ġaus ge +ĠE du +ĠC lo +Ġear rings +ĠTr ack +ĠR OS +ĠBe lle +çĻ ¾ +Ġpu eda +Ġday time +Ġsupp lier +ĠS V +ĠEx hale +Ġgal era +c ourse +Ġcent imeter +ĠB ast +m ud +Ġsang at +ĠPhys ical +Ġpriv ately +Ġtr ata +lyn n +ill i +Ġë© ĶìĿ´íģ¬ìĹħ +Ġcryst all +Ġpod s +ả n +in ator +ĠRec ords +å® ĺ +ÄŁim iz +isse ment +h are +h adow +ĠD K +ĠìķĮ ê³ł +Ġw yn +Ġrequest ing +ĠD onna +ĠìĹ ´ìĭ¬íŀĪ +ine a +Ġex ert +ĠDun can +Ġв еÑĩ +ĠH ah +ठĤ +ĠL if +ĠF inding +ĠNo v +Ġзн ак +Ġо ÑĦ +ĠQu è +Ġquarter back +ĠÑĦ ак +Ġbipart isan +ÄŁ in +Ġné cess +Ġrefer endum +Ġcomp iler +Ġprob abil +ед и +Ġtrad er +æĺ ĵ +ĠR um +ge me +Ġd io +ĠbÄĻdzie my +ĠÏĢ Î¬ +ê¾ ¸ +×ķ× ĺ +Ġठķ +Ġбл аг +Ġscal p +ĠPa use +Ġcapt ion +Ġend anger +Ġen lar +Ġrot ten +ãĥĥ ãĥĪ +Ġw ah +èĤ ī +Ġd zi +ĠInst all +A y +Ġcre ar +енÑĤ а +Ġwe ighing +Ġbutter flies +ĠG ast +äº ķ +h orn +war z +IC EOVER +Ġнай ÑĤи +Ġcoe fficients +ç°¡ åĸ® +ĠSp encer +ĠH igher +Ġcow ork +å¨ ĺ +ĠкоÑĤоÑĢ Ð¾Ðµ +Ġmon it +Ġdys function +ĠÑģÑĤ анов +Ġtour naments +Ġoy ster +B N +Ġtr ud +sl ow +ĠPen ny +ĠOd ys +æ r +Ġf ou +Ġenjoy ment +аÑĤ Ñĭ +Ġwygl Äħda +алÑĮ наÑı +ĠProt ect +Ġmo y +Ġcl aw +Ġsusp icion +Ġsacrific ed +Ġgost o +B ig +Ġaggress ively +Ġvor ne +ãĥ ł +Ġbl amed +ĠSe hr +פ ר +c ito +Ġse als +Ġmu jer +ĠWe ird +Ġfore ns +Ġcontrib utes +est ra +Ġp og +L OL +Ġhacer lo +о ÑĤÑĮ +f iction +7 9 +λ ο +大 æ¦Ĥ +å£ ° +ĠÑĤ об +ĠG S +ĠCl ara +ite z +Ġadvoc ating +ĠíĶ Ħë +s ung +Ġvert ices +Ġnavig ating +Ġeurop é +çļ Ĩ +Ġslow ed +Ġfore ground +ĠIndust rial +Ġad ore +ìĭ Ń +Ġcré er +æŀ Ĺ +chn itt +Ġun aware +Ġcur ly +ent ar +Ġl er +Ġprohib ited +ĠHero es +ĠRe ed +u ca +Ġsm ok +Ġkun na +zeit ig +im men +ĠL un +Ġаб ÑģолÑİÑĤ +Ġdeg li +Ġvill agers +Ġpres et +z ept +ud s +Ġem it +ä½ł è¦ģ +Ġë ī +ëĬĶ ì§Ģ +нак о +Ġos ób +Ġ196 9 +ĠÐIJ ÑĢ +Ġman chmal +ĠBro ck +Ġmant ra +ĠW IL +b ach +in ä +el as +kel n +Ġdisci ple +Ġqual c +Ġde hyd +ìĿ´ë Ŀ¼ëĬĶ +A f +ìĦ± ìĿ´ +R yan +Ġpupp et +ĠдÑĢÑĥг ие +Ġr ud +Ġp ending +P lus +ĠìķĬ ìĿĦ +Ġb á»ĭ +ĠSe ga +ç e +Ġprogram mer +b li +Ġun l +Ġensl aved +Ġsoci été +Äģ h +Ġinherit ance +ĠBang l +erm aid +Ġpractition er +ĠSt alin +ĠUs er +ci ble +Ġcard iac +ĠKore ans +Ġdump ed +Ġ×Ķ ×Ļ×Ķ +á is +Ġhydraul ic +oubt edly +ĠP it +Ġpic nic +Ġbehö ver +ĠÑģм ог +Ġbra king +é» ij +ut ar +ĠìĦ ¸ë +ub l +Ġü z +Ġmaj esty +Ġb ers +ut able +Ġhot ter +çħ § +ÛĮ ÙĨ +Ġbi ases +Ġsubject ed +Ġnaught y +Ġcir cus +ãģĹ ãģĭ +ĠIm medi +ĠSte fan +ĠTri ple +en k +Ġw it +Ġrecy cle +em ie +d ated +Ġun load +Ġpop ula +ch in +Ġyield s +Ġeng lish +ĠBon nie +Ġsp iders +à ģ +Ġer osion +éĥ¨ åĪĨ +ĠN ICK +иÑı Ñħ +Ġimp art +Ġк ни +Ġres olutions +Ġlith ium +Ġconver gence +ĠT ara +Ġдв е +th s +ĠCind y +æĪij è¦ģ +å¹ « +ĠD IE +Ġass urance +Ġоп иÑģ +Ġbu ckets +Ġc ues +ĠQu iet +Ġsimilar ity +Ġfound ational +ĠMin ist +æ» ¿ +Ġp ian +Ġcent r +Ġnum b +Ġmon ks +uj ourd +en zie +Ġskate board +Ġd latego +ĠÑģ оÑĤ +ĠA E +Ġmaster piece +ĠSol omon +ĠRed dit +Ġr iot +ab l +ĠJ azz +Ġelectromagn etic +Ġinsec ure +ĠComp et +ger ies +об од +ł ×ķ +ðŁ Ĵ +Ġsen ators +ĠBris bane +ĠAl b +utter ing +ĠAll ow +z ero +Ġp ai +ĠÐIJ лекÑģ +ĠDis play +ĠBl ade +ĠApp s +Ġp ä +Ġд еÑģÑı +Ġque lla +ĠGa o +ен нÑĭÑħ +Ġspoil ers +Ġgall ons +ĠÙĦ ÙĬ +ĠZ ion +æľī ä¸Ģ +on ie +rag t +ĠCh and +Ġë³ ij +Ġbl unt +Ġus u +ĠK ad +ra kt +Ġcin ematic +Ġam munition +re ne +Ġfour teen +ĠC arn +c rit +Ġten ure +v u +Ġprincipal mente +Ġalle en +éĢĻ ä¸Ģ +Ġkompl ett +Ġdü ny +J ames +Ġrecept or +Ġones elf +g uru +Ġmerch ant +l iness +Ġover looked +Ġharmon ic +éķ ¿ +ies o +×ķ× ŀ +col m +ĠпÑĢо екÑĤ +ĠAd a +ا س +T im +Ġrecur ring +Ġproceed s +ĠPart icularly +ĠDown load +et rical +Ġmat rices +Ġproyect o +anc ies +ĠUh m +Ġc aves +Ġìĸ´ë ł¤ +ĠLe af +Ġоб ÑĭÑĩ +ĠìĿ´ì ľł +Euro pe +Ġt Äħ +Ġpul s +Ġtak iego +ÐĿ е +G U +Ġfor s +Ïģ γ +Ġfot os +Ġ) ) +Ġë© ¤ë +Ġaqu ilo +ĠK urd +ï¸ ı +pt ic +ĠD ort +Ġmis ery +aus o +åĬ Ł +chuck ling +ĠR idge +ĠíĸĪ ìĬµëĭĪëĭ¤ +Ġ* ** +å® ¢ +ĠHmm m +Ġge ographic +Ġany s +Ġtal vez +Ġske let +Ġsign atures +Ġlit ers +IJë ©´ +ĠÑģво его +Ġski ing +ĠÐľ оÑģ +Ġadop ting +Ġha ft +Ġsymm etric +ĠL iqu +Ġthy roid +Ġmis in +lud e +Ġh ull +ĠX D +ĠG ust +ze ich +Ġvibr ations +Ġes emp +ĠвÑģ Ñİ +ĠQu em +Ġü brig +ĠS ke +ĠLyn ch +room s +art et +f est +Ġfr üher +Ġl ure +ä¸į好 æĦıæĢĿ +ĠìķĮ ìķĦ +ĠW IN +ĠR YAN +ĠкоÑĤоÑĢ ÑĥÑİ +ĠK ash +Ġ×Ķ× ŀ +Ġsaf eg +ĠHall elujah +Ġдв ÑĥÑħ +Ġstap le +Ġsed iment +ĠAct s +Ġbl aming +Ġmain land +Ġsport ing +Ġdecor ations +Ġexecut ing +Ġpar an +ĠDoll ar +Ġproject ions +Ġcommission ed +Ġb our +ö m +Ġste amed +ĠëŃ ĺ +Ġpet rol +Ġcel ular +å¸ ¶ +ĠHung ary +Ġrent ed +Ġв аÑĢи +bb ie +Ġsé cur +ü ll +Ġsw ings +bet ween +Ġи ÑĤ +est ro +Ġnie mand +ĠìĤ ¼ +ĠP ardon +ess es +ĠM ID +Ġcentral ized +ĠAl ien +cul os +Ġcr ise +裡 éĿ¢ +Ġcl asse +beit et +i ÄŁi +Ġwh ales +Ġper imeter +Ġty ing +Ġstr ony +Ġlike wise +ĠP unch +D a +ĠBapt ist +Ġsort ing +Ġ iv +Ġíķ © +Ġre hab +Ġet a +ri ver +Ġsa i +ãģĦãģŁ ãģł +od us +ãģĬé¡ĺãģĦ ãģĹãģ¾ãģĻ +Ġess ayer +Ġtur tles +ĠHaz rat +Ġfab rics +Ġcav ity +Ġpon ieważ +Ġschle cht +Ġs alsa +ÅŁ ekkür +Ġse ating +Ġeconom ists +Ġman g +Ġsegu inte +Ġr ang +Ġrat ios +Ġconst ell +Ġlong temps +u ating +Ġspo iled +Ġrecip ients +Ġsn iper +ä¹ĭ åīį +ìĬµ ëĭĪê¹Į +Ġw p +ĠLIN KE +Ġfl are +ĠAd ri +ñ as +Ġback l +mä ÃŁ +ĠB end +Ġworkload s +ĠÑģ Ñĥп +Ġ197 5 +им ÑģÑı +ан е +Ġм он +Ġaspir ations +ĠA er +ĠговоÑĢ Ð¸ÑĤÑĮ +ĠQ ian +å¦ Ī +Ġcomprom ised +Ġyol k +ла ÑģÑĤ +Ġhe men +ro ve +d ens +Ġком менÑĤ +Ġ- -- +Ġflu ores +но Ñģ +ĠLiver pool +ĠÑģоб ой +ĠZ we +Ġl umin +ĠO G +á ¸ +hol m +pro fits +S N +Ġproport ions +Ġm ica +ĠB oh +ĠAt las +Ġuns ure +Ġtour ing +Ġn ied +Ġt ÄĻ +Ġimper ative +Ġdem ek +ĠSher iff +r ance +Ġhom eland +ĠH ail +ĠG anz +y mm +M on +åĨ · +v ida +Ġdesar roll +æĬ Ģ +Ġintrig uing +ĠH ugo +Ġ ãĤĤ +é ¬ +а ÑĨ +ĠWiÄĻ c +att ed +ĠìķĦëĭĪ ê³ł +ĠV ari +á d +Ġsur real +Ġdispar ities +Ġm ó +ull en +ĠìŀĪ ëĭ¤ê³ł +Ġп ожалÑĥйÑģÑĤа +Ġma ins +Ġe ject +Ġmeth ane +Ġmarginal ized +Ġchill i +r ès +Ġy em +ä½ł æĺ¯ +ĠCh un +Ġdeb ts +Ġdownload ing +ĠAth ens +is ierung +ry n +Ġte kn +ĠQu indi +éľ Ģ +Ġtara f +Ġh é +Ġconscious ly +Ġfix es +uck le +may ın +Ġfre i +Ġsp a +Ġì§Ħ íĸī +ĠاÙĦØ ° +ĠÑĥ к +let t +Ġolm uÅŁ +Ġche esy +า à¸ģ +na ire +Ġw iden +Ġli en +Ġesca ping +igg s +ĠBl ick +c Äħ +ĠìĦ ľë +Ġ×Ķ× ¡ +Ġв пеÑĢ +oph one +ie ll +ĠSU BSCRI +Ġl ions +Ġê·¸ ê²ĥ +Ġinsp ires +Ġguarante es +Ġcome ça +ĠGrow ing +Ġneg lig +ĠFrank f +Ġge geben +ĠÄij ầu +Ġend lich +Ġì ፠+ĠT T +ĠL ith +ÏĢ Î± +aster n +ĠA zer +Ġlun ar +h ic +Ġна ÑĢод +Ġnen hum +è· ij +ĠSalv ador +ĠPro gress +Ġprivile ges +ĠëıĻ ìķĪ +Ġant agon +ĠImp f +Ġdesc ub +ĠLe i +ĠìĥĪë ¡ľ +Ñĩ е +Ġdó lares +ĠMeg han +ĠW ire +to o +ay ing +us c +Ġt ud +Ġappe als +ed uc +Ġp ane +Ġj i +Ġde cks +ĠAl ter +Ġ å°± +ìĦ ¤ +åĪĨ éIJĺ +Ġproduct ions +ĠWILL IAM +Ġimpl ied +Ġfulfill ment +ĠA ah +Ġsa ja +x us +ĠÎļ αι +Ãł s +uc ch +ок о +ĠDisc ord +ĠS Y +j sk +ĠWall ace +un ction +Dan iel +Ġk öt +ij ah +Ġmarch e +Ġdis gr +Ġm ungkin +Ġal ma +³ µ +Ġextensive ly +ĠFl oren +ĠAll ison +ãĤ ± +ÙĬ Ùħ +Ġju ven +ĠRena issance +Ġfundra ising +ĠCha os +Ġpar aly +Ġnarr ator +Ġecosystem s +A sh +Ġmitig ation +ĠA ujourd +ĠIde e +! , +Ġ ½ +Ġland lord +Ġdefect s +Ġac re +uls ive +Ġalg ae +pe k +Ġem ba +ĠR oc +éĽ ¢ +ks om +ä che +Ġle uk +Ġlever aging +Ġê·¸ëłĩ ì§Ģ +ĠPal m +Ġä ven +Ġl is +ĠIn sp +ĠR ita +ĠAb b +ith m +Ġsuper vision +Ġrevis it +Ġpi ÄĻ +Ġeu h +Ġf ades +Ġmot to +åį ¡ +ез ж +ĠSh im +Ġrelev ance +Ġo o +Ġo stat +n ica +Ġcho ix +ĠFac ulty +Ġì¤ij ìĹIJ +ĠAb ove +Ġнеб олÑĮÑĪ +Ġsequ encing +Ġnutri ent +Ġconqu ered +Ġdigest ive +Ġback drop +ĠL ori +ail able +G ame +Ġneglect ed +om orph +ill ah +Ġkn e +Ġsi itä +Ġworks pace +ĠVen ice +ĠK ne +Ñī о +ħ Ģ +ĠH ass +Ġv ita +Ŀ¼ë ©´ +Ġlay s +ên cias +é rica +ĠL l +æ± Ĥ +ĠCo ca +ĠWH Y +èĪ ŀ +Ġrout ing +Ġperm issions +Ġd ings +pre nd +pro gram +Ġcro cod +br al +AAAA AAAA +ag it +ĠN ä +Ġgek ommen +at ten +Ġrefer enced +Ġpair ing +ĠPart ner +ĠCoron avirus +Ñĸ Ñģ +è½ ī +Ġ×Ķ× ĵ +Ġespec ÃŃfic +ars i +qu elle +Ġspont aneous +çĨ ± +Ġê²ĥ ìĿĦ +ĠÐŁÐ¾Ñģ ле +ĠاÙĦ د +ĠSh out +Ġн ал +Ġdisgu ise +ĠJ ord +Ġwe e +Ġmiej sc +Ġser um +Ġplais ir +Ġcred ible +Ġb Ã¥ +ĠA J +ma res +Ġrod s +Ġer an +ãģ¾ ãģĤ +Ġp ää +ĠU A +ĠUn known +ĠÙĦ Ùħ +ĠRab bi +Ġla at +Ġhairst yle +ĠØ º +éģ ĭ +Ġc ach +ĠWr iting +оÑĩ ки +ab ad +Ġstraight en +-- " +w ife +Ġhott est +Ġpun ya +ĠF ashion +gr iff +ĠQ R +ot ch +ĠÐľ ожеÑĤ +Cl oud +ĠStri ke +ĠHe in +Ġ 羣çļĦ +Ġle i +ĠFl ow +weg s +Ġha br +åīĽ åīĽ +nah me +Ì ģ +Ġple asing +op ping +Ġ구ë ıħ +Ġdr an +Ġbang s +Ġ7 9 +Ġsk et +Ġcav al +ĠMac ron +Ġweight ed +Ġm uted +Ġnuest ras +EE P +Ġmath ematic +ĠM RI +ag us +Ġtherap ies +θ ε +Ġun pl +Ġcomm encer +f ull +Ġtow els +Ġpr ue +Ġlic enses +׼ ×ķ׾ +ĠÐŁ оÑĩемÑĥ +Ġpoint less +B ye +Ġelig ibility +Ġscra pe +Ġab usive +ĠM ant +Ġje unes +t al +ĠPrin cip +ĠOrth odox +Ġmel od +ĠмаÑĤ еÑĢи +Ġprosecut or +Ġopio id +ĠÑĥ веÑĢ +ĠBe en +Ġìłij ì¢ħ +Ġd ynasty +Ġajud a +Ġent reg +Ġweigh ed +Ġe ure +ĠB em +Ġab normal +8 2 +ĠJ R +ĠA kt +ĠB ri +ú t +Ġst agn +! * +Ġwe gen +Ġle aking +ĠW ords +ĠM au +Ġv ue +ĠL iam +ани ем +Ġclin icians +ĠP ump +Ġför st +? ... +Ġautom otive +ĠOw en +zus agen +ĠH undred +Ġdecentral ized +Ġbul bs +Ġ×ľ× Ľ +Ġprovin ces +ĠMil an +8 1 +k as +Ġëĵ £ +Ġfor ça +Ġright ly +å³ ¶ +r Äħ +Ġven ues +Ġw ai +Ġpred icting +ĠWi Fi +Ġê¶ģ ê¸Ī +ر ÙĪ +Ġ×Ķ× ĸ +cent ury +Ġgrad ual +ĠProblem e +ĠìĹ ħ +Ġcop ing +ĠBr us +Ġpean uts +irts chaft +Ġз ал +ĠT roy +Ġsper m +ĠM itar +ĠTür kiye +g rand +¦ Ń +Ġ×ŀ× ¡ +Ġp ans +ĠKnow ledge +ber ly +ĠÐķ го +Ġdan ced +ĠFr ost +ĠB urg +Ġbit ing +ìłķ ìĿĦ +me al +Ġhero ic +Ġmother board +ĠL icht +ãģ£ ãģ +ll an +ай н +ĠÑĢ Ñıд +Ġ à¹Ģภ+on en +ir ie +Ar t +r ang +ν η +Ġnew born +Ġam is +Ġا ÙĪØ± +Ġsoph om +ĠCare ful +Ġprospect s +ens en +Ġthr ill +ĠVi á»ĩt +A dam +r ition +ent ric +ud en +Ġcertific ates +Ġas hes +èª ¿ +play ing +Ġs adece +Ġo st +Ġairpl anes +ÑĢ Ð¾Ðº +on er +Ġmagnes ium +Ġgod damn +Ġ197 2 +ĠSch ule +Ġtem at +Ġpart out +௠Ĥ +Ġin ve +ĠScient ists +ĠHud son +win ning +ceks in +Ġcongress ional +or u +Ġro pes +в ед +Ġmad re +Ġf erry +ĠCoh en +ĠP red +Ġvag y +Ġб еÑģп +Ġmult im +Ġdrain age +Ġsim ulator +g iggles +ĠSt adium +об Ñī +Ġnot ices +Ġcraw ling +Ġgr oupe +åı ¸ +Ġkto ÅĽ +ĠY oga +Ġmed ida +ĠÑħ ваÑĤ +ĠL ite +Ġr av +or ama +Ġdisc ord +ĠDI RE +Ġte h +ĠN urs +ç² ī +Ġpitch ed +Ġbark ing +ĠC oke +wi ad +Ġpop ulated +éĻ ¤ +pe lled +Ġб ог +Ġpe wno +ĠC ube +Ġrecru ited +éĢĻ ç¨® +ĠC ara +ıģ ını +im ated +ĠÑĪ ÐºÐ¾Ð» +ic ional +ĠпÑĢо ÑĦ +Ġcontam ination +Ġúlt imos +Ġfear ful +Ġele phants +us i +ĠiT unes +ĠSw ami +ê ¼ +ĠìĦ¤ë ªħ +ĠRich ards +Ġmagn ets +ĠRicht ung +ĠLeg ion +èı ľ +Ġk itty +Ġkiss ed +Ġwater ing +Ġcon o +ĠPalest ine +id ir +Ġma ze +Ġflu ids +ĠProdu cer +ĠKr sna +好 åķ¦ +la f +Ġ×IJ ×ķ +Ġm iesz +ĠX ing +oint ed +se in +ĠF uk +ĠDep ression +ĠD uty +ĠPan ther +Ġsu nd +Ġref ere +Ġexc lusion +Ġnav al +ĠWin ston +Ġsl ogan +Ġhypoth etical +Ġelev ate +ë ł¹ +Ġcabe ça +ĠGes und +m eter +ĠìķĦëĭĪë ©´ +Ġcloud y +â̦ ? +ĠSch ritt +ĠJ S +ì į +ĠSpr ings +ĠB atter +· ° +Ġtail or +ĠPTS D +ĠG ent +Ġba ÄŁ +Ġspat ula +Ġcr ay +ĠLeg isl +Ġs ú +Ġle ve +า ม +Ġer ad +Ġdon g +Ġd erm +ĠBank s +ich o +åħĪ çĶŁ +ĠFr anz +ra vel +éģ Ķ +ол о +Ġfl ute +ĠE k +Ġjoy ful +Ġch ased +ĠLar ge +O ver +Ġentrepreneur ial +Ġcons iders +Ñĥ ем +op a +Ġdorm ir +ĠElement ary +Ġprzy pad +ÑĥÑģ ка +ĠоÑĩ еÑĢ +ug ene +Ġten ido +Ġlug ares +ë ¥ +ĠÑĩ аÑģÑĤ +Ġsa o +Ġbra id +ĠV ere +ĠRe ich +ĠP oss +Ġin an +w and +re f +Ġmont rer +Ġ198 1 +çķ ª +as ında +Ġch rome +ĠTr inity +Ġexplo itation +ĠS ense +ĠC MS +ĠNo ble +ĠìĦł íĥĿ +Ġswe lling +elect ronic +] ? +Ġbr ushing +Ġliquid ity +ĠH ook +ĠCon nor +ĠAl um +Ġgu cken +su ite +Ġwie le +Ġbarrel s +ĠReg el +ĠM ent +ĠT rip +ĠBr ush +ĠE rik +ur ate +ÉĻ r +ĠC yr +ou ble +ĠBe cca +Ġpass words +Å ± +bor g +Ġv endo +ĠCla us +ĠF az +ind est +Ġdece ased +Ġcompar isons +ĠL CD +ĠP ork +Ġevent ual +Ġpat reon +Ġin ability +Ġext inction +Ġì¢ĭìķĦ íķĺëĬĶ +ĠÑģ оÑģ +aj u +Ġ×ij× IJ× +Ġso fort +Ġdest ined +ĠR in +Ġmouth s +ĠNat ürlich +Ġpres erving +Ġlim p +é» ¨ +oc used +ин г +Ġexp osing +ĠÎ ¾ +ë į +la ugh +Ġhis s +ãģł ãģĭãĤī +Ġind ie +Ġdet al +ÑĢав ÑģÑĤв +Ġtr ên +æķ ° +Ġog ni +Ġsimple mente +Ġ197 8 +Ġgo o +Ġ196 7 +Ġgen ug +h ö +Ġhist ó +å® Ł +Ġlob ster +c endo +Ġte il +Ġalle vi +00 00 +OL D +Ġpes os +Ġbon uses +Ġam i +Ġrev ival +ĠHor se +Ġs ack +T alk +Ġmul her +ĠпоÑģÑĤо Ñıн +ĠH ood +H uh +Ġë¶ ģ +Ġhy ung +ĠMe eting +Ġimport a +Ġì°¾ ìķĦ +ĠV ern +Ġstri pped +Ġref uses +Ġqual ifications +op l +Ģë ıĦ +ix ÃŃ +Ġdi ab +it ime +fl ows +Ġin ac +ĠG ong +Ġmeaning less +Ġcourage ous +Ġmicro bi +az y +h ist +Ġvolunte ering +V IE +Ġviol ated +Ġsymp athy +ĠEd it +好 åĥı +elect ric +produ ct +Ġpand emia +Ġgeomet ric +ĠCon vers +g re +Ġgl ut +ist ed +ĠاÙĦ Ùĥ +ĠCh ain +ĠPres ent +ĠY in +ĠÑģ ог +ĠV log +Ġìĸ´ë ¨¸ +Ġdon n +Ġh itch +uck ing +ãģĬ ãģĦ +w ald +ris k +Ġhar i +ĠK ens +ĠId ol +Ġвним ание +Ġtod d +Ġsm ashed +Ġinv ari +Ġкон ÑĤÑĢ +Ġaut istic +ìŀ¥ ëĭĺ +R es +д Ñĭ +ch au +Ġsel v +Ġhät ten +ठ¿ +Ġexpect s +Ïģ η +Ġaç ık +ĠHT TP +le ÅŁ +Ġswe eping +ĠBet a +Ġcounterpart s +ab ile +ĠSim s +C s +Ġrep ar +s qu +Ġprovin cial +Ġshare holders +Ġrun ter +Ġged acht +ĠTe en +Ġgrand s +çĶ ¢ +ag les +Ġrock y +ven s +Ġr ivals +un al +Ġreact s +ë © +Ġmerc ury +ĠLu igi +Ġо г +ĠJ UST +Ġl od +Ġcort ex +w ig +Ġl akh +ì¤ij ìĹIJ +ĠV ic +ĠM und +Ġma pped +ĠD ell +ĠD ruck +Ġlif es +алÑĮ ное +ivid ual +ad ım +Ġat rav +ĠFl ug +ĠKle in +ê±° ìķ¼ +ห à¸Ļ +Ġapp li +ா ? +ü yorum +ĠинÑĤеÑĢеÑģ но +Ġdis infect +> - +Ġchamp agne +Ġk la +op ers +Tr ans +ĠDes ert +Ġcultiv ate +ĠFuck ing +idel ity +ĠÑĤ ан +Ġinc ub +Ġtem u +Ġlearn er +found er +ĠSy l +ãĤ Ģ +Ġf ato +z ier +ĠìĹĨ ìĿ´ +ĠìĪ ¨ +Ġpsych o +ĠÑĤел еÑĦ +Ġregard e +Ġrepresent ations +Ġlit igation +Ġsp ann +ult s +b ior +è¦ĭ ãģ¦ +ä¸į å¤ļ +ĠSur vey +ĠLED s +Ġtr ä +Ġl ên +Ġant ioxid +еÑĢ Ð¾Ð¼ +Ġindu ction +Ġfool ed +ät zlich +ĠговоÑĢ ÑıÑĤ +ĠF act +umb ai +Ġw iggle +NO UN +Ġdévelop p +ĠCl aro +Ġì ¸ +ë ¬ +ãģªãĤĵ ãģł +Ġaccum ulate +Ġmaint ains +ë Ħ +ĠFight er +íĨ ł +Ġmat in +Ġcoup on +Ġst unt +Ġdeb uted +å¾ħ ãģ£ãģ¦ +Ġpra g +ив аем +7 3 +Ġexp res +Ġìĺ¤ë ¹ł +ĠпеÑĢ Ñģон +Ġcalcul us +Ġab rupt +ĠInspect or +our t +æĸ Ļ +ź niej +int ense +B a +Ġl ounge +Ġast hma +ĠHi ç +ª » +Ġeditor ial +Ġse ize +Ġk ır +Ġm ouve +Ġtier ra +Ġtestoster one +Ġr h +ĠKing ston +EL LE +ĠRepresent ative +Ġ197 4 +Ġi ba +T s +Ġsort a +Ġ( ?) +Ġت ÙĪ +ĠëĤ´ë ł¤ +Ġbek ommt +Ġspirit ually +Ġdist orted +M ad +Ġre im +á nh +ĠOtt oman +ĠRel ig +ĠEl s +Ġret ained +ĠLa ughs +æĢ » +ĠS AS +ĠколиÑĩе ÑģÑĤво +×ķת ר +Ġinnov ate +Ġk ork +ĠÑĢаÑģÑģк азÑĭв +ond ere +iv i +ay e +ount y +ĠполÑĥÑĩ аеÑĤÑģÑı +Ġbun s +åħ « +Ġyüz den +Ġsur geries +Ø£ ÙĨ +Ġbankrupt cy +w elt +Ġsi amo +Ġdark est +ĠH ann +gg a +Ġform as +ĠD j +n amed +Ġshield s +ue ller +ĠF ew +Ġl ace +Ġfur ious +ĠY U +Ġsociet al +Ġjudge ment +ĠD os +Ġj ab +law s +Ġrein vent +ĠK atherine +ĠCh oi +ad ows +Ġr ans +od en +ĠMid west +n ın +Ġdep ort +ĠD ip +ç´ ħ +Ġaten ción +ĠCourt ney +ivid ad +ĠÚ© Ûģ +Ġeffic acy +ĠBrook s +Ġrefer ral +Ġкон ÑĨ +Ġmal icious +Ġk ir +ĠGod dess +Ġfun ky +Ġinter im +ĠK örper +Ġìĸ¼ë § +k ur +Ġк ли +Ġtruc s +ges etz +Ġz ug +ĠGl ück +ĠMin ute +Ġprest igious +Ġnie z +Ġconcent rations +ла ÑģÑĤи +ĠS is +ĠVit amin +ko v +ĠP BS +Ġне е +Ġretail ers +Ġcon ventions +ĠSam antha +Ġproud ly +J ordan +ĠJ ASON +at k +Ġtr iste +Ġst är +Ġreiter ate +Ġpos terior +Ġ197 3 +ĠP ine +ĠJul iet +Ġped ir +k il +Ġover lapping +Ġexclud e +Ġecon óm +Ġaccept s +ĠS ter +æ± º +Ġìļ ´ëıĻ +est ab +Ġt ug +ar g +Ġliv ro +Ø§Ø µ +Ġse ams +Ġbur aya +Ġe llo +ĠT M +ĠP aw +ĠInd ex +Ex c +Ġinspir ational +Ġd unk +è° ģ +ak ter +Ġcondition er +ĠSal ut +ÅĤ ec +Ġìī ½ +ĠÑĥз на +ĠRome o +f ruit +ĠY O +Ġchá» ī +б Ñĥ +b ons +Ġreprodu ctive +Ġor ada +Ġíļ ¨ +Ġtent ar +Ġma ñana +ãĤ ¬ +Ġsol vent +Jess ica +ĠLeg al +Ġtu a +Ġs ic +ĠE Q +au kee +ìĭľ ëĭ¤ +ĠÅŀ u +Ġad here +ĠT ul +Ġà® Ĩ +Ġtext books +ĠFif th +Ġexper i +Ġch ic +Ġhe ap +in ely +at ra +T wo +Ġhele maal +Ġf ren +æİ ¨ +Ġbis her +Ø§Ø ´ +ĠìĦł ìĥĿ +ĠT ages +Ġs á»± +Ġbull ied +Ø ¤ +Ġbenef ited +ĠPre viously +ĠÑį ÑĦÑĦ +Ù į +Ġsen ate +ĠM orm +ij ke +ĠF lu +Ġincorpor ating +j ack +Ġп иÑĤ +Ġimp ly +Ġha cks +ĠR ICH +Ġк ваÑĢ +ĠпÑĢек ÑĢаÑģ +Ġdepend ency +Ġìļ © +Ġì± ħ +Ġwäh rend +Ġsu lla +ĠPitts burgh +Ġesemp io +¼ë ¡ľ +pr ot +ĠR osen +ĠIndepend ence +Ġpars ley +ie gen +Ġha w +Ġaqu ell +ĠC AP +ĠÑĢабоÑĤ аÑĤÑĮ +ĠCl iff +ion ar +Ġsec uring +æĪijåĢij çļĦ +ν ε +Ġutil is +Ġcou le +ĠP ing +Ġtre k +Ġf ak +Ġenorm e +Ġìĭ « +è® © +Ġdoub ling +ĠнÑĢав иÑĤÑģÑı +Ġh ed +ho ven +ĠStand ing +Ġm ÃŃn +ĠJ imin +Ġmon arch +Ġco ke +Ġm r +Ġcl ic +à į +Ġimpe achment +Ġdur ability +Ġvar ios +Ġcommercial s +Ġgreet ings +ĠR i +ĠApp reci +ìŀĪ ëĬĶ +Ġrés ult +ér t +Ġsal ute +Ġpoder ia +Ġsun rise +ve ck +Ġreluct ant +Ġcommission er +å¿ µ +â te +ĠKen ny +ĠSir i +ãĥĥ ãĥĹ +ĠëĬ ĺ +ĠE E +Ġun ch +к он +ĠاÙĦØ ¥ +Ġbel ts +Ġhas s +Ġмо Ñı +Ġdispl aced +Ġab ra +ÎŃ Î» +Ġscratch es +Ġcom et +Ġauthor ization +ĠL LC +Ġprodu k +Ġrehabil itation +å ŀ +Ñĸ Ñĩ +ud ing +ol it +Ġ10 5 +Ġexp ands +Ġalt ri +ĠKom ment +Ġan f +P l +ĠM ana +f ed +Ġb ri +Ġor a +G s +ĠG ur +uck land +Ġjun ction +Ġiron ic +ĠFe ed +Ġpra kt +ĠHam mer +Įë ıĦ +ĠTr acy +çµ ± +ĠAs ide +н его +ĠиÑģполÑĮз оваÑĤÑĮ +Ġz aj +Ġequ itable +Ġcur b +Ġãģĵ ãĤĮ +Ġderiv atives +Ġpupp ies +ĠKenn eth +ĠCom pl +ig ram +ĠGar cia +) " +ĠHar bor +est ial +Ġ ä¾Ĩ +Ġ ers +æ ¹ +Ġunw anted +Ġbel ang +аР³Ð¾ +em b +d os +ĠìĻ ľë +ĠBud get +Ġbatt ling +ØŃ ت +k ok +наÑĩ ала +Ġpl ag +Ġcant idad +Ġgrup os +Ġplug ins +ler ini +Ġиме еÑĤ +Ġso zusagen +ol ics +Ġpue blo +Ġrem inis +r än +ĠMor rison +Ġl inha +Ġbreath s +ĠT aste +Ġenf rent +ĠDo cker +Ġд ен +Ġethnic ity +Ġw ob +Ġsuff ers +Ġtransition ing +ĠR ange +ÄĻd zy +Ġк аÑĤ +Ġsy ner +Ġdon ut +Ġprob abilities +ĠO mar +Wh ich +u ish +is in +Ġdem os +ĠìłĢ 기 +Ġëĺij ê°Ļ +Ġед ин +Ġc erve +Ġj oka +I AN +Ġkilomet er +Ġhorizont ally +ĠBh ag +Ġ- > +ĠMon itor +Ġknowledge able +Ġf av +Ġpin ned +Ġe Bay +ick er +Ġìŀłê¹ IJë§Į +ĠXia omi +Ġcap it +Ġn p +Ġ196 5 +ho e +Ġn ok +ĠS age +Ġн елÑĮзÑı +ĠT ow +g am +Ġdic en +ĠSUBSCRI BE +Ġrebo ot +Ġp aj +Ġë³´ìĹ ¬ë +Ġth icken +ĠRe ality +id än +N a +Ġê²ĥ ìĿĢ +!! ) +Ġrout ines +Ġод ного +Ġex ting +Ġì¦ Ŀ +Ġsulf ur +Ġcar ve +Ġastero id +ĠWarri or +Ġphotograph ers +Ġpe ll +Ġcros sover +æĪij çŁ¥éģĵ +Ġhace mos +ĠNe j +Ġsett ling +Ġir m +ĠBook s +ient ôt +Ġesp acio +ĠSchol ars +Ġdo omed +ĠIR S +w ohl +Ġseg ue +ĠëĪĦ ê°Ģ +Ġpr atic +B T +ĠConsider ing +ĠBuff alo +Ġtrain ings +Ġge bru +ĠG leich +Ġpir ates +Ġen velop +Ġre open +im at +Ġte e +Ġsu ed +fe h +Ġ×Ķ× § +Ġdi ets +Ġjunt os +ast o +Ġmisunder stood +Ġru im +Ġclass ify +ĠпÑĢод Ñĥк +Ġin se +Ġillust rated +Ġcorros ion +Ġacc red +ĠAunt ie +ĠпÑĢив еÑĤ +ĠLI VE +Ġre k +Ġrece ipt +åΰ åºķ +ĠBar bie +ĠSn ake +t urn +Je ff +ãģĬ ãģĬ +ķ Ħ +VO ICEOVER +co ll +Ġrun ners +ìł ľë +os os +mo on +Ġkey note +ĠInst it +S PEAK +Ġplug s +Ġcur v +ĠY uri +ĠTh eres +ĠP s +Ġμ ÏĢο +Ġconver ter +Ġref ine +Ġbad ass +Ġο ι +Ġreg en +az zi +ÙĬ Ùģ +Ġse ized +Ġiç er +ile e +Ġup stream +Ġbud s +Ġp im +Ġíķĺë £¨ +Ġall uded +Ġthem ed +Ġconsist ing +Ġb ons +un uz +ĠпÑĢов од +ĠLove ly +ॠĭ +Ġpar ach +ĠSta ats +éļ Ĭ +Ġselect ive +Ġf ase +ĠGeor get +Ġcoc aine +Ġreprodu ction +ĠL ara +ĠL D +Ġg h +J on +Ġl Ã¥ +Ġëij IJë +Ġtyp ed +ĠB ana +ë ĵľë +Ġsav ory +ĠZ omb +stand en +Ġpedest rian +Ġdifférent s +Ġìĭ ¸ +èī ¯ +Ġcompl ained +ç¦ ı +ĠÐļ ÑĤо +Ġ×ľ× ¤ +ali ÅĽmy +Ġmort ar +Ġverd ict +Ġsu ficiente +ĠMill ion +mitt el +in als +ĠاÙĦØ ® +аÑİ ÑģÑĮ +Ġmi ÄĻdzy +ĠO le +Ġin vert +czy Äĩ +озм ожно +star ter +Ġaud itor +ĠSc out +ch ien +ĠSver ige +uff led +Ġze hn +ĠA uckland +Ġarg ent +Ġ197 6 +ĠHo e +Ġboth ers +Ġsocial ist +Ġpl iers +Ġemer gen +ĠX P +еÑĢ Ð¾Ð² +M ore +ĠLe vi +ĠAnd ers +ibil idad +ĠP arents +Ġindu ced +ìĸ´ì ¤ +Ġbal ances +ĠвÑĭ ÑĪ +Ġsubmar ine +St art +Ġdri es +Ġvol ver +Ġtick ing +c ott +Ġf aj +pr és +ĠS abb +Ġза Ñĩ +Ġпок Ñĥп +Ġbapt ized +ĠBrill iant +ĠÐij ог +Ġm ots +b its +Ġlatt ice +æĪij è·Łä½ł +Ġcor iander +Ġresid ency +yn c +Ġpier wszy +ĠKn ock +ĠZ ap +ĠÐķ в +ê² ¬ +å°ı å¿ĥ +Ġune ven +ĠJ as +od or +ç¿ Ĵ +7 4 +ĠS ite +Ġacontece u +ym pt +Ġtril ogy +Ġlan tern +ĠZ ucker +v ari +we lling +ĠPot ato +gom ery +Ġreact ed +ĠChr on +Ġj ede +be eld +Ġtw ent +Ġl act +æ¨ Ĥ +Ġré se +Ġrel ent +Ġfurn ace +Ġwid get +Ġearthqu akes +ĠAd just +il it +ĠØ£ ÙĪ +Ġhear ings +Ġdefend ant +irs iniz +Ġbas k +c ja +ľ ¨ +Ġrif les +Ġinst al +ĠFor give +p ical +ĠÐŀÑĩ енÑĮ +Ġpet ites +Ġh p +Ġren owned +ĠIn n +Ġ주 ìĦ¸ìļĶ +Ġemphas ized +éĹ® é¢ĺ +ĠìŀĪ ì£ł +Ġê²ĥ ìľ¼ë¡ľ +ãĤ Ĩ +Å ĵ +g ili +D ave +Ġexha usting +ÅĤ ug +Ġsch ema +μ ά +cy cl +Ġaut ant +Ġpar cel +Ġmater ia +ĠB erry +ĠÑģ ами +Ġextract ed +ĠSay ing +ism atic +Ġпоп ÑĢоб +Ġneur on +g raph +ľë ©´ +Ġencl osure +ĠJoh ann +Ġafter math +ÑĤ об +Ġu ży +Ġs amp +3 60 +ĠMe i +Ġt aco +Ġrecept ors +Ġpunch es +ĠHo je +ĠÙĩ ÙĨا +=" # +ĠAng ular +Ġmus ique +Ġro l +Ġà ± +ster reich +Ġcl am +ĠTre asury +chem ical +Ġap ar +Ġapp end +Ġforb id +ĠHamb urg +ак ов +Ġê¸ Ī +ild a +Ġprepar ations +Ġmog Äħ +Ġcam ino +E ric +ĠBl ind +èĪ ĩ +å¹´ çļĦ +ĠDis covery +ì¸ ł +çĪ ¶ +Ġinterpre ter +Ġb red +ĠPsal m +Ġdef ended +ìī ¬ +ĠEr fahr +ĠPe ach +Ġmo ons +ĠO st +Ġspé cial +Ġarri ver +ĠW is +u ci +Ġrobot ics +I VE +Ġsie ge +ar la +Ġsepar ates +ĠT C +íı ° +quis ite +Ġparenth eses +ик е +ç« Ļ +Ġtr ous +å» º +ĠÑģ илÑĮ +Ġbe ers +Ġпл аÑĤ +ãģĻãģĶ ãģĦ +Ġso la +Ġd ès +ming ham +ik te +Ġo ops +Ġtw itch +å° ĩ +Ï Ī +ĠShould n +uv re +Ġle er +cript ions +Ġeyes hadow +ĠGu o +ĠPow ell +Ġsup uesto +Ġan a +r als +ĠMont real +Ġsurf ing +ĠÐŁÐµÑĢ Ð² +×ŀ ×ķ +Ġmillise conds +Ġsubur bs +Ġplanet a +ÑĥÑĪ ÐºÐ° +hr lich +ĠH Y +Ġس ÛĴ +ĠM M +ĠE ff +åı¯ æĦĽ +ĠH S +ans on +Ġì§ģ ìłij +Ġsu o +Ġdeploy ing +Ġk unt +ter ing +Ġere ct +ìŀ¥ ìĿ´ +ĠìĿĮ ìĭĿ +Ġspec imen +! ... +æĪij 說 +Ġlig ne +Ġk onst +ade qu +Ġìĥģ íĥľ +Ġaccess ed +ĠP ole +k ill +Ġë² Ħë +Ġauthentic ity +Ġapp elle +ull e +Ġrev ision +Ġgo ats +г ли +Ġp au +ĠR anger +ĠIm ag +aut hor +Ġe ve +ĠMess enger +Ġn ay +Ġwh oles +ät te +Ġon wards +ĠDep ois +Ġíijľ íĺĦ +ĠSAR S +Ġwszystk ich +Ġdest ru +umb ing +Ġcompat ibility +Ġmis information +od ore +ĠF avor +ek o +ı Į +w aukee +ĠTe aching +ĠK O +Ġbet ting +Ġquest s +Ġviv re +ĠмÑĥз Ñĭ +Ġs aga +Ġswe ll +Ġge he +æĢİ麼 樣 +ĠоÑĢг аниз +Ġg ide +ĠG ross +Ġdale j +Ġcl aws +á»Ļ c +Ġprejud ice +Ġins ign +i hood +Ġpl ed +Ġdó nde +ĠPolit ical +Ġprem ises +und ert +ع ت +on nen +Ġespa ço +Ġf é +ĠHarr ison +ĠC ensus +Ġcard io +Ġdi y +Ġmil ieu +Ġjourn ée +ĠRe lease +N IE +ĠM uk +id ée +á»į i +Ġiç inde +ŀ Ļ +Ġreson ate +Ġm oles +ĠF lying +ĠGl oria +ĠPast or +ĠAre na +好 ä¸į好 +N ON +ол ов +Ġall ÃŃ +om at +ìĸ´ë ıĦ +Ġcaracter ÃŃst +Ġdecl ining +Ñĸ Ñı +an co +ĠIn form +Ġbarg ain +Ġbus hes +ĠNat urally +Ġre chts +ĠT ensor +ĠPat ricia +Ġprincip io +ĠM umbai +Ġwom b +Ġnost ra +Ġdile mma +Ġirgendw ann +Ġ196 4 +Ġenerg ÃŃa +Ġна ÑĢ +Ġseg regation +ĠA thlet +Ġ» , +Ġy eni +ĠSe it +Ġven om +Ġdak ika +Ġëı Įë +ĠÃī l +Ġf us +ĠM og +¦½ ëĭĪëĭ¤ +Ġrem ar +ĠTed dy +Ġbreast s +ic ans +æĶ¶ çľĭ +k ap +Ġh Æ¡n +ĠJ P +ãĥ³ ãĤ¿ +Ġresur rect +ĠìĿ ¸ë +her ical +Ġfot ograf +ĠJos é +Ġlivel ihood +Ġbib li +ter i +Ġvor stellen +ĠA AA +Ġassess ing +Y A +Ġspl end +Ġexca v +Ġbapt ism +y ll +w ow +M ac +Ġpl astics +teok bokki +Ġintéress ant +Ġcommand ed +Ġfamous ly +ĠÐĺ ли +ĠMan uel +Ġsouth west +Ġde formation +ÃŃcul o +ĠнаÑħод иÑĤÑģÑı +ĠP atter +d egree +ĠczÄĻ sto +" - +Ġìħ ĭ +Ġman ger +ĠTrust ee +Ģë ¦¬ +Ġpunt os +iv able +Ġvol atile +ĠëĬ IJ +Ġinst ability +Ġc iel +ci Äħ +Ġpur ity +но ÑģÑĤ +S il +ed ar +åĻ ¨ +NOUN CER +Ġspe lled +G ER +Ġsanct uary +Ġacceler ating +Ġsc out +ĠпÑĢ ÐµÐ² +f ahren +ãģĵ ãģ¡ãĤī +ĠëĤĺìĺ ¨ +Ġpocz Äħt +ĠMe u +ka ar +³´ ê³ł +ak ra +D own +ĠÃĦ r +ĠEl ite +Ġall ons +Ġmay onnaise +ĠS ustain +prising ly +Ġsuper vis +Ġê·¸ëłĩ ì£ł +Ġunemploy ed +Ġfresh ly +Ġ×ŀ× ¢ +ĠD h +Ġtack ling +Ġo gr +Ġì´ Īë +ãĤĪ ãĤį +Ġlo ft +ar ah +ĠA irl +ĠD ir +ĠÐľ ожно +Ġbook ing +ĠC RA +Ġhtt ps +Ġcho ke +Ġg own +Ġno ite +Ġz ac +ist ol +Ġsec re +Ġresemb les +Ġcu ad +ìĤ¬ ê°Ģ +sh ow +Ġbl anc +Ġag u +ĠPr int +ast ed +ĠWe ather +i pl +Ġobsc ure +Ġcont e +ough s +) ; +ĠD ame +ä¸Ģ 缴 +Ġclar ification +Ġintim acy +Ġup hold +ĠMir ror +Ġw agon +x ide +Ġcl og +app er +ĠImmedi ately +ú de +Ġtouch down +Ġro oft +аÑĪ Ð° +Ġç ıkt +Ġla isser +ĠUn real +ens itive +Ġ12 3 +Ġpl aster +Ġduck s +Ġet me +Ġb ishop +bre vi +Ġb ic +ä¸ĭ åİ» +Ġrun time +Ġamb itions +м аÑĤ +ĠWe in +ĠMar i +ĠíĬ ¸ë +Ġresol ver +Ġng Ãły +ĠR ise +ãĤĪãģĨ ãģ« +ĠCr us +Ġmerchand ise +Ġel i +Ġstate wide +Ġow l +éģ ł +æĶ ¹ +Ġtwist ing +Ġcontam inated +ĠCom merce +hy thm +ĠÃ Ī +Ġìĭ ¤ë +Ġmus ste +u ir +Ġsum s +ĠSome where +ãĥ İ +Ġk ami +Ġa ired +ĠAND REW +Ġê º +Ġv iendo +Ġantib ody +Ġabsol ument +Ġprotest ers +ĠQué bec +st adt +Sha un +Ġcham bers +ĠWe ar +ĠEffect s +Ġhaz ards +Ġne i +Ġcoraz ón +Ġá ¼ +ĠS G +Ķ © +ĠìĹŃ ìĭľ +Ġcom fy +ĠC ody +Ġpens ando +Ġg anska +ĠAc ross +öll ig +aby te +Ġwed ge +Ġkal ian +Ġsig ue +end es +ĠGro ÃŁ +Ġutil iser +Ġfl own +ани Ñİ +Ġle var +rest rial +Ġillust rations +Ġas lında +BLE EP +Ġдо ÑģÑĤ +Ġtur ret +Ġsuit case +ziÄĻ ki +Ġsket ches +Ġac red +ĠRe i +Ġt sun +ĠS ag +Ġthird s +ĠKIR BY +ra i +Ġhuman os +Ġrecomm ends +Ġextraordin arily +Ġcommence ment +K N +ope z +Ġ×ij× © +Ġlet hal +ĠEst amos +Ġinspect or +ĠSe ok +e un +Ġoff shore +Ġget tin +ye ars +ĠSil ence +ĠNat ur +up un +Ġtr zy +Ġno get +Ġhamb urger +ĠPra ise +é nd +Ġ197 1 +yl ie +k rit +ĠìĥĿê°ģ ìĿ´ +çļ ® +Ġmoment os +Ġest é +Ġdisse min +Ġgig s +Ġdes af +Ġav is +ĠZ oo +ĠìķĬ ìĿĢ +h äng +åı ¥ +h ake +ĠB ism +Ġre think +ĠMal colm +Ġident ifies +l ower +ix el +Ġtv Ã¥ +k ed +ier z +Ġö ffentlich +Ġproc laim +so on +l ol +Ġlo i +Ġb itten +ro llo +Ġser mon +Ġes qu +Ġjack ets +Ġgr áfic +Ġпок азÑĭв +Ġcabe za +ch odzi +Ġpel vis +Ġnost algia +Ġbre w +Ġshort cuts +ĠAd emás +Ġsuperfic ial +åħ© åĢĭ +Ġbo ca +ĠæĪij æĺ¯ +iment os +åĽł 为 +Ġspr outs +é£ Ľ +ĠJon as +ĠFloren ce +st atic +da ughter +* ) +ÅĤ by +f ashion +ĠG inger +Ġë§ ¤ë +Ġhust le +ut os +ĠÑĤ Ñıж +ĠL ös +ש ×Ļ×Ŀ +any ch +tu ber +Ġtid y +Ġfront al +Ġwhis key +Ġhum id +ĠÎ Ł +Ġr idge +Ġmar in +Ġb ientôt +ĠCarr ie +ch w +Ġtah un +ĠEr geb +F R +Ġìłķ ë¶Ģ +ĠSold ier +Ġenlight enment +Ġexam ining +ĠNot re +Ġer am +ĠSun ny +Ġlay ered +ĠD azu +r ades +好 åIJĥ +ĠнаÑĪ ÐµÐ¹ +Ġtim ber +Ġman ners +ĠBir mingham +Ġmini ature +omet ers +Ġfill er +ĠR ip +ĠK omb +own er +ì ¿ +id ian +Ġdem ás +ĠÙĪ Øª +Ġpreca utions +Ġgovern o +z elf +ĠCom plete +å¸ ĥ +ĠPh antom +ãģ¾ ãģļ +Ġн ез +ĠкаÑĢ ÑĤ +ĠAnt wort +ĠPf izer +ĠFran co +Ġw ÅĤ +Ġfr ig +es per +Ġk ale +Ġfilm maker +Ġk urt +Ġinv alid +å± Ģ +are lla +Äĥ ng +ram ento +Ġnutr itional +Ġdict ators +Ġaf in +Ġf uzzy +ĠG ina +ó t +ĠExtrem adura +Ġdemonst rations +ĠMont gomery +íķ´ì Ħ¤ +ĠGand hi +ãĥ Ŀ +ç½ ® +Ġreun ion +Ġjaki ÅĽ +ĠZ ug +OU GH +l ifting +Ġ ಠ+á¹Ľ á¹£ +e b +ĠW OW +ĠSh iva +omet ry +Ġwild ly +Ġt ended +Ġmeg ap +ì² ĺ +Ġna use +Ġg erek +ãĥ ĭ +ĠMar cel +Ġn este +Ø® ر +Ġfe h +åĨ ħ +susp enseful +ĠWrest le +ĠPalestin ians +ĠG ORD +iy et +ĠÑĢ Ð°Ð´Ð¸ +Ġvers uchen +Ġtrans istor +ĠÐŁÑĢ Ð¾ÑģÑĤо +Ġпон ÑĢав +Ġrhy me +ĠVerm ont +pl atz +è® ° +ĠÄ°ÅŁ te +ĠH ag +ĠÐĺ м +ĠÑĢаÑģÑģк аз +Ġmet ros +ĠInfin ity +w olf +ib al +ft ig +Ġ ÚĨ +Ġíĺ¹ ìĭľ +Ġo ggi +Ġdisp osit +ĠпÑĢ Ð¸Ð» +ĠвÑĭ пол +Ġth ôi +ĠK ENN +Ġhand ing +act us +Ġtac os +Ġformer ly +ĠCorinth ians +ãģ« ãģ¯ +ÑĨÑĸ ÑĹ +Ġpad re +Ġcongreg ation +æ ij +fer t +Ġsub ir +ais er +qu a +ara oh +ĠCur ry +ĠìķĬ ëĬĶ +ел Ñİ +Ġf uss +Ġbo oty +Ġl ows +Ġh ommes +ĠM H +ĠDisney land +w ent +Ġresid ue +Ġbe eping +è¼ ķ +ät ta +Ġm ould +ĠPro jekt +st alk +Ġartif act +ĠAnt rag +ĠAM D +ĠCry pt +Ġë© Ķ +ĠFel ipe +ĠCO B +el u +Ġself ies +ĠS anti +ch utz +ĠУ кÑĢаÑĹ +ges amt +Ġflo ck +j az +pl ain +Ġwr inkles +Ġre ais +Ġpal jon +Ġempower ment +Ġattend ees +pp a +Ġn eden +он Ñĭ +Ġtime frame +ĠCher ry +Ġid ée +Ġg ag +Ġdon key +Ġô ng +ĠH are +éļ Ľ +ĠK ara +Ġacom pan +pl aces +im ientos +ĠH amm +б и +ub en +ili yor +Ġth irst +Ġk ry +ĠGeorget own +׳ ×Ķ +Ġor ch +Ġheart beat +Ġtransform ations +est ones +ĠK H +Ġcart oons +Ġan ci +Ġworth less +Ġtail ored +p u +Americ ans +Ġp iles +ĠMon key +Ġbas in +ĠTem per +ĠP aint +Ġpunch ing +Ġba ik +ĠOak land +v re +ÅŁ allah +yd d +Ġcas ually +od u +Ġc oded +ĠNorweg ian +ĠV ince +Ġprem ature +ĠProm ise +ек ÑģÑĤ +Ġdevast ated +ĠPrem ium +ĠPar am +ĠÃĸ yle +um uz +P O +r ators +Ġlamp s +Ġterritor ial +Ġback bone +list ed +D Y +ĠاÙĦ ر +Ġpurs ued +ĠComm ons +Ġê³ ¡ +lo cks +ed or +Ġconce ived +g ere +Ġdisappe aring +ĠS ull +ĠìĹ °ë +Ġho ffe +Ġdet ox +íĶ Į +Ġret ir +ĠëģĿ ëĤ +Ġper gunta +ĠB OY +ç² ¾ +Ġp enn +æĿ¥ äºĨ +h és +h on +Ġcatastroph ic +Ġa ust +Ġtor so +Ġìĸ´ ëĬIJ +ĠìĤ¬ëŀĮë ĵ¤ìĿ´ +Ġmarvel ous +ĠHar ley +ach ine +Ġti ế +itt o +ĠI ÃŃm +yl on +Ġshut down +.' ' +Ġap ologies +ĠCommun ication +ĠговоÑĢ Ñİ +ãģĤ ãĥ¼ +âĦ ¢ +ÃŃ veis +ac un +Ġret aining +Ġcontrad iction +ĠAD AM +C OM +Bry an +ĠM onsieur +Ġadap ting +Ш ÐIJ +ĠSc r +änd ert +Ġpl aus +ä»Ĭ天 çļĦ +Ġon set +Ġassist ants +Ġval ves +Ġsc atter +ĠR ust +aw ia +Ġread iness +Ġp ais +Ġb ible +Ġamb iente +Ġа меÑĢик +Ġunc ond +Ġk alk +åĬ ¨ +Ġmo c +un n +Ġact u +Ġhum ming +iss imo +ĠPat rol +g ow +ãĥ ¤ +ĠTHE Y +ĠBod en +ĠB ie +Ġre el +ĠÑĥÑģл ов +Ġende avor +ĠPer iod +ustom ed +m als +al on +B ox +ĠÏĥ αÏĤ +Ġom dat +Ġal tre +ĠHe h +k ad +Ġprotect or +Ġdomin ance +odynam ic +Ġcommunic ated +k ö +Ġprede cessor +ĠL uk +ĠFl ower +Ġãģ © +po que +ÑĤи ÑĢов +Ġret rospect +Ġdecis ive +Ġexem pel +{ \ +ĠR ück +r ite +ĠZe us +Ġcal orie +Ġattract ions +ĠH inter +Ġuh m +ĠíĮ IJ +Ġrul ers +Ġdiscour aged +Ġaconte cer +Ġacc ents +ĠOpt im +ĠAl g +k ids +20 21 +ĠLind say +Ġfilm makers +pr owad +Ġter ug +ëĭ ´ +ĠSom mer +20 18 +Ġborrow ing +ĠTrans fer +н оп +ari as +Ġhead phone +ì¼ ľ +Ġtransl ating +Ġauf ge +ப à®Ł +we is +av ant +pa id +b aby +Ġtough est +Ġrepe ats +ĠTer esa +L ord +Ġacab ar +ĠR ide +d ir +Ġl eng +Ġd wa +Ġhead aches +Ġn ữa +ĠнаÑģ ÑĤоÑıÑī +Ġbo ils +Ġlong ing +ri as +ó rio +ĠParad ise +ĠSeñ or +erd em +Ġrein st +Ġsal aries +Ġinsec urity +ÅĤo ÅĽci +ĠабÑģолÑİÑĤ но +ink en +ĠEd dy +ud os +Ġd ummy +Ðļ ак +s ix +Ġin box +Ạ© +Pe ople +á»ĵ ng +Ġorganiz ers +f ind +Ġü l +ĠCO M +ż a +we ile +Comment ary +íĬ¸ë ¥¼ +ĠMitt el +k us +èĽ ĭ +ठ¨ +ir al +Ġgar ment +ικ ά +Ġst ool +pay ers +Ġsh immer +ĠO llie +ĠJe żeli +è¿ĺ æľī +Ġ197 7 +Ġje ux +Ġext inct +ĠTransport ation +ĠM aker +Ġj ohn +Ġrich est +Ġtraum at +Ġli egen +´ë ¥¼ +è¿Ļ éĩĮ +Ġun rest +ĠSt raw +æĭľ æĭľ +Ġcom a +ĠKr isten +ĠÐļон еÑĩно +ĠBry ce +ĠÑıк Ñĸ +Ġpearl s +Ġпоним аÑİ +Ġadd itions +Ġas ympt +ĠменÑĮ ÑĪе +Ġsc ans +Ch ild +ĠH ide +к ÑĥÑİ +et as +Ġd ank +Ġple as +Ġess ays +Ġj ets +åħ Ĵ +Ġв ед +Ġposit ives +ho f +- ) +zz o +Ġstar ters +Ġsm iled +Ġ194 4 +qu iera +Ġro k +Ġpu esto +N ico +Ġsim ulations +Ġ à¶ +Ġintrig ued +ĠOver watch +åĸ Ĥ +s igh +b ai +Ġë§IJ ê³ł +id é +Ġcra bs +áºŃ p +ĠIraq i +ìĿ´ë ¥¼ +ÑĤ Ñı +ĠSoph ia +ĠDN S +Ġönem li +ĠLu o +Ŀ ¤ +ĠCoun sel +l igen +анÑĮ ÑĪе +Ġtrump et +Ġd apat +ĠJ M +ĠEVER Y +Ġå°į ä¸įå°į +å¤ ¢ +ĠL ayer +Ġc ô +н ал +ĠJ oo +ĠH ack +Ġs unt +ĠLeon ard +ĠFire base +äng er +Ġexpl oding +v oy +Ġì¦ IJ +ĠÑģ еÑĢÑĮ +Ġsever ity +Ġbest imm +çµIJ æŀľ +Ġt iring +Ġprocure ment +Ġdiplom acy +Ġdecor ative +ĠÙĬ ا +Ġpenet ration +Õ « +Ġout right +EN E +ĠUn i +od les +Ġz eros +Ġdelight ful +j m +Ġdo po +没 äºĭ +Ġposit ivity +ĠVIS TA +ĠRes ource +íĥ Ģë +ÑĪ Ð¸Ðµ +C arl +Ġpip ing +Ġchop ping +ĠGan ze +ü ss +ĠA o +Ġsh attered +ĠDet ective +Ġund oubtedly +Ġhall uc +Ġen ch +Ñĭ Ñĩно +ÑĥлÑı ÑĢ +is esti +Ġped als +Ġdur um +¤í Ķ +la imer +Ġprop re +C u +Ġtransl ator +Ġca ÅĤ +Ġê·¸ 걸 +Ġca ÅĤy +U A +Ġrev ised +Ġпод об +ĠArt icle +ĠHait i +Ġà ĵ +ĠC trl +Ġroz m +la it +Ġletz te +is pering +dis play +Ġalumin ium +Ġpalab ras +Ġconoc er +Ġz itten +Ġdir ig +åıª æľī +Ġbrain storm +Ġw ifi +ĠPart icip +Ġview point +ĠQu an +Ġhier arch +W elcome +å¯ ¾ +Ġoff en +ĠRe covery +gan o +W ould +Ġrep ro +Ġper ceptions +Ġdem asi +ĠBangl adesh +ĠIncred ible +Ġlet zt +Ġbehav ing +Ġaston ishing +Ġâ Ĩ +ĠëĤ¨ ìŀIJ +èµ° äºĨ +ãĥ Ķ +ĠGORD ON +C AR +? !" +ĠP rest +Ġë§ŀ ìķĦìļĶ +Ġt and +Ġl ash +ç Ĭ +ific ant +Ġint oler +Ġг еÑĢо +Ġte u +as o +ĠÑģов еÑĤ +Ġtravel ers +ĠSy nd +ĠвеÑĢ Ñģ +F onda +ad ı +Ġtrans cription +Ġtit anium +Ġtw ists +Ġgear box +ens ation +f at +C oll +ĠCommon wealth +z on +ĠPolize i +ĠAPP LAUSE +f ry +ĠJud a +este em +Ġso ck +ĠJug end +Ġк ÑģÑĤаÑĤи +ĠD ro +Ġproch aine +ãĥ¼ ãĥ« +Ġli ksom +ĠEner gie +ĠMar ina +Ġ2 30 +Ġê°Ģ ìĦľ +ump ing +Ġl one +ç´ ļ +Ġfont s +Ġbusiness man +Ġp ly +Ġdo e +gr id +ĠMil waukee +ĠE den +! ". +ĠÛĮ Ûģ +og ens +Ġteas er +Ġqui én +Ġincent iv +go vern +Ġchild care +Ġsneak ers +Ġimprison ed + ® +иÑĤ еÑģÑĮ +an bul +Ġreg ain +Ġtranqu il +Red ner +éĽ ¨ +IF A +Ġide ological +Ġmayor ÃŃa +Ġb ureau +et erm +ĠD ID +ìĬ · +Ġw aving +Ġbe b +Ġá r +Ġк в +Ġenv oy +an ut +ик Ñĥ +ĠEnviron ment +ĠAss ass +ãĤĵ ãģ§ +ĠB read +ĠТ ÑĥÑĤ +Ġstair case +ĠDise ase +Ġauc un +Ġëĭ Ī +Ġconfront ation +Ġ194 1 +Ġiron y +Ġwor sh +ãĤĮ ãĤĭ +Ġf ick +ĠNa omi +Ġback side +ie ux +K ap +Ġved ere +Ġlength y +Ġbreak er +ĠRoll e +Ġpred ator +Ġnoss os +Ġadvert ise +è³ ĩ +ÑĢод е +Redner wechsel +re ten +Ġcollect ors +ıģ ımız +Ġtr ig +Ġax es +in ters +Ġpen alties +ĠOs man +ĠJen na +Ġfl akes +Ġtrain ers +Ġstun ned +ĠSc roll +ĠP ip +Ġна ÑģÑĤ +Ġnh Ãł +ĠSm ack +ẫ n +rat os +ĠÑĢабоÑĤ Ñĭ +Ġu cz +ĠLem on +ĠS ind +Ġpsych ic +ĠAb g +Ġmamm als +Ġimmers ive +Ġb ots +Ġverschied ene +Ġg eral +Ġfoll ower +Ġ ä»ĸ +Ġsegur idad +Ġimmers ed +fe ito +c ross +Ġö ld +íĥ Ħ +Ġãģĵ ãģ® +Ġ×Ķ ×Ļ×IJ +ĠJ ian +Ġbili yor +are a +Ġk af +Ġgod t +缸 ä¿¡ +Ġë°© ìĨ¡ +Ġdet riment +æ¥ ļ +Ñĸ л +ĠÄij âu +Ġchlor ide +ø re +le i +Ġmont e +Ġdifférent es +à¯ģ . +Ġcareg ivers +Ġin adequ +Ġfare well +ĠÑĤип а +ont ec +ĠE ph +HH H +ĠTod os +ĠС ШÐIJ +Ġtro v +Ġl ige +Ġc ông +ĠC iv +Ġcap az +ĠV allahi +Ġquest e +Ġrepl ica +س ب +z na +ĠÑģл Ñĥж +ĠP T +w ave +ien i +Ġrel ied +de velop +Ġdem e +ĠA man +Ġ[ ...] +Ġcompl iments +u ais +ĠíĮ ¨ +Ġsmell ing +Ġdad urch +ÙĪ Øª +Ġor anges +Ġл ай +Ġstabil ization +åĢ į +ãĤĮ ãģŁ +æ¥ ½ +Ġappl iances +Ġh m +ĥ IJë©´ +odynam ics +Ġc iÄĻ +ĠC ott +M ON +ĠM ang +æĶ¯ æĮģ +Ġall erdings +ικ ή +sh ots +Ġt s +ĠG ör +ĠCH AR +Ġ: ( +Ġwr ath +Ġf ique +Ġfüh ren +Ġtest ament +Ġ^ ^ +á¹Ľá¹£ á¹ĩa +AL D +Ġtext o +ĠDog s +Ġs ib +Ġpath etic +ock s +Ġrad ically +ĠM ORE +ĠJAM ES +Ġing l +ĠTechn ical +Ġpor ch +ĠU T +ĠобÑıз аÑĤелÑĮно +Ġrenew al +Ġaesthet ics +ik um +Ġbe verage +der n +Ġpredict ive +Ġch uy +ĠRegard ing +ĠFor ward +ĠÙĪ ÙĦ +Ġcontext ual +Ġdwar f +Ġpre he +Ġgovern ed +ħ Ħ +Ġtrabal har +Ġnegó cio +ĠболÑĮÑĪ Ð¾Ð¹ +еÑĩ аÑĤ +Ġд ÑĥÑħ +Ġflood s +Ġbow ling +ĠO B +ĠH är +Ġgrad ing +주 ëĬĶ +Ġg ars +d ling +Ġr ak +ë Ī +c reat +ĠÑī е +Ġneighb ours +f ood +Qu ery +Ġhero in +ice ps +ĠK inda +N ET +Ġmar i +Ġim itate +Ġach ter +Ġsettle ments +ra re +cc iones +Ġë ĵľ +Ġf ik +it ung +Ġм акÑģим +Ġel f +Ġd alla +ĠPol sce +ĠP ul +Ч ÑĤо +ĠMor gen +ØŃ Ùħ +Ġsuprem acy +Ġk ys +ĠHur ricane +ĠG TA +ĠFe h +Ġfinal mente +m und +ĠK rie +é poque +ĠT ucker +IT T +Ġl ur +Ġdi pping +ä v +Ġeer ste +ĠFl int +bild ung +ู à¹ī +Ġto im +Ġpr acy +Ġtransform s +Ġspeed ing +Ġpresent er +Ġfellow s +f illed +ie za +Ġadv ising +ĠInter view +и гÑĢ +we hr +ĠD ante +pt ure +Īë¬ ¸ +¯ ¸ë +IJ IJ +ĠCoun ter +Ġcr ist +Ġì§ ľ +Ġje une +ĠÑģÑĤ ÑĢаÑĪ +Ġmie Äĩ +Ġtut or +Ġmas ala +Ġpowder ed +Ġn au +ĠFreder ick +Ġbill ing +ĠE isen +Ġд обÑĢ +Ġm est +æ ½ +Ġsn ipp +Ġmon o +ĠA lo +ĠMer cy +éri ence +Ġcasual ties +ĠAN NOUNCER +ä» İ +Ġto car +Ġbacter ial +H o +Ġstre ak +ĠJ ENN +Ġpl ast +Ñģ лед +Ġre app +Ġpay check +Ġmin ers +hab t +ĠJ ap +н ÑĥÑĤ +Ġred emption +Ġqu ir +hn lich +Ġaccum ulation +Ġsh ove +Ġadrenal ine +M ake +ĠH ern +oss ing +ĠV il +ub by +her tz +bre aks +Ġsp ur +ĠD aha +US TIN +Ġcontinu er +ĠSa ul +ãģ® ãģ¯ +Ġíı Ń +ĠëIJĺë ©´ +Ġë§IJìĶ Ģ +Ġо ж +Ġsuspect s +Ġla quelle +ĠMuch as +Ġv öllig +ul en +Ġimp res +Ġlo bb +ene e +Ġн аж +T a +Ġréal ité +ĠRe x +Ġharvest ing +Ġest r +æ ¶ +osp ace +OS S +Ġdisturb ance +ass ic +ĠIs ab +Ġdéc ouv +ĠHamp shire +Ġor nament +Ġlu ôn +ĠU W +Ġj Äħ +éĤ£ ä¹Ī +Ġrespect o +Ġcomun idad +Ġcom igo +ag na +Ġintrins ic +ĠAlum ni +Ġses leri +Ġestim ation +âĢĶ âĢĶ +Ġprodu it +ãĢĤ ãĢį +Ġв ÑĢ +Ġwh irl +Ġac ces +ç u +Ġvari ability +Ġv odka +its u +Ġinternship s +Ġalloc ate +R R +íĽ Ī +Ġinstruction al +t ant +Ġà®ħ த +Ġinv ites +Ġha k +Ġsca res +Ġe clipse +п ов +к олÑĮ +ativ as +Ġstab bed +ĠD OM +ä¸į åΰ +ro ots +ĠPict ure +íĺ ¼ +ĠC HA +ie c +ı ı +han ol +Ġmisunder stand +R ay +Ġroad map +ocument ed +iz ione +ĠOl ive +r ift +Ġ×Ķ× ł +æ¯ į +l est +; ; +ĠE A +éľĢ è¦ģ +од Ñĥ +Ġhob bies +Ġbur ial +ãģ« ãģ¡ãģ¯ +Ð ¤ +le ge +ĠH J +Ġobject ion +Ġãģ Ń +ct ory +Ġincre mental +Ġgym n +Ġepid emi +Ñģ Ñĭл +à ij +Ġadvance ment +Ġpar ch +New s +Ġa yr +л ам +Ġ×ľ× © +Ġdipl oma +ãģ¡ãĤĥ ãĤĵ +Ġrob bed +On ly +Ġinc ur +Ġch anting +Ġíķ´ë ıĦ +Ġrich es +ĠCar men +Ġnost ro +λ ÎŃ +ĠPow der +à¹Ģภ« +ĠìŀĪ ìľ¼ë©´ +Ġgerçek ten +ĠPik achu +ем он +OL L +Ġplanet ary +Ġsl ows +Ġclock wise +al ion +Ġì Į +Ġver n +Ġh omme +Ġend point +Ġinnoc ence +Ġelement os +Ġsophom ore +Ġnot ions +ĠCould n +p ur +Ġz at +Ġobs ess +Ġmotiv o +ĠK ub +ĠDr ug +A nt +ĠPlay ers +ĠHum ans +Ġme lee +ĠWild life +ĠV P +Ġvolcan ic +Ġcom in +ĠGu ang +ĠÏĦι ÏĤ +ĠоÑģоб енно +ĠS ize +L isten +ĠA aa +app ro +Ġbar bar +ĠPark inson +нÑı ÑĤÑĮ +å į° +Ġunderest imate +Ġsubst itution +Ġcosm etic +ä¸ĭ 次 +Ġwill en +Ġbe ide +ann i +Ġcondition ed +ĠDe bbie +Ġis to +ĠEd wards +ìĽĮ ìļĶ +ĠÑĤ ов +Ġab brevi +ĠM ün +ĠPr inc +ĠLi ang +Ġst ink +Ġradio active +ãģĨ ãĤı +Ġac ontec +Ġun con +ĠTur bo +ãģ IJ +Ġkiss es +æĺ¯ ä»Ģ麼 +еÑĤ ÑĢов +Ġfront ier +ĠSp y +ĠBel arus +ĠC BS +á» Ĺ +am oto +íķľë į° +ĠÑģÑĤ ÑĢо +ĠEn fin +Ġbread th +éĺ ² +ĠCa fe +ĠDaf ür +ĠB our +ar as +Ġbl ueprint +an ı +Ġconst ants +Ġattack er +ĠForm ula +za Äĩ +Ġs owie +Ġeyebr ow +ob ook +Ġset zen +第 ä¸ī +ons ider +aw ning +Ġsöyle ye +Ġinv aded +Ġpronoun s +Ġdob ry +S i +ĠÐ¥ оÑĤ +Ġvolley ball +Ġl ament +is ches +ar me +ap i +ĠW iki +ли ÑĪ +Ġkas ih +Ġp ess +ĠÑĦ оÑĤ +ĠS ul +å¾ · +Ġpse udo +Ġmem o +ĠìŰ ìĬµ +ĠдоллаÑĢ Ð¾Ð² +ĠпеÑĢ ÐµÐ¼ +ĠRe ach +mir al +alt ed +Ġstat ut +read ing +Ġsöy led +ĠLind sey +ĠAh mad +ë ¶Ģë +ĠС егоднÑı +Ġprzy got +Ġhy ster +U RE +ĠNe igh +Rep orter +ĠB unu +ĠTreat y +ĠR ank +ĠF ame +in ished +Ġge ared +Ġcomp ose +od ia +ĠL on +Ġjeste ÅĽmy +ĠDIRE CTOR +Ġel kaar +ĠV iel +×IJ× © +ynth ia +ä¸ ¦ +Ġm ère +ĠTom ato +Ġex atamente +ni ÄĻ +ĠFre i +ĠD if +Ġopen ings +Ġgraph ical +ĠÑĥд об +ĠвÑģ п +ĠWeek ly +ев а +Ġhang s +Ġuns afe +Ġem blem +ĠKolleg innen +al ay +Ġk si +Ġh ides +Ġol may +Ġent ste +Ġarth ritis +ÃŁ erdem +Ġbin nen +Ġlist ens +ĠH ess +åĨį ä¾Ĩ +ĠLou ise +ld en +ен Ñģ +ĠVers ion +ĠAgric ulture +ìĬ¤ë ¥¼ +м ан +ë Ħ¤ìļĶ +Ġw ines +ĠIN F +r ul +ĠJ K +ıyor lar +sh ield +reat h +Ġter us +ĠL um +Ġanticip ation +Ġacc ustomed +ĠM ina +Ġw ield +io è +mer a +Ġcount down +Ġcl ing +Ġcomm end +Ġfakt iskt +Ġdef enses +Ġcock pit +Ġком анд +Ġdish was +ĠThan os +Ġkid neys +Ġse he +Ġmicro bes +Ġc uff +ĠвÑĭÑģ ок +ĠSp icy +çŃī çŃī +வ à®° +cul us +or c +ç¾ ħ +ix es +ĠC redit +Ġr aj +Ġbring t +ĠN iss +Ġgr im +ĠS OL +Ġten im +ĠSud an +ĠSp art +Ġpromot es +ĠN ossa +ĠÑģоÑģÑĤо Ñıни +Ġì° © +Ġunc ont +ĠLiber al +ĠТ олÑĮко +ĠV iele +Ġktóre j +Ġ* *** +M ax +ĠЧ ÑĤобÑĭ +3 50 +Ġíĺ¼ ìŀIJ +Ġë¶Ħë ĵ¤ìĿ´ +Ġwar p +Ġteng a +Ġsympath etic +Ġbiz i +ĠZ ack +ied o +Ġëī ´ì +p iel +ĠÑĤ ол +Ġsc aled +ĠPET ER +ĠCO MM +ĠC ame +Ġcatast rophe +Ġsweat y +ig ration +Ġstuff ing +ĠÏĢολ Ïį +ĠDri ver +zy st +T ech +Ġassess ed +ĠSur face +ır ım +s ur +ler weile +Ġд ог +Ġshut ting +Ġfr actions +ĠÑģ ол +every one +Ġer n +ĠÐĿ ов +Ġdefend ers +Ġvers ucht +ãĥ³ãĥ Ģ +Ġpol ity +ĠÐŁ он +ver ständ +Ġbrows ers +Ġtransform ative +Ġdict ate +ĠLE GO +Ġning una +ê´ ij +Ġp izz +ĠHar old +ĠL opez +Ú¾ ÛĮ +an ız +atch et +ÙĬ ت +Ġl ernen +Ġê·Ģ ìŬ +Ġhous ed +Ġclean se +ĠW AT +lar ation +Ġby tes +Ġtuck ed +Ġfault s +д о +F X +Ġìĸ¼ë§ ĪëĤĺ +Ġde form +Ġcontract ing +ĠTIM E +ir se +Ġne ben +Ġc erc +ĠArm strong +Ġtest er +Ġparf ait +Ġjealous y +Ġtox ins +Ġdis bel +ÑĥÑĢ Ñĭ +imp ression +Ġprost ate +Ġfire wall +Ġclass ics +еÑĩ ÑĮ +Ġsocial ism +Ġgrac ious +ĠÑģ нова +Ġд нÑı +Ġburn er +ĠMin or +Ġìļ°ë ¦¬ë +Ġjed es +Ġcontinu um +Ġh ots +Ġoccur rence +Ġadminister ed +Ġзам еÑĤ +Ġhes itation +Ġdr ills +er ca +ĠвÑĤоÑĢ Ð¾Ð¹ +Ġstead ily +Ġinsan lar +Ġi han +í ij +Ġhel per +ĠSen in +åģ ľ +ов ание +ĠER IC +b la +ĠAcad emic +Ġhuman ities +bl ack +ump y +ort ex +Ġìł Īë +ĠØ¥ ÙĨ +Ġdiscl ose +ĠEl ijah +Ġλ ÎŃ +ĠQu er +ب ÙĦ +ãĤ ¡ +T ell +ar le +Ñĸ ÑĢ +Ġaug mented +Ġë¹Ħ ìĬ· +Ġand roid +ठ¤ +ar ma +Ġs zer +ge ord +Ġge ek +Ġye ux +Ġp ong +ĠãģĿ ãģĨ +Ġtort ured +ĠB ath +z ig +ason able +Ġn ets +Ġbar u +ĠFl at +ĠV ater +ĠTer ror +ĠA vo +Ġceremon ies +ro e +Ùģ Ø³ +O ps +Ġhy vin +Ġap resent +ol or +ĠигÑĢ Ñĭ +ort on +Ġê·¸ëŀ ¬ +Ġlook in +ĠT Y +ĠM int +Ad d +Ġm ite +ĠSm oke +Ġnot a +Ġm oss +ĠAb end +Ġì» ¨ +Ġexagger ated +f ires +Ġred ist +ff iti +Ġopen ness +ê°IJ ìĿ´ +ende u +ен ной +W atch +Ġav atar +ĠP ey +ur un +Ġsen za +Ġì§Ģ ìĹŃ +ĠNat omiast +Ġemer gence +ray s +Ġcraft ed +g ary +ãģł ãģij +ü ng +- " +Ġhack ed +Ġstr ay +en cie +em o +Ġcom en +ĠK ız +ĠJ asmine +ĠH indi +man as +Ġinfin itely +em on +ìĿ¸ëį° ìļĶ +j ak +Ġro aring +éri que +s weise +ĠRo lex +åł± å°İ +ĠStu art +bn b +Ġdiagn ose +Ġcoher ent +ĠM J +æºĸ åĤĻ +Ġp ike +l av +Ġorchest ral +а ÑģÑĤи +Ġterm inar +Ġgather ings +Ġcompl iant +Ġupgrad ing +Ġregul ator +Ġlan ç +éĢ £ +Ġmerch ants +ta wa +Ġmonit ored +Ġrend re +ä¸ ¤ +Ġunter wegs +ang uard +g ard +ĠBel ow +du ino +ĠЦ е +Ġimped ance +ìľ ¡ +ä» ½ +Ġakt uell +ĠV atic +åŃ © +Ġste wards +Ġbright est +Ġk enn +Ġk au +ĠMat rix +ĠB ark +ĠðŁ ij +Ġt aper +Ġcas ino +ר ×Ķ +ys ical +Ġbuild ers +ĠczÅĤ owie +ĠNep al +Ġ! " +Ġterm e +Ġin nych +Ġmath s +Ġdraft ed +ĠB alk +Ġhesit ant +Ġvolt ar +Ġrev ive +ĠÑĦилÑĮ ма +Ġassass in +ĠS olutions +Ġdu el +Ġbear ings +à¸Ħ ะ +Ġrook ie +ik at +Ġbisc uits +Ġc ords +Ñĥв аÑĤи +AR IN +Ġprogress ing +ĠG ir +Ġpenet rate +ĠSt orage +e ight +ĠÑĤ ÑĢÑĥ +Ġdon ÃŃt +Ġsiz in +Ġout dated +ĠнаÑĪ Ð¸ +Ġaff ir +Ġspo ons +Ġon i +Ġfl ank +ĠG ol +h ã +Ġp éri +Ġhonor able +ĠBreat he +sc enes +Ġob viamente +ик Ñģ +Ġש ×ŀ× +Ġsmooth ie +ŀ Īë +Ġd ime +ĠíĸĪ ìĸ´ìļĶ +Ġapp el +ĠCath olics +Ġsing les +Ġlat en +Ġç ünkü +ĠV ader +æı Ľ +Ġvard ı +ĠIst anbul +gr é +ĠEl sa +ë l +Ġinve ce +Ġcr ane +Ġo be +ĠSh ark +Ġsm ack +Ġrest oring +. \ +Ġë¹ łë +Ġf aded +um bers +S inging +Ġdep ressing +th est +ĠW ahr +Ġmult itude +ÑĢавÑģÑĤв ÑĥйÑĤе +rij k +ek a +Ġcomplet es +ĠWell s +Ġro y +ĠPr ay +ĠKal au +iz in +iaÅĤ em +Ġlo com +ĠNash ville +ĠPent agon +ë ¯¸ +ĠNE W +Äħ Äĩ +ÃŃ ss +Ġmarry ing +Ġfe ud +íĻ ķ +æĢ ¥ +) ! +ĠOper ations +Ñĥ ÑĶ +Ġmo je +Ġinstruct ed +ĠëĪĦ 구 +Ġ×Ķ× Ĵ +ĠпомоÑī ÑĮÑİ +Ġsab ia +ìķĺ ìĸ´ìļĶ +pl ane +p ri +Ġпол ноÑģÑĤÑĮÑİ +ĠK itty +Ġpróp rio +ed ere +Ġinteres ante +Ġд е +Ġcond ensed +Ġav ent +T OR +Ġgre asy +AR K +ort a +A J +Ġdis reg +Ġcorrect ions +Ġst ero +Ġinfluen za +Ġdess es +Ġball ots +Ġme get +Ġma fia +Ġb öl +n ost +ĠÑģÑĤ аÑĤÑĮ +Ġrespond er +Ġhint en +g rav +à¸Ń ะ +yn chron +Ġvi ens +Ġsam o +Ġd t +pan nt +ĠÅĽwi at +Ġзап иÑģ +Ġmer ged +Ġke p +Ġmis leading +Ġdig amos +Ġam mon +è¾ Ľ +ch et +Ġê°Ģ ìł¸ +Ġun i +ĠëIJĺ ëĬĶëį° +Ġнап ÑĢав +ĠкоÑĤоÑĢ Ð¾Ð³Ð¾ +Ġanim ate +×ķ× IJ× +еÑĢ Ð² +Ġmin ced +Ġka um +ãģĤ ãģģ +ÏĢ Îµ +л ег +exist ing +Ġplata form +ĠK RIS +ìĽ ł +ĠFamil ien +ĠLib ya +Ġbiod iversity +Ġidi ots +ird i +Ġszy b +ĠRoll ing +ü cht +ĠÑĥд ив +Ñģ Ñĥд +Ġreal izar +Ġcan ned +ĠÑĢ Ð°Ð½ +Ġmet abolic +ĠBe ef +Ġkil ka +лÑİ Ñģ +Ġreg istry +моÑĤÑĢ Ð¸ÑĤе +Ġviel ä +Ġod c +Ġcondem ned +æ© ĭ +f al +ĠD il +wo ÅĽci +A w +Ġstatist ically +Ġso gen +ĠB ETH +Ġsh aving +å¹ ¸ +oc al +ĠFun ny +Ġpeace fully +Ġaddict ive +ĠIns ert +la uf +Ġexperien cia +é¦ĸ åħĪ +иÑĤ елÑı +ÃŃ gen +ág ina +Ġabdom en +íķľ ëĭ¤ +ic us +im ana +ì ፠+arch ing +Ġkonk ret +ìķ ĺë +ек а +ou fl +ive l +Ġn ude +èt res +Ġm onsieur +Ġcl ash +Ġtherap ists +Ġcub ed +Ġretrou ver +Ġwave form +Ġpot em +ĠForm er +is ión +åº ľ +Ġ×IJ× Ŀ +und os +ĠMein ung +ص ÙĦ +ĠJ ude +Ġn Ã¥r +ĠLeon ardo +ĠCr isto +ĠG OT +ÑģÑĤÑĢÑĥ к +L AN +Ġg Ã¥ng +Ġdé b +ĠFrankf urt +Ġcra ppy +Ġli l +ann ée +ĠмеÑģÑĤ е +RE T +ĠN er +ĠCO STA +Ġjed em +Ġcurt ains +Ġiter ations +Ġun av +Ġpla que +or um +ĠÎ ¶ +Ġnúmer os +Ġdes ap +² ½ +Ġcomp iled +Ġref le +Ġrank ings +Ġrep aired +ĠÐĿап ÑĢ +Ġdownload s +Ġarm our +Ġ×Ļ ×ķתר +Ġlonge vity +ĠTON ER +ĠкомменÑĤ аÑĢ +Ġcz ego +Ġnot ify +Ġairport s +Ġend uring +let te +Ġapp arat +Ġhab il +á»ĩ c +n ad +IC O +ĠBra h +Ġseg ún +Ġgovern ors +k aha +ĠSchl uss +Ġodpow ied +ir ting +Ġrem pl +ĠAb original +ident ally +Ġenhan cing +lic ting +ĠHawai ian +Ġstri ving +ĠN iet +Ġzn aczy +Ġobed ience +ĠnÃ¥ got +Ġexp ired +Ġ19 18 +pres ented +Ġpr owad +ĠTer r +ĠPrinc eton +Ġmor gen +Ġattract ing +ĠS igma +ign er +ĠRe chts +ĠP eki +Ġmet hy +Ġha mm +Ġdire ito +Ġdeleg ation +ив аÑİÑĤ +Ġg in +You ng +Ġdepend encies +ĠBrad ley +bud s +Ġf is +Ġpyt anie +Ġinterconnect ed +Ġemba ixo +ĠS as +Ġr uh +ĠS icht +S ur +Ġsuper b +ĠSabb ath +ĠD anger +k ol +Ġh ou +s upp +ĠN acional +Ġsuccess ion +Ġv á +ĠMaÃŁ nahmen +ĠJess ie +ĠId aho +fore st +ħ ĺ +Ġ×ŀ× ĵ +ĠØ£ ÙĬ +Ġsweet heart +Ġneat ly +ĠEv angel +ê³ ¡ +ĠSu ite +úblic a +ĠÑĥ ли +ĠAnn ouncer +l igh +Ġsens ations +Ġshel ters +Ġh art +Ġsqueez ing +ĠR ivers +ĠCook ing +ì± ħ +person al +Ġman os +ÑijÑĤ ÑģÑı +w ij +Ġgo gg +ĠMill i +ĠF P +ün st +ĠL S +Ġspray ing +Ġf aux +Ġaut ograph +olog ic +Ġtor ment +Ġencry pted +á» ħ +Ġest re +ç¹ ¼ +à ± +Ġst umbled +Ġa ider +Ġsab en +x ter +ĠC ities +ĠTür k +ëĭ ¥ +ch ine +Ġto pping +Ġpoison ed +ĠRoman ia +×ĵ ×Ļ +Ģë ¡ľ +ĠпоÑĢ Ñıд +Ġchir ping +ĠìĻ Ħë +×ij× ¢ +Ġcu anto +Ġdon ating +ĠReg ent +ĠBer uf +Ġdistract ing +Ġstam ina +ĠDar ren +Ġì¶ ķ +l ists +d al +ch uss +Ġeconom ist +ãģĪ ãĥ¼ +org t +Ġist iyorum +è¿ Ľ +ĠSur prise +ĠHa o +Ġìµľ ê³ł +ĠG W +ĠIn ner +Ġqu ieren +Ġmind ed +Ġsupercom puter +Ġdiagram s +íĬ ľë +ê²ł ìĸ´ +ĠобÑĬ ÑıÑģ +Ġestab an +Ġdestro ys +ĠBre aking +Ġkar Ä±ÅŁ +Ġrebuild ing +ľë ĮĢ +ли во +ĠSau ce +ĠF usion +×ķ× ŀ× +ĠQu inn +Ġga uche +ĠÙĪ Ø£ +Ġ È +ç ĵľ +Ġtechn o +Ġdisp atch +ĠaÅŁ k +Ġein zel +ĠG mail +ç ŀ +Ġê°ľ ìĿ¸ +ĠÑģем ÑĮ +Ġjour neys +Ġi ht +Ġfib re +Ġdram as +ouch ed +Ġren ame +Ġоп еÑĢ +Ġpo o +ĠD ru +ĠиÑĤ ог +Ġz ast +Ġco z +Ġz ucch +Ġobt aining +Ġcomm ute +Ġsub mer +ĠV ish +ĠR abb +og g +Ġh ut +íĸĪ ìĸ´ +æ¯Ķ å¦Ĥ +ere mi +Ġμ α +Ġdisk ut +Ġб Ñĥк +Ġimp aired +d epend +ĠÙĪ Ø§ +ĠÑĢ Ñĥк +Ġб аÑĢ +Ġoxid ation +Ġsitu ação +ÉĻ n +u ção +Ġsag te +ĠS ER +ĠC ake +Ġtur meric +ĠK ak +b ung +ĠK á¹Ľá¹£á¹ĩa +Ġpoison ing +Ġsl ipping +ĠS ays +å°± åı¯ä»¥ +ò ng +çŁ ³ + « +ĠClaud ia +ĠChar acter +ни ÑĨ +co at +Ġprogress ed +ĠFer gus +Ġìĺ¤ ëĬ +Ġo at +ord able +ĠLe y +ĠHera us +Ġresult ados +ĠKay la +Ġr iff +Ġcheg ou +Ġx i +Ġsp acious +Ġrecogn ised +Ġe ch +ĠT ie +Ġlaunch er +J im +Ġsupp ression +ĠImp ossible +Ġguit ars +ĠFour ier +иÑĩеÑģ кий +ĠTh erap +ĠK af +cent ered +ĠÑģо оÑĤвеÑĤ +Ġk lim +Ġcarbohyd rates +ign ant +ĠAst ron +Ġem ple +Ġdr astic +ĠмиÑĢ Ðµ +в ин +u w +Ġpret tier +Ġdon uts +ĠAth ena +Ġdiss ert +Ġpl ante +Ġur anium +ìĿ Įë +ar é +Ġrze cz +Ġdisplay ing +æĪ ² +Ġsar c +r ão +Ġtamp oco +Ġphilosoph ers +ĠRe cht +æĵ ļ +Ġcoment arios +y se +Ġìľ ¤ +Ġm ise +ĠG in +Ġн ом +ĠFR OM +l iner +at if +Ġspo ÅĤec +x a +ĠÑĤ ÑĢÑĥд +Ġw ag +기 ìĹIJ +ĠM G +Ġoff spring +ĠUnder standing +åıª æĺ¯ +OR A +Ġwh irring +Ġsur rend +Ġpok er +Ġmon uments +ĠâĻ © +Ġorgan ised +ĠSo zial +ĠF actory +Ñħ а +Ġrese mble +з д +Ġexplos ions +Ġpay roll +Ġom n +ĠJ orge +ι Ïĥ +Ġfract ure +Ġpersec ution +Ġdem ais +E CH +, ) +Ġcri ar +ĠJ OSH +Ġdem ographics +Ġ16 00 +Ġcur rencies +ĠT ips +Ġ éĢĻåĢĭ +ĠRe fer +ĠDan cing +Ġincons istent +Ġde h +Ġimm ens +Ġme ist +Ġimpat ient +Ġbehav es +æĿ ¾ +ĠëĤ´ì ļ© +Ġback story +Ġagree ing +ĠÅ ģ +ih in +Ġtemper atura +ĠBack ground +Ġnut zen +Ġëħ ¹ +ĠM änner +Ġcollabor ations +ĠK os +éģİ åİ» +Ġnight mares +ë ĵ± +ĠQueens land +Ġassoci ates +ĠK ok +Ġfact orial +ĠHy ung +Ġê·¸ ëĭ¤ìĿĮ +Ġfil ho +Ġel ét +Ġíĸī ë³µ +° ± +Ġgef unden +Ġsemic ondu +Ġcounsel ors +ĠU pper +ĠA ub +ick ers +V er +Ġnorth west +ĠMainten ant +ĠL akes +аÑı в +int é +ì° ½ +Ġг аз +Ġgi orn +Ġdigit ally +ĠCirc uit +ì¼ Ģ +ãĤĬ ãģ¾ãģĹãģŁ +Ġcheer ful +ĠPet erson +ĠDan ish +ativ os +Ġli ken +Ġhar bor +али ÑģÑĤ +x e +Ġcur ls +ĠR hod +E nd +ĠE T +Ġacqu aint +ĠKel vin +Ġtr if +ĠA way +ìŀIJ ëĬĶ +v s +Ġp ágina +Ġin let +ĠSant os +Ġìļ° ìĻĢ +Ġyap ıyorsun +th eme +Ġsou ff +Ġinject ed +Ġpó źniej +iver so +amp ed +Ġda her +Ġd agger +ĠлÑİб им +Ġt ummy +Ġenlight ened +c ents +ĠD ah +Ġcu est +ä¾Ĩ 說 +IL Y +Ġ×ij ר +Ġbang ing +ĠEm il +ĠC ler +ĠB order +иж Ñĥ +Ġpresent ers +ĠST UD +co ins +ĠíĻ į +Ġper ks +Ġpar ap +Ġcertain es +ĠL ore +ö st +ĠMAR TIN +Ġb ios +Ġwhere by +ver ts +ĠMir anda +Ġst ip +æ¾ ¤ +and ez +׼ ׾ +uj in +Ġê ¾ +Ġaller gies +pl ate +Ġyap ıl +Ġundert ake +ĠëĤĺ ê°Ģ +P art +Ġkız ım +h guru +ãģĤ ãģ¨ +ĠJohn s +Ġeyel ashes +Ġdra ined +Ġst Ã¥r +ãģĤãĤĬ ãģ¾ãģĻ +ĠJ ade +Ġcal end +fil m +Ġmes a +Ġlud zie +Ġattract s +Ġju ices +Ġк ил +Ġnieu we +Ġmen cion +Ġign ition +Ġbl adder +anda ag +ĠExt ension +íĤ ¨ +fe ed +ĠÙĪ Ùĩ +Ġsp un +Ġt ät +оÑĢ Ð¾ÑĤ +ty ard +ron ics +ĠH uge +Ñĥж д +st ring +Ġun just +Ġpra wn +Ġfrost ing +Ġdisappear ance +ios a +Ġcard i +ĠPri est +Ġcient ÃŃfic +åĵª 裡 +ĠÐĴ аÑģ +Ġë¶Ģ íĥģ +Ġth ieves +Ġphys ique +ĠE ugene +Ġбли з +Ġmon opoly +Ġbi ography +Ġho ÅŁ +Ġt ö +m ac +Ġshock s +ìĦ ¸ë +h it +Ġsn ug +Ġinc l +Ġded ic +Ġult ras +Ġизв еÑģÑĤ +Ġutil ization +ĠÑģовеÑĢÑĪ ÐµÐ½Ð½Ð¾ +Ġserv i +st ag +1 80 +Ġse wer +ĠCh oice +Ġdis charged +ĠJ D +ол еÑĤ +ĠкваÑĢ ÑĤи +Ġteles cop +ĠJe ÅĽli +ĠN ana +c ale +ĠÑĤ он +mm m +äºĨ åIJ§ +Ġge habt +ëĤ ł +æĬ ķ +à¸Ļ à¸Ļ +Ġet her +Ġz en +Ġresearch ed +ĠCzy li +å®Į åħ¨ +work ers +Ġê²½ ì°° +Ġsher iff +all o +Ġtip os +Ġprosec ution +Ġfrog s +Ġf alt +j d +ĠíĮ Ķ +Ġfilter ed +ĠO ft +Ġì į +Ġdis fr +ĠMust ang +Ġwo ah +ĠRE ALLY +Ġмог ли +Ġentr ada +Ġиг ÑĢа +Ġmix es +ĠавÑĤом об +Ð Ļ +Ġsh in +Ġparan ormal +Ġsome place +Ġdish on +eta an +Ġfu erte +Ù ¹ +Ġdo om +ìĪ ľ +Ġexist ential +Ġbu ld +ĠSD K +ĠпÑĢав да +Ġturn over +ĠìĹ¬ê¸° ìĹIJ +Ġठ¹ +Ġmodel ed +Ġbug ün +Ġexperiment ation +Ġmorning s +Ġmed o +Ste vie +Ġplay able +Ġairl ines +g ments +Ġê¸°ë ¶Ħ +ĠT omb +ĠMV P +AUDI ENCE +Ġcheck out +Ġpas st +Ġbe ispiel +ĠLink s +he avy +Ġquestion able +Ġìĵ °ë +Ġs ill +Ġmanip ulated +ĠL oren +Ġìľ ¼ +Ġver ge +á k +I ES +Ġsab ot +ĠCustom er +ale ży +Ġnom inee +ĠG ad +Ġnouve lles +ĠS PE +ist ling +Ġo val +обÑĢ Ð°Ð¶ +if ty +éĩ İ +Ġbez el +y et +Ġfre ight +ĠHan ım +r ÃŃa +Ġz oning +Ġind em +ĠB ü +Ġfemin ism +Ġvo ix +Ġof icial +Ġdi yorum +» IJ +Ġar ose +Ġpar ar +ìĿ¸ ì§Ģ +ĠMart ine +ĠL ect +Ġrest er +Ġdrown ing +u ya +c ida +ĠAri el +Ġ0 2 +Ġ×Ķ ×Ķ +ç´ ł +ĠW ert +Т Ñĭ +Ġwid ow +Ġparch ment +Ġcott age +ĠX L +ĠSl ack +ĠN ES +Ġro be +Ġg imm +Ġcam inho +ĠHar per +Ġcit rus +Ġfirefight ers +Ġdop amine +el ets +Ġdemocr at +ìł ľë¡ľ +Ġplay back +o j +ĠпÑĢ Ð¾Ðº +ĠSull ivan +se mble +ĠW orth +ĠMust afa +า ร +Ġmet s +éĸ Ģ +л оÑģÑĮ +Ġinert ia +Ġuniform s +è¶ ³ +é rio +×ķר ×Ķ +é nt +Ġà® Ĵ +ĠÑģам ÑĭÑħ +Ġvou lais +ĠZ immer +ê² łë +Ġн оÑģ +en cias +Ġrel ación +Ġê± ¸ë +Ġfact ion +Ġg osp +пол ож +n ap +h ak +Ġproceed ings +ĠìĨ Ķ +ìķĦ ëĭĪ +ĠìŀIJ 기 +Ġwer d +Ġso f +Ġsch lim +Ġfl avored +Ġquad ratic +ĠBo ot +Ġpublic ity +ĠCar o +Ġ ?" +ни ÑĨа +man ia +ĠS UR +ĠB UR +l ance +ét ica +Ġzob aczy +Ġtri o +s ama +Ġta ÅŁ +Ġas ymm +ress er +Ġت ع +Ġп еÑģ +Ġbeginning s +lad ım +ĠбÑĭ ÑģÑĤÑĢ +Ġmo o +ĠGene va +Ġ åľ¨ +er us +bor ah +Ġref using +b ull +ĠWait ing +ĠInd ividual +Ġan onym +im ens +Ġmed idas +Ġfragr ant +Ġdirect ement +ĠìķĦ ë§Ī +ur ia +Ġsp herical +Ġab ge +ĠVictor ian +Ġspect acle +ĠRodrig uez +Ġoc up +ĠN är +mark s +ng ulo +ĠLu ci +Ġshout ed +Ġregul ators +ÄŁ ini +Ġdis ent +ĠÑĢÑĭ н +ëĤ ¨ +ĠìĤ ´ë +Ġprobl èmes +ĠF inger +asse mble +Ġpe ar +Ġdro ite +ĠEvery where +t am +оÑĤ ив +в ой +ordin ate +ĠL ak +Ġm Ỽi +ĠTele vision +Ġexpon entially +av as +Ġble v +ĠM T +ä¿ º +Con nell +ĠêµŃ 민 +ĠÑģво им +Ġach a +ĠD ynasty +J in +Ġto re +Ġfl or +Ġмног ие +æ²Ĵ äºĭ +ow an +b ah +Ġì£ Ħ +ĠC ela +Ġìµľ ê·¼ +Ġpermett re +Ġab ras +Ġverste hen +Ġesc ort +ĠThe m +är ke +por ter +Ġkah kaha +Ġhe ct +Ġda u +w ah +ol ve +ĠAg es +s chaft +ĠSt ell +ne lle +ĠEn suite +ĠÐĴÑģ ем +Ġcr éd +ĠP P +l ords +gr unting +Ġcontract ion +G ot +Ġacqu iring +Ġso pr +Ġpoison ous +R NA +Ġan ar +ĠH of +' ) +Ġremark ably +Ġintern acional +ü cke +in qu +Ġdu y +Ġbeast s +ĠL AN +Ġpreced ent +ĠRP M +åij ¨ +Ġsel on +Ġmort e +Ġcomeç ou +Ñı ла +Ġinterpre ting +ĠBur ke +ÑĤ ÑĢа +ĠìĿ´ë ٬ +Ġpess im +ĠN ok +íĮ Ŀ +F emale +Ġìĭ ¤í +Ļ Ģ +Ġstim ulation +Ġsl ick +Ġê°Ģ ëĬĶ +Ġк аз +ĠH BO +Ġpap ier +Ġkön nten +Ñĥб ли +ĠConst ant +SPEAK ING +Ġktó rÄħ +Ġcos metics +ĠT rend +Ġrob bery +Ġt itt +Ġgj ort +Ġdiet ary +ł Į +ĠKir by +ĠпÑĢимеÑĢ Ð½Ð¾ +Ġqual ification +Ġìķ ī +Ġcabin ets +Ġhtt p +ĠEric a +ç¾ © +Ġdisadvant ages +Ġch attering +y z +fe it +Ġgu ild +ĠE TF +ĠDrag ons +ĠH ERE +vent h +ÙĦ اÙħ +Ġmarch é +D am +Ġphot on +Ġest able +M ag +Ġol har +Ġcou pling +ĠHil fe +ĠW izard +Ġм ало +hel p +ĠlÃŃ nea +Ġì « +Ġstand alone +Ġmor ale +Ġzwe ite +ãĤĪãĤį ãģĹãģı +ähr t +Ġd otted +Ġdri pping +ĠFl ag +éĿ Ĵ +ro cket +rate gy +ir im +Ġíķĺë ©´ìĦľ +Ġsogen an +ĠUn o +ĠSch utz +Ġest ilo +ĠS ubs +ĠDais y +ÐĿ еÑĤ +' ... +Ġplat inum +Ġb irl +ĠSo vi +Ġviol ate +Ñĥ еÑĤÑģÑı +r ill +Ġtra z +Ġsn ip +Ġcum pl +à¸Ń à¸ģ +Ġc uk +éħ Ĵ +ĠParl ament +Ġhyper t +Ġpul p +Ġtong ues +at to +Ġbus ca +ih n +ER O +ĠÙĬ ع +Ġvari as +ĠMar ian +Ġbound ed +Ġpitch ing +Ġdefic iency +ĠBless ed +ĠEx erc +uch s +Ġnhư ng +æľ¬ å½ĵ +Ġrap ed +h ales +Ġmal a +p ic +Ġ40 1 +ÅĽ niej +ar ina +ëĵ¤ ìĿĦ +ott i +Ġдол го +Ġtrack er +ĠShel by +Ġvan ished +Ġbak ery +Kap ı +J esus +ĠK R +J O +ħ ¸ +Ġdisc s +ìĦ ¯ +ì§Ģ ë +×Ļ× ¦ +em ary +K endra +Ġy ük +ück t +Ġv az +Ġk up +akt u +ĠÑģп аÑģибо +Ġa ik +Ġnurs ery +Ġendanger ed +êm ement +emat ics +Ġrespond ers +ĠRepresent atives +Ġsculpt ures +ig keiten +Ġde pl +Ġinterpret ations +Ġdead lines +Ġ194 2 +Ã Ĺ +Ġsug ars +em u +l ively +Ġrecre ational +Ġdist ort +Ġunders core +Ġun quote +Ġsaf est +Ġsw ollen +Ġanalys es +Ġcommen cé +å¦ ¹ +and in +ĠÐ¥ оÑĢоÑĪо +Ġdi arr +ãģ¾ ãģģ +zi est +Ġtooth brush +éł» éģĵ +u ations +Ġc ade +Ġbackl ash +h ind +Ġris que +z ess +ĠìĿ´ìķ¼ ê¸° +Ġesper ar +Ġtransl ations +ion ed +gro ans +Ġп ÑĥÑĤ +Ġgen etically +éĢ ł +Ġhapp iest +Ġwer k +ato on +Ġmus i +Ġfun ção +Ġìŀħ ëĭĪëĭ¤ +ĠÑĢ Ð°Ð¹ +Ġbe vor +BL ANK +Ġrepent ance +P ut +Ġpotrze b +Ġsal a +Ġcamp a +W ER +Ġdec ÃŃa +Ġsécur ité +ĠAppreci ate +Ñĩ и +ĠR andom +ë³ Ħ +k ah +Ġmö j +Ġsä ger +Ġ×Ļ ×Ľ×ķ׾ +Ġ19 0 +xt ures +E u +Ġg ä +Ġ×ij× ª +ĠC roat +ap o +P LE +Ġpersist ence +åĬ © +Ġbl ends +Ġtre ffen +ĠSanti ago +yd ia +al do +ĠTensor Flow +ĠD ual +ãĥ ľ +Ġch iff +ìĹ ´ +Ġcontract ed +Ġseg reg +ĠFair y +Ġwis ely +Ġvulner abilities +Ġhand held +Ġgad gets +Ġbo ÅŁ +ĠPop ular +Ġcurv ature +ë ¬¸ +ĠMAR Y +ìĿ´ì Ĭ +Ġform ulation +Ġcel ery +Ġblur ry +ĠT S +ale z +Ġw s +Ġprogram m +ĠSt ack +ĠJ IM +ов али +ı ll +Ġp ère +ĠKan ye +ĠDel aware +Ġãģ ł +Ġda unting +Ġб еÑģ +ĠSt upid +b ig +ffic ial +Ġprecip itation +Ġpl ung +ụ c +bur se +Ġdar le +Ġcri pp +Ġpione er +Ġdis put +Ġse an +ãģĵ ãĤĵãģª +Ġresist or +Ġalle in +ipp les +are l +Ġend ors +z ust +ĠÑĢеб ÑıÑĤа +ed ed +Ġì¹´ë ©Ķë +Ġlle va +Ġken nt +Ġб ал +ĠDoc ument +ĠKn ights +Ġbuck le +Ġìī ¬ +Ġal k +ĠEvery day +atter s +Ġtoil ets +Ġj ugar +ĠìŀĪ ì§Ģ +Ġgen auso +ĠLandes regierung +ãģ£ãģ ± +ij e +Ġtrail ers +ĠT igers +Ġg itti +Ġforg iving +Ġconcur rent +ĠV u +ĠíĬ¹ íŀĪ +ĠBR OWN +ound ed +" ; +Ġtre mb +Ġt iet +ĠÑĢеж им +Ġnuts hell +ел иÑĩ +Ġlos ers +ric ting +Ġrede em +def ined +N ice +Ġbroad band +K O +Ġte asing +Ġpart isan +ı ma +Ġìŀ¬ë ¯¸ +ĠJour ney +Ġslop es +un ing +gr unts +Ġt äll +Ġuncover ed +Ġmy ÅĽlÄĻ +ĠEst her +äº İ +ĠHealth y +Ġë° ij +r ée +Ġpolar ization +Ġfl av +Ġcambi ar +Ġy r +ĠR anch +Ġspl its +Ġtrou vé +åľĭ å®¶ +Ġrecord er +Ġdé part +ÙĪ Ø¨ +ĠK ry +Ġinteress ant +Ġeder im +ÅĽ wiad +il ateral +w right +Ġpour ra +ê ter +Ġcam el +á ŀ +Ġrapid ement +Ġme j +Ġstiff ness +AD AS +Ġdiff ers +Ġal ot +ĠS ig +ÑıÑĤ елÑĮ +Ġabstract ion +åľ ĺ +Ġke iner +gr upp +ĠSher lock +íĺ Ķ +Ġc ite +Ġover flow +Ġt ại +ú car +b ula +Ġconjun to +ĠC I +Ġmoder ator +Ġindirect ly +Ġalle ine +â Ĥ +ÑĪ Ð¸Ð± +Ġб аб +Ġdan ach +Ġ19 39 +Ġpr omet +Ġdest inations +ĠIll ust +ικ ÏĮ +Ġsab es +Ġhe h +ĠGesetz ent +ĠM iz +ен ко +ĠM ys +Ð ¬ +ĠJuda ism +Ġmust ache +Ġst immt +ĠG aza +Ġvol te +Ġnu o +Ġm ón +ĠCom put +ู à¹Ī +ĠR adi +Ġexception ally +Ġassum es +éĸĭ å¿ĥ +ãģĪ ãģ° +in form +Ġshr ine +æĵ Ĭ +Ġimplic ation +ĠF itz +æ²Ĵ éĹľä¿Ĥ +! . +Ġl t +Ġall oy +Ġeth ic +Ġmonaster y +ìĭľ ì£ł +ica ção +Ġcoordin ating +ĠM oto +Ġover look +Ġcho is +Ġantibiot ic +ĠMin ne +ĠB J +ĠA pa +or ian +Ġsp illed +J am +Ġhus bands +Ġcre ations +Ġa ñ +üs sel +ĠìĿ´ì ļ© +Ġanaly se +r ose +Ġpunch ed +Ġpres que +Ġastron omy +Ġschwier ig +ĠEb ola +Ġc is +Ġac et +ĠF X +end re +ĠìĿĮ ìķħ +Ġweb page +Ġfre aked +Ġlat te +Ġì¿ ł +Ġë¨ ¸ë +N ever +G ra +íĻĶë ¥¼ +ey ed +Ġë°ľë Ŀ¼ +Ġesper a +Ġapare ce +ra ção +Ġdisrupt ive +ĠJo int +ur ous +re as +Ġquer ÃŃa +Ġdistrib utions +Ġexpon ent +ì¹ ĺ를 +Ġd l +z hou +ĠHe aring +å·® ä¸įå¤ļ +ĠC raw +Ġflo ats +oun ced +L ab +W orld +Ġbur dens +Ġauthor itarian +ĠB olt +Ġод нÑĥ +Ġpige on +Ġdistract ions +ĠHeraus forder +Ġz est +es c +Ġsh akes +at as +ĠÙħ Ø´ +hol es +Ġthink ers +al ta +Ġar che +ĠS uk +an ha +Ġtempt ing +Ġyou tuber +Ġv ì +Ġdz iaÅĤa +ĠVatic an +P ark +Ġsup ers +ĠNik ki +ëĬ IJë +or ang +ram ient +é ¬¼ +Ġê°ĸ ê³ł +Ġdessert s +Ġav ere +ĠGreg ory +Ġëĵ¤ìĸ´ì ĺ +Ġcost ing +ĠClin ic +Ġreb els +ĠM ob +Ġbun lar +ĠYour s +ert ime +Ġret ali +m ara +at us +all es +Ġд ÑĢ +Ġд иÑģ +Ġdiscount s +ĠGU Y +Ġкак ое +ĠExper iment +re ment +ĠXi ang +Ġb ate +W E +Ġspecial ize +Ġde ity +ĠL oki +m ag +ĠN it +W est +Ġmater nal +Ġqu is +åŁº æľ¬ +bro ken +Ġlas ers +Ġha kk +ĠAng els +Ġmaster y +ant is +T iffany +ee e +ç ij +ore m +Ġin acc +Ġjurisd ictions +ĠKard ash +æľ º +I l +ĠS inn +åĭķ çĶ» +Ġathlet ics +c ÄĻ +Ġlo osely +Ġdiet a +A g +Ġ? ? +ĠëĮĢ íijľ +Ġsuper v +Ġnut rit +Ġdr ifting +ĠìĦłìĥĿ ëĭĺ +Ġпон Ñıл +ĠVict ory +ÙĦ Ø© +×ķ׳ ×Ķ +Ġп иÑĪ +Ġsh aved +Ġmes ure +ond en +Ùĥ ر +Ġex ile +ĠDes de +ĠP interest +Ġattach ments +Ġh ombres +Ġfin es +ĠìĦ¸ ìĥģ +Ġsleep s +ĠT aco +ĠI RA +ri os +Ġo ll +et es +Ġun ut +fashion ed +Ġtre ball +ĠNear ly +ĠÑĢе алÑĮно +Ġch il +éĢ ± +ÄŁ a +ĠM EL +ros cop +ĠC G +Ġv enge +Ġdishwas her +al gic +Ġmod ifier +Ġemb assy +t imer +em ics +Ġintric ate +Ġev et +ĠëĮĢë °ķ +Ġis ot +Ġна ÑĥÑĩ +ĠQu iz +res o +δ Ïİ +Ġye lled +Ġfed er +ELL ER +Ġexceed ed +on as +ic ano +Ġжив оÑĤ +ĠMa o +ĠKaz uto +Ġ ãħĭãħĭãħĭãħĭ +Ġfront line +ĠHung arian +Ġüber all +aw at +Ġgri ps +i ções +arn ya +ĠÍ ¡ +Ġse id +Ġan ak +Ġacab ou +íķ ij +Ġnot orious +ĠGod zilla +Ġover coming +ĠP end +Ġol abilir +ül me +Ġer halten +ãĤī ãģĦ +ê· ¹ +ĠM eter +Ġsta an +O l +Ġch ats +ĠBu enos +ÃŃ ve +alu able +Ġstrateg ically +Ġcompr ised +ĠпеÑĢÑģон аж +Ġw ann +ĠC en +н иÑĤе +Ł ģ +ĠÑĤоб ой +i ad +ĠkardeÅŁ im +ĠCongress man +ream ing +h omme +Ġcommun aut +Ġalcohol ic +Ġpick led +Ġac ord +p osition +eg ól +Ġtrou bling +ĠMarch eg +Ġzum indest +Ġseam lessly +Ġol un +ĠTV s +ĠпÑĢакÑĤи ÑĩеÑģки +Ġback end +ãģĵãĤĵ ãģ«ãģ¡ãģ¯ +id able +Ġgad get +Ġfa ço +ĠMarcheg iani +Ġë° ¤ +Ġaccident al +ĠL P +Ġeld est +ĠAd miral +Ġn Äĥm +le ver +Ġpast el +Ġfond o +Con nie +Ġter cer +Ġp act +ĠMont e +Ġme ats +ĠS MS +ĠAustral ians +ç ¼ +Rh ett +Ġexact ement +Ġë¹ ¼ +ĠM OD +ç ¡ +ĠR apt +ĠNo ch +Ġab ort +ĠNav al +ĠFu ji +IN TER +Ġнов Ñĭй +Ġmiej sce +ĠIC U +ĠGrad uate +ĠGl en +ard i +ĠÈ ĺ +Ġsold er +Ġprofess ions +Ġorth og +om n +int rodu +ĠDen ise +ìŀIJë ¥¼ +Ġcorrespond ence +AM A +Ġinf lict +Ġf and +ĠG ü +ĠÑĩ еÑĤ +Ġtr aced +Ġpat ents +Ġamb ush +Ġlot ta +ff er +ĠW agner +Ġimp erson +Ġextr êmement +ÙĤ ت +cond uct +A tt +ĠM ueller +ĠAl icia +Ġcy c +Ġha cker +Ġt ys +Ġha il +Ġз аÑıв +Ġpas so +Ġì¶ Ķê°Ģ +ĠÎ Ī +Ġpack aged +ĠC ynthia +he et +ä¸Ń åĽ½ +ĠNiss an +ĠQuest o +é ¨ +d id +Ġμ ια +ĠEll is +ĠAnal ysis +ce mos +Ġas eg +ĠMy ster +ĠCa o +Ġtu v +ĠIndust ry +주 ê³ł +ot al +Ġpeque ño +br as +Ġcompreh end +ĠSim pson +ÑģÑĤв ие +ocr acy +иÑĩеÑģ ки +ĠM ush +ĠLaur ie +Ġtriang ular +ĠPres ents +ĠK unden +ç´ ¹ +æŃ ¦ +ĠIs s +ĠDe ck +á»ĥ n +ĠDark ness +Ġinflamm atory +eremi ah +Ġwar med +vey ard +ĠMem ory +et ty +Ġtax payers +ภĵ +Ø ¡ +Ġpract ise +ëĭ ¬ë +Ġdr illed +m Ã¼ÅŁ +log o +ĠF ach +¤ë ¡ľ +Ġübrig ens +Ġkon nten +Ġnormal mente +Ġarg ues +iling ual +°ë ¥¼ +eg al +Ġtrava ill +ov y +а ÑĤо +Ġr uth +ĠL ights +Ġconsist ed +×ijר ×Ļ×Ŀ +Ġstere otype +Ġpay er +ĠRe e +ĠAir bnb +Ġdr owned +ĠZ oe +Ġcan opy +Ġbar r +Ġн оÑĩ +Ġpag an +Ġj ars +Ġr ê +er ver +æĪ ¿ +ie ben +Ġes pect +ĠF i +Ġunw illing +Ġtechn ician +ặ t +m ember +ĠCan al +س Ùħ +Ġlie ber +Ġin ference +Ġhon oring +åij µ +ĠCamp aign +Ġline age +ĠSt ress +Ġvict ories +Ġde ja +× £ +ê tes +bl ick +Ġмен ее +oth s +ĠCou ple +J ason +ĠNic olas +ек Ñģ +l ib +Ġher ramient +Ġ×IJ ×ķ×ŀר +Ġвид им +mill imeter +Ġsil houette +Ġdrive way +Ġcher ish +ãħł ãħł +Ġrans om +Ġinter disciplinary +ĠPort al +Ġtra g +th ood +Ġted ious +Ġgloss y +Ġpré par +ĠC ay +ĠT ook +ĠBott om +Ġz ig +å « +åį ± +re presented +à¹Ģล ย +Ġdesar rollo +ìĦ ľë +Ġvis cos +Ġmill igram +ĠG und +Ġfer ment +d rum +Ġdraw ers +La ugh +Ġpel os +Ġpave ment +Ġmem oir +av ait +Ġ20 50 +¤ë ¥¼ +Ġraz ón +Ġflour ish +Ġst ern +ä¸ Ī +ĠCh ung +Ġser pent +ĠGentle men +羣çļĦ å¾Ī +k ook +Ġl ut +import e +p arent +Ġw sz +Ġsc ree +ĠMitar beiter +å· ´ +m ut +Ġìĸĺ 기를 +Ġsem ble +ĠO W +Ġinvestig ator +ĠCher yl +ĠG erald +Ġpr ere +Ġcomp ares +ny t +Ġdiferen ça +? - +Ġqu á +ר ×Ļ +S en +Ġhe ps +Ġgrat uit +Ġcons ort +ĠST OP +ĠProtest ant +Ġelectro de +â Ĺ +Ġsecure ly +иÑĩеÑģ кой +Ġt ää +Ġreg isters +ĠHeaven ly +og ly +iss ä +ĠPhys ics +ĠMer kel +Ġré v +éĻ ¢ +Ġer ased +ĠSac ramento +Ġcoff in +Ġex acer +Ġl anz +Ġpo ets +ul if +Ġì¹ ĺë +ĠN erd +ĠN CT +ĠH our +neh mer +ŀ ĺëıĦ +ĠPrin ci +S w +m ies +ar med +ĠBeat les +Ġpropag ation +Ġexch anged +Ġcum ulative +Ġì§ij ìĹIJ +Ġdefe ating +æĬ ± +b els +Ġw es +ĠOdys sey +ä½ł æĥ³ +av ior +ĠìľĦ ìĹIJ +Ġbr it +Ġhij o +D AY +ĠاÙĦت ÙĬ +ĠС еÑĢг +Ñĥ ка +eds iÄĻ +Ġimp os +Ġell as +Ġfire arms +ĠN R +Ġ×ij× IJ +ĠÐŁ ока +aw i +ĠìĦ± ê³µ +Ġpup ils +ĠT ack +Ġfr ase +ĠSh ip +Ġst ad +ä¸ ľ +ĠGreat er +un un +imm ung +gr own +ĠN XT +ĠAmeric as +f ox +Ġmant en +éłIJ åĤĻ +ĠÑģ ок +Ġr ikt +lect ric +de ep +Ġзна еÑĪÑĮ +Ġben ut +ĠInf rast +ĠEm ir +ĠоÑĤп ÑĢав +ĠKim chi +ĠFinn ish +´ìł ģ +ina ire +Ġo ike +æ¸ħ æ¥ļ +Ġhost age +ĠBut ton +ÙĤ ÙĬ +ek ing +ĠKaz akh +Ġcomfort ing +Ġso g +Ġgreet ed +g uitar +p ayer +Ġrel ational +Ġconstru ir +çī¹ åĪ¥ +op ian +ĠVol ume +iet h +ÑģÑĤв ом +ur rection +li ÅĽmy +Ġhem isphere +ĠBe an +IG N +Ġköt ü +ĠFall out +Ġbr ace +ç¹¼ çºĮ +ÏĢ Î¬ +ĠH AS +Ġg é +Ġcharacter ize +ặ c +ĠMil ky +Ġtum ors +Ġn uit +ĠG az +ĠìŀĪ ëĭ¤ëĬĶ +Ġг аÑĢ +ess ment +ĠA be +Ġë½ ij +ĠEins atz +J IN +j ä +C ry +ĠProm ised +ĠÑģеÑĢ Ð´ +ok us +Ġscal able +ĠпоÑģмоÑĤÑĢ ÐµÑĤÑĮ +ück lich +Ġreal ism +Ġmay o +Ġjuven ile +Ġhead lights +Ġgör Ã¼ÅŁ +ĠRe form +Ġhal ves +cz ne +Ġbreak up +że j +Ġr ätt +D ay +ĠìĿ¼ë ³¸ +Ġmu erte +Ġtun es +ĠSm ile +rec ord +Ġrecher che +atisf ied +Ġpo zi +Ġcelebr ations +ise xual +ĠRO B +third s +ĠF ortune +ĠÑĤ ой +Ġbrand ed +lo o +Ġd ud +Ġrandom ized +Ġcomb in +ä¸Ģ äºĽ +ier an +c zenia +į ãĥ« +Ġcur ator +Ġar tery +ĠÑĥ ÑĪ +ĠÑĩ иÑĤ +Ġsubsid ies +Ġbloss om +ĠTw ilight +Ġhy vä +ĠPom pe +ĠC isco +ĠÐŁÑĢ Ð¾ +Ġbir i +Ġg ern +Ġre built +Ġw cze +Ġbenefic i +Ġdrum mer +Ġsol ids +Ġdi yorsun +ãģĤãĤĬãģĮãģ¨ãģĨãģĶãģĸ ãģĦãģ¾ãģĹãģŁ +l ated +Ġmud dy +Ġh olog +Ġcl aps +ĠR ings +ĠO key +ĠBra ve +Ġvalu ation +Ġmig rant +Ġinter mitt +Ġeig ene +ili ary +ãĥ¼ ãĥĪ +mark t +k r +ĠR ib +á»Ļ i +Ġaccus ations +Ġa rab +w ash +ĠBard zo +Ġu gh +est ers +oph ren +Ġaliment os +ĠU z +Ö Ĥ +Ġ6 50 +ĠпÑĢи еÑħ +F I +Ġsamp ai +Ġparl é +hes ion +Ġs ır +Ġapparat us +Ġcor related +ĠPrincip al +Ġcor r +ĠOffic ial +иÑĩеÑģ кие +Ġtermin als +Sh ould +Ġvac un +Ġst ellt +Ġmo oi +etz ung +Ġк ÑĢа +Ġda i +Ġп ож +Te am +ĠP PE +ĠÐŀ Ñģ +ĠLe ah +ĠI vy +y st +Ġuh hh +Ġnight time +Ġtrend y +Ġsec urities +Ġcontin ents +Ġfirst hand +ĠVer on +ĠëĤ ® +Ġbrows ing +ĠC ada +t ro +Ġtr amp +re ib +Ġerst mal +irl er +Ġps ic +Ġget ir +ĠN P +Ġdzie ci +об ÑĢаз +Ġmagic ian +Ġscrut iny +Ġsl ab +ĠO T +ist y +ir ies +ore st +Ġtask ed +Ġmor ally +ìķ¼ ì§Ģ +ust ered +Ġfool s +Ġir respons +Ġein f +Ġvi á»ĩc +Ġsc or +Ġpill ows +ĠG egen +Ġtut te +Ġquarter ly +Ġdid nt +ĠG ym +ĠE ther +ĠØ « +лиÑĪ ÐºÐ¾Ð¼ +Ġsign aling +ĠN ode +ĠDonc s +Ġy ah +ĠKan al +Ġf ading +et in +Ġinfluen cers +Ġmed als +Ġengine ered +Ġfer mented +ê²ł ì§Ģë§Į +ĠBeet hoven +×ŀ× © +inent al +ĠìķĮë ł¤ +üt fen +al nya +Ġo vere +Ġden kt +ак ÑĤеÑĢ +Ġâ ĺ +Ġneces it +Ġgener ators +gr ass +Ġпод Ñĥм +lie ÃŁen +B ar +ľë ıĻ +ĠдеÑĤ ей +Ġsuck ing +Ġsten cil +Ġprim o +ĠBreat h +st rom +Ġimmens ely +Ġapp reh +ìłķ ìĿ´ +P op +Ġj ong +ĠGi ul +ĠAD HD +Ġhö ren +Ġe lo +iv ent +Ġr us +Ġoutrage ous +Ġmaster ed +Ġì» ¤ +ÙĪ Ùģ +ip es +ĠRud y +Jac ob +Ġbull ish +Ġt apped +Ġfa ud +iz ophren +ĠÑģо Ñħ +ĠDar ling +Ġ196 3 +ĠPre vention +² Ķ +Ġabdom inal +st ones +Ġav aient +á»ķ i +m ake +Ġs are +ĠInst ant +к ам +Ġkeep er +Ġblank ets +ãģ§ ãģĹãĤĩãģĨ +Ġswe ats +ĠMinne apolis +åħ¨ éĥ¨ +Ġgen ommen +Ġfast en +ĠBrus sels +åij ¼ +Ġcaf eter +Ġabsor bing +Ġha go +ĠEl mo +Ġgust o +ĠY ap +M úsica +Ġt ert +Ġband a +Ġm ily +Ġthere after +ĠStock holm +ĠC arson +Ġcalib ration +ava ÅŁ +ans a +ik ke +Ġfore see +Ġqual che +Ġdest e +æ ¤ +ün üz +Ġfor ge +D is +est en +Ġδ ια +Ġenca ps +ĠGes pr +Ġcher cher +ick ets +ÑĤоÑĢ Ñĭ +C r +ĠТак же +Ġrabb its +ĠD ot +he iten +Ġcaus al +ĠF oster +ajÄħ c +Ġbere it +Ġayud ar +é« Ļ +ãģ ³ +s ong +com b +Ġfr inge +Ġcyber security +Ġëľ ¨ +Ġk ier +Ġbesch äft +Ġкон ÑĨе +Ġfacil it +ĠNam en +Ġbil ateral +t x +ĠW issenschaft +Ġnu ances +Ġr ipping +Ġf y +ĠSicher heit +ĠGh ana +ol on +Ġto pped +ĠMoroc co +Ġrad ial +ĠL EE +ĠAndre as +ed d +ĠìĹ ´ë +ĠAirl ines +ãģĵ ãĤį +Ġval ores +ê· ľ +H y +Ġзад аÑĩ +ĠKend all +ĠÑħ аÑĢ +ĠV amp +Ġpy thon +Ġmanage able +ĠG ente +o ise +ici ary +Ġimp oss +ĠBun ny +iest a +And rew +Ġser t +ĠC ec +zz arella +Ġautom obile +ĠT iere +all ows +åĨ Ĩ +Ġë° Ģ +ĠSc orp +ĠJ elly +ag ara +ĠSt retch +Ġrede f +Ġexacer b +ĠS HA +é f +ors a +Ġflaw ed +ĠNo el +?! ? +Ġpro cent +Ġmen stru +ĠпÑĢо Ñĩ +Ġinf ants +ðŁİ µ +pa use +ĠR acing +Ġ194 8 +Ġsuper intendent +id ores +id y +bra him +Ġunl ucky +Ġper k +an ci +Ġë§Įë Ĥĺ +ĠÐľÐ¾Ñģ кв +Ġfin ans +Ġdiferen cia +łĪ ìĿ´ +éħ į +OR Y +ĠT ac +ÛĮ ا +Ġdes em +Ġваж но +ĠJ U +ĠìŀĪ ìŀĸìķĦìļĶ +ĠÎ Ŀ +Ġinform ations +ĠH EL +h st +Ġпог овоÑĢ +Ġvo iture +Ġre us +änd ig +ĠпоÑħ ож +j ing +Ġd ru +alt ra +Ġprodu its +Ġk ite +Ġeye ball +ĠB elt +ĠRestaur ant +Ġg amb +Ġpor ridge +it ters +Ġconver ts +Ġyard ım +Ġmáxim o +w irtschaft +Ġíķĺë Ĥĺë +Ġì¤ Ģ +Ġice berg +Ġvor bei +Ġ25 6 +ocr atic +Ġreck less +on ner +Ġm ús +Ġlog ically +ĠPr ison +ĠNet z +Ġvac ant +Ġn immt +ĠH ARR +Ġз ов +ĠDe e +ring e +ni est +ĠR ules +ìĬ¤ë ٽ +cuss ions +Ġfl oral +Ġconstra ined +Ġdifferent iation +ĠQue bec +ĠÛģ ÛĮÚº +Ġpúblic a +it el +Ġaccommod ations +ĠGr ü +í ľ +Ġpick les +иÑĩеÑģ киÑħ +Ġcomm issions +ĠBa ek +Ġçoc uÄŁ +ĠMed ium +Ġperiod ically +Ġwonder fully +Ġstaff ing +ìĽ IJë +ri re +f le +ĠMc L +ĠÑĤ еп +ĠпеÑĢ ÐµÐº +н олог +Ġíģ¬ ê²Į +çϼ çı¾ +Ġprosper ous +ĠSpirit ual +ĠCh ick +DI A +ĠÐŁÑĢ Ð¸Ð²ÐµÑĤ +Ġper ÃŃ +ÑĮ ÑİÑĤ +Ġconsult ants +ĠEar l +ä»Ĭ å¹´ +Ġru ining +оÑĢ Ðµ +Ġpens er +Ġtak iej +Ġstrength ened +ĠLiqu id +он еÑĨ +ав аÑĤÑĮ +Ġcam er +Ġdisagre ement +Ġbat hing +ĠY osh +a al +pre chen +RIS ADAS +Ġsuper star +æģ Ń +лÑı ÑĤÑĮ +Ġn ib +ĠTh erm +ĠDAN IEL +Ġp aw +Ġliqu ids +Ġcapac it +ark en +Ġvag ina +Ġm ashed +Ġemer ges +ys cy +Ġun related +ĠGu ild +Ġin verted +it ives +T ra +Ġbe gr +Ġal te +ì§ ķ +ãĤģ ãģ¦ +ĠÑĢазÑĢ Ð°Ð±Ð¾ÑĤ +f inder +Ġдал ее +Ġблаг одаÑĢ +walk er +Ġcr ater +ass adors +ren ces +ins ki +ĠK IM +ĠEll iot +20 17 +ĠS r +ink a +ano v +Ġìŀĺë ª» +Ġpropriet ary +display style +ĠÑģ им +Ġиз б +ĠPan el +Ġinstinct s +ĠCommun ications +éº » +mid t +Ġë§Įëĵ¤ ìĸ´ +ĠÑģл ова +ĠGil bert +缮 åīį +Т ак +voor beeld +е ÑİÑģÑĮ +ary n +que z +Ġd art +Ñĸ ÑĪ +ĠH ut +S al +Ġs outheast +Ġpestic ides +Ġhelicop ters +Ġend ured +i ada +Ġbre wing +ìĹ ¬ë +ĠÑģв обод +ĠS aints +ĠFr ançais +ĠEconom ics +Ġdis loc +oph obia +C amer +Ġnegoti ated +ĠÑģÑĤ али +ìĬ¤í ģ +og ie +Ġtsun ami +Ġpeel ed +Ġmotiv ations +è¨ Ń +ost at +fl an +ĠD AC +Ġk av +' RE +ĠPe arson +b be +c zenie +Ġaten ção +íĨµ ëł¹ +ãģ£ ãģ¡ +ĠÑĥд аÑĢ +Ġintrodu ctory +ĠI ci +ë ĮĢë +ak at +Ġt rench +Ġproceed ed +ĠCo in +Ġdere cho +ĠRed e +æ¯ Ľ +ан нÑĭй +Ġincarcer ated +ĠRich mond +R ock +ĠP av +ĠKar ma +ug es +Ġconte ú +ë ¹Ħ +Ġê·¸ë §Į +ĠG one +Ġwsp óÅĤ +ĠRah men +un ken +Ġì¤ijìļĶ íķľ +Ġi b +Ġatt aching +H ay +Ġsu ka +ìį ¹ +Ġpivot al +ĠRes pect +ÃŃ da +I B +ĠVer antwort +w iet +Ġforens ic +ÑĢи ÑģÑĤ +ĠпÑĢинÑĨип е +Ġmark ings +Ġk ettle +ĠOper a +ĠDo ctors +Ġshred ded +Ġrec uer +Ġvig il +ĠF ail +Ġentre v +Ġд ÑĥÑĪ +Ġout breaks +èµ° åIJ§ +ĠÏĢ Î¿ +Ġro gue +ang led +Ġyear ly +ĠCre ed +Ġw am +Ġlot us +ê³ ¼ë +ãĢģ ãĢģ +ĠSp it +ĠIt u +Ġstra ins +Ġstamp ed +Ġpl aint +Ġpot ion +Ġconsolid ation +è© ķ +оÑĩ кÑĥ +Ġvlog ging +Ġsl ate +ĠAu ft +ĠInc or +ừ ng +§ IJ +en h +Ġhe iÃŁ +Ġdom est +ĠSt rom +åį ³ +ak is +Ġfra gen +Ġfin er +ĠS ug +Ġup hill +Ġé én +â̦ ) +ĠÑģ оп +ĠCore y +Ġsie bie +Ġm use +Ġclo ves +Ġp ous +ĠFin anz +ĠR oute +am at +Ġmut ually +ĠвнÑĥÑĤ ÑĢи +ĠSel ena +ë Ķ +ĠGa ussian +ë ¶ĢíĦ° +Ġ×ij× Ľ +Ġej erc +å¾ ® +ke a +ĠG erry +ĠS ic +大 çļĦ +Ġ196 6 +ies e +Ġfoss ils +Ġest ad +ĠK ane +ci Äĩ +Ġìľł íĬľë +Ġп ам +ĠCru ise +int érieur +Ġbe kannt +ĠP ode +Ġdem ander +R em +Ġinv ade +Ġdecor ating +rop ic +Ġcow boy +ĠPh oto +opol it +Ġì»¬ë Ł¬ë +Ġre ap +Ġhand writing +à¹Ħ ร +Ġë ļ +Ġب عد +ĠM t +Ù Ģ +Ġspaces hip +Ġnational ism +Ġcouncil s +ĠGriff in +ĠAh med +Ġcl ich +ĠO L +w l +ĠPil ot +å® ® +Ġacron ym +Ġg els +Ġelectro ly +è ĵ +Ġм ной +Ġepis od +ĠDies es +ĠAT P +Ġed iyorum +Ġexpress es +Ġexhib its +C omm +Ġк ÑĢÑĥп +Ġmat ar +Ġ20 25 +ĠArt em +vas ive +r Ãł +Ġbe ÅŁ +é» ĥ +Ġliz ard +Ġfill e +Ġì§ Ī문 +Ġмо Ñī +Ġt ür +Ġcul prit +Ġwo ven +ĠAN Y +n im +Ġt ay +Ġprom in +Ġacom pa +Ġid é +Ġbo iler +ĠThe men +Ġaven ue +ĠM ud +Ġнов Ñĭе +Ġwitness ing +Ġl ance +ĠCH AN +ĠBe ver +ت Ùħ +Ġchem otherapy +K ing +ĠbÄĻd ÄĻ +Ġat ual +Ġt ive +Ġtalk in +Ġqued ar +ie ÃŁ +ed el +Ġìĸ´ì łľ +Ġjog ar +Ġö r +Ġundert aking +ĠStre ngth +Ġmil hões +ĠW ine +ĠM olt +è® ² +ãģij ãĤĮ +Ġunderm ine +ĠArch ives +v ana +mer cial +M C +Ġcast e +п ÑĢ +Ġlegisl ators +ul ators +ên io +Ġëį °ë +ĠÑħоÑĤ иÑĤе +Ġн ек +Ġs urn +Ġcons ci +ĠP OW +Ġcul inary +ĠK AT +ĠFol ks +Ñĭв аем +Ġв ок +ãģij ãĤĭ +s ervice +pt s +Ġпоб ед +æĺ¯ åķĬ +Ġt ents +Ġn ord +ST E +Ġrepublic an +Ġwy k +Ġmin ions +èĻ ķ +Ġmem ang +j est +Ġcompar ative +Ġty le +car bon +bed ingt +ks en +Ġneg ativity +Ġsjäl v +Ġd ú +æīĢ æľī +Ġrec alled +c ra +ĠT ada +ĠÑĢÑĥ ки +ĠопÑĢед ел +Ġproc rast +Ġjog os +ĠO o +ĠHe arts +Ġé ch +Ġksi Äħż +Ġco arse +ĠT ube +ĠG reens +Ġé n +Ġdumb bell +ĠÑĤ и +Ġquer er +ا ØŃ +Ïĥ ει +ĠпÑĢав илÑĮно +Ġп ап +Ġcomp ra +Ġt ér +ĠAnt es +Ġoptim um +Ġbisc uit +κ ι +acz ego +Ġìĭľê°Ħ ìĿ´ +ĠMar ines +ver o +Ġvacc inations +Ġpet ty +rit ers +Ġа л +count ry +Ġcoun ters +Ġattend ant +ĠH ui +ãģ¨ãģĦãģĨãģĵãģ¨ ãģ§ +ck a +ÑģÑĤвен нÑĭй +gu y +Ġtrick ed +ĠR ED +Ġthr illing +ÏĢο ι +Ġpig gy +Ġan unci +OR TER +ĠVal ue +Ġr ond +ĠA DA +Ġpos er +h ores +ĠR oland +ĵ ¯ +Ġno ir +Ġש ×IJ× +ë° ľ +iem and +ĠпоÑĤ еÑĢ +ê³ ³ +Ġê± ± +Ġformat ting +ĠL ed +è§Ģ çľ¾ +Ġkill ers +ĠÄij ấy +Ġha ar +ag ain +! </ +Ġsomet hin +Ġc oughing +Ġn ave +Ġprospect ive +ĠH K +ĠRes cue +may be +g ger +ĠÑĢабоÑĤ Ñĥ +×ķ׾ ×Ŀ +t ails +íķĺ íķĺ +Ġeyel id +Ġcustom ization +avil ion +Ġpro chain +Ġgla ze +æĥħ æ³ģ +S im +Ġоп аÑģ +Ġmosquito es +Ġf ent +Ġcapac ities +Ġapost les +Ġalt ura +Ġë¬ » +Ġser ont +ĠAny time +¥´ ëĬĶ +Ġcos play +Ġsp ac +Ġsam en +ãĥ Ħ +uc c +i ères +Ġsib ling +ĠC ock +Ġëı ħ +ĠпÑĢед ÑģÑĤавлÑı +Ġinstall ment +Ġdi je +ĠMC U +ĠE H +ĠN ing +Ġprep ares +Ġhyp ocr +pt y +Ġkad ın +ĠFro zen +ha ul +ĠK ylie +éĢĻæ¨£ çļĦ +Ġsh uffle +Ġelement al +Ġau ÃŁer +ĠKN OW +ĠAL ISSA +Z A +ì² ł +ç¾İ åħĥ +Ġrec ite +Ġsc rib +Ġ11 5 +ä¼ ij +Ġstar red +Ġle quel +Ġbre wer +ĠOpp ortun +Ġr ä +Ġchop sticks +ĠK ah +ĠEthi opia +Ġhand made +Ġer folg +ĠD z +itt ens +èªį çĤº +в ал +η ν +åĬ ŀ +ãĥ ĵ +br ingen +Ġunpl ug +Ġoff s +Ġher man +l ied +ason ic +ĠSer bia +ĠGu atem +Ġ... " +Ġer reichen +Ġamb iguous +ĠWhit ney +z uf +M AND +ł µ +Ġsqueez ed +ãģĿãģĨ ãģł +y as +é¾ į +ĠSh ock +Ġutil ise +uk o +b olt +Ġmot if +Ġin mates +Ġcorrupt ed +Ġconc ret +ĠCrit ical +ĠSing ing +ĠÑĦ Ñĥнк +éŃ Ķ +no va +reb be +d t +U nis +Ġweb cam +Ġcam oufl +K en +Ġlaws uits +ĠCons umer +Ġrec oll +Ġkle iner +ĠF IFA +Ġ196 2 +èŃ ¦ +Ġmal ad +Ġì° ½ +ĠÃ¥ t +Ġinfluen cer +ĠArt ist +st i +ãģªãĤĭ ãģ»ãģ© +ว ย +ys ÅĤ +ĠB ian +Īë Ħ¤ +Ġfire place +ĠApplic ation +Ġm niej +Ġacid ic +ĠMorm on +ss a +åĭ Ļ +Ġsneak y +Ġo jos +Ġv oud +ĠD ai +Ġgrass roots +ĠUn believable +ĠG abe +ĠExt reme +Ġhass le +Ġco b +m umbling +P ass +Įë Ł¬ +Ġsystem atically +Ġsev enteen +ÏĢ ÎµÎ¹ +âĻ ¡ +Ġк оÑĤ +Ġsend iri +Ġbathroom s +ĠS tern +ĠAr duino +è ¹ +cri bing +Ġreop ening +Ġc erv +pe e +AR I +Ġcad re +ĠAn ch +L ee +ĠMA X +Ġm änn +Ġch ores +Ġad esso +æĿ ij +ĠN ig +Ġdissert ation +ĠV ay +ST ALK +ак а +av at +çł ´ +Ġpun kt +Ġpad ding +ĠT empl +Ġe je +Ġí Ħ° +Ġa zt +ĠëĮĢ íĨµëł¹ +Ġrearr ange +á ch +ĠìĤ¬ëŀĮë ĵ¤ +Ġfre akin +cri re +Ġì» ¤ë +ĠExpl ain +ĠÏĦ Ïīν +Ġbod ily +ĠLe ist +Ġsig ui +Ġbunk er +Ġaz ul +ĠHa ush +S ub +ĠÐIJн д +ĠкÑĢ Ð°Ð¹ +Ġilleg ally +ĠM uy +ĠFe i +ĠBan ana +Ġscholar ly +ĠPr zy +ĠM oss +ĠFil ter +Ġìĸ´ëĸ ¡ +ĠMax well +ten se +Ġlong itud +Ġlang sam +Ġ×ŀ× § +sm ith +iz ada +ĠноÑĢм алÑĮно +ĠV oll +ĠEl ena +æĸ¹ éĿ¢ +ĠÑħ оÑĤÑĮ +ĠD abei +Ġconserv atives +Ġpróp ria +ĠDies er +ĠBrend a +ook ie +Ġb anc +ãĥ ¯ +ìĿ´ì ¦ +ìĽĥ ìĿĮ +Ġke h +Ġwed dings +Ġthunder storm +æĶ¾ å¿ĥ +ĠCo ordin +ìĪĺ ê°Ģ +Ġprze ci +éĴ ± +OS STALK +ma an +Ġê± ´ë +Ġب Ùĩ +Ġż ad +Ġy acht +Ġgö t +Ġble ach +Ġshort en +ĠÑģÑĤ ало +us an +ĠìŀIJ ìŰ +Ġd ers +x is +įĶ ëĭĪ +Ġquant idade +Ġopp ressed +Ġзакон Ñĩ +ä¸Ī 夫 +ãģĪ ãģĪ +ĠÑĩ еÑĤÑĭ +ĠÐĿапÑĢ Ð¸Ð¼ÐµÑĢ +ul p +æĢ ĸ +ÙĤ ÙĪÙĦ +оÑĩ е +ά λ +zen iu +Ġform ations +Ġspark ed +ĠEntwick lung +all s +Ġviv ir +Ġexp iration +ot ine +ĠЧ еÑĢ +ĠTur ning +Ġtar iffs +Ġnast ÄĻp +Ġab ide +ik si +Ġflash es +Ġdisp utes +Ġì² ´ +Ġmer ak +Ġenorm ously +z ahl +Ġf ührt +в он +Ġзав иÑģ +Ġpersever ance +Ġdivid ends +Ġcontest ants +Ġpros zÄĻ +ĠFrank en +ãĤį ãģĨ +Ġexpl orer +Ġbuff alo +âĢ ķ +Ġec ology +Ġsc alar +Ġcr an +ε ÏĦαι +ży Äĩ +ĠìļĶ ë +Ġg ia +ĠG og +ĠPri v +Ġë§IJ ìĿĦ +ĠRe ason +ra ktion +ĠDe borah +Ġk itten +ĠEd in +ä¹ ¾ +p iej +Ġëĭ ´ +Ġmá qu +Ġbid ding +Ġaff inity +Ġa ika +fol k +ĠCon se +Ġdeuts chen +è Ĩ +Ġdeb it +ıģ ın +is el +Ġì¤ij êµŃ +ĠëŃIJ ê°Ģ +Ġtrust worthy +ĠStart ed +æķ ij +ür d +Ġпон ÑıÑĤно +Ġscient ifically +P ods +CR OSSTALK +Ġpregunt as +Ġcal ming +ĠPrem iere +׼ ש +ĠÑħ олод +Ġcap ita +Ġto ma +Ġmur m +Ġfuer za +ĠH ani +æĪij æľī +ü f +arl os +Ġhä uf +ãģij ãģ¦ +Ġoso by +j ego +Ġп иÑģ +Ġcalm ly +id et +b uch +g one +Ġviscos ity +Ġmod al +Ġges am +ĠH z +Ġmunicip alities +Ġcircul ating +ol ina +S ho +é¢ ij +ĠBen ed +ol u +Ġrest s +Ġl Ã¥ng +ĠÐŀд нако +Ġprz ew +Ġpe pp +Ġmar riages +ĠB IG +and an +Ġmag ically +Ġbab ys +ĠëĮ ĵ +Ġhack ers +B aby +ĠM onst +Ġc ier +ĠAra bs +Ġмаг аз +ĠIndones ian +ãģĦãģĨ ãģĵãģ¨ +ĠMark t +Ġd achte +ĠSch üler +ĠV ND +Ġsp ielt +Ġper lu +ãĤ ´ +åŃ ĺ +ĠпÑĢо Ñħод +Ġsalt ed +Ġimpro vis +ĠInst r +vel mente +Ġn ess +Ġfun gus +Ġcollabor ators +ĠVir us +est ar +Ġproject or +ĠÐŁ ÑĢав +Ġag ility +×Ļ׳ ×ķ +ere l +Ġвоз в +Ġб аз +ĠCath y +ÄŁ u +ĠговоÑĢ Ð¸Ð» +b ility +ĠL anc +ĠKim berly +ĠBr ief +åħ · +Ġut veck +Ġgogg les +Ġpres chool +ç§ į +ATH ER +Ġmot ives +ĠB ong +E X +Ġch illy +ĠAdvis ory +âĢĭ âĢĭ +ĠкоÑĤоÑĢ Ð¾Ð¼ +Ġtra itor +Ġdemasi ado +ĠÑĨ ен +Ġмо и +åŀ ĭ +Ġmult if +ìĶ ¬ +ĠAlex is +Ġz iet +ĠR ama +br ance +Ġsan ction +it ous +×ķ× ļ +Ġë³´ë Ĥ +ÑģÑĤ анов +è¶ £ +ĠÑĢ ÐµÑģ +ĠChurch ill +ĠпÑĢ ÐµÐ· +ĠI O +ĠG ee +ĠG ather +ator i +Ty ler +Ġнем нож +ĠbÃ¥ de +ĠK iller +Ġtu ber +ĠRam adan +á ¿ +ie ht +Ġstrang ely +л Ñĥ +Ġredes ign +Ġinc umb +Ġber aber +ĠVolks wagen +met al +d zy +p ción +ĠìķĬ ìķĦ +åĶ ± +å¤ ´ +ĠGood ness +ив аеÑĤÑģÑı +b ahn +ĠAntar ctica +ек ÑĤоÑĢ +Ġhome owners +ze igt +ĠíĺĦ ìŀ¬ +ì§Ģ ëıĦ +Ġgeograph ical +th inking +Ġg osta +ĠIm am +ulif lower +d ag +an nt +ak ov +Ġdown wards +ì²´ ê°Ģ +CU BE +ĠÐļ ÑģÑĤаÑĤи +Ġпол ов +Ġplate au +ãģĦ ãģį +Ḡ¥ +Ġchlor ine +Ġacceler ator +Ġsol ves +ĠGr ass +p iano +ĠÚ© ا +Ġب ت +ĠRo chester +ĠÙĩ ÙĬ +Ġcollect s +įĶë Ŀ¼ +ĠChe er +ling en +ĠÑĢаз г +Ġam éric +ht a +EC T +Ġart ific +ĠPay Pal +h ana +Step hen +ĠG est +ph alt +Ġrepl ication +ĠWill ie +Ġneut r +Ġirr ational +Ġdad os +ĠA id +k am +an ter +Ġд Ñĥже +Ġdet on +Ġha re +Ġbet s +bag ai +Ġst ained +Ġplaus ible +Ġpe eling +Ġcr ÃŃt +Ġgr ote +ì¶ ° +¥´ ê²Į +alt et +P hone +F il +S QL +Ġge fallen +åı Ķ +Ġsa úde +ĠTam il +c ous +Ġглав ное +Ġatrav és +uss ia +Ġzwe iten +ĠEl vis +Ġmo ver +Ġlim ite +è¿ ½ +are z +¥´ ê³ł +ĠKr anken +ü re +ĠìķĬ ìķĦìļĶ +Ġth Ãłnh +Ġprofound ly +Ġbedroom s +Ġtoothp aste +ĠAc cept +ét ico +Ġkü ç +ĠA ry +ad in +Ġgran ular +ect ed +Ġmen jadi +Ġcompet ence +d oc +Ġspark ling +Ġì¢ĭ ìĿĦ +Ġconstruct ing +Ġam usement +ĠIns urance +ĠFe uer +Ġrenov ation +s uch +pl at +Ġpros th +Ġbe y +ĠComplet ely +Ġz od +al n +V ict +Ġconfir ms +ät z +â ĸ +ham mer +Ġзна еÑĤ +Ġadm ired +łë ¥¼ +ĠF ruit +ert en +Ġnie ce +ĠT iny +Ġpl umbing +erm a +Ġлег ко +Ġwind shield +ĠÑģм еÑĢ +Ġb zw +Ġabol ition +ĠSad hguru +Ġpre ached +ĠCreat ing +çī Ľ +per ed +Ġvol ont +Ġqu int +Ġprin ters +Ġneg ro +Ġgr osse +ĠTh y +ĠFell ows +æİ¥ ä¸ĭä¾Ĩ +Ġst anie +Ġnew com +ĠH ue +ĠFre unde +ĠConst ruction +Ġadvers ity +Ġneg atives +Ġhazard ous +Ġcompe lled +Ġw ok +ĠO y +п а +ª ¨ë +Ġrend ez +Ġover c +Ġwe aving +Ġид еÑĤ +Ġprosecut ors +Ġaudi obook +Ġancest or +Ġunder going +Ġpound ing +ãģĤãĤĬãģĮãģ¨ãģĨãģĶãģĸ ãģĦãģ¾ãģĻ +ĠíĴ Ģ +Ġì¶ ¤ +Ġtule e +ĠìĹ ´ì +Ġzo als +Ġne in +éŃ ļ +Ġo ke +ĠJoy ce +Ġn ud +Ġdil igence +ĠLab s +Ġv ents +Ġancest ral +ห ม +ĠмÑĥж Ñĩ +Ġnom és +表 示 +w ali +q ing +ĠMultip le +ĠCons ult +Ġist edi +ĠD oy +ak ah +Ġdiscipl ined +Ġaltern ating +ç Ĵ +Ġver me +Ġо Ñī +Ġto ta +ĠP rag +Ġsw orn +Ġbe ber +ĠAufg abe +ìļ ´ë +辦 æ³ķ +Ġy up +Ġrec laim +on ut +Ġauc une +Ġam ph +ĠÅĽ wie +Ġa a +isco ver +ĠAr g +cie ż +Ġdess as +ĠW äh +á» ¹ +Ġдав но +Ġsil ently +ar c +ĠíĽĦë ³´ +Ġtwe eting +ĠO nd +é¡ ŀ +¦¬ë ©´ +Ġbow el +ìħ¨ ìĸ´ìļĶ +èģ Ĭ +OS E +Ġprop io +ĠKun st +k ung +Ġdonn ées +ĠHor izon +ĠF rog +åĢĭ 人 +Ġar ist +â l +Ġк ож +Ġseg undos +ĠShort ly +ĠC rowd +ir an +ĠwÅĤa ÅĽci +ĠL ac +ident e +Ġê°Ģ ìŀIJ +Ġl en +ĠS US +ĠMot ors +ĠT rent +om ie +Ġtransmit ter +ĠAss ad +Ġpsych iatric +Ġж иÑĤÑĮ +Ġout lines +Ġeffective ment +ĠRelig ion +pre h +Ġдолж на +ĠÍ¡ ° +ĠCons ervation +Ġ á» +Ġз ай +Ġres ide +Ġcomplet o +K EN +ĠëĤĺìĺ¤ ëĬĶ +Ġsubur ban +Ġrépond re +ĠÑĢаз лиÑĩ +Ġgall eries +Ġr apt +æĦŁ è¬Ŀ +) ... +Ġcruel ty +ĠVM ware +í ά +Ġhay ır +Ġgroup ing +ĠR ider +Ġsyll able +Ġbeispiel sweise +Ġsafeg uard +ĠpelÃŃcul a +art i +ĠС о +Ġche ga +Ġк омÑĥ +Ġse ism +Ġharm less +ĠWarri ors +ãģĦ ãģ¤ +Ġп Ñģ +Ġsham eless +ĠBa um +inst all +Ġtool kit +Ġpip elines +Ġp ussy +Ġconce al +Ġprot esting +och ond +Ġdu a +ĠP ose +Ġhel ium +ĠU X +ik le +ĠS uff +ĠìĦ¸ ê³Ħ +ing ers +ĠÑģлÑĥÑĩ ай +Ġdesc ending +Ġ æ²Ĵæľī +Ġmont age +H igh +ĠìĿ´ì ĸ +ĠI di +Ġ×ij× ¡ +Ġexpress ive +ç§ ĭ +Ġпол ез +Ġp one +Ġadoles cent +ан нÑĭе +Ġassass ination +we isen +em atically +aut h +Ġur g +Ġgan har +Ġfund o +ĠRh ode +ĠиÑģÑĤоÑĢ Ð¸Ð¸ +Ġcompart il +æķ ¢ +Ġdimin ished +Ġapprent ice +ĠÐij Ñĥд +Ġphot ons +Ġcó d +å¹ ķ +æ¬ Ĭ +on ak +Ġadel ante +Ġch u +op ic +Ġa ixÃŃ +ed dar +ĠCong rats +m or +好 åIJ§ +Ġreserv ations +ĠT oby +ĠK ern +Ġraz em +Ġfor ged +Ġhorr ifying +ÙĬ ع +ĠJo ining +ãĥ© ãĤ¤ +ĠA uth +d ah +Ġcons ig +Ġintimid ated +Ġperipher al +Ġmen o +Ġdetect ing +Ġte or +Ġtag ged +Ġnost algic +Ġ미 ìķĪ +åĢ ¼ +Ġver di +Ġlabel ing +п од +ast es +Ġv ist +Ġcy t +Ġfl ips +ÑĢи з +bal anced +ãģª ãģı +Ġо ÑĪиб +Ġdest in +las se +ere i +Ġkal o +Ġar qu +Ġplan o +Ġordin ance +Ġcomp ilation +ĠVocê s +ĠE co +Ġì¶Ķ ì²ľ +Ġenc ima +ĠGar rett +ĠC ord +öl ker +ĠAr row +Ġprot ons +, âĢĭ +Ġì² ĺë +Ġsc and +Ġbe ige +c ong +Ġb iking +ĠT L +Ñĥн д +ĠìĨĶ ì§ģ +ĠV illa +ĠJ ACK +以 åıĬ +ĠÃ¶ÄŁ ren +Ġtem as +ĠKy ung +J enn +Ġc ud +Ġimp osing +Ġcommand ments +ĠMe ans +ĠD är +Ġrecom end +Ġdisp osition +ا Ùĩ +Ġth u +Ġredu ctions +Ġdi u +Ġ×ķ ×IJ× +ĠиÑģ Ñģлед +th ren +Ġl ados +ĠR B +ix ed +Ġì ı +F r +st ill +Ġol mas +CH UCK +ĠíĨ ł +ĠIndepend ent +ÐĴ Ðŀ +Ġp its +Ġundert aken +Ġf ør +ĠN aw +Ġìŀij ìĹħ +Ġsh epherd +Ġlang ue +ĠJ ab +ĠDr um +ĠEle kt +æĭ ¬ +ãģĺãĤĥ ãģªãģĦ +á»ij t +ĠìĿ´ì ª½ +Ġbegin nen +ĠF ury +á»ĥ u +se ctions +Ġspray ed +Ġmá r +ĠV olt +ĠSe ong +иÑĤ ел +du ction +as an +Ġjud gments +ima an +ŀ× ª +Ġs iento +ĠAC T +ĠB H +de v +Ġì¢ĭìķĦ íķĺ +Ġj orn +IS TIN +Ġro ar +Ġimmers ion +aff les +Ġtra inee +ĠBill board +ress es +ĠWar m +ĠRober to +Ġutil izz +ĠIg or +Ġr ash +Ġanalyt ic +ir am +Ġsymm etrical +Ġlifes pan +Ġe ater +ĠBloom berg +ater ial +Ġë¯ ¿ +Ġis ter +Ġinv aluable +Ġassist ing +Ġsh ack +μα ÏĦα +j is +en iz +ĠпÑĢед лож +Ġdecl aring +ĠV iking +ĠAss im +Ġexpend iture +Ġpos ing +ĠOn un +Ġin ic +аÑİ ÑĤÑĮ +re v +Ġm iedo +Ġfil thy +ĠI B +ĠDis cover +icht et +m illion +¶Ħë ĵ¤ìĿ´ +Ġamb igu +ĠF lynn +bard ziej +Ġinc omp +ав но +z ia +Ġinfluen cing +Ġworld ly +ĠSales force +z et +Ġparticul ier +ĠK och +Ġ194 3 +Ġton er +ĠÑįкÑģп еÑĢ +Ġsus cri +Ġtrigger ing +IC ES +ìĬ¤ ê°Ģ +δ α +ÑĢ Ð°Ð±Ð¾ÑĤ +Ġafter ward +p ine +ĠI L +are th +Ġп ал +Ġs aker +Ġ194 7 +A F +uy orsun +ĠìĬ ¤ë +Ġquant ify +Ġment orship +Ġll ega +ĠTam ara +Ġoptim izing +Ġfront s +os ters +Ġes quer +Ġsubm issions +Ġann ih +Ġsu ction +lu ence +chied en +ING S +Ġ×ij ×Ķ +ĠÑģ ÑĨен +Ġwiel u +Ġobjet o +Ġbo obs +ĠGesch äft +Ġear buds +ĠÑĢ Ð°Ð½ÑĮÑĪе +Ġrout inely +Ġcoll agen +од Ñĭ +ĠCin namon +Ġba ix +د Ùħ +f rage +Ġк ноп +Ġde ception +Ġunexpected ly +Ġsmell ed +Ġlo os +Ġhighlight er +Ġê¸°ë ³¸ +ĠGlas gow +ow ana +m n +ĠJ eremiah +ĠDat ab +iet e +Ġb aw +Ġprop ia +Ġprop ri +OOOO OOOO +ink er +Ġpert urb +ĠF ake +ìĿ´ì ĸ +im ming +Ġund ocumented +Ġtrabaj ando +Ġro am +Ġдолж но +Ġar be +Ġan i +at al +Ġar ada +ĠAnd a +ĠìĽ Ģ +ĠBr anch +o ires +Ġouts ider +d ollar +å½ĵ çĦ¶ +iss es +be ans +ĠG ig +çĿ ¡ +r ados +ĠS ut +ĠL ance +edsiÄĻ bior +Ġcol a +on ents +Ġrec onsider +ãĤ¹ ãĥĪ +Ġmond o +ãĥ³ãĥ įãĥ« +Ġuns uccess +ĠK ä +è¾ ¹ +Ġreg el +Ġbis og +et us +Ġun ravel +Ġsweet ie +Ġreprés ent +our ing +Ġground water +ĠBe w +Ġscratch ed +Ġcass ette +Ġc ider +p is +ĠÑģам а +Ġglobal ization +Ġdegrad ation +Ġde gener +ĠRos ie +ick t +Ġover weight +ĠM EM +Ġguard ians +Ġconse c +H mm +æĪij åľ¨ +ĠпоÑĤÑĢ ÐµÐ± +Ġme va +Ġgra ffiti +Ġfl irt +ĠB P +Ġjust o +ĠThous ands +çĶ ľ +٬ ìļ´ +. * +ĠRA W +Ġflu or +iy i +ant al +j ed +ĠSh eng +ĠEl ise +ĠChar ge +ìĿ´ íĬ¸ +Ġcon es +n ies +g ia +ĠнаÑĩ ала +ĠD harma +Ġëĭ¤ ìĸij +Ġf avors +ĠTr ung +het to +Ġpo zw +Ġlong o +Ġke lu +Ġdigest ion +ĠE ig +ĠTH ERE +Ġt iers +Ġs unk +Ġmyst ical +z ub +ĠÃī t +Ġanticip ating +ĠV ine +Y Y +Ġconcent rating +ĠAgre ement +Ġок оло +Ġlid t +ĠYa o +ĠÑģ лиÑĪком +r ÃŃ +ISTIN CT +ĠOFF IC +Ġso aking +Ġsi ihen +Ġrefer encing +ĠT ampa +ane y +Ġresp uesta +ĠCo alition +ĠÑģог лаÑģ +ank ind +Ġë Ľ +ĠY ummy +ë° ° +Ġon c +ui ção +Ġthe o +Ġm ural +ĠTeach ers +Ġwait s +Ġrent ing +ĠHar mon +Ġe ÅŁ +ĠMun ich +íĻ ľ +ìĸ ¼ +c ards +Ġrou ge +Ġn ên +cl ub +Ġun seen +Ġdep reci +Ġcomput ed +Ġwip ing +ĠEll i +ident ified +Ġcl utter +role um +Ġtele f +Ġlevel ing +ĠWo ody +ĠG us +ĠBenn ett +Ġsit io +i ÅĤ +Ġposs essions +ĠNat asha +old own +ĠÑģо обÑī +ĠL ic +Ġë§Įë ĵł +Ġlors que +we h +Ġм ам +l iter +ad omo +Ġfin i +Ïİ ÏĤ +ĠÑĥб ий +Ġind isp +Ġtele vis +Ġp á +ĠCre o +ÃŃ ll +Ġg ur +ĠM AL +ĠÑĢаз нÑĭÑħ +Ġzie hen +Ġfashion ed +Ġdeb ating +ĠS oup +ĠProv ince +ê·¸ë łĩ +Ġimpro per +Ġimag en +ĠÑģдел ал +Ġlog os +Ġevent o +è§ Ĩ +ả o +l arda +ĠназÑĭв аеÑĤÑģÑı +Ġver f +Ġscreens hots +×ķ×ĵ ×¢ +ĠAur ora +ĠB ali +ter ed +Ġcontag ious +Ġcompart ir +ven idos +ri ke +ĠвÑĭглÑıд иÑĤ +Ġfreed oms +nic as +ł¤ ìĦľ +Ġredu z +ĠE cu +Ġab onn +ĠSE Ãij +ĠB itch +Ġprojet o +иÑĩ но +ett re +AN NA +th ank +ĠA O +æīĢ以 åij¢ +arn ish +ie ÃŁen +Ġr ipple +Ġpant ry +ĠG H +γ α +ĠìĿ´ë²Ī ìĹIJ +Ġvalid ated +Ġbrush ed +ĠE min +ĠDar th +es in +, . +Ġv alle +Ġjer sey +ul an +R ead +ĠR angers +Ġso othing +Ġcomplement ary +ĠVer kehr +ac akt +Ġbat ht +ĠN D +S on +ĠíĻĶ ìŀ¥ +ĠA vi +ĠS AL +ais se +Ġsem aines +ĠSur v +w ier +Ġвид ел +Ġsi ete +Ķë ıĦ +ĠRams ay +ĠQueens borough +ĠM enge +ĠFood s +Ġthe ological +Ġ[ # +Ġв они +Ġim min +ios ity +ĠAb geord +ĠA cho +ĠÃ Ķ +Ġst ains +Ġreal istically +Ġfashion able +ĠCEO s +ĠSk ill +Ġв же +Ġde ver +ĠPl ug +æ ª +P od +Ġlo af +Ġge bracht +Ġabsor bs +ĠGr anny +Ġmal ware +ag ÄĻ +Ġcivil izations +ĠÏ ģ +Ġh ält +С Т +g reat +Ġlay ering +s ings +Ġв Ñĸн +Ġrecogn izable +Ġwo j +Ġwet en +第 ä¸ĢåĢĭ +γ ο +St udent +Ġdé fin +ple ase +en ch +Ġatt ic +ĠOt tawa +Ġopt ed +Ġcapt iv +Ġm ÅĤ +ĠY A +ĠW and +Ġb ounty +Ġ2 70 +Ġspec ulate +Ġenhance ment +Ġcommod ities +ĠMil ton +e j +al om +D as +Ġco oldown +ר ×IJ׾ +Ġ×IJ× ¤ +Ġwcze ÅĽniej +Ġel ong +Ġdi ode +ina ção +ĠI ris +ĠI b +Ġsummon ed +Ġres pe +ĠR ach +注 æĦı +Ġ» : +éĨ Ĵ +Ġv ur +Ġmov imento +Ġflu ent +ĠEv olution +ĠBut t +ific ación +ĶĶ ìĸ´ +ĠÑįн еÑĢг +Ġmanip ulating +Ġposit iv +м оÑģ +Ġw iz +Ġinto x +ÎŃ Ïģ +ем ÑģÑı +ives se +imiz i +Ġìļ ¸ +Ġknock s +Ġcongest ion +ĠIde ally +ĠHold ing +Ġpo bre +ĠJ UL +Ġë¶Ħëĵ¤ ìĿĢ +Ġα κ +ĠFergus on +ĠLabor atory +richt en +roph y +produ ction +ass ung +IT A +Ġsiè cle +ר ת +c ision +Ġפ ×Ķ +ĠIre ne +an ca +ĠìĤ¬ ê³ł +Ġpin point +Ġdesign ation +ÅŁ am +l Ä±ÅŁ +a at +ĠnÃ¥ gra +Ġmyth ical +ĠDec laration +Ġìŀ¡ ìķĦ +Ġby te +. âĻª +D el +Ġí į¼ +Ġnutrit ious +ĠÑĢÑĥб лей +åĤ ³ +S AY +M aster +ĠÑĦоÑĤ огÑĢаÑĦ +ĠëĴ¤ ìĹIJ +Ġne h +Ġdok ument +çª ģ +Ġczas u +Ġcontinu a +ĠSil ent +Ġtens or +Ġt anta +Ġirgend wo +ĠL ET +ĠSh akt +l ama +chl ag +Ġd ingen +ÑģÑĤ ÑĢа +Ġe hrlich +ĠM acht +rel s +Ãł cies +v ideo +Ġnatur ale +ĠSTE VE +um m +B ACK +Ġ7 20 +ãģ§ ãģĹãģŁ +Ġmom encie +ĠSw an +Ġtechn icians +Ġgee hr +ĠM end +R eg +Ġsca ff +Ġa ide +Ġë³´ ëĬĶ +Ġpress es +ler de +\ ' +Ġultras ound +Ġdisc laimer +ĠM its +ĠHol iday +Ġextern ally +ĠF ate +IN O +ĠC ats +ë° ķ +um o +cont rol +Ġthe CUBE +t ic +ier ungs +Ġзнак ом +Ġfre estyle +MAND ARIN +Ġis e +aur us +è¨ ± +ĠSt rategy +ĠBe am +rä ge +Ġexplo ited +ãģĪ ãģ£ +id is +Ġch ime +ĠPen insula +Ġmer its +Ġalt ro +ĠTO P +ĠS ens +ĠK ant +or as +Ġroyal ty +ĠID E +å¤ ī +r acy +ĠTH OM +om os +Ġläng er +Ġnumber ed +U m +ĠNi ye +θ η +zy ka +l ime +ĠPerson en +Ġvalid ity +Ġcont rat +ĠCom ic +ç ons +ĠHe idi +Ġz g +Ġren amed +Ġc umin +ĠJ F +in el +Ġenfor ced +Ġch ama +ли Ñĩно +Ạ» +Ġден ег +Ġprof und +Ġpel vic +Ġpalav ra +Ġext ras +Ġank les +ìĹIJ ìĦľëıĦ +ĠT F +Ġinsan ely +Ġм ÑıÑģ +Ġrép onse +Ġgö ster +ĠBB Q +ĠÑĥÑĩ аÑģÑĤ +Ġsh aken +ãĤ« ãĥ³ãĤ¿ +Ġalm onds +d ish +ĠP G +ĠBl izzard +ÑĮ ого +Ġ ãħ +Ġkn app +T oo +Ġund e +Ġmount s +ом ина +Ġnorth east +Ġcens orship +ÑıÑĤÑĮ ÑģÑı +l r +Ġlaw makers +ĠsÃ¥ dan +Ġins ider +Ġclean up +ĠN ada +ó c +Ġharvest ed +ĠDesp ués +íļ į +Ġredund ant +EN A +Ġdeleg ate +Ġbur g +ĠAl ison +æĸ° èģŀ +Ġcel estial +Ġsin ners +Ġmart yr +ĠP erm +Ġspec imens +Ġmit ochond +Ġmar avil +Ġcaval ry +Ġarray s +Ġanne x +Ġlabor atories +ĠBy z +Ġat ac +ĠÑģл ожно +Ġto pl +Ġger i +ĠCom bat +ÑģÑı ÑĤ +ek en +ĠÐĴ лад +Ġa just +Ġmar que +Ġlook out +ĠL ol +Ġrooft op +ĠOr ion +Ġб ой +Ġheart breaking +Ġdet to +z h +ät ter +c era +Ġhe ats +Ġant iqu +Ġunf inished +ĠK azu +ıl ı +Ġslight est +le o +ĠvÃ¥ ra +Ġverschied enen +Ġlot ion +ä½ł å°± +æĮ º +ÑĪ ÐµÐ³Ð¾ +ction al +ĠìĿ´ì ł +d ragon +Ġreson ates +Ġin m +av ic +Ġfulf il +Ġ기ë ĮĢ +Ġjust amente +Ġдо ÑģÑĤÑĥп +Ġê·¸ ê±´ +Ġrecon cile +ĠSch ön +ĠAvo id +ê¹ Ģ +' D +Ġconf inement +Ġí ij +Ġmotiv ating +ĠBritt any +Ġãģ Ļ +Ġscream ed +ob ject +Ġdec ree +Ġtrava ille +iss ible +Ġb usted +pro cess +Ġmass acre +Ġngh Ä© +ily n +Ġв ÑĢоде +Ġpo etic +Ġnh ất +Ġiron ically +us u +n io +Ġst aging +omed ical +le ased +ĠìĥĪë¡ľ ìļ´ +ĠN Z +act ing +ĠBattle field +play ful +V i +Ġseñ ora +Ġprompt s +lich keit +Ġçık ar +ji ang +Ġpick y +ĠC ave +Ġmirac ulous +ĠHugh es +20 16 +Ġx u +ĠDor othy +Ġvirt ues +Ġret ract +Ġty r +Ġchar ismatic +Ġb ola +é ¼ +Ġë§IJìĶ Ģë +Ġparent al +Ġmillion aire +ari at +æĶ¿ åºľ +Ġinv oke +żen ie +Ġextrem es +ĠA ku +ivid ade +Ġï· º +Ġìĭľ ì²Ń +ĠGar lic +RI A +Ġд оÑģ +ĠP ont +Ġmil j +ell i +Ġrack et +Ġcompet it +ĠWh is +Ġreal t +ign ment +est re +Ġper nah +ĠOp ening +ĠF S +ĠDemokrat en +ac ements +Ġworld view +Ġplay offs +ĠC AD +Ġét ant +Ġyem ek +Ġsent iments +od el +b uster +a ÅŁ +ĠK Y +cz ÄĻ +Ġschö ne +a pe +ĠR aspberry +Ġcred ited +ĠH idden +Ġsaus ages +ru ce +ĠBe v +ilant ro +Ġpoke mon +Ġê°Ģ 격 +Ġproceed ing +Ġve io +Ġ17 5 +è ¸ +ma x +Ġfr ater +ìłĦ ìĹIJ +Ġe gent +Ġ25 00 +us ch +T ube +Ġampl ify +Ġpraw d +Ġod or +ĠSc an +Ġplot ting +ithm etic +Ġres igned +ĠSC OTT +Ġstere oty +Ġdo able +ĠCom plex +Ùģ ÙĬ +t ım +ÑĢи г +l ardan +es o +D EN +Ġhood ie +ĠC AT +Ø§Ø · +Ġbond ed +ĠBurn s +оп аÑģ +Ġr ÄĻ +ει α +ĠоÑĤд елÑĮ +Ġtim eless +ĠV ij +ĠPan ama +Ġre organ +ĠT ä +ĠPl uto +O range +Ġп ойд +ĠBr istol +u ced +ĠëIJĺ ìĸ´ +Ġun bedingt +ad le +Ġvolunte ered +Ġm ieli +ĠEdin burgh +ik al +Ġal ten +ĠAr sen +Ġmouve ment +Ġant ique +Ġb h +ĠH ers +Ġsa ute +Ġasp ire +Ġsp heres +ĠW am +ắ m +Ġwip es +Ġ2 80 +ĠVe h +Ġcol oca +а ÑĦ +Ġвозмож ноÑģÑĤÑĮ +Ġphysi ological +h wa +et u +Ġprolong ed +Ġexperi ência +Ġвид но +Ġquar ant +Ġpued an +è Ķ +v ine +ĠUS DA +ph em +Ġform idable +Ġfl atter +ìĸ´ì §Ģ +Ġb én +à¹ģ à¸ķ +Ġë¬¼ë ¡ł +Ġfact ions +ĠLe aving +Ġ×IJת ×Ķ +ĠExper t +d io +ĠVer d +ãģ¿ ãģŁãģĦ +Ġs int +ÙĨ د +n umber +Ġow ed +Ġindu ce +ĠFred die +ab o +ĠFilip ino +¯ ¼ë +believ ably +ath lon +ama an +Ġde venir +ĠG os +ĠJen kins +b ait +Ġb ins +ĠM ICH +u yorum +ig rade +is so +ĠìĹ ´ +ĠìķĦë ¹ł +Ġdiarr hea +Ġtorn ar +ad din +Ġungef ähr +Ġrest room +Ġpsychiat rist +ĠKick starter +Ġg era +Ġal red +ĠW rap +ÏĮ Ïĥ +Ġsin ner +CH EERING +Ġkil ow +Ġdetermin ant +Ġdem onic +id ences +ch as +ĠD ed +å¼ ķ +Ġst umble +ĠUr s +Ġdece ived +ĠT ER +ĠC ó +ell ed +Ġnot wend +Ġì§Ģê¸Ī ê¹Įì§Ģ +Ġpart ido +Ġdesc ended +Ġvard ır +Ġenact ed +ĠczÄĻ ÅĽci +å·¥ ä½ľ +Ġtra inees +Ġaud ible +Ġm alf +Ġve o +ì n +ĠG PA +ĠApp e +åĤ · +Ġr ut +ĠCar la +k ach +Ġsav ior +itch ed +Ġclim ax +аÑĤ елÑı +ĠMc Connell +ол Ñı +ere ye +ĠÑģоз н +Ġcab o +ĠS ne +ĠAff ordable +Ġsar Ãł +Ġlegitim acy +Ġscar ce +... </ +Ġ10 8 +Ġac um +ĠFrank ly +Ġradi ator +Ġgener als +Ġdivid es +Ġcheese cake +Ġsor cer +Ġmiscon ception +Ġhardship s +ĠOne Plus +üy orsun +ĠSovi ets +ĠItal ia +ick i +ĠAfter wards +Ġridic ulously +Ġgdzie ÅĽ +ĠNot es +Ùĥ اÙĨ +Ġr oman +Ġorganiz er +Ġcour tyard +ĠÑĩелов еÑĩ +ĠW itness +Ġп ÑıÑĤ +ĠCh ill +ĠVal ve +Ġά λλ +ĠK P +chl uss +Ġdef lect +ĠTon i +Ġcl air +Ġstack ing +ä½ İ +ras zam +ĠSon ra +ãģ£ ãģ¡ãĤĥ +ĠAt ari +Ġpas ó +Ġchar ms +an st +Ġter ce +ĠL illy +Ġpsych ologically +ĠcÅ ĵ +ust e +¥ ´ì +CT V +Ġm iel +çļ ĩ +C are +ĠâĢ ij +Ġsna pped +ãģ© ãĤĤ +Ġê° IJë +оÑĤ Ñĭ +Ġm ês +. ? +Ġton nes +×ķ×ĵ ×Ķ +à¸Ħ à¸Ļ +T u +Ġdistrib uting +Ġcrack ers +Ġcor ação +äm än +ä½ł åľ¨ +cl amation +оÑĢ Ð´ +ĵľë¦´ ê²ĮìļĶ +ĠUnters chied +F ine +ck o +ĠÑĢеб ен +Ġsp ic +Ġdoctor al +ĠÑģкоÑĢ ÐµÐµ +un ivers +ac ula +ĠÃĸ sterreich +Ġgr inder +Ġamb os +Ġvast ly +éĢĻåĢĭ æĺ¯ +Ġconf essed +ĠSh h +and ers +ĠGu an +ĠнеобÑħод имо +Ġchampions hips +ĠV ul +ĠPh i +ĠMe asure +æľ ¨ +Ġins gesamt +æħ¢ æħ¢ +v ette +Ġgen om +ind ung +g li +D et +Ġunm ute +ãģ¾ ãĤĬ +Ġsau ces +ĠD w +×ij× ª +ĠB RE +Ġnurt ure +Ġdet ained +ĠBe er +Ġми ÑĢа +в е +ĠBird s +Ġmeille ur +Ġre wind +Ġp ore +×Ļ× ĸ +é ger +qu ela +Ġtrous ers +Ġsi inä +ĠG aga +ĠBR AND +le ben +Ġr aspberry +ä» ĺ +il ik +Ġvers ão +l ak +Ġlo gar +ĠMID I +ĠìľĦ íķľ +ĠпÑĢоиз оÑĪ +Ġster il +Ġhar med +ав лив +ĠÑģ ÑģÑĭл +Ġlack ed +Ġcontact ing +Ġ기 ìŀIJ +Ġgef ähr +Ġco y +ike l +Ġb inge +Ġorthog onal +Ġentend u +ĠTh irty +Ġsmart est +å¤ļ å°ij +Ġr asa +ĠQu á»ijc +Ñĭв аÑİÑĤ +Ġsl ut +л ÑĥÑĩ +ig ten +ĠÑĢ Ð°Ð± +Ġt aman +Ġqual idade +Ġdom ination +Ġsin us +Ġprogram mers +Ġaller gy +ĠTor res +ĠAust rian +n ants +å®Į æĪIJ +M el +ĠÑĥв елиÑĩ +ĠA gg +Ġso k +Ġpl uck +Ġbind s +Ġprop or +ĠM af +Ġoso b +ĠV IC +é ¥ +ĠзаÑĩ ем +Ġexhib itions +Ġett i +c za +ĠнаÑĪ Ð¸Ñħ +ĠM itte +обÑĭ ÑĤи +Ġclock s +Ġr ico +æĶ » +ĠиÑģÑĤоÑĢ Ð¸Ñı +Ġsch izophren +Ġfl uff +ĠÑģоб иÑĢ +Ġap oy +Ġprin ces +Ġbr aces +ĠF IR +ĠS na +Ġ; ) +ven es +Ġvuel ta +Ġm ies +Ġbro om +Ġmer ry +Ġespecial mente +ĠAl ban +ĠпоÑģÑĤоÑıн но +ĠL ena +ĠC ult +al so +Ġquot ing +Ġgen ere +ĠY ar +ĠL age +Ġdem ost +Ġd age +ĠEcu ador +Ġan vänd +u ÃŁen +Ġë°Ľ ìķĦ +Ġpsych ologists +ĠL ars +Ġposs a +Ġout going +Ġmet ic +Ġbag gage +er ia +Ġricht ige +ìĭľ ìĹIJ +ĠÑģоÑħ ÑĢан +Ġroot ing +Ġdro plets +çļĨ ãģķãĤĵ +Ġnas al +ĠCo x +X i +Ġdispos able +Ġbut cher +ĠZ ar +ĠArmen ian +Ġë¿ Įë +ĠF ool +ĠCB D +Ġs ost +Ġper ish +ĠR ép +ç´ ° +ãģĿãĤĮ ãģ§ãģ¯ +ĠFre ud +Ġf andom +Ġblo que +Ġinvent or +Ġab re +Ġénorm ément +Ġimport s +é Ī +Ġot ur +ĠRy u +ĠâĨ Ĵ +Ġsecond o +Ġincom pet +Ġincarcer ation +Ġasc end +b ene +åĸľ 欢 +Ġol urs +no ch +Ġbre eds +ли з +ĠVerf üg +Ġma iling +re ally +Ġes f +Ġpe le +Ġle ash +Ġdis ks +Ġзам еÑĩ +ìķĦ ìķĦ +ab outs +ĠM ull +ĠD ent +edere en +D rive +Ġt ipping +Ġnig ga +ord um +Ġpor ter +Ġkara oke +Ġdocument aries +ĠR IGHT +ĠP urd +ĠоÑģÑĤ ан +к лад +é rence +Ġê± ¸ë¡ľ +ĠÑĤ оп +ĠW ong +ä¸į 对 +ĠпÑĢ Ð¸ÑĢ +Ġnom inal +Ġa ula +ĠÑįк ÑĢан +Ġcher che +ĠTh r +åħ¶ å®ŀ +Ġla ufen +ĠKath leen +Ġreact ors +ih at +Ġs ided +ĠSim one +Ġguid eline +import ant +b umps +t one +Ġentre prises +Ġconst itute +osc ope +ĠMyst ery +cy cles +ĠWars aw +Ġburst s +ĠZh ong +å®Į äºĨ +ĠSAR AH +ĠëĬIJ ê» +é į +Ġbe acon +åį ĩ +AD E +Ġì§Ģë Ĥĺ +Ġ ersch +Ġinteg ers +ĠCross ing +s ource +Ġschool ing +ĠR OM +ator ium +ĠìŀĪ ê²Į +Ġr ôle +Ðķ ÐĿ +Ch at +Ġshr inking +Ġreim burse +Ġl umber +ü cks +Ġsal ah +M other +Ġk ali +ĠQ atar +ot ional +Ġop acity +Ġne e +ĠC ory +Ġì¸ ¡ +Ġturbul ent +z ers +ĠÑĤ еÑģÑĤ +Ġéc rit +Ġë³´ íĨµ +Ġdisgr ace +Ġì¹ ´ +Ġcourt esy +ing a +Ġhug ging +ĠA BS +m ith +Ġins ufficient +Ġcro oked +Ġê·¸ë ĮĢë¡ľ +ìĭ ¤í +Ġsim ulated +ĠëĦ¤ ê°Ģ +Ġb ö +ĠOt to +L ING +Ġillust rates +ĠDest roy +Ġ196 1 +ĠT agen +Ġmel on +ĠP ascal +Q UE +ĠполÑĥÑĩ иÑĤÑĮ +Ġinc idence +ĠSteven s +ĠG ins +r ue +Ġunre asonable +ĠJ ie +ys ics +Ġ몰ë Ŀ¼ +Ġfish es +© ´ì +Ġprec urs +Ġmog ÄĻ +t ight +et é +Ġmund ial +ìĹĪ ëĭ¤ +â̦ ! +B U +Ġsoci ology +Ġbrut ality +Ġperson aje +Ġn ÃŃvel +Ġfaz em +Ġess en +Ġd welling +Ġcommer cially +Ġed its +Ġd ues +ĠG SA +ìĿ¸ ê°Ģ +ĠíĹĪ íĮĿ +ĠYah oo +ен еÑĢ +ìľ ¨ +ÑĥÑĪ ÐºÐ¸ +le ft +Ġcapt ive +cip her +Ġ×ŀ× ŀ× +ĠгÑĢ Ð¾Ð¼ +Ġinn ate +Ġimp ul +ĠìŬ ìŀIJ +Ġswallow ed +ĠTab ii +ìĿ´ì ĭ +ĠÑģо ÑģÑĤав +Ġoy un +Ġobrig ado +ĠA ph +K atie +Ġc ena +ĠAll Äģh +ÙĪ Ø³ +Ġprzy p +Ġpe pt +Ġvolunt arily +ĠO ÄŁlum +ĠE lo +ou e +B ir +bur ger +ĠS BS +Ġ6 000 +Ġpromot ional +ĠHerr n +Ġstamp ing +Ġqual ifying +Ġcos mos +Ġaf ar +æ± Ł +ab us +Ġdad s +ãģŃ ãģĩ +ĠÑįк оном +inc arn +Ġìĸ´ë Ķ +Ġл еж +ĠB ET +Ġнай д +on ter +Ġreus able +Ġkomm a +ĠB ij +ĠTer az +ĠOl á +ĠìķĦ 침 +ĠÑĢаз меÑĢ +aw an +Ġcart a +æIJ ŀ +ic eless +Ġsm e +ĠTut aj +ĠÈĺ i +Ġprob ation +Ġadequ ately +ĠPresident ial +ind ruck +bl ade +Ġve ulent +Ġc ioè +åĮħ æĭ¬ +Ġrever b +Ġgegen über +ĠEsper o +Ġbe ge +ĠSTUD ENT +s ound +ĠD ü +Ġoff end +Ġ" .. +ken nt +ĠÑģл ÑĥÑĪ +Ġpurp osely +ĠL it +ĠíĽ ¨ +uch er +Ġh ina +ý ch +ign on +TH E +Ġgl ide +our cing +ĠØ£ ÙĨا +Ġoll ut +Ġarch ety +Ġsh ady +Ġs omm +Ġep ile +Ke ep +Ġnaj bardziej +ठķ +itution al +Ġм ай +Ġsin ful +ĠBron x +Ġгл Ñĥб +Ġv am +Ġpres ets +ĠD ag +ĠìĻĦ ìĦ± +Ġc reek +it ures +ĠLord s +ö tt +UN T +R a +Ġinequ alities +Ġcoll ateral +Ġwr ists +Ġgroup ed +Ġоб ÑĭÑĩно +Ġarm ored +Ġt ung +Ġconver ge +Ġb ok +ĠD odge +нÑı Ñı +Ġfle eing +ĠMartine z +ĠDream s +ke k +Ġsocial e +ĠPla za +د Ø© +Ġke ll +ĠSt ellen +f elt +ĠÑģп аÑģ +ĠP v +Ġcan ción +ĠH ert +ĠBal ance +Ġsel ves +Ġv andaag +Ġpr y +Ġnaj le +Ġвид иÑĤе +Ġvel vet +Ġgro ot +Ġf out +æ¨ ¡ +ĠSchul en +ĠMoh ammed +ĠCent ers +Ġha ver +Ġfre uen +¤í Ĭ¸ +л ан +P OS +ink i +Ġëĭ µ +Ġparaly zed +GL ISH +Ġcast s +ĠV C +ìĿ´ì ħĺ +Ġت Ú¾ +ç¥ ¨ +Ġì¤ ĺ +Ġר ×ķצ +Ġsu ced +Ġprogress es +ĠE ÄŁer +°ë ıĦ +Ġinstall ations +ped o +еÑĢ Ð± +inter pret +Ġê³łë ¯¼ +ĠAzer bai +ivid ades +Ġì£Ħ ìĨ¡ +Ġent fer +Ġchw il +ĠHer bert +ĠAlexand ria +y ty +Ġse chs +Ġcal iber +ĠWe ise +ĠHe ck +ĠY ug +ĠاÙĦØ · +Ġpes ar +Ġcig ar +Ġm él +Ġha ird +Ġprzypad ku +Ġconfident ly +Ġan arch +ĠG ian +Ġdo bre +c jÄĻ +aw y +ĠRe ce +ĠGob ierno +Ġcar ga +um sy +Ġn orte +Ġhand ler +Ġrespect ing +Ġall ied +ĠP iet +icht lich +Ġold s +Ġdust y +Ġg ry +Ġ- ... +GH T +Ġne o +Ñĩ ики +еж д +a ide +ĠбÑĥ ло +í į¼ +Ġtempor ada +Ġd oute +âĺ Ĩ +ĠìĪ ł +ĠJ USTIN +aut o +Ġration ale +pro b +Ġfish y +Ġdoor way +Ġempt iness +ен наÑı +Ġbra g +ĠÐĵ де +çĪ ¾ +Ġtrans ient +Ġmitt lerweile +ĠB ret +Ġf ij +Ġdepos ited +N S +Ġìķŀ ìĹIJ +Ġkim se +Ġchar ities +ĠMill enn +dog s +Ġmo yen +Ġnue vos +ĠCook ie +par able +do ing +ĠS ail +Ġ icy +h aba +Ġque ens +Ġchocol ates +ĠN ay +ĠÑĦ ин +Ġve c +Ġhelm ets +T M +ĠAr med +Ġimpair ment +ĠT us +ĠM ême +ome z +ĠRe qu +ĠInvest ig +íİ ĺ +Ġgol pe +ĠR ac +ig raph +Ġk west +Ġsail ors +Ġstatut ory +Ġmil estones +ĠM ash +ĠGesetzent wurf +é Ĭ +Ġcol oured +h uma +Ġy ere +Ġsubtit les +Ġembod ied +Ġmiss chien +ĠiP h +üt zen +Ġdet ached +Ġdescri ção +ci amo +Ġreco il +ĠÐŃÑĤо ÑĤ +Ġexport ed +ĠAl one +ant ry +Ġest an +ĠS od +Ġlavor o +æĬĬ å®ĥ +ר ×ij +ĠÄij á»ĭ +Ġsw ag +ĠPC B +ĠK aiser +ĠMod er +j ug +Ġtext ile +T w +Ġn ac +f rei +Ġret ard +isc ern +Ġtall est +ĠLu ca +R ah +Ġpre acher +Ġj ut +ĠR ica +ic iency +ĠÄiji á»ģu +Ġk aufen +Ġnet t +Ġdisc ut +Ġdepri ved +¡ Ń +Ġsp richt +Ġencl osed +ĠSub st +ç§ ij +ĠRab bit +pr ised +Ġbit ches +ì Łģ +çī Ī +Ġtap a +ĠEs sen +ĠBa o +Ġdev ient +ĠW uhan +ĠT ipp +Ġdis ast +ÑģÑĤв Ñĥ +ubl ique +Ġqual ité +Ġinadequ ate +Ġbarg aining +ĠGot cha +ев иÑĩ +iev ous +ert on +bl ue +ĠìĽĢ ì§ģ +Ġsand box +ĠRe in +è¦ ª +ĠìĿ´ê²ĥ ëıĦ +Ġsa x +z ogen +un ächst +Ġher kes +Ġ- , +zen i +r ising +Ġresp osta +Ġpromot ions +ĠUnter stüt +ĠM AS +N othing +ot ics +ĠвÑĭ й +Ġrot ates +k ien +Ġhab la +ĠDan i +un ion +Ġw ack +Ġarchae ological +ĠCurt is +ĠHor iz +Ġê³ ¨ë +Ġwai ver +åĺ ¿ +B on +Ġrot ated +Ġpitch er +Ġin ad +Ġhug s +ĠNorth east +×Ļת ×Ļ +Ġple a +Ġcup cake +ĠL Y +Ġfam ili +Ġgro o +ĠBla ir +Ġli j +Ġhabit ats +Ġcommun ism +os ium +b ars +ĠFre eman +ne o +Ġdiff use +Ġcylind ers +ĠDe bat +íĸĪ ëĬĶëį° +еÑĪ Ðµ +Ġfinger prints +Ġam ar +в ид +ĠìłķëıĦ ë¡ľ +Ġaffili ated +ĠÑħоÑĩ еÑĤ +ãģ° ãģĦ +Ġet iqu +Ġch ÃŃnh +æģŃ åĸľ +Ġcru ising +ĠWe ihn +çĶ µ +ĠTitan ic +ç´ Ģ +ĠN ast +Ġëĵ¤ ë +Ġв ал +Ġdem i +ĠKrist in +M IN +Ġrig or +Ġmot o +ĠL AKE +ĠíĻ ľ +Ġë§Į ìķ½ +ĠSt ro +Ġprot otypes +ĠL C +ìĿ¸ ìĿĦ +ÑĢ Ð¸Ð¼ +Ġviol ating +Ġgi orno +Ġchild ish +æ° Ķ +Ġ×IJ×Ĺ ×ĵ +Ġoverd ose +ag ogue +ад ÑĨ +he us +ĠговоÑĢ Ñı +Ġinc r +Ġdeb ated +Ùħ ÙĦ +Ġch icks +Ġqu in +LAUGH ING +Ġtight ening +Ġsupervis ors +ĠHaw k +ĠB az +Ġпов ÑĤоÑĢ +Ġбл ок +Äģ n +Ġdump ing +Ġfact o +ber ger +Ġarsen al +ĠAfric ans +¡ Ģ +Ġcafeter ia +fe eding +qu ila +ĠpaÅĦst wo +ı nt +Ħ ± +Ġenvironment ally +Ġdes prés +ĠWill y +ĠPaÅĦst wo +ĠG G +Ġch acun +Ġdirection al +Ġh ört +Ġ ðĿ +en ary +Ġvo iced +a ģı +Ġp ope +Ġcom rades +ĠGib son +ĠAC C +v ik +Ġmod elling +Ġag gi +ãģªãĤĵ ãģ§ãģĻ +Ġconvers ions +Ġaver ages +E llie +Ġgest ellt +ĠU E +osa ic +ÐĴ оÑĤ +S ay +ĠÑģам ого +Ġmes ures +is iert +g asp +vo ice +Ġcheck point +Ġpercent ages +Ġdisrupt ed +ĠT uc +ĠH omer +ĠW AY +ĠTur ks +he en +im oto +ĠO C +ÃŃ na +z iel +Ġmud ar +ãĥIJ ãĤ¤ +ges etzt +Ġmej ores +ĠC J +на ÑĢÑĥж +Ġmod ulus +Ġmod ulation +Ġrepl ies +Ġlar va +Ġg ider +ĠMand arin +ĠпоÑģмоÑĤÑĢ Ð¸Ð¼ +Ġsacrific ing +Ġpre ço +Ġoy sters +ĠMy an +olog ue +ĠW it +Ġd û +ĠLe uten +Ġp ater +ĠKENN ETH +аб аÑĤ +arth y +Ġsocied ad +Ġni ño +ев ой +Ġj ÄĻ +Ġadvert ised +ĠPep si +ute ur +Ġmas se +Ġsc attering +Ġy ön +Ġdesap are +ĠHub ble +ĠH é +k rä +ĠD are +Ġover ride +ĠEl aine +ĠDub lin +du llah +M at +ĠG arr +... ' +Ġadul thood +E Z +Ġbelang rijk +ien za +Ġun iverso +Ġstell ar +íĶ Ħë +Ġê²° êµŃ +Ġconstell ation +ĠShell ey +Ġmult it +Ġmasc ot +Ġhospital ized +Ġ ðĿĺ +оÑĢ Ñĭ +ad ia +ĠMike y +ĠAmer ika +Ġhair y +H old +ắ n +k iego +è§ Ĥ +à¹Ģà¸ Ķ +Ġrival ry +ĠJon ah +Ġsurge ons +Ġrelat able +è Ĵ +Ġswim s +Ġbillion aire +mod ern +Ġdocument ing +ĠDa e +Ġsw atch +Ġpu isse +Ġmas uk +Ġmar c +Ġk ró +ĠPeters burg +ĠArist otle +ix e +P rodu +Ġн ими +Ġk ana +ĠÐ © +Ġvom it +ĠWork ers +pop ular +ĠBie ber +еÑĤ и +ét ique +Ġenc ant +gr an +f ir +Ġanth em +ÑģÑĥд аÑĢ +L ast +Ġha g +Ġvic inity +rench ed +and ing +Ġгол оÑģ +ĠCor ner +ÐĴ Ñĭ +os as +ie vers +c ional +Ġvig or +Ġrejo ice +Ġci Äħ +Ġк оп +Ġqualc osa +dess us +Ġе в +ĠSc andin +ĠS mooth +ä½ł 说 +ha pe +Ġëĭ¬ë Ŀ¼ +ĠT U +Ġly ric +Ġb ess +é IJ +ÑģÑĤÑĢÑĥ менÑĤ +ĠAct ing +ĠOr chest +é cole +Ġdo lor +Ġíĭ ° +Ġverg essen +Ġeyel ids +ĠT anz +веÑĢ Ð¶ +Ġìķ łë +u é +Ġsc ène +Ġìļ°ë¦¬ ëĬĶ +Ġcr ate +k ick +ĠThe me +Ġ3 20 +Ġgarn ish +Ġmet re +Ġconve x +pl ants +es ian +Ġê±° ì§Ģ +Ġmé di +ĠMed al +1 30 +ĠAl ma +æľī é»ŀ +C ola +ĠваÑĢи анÑĤ +Ġg ord +Ġav anz +Ġwhis pering +Ġintest ine +Ðł Ðķ +ĠL ISA +am ız +S PD +Ġpe c +Ġpast ors +Ġmu á»ijn +oc re +S un +ĠÑĤак ÑĥÑİ +Ġrev ital +Ġincom es +Ġdetail ing +ĠB acon +Ġëħ¸ë ŀĺë +Ġpar rot +Ġcollabor ated +hes ia +Ġse va +Ġphysic ist +ĠB ACK +׾ ×Ļ +Ġbip olar +Ïģ εί +c ros +Ġk ed +Ġeconom ical +Ġend ings +Ġtick s +Ġê· ¼ +ĠOl iv +ong s +Ġcontin ental +Ġweiter hin +Ġactiv ating +Ġpoll en +ĠAn k +b ay +Ġ×ľ× Ĺ +ĠEgg s +ĠRAM SAY +ĠB ER +ĠíĽ¨ ìͬ +Ġpass ado +Ġground breaking +pres a +Ġhil ft +ĠTechn ically +ÑĨи й +N I +Ġturn out +ĠL ap +ĠG wen +ĠV ikt +Ġesc ola +ĠCin ema +æ° ¸ +Ġãģ Ĩ +Ġconsum o +ĠPurd ue +Ġse manas +ĠPRES ID +ư ng +Ġs ach +æĢİ麼 辦 +Ġsav age +ĠR W +Ġ5 50 +bo ld +ĠSim mons +Ġsl ang +ĠNar u +ĠThe o +íĸĪ ëĭ¤ +. � +Ġseiz ure +Ġh ive +Ġcell phone +å¥ ¶ +ii ii +ĠMus ical +ĠN uclear +è¡ Ĺ +á veis +Ġprest ige +Ġbal m +Ġref ill +y ah +h art +Ġt aps +Ġdisp ose +ĠM ick +Ġtherm ometer +ãģª ãĤī +Ġobed ient +Ġinform ações +ĠW ide +m om +S ud +Ġsusp end +ĠObs erv +Ġл еÑģ +Ġtr atar +ĠKat rina +Ġth eres +äº ŀ +Ġtext ed +Ġst ör +Ġsna il +ĠF iona +Ġvict orious +Ġlibr arian +pr act +Ġfin o +ĠAr ms +pp t +l uk +Ġty res +Ġto c +ĠKommun en +ç¯Ģ 缮 +Ġrev olt +Ġmotiv ates +Ġb isexual +Ġw us +Ġhand lar +ĠMU ELLER +Ġexpect ancy +Ġem body +ĠPrim ary +åİŁ åĽł +ÑĢ ÐµÐ¹ +Ġuns crew +i antly +, â̦ +Ġsn el +Ġpreval ence +Ġeru ption +Ġdescript ive +v ag +ĠбÑĥк в +Ġm êmes +Ġeth n +Ġhij os +ĠAbd ul +ĠZ ahl +b elt +Ġgö st +ĠTheres a +ĠS UN +ĠB ake +Ġ å¿« +Ġopt ics +Ġap ocalypse +p urpose +Ġróż nych +Ġcr us +ĠÐĹ ÐµÐ¼ +Ġhard ened +ĠT D +Ġgra veyard +ĠSi ber +ĠPor ter +Ġexpl odes +ĠSo fia +ĠÐĴ едÑĮ +Ġweak ened +æĺ¯ æĪij +UL L +Ġpink y +Ġchap el +ĠF res +ĠпÑĢи г +M ER +ĠSch midt +ĠD ud +æŁ ¥ +est ens +Ġnu ance +Ġmod ifying +ĠMöglich keiten +ĠAn at +Ġecc entric +ĠSc rew +ĠLe h +Ġhom ogeneous +ĠT all +ĠRic ardo +à ļ +ign s +Ġли ÑĪ +Ġgef ragt +R un +c aster +no ise +Ġas ynchron +ÄĻd zie +Ġ×ŀ× Ĺ +Ġsupp ressed +Ar thur +ή ÏĤ +â r +d ist +Ġк ад +Ġh ör +Ġ13 5 +ĠMoz art +ĠÑģ обÑĭÑĤи +ĠNurs ing +ĠH ahah +ĠD op +Ġpolic eman +´ìĹIJ ìĦľ +Ġê´Ģë ł¨ +hy uk +Ġrug ged +Ġnug gets +ĠComm s +St ud +ĠÑģв ое +Ġczas ie +ãĤ ½ +Ġrég ion +Ġfisher men +ĠL T +à ĵ +cia ż +he i +Ġcr umbs +ĠIm mer +ĠF eld +th ese +Ġadvertis ers +Ġro aming +Ġfun niest +ĠN YU +Ġhe he +Ġp oking +ĠìķĪë ı¼ +ist ical +Ġop aque +u ç +w ire +ĠWe ber +ĠJac ques +Ġ2 10 +ü p +uy u +Ġenfer med +Ġbump ed +ĠS ew +ĠChan el +Ġpersön lich +Ġbetray al +Ġallevi ate +Ġv ähän +Ġguess es +ĠC eline +ass ing +stro ke +Ġì¡ °ë +å¤ ı +ĠÑĤеÑħ нолог +Ġо ÑģÑĤÑĢ +Ġso ient +De ar +Ġj s +Ġges prochen +ath i +ç¿ » +Å¡ e +S et +og er +ĠR ig +Ġм еÑĩ +Ġserv icios +ĠR ut +ĠÐŀ й +ĠMyan mar +if ie +Ġsna pping +ĠKam era +Ġfest ive +ĠF Y +ĠCaro lyn +Ñĸ б +Ġlegg ings +Ġy at +Ġer gon +Ġepis ód +Ġanom aly +uest os +I d +Ġevac uation +Ġgig abytes +Ġand are +ĠR ent +m t +ist ine +Ġest rat +ett u +Ġrece ber +Ġdram at +ric ular +aln ız +ĠSen i +Ġo yn +ĠChem ical +ĠÑģ Ñħ +Ġtur f +Ġ19 17 +iscern ible +Ġmant ener +Ġexc er +Ġspect ral +Ġneuros cience +Ġmicro f +Ġforeign er +ĠL anka +ä½ł åı¯ä»¥ +ĠÑĤ воÑĢ +Ġtoss ed +Ġpobl ación +Ġmate ix +Ġsie llä +Ġot t +Ġcomp uls +ak ukan +Ġmanifest ed +Ġìĵ ¸ +Ġut most +Ġrevers al +Ġplace bo +Ġbl at +ĠSt unde +m anship +Ġatt e +ĠìĨĮ ê°ľ +Ġist em +Ġann at +ĠPlay station +Ġz ad +Ġqu itting +Ġfam ine +ĠR ough +ĠFl ame +Ġhe ut +Ġoportun idad +Ġfais ait +ĠD P +Ġdic iendo +ĠMel anie +ĠCar ne +m eg +pet to +J UN +ĠлÑİб ой +Ġo ste +ĠJJ onak +Ġtheat rical +Ġinv inci +Ġcommun ion +voc al +E h +ĠDet ails +Ġst roll +ĠRay mond +ĠAm elia +ij ¥ +Ġprodu kt +Ġnue vas +Ġmust n +may ı +col ored +de c +Ġhj äl +Ġsentiment al +Ġreal ms +Ġk rit +Ġse xt +ĠPsych ology +èĪ ī +h il +ĠкоÑĢ Ð°Ð± +ĠëĤ´ ìĿ¼ +ĠUnder stood +ĠG uten +Ġgang s +Ġeven ings +æĢİ æ¨£ +E nt +ĠLeg acy +ĠCong o +Ġdurch aus +Ġbu oy +ere lla +W AN +P re +ĠÑĢ ÐµÐ´ +ĠCr isis +ãģª ãģŁ +ĠìĿ¼ ìĿ´ +Ġmanuscript s +еÑĤ ÑĢ +Ġnon profits +Ġdict ator +Ġbask ets +ĠIs h +Ġper to +Ġdatas ets +Ġam ple +geb aut +Ġcontrib utor +Ġc iao +Ġconfir ming +ĠUC LA +âĻ ¬ +ĠÑģ н +Ġovert urn +åIJ ī +Ġunreal istic +ĠPie ce +oc ate +Ġf ällt +po x +Ġë³´ìĭ ľë©´ +Ġë© Ķë +ĠCre ation +Ñİ Ð´Ð° +Ġ×Ķ× IJ +Ġwh ack +olith ic +c ely +ĠÑģов ÑĢем +Ġsequ ential +Ġprofes ional +Ġcool s +Ġrep ente +Ġa ire +enn es +rit os +ĠÐĴ ид +Ġk ör +ĠB itte +ul ars +Ġincorrect ly +Ġshar ply +Ġbomb ard +ëĭĺ ìĿ´ +Ġchromos ome +Ġadvertis ements +h un +ĠÑī об +ĠÐĶ Ð°Ð¶Ðµ +Ġbatht ub +ĠS no +ÙIJ Ùij +Ġbuff et +ĠGr id +ĠB rew +is et +ĠImport ant +üm üz +Ġvet o +ĠW erk +ĠSh am +k ra +ile en +he ard +Ġdra ining +Ġkl ass +Ġbak ayım +ct ure +ä½ł 說 +am our +Ġspons orship +Ġdist ill +Ġpat io +Ġk omb +Ġoverwhelming ly +ĠJama ica +uit en +L ittle +ĠL OT +ta Äĩ +Ġcommand ers +ĠWat ts +ĠO ptions +ìĿ´ë ©´ +AC T +Ġindisp ens +ĠF orsch +ot om +ĠÎŃÏĩ ει +Ġpra ising +Ġìĺģìĥģ ìĿĦ +Ġam an +Ġhyp not +th ms +Ġnas zej +Ġmour ning +ĠS AY +cy j +Ġго ÑģÑĥдаÑĢ +Ġca u +me e +Ġt adi +M ed +Ġcal idad +ãĥŁ ãĥ¼ +Ġstri pe +Ġε ν +ĠKat y +ĠEs cape +Ġ ãĤĵ +Ġmüs ste +ĠاÙĦ ا +к ÑĤ +Ġjob bar +ĠJe ju +or ar +ĠSer á +ĠMess i +á z +ĠTr an +Ġpier cing +Ġar ithmetic +Ġstagger ing +Ġplug ging +ĠK AR +v l +´ì ĺ +ĠReg ierung +ĠO czywiÅĽcie +ĠEd gar +Ġconduct ivity +y elling +v ais +ad ian +Ġbul ky +ĠÑģ ÑĢав +ĠпÑĢ Ð¾Ð¼ +Ġp aved +Ġb ends +ĠSkills hare +ĠMmm m +ĠHor ror +Ġt umb +Ġgoof y +ĠMe ow +×Ļ׾ ×ķ +ĠW ass +ĠSc ale +ĠR ak +Ġproject ing +Ġlingu istic +ĠWorld s +ense mble +Ġpe ga +stop pable +Ġim balance +Ġà ¸ +Ġthr iller +колÑĮ кÑĥ +Ġleft overs +Ġcave at +ĠST R +und ai +Ġwater y +ĠMar in +ãĥ³ ãĤ° +Ġegg plant +ĠJ B +Ùħ ÙĥÙĨ +vid ia +ĠF IN +ic able +Ġpod ob +Ġco hesive +ĠVerfüg ung +ĠPl ato +аÑĢи Ñī +Ġk ot +ĠÐŁ ом +Ġдок Ñĥм +Ġimpl ants +isse z +B re +Ġgas ps +ĠT ED +r ato +J I +Ġaven ues +ĠCh ong +lad ı +ر ض +Ġin ici +ĠSub aru +æķ ħ +éģĬ æĪ² +ภĭ +Ġach t +ĠArchitect ure +ĠвеÑī и +ĠDev Ops +Ġto ppings +Ġobs ol +ain a +ĠBang kok +est ruct +Ġk ob +Ġëĵ ¯ +ĠÑĢаз нÑĭе +Ġre e +Ġbij voorbeeld +ĠDemocr acy +à¹Ģร า +Ġкон ÑĤ +Ġse ç +Ġrah at +Ġparliament ary +ĠB ash +æĬ ĵ +z iaÅĤ +IT CH +ĠBub ble +kt ó +Who a +Ġfl ats +æķ Ī +z ne +Ġserv icio +ĠD ew +Õ¸ ÖĤ +Ġunterstüt zen +ĠWind s +éĤ£ 个 +Ġìĸĺ ëĬĶ +Ġevalu ations +Ġre ca +Ġel ves +che er +Ġj al +Ġrest ed +Ġquien es +ĠBro oke +Ġë§ĪìĿĮ ìĹIJ +Ġint en +Ġo ats +Ġrefere e +Ġpneum onia +Ġdel ve +pe ace +en y +Ġmost ra +ĠC annon +Ïģο Ïį +ĠÐIJ л +Ġmonument al +οÏį με +imm ers +av ian +Ġдел аеÑĤ +Ġpitch es +ĠGro ve +Ġsemin ars +Ġré cup +ĠVo or +Ġde ven +Ġd B +Ġboost ing +eg an +Ġwel t +ĠGuatem ala +Ġmile age +Ġbeh and +ĠWa ar +ĠSur f +Ġca uliflower +ĠTy r +Ġmite inander +Ġd aring +ĠS itting +d led +Ġresent ment +mÃ¤ÃŁ ig +Ġfilm making +w arts +th ought +olog ique +ĠC OR +Ġaccount ed +Ġa per +ĠIN T +ol are +Ġacompa ñ +èŃ ĺ +Ġ Æ¡i +ä¹ Ŀ +Ġm ermaid +ĠBent ley +at ore +Ġpre n +Ġet hanol +Ġastronom ers +se at +keep ers +Ġexem ption +Ġam o +ĠëĤĺ ìĦľ +Ġin hal +Ġb ows +Ñģк ÑĥÑİ +3 000 +Ġfer mentation +Ġsink s +Ġcomer cial +Ġst ump +Ġce le +ĠS isters +ĠReg ister +Ġso ort +Ġnat omiast +Ġê·¸ë ¦¼ +ĠÅŀ ey +Ġhy ped +ĠRaf ael +ĠE is +ĠBas il +ĠAssass in +ĠA de +rÃ¥ n +Ġon lar +Ġmov imiento +Ġaddition ally +Ġsl it +ĠCh ry +ĠInter viewer +׾ ×§ +Ġdis l +Ġl igger +Ñĥ ки +ber ish +ĠÑĢÑıд ом +AR ON +], , +Ġlum ière +Ġol vid +Ġfre ue +ĠT ing +ĠK ö +Ġge o +Ġdy ed +ãģ§ ãģį +ÑĪ ÐµÐ¹ +Ġży cie +Ġ ie +Ġtax payer +Ġpe ÅĤ +Ġdéc idé +ĠcÅĵ ur +Ġentwic kelt +ĠH Q +K K +od ar +Ġh one +Ġconf iance +Ġiss uing +Ġdiagn ost +Ġìŀ Ħ +ĠкÑĢÑĥ ÑĤ +Ġк аÑģ +Ġà ¾ +Ġrestrict ive +ĠCast ro +Ġu ÄŁ +Ġem pre +ĠM oo +ĠFig ure +phon etic +Pro f +ĠпÑĢ Ðµ +Ġtilt ed +ĠNeg ative +ĠLim ited +men o +lam ation +Ġtrust ees +Ġintens ely +Ġaç ıl +ĠUs ed +Ġz ul +Ġappreci ative +Ġt inc +Ġconqu est +ĠعÙĨ د +Ġsuic idal +Ġmul heres +Ġdet ach +Ġkam era +ĠAir Pods +IND ISTINCT +гли й +Ġëĥ Ħ +Ġwrest le +æ´ Ĺ +Ġfire arm +Ġli re +p ra +Ġjew els +ĠCor nell +Ġíķł ê²ĮìļĶ +Ġsu cker +Ġnombre ux +ĠF erm +ìĽIJ ìĿ´ +ĠP is +Ġиз ÑĥÑĩ +Ġmit en +Ġce v +ĠURL s +ĠC AS +Ġ åı¯ä»¥ +f inden +Ġbra very +ĠÑģлов о +Ġnen huma +Ġencuent ra +ĠShir ley +Ġper cept +fr ames +ĠRo ver +ĠAlber ta +oc c +Ġë Ŀ¼ê³ł +Ġsú per +Ġpres ume +Ġgl and +Ġp acing +Ġneur ot +Ġs no +Ġpl otted +ĠpaÅĦst wa +ĠOwn er +ĠDef ence +rid ges +Ġwall paper +on ian +B ro +ĠAri ana +缴 æİ¥ +k ry +Ġnarr ation +Ġcrian ça +ĠAlright y +ĠìĿ ½ +Ġìĵ° ê³ł +Ġliber ated +Ġexceed s +Ġdom inating +Ġbak ın +l k +Ġsla pped +ÐĹ Ð´ +ument al +get table +ĠRo z +ĠG ul +ou vert +Ġsm ashing +azu je +S ir +Ġgr ated +ä½ł æľī +AT T +Ġartic ulated +Ġst ora +Ġextr ater +á» ī +Ïĥ Ïī +w ir +ĠM ete +I mp +Ġho or +ph ase +ĠÑĩ Ñĥд +Ġб ÑĢаÑĤ +Ġid ag +Ġcin q +Ġapare cer +ĠI CE +åĪ Ĺ +Ġquiet er +Ġfals ch +ad ic +Ġп лÑİÑģ +ĠMen u +ux e +ĠT ôi +ĠM IL +ĠH aj +ver bs +Ġtub ing +Ġmach st +Ġd all +T er +Ġgel en +Ġcuc umbers +Ġwid gets +Ġdev rait +Ġm ike +Ġint ra +íķ Ń +Ġà ħ +ĠH und +æ§ ĭ +qu arter +Ġe w +Ġkelu ar +Ġm ats +ĠTr ick +ĠInfin ite +ŀ ¨ +Ġpe ac +ĠPr ote +à¥ Ī +Ġ17 00 +ĠR ais +๠Ĭ +äh lt +ific a +a imer +a Äĩ +Ġa kl +ĠVol vo +ĠT yson +ĠR ong +irs in +ĠâĻ ¥ +Ġpar ody +n ational +p od +ay d +amb led +Ġgovernment al +Ġconf ort +ic ides +Ġnas ze +ĠSh epherd +ĠKont akt +Ġdisproportion ately +Ġк лÑİÑĩ +Ġt ÃŃtulo +Ġs ina +Ġcompos itions +ĠP F +Ġver kl +Ġsuiv re +Ġast a +Ġstake holder +Ġsam ma +ĠBL ACK +Ġnod ig +Ġle va +Ġjue gos +Ġern st +Ġbottom s +ĠSign al +Ġpoll ut +Ġd ura +Mus ik +Ġком на +ĠвÑģ ей +al ter +ĠSte f +ĠBig Query +ĠVerantwort ung +Ġëĭ¹ ìŰ +Ġqu izz +ĠLet ter +ĠInvest ment +ÑĪ ÑĤ +IJë į° +Ġenc oding +Ġtän ker +ĠK w +ann ie +åĭ Ŀ +1 10 +Ġz wy +Ġì§ § +Ġda w +est ä +Ġdece ive +ĠL änder +is ko +Ġpod staw +ĠPh araoh +ì³ ¤ +éĻ IJ +ú lt +Ġty ö +Ġmus imy +è³ ª +Ġp c +ĠN T +ĠCost co +Ġ å°ı +ĠÏĥ οÏħ +Ġun in +r ounds +Ġremind ers +Ġpuis qu +Ġkrij gen +Ġwork flows +net en +ĠëIJĺ ì§Ģ +Ġsle ek +Ġcowork ers +am ientos +Ġwitch es +ba ar +et ies +Ġun natural +ĠS ick +ĠEf endi +ãĥ³ãĥĢ ãĥĽ +j cie +Ġcham ado +ìĺĢ ìĬµëĭĪëĭ¤ +Ġprz edsiÄĻbior +Ġbook store +Ġìŀłê¹ IJ +ĠSep ar +ang i +E vet +Ġemergen cies +ĠX ML +н д +¥´ë ©´ +Ġê¿ Ī +Ġëĵ¤ ê³ł +Ġs ut +ĠW iz +å± ķ +Ġdynam ically +op eration +d ot +Ġine fficient +cle ars +Ġmund ane +ĠVeron ica +èĮ ¶ +ر ت +p ose +p ai +Ġn ylon +Ġaument ar +Ġall tsÃ¥ +v ak +Ġcapac idad +ĠWrest ling +Ġfert ile +Ġm ég +ĠN ano +аÑĤ ели +Ġìĸ´ì © +Ġto ca +ĠE g +â ģ +Ġì ³ +lu ent +Ġso lem +Ġcin emat +ĠQu el +Ġorb its +ĠHar m +ric anes +Ġblur red +å¦Ĥ ä½ķ +ĠاÙĦذ ÙĬ +Ġj in +Ġgren ades +Ġat roc +Ġwhere in +Ġrepl en +ĠCom ics +eda an +Ġden im +Ġembarrass ment +ĠG omez +ĠBus an +iv ities +Ġsal iva +Ġmer k +Ġil gili +Ġк ÑĢÑĥг +Ġoccup ational +ĠSah ib +S ta +Ġadv iser +ĠTru ly +ĠYE AH +ĠìŀĪ ëĬĶëį°ìļĶ +z ew +b aren +Ġst ol +Ġbelong ings +ĠResearch ers +Ġe fendim +Ïħ Ïĩ +ÅĤÄħ cz +ĠU ng +ĠJ ub +Ġcere bral +á»ĩ u +Ġצ ר +Ġпод аÑĢ +Ġmarch ed +Ġaw aken +Ġa ko +Ġa cept +Ġiniti ation +è¯ ī +l ot +ĠwÅĤ as +ĠMong ol +ut ral +Ġtent ang +Ġinvers ion +ĠìĿ´ íĽĦ +Ġlo k +ÅĤby m +R S +Ġst os +Ġinteract s +ĠCal endar +Ġvan ish +Ġphysi ology +Ġlinear ly +ĠJ Y +ÄŁ an +fund ed +iz iert +Ġzm ian +ĠGr ill +Ġun believably +otechn ology +ĠC ars +ĠÙĨ Ûģ +ĠFol ge +ĠBever ly +ä ischen +Ġaument o +ìĽĮ ìĦľ +Ġmail box +Ġste eds +ĠPe ak +å· § +Ġwy kor +Ġpraw da +иÑĤ Ñĭ +Ġdisc ours +Ġacc use +cess o +u ire +Ġпоп ад +Ġth a +Ġmeas urable +be eping +ĠIn nen +Ġп ÑıÑĤÑĮ +Ġcompet ed +ĠItal ians +Ġencont ra +Ġn iew +Ġfilt ration +ĠпÑĢоÑĦ еÑģÑģ +Ġpaj amas +Ġc ilantro +ĠSo c +L uc +Ġê¹ Ģë +ĠOd d +Ġhyd ration +м ов +Ġply wood +ĠCompet ition +из неÑģ +f light +ĠBe it +bour g +Ġco ils +Ġcâ mera +Ġam ended +Äģ m +Ang el +ĠSt acy +f lo +Ġnorm ale +Ġconson ant +Ġaccompany ing +к Ñĸ +Ġirrit ated +ĠfÃ¥ tt +Ġcrocod ile +IJĺ ëĬĶ +Ġal beit +ĠPhilos ophy +ç´ ¯ +Å Ĩ +yt ic +Ġr èg +Ġfr ança +Ġattent ive +H am +Ġalred edor +æĿ ¿ +se i +ĠÑģв ид +Ġgim bal +Ġch ina +ĠðŁİ ¶ +ĠÐĴ ам +Ġstim ulating +ĠO ra +yt es +Ġhe ft +Ġhat ers +Ġcomplex es +Ġ0 3 +ró d +cle ar +Ġbeste ht +çķĻ è¨Ģ +wn y +mo il +Ġslop py +Ġinsign ificant +Ġdub bed +Ġëĸ ł +Ġcons igo +лÑĥÑĪ Ð°Ð¹ +S n +Ġ×Ķ× ¦ +ĠÎ Į +Ġnad zie +Ġfresh men +ta a +Ġuw agÄĻ +ĠFavor ite +ĠCrim inal +Ġev iden +Ġsy mb +L es +ĠBe au +un ed +ple ment +A c +Ġderm at +ĠN olan +Ñĭ п +Ġs itt +Ġever lasting +Ġest avam +Ġм ик +Ġkh ác +Ġinv it +Ġtre ble +Ġj ig +man i +Ġtu vo +ĠR US +ĠEr de +ĠD ziÄĻkujÄĻ +Ġblue berries +ke ll +ac ions +çĪ · +в и +LE T +Ġspr out +Ġsp or +Ġb ên +ĠM ona +ĠCont ain +ĠKe ys +оз Ñı +Ġfun ción +Ġrapp elle +Ġevol ves +Ġscra ping +Ġcoment ários +Ġpr atique +Ġaux iliary +ĠSp onge +Ñģк им +u vo +ĠÑģам о +Ġs ank +Ġhigh ways +Ġinvent ions +Ġин огда +Ġcreat ively +Ġbenchmark s +on cé +al al +Ġs otto +Ġcal ves +ĠMo v +Ġlav ender +Ġeye balls +Ġawait ing +ĠPat y +ÙĦ Ùĩ +Ġembroider y +Ġdu h +Ġcam ar +ĠBO B +Ġsp aced +ĠgÅĤ os +аем ÑģÑı +Ġesca pes +ĠR ogue +z cz +è ŀ +¬ë ¥¼ +ĠMo że +Ġе ÑģÑĤе +ĠBur ada +éĮ ² +w d +uu uu +Ġs ash +ĠL ub +Ġnote books +Ġma e +Ġconf licting +Ġsumm ertime +ac as +Ġb auen +bl owing +ạ o +Ġìĸ¸ ìłľ +ä»ĬæĹ¥ ãģ¯ +ĠSen hor +ĠiPh ones +ĠQu arter +Ġìłľë ĮĢë¡ľ +u ÃŁ +Ġë§ Ī무ë +Ġsett lers +Ġcre st +Ġtrans c +æĽ ¾ +Ġri ots +Ġcl ones +ĠOp rah +ί ζ +Ġp als +.... ... +ãģĶãģĸ ãģĦãģ¾ãģĻ +ĠÑĢ Ð¾ÑģÑģ +ĠLas er +Ġz aczy +Ġse vi +Ġregen eration +ìĹ ¼ +w ould +Ġüzer ine +ĠStra ÃŁe +Ġvenge ance +Ġr er +ĠSaf ari +ĠHE Y +çķ « +Ġsac ar +Ġimage m +ĠBund est +mes an +ĠP aste +Ġs izz +Ġпо ÑģÑĤÑĥп +×Ķ ×ķ +t rad +Ġfrança ise +ĠB ou +Ġbar re +ĠZ hi +ĠG eez +ih ad +Ġrecon oc +Ġpel ig +Ġind ices +Ġë°Ķë Ģ +Ġcondu ction +Ġìķ ħ +Ġz eker +Ġf um +ĠW ür +bre aker +Ġspr ite +C rowd +Ġopen er +Ġol v +Ġbu enas +ĠSil k +ĠH IM +k op +com pl +Ġposs ono +³ Ģ +Ġoscill ator +ĠS ith +èĥ ¡ +аж и +Ġra ft +h all +Ġschne ller +Ġimport ing +Ġassemb ling +Ġub iqu +Ġactiv ates +ac ci +ĵľë ¥¼ +Ġcompos ers +ĠAC L +Con f +Ġì½ ĺ +ĠнекоÑĤоÑĢ Ñĭе +Ġcand ies +åĬł åħ¥ +ĠM uss +à¹ĥ à¸Ĭ +Ġd uda +ник ом +med en +Ġìĸ´ë ķĮ +ĠYes hua +z ag +hod ou +Ġal oud +ĠPal mer +im ize +ãĤ· ãĥ§ +Ġmar itime +Ġcommun al +Ġbad ges +Ġrug by +Ġmarshm allow +Ġfier y +Ġaccount ant +Ġab la +ĠMon roe +ĠF ont +ĠBo ost +ĠBarn es +ans wer +ĠBur ning +Ġ ä¸įæĺ¯ +Ġan gef +ĠWes ley +ll s +ì µ +ש ׾ +ili ÅĽmy +×IJ× Ł +am ura +ĠF uj +Ġpan i +ĠT rop +ar beiten +Ġr ue +ĠR are +äng en +ĠÑģмоÑĤÑĢ ÐµÑĤÑĮ +ĠÐļ аÑĢ +ĠM TV +board ing +] [ +Ġë łĪë +stan bul +p ielt +ĠHard y +ĠEng agement +ĠD ienst +Ġw ären +Ġfue go +Ġest ruct +Ġcal am +ĠResp onse +Ġ ãĤĦ +ĠMoh ammad +Ġresist ing +Ġdur ant +èģ ¯ +åĨ µ +ĠO LED +Ġver z +m än +ĠÙĨ ÛĴ +Ġparano id +ĠA ware +ĠEngine ers +Ġproced ural +Ġpersonn age +Ġfark lı +é¡ Ĩ +fl owing +ĠмеÑģÑĤ а +ĠB are +ist em +ĠpoczÄħt ku +Ġperson ajes +Ġìĸ´ë łµ +Ń ī +ĠХоÑĤ Ñı +Ġuns ett +ĠAbs ol +Ġ ấy +ĠMAY OR +пол не +Ġinform ing +Ġam ps +ÐŁ ÑĢ +ĠëŃ Ķ +a eda +Ġ×Ķ× ij× +ấ n +kel ijk +Ġathe ist +Ġtr out +Ġne ues +ĠNok ia +m achen +Ġwholes ale +ır d +I ns +ĠÑį п +Ġpr ick +ĠKind ern +à¸Ĺ ำ +Ġclass y +Ġî nt +ĠShop ify +ĠÑģ оÑĢ +Ġзак ÑĢÑĭ +z uk +Ġunivers ally +Ġteas poons +Ġrec ount +ĠnÃ¥gon ting +ĠX ue +isi ème +Ġweak est +Ġte ÅŁekkür +Ġmath ematically +ĠH os +Ġíķľ ëĭ¤ +Ġpart ager +ĠD arr +ê º +Ġε κ +Ġgerm s +Ġgel ir +Ġd ul +, - +Ġìĸ ¸ë +Ġ×ŀ× ¦ +ĠÑı ÑĢ +Ġquot id +Ġprz ysz +Ġhard ness +Ġaqu atic +ĠJung le +ĠPC R +ĠEli ot +Ġo str +Ġma pa +ess ä +ĠG IR +ĠDri ving +ĠS ami +ĠMed ien +ĠCompan ies +ĠPh arm +se its +ĠR im +Ġο ÏĢο +Ġweiter en +Ġpizz as +ĠL ydia +ĠHe ights +Ġsincer ity +Ġnoss as +Ġd ÅĤ +Ġalar ming +ĠC auc +ĠÑģм ÑĭÑģ +f acing +b ags +W W +ĠØ´ ÙĬ +Ġcourt room +ĠPhill ip +Ġê²ĥ ì²ĺëŁ¼ +ĠSpiel er +ãĤı ãģĭ +Ġk ant +Ġadm itting +ãĥģãĥ£ ãĥ³ãĥįãĥ« +Ġcontain ment +å¼ ł +Ġremo vable +Ġjum per +f ocused +ĠиÑĤог е +ĠТ ем +Ġv ase +ĠUS C +ĠMon ate +ĠJac obs +ĠH OL +ik ed +er weise +Ġgood ies +Ġhom age +׼ש ×Ļ×ķ +Ġqu ais +Ġin icial +Ġguard ing +Ġd azz +Ġcomb os +ĠÑĥп ÑĢав +ĠTal ent +å¥ĩ æĢª +Ġó r +Ġintermitt ent +ĠMcC arthy +Ġsp ans +Ġty re +Ġqu y +èĪ Ī +j ut +ĠZ ent +Ġg at +大 åĵ¥ +Ġscaff old +Ġneces ario +ĠZ ahlen +ĠS AND +ĠP U +Every thing +-------- -------- +Ġвз ÑıÑĤÑĮ +Ġspark s +Ġpend ulum +×ŀ× Ł +Ġìĥī ê¹ +Ġmultipl ier +Ġл адно +ur at +Ġupset ting +è¡ Ģ +b ak +Ġìµ ľëĮĢ +Ġan ál +ĠJO E +Ġk osten +ĠPat ty +ĠGu in +ck ed +ĠEgypt ians +ĠCit izens +ר ׼ +ĠÐķ Ñīе +Ġй ого +Ġsnow fl +Ġlek ker +Ġac ost +ĠB abe +Ġgam ble +Ġadject ive +к ими +o ys +Ġmont re +ĠHy undai +Ġmoistur izing +Ġmo zzarella +OO O +Ġfac ult +Ġdo et +Ġfear less +Ġesp resso +Ġall ora +ĠC inc +ãĥ¼ ãĤ¸ +Ġconteú do +ĠPel osi +Ġmind er +ro ot +Ġíķ łë +Ġп ад +ĠCall ing +ĠConf ig +ĠCon sole +ins ky +éner gie +Ġsol itary +од е +Ġguard ed +1 60 +ĠпÑģ иÑħ +ĠSh ap +Ġtit re +olog ne +ĠпаÑĢ Ñĥ +ĠP RE +ãĥ¼ ãĥī +Ġl n +ĠMit gl +ĠCar ry +Ġsp ind +ĠCant on +Ġkingdom s +rem o +Ġr aging +Ġincap able +ĠW R +åĨį è§ģ +ĠÑģоб ÑģÑĤвен +Ġкак иÑħ +ĠSH E +ëĭ¹ íŀĪ +Ġscar city +Ġper de +Ġex its +ĠS inger +Ġsupp er +Ġmunicip ality +ĠD iversity +Ġt iro +iel s +ĠlÃŃ der +Ġbl uff +Ġat ra +ly s +Ġma hd +Ġcód igo +ĠHar lem +ru le +ic ity +Ġsimpl istic +ĠK onst +åģ ¥ +ELL I +Ġför sta +Ġconstit utes +ĠÑģÑĤоÑĢон Ñĥ +Ġur ged +ĠP anda +ì° ¨ë +re ce +Ġpatri ot +ĠCr ush +Ġw ink +ой ÑĤи +uran ça +Ġseiz ures +Ġelect rod +ĠDon key +ĠI U +ĠM OS +Ġal kal +ì´ ī +bes ondere +Ġparall els +Ġbitter ness +ät tre +ess ional +Ġsoy bean +Ġcoll ab +ĠReport ing +å§ Ķ +Ġкомп ании +Ġwsz yscy +ĠCr unch +ise en +Ġamb assadors +ĠChe v +åį Ī +ов Ñĭе +s ca +ĠÑĢеÑĪ Ð¸Ð» +о ÑĤо +Ġgleich zeitig +mer n +ü st +ĠHa e +³´ ê²łìĬµëĭĪëĭ¤ +Ġsh ores +Ġdep ress +Ġa hor +ĠSte uer +ah h +Ġrev ise +ĠÑģам Ñĭе +j at +Ġher bal +Ġcu ánt +Ġb una +niejs ze +F inally +×ķ× ĸ +c je +ĠìŀĪ ê±°ëĵłìļĶ +ĠëĤĺë Ī +Ġprz est +ãĥ¼ãĥ ł +lic a +ĠD uch +å°į å°į +Ñĸй ÑģÑĮ +p assen +Ġsatisf ies +ĠAdd itional +Ġcá mara +еÑĩ ение +Ġp omp +Ġë§IJ ìĿ´ +ĠM ills +ев ид +Ġrespect able +Ġfil ament +Ġv ender +Ġmatter ed +ou re +ì¸ µ +K orean +Ġestud io +Ġc actus +ĠV ive +ĠR ag +Ġcompl iqué +ĠÙĪ Ûģ +Ġta o +¦ ¿ +S ince +Ġje opard +ĠS ell +åº Ķ +Ġìĺ Ľ +Ġket o +Ġintel ig +ĠAn geb +Ġt iden +Ġsoci o +Ġreminis cent +Ġcareg iver +Sp ace +ĠExerc ise +ĠBe come +ê ts +ak k +! .. +ĠÑģп ÑĢоÑģ +Ġα ÏĢο +Ġshoot ings +Ġa pe +ĠSam my +ĠK ung +Ġcu ál +ĠL up +æĿ Ł +ä¾Ĩ åΰ +ĠÑģÑĤ Ñĥд +Ġswe eter +Ġcom um +ĠAd s +hy ung +ĠбÑĥд ÑĥÑī +Ġw affle +ĠOr b +Ġla ut +Ġforecast ing +å ª +Ġrapp ing +Ġpref ers +Ġben z +Ġn ik +ĠB ahn +Ġsand ing +Ġimmin ent +ĠпÑĢоблем Ñĭ +Ġdo ivent +ол а +Ġży cia +ih u +Ġexist em +ĠInter ior +ĠT akes +Ġtodd ler +Ġdictators hip +ĠSmith son +ĠAllah u +Ïİ Ïģα +ìķĺ ìĬµëĭĪëĭ¤ +ĠV ote +ĠSm ells +од но +Ġhind sight +V R +ĠP atch +ĠJah res +Ġsou venir +Ġneut ron +Ġlong time +Ġsay in +ä¹ IJ +as aki +ĠоÑģÑĤ анов +Ġex pelled +Ġcryptocur rencies +ĠMur der +ĠCit izen +W AY +Ġpl u +Ġlemon ade +Ġconvenient ly +ĠH I +Ġ20 23 +ש ×ķת +аÑĨи он +ĠëĽ ° +ĠÙĦ ÙĥÙĨ +Ġнемнож ко +Ġun used +Ġmaior ia +Ġastrolog y +ĠDow nt +N ick +Ġpreoc cup +Ġdem ain +×ŀ× ¢ +Ġвод Ñĭ +ĠSans krit +Ġpr êt +Ġstrand ed +Ġref in +ĠпÑĢи ним +Ġпов еÑĢÑħ +à¯į ? +Ġz rob +Ġinter tw +ĠDavid son +лен а +Ġпон ÑıÑĤÑĮ +ĠR eno +ĠполÑĥÑĩ илоÑģÑĮ +Ġcorrespond ent +ĠU ran +el se +· · +Ġtut oring +Ġgrand daughter +lud ed +Ġst esso +Ġh ết +Ġgeg angen +ĠÐĿ ÐIJ +Ġant ig +back ground +Ġg edaan +Ġf avored +ĠEm manuel +Ġi od +Ġclamp s +Ġcomp le +ĠAdv ance +ĠìŀĪ ê³łìļĶ +ĠRo x +ĠìĹ IJë +Ġintest ines +Ġper cussion +Ġlegit imately +ĠE ternal +f amily +al og +B rad +ени ÑĤÑĮ +ĠÑģ наÑĩала +Ġcert a +Ġak kor +Ġε δÏİ +Ġoct ave +ĠV ac +м оÑĤÑĢи +ĠÃīt ats +Ġlong ue +Ġdis soci +ÑĢ Ñıд +he in +Ġpant alla +Ġindic ations +ĠL t +ĠGr ade +è£ Ŀ +o ine +b ug +ĠVer izon +ĠAl ém +Ġv iennent +ĠÑĩ иÑģÑĤ +ĠBen i +ĠT sch +ĠT P +Ġinsult ing +ĠWe ight +Ġadapt ations +Ġhab ÃŃan +Ġcl ique +o ÅĽci +j una +Ġsuch en +ĠGo es +ĠEx odus +C ho +Ġant is +ĠíĮ Įë +se ven +ĠÑĩаÑģ ов +Ġball istic +z ony +IC IA +ĠпÑĢ ÐµÑģÑĤ +Ġsimples mente +ĠColl abor +F red +ĠÑĤелеÑĦ он +ĠR avi +íķ´ì ¤ +пеÑĢ Ð² +ĠìŀĪ ìľ¼ëĭĪê¹Į +Ġó t +Ġa leg +ú p +Ġdisreg ard +Ġind ent +cl oud +chl agen +Ġiter ate +Ġgeneral ized +ãģĹ ãģ¾ãģĹãģŁ +ठ¹ +eler i +Ġdisast rous +ĠÑģÑĤ ала +³ ij +KN OWN +Ġrich ness +Ġcons cient +icht s +ĠÑį лем +ب د +ire ns +Ġha unting +ruct ures +att ack +Ġcup cakes +s que +Ġnas zego +Ġanthrop ology +ãģŁ ãģł +ãģµ ãģµ +cha e +Ġdisco vers +ĠPerson ality +ĠΤ ο +Ġdi ÄŁer +åį Ģ +Ġне Ñij +ĠAn ita +Ġ[ âĻª +ĠCar m +ĠBen ny +ìĬ ¬ +Ġpup il +Ġoc as +äll et +j ÅĽÄĩ +大 ä¸Ī夫 +ament al +ĠоÑĤно Ñģ +Ġp id +Ġar mp +RE E +ĠоÑĤк ÑĢÑĭв +Ġu da +ĠSynd rome +ĠStand ards +ãģĪ ãĤĭ +Ġpo inters +Ġen am +ĠT ig +ÃŃ z +Ġн ами +Ġunch anged +Ġtur moil +ứ ng +!! " +5 000 +Ġ물 ìĸ´ë +Ġmer ging +Ġentsche iden +åĩº æĿ¥ +form e +Ġtrim med +Ġd ared +Ġasp iration +ĠMyth ical +ĠHe j +ĠAle j +ÑĨ о +оÑĤ Ñĥ +Z e +Ġин ÑģÑĤÑĢÑĥменÑĤ +ĠRT X +Ġlocal ized +çļĦ è¯Ŀ +Ġsur rounds +Ġemp ieza +Ġcl ase +Ġ à¸ģ +ĠRap id +omin ous +ig ail +ĠÑĪ Ð¸ÑĢ +Ġl æ +Ġzas ad +Ġunfold ing +?! ?! +ĠìĪľ ê°Ħ +ĠPol ski +ĠK auf +ĠC elt +it ic +Ġtool box +ĠP ocket +ĠìĦ ľë¡ľ +Ġbel ki +Ġadm iration +ph r +ĠProdu kt +ĠT ruck +ãģ İ +Ġdra uÃŁen +wa ÅĤ +ĠHebrew s +Ġíķĺ ê²Į +ĠA CE +urg ence +aur ais +Ġchar itable +ı t +Ġarm as +ĠGed anken +reat ing +port e +Ġimp rint +f äh +Ġпод Ñħод +Ġout set +ว à¸ģ +ен ного +Cl ass +Ġvan ity +ĠVO ICES +Ġ2 60 +res ident +US E +Ġê°Ģ ìļ´ëį° +é ½ +Ġthrough put +Ġc uma +ìļ ± +ãĥ¼ ãĥ³ +Ġпло Ñī +Ġpart is +ĠAnim ation +§ Īë +C re +öt zlich +Ġma gg +Ġcl umsy +Ġbott lene +Ġbirl ikte +ĠG amb +Ġ׼ ף +Ġmet ropolitan +è¯ ¥ +æİ Ĵ +O oh +Ġobject ions +ĠÙħ ت +Ġм ел +Ġrem nants +ĠX avier +R ich +Ġol sa +ĠP ill +Ġgro ans +ĠNaru hodou +ĠCont ract +ад а +na i +ĠÑĦ из +Ġop s +ạ t +Ġparach ute +Ġne ll +ĠEntscheid ung +׾ ×Ļ×Ŀ +Ġtruth ful +Ġshar per +Ġbureauc racy +c art +Ġин ÑĤ +wie k +Ġwilling ly +ĠH erman +Ġmehr ere +Ġel ites +ĠArm or +ãĥĪ ãĥŁãĥ¼ +Ġemb ora +ĠRec ogn +ĠлÑİб лÑİ +ĠEx cellence +ib el +Ġexport ing +ì² ´ìłģ +K elly +Camer aman +Ġsl ips +Ġfig ura +Ġãģ ¡ +Ġk oll +ĠPand emie +çı Ń +Ġtim ed +lieÃŁ lich +Ġ×ŀ× Ľ +ĠperÃŃ odo +å¿ Ĺ +iv at +Ġquestion naire +Ġpéri ode +ç© ¶ +Ġs ighs +Ġalleg iance +ĠX V +ĠKens uke +ĠGesund heits +Ġposit ivo +ĠJane iro +ĠS EE +Ġا ست +ĠKel sey +to ber +Ġα λλά +ĠP arent +ĠDay ton +ĠBild er +our age +Ġser es +ĠmuchÃŃs imo +ĠReal m +ĠOFFIC ER +erson ic +ãĤĤ ãģ® +ony a +Ġê¸ ī +Ġancest ry +ĠJur assic +Ġcent igrade +ấ u +ujÄħ c +m ans +Ġt io +ĠMo ż +Ġtra gen +Ġst ared +Ġsch ematic +Ġpass ou +Ġmeat balls +ÅĤo ÅĽÄĩ +Ġsynchron ous +Ġperm is +ar ial +Ġz er +Ġpar ity +ĠAv atar +inde er +est on +Ġme idän +ĠC ly +´ ī +Ġest rogen +Ġcent imet +çĻ º +Ġconv ictions +Ġposs iamo +Ġper du +Ġpath ogens +ĠQu in +ĠProgram s +ĠPoint s +ram ent +ra il +Ġv y +Ġgra ft +Ġb art +ĠLot us +à ¨ +Ġë³´ìĭ ľ +ram er +F ather +Ġëľ » +Ġ×Ķ× Ŀ +Ġtra zer +Ġt ark +è ces +f orth +ĠÑģдел али +Ġzucch ini +Ġw aktu +Ġentertain ed +ĠMilli arden +Ġsh aky +Ġprz ede +¸ Įë +Ġrevers ible +ĠN AU +u ins +ér êt +ann en +ĠHun ting +ĠF ellow +él ior +Ġrot ations +Ġgr anny +xt on +ĠÑģÑĤанов иÑĤÑģÑı +ĠнаÑĩ ал +Ġarter ies +ri ó +ĠполÑĮз ов +ĠÐij Ñĭ +Ġnovel ty +p ound +Ġweird est +Ġbo is +ém ie +u pl +AT A +Ġte hd +ĠN ir +s ınız +! ", +åijĬ è¯ī +Ġimm ort +Ġel k +ани Ñĩ +Ġfabric ation +ĠNo ise +ĠA vant +ر ÛĮ +w at +Ġwho oshing +Ġ׼ ×Ļ +ĠÐĹна ÑĩиÑĤ +Ġcent rif +ans ing +S ound +Ġë Ŀ¼ë +Ġcapt ions +à³ į +Ġor gas +Ġdolph ins +ĠBl end +ĠT aj +ĠC CTV +Ġin om +Ġed itions +Ġburn out +Ġb ättre +ĠC asa +ov ich +Ġmol ten +Ġblind fold +ĠG ue +æĹ¶ éĹ´ +Ġspin ner +Ġmöglich st +ĠV Ãł +ene ca +Ġméd ico +å¹¹ åĺĽ +ást ico +Ġar d +ĠSund ays +ĠRem ote +Ġìĸ¼ë §Ī +Ġtr Æ°á»Ľc +ìħ ¨ë +Ġdo pp +Ġbe ÄŁ +ic ana +ĠëĤĺ ì¤ijìĹIJ +çİ ĩ +Ġhol iness +d irect +Ġìĺģ íĻĶ +Ġcul pa +ĠSt itch +light ly +ам ен +Ġм еÑĪ +Ġп еÑĩ +Ġy hte +os phere +Ġìĵ° ëĬĶ +é k +Ġserious ness +Ġgar ments +Ġconc ise +ĠS J +Ġverl oren +Ġpare cer +ĠUN C +ìĬ¤í ĥĢ +Ġenf ant +Ġbom ber +ĠG ift +Ġì¢ĭ ëĭ¤ +Ġrhy thms +ĠK lar +人 æ°ij +own ik +ĠRever end +Ġem itted +l assen +Ġreven ir +Ġar ising +Ġprecis amente +Ġinter pol +ĠTen emos +ob ed +Ġtecn ologia +Ġnered e +ĠV isa +Ġsa va +Ġescre ver +Ġassault ed +ĠFle isch +ĠCouncill ors +Ġê°Ģ ê¹Į +Ġbe gg +ĠDevelop er +ĠBron ze +ĠBon us +Ġר ×§ +f act +Ġend lessly +Ġmac am +Ġrze czywiÅĽcie +Ġhover ing +è ge +Ġpo orest +ĠSch ed +m ile +isse ments +ac Äĥ +Ġë ¦½ +Ġvacc in +Ġfutur istic +ĠWind ow +п аÑĢ +ĠÑĢ Ð¾Ñģ +Ġlow ers +ac s +ĠÐIJлекÑģ анд +ĠAl ert +ie me +ĠCauc as +Ġj aws +Ġhunt ed +ìĹ ½ +Ġب ÙĨ +Ġ×ľ× ł×ķ +Ġturb ines +Ġl umps +ĠAll ies +ah lt +Ġsubscri ptions +Ġnouve aux +ug er +b ones +Ġb erry +ĠìĦłë ¬¼ +ĠMan ufact +ĠL unch +ê·¸ë ŀĺ +Ġhyd rated +Ġache i +ĠY az +ĠTibet an +ĠQuant um +ĠJer ome +ĠоÑī ÑĥÑī +ов ан +m otion +ĠCont roller +ener getic +ĠÑģкоÑĢ Ð¾ +Ġvow els +ĠÑĥж аÑģ +Ġho of +ĠBull et +imag in +׳ ×Ļ×Ŀ +Ġengage ments +ĠBl ues +Ġañ ad +Ġf ps +Ġcater p +Ġs á»ij +ĠTri be +ç¶ ļ +п он +if eration +Ġrum ah +ĠPun j +l ab +Ġcompreh ension +br inging +W o +Ġt ik +Ġany how +以 åīį +át icas +Ġsit zen +Ġkol ay +ĠConfeder ate +ĠCall ed +Ġnas zych +Ġd ziÄĻki +Ġclo ak +ĠGo og +ĠAs he +è± ¡ +en an +ĠмÑĭ ÑĪ +Ġв еÑĤ +ĠSp o +ĠS ket +ĠHend erson +il ah +Ġбез опаÑģ +Ġsek ali +ìĸ´ ê°Ģ +Ġsn are +Ġr ằng +Ġförs ö +szy ch +Ġü bers +Ġstrat ég +Ġìº IJë +Ġrapp ers +Ġc ep +ĠH asta +Ġhor ribly +Ġfr üh +Ġب ع +Ġmant le +ãĢ ħ +fund ing +Ġz ust +ĠP ens +s ed +ĠíĹ ¤ +Ġgere ki +Ġal arms +ĠWh a +ĠMark us +aks i +ĠÐIJ ле +kl ore +Ġé ner +Ġt ilde +box ing +ĠìĦ ŀ +Ġencont ramos +ĠPh ar +нак ом +ó st +Ġİ s +Ġëĭ ĺ +Ġsqu ats +Ġpret ended +Ġde z +Ġê´ľì°® ìķĦ +j ach +ë Ŀ¼ê³ł +ĠíĻķ ì§Ħ +ĠAn sch +imer k +Ġconjug ate +Ġpen insula +Ġgor illa +Ġphotograph ed +ĠAun que +Ġent ren +ĠDeuts chen +ĠAl addin +Ġë¬ ´ìĦľ +ĠSt ella +ĠE lection +out ine +G rand +ĠW ak +ĠSer gio +hor se +ah on +ĠFamil ies +Ġh ating +ĠB ett +à¸Ļะ à¸Ħะ +Ġcur ling +ĠIsrael is +Ġ×ľ× IJ× +ĠMy ers +Ġsc anned +ĠB EC +iler i +Ġcall e +ĠMin h +Ġmic ron +Ġcon duc +ÃŃ v +Ġвоз ÑĮ +Ġaction able +ĠTrust ees +Ġt ief +Ġhead ers +Ġanim ales +ìĽ Ģ +ло Ñħ +un ity +ly a +Ġj angan +Ġh ani +Ġcas ing +Ġjó venes +ĠSpl it +ĠCar lo +ĠBe im +å°į ä¸įèµ· +Ġnu anced +Ġted dy +ĠCl an +ä chen +p ier +Ġдоп олн +Ġdi aper +e ffective +ĠNi agara +Ġw art +Ġcor ro +ĠK ampf +z te +Ġdévelopp ement +Ġattack ers +ĠSher man +Ġ19 14 +Ġme ow +ĠP Ã¥ +ì º +c it +Ġcou pe +Ġê·¸ëĭ¤ìĿĮ ìĹIJ +Ġhum our +Ġco le +ĠW arning +ĠT il +cal m +bu at +Ġc ine +k iej +Ke vin +Ġmilligram s +×ĵ ר +ar iamente +Ġo ro +ĠH od +ert os +Ġli hat +Ġfull est +Ġgrand i +Ġб ок +Ġwho lly +Ġmahd oll +Ġcontro ll +ĠBun un +èĬ Ĥ +Ġdi pped +Ġreg ión +ĠÙĦ ÙĪ +Ġб аг +Ġpremi ers +Ġch á»ĭ +Ġ æīĢ以 +è± Ĩ +ide z +Ġqu ota +Ġg hee +ark an +Ġgel atin +ĠCler k +bb les +ĠPa ige +Ġst aged +Ġsoci ais +ĠBiz im +Ġveloc idade +Ġmal aria +Ġshort ened +Ġsal ut +ĠHe he +Ġv á»ĭ +ĠTaiwan ese +ĠAr ri +g res +åİ» äºĨ +( ) +ri ad +ij IJë +Ġãģ¾ ãģĻ +Ġmascul inity +L P +Ġëĸ ¡ +Ġtér min +ĠV ä +ĠSe iten +Ġrespect fully +á o +Ġtotal ement +Ġscra ps +Ġinf ring +ĠB ose +am ar +ĠLu iza +ĠAR M +ĠплоÑħ о +Ġme illä +ĠD ion +å¼Ģ å§ĭ +Ġsou ha +Ġgesch afft +Ġconv olution +ĠâĢij âĢij +Ġ14 4 +ling t +Ġmänn isk +Ġgust ado +Ġco ined +ĠL ulu +å®ĥ çļĦ +op ot +ĠPr ayer +Ġroast ing +Ġchromos omes +é£ ¯ +ел е +Bl ue +ĠEr folg +èĩª çͱ +ĠпÑĢид Ñĥм +Ġrisk ing +ĠGuard ians +Ġ20 24 +è se +ĠбÑĥд ÑĤо +Ġcons erve +ĠBr inging +ĠAst ra +à¹ĢภĤ +Ġкак ÑĥÑİ +resp ace +ĠÐŀ п +Ġвок ÑĢÑĥг +æħ ĭ +Ġmask ed +ĠSh y +ĠN im +end as +Ġíı¬ ìĿ¸ +Ġ모 ìĸij +Ġvale ur +ĠNeg ro +ĠCD s +ink ling +Ġmont ón +ĠH ond +Re al +Ġfull ness +ĠWho ops +ĠSh ank +ĠBr an +Ġtransl uc +Ġer r +ĠGard ens +oy u +Ġaffirm ative +ä¸ĭ ä¸Ģ +Ġpot tery +l ive +ia u +m ount +Ġfluct uations +åŁ İ +ÃŃ em +Ġpul ses +Ġcrian ças +ία ÏĤ +Ġbast a +ENN IS +ĠкоÑĢ Ð¿ +ĠF unk +Ġ éĢĻ +Ã¥r t +ĠзаÑĤ ем +Ġparas ites +ãĥ Ļ +Ġair flow +ĠX uan +G ülme +Ġblo oming +Ġm ummy +Ġba o +ĠCl ap +ant ics +sk in +cent ric +be fore +ĠRICH ARD +ĠH ahn +T AKE +ĠÑĤÑĢ ÐµÑĤÑĮ +Ġpressure d +ĠKur z +ist i +ĠнаÑĪ ÐµÐ³Ð¾ +Ġsemicondu ctor +ĠCl int +Ġpl up +ĠOrig in +ĠEv ents +Ġê±± ìłķ +mp fen +NE Y +ĠD W +Ġë¶ģ íķľ +Ġinform s +Ġfor sk +Ġam iga +ĠCinc inn +St r +Ġpar ish +Ġì» ¤íĶ +Ġsiz i +Ġplant ation +Ġbl iver +Ġпол иÑĤ +Ġsubd iv +Ġr ant +Ġprincip als +åIJ ¦ +Ġkun ne +ü gen +a respace +Ġv allahi +Ġcollaps ing +اÙĦ Ùħ +Ġl ider +Ġt ama +Ġg agner +ro lle +Ġë§IJìĶĢë ĵľë +Ġcath edral +ĠWe bs +ĠPolit ics +ãģĹ ãģ¾ +ãģ£ãģ¦ ãĤĭ +ĠDen is +Ġtu o +Ġref ract +Ġdis integr +st es +ĠлÑİб ов +Ġw ilt +Ġtrust s +Ġkom un +ĠB asket +~ !! +na e +ĠÐļ ол +Ġsyll ables +ĠHen ri +ĠN ab +ÙĪ Ø¹ +Ġw n +Ġk amp +ĠPrag ue +ĠBreak fast +Ġê·¸ëŁ ´ +Ġch ut +Ġ3 30 +ĠIndust ries +ä¸į 管 +ĠiÅŁ i +ĠGold man +Ġİ ns +uss a +ith e +Ħ IJ +ĠSO UND +алÑĮ нÑĭм +. ( +Ġго ÑĢаз +Ġdage gen +Ġë ® +Ġwait er +l ength +ĠÏĥ ÏĦα +Ġchunk y +S a +Ġrust y +ĠJud ith +7 50 +Ġepo xy +ì¹ ł +åı ² +met ro +Ġreject ing +Ġsquish y +Ġplup art +Ġmé th +Ġasp iring +ĠD rama +Ġup lift +§Ī ëĭ¤ +........ ........ +ł¤ ìļĶ +Ġtéc nica +Ġpas ando +Th ose +ĠÑĢаз дел +Ġmedi ocre +ĠNic kel +Ġsuperhero es +Ġmission ary +ĠPare ce +Ġrot ational +Ġpret t +ãģĿãģĨ ãģĿãģĨ +Ġl ama +Ġcan yon +Ġbet er +ĠProv ost +Ġh vis +Ġde activ +ĠH els +pf licht +S omething +ĠPier ce +Ġê²Ģ ì°° +l ungen +Ġs izing +Ġlat itude +ĠNon etheless +om nia +ĠSab rina +ĠDynam ic +åĥ ¹ +ont a +ìĨ IJ +Ġdirect ive +ĠDep ot +Ġfuel ed +Ġexp ire +Ġcom ún +ĠSe xual +ĠG ore +Ġrest less +ĠJ AKE +ÑĤеÑĢ ÐµÑģ +ĠÑĤÑĢ Ð°Ð½ +ĠHol z +å° Ĩ +ĠA ctor +æĿ ¯ +c all +Ġemail ed +ĠPe ar +Ñĥд и +ÑĢ Ð°Ð» +Ġm Ãły +ĠCH EERING +å®ī åħ¨ +Ġretail er +Ġprot r +Ġdisc arded +ĠH IS +Ġevangel ical +ĠEl se +Ġexpl ores +Ġcritic izing +if ik +Ġwh ipping +Ġop is +ous ed +F ree +ĠíĮ ¬ +Ġm ics +run ning +O b +iti é +Ġneces ita +ĠDomin ican +ĠB agh +Ġtend encies +ĠMet ropolitan +Åij l +Ġзна ем +ĠZ am +ĠDead pool +ale ż +Ġinvestig ative +ĠPron unciation +Ġem ulate +Ġban co +Ġ- âĻª +åĪ » +Ġover arching +lich es +Ġвозв ÑĢаÑī +ĠSc ary +ĠK ia +åľ Ł +ron ting +inn ed +ĠÛģ ÙĪ +ìĪ ĺ를 +ç¾İ åij³ +w el +Ġë³ Ħë¡ľ +Ġunint ention +aa S +Ġnic est +ĠT esting +ĠIS IL +ogen ous +ĠØ Ł +Ġlie utenant +Ġbra uch +ĠT ir +d rive +Ġtoler ant +Ġshoot ers +ĠìĺĪë »IJ +æ® º +ont on +Ġter ia +iet et +R on +le igh +ga e +Ġol mak +ĠCl one +so ld +Ġskelet ons +Ġincumb ent +ом е +C ON +Ġle ven +Ġmillenn ials +Ġequ ator +ĠFed er +ĠAlex andra +Ġv rij +ĠHealth care +Ġíķ ij +Ġemphas izing +Ġdialog ues +Ġch illed +Ġpr ow +ĠPass ion +ĠLad en +ari est +aph rag +Ġadd itive +ĠSta at +ĠN ept +ĠH AM +à¹Ģ à¸Ń +day s +Ġíĸ Īëįĺ +Ġvo ila +ĠÑħ л +ĠDeuts che +qu ir +O pen +Ġr anged +Ġle vers +ĠMans ion +p ared +ĠTit ans +ato ire +Ġeng ages +ye z +n aden +Ġobst ruct +ĠEmm y +åķ Ĩ +° ¥ +Ġtro ph +Ġtake aways ++ . +ty cznie +hés itez +Ġpod ÃŃa +Ġ주 ëĬĶ +Ġc itation +ĠAqu a +Ġdebug ging +в ан +Ġëĭ¹ ìĭł +ĠاÙĦ ÙĬ +Ġinstant aneous +ĠAut umn +Ġkep ada +Ġget an +h ini +ynth esis +ĠпеÑĢ Ð¸ +ĠM aced +P ac +unt u +B ra +ĠгоÑĢаз до +Ġ195 9 +ĠÑĤем пеÑĢ +Ġs ane +ĠO UR +as u +Ġ무ì Ĺ +Ġvalle ys +Ġlist ings +Ġprzed staw +Ġg ummy +Ġcort isol +ĠO brig +ĠAll ied +ож Ñĥ +Ġgén ér +Ġdoc s +ĠCh ili +ĠAb dullah +K it +Ġcontrib utors +го ÑĢ +л еÑĢ +Ġb inder +Ġmod èle +íħ IJ +Ġinte iro +m is +fer a +Ø§Ø ° +M ania +ĠíĻ ľëıĻ +Ġë´IJ ìļĶ +ĠJ az +ç» ĵ +ÑĸлÑĮ ки +r ishna +Ġêµ ° +Ġtaman ho +Ġappli ance +ĠRes istance +ĠL OOK +ĠHy p +ĠHe il +F ire +uj u +Ġhe als +Ġmal t +ĠVER Y +ĠÑħоÑĩ еÑĪÑĮ +Ġl inger +ĠN arr +ĠReg ular +ĠLo op +ĠL eno +Ġsort ie +ĠS erve +ĠìĿ µ +ĠL uego +itt ä +Ġund es +è³ ½ +å¦Ĥæŀľ ä½ł +Ġslipp ers +Ġon da +ĠÄIJ ây +Ġtap ed +Ġtra verse +Ġrelat ivity +ĠY oshi +c jon +il ated +act ively +ĠС ов +æĪij è§īå¾Ĺ +ĠP OL +Ðł Ðĺ +infl amm +cheer ful +Ġ×ŀ× IJ× +Ġ>> [ +min ster +Ġв ли +Ġident ifier +ĠLamb da +Ġtr os +Ġflaw less +Ġdetriment al +Ġbun ları +W ar +Ġreg ião +羣çļĦ æĺ¯ +ĠB ike +cess ors +Ġc ùng +ĠR N +Ġê½ ĥ +Ġküç ük +ĠBegin ning +íĺ ¸ë +Ġge we +Ġden ote +ĠAlber to +Ġprob iot +Ġo de +Ġmol ar +Ġburst ing +ass umed +Ġfoot prints +ved a +Ġstero ids +Ġfl aming +ĠE ller +Ġerk ennen +ät zen +Ġlife cycle +ĠD OU +ĠK arena +ĠGuer ra +è¿ĺ æĺ¯ +Ġsin ister +Ġpod éis +Ġpar ab +Ġok o +Ġmat éri +Ġcar ic +son aro +Ġpratic amente +ÑĥÑģ а +Ġcomun que +Ġvig ilant +Ġreg imes +ĠShoot ing +Ġra ids +ĠN ora +ĠW ieder +m ens +ĠÑģ од +Ġê²½ìļ° ìĹIJëĬĶ +Ġв Ñħод +Ġaut obi +ĠS chn +ĠRob bie +ĠF itness +Ġкон ÑĦ +Ġpeng uin +моÑĤÑĢ Ñı +Ġми ним +play s +Ġdeleg ates +M er +Ġsist em +ĠMicha els +m ale +ا ع +Ġcá ch +ĠH ä +Ġ×Ļ ×ķ×ĵ×¢ +Ġsuper power +Ġstr on +Ġro ver +Ġdé pend +éĻ ³ +Ġret iring +Ġvamp ires +Ġmer de +ĠCh anging +Ġt ame +Ġspokes person +Ġc ay +Ġfl irting +ĠGr ö +Ġw är +Ġwy b +Ġcoe ur +ạ nh +ĠìĻĢ ìĦľ +Ġconna is +ĠHundred s +ĠBe a +Ġα ÏĢ +pr uch +Ġsocied ade +ĠWh ilst +ĠK ait +esp ace +Ġch ia +ĠEr m +Ġë°Ķ ê¿ +Ġf ences +ĠM ortal +ê² ģ +Ġг ÑĢаÑĦ +ĠHom eland +ĠJ UN +is st +Ġpar lar +Ġsport y +é o +Ġdeep en +ĠBeh avior +éĢ ı +åĵĪåĵĪ åĵĪ +Ġer rand +Ġrot ary +ĠWell ington +W ind +Ġmes ela +ả ng +iend e +Ġex cell +ĠGen ius +ĠEdu ardo +æľī 人 +ĠÅŁ unu +Ġİ stanbul +Ġprod uto +Ġ ãħİãħİ +O FF +Ġwoll t +çĪ Ĩ +Ġëī´ì Ĭ¤ +Ġl ass +Ġher tz +Ġar omatic +Ġзв он +Ġaut oc +ĠL ust +Ġ11 2 +ĠÎ Ĺ +Ġreview ers +Ġrecept ive +å°į äºĨ +â nd +og lo +ĠìķĦëĭ Ļ +Ġn go +Ñĸ ÑĤи +Ã¥ t +con o +Ġtek rar +Ġ주 ê³ł +Ġgel miÅŁ +Ġbed time +ĠAr gh +AD A +ĠгоÑĢод а +ĠÄ ĩ +Ġall iances +g iggling +Ġyer de +Ġsp ies +Ġg utes +ç i +Ġallt id +ĠL ah +ŀ IJë +Ġdo kÅĤad +ÙĪ ÙĬ +Ġtoxic ity +Ġcancell ation +Ġ195 8 +d ro +Ġìŀij ìĿĢ +ĠMotor ola +Ġmult in +Ġenthusi asts +ĠM ighty +ĠCoc onut +: ãĢĮ +ĠPict ures +Ġsang re +Ġbl inking +ol esome +ĠìĬ¤íĥĢ ìĿ¼ +F P +Ġboom ing +ĠдеÑģÑı ÑĤ +Ġr atchet +Ġtim elines +len ess +Ġc ages +ĠGood night +omet imes +Ġc unning +ĠR isk +ul ed +d ade +Ġpr ata +Ġgust arÃŃa +am us +ĠJin ping +Ġest rut +Ġdescob rir +ĠM Äģ +ĠAll an +Ġ åĪĨ +Ġ×ľ× § +Ġpres erv +ĠStraw berry +Ä ı +L u +Ġk ro +ĠRep orts +ìħĶ ìķ¼ +Ġval t +Ġpouv ait +Ġapp ar +ĠB one +Ġprefer ably +ĠRep ública +å°± åΰ +Ġher zlich +Ġchim ney +Ġç ev +Ġvis as +Ġver r +Ġcultiv ation +ĠArmen ia +Ġвд ÑĢÑĥг +Ġcock ro +retch ed +art z +ĠлÑİд Ñıм +ĠpolÃŃt icas +ĠP anz +ĠA KA +ĠëĪ Į룬 +Ġer ro +Ġcam per +Ġ10 2 +ठ¸ +d one +Ġho ard +ĠÐŁÐ¾ÑĤ ом +je ong +Ġdest a +p ak +Ġin im +Ġgrow ers +ĠMess age +Ġele ctor +eng age +ĠFor bes +ĠCincinn ati +Ġdiffé rence +d f +Ġsp ar +Ġawait s +ĠUSS R +ĠR ising +ĠHo ÅŁ +Ġfoot ing +Ġcond iciones +ÑĤоÑĢ Ð¾Ð² +Ġclin ician +ĠDisk uss +å£ ĵ +ר ×Ĵ +× ¥ +ite it +g ren +Ġchar isma +Ġle uke +Ġirrit ating +Ġcir ca +ĠRhod es +Ġp ior +Ġhandic ap +roy able +Ġv ull +O G +Ġin ÃŃcio +ier i +Ġspl ashing +Ġdem ise +Ġassist ir +Ñĩ ÑĤо +Ġcover t +ĠG ud +ภī +kl är +ĠìŀIJ 꾸 +Ġver ändert +ĠR EM +ĠCon ven +at ge +Ġpierws ze +Ġcler gy +ling ton +l iv +V PN +ĠÑģ ожал +ĠH ate +ãģ¨ ãģĵãĤį +ÏĨ ο +ĠResp ons +оз д +Ġet mek +Ġchem in +Ùħ Ø© +Ġê°Ģ 족 +T re +Ġum as +ĠBur ton +Ġpatri arch +ĠSmithson ian +¥ ĺ +M oon +A ir +Ġmed ios +Ġer aser +Ġwoll ten +Ġpare il +ĠBill ie +æĬ ½ +еÑĢÑĤ в +Ġparl ament +Ġag ony +ĠQU E +sequ ently +An other +ĠWh ew +ĠAnn ual +Ġse ben +ìĥģ ìĿĦ +val ues +ŀľë §Į +Ġsin on +ere al +ĠEn light +ĠChem istry +ĠCatal unya +Ġdoct r +ant on +Ġst uk +ĠPl ate +ĠKardash ian +Ġfil os +ĠW et +Ġпоп ÑĭÑĤ +Ġunknown s +ĠSch on +ĠBald win +Ġtelescop es +ĠG ucci +ox ide +ĠConserv ative +ìĦ± ìĿĦ +Ġhina us +P ower +Ġê±´ ê°ķ +Ġprev ail +orm an +m achine +Ġ194 6 +Ġun bel +Ġsch aut +Ġp iel +e enth +Ġobject ively +Ġch akra +aud io +Ġch icos +ĠV ault +å° Ī +Ġmedic inal +ĠT ail +Wh ile +Ġas phalt +Ġfro ze +ĠE K +unch ing +n osis +20 15 +ĠG ri +Ġodd ly +ĠM är +ĠA eg +c olo +P ar +Ġëĵ¤ ìĸ´ë +Ġv inden +ĠO VER +Ġ iced +Ġsc orp +Ġha c +qual ified +ĠÑĥвид еÑĤÑĮ +erm o +H EN +Ġso i +Ġmulti ples +Ġlay outs +Ġblind ness +ĠB owser +Ġпод ÑĤ +ĠÃ İ +vention al +Ġm ata +mad ı +Ġge ez +Ġcad ence +Ġważ ne +ĠChrist ie +ven ge +C all +Ġturn around +Ġblo b +ĠЯ к +ĠVoice over +Ġper il +ĠJa ime +ĠH OY +l ane +Ġse bel +ĠDu o +ĠHistor ical +Ġd ni +Ġg ema +y k +Ġsab em +ắ ng +Ġv ars +ĠRon nie +ĠRon aldo +ĠPer què +ns inn +h air +Ġrelent less +Ġl yn +Ġtravel er +æĢİ麼 äºĨ +n ine +Ġant im +Ġì¼ Ģ +Ġsnow ball +ĠÑħаÑĢ Ð°ÐºÑĤеÑĢ +Ġintern s +Ġconstitu ency +ĠÐĿ ам +׾ ׾ +V EL +Ġvikt igt +Ġap oyo +ÙĦ ب +Ġj ard +Ġheight ened +ÑĢо ÑģÑĤ +ĠSM ITH +Ġдел а +Ġrepair ing +Ġr igt +ĠShe ikh +ĠBrit ney +Ġevery time +Ġadvent urous +oc key +er nt +Ġat aque +ĠAltern atively +e ffect +Ġpalav ras +ĠElli ott +Ġréuss i +Ġhypert ension +ĠMan ual +Ġproph etic +Ġhand c +ÑĮ е +Ġref rain +ĠSqu id +ìŀ ¡ +Ġком ан +äll en +Ġlleg ó +Ġbas h +ion y +ĠÑģк лад +Ġк аб +Ġcare less +ĠP ool +Ġtr ás +Ġfil s +ĠSch r +Ġsp rawd +ĠMon aten +Ġunfor gettable +ĠCott on +Ġinconven ient +ĠR X +or is +Ġhum bled +ת ×Ĺ +ĠØ¢ Ù¾ +Ġincre ÃŃ +ĠKomment are +èĪ Ĵ +r ación +Ġv antage +ĠSe al +ĠìĿ´ 거를 +Ġjou e +ãģĿãģĨ ãģ§ãģĻãģŃ +Ġìĺ¤ë ŀĺ +ĠиÑģп ÑĭÑĤ +ob en +Ġgr ate +Ġcontro le +ĠPer cy +ÅĤ ada +Ġsimult aneous +Ġprot oty +ĠgroÃŁ er +Ġbew usst +iniz i +Ġpass ieren +ĠHapp iness +åī ĩ +sh i +ge ht +Ġstation ed +ĠErgeb nis +Ġdirect amente +Ġsurv ives +Ġperson es +BER G +Ġvom iting +Ġconhe cer +Ġad jour +ĠCiv ic +pe i +bur st +Ġëĭ¤ ëĭĪ +é ı +Ġsl ed +Ġplataform a +ĠS ect +ĠDe fin +çĻ» éĮ² +én om +chn et +Ġprofit ability +Ġerre icht +á»ı i +c ation +Ġì§Ģ ê¸ +Ġperd re +Ġfel ony +Ġ195 7 +æĪij å¾Ī +Ġunsuccess ful +Ġnag yon +Ġelastic ity +Ġfac ade +Ġearth ly +ĠамеÑĢик ан +Ġcon n +c la +D u +Ġpolit iques +Ġhal o +iant es +Ġмо ей +ãĥ³ ãĥī +ton es +el ier +è® ļ +ht aking +Ġwicht ige +Ġan no +ĠL ok +ill ions +Ġv iver +Ġsol chen +Ġsu f +ĠSal z +ĠN vidia +z uge +ĠSp ike +V ideo +Ġtw or +ĠA la +èij ī +Ġh anya +ĠAd m +ìĿ µ +ĠPatient en +ĠOn ion +ĠKo be +ĠSc ene +ĠR ash +æ¨ Ļ +ÑĢа ÑģÑĤ +ist ani +Gen eral +le ye +imb ap +Ġconce aled +ĠFr idays +ĠW ool +Ġнов ÑĭÑħ +Ø´ ر +Ġê²° ê³¼ +Ġjed och +´ìĭ ľ +ĵ¤ ëıĦ +Ġìŀ¥ ëĤľ +uk t +L ou +Ġ먹 ìĸ´ +ĠEx pect +Ġдом ой +Ġirrespons ible +Ġac erca +ĠZ ust +ר ×ĺ +U I +Ġyout ubers +ĠPos itive +Ġsoci oe +Ġsn atch +èĥ Į +Ġrefresh ed +Ġnom inations +ĠP att +Ġobsol ete +Ġdem iÅŁ +åı ¤ +orm uÅŁ +ĠìĨĶì§ģ íŀĪ +Ġf la +Ġcra ziest +ĠZ ie +ĠT ú +z ep +ic em +Ġë©ĭ ìŀĪ +Ġcyn ical +ãģĿ ãĤĵãģª +Ġt resp +Ġcra z +Õ¥ Õ +Ġne lle +Ġm ph +ĠN ered +ĠK ob +ĠE ck +¨¸ ëĭĪ +J an +ĠТ огда +Ġde ci +ĠV og +Ġbubb ling +éĢ Ģ +ú a +Ġproduct os +iber al +Ġrepl icated +ĠImp rove +ill ary +C ha +Ġré du +ĥIJ íķĺë©´ +Ġcon not +ĠK rit +ĠдÑĥÑħ ов +Ġtread mill +ĠP W +Ġзов ÑĥÑĤ +Ġcl ams +Ġdra fting +Ġ195 6 +un ta +Ġexpend itures +ĠHoo ver +W OO +ÑĪе е +Ġded uction +mon ary +Ġreci b +Ġpo vo +Ġëį Ķë +ĠP AL +ĠBl ow +Ġwy p +Ġdest ac +de al +Gra eme +Ġnécess aire +Ġdamn ed +Ġ19 38 +Ġìĭ¤ ìłľë¡ľ +Ġtro op +Ġinsight ful +ĠT J +ĠоÑģ в +Ġf idelity +ĠSk ip +ĠMay o +ë§ Ŀ +app e +Ġbl as +ĠW Y +ĠG N +ct ar +S u +Ġcu ent +he ws +Ġcorps es +A bs +Ġwaste water +Ġc iek +ĠOn u +Ġexplos ives +Ġar ma +ĠSTEP HAN +polit ik +ĠOs aka +ta ÅĤ +Ġyap ıyor +Ġiz quier +Ġbele za +ĠWy att +åIJ ¸ +Ġsu k +Ġspec jal +Ġdan ke +wh istle +ĠfÃŃs ica +ĠHar riet +ĠìķĦ íĮĮ +Ġwill kommen +ip ing +ĠÑģмоÑĤÑĢ Ð¸ÑĤе +Ġмож еÑĪÑĮ +Ġinacc urate +Ġarrog ance +ĠRem o +γ ά +ass ed +Ġdeliver ies +Ġst inky +ĠпеÑĢ ÐµÐ¶ +j ay +Ġtrans itional +Ġr ere +ĠNGO s +ĠAT M +Ø® ت +i ology +Ġв лад +Ġsch me +ĠSh ine +ìķ ¡ +p ants +Ġser ge +Ġsen hor +Ġab duct +ĠBry ant +V ES +Ġawak ened +ĠL az +rop olis +ĠLa o +è¾Ľ èĭ¦ +Ġvill a +Ġsumm ers +Ġent hal +Ġ194 9 +V ia +Ġìĸ´ì ¨ +Ġtend on +Ġviol et +Ġintellect ually +Ġboun ced +ara us +Ġ19 19 +Ġvra ag +Ġsp el +ĠSch war +Sc ott +ĠInd o +Ġë§ Ŀ +Ġcanon ical +ĠI KE +Ġthat ÃŃs +Ġme llan +æ¯ Ĵ +ig mat +C ould +... ?) +Ġfo arte +ĠKum ar +rend o +Ġél é +à ´ +val uation +c ases +Ġintuit ively +h ong +ett ed +Ġsou ven +Ġmor b +Ġc ors +ĠN V +ĠHas an +æĥħ åĨµ +ie ved +Ġì§Ģê¸Ī ìĿĢ +Ġdum pling +Ġcontr ôle +Ġambigu ity +æ©Ł æľĥ +Ġco g +ĠScript ures +Ġc ai +Ġbe ver +大家 éĥ½ +Ġhu is +Ġa ime +Ġerkl ären +ĠL M +ĠF ey +éļ ¾ +à®± த +Ġsuper vised +Ġje we +s pl +ĠÑĨенÑĤ ÑĢ +Ġcoll isions +ÙĦ Ùģ +ĠHog warts +ĠDur ham +×ķ× £ +Ġphosph ate +Ġoverse e +Ġinspect ions +Ġbr inc +ĠZ ak +Ġpay off +Ġch aud +ĠHung er +ã os +v ir +Ġf iance +Ġb oug +l ived +c ry +åĽŀ ä¾Ĩ +Ġjoint ly +Ġgirl friends +ĠNe xus +¦¬ ê²łìĬµëĭĪëĭ¤ +ĠK wang +åĵĪ åĽī +å§ ij +ÅĤ ÄĻ +ĠN eden +ie ce +Ġins erting +æŁ ĵ +ĠM ummy +ĠGlo be +Ġle e +Ġg erman +Ġcre ams +ach o +Ġch ưa +ĠGal ile +Ġfür s +Ġest iver +c idos +Christ ian +Ġlors qu +Ġcut est +v ale +ĠкÑĢ ÐµÐ¿ +Ġw ary +Ġslic ing +Ġesper ando +ĠV ander +ĠDe ixa +Ġ195 4 +Ġmów iÄħ +Ñĸ ÑĶ +Ġtool ing +Ġrest or +Ġpos ición +Ġintent ar +ĠAp ache +OU L +ĠÙĪ Ø¨ +Ġmat ière +ãĥ¼ ãĤĵ +Ġl inen +Ġestrat ég +ĠMut ta +é¡ ¯ +è¡Į äºĨ +Ġpart ing +Ġminim izing +Ġapp rendre +æľ Ŀ +Ġан глий +ĠDo o +ĠFire fox +c ómo +Ġge opolit +Ġmak an +Ġmog elijk +ĠÏĢε Ïģι +Ġcá» © +Ġinstall er +Ġdib uj +ĠHe ath +lo op +ĠBro ken +HY UN +sh elf +Ġf izer +Ġenh ances +ä¾ĭ ãģĪãģ° +Ġдо ÑģÑĤи +ĠP UB +ĠKolleg in +Ġatt ained +Ä ¾ +Ġmist ress +ĠOft entimes +×ŀ ×Ļ×Ŀ +Ġbe we +ĠS ora +ra uen +ba um +Ġroll ers +Ġm ering +ĠP AC +Ġн Ñĸ +ĠRép ublique +ĠÑĤ ÑĢав +ĠV anguard +uc iones +Ġ무ë ĮĢ +Ġg our +¯ ¤ +ĠÏ ī +Ġsa una +Ġpe ine +ĠVal erie +ĠS ikh +fend imiz +ber o +ĠÑĩ и +Ġdo ÅĽwiad +ĠE uros +Ġcomment aires +Ġtwe aks +ĠF aster +ĠÑĢаÑģ к +Ġprogress ively +ĠE uch +bor o +ĠIng red +C ap +Ġun check +Ġìĺ¤ë ¥¸ +Ġw re +ĠF T +ör ung +Ġmemor ized +ĠD inner +ĠP hew +ou bl +Ġput a +Ġadm its +ез де +op od +Ġpand a +Ġhing es +ci pe +Ġtrans act +Ġpod ia +Ġp ics +Ġcriter ion +ĠOrchest ra +ĠBl og +Ġsolem n +ĠPix ar +Th ree +Ġв низ +ĠVol unte +ĠSav age +ĠPV C +ĠC af +Ġwy kon +Ġgrad ers +Ġcr ouch +Ġcl iche +Ġsoy beans +ĠM UR +ĠGonz alez +ĠM imi +ĠBol sonaro +Ġdi aphrag +Ġbil ang +ëIJĺ ëĬĶ +éĤ£ æĪijåĢij +Ġregul ating +M c +J udge +Ġн ож +Ġjak Äħ +ites se +ĠW ij +Ġl ata +gro aning +POS ING +Ġ×IJ×ķת ×ķ +Ġha ga +Ġground ing +Ġviol ently +Ġt ills +Ġeng ag +ĠHo llow +Ġпоп ÑĥлÑıÑĢ +Ġw prowad +Ġrepl aces +Ġfluores cent +urg ical +igg ly +ĠTrad itional +t te +ĠÙĦ Ùĩ +Ġphosph orus +Ġapr on +ĠWat ers +ĠK ultur +ав ай +Ġol ives +Ġ×Ķ×IJ× ľ +Ġteil weise +Ġsen cill +Ġprend s +Ġnarr ower +Ġj ätte +ĠInformation en +ìĥģ ìĿ´ +Ġstar ve +Ġfr ick +ĠBe weg +ठ² +Ġdolph in +ĠLAUGH TER +ĠINTER VIE +åĶ ī +Ġyan lÄ±ÅŁ +Ġtor pedo +Ġshort ages +ìĿ´ë ĵľ +ıld ı +Ġp aws +Ġo zone +Ġcultiv ated +ĠF ot +Ġnot or +н оз +Ġко ÑĪ +Ġtouch screen +ĠAll y +æľĢ è¿ij +Ġë§ĽìŀĪ ìĸ´ìļĶ +ĠС еÑĢ +Ġв полне +Ġpap rika +ĠDust in +Ġefect o +Ġop ini +Ġmu ut +Ġhá»į c +Ġinter ject +ÄĻ t +Ġbut ts +ure z +ĠP ike +ĠH ok +ĠGu inea +ĠCath edral +Ġ14 00 +C ra ++ , +ë§ Ľ +³´ë ıĦë¡Ŀ +aby rin +Ġvide og +Ġо ÑĢÑĥж +Ġu ž +Ġbus cando +ĠAss istance +éĻ ½ +Ġmel hores +ì¡ ´ +Ġëģ ¼ +ĠR J +Ġت Ùħ +Ġo min +Ġmotor cycles +ĠS app +Ġsupply ing +ĠAl gun +Ġaer ospace +×¢ ׾ +oc cup +le ist +Ġê±° ëĬĶ +Ġcomplet a +b res +! ( +ĠÐŁÑĢ ÐµÐ´ +Ġdisadvant aged +ĠAtt end +ĠJud ah +á»ĭ ch +yl ene +act ly +Ġset ups +Ġammon ia +ĠSchwe iz +ĠSh ame +Ġband e +ĠF uel +Ġtroubles ome +Ġnum ero +ĠM OM +ĠпÑĢед лаг +ment ioned +ĠболÑĮÑĪ Ð¾Ðµ +ĠVikt or +ĠSty les +Ġcruc ified +ructure d +en viron +Ġmor als +Ġmed itating +Ġax ial +is ance +ĠAb st +G reen +Ġê± ´ì +Ġquad rant +Ġper gi +Ġcamer aman +ĠSe qu +Ġpa used +ĠLa ughing +ê· Ģ +? .. +ĠÅ» e +Ġpermit ir +Ġdetect ors +ĠH UD +av al +ĠìĹ¬ê¸° ê¹Įì§Ģ +Ġh ubs +Ġbest immt +ĠбÑĥдеÑĤ е +INTER POSING +Ġten gan +Ġcra ve +ĠBundes regierung +ĠBlo ody +Ġus ability +ĠE as +ĠÄijá»Ļ ng +Ġ195 5 +Ġkrie gen +Ġhabit ual +Ġessential s +rim inal +Ġroomm ates +éĤ£ å°± +ĠпеÑĢе Ñħод +Ġng hi +Ġmen ing +ĠSym phony +ĠH ug +ag gi +Ġw ied +Ġmit ad +ãģ£ãģ¦ ãģĦãģĨ +te enth +ida Äĩ +S ave +Ġrob iÄĩ +Ġboun ces +° ĸìĹIJ +st ars +Ġprag matic +Ġcogn ition +Ġwra pper +Ġw arten +ad h +Ġpens a +ĠHert z +Ġn ÄĽ +ĠRe id +ĠPC s +ĠMo le +Ġ.. ... +Ġpre cio +ĠChampions hips +ê°Ģë Ŀ½ +Ġv ér +Ġcorrid ors +ĠElect ronic +S l +Ġа ле +Ġoverth row +Ġk abul +ĠR ES +ĠCyber punk +ог од +ĠÐĿ ав +Ġw an +Ġmanifest ations +Ġcual es +ĠW ise +ĠLös ung +Ġex fol +Ġearn s +ÑĥÑģÑĤ иÑĤÑĮ +Ġsa pp +ĠBra un +ĠBRAND ON +ì¹ Ļ +Ġs ano +ĠF EL +Ñĭв айÑĤеÑģÑĮ +ожд ениÑı +Ġse wn +F un +Ġrecipro cal +Ġexpans ive +ĠTra ffic +Ġktóre go +ĠÙĪ Ø³ +æĺ ¥ +Ġë¹ ¨ +pro ve +ig are +Ġlo h +Ø§Ø ¶ +H ope +Ġdevote es +ĠG om +Ġste als +ĠU ms +ĠTw ice +ãĤ ² +iy im +Ġrhythm ic +ĠV orte +Ġpref ix +om ination +Ġdat o +Ġcust ard +ĠVO ICE +å· ŀ +Ġmen y +ist ors +Ġíĺ ij +ĠìĤ´ì ķĦ +Ġíĥ Ħ +Ġk ort +Ġab a +ĠV era +ep y +Ġì¹´ë©Ķë Ŀ¼ +Ġsubmer ged +ĠC lock +Ġthumbna ils +Ġbo ast +ĠF are +!! ] +ĠÅĽ m +Ġkaik ki +ĠTechn ologies +ìĻ ¸ +ãĥ Ĵ +иÑĤ ай +å°ı æĻĤ +Ġа ÑĤ +Ġkn obs +Ġre icht +ượ ng +gl io +Ġë§Ľ ìĿ´ +ê°IJ ìĿĦ +Ġjot ka +ĠHand y +ĠHab en +n ous +Ġin land +Ġam azon +ho oting +S L +Ġle isten +~ " +Ġprov oke +ĠTw ist +Ġ×ij× Ĺ +Ġdepart ed +ê° ľë¥¼ +Ġk onse +ĠCar wyn +íķĺ ìĭł +ident al +ES CO +Ġt teokbokki +Ġdiz endo +ç· ´ +ınd aki +imas u +af ar +Ġland fill +Ġcorrect ing +Ġcle ars +ĠNum mer +H AM +Ġcart ridges +ĠDies el +p aced +Ġobl iv +Ġmoy ens +ĠSin ne +ĠPre is +il iz +ĠÑģм ож +Ġbroad en +ä»ĸ æĺ¯ +x es +Ġcarbohyd rate +íĺ ¹ +se ok +Ġecho es +Ġc ess +ë° Ķ +Ġб изнеÑģ +Ġllam ado +Ġess ent +ĠìĿ¼ë °ĺ +ĠA ires +ph en +Ġze bra +Ġsymbol ism +On ce +Ġr acks +ĠKaf ka +ĠÑģеÑĢÑĮ ез +Ġsin n +p icious +ka a +Ġmotherf ucker +Ġapprentices hip +Ġr pm +Ġtax ation +Ġfur ry +ĠSac red +ĠÑĢаз м +por a +eng es +ĠíĹ Īë +ĠÑģ ин +Ġsanit izer +Ġcr inge +ĠS ca +оÑĩ но +Ġof ere +Ġmel odies +ĠVel vet +ĠIhr er +ĠHy brid +ĠG iov +Ġirgend was +Ġdep ende +ĠUs ers +Ġh ump +dri ving +Ġs f +Ġruth less +à¹ĢภĦ +Ġlem ons +Ġfö ret +ĠO j +Ġм ама +Ġinter personal +Ġge v +Ġab norm +иÑģ л +Ġин д +Ġkont roll +Ġreg res +Ġled ge +Ġerzäh lt +ĠT act +Ġarri vé +Ġsubstant ive +Ġspoon ful +zw ischen +oooo o +Ġconten ido +Ġbes l +á»ĥ m +k ten +Jam ie +Ġsand y +ä¸į åIJĮ +â ĭ +Ġp ase +Ġdet te +ĠBelg ian +ê° ľë +ula res +r ud +ig or +ĠíĮ ¬ë +Ġremed ies +Ġblast ing +ĠS ich +Ġож ид +Ġmon str +Ġmanif old +Ġglaub en +ĠE ST +Ġstream line +Ġlobb ying +ĠGoth ic +to ire +.. ' +Ġdém ocr +Ġнаб лÑİд +Ġwsp ól +ĠczÄĻ ÅĽÄĩ +ä¸ĭ éĿ¢ +is és +g angen +Ġbez pie +rem lin +ê° Ŀ +St ill +Ġres ides +Ġgele cek +Ġtélé phone +Ġpe wn +Ġle opard +Ġcompliment ary +Ġc rib +ĠAnim als +Ġge il +ess el +Ġgard er +Ġcatch y +æ¨ ¹ +ĠE ts +ĠCom mercial +ĠD ENNIS +ĠCoordin ator +ĠAb igail +ffff ff +ấ p +Ġpeque ña +Ġinject ions +ce kt +Ġphilanthrop y +Ġp uck +Ġcelebr ates +ĠD unk +ĠD latego +ãģ¾ ãģł +δ ή +grad uate +ĠM obil +t ill +ac am +Ġyol ks +Ġtang led +Ġman iac +Ġoblig ed +ĠLa ink +Ġver der +ĠDam on +Ġmut ant +Ġhop ping +Ġre ins +Ġinver ter +Ġcont empt +׳ ס +le arning +M iss +ĠÐĵ оÑģ +ĠMe yer +ê»ĺ ìĦľ +é£ İ +×ķ׳ ×Ļ×Ŀ +ask ing +Ġtrim ming +Ġtre asury +Ġs ente +A ust +ĠUnterstüt zung +ĠCom edy +ĠAn akin +é ¹ +ÑĢÑĥ ÑĤ +ĠH ari +ograph ers +Ġoat meal +ĠB ots +ä¸į äºĨ +Ġп алÑĮ +Ġacknowledge ment +x ic +Ġê´Ģ ìĭ¬ +gas ping +Ġãģ ķ +Ġterr ace +Ġor naments +ĠM ER +comm ittee +ĠìĹĨ ìĬµëĭĪëĭ¤ +Ġr ij +é ³ +צ ×Ŀ +le me +Ġlibert ies +Ġfell as +ĠCop per +ben ch +ĠIde a +á»į n +ÑĪ Ð° +Ġvers ión +ÏĦο Ïį +ĠÐľ и +ĠпÑĢил ож +Ġbox er +ĠT anner +ĠM oy +ì¹ĺ ëĬĶ +T hr +Ġtin ham +Ġpol ishing +Ġconsequ ently +Ġamen ities +ĠK I +ĠGRE EN +ĠFrank ie +н иÑĤ +itt el +Ñģ кое +urs ed +Ġup bringing +Ġth ứ +ĠìĭĿ ìľ¼ë¡ľ +Ġwh im +Ġchin ese +conf idence +ĠJ eder +ãģª ãģ®ãģ§ +aj cie +ĠT ous +ĠPow ers +ừ a +other mal +ĠвÑĭ ÑĪе +r ale +Ø§Ø ® +Ġì§Ģ ìĽIJ +Ġép isode +Ġsul ph +Ġenc ara +k raft +alar ı +ĠCom es +Ġdiv ul +ĠRud olph +ĠM use +Ġut ens +ĠìŀIJ 주 +Ġp ana +ĠVeget a +ĠPH P +ĠN SA +ent in +ĠCarne gie +ا ÙĬ +iÄĻ cy +H arry +Ġf ır +С п +Ġglad ly +Ġaver aging +íķĺ ê²łìĬµëĭĪëĭ¤ +лÑı ÑİÑĤÑģÑı +ĠÐľ енÑı +Ġquot ation +ri res +itch ens +ay ed +Ġun att +ĠP erez +ĠоÑĤ меÑĤ +Ġtact ile +ĠEu h +is ini +b uh +Ġhat ır +ĠìŀĪ ìľ¼ +Ġpolicy makers +³´ì Ħ¸ìļĶ +ac ı +Ġκ ι +Ġregister ing +re to +ĠSpr inkle +ĠGram my +ax ter +Ġб и +Ġsit ter +Ġpred ic +Ġthin ly +Ġstr um +Ġag grav +Ġa ha +ر ج +m ellow +Ġconst ante +ĠL aut +ist on +Ġtransition ed +ĠCamb odia +ãģĦ ãģįãģ¾ãģĻ +è·Ł 大家 +art ed +Ġmis f +ĠPunk te +Įë ĵł +Ġtremb ling +Ġges pannt +ĠعÙĦÙĬ Ùĩ +Ġникак иÑħ +Ġë¶Ģë ĵľë +ĠÑĢазв иÑĤ +Ġit chy +Ġc iento +Ġpl ains +Ġk ittens +Ġback log +ĠPres iding +pt a +Ġha voc +ĠDarr in +ĠÐĽÑİ Ð± +Ġsegreg ated +Ġg hetto +Ġerle bt +Ġdrug iej +ĠSi xt +åı ĥ +ร ะ +uen cia +Ġíķĺ 기 +ĠëĨ į +Ġrob i +Ġpione ers +Ġmilli ards +ĠWitch er +Ġ무ìĹ ĩ +or ro +m ass +Ġdiver gence +ĠRiver a +ĠNo odles +Ġend roit +ĠK osten +ĠдÑĢÑĥг а +ĠmÃŃn imo +ĠKazakh stan +ت Ùĩ +Ġвоз дÑĥ +Ġgesch rieben +ĠN il +Ñģ ки +ĠFr üh +Ġbever ages +æº IJ +ĠG on +æĺ ¨ +Ar in +ĠInt ro +ocaly ptic +Ġexhaust ion +ĠStat us +ĠBatter y +és z +£ ¼ë +air y +Ġë³´ìŬë ĵľë +Ġdispar ity +Ù Į +ĠTuc son +Ġbright ly +pro blem +Ġbiom ass +éĻ į +§ ī +Ġhur dle +Ġwavelength s +Ġ< < +Ġteam ed +FF FF +ĠS lim +om ial +Ġunve iled +ĠVere in +ÙĤ Ø· +est ry +Ġcl ás +Ġch eddar +Ġaccus ing +ĠScient ific +ĠбÑĥд е +ĠCyr us +ε ÏĦε +Ĩĵ ê³ł +Ġë³ Ħ +Ġcur d +Ġrefer rals +sh ift +åį ķ +nik ów +Ġm ier +Ġconf ronting +ê²ĥ ëıĦ +aw l +Ġtry in +Ġê·¸ëŀĺ ìļĶ +Ġch iar +Ġìĺ¤ëĬ ĺëıĦ +æĶ¿ æ²» +es que +Ġmism os +ĠSh ak +Ġsoci aux +Ġpi ÅŁ +ĠkiÅŁ i +Ġcy an +h ay +be w +b od +ĠÎ ¹ +ĠMain ly +Ñİ ÑĤÑĮ +hab itude +ĠÑģп окой +è·Ł æĪij +Ġpre con +ĠM andy +ðŁ¤ £ +ill os +Ġgr upp +Ġcr umble +Ġconstru ctor +erv ices +Ġlight house +ĠCon cept +ан ÑĤи +alt ro +h ope +ĠAll eg +ìĸ´ë ¥¼ +pie ces +oun ter +Ġíķĺ ëĭĪê¹Į +ĠìĿ¸ íĦ°ë +Ġvérit able +Ġthread ed +bl ind +Ĥĺë Ŀ¼ +Ġtr ays +ĠEd ison +ĠÃĸ z +ĠSte vie +Ġl ender +Ġbrig ade +Ġdeuts che +m uffled +b art +Ġinsan ity +Ġsav vy +Ġsens ational +Ġdere chos +ĠM X +ĠпÑĢ ÐµÐ¿ +Ġthreat ens +Ġrealt Ãł +Ġindic ative +Ġch ops +Ġbenef iting +ĠVern on +ĠSt rand +n un +qu ently +10 1 +Ġe el +ìĪ Ļ +r ints +ĠÙħ س +Ġب د +Ġпо ÑģÑĤÑĢо +Ġyap mÄ±ÅŁ +Ġol ması +Ġi edereen +ol é +ke f +Ġë°ľ ìĥĿ +Ġr ained +Ġalm ighty +ĠвÑĭ д +ĠC PR +F re +Ġinhab ited +Ġarb ets +Ġa kin +а ÑģÑĤв +v ania +Ġhäuf ig +ĠMat te +s orry +Jen ny +ĠгÑĢ Ð°Ð´ +Ġwh it +Ġbro kers +å¯ Ł +Ġh ine +ast en +Ġг ÑĢÑĥ +M B +ĠP RI +S ab +Ġwrest ler +Ġfacil itating +Ġeh kä +ĠC red +Ġ12 7 +Ġnot hin +Ġmand ated +å¯ Į +ÑĥÑĤ ÑģÑĤв +F rank +Ġwor s +Ġdzie ÅĦ +ĠUnder ground +Ġznaj du +ĠB ä +ĠPrin zip +аÑĤ елей +Ġveter inar +Ġsplend id +Ġroz p +Ġpsych opath +ig on +Ġh ops +Ġc ần +ĠX ian +Ġtro isième +Ġproduct o +ĠdeÄŁ er +ĠContin uing +ив ал +c ık +Ġmoistur izer +Wh ite +Ġsi is +ĠEver est +ien ced +Ġcả m +ĠJ apon +´ìł Ħ +Ġten ÃŃan +Ġenc anta +M m +Ġdrop down +ĠI ya +³´ë ©´ +Ġword ing +ĠSque eze +ĠMap le +Ġclar ified +ĠMun icip +ĠRou ge +ĠNick i +ĠGo o +v olt +t ek +fect ure +f red +ar rive +ãĥ¼ ãģĦ +te z +E p +Ġob ras +ĠV ID +ĠR iv +ĠMod i +i be +Ġacontec endo +Ġim itation +Ġcamoufl age +Ġspan ning +ĠSEC RET +ĠOre o +ìĨĮë ¦¬ +Ġh unch +Ġca ÅĤe +Ġspont aneously +ĠPer d +Ġet ap +ĠHo le +ĠDis ability +Ġafter life +æģ © +Ġtest ified +Ġpres up +Ġpet roleum +Ġcontr ario +ĠAss essment +ÄŁ lu +Ġp ests +Ġdil ig +ĠвÑģÑĤÑĢ ÐµÑĤ +Ġcons équ +Ġcann ons +Ġcan oe +ĠM ile +Ġcit oy +Ġbe gged +ĠMin nie +ÅĤy ch +Ġprinci pe +ÏĢÏĮ ν +m niej +Ġw ert +Ġëĭ¤ë ĵ¤ +an se +Ġunc les +Ġprovoc ative +Ġinter sections +Ġdemocr ats +ĠJul ius +ин ки +yg usal +Ġ׾ ×ķ +Ġgj orde +Ġg asket +ĠB ock +Ġİ n +b reat +ĠEqu ity +ard ı +Ġкан але +Ġд ней +Ġt Ỽi +Ġfi xture +Ġab uses +Ġv aya +Ġou vert +Ġmultic ultural +Ġcontext o +ĠSes ame +Ġdé pl +Ġcons omm +ĠPart e +Ġp em +ĠCon an +Ġб ÑĸлÑĮ +Ġpersu aded +Ġdra ins +M oo +F ORE +Ġб аÑĤ +Ġf od +ĠProduct s +ì§Ħ ì§ľ +Ġ" [ +ĠW ick +ĠNar uto +н али +ry w +Ġl odge +Ġin h +Ġvont ade +Ġdi j +ĠJes ús +Look ing +Ġfore arm +ĠIntegr ation +ĠHARR IS +Ġtool bar +le ader +Ġsel dom +Ġб ÑĢоÑģ +ĠK ook +он д +Ġmon opol +Ġmill et +Ġl ira +ĠAs ians +Ġ18 90 +ci ÄŁim +Ġed en +ĠIKE A +ĠNeigh bor +ĠKazu ya +ü d +Ġpsych edel +Ġenvision ed +åĿ Ĺ +Ġï· » +Ġw under +ĠBulgar ia +B rid +Ġmar row +Ġdep iction +ĠT in +ĠPhar ise +Ġeinz ige +Ġblind ly +ãģĽ ãģ¦ +Ġdef ens +D ire +Ġvibr ating +Ġtroll s +Ġdisrespect ful +Ġw od +Ġstimul i +Ġcreep ing +Ġcla irement +Ġsc ariest +Ġdécouv rir +Ġ10 4 +ĠвеÑĢ Ñħ +ĠÅĤ at +Ġróż ne +Ġbar ley +ĠRe pl +ĠT we +k ke +ĠãģĿ ãĤĮ +ĠRed mi +ĠMet roid +Ġή ÏĦαν +Che ck +ĠS EN +Ġ ido +ÑĤоÑĢ Ð¸Ð¸ +ó p +UN KNOWN +Ġänd ern +ĠJu ice +ĠGes icht +å°± æľĥ +ĠнаÑģÑĤ олÑĮко +íĥ ķ +Â Ń +ex hales +Ġì´ ī +Ġj sem +ÏĢ ÏīÏĤ +Ġit t +ëªħ ìĿ´ +Ġrem ix +Ġbloss oms +ĠR enee +is ations +ìĬ¤í Ħ° +Ġë³´ ìĿ´ëĬĶ +uest as +op edia +ĠA im +ìĿ´ì¦ Ī +sc ene +Ġleak age +uck t +S ad +A sk +Ġsusp ense +Ġimp ost +ĠStrateg ic +ĠIt ÃŃs +âĢ Į +Ġkey boards +Ġam using +og r +id erman +ŀ ĸ +Ġв ижÑĥ +Ġd ips +Ġapolog ized +ĠST AR +Ġesc uela +ĠC hing +н ениÑı +Ġë¶Ģë¶Ħ ìĿ´ +ĠFle et +Ġs amb +Ġentsprech end +Ġelectrod es +ĠFrei heit +æĪij ä¸įçŁ¥éģĵ +ĠSh rim +iÃŁ e +Ġselect ions +Ġfor di +Ġd oss +Ñı Ñĩ +Ġdiscrimin ate +ĠAu ÃŁerdem +Ġdesenvol v +ĠIntern al +ĠBened ict +å¯ Ĩ +ĠSh iv +M issy +Ġоб наÑĢÑĥж +Ġна ÑģÑĤÑĢо +Ġcontrol ar +ĠL ia +Ġopio ids +ant u +Ġcup board +æģ IJ +г е +acht s +Ġcur ated +Ġx em +Ġwe ary +Ġbre thren +Ġbudget ing +Ġpour tant +éļ » +ais ia +ĠоÑĤв еÑĩ +ĠG IS +μ αι +Ġש×Ķ ×ķ×IJ +Ġsa ud +Ġl Ỽ +Ðķ Т +ub ine +ĠнÑĥж ен +Ġkidna pping +Ġbr at +ĠTer re +ĠMon et +Ġë§Ī ìĬ¤íģ +Ġflash y +ĠIS BN +Ġfreel ance +i age +Ġjun ge +ì¶ © +cer al +ĠÑĤоÑĩ ки +Ġform ulate +ĠF ER +ĠDart mouth +ìľ¼ë ©´ìĦľ +å¢ ĥ +ow iÄħ +ĠëĶĶ ìŀIJ +Ġreg iment +Ġmetabol ismo +ĠP arr +Ġì¶© ë¶Ħ +Ġsan ity +ĠL al +ĠG ö +ĠG la +Ġprot o +Ġmicroscop ic +Ġk ang +ĠSc alia +Ġp ug +ĠSc ore +ĠSav annah +Ġgard e +ĠN OR +å°į åIJ§ +Ġsche int +Ġp óÅĤ +Ġcor ri +Ġbr ute +Ġ ÅĤad +ä»ĸ 们 +Ġsucceed ing +Ġbicy cles +N on +Ġseek ers +Ġuncond itional +Ġrhy mes +ĠGar age +Ġinv oice +Ġcan vi +ne ck +Ġcustom izable +irit ual +Que en +íķĺ ìĭľëĬĶ +Ġpower less +Ġcs ak +ä¸į ä¼ļ +is oft +Ġìłķ íĻķ +Ġnh ân +ĠM AND +ĠH af +Ġrevol ves +ä¹Ł åı¯ä»¥ +ov an +ar oo +ĠGr ind +éĽ ª +Ġindispens able +Ġconsult ed +ĠClin ical +A cc +Ġol hos +Ġmon ter +ĠH ana +et ah +Ġva an +Ġt igers +Ġcau cus +ðŁĺ Ĥ +³´ì ŀIJ +pow ers +ium s +ĠíĨ łë +Ġtrad icional +Ġreson ated +Ġìĭł 기 +th em +Ro bert +Ġelement o +Ġant id +Ġоб Ñģ +Ġnat ives +Ġlo ca +ow ment +ĠT ight +Ġ æĢĿ +Ġmel an +ĠN ue +am is +Ġsor gen +as ına +H ome +ĠPUB G +Ġaw fully +ĠSh ore +ĠPer ché +ĠL au +ĠCind erella +ĠCh est +Ġsem antic +Ġdesert ed +ĠMom o +ĠHern andez +gen es +ĠAd ult +иÑĩеÑģ кого +osh ima +ĠcaracterÃŃst icas +ĠK L +´ìŀ ¥ +oc ar +Ġfeh lt +Ġd ruk +ĠPop py +EN GLISH +ĠVerg leich +B rien +Ġrec omp +ĠÑģ д +Ġmer ger +Ġmarket ers +Ġhoney moon +Ġpen so +Ġbell i +еÑĤ Ñĥ +Ġbank er +Cam era +ĠSt all +ĠSt amp +ĠB ite +еж де +Ġs ür +Ġgü ç +ĠPas sover +ĠBug ün +ĠÑģожал ениÑİ +Ġн из +Ġman ure +Ġglac ier +è« ĩ +RA Y +ter ror +Ġsal ads +Ġhur ricanes +ĠDesign er +ator io +Ġfact ual +ĠTam my +Ġзв ÑĥÑĩ +Ġintrodu ctions +Ġhouse keeping +Ġh anger +ëĭ ĺë +ak te +ĠCol a +' ] +ĠG ender +оÑĢ Ð¾Ð½ +ip se +ic ias +Ġsuccess ive +Ġpolit ic +Ġhö her +ĠQ iao +ĠG imme +Ġл ож +Ġse b +ĠWe iter +ĠSak ura +ĠB oulder +ĠAm érica +peÅĤ nie +Ġtecn ologÃŃa +ish ops +f ur +Ġmoon light +Ġdispers ed +Ġre z +ен ное +алÑĮ нÑĥÑİ +ĠTw elve +ĠH OR +ìĭ¤í ŀĪ +il age +Ġshad ed +Ġres umes +ĠPe anut +ĠM ILL +ap ons +ĠU FC +ĠSo le +Ġjoy stick +ĠOliv ier +war ming +Ġsyll abus +Ġоб Ñīе +Ġhi á»ĩn +Ġfest a +Ġcr adle +ĠZ ac +Ġremem brance +Ġê°Ļ ìķĦìĦľ +ĠpiÄĻ k +Ġco exist +ĠV II +Ġá reas +Ġu waż +Ġobser vers +Ġmännisk or +co on +ĠD AM +Ġnas zym +Ġall igator +ĠFree ze +ĠEst ate +ĠÑĤÑĢ Ð°Ð´Ð¸ +Ġunder cover +Ġn ies +ĠFeh ler +pl in +ĠK abul +il ate +Ġê³ł ìĸij +Ġm op +ìĦ ¼ +Ġand erer +ĠK ELL +ок и +Ġж еÑģÑĤ +Ġgra zing +Ġda ÃŃ +Ġcapital ize +Ġa pex +Ġnurt uring +Ġcort ar +Ġcontr ac +ımız ı +Ġtand em +éĥ½ æľī +ge ment +ĠÑģиÑģÑĤем а +Ġman que +ia jÄħ +W OR +Ġا ب +Ġcart s +AN O +Ġë°Ľ ê³ł +ĠC ena +ĠBi ology +id ar +Ġa ż +er ne +an u +Ġthank ed +Ġsubmar ines +Ġman ic +Ġм оз +ä¼ Ĭ +inst ant +ess ential +Ġsam urai +Ġpast i +Ġal an +Ġbro ch +Ġb aker +ĠGu ill +¨ ¼ +Ġwithd rawn +ëĭ Ŀ +Per fect +qu ency +Ġstream lined +Ġ13 00 +´ë ıĦ +Ġëĸ łë +Ġãģ¯ ãģĦ +Ġh vad +ä¸Ģå®ļ è¦ģ +Ġverb ally +ĠK ons +Ġì¡° ìĭ¬ +Ġdie z +æİ° æİ° +Ġchuck ling +ĠM ih +Ġrall ies +Ġman ter +Ġearn est +s uper +Ġge ce +ĠR end +ĠGer ade +jen igen +ĠV all +Ġìŀ ĪëĤĺ +ĠÑģказ ала +Ġtrabal h +ĠнаÑĪ ÐµÐ¼ +Ġм еÑħ +ik it +Ġnoun s +Ġneurolog ical +Ġmotiv ational +ĠMcM ahon +ĠFin ished +Ġë³´ ìĿ´ +ĠField s +Ġadoles cents +ĠT isch +ĠNe ben +ĠFl owers +ĠEner g +Ġdire t +ĠTh i +ĠP icas +æĥ ľ +æĢİä¹Ī æł· +Ġav ete +ĠF ors +ĠChap el +N ão +E t +ĠÑģод еÑĢж +ren o +Ġs ven +Ġdost ÄĻp +ne e +ĠSnap dragon +ĠID s +ìķĺ ëĬĶëį° +ר ×ļ +Ġsun flower +Ġperpet ual +ç³ ĸ +Ġkn ights +Ġg ird +ĠTo ld +Ġvolcano es +Ġadvers ary +ĠEconom y +Ġextra pol +Ġbl uetooth +Ġzoom ing +Ġsk ys +Ġgen ial +ÃŃcul os +amb re +Ġм еÑĢ +Ġteen y +Ġstress ing +ìķ Į +ON Y +Ġtransluc ent +Ġround ing +Ġgr ues +×Ļ׳ ×Ķ +ap rès +Ġprue ba +Ġpoly gon +Ġblue berry +ĠProgram m +Ġtren ches +Ġse bagai +Ġpal ate +Ġla ude +Ġbehav ed +Ġlongitud inal +ĠMod ule +Ġadm ir +λ ι +G reg +Ġwy st +Ġpropag ate +Ġmold s +ĠT ub +ĠL oud +ust o +Ġun stoppable +Ġreinfor cing +éĿŀ常 çļĦ +ĠпÑĢоблем а +Ġpot encial +Ġhe mp +ìŀ Ķ +ठ¯ +Ġopt ic +Ġerfolg reich +Ñģ Ñĭ +олÑĮ ÑĪе +ur st +ĠPo is +Ġrespond ents +Ġneh me +ĠEx ternal +ol ate +H yun +Ġquart z +Ġmathematic ian +Ġbás icamente +Ġa il +ìł ľë¥¼ +att utto +Ġno oit +Ġaff lict +ĠOl ga +èŃ · +Ġна ÑĤ +Ġd ites +Ġreal idade +Ġk än +Ġuniqu eness +Ġpad res +Ġsubs idi +Ġpige ons +β α +st ad +Ġder en +ĠС лед +d oo +ĠопиÑģ ании +Ġam ber +Ġgoose bumps +ĠfrÃ¥ gor +ĠV ital +ĠIsrael ites +w asser +Is n +Ġcomm its +ĠSTE VEN +ĠBev ölker +uit ive +Ġleg en +Ġbr uk +иÑĢов ан +yn en +hel m +Ġgener ational +ĠL ändern +οι ÏĢÏĮν +uz u +Ġcall er +он ÑĮ +üm ü +Ġbes ar +Ġpl ats +Ġmig rated +Ġj ap +ĠW AR +Ġdis sect +ĠZus ch +ĠZe iten +ĠL ions +ĠD F +â Ķ +ки в +Ġpedest rians +ĠMar ilyn +d ock +Ġy ht +Ġre incarn +ĠSon o +ĠGrow th +ÑĥÑģ ов +Ġdun geons +Ġbag us +k ich +ĠÑĥ кÑĢаÑĹ +éĨ « +ĠK eller +chem istry +J apanese +Ġwill st +Ġdecomp osition +ĠÑģÑĤ ен +Ġrev ived +íķĻ êµIJ +ĠÅ ĵ +ä½ IJ +ìĭ ¸ +ipp y +Ġhour ly +j än +ĠWork shop +Ŀ¼ ìĦľ +Ġcu arto +Ġpat rim +ĠB urch +ĠìŀĪ ê¸° +Ġhe pat +Ġh Ãłng +ĠëĮĢ íķ´ +ĠваÑĪ Ð¸ +Ġre work +Ġpar se +Ġçıkt ı +ĠS ax +ĠMong o +ĠAa ah +ram ble +D J +Ġstabil ized +ĠSpe ech +Book s +Ġhur dles +ĠW O +ĠLamb org +Ġ19 33 +Ġvor bere +Ġclin ically +Ġbreat htaking +ĠGate way +пеÑĢв ÑĭÑħ +ut ers +Ġë¹ µ +Ġyet er +Ġpull ey +Ġmuff in +ĠPre fer +ĠP ence +Ġinform ação +ìĬ¤í Ĭ¸ë +ãĤ¸ ãĥ£ +ĠTur tle +ĠReg ina +ĠLo ad +do es +pan ze +¸ Ķ +Ġmin a +ĠLatin os +amm ers +ĠT ort +ĠBey once +имо ÑģÑĤи +ĠвопÑĢоÑģ Ñĭ +Ġbul un +èĢĮ å·² +ine k +bere ich +Ġpast ure +ĠO A +ĠM elt +ĠEt t +ĠD Y +Ġob wohl +Ġle agues +ÑĤ еÑģÑĮ +Ġк ÑĥÑģ +Ġv ors +Ġto pp +ograph ical +as st +Ġl indo +Ġë°Ŀ íĺĶ +Ġré fl +Ġclim bs +Ġv arsa +Ġmethy l +ĠKar ere +Æ°á» Ł +R ad +Ġprepared ness +он Ñĩ +ĠO D +ĠC GI +Ġठ® +Ġspeech less +Ġlas ci +Ġbol ag +ĠÑħоÑĩ еÑĤÑģÑı +Ġgr ieving +ĠJohann es +ĠCar roll +ad aki +Ī ¬ë +ĠsÅĤ u +Ġinner halb +Ġgymn astics +п ÑĢи +if iques +Ġkar ate +Ġdom u +ãģĿãĤĮ ãģ§ +OTH ER +Ġdemand é +Ġbook let +ĠKy oto +Ġw oh +ĠMar ÃŃa +viol ent +J E +Ġl óg +Ġbrut ally +c ot +ĠÙħ ÛĮ +ĠWars z +å® Ī +w ol +Ġmik ä +ĠPron ounce +ĠBrend an +Ġr oup +Ġital iano +å¦Ĥ æŃ¤ +Ġкомп ÑĮÑİÑĤ +Ġur ging +ed es +Ġcarbon o +ĠRichards on +ĠÐĿ аÑĩ +ĠTra iner +ĠCrime a +Ġdi apers +Ġco vet +ĠMah ar +ĠH utch +ĠAus w +ber ty +Ġind ifferent +кÑĢ ÐµÑĤ +uld ade +Ġhar ms +¢ ÙĨ +les ia +Ġg io +ĠMist ress +ĠK nox +ĠFRE E +Ġë £¨ë +ĠнаÑĪ Ð° +Ġinvinci ble +Ġma iden +ĠJ eez +Ġbre ve +po le +Ġcritic isms +ĠRus ia +ठ® +ph in +ĠComp are +ĠB ON +Ġsne aking +ĠR ails +ĠG eral +Ġ195 3 +H ola +Ġоп ÑĭÑĤ +Ġrain forest +Ġbel um +ĠOb i +ĠIS S +ãĤĮ ãģªãģĦ +ĠС в +Ġbl ond +Ġwz gl +Ġpowiedz iaÅĤ +Ġch oking +ĠSong s +ĠBir az +Ġyell s +Ġstyl ist +ÏĮ ÏĦε +Ġsch reiben +ĠJ aw +ĠEle ven +ĠR if +/ . +Ġìĺ¤ë ŀľë§Į +Ġtreat ies +uff ed +ĠâĪ Ĵ +Ġroof s +à¹Ģภª +Ġë » +Ġspark le +ĠK iev +ĠAr gu +ere cht +ĠÐĿад о +ĠF IL +Ġmol ta +ĠDe vi +Ġcam pe +Ġbene vol +ĠT ough +Ġmo im +Ġevac uate +Ġer rado +å© Ĩ +ÑĢÑĥ го +Ġíİ ĺ +ĠÎĵ ια +Ġweak en +Ġillum inated +Ġsig lo +ĠV acc +и ей +al is +ĠÑĥ ÑģÑĤÑĢой +Ġdon a +ÅĤ os +ü man +Ġprodu cción +Ġcl ot +ĠM ango +Ġune asy +Ġsh uts +ĠExam ples +ve ll +e be +Ġprompt ly +ĠT eles +ĠпÑĢоÑĪ Ð» +Ġpu erta +Ġüber zeug +Ġco ch +so cial +ĠB enson +ĠM eth +ĠEx ped +Ġsupplement al +Ġconce ive +Ġ×ĺ ×ķ×ij +Ġcapt ivity +ıĻ ìķĪ +ĠÑħ Ñĥд +form ing +Ġupload s +Ġturbul ence +j oint +Ġsatisf actory +ĠAn ime +Ġwash es +Ġliber als +ĠSun shine +ĠRE AL +ub lik +b inary +T ony +Ġpolar ized +Ġenrich ed +t aking +ĠëģĿ ëĤĺ +Ġple asures +Ġex termin +in ese +at l +v är +аÑĢ Ñĭ +Ġmy ÅĽ +n arrator +Ġод ном +Ġnaj wiÄĻ +Ġmobil ize +Ġmill or +Ġat a +æ· · +ĠpolÃŃt ico +Ġple ad +Ġpain ters +ĠS ow +о ÑĦ +ĠìĺĽ ëĤł +ĠÑĩ ÑĤоб +Ġs abor +ĠUnd ert +ĠJER RY +Å¡ ÃŃ +Ġë° ĸìĹIJ +Ġpréc éd +Ġannot ation +ĠI naudible +Ġtext ured +Ġfisher man +v ordan +icher ung +Ġìłģ ìĿ´ +Ġge zeigt +Ġmand ates +Ġbe ak +ĠTW O +ĠAk bar +il ian +Ġtiế p +Ġsuperior ity +ink u +Ġl ys +ĠF CC +ĠC PA +ust ering +nic os +an ja +Ġch ills +ĠC age +Ġse aling +Ġsa ç +Ġded ans +ĠAl ger +Ġspe zie +Ġcol oss +ıy ı +clock wise +Ġexact amente +Ġ iemand +am ı +Ġmand ar +ra j +f aced +ag ua +Ġê¹ Ķë +Ġins besondere +Ġdri zzle +Ġdimin ish +ĠY oda +A I +Ġbil miyorum +ĠM MA +ateg ory +ĠпеÑĢ ÐµÐ¿ +Ġparticip ar +Ġnormal ized +Ġcomplex ities +æ´ ² +æİ § +аÑĢ Ð¾Ð² +m ist +ich a +Gr oup +Ġresil iency +Ġnog le +ĠCN C +pr ü +Ġphysic ists +н ок +L I +Ġstuff s +Ġsist emas +Ġinterfer ing +ĠMar vin +ér cito +ĠìĹĨ ê³ł +Ġson ic +Ġequ iv +Ġab ord +ĠRam en +Ġ0 9 +med im +at iques +Ġдел аÑİÑĤ +Ġunanim ously +Ġsk irts +ĠíĬ¹ ë³Ħ +ĠP rix +k ami +Ġfr uition +Ġbirthday s +ик ом +Ġinaug ural +Ġcorrel ate +ĠT ory +ĠëĤĺ ìģ +Ġde w +ĠPre cis +ih i +Ġë¬¸ìłľ ê°Ģ +Ġc iting +ĠL ana +ĠK ag +Ġplay through +ĠProt ocol +fr ist +hov ah +Ġmerc iful +Ġb ilingual +ĠG uitar +r h +Ġglam orous +ĠVik ings +ĠOoo oh +íķĺ ëĬĶëį° +ĠUg anda +Ġcollaps es +ent ry +Ġantioxid ants +ëĤ ĺë +ÑĪ Ð°Ñı +Ġtri via +Ġgä ller +Ġfun gi +Ġmil ks +Ġd icht +μ η +po ke +ĠвÑĭп ÑĥÑģк +Ġfeed er +ĠAl cohol +h ower +Ġdes erving +ĠRe bel +ios is +Ġ10 3 +Ġhand out +Ġen m +Ġland lords +Ġge ology +r ils +Ġco bra +ĠV old +ĠP anch +ĠGRE G +Ġpr oss +Ġbrac elets +ĠV ega +Ġroz um +æ¬ ¾ +аз д +ĠLy nd +ĠHon ors +Ġsurrend ered +Ġlibr arians +12 5 +ĠÑģ иг +Ġuniform ly +ĠE agles +ìķ Ļ +иÑĤ ан +and id +ĠìłĪë ĮĢ +ĠØ ¶ +Ġarrest s +ĠCS V +ĠAzerbai jan +ort ic +ĠD X +ĠAdvent ures +Ġab us +ĠF au +Ġschlim m +Ġratt ling +Ġconsum es +ĠTol kien +Ġresurrect ed +ĠX Y +íĬ¸ ê°Ģ +ĠвÑĭ ÑģÑĤÑĥп +ĠAng ie +żen ia +M ic +ĠShe ila +acht et +Ġover st +Ġl â +Ġine ffective +æĿ ¡ +æĢİä¹Ī äºĨ +å¿ Ļ +Ġwicht iger +Ġv ino +Ġp um +Ġang led +ĠP ione +ĠM ỹ +ãģĿãĤĮ ãģ¯ +wo ÅĽÄĩ +d raw +ั à¹Ī +mark ets +Ġcaf es +ĠC em +â Ŀ¤ +ĠS uit +M K +Ġemphas izes +Ġtort illa +Ġmejor ar +ĠSur viv +cast ing +Ġeduc ación +ĠG um +u ely +ĠìĹ¬ê¸° ëĬĶ +Ġstretch y +en ça +Ġwith hold +Ġex iting +Ġenthal py +ĠTrans it +ıl mÄ±ÅŁ +al ies +Ġsal var +Ġlean ed +ĠgroÃŁ es +Ġf itt +ак и +S arah +Ġhost el +Ġfinger na +Ġnadzie jÄĻ +w ives +R ec +Ġsp ool +аÑĤ ов +ĠEn emy +Ġf ury +Ġdet ta +ĠF ay +éļ ¨ +Ñı ÑİÑĤ +Ġaproxim adamente +Ġsil os +Ġmag ist +Ġc ree +ĠKr ank +ĠD OWN +Ġstart led +Ġre born +ĠUm welt +ĠSuz anne +ни ÑĨÑĭ +out ez +ĠJ AC +y ards +rad as +ra u +ip ts +h ail +Ġparagraph s +Ġme glio +Ġisol ating +Ġace ite +ĠH arsh +Ġcy st +ĠBlock chain +ĠÑħоÑĢоÑĪ Ð¸Ð¹ +Ġvirt uous +Ġinvestig ación +Ġdev oir +Ġmast urb +ĠS ale +ÙĬر Ø© +ĠÎ § +ĠStra ÃŁen +Ġdi kk +Ġa fore +ĠJung kook +Ġcho ciaż +ĠDebat te +Ġweird ly +Ġvia je +reg ist +H elp +Ġkind eren +Ġform ulated +Ġenf im +ĠTow ards +ко ÑĹ +iver ing +ĠдеÑĤ и +char ger +Ġpur l +Ġacadem ically +ĠNur se +Ġdel eting +ay o +Ġref usal +Ġdepict s +ĠDr acula +Ġtoast ed +ĠZomb ie +ĠSuper ior +ĠB old +Ġquizz es +Ġg le +4 50 +Ġcome ço +yn n +Ġver st +ĠO laf +Ġpom oc +ĠS ask +ë ĺ +ĠT CP +ĠProper ty +íķĺ ì£ł +à¸ľ ม +bo om +ar os +ĠÑĢоÑģÑģ ий +ĠбÑĭв аеÑĤ +åĩº åİ» +ĠìĿ´ìķ¼ ê¸°ë¥¼ +Ġcomb ien +v acc +Ġeben falls +par a +Ġз м +Ġdesper ation +ord re +Ġש׾ ×Ļ +Ġgener ously +ĠÐŀ к +Ġorb iting +> </ +Ġesp ÃŃ +ĠCO P +åŃ© åŃIJ +vis ible +ĠпÑĢеÑģÑĤ Ñĥп +Ġstitch ed +à¯Ī . +Ġlat ent +ĠP rab +ĠMc N +ĠHe aling +ĠCur iosity +c ert +Ġ민 주 +Ġpatient ly +ĠY T +fore ign +Ġv ẫn +Ġindust ri +Ġcock tails +Ġbright en +Ġconsolid ated +аÑĢ Ð´ +lt ry +Ġgr ille +Ġb ona +Ġdilig ently +ĠWrestle Mania +er kt +ener gy +99 9 +à®ķ வ +Ġto te +ion o +DI O +Ġschizophren ia +Ġpostp oned +ĠQ iu +ĠÏĥÏħ ν +Ġzd jÄĻ +Ġspann end +ĠD IS +R el +Ġr hin +imm une +O ld +Ġpl ötzlich +Ġm ound +Ġastronom ical +ĠGu id +ĠC ul +H I +ĠÅ ł +Ġrep o +ĠMaur ice +ä¸Ģ çĤ¹ +Ġband its +ĠDes ktop +ä ss +ft a +Ġlic ence +Ġimag inar +ĠEntre prene +x o +Ġë§ĽìŀĪ ëĬĶ +Ġ×Ķ× ij +Ġpump kins +Ġkans sa +ĠjÄĻ zy +Ġcommunaut é +b ür +Ġer hö +ĠWol ver +ĠSh aring +ä» ¤ +Ġpak ai +Ġinsult ed +Ðľ Ñĭ +о ÑĹ +Ġconsist e +æĮ ij +Ġyoung sters +Ġgleich en +w eder +Ġm ote +Ġcla uses +ét at +pr us +Ġwas t +ç»Ļ æĪij +ĠCr isp +Ġ çĦ¶å¾Į +Ġoff enders +Ġconve ction +Ġconf ian +o llow +am et +ĠÑĹ Ñħ +第äºĮ åĢĭ +ffic iency +Ġung laub +ig ans +Ġmarket ed +ĠV AN +Ġproc laimed +Ġcél ulas +Ġcoll ide +ĠO culus +ad ore +J i +Ġsust aining +ĠF asc +Ġset zt +Ġnos altres +M ost +Ġв Ñĩ +Ġna uc +ĠB har +çΏ çΏ +æĪijè·Łä½ł è¬Ľ +Ġy êu +Ġtim est +Ġpert ama +ir mi +Ġz wr +Ġverb ess +Ġv ortex +ĠST ACK +Ø« ر +¹ Ħë +ĶĶ ìĺ¤ +Ġlink age +ĠFr aser +en ario +Ġë Ŀ¼ëĬĶ +ĠìĦłë °° +ht hal +Ġê¹ Į +ĠKh ông +à ĥ +Ġscr ambled +ĠE ink +Ġmicro organ +Ġnarciss ist +ĠKomb at +Ġë§ ¡ +ĠA GA +Ġperf ekt +ĠSer ie +det erm +- ' +Ġpony tail +Ġkos ka +ì ĵ +Ġo bec +Ġch ests +ve er +Ġup rising +Ġst oked +as soci +Ġprodu ção +ĠSha pe +ìłľ ê°Ģ +ĠëĶ ° +Ġj on +Ġinad vert +ant as +Ġнак онеÑĨ +Ġå°į åķĬ +ĠArsen al +Ġprot eg +Ġlibert é +Ġgl are +åĪ ļ +å·² ç»ı +Ġvere in +Ġinsert s +ĠJ ana +Ġwyd aje +ÅĤ um +Ġ %. +orig ine +Ġsyn agogue +Ġfall ait +Ġdis obed +Ġant ic +ĠCy cl +Ġasynchron ous +Ġë²Į ì፠+Ġges und +Ġg agn +Ġpe a +Ġgr in +é st +Ġsa uc +ĠM äd +íķ´ë ıĦ +pp s +ĠεÏĢ Î¹ +Ġpeu ple +Ġde ben +ĠB ree +ĠÑĢ Ð¾Ð»ÑĮ +Ġкак им +Ġú til +Ġdistrib utor +ал Ñĭ +Ġswo jÄħ +Ġfol klore +Ġrece ivers +ĠM OO +b ins +ast re +ìķ Īë +ĠëĦ£ ê³ł +Ġmultim edia +Ġgeb aut +ов ÑĭÑħ +ã y +Ġd ane +ok ol +emit ism +ONE Y +Ġya ÄŁ +Ġcha uff +容 æĺĵ +Ġesf uer +Äĥ n +ert as +Ġfonction ne +om ina +Ġiv ory +ĠYout uber +ĠSky walker +иÑĩеÑģ каÑı +to i +Ġve ya +Ġgel ernt +Ġchance llor +ĠStat istics +Ġweld ed +Ġon dan +ĠSe i +Ġmed ically +Ġenerg ized +ĠV ia +Ġв ик +Ġun inter +Ġhigh ness +ĠíĮ Ķë +Ġampl ified +ĠSerge y +ĠM ins +w arm +pe ll +oph ile +Ġh è +ĠBel o +ĠSket ch +Ġcharacter ization +ans en +ĠÑĤ ÑĥÑĢ +Ġ ãħĭãħĭãħĭ +N ote +Ġko ÅŁ +Ġc iert +f lu +Ġba ht +ĠDownt own +ĠC RIS +od ie +1 40 +Ġlit res +Ġgr iev +æ§ ĺ +Ġì͍ ê°Ģ +Ġsucceed s +Ġ __ +ent ing +Ġv imos +Ġs ì +def ense +ĠMc D +ĠMar ion +ĠD ont +ĠD DR +ĠL azar +ĠD AR +Ġk uv +K n +Ġsemb la +Ġair borne +ĠViol ence +ëIJ IJ +Ġrestra int +Ġwh istles +Ġscold ed +Ġacces o +Ġabsolut amente +ĠT yl +ĠS ap +¶Ģë ¶Ħ +itä ten +ad em +Ġà ½ +Ġpres cribe +ĠM age +ĠHel ena +å¾Ī æľī +äº ² +v t +Ġv ienen +Ġsne ez +Ġmol é +Æ°á»Ł ng +Ġtransport ing +ĠLe an +Ġk ung +Ñĥ ÑĢа +ÏĦ ÎŃ +ut ches +ond ers +li yor +N at +Ġz ij +Ġmamm al +Ġkä yt +ĠJo anna +s ent +Ġठ¸ +Ġvest ed +ĠErfahr ung +oke e +Ġcl ipping +ĠList ening +Ġ( # +f ö +Ġvid are +Ġbr ittle +ĠST ART +ĠDam as +ĠY og +ãĤĵ ãģ¨ +g art +Ġver lier +Ġheart felt +Ġdo ÅĽÄĩ +ì¹ĺ ê°Ģ +. » +Ġmaxim al +Ġdistint os +ĠìĻľë ĥIJíķĺë©´ +Ġsa iled +Ġconvey ed +ĠT inder +ĠSU PER +ни ÑĨÑĥ +cont rolled +Ġfun z +Ġbast ards +ĠGins burg +Ġnuo vo +ĠP ere +ĠJ ES +ĠD ingen +ĠB ets +umb a +ac ción +ĠìŀĪ ì§Ģë§Į +Ġret ra +ĠLaure nt +Ġpo zy +Ġgroo ves +Ġmáqu ina +Ġmin ion +Ġde inen +ĠSha un +×Ļ ×Ļ +Ġhonor ary +osaur us +Ġze it +Ġespe cie +ĠB CE +аÑĤ е +Just in +ĠWhe els +ĠìĿ´ íķ´ +Ġب ÙĬÙĨ +Ġprop ulsion +Ġperce ber +ĠNew man +å ´ +cul osis +M i +Ġак кÑĥ +Ġmaster ing +Ġl äh +Ġf ists +ä» Ķ +Ġmarin ade +L illy +Ġëħ¸ë ł¥ +ĠY H +Ġurg ently +Ġinform ational +Ġac ordo +iz zy +ãģĦ ãģı +ìĿ´ì ĸ´ +im ar +ĠëĤĺìĺ ¤ë +Ġtwent ies +Ġr asp +Ġbump y +ب Ø© +work er +Ġquick est +Ġattach es +в иг +ĠëĤĺ íĥĢë +Ġpure e +Ġovers ized +Ġstir red +Ġjak im +Ġhom icide +ãĤĤ ãģĹ +isc illa +Ġì± Ļ +Ġspec ulative +Ġass ists +m ain +j ähr +ind et +ĠÅŁ ur +Ġforecast s +Ġdivers ion +Ġt are +Ġog l +ĠOrgan isation +ĠChe vy +Ġb aja +and ır +ĠÙĪ ÙĦا +Ġrad iant +Ġli aison +Ġdem okrat +ĠMAR C +ÏĢ Î¿Ïħ +Ġrun t +Ġpréc is +Ġge ven +Ġvé hic +ĠJ ESS +ST R +Ġìĸ ĺë +Ġvision ary +Ġbur adan +ĠãģĤ ãĤĬ +Ġre birth +Ġexhib ited +ĠMet all +ol ie +ely n +Ġflav ours +Ġesc rito +ĠDel ete +ĠìķĮ ìķĺìĸ´ +ĠÑĥкÑĢаÑĹ Ð½ +Ġinterrupt ing +Ġident ific +ĠSuz uki +ĠLand ing +ä»¶ äºĭæĥħ +and i +Ġest ran +Ġcoule ur +Ġag rad +ĠS ny +Ġà®ĩ ல +Ġand er +Ġr ua +Ġpr ise +Ġla ure +ĠíĬ Ģ +Ġmod eration +Ġerf ahren +Ġdec onst +ĠRe ese +ĠP K +et os +ãģĵãĤĮ ãģ§ +ĠGra vity +ĠE ren +Ġover board +Ġmü sst +ĠEm ail +еÑĢ Ð¼ +y di +iÄĻ dzy +ĠL OU +ĠFuÃŁ ball +ĠR D +al ts +ĠìĬ¤í Ĭ¸ë +ĠÐļ ÑĢаÑģ +Ġtele v +ĠÑĢ Ð¾ +Ġresign ation +Ġj ingle +ĠStud ien +ĠI X +ĠSent inel +ĠP ang +é Ħ +J ake +Ġperson agem +Ġméd ia +ĠC hern +ant ically +Ġth á»Ŀi +Ġparal ysis +Ġj apanese +Ġcon ex +Ġe fic +Ġunders ide +Ġne ol +Ġf ian +имо ÑģÑĤÑĮ +Ġquir ky +Ġp ista +ĠC lement +not hing +Ġпо еÑħ +Ġhor rend +Ġconsolid ate +plo ys +em aker +Jenn ifer +Ġnum éro +Ġfam oso +ĠNept une +ĠíĸĪ ìĸ´ +ĠпÑĢез ид +Ġsit com +Ġser io +Ġm ue +Ġgl ands +Ġbör jar +ĠY J +ĠR iot +par agus +Ġseg urança +Ġimm ature +ĠMad onna +ภį +Ġling ering +Ġac esso +ĠOri ent +ĠRe comm +Ġcompl ac +found ed +att end +Ġciel o +ĠZ han +na ires +c co +Ġ×IJ× ł +Ġstat a +Ġcontradict ory +ĠS é +ĠS AN +ĠCon nie +Ġëĭ¹ ìĭľ +ĠÑģам ой +Ġmaj estic +ĠPeng uin +ĠCO ME +ÃŃ cios +per o +Ġm g +Ġfa uc +Ġcor rer +ĠGott es +ĠAng lo +H ar +á»Ĺ i +Ġv itesse +Ġannoun cer +ĠOm aha +k um +Ġsp ared +ĠÑĢаз а +ĠполÑĥÑĩ иÑĤÑģÑı +Ġt ähän +Ġпон ад +Ġpert aining +ĠR ate +ier n +G old +Ġtest e +ĠdeÄŁ ild +Ġdamp ing +ĠPartners hip +zy sta +g eld +Ġsm okes +ĠMar riage +쪽 ìĹIJ +èħ ³ +is ce +Ġtry na +ĠDirect ory +ĠëĤĺìĺ ¬ +Ġshame ful +Ġment re +Ġass igning +æĺ¯ éĢĻæ¨£ +Ġreper toire +Ġobjet os +ç¨ ± +Ġunder world +Ġende avors +Ġign ite +ĠÙĪ Ø¬ +Ġexper ient +ĠÐĹ Ð°Ð¿ +Ġзак лÑİÑĩ +Ġvolt ages +Ġnie go +Ġdefic its +Ġbu enos +ĠSleep ing +ĠSal em +Ġunlock ing +Ġinteract ed +Ġent endeu +ĠSuper intendent +Ġszcz egól +Ġqu as +Ġpal ing +Ġk ho +ب ØŃ +Ġcol abor +ĠпÑĢи гоÑĤов +Ġma uv +ĠJud as +ĠAss ist +ĠÑĤеÑĢ ÑĢи +Ġна ÑģколÑĮко +Ġsubsid y +ĠEmb assy +Ġd agen +ĠS anto +èĪ ¬ +ש ×ķ×ij +Ġabrupt ly +ĠAd apt +Ġva ak +Ġpost al +Ġinvest ir +Ġfique i +Ġdownt ime +ĠWe bb +ĠNC AA +ĠEst oy +ол оÑĤ +ĠìĤ¬ ê±´ +Ġnational ist +ĠKath ryn +ĠK op +é ª +Se an +ON A +ĠB j +×¢ ×Ŀ +ÃŃ b +id amente +Ġглаз а +Ġun nie +Ġgema akt +ĠINTERVIE WER +ĠH aut +ί ο +ge ois +w ydd +Ġкол и +Ġtight ened +Ġpl anners +Ġher um +Ġgör ün +Ġelectron ically +Ġcer am +Ġëĭ¤ìĸij íķľ +Ġepile psy +Ġe ÄŁ +l ins +ĠSh iny +æł ¡ +ĠÑģ олн +Ġmac aron +Ġimpact o +ĠVeg an +ze ÅĦ +ĠRap ha +ĠP ars +ĠL EO +ãģĬ ãģ£ +c ü +Ġ׾×Ķ ×Ļ×ķת +Ġä hnlich +Ġfl oss +ĠA Z +Ġmö chten +Ġgroom ing +Ġgrass es +ran ch +Ġreci bir +Ġboun cy +ĠHob by +Ġvikt ig +Ġbeg itu +ĠPicas so +ĠK ush +ë ª¨ +Ġobst ruction +Ġë¶Ħ ìľĦ +Ġmicro b +ĠWest minster +rop s +d ul +Ġdev o +ĠLehr er +ĠAd visor +uck en +Ġб Ñĥм +Ġfl attering +ĠTr uman +ĠSem pre +ĠMcC ain +ĠHind us +Jul ia +Ġwaters hed +Ġl ush +ìł Ħë +Be fore +ĠÐĴ ÑĤоÑĢ +ĠS aaS +Ġsit zt +Ġbeet le +ĠEss ential +en ko +ĠëķĮë ıĦ +Ġrev ving +Ġpoor er +Ġco erc +Ġide e +Ġco û +al et +Ġzd row +Ġf ender +grow th +D ING +Ġz de +ä¸Ĭ éĿ¢ +EN TS +Ġfac ets +éļ ª +ush ima +ĠÅŁ eh +Ġparas ite +Ġl apse +ĠMe er +ĠK und +Ġsl og +Ġbr unch +ĠCh art +ar z +ĠM US +Ġoff enses +Ġingl és +Ġfol iage +op lan +A ut +ĠJac qu +t ak +ie mbre +Ġx en +Ġnom inees +Ġbi omedical +és us +Ġest uv +ÏĦ ÏĮ +ATH AN +Ġíķľë į° +Ġhe ed +cros stalk +B ill +Ġsp ouses +ĠÑģÑİ Ð¶ +Ġver so +ĠS ven +ĠC au +c uz +Ġë³´ì Ħ¸ìļĶ +ĠÑħ озÑı +Ġmock ing +ĠO na +ĠD á +Ġfruit ful +Ġban quet +ud ding +in ctions +d ert +s ud +Ġdes con +ĠJ C +Ġ § +Ġpub li +ëĪ Ī +éģķ ãģĨ +Ġents chieden +ĠRO I +ãģį ãģŁ +ĠìĥĿ ê²¼ +Ġkä ytt +y ani +sh aw +Ġunle ash +Ġman ne +Ġhist ogram +æĬ ¥ +à¸Ńะ à¹Ħร +Ġg n +Ġfe lla +Ġeing es +ĠBu ilt +Ġrepresent a +Ġpun ishing +Ġouts iders +нÑĥ ÑĤÑĮÑģÑı +cur rent +Ġfamiliar ity +Ġд ив +Ġpro jets +Ġaqu eles +ĠGl ue +th ose +Ġin ception +Ġaquell os +Ġill usions +Ġatt ends +re se +Ġsw arm +Ġsw ab +Ġregard ez +Ġpos ição +Ġak hir +Ġextract ing +Ġanecd ote +ĠT ale +Ġв ин +Ġab ges +Ġol uÅŁ +Ġcomplic ado +Ġco vari +Ñĸ ÑĤÑĮ +D er +Ġ×Ļ ×Ķ +F orm +Ġìĸ´ì¨ Įëĵł +Ġread able +Ġinhib it +Ġde cipher +ĠAng ry +p g +வ த +ĠÑģоб ÑģÑĤвенно +Ġsam h +Ġesc r +Ġencompass es +Ġa uster +Ġconf isc +ĠMand al +Ġ } +atch er += # +çļĦ æĹ¶åĢĻ +Ġк ино +Ġst al +l ungs +Ġvo le +Ġrequ is +Ġ ãĤĪ +Ġp én +Ġlect urer +Ġins cription +Ġcerv ical +ĠTre asure +ĠJ W +com ings +Ġeyes ight +ĠT ails +ÃŃs imo +Ġworks heet +Ġswift ly +Ġcon os +Ġelimin ates +ĠBla ze +ал ог +Ġpicture d +Ġgir affe +ĠLog ic +åĺ ī +Ġenrich ment +F it +Ġunint ended +Ġpersec uted +ak ap +ë° ĺ +Ġbar ber +Ġar beitet +ĠSur prisingly +ĠAut ob +unk u +pro v +ĠLo ch +ob yl +Ġпод гоÑĤов +Ġéconom ique +Ġp att +Ġce ased +ĠÑģп иÑģ +Ġnucle i +Ġis te +ĠW ag +Ġzu peÅĤnie +Ġpro verb +ĠAh ÃŃ +åĽŀ åİ» +li amo +Ġreli ably +Ġp ik +ĠTr ading +ĠCole man +Ġα να +Ġmag ari +ĠPH IL +Ġshed ding +oh ner +Ġporn ography +Ġbenefici aries +âĢ ¢ +en in +Ġresol ving +ĠÑģп оÑĢÑĤ +Ġб ег +Ġne ctar +ult ura +ims ical +ĮĢë ¥¼ +å¹´ åīį +ãģĹ ãĤĥ +Ġvis ão +éģİ ä¾Ĩ +ÿÿÿÿ ÿÿÿÿ +att form +Ġë§ŀ ëĬĶ +Ġpilgr image +Ġm ating +ĠRe aper +ĠBre f +çĶŁ æ´» +Ġ×ij× ĵ +Ġnov amente +Ġgr illing +ĠWire less +ĠRoman ian +Ò Ľ +ìľ łë +h ait +ĠB ora +ARR Y +Ġhypothes es +é© ¬ +ik ut +ĠìķĦë ²Ħ +ĠÑĸ з +Ġnation ale +ت Ùī +üll t +Ġélé ments +ĠW are +Ġ( - +алÑĮ ном +Ġind ict +ĠSt ones +ãģŁ ãĤģ +expl osion +ĠëĥĦ ìĥĪ +Ġfel ic +Ġjud iciary +Ġincarn ation +Ġin ning +Ġform ul +Ġship ment +Ġre indeer +æĴ Ń +Ġоз наÑĩ +Ġenv ol +und y +Ġзна ÑĤÑĮ +Ġвид ели +Ġexclud ing +de ath +Ġb erm +Ġsopr attutto +Ġdeb ido +ĠZ ig +ĠO v +ĠKE VIN +ĠP ale +ĠM ire +Ġand ar +inc luding +Ġsw apped +Ġmiscon ceptions +Ġsp ong +ré al +Ġorbit als +Ġhasht ags +or it +Ġmauv ais +иÑģ а +Ġliv res +ĠI PS +Ġ0 4 +ö g +in str +Ġвн еÑĪ +Ġh ice +is ée +Ġow es +Ġes imerk +ĠU H +Ġirrit ation +Ġg iggles +Ġcolonial ism +ĠBl iss +str ings +Ġreun ited +ĠPs aki +w ach +Ġcl iffs +ĠFal se +ä g +p ipe +Ġwh opping +Ġmering ue +Ġb ung +indust rie +Ġle che +ĠL oy +Ġdr ie +Ġpass at +Ġo leh +Ġcé u +ĠGab rie +Ġreef s +Ġbom bers +Ġepisód io +ĠR ug +ĠPr ose +on os +Ġob ese +Ġgo og +Ġpi ace +flan zen +éĴ Ł +Ġfl aps +ĠAl to +é£Ł ãģ¹ +F in +Ġres ize +ê·¸ë ŀ¨ +è² » +N athan +ŀĪë ł¤ +ĠÑĤ ай +ĠNF T +Ġsne eze +Ġshr oud +i é +Ġver amente +Ġcas cade +ĠO ok +ìĹĨ ìĿ´ +Ġinf used +f ps +cent er +Ġgrapp ling +ĠWohn ung +ĠT umb +ĠIm ma +ĠDu ygusal +ен ÑĤи +Ġstewards hip +Ġhar p +Ġendors ed +ıl an +Ġод ним +Ġcompet ency +Ġb ert +ĠT ales +Ġr he +Ġoh h +Ġê°Ħ ëĭ¨ +Ġm RNA +Ġgang ster +ĠRun ner +ен нÑĭм +ph oria +ĠwÅĤaÅĽci wie +Ġqu arto +Ġorgan ise +ĠV et +P ad +ĠÙħ Ø« +Ġst inks +ĠD ul +u em +is iej +T op +Ġt ussen +ĠE fendimiz +ĠB oule +ĠSlo ven +ĠL ö +Ñij з +ÑĢи п +ca ve +Ġbo î +Ġapolog ise +ĠMar ly +ĠEx port +ĠCait lin +Ġtav alla +Ġent ails +Ġbr om +ĠCop enh +Ġwal nut +Ġins ists +Ġcu á»Ļc +ĠQ uit +ĠDev ice +×Ĵ ×Ŀ +ĠD OT +Ġveloc idad +L IE +C ool +Ġsan itation +Ġol ho +ĠE B +ĠíĻķ ìĭ¤íŀĪ +ĠÐľ иÑħ +Ġz uk +Ġsurn ame +ĠSch uld +ru ff +c ultural +ĠÑģÑĤ олÑĮко +æĻļ ä¸Ĭ +Įë į° +Ġtor to +Ġback ups +ÑĢи й +re lax +Ġsyner gy +Ġbuff s +Ġap o +ĠWell ness +round ed +Ġunivers es +Ġf era +Ġstand by +ĠSil va +ĠJ I +ens ored +ĠìĹĨ ëĭ¤ +ĠÐIJ в +ĠоÑĤ дел +Ġf ø +ĠRoc kef +ĠComp ass +ĠBe ars +Ġ ä¸įè¦ģ +T urn +Ġth á»±c +Ġposs ibile +Ġest em +ĠCroat ia +Ġtät ä +ĠC AL +à¹Ģภŀ +ĠÑģÑĤ ÑĢаÑħ +Ġsal ts +Ġminimal ist +Ġincorpor ates +ĠÙĨÛģ ÛĮÚº +aca o +Ġsl ammed +Ġcam a +T ext +!!!! !! +Ġalc anz +é ma +Ġinc ense +Ġhard en +Ġgrant ing +ĠN ai +ĠFir ma +Ġhyp oc +j ob +ĠR H +z ur +ил Ñı +ĠÅ º +Ġda res +an h +Ġë§Į íģ¼ +Ġcuest ión +ĠL ima +æĻ ¯ +Ġass unto +ĠIP O +ĠBeng al +ĠB ier +Ġpsy che +Ġacqu ainted +ĠG ün +оз и +ÅĽci Äħ +A G +Ġmalf unction +Ġastero ids +ire z +am orph +ĠÑģоÑĤ ÑĢÑĥд +Ġfresh water +Ġar ran +ĠпÑĢ Ñĭ +н ог +Ġdiab etic +ĠÙĤ اÙĦ +Ġopp ress +Ġcapacit ance +perform ance +cr ates +Ġapost le +ĠJ EN +OU LD +Int ro +Ġstall s +ĠAB OUT +ctic amente +Ġdil igent +Ġmanif ests +ĠPak istani +Ġ( ' +åľ º diff --git a/whisper/assets/multilingual/special_tokens_map.json b/whisper/assets/multilingual/special_tokens_map.json new file mode 100644 index 0000000000000000000000000000000000000000..817762d631ad6f9c799f6b9dc713c46420e65546 --- /dev/null +++ b/whisper/assets/multilingual/special_tokens_map.json @@ -0,0 +1 @@ +{"bos_token": "<|endoftext|>", "eos_token": "<|endoftext|>", "unk_token": "<|endoftext|>"} \ No newline at end of file diff --git a/whisper/assets/multilingual/tokenizer_config.json b/whisper/assets/multilingual/tokenizer_config.json new file mode 100644 index 0000000000000000000000000000000000000000..0235ccff90efbc022fbe30360220375c5bad68da --- /dev/null +++ b/whisper/assets/multilingual/tokenizer_config.json @@ -0,0 +1 @@ +{"unk_token": {"content": "<|endoftext|>", "single_word": false, "lstrip": false, "rstrip": false, "normalized": true, "__type": "AddedToken"}, "bos_token": {"content": "<|endoftext|>", "single_word": false, "lstrip": false, "rstrip": false, "normalized": true, "__type": "AddedToken"}, "eos_token": {"content": "<|endoftext|>", "single_word": false, "lstrip": false, "rstrip": false, "normalized": true, "__type": "AddedToken"}, "add_prefix_space": false, "model_max_length": 1024, "special_tokens_map_file": null, "name_or_path": "multilingual", "errors": "replace", "tokenizer_class": "GPT2Tokenizer"} \ No newline at end of file diff --git a/whisper/assets/multilingual/vocab.json b/whisper/assets/multilingual/vocab.json new file mode 100644 index 0000000000000000000000000000000000000000..406c3f2600089389bd8bf3920c2fa3770d11789a --- /dev/null +++ b/whisper/assets/multilingual/vocab.json @@ -0,0 +1 @@ +{"!": 0, "\"": 1, "#": 2, "$": 3, "%": 4, "&": 5, "'": 6, "(": 7, ")": 8, "*": 9, "+": 10, ",": 11, "-": 12, ".": 13, "/": 14, "0": 15, "1": 16, "2": 17, "3": 18, "4": 19, "5": 20, "6": 21, "7": 22, "8": 23, "9": 24, ":": 25, ";": 26, "<": 27, "=": 28, ">": 29, "?": 30, "@": 31, "A": 32, "B": 33, "C": 34, "D": 35, "E": 36, "F": 37, "G": 38, "H": 39, "I": 40, "J": 41, "K": 42, "L": 43, "M": 44, "N": 45, "O": 46, "P": 47, "Q": 48, "R": 49, "S": 50, "T": 51, "U": 52, "V": 53, "W": 54, "X": 55, "Y": 56, "Z": 57, "[": 58, "\\": 59, "]": 60, "^": 61, "_": 62, "`": 63, "a": 64, "b": 65, "c": 66, "d": 67, "e": 68, "f": 69, "g": 70, "h": 71, "i": 72, "j": 73, "k": 74, "l": 75, "m": 76, "n": 77, "o": 78, "p": 79, "q": 80, "r": 81, "s": 82, "t": 83, "u": 84, "v": 85, "w": 86, "x": 87, "y": 88, "z": 89, "{": 90, "|": 91, "}": 92, "~": 93, "¡": 94, "¢": 95, "£": 96, "¤": 97, "¥": 98, "¦": 99, "§": 100, "¨": 101, "©": 102, "ª": 103, "«": 104, "¬": 105, "®": 106, "¯": 107, "°": 108, "±": 109, "²": 110, "³": 111, "´": 112, "µ": 113, "¶": 114, "·": 115, "¸": 116, "¹": 117, "º": 118, "»": 119, "¼": 120, "½": 121, "¾": 122, "¿": 123, "À": 124, "Á": 125, "Â": 126, "Ã": 127, "Ä": 128, "Å": 129, "Æ": 130, "Ç": 131, "È": 132, "É": 133, "Ê": 134, "Ë": 135, "Ì": 136, "Í": 137, "Î": 138, "Ï": 139, "Ð": 140, "Ñ": 141, "Ò": 142, "Ó": 143, "Ô": 144, "Õ": 145, "Ö": 146, "×": 147, "Ø": 148, "Ù": 149, "Ú": 150, "Û": 151, "Ü": 152, "Ý": 153, "Þ": 154, "ß": 155, "à": 156, "á": 157, "â": 158, "ã": 159, "ä": 160, "å": 161, "æ": 162, "ç": 163, "è": 164, "é": 165, "ê": 166, "ë": 167, "ì": 168, "í": 169, "î": 170, "ï": 171, "ð": 172, "ñ": 173, "ò": 174, "ó": 175, "ô": 176, "õ": 177, "ö": 178, "÷": 179, "ø": 180, "ù": 181, "ú": 182, "û": 183, "ü": 184, "ý": 185, "þ": 186, "ÿ": 187, "Ā": 188, "ā": 189, "Ă": 190, "ă": 191, "Ą": 192, "ą": 193, "Ć": 194, "ć": 195, "Ĉ": 196, "ĉ": 197, "Ċ": 198, "ċ": 199, "Č": 200, "č": 201, "Ď": 202, "ď": 203, "Đ": 204, "đ": 205, "Ē": 206, "ē": 207, "Ĕ": 208, "ĕ": 209, "Ė": 210, "ė": 211, "Ę": 212, "ę": 213, "Ě": 214, "ě": 215, "Ĝ": 216, "ĝ": 217, "Ğ": 218, "ğ": 219, "Ġ": 220, "ġ": 221, "Ģ": 222, "ģ": 223, "Ĥ": 224, "ĥ": 225, "Ħ": 226, "ħ": 227, "Ĩ": 228, "ĩ": 229, "Ī": 230, "ī": 231, "Ĭ": 232, "ĭ": 233, "Į": 234, "į": 235, "İ": 236, "ı": 237, "IJ": 238, "ij": 239, "Ĵ": 240, "ĵ": 241, "Ķ": 242, "ķ": 243, "ĸ": 244, "Ĺ": 245, "ĺ": 246, "Ļ": 247, "ļ": 248, "Ľ": 249, "ľ": 250, "Ŀ": 251, "ŀ": 252, "Ł": 253, "ł": 254, "Ń": 255, "Ġt": 256, "Ġa": 257, "Ġth": 258, "in": 259, "er": 260, "Ġw": 261, "Ġs": 262, "ou": 263, "Ġthe": 264, "re": 265, "on": 266, "at": 267, "en": 268, "Ġc": 269, "it": 270, "is": 271, "Ġb": 272, "nd": 273, "Ġd": 274, "Ġm": 275, "Ġh": 276, "Ġo": 277, "ing": 278, "es": 279, "Ġp": 280, "Ġto": 281, "an": 282, "Ġf": 283, "or": 284, "ll": 285, "ĠI": 286, "Ġl": 287, "Ġy": 288, "ar": 289, "Ġg": 290, "Ġyou": 291, "ed": 292, "Ġand": 293, "Ġin": 294, "Ġof": 295, "as": 296, "Ġn": 297, "om": 298, "ic": 299, "Ġthat": 300, "us": 301, "et": 302, "ve": 303, "al": 304, "ow": 305, "le": 306, "Ġis": 307, "Ġe": 308, "Ġit": 309, "ot": 310, "'s": 311, "Ġbe": 312, "ion": 313, "ĠT": 314, "Ġwh": 315, "ĠA": 316, "ent": 317, "ĠS": 318, "Ġre": 319, "ay": 320, "Ġwe": 321, "Ġon": 322, "ere": 323, "Ġha": 324, "ut": 325, "ac": 326, "id": 327, "ig": 328, "os": 329, "ke": 330, "ver": 331, "im": 332, "ĠÐ": 333, "ĠTh": 334, "am": 335, "all": 336, "Ġfor": 337, "el": 338, "ch": 339, "ro": 340, "Ġthis": 341, "Ġst": 342, "ĠW": 343, "Ġu": 344, "ad": 345, "out": 346, "ir": 347, "ld": 348, "ct": 349, "Ġk": 350, "if": 351, "Ġgo": 352, "..": 353, "о": 354, "ith": 355, "ly": 356, "ht": 357, "qu": 358, "Ġ-": 359, "Ġdo": 360, "Ġj": 361, "Ġhave": 362, "ĠB": 363, "Ġan": 364, "Ġwith": 365, "Ġare": 366, "Ġr": 367, "Ġde": 368, "Ġse": 369, "Ġso": 370, "Ġv": 371, "st": 372, "ill": 373, "ur": 374, "Ġli": 375, "ĠM": 376, "est": 377, "od": 378, "ally": 379, "'t": 380, "ust": 381, "Ġas": 382, "ĠC": 383, "ce": 384, "Ġme": 385, "а": 386, "е": 387, "il": 388, "ĠH": 389, "Ġwas": 390, "ter": 391, "th": 392, "Ġcan": 393, "ant": 394, "Ġcom": 395, "our": 396, "ight": 397, "ĠY": 398, "ation": 399, "ĠAnd": 400, "ol": 401, "Ġsh": 402, "ÑĤ": 403, "op": 404, "se": 405, "Ġnot": 406, "ĠSo": 407, "Ġne": 408, "un": 409, "Ġab": 410, "Ġlike": 411, "Ġat": 412, "ĠD": 413, "ie": 414, "Ġhe": 415, "Ġcon": 416, "Ġch": 417, "ore": 418, "Ġal": 419, "Ġor": 420, "Ġqu": 421, "ĠO": 422, "ome": 423, "ra": 424, "ul": 425, "ĠN": 426, "pp": 427, "Ġyour": 428, "ould": 429, "ĠP": 430, "Ġfr": 431, "ge": 432, "ers": 433, "'re": 434, "и": 435, "Ġthey": 436, "Ġwhat": 437, "use": 438, "Ġall": 439, "ĠThe": 440, "ĠL": 441, "ess": 442, "em": 443, "Ġkn": 444, "Ġjust": 445, "art": 446, "Ġpro": 447, "very": 448, "um": 449, "Ġlo": 450, "Ġì": 451, "Ġmy": 452, "ok": 453, "Ġex": 454, "ab": 455, "Ġthere": 456, "Ġbut": 457, "Ġknow": 458, "Ġsu": 459, "ĠG": 460, "Ñģ": 461, "ĠE": 462, "Ġma": 463, "оÐ": 464, "Ġen": 465, "Ġabout": 466, "ĠIt": 467, "ist": 468, "Ġwor": 469, "ri": 470, "ind": 471, "Ġone": 472, "ate": 473, "and": 474, "ink": 475, "Ġle": 476, "ort": 477, "'m": 478, "ĠF": 479, "ich": 480, "ÑĢ": 481, "ide": 482, "Ġget": 483, "Ġout": 484, "...": 485, "Ġwill": 486, "ãģ": 487, "ive": 488, "н": 489, "Ġfrom": 490, "ain": 491, "ĠWe": 492, "Ġup": 493, "pe": 494, "res": 495, "ca": 496, "ĠR": 497, "Ġif": 498, "Ġpl": 499, "Ġdon": 500, "ack": 501, "Ġ1": 502, "Ġ\"": 503, "Ġtr": 504, "Ġus": 505, "ĠWh": 506, "ity": 507, "ĠJ": 508, "ĠYou": 509, "Ġhere": 510, "her": 511, "Ġsome": 512, "oug": 513, "ak": 514, "ard": 515, "Ġgoing": 516, "Ġun": 517, "ment": 518, "Ġthink": 519, "Ġpe": 520, "end": 521, "Ġ(": 522, "cause": 523, "Ġtim": 524, "ast": 525, "é": 526, "Ġour": 527, "Ġwant": 528, "ame": 529, "ies": 530, "Ġë": 531, "ud": 532, "ine": 533, "Ġreally": 534, "Ġte": 535, "Ġsee": 536, "ci": 537, "Ġby": 538, "so": 539, "ure": 540, "ose": 541, "Ġ[": 542, "are": 543, "Ġmore": 544, "ah": 545, "one": 546, "ck": 547, "ople": 548, "аÐ": 549, "Ġthen": 550, "Ġthing": 551, "Ġthem": 552, "ven": 553, "ound": 554, "ost": 555, "ong": 556, "ect": 557, "Ġright": 558, "ag": 559, "Ġint": 560, "Ġpeople": 561, "Ġwhen": 562, "ous": 563, "pl": 564, "Ġtime": 565, "Ġim": 566, "Ġwho": 567, "Ġ2": 568, "ap": 569, "Ġbecause": 570, "hing": 571, "Ġno": 572, "ice": 573, "Ġlook": 574, "Ġhas": 575, "Ġwould": 576, "Ġhow": 577, "act": 578, "Ġfe": 579, "nt": 580, "ough": 581, "Ġpr": 582, "ĠBut": 583, "Ġsay": 584, "Ñĥ": 585, "Ġnow": 586, "Ġman": 587, "Ġvery": 588, "Ġwork": 589, "iz": 590, "ĠK": 591, "iv": 592, "itt": 593, "Ġar": 594, "ep": 595, "Ġcl": 596, "Ġwhich": 597, "Ġco": 598, "ans": 599, "'ve": 600, "Ġsa": 601, "ff": 602, "'ll": 603, "Ġany": 604, "Ġact": 605, "Ġye": 606, "ber": 607, "ach": 608, "age": 609, "per": 610, "Ġalso": 611, "fer": 612, "Ġthese": 613, "Ġad": 614, "еÐ": 615, "ther": 616, "ace": 617, "ick": 618, "ake": 619, "reat": 620, "ire": 621, "ue": 622, "Ġag": 623, "ĠU": 624, "uch": 625, "ions": 626, "ry": 627, "00": 628, "na": 629, "Ġdid": 630, "Ġque": 631, "Ġhad": 632, "Ġevery": 633, "ĠHe": 634, "Ġla": 635, "Ġway": 636, "Ġsp": 637, "ble": 638, "ĠThis": 639, "ass": 640, "Ġtheir": 641, "ite": 642, "Ġneed": 643, "Ġpart": 644, "Ġwere": 645, "Ġback": 646, "ip": 647, "own": 648, "omet": 649, "be": 650, "ase": 651, "Ġmake": 652, "irst": 653, "ia": 654, "ence": 655, "ang": 656, "ank": 657, "Ġgot": 658, "Ġpre": 659, "Ġcont": 660, "Ġother": 661, "pt": 662, "ĠThat": 663, "og": 664, "Ġgood": 665, "Ġinto": 666, "alk": 667, "Ġbeen": 668, "Ġam": 669, "Ġover": 670, "ually": 671, "Ġâ": 672, "ìĿ": 673, "Ġund": 674, "he": 675, "way": 676, "Ġgr": 677, "ÑĮ": 678, "Ġdif": 679, "Ġper": 680, "Ñı": 681, "ĠIn": 682, "Ġtw": 683, "ond": 684, "ars": 685, "int": 686, "orm": 687, "Ġlot": 688, "Ġwhere": 689, "ĠÃ": 690, "ĠV": 691, "Ġsomet": 692, "л": 693, "ens": 694, "Ġgu": 695, "Ġac": 696, "ug": 697, "Ñĭ": 698, "ı": 699, "Ġfirst": 700, "ree": 701, "Ġhis": 702, "ittle": 703, "Ġimp": 704, "Ġmo": 705, "av": 706, "Ġlittle": 707, "ĠWhat": 708, "Ġmuch": 709, "Ġz": 710, "Ġê": 711, "able": 712, "Ġп": 713, "Ġpo": 714, "Ġcomp": 715, "ne": 716, "Ġdis": 717, "Ġlet": 718, "ance": 719, "Ġher": 720, "Ġthings": 721, "Ġstart": 722, "ult": 723, "Ġapp": 724, "Ġres": 725, "Ġfo": 726, "Ġcould": 727, "Ġinter": 728, "Ġthose": 729, "Ġdes": 730, "Ġwell": 731, "Ġtwo": 732, "Ġkind": 733, "xt": 734, "ress": 735, "ely": 736, "ä": 737, "Ġbr": 738, "Ġthr": 739, "Ġв": 740, "Ġi": 741, "ish": 742, "Ġdiffer": 743, "Ġro": 744, "ĠSt": 745, "Ġsomething": 746, "Ġtake": 747, "Ġbo": 748, "ys": 749, "Ġshe": 750, "Ġtalk": 751, "lo": 752, "Ñĩ": 753, "Ġeven": 754, "к": 755, "ãĢ": 756, "Ġн": 757, "Ġbu": 758, "ĠIf": 759, "Ġdown": 760, "ĠCh": 761, "ade": 762, "ations": 763, "Ġuse": 764, "ord": 765, "Ġoff": 766, "Ġactually": 767, "Ġspe": 768, "du": 769, "ated": 770, "ater": 771, "oss": 772, "ning": 773, "ü": 774, "Ġdoes": 775, "ĠÑģ": 776, "Ġnew": 777, "Ġbet": 778, "vel": 779, "cess": 780, "ple": 781, "Ġhapp": 782, "ting": 783, "onna": 784, "Ġes": 785, "Ġday": 786, "Ġonly": 787, "ign": 788, "kay": 789, "sel": 790, "ents": 791, "ount": 792, "ild": 793, "ile": 794, "Ġsc": 795, "Ġhim": 796, "Ġagain": 797, "ving": 798, "Ġgonna": 799, "Ġcomm": 800, "Ġhel": 801, "other": 802, "Ġke": 803, "ical": 804, "Ġ3": 805, "Ġel": 806, "Ġthrough": 807, "Ġcome": 808, "ark": 809, "day": 810, "ier": 811, "ó": 812, "Ġthan": 813, "ĠThey": 814, "Ġmay": 815, "Ġser": 816, "íķ": 817, "Ġcall": 818, "Ġdifferent": 819, "Ġshould": 820, "ĠThere": 821, "ary": 822, "ĠNow": 823, "ãĤ": 824, "thing": 825, "we": 826, "ory": 827, "fter": 828, "Ġput": 829, "ors": 830, "ial": 831, "ëĭ": 832, "Ġunder": 833, "Ġinc": 834, "ĠYe": 835, "ub": 836, "form": 837, "Ġvide": 838, "à¸": 839, "vers": 840, "Ġfeel": 841, "á": 842, "ody": 843, "ft": 844, "fore": 845, "Ġem": 846, "get": 847, "Ġsaid": 848, "ition": 849, "Ġrec": 850, "ious": 851, "atch": 852, "Ġtry": 853, "Ġhelp": 854, "Ġshow": 855, "д": 856, "Ġbit": 857, "ull": 858, "в": 859, "ÑĤо": 860, "gr": 861, "Ġplay": 862, "ife": 863, "ail": 864, "ĠYeah": 865, "Ġquest": 866, "Ġmany": 867, "Ġpers": 868, "Ġgreat": 869, "ÃŃ": 870, "Ġest": 871, "ng": 872, "ĠâĻ": 873, "ty": 874, "la": 875, "ĠOh": 876, "Ġ×": 877, "à®": 878, "ĠBe": 879, "ady": 880, "Ġmost": 881, "ction": 882, "ĠNo": 883, "Ġdoing": 884, "Ġbeing": 885, "Ġtoo": 886, "ces": 887, "Ġbl": 888, ".\"": 889, "Ġrem": 890, "iss": 891, "ons": 892, ">>": 893, "ru": 894, "wn": 895, "ont": 896, "ib": 897, "ell": 898, "Ġsm": 899, "oth": 900, "ual": 901, "Ġ>>": 902, "Ġph": 903, "les": 904, "oc": 905, "ful": 906, "Ġsec": 907, "ise": 908, "Ġadd": 909, "igh": 910, "ert": 911, "Ġsame": 912, "âĢ": 913, "Ġmean": 914, "Ġfind": 915, "ek": 916, "Ġend": 917, "--": 918, "м": 919, "Ġstill": 920, "az": 921, "Ġ'": 922, "Ġmin": 923, "Ġyears": 924, "urn": 925, "Ġaround": 926, "self": 927, "Ġwr": 928, "bs": 929, "ought": 930, "ĠâĻª": 931, "Ġfl": 932, "ange": 933, "Ġafter": 934, "Ġpoint": 935, "mer": 936, "ved": 937, "Ġlong": 938, "oy": 939, "ä¸": 940, "Ġcr": 941, "ways": 942, "Ġsy": 943, "Ġtra": 944, "Ġ20": 945, "ave": 946, "Ġche": 947, "Ġent": 948, "Ġbefore": 949, "ph": 950, "Ġatt": 951, "ian": 952, "ily": 953, "Ġperson": 954, "Ġbig": 955, "Ġsch": 956, "Ġreal": 957, "Ġnext": 958, "Ġlove": 959, "Ġvideo": 960, "ĠLet": 961, "Ġfin": 962, "Ġmak": 963, "ible": 964, "Ġtoday": 965, "erm": 966, "ĠAl": 967, "ower": 968, "ann": 969, "ix": 970, "Ġpar": 971, "Ġstud": 972, "ö": 973, "Ġimport": 974, "te": 975, "Ġgive": 976, "ves": 977, "Ġdie": 978, "Ġdec": 979, "Ġtell": 980, "Ġк": 981, "ÑģÑĤ": 982, "Ġwhy": 983, "ically": 984, "ict": 985, "red": 986, "Ġbas": 987, "Ġsure": 988, "Ġbel": 989, "ating": 990, "Ġtak": 991, "Ġset": 992, "Ġlife": 993, "Ġdidn": 994, "ا": 995, "ob": 996, "und": 997, "ath": 998, "Ġop": 999, "Ġо": 1000, "ait": 1001, "Ġworld": 1002, "Ġsupp": 1003, "io": 1004, "Ġcour": 1005, "Ġи": 1006, "ward": 1007, "ен": 1008, "Ġalways": 1009, "up": 1010, "Ġhand": 1011, "ĠHow": 1012, "cial": 1013, "Ġcons": 1014, "ĠÑ": 1015, "Ġind": 1016, "Ġ4": 1017, "ĠAs": 1018, "Ġfun": 1019, "ject": 1020, "Ġimportant": 1021, "Ġsur": 1022, "ew": 1023, "ates": 1024, "Ġ5": 1025, "Ġdi": 1026, "Ġmade": 1027, "Ġins": 1028, "Ġask": 1029, "Ġet": 1030, "Ġnum": 1031, "Ġcar": 1032, "ĠOkay": 1033, "Ġsim": 1034, "ik": 1035, "Ġlast": 1036, "ĠGo": 1037, "Ġmus": 1038, "Ġrel": 1039, "ular": 1040, "´ì": 1041, "ĠWell": 1042, "pect": 1043, "ĠThank": 1044, "Ġthree": 1045, "ã": 1046, "ãĥ": 1047, "Ġinv": 1048, "Ġgen": 1049, "lic": 1050, "Ġhappen": 1051, "ëĬ": 1052, "ien": 1053, "ever": 1054, "ов": 1055, "Ġstr": 1056, "ĠAll": 1057, "Ġinst": 1058, "ĠâĢ": 1059, "Ġdef": 1060, "Ġsl": 1061, "Ġmight": 1062, "ung": 1063, "Ġyear": 1064, "Ġown": 1065, "Ġkeep": 1066, "body": 1067, "der": 1068, "ĠÑĤ": 1069, "Ġд": 1070, "Ġanother": 1071, "Ġmod": 1072, "Ġev": 1073, "Ġguys": 1074, "Ġable": 1075, "ão": 1076, "que": 1077, "ident": 1078, "ĠYes": 1079, "Ġits": 1080, "Ġplace": 1081, "Ġprodu": 1082, "arn": 1083, "Ġм": 1084, "Ġrep": 1085, "Ġexper": 1086, "Ġfam": 1087, "ities": 1088, "ific": 1089, "Ġhigh": 1090, "ied": 1091, "ool": 1092, "iew": 1093, "еÑĤ": 1094, "ren": 1095, "Ġdone": 1096, "Ġ...": 1097, "ëĬĶ": 1098, "stem": 1099, "ĠSe": 1100, "Ġbetter": 1101, "come": 1102, "Ġdel": 1103, "Ġty": 1104, "Ġum": 1105, "Ġho": 1106, "ĠAn": 1107, "Ġmon": 1108, "ings": 1109, "Ġsk": 1110, "Ġob": 1111, "com": 1112, "blem": 1113, "ope": 1114, "stand": 1115, "'d": 1116, "ments": 1117, "Ġele": 1118, "ĠIs": 1119, "Ġda": 1120, "Ġreg": 1121, "lease": 1122, "ike": 1123, "als": 1124, "ize": 1125, "ê°": 1126, "Ġcare": 1127, "Ġnever": 1128, "ìĿ´": 1129, "ese": 1130, "Ġmet": 1131, "olog": 1132, "ĠWhen": 1133, "uck": 1134, "еÑĢ": 1135, "Ġé": 1136, "Ġdat": 1137, "ç": 1138, "Ġexam": 1139, "ility": 1140, "Ġdet": 1141, "cri": 1142, "Ġused": 1143, "ĠDo": 1144, "Ġtrans": 1145, "eg": 1146, "ten": 1147, "Ñİ": 1148, "cus": 1149, "Ġsecond": 1150, "Ġbest": 1151, "Ġhard": 1152, "Ġide": 1153, "Ġproblem": 1154, "ê³": 1155, "ĠUn": 1156, "Ñħ": 1157, "ĠÎ": 1158, "Ġwatch": 1159, "ĠSh": 1160, "atter": 1161, "Ġpret": 1162, "Ġder": 1163, "Ġcourse": 1164, "ÅŁ": 1165, "ative": 1166, "ics": 1167, "Ġquestion": 1168, "ute": 1169, "ìĹ": 1170, "ĠFor": 1171, "ather": 1172, "Ġcol": 1173, "iend": 1174, "Ġí": 1175, "ĠZ": 1176, "Ġdoesn": 1177, "arch": 1178, "Ġinterest": 1179, "Ġpol": 1180, "Ġcor": 1181, "ience": 1182, "Ġpres": 1183, "Ġeach": 1184, "Ġsystem": 1185, "Ġfact": 1186, "iel": 1187, "ably": 1188, "Ġer": 1189, "Ġrun": 1190, "ĠìĿ": 1191, "Ġtop": 1192, "ner": 1193, "Ġthought": 1194, "Ġeas": 1195, "ient": 1196, "Ġcre": 1197, "ÑĪ": 1198, "Ġcommun": 1199, "ye": 1200, "ready": 1201, "llow": 1202, "Ġeverything": 1203, "omm": 1204, "Ġmed": 1205, "ļĶ": 1206, "Ġcount": 1207, "its": 1208, "Ġcompl": 1209, "hip": 1210, "ÙĦ": 1211, "ook": 1212, "Ġtoget": 1213, "Ġtogether": 1214, "amp": 1215, "Ġgame": 1216, "Ġalready": 1217, "ал": 1218, "Ġcalled": 1219, "ale": 1220, "ÅĤ": 1221, "ĠMy": 1222, "Ġunderstand": 1223, "Ġdr": 1224, "Ġmom": 1225, "ited": 1226, "ол": 1227, "Ġusing": 1228, "zy": 1229, "Ġnumber": 1230, "ãĢģ": 1231, "ced": 1232, "Ġcle": 1233, "но": 1234, "ëĭ¤": 1235, "ince": 1236, "Ġlooking": 1237, "Ġpretty": 1238, "Ġprob": 1239, "ĠShe": 1240, "Ġve": 1241, "Ġgetting": 1242, "Ġweek": 1243, "Ġeff": 1244, "uff": 1245, "air": 1246, "ues": 1247, "ern": 1248, "ĠQ": 1249, "oup": 1250, "ention": 1251, "Ġside": 1252, "ом": 1253, "Ġform": 1254, "Ġbus": 1255, "Ġass": 1256, "Ġed": 1257, "ason": 1258, "ween": 1259, "â̦": 1260, "Ġturn": 1261, "Ġcur": 1262, "Ġcoll": 1263, "Ġdire": 1264, "ĠGod": 1265, "Ġ10": 1266, "Ġequ": 1267, "Ġб": 1268, "Ġopen": 1269, "Ġsuch": 1270, "ird": 1271, "ак": 1272, "Ġear": 1273, "ÄĻ": 1274, "gan": 1275, "Ġpartic": 1276, "Ġfriend": 1277, "Ġexp": 1278, "Ġext": 1279, "Ġhome": 1280, "Ġwater": 1281, "ĠOn": 1282, "ÑĤÑĮ": 1283, "ork": 1284, "ĠпÑĢ": 1285, "Ġmove": 1286, "ness": 1287, "ense": 1288, "ho": 1289, "Ġchar": 1290, "co": 1291, "ins": 1292, "Ġboth": 1293, "Ġ19": 1294, "Ġgra": 1295, "Ġbetween": 1296, "á»": 1297, "Ġìķ": 1298, "ash": 1299, "ĠRe": 1300, "ai": 1301, "alth": 1302, "ures": 1303, "ember": 1304, "Ġav": 1305, "Ġver": 1306, "ê": 1307, "oney": 1308, "Ġthank": 1309, "Ġmaybe": 1310, "uc": 1311, "ime": 1312, "ê³ł": 1313, "Ġaway": 1314, "Ġname": 1315, "ouse": 1316, "Ġacc": 1317, "Ġmusic": 1318, "Ġchange": 1319, "Ġpass": 1320, "ger": 1321, "Ġbuild": 1322, "Ġval": 1323, "iness": 1324, "any": 1325, "Ġfew": 1326, "´ë": 1327, "ta": 1328, "Ġlist": 1329, "Ã¥": 1330, "Ġold": 1331, "Ġìŀ": 1332, "Ġsort": 1333, "Ġmem": 1334, "Ġca": 1335, "cept": 1336, "Ġgener": 1337, "Ġyeah": 1338, "Ġwhile": 1339, "Ġanything": 1340, "ric": 1341, "gram": 1342, "Ġein": 1343, "cy": 1344, "uring": 1345, "ĠDe": 1346, "Ġpower": 1347, "Ġcoming": 1348, "Ġword": 1349, "Ġ--": 1350, "Ġbelie": 1351, "Ġfound": 1352, "to": 1353, "п": 1354, "Ġmeans": 1355, "Ġinform": 1356, "ĠØ": 1357, "ĠÑĩ": 1358, "Ġsmall": 1359, "000": 1360, "Ġcame": 1361, "Ġíķ": 1362, "wh": 1363, "Ġworking": 1364, "Ġexample": 1365, "Ġpos": 1366, "Ġdep": 1367, "ê²": 1368, "äº": 1369, "ote": 1370, "Ġdem": 1371, "ì§": 1372, "ts": 1373, "Ġvar": 1374, "aut": 1375, "Ġtri": 1376, "chn": 1377, "Ġhead": 1378, "Ġwhole": 1379, "×Ļ": 1380, "ze": 1381, "Ġtrying": 1382, "Ġtem": 1383, "Ġcou": 1384, "ets": 1385, "Ġ6": 1386, "Ġfil": 1387, "velop": 1388, "Ġcase": 1389, "à¯": 1390, "Ġprobably": 1391, "Ġokay": 1392, "Ġplan": 1393, "Ġsit": 1394, "Ġschool": 1395, "ĠThen": 1396, "¸ë": 1397, "me": 1398, "Ġprocess": 1399, "Ġfar": 1400, "Ġread": 1401, "Ġposs": 1402, "Ġbre": 1403, "Ġsol": 1404, "icht": 1405, "Ġsupport": 1406, "ĠTo": 1407, "ertain": 1408, "Ġstarted": 1409, "Ġcap": 1410, "Ġleft": 1411, "Ġdata": 1412, "Ġtimes": 1413, "ел": 1414, "Ġwanted": 1415, "ан": 1416, "Ġtalking": 1417, "Ġist": 1418, "Ġhaving": 1419, "ump": 1420, "Ġcontin": 1421, "Ġsub": 1422, "Ġз": 1423, "pr": 1424, "ëĭĪ": 1425, "ina": 1426, "ż": 1427, "Ġcreat": 1428, "ode": 1429, "×ķ": 1430, "æĺ": 1431, "!!": 1432, "Ġterm": 1433, "ism": 1434, "од": 1435, "ĠBecause": 1436, "Ġwent": 1437, "ider": 1438, "Ġprov": 1439, "Ġchild": 1440, "Ġden": 1441, "Ġlight": 1442, "br": 1443, "³Ð¾": 1444, "oh": 1445, "Ġbook": 1446, "ĠÙ": 1447, "ution": 1448, "ĠJust": 1449, "ene": 1450, "Ġfour": 1451, "Ġvis": 1452, "ê°Ģ": 1453, "Ġhope": 1454, "Ġmaking": 1455, "ĠLe": 1456, "ìķ": 1457, "Ġopp": 1458, "au": 1459, "Ġmoney": 1460, "Ġprogram": 1461, "è": 1462, "Ġstand": 1463, "IN": 1464, "Ġsign": 1465, "Ġlearn": 1466, "Ãł": 1467, "ĠDon": 1468, "Ġteam": 1469, "Ġна": 1470, "lud": 1471, "Ġrest": 1472, "ices": 1473, "æľ": 1474, "ĠÑĢ": 1475, "Ġaut": 1476, "Ġlead": 1477, "ational": 1478, "de": 1479, "gy": 1480, "Ġnice": 1481, "Ġdas": 1482, "Ġdist": 1483, "Ġhum": 1484, "ĠOne": 1485, "æĪ": 1486, "Ġcomes": 1487, "Ġjo": 1488, "Ġcent": 1489, "Ġexpl": 1490, "Ġmark": 1491, "reen": 1492, "led": 1493, "gin": 1494, "ìļĶ": 1495, "Ġlevel": 1496, "Ġconf": 1497, "ush": 1498, "Ġdevelop": 1499, "Ġtest": 1500, "eng": 1501, "vious": 1502, "ature": 1503, "ем": 1504, "ret": 1505, "Ġje": 1506, "Ġstuff": 1507, "Ġclass": 1508, "ows": 1509, "Ġê·": 1510, "Ġsi": 1511, "Ġles": 1512, "rop": 1513, "çļ": 1514, "Ġpor": 1515, "Ġwar": 1516, "ìĹIJ": 1517, "Ġeveryone": 1518, "Ġge": 1519, "Ġcheck": 1520, "ott": 1521, "Ġsing": 1522, "Ġart": 1523, "Ġfollow": 1524, "Ġ201": 1525, "ĠFr": 1526, "ais": 1527, "ìĸ": 1528, "α": 1529, "å°": 1530, "ĠÃł": 1531, "imes": 1532, "Ġret": 1533, "Ġchang": 1534, "Ġpub": 1535, "Ġinf": 1536, "Ġtechn": 1537, "ada": 1538, "ives": 1539, "Ġbeh": 1540, "æĺ¯": 1541, "Ġlooks": 1542, "ãĢĤ": 1543, "з": 1544, "ĠWhy": 1545, "çļĦ": 1546, "Ġenough": 1547, "Ġbra": 1548, "itch": 1549, "ä»": 1550, "Ġadv": 1551, "б": 1552, "Ġwithout": 1553, "wer": 1554, "meric": 1555, "den": 1556, "Ġcomplet": 1557, "Ġidea": 1558, "ters": 1559, "ock": 1560, "Ġdefin": 1561, "Ġever": 1562, "Ġgl": 1563, "Ġonce": 1564, "Ġbring": 1565, "Ġsaying": 1566, "Ġans": 1567, "Ġhear": 1568, "nect": 1569, "Ġless": 1570, "go": 1571, "ream": 1572, "ado": 1573, "ìŀ": 1574, "Ġmind": 1575, "ente": 1576, "Ġfull": 1577, "Ġbad": 1578, "Ġwom": 1579, "Ġsomeone": 1580, "Ġdu": 1581, "Ġwon": 1582, "Ġcontro": 1583, "ortun": 1584, "Ġhealth": 1585, "Ġcho": 1586, "ĠAr": 1587, "Ġconc": 1588, "Ġinformation": 1589, "Ġstop": 1590, "att": 1591, "ately": 1592, "ä½": 1593, "Ġgroup": 1594, "ĠÑĥ": 1595, "Ġquite": 1596, "Ġresp": 1597, "ER": 1598, "ught": 1599, "ê¸": 1600, "man": 1601, "ized": 1602, "ĠBr": 1603, "Ġremember": 1604, "Ġfamily": 1605, "Ġbusiness": 1606, "aw": 1607, "Ġspec": 1608, "Ġau": 1609, "ĠOr": 1610, "Äħ": 1611, "Ġseen": 1612, "Ġlar": 1613, "Ġ7": 1614, "gg": 1615, "bers": 1616, "Ġdra": 1617, "Ġmonth": 1618, "Ġsays": 1619, "Ġiss": 1620, "Ġlive": 1621, "Ġline": 1622, "Ġmoment": 1623, "Ġexc": 1624, "els": 1625, "Ġsound": 1626, "Ġcool": 1627, "Ġloc": 1628, "Ġcertain": 1629, "Ġdri": 1630, "оÑĤ": 1631, "ames": 1632, "Ġmust": 1633, "ny": 1634, "иÑĤ": 1635, "Ġkid": 1636, "Ġinclud": 1637, "ìĿĦ": 1638, "ator": 1639, "ÄŁ": 1640, "ha": 1641, "ared": 1642, "Ġseem": 1643, "й": 1644, "ìĦ": 1645, "Ġelse": 1646, "Ġìł": 1647, "irl": 1648, "Ġ8": 1649, "Ġvo": 1650, "Ġquestions": 1651, "ines": 1652, "ee": 1653, "æĪij": 1654, "ür": 1655, "ĠAmeric": 1656, "Ġstory": 1657, "Ġserv": 1658, "vern": 1659, "ages": 1660, "land": 1661, "ĠâĢĵ": 1662, "era": 1663, "ĠCan": 1664, "Ġpop": 1665, "ether": 1666, "Ġna": 1667, "Ġorder": 1668, "Ġmakes": 1669, "Ġsince": 1670, "con": 1671, "ctor": 1672, "Ġthough": 1673, "Ġproduct": 1674, "ли": 1675, "Ġleg": 1676, "Ġmeet": 1677, "alf": 1678, "ÑģÑı": 1679, "unch": 1680, "iter": 1681, "ove": 1682, "×ķ×": 1683, "iet": 1684, "ам": 1685, "ital": 1686, "Ġsuper": 1687, "ling": 1688, "Ġpay": 1689, "Ġpara": 1690, "Ġjob": 1691, "ĠHere": 1692, "Ġsw": 1693, "ks": 1694, "ption": 1695, "ma": 1696, "Ġbelieve": 1697, "¬ë": 1698, "Ġwait": 1699, "ой": 1700, "Ġunt": 1701, "Ġquick": 1702, "hr": 1703, "ĠÑį": 1704, "ĠPro": 1705, "Ġmen": 1706, "à¹": 1707, "Ġdays": 1708, "Ġgoes": 1709, "Ġspeak": 1710, "ĠAt": 1711, "ement": 1712, "Ġmiss": 1713, "Ġaw": 1714, "Ġdesign": 1715, "Ġproject": 1716, "оÑĢ": 1717, "ij": 1718, "ants": 1719, "ats": 1720, "ĠChr": 1721, "Ġ9": 1722, "Ġcut": 1723, "Ġrequ": 1724, "Ġне": 1725, "ĠNot": 1726, "aster": 1727, "Ġmill": 1728, "Ġparticular": 1729, "Ġpie": 1730, "Ġstudents": 1731, "Ġfive": 1732, "oun": 1733, "ĠNe": 1734, "Ġgi": 1735, "Ġpas": 1736, "Ġfree": 1737, "ĠSp": 1738, "lich": 1739, "Ġprof": 1740, "Ġeng": 1741, "Ġprot": 1742, "ĠLike": 1743, "osed": 1744, "Ġconnect": 1745, "app": 1746, "Ġë§": 1747, "iting": 1748, "Ġblo": 1749, "Ġlos": 1750, "ists": 1751, "Ġexperience": 1752, "rent": 1753, "Ġstay": 1754, "Ġfood": 1755, "ton": 1756, "ruct": 1757, "Ġhist": 1758, "view": 1759, "ining": 1760, "most": 1761, "ivers": 1762, "bo": 1763, "ãģĦ": 1764, "ĠTr": 1765, "gen": 1766, "Ġplease": 1767, "Ġcommunity": 1768, "Ġce": 1769, "AN": 1770, "no": 1771, "Ġbody": 1772, "Ġhour": 1773, "Ġvers": 1774, "áº": 1775, "cer": 1776, "Ġê°": 1777, "Ġreason": 1778, "ĠRight": 1779, "Ġlater": 1780, "ÏĦ": 1781, "Ġhouse": 1782, "ĠX": 1783, "он": 1784, "Ġstate": 1785, "fic": 1786, "å¤": 1787, "ÅĽ": 1788, "ield": 1789, "Ġpri": 1790, "Ġpast": 1791, "Ġwalk": 1792, "ology": 1793, "ering": 1794, "anna": 1795, "Ġter": 1796, "Ġhold": 1797, "Ġorgan": 1798, "ben": 1799, "ο": 1800, "ón": 1801, "Ġeffect": 1802, "Ġyourself": 1803, "Ġplus": 1804, "aj": 1805, "ando": 1806, "ural": 1807, "Ġroom": 1808, "lect": 1809, "ê²Į": 1810, "?\"": 1811, "side": 1812, "Ġbecome": 1813, "ÑĨ": 1814, "ĠÂ": 1815, "ood": 1816, "Ġconst": 1817, "Ġnight": 1818, "utes": 1819, "ж": 1820, "Ġbreak": 1821, "Ġpain": 1822, "Ġstep": 1823, "ired": 1824, "Ġnothing": 1825, "Ġuntil": 1826, "Ñĸ": 1827, "ав": 1828, "ÙĬ": 1829, "Ġduring": 1830, "ì§Ģ": 1831, "less": 1832, "oll": 1833, "нÑĭ": 1834, "ι": 1835, "fect": 1836, "iver": 1837, "ıĦ": 1838, "ither": 1839, "ying": 1840, "Ġbegin": 1841, "×Ļ×": 1842, "ivid": 1843, "Ġç": 1844, "Ġsal": 1845, "Ġta": 1846, "Ġpot": 1847, "Ġ$": 1848, "Ġmar": 1849, "Ġclear": 1850, "Ġface": 1851, "Ġgrow": 1852, "Ġ*": 1853, "Ġinside": 1854, "Ġfriends": 1855, "Ġleave": 1856, "enn": 1857, "Ġeasy": 1858, "Ġarea": 1859, "ality": 1860, "oud": 1861, "Ġeat": 1862, "ÙĨ": 1863, "Ġpur": 1864, "orn": 1865, "Ġsaw": 1866, "Ġanswer": 1867, "Ġfront": 1868, "Ġbeaut": 1869, "¼ë": 1870, "Ġmatter": 1871, "Ġson": 1872, "ĠNew": 1873, "Ġresult": 1874, "ides": 1875, "che": 1876, "Ġfut": 1877, "ps": 1878, "Ġfocus": 1879, "Ġinteresting": 1880, "å¥": 1881, "Ġap": 1882, "\".": 1883, "Ġcreate": 1884, "оÑģ": 1885, "Ġpress": 1886, "ross": 1887, "Ġpick": 1888, "line": 1889, "Ġtook": 1890, "ĠMay": 1891, "row": 1892, "Ġich": 1893, "ĺë": 1894, "Ġref": 1895, "Ġmor": 1896, "ract": 1897, "arent": 1898, "AR": 1899, "Ġexact": 1900, "Ġspace": 1901, "work": 1902, "ни": 1903, "Ġbir": 1904, "Ġdev": 1905, "г": 1906, "Ġtold": 1907, "Ġpublic": 1908, "cially": 1909, "Ġview": 1910, "ĠHey": 1911, "med": 1912, "llo": 1913, "cc": 1914, "Ġfac": 1915, "Ġcouple": 1916, "Ġheart": 1917, "ler": 1918, "Ġready": 1919, "Ġalmost": 1920, "aring": 1921, "Ġhalf": 1922, "ĠMe": 1923, "avor": 1924, "ique": 1925, "Ġcharac": 1926, "Ġpract": 1927, "ON": 1928, "ane": 1929, "Ġil": 1930, "на": 1931, "Ġvi": 1932, "lish": 1933, "head": 1934, "Ġleast": 1935, "Ġbasically": 1936, "ased": 1937, "right": 1938, "Ġyet": 1939, "Ġtaking": 1940, "Ġcountry": 1941, "Ġwin": 1942, "Ġisn": 1943, "Ġpossible": 1944, "Ġcam": 1945, "Ġincre": 1946, "Ġpat": 1947, "Ġwanna": 1948, "Ġconsider": 1949, "Ġabs": 1950, "Ġwithin": 1951, "Ġhuman": 1952, "Ġthinking": 1953, "Ġoh": 1954, "¡ľ": 1955, "Ġqui": 1956, "ases": 1957, "Ġ0": 1958, "itely": 1959, "ä¸į": 1960, "Ġkill": 1961, "Ġmil": 1962, "Ġinvest": 1963, "ister": 1964, "Ġsuc": 1965, "ional": 1966, "elf": 1967, "Ġwhether": 1968, "Ġcontrol": 1969, "Ġagainst": 1970, "ots": 1971, "ëĭĪëĭ¤": 1972, "ior": 1973, "Ġpresent": 1974, "Ġا": 1975, "Ġwatching": 1976, "ube": 1977, "erv": 1978, "Ġnicht": 1979, "Ġgovern": 1980, "ĠThese": 1981, "Ġ:": 1982, "uit": 1983, "ugh": 1984, "Ġworks": 1985, "oo": 1986, "Ġwir": 1987, "Ġair": 1988, "ĠTe": 1989, "аз": 1990, "ision": 1991, "where": 1992, "Ġtot": 1993, "joy": 1994, "ìĭ": 1995, "Ġvol": 1996, "Ġе": 1997, "Ġclose": 1998, "ĠAd": 1999, "Ñī": 2000, "ined": 2001, "Ġuna": 2002, "Ġê·¸ë": 2003, "°ë": 2004, "orry": 2005, "Ġbro": 2006, "Ġfilm": 2007, "ift": 2008, "20": 2009, "Ġtype": 2010, "Ġhappened": 2011, "ĠAm": 2012, "Ġgirl": 2013, "ĠAre": 2014, "wards": 2015, "Ġpour": 2016, "Ġcolor": 2017, "elt": 2018, "аÑģ": 2019, "Ġsense": 2020, "lex": 2021, "ĠWith": 2022, "uss": 2023, "rib": 2024, "Ġrese": 2025, "Ġnorm": 2026, "Ġfuture": 2027, "Ġdeal": 2028, "ending": 2029, "ey": 2030, "Ġx": 2031, "ero": 2032, "ĠCl": 2033, "uk": 2034, "Ġwhatever": 2035, "selves": 2036, "Ġyoung": 2037, "ìĬ": 2038, "ĠMar": 2039, "ĠChrist": 2040, "Ġguess": 2041, "Ġperform": 2042, "Ġener": 2043, "ron": 2044, "Ġhit": 2045, "Ġwond": 2046, "Ġdirect": 2047, "ĠEvery": 2048, "Ġoften": 2049, "Ġfa": 2050, "Ġalong": 2051, "Ġclick": 2052, "ĠLook": 2053, "Ġsitu": 2054, "Ġhappy": 2055, "ead": 2056, "Ġago": 2057, "Ġenc": 2058, "Ġmyself": 2059, "Ġcover": 2060, "об": 2061, "Ġmid": 2062, "Ġcost": 2063, "Ġten": 2064, "ĠSch": 2065, "Ġexpect": 2066, "Ġwasn": 2067, "Ġstrong": 2068, "iful": 2069, "Ġopportun": 2070, "inal": 2071, "yle": 2072, "Ġshare": 2073, "Ġtrue": 2074, "Ġappro": 2075, "Ġchall": 2076, "Ġminutes": 2077, "Ġchann": 2078, "ĠëĤ": 2079, "ε": 2080, "li": 2081, "Ġmess": 2082, "ories": 2083, "pecially": 2084, "Ġwrong": 2085, "Ġyes": 2086, "ĠìĹ": 2087, "iron": 2088, "Ġallow": 2089, "Ġsubs": 2090, "Ġfore": 2091, "Ġfight": 2092, "Ġsocial": 2093, "Ġcra": 2094, "ana": 2095, "Ġaff": 2096, "Ġess": 2097, "Ġways": 2098, "Ġshort": 2099, "Ġfall": 2100, "Ġlaw": 2101, "ĠWho": 2102, "Ġenjoy": 2103, "Ġcal": 2104, "Ġaccess": 2105, "fe": 2106, "Ġnon": 2107, "Ġacross": 2108, "ery": 2109, "viously": 2110, "ĠEx": 2111, "ided": 2112, "Ġlink": 2113, "ĠPr": 2114, "Ġterms": 2115, "aces": 2116, "Ġland": 2117, "azing": 2118, "Ġ15": 2119, "Ġmult": 2120, "Ġspecial": 2121, "åĢ": 2122, "iving": 2123, "ìĿĢ": 2124, "Ġtyp": 2125, "Ġste": 2126, "ĠÄ": 2127, "Ġforward": 2128, "åı": 2129, "Ġfre": 2130, "好": 2131, "Ġresearch": 2132, "à¯į": 2133, "аÑĤ": 2134, "Ġmain": 2135, "Ġrecord": 2136, "Ġhu": 2137, "Ġdefinitely": 2138, "Ġeither": 2139, "Ġlisten": 2140, "Ġkey": 2141, "Ġmarket": 2142, "ĠÑĩÑĤо": 2143, "ization": 2144, "Ġvideos": 2145, "Ġguy": 2146, "Ġfig": 2147, "Ġstra": 2148, "ĠPl": 2149, "ully": 2150, "amos": 2151, "Ġmention": 2152, "Ġsong": 2153, "Ġintern": 2154, "ral": 2155, "urs": 2156, "Ġhon": 2157, "Ġvalue": 2158, "Ġbar": 2159, "cle": 2160, "ож": 2161, "Äĩ": 2162, "ľë": 2163, "Ġzu": 2164, "им": 2165, "ä½ł": 2166, "Ġsingle": 2167, "Ġauch": 2168, "cuss": 2169, "Ġgets": 2170, "Ġsometimes": 2171, "å¾": 2172, "amb": 2173, "mm": 2174, "cing": 2175, "Ġperfect": 2176, "ĠBl": 2177, "outh": 2178, "ìł": 2179, "Ġsci": 2180, "par": 2181, "Ġred": 2182, "Ġpost": 2183, "Ġmot": 2184, "Ġelect": 2185, "ĠEu": 2186, "itive": 2187, "ĠSome": 2188, "Ġdescri": 2189, "Ġcurrent": 2190, "és": 2191, "Ġtre": 2192, "ĠEn": 2193, "Ġmit": 2194, "EN": 2195, "Īë": 2196, "ium": 2197, "Ġheard": 2198, "Ġsimple": 2199, "lar": 2200, "Ġeverybody": 2201, "ilar": 2202, "Ġneeds": 2203, "Ġdiffic": 2204, "ĠGood": 2205, "ument": 2206, "cent": 2207, "Ġoper": 2208, "аÑĤÑĮ": 2209, "ety": 2210, "Ġblack": 2211, "Ġgiven": 2212, "ones": 2213, "Ġwel": 2214, "éĢ": 2215, "ĠìķĦ": 2216, "Ġ30": 2217, "AT": 2218, "Ġstat": 2219, "ouch": 2220, "ĠMr": 2221, "аÑĢ": 2222, "Ġsho": 2223, "Ġcond": 2224, "×Ķ": 2225, "my": 2226, "Ġchildren": 2227, "Ġeu": 2228, "ед": 2229, "ìķĦ": 2230, "tern": 2231, "Ġuh": 2232, "Ġhar": 2233, "Ġprom": 2234, "Ġpull": 2235, "rew": 2236, "Ġcompany": 2237, "Ġbeautiful": 2238, "ustom": 2239, "íķĺ": 2240, "ки": 2241, "Ġstre": 2242, "Ġamazing": 2243, "ries": 2244, "Ġsuccess": 2245, "Ġmach": 2246, "not": 2247, "Ġdiscuss": 2248, "Ġnat": 2249, "¦¬": 2250, "Ġune": 2251, "Ġdifficult": 2252, "Ġris": 2253, "ν": 2254, "Ġcamp": 2255, "Ġbuy": 2256, "ä¸Ģ": 2257, "Ġmag": 2258, "po": 2259, "ĠYour": 2260, "Ġbehind": 2261, "ica": 2262, "ın": 2263, "ĠOK": 2264, "Ġlang": 2265, "Ġwomen": 2266, "Ġenv": 2267, "Ġrece": 2268, "Ġchannel": 2269, "ially": 2270, "ule": 2271, "Ġ12": 2272, "thers": 2273, "Ġbott": 2274, "Ġreport": 2275, "ently": 2276, "fully": 2277, "The": 2278, "Ġsent": 2279, "Ġevent": 2280, "Ġenergy": 2281, "lt": 2282, "Ġwords": 2283, "arr": 2284, "dle": 2285, "Ġahead": 2286, "ards": 2287, "ر": 2288, "äºĨ": 2289, "Ġtool": 2290, "conom": 2291, "еÑģ": 2292, "Ġexactly": 2293, "Ġfavor": 2294, "Ġlow": 2295, "Ġproper": 2296, "ĠìŀĪ": 2297, "Ġ!": 2298, "Ġrelations": 2299, "Ġmas": 2300, "Ġkids": 2301, "Ġentire": 2302, "ude": 2303, "Ùħ": 2304, "ĠWhere": 2305, "Ġones": 2306, "Ġcity": 2307, "olut": 2308, "Ġsix": 2309, "ability": 2310, "ör": 2311, "ili": 2312, "ĠEs": 2313, "Ġhappens": 2314, "ains": 2315, "Ġmodel": 2316, "Ġpict": 2317, "Ġespecially": 2318, "Ġ100": 2319, "kt": 2320, "Ġsoon": 2321, "by": 2322, "rodu": 2323, "Ġann": 2324, "Ġsubscri": 2325, "ĠQu": 2326, "Ġavail": 2327, "iment": 2328, "Ġvoc": 2329, "ka": 2330, "Ġ200": 2331, "aper": 2332, "ĠInd": 2333, "Ġì§": 2334, "hor": 2335, "į°": 2336, "jor": 2337, "ил": 2338, "Ġsqu": 2339, "AU": 2340, "arning": 2341, "Ġг": 2342, "IS": 2343, "Ġл": 2344, "ей": 2345, "yes": 2346, "åħ": 2347, "ĠÐĴ": 2348, "Ġorig": 2349, "ого": 2350, "Ġasked": 2351, "ilt": 2352, "ог": 2353, "Ġcontinue": 2354, "Ġìĺ": 2355, "ram": 2356, "Ġothers": 2357, "ES": 2358, "ohn": 2359, "Ġlay": 2360, "Ġbased": 2361, "Ġpu": 2362, "Ġappe": 2363, "Ġlim": 2364, "Ġprop": 2365, "Ģë": 2366, "min": 2367, "Ġhot": 2368, "ĠLa": 2369, "Ġfast": 2370, "Ġprotect": 2371, "Ġamount": 2372, "Ġaqu": 2373, "Ġfund": 2374, "Ġcustom": 2375, "Ġcult": 2376, "Ġhands": 2377, "Ġhaven": 2378, "Ġaud": 2379, "Ġoutside": 2380, "ĠAfter": 2381, "aps": 2382, "Ġanim": 2383, "ploy": 2384, "Ġhat": 2385, "ĠFirst": 2386, "Ġtreat": 2387, "Ġep": 2388, "Ġmater": 2389, "Ġbuilding": 2390, "Ġë°": 2391, "åIJ": 2392, "ìĦľ": 2393, "za": 2394, "ughter": 2395, "ĠPe": 2396, "ney": 2397, "eter": 2398, "atic": 2399, "Ġeduc": 2400, "기": 2401, "Ġmov": 2402, "ĵ¤": 2403, "ama": 2404, "ration": 2405, "Ġsn": 2406, "ÙĪ": 2407, "Ġsum": 2408, "Ġphot": 2409, "ĠÐĿ": 2410, "Ġ.": 2411, "æľī": 2412, "Ġfinish": 2413, "itting": 2414, "å®": 2415, "Ġlarge": 2416, "Ġìĸ": 2417, "Ġwhite": 2418, "ara": 2419, "Ġmais": 2420, "ĠHi": 2421, "Ġdam": 2422, "ĠاÙĦ": 2423, "Ġbox": 2424, "ĠHello": 2425, "Ġsle": 2426, "Ġopt": 2427, "ried": 2428, "¥¼": 2429, "Ġactiv": 2430, "Ġnão": 2431, "ĠCom": 2432, "Ġplaying": 2433, "Th": 2434, "Ġavailable": 2435, "Ġport": 2436, "åĪ": 2437, "ĠAh": 2438, "Ġlas": 2439, "Ġearly": 2440, "Ġwonder": 2441, "±°": 2442, "Ġ18": 2443, "cul": 2444, "Ġfunction": 2445, "Ġmorning": 2446, "lle": 2447, "ients": 2448, "ux": 2449, "Ġcir": 2450, "itions": 2451, "Ġdeep": 2452, "Ġpolit": 2453, "yor": 2454, "mp": 2455, "aking": 2456, "Įë": 2457, "ĠMan": 2458, "Ġmillion": 2459, "Ġ/": 2460, "Ġindivid": 2461, "Ġpan": 2462, "Ġgovernment": 2463, "Ġwrite": 2464, "ĠTod": 2465, "ament": 2466, "ĠÏ": 2467, "Ġwind": 2468, "ĠEng": 2469, "chen": 2470, "Wh": 2471, "ìľ": 2472, "Ġident": 2473, "ãģ§": 2474, "vent": 2475, "urch": 2476, "Ġhy": 2477, "Ġya": 2478, "Ġtrad": 2479, "Ġrelationship": 2480, "ú": 2481, "Ġdou": 2482, "OR": 2483, "Ġswe": 2484, "Ġneg": 2485, "ination": 2486, "Ġtext": 2487, "ipp": 2488, "Ġfine": 2489, "ás": 2490, "ĠDr": 2491, "ĠCome": 2492, "Ġmonths": 2493, ",\"": 2494, "ени": 2495, "Ġhours": 2496, "Ġpod": 2497, "irt": 2498, "Ġinvol": 2499, "Ġcollect": 2500, "Ġauf": 2501, "Ġpa": 2502, "Ġhistory": 2503, "mb": 2504, "ify": 2505, "Ġ?": 2506, "Ġbelow": 2507, "asure": 2508, "aby": 2509, "Ġlangu": 2510, "Ġant": 2511, "Ġcomb": 2512, "ato": 2513, "Ġexist": 2514, "Ġëĭ": 2515, "Ġtakes": 2516, "Ġcharacter": 2517, "aff": 2518, "Ġfield": 2519, "Ġeconom": 2520, "ief": 2521, "Ġpiece": 2522, "åľ": 2523, "Ġreach": 2524, "Ġê²": 2525, "ony": 2526, "Ġmaterial": 2527, "Ġdig": 2528, "Ġphys": 2529, "Ġimpro": 2530, "Ġsimilar": 2531, "IC": 2532, "Ġnet": 2533, "yn": 2534, "Ġposition": 2535, "ÃŁ": 2536, "Ġbene": 2537, "read": 2538, "Ġlearning": 2539, "ume": 2540, "Ġclean": 2541, "ÑĤоÑĢ": 2542, "Ġcook": 2543, "Ġseems": 2544, "Ġol": 2545, "ĠUS": 2546, "ĠJes": 2547, "Ġà®": 2548, "ential": 2549, "iversity": 2550, "acy": 2551, "ĠÑı": 2552, "olutely": 2553, "rect": 2554, "ĠPlease": 2555, "Ġrepres": 2556, "Ġtouch": 2557, "men": 2558, "Ġа": 2559, "ión": 2560, "ĠThanks": 2561, "Ġang": 2562, "Ġmajor": 2563, "Ġitself": 2564, "ills": 2565, "\",": 2566, "ians": 2567, "Ġscreen": 2568, "Ġhor": 2569, "Ġknown": 2570, "Ġenviron": 2571, "Ġfinal": 2572, "Ġfigure": 2573, "ĠTw": 2574, "Ġeyes": 2575, "Ġimag": 2576, "Ġseeing": 2577, "Ġhair": 2578, "rem": 2579, "Ġapplic": 2580, "ends": 2581, "put": 2582, "Ġnews": 2583, "Ġcompletely": 2584, "ughs": 2585, "Ġknew": 2586, "ified": 2587, "ĠJe": 2588, "ĠDid": 2589, "Ġsituation": 2590, "Ġflo": 2591, "ms": 2592, "Ġphone": 2593, "Ġball": 2594, "do": 2595, "Ġparent": 2596, "Ġsorry": 2597, "ury": 2598, "ин": 2599, "ips": 2600, "ад": 2601, "Ġinstead": 2602, "Ġhuge": 2603, "Ġtu": 2604, "Ġãģ": 2605, "ĠGr": 2606, "Ġdetail": 2607, "ĠÐŁ": 2608, "Ġindividual": 2609, "Ġfire": 2610, "Ġclos": 2611, "Ġwer": 2612, "une": 2613, "Ġrunning": 2614, "Ġconvers": 2615, "Ġrecomm": 2616, "Ġcomo": 2617, "Ġsomebody": 2618, "ĠJohn": 2619, "ĠìĿ´": 2620, "ĠOur": 2621, "ples": 2622, "ĠPh": 2623, "Ġanal": 2624, "Ġ50": 2625, "Ġoffer": 2626, "Ġ<": 2627, "itional": 2628, "gest": 2629, "Ġvous": 2630, "let": 2631, "icy": 2632, "Ġfeeling": 2633, "LE": 2634, "ros": 2635, "Ġthird": 2636, "ок": 2637, "Ġseries": 2638, "ĠAny": 2639, "ised": 2640, "old": 2641, "Ġdraw": 2642, "Ġservice": 2643, "Ġcannot": 2644, "bal": 2645, "ãģĨ": 2646, "Ġliving": 2647, "ım": 2648, "Ġdifference": 2649, "Ġopportunity": 2650, "Ġnear": 2651, "orth": 2652, "ken": 2653, "Ġlocal": 2654, "ت": 2655, "ĠCon": 2656, "Ġobject": 2657, "Ġdass": 2658, "ãģĻ": 2659, "IJ×": 2660, "Ġquickly": 2661, "raph": 2662, "Ġissues": 2663, "éĢĻ": 2664, "ĠAmerican": 2665, "Ġprep": 2666, "ences": 2667, "Ġprofess": 2668, "lling": 2669, "of": 2670, "Ġfoot": 2671, "bre": 2672, "Ġusually": 2673, "Ġgeneral": 2674, "da": 2675, "ances": 2676, "Ġdest": 2677, "Ġocc": 2678, "Ġmembers": 2679, "Ġdans": 2680, "Ġequal": 2681, "zt": 2682, "Ġbecom": 2683, "Ġmoving": 2684, "Ġspecific": 2685, "ÃŃa": 2686, "Ġfur": 2687, "Ġnecess": 2688, "Ġcommon": 2689, "Ġattack": 2690, "ĠÑįÑĤо": 2691, "ĠToday": 2692, "Ġuns": 2693, "ĠGu": 2694, "iod": 2695, "Ġaccount": 2696, "Ġgrand": 2697, "Ġself": 2698, "ĠEl": 2699, "Ġtast": 2700, "Ġcontent": 2701, "Ġcu": 2702, "Ħë": 2703, "ĠMaybe": 2704, "ĠJesus": 2705, "ores": 2706, "port": 2707, "©´": 2708, "Ġgives": 2709, "Ġnormal": 2710, "ÑĢÑĥ": 2711, "Ġimpact": 2712, "är": 2713, "Ġdies": 2714, "Ġlab": 2715, "sh": 2716, "ios": 2717, "ĠPres": 2718, "ĠUnd": 2719, "ĠOf": 2720, "Ġfinally": 2721, "Ġdoll": 2722, "Ġvocê": 2723, "ply": 2724, "ĠAg": 2725, "Ġtaken": 2726, "Ġground": 2727, "fort": 2728, "Ġgave": 2729, "ĠInst": 2730, "Ġlost": 2731, "Ġworked": 2732, "Ġliter": 2733, "Ġissue": 2734, "Ġindust": 2735, "Ġreturn": 2736, "Ġhappening": 2737, "Ġwants": 2738, "ив": 2739, "Ġproblems": 2740, "ĠCar": 2741, "Ŀ¼": 2742, "ĠAlso": 2743, "Ġsize": 2744, "Ġobviously": 2745, "ĠSu": 2746, "ĠSc": 2747, "Ġrecommend": 2748, "ources": 2749, "astic": 2750, "....": 2751, "Ġmi": 2752, "lier": 2753, "ĠEven": 2754, "cia": 2755, "Ġhur": 2756, "va": 2757, "Ġmass": 2758, "Ġwouldn": 2759, "unt": 2760, "cks": 2761, "Ġfelt": 2762, "osp": 2763, "light": 2764, "олÑĮ": 2765, "nie": 2766, "Ġbottom": 2767, "ĠбÑĭ": 2768, "ored": 2769, "ison": 2770, "Ġgrad": 2771, "Ġuma": 2772, "Ġva": 2773, "ĠìĤ": 2774, "ression": 2775, "ulation": 2776, "ID": 2777, "idence": 2778, "Ġbur": 2779, "Ġgone": 2780, "lu": 2781, "ìĸ´ì": 2782, "Ġredu": 2783, "Ġja": 2784, "ìĿĺ": 2785, "ita": 2786, "Ġsoft": 2787, "Ġça": 2788, "ico": 2789, "eral": 2790, "ñ": 2791, "af": 2792, "Ġpoints": 2793, "gu": 2794, "Ġdé": 2795, "apt": 2796, "ax": 2797, "ĠAlright": 2798, "Ġcamera": 2799, "Ġach": 2800, "Ġпо": 2801, "Ġsever": 2802, "50": 2803, "Ġsie": 2804, "Ïģ": 2805, "Ġmal": 2806, "Ġcomput": 2807, "Ġmiddle": 2808, "Ġcouldn": 2809, "ming": 2810, "Ġìĭ": 2811, "ĠHis": 2812, "Ġgames": 2813, "Ġintrodu": 2814, "Ġcell": 2815, "por": 2816, "Ġsleep": 2817, "Ġë³": 2818, "iding": 2819, "Ġou": 2820, "Ġdeg": 2821, "Ġdrink": 2822, "Ġenvironment": 2823, "ĠUnited": 2824, "Ġtalked": 2825, "Ġchoose": 2826, "Ġjour": 2827, "ege": 2828, "ĠMin": 2829, "Ġinte": 2830, "Ġrather": 2831, "Ġoffic": 2832, "ка": 2833, "aching": 2834, "Ġmentioned": 2835, "Ġfill": 2836, "Ġtrack": 2837, "Ġnie": 2838, "Ġut": 2839, "ĠвÑĭ": 2840, "ibility": 2841, "Ġvac": 2842, "Ġrad": 2843, "Ġpack": 2844, "Ġsend": 2845, "ĠDas": 2846, "ĠAb": 2847, "Ġengine": 2848, "ãģĹ": 2849, "Ġcompet": 2850, "ô": 2851, "ĠвÑģ": 2852, "Ġdoor": 2853, "Ġlonger": 2854, "å°į": 2855, "Ġlanguage": 2856, "Ġextra": 2857, "play": 2858, "Ġwebs": 2859, "umb": 2860, "room": 2861, "çľ": 2862, "Ġbeginning": 2863, "Ġrefer": 2864, "AM": 2865, "nen": 2866, "igher": 2867, "face": 2868, "erc": 2869, "Ġforget": 2870, "Ġcomment": 2871, "ек": 2872, "лÑı": 2873, "ror": 2874, "że": 2875, "ĠGe": 2876, "Ġdark": 2877, "Ġanyone": 2878, "ante": 2879, "ges": 2880, "ìĬµ": 2881, "Ñij": 2882, "bed": 2883, "je": 2884, "ructure": 2885, "Ġprim": 2886, "ida": 2887, "è¦": 2888, "ãģ¾": 2889, "Ġmix": 2890, "Ġstarting": 2891, "ĠìĿ´ë": 2892, "Ġprovide": 2893, "action": 2894, "Ġmother": 2895, "Ġperiod": 2896, "Ġstick": 2897, "ĠYouT": 2898, "Ġtechnology": 2899, "ê¹": 2900, "Ġbed": 2901, "Ġgiving": 2902, "Ġexplain": 2903, "zen": 2904, "imate": 2905, "Ġrepresent": 2906, "load": 2907, "ĠHowever": 2908, "Ġlives": 2909, "uth": 2910, "irit": 2911, "ogn": 2912, "Ġlik": 2913, "Ġrespons": 2914, "Ġpriv": 2915, "Ġtom": 2916, "ção": 2917, "iam": 2918, "Ġexcited": 2919, "Ġcard": 2920, "ground": 2921, "Ġ×Ķ": 2922, "Ġsens": 2923, "Ġteach": 2924, "ido": 2925, "hod": 2926, "Ġepis": 2927, "Ġwelcome": 2928, "Ġwall": 2929, "ä¹": 2930, "Ġchance": 2931, "hen": 2932, "ĠС": 2933, "ĠÄij": 2934, "Ġsimply": 2935, "ĠÑĤак": 2936, "ring": 2937, "ja": 2938, "book": 2939, "Ġseveral": 2940, "ste": 2941, "Ġcreated": 2942, "ĠоÑĤ": 2943, "Ġpush": 2944, "==": 2945, "Ġhigher": 2946, "uf": 2947, "ource": 2948, "oke": 2949, "Ġonline": 2950, "Ġrele": 2951, "Ġton": 2952, "ensive": 2953, "Ġfavorite": 2954, "Ñĥд": 2955, "Ġlooked": 2956, "Ġvon": 2957, "âĢĶ": 2958, "Ġfür": 2959, "Ġbutton": 2960, "Ġbill": 2961, "Ġchanges": 2962, "!\"": 2963, "Ġslow": 2964, "ables": 2965, "Ġdeath": 2966, "ands": 2967, "ateg": 2968, "Ġthemselves": 2969, "ãģ£": 2970, "Ġcop": 2971, "ãģ®": 2972, "Ġpersonal": 2973, "ughing": 2974, "Ġ11": 2975, "gar": 2976, "ades": 2977, "Ġneeded": 2978, "Ġstudy": 2979, "aged": 2980, "ÑģÑĤв": 2981, "ino": 2982, "Ġdisc": 2983, "ki": 2984, "Ġaddress": 2985, "ר": 2986, "itten": 2987, "esome": 2988, "Ġж": 2989, "¤ë": 2990, "ura": 2991, "Ġmu": 2992, "Ġcontinu": 2993, "for": 2994, "Ġmatch": 2995, "ãģ¦": 2996, "Ġstraight": 2997, "IJë": 2998, "ners": 2999, "Ġdog": 3000, "Ġdeb": 3001, "ĠCO": 3002, "Ġos": 3003, "ged": 3004, "came": 3005, "Ġcorrect": 3006, "ette": 3007, "ĠSee": 3008, "Ġincluding": 3009, "ĠEuro": 3010, "ester": 3011, "Ġjump": 3012, "ĠWhich": 3013, "Ġкак": 3014, "son": 3015, "ya": 3016, "ING": 3017, "Ġeine": 3018, "osh": 3019, "ency": 3020, "Ġmedia": 3021, "Ġsubscribe": 3022, "éĤ": 3023, "Ġprin": 3024, "Ġhab": 3025, "ĠPer": 3026, "ĠWas": 3027, "Ġpage": 3028, "itor": 3029, "Ġtowards": 3030, "Ġtried": 3031, "enge": 3032, "artment": 3033, "Ġvari": 3034, "Ġpaper": 3035, "Ġpicture": 3036, "Ġversion": 3037, "Ġbrought": 3038, "ware": 3039, "ĠStates": 3040, "Ġsich": 3041, "ledge": 3042, "Ġpercent": 3043, "Ġgod": 3044, "ec": 3045, "ĠComm": 3046, "Ġdecided": 3047, "Ġselect": 3048, "íķľ": 3049, ").": 3050, "urity": 3051, "Ġfurther": 3052, "Ġcomments": 3053, "lement": 3054, "Ġdream": 3055, "Ġcenter": 3056, "mi": 3057, "Ġcas": 3058, "Ġwoman": 3059, "Ġroad": 3060, "Ġfail": 3061, "Ġbecame": 3062, "lus": 3063, "ilities": 3064, "ãģ¯": 3065, "ĠCo": 3066, "Ġmanage": 3067, "Ġrecogn": 3068, "Ġaction": 3069, "Ġbenef": 3070, "Ġearlier": 3071, "׾": 3072, "Ġspeed": 3073, "Ġment": 3074, "Ġsoci": 3075, "Ġshoot": 3076, "ui": 3077, "Ġä": 3078, "Ġapply": 3079, "vo": 3080, "xim": 3081, "Ġcause": 3082, "Ġsurpr": 3083, "Ġhaben": 3084, "DI": 3085, "Ġfather": 3086, "ĠNext": 3087, "ĠYouTube": 3088, "Ġcode": 3089, "Ġrole": 3090, "gress": 3091, "Ġgreen": 3092, "ett": 3093, "Ġbuilt": 3094, "Ġflow": 3095, "Ġbase": 3096, "Ġtraining": 3097, "Ġround": 3098, "ĠWill": 3099, "Ġpath": 3100, "ĠRo": 3101, "Ġinterested": 3102, "ìĸ´": 3103, "Ġrespect": 3104, "Ġchanged": 3105, "ission": 3106, "Ġstudent": 3107, "ograph": 3108, "Ġapproach": 3109, "Ġshows": 3110, "å°±": 3111, "Ġtar": 3112, "Ġcrit": 3113, "Ġglo": 3114, "ìĬµëĭĪëĭ¤": 3115, "Ġdead": 3116, "ĠPresident": 3117, "Ġthous": 3118, "Ġbal": 3119, "ster": 3120, "ex": 3121, "Ġabsolutely": 3122, "Ġmic": 3123, "Ġpractice": 3124, "Ġquality": 3125, "Ġlower": 3126, "ogle": 3127, "Ġsepar": 3128, "ball": 3129, "medi": 3130, "Ġreview": 3131, "ĠApp": 3132, "Ġok": 3133, "âĢĭ": 3134, "Ġexperien": 3135, "Ġconcern": 3136, "entially": 3137, "more": 3138, "ĠJo": 3139, "apan": 3140, "ĠIch": 3141, "istic": 3142, "Ġfair": 3143, "Ġwebsite": 3144, "ires": 3145, "ĠBy": 3146, "Ġtravel": 3147, "Ġrisk": 3148, "Ġmir": 3149, "Ġboard": 3150, "Ġsen": 3151, "Ġparents": 3152, "ĠWow": 3153, "Ġfeed": 3154, "Ġsave": 3155, "Ġserious": 3156, "Ġinit": 3157, "EL": 3158, "undred": 3159, "AS": 3160, "Ġvan": 3161, "orrow": 3162, "Ġworth": 3163, "Ġsearch": 3164, "Ġ16": 3165, "Ġparts": 3166, "ÑģÑĤÑĮ": 3167, "Ġcompan": 3168, "Ġmovie": 3169, "Ġmethod": 3170, "Ġill": 3171, "Ġwish": 3172, "dy": 3173, "Ġitem": 3174, "Ġminus": 3175, "anger": 3176, "Ġvoice": 3177, "Ġskin": 3178, "Ġareas": 3179, "Ġeight": 3180, "Ġobs": 3181, "Ġ,": 3182, "ай": 3183, "Ġoil": 3184, "Ġcy": 3185, "Ġbaby": 3186, "sy": 3187, "Ġemploy": 3188, "ĠKe": 3189, "Ġplaces": 3190, "Ġfix": 3191, "Ġestá": 3192, "ãģ¨": 3193, "ived": 3194, "Ġlots": 3195, "Ġseason": 3196, "unk": 3197, "alt": 3198, "Ġtable": 3199, "ĠТ": 3200, "â": 3201, "Ġattention": 3202, "ãģª": 3203, "ĠHer": 3204, "Ġage": 3205, "Ġpra": 3206, "back": 3207, "cil": 3208, "Ġnetwork": 3209, "rit": 3210, "Ġdoc": 3211, "Ġaren": 3212, "igen": 3213, "ĠëĦ": 3214, "د": 3215, "ender": 3216, "Ġtotal": 3217, "Ġprice": 3218, "Ġcrazy": 3219, "ìļ": 3220, "iqu": 3221, "though": 3222, "You": 3223, "Ùĩ": 3224, "ãĤĵ": 3225, "Ïħ": 3226, "Ġsat": 3227, "Ġbi": 3228, "ĠDie": 3229, "Ġsha": 3230, "Ġthanks": 3231, "uh": 3232, "Ġstage": 3233, "аж": 3234, "ĠFl": 3235, "Ġleav": 3236, "Ġboy": 3237, "Ġaf": 3238, "ön": 3239, "ĠGet": 3240, "Ġaccept": 3241, "Ġenter": 3242, "Ġtur": 3243, "ĠsiÄĻ": 3244, "Ġhonest": 3245, "ãĢĮ": 3246, "Ġsam": 3247, "Ġrepl": 3248, "ging": 3249, "Ġdevelopment": 3250, "ĠAct": 3251, "ora": 3252, "ãĢį": 3253, "ä¾": 3254, "Ġknows": 3255, "Ġimage": 3256, "ĠLord": 3257, "иÑĤÑĮ": 3258, "Ġweeks": 3259, "Ġsex": 3260, "Ķë": 3261, "Ġhundred": 3262, "Ġsounds": 3263, "Ġlearned": 3264, "Ġbud": 3265, "ĠÑģÑĤ": 3266, "Ġincred": 3267, "âĻ": 3268, "Ġnos": 3269, "Ġdrop": 3270, "Ġben": 3271, "ĠÐĺ": 3272, "Ġsafe": 3273, "ata": 3274, "Ġfuck": 3275, "soci": 3276, "Ġdan": 3277, "Ġcross": 3278, "10": 3279, "mo": 3280, "vert": 3281, "Ġ17": 3282, "zie": 3283, "åķ": 3284, "Ġdom": 3285, "ĠBo": 3286, "Ġsetting": 3287, "Ġinvolved": 3288, "arily": 3289, "Ġsind": 3290, "Ġsus": 3291, "Ġworry": 3292, "eth": 3293, "ê¹Į": 3294, "Ġsun": 3295, "Ġhier": 3296, "Ġcertainly": 3297, "oul": 3298, "orts": 3299, "ĠEr": 3300, "ĠUm": 3301, "Ġcaus": 3302, "Ġnatural": 3303, "Ġü": 3304, "Ġcry": 3305, "ĠSec": 3306, "Ġsom": 3307, "æ²": 3308, "Ġeducation": 3309, "аеÑĤ": 3310, "Ġmultip": 3311, "Ġalone": 3312, "Ġeye": 3313, "Ġrate": 3314, "ĠEurope": 3315, "è¿": 3316, "mon": 3317, "Ġfit": 3318, "izing": 3319, "pped": 3320, "Ġpressure": 3321, "the": 3322, "иÑģ": 3323, "ites": 3324, "ĠAf": 3325, "reci": 3326, "attle": 3327, "Ġservices": 3328, "ĠGoogle": 3329, "éģ": 3330, "Ġcases": 3331, "Ġdrive": 3332, "Ġchalleng": 3333, "uz": 3334, "ĠMo": 3335, "ìľ¼ë": 3336, "val": 3337, "åĢĭ": 3338, "Ġfol": 3339, "Ġì¢": 3340, "ffic": 3341, "Ġra": 3342, "Ġsin": 3343, "Ġblue": 3344, "Ġaffect": 3345, "Ġmis": 3346, "Ġshot": 3347, "Ġоб": 3348, "asing": 3349, "Ġsignific": 3350, "ĠChe": 3351, "Ġê³": 3352, "Ġpositive": 3353, "ì£": 3354, "Ġwie": 3355, "Ġ40": 3356, "ording": 3357, "ĠFrom": 3358, "êµ": 3359, "Ġbrand": 3360, "Ġtrust": 3361, "Ġple": 3362, "Ġcommunic": 3363, "Ġweight": 3364, "Ġasking": 3365, "Ġtax": 3366, "ĠJapan": 3367, "ãģŁ": 3368, "Ġíķĺ": 3369, "ops": 3370, "ÏĤ": 3371, "Ġputting": 3372, "Ġroll": 3373, "ĠAmerica": 3374, "reg": 3375, "ŀ×": 3376, "atures": 3377, "ension": 3378, "ĠSomet": 3379, "Ġoriginal": 3380, "ping": 3381, "ĠÅŁ": 3382, "Ġproducts": 3383, "ãĥ¼": 3384, "Ġcontact": 3385, "olution": 3386, "Ġgoal": 3387, "Ġpow": 3388, "Ġperformance": 3389, "Ġblood": 3390, "ators": 3391, "ĠMich": 3392, "Ġtemper": 3393, "ĠDan": 3394, "Ġsugg": 3395, "ÑĤи": 3396, "Ġimm": 3397, "Ġoffice": 3398, "Ġarri": 3399, "Ġcomfort": 3400, "ĠÐĶ": 3401, "Ġsuggest": 3402, "Ġplat": 3403, "Ĥĺ": 3404, "19": 3405, "Ġom": 3406, "Ġseven": 3407, "ĠCent": 3408, "ille": 3409, "Ġconcept": 3410, "Ġbag": 3411, "ün": 3412, "ively": 3413, "Ġdiv": 3414, "mos": 3415, "æī": 3416, "Ġfeels": 3417, "Ġir": 3418, "akes": 3419, "ley": 3420, "Ġparticip": 3421, "ĠÐļ": 3422, "fl": 3423, "just": 3424, "Ġsil": 3425, "ĠPa": 3426, "AL": 3427, "Ġgotta": 3428, "Ġfan": 3429, "Ġchallenge": 3430, "Ġcompanies": 3431, "ĠPeople": 3432, "</": 3433, "оз": 3434, "Ġpen": 3435, "ising": 3436, "Ġaus": 3437, "emic": 3438, "amente": 3439, "Ġmeeting": 3440, "Ġvisit": 3441, "Ġsupposed": 3442, "ĠOnce": 3443, "да": 3444, "orld": 3445, "30": 3446, "US": 3447, "Ġviol": 3448, "Ġnotice": 3449, "ĠÐIJ": 3450, "han": 3451, "ped": 3452, "ìĺ": 3453, "hh": 3454, "Ġtrou": 3455, "Ġminute": 3456, "ĠPar": 3457, "ray": 3458, "Ġtit": 3459, "Ġupd": 3460, "Ġblock": 3461, "Ġdue": 3462, "aur": 3463, "Ġforce": 3464, "Ġcoun": 3465, "ĠâĢĶ": 3466, "Ġtypes": 3467, "ë§": 3468, "Ġlate": 3469, "Ġimprove": 3470, "ĠìĪ": 3471, "Ġave": 3472, "ules": 3473, "cl": 3474, "amed": 3475, "Ġawesome": 3476, "ĠOk": 3477, "Ġvot": 3478, "Ġmachine": 3479, "Ġfollowing": 3480, "Ġmeasure": 3481, "ación": 3482, "uel": 3483, "chan": 3484, "Ġability": 3485, "Ġtout": 3486, "Ġideas": 3487, "Ġincrease": 3488, "Ġens": 3489, "ĠÑħ": 3490, "Ġëª": 3491, "Ġjest": 3492, "ĠÐľ": 3493, "Ġtruth": 3494, "hy": 3495, "Ġspend": 3496, "Ġscience": 3497, "ete": 3498, "Ġ14": 3499, "Ġepisode": 3500, "Ġalg": 3501, "ended": 3502, "ãģĵ": 3503, "ari": 3504, "lla": 3505, "Ġfish": 3506, "Ġthrow": 3507, "mit": 3508, "å¹": 3509, "Ġcirc": 3510, "ĠCal": 3511, "Ġtour": 3512, "Ġdirection": 3513, "Ġnoch": 3514, "ев": 3515, "én": 3516, "Ġcountries": 3517, "Ġindustry": 3518, "iny": 3519, "icle": 3520, "Ġfeet": 3521, "It": 3522, "Ġleaders": 3523, "etzt": 3524, "Ġstaff": 3525, "çĶ": 3526, "Ġpurp": 3527, "ito": 3528, "?!": 3529, "ĠJa": 3530, "Ġstore": 3531, "etic": 3532, "ĠChina": 3533, "ĠëIJ": 3534, "ĠUniversity": 3535, "Ġ#": 3536, "Ġdecision": 3537, "Ġachie": 3538, "Ġactual": 3539, "uly": 3540, "Ġsection": 3541, "Ġresults": 3542, "Ġstar": 3543, "Ġmist": 3544, "ibly": 3545, "Ġdad": 3546, "Ġnumbers": 3547, "omb": 3548, "èª": 3549, "ĠSpe": 3550, "Ġmer": 3551, "Ġ25": 3552, "Ġautom": 3553, "Ġcold": 3554, "ب": 3555, "Ħľ": 3556, "ager": 3557, "ĠTV": 3558, "ĠSie": 3559, "ĠHave": 3560, "Ġże": 3561, "ugg": 3562, "ained": 3563, "Ġupon": 3564, "Ġlog": 3565, "Ġcomplete": 3566, "Ġbrain": 3567, "aging": 3568, "ĠMus": 3569, "over": 3570, "Ġeasier": 3571, "Ġintegr": 3572, "Ġmás": 3573, "Ġturned": 3574, "Ġstri": 3575, "ival": 3576, "Ġheav": 3577, "ĠTH": 3578, "Ġwriting": 3579, "ÑĢа": 3580, "åľ¨": 3581, "大": 3582, "Ġcla": 3583, "ding": 3584, "Ġtelling": 3585, "ид": 3586, "icated": 3587, "以": 3588, "acht": 3589, "ãģĤ": 3590, "haps": 3591, "ĠSte": 3592, "Ġresources": 3593, "Ġdann": 3594, "Ġparty": 3595, "ĠÏĦ": 3596, "Ġsaf": 3597, "ises": 3598, "tre": 3599, "oint": 3600, "Ġknowledge": 3601, "Ġanymore": 3602, "Ġfly": 3603, "Ġmaint": 3604, "ик": 3605, "åij": 3606, "Ġsell": 3607, "laughs": 3608, "ĠYork": 3609, "Ġbien": 3610, "Ġod": 3611, "Ġeasily": 3612, "Ġrange": 3613, "Ġoption": 3614, "ع": 3615, "Ġappreci": 3616, "ocr": 3617, "Ġdeterm": 3618, "ÑĦ": 3619, "Ġmeaning": 3620, "Ġsite": 3621, "Ġdisco": 3622, "verage": 3623, "Ġlose": 3624, "Ġinstall": 3625, "Ġemot": 3626, "antly": 3627, "ät": 3628, "Ġtamb": 3629, "ĠWar": 3630, "ĠHo": 3631, "ĠGen": 3632, "emy": 3633, "ез": 3634, "ĠPol": 3635, "Ġmessage": 3636, "Ġnote": 3637, "ĮĢ": 3638, "Ġhet": 3639, "Ġimmedi": 3640, "Ġavo": 3641, "Ġbooks": 3642, "Ġbecomes": 3643, "resh": 3644, "ès": 3645, "asons": 3646, "Ġhimself": 3647, "uts": 3648, "Ġju": 3649, "Ġaware": 3650, "Ġrequire": 3651, "Ġsystems": 3652, "ĠHar": 3653, "Ġamong": 3654, "Ġhom": 3655, "Ġbreat": 3656, "Ġweird": 3657, "Ġë¶": 3658, "λ": 3659, "Ø©": 3660, "iff": 3661, "oring": 3662, "Ġplatform": 3663, "ĠTake": 3664, "Ġhelps": 3665, "utions": 3666, "Ġforg": 3667, "Ġluck": 3668, "ĠEnglish": 3669, "Ġweb": 3670, "Ġnegative": 3671, "Ġtut": 3672, "Ġabove": 3673, "ngth": 3674, "Ġê±°": 3675, "Ġstories": 3676, "Ġload": 3677, "Ġbackground": 3678, "Ġswitch": 3679, "ga": 3680, "Ġprinci": 3681, "Ġfinan": 3682, "Ġvarious": 3683, "ĠlÃł": 3684, "Ġkinds": 3685, "aining": 3686, "Ġnature": 3687, "ĠÐŀ": 3688, "cz": 3689, "Ġpray": 3690, "Ġgar": 3691, "irm": 3692, "Ġ&": 3693, "Ġìĥ": 3694, "ns": 3695, "ĠRep": 3696, "ĠFe": 3697, "Ġrev": 3698, "rand": 3699, "Ġlikely": 3700, "Ġunderstanding": 3701, "ır": 3702, "ãģĭ": 3703, "Ġfal": 3704, "Ġ13": 3705, "ÑĨи": 3706, "Ġsud": 3707, "Ġbrother": 3708, "Ġplant": 3709, "Ġthroughout": 3710, "wise": 3711, "pre": 3712, "Ġculture": 3713, "ĠÙħ": 3714, "Ġwonderful": 3715, "Ġah": 3716, "pper": 3717, "Ġsold": 3718, "Ġstarts": 3719, "Ġwritten": 3720, "ί": 3721, "ni": 3722, "Ġ×Ķ×": 3723, "ĠDav": 3724, "Ġult": 3725, "Ġarm": 3726, "Ġrock": 3727, "Ġwear": 3728, "ëį°": 3729, "ano": 3730, "rag": 3731, "Ġsquare": 3732, "ани": 3733, "cast": 3734, "lebr": 3735, "Ġliterally": 3736, "Ġplayed": 3737, "Ġheat": 3738, "onse": 3739, "rict": 3740, "Ġinsp": 3741, "ids": 3742, "Ġpopular": 3743, "ëıĦ": 3744, "Ġcatch": 3745, "Ġmount": 3746, "Ġjud": 3747, "What": 3748, "еб": 3749, "RA": 3750, "aud": 3751, "ко": 3752, "Ġsurface": 3753, "Ġconv": 3754, "Ġpieces": 3755, "Oh": 3756, "æĢ": 3757, "Ġstyle": 3758, "pping": 3759, "Ġreading": 3760, "Ġconversation": 3761, "оп": 3762, "ä¾Ĩ": 3763, "ĠAgain": 3764, "Ġbank": 3765, "time": 3766, "ÑĥÑĤ": 3767, "erve": 3768, "ĠGreat": 3769, "Ġcapt": 3770, "аб": 3771, "ays": 3772, "ĠFin": 3773, "ification": 3774, "Ġär": 3775, "аÑİ": 3776, "Ġegg": 3777, "ĠWel": 3778, "Ġtarget": 3779, "ula": 3780, "ches": 3781, "ani": 3782, "OO": 3783, "icious": 3784, "now": 3785, "Ïĥ": 3786, "board": 3787, "Ġgente": 3788, "Ġdro": 3789, "ĠEt": 3790, "Ġdin": 3791, "Ġcos": 3792, "Ġauthor": 3793, "س": 3794, "Ġoch": 3795, "Ġemail": 3796, "Ġspirit": 3797, "Ġsitting": 3798, "mas": 3799, "Ġstrength": 3800, "Ġbigger": 3801, "ĠWait": 3802, "Ġmat": 3803, "Ġpolice": 3804, "ressed": 3805, "Ġwaiting": 3806, "ishing": 3807, "Ġdollars": 3808, "hood": 3809, "ss": 3810, "Ġimagine": 3811, "ini": 3812, "Ġmes": 3813, "Ġdise": 3814, "idge": 3815, "abor": 3816, "Ġpet": 3817, "Ġhop": 3818, "ĠKing": 3819, "Ġcomputer": 3820, "Ġgold": 3821, "Ġnu": 3822, "Ġfing": 3823, "),": 3824, "Ġsecurity": 3825, "ruction": 3826, "Ġsolution": 3827, "ext": 3828, "Ġpatter": 3829, "icken": 3830, "ured": 3831, "Ġstandard": 3832, "ìĭľ": 3833, "Ġdouble": 3834, "η": 3835, "Ġwife": 3836, "isa": 3837, "Ġdirectly": 3838, "aced": 3839, "Ġbunch": 3840, "Ġ¿": 3841, "алÑĮ": 3842, "Ġregard": 3843, "Ġsweet": 3844, "Ġunique": 3845, "ĠâĻ«": 3846, "Ġtrain": 3847, "ĠGerm": 3848, "ά": 3849, "RE": 3850, "Ġbehav": 3851, "Ġpred": 3852, "ìĥ": 3853, "set": 3854, "Ġdescription": 3855, "ée": 3856, "Ġcat": 3857, "åĵ": 3858, "Ġcollege": 3859, "ìĽ": 3860, "Ġapplication": 3861, "ĠSen": 3862, "ask": 3863, "Ġcred": 3864, "ublic": 3865, "Ġmultiple": 3866, "Ġni": 3867, "Ġpresident": 3868, "Ġadded": 3869, "Ġrob": 3870, "Ġaqui": 3871, "Ġhosp": 3872, "Ġtools": 3873, "Ġgun": 3874, "Ġbasic": 3875, "Ġlines": 3876, "Ġstructure": 3877, "ĠRuss": 3878, "Ġtotally": 3879, "Ġbiggest": 3880, "Ġeen": 3881, "Ġarg": 3882, "Ġ׾": 3883, "Ġpark": 3884, "ĠDes": 3885, "Ġcelebr": 3886, "Ġfait": 3887, "енÑĮ": 3888, "Ġsuff": 3889, "Ġregular": 3890, "¨ë": 3891, "Ġmine": 3892, "ĠKore": 3893, "Ġprevious": 3894, "Ġpi": 3895, "Ġseg": 3896, "Ġpolicy": 3897, "Ġко": 3898, "ĠTrump": 3899, "Ġvacc": 3900, "ów": 3901, "ĠSy": 3902, "иÑĩ": 3903, "itter": 3904, "Ġpolitical": 3905, "ras": 3906, "Ġals": 3907, "елÑĮ": 3908, "Ġshape": 3909, "anz": 3910, "Ġonto": 3911, "Ġarch": 3912, "Ġamb": 3913, "agram": 3914, "ĠSm": 3915, "ctions": 3916, "Ġjoin": 3917, "bor": 3918, "åĽ": 3919, "Ġframe": 3920, "łĩ": 3921, "Ġchoice": 3922, "à¯ģ": 3923, "ÑĥÑİ": 3924, "ĠCor": 3925, "ĠSw": 3926, "IT": 3927, "Ġtend": 3928, "ĠEar": 3929, "Ġtor": 3930, "Ġevents": 3931, "Ġclaim": 3932, "ĠDa": 3933, "ĠMark": 3934, "Ġgroups": 3935, "Ġeating": 3936, "ĠWorld": 3937, "Ġrecently": 3938, "Ġtaste": 3939, "Ġsurv": 3940, "à¤": 3941, "Ġskills": 3942, "Ġиз": 3943, "itted": 3944, "Ġshop": 3945, "ìĿ´ì": 3946, "Ġestab": 3947, "ĠëĤĺ": 3948, "Ġseconds": 3949, "ĠThose": 3950, "ĠEnt": 3951, "ĠìĦ": 3952, "erson": 3953, "Ġtown": 3954, "Ġcand": 3955, "Ġoptions": 3956, "Ġing": 3957, "VID": 3958, "Ġencour": 3959, "Ġré": 3960, "âĻª": 3961, "Ġentre": 3962, "Ġmovement": 3963, "ĠBen": 3964, "Ġbirth": 3965, "Ġwhe": 3966, "Ġhang": 3967, "ĠEm": 3968, "ige": 3969, "roll": 3970, "Ġunf": 3971, "ìĤ": 3972, "Ġrid": 3973, "Ġspread": 3974, "Ġhost": 3975, "ald": 3976, "ĠEd": 3977, "Ġconsum": 3978, "UN": 3979, "Ġopin": 3980, "itar": 3981, "ĠMed": 3982, "Ġsubject": 3983, "Ġpal": 3984, "Ġcarry": 3985, "Ġagree": 3986, "ĠWhile": 3987, "Ġcareer": 3988, "Ġscient": 3989, "Ġsudden": 3990, "Ġfile": 3991, "zi": 3992, "Ġexcept": 3993, "éº": 3994, "Ġpotential": 3995, "ĠAnother": 3996, "Ġcomplex": 3997, "ĠSim": 3998, "endo": 3999, "Ġrais": 4000, "Ġphysical": 4001, "Ġdate": 4002, "aker": 4003, "ĠCol": 4004, "Ġpowerful": 4005, "Ġmember": 4006, "rap": 4007, "Ġspot": 4008, "Ġsource": 4009, "Ġfem": 4010, "ém": 4011, "Ġemp": 4012, "ji": 4013, "iety": 4014, "Ġinflu": 4015, "Ġdry": 4016, "Ġlock": 4017, "Ġzero": 4018, "ĠUh": 4019, "Ġrout": 4020, "Ġporque": 4021, "Ġ24": 4022, "Ġtal": 4023, "Ġfolks": 4024, "Ġlaunch": 4025, "Ġcompon": 4026, "ĠWelcome": 4027, "Ġkann": 4028, "än": 4029, "ĠÑįÑĤ": 4030, "ees": 4031, "ĠÙĪ": 4032, "Ġanyway": 4033, "Ġaudience": 4034, "人": 4035, "Ġslight": 4036, "ona": 4037, "Ġur": 4038, "Ġrelig": 4039, "Ġextrem": 4040, "ız": 4041, "ĠMa": 4042, "μ": 4043, "Ġö": 4044, "Ġallows": 4045, "Ġfat": 4046, "ĠFace": 4047, "Ġnational": 4048, "Ġinterview": 4049, "ĠMc": 4050, "ét": 4051, "Ġcute": 4052, "ela": 4053, "Ġsecret": 4054, "ĠWest": 4055, "ĠDep": 4056, "Ġexerc": 4057, "Ġhistor": 4058, "Ġprior": 4059, "Ġ60": 4060, "ava": 4061, "acher": 4062, "yond": 4063, "ĠHa": 4064, "Ġeste": 4065, "inary": 4066, "ĠNorth": 4067, "onst": 4068, "Ġsmart": 4069, "ams": 4070, "али": 4071, "Ġdar": 4072, "ered": 4073, "Ġfunny": 4074, "ĠOb": 4075, "ĠBlack": 4076, "Ġrelated": 4077, "ĠBu": 4078, "Ġsomewhere": 4079, "ĠRem": 4080, "nes": 4081, "mente": 4082, "ĠReally": 4083, "Ġcreating": 4084, "Ġfamil": 4085, "Ġsociety": 4086, "Ġgel": 4087, "Ġtransform": 4088, "Äĥ": 4089, "Ġinclude": 4090, "Ġhol": 4091, "like": 4092, "ko": 4093, "airs": 4094, "Ġпод": 4095, "Ġperspect": 4096, "Ġbes": 4097, "Ġparticularly": 4098, "Ġshowing": 4099, "ĠPart": 4100, "Ġqual": 4101, "lock": 4102, "Ġreality": 4103, "hold": 4104, "iction": 4105, "oon": 4106, "Ġvir": 4107, "ãģ«": 4108, "itary": 4109, "Ġdrug": 4110, "Ġfeature": 4111, "Ġreasons": 4112, "Ġש": 4113, "Ġwrote": 4114, "Ġfant": 4115, "Ġband": 4116, "Ùĥ": 4117, "ena": 4118, "key": 4119, "Ġearth": 4120, "dom": 4121, "Ġfeatures": 4122, "Ġfloor": 4123, "Ġspeaking": 4124, "Ġtip": 4125, "ĠAust": 4126, "Ġstock": 4127, "Ġchurch": 4128, "Ġrac": 4129, "ìľ¼ë¡ľ": 4130, "à¸Ļ": 4131, "ãĤĮ": 4132, "ky": 4133, "Ġresponse": 4134, "ÛĮ": 4135, "ulations": 4136, "Ġslide": 4137, "Ġgradu": 4138, "cious": 4139, "Ġmeant": 4140, "Ġ==": 4141, "Ġ×IJ×": 4142, "ãħ": 4143, "Ġkinda": 4144, "Ġscene": 4145, "Ġmuit": 4146, "Ġê°Ģ": 4147, "rast": 4148, "rest": 4149, "Ġplayers": 4150, "wa": 4151, "Ġbroad": 4152, "Ġtomorrow": 4153, "ocol": 4154, "ĠÑģв": 4155, "ĠBar": 4156, "ık": 4157, "Ġsea": 4158, "Ġremove": 4159, "Ġremind": 4160, "омÑĥ": 4161, "ĠSince": 4162, "Ġavec": 4163, "cell": 4164, "иÑħ": 4165, "Ġdocument": 4166, "Ġê·¸ëŁ": 4167, "Ġneigh": 4168, "beat": 4169, "ĠpÃ¥": 4170, "Ġaspect": 4171, "Ġded": 4172, "lished": 4173, "ils": 4174, "Ġourselves": 4175, "uce": 4176, "Ġhey": 4177, "ĠпÑĢо": 4178, "enty": 4179, "Ġassoci": 4180, "ados": 4181, "umber": 4182, "Ġ]": 4183, "éĤ£": 4184, "nov": 4185, "ĠìĻ": 4186, "ÑĥÑĩ": 4187, "Ġcondition": 4188, "ëĬĶëį°": 4189, "Ġvalues": 4190, "Ġscen": 4191, "minist": 4192, "Ġcast": 4193, "Ġgrowing": 4194, "Ġuser": 4195, "Ġrespond": 4196, "lim": 4197, "ér": 4198, "ym": 4199, "çľĭ": 4200, "oses": 4201, "sych": 4202, "ĠÑĢаз": 4203, "Ġappear": 4204, "Ġprogress": 4205, "ength": 4206, "Ġjak": 4207, "ĠDis": 4208, "Ġpatients": 4209, "ĠSer": 4210, "Ġgas": 4211, "ère": 4212, "ìĸ´ìļĶ": 4213, "Ġreci": 4214, "ìĿ¸": 4215, "Ġsca": 4216, "epend": 4217, "Ñģк": 4218, "ап": 4219, "Ġbatter": 4220, "Ġveh": 4221, "ðŁ": 4222, "Ġaccom": 4223, "Ġbeat": 4224, "Ġpaint": 4225, "Ġcontrib": 4226, "Ġsad": 4227, "ư": 4228, "ales": 4229, "Ġtree": 4230, "ba": 4231, "Ġborn": 4232, "iced": 4233, "à®ķ": 4234, "band": 4235, "Ġmechan": 4236, "ĠDet": 4237, "Ġcapital": 4238, "Ġdeliver": 4239, "Ġfear": 4240, "ŀĺ": 4241, "ĠSouth": 4242, "Ġbought": 4243, "Ġstress": 4244, "Ġvor": 4245, "??": 4246, "ih": 4247, "ìķ¼": 4248, "Ġera": 4249, "ìĿ´ë": 4250, "аÑı": 4251, "isions": 4252, "ivity": 4253, "Ġhelped": 4254, "Ġassist": 4255, "Ġplayer": 4256, "ran": 4257, "Ġimmediately": 4258, "Ġmoved": 4259, "cie": 4260, "ê±": 4261, "Ġannoun": 4262, "å¿": 4263, "ìŀIJ": 4264, "Ġproduction": 4265, "Ġsummer": 4266, "Ġtun": 4267, "Ġprograms": 4268, "GH": 4269, "aling": 4270, "ira": 4271, "eless": 4272, ".)": 4273, "Ġaverage": 4274, "è¦ģ": 4275, "Ġglass": 4276, "oman": 4277, "ifically": 4278, "Ġëĭ¤": 4279, "ĠCong": 4280, "ĠVer": 4281, "Ġtrick": 4282, "Ġbegan": 4283, "Ġvill": 4284, "ê±°": 4285, "how": 4286, "æŃ": 4287, "Ġtill": 4288, "Ġ90": 4289, "bert": 4290, "Ġê¸": 4291, "Ġtemperature": 4292, "ò": 4293, "à¹Ī": 4294, "Ġgraph": 4295, "Ġê·¸": 4296, "Ġrot": 4297, "Ġmob": 4298, "AY": 4299, "ael": 4300, "Ġrepe": 4301, "Ġdevice": 4302, "Ġ199": 4303, "Ġtele": 4304, "Ġkept": 4305, "pa": 4306, "æĸ": 4307, "verse": 4308, "Ġstream": 4309, "еÑĩ": 4310, "ession": 4311, "Ġstrugg": 4312, "zz": 4313, "Ġdegree": 4314, "Ġhelping": 4315, "Ġsmell": 4316, "Ġperhaps": 4317, "pro": 4318, "Ġcontext": 4319, "Ġik": 4320, "ĠпеÑĢ": 4321, "Ġcalcul": 4322, "麼": 4323, "bing": 4324, "Ġrealize": 4325, "lam": 4326, "ĠChar": 4327, "yt": 4328, "ĠìĿ´ì": 4329, "Ġdanger": 4330, "ĠIm": 4331, "aa": 4332, "Ġloved": 4333, "Ġpurpose": 4334, "Ġfinished": 4335, "Ġpeace": 4336, "Ġot": 4337, "Ġglobal": 4338, "ÏĢ": 4339, "Ġaber": 4340, "ĸĪ": 4341, "Ġcharacters": 4342, "Ġnur": 4343, "Ġdamage": 4344, "Ġemer": 4345, "Ġprec": 4346, "ĠWir": 4347, "Ġinstit": 4348, "ij×": 4349, "Ġallowed": 4350, "bon": 4351, "Ġtod": 4352, "его": 4353, "Ġjetzt": 4354, "Ġmedic": 4355, "Ġsmaller": 4356, "ceed": 4357, "Ġlevels": 4358, "Ġintell": 4359, "We": 4360, "Ġsem": 4361, "Ġcurrently": 4362, "Ġmodern": 4363, "Ġcontract": 4364, "Ġdetails": 4365, "ortunately": 4366, "OS": 4367, "Ġstates": 4368, "Ġadjust": 4369, "antage": 4370, "ez": 4371, "ĠVery": 4372, "Ġscale": 4373, "Ġrelease": 4374, "Ġfaz": 4375, "Ġic": 4376, "itude": 4377, "AC": 4378, "ĠPat": 4379, "iden": 4380, "ŃIJ": 4381, "Ġprefer": 4382, "ological": 4383, "ĠFacebook": 4384, "Ġê°Ļ": 4385, "Ġ..": 4386, "ĠMake": 4387, "ĠкоÑĤоÑĢ": 4388, "ĠDavid": 4389, "ĠAfric": 4390, "Ġmode": 4391, "ĠCity": 4392, "Ġshall": 4393, "ĠÑĦ": 4394, "imin": 4395, "Ġза": 4396, "rom": 4397, "ua": 4398, "Ġbeyond": 4399, "Ġdistrib": 4400, "кÑĥ": 4401, "ĠDoes": 4402, "Ġvict": 4403, "rate": 4404, "Ġvai": 4405, "Ġsuccessful": 4406, "Ġhous": 4407, "aha": 4408, "ests": 4409, "ĠEst": 4410, "Ġdiscover": 4411, "Ġtherefore": 4412, "cha": 4413, "Ġcup": 4414, "Ġpopulation": 4415, "ĠIl": 4416, "sc": 4417, "Ġspent": 4418, "rel": 4419, "Ġuseful": 4420, "Ġtab": 4421, "æĿ": 4422, "ĠÅ": 4423, "Ġìłľ": 4424, "Ġconse": 4425, "Ġquant": 4426, "aya": 4427, "Ġbon": 4428, "åı¯": 4429, "ĠChin": 4430, "Ġê²ĥ": 4431, "ounds": 4432, "еÑĪ": 4433, "elle": 4434, "Ġice": 4435, "21": 4436, "Ġkick": 4437, "ä¸ĭ": 4438, "Ġsteps": 4439, "Ġtonight": 4440, "нÑĭй": 4441, "rench": 4442, ".'": 4443, "Ġgrab": 4444, "Ġimplement": 4445, "ĠìĪĺ": 4446, "Ġmission": 4447, "Ġclearly": 4448, "Ġappreciate": 4449, "èĢ": 4450, "Ġfresh": 4451, "arm": 4452, "ĠTwo": 4453, "Ġexec": 4454, "Ġprojects": 4455, "Ġcommunities": 4456, "rible": 4457, "Ġregion": 4458, "Ġfrequ": 4459, "roy": 4460, "Ġhowever": 4461, "Ġpartners": 4462, "anc": 4463, "Ġminim": 4464, "Ġlat": 4465, "Ġfamilies": 4466, "Ġevidence": 4467, "Ġpun": 4468, "raft": 4469, "Ġloss": 4470, "Ġmap": 4471, "Ġanybody": 4472, "Ġchanging": 4473, "Ġrules": 4474, "Ġorganization": 4475, "Ġessentially": 4476, "ĠRed": 4477, "Ġelement": 4478, "æĹ": 4479, "Ġvirt": 4480, "rat": 4481, "Ġprint": 4482, "ander": 4483, "aren": 4484, "emos": 4485, "οÏħ": 4486, "Ġconditions": 4487, "abe": 4488, "Ġdance": 4489, "иÑĢ": 4490, "Ġdos": 4491, "оÑĩ": 4492, "ĠQue": 4493, "Ġwalking": 4494, "Ġtro": 4495, "Ġid": 4496, "Ġadditional": 4497, "Ġfully": 4498, "Ġfans": 4499, "Ġaddition": 4500, "Ġliked": 4501, "Ġüber": 4502, "Ġbow": 4503, "di": 4504, "Ġmaster": 4505, "off": 4506, "):": 4507, "mber": 4508, "Ġë¬": 4509, "å¯": 4510, "åΰ": 4511, "lause": 4512, "Ġoder": 4513, "Ġsafety": 4514, "Ġreact": 4515, "ி": 4516, "bt": 4517, "Ġdisapp": 4518, "Ġgirls": 4519, "St": 4520, "ĠAng": 4521, "Ġfaith": 4522, "Ġturns": 4523, "Ġtight": 4524, "Ġmouth": 4525, "ami": 4526, "zer": 4527, "Ġweap": 4528, "ĠбÑĥд": 4529, "Ġhospital": 4530, "raid": 4531, "Ġmicro": 4532, "ĠState": 4533, "ĠMost": 4534, "agn": 4535, "Ġdecide": 4536, "Ġpatient": 4537, "Ġcorner": 4538, "Ġdied": 4539, "No": 4540, "ĠStud": 4541, "rend": 4542, "empt": 4543, "Ġlie": 4544, "Ġlif": 4545, "ĠBefore": 4546, "tó": 4547, "ĠSuper": 4548, "Ġbell": 4549, "60": 4550, "Ġprivate": 4551, "ĠPaul": 4552, "Ġgib": 4553, "Ġagre": 4554, "´ìĦľ": 4555, "Ġsig": 4556, "Ġinvestig": 4557, "ÑıÑĤ": 4558, "ening": 4559, "Ġdistance": 4560, "Ġwarm": 4561, "Ġdigital": 4562, "å¾Ī": 4563, "iner": 4564, "Ġpand": 4565, "ĠCOVID": 4566, "го": 4567, "gn": 4568, "Ġrace": 4569, "Ġproud": 4570, "Ġteaching": 4571, "ĠÑĤо": 4572, "ìŀ¥": 4573, "ĠAllah": 4574, "In": 4575, "Ġwood": 4576, "Ġcolors": 4577, "Ġwird": 4578, "uj": 4579, "idad": 4580, "Ġcustomers": 4581, "Ġconnected": 4582, "Ġlayer": 4583, "Ġachieve": 4584, "Ġperspective": 4585, "ĠColl": 4586, "ÙĤ": 4587, "Ġcloud": 4588, "!!!": 4589, "Ġended": 4590, "łĩê²Į": 4591, "Ġmanagement": 4592, "Ġrich": 4593, "Ġsubst": 4594, "Ġremo": 4595, "Ġserve": 4596, "Ġresist": 4597, "Ġthoughts": 4598, "Ġgrowth": 4599, "iliar": 4600, "Ġrights": 4601, "Ġcharge": 4602, "Ġconsist": 4603, "Ġwerden": 4604, "Ġemb": 4605, "andom": 4606, "Ġhurt": 4607, "Ġkan": 4608, "ias": 4609, "ло": 4610, "Ġshit": 4611, "Ġbeg": 4612, "Ġreceived": 4613, "itation": 4614, "Ġmeat": 4615, "Ġisso": 4616, "ffee": 4617, "Ġfamous": 4618, "Ġcomfortable": 4619, "IL": 4620, "ĠBye": 4621, "說": 4622, "åĢij": 4623, "othes": 4624, "Ġmedical": 4625, "Ġenjoyed": 4626, "Ġhealthy": 4627, "Ġwy": 4628, "cies": 4629, "Ġeffort": 4630, "Ġdoctor": 4631, "Ġmilitary": 4632, "LAU": 4633, "Ġgro": 4634, "Ġbattle": 4635, "Ġfed": 4636, "Ġcapac": 4637, "Ġafraid": 4638, "ivil": 4639, "ĠвÑģе": 4640, "Ġlength": 4641, "ysis": 4642, "Ġbei": 4643, "¤í": 4644, "Ġorganiz": 4645, "org": 4646, "inc": 4647, "Ġinteract": 4648, "ĠChinese": 4649, "Ġaccording": 4650, "Ġincredible": 4651, "Ġkilled": 4652, "Ġdaughter": 4653, "ĠÏĢ": 4654, "Ñĭв": 4655, "Ġschools": 4656, "Ġ«": 4657, "ller": 4658, "Ġshouldn": 4659, "nal": 4660, "Ġcris": 4661, "Ġchicken": 4662, "Ġfaster": 4663, "Ġextremely": 4664, "Ġoppos": 4665, "Ġnous": 4666, "Ġ+": 4667, "ria": 4668, "Ġfinancial": 4669, "Ġexciting": 4670, "Ġjourney": 4671, "×Ļ×Ŀ": 4672, "łë": 4673, "Ġdisplay": 4674, "Ġmemory": 4675, "Ġheavy": 4676, "не": 4677, "Ġpassed": 4678, "ÑĢи": 4679, "iles": 4680, "Ġpsych": 4681, "Ġspecifically": 4682, "Ġengage": 4683, "Ġled": 4684, "orge": 4685, "ĠDem": 4686, "order": 4687, "Ġ80": 4688, "Ġcream": 4689, "esterday": 4690, "Ġedge": 4691, "Ġпол": 4692, "Ġbull": 4693, "Ġindic": 4694, "Ġktó": 4695, "Ġhopefully": 4696, "uments": 4697, "agen": 4698, "ного": 4699, "Ġhate": 4700, "cht": 4701, "80": 4702, "Ġeffic": 4703, "Ġì§Ģ": 4704, "Ġinternet": 4705, "Ġbudget": 4706, "Ġproperty": 4707, "iday": 4708, "Ġìļ": 4709, "Ġмож": 4710, "ola": 4711, "Ġshowed": 4712, "ĠMon": 4713, "Ġthousand": 4714, "AP": 4715, "Ġpoor": 4716, "used": 4717, "ĠJack": 4718, "ĠsÃ¥": 4719, "ĥ½": 4720, "Ġesc": 4721, "Ġsoftware": 4722, "Ġquar": 4723, "Ġب": 4724, "Ġnecessarily": 4725, "omen": 4726, "iy": 4727, "Ġeventually": 4728, "ished": 4729, "Ġbright": 4730, "ED": 4731, "Ġspl": 4732, "Ġdemand": 4733, "Ġthreat": 4734, "Ġsir": 4735, "Ġreleased": 4736, "cket": 4737, "ĠâĢ«": 4738, "Ġrequired": 4739, "Ġvote": 4740, "ì¹": 4741, "த": 4742, "Ġdeveloped": 4743, "ĠìĤ¬": 4744, "atory": 4745, "Ġdir": 4746, "cape": 4747, "Ġslightly": 4748, "ì": 4749, "à¹ī": 4750, "reet": 4751, "Ġdisease": 4752, "Ġcourt": 4753, "Ġitems": 4754, "ĠEarth": 4755, "ÑģÑĤи": 4756, "же": 4757, "ì²": 4758, "Ġchallenges": 4759, "ĠBrit": 4760, "Ġdesigned": 4761, "12": 4762, "Ġhearing": 4763, "Ġlistening": 4764, "zo": 4765, "ĠÑģл": 4766, "ãģ§ãģĻ": 4767, "Ġpero": 4768, "Ġwearing": 4769, "plic": 4770, "Ġchem": 4771, "Ġbalance": 4772, "Ġba": 4773, "Ġreceive": 4774, "ima": 4775, "Ġsignificant": 4776, "ĠмÑĭ": 4777, "anch": 4778, "ĠCr": 4779, "ĠCoun": 4780, "ê¸Ī": 4781, "Ġjobs": 4782, "Ġofficial": 4783, "Ġperm": 4784, "oms": 4785, "Ġopportunities": 4786, "Ġoverall": 4787, "Ġhus": 4788, "odes": 4789, "Ġnation": 4790, "ĠReg": 4791, "Ġord": 4792, "Ġrestaur": 4793, "ĠìĨ": 4794, "Ġmel": 4795, "vin": 4796, "Ġwenn": 4797, "Ġkön": 4798, "æĥ": 4799, "Ġopinion": 4800, "ãĤĤ": 4801, "è¬": 4802, "ĠSometimes": 4803, "çĤ": 4804, "Ñīе": 4805, "asc": 4806, "OU": 4807, "Ġ2020": 4808, "Ġdelicious": 4809, "iger": 4810, "ĠìķĪ": 4811, "ole": 4812, "Ġhandle": 4813, "Ġcit": 4814, "Ġíķľ": 4815, "Ġför": 4816, "ooth": 4817, "Ġnecessary": 4818, "Ġindepend": 4819, "æĦ": 4820, "isten": 4821, "ham": 4822, "Ġét": 4823, "ãĥ³": 4824, "Ġmulti": 4825, "ÏĮ": 4826, "?)": 4827, "Ġcampus": 4828, "Ġtopic": 4829, "Ġrain": 4830, "Ġpanel": 4831, "ĠSam": 4832, "Ġlarger": 4833, "audience": 4834, "Ġpaid": 4835, "Ġeconomic": 4836, "olt": 4837, "Ġstreet": 4838, "ĠCont": 4839, "Ġdriving": 4840, "ĠìłĢ": 4841, "Ġhay": 4842, "Ġprofessional": 4843, "ĠIntern": 4844, "å¸": 4845, "Ġinput": 4846, "Ġcateg": 4847, "Ġcro": 4848, "Ġll": 4849, "ET": 4850, "Ñĭй": 4851, "**": 4852, "ĠZe": 4853, "BLE": 4854, "Ġì¤": 4855, "rees": 4856, "ĠЯ": 4857, "ede": 4858, "iert": 4859, "Ġfold": 4860, "Ġdur": 4861, "ĠNational": 4862, "Ġìĸ´ë": 4863, "anced": 4864, "Ġfaire": 4865, "uted": 4866, "Ġking": 4867, "Ġwild": 4868, "oi": 4869, "upbeat": 4870, "Ġprevent": 4871, "ius": 4872, "Ġè": 4873, "Ġwide": 4874, "Ġring": 4875, "Ġtitle": 4876, "Ġstanding": 4877, "Ġalthough": 4878, "Ġhi": 4879, "Ġsauce": 4880, "Ġsides": 4881, "Ġanimals": 4882, "iling": 4883, "atives": 4884, "ìĹIJìĦľ": 4885, "ĠOver": 4886, "Ġdesp": 4887, "Ġconsidered": 4888, "aries": 4889, "iers": 4890, "Ġeinen": 4891, "Ġsister": 4892, "Ġëķ": 4893, "ĠSure": 4894, "ãĤĭ": 4895, "riend": 4896, "aign": 4897, "Ġshown": 4898, "Ġsac": 4899, "Ġsont": 4900, "Ġcentury": 4901, "Ġtien": 4902, "Ġκ": 4903, "ĠST": 4904, "åķĬ": 4905, "Ġolder": 4906, "iem": 4907, "Ġtruly": 4908, "ĠSi": 4909, "Ġwindow": 4910, "iques": 4911, "ario": 4912, "æ²Ĵ": 4913, "Ġlocation": 4914, "κ": 4915, "Ġìľ": 4916, "vi": 4917, "ague": 4918, "ĠSorry": 4919, "Ġdisp": 4920, "Ġhell": 4921, "ĠÃī": 4922, "Ġtrade": 4923, "Ġcritical": 4924, "Ġê±": 4925, "Ġnamed": 4926, "Ġprepared": 4927, "ĠHouse": 4928, "alu": 4929, "Ġtough": 4930, "Ġtrip": 4931, "Ġsand": 4932, "cel": 4933, "üz": 4934, "ĠPut": 4935, "Ġapart": 4936, "isf": 4937, "vis": 4938, "Ġlibr": 4939, "aven": 4940, "Ġvie": 4941, "Ġeffective": 4942, "า": 4943, "Ġmagn": 4944, "Ġmuito": 4945, "Ġêµ": 4946, "hal": 4947, "Ġlimit": 4948, "Ġnine": 4949, "Ġwilling": 4950, "Ä±ÅŁ": 4951, "sp": 4952, "ег": 4953, "hi": 4954, "Ġalt": 4955, "ĠJan": 4956, "Ġorigin": 4957, "ĠUs": 4958, "Ġelements": 4959, "Ġuses": 4960, "Ġhelpful": 4961, "Ġflat": 4962, "Ġfamiliar": 4963, "ĠPark": 4964, "Ġcore": 4965, "Ġcloser": 4966, "Ġactive": 4967, "Ġadminist": 4968, "CE": 4969, "нÑĭе": 4970, "çĦ": 4971, "Ġrelative": 4972, "Ġmental": 4973, "Ġrandom": 4974, "Ġpartner": 4975, "Ġutil": 4976, "phone": 4977, "Ġrule": 4978, "ww": 4979, "Ġìłķ": 4980, "Ġschon": 4981, "Ġcoffee": 4982, "HA": 4983, "Ġconnection": 4984, "Ġunit": 4985, "laughing": 4986, "log": 4987, "Ġappl": 4988, "ла": 4989, "usic": 4990, "ĠBra": 4991, "Ġanywhere": 4992, "AUDI": 4993, "Ġseparate": 4994, "box": 4995, "Ġdivid": 4996, "Ġtesting": 4997, "Ġsick": 4998, "Ġweren": 4999, "ä»ĸ": 5000, "Ġ׾×": 5001, "Ġadvantage": 5002, "Ġtransfer": 5003, "'.": 5004, "Ġë¹": 5005, "Ġfinding": 5006, "ной": 5007, "Ġì¢ĭ": 5008, "Ġfort": 5009, "Ġeconomy": 5010, "Ġlack": 5011, "Ġleaving": 5012, "Ġdim": 5013, "åİ": 5014, "ĠRes": 5015, "ØŃ": 5016, "Ġdiscussion": 5017, "еп": 5018, "Ġges": 5019, "duct": 5020, "Ġchain": 5021, "Ġusers": 5022, "ech": 5023, "ÅĤa": 5024, "Ġdish": 5025, "Ġcareful": 5026, "Ġteacher": 5027, "Ġoptim": 5028, "Ġflu": 5029, "atically": 5030, "Ġreflect": 5031, "Ġtreatment": 5032, "eed": 5033, "iÄĻ": 5034, "ù": 5035, "ா": 5036, "Ġequip": 5037, "Ġplanning": 5038, "Ġsolve": 5039, "ãģĿ": 5040, "ĠTom": 5041, "Ġavoid": 5042, "Ġpou": 5043, "Ġgreater": 5044, "lin": 5045, "OL": 5046, "ĠLu": 5047, "ĠMore": 5048, "Ġattract": 5049, "ên": 5050, "una": 5051, "Ġphoto": 5052, "eration": 5053, "Ġplanet": 5054, "Ġcopy": 5055, "Ġvisual": 5056, "iring": 5057, "Ġinternational": 5058, "Ġlaughing": 5059, "Ġthick": 5060, "Ġholding": 5061, "Ġbringing": 5062, "Ġletter": 5063, "Ġburn": 5064, "Ġeffects": 5065, "ité": 5066, "ours": 5067, "OT": 5068, "ême": 5069, "ĠSchool": 5070, "×ķת": 5071, "ropri": 5072, "lig": 5073, "αι": 5074, "Ġadult": 5075, "Ġsugar": 5076, "Ġride": 5077, "Ġhighlight": 5078, "Ġnobody": 5079, "Ġ21": 5080, "Ġchat": 5081, "ĠпÑĢи": 5082, "Ġinnov": 5083, "ungen": 5084, "Ġattach": 5085, "edom": 5086, "åĬ": 5087, "yl": 5088, "Ġlegal": 5089, "Ġrice": 5090, "Ġcollabor": 5091, "king": 5092, "down": 5093, "æĻ": 5094, "ãĤĬ": 5095, "Ġih": 5096, "ĠAc": 5097, "ously": 5098, "Ġrap": 5099, "Ġsolid": 5100, "Ġgenerally": 5101, "Ġpattern": 5102, "ali": 5103, "à¸Ń": 5104, "Ġtransl": 5105, "inter": 5106, "ault": 5107, "Ġë¨": 5108, "Ġexpress": 5109, "Ġexamples": 5110, "Ġchose": 5111, "Ġtells": 5112, "ÃŃs": 5113, "aint": 5114, "ĠTell": 5115, "ĠMichael": 5116, "æ¨": 5117, "ĠNumber": 5118, "Ġtap": 5119, "Ġexperiment": 5120, "Ġbenefit": 5121, "Ġì°": 5122, "Ġsequ": 5123, "Ġexpensive": 5124, "Ġgeneration": 5125, "ĠMany": 5126, "Ġadding": 5127, "Ġkil": 5128, "Ġcampaign": 5129, "ĠAnt": 5130, "raw": 5131, "ommen": 5132, "Ġsoul": 5133, "jo": 5134, "ĠActually": 5135, "amm": 5136, "ê²ł": 5137, "Ġmaxim": 5138, "Ġsalt": 5139, "Ġcru": 5140, "Ġcalling": 5141, "ãģĮ": 5142, "Ġbasis": 5143, "ban": 5144, "Ġkeeping": 5145, "ĠMor": 5146, "eds": 5147, "ìĨ": 5148, "Ġtodo": 5149, "ами": 5150, "нÑı": 5151, "Ġlived": 5152, "ĠDu": 5153, "ãĤī": 5154, "å®¶": 5155, "force": 5156, "å¹´": 5157, "ference": 5158, "ala": 5159, "Ġoccur": 5160, "sk": 5161, "Ġrecent": 5162, "Ġcars": 5163, "Ġtraditional": 5164, "entle": 5165, "²Ī": 5166, "Ġheld": 5167, "Ġnach": 5168, "ĠCenter": 5169, "eren": 5170, "Ġbin": 5171, "Ùģ": 5172, "Ġcomme": 5173, "Ġreve": 5174, "Ġìĺ¤": 5175, "Ġexpected": 5176, "abil": 5177, "Ġfocused": 5178, "ov": 5179, "ĠiP": 5180, "orial": 5181, "iro": 5182, "Ġetc": 5183, "aming": 5184, "ĠSon": 5185, "Ġyesterday": 5186, "Ġstrate": 5187, "ĠÑĨ": 5188, "Ġëı": 5189, "pes": 5190, "Ġactivity": 5191, "Ġadvice": 5192, "Ġopening": 5193, "fin": 5194, "Ġrela": 5195, "éĸ": 5196, "Ġinstance": 5197, "ĠEveryone": 5198, "bl": 5199, "pen": 5200, "Ġvision": 5201, "ĠAlex": 5202, "iforn": 5203, "Ġtick": 5204, "He": 5205, "Ġstrategy": 5206, "Ġkom": 5207, "PE": 5208, "ĠGl": 5209, "Ġelectric": 5210, "15": 5211, "Ġdaily": 5212, "Ġhusband": 5213, "Ġstation": 5214, "Ġanalysis": 5215, "ynam": 5216, "Ġattempt": 5217, "Ġbillion": 5218, "vant": 5219, "Ġforth": 5220, "Ġmath": 5221, "aly": 5222, "Ġbehavior": 5223, "ĠMas": 5224, "kan": 5225, "ĠDay": 5226, "Ġbless": 5227, "Ġgut": 5228, "ĠHigh": 5229, "ox": 5230, "Ġdress": 5231, "Ġjed": 5232, "è¯": 5233, "åĸ": 5234, "Ġexperiences": 5235, "ista": 5236, "Ġfighting": 5237, "å·": 5238, "ĠÑģк": 5239, "Ġmostly": 5240, "ause": 5241, "Ġpictures": 5242, "енÑĤ": 5243, "Ġmad": 5244, "Ġmodels": 5245, "ÑĪе": 5246, "ĠCount": 5247, "ÅĦ": 5248, "ÅĤo": 5249, "ept": 5250, "OM": 5251, "ĠAN": 5252, "Ġtrouble": 5253, "40": 5254, "Ġbird": 5255, "ulate": 5256, "Ġmur": 5257, "Ġproduce": 5258, "Ġmarried": 5259, "bit": 5260, "Ġtheory": 5261, "íĺ": 5262, "Ġleader": 5263, "ĠLast": 5264, "AA": 5265, "èµ": 5266, "Ġimages": 5267, "Ġexpand": 5268, "ĠPor": 5269, "Ġpurch": 5270, "ĠSan": 5271, "ĠChristmas": 5272, "ĠAustral": 5273, "Ġwid": 5274, "ĠMiss": 5275, "Ġknowing": 5276, "Ġze": 5277, "ship": 5278, "ku": 5279, "Ñħод": 5280, "ĠInstagram": 5281, "ĠIndia": 5282, "Ġesta": 5283, "ĠCaliforn": 5284, "Ġ70": 5285, "Ġdrag": 5286, "Ġbrush": 5287, "Ġnames": 5288, "And": 5289, "Ġyo": 5290, "illa": 5291, "Ġsched": 5292, "Ġdestroy": 5293, "year": 5294, "Ġvamos": 5295, "ĠÙĦ": 5296, "ça": 5297, "Ġforgot": 5298, "ие": 5299, "Ġraise": 5300, "reme": 5301, "íķ´": 5302, "ĠGive": 5303, "Ġcontain": 5304, "rab": 5305, "Ġgift": 5306, "ĠÑģп": 5307, "Ġrequest": 5308, "Ġshut": 5309, "Ġdegrees": 5310, "Ġbenefits": 5311, "Ñĭе": 5312, "Ġstudies": 5313, "Ġends": 5314, "Ġeverywhere": 5315, "Ġhero": 5316, "oph": 5317, "erry": 5318, "Ġmaterials": 5319, "ened": 5320, "NA": 5321, "åį": 5322, "Ġmuy": 5323, "Ġworse": 5324, "ä»Ģ": 5325, "ĠMad": 5326, "Ġdecisions": 5327, "ione": 5328, "Ġforeign": 5329, "laughter": 5330, "iber": 5331, "ениÑı": 5332, "ãħĭ": 5333, "Ġrealized": 5334, "Ġign": 5335, "Ġweak": 5336, "Ġμ": 5337, "Ġscared": 5338, "Ġassum": 5339, "AK": 5340, "ï¿": 5341, "�": 5342, "Ġcovered": 5343, "ĠSat": 5344, "Ġон": 5345, "Ġindividuals": 5346, "Ġcompared": 5347, "11": 5348, "ĠAdd": 5349, "icles": 5350, "Ġcert": 5351, "rar": 5352, "Ġbrief": 5353, "Ġactivities": 5354, "Ġfab": 5355, "bar": 5356, "Ġast": 5357, "ĠOther": 5358, "Ġclasses": 5359, "Ġog": 5360, "Ġmissing": 5361, "ãģł": 5362, "éĿ": 5363, "wers": 5364, "ש": 5365, "Ġintroduce": 5366, "Ġequation": 5367, "ãģ¾ãģĻ": 5368, "Ġnom": 5369, "Ġpainting": 5370, "ushing": 5371, "ĠAP": 5372, "Ġencourage": 5373, "Ġship": 5374, "ittee": 5375, "iverse": 5376, "ota": 5377, "nam": 5378, "ãĥ»": 5379, "Ġexercise": 5380, "ĠÐŃ": 5381, "Ġnas": 5382, "Ġthousands": 5383, "ĠCalifornia": 5384, "Ġses": 5385, "Ġrow": 5386, "ŀĪ": 5387, "Ġpandemic": 5388, "Ġskill": 5389, "bel": 5390, "Ġdirector": 5391, "Ġmilk": 5392, "Ġnut": 5393, "Ġmotion": 5394, "Ġclosed": 5395, "è¨": 5396, "Ġcredit": 5397, "ahr": 5398, "Ġcheese": 5399, "Ġaltern": 5400, "imately": 5401, "Ġsust": 5402, "ĠTra": 5403, "Ġglad": 5404, "Ġhighly": 5405, "Ġwa": 5406, "Ġreduce": 5407, "Ġble": 5408, "ador": 5409, "inated": 5410, "iones": 5411, "cient": 5412, "Ġdepending": 5413, "Ġsharing": 5414, "Ġcaught": 5415, "rael": 5416, "Ġmehr": 5417, "Ġpassion": 5418, "çĽ": 5419, "Ġru": 5420, "Ġfarm": 5421, "TI": 5422, "aves": 5423, "ĠRob": 5424, "ĠBro": 5425, "Ġmotiv": 5426, "retch": 5427, "rupt": 5428, "ĠBig": 5429, "Ġalle": 5430, "Ġett": 5431, "ubs": 5432, "ĠJapanese": 5433, "ĠHall": 5434, "или": 5435, "AUDIBLE": 5436, "ç¬": 5437, "Ġcells": 5438, "ika": 5439, "eline": 5440, "iler": 5441, "Ġì£": 5442, "Ġsky": 5443, "INAUDIBLE": 5444, "ende": 5445, "apter": 5446, "Ġpin": 5447, "Ġgather": 5448, "hol": 5449, "lection": 5450, "Ġsyn": 5451, "Ġplug": 5452, "round": 5453, "Ġuniversity": 5454, "hib": 5455, "Ġfantastic": 5456, "kn": 5457, "Ġhole": 5458, "ĠRemember": 5459, "inct": 5460, "aks": 5461, "CH": 5462, "Ġbroken": 5463, "Ġstrateg": 5464, "Ġalive": 5465, "Ġtank": 5466, "Ġcart": 5467, "rated": 5468, "rie": 5469, "ĠStep": 5470, "ĠEverything": 5471, "Ġbound": 5472, "Ġsobre": 5473, "Ġcustomer": 5474, "¡Į": 5475, "urg": 5476, "ĠBill": 5477, "La": 5478, "what": 5479, "Ġreaction": 5480, "Ġsession": 5481, "Ġplans": 5482, "ĠìĿ´ëłĩê²Į": 5483, "Ġdownload": 5484, "ìĻ": 5485, "uer": 5486, "Ġcab": 5487, "Ġinstr": 5488, "ifying": 5489, "ĠNice": 5490, "Ġteams": 5491, "ıl": 5492, "Ġgoals": 5493, "isch": 5494, "Ġtransport": 5495, "Ġanimal": 5496, "Ġcosts": 5497, "Ġcalls": 5498, "Ġsehr": 5499, "ìĪ": 5500, "rian": 5501, "Ġdial": 5502, "Ġweather": 5503, "à¹Ģ": 5504, "ĠвоÑĤ": 5505, "ĠPlay": 5506, "Ġshared": 5507, "Ġsmooth": 5508, "aba": 5509, "Ġleaves": 5510, "ன": 5511, "Ġconcent": 5512, "Ġshift": 5513, "ĠëIJĺ": 5514, "ĠGovern": 5515, "Ġdemonst": 5516, "Ġbutter": 5517, "ĠìŬ": 5518, "Ġsatisf": 5519, "Īë¬": 5520, "Ġrecognize": 5521, "ĠFrench": 5522, "Ġvolume": 5523, "änd": 5524, "Ñĥм": 5525, "Ġì§Ħ": 5526, "ĠKeep": 5527, "owa": 5528, "ipped": 5529, "ÑģÑĤÑĢ": 5530, "Ġdetect": 5531, "ĠÏĥ": 5532, "Ġlift": 5533, "Ġclothes": 5534, "ĠStop": 5535, "õ": 5536, "met": 5537, "Ġclin": 5538, "Ġarr": 5539, "friend": 5540, "Ġstuck": 5541, "Ye": 5542, "hand": 5543, "uma": 5544, "Ġscri": 5545, "Ġfucking": 5546, "ctors": 5547, "ת": 5548, "Ġjoining": 5549, "Ġcette": 5550, "ĠØ£": 5551, "ĠWhite": 5552, "Ġihr": 5553, "ÎŃ": 5554, "ãģŃ": 5555, "Ġincluded": 5556, "esso": 5557, "Ġacad": 5558, "bum": 5559, "Ġsab": 5560, "ĠдлÑı": 5561, "è¿Ļ": 5562, "ufact": 5563, "ĠRepublic": 5564, "rim": 5565, "Ġyellow": 5566, "Ġlimited": 5567, "TER": 5568, "ĠTy": 5569, "Ġnotes": 5570, "vest": 5571, "из": 5572, "aled": 5573, "Ġphase": 5574, "anda": 5575, "ĠMom": 5576, "RI": 5577, "Ġimmer": 5578, "mal": 5579, "Ġinj": 5580, "Ġyang": 5581, "udible": 5582, "аг": 5583, "Ġsett": 5584, "Ġmagic": 5585, "Ġensure": 5586, "Ġspring": 5587, "Ġshock": 5588, "Ġwheel": 5589, "огда": 5590, "ãĤĪ": 5591, "Ġcancer": 5592, "Ġroot": 5593, "ÐIJ": 5594, "gency": 5595, "Ġëį": 5596, "ii": 5597, "Ġoutput": 5598, "Ġcommit": 5599, "Ġworkers": 5600, "ìķĦìļĶ": 5601, "ĠÑģам": 5602, "vey": 5603, "Ġpeu": 5604, "Ġcivil": 5605, "isc": 5606, "Ġbrings": 5607, "ÑĢав": 5608, "ania": 5609, "Äģ": 5610, "craft": 5611, "mbol": 5612, "Ġintellig": 5613, "bi": 5614, "acing": 5615, "you": 5616, "Ġbecoming": 5617, "ĠDer": 5618, "ema": 5619, "å°±æĺ¯": 5620, "Ġingred": 5621, "Ġcommand": 5622, "Ġupdate": 5623, "Ġprem": 5624, "Ġopened": 5625, "Ħ¤": 5626, "ение": 5627, "Ġgard": 5628, "Ġstatement": 5629, "Ġscrew": 5630, "Ġprote": 5631, "Ġcards": 5632, "Ġtask": 5633, "Ġevening": 5634, "Ġstitch": 5635, "inen": 5636, "ĠBer": 5637, "mark": 5638, "ĠDad": 5639, "ĠеÑģÑĤÑĮ": 5640, "Ġ×ŀ×": 5641, "ìĹĪ": 5642, "Ġban": 5643, "Ġclim": 5644, "Ġfreedom": 5645, "Ġnormally": 5646, "еÑģÑĮ": 5647, "å¦": 5648, "Ġprovided": 5649, "ĠìŀIJ": 5650, "ĠìķĦëĭĪ": 5651, "ĠKim": 5652, "ieder": 5653, "ìĿĮ": 5654, "Ġcitiz": 5655, "Ġbike": 5656, "Ġbak": 5657, "Ġnoise": 5658, "Ġclimate": 5659, "izes": 5660, "å¾Į": 5661, "Ġincreasing": 5662, "ĠTHE": 5663, "Ġliqu": 5664, "Ġpersonally": 5665, "ef": 5666, "resp": 5667, "Ġlegs": 5668, "inder": 5669, "Ġped": 5670, "Ġë§İ": 5671, "Ġdepend": 5672, "Ġvariety": 5673, "ĠIsrael": 5674, "Ġwash": 5675, "åĨ": 5676, "Ġquiet": 5677, "ĠJames": 5678, "ĠJew": 5679, "Ġforever": 5680, "ĠInt": 5681, "Ġcounter": 5682, "urance": 5683, "ĠAnyway": 5684, "care": 5685, "ĠOnly": 5686, "ción": 5687, "adi": 5688, "ĠEv": 5689, "ëĭĪê¹Į": 5690, "Ġα": 5691, "Ġslowly": 5692, "Ġод": 5693, "Ġnoticed": 5694, "ieren": 5695, "Ġfell": 5696, "ĠÐij": 5697, "Ġmême": 5698, "Ġwhenever": 5699, "!)": 5700, "ĠHy": 5701, "å¼": 5702, "ords": 5703, "usion": 5704, "ĠStar": 5705, "Ġíĺ": 5706, "ĠMac": 5707, "ä¸Ĭ": 5708, "iven": 5709, "Ġìĭľ": 5710, "ĠìĹĨ": 5711, "ĠTur": 5712, "Ġger": 5713, "ris": 5714, "Ġvez": 5715, "ĠлÑİ": 5716, "Ġversus": 5717, "اØ": 5718, "ocolate": 5719, "Ġplane": 5720, "Ġzo": 5721, "Ġsuit": 5722, "This": 5723, "Ġnerv": 5724, "ĠAcc": 5725, "Ñĥж": 5726, "ìĤ¬": 5727, "nh": 5728, "eme": 5729, "Ġauss": 5730, "Ġmeas": 5731, "Ġtrès": 5732, "Ïī": 5733, "Ñģли": 5734, "ĠArt": 5735, "ĠSecond": 5736, "олÑĮко": 5737, "cho": 5738, "itect": 5739, "еÑģÑĤ": 5740, "Ġboss": 5741, "Ġincome": 5742, "ł¤": 5743, "Ġshad": 5744, "Ġappropri": 5745, "ĠMal": 5746, "opt": 5747, "Ġartist": 5748, "Ġplays": 5749, "others": 5750, "ĠInter": 5751, "Ġvirus": 5752, "Ġhung": 5753, "Ġconstant": 5754, "Ġscript": 5755, "Ġsnow": 5756, "ulf": 5757, "ket": 5758, "Ġdevices": 5759, "Ġmetal": 5760, "ights": 5761, "ìĦ¸": 5762, "Ġsales": 5763, "Ġveget": 5764, "Ġcollection": 5765, "Ġvia": 5766, "ker": 5767, "Ġgotten": 5768, "OW": 5769, "ién": 5770, "Ġaccur": 5771, "Ġwave": 5772, "ulty": 5773, "ĠAir": 5774, "Ġleading": 5775, "icing": 5776, "Ġcentral": 5777, "ĠChristian": 5778, "fr": 5779, "ĠAlthough": 5780, "Ġsongs": 5781, "Ġfif": 5782, "нÑĭÑħ": 5783, "Ġbelong": 5784, "ossible": 5785, "ì°": 5786, "Ġphotos": 5787, "isl": 5788, "Ġrelax": 5789, "sa": 5790, "USIC": 5791, "ê·": 5792, "Ġmanufact": 5793, "ĠTwitter": 5794, "Ġdangerous": 5795, "Ġhyd": 5796, "lear": 5797, "iant": 5798, "Ġâ̦": 5799, "Ġsuddenly": 5800, "Ġlaugh": 5801, "Ġangle": 5802, "ĠGot": 5803, "Ġworried": 5804, "ое": 5805, "Ġpap": 5806, "ĠMart": 5807, "eno": 5808, "Ġbattery": 5809, "ĠпоÑģ": 5810, "Ġlights": 5811, "Ġarms": 5812, "ĠAbs": 5813, "mes": 5814, "âĢĵ": 5815, "useum": 5816, "Ġtea": 5817, "ĠMic": 5818, "Ġformer": 5819, "ography": 5820, "Ġapplications": 5821, "ĠDire": 5822, "çĦ¶": 5823, "Ġfeedback": 5824, "itchen": 5825, "yorum": 5826, "ued": 5827, "igt": 5828, "ưá»": 5829, "osition": 5830, "ĠDel": 5831, "Ġíķĺë": 5832, "ĠBack": 5833, "ads": 5834, "Ġprime": 5835, "주": 5836, "ì£ł": 5837, "×ij": 5838, "Ġmut": 5839, "].": 5840, "ĠÐĹ": 5841, "loc": 5842, "kin": 5843, "Ġexpert": 5844, "Ġalright": 5845, "ungs": 5846, "Ġsupply": 5847, "Ġleadership": 5848, "ĠFra": 5849, "Ġtypically": 5850, "Ġsel": 5851, "Ġtrees": 5852, "Ġ22": 5853, "har": 5854, "Ġworst": 5855, "Ġbusy": 5856, "anto": 5857, "ĠUp": 5858, "ĠBas": 5859, "Ġpresentation": 5860, "Ġstrange": 5861, "Ġthin": 5862, "ÑĤе": 5863, "Ġvehicle": 5864, "Ġдо": 5865, "cellent": 5866, "70": 5867, "Ġtired": 5868, "Ġcrisis": 5869, "Ġtiny": 5870, "asy": 5871, "Ġran": 5872, "éĩ": 5873, "Ġforces": 5874, "ĠоÑĩ": 5875, "Ġidentify": 5876, "Ġassess": 5877, "иÑĤе": 5878, "SE": 5879, "Ġcreative": 5880, "çŁ": 5881, "Ġdepartment": 5882, "Ġinitial": 5883, "æĪijåĢij": 5884, "ĠDam": 5885, "akt": 5886, "vere": 5887, "Ġinfect": 5888, "Ġpump": 5889, "ạ": 5890, "Ġviel": 5891, "Ġrare": 5892, "Ġdot": 5893, "ashion": 5894, "empl": 5895, "Ġflex": 5896, "Ġkon": 5897, "Ġtruck": 5898, "Ġlect": 5899, "Ġplastic": 5900, "law": 5901, "Ġlikes": 5902, "Ġrough": 5903, "ĠMAT": 5904, "íŀĪ": 5905, "Ġcommer": 5906, "Ġasse": 5907, "Ġcake": 5908, "Ġactions": 5909, "Ġadm": 5910, "Ġotherwise": 5911, "ĠHealth": 5912, "Ġcolle": 5913, "à¹Ģà¸": 5914, "Ġrub": 5915, "å¾Ĺ": 5916, "æĶ": 5917, "Ġscr": 5918, "Ġzum": 5919, "ĠHim": 5920, "Ġchamp": 5921, "Ġconcerned": 5922, "Ġ500": 5923, "Ġplate": 5924, "ĠOut": 5925, "Ġdonc": 5926, "Ġequipment": 5927, "Ġtaught": 5928, "lled": 5929, "ĠíĻ": 5930, "iva": 5931, "Ġmotor": 5932, "»": 5933, "Ġguide": 5934, "åī": 5935, "Ġstopped": 5936, "Ġrat": 5937, "Ġlabor": 5938, "Ġaim": 5939, "Ġprepare": 5940, "ĠÑĪ": 5941, "Ġshooting": 5942, "anned": 5943, "cript": 5944, "Ġenemy": 5945, "Ġdepends": 5946, "Ġnav": 5947, "Ġber": 5948, "Ġlands": 5949, "Ġunivers": 5950, "iu": 5951, "Ġfactor": 5952, "oking": 5953, "Ġcarbon": 5954, "but": 5955, "ĠLove": 5956, "eld": 5957, "Ġε": 5958, "Ġga": 5959, "Ġés": 5960, "Ġbread": 5961, "Ġvolt": 5962, "íĬ": 5963, "Ġwaste": 5964, "Ġkeeps": 5965, "æīĢ": 5966, "Ġstor": 5967, "Ġhonor": 5968, "Ġunless": 5969, "Ġcolum": 5970, "ĠëĮĢ": 5971, "Ġplants": 5972, "Yeah": 5973, "Ġincludes": 5974, "ä¸Ń": 5975, "Ġox": 5976, "Ġpeut": 5977, "ë§Į": 5978, "ìĥģ": 5979, "istry": 5980, "ั": 5981, "ĠDepartment": 5982, "anta": 5983, "Ġfinger": 5984, "Ġstretch": 5985, "Ġsymbol": 5986, "Ġneighbor": 5987, "æ¬": 5988, "ê°Ħ": 5989, "~~": 5990, "ĠÑĤÑĭ": 5991, "ĠAber": 5992, "kes": 5993, "Ġmassive": 5994, "ĠCH": 5995, "ĠSal": 5996, "׳": 5997, "ãĤĴ": 5998, "Ġdynam": 5999, "ache": 6000, "ĠPre": 6001, "Ġmonitor": 6002, "ented": 6003, "EO": 6004, "Ġraised": 6005, "istics": 6006, "Ú©": 6007, "Ġvou": 6008, "iten": 6009, "¡°": 6010, "Ġbusinesses": 6011, "Ġearn": 6012, "Ġmobile": 6013, "idade": 6014, "Ġhabe": 6015, "yr": 6016, "lict": 6017, "Ġconduct": 6018, "Ġfederal": 6019, "Ġwo": 6020, "bu": 6021, "Ġnone": 6022, "Ġteachers": 6023, "ĠاÙĦØ": 6024, "éģĵ": 6025, "idents": 6026, "اÙĦ": 6027, "Ġtrend": 6028, "еж": 6029, "Ġalbum": 6030, "Ġmich": 6031, "based": 6032, "ี": 6033, "Ġtransition": 6034, "Ġно": 6035, "ões": 6036, "host": 6037, "edy": 6038, "ĠProf": 6039, "pan": 6040, "ijn": 6041, "Ġcapacity": 6042, "undo": 6043, "Ġ×ij×": 6044, "Ġbreath": 6045, "Ġмен": 6046, "Ġmü": 6047, "íĻ": 6048, "ĠAut": 6049, "hington": 6050, "Ġnor": 6051, "Ġgain": 6052, "point": 6053, "Yes": 6054, "Ġت": 6055, "ĠNa": 6056, "Ã¥r": 6057, "Ġiç": 6058, "ĠMary": 6059, "Ġspin": 6060, "Ġanti": 6061, "åIJ§": 6062, "Ġsomehow": 6063, "Ġlaws": 6064, "Ġmoments": 6065, "Ġgre": 6066, "Ġmoves": 6067, "ĠWould": 6068, "Ġpredict": 6069, "Ġvra": 6070, "Ġ2019": 6071, "¶Ħ": 6072, "Ġfundament": 6073, "25": 6074, "Ġpure": 6075, "Ġwow": 6076, "Ġisland": 6077, "Ġinvestment": 6078, "Ġbath": 6079, "ĠYa": 6080, "Ġharder": 6081, "Ġtips": 6082, "åĹ": 6083, "Ġelectron": 6084, "ĠBob": 6085, "Ġbond": 6086, "odies": 6087, "ĠAug": 6088, "Ġgibt": 6089, "Ġchair": 6090, "Ġtwice": 6091, "wood": 6092, "Ġclar": 6093, "Ġmask": 6094, "Ġhonestly": 6095, "Ġ2018": 6096, "ties": 6097, "',": 6098, "Ġpens": 6099, "Ġsurprised": 6100, "Ġcommunication": 6101, "ãģ£ãģ¦": 6102, "Ġspr": 6103, "Ġwhose": 6104, "Ġstars": 6105, "×IJ×": 6106, "ĠâĢĭ": 6107, "Ġproperly": 6108, "Ġgrew": 6109, "osing": 6110, "Ġdivers": 6111, "AD": 6112, "Ġempt": 6113, "Ġexpression": 6114, "ế": 6115, "ĠPal": 6116, "ãģĬ": 6117, "Ġjustice": 6118, "Ġpair": 6119, "wo": 6120, "Ġseat": 6121, "orter": 6122, "Ġlinks": 6123, "ĠMer": 6124, "Ġrend": 6125, "ное": 6126, "upid": 6127, "ĠHel": 6128, "ĠMarch": 6129, "ĠLo": 6130, "ÑģÑĮ": 6131, "Ġhasn": 6132, "Ġevalu": 6133, "ãģı": 6134, "天": 6135, "ilos": 6136, "Ġfunding": 6137, "Ġven": 6138, "uan": 6139, "ĠMaster": 6140, "ĠOl": 6141, "ĠFre": 6142, "Ġyap": 6143, "ĠSir": 6144, "sch": 6145, "Ġmistake": 6146, "aman": 6147, "Ġdinner": 6148, "ĠWashington": 6149, "Ġorganizations": 6150, "Ġже": 6151, "aving": 6152, "ĠvÃŃ": 6153, "Ġbirthday": 6154, "Ġbear": 6155, "ĠÙģ": 6156, "Ġafford": 6157, "Ġreven": 6158, "Ġrelationships": 6159, "rough": 6160, "ĠTime": 6161, "Ġtag": 6162, "ĠSun": 6163, "uary": 6164, "ĠPo": 6165, "car": 6166, "abilities": 6167, "Ġprison": 6168, "Ġlic": 6169, "ìłķ": 6170, "idden": 6171, "Ġspecies": 6172, "é»": 6173, "Ġfirm": 6174, "Ġscore": 6175, "Ġdit": 6176, "Ġspect": 6177, "Ġpel": 6178, "Ġcomplicated": 6179, "樣": 6180, "Ġrank": 6181, "Ġopposite": 6182, "Ġpicked": 6183, "Ġкон": 6184, "eler": 6185, "Ġmig": 6186, "ĠSl": 6187, "ĠNet": 6188, "Ġneck": 6189, "ĠFrance": 6190, "Ġtechnical": 6191, "ม": 6192, "Ġmiles": 6193, "Ġprimary": 6194, "Ġsein": 6195, "ses": 6196, "Ġlaughs": 6197, "bra": 6198, "ÅĽci": 6199, "riage": 6200, "Ġnic": 6201, "eters": 6202, "Ġê": 6203, "ologies": 6204, "ĠIS": 6205, "rad": 6206, "udo": 6207, "ınd": 6208, "mar": 6209, "Ġexch": 6210, "Ġcompetition": 6211, "Ġaussi": 6212, "ĠServ": 6213, "Ġrent": 6214, "Ġchocolate": 6215, "Ġwieder": 6216, "Ġnearly": 6217, "Ġspeech": 6218, "Ġunc": 6219, "Ġparam": 6220, "ĠBritish": 6221, "Ġremain": 6222, "à¸ģ": 6223, "urt": 6224, "Ġع": 6225, "Ġcrack": 6226, "ails": 6227, "Ġpromise": 6228, "Ġpaying": 6229, "iÃŁ": 6230, "Ġadapt": 6231, "ала": 6232, "Ġmovies": 6233, "Ġwire": 6234, "٬": 6235, "æľĥ": 6236, "Ġterrible": 6237, "Ġsó": 6238, "Ġperfectly": 6239, "åij¢": 6240, "ordin": 6241, "Ġjá": 6242, "Ġimpossible": 6243, "ĠThree": 6244, "Ġnh": 6245, "Ġturning": 6246, "rum": 6247, "ĠBel": 6248, "igg": 6249, "Ġresponsible": 6250, "ий": 6251, "Ġincredibly": 6252, "wi": 6253, "iano": 6254, "Ġhumans": 6255, "ĠÃĩ": 6256, "Ġsettings": 6257, "Ġjoy": 6258, "oot": 6259, "Ġdealing": 6260, "illed": 6261, "Ġsurround": 6262, "Ġfollowed": 6263, "Ġpossibly": 6264, "Ġiniti": 6265, "sten": 6266, "Ġpros": 6267, "Ġcandid": 6268, "Ġassign": 6269, "Ġviolence": 6270, "Well": 6271, "Ġrise": 6272, "PS": 6273, "Ġtambém": 6274, "Ġëĵ¤": 6275, "iance": 6276, "yan": 6277, "Ġaudio": 6278, "ĠBet": 6279, "ĠAmericans": 6280, "ĠAss": 6281, "ischen": 6282, "ìŀħ": 6283, "Ġultimately": 6284, "Ġpolic": 6285, "Ġmajority": 6286, "éĢĻåĢĭ": 6287, "ĠFinally": 6288, "erap": 6289, "Ġguard": 6290, "ĠMATT": 6291, "Ġbrown": 6292, "ми": 6293, "Ġcha": 6294, "ĠHoly": 6295, "Ġnervous": 6296, "ipping": 6297, "ÄĻd": 6298, "ĠSa": 6299, "ĵľë": 6300, "¶Ģ": 6301, "lie": 6302, "羣": 6303, "Ġnuc": 6304, "ĠApr": 6305, "éĽ": 6306, "ĠKorea": 6307, "ego": 6308, "ĠCanada": 6309, "Ġkönnen": 6310, "Ġcompar": 6311, "Ġganz": 6312, "ĠMais": 6313, "Ġtheme": 6314, "Ġki": 6315, "Ġdrawing": 6316, "azon": 6317, "ĠOff": 6318, "tt": 6319, "ĠWind": 6320, "Ġtodos": 6321, "Ġobvious": 6322, "наÑı": 6323, "IM": 6324, "ĠÐł": 6325, "well": 6326, "Ġblow": 6327, "Ġhook": 6328, "Ġcircle": 6329, "Ġë³´": 6330, "Ġarchitect": 6331, "ĠKr": 6332, "Ġcó": 6333, "Ġprotection": 6334, "ega": 6335, "åĩ": 6336, "Ġwatched": 6337, "Ġanswers": 6338, "Ġdiet": 6339, "ivo": 6340, "Ġpowder": 6341, "Ġyours": 6342, "Ġhighest": 6343, "çĤº": 6344, "FF": 6345, "åº": 6346, "Ġboys": 6347, "öyle": 6348, "Ġlunch": 6349, "è¬Ŀ": 6350, "ĠII": 6351, "Ġsets": 6352, "Ġmole": 6353, "Ûģ": 6354, "Ġwinter": 6355, "Ġlucky": 6356, "Ġresponsibility": 6357, "Ġsignal": 6358, "Ġwondering": 6359, "Ġax": 6360, "Ġcooking": 6361, "овоÑĢ": 6362, "leg": 6363, "ĠпоÑĤ": 6364, "Ġsurprise": 6365, "Ġdemocr": 6366, "Ġloop": 6367, "Ġjag": 6368, "Ġcurious": 6369, "Ġmarketing": 6370, "ÐĿ": 6371, "aron": 6372, "ĠApple": 6373, "Ġvirtual": 6374, "Ġ198": 6375, "noon": 6376, "ĠMet": 6377, "оÑģÑĤо": 6378, "обÑĭ": 6379, "itu": 6380, "ĠAw": 6381, "Ġbuying": 6382, "Ġrestaurant": 6383, "ĠBud": 6384, "Ġdoubt": 6385, "Ġgrant": 6386, "Ġverd": 6387, "Ġcash": 6388, "Ġfaculty": 6389, "That": 6390, "ĠEin": 6391, "å¤ļ": 6392, "Ġwed": 6393, "itness": 6394, "ĠMag": 6395, "nel": 6396, "Ġnarr": 6397, "Ġaccident": 6398, "Ġmedium": 6399, "ements": 6400, "Ġcrow": 6401, "night": 6402, "ìĿ¼": 6403, "ä¹Ł": 6404, "Ġlibrary": 6405, "аÑİÑĤ": 6406, "Ġtambién": 6407, "Ġreference": 6408, "Ġfourth": 6409, "house": 6410, "vention": 6411, "Ġfilled": 6412, "ĠCour": 6413, "ibr": 6414, "Ġng": 6415, "Ġdeveloping": 6416, "Ġprovides": 6417, "Ġpoll": 6418, "Ġtraffic": 6419, "arently": 6420, "à®Ł": 6421, "Ġforms": 6422, "Ġclient": 6423, "Ġgentle": 6424, "Ġmuss": 6425, "ĠCongress": 6426, "ĠIndian": 6427, "cean": 6428, "Ġpil": 6429, "Ġczy": 6430, "stood": 6431, "uty": 6432, "Ġnä": 6433, "Ġspending": 6434, "Ġconstruction": 6435, "inaudible": 6436, "Ġë§Ī": 6437, "Ī무": 6438, "ĠìĥĿ": 6439, "oma": 6440, "osen": 6441, "ago": 6442, "Ġlargest": 6443, "ãħĭãħĭ": 6444, "Ġuniverse": 6445, "bes": 6446, "osa": 6447, "Ġего": 6448, "Ġdude": 6449, "ĠMAR": 6450, "Ġindeed": 6451, "ει": 6452, "Ġmanaged": 6453, "ĠShould": 6454, "So": 6455, "Ġapplied": 6456, "Ġfairly": 6457, "ĠDen": 6458, "Ġanaly": 6459, "Ġconstantly": 6460, "Ñģп": 6461, "How": 6462, "ĠSay": 6463, "encies": 6464, "ĠPC": 6465, "Ġeggs": 6466, "à®°": 6467, "Ġeth": 6468, "ĠEntão": 6469, "inar": 6470, "iot": 6471, "Ġcz": 6472, "ĠEuropean": 6473, "ãģĪ": 6474, "ĠAM": 6475, "Ġcá": 6476, "Ġradio": 6477, "§Į": 6478, "Ġhide": 6479, "ä»Ĭ": 6480, "ĠStart": 6481, "Ġclub": 6482, "ĠHope": 6483, "Ġefforts": 6484, "lusion": 6485, "Ġcities": 6486, "hone": 6487, "Ġreached": 6488, "Ġguid": 6489, "roid": 6490, "Ġharm": 6491, "Ġcutting": 6492, "Ġbul": 6493, "18": 6494, "iest": 6495, "ĠMex": 6496, "Ġiron": 6497, "çŁ¥": 6498, "Ġafternoon": 6499, "Ġhall": 6500, "Ġprzy": 6501, "Ġgosh": 6502, "Ġinfluence": 6503, "Ġвид": 6504, "Ġincreased": 6505, "ĠMinister": 6506, "Ġdisci": 6507, "ĠPeter": 6508, "Ġvert": 6509, "Ġmenu": 6510, "Ġselling": 6511, "urally": 6512, "Ġquote": 6513, "Ġ¡": 6514, "Ġcontinues": 6515, "mpre": 6516, "ĠÅŁey": 6517, "itution": 6518, "ĠнаÑģ": 6519, "cles": 6520, "ĠGerman": 6521, "czy": 6522, "ĠУ": 6523, "Be": 6524, "Ġkitchen": 6525, "ĠTry": 6526, "ipe": 6527, "Ġicon": 6528, "arp": 6529, "Ġproviding": 6530, "ĠTrans": 6531, "Ġtechnique": 6532, "Ġhär": 6533, "Ġinfrast": 6534, "Ġsusp": 6535, "ück": 6536, "icip": 6537, "ĠÐķ": 6538, "Ġcin": 6539, "ìĸ´ë": 6540, "Ġprz": 6541, "Ġcomponent": 6542, "Ġbye": 6543, "ĠBible": 6544, "izer": 6545, "Ch": 6546, "Ġsolutions": 6547, "Ġaccompl": 6548, "Ġ2016": 6549, "IE": 6550, "ĠTa": 6551, "Ġassume": 6552, "Ġliquid": 6553, "Ġ먹": 6554, "Ġquarter": 6555, "Ġfemale": 6556, "ĠThink": 6557, "Ġstatus": 6558, "itute": 6559, "Ġcoach": 6560, "Ġrein": 6561, "Ġcombination": 6562, "è·": 6563, "ĠTer": 6564, "Ġobjects": 6565, "Ġdistrict": 6566, "Ġmakeup": 6567, "Ġmurder": 6568, "was": 6569, "fen": 6570, "Ġbowl": 6571, "Ġpublished": 6572, "Ġsports": 6573, "ãģ¡": 6574, "Ġidentity": 6575, "Ġseemed": 6576, "Ġacting": 6577, "лÑİ": 6578, "rix": 6579, "Ġupload": 6580, "Ġhast": 6581, "Ġboat": 6582, "ĠMod": 6583, "rio": 6584, "Ġ=": 6585, "Ġcycle": 6586, "¯¸": 6587, "Ġloud": 6588, "usted": 6589, "coming": 6590, "Ġ2017": 6591, "Ġont": 6592, "Ġlegisl": 6593, "Ġstruct": 6594, "ĠSomething": 6595, "Ġconflict": 6596, "Ġupper": 6597, "Ġmanager": 6598, "Ġmort": 6599, "Ġfra": 6600, "Ġİ": 6601, "ĠMike": 6602, "ĠWork": 6603, "Ġnó": 6604, "phere": 6605, "ĠìĤ¬ë": 6606, "ĠLand": 6607, "Ġfilter": 6608, "Ġpromot": 6609, "æ°": 6610, "æĻĤ": 6611, "ķ¼": 6612, "Ġrecording": 6613, "×Ŀ": 6614, "Ġassociated": 6615, "Ġfuel": 6616, "under": 6617, "Ġelection": 6618, "Ġemployees": 6619, "ĠComp": 6620, "ÑĢÑĥг": 6621, "ĠWo": 6622, "rol": 6623, "Ġsaved": 6624, "ĠHon": 6625, "ĠVi": 6626, "åĪĨ": 6627, "aca": 6628, "pret": 6629, "Ġwet": 6630, "Ġstupid": 6631, "Ġlad": 6632, "Ġfest": 6633, "Ġwake": 6634, "Ġин": 6635, "Ġgreatest": 6636, "ĠJim": 6637, "Ġseriously": 6638, "Ġì¹": 6639, "Ġfeelings": 6640, "Ġ300": 6641, "iation": 6642, "Ġbeauty": 6643, "Ġìŀĺ": 6644, "Ġsan": 6645, "ĵł": 6646, "Ġ-(": 6647, "Ġconscious": 6648, "Ġдел": 6649, "bye": 6650, "çĻ": 6651, "Man": 6652, "Ġlets": 6653, "Ġshoes": 6654, "yd": 6655, "ä¹Ī": 6656, "Ġdisappe": 6657, "ĠCounty": 6658, "ĠScott": 6659, "Ġbutt": 6660, "ĠaquÃŃ": 6661, "Ġconfig": 6662, "respond": 6663, "LAUGH": 6664, "©ëĭĪëĭ¤": 6665, "Ġdivided": 6666, "Ġacqu": 6667, "Ġzone": 6668, "Ġkomm": 6669, "ação": 6670, "ì§ľ": 6671, "cut": 6672, "Ġ23": 6673, "Ġmaximum": 6674, "rog": 6675, "Ġruns": 6676, "Ġcomponents": 6677, "Ġarrived": 6678, "Ġconfident": 6679, "ÑĢов": 6680, "Ġheight": 6681, "Ġproced": 6682, "EM": 6683, "ĠÐŃÑĤо": 6684, "ĠMen": 6685, "Ġtalks": 6686, "Ġconfidence": 6687, "ĠChris": 6688, "Ġleads": 6689, "Ġnose": 6690, "fall": 6691, "bb": 6692, "ĠNothing": 6693, "iser": 6694, "Ġindependent": 6695, "Ġminor": 6696, "Ġsym": 6697, "len": 6698, "cience": 6699, "Ġfashion": 6700, "Ġsexual": 6701, "Ġbun": 6702, "here": 6703, "Ġsoil": 6704, "Ġdiese": 6705, "Ġshap": 6706, "Ġempty": 6707, "Ġjournal": 6708, "agon": 6709, "ĠTheir": 6710, "Ġweekend": 6711, "ÃŃt": 6712, "Ġerror": 6713, "Ġnar": 6714, "ø": 6715, "è©": 6716, "ancy": 6717, "ĠìķĬ": 6718, "Ġforest": 6719, "Ġhacer": 6720, "Ġmissed": 6721, "ãģķ": 6722, "åı¯ä»¥": 6723, "Ġevil": 6724, "Ġstorage": 6725, "Ġsinging": 6726, "inha": 6727, "Ġknock": 6728, "Ġimpress": 6729, "ĠоÑĩенÑĮ": 6730, "ĠGold": 6731, "ĠSur": 6732, "ĠPort": 6733, "åİ»": 6734, "ĠLond": 6735, "Ġfazer": 6736, "oty": 6737, "oto": 6738, "Ġanx": 6739, "ĠWilliam": 6740, "Ġexisting": 6741, "place": 6742, "ĠCD": 6743, "γ": 6744, "ĠCollege": 6745, "lor": 6746, "ĠEast": 6747, "sen": 6748, "fach": 6749, "oft": 6750, "Ġexperienced": 6751, "Ġloves": 6752, "imm": 6753, "Ġpoly": 6754, "Ġesse": 6755, "ì¤": 6756, "ĠGrand": 6757, "è§": 6758, "cher": 6759, "Ġvictim": 6760, "ĠGes": 6761, "лÑĮ": 6762, "vision": 6763, "Ġtall": 6764, "Ġlens": 6765, "Ġзна": 6766, "ĠBoth": 6767, "Ġì²": 6768, "Ġsustain": 6769, "Ġargument": 6770, "Ġfactors": 6771, "Ġautomatically": 6772, "Ġfruit": 6773, "Ġliber": 6774, "Ġale": 6775, "ĠPress": 6776, "ĠBa": 6777, "Ġго": 6778, "Ġhundreds": 6779, "that": 6780, "ĠRich": 6781, "Ġrecipe": 6782, "ĠIT": 6783, "èĩ": 6784, "ấ": 6785, "Ġdescribe": 6786, "Ġdriver": 6787, "ĠOct": 6788, "ĠMat": 6789, "де": 6790, "Ġmeal": 6791, "Ġlatest": 6792, "Ġtherap": 6793, "Ġcompare": 6794, "ĠAmazon": 6795, "Ġì¢Ģ": 6796, "ĠRussia": 6797, "Ġstring": 6798, "Ġka": 6799, "ĠCommun": 6800, "Ġdia": 6801, "Is": 6802, "Ġmillions": 6803, "Ġcorpor": 6804, "Ġcorrespond": 6805, "Ġfixed": 6806, "ĠJoe": 6807, "Ùİ": 6808, "Ġviews": 6809, "Ġriver": 6810, "Ġstudio": 6811, "igger": 6812, "Ġflavor": 6813, "Ġpresence": 6814, "Ġunits": 6815, "Ġsaving": 6816, "avour": 6817, "Ġpesso": 6818, "orith": 6819, "Ġhers": 6820, "ĠNat": 6821, "asion": 6822, "ĠFrank": 6823, "оÑĪ": 6824, "ÅĤy": 6825, "íĦ": 6826, "Ġeinem": 6827, "Ġfunctions": 6828, "uman": 6829, "Ġnorth": 6830, "ĠìłĦ": 6831, "Ġhorse": 6832, "vid": 6833, "Ġpleasure": 6834, "аÑĪ": 6835, "ées": 6836, "inda": 6837, "Ġtail": 6838, "Ġexplore": 6839, "ST": 6840, "Ġcommercial": 6841, "ĠDuring": 6842, "arl": 6843, "]:": 6844, "fit": 6845, "Ġrates": 6846, "æ³": 6847, "MUSIC": 6848, "Ġhousing": 6849, "Ġeiner": 6850, "Ġsituations": 6851, "æĭ": 6852, "Ġdecre": 6853, "Ġappropriate": 6854, "енно": 6855, "%.": 6856, "Ġbac": 6857, "Ġwat": 6858, "ensity": 6859, "äh": 6860, "known": 6861, "itz": 6862, "Ġemotional": 6863, "ervation": 6864, "Ġblind": 6865, "16": 6866, "íĥ": 6867, "大家": 6868, "Ġjoined": 6869, "Ġlocated": 6870, "ĠÑģм": 6871, "adas": 6872, "berg": 6873, "Ġdess": 6874, "Ġdear": 6875, "eden": 6876, "cos": 6877, "Ġadopt": 6878, "100": 6879, "owe": 6880, "ĠCheck": 6881, "ismo": 6882, "Ġsimpl": 6883, "Ġangry": 6884, "ĠменÑı": 6885, "ĠCam": 6886, "Ġpad": 6887, "Ġattend": 6888, "Ġsample": 6889, "æĹ¥": 6890, "ĠìĽ": 6891, "ĠIN": 6892, "ulous": 6893, "ĠSar": 6894, "ĠShow": 6895, "Ġinfrastructure": 6896, "ĠAugust": 6897, "Ġlesson": 6898, "Ġniet": 6899, "æİ": 6900, "Ġfoi": 6901, "Ġbroke": 6902, "tr": 6903, "çķ": 6904, "Ġ45": 6905, "Ġgew": 6906, "Ñĥп": 6907, "ati": 6908, "Ġmaintain": 6909, "Ġartists": 6910, "inger": 6911, "æĿ¥": 6912, "erved": 6913, "IA": 6914, "Ġequals": 6915, "Ġoperation": 6916, "illy": 6917, "ĠëĤ´": 6918, "Ġcrowd": 6919, "Ġinternal": 6920, "Ġtests": 6921, "ĠRock": 6922, "ĠCons": 6923, "ĠëĦĪ무": 6924, "war": 6925, "Ġsou": 6926, "Ġchart": 6927, "ĠJune": 6928, "ĠApril": 6929, "gent": 6930, "Ġvent": 6931, "Ġquand": 6932, "ĠKorean": 6933, "imo": 6934, "çī": 6935, "iders": 6936, "Ġmountain": 6937, "ÑģÑĤав": 6938, "æľĪ": 6939, "ijk": 6940, "Ġdiscovered": 6941, "ĠSund": 6942, "ĠSil": 6943, "Ġsolo": 6944, "´": 6945, "Ġschol": 6946, "ĠEach": 6947, "çµ": 6948, "Ġbare": 6949, "ĠíĮ": 6950, "ĠvÃŃde": 6951, "Ġingredients": 6952, "ĠIts": 6953, "Ŀ¼ê³ł": 6954, "ĠìĬ": 6955, "Ïį": 6956, "ĠLee": 6957, "Ġscary": 6958, "Ġprincip": 6959, "Ġspiritual": 6960, "ìħ": 6961, "ĠHold": 6962, "æ²Ĵæľī": 6963, "Ġdefine": 6964, "ĠLes": 6965, "ĠNor": 6966, "ĠEnd": 6967, "Ġblog": 6968, "ĠGreen": 6969, "аеÑĤÑģÑı": 6970, "part": 6971, "eles": 6972, "äºĭ": 6973, "ĠUnder": 6974, "Ġparte": 6975, "Ġ35": 6976, "Ġsector": 6977, "ĠSept": 6978, "Ġauth": 6979, "à®®": 6980, "omin": 6981, "Ġclients": 6982, "Ġci": 6983, "ĠFriday": 6984, "eras": 6985, "Ġtwe": 6986, "ulated": 6987, "Ġcultural": 6988, "ĠÑģво": 6989, "ĠëįĶ": 6990, "Ġú": 6991, "Ġparce": 6992, "ல": 6993, "Ġtradition": 6994, "Ġjudge": 6995, "ĠGeneral": 6996, "Ġdetermine": 6997, "ĠIsn": 6998, "ĠPL": 6999, "neath": 7000, "Ġmatters": 7001, "íķ´ì": 7002, "!]": 7003, "аÑħ": 7004, "Ġpool": 7005, "Ġvariable": 7006, "Ġvaccine": 7007, "Ġcaused": 7008, "Ġwest": 7009, "ĠYep": 7010, "fast": 7011, "Ġphilos": 7012, "hora": 7013, "Ġcontinued": 7014, "Ġunfortunately": 7015, "ãģį": 7016, "æķ": 7017, "Ġflight": 7018, "Ġwrap": 7019, "Ġhuh": 7020, "ĠAbsolutely": 7021, "Ġpink": 7022, "Ġremains": 7023, "Ġné": 7024, "Ġfle": 7025, "ĠSol": 7026, "Ġlosing": 7027, "Ġalgorith": 7028, "Ġrequires": 7029, "Ġfoundation": 7030, "ĠBur": 7031, "Ġprofession": 7032, "ĠMid": 7033, "ĠëŃIJ": 7034, "can": 7035, "ĠMil": 7036, "Ġyounger": 7037, "Ġappears": 7038, "term": 7039, "íķĺê³ł": 7040, "acle": 7041, "ĠLondon": 7042, "Ġengineering": 7043, "ย": 7044, "Ġadvent": 7045, "ìĦ¸ìļĶ": 7046, "Ġ기": 7047, "ĠMaj": 7048, "ÑĢем": 7049, "ingu": 7050, "ĠUK": 7051, "uro": 7052, "spe": 7053, "Ġtent": 7054, "Ġreported": 7055, "ĠAL": 7056, "Hey": 7057, "Ġë§IJ": 7058, "Ġdent": 7059, "ĠAustralia": 7060, "ĠJanuary": 7061, "³´": 7062, "agues": 7063, "arsh": 7064, "rig": 7065, "Ġtiene": 7066, "ร": 7067, "ή": 7068, "Ġmachen": 7069, "unte": 7070, "ÑĥÑģ": 7071, "Ġelectr": 7072, "Ġtutorial": 7073, "Ġplaced": 7074, "ĠìĿ´ê±°": 7075, "ĠCouncil": 7076, "íĸĪ": 7077, "°ë¦¬": 7078, "ahren": 7079, "Ġê·¸ëŀĺ": 7080, "Ġprove": 7081, "fol": 7082, "Ġquer": 7083, "Ġcheap": 7084, "ĠFather": 7085, "ĠPower": 7086, "ĵľ": 7087, "Ġpurs": 7088, "Ġesp": 7089, "ĠBre": 7090, "기ë": 7091, "omas": 7092, "æĥ³": 7093, "илÑĮ": 7094, "Ġgeht": 7095, "oster": 7096, "ê³¼": 7097, "Ġfiles": 7098, "ĠЧ": 7099, "bell": 7100, "Ġwhom": 7101, "Ġëĺ": 7102, "Ġexcellent": 7103, "Ġdatab": 7104, "Ġgö": 7105, "Ġì§Ħì§ľ": 7106, "Ġbelief": 7107, "jet": 7108, "Ġjack": 7109, "Ġswim": 7110, "rial": 7111, "umin": 7112, "auc": 7113, "Ġsoll": 7114, "Ġessential": 7115, "íķĺëĬĶ": 7116, "Ġevol": 7117, "chaft": 7118, "aine": 7119, "thlet": 7120, "Ġincor": 7121, "Ġreports": 7122, "Ġdefinition": 7123, "kel": 7124, "Ġcircum": 7125, "Ġproduced": 7126, "Ġ׼": 7127, "antic": 7128, "net": 7129, "Ġaward": 7130, "Ġdurch": 7131, "Ġtransp": 7132, "Ġmale": 7133, "¦¬ë": 7134, "Ġmoon": 7135, "ĠGeorge": 7136, "Ġflying": 7137, "ió": 7138, "Ġsources": 7139, "Ġplenty": 7140, "ĠDemocr": 7141, "RO": 7142, "Ġ00": 7143, "Ġsecure": 7144, "ĠBir": 7145, "rain": 7146, "Ġzur": 7147, "Ġefficient": 7148, "Ġrepeat": 7149, "Ġmethods": 7150, "Ġcalm": 7151, "Ġdiscussed": 7152, "ĠìŀĪëĬĶ": 7153, "Ġserver": 7154, "anie": 7155, "ĠInstead": 7156, "Ġideal": 7157, "Ġconven": 7158, "Ġhoping": 7159, "ĠTor": 7160, "Ġdepth": 7161, "Ġheaven": 7162, "ENCE": 7163, "Ġhabit": 7164, "grad": 7165, "Ġflag": 7166, "Ġine": 7167, "Ġkh": 7168, "ĠLI": 7169, "Ġfacing": 7170, "ĠAU": 7171, "ĠTim": 7172, "Ġgem": 7173, "ĠJul": 7174, "Ġela": 7175, "izza": 7176, "Ġfellow": 7177, "Ġquel": 7178, "Ġspoke": 7179, "Ġcitizens": 7180, "uge": 7181, "éĥ½": 7182, "Ġpages": 7183, "Ġfasc": 7184, "Ġreligious": 7185, "aten": 7186, "Ġchapter": 7187, "ĠVal": 7188, "Ġconsult": 7189, "ĠMill": 7190, "gl": 7191, "oper": 7192, "Ġinfin": 7193, "Ġmarriage": 7194, "Ġmedicine": 7195, "Ġдв": 7196, "Ġdogs": 7197, "Ġinstrument": 7198, "ĠExact": 7199, "án": 7200, "Ġ2021": 7201, "Ġfer": 7202, "Ġwealth": 7203, "Ġgrade": 7204, "ÑĭÑħ": 7205, "Ġcrime": 7206, "Ġthread": 7207, "Ġessa": 7208, "Ġwine": 7209, "cohol": 7210, "pha": 7211, "à¸ĩ": 7212, "ogue": 7213, "Ġinsurance": 7214, "arrator": 7215, "ĠSeptember": 7216, "Ġvid": 7217, "ĠSpirit": 7218, "Ġgest": 7219, "ĠRussian": 7220, "Ġproperties": 7221, "Ġarticle": 7222, "Ġunderneath": 7223, "yer": 7224, "Ġjoint": 7225, "Ġrelatively": 7226, "Ġinch": 7227, "Ġdespite": 7228, "ĠGree": 7229, "Ġclassic": 7230, "Ġsupporting": 7231, "Ġinstruct": 7232, "lusive": 7233, "Ġdiagn": 7234, "æĬ": 7235, "Ġadministration": 7236, "абоÑĤ": 7237, "ĠOpen": 7238, "æīĢ以": 7239, "Ġпок": 7240, "Ġdollar": 7241, "Ġconsequ": 7242, "ober": 7243, "ĠGermany": 7244, "Ġterr": 7245, "ĠQU": 7246, "ĠÐĵ": 7247, "ç¾": 7248, "Ġstronger": 7249, "ÉĻ": 7250, "ĠÙĬ": 7251, "ĠiPhone": 7252, "Ġfabric": 7253, "üh": 7254, "Ġenem": 7255, "æ¯": 7256, "Ġsubt": 7257, "EE": 7258, "onde": 7259, "Ġcrew": 7260, "Ġremoved": 7261, "Ġlady": 7262, "Ġpotentially": 7263, "ĠÐĿо": 7264, "yal": 7265, "Ġsympt": 7266, "Ġarmy": 7267, "Ġintroduced": 7268, "tes": 7269, "Ġaspects": 7270, "14": 7271, "ĠLou": 7272, "Ġ)": 7273, "Ġdeploy": 7274, "pet": 7275, "Ġhan": 7276, "ĠWatch": 7277, "Ġweapons": 7278, "Ġphen": 7279, "Ġregister": 7280, "Ġeinfach": 7281, "Ġsport": 7282, "Ġbridge": 7283, "Ġinner": 7284, "Ġminimum": 7285, "Ġwitness": 7286, "Ġeso": 7287, "Ġvillage": 7288, "Ġowner": 7289, "¦¬ê³ł": 7290, "Ġscream": 7291, "iled": 7292, "Ġpitch": 7293, "bru": 7294, "Ġadvance": 7295, "ä¸įæĺ¯": 7296, "Ġsuppose": 7297, "ĠAtt": 7298, "еÑĤÑģÑı": 7299, "Ġdifferences": 7300, "aked": 7301, "Ġinterpret": 7302, "æ": 7303, "iendo": 7304, "Ġabsol": 7305, "ĠбÑĥдеÑĤ": 7306, "Ġë²": 7307, "Ġtrial": 7308, "Ġthinks": 7309, "lying": 7310, "ception": 7311, "ĠAfrican": 7312, "Ġchemical": 7313, "Ġtape": 7314, "Ġconversations": 7315, "Ġdistribution": 7316, "ti": 7317, "ĠAI": 7318, "Ġflash": 7319, "Ġunderstood": 7320, "ĠGovernment": 7321, "å°ı": 7322, "!?": 7323, "ĠSk": 7324, "ê±°ë": 7325, "rier": 7326, "TS": 7327, "ĠAccording": 7328, "ÑİÑĤ": 7329, "Ġspons": 7330, "ÑĤобÑĭ": 7331, "Ġvalu": 7332, "erem": 7333, "ichtig": 7334, "Ġresistance": 7335, "ĠGal": 7336, "gery": 7337, "Ġbegins": 7338, "Ġadvanced": 7339, "Ġrelevant": 7340, "Ġpolitics": 7341, "ĠFam": 7342, "Ġçok": 7343, "ĠNever": 7344, "illing": 7345, "Ġfootball": 7346, "ии": 7347, "ĠID": 7348, "ĠAfrica": 7349, "Ġfingers": 7350, "ĠболÑĮ": 7351, "Ġá": 7352, "Ġclip": 7353, "ĠLat": 7354, "ãĤĦ": 7355, "Ġì§Ģê¸Ī": 7356, "esse": 7357, "Ġvoor": 7358, "Ġaside": 7359, "æŀ": 7360, "Ġtoward": 7361, "Ġbat": 7362, "Ġvalid": 7363, "ĠMens": 7364, "Ġcompleted": 7365, "ıģ": 7366, "Ġpodcast": 7367, "ĠBon": 7368, "ÛĴ": 7369, "ĠJuly": 7370, "ila": 7371, "Ġpackage": 7372, "Ġpulled": 7373, "char": 7374, "ĠMel": 7375, "ois": 7376, "Ġsouth": 7377, "ĠëĶ": 7378, "Ġimportance": 7379, "Ġpushing": 7380, "Ġisol": 7381, "Ġstands": 7382, "cill": 7383, "ä¼": 7384, "ĠðŁ": 7385, "ori": 7386, "ê°ģ": 7387, "Ġhomes": 7388, "Ġconcerns": 7389, "Ġbiz": 7390, "å½": 7391, "bie": 7392, "Ġbis": 7393, "Ġgear": 7394, "ĠMS": 7395, "Ġhun": 7396, "ĠMatt": 7397, "ả": 7398, "sey": 7399, "ĠSecret": 7400, "Ġodd": 7401, "ĠMax": 7402, "olly": 7403, "ford": 7404, "ĠSH": 7405, "Ġreplace": 7406, "Ġnavig": 7407, "Ġini": 7408, "иÑı": 7409, "Ġgiant": 7410, "Ġmand": 7411, "ĠHapp": 7412, "TION": 7413, "gun": 7414, "iamo": 7415, "ìŀħëĭĪëĭ¤": 7416, "Ġgap": 7417, "Ġêtre": 7418, "Ġclassroom": 7419, "Ġhyp": 7420, "aki": 7421, "è®": 7422, "isters": 7423, "acks": 7424, "ĠÑģо": 7425, "Ġbug": 7426, "Ġgrav": 7427, "amin": 7428, "Ġeveryday": 7429, "Ġì¡°": 7430, "Ġgarden": 7431, "cember": 7432, "Ġesto": 7433, "åĹİ": 7434, "ج": 7435, "ٰ": 7436, "åģ": 7437, "Ġrom": 7438, "Ġìłľê°Ģ": 7439, "Ġfalling": 7440, "Ġfault": 7441, "elly": 7442, "Ġchest": 7443, "Ġли": 7444, "Ġpotato": 7445, "Ġbuildings": 7446, "Ġoperating": 7447, "Ġpare": 7448, "wr": 7449, "Don": 7450, "ĠFour": 7451, "Ġvul": 7452, "Ġlá": 7453, "Ġfrust": 7454, "ĠDann": 7455, "oles": 7456, "nya": 7457, "Ġì¶": 7458, "ĠÑĢаÑģ": 7459, "׼": 7460, "ĠaÃŃ": 7461, "word": 7462, "Ġweapon": 7463, "Ġobt": 7464, "ĠFall": 7465, "ĠSteve": 7466, "Ġmixed": 7467, "Ġpode": 7468, "ĠAS": 7469, "ĠLeg": 7470, "Ġdesc": 7471, "Ġsplit": 7472, "Ġemergency": 7473, "ĠSing": 7474, "Ġprofit": 7475, "Ġtypical": 7476, "ĠDonc": 7477, "Ġannounce": 7478, "ĠTex": 7479, "Ġsacr": 7480, "ternal": 7481, "Ġcommittee": 7482, "igo": 7483, "Ġdiam": 7484, "phas": 7485, "Ġdefe": 7486, "ĠProfess": 7487, "Ġdecl": 7488, "ÑĥÑĢ": 7489, "22": 7490, "olf": 7491, "ĠMond": 7492, "uy": 7493, "Ġay": 7494, "Ġlem": 7495, "Ġlovely": 7496, "ĠCould": 7497, "Ġguar": 7498, "HH": 7499, "Ġcarefully": 7500, "ĠListen": 7501, "ĠкÑĢ": 7502, "Ġyouth": 7503, "ĠTherefore": 7504, "Ġdreams": 7505, "ĠJeff": 7506, "?]": 7507, "ĠëĪ": 7508, "DA": 7509, "Ġbodies": 7510, "aux": 7511, "Ġtechniques": 7512, "Ġmechanism": 7513, "×ĵ": 7514, "Ġони": 7515, "Ġdesire": 7516, "î": 7517, "ĠVo": 7518, "ques": 7519, "ĠÑĥже": 7520, "ĠWhoa": 7521, "ĠGame": 7522, "Ġhal": 7523, "anish": 7524, "Ġpractices": 7525, "500": 7526, "Ġsorts": 7527, "ups": 7528, "ateful": 7529, "Ġherself": 7530, "Ġguitar": 7531, "Ġpropos": 7532, "Ġsites": 7533, "Ġbeach": 7534, "Ġ×¢": 7535, "第": 7536, "нÑĥ": 7537, "Ġdram": 7538, "ĠNove": 7539, "VE": 7540, "rant": 7541, "Ġplot": 7542, "ĠìĹ¬ê¸°": 7543, "ĠCa": 7544, "Ġestablished": 7545, "Ġ2015": 7546, "Ġinspired": 7547, "Ġannounced": 7548, "个": 7549, "ĠÑĤÑĢ": 7550, "Ġ26": 7551, "Ġvoy": 7552, "Ġtech": 7553, "ìłģ": 7554, "Ġprocesses": 7555, "onto": 7556, "ĠPan": 7557, "Ġrapid": 7558, "istan": 7559, "Ġ197": 7560, "Ġreligion": 7561, "Ġ28": 7562, "Ġsmile": 7563, "Ġbab": 7564, "ĠÚ©": 7565, "ĠVir": 7566, "Ġschedule": 7567, "Ġexecut": 7568, "Ġpron": 7569, "Ñį": 7570, "ĠÐĿÑĥ": 7571, "music": 7572, "ìĽIJ": 7573, "Ġgan": 7574, "ìĭł": 7575, "Ġdefault": 7576, "Ġbem": 7577, "Ùī": 7578, "Ġforced": 7579, "ĠObviously": 7580, "Ġstone": 7581, "Ġtie": 7582, "Ġdrinking": 7583, "Ġserved": 7584, "Cause": 7585, "Ġconference": 7586, "ĠExactly": 7587, "ãĥĪ": 7588, "łľ": 7589, "ìĻĢ": 7590, "ĠRa": 7591, "Ġfake": 7592, "Ġdiff": 7593, "ãģ©": 7594, "Ġchallenging": 7595, "Ġì¤ij": 7596, "Ïĩ": 7597, "ä»Ģ麼": 7598, "Ġintelligence": 7599, "rete": 7600, "Ġstudying": 7601, "Ġappoint": 7602, "Ġtan": 7603, "Ġим": 7604, "Ġcurve": 7605, "ĠTeam": 7606, "ĠAz": 7607, "Ġзд": 7608, "ĠMusic": 7609, "field": 7610, "iration": 7611, "Ġfailed": 7612, "Ġnovel": 7613, "Ġdifferently": 7614, "Ġescape": 7615, "ĠYo": 7616, "ĠOctober": 7617, "ıyor": 7618, "Ġdescribed": 7619, "Ġconvert": 7620, "acement": 7621, "Ġhotel": 7622, "isation": 7623, "Ġsuis": 7624, "ãģij": 7625, "åŃIJ": 7626, "æĢİ": 7627, "Ġwalked": 7628, "200": 7629, "Ġneighborhood": 7630, "isp": 7631, "ĠLos": 7632, "Ġhidden": 7633, "Ġ27": 7634, "ле": 7635, "Ġphr": 7636, "ĠIsland": 7637, "ĠStreet": 7638, "enda": 7639, "hips": 7640, "osure": 7641, "Ġdefined": 7642, "ว": 7643, "Ġvida": 7644, "Ġlabel": 7645, "ĠEverybody": 7646, "Ġjoke": 7647, "iao": 7648, "اÙĨ": 7649, "Ġathlet": 7650, "...\"": 7651, "ĠFire": 7652, "Do": 7653, "Ġdefense": 7654, "Ġentertain": 7655, "át": 7656, "Ġpolicies": 7657, "Ġalcohol": 7658, "ĠEngine": 7659, "Ġgal": 7660, "ĠJud": 7661, "Ġvolunte": 7662, "icks": 7663, "eta": 7664, "agt": 7665, "Ġ×ķ": 7666, "Ġmö": 7667, "13": 7668, "Ġencoun": 7669, "Ġeh": 7670, "Ġorange": 7671, "Ġabsor": 7672, "Ġspaces": 7673, "ĠNovember": 7674, "구": 7675, "iat": 7676, "Ġtam": 7677, "cknow": 7678, "Ġstorm": 7679, "ĠDirector": 7680, "Ġpregn": 7681, "ĠìĿ¼": 7682, "Ġоп": 7683, "Ġresource": 7684, "Ġbard": 7685, "new": 7686, "ĠDecember": 7687, "uits": 7688, "Ġweil": 7689, "Ġconstruct": 7690, "si": 7691, "nic": 7692, "Ġflour": 7693, "Ġrestrict": 7694, "üt": 7695, "Ġentirely": 7696, "Ġbreaking": 7697, "entlich": 7698, "Ġtwenty": 7699, "Ġcauses": 7700, "Ġelev": 7701, "ĠSpr": 7702, "ĠInternet": 7703, "Ġkiss": 7704, "Ġoperations": 7705, "szy": 7706, "ĠëĬ": 7707, "Ġscientists": 7708, "Ġgrown": 7709, "Ġowners": 7710, "outs": 7711, "Ġcourses": 7712, "Ġusual": 7713, "Ġinn": 7714, "Ġtransm": 7715, "ño": 7716, "Ġnuest": 7717, "ков": 7718, "Ġcategory": 7719, "ĠLife": 7720, "ĠPlus": 7721, "Ġatmos": 7722, "while": 7723, "Ġrecords": 7724, "ĠdeÄŁ": 7725, "ëĭ¤ê³ł": 7726, "ĠìĤ¬ëŀ": 7727, "Ġrequirements": 7728, "inn": 7729, "Ġimmig": 7730, "Ġdeeper": 7731, "ç´": 7732, "Ġapps": 7733, "Ġcolleagues": 7734, "ży": 7735, "Ġoffers": 7736, "Ġtá": 7737, "Ġcolumn": 7738, "laud": 7739, "IR": 7740, "ĠMs": 7741, "Ġexchange": 7742, "las": 7743, "ĠLaw": 7744, "ĠJon": 7745, "isse": 7746, "rogen": 7747, "Ġmoi": 7748, "×Ĺ": 7749, "Ġsending": 7750, "Ġhello": 7751, "ее": 7752, "ÅĽÄĩ": 7753, "Ġsucceed": 7754, "Ġsuffering": 7755, "Ġadvert": 7756, "Ġ주": 7757, "çŁ¥éģĵ": 7758, "Ġreco": 7759, "ını": 7760, "Ġком": 7761, "alley": 7762, "Ġfailure": 7763, "iej": 7764, "ĠëķĮ": 7765, "Ġdrugs": 7766, "Ġcuando": 7767, "Ġìĸ´ëĸ": 7768, "ĠAbout": 7769, "Ġquando": 7770, "90": 7771, "ĠFed": 7772, "17": 7773, "Sh": 7774, "inho": 7775, "ĠSunday": 7776, "ĠPhil": 7777, "Ġacademic": 7778, "ĠInc": 7779, "Ġmainten": 7780, "åĩº": 7781, "Ġreward": 7782, "erd": 7783, "Ġcommitted": 7784, "ìĬ¤": 7785, "гÑĢ": 7786, "Ġstandards": 7787, "Ġkal": 7788, "Ġintention": 7789, "ĠZh": 7790, "Ġacknow": 7791, "ä¿": 7792, "Ġ===": 7793, "ogy": 7794, "å§": 7795, "Ġfilms": 7796, "isk": 7797, "Ġteeth": 7798, "Ġstruggle": 7799, "rd": 7800, "uen": 7801, "Ġdiss": 7802, "ĠDar": 7803, "amy": 7804, "Ġenemies": 7805, "Ġveloc": 7806, "ĠCall": 7807, "umbs": 7808, "иÑĤелÑĮ": 7809, "Ġocean": 7810, "éd": 7811, "ìļ°": 7812, "Ġtrem": 7813, "iento": 7814, "еÑĪÑĮ": 7815, "fficient": 7816, "Ġbottle": 7817, "Ġinstitution": 7818, "esty": 7819, "ĠHan": 7820, "hab": 7821, "ëĬĺ": 7822, "Ġarrest": 7823, "éĤĦ": 7824, "Ġletters": 7825, "ounce": 7826, "íĮ": 7827, "An": 7828, "Ġcreates": 7829, "Ġclock": 7830, "Ġdebt": 7831, "Ġancient": 7832, "ifications": 7833, "gi": 7834, "But": 7835, "ĠTu": 7836, "kl": 7837, "Ġborder": 7838, "Ġook": 7839, "ĠBay": 7840, "esta": 7841, "Ġë³´ì": 7842, "Ġwra": 7843, "prene": 7844, "Ġê²Į": 7845, "angle": 7846, "Ġbelieved": 7847, "iency": 7848, "aka": 7849, "Ġcritic": 7850, "Ġbomb": 7851, "Ġham": 7852, "ĠÐĽ": 7853, "êµŃ": 7854, "ĠGuys": 7855, "rosoft": 7856, "Ġcrim": 7857, "etch": 7858, "ARR": 7859, "Ġsight": 7860, "ина": 7861, "Ġain": 7862, "á»ij": 7863, "ische": 7864, "Ġaux": 7865, "Ġnumer": 7866, "Ġsurvive": 7867, "All": 7868, "BC": 7869, "Ġsz": 7870, "٬ë": 7871, "Ġjam": 7872, "ĠCourt": 7873, "Ġalles": 7874, "Ġtrigger": 7875, "Ðŀ": 7876, "Ġformat": 7877, "Ġdecades": 7878, "Ġces": 7879, "Ġsigns": 7880, "Ġrobot": 7881, "ĠChurch": 7882, "Ġaz": 7883, "Ġsoup": 7884, "ĠTexas": 7885, "uten": 7886, "ĠÑĩÑĤобÑĭ": 7887, "Ġneighb": 7888, "ĸ×Ķ": 7889, "Ġcommunicate": 7890, "Å¡": 7891, "Ġelimin": 7892, "Ġfrequency": 7893, "hern": 7894, "idos": 7895, "Ġemphas": 7896, "Ġmessages": 7897, "Ġgender": 7898, "ĠWenn": 7899, "Ġво": 7900, "Ġprices": 7901, "olo": 7902, "Ġпон": 7903, "wing": 7904, "ĠFil": 7905, "аем": 7906, "ĠCur": 7907, "Ġfalse": 7908, "Ġfields": 7909, "Ġsé": 7910, "24": 7911, "Ġmac": 7912, "uÅŁ": 7913, "Ġlayers": 7914, "Ġadvoc": 7915, "wan": 7916, "Ġkar": 7917, "ĠÅŀ": 7918, "Ġdecor": 7919, "Ġwalls": 7920, "oe": 7921, "issions": 7922, "Ġresol": 7923, "×¢": 7924, "ĠCarol": 7925, "ĠVide": 7926, "leep": 7927, "ĠYOU": 7928, "Ġflip": 7929, "Ġsurgery": 7930, "Ġchop": 7931, "UR": 7932, ".,": 7933, "Ġagency": 7934, "Ġwanting": 7935, "Ġsolar": 7936, "Ġhoriz": 7937, "ĠAdam": 7938, "Ġstaying": 7939, "olic": 7940, "Ġgrateful": 7941, "Ġremark": 7942, "Ġtechnologies": 7943, "Ġprotein": 7944, "å¿ĥ": 7945, "дел": 7946, "ĠMont": 7947, "Ġshoulder": 7948, "Ġza": 7949, "rey": 7950, "ĠOoh": 7951, "Ġsty": 7952, "icar": 7953, "оÑĤÑĢ": 7954, "Ġroute": 7955, "ĠTurn": 7956, "Ġbom": 7957, "Ġdebate": 7958, "Ġpossibility": 7959, "Ġíķ´ì": 7960, "apa": 7961, "Ġinvent": 7962, "ürlich": 7963, "Ġprofile": 7964, "Ġsenior": 7965, "ppy": 7966, "vas": 7967, "Ġmundo": 7968, "atever": 7969, "Ġapparently": 7970, "ener": 7971, "×IJ": 7972, "çŃ": 7973, "Ġprecis": 7974, "Ġalign": 7975, "Ġknife": 7976, "ĠRobert": 7977, "åĭ": 7978, "Ġfool": 7979, "Ġinvite": 7980, "using": 7981, "Ġcircumst": 7982, "Ġcapture": 7983, "Ġdough": 7984, "ĠSand": 7985, "Ġseu": 7986, "ĠNews": 7987, "Ġbite": 7988, "Ġneut": 7989, "wide": 7990, "Ġlecture": 7991, "ĠëĺIJ": 7992, "Ġoriginally": 7993, "Ġchoices": 7994, "ĠGar": 7995, "Ġverse": 7996, "Ġlit": 7997, "Ġ196": 7998, "íķł": 7999, "Ġmeasures": 8000, "ções": 8001, "water": 8002, "rive": 8003, "Ġzijn": 8004, "íģ": 8005, "ĠBus": 8006, "Ġheb": 8007, "еÑħ": 8008, "ĠKar": 8009, "ĠNão": 8010, "Ġkilling": 8011, "ப": 8012, "Ġmirror": 8013, "mod": 8014, "Ġmol": 8015, "Ġcreation": 8016, "Ġestim": 8017, "Ġatmosphere": 8018, "Ġgam": 8019, "Ġtables": 8020, "isi": 8021, "ĠLittle": 8022, "Ġtas": 8023, "ĠEle": 8024, "él": 8025, "Ġscenes": 8026, "Ġtone": 8027, "Ġaffected": 8028, "ĠAUDI": 8029, "ĠBrown": 8030, "If": 8031, "ĠÙĩ": 8032, "ĠDaniel": 8033, "羣çļĦ": 8034, "quer": 8035, "chi": 8036, "íķĺë": 8037, "Ġmistakes": 8038, "Ġsla": 8039, "ãĤ¤": 8040, "Ġentr": 8041, "ĠеÑģли": 8042, "Ġshout": 8043, "Ġportion": 8044, "ÑĹ": 8045, "Ġpreviously": 8046, "á»Ļ": 8047, "ĠпÑĢед": 8048, "оÑģÑĮ": 8049, "Ġheads": 8050, "çİ": 8051, "åŃ": 8052, "åľĭ": 8053, "Ġgrass": 8054, "ะ": 8055, "cribe": 8056, "Ġqué": 8057, "ĠSpanish": 8058, "Ġoffered": 8059, "ĠбÑĭло": 8060, "ĠCloud": 8061, "Ġvector": 8062, "ĠHuh": 8063, "Ġkad": 8064, "ifts": 8065, "Ġν": 8066, "Ġhungry": 8067, "С": 8068, "Ġparall": 8069, "AND": 8070, "ĠvÃŃdeo": 8071, "izz": 8072, "Ġoccup": 8073, "ĠíĶ": 8074, "Ġseek": 8075, "hes": 8076, "Ġdoors": 8077, "Ġhouses": 8078, "Ġconsidering": 8079, "Ġgraduate": 8080, "Ġfulf": 8081, "è¡Į": 8082, "è£": 8083, "Ġextreme": 8084, "Ġflowers": 8085, "itate": 8086, "ĠPri": 8087, "Ġfundamental": 8088, "ÑĩаÑģ": 8089, "说": 8090, "Ġtexture": 8091, "įĺ": 8092, "ĠAND": 8093, "à®±": 8094, "ĠTem": 8095, "Ġnada": 8096, "ì§Ħ": 8097, "Ġcelebrate": 8098, "ums": 8099, "Ġpill": 8100, "Ġили": 8101, "going": 8102, "Ġhip": 8103, "Ġsupported": 8104, "Ġperman": 8105, "Ġagreement": 8106, "Ġtym": 8107, "Ġëij": 8108, "ĵ¤ìĿ´": 8109, "Ġpurchase": 8110, "íĶ": 8111, "ĠPlan": 8112, "egen": 8113, "Ġrecover": 8114, "PU": 8115, "ĠMicrosoft": 8116, "duc": 8117, "Ġholes": 8118, "Ġdropped": 8119, "Ġpig": 8120, "Ġending": 8121, "Ġattacks": 8122, "bec": 8123, "Ġren": 8124, "Ġrapp": 8125, "Ġìļ°ë¦¬": 8126, "Ġterror": 8127, "Ġ×Ļ": 8128, "Ġedit": 8129, "Ġao": 8130, ".</": 8131, "Ġ2000": 8132, "ĠUnion": 8133, "Ġscientific": 8134, "Ġpunch": 8135, "ortion": 8136, "Ġputs": 8137, "ĠMonday": 8138, "ĠJer": 8139, "EC": 8140, "Ġmatrix": 8141, "Ġinstitutions": 8142, "Ġmont": 8143, "Ġexhib": 8144, "Ġspeaker": 8145, "Ġmeters": 8146, ".]": 8147, "Ġserving": 8148, "Ġdatabase": 8149, "ĠLAU": 8150, "Ġdamn": 8151, "Ġpoder": 8152, "!!!!": 8153, "ĠíĸĪ": 8154, "ĠAUDIENCE": 8155, "Ġjun": 8156, "ĠAC": 8157, "ĠItal": 8158, "sec": 8159, "ĠYoung": 8160, "ruck": 8161, "ouve": 8162, "à¸Ħ": 8163, "çĪ": 8164, "Ġë§Įë": 8165, "ading": 8166, "uration": 8167, "ĠPS": 8168, "Ðļ": 8169, "ĠUnf": 8170, "èģ": 8171, "oria": 8172, "Ġmanif": 8173, "Ġsentence": 8174, "Ġsigned": 8175, "BS": 8176, "Ġproof": 8177, "ĠMuslim": 8178, "Ġnuclear": 8179, "ĠговоÑĢ": 8180, "Ġwoll": 8181, "Ġfavour": 8182, "ĠWH": 8183, "Ġvulner": 8184, "Ġclosely": 8185, "Ġindex": 8186, "ÑĤеÑĢ": 8187, "achel": 8188, "Ġcapable": 8189, "ĠBes": 8190, "Ġcroch": 8191, "ekt": 8192, "Ġsheet": 8193, "Ġsees": 8194, "Ġnaturally": 8195, "ĠEngland": 8196, "Ġparticipate": 8197, "Ġexists": 8198, "Ġsharp": 8199, "py": 8200, "Ġbreakfast": 8201, "bow": 8202, "Ġtwist": 8203, "ç§": 8204, "inating": 8205, "oti": 8206, "ĠFound": 8207, "Ġdeux": 8208, "Ġselected": 8209, "ìłĦ": 8210, "osis": 8211, "Ġpresented": 8212, "Ġlinear": 8213, "Ġê´": 8214, "Ġkun": 8215, "é»ŀ": 8216, "ông": 8217, "ĠbÄĻd": 8218, "Ġtempor": 8219, "Ġcable": 8220, "ĠпÑĢоÑģÑĤо": 8221, "ке": 8222, "ĠÑĤам": 8223, "Ġwinning": 8224, "èĥ½": 8225, "ĺëıĦ": 8226, "Ġ2014": 8227, "ĠìŬë": 8228, "ĠUN": 8229, "ĠClick": 8230, "Ġprepar": 8231, "ĠTO": 8232, "Ġsua": 8233, "ĠHam": 8234, "Ġlä": 8235, "Ġabsolute": 8236, "Ġengaged": 8237, "å¦Ĥ": 8238, "ĠHmm": 8239, "Ġdash": 8240, "TA": 8241, "ños": 8242, "Ġspo": 8243, "çĶŁ": 8244, ")]": 8245, "Ġtested": 8246, "Ġblank": 8247, "Ġreject": 8248, "Ġassim": 8249, "Ġrear": 8250, "ĠStr": 8251, "Ġcrash": 8252, "ĠнаÑĪ": 8253, "иÑĤÑģÑı": 8254, "Ġcolon": 8255, "ĠUnt": 8256, "ĠCe": 8257, "Ġacid": 8258, "éĹ": 8259, "Ġkit": 8260, "ibilities": 8261, "uto": 8262, "Ġvaluable": 8263, "list": 8264, "Ġparties": 8265, "ĠMm": 8266, "Ġcolour": 8267, "Ġcham": 8268, "Ġsteel": 8269, "ĠImp": 8270, "Ġfunds": 8271, "ĠDNA": 8272, "ĠKen": 8273, "inde": 8274, "íķ´ìĦľ": 8275, "ãĥĥ": 8276, "ĠHappy": 8277, "ĠUse": 8278, "ĠLight": 8279, "Ġlip": 8280, "Ġauthority": 8281, "ĠLong": 8282, "ĠIran": 8283, "Ġell": 8284, "Ġcoordin": 8285, "Ġsubm": 8286, "Ġrecorded": 8287, "ÑĥÑĪ": 8288, "Ġdelta": 8289, "Ġreform": 8290, "ĠStill": 8291, "Ġoppon": 8292, "Ġallowing": 8293, "Ġpatterns": 8294, "Ġletting": 8295, "Ġsleeping": 8296, "Okay": 8297, "Ġpizza": 8298, "ĠÅĽ": 8299, "Ġдол": 8300, "Ġtalent": 8301, "ensions": 8302, "Ġenvironmental": 8303, "Ġprofessor": 8304, "Ġshots": 8305, "Ġcontains": 8306, "ugar": 8307, "yo": 8308, "ıĻ": 8309, "Ġsequence": 8310, "ια": 8311, "ader": 8312, "éł": 8313, "аÑĩ": 8314, "ÙĨا": 8315, "ĠIk": 8316, "Ġtous": 8317, "uries": 8318, "Ġpounds": 8319, "Ġexternal": 8320, "iments": 8321, "Ġvraiment": 8322, "ìĭ¤": 8323, "Ġhappiness": 8324, "Ġprze": 8325, "estic": 8326, "Ġestablish": 8327, "ĠFlor": 8328, "Ġrig": 8329, "Ġhoney": 8330, "Ġpul": 8331, "Ġsymptoms": 8332, "Ġbrows": 8333, "ели": 8334, "ĠÏĦο": 8335, "Ġshirt": 8336, "ĠTechn": 8337, "ĠProgram": 8338, "емÑĥ": 8339, "Ġupset": 8340, "Ġguest": 8341, "burg": 8342, "Ġunlike": 8343, "Ġsomewhat": 8344, "Ġhanging": 8345, "ae": 8346, "Ġrum": 8347, "Ġphotograph": 8348, "ĠLi": 8349, "åĽŀ": 8350, "Ġstable": 8351, "Ġvoltage": 8352, "ĠEll": 8353, "Ġentreprene": 8354, "uses": 8355, "assen": 8356, "¬¸": 8357, "Ġë§İìĿ´": 8358, "Ġghost": 8359, "Ġsagen": 8360, "Ġcombat": 8361, "Ġgör": 8362, "ĠCap": 8363, "Ġsão": 8364, "ĠKat": 8365, "Ġforma": 8366, "Ġsumm": 8367, "Ġmarch": 8368, "Ġvast": 8369, "ük": 8370, "Ġcommitment": 8371, "imos": 8372, "Let": 8373, "Ġdedicated": 8374, "iste": 8375, "lay": 8376, "éĢĻæ¨£": 8377, "Ġtopics": 8378, "Ġmachines": 8379, "ĠParis": 8380, "ĠìĿ´ëٰ": 8381, "Ġmini": 8382, "Ġmarkets": 8383, "Ġko": 8384, "δ": 8385, "ville": 8386, "Ġgoodness": 8387, "Ġframework": 8388, "ulture": 8389, "Ġbasket": 8390, "essa": 8391, "аÑĨи": 8392, "uster": 8393, "Ġê¹": 8394, "ä½Ĩ": 8395, "Ġextent": 8396, "ĠMenschen": 8397, "Ġconsistent": 8398, "Ġauto": 8399, "rip": 8400, "Ġmere": 8401, "à¯Ī": 8402, "ÑĶ": 8403, "Ġelle": 8404, "ĮĢë": 8405, "oken": 8406, "Ġpulling": 8407, "Ġcow": 8408, "outhern": 8409, "Ġmeetings": 8410, "Ġcada": 8411, "нÑĭм": 8412, "iente": 8413, "Ġbast": 8414, "aning": 8415, "Ġfocusing": 8416, "road": 8417, "Ġroof": 8418, "ĠProfessor": 8419, "ĠSP": 8420, "ÑĢаз": 8421, "Ġnood": 8422, "Ġ400": 8423, "ĠìĿ´ìłľ": 8424, "ìŀĪ": 8425, "ĠMount": 8426, "ейÑĩаÑģ": 8427, "Ġ×IJ": 8428, "Why": 8429, "×ŀ": 8430, "ında": 8431, "Ġpositions": 8432, "ème": 8433, "çı": 8434, "ĠдÑĢÑĥг": 8435, "iyor": 8436, "Ġpassing": 8437, "Ġassemb": 8438, "Ġsmoke": 8439, "Ġtil": 8440, "Ġmuseum": 8441, "ÐĶ": 8442, "ĠPerson": 8443, "ним": 8444, "leich": 8445, "Ġintent": 8446, "Ġsque": 8447, "Ġcraft": 8448, "ìĪĺ": 8449, "orsun": 8450, "Ġ150": 8451, "Ġbrothers": 8452, "vor": 8453, "ĠSpeaker": 8454, "icians": 8455, "Ġofficer": 8456, "Ġiçin": 8457, "ĠÑĤеб": 8458, "Ġscratch": 8459, "Ġgenerate": 8460, "yi": 8461, "Ġemotions": 8462, "aus": 8463, "ì¹ĺ": 8464, "45": 8465, "ĠLink": 8466, "ĠReal": 8467, "Ġate": 8468, "Ġнад": 8469, "Ġnative": 8470, "á»ĩ": 8471, "ıy": 8472, "Ġenorm": 8473, "Ġblocks": 8474, "Ġfaces": 8475, "acc": 8476, "iveness": 8477, "Ġinches": 8478, "uis": 8479, "heit": 8480, "Ġstreets": 8481, "Ġprobability": 8482, "asi": 8483, "Ġimpl": 8484, "Ġà¤": 8485, "urday": 8486, "Ġfaut": 8487, "omy": 8488, "Ġpip": 8489, "Ġillust": 8490, "ய": 8491, "ĠJun": 8492, "Ġlying": 8493, "99": 8494, "Ġmemories": 8495, "Ġpractical": 8496, "iana": 8497, "onces": 8498, "Ġviewers": 8499, "ĠThomas": 8500, "æĮ": 8501, "ĠGirl": 8502, "ĠWhether": 8503, "Ġinnovation": 8504, "Ġdisappoint": 8505, "My": 8506, "Ġwinner": 8507, "Ġig": 8508, "Ġratio": 8509, "ĠBlue": 8510, "ĠSub": 8511, "Ġdocuments": 8512, "Ġformula": 8513, "Ġë©": 8514, "ÑĬ": 8515, "Ġappeared": 8516, "var": 8517, "andon": 8518, "Ġspray": 8519, "mak": 8520, "ĠQUES": 8521, "KE": 8522, "Ġwedding": 8523, "Re": 8524, "аÑĤÑĮÑģÑı": 8525, "Ġuno": 8526, "Ġgall": 8527, "íĦ°": 8528, "cio": 8529, "cers": 8530, "Ġмне": 8531, "Ġpepper": 8532, "ãģĹãģŁ": 8533, "ĠFebru": 8534, "Ġalternative": 8535, "Ġfu": 8536, "ĠBasically": 8537, "ĠSmith": 8538, "Ġgate": 8539, "ĠTam": 8540, "ĠWhatever": 8541, "Ġapproxim": 8542, "Ġconcert": 8543, "Ġjuice": 8544, "ĠEspecially": 8545, "Ġdynamic": 8546, "Qu": 8547, "onder": 8548, "ivery": 8549, "Ġbang": 8550, "Ġrul": 8551, "ĠParty": 8552, "Ġscholars": 8553, "Ġcrying": 8554, "jÄħ": 8555, "Т": 8556, "ĠQUESTION": 8557, "rid": 8558, "Ġaccurate": 8559, "ço": 8560, "ĠCool": 8561, "coin": 8562, "Ġìĥģ": 8563, "ĠFo": 8564, "Ġpró": 8565, "ĠRoman": 8566, "ĠÐŁÑĢ": 8567, "Ġchecking": 8568, "?'": 8569, "Ġattached": 8570, "ĠIslam": 8571, "Ġexperts": 8572, "×§": 8573, "ĠConst": 8574, "ÑĢан": 8575, "Ġshadow": 8576, "Ġdelay": 8577, "ÐĴ": 8578, "Ġorient": 8579, "ëĤ": 8580, "ellen": 8581, "ĠasÃŃ": 8582, "кий": 8583, "Ġhistorical": 8584, "Ġuncom": 8585, "omp": 8586, "hm": 8587, "Ġbil": 8588, "Ġplanned": 8589, "ĠUnfortunately": 8590, "ĠWindows": 8591, "Ø´": 8592, "Ġencounter": 8593, "ĠìĥĿê°ģ": 8594, "Ġregarding": 8595, "arrass": 8596, "Ġrecovery": 8597, "ĠHur": 8598, "ĠEmp": 8599, "ĠsÃŃ": 8600, "íķĺê²Į": 8601, "Ġdefend": 8602, "Ġcet": 8603, "asse": 8604, "ëĭ¨": 8605, "okes": 8606, "Ġremote": 8607, "Ġس": 8608, "Ġarts": 8609, "isco": 8610, "aucoup": 8611, "ĠMexico": 8612, "Ġпом": 8613, "Ġchosen": 8614, "emat": 8615, "oding": 8616, "Ġflower": 8617, "standing": 8618, "ĠAssoci": 8619, "ummy": 8620, "ILL": 8621, "Ġcameras": 8622, "åĨį": 8623, "ĠæĪij": 8624, "ĠArab": 8625, "ĠSum": 8626, "Ġtego": 8627, "Ġcriminal": 8628, "iform": 8629, "Ġstack": 8630, "ìĦ±": 8631, "ĠDonald": 8632, "ĠOld": 8633, "Ġdust": 8634, "ĠJose": 8635, "Ġhem": 8636, "Ġincreases": 8637, "osta": 8638, "Ġdying": 8639, "ĠRiver": 8640, "Ġmoist": 8641, "ÑĤов": 8642, "ares": 8643, "Ġdiscipl": 8644, "rait": 8645, "ĠHas": 8646, "ygen": 8647, "ĠTre": 8648, "Ġë´": 8649, "Ġlanguages": 8650, "ĠHen": 8651, "Ġ36": 8652, "ĠDisney": 8653, "ints": 8654, "Ġalgo": 8655, "Ġfoods": 8656, "Ġsetup": 8657, "lan": 8658, "Ġeffectively": 8659, "Ġwherever": 8660, "æľĢ": 8661, "Ġunter": 8662, "formation": 8663, "Ġhits": 8664, "Ġprinciple": 8665, "Ġtastes": 8666, "§Ī": 8667, "Ġtreated": 8668, "Ġresolution": 8669, "Ġprivile": 8670, "ĠIP": 8671, "ë°": 8672, "Ġterrit": 8673, "Ġpowers": 8674, "Ġíĥ": 8675, "ĠVict": 8676, "Ġbother": 8677, "ĠChair": 8678, "Ġmuscle": 8679, "Ġsale": 8680, "Ġdecent": 8681, "Ġcoup": 8682, "ĠSqu": 8683, "Ġcoast": 8684, "Ġrod": 8685, "ĠFranc": 8686, "Ġbathroom": 8687, "Ġshopping": 8688, "ĠможеÑĤ": 8689, "ĠiÅŁ": 8690, "ĠStay": 8691, "grade": 8692, "Ġformed": 8693, "ĠbaÅŁ": 8694, "Ġbrill": 8695, "jour": 8696, "íĸ": 8697, "åĽł": 8698, "wie": 8699, "icate": 8700, "ĠâĢĭâĢĭ": 8701, "ĠNorm": 8702, "à¥": 8703, "Ġmainly": 8704, "ĠSpace": 8705, "Ġtremend": 8706, "iti": 8707, "வ": 8708, "UT": 8709, "Music": 8710, "ĠFebruary": 8711, "Ġcontrast": 8712, "对": 8713, "esting": 8714, "Ġδ": 8715, "inging": 8716, "ĠÙĨ": 8717, "ssen": 8718, "ĠHome": 8719, "Ġshell": 8720, "ĠHay": 8721, "Ġaller": 8722, "ĠAp": 8723, "ĠWestern": 8724, "ĠWord": 8725, "ĠPLAY": 8726, "Ġëħ": 8727, "ĠAqu": 8728, "Ġentry": 8729, "Ġlaunched": 8730, "ĠMem": 8731, "ĠPour": 8732, "Ġzwe": 8733, "ĠSomeone": 8734, "inge": 8735, "ĠProb": 8736, "mble": 8737, "ĠRel": 8738, "uru": 8739, "Ġrhy": 8740, "Ġgig": 8741, "Ġengagement": 8742, "Ã¼ÅŁ": 8743, "ãĤĩ": 8744, "Ġoffering": 8745, "whel": 8746, "Ġactor": 8747, "Ġå°į": 8748, "APP": 8749, "west": 8750, "ĠRoy": 8751, "Ġreturned": 8752, "Ġsilver": 8753, "rating": 8754, "Ġestar": 8755, "Ġske": 8756, "Ġti": 8757, "ication": 8758, "Ġannoy": 8759, "Ġdeeply": 8760, "ìļ©": 8761, "Ġnatürlich": 8762, "ELL": 8763, "ĠCath": 8764, "Ġrail": 8765, "нов": 8766, "Ġprayer": 8767, "col": 8768, "GB": 8769, "ĠТак": 8770, "Ġgla": 8771, "ĠWater": 8772, "ÑıÑĤÑĮ": 8773, "ĠNon": 8774, "ôt": 8775, "agers": 8776, "Ġhug": 8777, "Ġdoctors": 8778, "ancing": 8779, "ĠTalk": 8780, "zing": 8781, "Ġhadn": 8782, "Ġlui": 8783, "Ġaté": 8784, "Ġê·¸ë¦¬ê³ł": 8785, "ê¹Įì§Ģ": 8786, "ici": 8787, "Ġincorpor": 8788, "ĠDi": 8789, "zil": 8790, "anya": 8791, "ªħ": 8792, "Ġ»": 8793, "35": 8794, "Ġbeer": 8795, "Ġbeaucoup": 8796, "ĠMC": 8797, "Ġears": 8798, "ogen": 8799, "ĠQuest": 8800, "eda": 8801, "æľ¬": 8802, "ĠSaturday": 8803, "Ġfalls": 8804, "ston": 8805, "bles": 8806, "Ġthus": 8807, "ĠëĦ¤": 8808, "à¹Ħ": 8809, "Ġtherm": 8810, "Ġdiversity": 8811, "Ġsoy": 8812, "azu": 8813, "imp": 8814, "Ġtelevision": 8815, "éģİ": 8816, "Ġש׾": 8817, "Ġwur": 8818, "Ġedges": 8819, "Ġlessons": 8820, "ĠAud": 8821, "ãģĹãģ¦": 8822, "voir": 8823, "amento": 8824, "Ġexplained": 8825, "Ġона": 8826, "Ġtemps": 8827, "Ïİ": 8828, "They": 8829, "Ġsurprising": 8830, "аниÑı": 8831, "ĠDrag": 8832, "éĿ¢": 8833, "ĠCle": 8834, "Ġnam": 8835, "ĠлÑİд": 8836, "Ġhardware": 8837, "Ġthumbs": 8838, "Ġκαι": 8839, "ĠTop": 8840, "ĠÃ¥": 8841, "éĻ": 8842, "×ķר": 8843, "Ġê·¸ëŀĺìĦľ": 8844, "ĠBudd": 8845, "thern": 8846, "Ġinterests": 8847, "ذ": 8848, "Ġdevelopers": 8849, "Ġhitting": 8850, "Ġopposed": 8851, "Ġhearts": 8852, "ĠAndroid": 8853, "ĠHand": 8854, "Ġrepresents": 8855, "glich": 8856, "íĬ¸": 8857, "Ġ32": 8858, "Ġdomin": 8859, "ĠAnn": 8860, "ä¸Ģä¸ĭ": 8861, "Ġété": 8862, "Ġzoom": 8863, "Ġktóre": 8864, "Ġadults": 8865, "Ġordered": 8866, "Ġpicking": 8867, "ĠHong": 8868, "Ġfilming": 8869, "æĢĿ": 8870, "Ġseed": 8871, "ĠAT": 8872, "Ġcalculate": 8873, "Ġкогда": 8874, "ĠOs": 8875, "icit": 8876, "Ġremaining": 8877, "Ġsegu": 8878, "û": 8879, "Ġìĺ¤ëĬĺ": 8880, "Ġarrive": 8881, "Ġcongr": 8882, "Ġgrande": 8883, "Ġhealthcare": 8884, "Ġможно": 8885, "SA": 8886, "este": 8887, "Ġawareness": 8888, "Ġsquared": 8889, "xture": 8890, "ĠBeing": 8891, "Ġsoldiers": 8892, "Ñĥб": 8893, "Ġrevolution": 8894, "Ġtrained": 8895, "enden": 8896, "è°": 8897, "Ġdancing": 8898, "Ġinstalled": 8899, "prise": 8900, "Ġveter": 8901, "Ġmenos": 8902, "nell": 8903, "ĠBrother": 8904, "Ġnun": 8905, "Ġimportantly": 8906, "alled": 8907, "iaÅĤ": 8908, "abled": 8909, "ĠSystem": 8910, "ĠVol": 8911, "Ġeld": 8912, "Ġemotion": 8913, "ican": 8914, "ĠBank": 8915, "ikes": 8916, "Ġvlog": 8917, "Ġвоз": 8918, "Ġpuede": 8919, "ìĺ¤": 8920, "Ġteen": 8921, "Ġsevere": 8922, "%,": 8923, "Ġcleaning": 8924, "zÄħ": 8925, "ĹIJ": 8926, "ĠThrough": 8927, "ĠSet": 8928, "EP": 8929, "\"?": 8930, "ĠMother": 8931, "Ġfigured": 8932, "Ġmud": 8933, "ĠÑĸ": 8934, "ĠOffice": 8935, "Ġraw": 8936, "Ġdestroyed": 8937, "enta": 8938, "Ġaggress": 8939, "ĠоÑģ": 8940, "Ġ모ë": 8941, "ää": 8942, "ĠAR": 8943, "Ġcorrectly": 8944, "åīį": 8945, "Ġstir": 8946, "Ġextract": 8947, "Ġvehicles": 8948, "éĸĭ": 8949, "ĠRun": 8950, "ĠвÑĢем": 8951, "Ġparallel": 8952, "Ġlag": 8953, "ju": 8954, "Ġdare": 8955, "ĠMot": 8956, "ono": 8957, "Ġbeings": 8958, "Ġstro": 8959, "Ġexcuse": 8960, "Ġalpha": 8961, "Ġasks": 8962, "Ġpocket": 8963, "...?": 8964, "Ġkita": 8965, "üm": 8966, "Ġappearance": 8967, "ordan": 8968, "Ġinsert": 8969, "ĠнаÑĩ": 8970, "Ľi": 8971, "Ġtempo": 8972, "Ġfacility": 8973, "Ġvisible": 8974, "åĴ": 8975, "ĠScience": 8976, "uros": 8977, "ĠÙģÙĬ": 8978, "ĠVan": 8979, "Ġtension": 8980, "Ġíķł": 8981, "Ġdelivery": 8982, "Ġstim": 8983, "Ġsurvey": 8984, "ĠGra": 8985, "Ġbol": 8986, "æł": 8987, "Ġweiter": 8988, "ÃŁen": 8989, "ä¸ĢåĢĭ": 8990, "Ġproceed": 8991, "Ġimpressive": 8992, "ĠVoc": 8993, "iously": 8994, "Ġда": 8995, "hale": 8996, "och": 8997, "Ġglue": 8998, "phet": 8999, "cont": 9000, "Ġfits": 9001, "Ġboxes": 9002, "Ġcontrols": 9003, "ĠChild": 9004, "Ġscenario": 9005, "Ġtrop": 9006, "Ġprocessing": 9007, "ĠÑĤолÑĮко": 9008, "Ġbirds": 9009, "ĠChic": 9010, "Ġнап": 9011, "Ġ2013": 9012, "Ġmüssen": 9013, "ĠJag": 9014, "ĠsÄħ": 9015, "Ġperce": 9016, "reh": 9017, "ĠFore": 9018, "Ġconfused": 9019, "aire": 9020, "Ġaccomplish": 9021, "Ġcasa": 9022, "clock": 9023, "Ġinfluen": 9024, "ĠRO": 9025, "Ġbone": 9026, "ician": 9027, "ĠSC": 9028, "Ġstrategies": 9029, "gh": 9030, "дÑĥ": 9031, "Ġitu": 9032, "Ġpersonality": 9033, "Ġbardzo": 9034, "Ġaccepted": 9035, "Ġstom": 9036, "iev": 9037, "ĠHist": 9038, "ĠAus": 9039, "Ġë°Ķë": 9040, "ATOR": 9041, "æĦı": 9042, "oir": 9043, "Ġmagaz": 9044, "Ġexplan": 9045, "Ġcorn": 9046, "Ġils": 9047, "Ġcircuit": 9048, "Ġgay": 9049, "hop": 9050, "ãĤĥ": 9051, "Ġequival": 9052, "Ġdieser": 9053, "erves": 9054, "comes": 9055, "klich": 9056, "ĠëķĮë": 9057, "abet": 9058, "Ġexha": 9059, "Ġmanner": 9060, "ĠâĻªâĻª": 9061, "éc": 9062, "äl": 9063, "Ġconfirm": 9064, "Ġentered": 9065, "emplo": 9066, "ĠFar": 9067, "Ġoù": 9068, "essions": 9069, "Ġnurs": 9070, "Ġentão": 9071, "Ġabandon": 9072, "life": 9073, "Ġwis": 9074, "Narrator": 9075, "Ġìĸ´": 9076, "There": 9077, "ĠRam": 9078, "aste": 9079, "Ġattrib": 9080, "ĠAy": 9081, "Ġmesmo": 9082, "Ġνα": 9083, "é«": 9084, "enses": 9085, "Ġcrop": 9086, "ĠздеÑģÑĮ": 9087, "ĠUntil": 9088, "stein": 9089, "Ġoven": 9090, "Ġsuspect": 9091, "het": 9092, "Ġpuis": 9093, "Ġcarried": 9094, "ég": 9095, "ĠDev": 9096, "ems": 9097, "reens": 9098, "berry": 9099, "Ġtempl": 9100, "ĠBit": 9101, "Ġvariables": 9102, "Ġoverwhel": 9103, "με": 9104, "Ġinitially": 9105, "ìķĺ": 9106, "othing": 9107, "еÑĤÑĮ": 9108, "ĠHill": 9109, "Ġdepart": 9110, "Ġmyst": 9111, "azz": 9112, "Ġfluid": 9113, "ĠDC": 9114, "Ġclinical": 9115, "ĠRyan": 9116, "ĠFlorida": 9117, "ĠTak": 9118, "Ġanxiety": 9119, "bro": 9120, "Ġcircumstances": 9121, "ĠÙĥ": 9122, "Ġexistence": 9123, "Ġtong": 9124, "Ġ2012": 9125, "ĠSecretary": 9126, "Ġspicy": 9127, "Ġ[(": 9128, "ĠWithout": 9129, "Ġfacts": 9130, "Ġtons": 9131, "App": 9132, "ĠStand": 9133, "Ġlies": 9134, "ĠAD": 9135, "win": 9136, "ÏĦε": 9137, "applause": 9138, "IP": 9139, "sta": 9140, "ĠSup": 9141, "phones": 9142, "ŀij": 9143, "pie": 9144, "ĠPot": 9145, "ĠNO": 9146, "èµ·": 9147, "Ġ×ŀ": 9148, "ĠÐĶа": 9149, "icas": 9150, "ĠIr": 9151, "Ġpushed": 9152, "Ġuncle": 9153, "ĠÙħÙĨ": 9154, "Ġlon": 9155, "Ġprinciples": 9156, "ĠInternational": 9157, "ĠÃĸ": 9158, "ž": 9159, "Ġsaya": 9160, "Ġê³ł": 9161, "Ġrib": 9162, "Ġpaste": 9163, "Ġwarning": 9164, "Ġmusical": 9165, "Ġagreed": 9166, "оÑĢм": 9167, "Ġgarlic": 9168, "Ġoxygen": 9169, "ìĺĪ": 9170, "Al": 9171, "Ġë§ŀ": 9172, "elines": 9173, "LAUSE": 9174, "ç¾İ": 9175, "gypt": 9176, "GE": 9177, "cker": 9178, "tu": 9179, "Ġshel": 9180, "Ġstayed": 9181, "Ġгод": 9182, "Ġlapt": 9183, "ĠMartin": 9184, "Ġinvited": 9185, "Ġconfir": 9186, "Ġembarrass": 9187, "aciones": 9188, "ĠCamp": 9189, "Ġholds": 9190, "axy": 9191, "Ġdive": 9192, "uckles": 9193, "Ġboost": 9194, "Ġwür": 9195, "stal": 9196, "ĠÑĢабоÑĤ": 9197, "Ġdéc": 9198, "Ġofficers": 9199, "ĠìķĦë": 9200, "ologist": 9201, "×ŀ×": 9202, "Ġseeds": 9203, "Ġbuff": 9204, "Ġupdates": 9205, "ãĤı": 9206, "ded": 9207, "Ġfriendly": 9208, "Ġcouncil": 9209, "ĠProbably": 9210, "Ġpiano": 9211, "Ġreduced": 9212, "ÏĦα": 9213, "Ġauthent": 9214, "Ġexplos": 9215, "pass": 9216, "ĠHit": 9217, "jud": 9218, "ĠNav": 9219, "omi": 9220, "Ġcommission": 9221, "Ġgym": 9222, "ÐŁ": 9223, "Ġpon": 9224, "ÑĢоÑģ": 9225, "Ġinterface": 9226, "Ġstructures": 9227, "ĠJen": 9228, "Ġyok": 9229, "Ġmeu": 9230, "ì§Ģë§Į": 9231, "ned": 9232, "ĠWie": 9233, "Ġidentified": 9234, "Ġchannels": 9235, "ına": 9236, "Ġphilosop": 9237, "keit": 9238, "Ġbits": 9239, "entes": 9240, "Ġfrag": 9241, "ĠKind": 9242, "Ġdoch": 9243, "Ġsne": 9244, "inding": 9245, "ĠJewish": 9246, "оÑĢоÑĪ": 9247, "Ġfue": 9248, "æĸ¹": 9249, "Ġíı": 9250, "Ġmı": 9251, "Ġkeine": 9252, "Ġlocations": 9253, "ç͍": 9254, "Ġmeter": 9255, "Ġbeef": 9256, "ãģĺ": 9257, "Ġmanip": 9258, "Ġsono": 9259, "zzle": 9260, "ç¶": 9261, "Ġpes": 9262, "Ġhorrible": 9263, "ĠSn": 9264, "Ġfactory": 9265, "Ġfifth": 9266, "Ġcooked": 9267, "Ġmood": 9268, "Ġvelocity": 9269, "Ġoblig": 9270, "Ġconnections": 9271, "ÄŁim": 9272, "Ġê³µ": 9273, "Ġdomain": 9274, "Ġapplying": 9275, "Ġridic": 9276, "Ġcel": 9277, "Ġchildhood": 9278, "ĠTest": 9279, "ratulations": 9280, "ĠVirgin": 9281, "ĠCEO": 9282, "Ġпл": 9283, "Ġalgorithm": 9284, "Ġinteraction": 9285, "aga": 9286, "Ġkidding": 9287, "Ġtomato": 9288, "Ġcontinuing": 9289, "lad": 9290, "stream": 9291, "оже": 9292, "Ġìĺģ": 9293, "елов": 9294, "BA": 9295, "Ġnap": 9296, "ĠNobody": 9297, "Ġthumb": 9298, "ĠON": 9299, "Ġrush": 9300, "DR": 9301, "Ġstrike": 9302, "Ġevolution": 9303, "iche": 9304, "Ġì»": 9305, "Ġê·¸ëŁ°": 9306, "ات": 9307, "Ġak": 9308, "Ġwindows": 9309, "Ġexcess": 9310, "ãģªãģĦ": 9311, "Ġconclud": 9312, "Ġepisodes": 9313, "Ġstruggling": 9314, "ĠDat": 9315, "Ŀ¼ë": 9316, "Ġkeys": 9317, "Ġkle": 9318, "æŀľ": 9319, "Ġvegetables": 9320, "ystem": 9321, "ência": 9322, "rick": 9323, "Ġrevenue": 9324, "ĠHaw": 9325, "Ġlan": 9326, "antes": 9327, "iniz": 9328, "ãģĵãĤĮ": 9329, "иÑģÑĤ": 9330, "Ġsup": 9331, "©´ìĦľ": 9332, "Ġmomento": 9333, "isto": 9334, "ãģ¤": 9335, "ĠEric": 9336, "iors": 9337, "baj": 9338, "Ġintroduction": 9339, "irty": 9340, "Ġdeck": 9341, "real": 9342, "ĠMario": 9343, "Ġloving": 9344, "à¸Ķ": 9345, "Ġsupports": 9346, "иÑĩеÑģ": 9347, "Ġincident": 9348, "utch": 9349, "uv": 9350, "Ġboom": 9351, "еÑĢÑĮ": 9352, "ĠнÑĥж": 9353, "Ġcombined": 9354, "ĠLin": 9355, "23": 9356, "oration": 9357, "nte": 9358, "Ġsor": 9359, "Ġdirty": 9360, "ifer": 9361, "ĠAPI": 9362, "Ġcollaboration": 9363, "iable": 9364, "Ġpriority": 9365, "ĠAle": 9366, "ĠPrin": 9367, "ĠExc": 9368, "Ġvais": 9369, "Ġgran": 9370, "Ġstood": 9371, "Ġrecru": 9372, "ĠMur": 9373, "esis": 9374, "asp": 9375, "Ġlocked": 9376, "ĠPero": 9377, "ĠHarry": 9378, "Ġtudo": 9379, "ĠTen": 9380, "ص": 9381, "forcement": 9382, "))": 9383, "oli": 9384, "ĠìĿ¸": 9385, "Ġsuppl": 9386, "Ġcrochet": 9387, "Ġphenomen": 9388, "los": 9389, "athan": 9390, "ĠSupp": 9391, "Ġembr": 9392, "Ġbek": 9393, "ĠZeit": 9394, "gend": 9395, "Ġrooms": 9396, "ª½": 9397, "VER": 9398, "nych": 9399, "Ġdont": 9400, "Ġcabin": 9401, "Ġaccounts": 9402, "ĠEaster": 9403, "×ķ׾": 9404, "ãĥ«": 9405, "Ġfacilities": 9406, "beit": 9407, "Ġlinked": 9408, "ĠGer": 9409, "Ġprogramming": 9410, "otic": 9411, "Ġdrama": 9412, "Ġ29": 9413, "Ġíģ": 9414, "Ġinstructions": 9415, "Ġimportante": 9416, "Ġwaves": 9417, "Ġaid": 9418, "CK": 9419, "ê²łìĬµëĭĪëĭ¤": 9420, "ĠMir": 9421, "Ġtid": 9422, "ĠHot": 9423, "Ġarrange": 9424, "ĠBaby": 9425, "Ġtack": 9426, "ĠÑī": 9427, "íĿ": 9428, "Ġvertical": 9429, "Ġheel": 9430, "ĠCut": 9431, "Ġnarrow": 9432, "ĠAri": 9433, "Ġknee": 9434, "ĠBrazil": 9435, "ĠFive": 9436, "Ġposted": 9437, "UD": 9438, "Ġrolling": 9439, "θ": 9440, "Ġclaims": 9441, "ĠIns": 9442, "OK": 9443, "ãģĦãģĨ": 9444, "uin": 9445, "ĠInstitute": 9446, "Ġintense": 9447, "iar": 9448, "ĠNick": 9449, "Ġselection": 9450, "Ġlegend": 9451, "Ġuniform": 9452, "ún": 9453, "Ġstudied": 9454, "太": 9455, "ĠÐ¥": 9456, "ĠìķĮ": 9457, "gers": 9458, "Ġdow": 9459, "ĠCS": 9460, "Ġagent": 9461, "ĠAuf": 9462, "覺": 9463, "Ġjog": 9464, "Ġaircraft": 9465, "ëĭĺ": 9466, "Ġvit": 9467, "uls": 9468, "Ġsegment": 9469, "Ġorders": 9470, "ĠClass": 9471, "Ġapolog": 9472, "Ġplatforms": 9473, "Ġmyth": 9474, "аже": 9475, "ĠBook": 9476, "Ġsensitive": 9477, "ĠполÑĥÑĩ": 9478, "Ġdamit": 9479, "ĠCapt": 9480, "sole": 9481, "Ġarchitecture": 9482, "ĠWil": 9483, "Ġinher": 9484, "cap": 9485, "ĠBoy": 9486, "次": 9487, "Ġburning": 9488, "ĠPublic": 9489, "Ġbehalf": 9490, "ĠìľĦ": 9491, "Ġtherapy": 9492, "ubscribe": 9493, "Ġinvolve": 9494, "Ġexposed": 9495, "iÅŁ": 9496, "们": 9497, "être": 9498, "Ġtoil": 9499, "Ġsink": 9500, "pir": 9501, "åĥ": 9502, "II": 9503, "Ġagencies": 9504, "Ġq": 9505, "ĠDown": 9506, "auf": 9507, "Ġë§Ľ": 9508, "ãĥ»ãĥ»": 9509, "Ġproc": 9510, "oked": 9511, "Ġstores": 9512, "power": 9513, "ĠThings": 9514, "Ġaccessible": 9515, "Ġteż": 9516, "ĠEduc": 9517, "Ġspeakers": 9518, "ĠSarah": 9519, "ĶĶ": 9520, "Ġdiverse": 9521, "ìŀĸ": 9522, "ĠUlt": 9523, "Ãły": 9524, "ĠChicago": 9525, "She": 9526, "athy": 9527, "Ġenable": 9528, "Ġtrading": 9529, "Ġmuscles": 9530, "æĽ": 9531, "ĠCare": 9532, "ĠUr": 9533, "ĠScot": 9534, "Ġphrase": 9535, "ENT": 9536, "Ġê²½": 9537, "ĠJac": 9538, "pack": 9539, "Ġdetermined": 9540, "ünd": 9541, "Ġnegoti": 9542, "Ġvidé": 9543, "Ġroz": 9544, "ĠSus": 9545, "Ġriding": 9546, "hmen": 9547, "ĠDef": 9548, "ĠCre": 9549, "ãĤ¹": 9550, "ĠWall": 9551, "igan": 9552, "Ġsempre": 9553, "Ñĸд": 9554, "Ġdriven": 9555, "Ġfootage": 9556, "Ġfond": 9557, "ĠWay": 9558, "äm": 9559, "ĠObama": 9560, "ĠService": 9561, "Ġ75": 9562, "ĠDark": 9563, "Ġê·¼ë": 9564, "ĠCat": 9565, "Ø·": 9566, "éĮ": 9567, "Ġjug": 9568, "Ġetwas": 9569, "Ġbreathing": 9570, "á»ĥ": 9571, "åħ¶": 9572, "ĠWeb": 9573, "ä¹ĭ": 9574, "èµ°": 9575, "Ġfois": 9576, "Ġlighting": 9577, "ĠDA": 9578, "Ġobst": 9579, "Ġleur": 9580, "çı¾": 9581, "ĠEgypt": 9582, "ĠArmy": 9583, "icide": 9584, "аÑĤи": 9585, "Ġëĭ¤ë": 9586, "Ġapartment": 9587, "Ġchief": 9588, "ĠWed": 9589, "Ġnetworks": 9590, "Ġbatt": 9591, "æ¸": 9592, "ĠLuc": 9593, "Ġnicely": 9594, "Ġverb": 9595, "ิ": 9596, "ì¶": 9597, "osit": 9598, "Ġrevealed": 9599, "Ġtat": 9600, "Ġtied": 9601, "á»ģ": 9602, "Ġanimation": 9603, "Ġroles": 9604, "ìĬ¤í": 9605, "Ġversions": 9606, "ÑĩиÑĤ": 9607, "Ġtasks": 9608, "¯¼": 9609, "Ġresc": 9610, "she": 9611, "Ġloose": 9612, "Ġcá»": 9613, "Ġcoisa": 9614, "Ġalert": 9615, "Ġnin": 9616, "ĠSAM": 9617, "Ġtrabaj": 9618, "irus": 9619, "TH": 9620, "Æ¡": 9621, "ogether": 9622, "ĠTai": 9623, "Ġfigures": 9624, "Ġ×IJת": 9625, "Ġcreep": 9626, "Ġinvestigation": 9627, "Ġrecommended": 9628, "ĠAk": 9629, "Ġresidents": 9630, "ÑģÑĤво": 9631, "sect": 9632, "ание": 9633, "Ġminds": 9634, "uing": 9635, "å±": 9636, "owing": 9637, "Ġnog": 9638, "Ġraz": 9639, "ار": 9640, "Ġquot": 9641, "ĠиÑħ": 9642, "Ġsed": 9643, "Ġapplaud": 9644, "Ġcoverage": 9645, "vol": 9646, "ĠRec": 9647, "ÄĽ": 9648, "ĠвÑģÑij": 9649, "Ġexpecting": 9650, "Ġoperate": 9651, "Ġconver": 9652, "ĠSuch": 9653, "ĠRad": 9654, "ĠPrime": 9655, "Ġpurple": 9656, "Ġ2010": 9657, "ĠìķĪë": 9658, "Ġexem": 9659, "Ġcomparison": 9660, "Ġlandscape": 9661, "Ġneither": 9662, "ĠEh": 9663, "ëħ": 9664, "Ġstomach": 9665, "Ġcaso": 9666, "ân": 9667, "Ġpercentage": 9668, "wich": 9669, "itan": 9670, "Ġkl": 9671, "Ġexpans": 9672, "ĠاÙĦÙħ": 9673, "Ġoccasion": 9674, "rets": 9675, "igning": 9676, "Ġkilomet": 9677, "è·Ł": 9678, "Ġgust": 9679, "cze": 9680, "Ġurban": 9681, "Ġagric": 9682, "Ġassistance": 9683, "Ġsurf": 9684, "imeter": 9685, "Ġpetit": 9686, "Ġassessment": 9687, "Ġmanual": 9688, "Ġimproved": 9689, "bst": 9690, "Ġpilot": 9691, "ĠMars": 9692, "Ġviele": 9693, "ĠCongratulations": 9694, "Ġargue": 9695, "Ġwirklich": 9696, "Ġclicking": 9697, "RIS": 9698, "Ġlogo": 9699, "Ġoutcome": 9700, "ĠCentral": 9701, "ĠJi": 9702, "Ġgaming": 9703, "Ġconserv": 9704, "Ġultimate": 9705, "ĠVe": 9706, "ĠWal": 9707, "aro": 9708, "æĦŁ": 9709, "star": 9710, "Ġconsumer": 9711, "Ġtraveling": 9712, "imer": 9713, "Ġ1000": 9714, "ник": 9715, "Ġprincipal": 9716, "Ġsake": 9717, "Ñĸв": 9718, "Ġmouse": 9719, "arios": 9720, "Ġrelation": 9721, "èĩª": 9722, "Ġmoral": 9723, "åķ¦": 9724, "Ġtheta": 9725, "wy": 9726, "Ġkam": 9727, "Ġeig": 9728, "Ġgolden": 9729, "פ": 9730, "Ġampl": 9731, "Ġvu": 9732, "str": 9733, "rors": 9734, "Ġwhereas": 9735, "izar": 9736, "Ġadministr": 9737, "Ġnós": 9738, "ĠPret": 9739, "ĠAcad": 9740, "anging": 9741, "bage": 9742, "était": 9743, "uri": 9744, "Ġhealing": 9745, "Ġtipo": 9746, "Ġmarry": 9747, "Ñĥв": 9748, "Ġestate": 9749, "uu": 9750, "ìĶ": 9751, "ĠBest": 9752, "Ġsuffer": 9753, "Ġ194": 9754, "Ġbacter": 9755, "ĠÐĴоÑĤ": 9756, "ĠOm": 9757, "Ġdz": 9758, "è¶": 9759, "ì¦": 9760, "Ġoldu": 9761, "Ġphysically": 9762, "ĠLouis": 9763, "etime": 9764, "case": 9765, "Ġpier": 9766, "ìłľ": 9767, "van": 9768, "Ġassets": 9769, "Ġëģ": 9770, "vet": 9771, "иб": 9772, "Ġpromote": 9773, "Ġcongrat": 9774, "uesday": 9775, "Ġduty": 9776, "ĠVideo": 9777, "Ø®": 9778, "ĠJohnson": 9779, "ktion": 9780, "ĠVocê": 9781, "ãĢĭ": 9782, "Ġai": 9783, "Ġannual": 9784, "ĠJosh": 9785, "itte": 9786, "ĠJO": 9787, "Ġslides": 9788, "Ġanc": 9789, "¹Ħ": 9790, "teen": 9791, "Ġcarrying": 9792, "lymp": 9793, "eding": 9794, "Ġfro": 9795, "Ġadmit": 9796, "rer": 9797, "Ġofficials": 9798, "ptions": 9799, "gal": 9800, "Ġheute": 9801, "Ġvoices": 9802, "Ġballs": 9803, "Ġguests": 9804, "anner": 9805, "ãĢĬ": 9806, "isher": 9807, "ĠMR": 9808, "ĠRichard": 9809, "Ġroughly": 9810, "lı": 9811, "Ġvictory": 9812, "Ġalgun": 9813, "ĠMrs": 9814, "ÅĽcie": 9815, "ĠUk": 9816, "Ġey": 9817, "ĠWars": 9818, "Ġbranch": 9819, "asty": 9820, "ĠPrince": 9821, "екÑĤ": 9822, "Ġrecognized": 9823, "Ġmucho": 9824, "ĠLeave": 9825, "connect": 9826, "Ġspell": 9827, "Ġtouched": 9828, "Ġagenda": 9829, "è¾": 9830, "aria": 9831, "ĠKong": 9832, "oga": 9833, "Ġparameters": 9834, "ëĭ¤ë": 9835, "Ġinstant": 9836, "Ġregul": 9837, "Con": 9838, "Ġeditor": 9839, "ĠDist": 9840, "Ġunknown": 9841, "Ġpunish": 9842, "Ġexpectations": 9843, "Ġcrypt": 9844, "Ġdivide": 9845, "aken": 9846, "ĠMess": 9847, "Ġhyper": 9848, "ĠProject": 9849, "iki": 9850, "Ġagora": 9851, "Ġabuse": 9852, "Ġcausing": 9853, "Ġconvin": 9854, "ĠLA": 9855, "Ġconcentration": 9856, "Ġbreaks": 9857, "urer": 9858, "Ġconcrete": 9859, "Ġformal": 9860, "Ġbeta": 9861, "itors": 9862, "ĠChamp": 9863, "Ġheading": 9864, "ĠBlo": 9865, "Ġprend": 9866, "ĠSenate": 9867, "Ġadventure": 9868, "oso": 9869, "Ġopens": 9870, "ĠPLAYING": 9871, "ĠSU": 9872, "uren": 9873, "ikt": 9874, "ĠлÑİб": 9875, "ĠFollow": 9876, "ĠBiden": 9877, "eln": 9878, "ĠSky": 9879, "eting": 9880, "ĠExt": 9881, "нÑĥÑİ": 9882, "ĠìĻľ": 9883, "Ġshr": 9884, "ella": 9885, "ĠDiv": 9886, "Ġtransformation": 9887, "Ġhousehold": 9888, "etry": 9889, "è¡": 9890, "ĠDesp": 9891, "Ġcourage": 9892, "Ġparking": 9893, "Ġettä": 9894, "cal": 9895, "lyn": 9896, "Ġlaid": 9897, "Ġtries": 9898, "irts": 9899, "iga": 9900, "Ġrecall": 9901, "ifier": 9902, "Ïģα": 9903, "Ġaan": 9904, "Ġbuttons": 9905, "Ġreaching": 9906, "Ġê·¼ëį°": 9907, "Ġspark": 9908, "ĠSocial": 9909, "ĠеÑīе": 9910, "Ġcanal": 9911, "Ġcriter": 9912, "Ġktóry": 9913, "Ġtenemos": 9914, "Ĥ¬": 9915, "ĠнеÑĤ": 9916, "Ġtube": 9917, "acles": 9918, "иÑĪ": 9919, "ĠdeÄŁil": 9920, "Ġstamp": 9921, "Ġinfl": 9922, "Ġahora": 9923, "Ġtrail": 9924, "Ġmixture": 9925, "ĠRoll": 9926, "Ġroutine": 9927, "Ġcounty": 9928, "Ġenjoying": 9929, "ноÑģÑĤÑĮ": 9930, "eres": 9931, "Ġpurposes": 9932, "ĠSanta": 9933, "Ġbreast": 9934, "äng": 9935, "Ġwriter": 9936, "åĮ": 9937, "ÑĢо": 9938, "Ġnem": 9939, "icos": 9940, "аÑģÑĤ": 9941, "Ġdetailed": 9942, "Ġreverse": 9943, "ĠReady": 9944, "Ġdistract": 9945, "ĠAlors": 9946, "utter": 9947, "Ġdeserve": 9948, "ĠRon": 9949, "ном": 9950, "Ġobserv": 9951, "Ġlogic": 9952, "ĠPy": 9953, "ĠKevin": 9954, "ãģĿãģĨ": 9955, "¥´": 9956, "ÙĬÙĨ": 9957, "Ġska": 9958, "Ġtact": 9959, "Ġholiday": 9960, "Ġbump": 9961, "Ġмог": 9962, "Ġdeix": 9963, "íħ": 9964, "Ġworship": 9965, "Cl": 9966, "Ġsuck": 9967, "ĠÑģеб": 9968, "Ġapplause": 9969, "ĠEp": 9970, "Ġмо": 9971, "Ġpatch": 9972, "áºŃ": 9973, "Ġladies": 9974, "Ġbroadcast": 9975, "Ġilleg": 9976, "Ġnarrative": 9977, "ossa": 9978, "ARRATOR": 9979, "Ġsang": 9980, "Ġmovements": 9981, "Ġpartnership": 9982, "Ġorganized": 9983, "Ġnode": 9984, "estyle": 9985, "ĠMeg": 9986, "Ġindustrial": 9987, "Ġgol": 9988, "Ġboring": 9989, "åĬł": 9990, "ãģĶ": 9991, "Ġcuts": 9992, "Ġrecon": 9993, "asa": 9994, "Ġimpression": 9995, "ìļ´": 9996, "gie": 9997, "MA": 9998, "Ĩµ": 9999, "Ġediting": 10000, "ront": 10001, "Ġfollows": 10002, "ĠItalian": 10003, "ÑĢод": 10004, "Ġê°ĻìĿĢ": 10005, "Ġë°©": 10006, "Ġparticles": 10007, "ĠBoard": 10008, "×Ļת": 10009, "jun": 10010, "ronic": 10011, "Ġej": 10012, "ĠÏĦη": 10013, "×ķ×ĵ": 10014, "cion": 10015, "itty": 10016, "ĠTuesday": 10017, "umes": 10018, "ĠProt": 10019, "eder": 10020, "Ġpessoas": 10021, "Ġнов": 10022, "Ġskip": 10023, "Ġobjective": 10024, "ÃŃas": 10025, "Ġdesk": 10026, "ĠLooks": 10027, "unden": 10028, "Ġprimarily": 10029, "imento": 10030, "Ġreporting": 10031, "Ġhace": 10032, "Ġchecked": 10033, "éĺ": 10034, "Ġë³´ë": 10035, "Ġsmells": 10036, "Ġactors": 10037, "ĠAsia": 10038, "ilÃł": 10039, "Ġreceiving": 10040, "Ġtaxes": 10041, "Ġgrace": 10042, "Ġcompetitive": 10043, "Ġdivision": 10044, "Ġesper": 10045, "Ġwheels": 10046, "Ġkommt": 10047, "Ġtremendous": 10048, "Ġespe": 10049, "...)": 10050, "Ġìŀħ": 10051, "Ġlisted": 10052, "äll": 10053, "Ġunus": 10054, "ĠHolly": 10055, "Ġguidance": 10056, "Ġcub": 10057, "Ġintellect": 10058, "ĠбÑĭл": 10059, "Ġregardless": 10060, "ĠStan": 10061, "没": 10062, "Ġconclusion": 10063, "acaÄŁ": 10064, "Ġlol": 10065, "ĠBat": 10066, "Ġmanifest": 10067, "ĠChief": 10068, "Ġshame": 10069, "Ġoutcomes": 10070, "Ġmail": 10071, "Ġkur": 10072, "ικ": 10073, "etz": 10074, "Ġpreparing": 10075, "27": 10076, "ĠQueen": 10077, "ள": 10078, "Ġë¹Ħ": 10079, "Ġtiss": 10080, "Ġconsciousness": 10081, "Ġpants": 10082, "Ġmelt": 10083, "ucht": 10084, "inh": 10085, "ìĽĮ": 10086, "Ġvotre": 10087, "Ġmodule": 10088, "owy": 10089, "Ġmonster": 10090, "ĠëĨ": 10091, "Ġelectronic": 10092, "Ġcentre": 10093, "Ġstops": 10094, "Ġtou": 10095, "ĠëŃ": 10096, "Ġlamb": 10097, "Ġconsequences": 10098, "Ġstraw": 10099, "Ġimper": 10100, "Ġextend": 10101, "ãģ£ãģŁ": 10102, "Ġanswered": 10103, "ĠMah": 10104, "ĠLAURA": 10105, "ifting": 10106, "uate": 10107, "åħĪ": 10108, "ĠUSB": 10109, "ĠAndrew": 10110, "ãĤ«": 10111, "ĠFred": 10112, "ĠDE": 10113, "ĠGeorg": 10114, "ç»": 10115, "ình": 10116, "Ġdrawn": 10117, "Ġlips": 10118, "bir": 10119, "Ġmayor": 10120, "imi": 10121, "Ġencore": 10122, "åIJĥ": 10123, "fortable": 10124, "ursday": 10125, "ĠForm": 10126, "Ġblame": 10127, "Ġshower": 10128, "Ġcontainer": 10129, "sters": 10130, "udes": 10131, "ĠTay": 10132, "ล": 10133, "ĠìĺĪ": 10134, "Ġvom": 10135, "Ġbass": 10136, "ĠLab": 10137, "issa": 10138, "Ġdimension": 10139, "Ġexecutive": 10140, "ĠRom": 10141, "ê²ĮìļĶ": 10142, "ĠDoctor": 10143, "Ġdelivered": 10144, "Ġgang": 10145, "Ġcer": 10146, "Ġpit": 10147, "eli": 10148, "Ġextraord": 10149, "jar": 10150, "Ġderiv": 10151, "Ġillness": 10152, "Ġguns": 10153, "Ġ2011": 10154, "Ġairport": 10155, "Ðķ": 10156, "Ġattitude": 10157, "Ġgrat": 10158, "ĠWr": 10159, "ĠNARRATOR": 10160, "ĠìļĶ": 10161, "Ġrenew": 10162, "Ġcosa": 10163, "Ġcontrolled": 10164, "ommy": 10165, "onds": 10166, "Ġese": 10167, "äch": 10168, "Ġvend": 10169, "dam": 10170, "Ġargu": 10171, "Ġacceler": 10172, "Ġnail": 10173, "iene": 10174, "ìĥĿ": 10175, "Ġencont": 10176, "esearch": 10177, "é¡": 10178, "Ġgoods": 10179, "Ġfishing": 10180, "APPLAUSE": 10181, "ĠNAS": 10182, "ection": 10183, "Ġtemple": 10184, "liche": 10185, "Ġkeyboard": 10186, "çŃī": 10187, "Ġdesde": 10188, "Ġeducational": 10189, "ĠNight": 10190, "33": 10191, "Ġbreathe": 10192, "lichen": 10193, "thm": 10194, "ière": 10195, "à¸ļ": 10196, "ları": 10197, "Ġali": 10198, "Ġcompos": 10199, "Ġsensor": 10200, "Ġë¶Ģë": 10201, "Ġnewsp": 10202, "ĠBund": 10203, "ĠMi": 10204, "Ġperforming": 10205, "Ġdrum": 10206, "BE": 10207, "Ġpork": 10208, "Ġcoal": 10209, "enger": 10210, "Ġram": 10211, "Ġë²Ī": 10212, "çĦ¶å¾Į": 10213, "иÑĢов": 10214, "ĠPop": 10215, "Ġphones": 10216, "Ġfacil": 10217, "Ġtracks": 10218, "onte": 10219, "Ġorganic": 10220, "Ġdialogue": 10221, "ĠHaving": 10222, "ĠPost": 10223, "Ġpayment": 10224, "Ġarray": 10225, "Ġintended": 10226, "ús": 10227, "Ġbars": 10228, "Ġreviews": 10229, "lands": 10230, "Ġkingdom": 10231, "Ġstages": 10232, "Ġmountains": 10233, "Ġdun": 10234, "Ġdecir": 10235, "Äį": 10236, "Ġbanks": 10237, "Ġthrowing": 10238, "Ġ못": 10239, "Ġanger": 10240, "ĠÑģейÑĩаÑģ": 10241, "Ġdistur": 10242, "Ġhumanity": 10243, "Ġeles": 10244, "Ġshoulders": 10245, "ĠPerfect": 10246, "Ġfancy": 10247, "Ġbrilliant": 10248, "Ġinspiration": 10249, "hmm": 10250, "å¿«": 10251, "Ġlid": 10252, "UL": 10253, "ĠmÃ¥": 10254, "indi": 10255, "èĪ": 10256, "Ġshield": 10257, "Ġìĺ¤ë": 10258, "CT": 10259, "agine": 10260, "uber": 10261, "ĠBR": 10262, "Ġquesto": 10263, "Ġзак": 10264, "ĠKnow": 10265, "Ġtang": 10266, "íķ©ëĭĪëĭ¤": 10267, "Ġbarely": 10268, "ĠSE": 10269, "Ġmargin": 10270, "rei": 10271, "аÑĤелÑĮ": 10272, "Ġcontr": 10273, "ĠvÃł": 10274, "Ġlegit": 10275, "Ðĺ": 10276, "kins": 10277, "ÑĢед": 10278, "ĠAsh": 10279, "Ġadvis": 10280, "ĠGreek": 10281, "Ñĥк": 10282, "Ġshake": 10283, "idades": 10284, "аÑģÑĮ": 10285, "Ġconvention": 10286, "Ġcontest": 10287, "MS": 10288, "ĠYear": 10289, "Ġrepresentation": 10290, "inden": 10291, "endar": 10292, "Ġprost": 10293, "ĠHuman": 10294, "ĠCy": 10295, "anged": 10296, "PA": 10297, "Ġaxis": 10298, "Ġtheore": 10299, "atz": 10300, "Ġíķĺê³ł": 10301, "Ġels": 10302, "ĠResearch": 10303, "Ġbenefic": 10304, "Ġdensity": 10305, "indo": 10306, "ìľ¼": 10307, "imdi": 10308, "Ġresearchers": 10309, "ê±°ëĵł": 10310, "ighs": 10311, "dan": 10312, "Ġdice": 10313, "Ġmaar": 10314, "Ġsubmit": 10315, "Ġdumb": 10316, "Ġbij": 10317, "away": 10318, "ĠPass": 10319, "Ġextension": 10320, "Ġcrush": 10321, "Ġcovering": 10322, "edi": 10323, "born": 10324, "inations": 10325, "ĠÑģдел": 10326, "веÑĢ": 10327, "ĠOtherwise": 10328, "istant": 10329, "айÑĤе": 10330, "Ġtanto": 10331, "Ġperformed": 10332, "Ġзап": 10333, "alo": 10334, "ĠFoundation": 10335, "Ġprotocol": 10336, "ĠZo": 10337, "may": 10338, "Ġhack": 10339, "Ġbuddy": 10340, "made": 10341, "Ġads": 10342, "Ġfascinating": 10343, "Ġequivalent": 10344, "gel": 10345, "Ġarc": 10346, "ĠÑĩелов": 10347, "Ġproposed": 10348, "Ġnotre": 10349, "anges": 10350, "Ġcounsel": 10351, "alla": 10352, "Ġ31": 10353, "weet": 10354, "ÈĻ": 10355, "Ġelectricity": 10356, "Ġtox": 10357, "ÅĤad": 10358, "Ġì´": 10359, "Ġdifficulty": 10360, "ł×Ļ": 10361, "nesday": 10362, "иÑģÑĮ": 10363, "Ġalleg": 10364, "ĠGO": 10365, "Ġquit": 10366, "ĠHerr": 10367, "Ġestán": 10368, "Ġgirlfriend": 10369, "Ġteng": 10370, "ificial": 10371, "ĠJam": 10372, "Ġcancel": 10373, "Ġfrequently": 10374, "IV": 10375, "實": 10376, "Ġclosing": 10377, "Ġdecade": 10378, "Ġrepresented": 10379, "ĠCanad": 10380, "ĠкоÑĤоÑĢÑĭе": 10381, "Ġestamos": 10382, "ĠThursday": 10383, "ĠGa": 10384, "ĠLive": 10385, "lem": 10386, "bble": 10387, "SON": 10388, "Ġ2008": 10389, "Ġdich": 10390, "ĠAwesome": 10391, "Ġconcepts": 10392, "PEAK": 10393, "Ġliterature": 10394, "ĠOlymp": 10395, "лад": 10396, "Ġnost": 10397, "vit": 10398, "ĠEnter": 10399, "orders": 10400, "icking": 10401, "niej": 10402, "Ġeuch": 10403, "ĠThough": 10404, "Ġbags": 10405, "Ġlimits": 10406, "Ġstake": 10407, "ĥ¥": 10408, "Ġoc": 10409, "ĠVis": 10410, "Ġ120": 10411, "Ġnue": 10412, "Ġconce": 10413, "Ġdisag": 10414, "ç¨": 10415, "Ġanticip": 10416, "łĪ": 10417, "sl": 10418, "Ġvoting": 10419, "Ġexposure": 10420, "ĠCommunity": 10421, "ĠJustice": 10422, "orney": 10423, "szyst": 10424, "Ġfried": 10425, "ìĭľë": 10426, "ĠWin": 10427, "Ġ@": 10428, "ĠHopefully": 10429, "esz": 10430, "Ġmonde": 10431, "Ġcombine": 10432, "gment": 10433, "Ġrecommendations": 10434, "Ġpregnant": 10435, "ìĭĿ": 10436, "raf": 10437, "Ġlu": 10438, "èĢģ": 10439, "ä»Ģä¹Ī": 10440, "door": 10441, "азÑĭв": 10442, "uego": 10443, "Ġimprovement": 10444, "Ġtrim": 10445, "Ġeigen": 10446, "Ġapproximately": 10447, "Ġвам": 10448, "awa": 10449, "ĠÑģоб": 10450, "Ġcoron": 10451, "Ġongoing": 10452, "Ġhes": 10453, "Ġinjury": 10454, "Ġfrank": 10455, "Ġkadar": 10456, "rency": 10457, "ĠColor": 10458, "ĠGru": 10459, "Ġdip": 10460, "ÑĢÑĭ": 10461, "Ġtears": 10462, "gt": 10463, "ĠPD": 10464, "Ġpause": 10465, "osc": 10466, "Ġusted": 10467, "ĠWoo": 10468, "ĠwiÄĻ": 10469, "è¦ĭ": 10470, "Ġdenn": 10471, "ĠPet": 10472, "Ġovercome": 10473, "ĠëĤ´ê°Ģ": 10474, "ĠMove": 10475, "Ġlicense": 10476, "Ġrepeated": 10477, "à¯ĩ": 10478, "Ġcategories": 10479, "Ġnoodles": 10480, "Ġflood": 10481, "ĠMass": 10482, "Ġnuts": 10483, "ĠJess": 10484, "ĠIh": 10485, "Ġchances": 10486, "IJĺ": 10487, "Ġdonde": 10488, "IG": 10489, "Ġandere": 10490, "Ġbones": 10491, "ìŀij": 10492, "Ġefficiency": 10493, "Ġmoder": 10494, "roat": 10495, "ĠìĿ´ê²Į": 10496, "iller": 10497, "Ġomega": 10498, "Ġпов": 10499, "ĠGroup": 10500, "Ġproducing": 10501, "amo": 10502, "Ġparticipants": 10503, "upp": 10504, "ifice": 10505, "Ġfortun": 10506, "ietnam": 10507, "acak": 10508, "ĠKo": 10509, "miÅŁ": 10510, "Ġjail": 10511, "ĠJones": 10512, "ÅĽmy": 10513, "ĠDeuts": 10514, "Ġbriefly": 10515, "ĠTal": 10516, "ĠPerhaps": 10517, "ĠRub": 10518, "ĠKn": 10519, "ëĭ¤ëĬĶ": 10520, "ré": 10521, "Ġvocês": 10522, "ĠCharles": 10523, "еÑĤе": 10524, "riers": 10525, "Ġheal": 10526, "antee": 10527, "Ġdemocracy": 10528, "Ġloan": 10529, "Ġchef": 10530, "Ñıм": 10531, "Ġuncomfortable": 10532, "Ġetern": 10533, "apping": 10534, "Ġrepair": 10535, "rot": 10536, "ĠTar": 10537, "Ġcovers": 10538, "oming": 10539, "ĠEth": 10540, "ĠÎŃ": 10541, "Ñĩно": 10542, "Ġafterwards": 10543, "ĠвеÑĢ": 10544, "Ġdaha": 10545, "Ġknees": 10546, "Ġordinary": 10547, "ül": 10548, "gas": 10549, "Ġticket": 10550, "ĠìłĢëĬĶ": 10551, "ĠìŀĪìĬµëĭĪëĭ¤": 10552, "chte": 10553, "Mr": 10554, "Ġsist": 10555, "hui": 10556, "ê·¸ë": 10557, "ìŬ": 10558, "Ġvary": 10559, "Ġmemor": 10560, "Ġcontroller": 10561, "ĠbÄĻdzie": 10562, "Ġminister": 10563, "×Ĵ": 10564, "flow": 10565, "AH": 10566, "Ġtower": 10567, "çIJ": 10568, "Ġscar": 10569, "æĥħ": 10570, "ĠPen": 10571, "ĠpaÃŃs": 10572, "×ĺ": 10573, "ìĿ¸ë": 10574, "Ġenerg": 10575, "Ġsword": 10576, "Ġpapers": 10577, "ила": 10578, "ĠWednesday": 10579, "ĠForce": 10580, "Ġextraordinary": 10581, "ĠLake": 10582, "Ġê°Ģë": 10583, "ĠBeaut": 10584, "Ġreasonable": 10585, "Ġcontribute": 10586, "Ġpleased": 10587, "Ġupdated": 10588, "Ġpiù": 10589, "elo": 10590, "Ġsignificantly": 10591, "Ġbot": 10592, "Ġgenerations": 10593, "Ġprotected": 10594, "åĵĪ": 10595, "Ġhiding": 10596, "ĠIll": 10597, "Ġneutral": 10598, "],": 10599, "ÏĦο": 10600, "Ġtongue": 10601, "Thank": 10602, "Ġê³Ħ": 10603, "Ġpays": 10604, "ίν": 10605, "Ġapple": 10606, "01": 10607, "erk": 10608, "iera": 10609, "Ġjeg": 10610, "ĠSubscribe": 10611, "Ġtheater": 10612, "Ġstrongly": 10613, "ĠìĨĮ": 10614, "ĠпÑĢав": 10615, "ucky": 10616, "ĠJin": 10617, "kward": 10618, "ê±´": 10619, "Ġopponent": 10620, "ĠSO": 10621, "Ġholy": 10622, "Ġfilling": 10623, ":]": 10624, "Ġhij": 10625, "Ðľ": 10626, "Ġbiss": 10627, "Ġblend": 10628, "Ġimplic": 10629, "Ġì½": 10630, "lleicht": 10631, "ÙĬØ©": 10632, "asant": 10633, "erte": 10634, "ĠSame": 10635, "Ġinterior": 10636, "Se": 10637, "Ġbench": 10638, "Ġpoco": 10639, "Ġmarks": 10640, "Ġwins": 10641, "åĸĶ": 10642, "Ġγ": 10643, "Ġdistinct": 10644, "ĠAsian": 10645, "Ġmolec": 10646, "ĠJackson": 10647, "Ġeast": 10648, "Ġphysics": 10649, "imal": 10650, "Ġpeak": 10651, "arian": 10652, "eps": 10653, "Ġneat": 10654, "ĠваÑģ": 10655, "urning": 10656, "Ġsynth": 10657, "Ġreveal": 10658, "ź": 10659, "gon": 10660, "nis": 10661, "ativ": 10662, "ĠLas": 10663, "Ġpy": 10664, "ĠMajesty": 10665, "ĠValley": 10666, "Ġenf": 10667, "Ġgens": 10668, "Ġroots": 10669, "eze": 10670, "bet": 10671, "Ġacts": 10672, "éļ": 10673, "èIJ": 10674, "Ġphilosophy": 10675, "Ġmatches": 10676, "Ŀi": 10677, "Ġjuż": 10678, "Ġdesper": 10679, "ĠEducation": 10680, "Ġspots": 10681, "Ġregions": 10682, "Ar": 10683, "ĠNam": 10684, "een": 10685, "Ġdiagram": 10686, "Ġrely": 10687, "Ġtens": 10688, "Ġdating": 10689, "Ġcoat": 10690, "ĠHor": 10691, "Ġacknowledge": 10692, "ĠPretty": 10693, "Ġпоп": 10694, "Ġvoir": 10695, "Ġfavourite": 10696, "Ġmoż": 10697, "Ġkm": 10698, "ĠDO": 10699, "Ġfert": 10700, "ĠëıĦ": 10701, "ĠPac": 10702, "Ġfont": 10703, "Ġfinds": 10704, "ĠItaly": 10705, "Ġкол": 10706, "Ġcompass": 10707, "ë³": 10708, "liament": 10709, "Ġnotion": 10710, "Ġinject": 10711, "Ġwisdom": 10712, "ĠÃľ": 10713, "ĠMoon": 10714, "ĠBusiness": 10715, "rics": 10716, "ĠYout": 10717, "Ġforgive": 10718, "Ġfinance": 10719, "ilo": 10720, "Ø£": 10721, "ahl": 10722, "Ġdemo": 10723, "Ġclimb": 10724, "Ġexport": 10725, "åł": 10726, "Ġsuccessfully": 10727, "ĠFer": 10728, "pected": 10729, "dem": 10730, "Ġretire": 10731, "Ġlaptop": 10732, "Ġspir": 10733, "ĠAssociation": 10734, "Ġгл": 10735, "ĠSel": 10736, "Ġíķľë": 10737, "Ġemployee": 10738, "Ġmolt": 10739, "RL": 10740, "Я": 10741, "Ġcontra": 10742, "Ġug": 10743, "ĠBall": 10744, "ĠJava": 10745, "érie": 10746, "Ġprocedure": 10747, "Ġgrid": 10748, "ĠëĬIJë": 10749, "Ġbelt": 10750, "ĠÑįÑĤого": 10751, "urd": 10752, "Ġcompreh": 10753, "Ġdeveloper": 10754, "ĠÑįÑĤом": 10755, "åĺ": 10756, "cr": 10757, "Ġëĵ": 10758, "Ġspoken": 10759, "rence": 10760, "Ġtermin": 10761, "Ġaggressive": 10762, "Ġbisschen": 10763, "Ġhasta": 10764, "ĠBrian": 10765, "ĠCommission": 10766, "ĠYu": 10767, "Ġpromised": 10768, "Ġequity": 10769, "iko": 10770, "verty": 10771, "Ġreplaced": 10772, "ĠHelp": 10773, "Ġpose": 10774, "ĠMiddle": 10775, "Ġkim": 10776, "Ġmein": 10777, "ĠCouncill": 10778, "ĠÐĴÑģ": 10779, "oro": 10780, "ĠBern": 10781, "Ġbez": 10782, "Ġanalyt": 10783, "angen": 10784, "Ġìĭ¶": 10785, "ĠGlo": 10786, "Ġquad": 10787, "ÑĤа": 10788, "Ġspeaks": 10789, "ìĺĪìļĶ": 10790, "ĠìŬ룬ë": 10791, "free": 10792, "нÑĸ": 10793, "rich": 10794, "Ġ미": 10795, "ĠDies": 10796, "abb": 10797, "¥¸": 10798, "Ġdepression": 10799, "Ġretail": 10800, "Ħëĵ¤": 10801, "ĠVous": 10802, "ĠLatin": 10803, "á¹": 10804, "Ġì¢ĭìķĦ": 10805, "Ġtort": 10806, "Ġcomputers": 10807, "Ġsearching": 10808, "Ġtub": 10809, "atell": 10810, "Ġmerc": 10811, "Ġglasses": 10812, "person": 10813, "Ġdishes": 10814, "Ġguarantee": 10815, "Ġmeg": 10816, "sm": 10817, "ĠWalk": 10818, "ìľ¼ë©´": 10819, "Ġfolder": 10820, "ĠMit": 10821, "Ġtiming": 10822, "Ġabst": 10823, "ĠLog": 10824, "ãĤ¯": 10825, "Ġapproved": 10826, "ĠUSA": 10827, "веÑĤ": 10828, "Ġwise": 10829, "essed": 10830, "Ġdoub": 10831, "Ġresident": 10832, "Ġgenerated": 10833, "Ġstays": 10834, "Ġexplanation": 10835, "Ġpoison": 10836, "atre": 10837, "Ġinsane": 10838, "Ġreferred": 10839, "aires": 10840, "ĠTRA": 10841, "Ġsei": 10842, "Ġinnoc": 10843, "Ah": 10844, "Ġmant": 10845, "hus": 10846, "Ġouter": 10847, "geb": 10848, "oice": 10849, "Ġdiscussing": 10850, "Ġconvenient": 10851, "__": 10852, "Ġavoir": 10853, "Ġshapes": 10854, "Ġgray": 10855, "Ġdentro": 10856, "Ġmacht": 10857, "Ġ195": 10858, "Ùı": 10859, "Ġadds": 10860, "uting": 10861, "Ġcapabilities": 10862, "Ġsections": 10863, "Ġtune": 10864, "ĠCause": 10865, "arde": 10866, "ĠÑģказ": 10867, "avirus": 10868, "ĠRE": 10869, "Ġtuned": 10870, "Ġleaf": 10871, "terior": 10872, "ĠCaptain": 10873, "Ġج": 10874, "Ġchoosing": 10875, "hin": 10876, "gging": 10877, "viet": 10878, "Ġregret": 10879, "26": 10880, "ondern": 10881, "Ġbonus": 10882, "ĠRay": 10883, "As": 10884, "Ġtorn": 10885, "ĠHier": 10886, "ĠEU": 10887, "Ġrisks": 10888, "Ġama": 10889, "ĠYet": 10890, "Ġcharacteristics": 10891, "Ġê°IJ": 10892, "ĠSenator": 10893, "ĠVamos": 10894, "Ġrose": 10895, "Ġcorporate": 10896, "ghan": 10897, "Ġcenters": 10898, "stairs": 10899, "Ġnit": 10900, "Ġunusual": 10901, "ĠTony": 10902, "ĠGR": 10903, "ĠWild": 10904, "ĠSimilar": 10905, "Ġtodas": 10906, "åģļ": 10907, "Ġhorizont": 10908, "mel": 10909, "Ġstrict": 10910, "Ġcual": 10911, "Ġwrit": 10912, "Ġextended": 10913, "ĠíķĺëĬĶ": 10914, "Ġrelief": 10915, "Ġonion": 10916, "Ġbabies": 10917, "Ġdifer": 10918, "Ġintegrated": 10919, "üzik": 10920, "eping": 10921, "----": 10922, "Ġmens": 10923, "Ġstrategic": 10924, "finitely": 10925, "Ġeigentlich": 10926, "Who": 10927, "åľ°": 10928, "Ġ{": 10929, "Ġä½ł": 10930, "ĠTri": 10931, "Ġpointed": 10932, "ðĿ": 10933, "nament": 10934, "еÑĨ": 10935, "Ġpride": 10936, "ĠRepublican": 10937, "Ġsamples": 10938, "Ġdomestic": 10939, "LY": 10940, "vez": 10941, "Ġwebinar": 10942, "اÙħ": 10943, "Ġenh": 10944, "Ġsuggested": 10945, "Ġmeine": 10946, "Ġpued": 10947, "oren": 10948, "rir": 10949, "Ġheavily": 10950, "Ġinstruction": 10951, "Ġmicrophone": 10952, "Ġigual": 10953, "ĠIra": 10954, "Ġvulnerable": 10955, "ĠVirginia": 10956, "Ġcontinuous": 10957, "Ġpoverty": 10958, "Ġblade": 10959, "ä¸ī": 10960, "Ġrelate": 10961, "Ġcara": 10962, "ĠGoing": 10963, "Ġregional": 10964, "ĠFuck": 10965, "Ġtow": 10966, "ĠMuseum": 10967, "rants": 10968, "Ġбез": 10969, "laim": 10970, "Ġchampion": 10971, "tle": 10972, "ÃŃn": 10973, "encia": 10974, "Ġdiesem": 10975, "ĠDig": 10976, "mates": 10977, "Ġinvesting": 10978, "ĠJordan": 10979, "Ġintegration": 10980, "Ġíİ": 10981, "ห": 10982, "ensus": 10983, "ĠArch": 10984, "Ġpencil": 10985, "алÑĮно": 10986, "issen": 10987, "ĠKa": 10988, "Ġrocks": 10989, "Ġrating": 10990, "Ġrefuge": 10991, "Ġapr": 10992, "eted": 10993, "Ġassistant": 10994, "Ġmeaningful": 10995, "Ġpermanent": 10996, "Ġhill": 10997, "Ġwszyst": 10998, "Ġwound": 10999, "ĠAtl": 11000, "Ġlake": 11001, "ĠFort": 11002, "è¬Ŀè¬Ŀ": 11003, "Ġreduction": 11004, "Ġviv": 11005, "Ġsour": 11006, "Ġecos": 11007, "Ġhaz": 11008, "Ġsteal": 11009, "Ġmyster": 11010, "ĠÐļак": 11011, "ĠÑįÑĤи": 11012, "ĠVietnam": 11013, "Ġantes": 11014, "Ġconnecting": 11015, "éĸĵ": 11016, "ĠDave": 11017, "Ġböyle": 11018, "ĠCast": 11019, "Le": 11020, "Ġcul": 11021, "Ġgenre": 11022, "ë§IJ": 11023, "Ġcomplain": 11024, "Ġhurry": 11025, "arte": 11026, "greg": 11027, "Ġmonitoring": 11028, "Ġdesert": 11029, "ĠÑģов": 11030, "eling": 11031, "ĠSupreme": 11032, "Ġgibi": 11033, "Ġlarg": 11034, "Ġnations": 11035, "ĠTok": 11036, "Ġneedle": 11037, "æµ": 11038, "Ġasleep": 11039, "Ġcomun": 11040, "ĠJews": 11041, "Ġachieved": 11042, "Ġexit": 11043, "Ġdiseases": 11044, "lines": 11045, "ãģĭãĤī": 11046, "riends": 11047, "Ġrect": 11048, "Ġscan": 11049, "ãģ¯ãģĦ": 11050, "Ġhurts": 11051, "zÄĻ": 11052, "ĠLooking": 11053, "ãĤ·": 11054, "íĴ": 11055, "ultural": 11056, "á»ĵ": 11057, "inent": 11058, "Ġpues": 11059, "Ġcheering": 11060, "§Ģ": 11061, "agger": 11062, "Ġada": 11063, "Laughter": 11064, "ĠWomen": 11065, "裡": 11066, "è«": 11067, "Ġoccurred": 11068, "Ġseats": 11069, "èĢĮ": 11070, "Ġempower": 11071, "unu": 11072, "elling": 11073, "BER": 11074, "ensional": 11075, "Ġconsole": 11076, "ashing": 11077, "Ġeinmal": 11078, "fare": 11079, "Ġëı¼": 11080, "Ġsessions": 11081, "ÙIJ": 11082, "Ġridiculous": 11083, "ÃŃan": 11084, "ĠHenry": 11085, "ĠHol": 11086, "Ġcollected": 11087, "Ġdiscussions": 11088, "De": 11089, "Ġdisability": 11090, "ĠíĽ": 11091, "Ġsubscribers": 11092, "Ġirgend": 11093, "Ġfel": 11094, "Ġdirections": 11095, "Ġmanufacturing": 11096, "ĠRod": 11097, "Ġìĸĺ": 11098, "à¸Ĺ": 11099, "æĺİ": 11100, "Ġcriteria": 11101, "Ġmold": 11102, "話": 11103, "Ġentering": 11104, "rij": 11105, "isen": 11106, "ĠPara": 11107, "ieve": 11108, "Ġcharged": 11109, "Ġjou": 11110, "Ġcats": 11111, "лед": 11112, "adays": 11113, "анов": 11114, "jÄĻ": 11115, "vation": 11116, "Ġastron": 11117, "itals": 11118, "ĠBrand": 11119, "ĠKan": 11120, "Ġplain": 11121, "Ġanderen": 11122, "ande": 11123, "Ñıз": 11124, "Ġtoler": 11125, "ÅĤem": 11126, "Ġpré": 11127, "моÑĤÑĢ": 11128, "agement": 11129, "uct": 11130, "ché": 11131, "ĠEner": 11132, "ajÄħ": 11133, "Ġíķ´ë": 11134, "Ġsta": 11135, "Ġrings": 11136, "Ġtoilet": 11137, "ĠCra": 11138, "Ġexperiencing": 11139, "Ġslip": 11140, "Ġsandwich": 11141, "ĠUsing": 11142, "Ġspectrum": 11143, "ĠRos": 11144, "apse": 11145, "ĠJay": 11146, "мÑĥ": 11147, "æ³ķ": 11148, "Ex": 11149, "Ġrecognition": 11150, "ĠDidn": 11151, "uda": 11152, "aje": 11153, "estly": 11154, "Ġfemin": 11155, "iture": 11156, "ÑĢаÑĤ": 11157, "Ġhire": 11158, "Ġnowhere": 11159, "ä½į": 11160, "ầ": 11161, "Ġwing": 11162, "Ġsav": 11163, "ĠSecurity": 11164, "Ġrural": 11165, "ĠFun": 11166, "ayer": 11167, "Ġaccus": 11168, "Ġmm": 11169, "ĠJoseph": 11170, "Ġscreens": 11171, "Ġborrow": 11172, "Ġswing": 11173, "Ġ48": 11174, "Ġtouching": 11175, "this": 11176, "intendo": 11177, "éĥ": 11178, "Ðł": 11179, "ĠScotland": 11180, "ĠJason": 11181, "ĠVen": 11182, "Ġexception": 11183, "Ġnearby": 11184, "Ġbrowser": 11185, "angers": 11186, "ĠSin": 11187, "ÏĢο": 11188, "ä½Ĩæĺ¯": 11189, "ospel": 11190, "Ġwurde": 11191, "Ġdrunk": 11192, "íļ": 11193, "ìĨį": 11194, "ãĥī": 11195, "ĠìĬ¤í": 11196, "ĠLie": 11197, "oco": 11198, "ĠLeague": 11199, "Ġignore": 11200, "Ġ:)": 11201, "Ġlanding": 11202, "ĠعÙĦ": 11203, "ĠTag": 11204, "28": 11205, "Ġdraft": 11206, "Ġaer": 11207, "Ġê·¸ëĥ¥": 11208, "Ġpense": 11209, "Ġдаже": 11210, "Ġbedroom": 11211, "Ġnaj": 11212, "ì§Ģê³ł": 11213, "igenous": 11214, "Ġdeals": 11215, "ello": 11216, "äºĮ": 11217, "Ġposit": 11218, "ê»": 11219, "Ġvisited": 11220, "ifies": 11221, "Ġpremi": 11222, "Ġcant": 11223, "ĠRick": 11224, "Ġraising": 11225, "Ġpermission": 11226, "Ġpubl": 11227, "unci": 11228, "Ġbend": 11229, "Ġchampions": 11230, "die": 11231, "Ġawful": 11232, "Ġjumping": 11233, "Ġlleg": 11234, "Ġsustainable": 11235, "ĠTot": 11236, "Ġcandy": 11237, "åĢĻ": 11238, "Ġsatisfied": 11239, "Ġpipe": 11240, "Ġcock": 11241, "ض": 11242, "stone": 11243, "Ġmomentum": 11244, "ĠÐĿа": 11245, "Ġalors": 11246, "Ġreturns": 11247, "ammen": 11248, "ç®": 11249, "Ñĭм": 11250, "awn": 11251, "otted": 11252, "Ġwollen": 11253, "icted": 11254, "Ġcandidates": 11255, "ĠLady": 11256, "Ġyield": 11257, "Ġmaintenance": 11258, "ffect": 11259, "Ġexpansion": 11260, "ĠLED": 11261, "Ġdarkness": 11262, "Ġoutfit": 11263, "ìķĪ": 11264, "ĠиÑģп": 11265, "ruption": 11266, "ãģĦãģ¾ãģĻ": 11267, "Ġengaging": 11268, "Ġinsight": 11269, "ĠAlways": 11270, "Ġgef": 11271, "rak": 11272, "Ġpix": 11273, "覺å¾Ĺ": 11274, "Ġquantity": 11275, "Ġink": 11276, "ĠKingdom": 11277, "Ġcort": 11278, "常": 11279, "Ġgovernments": 11280, "Ġprotest": 11281, "poon": 11282, "ĠÑĤого": 11283, "å®ĥ": 11284, "uchen": 11285, "quality": 11286, "ĠPorque": 11287, "ĠClub": 11288, "Ġrit": 11289, "Ġarticles": 11290, "BI": 11291, "igible": 11292, "Ġdisaster": 11293, "иг": 11294, "Ġник": 11295, "Ùĩا": 11296, "를": 11297, "aret": 11298, "Ġunable": 11299, "Ġî": 11300, "Ġerst": 11301, "Ġ׳": 11302, "vard": 11303, "Ġannoying": 11304, "ĠKir": 11305, "еÑĢж": 11306, "ennis": 11307, "Ġuncertain": 11308, "36": 11309, "ös": 11310, "Ġecosystem": 11311, "zed": 11312, "jÃł": 11313, "sun": 11314, "ìĸ´ìĦľ": 11315, "Ġżeby": 11316, "Ġmaps": 11317, "ëĤĺ": 11318, "åħ¨": 11319, "ĠJustin": 11320, "Ġtrash": 11321, "Ġenormous": 11322, "Ġstated": 11323, "Ġbrands": 11324, "Ġyout": 11325, "ĠÑĩеловек": 11326, "ĠMatth": 11327, "Ġtransportation": 11328, "Ġlegislation": 11329, "Ġproviders": 11330, "ĠØŃ": 11331, "Ġmagazine": 11332, "Ġsehen": 11333, "ĠDespite": 11334, "Ġpasses": 11335, "æĪIJ": 11336, "Ġalter": 11337, "adan": 11338, "Ġfarmers": 11339, "è°¢": 11340, "Ġconfirmed": 11341, "Ġesa": 11342, "itos": 11343, "Ġroads": 11344, "VIS": 11345, "Ġworker": 11346, "Ġdesigns": 11347, "ĠSoviet": 11348, "brid": 11349, "Ġpracticing": 11350, "Ġë¶Ģ": 11351, "ĠSea": 11352, "ãĥ©": 11353, "ĠпÑĢод": 11354, "Ġchill": 11355, "Ġlemon": 11356, "ìĹIJëĬĶ": 11357, "Ġflexible": 11358, "ĠExcuse": 11359, "Ġterritory": 11360, "åķı": 11361, "ãģ¿": 11362, "Ġlux": 11363, "Ġlifetime": 11364, "Ġdistingu": 11365, "ĠTimes": 11366, "Ġgross": 11367, "enz": 11368, "Ġscroll": 11369, "mÄ±ÅŁ": 11370, "cip": 11371, "£¼": 11372, "DP": 11373, "Ġpublish": 11374, "Ġeben": 11375, "Ġregist": 11376, "Ġedition": 11377, "ĠLE": 11378, "Ġcharging": 11379, "utation": 11380, "yrics": 11381, "idas": 11382, "Ġο": 11383, "ĠкоÑĢ": 11384, "ĠTon": 11385, "缮": 11386, "Ġwhoever": 11387, "ĠFox": 11388, "æīĭ": 11389, "ê±°ëĵłìļĶ": 11390, "Ġfought": 11391, "Ġdrill": 11392, "ĠAfghan": 11393, "~!": 11394, "ĠToo": 11395, "Ġsecondary": 11396, "rä": 11397, "ĠHead": 11398, "innen": 11399, "Ġyarn": 11400, "Ġнам": 11401, "Ġwidth": 11402, "Ġengineer": 11403, "iÄħ": 11404, "Ġwings": 11405, "ĠëķĮ문": 11406, "Ġtrauma": 11407, "Ġreprodu": 11408, "Ġchip": 11409, "Ġpassionate": 11410, "Ġawkward": 11411, "ĠíĬ": 11412, "ажд": 11413, "ĠBitcoin": 11414, "Ġkhông": 11415, "Ġró": 11416, "rection": 11417, "Ġгде": 11418, "ĠUsually": 11419, "Ġimplementation": 11420, "Ġgameplay": 11421, "Ġmystery": 11422, "Ġок": 11423, "Ġaños": 11424, "andy": 11425, "ими": 11426, "Ġprivacy": 11427, "aco": 11428, "ãĤģ": 11429, "Ġdump": 11430, "ĠPay": 11431, "Ġdipl": 11432, "Ġfurn": 11433, "Ġships": 11434, "LA": 11435, "ĠÑħоÑĢоÑĪ": 11436, "Ġec": 11437, "Ġdrops": 11438, "chl": 11439, "32": 11440, "Ġobserve": 11441, "ĠDevelop": 11442, "Müzik": 11443, "annel": 11444, "owaÄĩ": 11445, "Ġfaced": 11446, "ál": 11447, "Ġvictims": 11448, "Ġgifts": 11449, "Ġboot": 11450, "ÃŁe": 11451, "rod": 11452, "Ġ2009": 11453, "ört": 11454, "Ġuniversal": 11455, "Ġnouve": 11456, "Ġboyfriend": 11457, "Ġcetera": 11458, "ÑģÑĤа": 11459, "'S": 11460, "Ġnive": 11461, "Ġcrucial": 11462, "Ġsurve": 11463, "Ġcoin": 11464, "Ġsondern": 11465, "Ġshade": 11466, "Ġlugar": 11467, "Ġsurely": 11468, "Ġmax": 11469, "Ġimproving": 11470, "åĽłçĤº": 11471, "Ġwen": 11472, "Ġ×ij": 11473, "Ġìĸ´ì": 11474, "Ġenforcement": 11475, "ibl": 11476, "Ġliv": 11477, "leri": 11478, "Ġmejor": 11479, "ĠCarolina": 11480, "Ġvas": 11481, "Ġcomprom": 11482, "Ġdirt": 11483, "Ġupgrade": 11484, "ĠBell": 11485, "Ġrestaurants": 11486, "Ġtrap": 11487, "Ġteas": 11488, "blic": 11489, "ĠGreg": 11490, "san": 11491, "Ġow": 11492, "uest": 11493, "Ġproposal": 11494, "ĠRet": 11495, "front": 11496, "Ġpassage": 11497, "Ġsurrounding": 11498, "Ġúlt": 11499, "Ġupcoming": 11500, "Ġhorror": 11501, "Ġclothing": 11502, "Ġìķ½": 11503, "Ġdil": 11504, "rome": 11505, "ĠId": 11506, "ĠRoad": 11507, "ĠÑįÑĤоÑĤ": 11508, "chain": 11509, "ĠбÑĭÑĤÑĮ": 11510, "ĠOffic": 11511, "ĠÐĿе": 11512, "Ġinsan": 11513, "Ġdecrease": 11514, "ĠÑħоÑĤ": 11515, "build": 11516, "ĠDragon": 11517, "åĤ": 11518, "Ġinvestors": 11519, "anti": 11520, "Ġsacrifice": 11521, "Ġtroops": 11522, "ĠBad": 11523, "Ġpassword": 11524, "Ġconstra": 11525, "à¸ķ": 11526, "ĠÃĩa": 11527, "adow": 11528, "through": 11529, "ÑĨа": 11530, "Can": 11531, "Ġcandidate": 11532, "Ġantib": 11533, "ìĹħ": 11534, "Ġtasty": 11535, "ÙĪÙĨ": 11536, "ĠInf": 11537, "ĠBang": 11538, "iÃŁt": 11539, "inity": 11540, "father": 11541, "Ġcontrovers": 11542, "ĠPak": 11543, "ilty": 11544, "구ë": 11545, "Ġlighter": 11546, "Ġfallen": 11547, "Ġzus": 11548, "ĠGuard": 11549, "Ġcott": 11550, "ĠFree": 11551, "Ġinitiative": 11552, "alous": 11553, "Ġnotification": 11554, "ĠMedic": 11555, "ĠCommittee": 11556, "ìŰ": 11557, "ĠWood": 11558, "Ġmush": 11559, "ulum": 11560, "è²": 11561, "ahah": 11562, "Ġsufficient": 11563, "Ġsinger": 11564, "кой": 11565, "ALI": 11566, "ätt": 11567, "ĠPR": 11568, "ĠLar": 11569, "cules": 11570, "iempo": 11571, "Ġunex": 11572, "Ġintegral": 11573, "Ġtransmission": 11574, "Ġici": 11575, "ÑĥÑħ": 11576, "gic": 11577, "ĠNintendo": 11578, "ĠCop": 11579, "ĠTrust": 11580, "enas": 11581, "Ġabilities": 11582, "Ġchips": 11583, "pat": 11584, "Ġanche": 11585, "лен": 11586, "Ġapproaches": 11587, "Ġthor": 11588, "Ġsisters": 11589, "Ġdrivers": 11590, "Ġalla": 11591, "03": 11592, "Ġrubber": 11593, "ĠnÃ¥": 11594, "ACK": 11595, "Ġdisappear": 11596, "ê°ľ": 11597, "Ġcompens": 11598, "Ġvibr": 11599, "ç¬ij": 11600, "GO": 11601, "Ġsizes": 11602, "Ġtracking": 11603, "íĻĶ": 11604, "ĠìĦ¸": 11605, "Ġimpacts": 11606, "ibil": 11607, "fish": 11608, "BR": 11609, "Ġarrow": 11610, "Ġlargely": 11611, "anny": 11612, "Ġlawyer": 11613, "æĢİ麼": 11614, "jours": 11615, "Úº": 11616, "via": 11617, "Ġdella": 11618, "Ġmathemat": 11619, "ĠMine": 11620, "ĠKoll": 11621, "ز": 11622, "ĠCross": 11623, "Ġ65": 11624, "Ġgrac": 11625, "Ġinvolves": 11626, "Ġdelight": 11627, "ĠHollywood": 11628, "Ġimmediate": 11629, "onic": 11630, "Ġlado": 11631, "Ġbullet": 11632, "ĠNote": 11633, "Ġunlock": 11634, "Ġdiscount": 11635, "Ġrising": 11636, "press": 11637, "Ġpace": 11638, "Ġshorter": 11639, "Ġtener": 11640, "geon": 11641, "Ġmanaging": 11642, "Ġcere": 11643, "Chr": 11644, "When": 11645, "achen": 11646, "Ġìĵ": 11647, "ĠHun": 11648, "Ġoft": 11649, "Ġ250": 11650, "ierung": 11651, "Ġstabil": 11652, "ĠConnect": 11653, "Ġyani": 11654, "Ġdownt": 11655, "μα": 11656, "Ġvocal": 11657, "να": 11658, "Ġlean": 11659, "Ġvidéo": 11660, "ĠFamily": 11661, "resent": 11662, "Ġamounts": 11663, "ì§ģ": 11664, "class": 11665, "Ġvib": 11666, "ĠAv": 11667, "arse": 11668, "Ġgentlemen": 11669, "Ġseeking": 11670, "Ġunion": 11671, "Ġregularly": 11672, "æı": 11673, "ĠJahr": 11674, "ĠFood": 11675, "ĠProblem": 11676, "Ġartificial": 11677, "ĠSix": 11678, "Ġimpressed": 11679, "Ġtooth": 11680, "ĠKh": 11681, "Ġyard": 11682, "Ġíķ´": 11683, "Ġowned": 11684, "ĠëıĻ": 11685, "ì²Ń": 11686, "Ġtoda": 11687, "Ġportfol": 11688, "ĠëĤ¨": 11689, "orgeous": 11690, "Ġdates": 11691, "олÑĮз": 11692, "еÑĩно": 11693, "Ġconfiguration": 11694, "Ġrequirement": 11695, "Ġbelly": 11696, "Ġpainful": 11697, "Ġdemonstrate": 11698, "Ġgleich": 11699, "Ġvisiting": 11700, "ĠConf": 11701, "Ġdal": 11702, "Ùij": 11703, "Ġamend": 11704, "ĠFur": 11705, "æ¯Ķ": 11706, "Ġvital": 11707, "á»ĭ": 11708, "Ġmate": 11709, "ĠOu": 11710, "Ġlegacy": 11711, "usting": 11712, "Ġaccommod": 11713, "Ġquoi": 11714, "auen": 11715, "Ġlifestyle": 11716, "CC": 11717, "ään": 11718, "arten": 11719, "Ġminha": 11720, "ró": 11721, "Ġ모": 11722, "Ġformation": 11723, "Ġtrailer": 11724, "peror": 11725, "ĠгÑĢ": 11726, "Ġud": 11727, "zu": 11728, "Ġkommen": 11729, "Ġcave": 11730, "ĠCouncillor": 11731, "Ġthrown": 11732, "Ġtricks": 11733, "LAUGHTER": 11734, "ĠAcademy": 11735, "rowd": 11736, "¡Ŀ": 11737, "ìłĢ": 11738, "ĠImagine": 11739, "Ġinformed": 11740, "roph": 11741, "Ġlig": 11742, "Ġskull": 11743, "abeth": 11744, "Ġfunctional": 11745, "erek": 11746, "On": 11747, "é¦": 11748, "Ġancest": 11749, "Ġsafely": 11750, "ĠHT": 11751, "ëĭ¹": 11752, "Ġdav": 11753, "Ġdrives": 11754, "Americ": 11755, "Ġtire": 11756, "Ġsais": 11757, "ári": 11758, "avors": 11759, "Ġcorresponding": 11760, "Ġprés": 11761, "chest": 11762, "Ġbacteria": 11763, "Ġinfection": 11764, "usal": 11765, "Ġavez": 11766, "Ġbasketball": 11767, "Ġsupplies": 11768, "Ġexpertise": 11769, "ł¥": 11770, "fa": 11771, "Ġtiempo": 11772, "ĠWant": 11773, "Ġsilly": 11774, "Ġupp": 11775, "Ġelected": 11776, "Ġfired": 11777, "Ġد": 11778, "Ġuniversities": 11779, "alle": 11780, "Ġjacket": 11781, "°": 11782, "Ġtrav": 11783, "ls": 11784, "Ġdefeat": 11785, "Ġcogn": 11786, "Ġequations": 11787, "uki": 11788, "ĠSher": 11789, "Ġthirty": 11790, "Ġstreaming": 11791, "otros": 11792, "ĠProdu": 11793, "nej": 11794, "Ġdesigner": 11795, "ĠëĬIJëĤ": 11796, "Ġpainted": 11797, "raine": 11798, "mail": 11799, "Ġvess": 11800, "Ġrhythm": 11801, "lesh": 11802, "Ġ99": 11803, "Ġainda": 11804, "chied": 11805, "Ġétait": 11806, "Ġaffects": 11807, "é£": 11808, "ĠFind": 11809, "Ġél": 11810, "Ġpotatoes": 11811, "Ġpag": 11812, "ĠпаÑĢ": 11813, "arts": 11814, "ĠNach": 11815, "Ġ33": 11816, "ĠHard": 11817, "ĠIraq": 11818, "Ġopinions": 11819, "with": 11820, "erman": 11821, "ý": 11822, "èŃ": 11823, "ĠSPEAK": 11824, "¬¼": 11825, "Ġstability": 11826, "ĠHE": 11827, "Ġcaptured": 11828, "Ġbucks": 11829, "Ġmasks": 11830, "Ġcompete": 11831, "Ġforgotten": 11832, "лÑİÑĩ": 11833, "sequ": 11834, "ĠìĦł": 11835, "illion": 11836, "Ġgraphics": 11837, "Ġhub": 11838, "ĠìŰ": 11839, "empor": 11840, "Ġcrown": 11841, "Ġwider": 11842, "Ġoccurs": 11843, "DS": 11844, "æģ": 11845, "ĠBattle": 11846, "ãģªãĤĵ": 11847, "Ġdual": 11848, "Ġ600": 11849, "athers": 11850, "à¹ģ": 11851, "Ġsettle": 11852, "Ġavait": 11853, "Ġdois": 11854, "киÑħ": 11855, "adores": 11856, "Ġó": 11857, "nego": 11858, "ĠGeorgia": 11859, "ĠRog": 11860, "Ġdivor": 11861, "ĠSong": 11862, "ĠSpecial": 11863, "Ġmun": 11864, "Ġpretend": 11865, "MAN": 11866, "Ġviolent": 11867, "Ġbesides": 11868, "vy": 11869, "ĠNaz": 11870, "29": 11871, "Ġsweat": 11872, "Ġzw": 11873, "ĠHu": 11874, "ĠBuild": 11875, "Ġhorm": 11876, "ĠCard": 11877, "Ġìľł": 11878, "Ġrecommendation": 11879, "called": 11880, "stick": 11881, "ĠPolice": 11882, "Ġconsumers": 11883, "Ġgrocer": 11884, "Ġstun": 11885, "ĠÐĴÑĭ": 11886, "У": 11887, "ĠData": 11888, "Ġsubstant": 11889, "irect": 11890, "à²": 11891, "Ġвз": 11892, "ĠArm": 11893, "Ġsemester": 11894, "ĠBrad": 11895, "Ġours": 11896, "ĠкоÑĤоÑĢÑĭй": 11897, "§a": 11898, "Ġgrams": 11899, "Ġexercises": 11900, "75": 11901, "Ġswear": 11902, "Ġincent": 11903, "Ïģο": 11904, "Ġillegal": 11905, "ä½ķ": 11906, "ĠDamn": 11907, "Ġnú": 11908, "Ġneces": 11909, "Ġcuz": 11910, "icon": 11911, "Ġhors": 11912, "ĠComo": 11913, "ä½ľ": 11914, "ĠëijIJ": 11915, "Ġoverse": 11916, "Ġharvest": 11917, "Ġthrew": 11918, "ĠпоÑĤомÑĥ": 11919, "×Ļ×Ķ": 11920, "Ġotro": 11921, "ĠпеÑĢв": 11922, "Ġscope": 11923, "Ġglory": 11924, "ĠMichigan": 11925, "Ġassuming": 11926, "ĠÑĥд": 11927, "Ġbold": 11928, "gue": 11929, "mother": 11930, "Ġwaren": 11931, "è¬Ľ": 11932, "ĠØ¥": 11933, "ĠKam": 11934, "ispiel": 11935, "Ġtoujours": 11936, "Ġconstitution": 11937, "Ġ~": 11938, "Ġfrankly": 11939, "olen": 11940, "onscious": 11941, "Ġwürde": 11942, "thon": 11943, "ĠOF": 11944, "ìŀIJë": 11945, "unda": 11946, "Ġæĺ¯": 11947, "ĠпоÑĢ": 11948, "Ġemployment": 11949, "ÑijÑĤ": 11950, "ãģģ": 11951, "Ġsteam": 11952, "ĠDI": 11953, "Ġprofessionals": 11954, "Ġengineers": 11955, "ĠXia": 11956, "ç«": 11957, "ìĺģ": 11958, "ĠDun": 11959, "Ġbitch": 11960, "ĠFord": 11961, "ä¸įè¦ģ": 11962, "section": 11963, "Ġvice": 11964, "ĠLater": 11965, "oston": 11966, "ÑįÑĤ": 11967, "צ": 11968, "ĠAzure": 11969, "pling": 11970, "Ġ180": 11971, "ĠCreat": 11972, "ISHA": 11973, "Ġbueno": 11974, "íĿ¬": 11975, "rup": 11976, "lers": 11977, "ĠYang": 11978, "ĠHA": 11979, "bat": 11980, "ĠCatholic": 11981, "Ġaccent": 11982, "Ġmixing": 11983, "ckets": 11984, "Ġenhance": 11985, "ühr": 11986, "ês": 11987, "Ġíĸ": 11988, "Ġswimming": 11989, "Ġcá»§a": 11990, "ĠEliz": 11991, "Ġimmune": 11992, "Ġбол": 11993, "Ġfare": 11994, "ĠGab": 11995, "ס": 11996, "Ġsatell": 11997, "ĠAnything": 11998, "Ġasset": 11999, "Ġschedul": 12000, "Ġradical": 12001, "Ġzwei": 12002, "ĠME": 12003, "related": 12004, "Ġseparated": 12005, "ĠLibr": 12006, "Ġgrip": 12007, "Ġப": 12008, "è¨Ģ": 12009, "Ġbeans": 12010, "ĠOp": 12011, "ìĨĮ": 12012, "Ġpound": 12013, "Ġentrance": 12014, "ÏĨ": 12015, "ĠNie": 12016, "ĠRepublicans": 12017, "Ġatom": 12018, "Ġpersonas": 12019, "ĠAli": 12020, "ähr": 12021, "å¤ĸ": 12022, "Ġdramatic": 12023, "ĠFine": 12024, "Ġreminds": 12025, "èĻ": 12026, "ĠdéjÃł": 12027, "Ġaffordable": 12028, "Ġbran": 12029, "iero": 12030, "alar": 12031, "cu": 12032, "Ġ\\": 12033, "Ġmoże": 12034, "Ġblast": 12035, "Ġrecy": 12036, "fire": 12037, "Ġlle": 12038, "ĠвÑĢемÑı": 12039, "ĠWW": 12040, "Ġvs": 12041, "ĠDude": 12042, "ĠRome": 12043, "Ġgreet": 12044, "ĠHet": 12045, "cias": 12046, "Ġëĭ¹": 12047, "lessly": 12048, "Ġpremium": 12049, "Ġexperiments": 12050, "atar": 12051, "éri": 12052, "Ġofficially": 12053, "Ġfee": 12054, "à¹ĩ": 12055, "ĠÑĩем": 12056, "rea": 12057, "Ġtoy": 12058, "OP": 12059, "ĠTaylor": 12060, "ĠMcC": 12061, "iley": 12062, "ĠBak": 12063, "Ġalum": 12064, "ĠUnter": 12065, "Ġmagical": 12066, "Ġtrabal": 12067, "Ġvotes": 12068, "itage": 12069, "Ġmechanical": 12070, "hn": 12071, "ãģ¾ãģĹãģŁ": 12072, "ĠинÑĤеÑĢ": 12073, "ä»Ĭ天": 12074, "Ġhint": 12075, "Ġauthorities": 12076, "ĠNASA": 12077, "iversary": 12078, "ĠпоÑĩ": 12079, "rac": 12080, "ĠSPEAKER": 12081, "öt": 12082, "Ġframes": 12083, "Ġgoodbye": 12084, "Ġcher": 12085, "hu": 12086, "Ġneur": 12087, "å®ļ": 12088, "ĠMach": 12089, "ĠHell": 12090, "Ġfestival": 12091, "ëħĦ": 12092, "uta": 12093, "Ġmushroom": 12094, "Ġtant": 12095, "Ġtatto": 12096, "Ġdelete": 12097, "Ġdiz": 12098, "Ġvä": 12099, "Ġsevent": 12100, "ĠQuick": 12101, "Ġbaking": 12102, "Ġassembly": 12103, "Go": 12104, "ĠDream": 12105, "ĠLad": 12106, "éĿŀ": 12107, "ây": 12108, "ags": 12109, "Ġgravity": 12110, "Ġì§ij": 12111, "employ": 12112, "Ġdieses": 12113, "Ġdiscovery": 12114, "ÑģÑĤва": 12115, "Ġhebben": 12116, "Ġgerade": 12117, "ĠDR": 12118, "Ġ''": 12119, "Ġtechnically": 12120, "ĠÐŁÐ¾": 12121, "Ġprivilege": 12122, "ĠEver": 12123, "ĠServices": 12124, "uran": 12125, "Ġconsumption": 12126, "ĠRev": 12127, "ĠShall": 12128, "asser": 12129, "¶ĢíĦ°": 12130, "Ġracial": 12131, "ĠYoutube": 12132, "ĠPra": 12133, "ÑģÑĤвен": 12134, "cek": 12135, "æ´": 12136, "asha": 12137, "ĠÛģ": 12138, "ijľ": 12139, "ahn": 12140, "ICK": 12141, "Ġdrinks": 12142, "Ġcarb": 12143, "ãĤ¿": 12144, "Ġ64": 12145, "ĠMmm": 12146, "Ġelectrical": 12147, "Ġfruits": 12148, "ĠHD": 12149, "ña": 12150, "ĠDefinitely": 12151, "Ġë°Ľ": 12152, "Ġfais": 12153, "rations": 12154, "Ġcoe": 12155, "ahu": 12156, "ĠFair": 12157, "Ġeaten": 12158, "Ġfir": 12159, "ĠAu": 12160, "Ñĥн": 12161, "ulating": 12162, "ingly": 12163, "Ġvaccines": 12164, "Ġdragon": 12165, "Ġpointing": 12166, "Ġpelo": 12167, "orters": 12168, "Ġworkout": 12169, "имеÑĢ": 12170, "mond": 12171, "ĠNope": 12172, "Ġ×ĸ×Ķ": 12173, "ĠÙĤ": 12174, "Ġadopted": 12175, "bul": 12176, "Ġsans": 12177, "Ġpossibilities": 12178, "Ġpend": 12179, "Ġzaman": 12180, "hou": 12181, "Ġshares": 12182, "Ġcalendar": 12183, "Ġpersona": 12184, "Ġseal": 12185, "Ġgene": 12186, "Ġstored": 12187, "Ġпоз": 12188, "Ġlyrics": 12189, "Ġinstruments": 12190, "ĠMA": 12191, "ĢìĿ´": 12192, "Ġclouds": 12193, "hot": 12194, "ắ": 12195, "Ġê°ĻìķĦìļĶ": 12196, "ĠEmpire": 12197, "Ġbio": 12198, "wind": 12199, "iego": 12200, "ĠEurop": 12201, "Ġ好": 12202, "edge": 12203, "Ġbackwards": 12204, "Ġì§Ģë": 12205, "Ġqueen": 12206, "Ġshine": 12207, "Ġçık": 12208, "Ġcad": 12209, "ĠOd": 12210, "ĠìĤ¬ëŀĮ": 12211, "Ġbubble": 12212, "ôi": 12213, "zes": 12214, "Ġreactions": 12215, "Ġjudgment": 12216, "ĠDemocrats": 12217, "Ġcosas": 12218, "ashed": 12219, "Ġдолж": 12220, "ÅĽnie": 12221, "ê´": 12222, "Ġexemple": 12223, "MP": 12224, "ữ": 12225, "ĠVers": 12226, "Ġresil": 12227, "Ġmá": 12228, "ÅĦst": 12229, "believ": 12230, "ĠVor": 12231, "Ġscheme": 12232, "onda": 12233, "Ġpodemos": 12234, "Ġcharges": 12235, "Ġdestination": 12236, "ĠKy": 12237, "ĠSS": 12238, "Ġsilence": 12239, "Ġembed": 12240, "nat": 12241, "Ỽi": 12242, "ANT": 12243, "gged": 12244, "Ġreducing": 12245, "Ġugly": 12246, "Ġmim": 12247, "Ñĥда": 12248, "34": 12249, "Ġcord": 12250, "ĠÑĤоже": 12251, "ĠLisa": 12252, "Ġön": 12253, "ĠChristians": 12254, "umbles": 12255, "ologists": 12256, "aza": 12257, "Ġtends": 12258, "ĠCook": 12259, "Ġgesagt": 12260, "ĠíķĺëĤĺ": 12261, "ĠTes": 12262, "eremony": 12263, "ĠнÑĥжно": 12264, "ĠMARISHA": 12265, "Ġenroll": 12266, "ĠCry": 12267, "ESS": 12268, "ĠSad": 12269, "Ġimplemented": 12270, "ĠdÃŃa": 12271, "Ãľ": 12272, "Ġpist": 12273, "Dr": 12274, "Ġsabe": 12275, "ĠSoci": 12276, "äre": 12277, "ĠкÑĤо": 12278, "ĠFrancisco": 12279, "Ġìŀ¥": 12280, "Ġcreatures": 12281, "aws": 12282, "Ġearned": 12283, "Ġcheaper": 12284, "Ġdla": 12285, "Ġwarn": 12286, "sche": 12287, "Ġblah": 12288, "Ġnutr": 12289, "è¼": 12290, "Ġgorgeous": 12291, "ĠAngeles": 12292, "Ġgemacht": 12293, "Ġhomeless": 12294, "ographic": 12295, "ĠTaiwan": 12296, "ĠSom": 12297, "ĠHad": 12298, "actions": 12299, "Ġposts": 12300, "Ġoutra": 12301, "ĠMean": 12302, "kar": 12303, "Ġcous": 12304, "Ġbrack": 12305, "иÑĤÑĮÑģÑı": 12306, "Ġbelieves": 12307, "Ġsuicide": 12308, "Ġequally": 12309, "Ġcares": 12310, "ожно": 12311, "Ġstem": 12312, "ĠMuch": 12313, "Ġproducer": 12314, "×ķ×IJ": 12315, "Ġprotecting": 12316, "ĠTRAVIS": 12317, "Ġinterviews": 12318, "Ġalien": 12319, "ĠAsk": 12320, "Ġsole": 12321, "CO": 12322, "ĠSud": 12323, "Ġsurviv": 12324, "Ġsketch": 12325, "ĠwÅĤa": 12326, "Ġcoloc": 12327, "Ġapologize": 12328, "weight": 12329, "Ġ55": 12330, "Ġ>": 12331, "Ġheroes": 12332, "ĠBoston": 12333, "Ġdependent": 12334, "Ġmotivation": 12335, "flix": 12336, "Ġseam": 12337, "кие": 12338, "Ġdrain": 12339, "oded": 12340, "Ġguilty": 12341, "ĠJenn": 12342, "ingen": 12343, "Ġgranted": 12344, "ĠKelly": 12345, "ĠSav": 12346, "ĠUncle": 12347, "ĠHonestly": 12348, "ELI": 12349, "Ġnavigate": 12350, "Ġblessed": 12351, "core": 12352, "Ġearning": 12353, "Ġsignals": 12354, "Ġdisk": 12355, "ials": 12356, "Ġages": 12357, "æħ": 12358, "Ġparticle": 12359, "ĠÑĩеÑĢ": 12360, "Ġcann": 12361, "Ġtier": 12362, "Ġstatements": 12363, "ê³łìļĶ": 12364, "ĠëķĮ문ìĹIJ": 12365, "ĠCho": 12366, "Ġpolar": 12367, "anç": 12368, "ĠKenn": 12369, "ĠNi": 12370, "ĠFight": 12371, "organ": 12372, "éķ": 12373, "ĠCha": 12374, "ĠSÃŃ": 12375, "ãĥª": 12376, "Ġslic": 12377, "Ġcertific": 12378, "Ġtemplate": 12379, "ĠFederal": 12380, "Ġconsideration": 12381, "Ġexplo": 12382, "ĠMain": 12383, "ĠNE": 12384, "Ġalongside": 12385, "Ġdressed": 12386, "ĠPoint": 12387, "Ġenvironments": 12388, "Ġpróxim": 12389, "Ġdaar": 12390, "Ġprompt": 12391, "Ġpursue": 12392, "Ġentertainment": 12393, "Ġthroat": 12394, "Ġproblema": 12395, "Ġmart": 12396, "ì¼": 12397, "Ġprovider": 12398, "ØĮ": 12399, "Ġ×Ĺ": 12400, "inte": 12401, "making": 12402, "Ġstroke": 12403, "Ġtissue": 12404, "Un": 12405, "Ġprecious": 12406, "ĠArts": 12407, "inking": 12408, "ĠÐŀн": 12409, "ĠиÑģ": 12410, "nah": 12411, "ĠÐķÑģли": 12412, "Ġcorners": 12413, "Ġtricky": 12414, "inch": 12415, "lijk": 12416, "Ġpressing": 12417, "level": 12418, "ANG": 12419, "Ġradiation": 12420, "ìĦł": 12421, "Ġconfront": 12422, "Ġvet": 12423, "Ġrepresentative": 12424, "Ġpropag": 12425, "Ġcrap": 12426, "ĠDec": 12427, "Ġramp": 12428, "епеÑĢÑĮ": 12429, "ués": 12430, "essen": 12431, "cription": 12432, "Ġbills": 12433, "ĠMatthew": 12434, "Ġanime": 12435, "ất": 12436, "Ġlowest": 12437, "has": 12438, "screen": 12439, "ograp": 12440, "ало": 12441, "inton": 12442, "ĠJah": 12443, "èĢħ": 12444, "itÃł": 12445, "Ġkay": 12446, "Ġrotation": 12447, "ĠWere": 12448, "abei": 12449, "Ġtrials": 12450, "Ġlever": 12451, "ighty": 12452, "Ġspoon": 12453, "Ġhunt": 12454, "cling": 12455, "Ġdism": 12456, "ĠболÑĮÑĪ": 12457, "Ġassault": 12458, "Ġíĺķ": 12459, "Ġweekly": 12460, "Ġmismo": 12461, "Ġgenetic": 12462, "ulpt": 12463, "ĠStudent": 12464, "Ġrealistic": 12465, "Ġauthentic": 12466, "æīĵ": 12467, "asta": 12468, "Ġarrested": 12469, "Ġguidelines": 12470, "Ġ׾×IJ": 12471, "Ġдав": 12472, "ĠComing": 12473, "für": 12474, "Ġrequests": 12475, "ĥIJ": 12476, "Ġanalyze": 12477, "Ġinteress": 12478, "Ġhalt": 12479, "ĠOper": 12480, "onom": 12481, "Ġduck": 12482, "Ġwithd": 12483, "ser": 12484, "ĠÏĮ": 12485, "ĠHistory": 12486, "Ġyoutube": 12487, "ãĤį": 12488, "Ġsaber": 12489, "walk": 12490, "font": 12491, "Ġoverview": 12492, "39": 12493, "üy": 12494, "etti": 12495, "Ġfrozen": 12496, "Ġflesh": 12497, "ÄŁi": 12498, "ĠPM": 12499, "ĠìĻĢ": 12500, "é¢": 12501, "ÑĨии": 12502, "Ġ기ë": 12503, "íģ¬": 12504, "Ġprose": 12505, "oooo": 12506, "rates": 12507, "WS": 12508, "Ġautomatic": 12509, "Ġcollecting": 12510, "Åij": 12511, "Ġneighbors": 12512, "».": 12513, "ĠExpl": 12514, "Ġcircul": 12515, "cover": 12516, "weg": 12517, "Ġsticks": 12518, "Ġeller": 12519, "Ġwww": 12520, "Ġdorm": 12521, "ĠExper": 12522, "Ġstatistics": 12523, "Ġemails": 12524, "Ġgrave": 12525, "imiz": 12526, "HS": 12527, "Ġuit": 12528, ",'": 12529, "Ġlaser": 12530, "èī": 12531, "ĠÑĤем": 12532, "ÑĭÑĪ": 12533, "ÑīÑij": 12534, "Ġgenau": 12535, "Ġtienen": 12536, "Ġmeditation": 12537, "ĠOrgan": 12538, "Ġestimate": 12539, "Ġ무ì": 12540, "lets": 12541, "ĠnÃły": 12542, "Ġmindset": 12543, "Ġreson": 12544, "Ġmés": 12545, "Ġnumerous": 12546, "Ġvielleicht": 12547, "ĠThird": 12548, "uous": 12549, "ĠDead": 12550, "анд": 12551, "HN": 12552, "Ġracing": 12553, "Ġagents": 12554, "ĠUt": 12555, "Ġtear": 12556, "ĠHP": 12557, "Ġchemistry": 12558, "Ġsurvival": 12559, "æĸ°": 12560, "Ġconvinced": 12561, "Ġ;": 12562, "Ġregulations": 12563, "ĠES": 12564, "åĴĮ": 12565, "300": 12566, "Ġense": 12567, "Ġìµ": 12568, "Ġdict": 12569, "GA": 12570, "ĠahÃŃ": 12571, "åĭķ": 12572, "Ġtej": 12573, "ĠоÑģÑĤ": 12574, "ĠElect": 12575, "Ġintellectual": 12576, "Ġbias": 12577, "Ġburden": 12578, "çĤ¹": 12579, "Ġìĸ´ëĸ»": 12580, "Ġcheer": 12581, "Ġsoph": 12582, "Ġportfolio": 12583, "uba": 12584, "Ġestos": 12585, "TV": 12586, "For": 12587, "Ġash": 12588, "Ġkommer": 12589, "Ġcollective": 12590, "Ġwrest": 12591, "ĠJetzt": 12592, "ĠWat": 12593, "reich": 12594, "Ġprimer": 12595, "active": 12596, "Ġmie": 12597, "icked": 12598, "Ġhunting": 12599, "Ġtestim": 12600, "Ġcompassion": 12601, "Ġر": 12602, "Ġbrut": 12603, "Ġsalad": 12604, "обÑīе": 12605, "Ġsolving": 12606, "Ġfloating": 12607, "ç·": 12608, "Ġattractive": 12609, "ÙĪÙĦ": 12610, "Ġperd": 12611, "iffer": 12612, "Ġsculpt": 12613, "hhh": 12614, "ĠWeek": 12615, "Ġenthus": 12616, "Ġnad": 12617, "Ġmerch": 12618, "ĠíĻķ": 12619, "Ġmile": 12620, "好äºĨ": 12621, "Ġθ": 12622, "ĠëĤĺë": 12623, "éĩį": 12624, "38": 12625, "Ġchains": 12626, "ĠAlmost": 12627, "Ġtickets": 12628, "rin": 12629, "ĠCC": 12630, "Ġdistributed": 12631, "abetes": 12632, "Ġtemperatures": 12633, "Ġgained": 12634, "Ġflexibility": 12635, "Ġscreaming": 12636, "Ġabroad": 12637, "uno": 12638, "Ġentrepreneurs": 12639, "ĠNetwork": 12640, "ĠCanadian": 12641, "Ġprev": 12642, "Ġsö": 12643, "ĠÑĤебÑı": 12644, "ĠPoke": 12645, "ĠPod": 12646, "ĠTurkey": 12647, "çı¾åľ¨": 12648, "Ġabstract": 12649, "Ġsnake": 12650, "ĠAmy": 12651, "ĠëĬIJëĤĮ": 12652, "Ġbrave": 12653, "ĠìŀĪìĸ´ìļĶ": 12654, "ĠKal": 12655, "Ġ2007": 12656, "ário": 12657, "Ġmarked": 12658, "gines": 12659, "Ġalloc": 12660, "ONG": 12661, "Ġscientist": 12662, "Ġesca": 12663, "Ġracism": 12664, "×ij×": 12665, "ĠSams": 12666, "ĠPenn": 12667, "Ġloads": 12668, "Ġந": 12669, "über": 12670, "Me": 12671, "ixò": 12672, "Ġperò": 12673, "anne": 12674, "Ġexpressed": 12675, "меÑĢ": 12676, "Ġmoet": 12677, "Ġreturning": 12678, "nia": 12679, "Ġexpon": 12680, "Pro": 12681, "Ġloyal": 12682, "ML": 12683, "Ġlamp": 12684, "Ġshy": 12685, "Ġcomposition": 12686, "ĠLy": 12687, "Ġmagnetic": 12688, "Ġpremier": 12689, "Ġmeasured": 12690, "Ġsummary": 12691, "Ġattacked": 12692, "Ġfinishing": 12693, "ÐĹ": 12694, "ç¥": 12695, "Ġsits": 12696, "Ġhydrogen": 12697, "Ġmai": 12698, "ĠDeutsch": 12699, "ası": 12700, "Ġobtain": 12701, "vie": 12702, "Ġsoit": 12703, "Ġë°Ķ": 12704, "Ġlane": 12705, "Ġconsegu": 12706, "во": 12707, "Ġease": 12708, "akin": 12709, "ĠFa": 12710, "Ġuntuk": 12711, "Ġburst": 12712, "Ġcum": 12713, "alım": 12714, "úblic": 12715, "idi": 12716, "ĠRoyal": 12717, "ĠKon": 12718, "Ġcommonly": 12719, "Ġremoving": 12720, "Ġjur": 12721, "ilib": 12722, "Ġanch": 12723, "íĸī": 12724, "ượ": 12725, "ĠÐľÑĭ": 12726, "ĠAnth": 12727, "ĠSÃ¥": 12728, "Ġinterrupt": 12729, "Ġstere": 12730, "ĠOS": 12731, "onym": 12732, "tery": 12733, "ĠMaria": 12734, "ê²ĥ": 12735, "Ġexploring": 12736, "Ġtransparent": 12737, "Ġfate": 12738, "ĠJung": 12739, "Ġgrup": 12740, "Ġdarker": 12741, "ĠDoug": 12742, "Ġmane": 12743, "æĶ¾": 12744, "ại": 12745, "dri": 12746, "look": 12747, "ĠDesign": 12748, "Ġtutaj": 12749, "Ġhorizontal": 12750, "reon": 12751, "orte": 12752, "ĠCorrect": 12753, "ĠSteven": 12754, "Ġvine": 12755, "02": 12756, "iÄĩ": 12757, "Ġsiempre": 12758, "ĠKey": 12759, "åĥı": 12760, "ĠGames": 12761, "Ġnaar": 12762, "Ġshocked": 12763, "elve": 12764, "ĠRose": 12765, "ìĭ¬": 12766, "Ġstopping": 12767, "ohl": 12768, "ĠMix": 12769, "Ġsuffered": 12770, "Ġsigma": 12771, "Ġweakness": 12772, "ĠOw": 12773, "ีà¹Ī": 12774, "IF": 12775, "Ġà®ħ": 12776, "aded": 12777, "ĠNetflix": 12778, "anes": 12779, "Ġremained": 12780, "iry": 12781, "Ġrip": 12782, "ellt": 12783, "Ġsilent": 12784, "Ġproven": 12785, "Ġtoxic": 12786, "Ġalumin": 12787, "Ġmultipl": 12788, "aland": 12789, "Ġ34": 12790, "06": 12791, "ĠBru": 12792, "Ġìłķë§IJ": 12793, "Just": 12794, "boy": 12795, "Ġshoe": 12796, "Ġcreature": 12797, "Ġheaded": 12798, "ĠоÑĤк": 12799, "æ±": 12800, "Ġessence": 12801, "Ġremarkable": 12802, "Ġnúmer": 12803, "Ġdrew": 12804, "Ġpuzzle": 12805, "ĠLibrary": 12806, "ĠFu": 12807, "ashes": 12808, "kk": 12809, "ĠIst": 12810, "¦°": 12811, "ĠBry": 12812, "Ġceremony": 12813, "Ġà®İ": 12814, "Ġcri": 12815, "equ": 12816, "ãĤ¢": 12817, "Ġprize": 12818, "Ġdimensions": 12819, "ogram": 12820, "Ġleather": 12821, "Ġpopulations": 12822, "uum": 12823, "Ġvegan": 12824, "Ñıд": 12825, "Ġcómo": 12826, "åĦ": 12827, "Ġstrip": 12828, "å£": 12829, "Ġvacation": 12830, "ħķ": 12831, "Ġmeals": 12832, "ilipp": 12833, "Ġents": 12834, "aram": 12835, "richt": 12836, "Ġgrain": 12837, "ĠSpain": 12838, "Ġcheek": 12839, "ĠAff": 12840, "ION": 12841, "ĠBring": 12842, "Ġ38": 12843, "ielen": 12844, "ulu": 12845, "ĠболÑĮÑĪе": 12846, "Ġannouncement": 12847, "ĠÑĤÑĥÑĤ": 12848, "ĠProphet": 12849, "ardo": 12850, "37": 12851, "Ġwoke": 12852, "Ġtranslation": 12853, "ĠNOT": 12854, "ĠCL": 12855, "ĠdÃ¼ÅŁ": 12856, "ÑĨÑĸ": 12857, "acer": 12858, "ĠLoc": 12859, "Ġperception": 12860, "NO": 12861, "Ġdiesen": 12862, "Look": 12863, "heart": 12864, "aved": 12865, "Ġboundary": 12866, "Ġflows": 12867, "Ñijм": 12868, "Ġarguments": 12869, "Ġelections": 12870, "ıs": 12871, "Ġheck": 12872, "Ġsuitable": 12873, "Ġfiber": 12874, "ĠStra": 12875, "xy": 12876, "ĠHum": 12877, "Ġmonthly": 12878, "uper": 12879, "Ġgolf": 12880, "Ġlately": 12881, "ĠGard": 12882, "ĠRen": 12883, "ĠAst": 12884, "ĠFant": 12885, "аÑģÑģ": 12886, "Ġobser": 12887, "ë¡ľ": 12888, "Ġeasiest": 12889, "įĶë": 12890, "Ġwebsites": 12891, "pol": 12892, "Ġcocon": 12893, "Ġà®ĩ": 12894, "ĠVeg": 12895, "Ġwalks": 12896, "Ġintro": 12897, "Ġdirected": 12898, "ĠAnna": 12899, "Ġëĵ¤ìĸ´": 12900, "ĠEastern": 12901, "ĠSaint": 12902, "ĠBow": 12903, "Ġroast": 12904, "ĠURL": 12905, "Ġjeden": 12906, "uras": 12907, "aja": 12908, "Ġsemi": 12909, "Ġrapidly": 12910, "Ġtargets": 12911, "ĠControl": 12912, "Ġbah": 12913, "Ġreflection": 12914, "Ġcreativity": 12915, "holders": 12916, "Ġìĺ¬ë": 12917, "Ġamongst": 12918, "Ġfeeding": 12919, "ÑįÑĤомÑĥ": 12920, "Ġвиде": 12921, "Ġë§Įëĵ¤": 12922, "ĠSmart": 12923, "Ġreliable": 12924, "Ġvezes": 12925, "Ġר": 12926, "chuckles": 12927, "azione": 12928, "ĠWilliams": 12929, "Ġaç": 12930, "Ġslee": 12931, "еÑī": 12932, "Ġtimeline": 12933, "Ġthorough": 12934, "á»į": 12935, "ĠOt": 12936, "ạn": 12937, "Ġimagination": 12938, "Ġmechanics": 12939, "rist": 12940, "Ġclaimed": 12941, "ÏĦη": 12942, "ête": 12943, "ĠHurry": 12944, "ĠiPad": 12945, "Ġconstru": 12946, "ĠCla": 12947, "ĠAls": 12948, "ä¼ļ": 12949, "utz": 12950, "Ġcultures": 12951, "Ġìĸ´ëĸ»ê²Į": 12952, "Ġbelongs": 12953, "Ġyer": 12954, "ĠDoesn": 12955, "Ġgeomet": 12956, "Ġbid": 12957, "Ġfoam": 12958, "Ġhob": 12959, "ĠBritain": 12960, "Ġsubstance": 12961, "Ġanniversary": 12962, "ĠëĦĪ": 12963, "Ġnoted": 12964, "Ġgovernor": 12965, "Ġstocks": 12966, "31": 12967, "Ġdiye": 12968, "ìĬ¤ë": 12969, "Ġreb": 12970, "zel": 12971, "Ġmultiply": 12972, "Ġoperator": 12973, "Ħ¤ìļĶ": 12974, "Ġwaters": 12975, "Ġdär": 12976, "Ġunser": 12977, "ĠElizabeth": 12978, "é«ĺ": 12979, "Ġincreasingly": 12980, "ĠGro": 12981, "Ġengines": 12982, "irs": 12983, "Ø«": 12984, "Ġtreasure": 12985, "PC": 12986, "inction": 12987, "iri": 12988, "Ġaccum": 12989, "Ġvariation": 12990, "Ġpom": 12991, "Ġtitles": 12992, "ĠFest": 12993, "ós": 12994, "Ġelder": 12995, "nym": 12996, "run": 12997, "Ñıв": 12998, "Ġinnovative": 12999, "Ġnombre": 13000, "Ġcoinc": 13001, "Ġfranch": 13002, "Ġentonces": 13003, "Ġnichts": 13004, "Ġexclusive": 13005, "ĠCheers": 13006, "ĠBi": 13007, "uje": 13008, "æŃ¡": 13009, "Ġpok": 13010, "ĠPrem": 13011, "Ġrocket": 13012, "ELIPE": 13013, "Ġhospitals": 13014, "rium": 13015, "Ġjuste": 13016, "Ġhammer": 13017, "Ġquantum": 13018, "Ġresponses": 13019, "lly": 13020, "endi": 13021, "Ġactively": 13022, "Ġfridge": 13023, "iate": 13024, "long": 13025, "Ġquem": 13026, "Ġdeaths": 13027, "Ġsuperior": 13028, "cken": 13029, "ìĿ´ìĹIJ": 13030, "ktop": 13031, "Ġgathered": 13032, "£¨": 13033, "Ġdazu": 13034, "Ġrecipes": 13035, "Ġbuzz": 13036, "cen": 13037, "Ġanytime": 13038, "onsense": 13039, "Ġcircles": 13040, "Ġsolved": 13041, "Ġìĭł": 13042, "Ġcoronavirus": 13043, "ĠLuke": 13044, "Ġbubb": 13045, "Ġcontempor": 13046, "rzy": 13047, "ĠJane": 13048, "Ġдом": 13049, "Ġscrews": 13050, "Ġhybrid": 13051, "Ġcasual": 13052, "Ġselbst": 13053, "being": 13054, "ĠÄIJ": 13055, "ĠColumb": 13056, "ĠÑħоÑĩ": 13057, "Ġbucket": 13058, "Ġevaluate": 13059, "Ġidol": 13060, "Ġreputation": 13061, "ĠìĨĮë": 13062, "ÙĪØ±": 13063, "Ġhecho": 13064, "Ġpoem": 13065, "Ġsubjects": 13066, "plant": 13067, "ĠBeh": 13068, "ĠSpeaking": 13069, "Ġbatteries": 13070, "Ġfollowers": 13071, "öl": 13072, "Ġgently": 13073, "Ġsixt": 13074, "Ġparameter": 13075, "Ġikke": 13076, "ĠTour": 13077, "ĠDJ": 13078, "otte": 13079, "ĠJahren": 13080, "Ġpreparation": 13081, "ĠдÑĥм": 13082, "Ġ800": 13083, "cop": 13084, "iking": 13085, "Ġ문": 13086, "ĠнÑĥ": 13087, "ĠлеÑĤ": 13088, "åIJĮ": 13089, "ĠIde": 13090, "Ġì¡°ê¸Ī": 13091, "Ġlaughter": 13092, "Ġmolecules": 13093, "ĠRest": 13094, "Ġobserved": 13095, "dzie": 13096, "Ġadvertising": 13097, "erto": 13098, "Ġmoins": 13099, "ĠMIT": 13100, "Ġexcit": 13101, "Ġtum": 13102, "Ġtyl": 13103, "Ġinvested": 13104, "Ġpharm": 13105, "Ġunexpected": 13106, "Ġphi": 13107, "otype": 13108, "weise": 13109, "Ġgeç": 13110, "jourd": 13111, "Ġhorses": 13112, "nÄħ": 13113, "=\"": 13114, "ĠSM": 13115, "Ġfib": 13116, "Ġclips": 13117, "çķ¶": 13118, "å¦Ĥæŀľ": 13119, "Ġregime": 13120, "Ġrotate": 13121, "rou": 13122, "nik": 13123, "Ġarmor": 13124, "ðŁĺ": 13125, "еÑĢа": 13126, "度": 13127, "ĠOch": 13128, "Ġrichtig": 13129, "üzel": 13130, "aneously": 13131, "mek": 13132, "éĮ¯": 13133, "ĠXiao": 13134, "Ġexisted": 13135, "worth": 13136, "ãģ£ãģ¨": 13137, "Ġnaught": 13138, "ĠheiÃŁt": 13139, "ĠBal": 13140, "Ġresid": 13141, "ivot": 13142, "omatic": 13143, "Ġhired": 13144, "Ġgradually": 13145, "Ġonions": 13146, "Ġcompat": 13147, "Ġintim": 13148, "Ġjew": 13149, "Ġcontribution": 13150, "ĠIre": 13151, "acji": 13152, "Ġslice": 13153, "Ġimmun": 13154, "ĠRus": 13155, "Ġgrows": 13156, "ĠSimilarly": 13157, "Ġhardest": 13158, "Ġstruck": 13159, "Ġmeasurement": 13160, "...]": 13161, "they": 13162, "ĠìłĢë": 13163, "Ġsneak": 13164, "Ġapplies": 13165, "Ġнем": 13166, "æĵ": 13167, "×ijר": 13168, "ĠЧÑĤо": 13169, "Ġoutro": 13170, "Ġinnocent": 13171, "Ġmog": 13172, "ĠSamsung": 13173, "Ġmercy": 13174, "Ġhandling": 13175, "Ġintervention": 13176, "idays": 13177, "got": 13178, "Ġcurric": 13179, "Ġboundaries": 13180, "Ġconfusing": 13181, "Ŀ¼ëĬĶ": 13182, "æĩ": 13183, "Ġstitches": 13184, "ÃŃvel": 13185, "Ġtunnel": 13186, "itä": 13187, "Ġgost": 13188, "imy": 13189, "Ġczas": 13190, "Ġmé": 13191, "Ġcatal": 13192, "ĠSimon": 13193, "ĠLIAM": 13194, "mic": 13195, "ĠФ": 13196, "Ġeyel": 13197, "isas": 13198, "ĠCPU": 13199, "ĠDou": 13200, "Ġnäch": 13201, "Ġinfinity": 13202, "Ġrif": 13203, "ĠPeace": 13204, "ĠCu": 13205, "Ġminimal": 13206, "Ġlistened": 13207, "Ġpole": 13208, "halb": 13209, "Ġloaded": 13210, "Ġsteady": 13211, "ĠBesides": 13212, "êm": 13213, "Ġlap": 13214, "Ġcoop": 13215, "Ġfriendship": 13216, "world": 13217, "Ġgeh": 13218, "Ġtylko": 13219, "ĠLaura": 13220, "Ġsurrounded": 13221, "ĠEvent": 13222, "Ġchap": 13223, "ĠWonder": 13224, "break": 13225, "Ġdrove": 13226, "Ġbroader": 13227, "Ġchi": 13228, "Fi": 13229, "Ġgehen": 13230, "Ġwestern": 13231, "Ġintelligent": 13232, "Ġpersist": 13233, "Ġfounded": 13234, "ãģĵãģ¨": 13235, "Ġhistoric": 13236, "ĠfrÃ¥": 13237, "cksÃ¥": 13238, "Ġhandy": 13239, "Ġsymp": 13240, "Ġrows": 13241, "Ġnutri": 13242, "bur": 13243, "ĠLeon": 13244, "Ġsistema": 13245, "Ġextensive": 13246, "ĠÑĥв": 13247, "íı": 13248, "Ġnights": 13249, "Ġcác": 13250, "Ġcounting": 13251, "ĠMust": 13252, "allow": 13253, "еÑģÑģ": 13254, "Mom": 13255, "Ġнадо": 13256, "Ġbarrel": 13257, "ãĥŀ": 13258, "ARD": 13259, "Ġinstallation": 13260, "Ġinsect": 13261, "Ġëħ¸ë": 13262, "ujÄħ": 13263, "ĠÄiji": 13264, "Ġpacked": 13265, "Ġfiction": 13266, "Now": 13267, "ĠYay": 13268, "Ġpert": 13269, "rons": 13270, "unde": 13271, "aches": 13272, "Ġstyles": 13273, "Ġaprès": 13274, "oku": 13275, "ĠVice": 13276, "ınız": 13277, "comm": 13278, "Ġassigned": 13279, "Ġinteractions": 13280, "Ġacab": 13281, "FELIPE": 13282, "Ġrescue": 13283, "Ġindustries": 13284, "ĠAndy": 13285, "Ġpraise": 13286, "Ġflame": 13287, "Ġsnack": 13288, "íĤ": 13289, "çģ": 13290, "Ġswo": 13291, "render": 13292, "Ġboards": 13293, "ĠÑĤом": 13294, "enne": 13295, "Ġpasta": 13296, "Ġdevil": 13297, "ĠFel": 13298, "Ġhatte": 13299, "Ġcolleg": 13300, "eh": 13301, "ì»": 13302, "ãģĵãģ®": 13303, "Ġproductive": 13304, "forward": 13305, "ип": 13306, "Ġsmartphone": 13307, "Ġinvis": 13308, "Ġbum": 13309, "Ġwhoa": 13310, "ìŀĦ": 13311, "ĠocksÃ¥": 13312, "ĠLang": 13313, "ĠSyria": 13314, "Ġsesi": 13315, "ία": 13316, "Ġapproval": 13317, "48": 13318, "Ġодин": 13319, "Ġëĸ": 13320, "ĠHarr": 13321, "ĠAdminist": 13322, "Ġפ": 13323, "ĠDean": 13324, "fi": 13325, "Ġcitizen": 13326, "Ġshark": 13327, "05": 13328, "Ġboil": 13329, "Ġindicate": 13330, "å¡": 13331, "Are": 13332, "Ġlayout": 13333, "Ġrefr": 13334, "ĠPacific": 13335, "AAAA": 13336, "ĠAustralian": 13337, "gression": 13338, "Voice": 13339, "алÑģÑı": 13340, "Ġshelter": 13341, "To": 13342, "aupt": 13343, "Ġevaluation": 13344, "apor": 13345, "Ġcurrency": 13346, "Ġмного": 13347, "igos": 13348, "ãģ°": 13349, "Ġoct": 13350, "Ġroyal": 13351, "è³": 13352, "asil": 13353, "ĠChildren": 13354, "Ġrien": 13355, "Ġëĵľë": 13356, "Ġbarrier": 13357, "Ġejemplo": 13358, "Ġek": 13359, "ND": 13360, "esp": 13361, "ена": 13362, "Ġpic": 13363, "Ġkiller": 13364, "Ġintegrate": 13365, "Ġfewer": 13366, "Ġdisabilities": 13367, "Ġ....": 13368, "Ġtriangle": 13369, "Ġfees": 13370, "Ġwidely": 13371, "emi": 13372, "Ġoverwhelming": 13373, "Ġzomb": 13374, "Ġbere": 13375, "Ġhood": 13376, "ĠAye": 13377, "ĠHarvard": 13378, "ev": 13379, "ĠÏĦοÏħ": 13380, "Ġcups": 13381, "ĠAuch": 13382, "zona": 13383, "Ġ1990": 13384, "ĠweiÃŁ": 13385, "Ġcrunch": 13386, "æ¥": 13387, "Ġзав": 13388, "Ġmeasuring": 13389, "Ġstations": 13390, "ĠStephen": 13391, "Ġshortly": 13392, "Ġsigning": 13393, "Ġcomedy": 13394, "omo": 13395, "Ġsuggestions": 13396, "Ġsignature": 13397, "ĠпÑĢив": 13398, "Ġdisorder": 13399, "aska": 13400, "Ġworlds": 13401, "Ġprecisely": 13402, "norm": 13403, "rav": 13404, "ĠCivil": 13405, "Inter": 13406, "ĠCertain": 13407, "Ġinjured": 13408, "Ġsuggests": 13409, "ĠGolden": 13410, "Ġcyber": 13411, "ĠØ´": 13412, "Ġtemporary": 13413, "Ġcooper": 13414, "Ġvoted": 13415, "Ġought": 13416, "ấy": 13417, "xual": 13418, "Ġpanels": 13419, "Ġ95": 13420, "Ġhandsome": 13421, "ĠпÑĢов": 13422, "Ġpermit": 13423, "Ġkein": 13424, "Ġbadly": 13425, "Ġnotifications": 13426, "iza": 13427, "ĠNotice": 13428, "Ġinclusive": 13429, "Ġanswering": 13430, "ĠíĹ": 13431, "uld": 13432, "íħĮ": 13433, "Ġnowadays": 13434, "Ġ37": 13435, "Ġbolt": 13436, "Ġstatic": 13437, "ĠHop": 13438, "Ġavant": 13439, "ajo": 13440, "Ġë§ĽìŀĪ": 13441, "Ġfifty": 13442, "ĠFinal": 13443, "Ġscores": 13444, "ĠTap": 13445, "Ġcyl": 13446, "Ġconvince": 13447, "Ġanyways": 13448, "oda": 13449, "Ġìķ¼": 13450, "Ġserves": 13451, "ĠÑĤакой": 13452, "ĠZoom": 13453, "Ġsavings": 13454, "ulo": 13455, "Ġsouthern": 13456, "viewer": 13457, "Ġhoje": 13458, "Ġseja": 13459, "Ġrepresenting": 13460, "Īëįĺ": 13461, "lik": 13462, "ĠSomebody": 13463, "Ġbeast": 13464, "Ġsticking": 13465, "Ġinsist": 13466, "Ġtalented": 13467, "Ġexplaining": 13468, "Ġattorney": 13469, "éĥ¨": 13470, "Ġstairs": 13471, "ĠDog": 13472, "íĭ": 13473, "Ġcig": 13474, "Ġshaped": 13475, "Ġsons": 13476, "Ïģι": 13477, "utt": 13478, "ĠìĶ": 13479, "Ġparad": 13480, "ìĿ¸ëį°": 13481, "Ġhorn": 13482, "ĠJour": 13483, "anno": 13484, "Ġworldwide": 13485, "åĬĽ": 13486, "Ġparticipation": 13487, "¦Ħ": 13488, "Ġmów": 13489, "Ġburned": 13490, "Ġwriters": 13491, "allah": 13492, "ĠFund": 13493, "Ġclever": 13494, "ĠLeute": 13495, "bin": 13496, "Ġbeating": 13497, "foot": 13498, "ĠìĽIJ": 13499, "ĠStudio": 13500, "Ġvag": 13501, "bey": 13502, "rze": 13503, "Ġopposition": 13504, "Ġжиз": 13505, "who": 13506, "Ġê±´": 13507, "Ġtrace": 13508, "ĠденÑĮ": 13509, "Ġepid": 13510, "Ġgesch": 13511, "ĠNar": 13512, "ĠBE": 13513, "Ñĥй": 13514, "ĠSign": 13515, "edly": 13516, "Ġclay": 13517, "Ġinstantly": 13518, "Ġgathering": 13519, "ĠGalaxy": 13520, "Ġbored": 13521, "ĠBuddh": 13522, "cé": 13523, "Ġmam": 13524, "Ġslope": 13525, "Ġëĭ¤ìĿĮ": 13526, "Ġschön": 13527, "Ġpir": 13528, "gef": 13529, "amer": 13530, "Ġhö": 13531, "Ġcolleague": 13532, "Ġpresents": 13533, "adium": 13534, "Ġவ": 13535, "Ġfalar": 13536, "beep": 13537, "Ġdried": 13538, "isms": 13539, "Ġrope": 13540, "Ġworkshop": 13541, "Ġestud": 13542, "Ġbands": 13543, "Ġthemes": 13544, "åħ¬": 13545, "ÙĬر": 13546, "åIJİ": 13547, "Ġreminder": 13548, "ÑĤÑĥ": 13549, "ĠBh": 13550, "Ġcoconut": 13551, "ĠÑģÑĤо": 13552, "ĠChannel": 13553, "Ġimmigration": 13554, "äs": 13555, ".....": 13556, "主": 13557, "çϽ": 13558, "stop": 13559, "ĠкаÑĢ": 13560, "Ġcoins": 13561, "ĠÑĩаÑģ": 13562, "Ġdestruction": 13563, "lined": 13564, "Ġbarriers": 13565, "antine": 13566, "Ġprinted": 13567, "Ġcongratulations": 13568, "ĠHeart": 13569, "Ġinqu": 13570, "tha": 13571, "Ġhardly": 13572, "ĠAven": 13573, "Ġtinha": 13574, "ĠSony": 13575, "ĠNF": 13576, "Ġgraduates": 13577, "Ġsqueeze": 13578, "eremy": 13579, "ÏĦι": 13580, "Ġepic": 13581, "ĠJu": 13582, "Ġolm": 13583, "ĠLaughter": 13584, "Ġbeliefs": 13585, "ĠCru": 13586, "ĠTrue": 13587, "ĠSoul": 13588, "oween": 13589, "Ġromantic": 13590, "Ġзв": 13591, "Ġanos": 13592, "ĠYup": 13593, "éĺ¿": 13594, "dim": 13595, "Ġinfer": 13596, "Ġзам": 13597, "Ġsoc": 13598, "uka": 13599, "Ġprecise": 13600, "Ġdropping": 13601, "Ġclue": 13602, "Ġerrors": 13603, "charge": 13604, "ĠPu": 13605, "ometer": 13606, "Ġlambda": 13607, "acional": 13608, "ĠDong": 13609, "Ġchamber": 13610, "Ġthankful": 13611, "ĠNu": 13612, "ĠHawai": 13613, "Ġinfo": 13614, "Ġactivate": 13615, "ĠQual": 13616, "Ġqued": 13617, "ÑĥлÑĮ": 13618, "Ġcloth": 13619, "åĸľ": 13620, "Ġwichtig": 13621, "55": 13622, "Ġotra": 13623, "ographer": 13624, "Ġcurios": 13625, "Ġ1980": 13626, "Ġempres": 13627, "dess": 13628, "eur": 13629, "Ġcluster": 13630, "arter": 13631, "obile": 13632, "ĠYan": 13633, "ĠAdv": 13634, "Ġdiscipline": 13635, "ĠìłķëıĦ": 13636, "ĠPlace": 13637, "ĠSelect": 13638, "TE": 13639, "ĠбÑĭла": 13640, "Ġwhis": 13641, "Ġbay": 13642, "ĠDor": 13643, "encing": 13644, "Ġrepet": 13645, "Ġficar": 13646, "pad": 13647, "Ġfog": 13648, "uyor": 13649, "Ġsnap": 13650, "ibt": 13651, "Ġsobie": 13652, "Ġappointment": 13653, "ĠRy": 13654, "Ġceiling": 13655, "ourse": 13656, "Ġwrites": 13657, "ĠAfghanistan": 13658, "Ġmos": 13659, "aze": 13660, "Ġpenal": 13661, "Ġcrystal": 13662, "ICE": 13663, "ê°IJ": 13664, "éŁ": 13665, "ĠTesla": 13666, "Ġtheories": 13667, "Ġappeal": 13668, "Ġnewspaper": 13669, "Ġcookies": 13670, "æ©": 13671, "ĠاÙĦÙĦ": 13672, "Ġmaj": 13673, "ĠGetting": 13674, "kommen": 13675, "ĠHeaven": 13676, "ells": 13677, "Ġdivine": 13678, "Ä«": 13679, "Ġakt": 13680, "Ġhopes": 13681, "ĠChen": 13682, "wegen": 13683, "***": 13684, "ĠFrage": 13685, "Ġни": 13686, "ู": 13687, "minister": 13688, "nesota": 13689, "which": 13690, "Ġexplicit": 13691, "Ġverdad": 13692, "Ġgraduated": 13693, "ĠPhilipp": 13694, "QL": 13695, "ĠMI": 13696, "Ġdevot": 13697, "Ġcure": 13698, "Ġclosest": 13699, "ĠÃĦ": 13700, "Ġsexy": 13701, "ãģĽ": 13702, "ĠDeath": 13703, "oko": 13704, "ugu": 13705, "ĠAnne": 13706, "itarian": 13707, "esa": 13708, "егод": 13709, "ĠDur": 13710, "Ġ000": 13711, "zeit": 13712, "Ġtournament": 13713, "Ġmelhor": 13714, "ส": 13715, "Ġindu": 13716, "Ġflaw": 13717, "Ġwars": 13718, "ĠMind": 13719, "ĠIron": 13720, "ÑĤак": 13721, "ĠVR": 13722, "Ġsiz": 13723, "ĠSouthern": 13724, "Ġê·¸ëŁ¬ë": 13725, "Ġawak": 13726, "Ġìķŀ": 13727, "Ġcube": 13728, "believable": 13729, "ifall": 13730, "dis": 13731, "Ġabandoned": 13732, "mind": 13733, "Ġparl": 13734, "Ġclassical": 13735, "èĭ": 13736, "á»Ļt": 13737, "ĠAuto": 13738, "ĠBor": 13739, "ç©": 13740, "400": 13741, "ĠSociety": 13742, "Ġsubtle": 13743, "Ġmissions": 13744, "Ġremembered": 13745, "ĠEither": 13746, "Ġdafür": 13747, "ORD": 13748, "Ġintensity": 13749, "ESIN": 13750, "ĠCup": 13751, "Ġrarely": 13752, "Ġtoys": 13753, "ĠCharlie": 13754, "ợ": 13755, "Ġglaube": 13756, "Ġrounds": 13757, "TIN": 13758, "Ġcapability": 13759, "Ġderivative": 13760, "Ġreferring": 13761, "ĠdÃ¥": 13762, "ĠTALI": 13763, "Ġcotton": 13764, "Ġconfer": 13765, "Ġcolumns": 13766, "Ġliberal": 13767, "Ġnunca": 13768, "Ġμε": 13769, "Ġindo": 13770, "iben": 13771, "ĠBeispiel": 13772, "Ġê·¸ëłĩ": 13773, "ĠÑĥÑĩ": 13774, "Ġhoy": 13775, "Ġfry": 13776, "ĠScottish": 13777, "èĬ": 13778, "Ġciv": 13779, "Ġconservative": 13780, "Ġairpl": 13781, "Ġsar": 13782, "rus": 13783, "Ġinvestments": 13784, "Ġinfinite": 13785, "Ġà®ķ": 13786, "ĠTALIESIN": 13787, "ĠGary": 13788, "uell": 13789, "Ġак": 13790, "ĠCir": 13791, "Ġritual": 13792, "Ġ>>>": 13793, "Ġtempt": 13794, "ĠTech": 13795, "ĠPokemon": 13796, "Ġimprovements": 13797, "Ġspare": 13798, "Ġtranslate": 13799, "Ġsonra": 13800, "ĠFilm": 13801, "wort": 13802, "Ġми": 13803, "Ġperiods": 13804, "Ġjealous": 13805, "ãģĦãģĦ": 13806, "Ġtir": 13807, "MI": 13808, "Ġconducted": 13809, "ĠìķĪëħķ": 13810, "09": 13811, "ĠPolit": 13812, "ĠWhereas": 13813, "Ġmoisture": 13814, "Ġsins": 13815, "Ġkap": 13816, "ĠÑįк": 13817, "Ġbenim": 13818, "Ġeliminate": 13819, "Ġathletes": 13820, "ĠManager": 13821, "Ġfeatured": 13822, "apore": 13823, "äºĽ": 13824, "Ġë°ľ": 13825, "Ġperf": 13826, "ĠThus": 13827, "Ġdebut": 13828, "обÑĢ": 13829, "Ġseñ": 13830, "Ġmysterious": 13831, "words": 13832, "Ķê°Ģ": 13833, "Ġchecks": 13834, "Ġvolunteer": 13835, "Ġwashing": 13836, "ĠMarvel": 13837, "ĠAB": 13838, "issors": 13839, "!'": 13840, "ĠFull": 13841, "yeon": 13842, "Ġweigh": 13843, "ĠJOHN": 13844, "Ġvos": 13845, "Ġprocedures": 13846, "Ġaddressed": 13847, "ĠBerlin": 13848, "puter": 13849, "ĠBan": 13850, "Ġmedication": 13851, "Ġdrone": 13852, "ĠÑĥб": 13853, "ĠJean": 13854, "Ġcaps": 13855, "Ġdisappointed": 13856, "Ġwore": 13857, "ĠêµŃ": 13858, "Ġorganize": 13859, "ĠHalloween": 13860, "Ġfantasy": 13861, "yard": 13862, "Ġnosotros": 13863, "Ġjumped": 13864, "Ġphotography": 13865, "ĠName": 13866, "rec": 13867, "AB": 13868, "Ġblessing": 13869, "ĠShut": 13870, "Ġbitter": 13871, "pop": 13872, "ãģĿãĤĮ": 13873, "Ġdei": 13874, "Ġfulfill": 13875, "çIJĨ": 13876, "Ġdengan": 13877, "Ġbelo": 13878, "ĠMeanwhile": 13879, "Ġdepois": 13880, "Ġdiabetes": 13881, "Ġbund": 13882, "ĠZealand": 13883, "Ġdigest": 13884, "Ġtires": 13885, "Ġdod": 13886, "agne": 13887, "ết": 13888, "Ġpeel": 13889, "Ġзаб": 13890, "Ġnodes": 13891, "Ġtrends": 13892, "ĠSwitch": 13893, "ĠAward": 13894, "ĠOrig": 13895, "ĠHal": 13896, "Ġestas": 13897, "Ġ360": 13898, "Ġsimult": 13899, "Ġcomic": 13900, "ĠmÃł": 13901, "Ġbalanced": 13902, "ĠPrincess": 13903, "Ġkilometers": 13904, "ứ": 13905, "Ġpartir": 13906, "ì¤ij": 13907, "soft": 13908, "ĠView": 13909, "Ġbiological": 13910, "inst": 13911, "44": 13912, "Ġmanera": 13913, "Ġcomprehensive": 13914, "ĠSab": 13915, "Ġcrimes": 13916, "yers": 13917, "ĠCompany": 13918, "ĠPhot": 13919, "Ġpouco": 13920, "iac": 13921, "Ġbeim": 13922, "inate": 13923, "Ġsubsequ": 13924, "ĠMayor": 13925, "Ġcenturies": 13926, "ères": 13927, "ìŀĸìķĦìļĶ": 13928, "Ġê·¸ëŁ¼": 13929, "ĠFrau": 13930, "ĠOH": 13931, "ĠëģĿ": 13932, "ĠNah": 13933, "ĠSeries": 13934, "Ġovernight": 13935, "íĴĪ": 13936, "ĠâĢ¢": 13937, "Ġtrave": 13938, "attered": 13939, "Ġwarri": 13940, "ĠGrund": 13941, "ĠIndones": 13942, "Ġscra": 13943, "oby": 13944, "ĠBrook": 13945, "Ġcurs": 13946, "Ġë¸": 13947, "Ġexplains": 13948, "ramatic": 13949, "Ġparticipating": 13950, "Ġminut": 13951, "Ġcontracts": 13952, "Ġgegen": 13953, "Ġdisappeared": 13954, "ĠSN": 13955, "Ġrobust": 13956, "aph": 13957, "Ġshrim": 13958, "Ġdevast": 13959, "cope": 13960, "Ġmeets": 13961, "Ġpeaceful": 13962, "mate": 13963, "Ġweld": 13964, "Ġת": 13965, "don": 13966, "ÑĥÑĤÑĮ": 13967, "Ġregistered": 13968, "ĠNik": 13969, "jin": 13970, "Ġcav": 13971, "Ġecht": 13972, "iox": 13973, "Ġflowing": 13974, "ноÑģÑĤи": 13975, "Ġtoe": 13976, "Ġentity": 13977, "ова": 13978, "fits": 13979, "ĠPatrick": 13980, "ÑĤÑĢ": 13981, "Ġleverage": 13982, "Ġcorrel": 13983, "iah": 13984, "Ġstrings": 13985, "istinct": 13986, "Ġgue": 13987, "archy": 13988, "Ġtengo": 13989, "ımız": 13990, "Ġorbit": 13991, "为": 13992, "ĠеÑīÑij": 13993, "cake": 13994, "Ġ׾×Ķ": 13995, "ĠMinnesota": 13996, "Ġbrake": 13997, "owie": 13998, "Ġcraw": 13999, "기를": 14000, "Ġprogramme": 14001, "ĠÑģлÑĥÑĩ": 14002, "åıª": 14003, "iences": 14004, "ĠOui": 14005, "ĠPers": 14006, "imiento": 14007, "ĠInvest": 14008, "Ġslower": 14009, "æĻĤåĢĻ": 14010, "ĠBeth": 14011, "Ġnurse": 14012, "ĠSpring": 14013, "Sp": 14014, "Ġunemploy": 14015, "ди": 14016, "Ġgenius": 14017, "ĠAaron": 14018, "Ġê·¸ëŁ¬": 14019, "Ġei": 14020, "ãģĹãĤĩ": 14021, "Ġtanks": 14022, "Ġaujourd": 14023, "Ġcomplexity": 14024, "ĠÑĢеÑĪ": 14025, "Ġoldest": 14026, "Ġletz": 14027, "åħ¥": 14028, "Ġphenomenon": 14029, "print": 14030, "ĠBundes": 14031, "itat": 14032, "ê»ĺ": 14033, "Ġ42": 14034, "ĠWi": 14035, "Ġincom": 14036, "Ġgek": 14037, "Ġembrace": 14038, "Ġties": 14039, "oute": 14040, "Ġdose": 14041, "ĠFriends": 14042, "ÑĭÑĤ": 14043, "егоднÑı": 14044, "Ġorg": 14045, "Ħë¡ľ": 14046, "óg": 14047, "Ġexceed": 14048, "Ġgods": 14049, "Ġê±°ìĺĪìļĶ": 14050, "Ġsociet": 14051, "ĠUnivers": 14052, "ität": 14053, "Ġworden": 14054, "Ġsmoking": 14055, "Ġintens": 14056, "abul": 14057, "emia": 14058, "èij": 14059, "47": 14060, "fly": 14061, "Ġ2006": 14062, "ĠSeriously": 14063, "Ġprzez": 14064, "æ¼": 14065, "cre": 14066, "Ġnan": 14067, "Ġmodes": 14068, "оваÑĤÑĮ": 14069, "ĠHang": 14070, "emen": 14071, "Ġbeneficial": 14072, "Ġvoters": 14073, "ĠBroad": 14074, "Ġbent": 14075, "Wow": 14076, "Ġmul": 14077, "åĵ¥": 14078, "ĠUC": 14079, "Ġdamaged": 14080, "ĠUkraine": 14081, "Ġwipe": 14082, "Ġstones": 14083, "Ġmanagers": 14084, "Ġrab": 14085, "ÑģÑĤÑĢо": 14086, "lat": 14087, "Ġdece": 14088, "Ġgraphic": 14089, "Ġfoss": 14090, "Ġdisagree": 14091, "ĠAmen": 14092, "Ġsecrets": 14093, "hole": 14094, "inkle": 14095, "Ġfortunate": 14096, "Ġì±": 14097, "ìľĦ": 14098, "èIJ¬": 14099, "Ġhabits": 14100, "Ġburied": 14101, "Ġhin": 14102, "Ġvirtually": 14103, "olas": 14104, "ĠRP": 14105, "ĠTab": 14106, "low": 14107, "Ġsacrific": 14108, "Ġestimated": 14109, "oln": 14110, "Ùĭ": 14111, "cur": 14112, "ĠFeel": 14113, "Ġcastle": 14114, "Ġuseless": 14115, "Ġdisg": 14116, "ĠJacob": 14117, "Ġgaan": 14118, "Ġupside": 14119, "Ġparece": 14120, "ãĥ³ãĥ": 14121, "Ġshipping": 14122, "ĠCR": 14123, "Ġdisrupt": 14124, "acter": 14125, "UND": 14126, "fu": 14127, "å®Į": 14128, "ĠPick": 14129, "ĠCharl": 14130, "ĠBull": 14131, "Ġenterprise": 14132, "Ġpunishment": 14133, "acking": 14134, "Ġfraction": 14135, "Ġtablet": 14136, "Ġchord": 14137, "Ġsimilarly": 14138, "åħ¶å¯¦": 14139, "ĠToronto": 14140, "Ġcourts": 14141, "ÄŁl": 14142, "eszcze": 14143, "Ġpronoun": 14144, "ĠSister": 14145, "ĠMP": 14146, "Ġgreatly": 14147, "ĠDank": 14148, "icop": 14149, "Ġgarbage": 14150, "Ġresolve": 14151, "ĠSaf": 14152, "ĠGun": 14153, "Ġcompound": 14154, "Ġë°°": 14155, "ĠMusik": 14156, "âĻ«": 14157, "Ġchaos": 14158, "ĠWhenever": 14159, "Ġeuros": 14160, "Ġorchest": 14161, "Ġrefriger": 14162, "alan": 14163, "ื": 14164, "ĠAmazing": 14165, "Ġpud": 14166, "agan": 14167, "Ġjeszcze": 14168, "isy": 14169, "Ġaccuracy": 14170, "ĠAma": 14171, "isode": 14172, "ëĮĢ": 14173, "Ġinterpretation": 14174, "ĠLiber": 14175, "æ·": 14176, "cam": 14177, "Ġevolved": 14178, "ĠKay": 14179, "ÑĨÑĭ": 14180, "Ġcreator": 14181, "itas": 14182, "Ġalarm": 14183, "Ġcelebration": 14184, "zent": 14185, "Ġfuncion": 14186, "Ġov": 14187, "umbling": 14188, "Ġ%": 14189, "à¸Ī": 14190, "Ġrestrictions": 14191, "Ġнав": 14192, "ĠKinder": 14193, "Ġbanana": 14194, "ÑĮÑı": 14195, "Ġdiameter": 14196, "Ġnorthern": 14197, "urers": 14198, "ĠPas": 14199, "æĪijçļĦ": 14200, "Ġworkforce": 14201, "Ġjung": 14202, "Ġguarante": 14203, "Ġequilib": 14204, "Ġsuite": 14205, "Ġeuro": 14206, "Ġdeliber": 14207, "Ste": 14208, "Ġdowntown": 14209, "Ġchin": 14210, "Ġcodes": 14211, "edia": 14212, "Ġsheep": 14213, "reshold": 14214, "wnie": 14215, "ób": 14216, "Ġunderlying": 14217, "lia": 14218, "jer": 14219, "ÏĢÏĮ": 14220, "çĿ": 14221, "throp": 14222, "Ġzap": 14223, "Ġvacuum": 14224, "ĠHab": 14225, "Ġwrapped": 14226, "ì¢": 14227, "Ġinventory": 14228, "ма": 14229, "Ġcoord": 14230, "Ġplates": 14231, "Ġsymm": 14232, "Te": 14233, "ĠwÅĤaÅĽnie": 14234, "Ġreaches": 14235, "Ġlonely": 14236, "Script": 14237, "lee": 14238, "esser": 14239, "Ġ걸": 14240, "ĠGesch": 14241, "ĠMoving": 14242, "Ġrép": 14243, "ĠVill": 14244, "åIJĪ": 14245, "ĠRachel": 14246, "Ġtemos": 14247, "ONE": 14248, "Ġstrain": 14249, "Ġangel": 14250, "ĠfÃ¥": 14251, "Tr": 14252, "Ġacho": 14253, "Ġhighlights": 14254, "ĠWer": 14255, "ĠCarl": 14256, "Ġblur": 14257, "Ġregards": 14258, "·": 14259, "илÑģÑı": 14260, "Ġrecre": 14261, "ĠYani": 14262, "UCK": 14263, "ł¸": 14264, "Ġelectrons": 14265, "ĠSpiel": 14266, "Ġved": 14267, "Ú¾": 14268, "Ġbeam": 14269, "Ġidiot": 14270, "ëĵ¤": 14271, "наÑĩ": 14272, "idd": 14273, "Ġski": 14274, "itative": 14275, "Ġhypothes": 14276, "ãģ§ãģĻãģŃ": 14277, "enter": 14278, "ĠìķĦëĭĪë": 14279, "Ġihre": 14280, "Ġpreview": 14281, "angel": 14282, "Ġdemon": 14283, "Ġdus": 14284, "Ġdic": 14285, "ĠKom": 14286, "LEY": 14287, "...!": 14288, "Ġsieht": 14289, "ĠSonic": 14290, "Ġtenho": 14291, "anas": 14292, "Ġdigit": 14293, "ĠMaar": 14294, "Ġundergrad": 14295, "ouncer": 14296, "uffy": 14297, "Ġconversion": 14298, "Ġdisconnect": 14299, "Ġecho": 14300, "omer": 14301, "Ġcurriculum": 14302, "Ġperché": 14303, "Ġwand": 14304, "..?": 14305, "Ġrolled": 14306, "Ġentrepreneur": 14307, "Ġtheoret": 14308, "ĠÑīо": 14309, "Ġinsights": 14310, "Ġzusammen": 14311, "oin": 14312, "rett": 14313, "produ": 14314, "Ġvisitors": 14315, "eous": 14316, "Ġgrandmother": 14317, "Ġhumor": 14318, "ĠниÑħ": 14319, "zenia": 14320, "inson": 14321, "Ġreset": 14322, "Ġbaseball": 14323, "Ġmatching": 14324, "ëĭ¤ê°Ģ": 14325, "Ġpunto": 14326, "ì¡": 14327, "Ġrede": 14328, "Ġaddressing": 14329, "Ġforecast": 14330, "ĠBol": 14331, "Ġcolored": 14332, "Ġdocumentation": 14333, "Ġexpectation": 14334, "ĠNorthern": 14335, "Ġcreo": 14336, "Ġà®ļ": 14337, "fon": 14338, "Ġunsere": 14339, "UM": 14340, "Ġcopies": 14341, "Ġexpanded": 14342, "Ġveterans": 14343, "ĠAlm": 14344, "ĠвообÑīе": 14345, "Ġpsychological": 14346, "Ġnosso": 14347, "Ġpayments": 14348, "imeters": 14349, "Ġ-->": 14350, "ĠJennifer": 14351, "Ġvolunteers": 14352, "osse": 14353, "orious": 14354, "ĠбÑĭли": 14355, "èĤ": 14356, "ĠEss": 14357, "ws": 14358, "ĠBC": 14359, "ĠIC": 14360, "Woman": 14361, "Ġvont": 14362, "Ġethnic": 14363, "ENN": 14364, "имо": 14365, "Ġlob": 14366, "Ġoui": 14367, "cs": 14368, "Ġrehe": 14369, "Ġìłģ": 14370, "Ġchick": 14371, "úsica": 14372, "Ġkont": 14373, "ĠDistrict": 14374, "Ġpile": 14375, "Ġав": 14376, "ейÑģÑĤв": 14377, "Ġ£": 14378, "Ġissued": 14379, "Ġкомп": 14380, "Ġprosper": 14381, "Ġprofound": 14382, "ĠDear": 14383, "Ġãģĵ": 14384, "Ġfunded": 14385, "Ġbisa": 14386, "ŀĺë": 14387, "ף": 14388, "ĠìĿĺ": 14389, "Ġtwelve": 14390, "ĠChampions": 14391, "éĿŀ常": 14392, "Ñģл": 14393, "Ġ2005": 14394, "pm": 14395, "Ġonde": 14396, "Ġdiffé": 14397, "ĠChall": 14398, "Ġdifficulties": 14399, "Ġgarage": 14400, "Ġdá": 14401, "ünk": 14402, "Ġ물": 14403, "Ġtran": 14404, "Ġsubmitted": 14405, "zw": 14406, "ÙĪØ§": 14407, "Ġark": 14408, "ĠìĦ±": 14409, "Ġgrocery": 14410, "она": 14411, "iere": 14412, "Ġaest": 14413, "Ġexhibition": 14414, "Ġrés": 14415, "Ġconsistency": 14416, "Ġcookie": 14417, "ней": 14418, "Ġreplacement": 14419, "æ²¹": 14420, "ĠSem": 14421, "ĠìĤ¬ìļ©": 14422, "800": 14423, "Ġgenes": 14424, "Ġtransaction": 14425, "ĠEL": 14426, "Ġdurante": 14427, "ibles": 14428, "ĠEat": 14429, "tail": 14430, "issance": 14431, "Ġtoss": 14432, "Ġsurvived": 14433, "Ġoffices": 14434, "Ġsupportive": 14435, "Where": 14436, "Ġtoutes": 14437, "Ġë§ī": 14438, "Ġjokes": 14439, "ieron": 14440, "apers": 14441, "Ġmature": 14442, "ĠMarsh": 14443, "Ġsido": 14444, "kind": 14445, "Ġrealmente": 14446, "ĠChef": 14447, "Ġquelque": 14448, "Ġjudges": 14449, "eft": 14450, "ERS": 14451, "Ġjet": 14452, "Ġpersons": 14453, "è»": 14454, "izations": 14455, "rik": 14456, "Ġshops": 14457, "ĠWy": 14458, "Ġeleg": 14459, "què": 14460, "quoi": 14461, "Ġjuga": 14462, "Ġíķľë²Ī": 14463, "ĠQuestion": 14464, "ĠGlobal": 14465, "Ġìķ½ê°Ħ": 14466, "ĠStation": 14467, "æİ¥": 14468, "ĠOhio": 14469, "Ġsticky": 14470, "Ġstressed": 14471, "Ġgün": 14472, "ĠíĿ": 14473, "ÑģÑĤÑĥп": 14474, "é¡Į": 14475, "ĠPhD": 14476, "immer": 14477, "Ġmentor": 14478, "Ġinvented": 14479, "Ġreun": 14480, "Ġinevit": 14481, "ĠpolÃŃt": 14482, "Ġexecute": 14483, "ĠStory": 14484, "Ġoutstanding": 14485, "Ġguer": 14486, "ĠRain": 14487, "Ġchoses": 14488, "ĠTit": 14489, "ĠÑģеÑĢ": 14490, "ĠSingapore": 14491, "ĠNone": 14492, "Ġchronic": 14493, "°ëį°": 14494, "Ġego": 14495, "æł·": 14496, "EST": 14497, "ãģĤãĤĬ": 14498, "ĠWang": 14499, "ĠNAT": 14500, "Ġaug": 14501, "Ġdesktop": 14502, "Ġeternal": 14503, "ĠìĤ¬ìĭ¤": 14504, "ĠConstitution": 14505, "ìĤ¬ë": 14506, "×Ļ׾": 14507, "pres": 14508, "ĠТÑĭ": 14509, "Ġinterf": 14510, "Ġlists": 14511, "Ġfights": 14512, "ften": 14513, "ĠIowa": 14514, "Ġmotivated": 14515, "ĠHosp": 14516, "Ġelsewhere": 14517, "Ġpaths": 14518, "Ġinstances": 14519, "Bl": 14520, "range": 14521, "á»±": 14522, "ĠSit": 14523, "mana": 14524, "Ġìĭľìŀij": 14525, "Ġmình": 14526, "ansas": 14527, "Ġsna": 14528, "Ġphilosoph": 14529, "Ġpasse": 14530, "ưá»Ŀi": 14531, "akh": 14532, "ental": 14533, "Ġihn": 14534, "ructor": 14535, "ĠваÑĪ": 14536, "Ġgenerous": 14537, "Ġpivot": 14538, "пол": 14539, "Ġjamais": 14540, "Ġcoment": 14541, "ĠLew": 14542, "odzi": 14543, "ĠXbox": 14544, "Ġвод": 14545, "Ġconsent": 14546, "īìŀ¥": 14547, "Ġdispar": 14548, "lass": 14549, "ĠGovernor": 14550, "Beifall": 14551, "Ġê°ľ": 14552, "Ġbeloved": 14553, "׳×ķ": 14554, "sell": 14555, "Ġhonored": 14556, "leh": 14557, "Ġwäre": 14558, "unting": 14559, "Ġfraud": 14560, "ĠRAM": 14561, "걸": 14562, "Ġkills": 14563, "Ġeconomics": 14564, "04": 14565, "пеÑĢ": 14566, "Ġcoisas": 14567, "ĠигÑĢ": 14568, "ÃŃm": 14569, "Ġmöchte": 14570, "Ġìµľ": 14571, "Ġstimul": 14572, "Ġfastest": 14573, "lv": 14574, "Ġgén": 14575, "ĠSounds": 14576, "Ġ1970": 14577, "Ġhomework": 14578, "speaking": 14579, "Ġencouraging": 14580, "Ġquery": 14581, "Ġrevers": 14582, "profit": 14583, "Ġdy": 14584, "Ġìŀij": 14585, "ëĬĶëį°ìļĶ": 14586, "Ġsoap": 14587, "ĠGall": 14588, "ĠCN": 14589, "ĠAns": 14590, "Ġfic": 14591, "anks": 14592, "Ġdessert": 14593, "ĠìłĢíĿ¬": 14594, "ĠMaking": 14595, "Ġcomeç": 14596, "ê³Ħ": 14597, "Ġassociation": 14598, "Dad": 14599, "hee": 14600, "Ġhogy": 14601, "Ġapro": 14602, "Ġinvisible": 14603, "American": 14604, "íİ": 14605, "Ġvibe": 14606, "Ġemissions": 14607, "Ġadvocate": 14608, "Ġkicked": 14609, "Ġvel": 14610, "Ġsummar": 14611, "Ġfreaking": 14612, "chron": 14613, "Ġpinch": 14614, "Ġwszystk": 14615, "iscal": 14616, "Ġproved": 14617, "Ġmindful": 14618, "Ġtä": 14619, "Ġnoises": 14620, "Ġisolated": 14621, "Ġcrossed": 14622, "Ġê°ķ": 14623, "ĠvoilÃł": 14624, "Ġchore": 14625, "ĠRA": 14626, "Com": 14627, "Ġrelaxed": 14628, "atro": 14629, "Ġprevention": 14630, "Voiceover": 14631, "OD": 14632, "ĠCovid": 14633, "Ġseparation": 14634, "Ġ-[": 14635, "иÑĩего": 14636, "çϼ": 14637, "ĠSD": 14638, "bleep": 14639, "Ġindependence": 14640, "Ġpartial": 14641, "Ġalgorithms": 14642, "ĠAnyone": 14643, "Ġassociate": 14644, "hum": 14645, "icular": 14646, "Ġbạn": 14647, "Ġbattles": 14648, "Good": 14649, "Applause": 14650, "Ġbastante": 14651, "Ġadvant": 14652, "ĠSweet": 14653, "Ġrefused": 14654, "ãĤ¸": 14655, "ĠÑĤебе": 14656, "plet": 14657, "Ġencouraged": 14658, "åĵ¦": 14659, "Ġmiracle": 14660, "ĠBun": 14661, "ĠVar": 14662, "rimination": 14663, "elect": 14664, "ĠMult": 14665, "Ġdelivering": 14666, "eing": 14667, "Ġcm": 14668, "nehmen": 14669, "ĠLine": 14670, "Ġë§Į": 14671, "enced": 14672, "ĠSound": 14673, "ĠContin": 14674, "ijd": 14675, "UNG": 14676, "kle": 14677, "Ġthreshold": 14678, "Ġcompact": 14679, "adt": 14680, "Ġtoes": 14681, "ĠPur": 14682, "owned": 14683, "mented": 14684, "Ġdesigning": 14685, "Ġvaccinated": 14686, "Ġexhaust": 14687, "Ġbasics": 14688, "Ġconsists": 14689, "ĠGuy": 14690, "aczy": 14691, "ĠmÃŃ": 14692, "won": 14693, "害": 14694, "Ġ85": 14695, "æĤ": 14696, "Ġmum": 14697, "Ġignor": 14698, "Ġprinting": 14699, "acular": 14700, "pow": 14701, "Ġexpanding": 14702, "Ġgir": 14703, "ĠCab": 14704, "íĺ¸": 14705, "ÑĤÑĮÑģÑı": 14706, "ĠìŬ룬ë¶Ħ": 14707, "Ġangles": 14708, "Ġterminal": 14709, "ĠWon": 14710, "ĠInteresting": 14711, "Ġcrossing": 14712, "Ġbonds": 14713, "Ġpueden": 14714, "Ġorb": 14715, "ların": 14716, "Ġcreepy": 14717, "Ġnutrition": 14718, "Ġallies": 14719, "Ġwireless": 14720, "Ġdesired": 14721, "Ġcompute": 14722, "ĠArizona": 14723, "ĠBeautiful": 14724, "Ġproduces": 14725, "Ġnuestro": 14726, "ted": 14727, "Ġeligible": 14728, "ĠÑģоз": 14729, "icial": 14730, "ĠHero": 14731, "Ġconsume": 14732, "Ġrobots": 14733, "Ġpurchased": 14734, "cción": 14735, "Ġiz": 14736, "ược": 14737, "ίναι": 14738, "ĠØ£ÙĨ": 14739, "Ġshadows": 14740, "ĠMedia": 14741, "Ġprincess": 14742, "Ġklar": 14743, "Ġwooden": 14744, "Ġusar": 14745, "Ġgüzel": 14746, "Ġslot": 14747, "rade": 14748, "ĠëĴ": 14749, "Ġharmon": 14750, "Ġingredient": 14751, "orship": 14752, "eki": 14753, "Ġgrandfather": 14754, "Ġexcitement": 14755, "Ġpoliticians": 14756, "..!": 14757, "Ġouts": 14758, "Ġseparately": 14759, "ĠÑıк": 14760, "ĠWelt": 14761, "ĠPow": 14762, "jan": 14763, "Ġorientation": 14764, "åıĭ": 14765, "LC": 14766, "agem": 14767, "ÛĮÚº": 14768, "åIJĹ": 14769, "Ġbranches": 14770, "aden": 14771, "rente": 14772, "ĠIhr": 14773, "asm": 14774, "Ġestão": 14775, "ĠNic": 14776, "Ġslave": 14777, "Ġcompress": 14778, "crowd": 14779, "Ġclimbing": 14780, "ĠManagement": 14781, "ĠBah": 14782, "Ġpanic": 14783, "Ġkor": 14784, "Ġcooling": 14785, "Ġbind": 14786, "Ġзад": 14787, "Ġrack": 14788, "Ġentit": 14789, "Ġsends": 14790, "Ġyourselves": 14791, "des": 14792, "ĠMuslims": 14793, "Ġíļ": 14794, "isma": 14795, "cycle": 14796, "unkt": 14797, "ĠCore": 14798, "Ġinjuries": 14799, "Ġidentical": 14800, "каÑı": 14801, "ĠDeutschland": 14802, "Ġее": 14803, "isan": 14804, "Ġtruc": 14805, "leton": 14806, "Ġbackup": 14807, "Ġultra": 14808, "Ġabund": 14809, "illeurs": 14810, "ĠbyÅĤo": 14811, "åħĥ": 14812, "orted": 14813, "Ġearthqu": 14814, "Ġкл": 14815, "Ġobservation": 14816, "Ġmaintenant": 14817, "elen": 14818, "Ġsettled": 14819, "Ġpela": 14820, "ĠEconom": 14821, "ĠÕ": 14822, "Ġsteering": 14823, "ĠALL": 14824, "ĠCher": 14825, "Ġpatience": 14826, "ĠSnow": 14827, "Ġbor": 14828, "Ġworthy": 14829, "Ġcái": 14830, "Ġ×§": 14831, "Ġκα": 14832, "dog": 14833, "ĠKaren": 14834, "illes": 14835, "β": 14836, "Ġagriculture": 14837, "×ķף": 14838, "ĠSean": 14839, "Ġsensors": 14840, "íķ´ë": 14841, "agh": 14842, "Ġpublicly": 14843, "Ġpeux": 14844, "ĠAlexander": 14845, "Ġpriorit": 14846, "Ġlazy": 14847, "ardon": 14848, "attering": 14849, "Ġcostume": 14850, "ست": 14851, "è¿ĺ": 14852, "Ġunw": 14853, "ÐĽ": 14854, "Ġthickness": 14855, "quito": 14856, "gunt": 14857, "istas": 14858, "neys": 14859, "ĠëIJĺê²Į": 14860, "ĠBrasil": 14861, "Ġtoken": 14862, "Ġaffili": 14863, "lon": 14864, "ĠfÃ¥r": 14865, "ĠBeach": 14866, "Ġwitch": 14867, "ĠSeven": 14868, "Ġpant": 14869, "λλ": 14870, "Ġcaptain": 14871, "åĿ": 14872, "Ġveut": 14873, "Ġpouvoir": 14874, "acz": 14875, "ĠBarb": 14876, "Ġutility": 14877, "Ġcontemporary": 14878, "Ġobtained": 14879, "Ġpaintings": 14880, "ear": 14881, "Ġpean": 14882, "ĠOg": 14883, "Ġcust": 14884, "лем": 14885, "Ĥĺë": 14886, "ĠIsso": 14887, "Ġaconte": 14888, "ĠTele": 14889, "ĠAssistant": 14890, "Ãī": 14891, "íĸĪìĬµëĭĪëĭ¤": 14892, "Ġcounts": 14893, "Ġbuck": 14894, "ĠDeep": 14895, "Ġtackle": 14896, "Ġharsh": 14897, "Ġdecides": 14898, "éĹľ": 14899, ".âĢĭ": 14900, "éĤĬ": 14901, "ĠAngel": 14902, "Ġlaying": 14903, "Ġcalories": 14904, "Ġcontrolling": 14905, "Ġadvantages": 14906, "ĠÑįÑĤой": 14907, "Ġapproaching": 14908, "Ġthreats": 14909, "akan": 14910, "ematic": 14911, "mann": 14912, "ê³µ": 14913, "mumbles": 14914, "ació": 14915, "Ġmaintaining": 14916, "Ġfounder": 14917, "lah": 14918, "fight": 14919, "Ġadmitted": 14920, "â̦.": 14921, "ķĮ": 14922, "abol": 14923, "Ġusage": 14924, "Ġnonsense": 14925, "ĠPalest": 14926, "Ġcontre": 14927, "ĠDemocratic": 14928, "ĠER": 14929, "jekt": 14930, "Ġarbit": 14931, "Ġгол": 14932, "ĠMichelle": 14933, "icher": 14934, "esh": 14935, "ĠPho": 14936, "ком": 14937, "49": 14938, "ĠEnergy": 14939, "οÏį": 14940, "Ġcents": 14941, "Ġrefers": 14942, "Ġgospel": 14943, "ĠSha": 14944, "ĠShare": 14945, "×Ļ׳": 14946, "Ġclinic": 14947, "ĠëĦ£": 14948, "Ġequality": 14949, "ugs": 14950, "Ġshed": 14951, "Ġplanes": 14952, "Ġtoute": 14953, "reck": 14954, "Ġstrand": 14955, "Ġbiology": 14956, "Ġleague": 14957, "ĠPok": 14958, "Ġnúmero": 14959, "ĠCoast": 14960, "Ġconsistently": 14961, "Ġnucle": 14962, "OOOO": 14963, "Ġobjet": 14964, "Ġchor": 14965, "Ġginger": 14966, "Ġdabei": 14967, "Ġcooperation": 14968, "à¯į.": 14969, "nten": 14970, "ç¤": 14971, "lÃł": 14972, "ìĸij": 14973, "rado": 14974, "Ġpassive": 14975, "Ġgloves": 14976, "Ġunderground": 14977, "Ġlogical": 14978, "Ġket": 14979, "Ġfunctionality": 14980, "¸ë¦¬": 14981, "Ġportal": 14982, "eller": 14983, "×Ļר": 14984, "ĠTed": 14985, "ĠGre": 14986, "IJľ": 14987, "Ġpersonnel": 14988, "Ġemerging": 14989, "ĠFür": 14990, "Ġmeantime": 14991, "usalem": 14992, "ĠClear": 14993, "Ġtrapped": 14994, "Ġìļ°": 14995, "Ġdispl": 14996, "Ġmettre": 14997, "Ġmunicip": 14998, "Ġwithdraw": 14999, "Ġspat": 15000, "unes": 15001, "Ġaccessibility": 15002, "æĪij们": 15003, "Ġapare": 15004, "Ġprospect": 15005, "Ġназ": 15006, "Ġcopper": 15007, "ĠPRO": 15008, "ÏħÏĦ": 15009, "Ġattacking": 15010, "ĠVin": 15011, "ĠStone": 15012, "Ġinvestigate": 15013, "style": 15014, "Ġλ": 15015, "ë¡Ŀ": 15016, "ë§Ī": 15017, "Ġinspect": 15018, "Ġliver": 15019, "алиÑģÑĮ": 15020, "Ġsera": 15021, "halten": 15022, "eman": 15023, "Ġministry": 15024, "''": 15025, "Ġdots": 15026, "ãħĭãħĭãħĭãħĭ": 15027, "ÑĥÑģÑĤ": 15028, "ĠJak": 15029, "AKE": 15030, "Ġgaps": 15031, "ucker": 15032, "ĠинÑĤеÑĢеÑģ": 15033, "ĠEmily": 15034, "Ġinterval": 15035, "Ġtender": 15036, "ĠTechnology": 15037, "game": 15038, "Ġtrib": 15039, "ÙĦا": 15040, "ĠDevelopment": 15041, "Ùħا": 15042, "Ġwrist": 15043, "Ġfires": 15044, "Ġtargeted": 15045, "ìłIJ": 15046, "Ġsod": 15047, "íļĮ": 15048, "ĠolduÄŁ": 15049, "Ġseasons": 15050, "ventions": 15051, "Ġнего": 15052, "Ġsometime": 15053, "лив": 15054, "né": 15055, "Ġtú": 15056, "ĠDeus": 15057, "Ġexecution": 15058, "áp": 15059, "ĠChange": 15060, "ĠIndeed": 15061, "Ġregulation": 15062, "ĠHung": 15063, "éis": 15064, "Ġwishes": 15065, "Ġjazz": 15066, "Ġstructural": 15067, "Ġblowing": 15068, "ĠbyÄĩ": 15069, "Ġthermal": 15070, "phant": 15071, "ÑĢÑĥз": 15072, "анÑĤ": 15073, "ĠPull": 15074, "Ġconfusion": 15075, "нÑĭми": 15076, "Ġscenarios": 15077, "ìłģìľ¼ë¡ľ": 15078, "ĠдеÑĤ": 15079, "Ġtattoo": 15080, "Ġautre": 15081, "Ġheating": 15082, "Ġtreating": 15083, "Ġпоним": 15084, "Ġexclus": 15085, "ĠLOL": 15086, "wear": 15087, "agle": 15088, "Ġzurück": 15089, "Ġrational": 15090, "su": 15091, "Ġdeter": 15092, "ĠNative": 15093, "à®ķள": 15094, "ached": 15095, "Ġãĥ": 15096, "ĠEntonces": 15097, "Ġhora": 15098, "ìĿ´ìĹIJìļĶ": 15099, "Ġlite": 15100, "ë": 15101, "Ġsixth": 15102, "Ġболее": 15103, "actor": 15104, "Ġpsychology": 15105, "缸": 15106, "Ġdemands": 15107, "Ġpeer": 15108, "Ġnewly": 15109, "ĠWWE": 15110, "Donald": 15111, "ĠBox": 15112, "Ġpine": 15113, "Ġloading": 15114, "ĠNico": 15115, "ĠsÅĤ": 15116, "omme": 15117, "ART": 15118, "Ġrecruit": 15119, "Ġbugs": 15120, "arents": 15121, "ĠпÑĢоб": 15122, "ĠInside": 15123, "ipper": 15124, "dramatic": 15125, "Ġplanets": 15126, "orde": 15127, "Ġyoga": 15128, "child": 15129, "ĠMarie": 15130, "ĠãģĤ": 15131, "ĠBL": 15132, "Ġfilmed": 15133, "Ġrefresh": 15134, "Ġtomatoes": 15135, "Ġfet": 15136, "Qué": 15137, "Ġ!!": 15138, "ĠëĤ´ë": 15139, "rine": 15140, "Ġinteractive": 15141, "sal": 15142, "annah": 15143, "pez": 15144, "ç¶ĵ": 15145, "Ġunderstands": 15146, "ĠTokyo": 15147, "Ġlibraries": 15148, "Ġreader": 15149, "ijIJ": 15150, "oz": 15151, "ĠEnde": 15152, "ĠFlo": 15153, "Ġmild": 15154, "Ġpoetry": 15155, "Ġжив": 15156, "æĦĽ": 15157, "Ġbehave": 15158, "Ġdoen": 15159, "ĠSusan": 15160, "page": 15161, "raham": 15162, "Ġcommunications": 15163, "Ġtuning": 15164, "Ġpac": 15165, "Ġanxious": 15166, "IO": 15167, "Mark": 15168, "Ġhiç": 15169, "books": 15170, "Ġpiss": 15171, "Ġenabled": 15172, "achelor": 15173, "ĠFOR": 15174, "Ġéc": 15175, "ĠTR": 15176, "ilst": 15177, "hat": 15178, "ĠìĿĮ": 15179, "Ġtych": 15180, "Ġjar": 15181, "Ġbuilds": 15182, "ĠArgent": 15183, "Ġintermedi": 15184, "Ġlou": 15185, "Ġara": 15186, "Ġassignment": 15187, "Ġcabinet": 15188, "Ġretirement": 15189, "ãģ»": 15190, "Ġdisabled": 15191, "rica": 15192, "Ġawards": 15193, "Ġboots": 15194, "Ġacknowled": 15195, "Ġthy": 15196, "Ġ구": 15197, "Ġsynd": 15198, "ний": 15199, "ilton": 15200, "Ġprobl": 15201, "ĠFal": 15202, "Ġverdade": 15203, "Ġ700": 15204, "ĠLearning": 15205, "ocus": 15206, "Ġpalace": 15207, "Not": 15208, "tain": 15209, "cm": 15210, "Ġmagnet": 15211, "incoln": 15212, "Ġfiguring": 15213, "ĠLyn": 15214, "ĠBoss": 15215, "ĠVO": 15216, "Ġdiagnosis": 15217, "Ġequipped": 15218, "watch": 15219, "inos": 15220, "aders": 15221, "Ġshelf": 15222, "Ġorganis": 15223, "Ġnod": 15224, "Ġkız": 15225, "ppers": 15226, "Ġrestore": 15227, "Ġartic": 15228, "ĠVoice": 15229, "ıyorum": 15230, "격": 15231, "Ġspreading": 15232, "Ġhips": 15233, "Ġward": 15234, "ureau": 15235, "Ġintersection": 15236, "66": 15237, "Ġ39": 15238, "ç³": 15239, "Ġwaited": 15240, "ì´": 15241, "hhhh": 15242, "Ġdys": 15243, "ĠEN": 15244, "Ġbatch": 15245, "Ġcaf": 15246, "Ġmarker": 15247, "大家好": 15248, "orable": 15249, "ória": 15250, "Ġstepped": 15251, "Ġcelebrating": 15252, "ана": 15253, "Ġworn": 15254, "ĠFol": 15255, "Ġpla": 15256, "Ġattempts": 15257, "Ġtweet": 15258, "Ġrust": 15259, "gence": 15260, "íĨµ": 15261, "Ġrevel": 15262, "Ġrecept": 15263, "eness": 15264, "Ġ((": 15265, "ãĥ¼ãĥ": 15266, "!âĢĭ": 15267, "ĠìĨIJ": 15268, "Ġinfluenced": 15269, "иж": 15270, "ĠконеÑĩно": 15271, "Ġcolleges": 15272, "ioni": 15273, "Ġsag": 15274, "Ann": 15275, "olar": 15276, "Ġexpressions": 15277, "Ġsuits": 15278, "Ġownership": 15279, "eland": 15280, "piece": 15281, "æĢİä¹Ī": 15282, "Ġdespués": 15283, "Ġtel": 15284, "Ġinsult": 15285, "Ġêµīìŀ¥": 15286, "ĠSmall": 15287, "ĠFR": 15288, "oka": 15289, "berries": 15290, "ĠAnton": 15291, "елÑı": 15292, "ÑıÑģ": 15293, "Ġvalve": 15294, "acts": 15295, "Ġwoods": 15296, "ண": 15297, "Ġcultiv": 15298, "Ġfá": 15299, "ãģ¨ãģĦãģĨ": 15300, "Ġcheers": 15301, "Ġassumption": 15302, "Ġfitness": 15303, "ÃŃcul": 15304, "Ġpodr": 15305, "Ġweit": 15306, "ĠHind": 15307, "Ġdign": 15308, "Ġзн": 15309, "Ġsquad": 15310, "Ġdestro": 15311, "cere": 15312, "shirt": 15313, "immt": 15314, "engers": 15315, "Ġsä": 15316, "kÅĤad": 15317, "ĠÈĻ": 15318, "Ġoccas": 15319, "Ġì¤Ħ": 15320, "Ġprocessor": 15321, "ĠDM": 15322, "ĠDaddy": 15323, "Ġsooner": 15324, "Ġstraightforward": 15325, "Ġdepartments": 15326, "ĠChrome": 15327, "Ġworkplace": 15328, "ĠPython": 15329, "Ġmeng": 15330, "ĠDAN": 15331, "ĠIce": 15332, "ĠëĪĪ": 15333, "ĠGi": 15334, "Ġhiring": 15335, "Ġlanded": 15336, "Ġdemocratic": 15337, "iedz": 15338, "ãģĺãĤĥ": 15339, "Ġsev": 15340, "icia": 15341, "Ġespecial": 15342, "ĠNous": 15343, "Ġhät": 15344, "Ġbou": 15345, "pert": 15346, "iesz": 15347, "åijĢ": 15348, "Ġvil": 15349, "ÅĽli": 15350, "Ġîn": 15351, "Ġlosses": 15352, "éķ·": 15353, "Ġtoast": 15354, "Ġrealm": 15355, "ĠAustin": 15356, "ĠInformation": 15357, "Ġresume": 15358, "Ġchase": 15359, "Ġsalary": 15360, "Ġë¶Ħ": 15361, "лиÑĩ": 15362, "ĠÑģлед": 15363, "ĠFurther": 15364, "Ġcaring": 15365, "Ġvig": 15366, "Ġvalor": 15367, "è¿Ļ个": 15368, "ĠÑĩа": 15369, "Ġanalytics": 15370, "Ġglobe": 15371, "ĠMAN": 15372, "Ġnel": 15373, "ìĿ´ìķ¼": 15374, "ټ": 15375, "Ġoy": 15376, "íķĺìĦ¸ìļĶ": 15377, "jen": 15378, "Ġtroubles": 15379, "ahaha": 15380, "Ġchurches": 15381, "uet": 15382, "Ġmeasurements": 15383, "bil": 15384, "ì½": 15385, "ifully": 15386, "инÑĥ": 15387, "ĠWilson": 15388, "¦´": 15389, "ĠíĮĮ": 15390, "Ġì°¨": 15391, "Ġpúblic": 15392, "ĠJerusalem": 15393, "Ġnails": 15394, "Ġspine": 15395, "Ġhemos": 15396, "Ġzn": 15397, "quis": 15398, "ĠLeben": 15399, "Ġreferences": 15400, "ITH": 15401, "iper": 15402, "ĠÑģебÑı": 15403, "ìģ": 15404, "ĠWa": 15405, "state": 15406, "§Ŀ": 15407, "åħ±": 15408, "ĠGener": 15409, "Ġactress": 15410, "ĠEnjoy": 15411, "à¹ĥ": 15412, "Ġ×Ĵ": 15413, "Ġinfected": 15414, "Ġshaking": 15415, "Ġnick": 15416, "ุ": 15417, "Ġfot": 15418, "Ġaccomplished": 15419, "uke": 15420, "Ġsheets": 15421, "Ġfence": 15422, "Ġnursing": 15423, "Ġintroducing": 15424, "Ġfeat": 15425, "One": 15426, "TO": 15427, "Ġclubs": 15428, "ĠBruce": 15429, "onge": 15430, "change": 15431, "ĠBatman": 15432, "åı°": 15433, "ĠOfficer": 15434, "Ġhydro": 15435, "Ġsupplement": 15436, "Ġcela": 15437, "Ġlongest": 15438, "Ġcompeting": 15439, "Ġconhe": 15440, "giving": 15441, "Ġbrains": 15442, "Ġloans": 15443, "Ġwage": 15444, "ĠClinton": 15445, "ĠsÄĥ": 15446, "aneous": 15447, "Ġlord": 15448, "ÑĢÑĥж": 15449, "Ġquiz": 15450, "Ġstiff": 15451, "ĠLGB": 15452, "sz": 15453, "ME": 15454, "mare": 15455, "there": 15456, "Ġnär": 15457, "ĠMand": 15458, "last": 15459, "Ġdag": 15460, "Ġhalfway": 15461, "ĠBand": 15462, "Ġëĭ¤ìĭľ": 15463, "ĠAren": 15464, "Ġile": 15465, "PN": 15466, "ento": 15467, "Ġalgum": 15468, "Ġsoccer": 15469, "Ġblocked": 15470, "ĠJonathan": 15471, "Ġsew": 15472, "ĠTestament": 15473, "Ġvale": 15474, "Ġbehavi": 15475, "å§ĭ": 15476, "Ġconna": 15477, "ICH": 15478, "Ġaudiences": 15479, "ml": 15480, "ammad": 15481, "ĠìĤ´ì": 15482, "IGH": 15483, "Ġraces": 15484, "emed": 15485, "Ġmá»Ļt": 15486, "ï": 15487, "Ġovers": 15488, "Ġdeclared": 15489, "Ġsana": 15490, "ĠUna": 15491, "ĠÑĢе": 15492, "ucks": 15493, "Ġpairs": 15494, "Ġange": 15495, "Ne": 15496, "Ġups": 15497, "avy": 15498, "ør": 15499, "reek": 15500, "Ġbehaviors": 15501, "Ġreflected": 15502, "Ġpriorities": 15503, "Ġcondu": 15504, "Ġretreat": 15505, "Ġexpenses": 15506, "Ġë´IJ": 15507, "Ġtriple": 15508, "Ġêµīìŀ¥íŀĪ": 15509, "ält": 15510, "Ġindigenous": 15511, "Ġmining": 15512, "Ġacceptable": 15513, "Ġruin": 15514, "CA": 15515, "uine": 15516, "Ġpipeline": 15517, "ctic": 15518, "êt": 15519, "ĠвÑģего": 15520, "Ġboun": 15521, "ĠDigital": 15522, "ĠBoom": 15523, "ÑĨе": 15524, "ĠлÑĥÑĩ": 15525, "Ġasc": 15526, "ĮĢë¡ľ": 15527, "ĠGoodbye": 15528, "Ġrender": 15529, "enez": 15530, "arre": 15531, "ĠTHAT": 15532, "bour": 15533, "ición": 15534, "ãĤŃ": 15535, "Every": 15536, "Ġwires": 15537, "ĠParliament": 15538, "nung": 15539, "ateur": 15540, "ĠSave": 15541, "ĠPhys": 15542, "Ġamor": 15543, "ĠEve": 15544, "Ġfright": 15545, "Ġgamma": 15546, "Ġmicros": 15547, "mitt": 15548, "ĠCode": 15549, "ĠBey": 15550, "pled": 15551, "ĠиÑģполÑĮз": 15552, "çĹ": 15553, "ìĥī": 15554, "她": 15555, "Ġmonet": 15556, "ĠJahre": 15557, "Ġluxury": 15558, "Ġdeaf": 15559, "Ġbetray": 15560, "Ġê²°": 15561, "ики": 15562, "Ġdefeated": 15563, "Ġundert": 15564, "Ġweg": 15565, "Ġcooler": 15566, "ãģķãĤĵ": 15567, "iami": 15568, "éĤĦæľī": 15569, "ĠJessica": 15570, "ĠJoy": 15571, "Ġsophistic": 15572, "ении": 15573, "ðĿĺ": 15574, "Ġchili": 15575, "ĠType": 15576, "Ġproteins": 15577, "Ġpresenting": 15578, "alia": 15579, "ìļ¸": 15580, "ĠMajor": 15581, "Ġmolecule": 15582, "umer": 15583, "Ġcollapse": 15584, "ĠAnyways": 15585, "ĠMountain": 15586, "anted": 15587, "ãĢIJ": 15588, "Ġвидео": 15589, "æ°´": 15590, "Aud": 15591, "Ġconqu": 15592, "Ġvoll": 15593, "Ġknit": 15594, "Ġmembr": 15595, "ĠMarket": 15596, "Ġdari": 15597, "Ġcalculated": 15598, "ги": 15599, "Ġshrimp": 15600, "ĠMu": 15601, "ĠпÑĢоÑĤ": 15602, "Ġìĺģìĥģ": 15603, "Ġproductivity": 15604, "Ġcognitive": 15605, "ĠHeb": 15606, "ictions": 15607, "ê²½": 15608, "Ġcré": 15609, "för": 15610, "Ġpraying": 15611, "ashi": 15612, "ĠTik": 15613, "ór": 15614, "wen": 15615, "ÑĮÑİ": 15616, "ixo": 15617, "Ġ(\"": 15618, "ĠÑĤел": 15619, "Ġìĸ´ëĸ¤": 15620, "ĠпеÑĢед": 15621, "ĠDrive": 15622, "ãĢij": 15623, "ĠEqu": 15624, "Ġequilibrium": 15625, "Ġdescribes": 15626, "нее": 15627, "42": 15628, "ĠCurrent": 15629, "yy": 15630, "Ġabsorb": 15631, "Ġsoldier": 15632, "ders": 15633, "Ġtestimony": 15634, "Ġdecline": 15635, "ľë¡ľ": 15636, "gage": 15637, "Ġinspire": 15638, "lapping": 15639, "Ġspinning": 15640, "Ġslavery": 15641, "Ġfacial": 15642, "Ġtraditions": 15643, "ários": 15644, "ĠHospital": 15645, "Ġnest": 15646, "ĠëĪĦ": 15647, "Ġtoi": 15648, "Ġfears": 15649, "ìħ¨": 15650, "ĠMuh": 15651, "Ġgraduation": 15652, "Ġimpacted": 15653, "Ġaunt": 15654, "ĠLets": 15655, "Ġaluminum": 15656, "Ġdominant": 15657, "ĠDavis": 15658, "ĠNavy": 15659, "Ġcompt": 15660, "oples": 15661, "Ġestava": 15662, "è¥": 15663, "Ġscal": 15664, "Ġpreserve": 15665, "ĠOpp": 15666, "Ġpractically": 15667, "Ġmagnitude": 15668, "Ġfitting": 15669, "Ġcoordinate": 15670, "Ġfurniture": 15671, "ĠFamil": 15672, "Ġexplosion": 15673, "Ġdocumentary": 15674, "ĠScript": 15675, "Ġportray": 15676, "mat": 15677, "Ġscheduled": 15678, "Ġdynamics": 15679, "phy": 15680, "aky": 15681, "ĠUI": 15682, "Che": 15683, "Ġcontinuously": 15684, "ĠProv": 15685, "å°ij": 15686, "Ñĥз": 15687, "rah": 15688, "Ġgerne": 15689, "proof": 15690, "Ġsecretary": 15691, "ĠPatreon": 15692, "scream": 15693, "ĠKids": 15694, "á»ĵi": 15695, "Ġkg": 15696, "Ġuncertainty": 15697, "Ġкажд": 15698, "Ġmitig": 15699, "Ġreads": 15700, "å·²": 15701, "ĠRu": 15702, "Ġpriest": 15703, "Ġнед": 15704, "Ġlimitations": 15705, "Ġfloat": 15706, "600": 15707, "ĠToy": 15708, "ĠJimmy": 15709, "Ġoffensive": 15710, "eni": 15711, "ĠXi": 15712, "Ġeyebr": 15713, "ĠTurk": 15714, "Ġaccidentally": 15715, "Ġohne": 15716, "ĠSaud": 15717, "95": 15718, "ĠDutch": 15719, "анÑģ": 15720, "ĠSeattle": 15721, "Ġëĵ±": 15722, "check": 15723, "kÄĻ": 15724, "Ġcontributions": 15725, "Ġbeside": 15726, "Ġquindi": 15727, "Ġflew": 15728, "æĹ¶": 15729, "ذا": 15730, "ĠLO": 15731, "Ġwaist": 15732, "ĠEV": 15733, "Ġholidays": 15734, "jon": 15735, "Ġmisunder": 15736, "Ñıн": 15737, "Ġbout": 15738, "Ġdimin": 15739, "ẽ": 15740, "ól": 15741, "ĠGrace": 15742, "Ġinputs": 15743, "Ġdeny": 15744, "Ġforming": 15745, "ĠBild": 15746, "Ġadequ": 15747, "Ġfolk": 15748, "Ġrejected": 15749, "semb": 15750, "Ġfrustrated": 15751, "open": 15752, "ĠBetter": 15753, "ilon": 15754, "Ġtowel": 15755, "Ġdifferential": 15756, "Ġsacred": 15757, "Ġsail": 15758, "éĩĮ": 15759, "entimes": 15760, "Ġgentleman": 15761, "Ġiconic": 15762, "Ġcomparing": 15763, "Ġsagt": 15764, "Ġtexts": 15765, "Ġgrandma": 15766, "Ġrolls": 15767, "Ġcontents": 15768, "ä¸į好": 15769, "оÑģÑģ": 15770, "Ġsuspension": 15771, "roit": 15772, "¦¼": 15773, "Ġassez": 15774, "Ġdort": 15775, "ĠMath": 15776, "ĠVictor": 15777, "ĠJavaScript": 15778, "ä¸įå°į": 15779, "Ġenhan": 15780, "ÅĻ": 15781, "ĠBush": 15782, "Ġpromotion": 15783, "Ġkin": 15784, "Ġmonsters": 15785, "ĠColorado": 15786, "Ġβ": 15787, "íķ´ìļĶ": 15788, "æŃ£": 15789, "ifferent": 15790, "Ġnaked": 15791, "Ġprod": 15792, "etics": 15793, "ĠWoman": 15794, "Ġtreatments": 15795, "Ġestoy": 15796, "vé": 15797, "Ġlifting": 15798, "Ġyapt": 15799, "ĠRober": 15800, "Ġì¹ľ": 15801, "Ġsubstitute": 15802, "aku": 15803, "ridge": 15804, "Ġê±°ë": 15805, "Ġresponded": 15806, "Ġbé": 15807, "ĠEngineer": 15808, "Ġtransferred": 15809, "ë²": 15810, "Ġhaber": 15811, "oop": 15812, "ĠWE": 15813, "Ġvest": 15814, "Ġforty": 15815, "ĠDS": 15816, "Ġ2004": 15817, "Ġcoaching": 15818, "nom": 15819, "ĠBab": 15820, "Ġnossa": 15821, "ĠJake": 15822, "Ġgy": 15823, "Ġdeleg": 15824, "Ġìŀł": 15825, "ĠкÑĢаÑģ": 15826, "Ġstandpoint": 15827, "Ġdisad": 15828, "Ġartwork": 15829, "Ad": 15830, "illo": 15831, "ĠÄijược": 15832, "ĠProm": 15833, "ĠLib": 15834, "Ġcriticism": 15835, "Ġcontacts": 15836, "ÑĢам": 15837, "Ġachievement": 15838, "ÐĶа": 15839, "Ġdissol": 15840, "ĠVegas": 15841, "Ġstreams": 15842, "ĠKent": 15843, "ĠعÙĦÙī": 15844, "Ġradius": 15845, "Ġsucks": 15846, "ĠAch": 15847, "Ġfi": 15848, "oust": 15849, "ĠлÑİди": 15850, "Ġpalette": 15851, "ĠHaz": 15852, "ĠAnthony": 15853, "Ġtema": 15854, "ĠCos": 15855, "Ġsafer": 15856, "αÏĤ": 15857, "Ġcontrad": 15858, "Ġmaior": 15859, "Ġinflation": 15860, "ĠSilver": 15861, "Ġattending": 15862, "íķľíħĮ": 15863, "arto": 15864, "Ġapplauding": 15865, "Ġcomputing": 15866, "ĠHat": 15867, "æ»": 15868, "know": 15869, "makers": 15870, "Ġconoc": 15871, "Ġeducated": 15872, "Ġmodified": 15873, "Ġinclusion": 15874, "mental": 15875, "ŀIJ": 15876, "isia": 15877, "ĠÏĢοÏħ": 15878, "Ġaun": 15879, "ĠIreland": 15880, "Ġkö": 15881, "Ġcompliance": 15882, "Ġinspiring": 15883, "иÑĤелÑĮно": 15884, "Ġdispos": 15885, "ì°¨": 15886, "Ġwip": 15887, "rical": 15888, "rawd": 15889, "Ġtres": 15890, "Ġmobil": 15891, "olutions": 15892, "BO": 15893, "Ġbounce": 15894, "Ġassumed": 15895, "ĠMedical": 15896, "Ġfiscal": 15897, "Ġngưá»Ŀi": 15898, "itionally": 15899, "Ġstolen": 15900, "ĠBM": 15901, "Ġmechanisms": 15902, "εί": 15903, "Ġqualified": 15904, "ĠìŀIJë": 15905, "ughters": 15906, "ĠHIV": 15907, "ĠLots": 15908, "Ġservers": 15909, "Ġcarr": 15910, "ĠTogether": 15911, "Ġattracted": 15912, "Ġkr": 15913, "æĪijæĺ¯": 15914, "thur": 15915, "inin": 15916, "ĠHalf": 15917, "ÈĽ": 15918, "ĠPap": 15919, "Ġreminded": 15920, "ALL": 15921, "Ġhelmet": 15922, "Ġbottles": 15923, "Ġprofessors": 15924, "Ġseine": 15925, "ÅĤÄħ": 15926, "ãĥı": 15927, "Ġê±°ìķ¼": 15928, "Ġ×¢×ľ": 15929, "fun": 15930, "ĠBird": 15931, "Ġfighter": 15932, "ĠëͰë": 15933, "ĠTool": 15934, "Ġtin": 15935, "inois": 15936, "ë¶Ħ": 15937, "×Ļף": 15938, "ĠCAR": 15939, "åIJį": 15940, "irsty": 15941, "Ġoutdoor": 15942, "ĠNS": 15943, "ãħİ": 15944, "ffen": 15945, "Ġlud": 15946, "Hello": 15947, "Ġroller": 15948, "iele": 15949, "ĠPoland": 15950, "Ġapa": 15951, "exp": 15952, "Ġcertificate": 15953, "ĠTown": 15954, "аÑİÑĤÑģÑı": 15955, "ilde": 15956, "Ġdetermin": 15957, "PR": 15958, "Ġfreeze": 15959, "Ġmainstream": 15960, "Ġobjectives": 15961, "blo": 15962, "Ġtakie": 15963, "åĵĪåĵĪ": 15964, "Ġë°Ķë¡ľ": 15965, "elet": 15966, "ĠIV": 15967, "ĠFast": 15968, "Ġdere": 15969, "emp": 15970, "ĠDra": 15971, "ĠìŀĪìĹĪ": 15972, "Ġdiscrimination": 15973, "Ġείναι": 15974, "necess": 15975, "æ®": 15976, "ıģı": 15977, "Ġposting": 15978, "wiÅĽcie": 15979, "Ġlub": 15980, "Ġolive": 15981, "Ġrim": 15982, "Ġmodeling": 15983, "Ġaño": 15984, "ĠPakistan": 15985, "Ġoverl": 15986, "Ġinflam": 15987, "NE": 15988, "ìĹIJê²Į": 15989, "Ġattended": 15990, "Ġdealt": 15991, "ĠAlt": 15992, "ĠLincoln": 15993, "Ġawake": 15994, "Ġfilters": 15995, "ĠWithin": 15996, "czywiÅĽcie": 15997, "Ġsû": 15998, "ĠJohnny": 15999, "Ġintegrity": 16000, "Ġisolation": 16001, "ĠEasy": 16002, "ĠпÑĢин": 16003, "ĠAlice": 16004, "Ġsmiling": 16005, "enix": 16006, ",...": 16007, "ζ": 16008, "Ġbegun": 16009, "Ġjewel": 16010, "Ġconventional": 16011, "Ġstatist": 16012, "Ġhanded": 16013, "Ġirre": 16014, "Ġprohib": 16015, "Ġsatellite": 16016, "é¦Ļ": 16017, "ĠIndust": 16018, "Ġtraged": 16019, "Ġtrava": 16020, "Ġihm": 16021, "Ġcruel": 16022, "ĠAgora": 16023, "ĠDoc": 16024, "Ġzones": 16025, "Ġmall": 16026, "Ġtray": 16027, "×ķ׳": 16028, "Ġirrit": 16029, "Ġkans": 16030, "ĠBeat": 16031, "udge": 16032, "ielle": 16033, "Ġtrusted": 16034, "Ġbikes": 16035, "ĠÑĥп": 16036, "ĠMember": 16037, "wick": 16038, "Ġcreators": 16039, "Ġheritage": 16040, "indistinct": 16041, "Ġresur": 16042, "ennen": 16043, "Come": 16044, "Ġfiring": 16045, "ĠBueno": 16046, "ĠТо": 16047, "ikan": 16048, "ettes": 16049, "Ġkes": 16050, "Ġtrips": 16051, "Ġdivorce": 16052, "ĠKl": 16053, "Ġconsol": 16054, "keep": 16055, "기ê°Ģ": 16056, "ĠReport": 16057, "Ġhosting": 16058, "Ġdiamond": 16059, "Ġcomplic": 16060, "Ġhelicop": 16061, "Ġdepuis": 16062, "ds": 16063, "ĠChan": 16064, "Ñıл": 16065, "Ġscissors": 16066, "ilation": 16067, "Ġproportion": 16068, "ERE": 16069, "ĠÙĪØ§ÙĦ": 16070, "inta": 16071, "Ġmuchas": 16072, "uation": 16073, "itis": 16074, "æĬĬ": 16075, "ÑıÑī": 16076, "Ġniin": 16077, "Ġemphasize": 16078, "uela": 16079, "Ġproducers": 16080, "Ġrze": 16081, "änder": 16082, "ETH": 16083, "æº": 16084, "Ġconstitu": 16085, "åĽ½": 16086, "Ġperformances": 16087, "istle": 16088, "gov": 16089, "ĠLiter": 16090, "Ġincorporate": 16091, "Ġeducate": 16092, "ĠNin": 16093, "쪽": 16094, "ÙĩÙħ": 16095, "eleration": 16096, "×ķ×ij": 16097, "ĠyaÅŁ": 16098, "orous": 16099, "ĠCas": 16100, "Ġgrants": 16101, "ëĬ¥": 16102, "amel": 16103, "Ġê·¸ëłĩê²Į": 16104, "ĠEste": 16105, "ÑħодиÑĤ": 16106, "ĠпоÑģле": 16107, "Ġgent": 16108, "Ġfocuses": 16109, "alities": 16110, "ĠRh": 16111, "ë³´": 16112, "æ°ij": 16113, "ĠDance": 16114, "rr": 16115, "Ġamer": 16116, "Ġutilize": 16117, "ĠlÃŃ": 16118, "ĠAmong": 16119, "Ġpregnancy": 16120, "Ġloops": 16121, "алоÑģÑĮ": 16122, "ĠMoh": 16123, "Ġcatching": 16124, "Ġglob": 16125, "Ġajud": 16126, "Ġ[?": 16127, "ĠAnal": 16128, "looking": 16129, "Ġsurfaces": 16130, "Ġprogressive": 16131, "Ġviral": 16132, "08": 16133, "ξ": 16134, "KA": 16135, "Ġży": 16136, "Ġpicks": 16137, "annon": 16138, "Ġbulk": 16139, "ĠRoss": 16140, "Ġdescribing": 16141, "ĠGel": 16142, "Ġlocally": 16143, "Ġendless": 16144, "Ġmassage": 16145, "Ġcleaned": 16146, "Ġtraveled": 16147, "енÑĭ": 16148, "Ġsentiment": 16149, "igma": 16150, "ĠNas": 16151, "Ġchemicals": 16152, "Ġrighteous": 16153, "ĠMagic": 16154, "Ġrelates": 16155, "Ġtrucks": 16156, "Ġ1960": 16157, "åĪ¥": 16158, "Ġappet": 16159, "Ġsnacks": 16160, "ĠSummer": 16161, "Ġyüz": 16162, "Ġpris": 16163, "ĠMexican": 16164, "Ġtransparen": 16165, "Ġminority": 16166, "Ġverte": 16167, "Ġlassen": 16168, "46": 16169, "лек": 16170, "ép": 16171, "ĠÑĦилÑĮ": 16172, "Ġiyi": 16173, "Ġspan": 16174, "íķĺì§Ģ": 16175, "Ġindicated": 16176, "quar": 16177, "Ġscholarship": 16178, "ĠLGBT": 16179, "Ġhistorically": 16180, "óÅĤ": 16181, "Ġminist": 16182, "Ġpenet": 16183, "ĠRap": 16184, "Ġconservation": 16185, "缴": 16186, "ĠHoney": 16187, "ĠBei": 16188, "idel": 16189, "Ġresponsibilities": 16190, "Ġmessy": 16191, "ĠExcept": 16192, "ORE": 16193, "Ġinitiatives": 16194, "Ġjunior": 16195, "Ġdesigners": 16196, "Ġexploration": 16197, "Ġsponsor": 16198, "Ġmobility": 16199, "Ġinteg": 16200, "lando": 16201, "Ġbark": 16202, "Ġindicates": 16203, "à¶": 16204, "Ġemployer": 16205, "å®ī": 16206, "Ġcousin": 16207, "Ġboiling": 16208, "Ġchrom": 16209, "Ġçal": 16210, "Ġperpet": 16211, "Ġcontained": 16212, "Ġparks": 16213, "Ы": 16214, "ĠEngineering": 16215, "Please": 16216, "ĠStarting": 16217, "hero": 16218, "Ġlawyers": 16219, "西": 16220, "Ġzd": 16221, "Ġfranchise": 16222, "rage": 16223, "Ġintuit": 16224, "ĠGL": 16225, "reach": 16226, "ĠElle": 16227, "Ġnhư": 16228, "ĠNord": 16229, "Ġbean": 16230, "07": 16231, "Ġpleasant": 16232, "å½ĵ": 16233, "viron": 16234, "Ġgradient": 16235, "zus": 16236, "ĠEM": 16237, "Ġessay": 16238, "ìĹIJìļĶ": 16239, "ến": 16240, "nu": 16241, "ừ": 16242, "ĠÃīs": 16243, "Ġdenomin": 16244, "ĠGirls": 16245, "Ġpersonnes": 16246, "ĠاÙĦØ£": 16247, "bild": 16248, "ĠStat": 16249, "Ġcompliment": 16250, "ĠKate": 16251, "Ġoptimal": 16252, "Ġhid": 16253, "دÙĬ": 16254, "Ġquicker": 16255, "wall": 16256, "En": 16257, "INE": 16258, "???": 16259, "ì²´": 16260, "ĠAction": 16261, "åŁ": 16262, "Ġpenalty": 16263, "ĠKaz": 16264, "'?": 16265, "Ġcried": 16266, "Ġcanvas": 16267, "fte": 16268, "Ġexclud": 16269, "¸ë¡ľ": 16270, "Ġemphasis": 16271, "Ġenzy": 16272, "ĠHou": 16273, "Ġoverseas": 16274, "ÃŃamos": 16275, "師": 16276, "öglich": 16277, "Ġheadphones": 16278, "cn": 16279, "ĠAge": 16280, "Ġakan": 16281, "Ġcharacteristic": 16282, "íķĺë©´": 16283, "gets": 16284, "Ġë¶Ī": 16285, "Ġrival": 16286, "Ġborders": 16287, "emente": 16288, "emás": 16289, "Ġyol": 16290, "Ġcompe": 16291, "enders": 16292, "ından": 16293, "Ġmöglich": 16294, "Ġbubbles": 16295, "natural": 16296, "Ġarmed": 16297, "Ġelabor": 16298, "ĠìĿ´ë²Ī": 16299, "Ġwashed": 16300, "οÏħμε": 16301, "è«ĭ": 16302, "Ġflavors": 16303, "Ġexiste": 16304, "Ġprest": 16305, "ĠThema": 16306, "опÑĢоÑģ": 16307, "eron": 16308, "UE": 16309, "eri": 16310, "Ġconcer": 16311, "Ġaixò": 16312, "åħ©": 16313, "Ġprotective": 16314, "ĠзнаÑİ": 16315, "ĠëĤł": 16316, "ĠIII": 16317, "Ġmeer": 16318, "ĠShop": 16319, "lli": 16320, "ĠOrder": 16321, "ĠMY": 16322, "ĠGhost": 16323, "ãĤĤãģĨ": 16324, "adel": 16325, "Ġstole": 16326, "Ġreleasing": 16327, "ĠComment": 16328, "Ġtrains": 16329, "ëªħ": 16330, "Ġwissen": 16331, "ensed": 16332, "Ġdescend": 16333, "Ġfier": 16334, "Ġradi": 16335, "Ġpersu": 16336, "ç¢": 16337, "Ġмн": 16338, "ĠDest": 16339, "Ġworries": 16340, "itet": 16341, "bas": 16342, "Ġstab": 16343, "name": 16344, "oric": 16345, "ĠClose": 16346, "Ġalumni": 16347, "ĠSelf": 16348, "ffe": 16349, "itating": 16350, "atherine": 16351, "ĠRights": 16352, "Ġellos": 16353, "Ġwarrant": 16354, "Ġnerve": 16355, "Ġvegetable": 16356, "ĠTeil": 16357, "Ġê°ĻìĿ´": 16358, "RY": 16359, "Ġsustainability": 16360, "Ġsteht": 16361, "Ġbrid": 16362, "adaÅŁ": 16363, "Ġtv": 16364, "Ġduration": 16365, "Ġpessoa": 16366, "Ġmetrics": 16367, "Ġadam": 16368, "cas": 16369, "аÑĢи": 16370, "Ġevident": 16371, "Ġdisplayed": 16372, "ائ": 16373, "Ġreck": 16374, "ĠBuddha": 16375, "Ġdele": 16376, "ĠDiego": 16377, "osph": 16378, "Ġbla": 16379, "ĠMik": 16380, "ulator": 16381, "Ġ2001": 16382, "Ġpromoting": 16383, "ych": 16384, "ĠEX": 16385, "Ġlastly": 16386, "Ġoutline": 16387, "Ġspirits": 16388, "Ġveux": 16389, "Ġsubtract": 16390, "ĠÅŁimdi": 16391, "Ġpins": 16392, "Ġburger": 16393, "Ġmolto": 16394, "ĠhabÃŃa": 16395, "Ġë°ĺ": 16396, "igu": 16397, "erst": 16398, "Ġnen": 16399, "Ġbacon": 16400, "itious": 16401, "Ġcarries": 16402, "Ġpromises": 16403, "nde": 16404, "ĠLeft": 16405, "ĠLim": 16406, "æ£": 16407, "Ġ44": 16408, "Ġcareers": 16409, "Ġ주ë": 16410, "Ġspeeds": 16411, "qué": 16412, "mad": 16413, "market": 16414, "isme": 16415, "Ġ2003": 16416, "Ġrecess": 16417, "ĠJUD": 16418, "Ġracist": 16419, "ĠSchl": 16420, "Ġparler": 16421, "Ġotros": 16422, "ishes": 16423, "Ġconverted": 16424, "aaaa": 16425, "ании": 16426, "ĠArk": 16427, "ĠChance": 16428, "Ġelementary": 16429, "εν": 16430, "inks": 16431, "Interviewer": 16432, "Ġfreely": 16433, "alah": 16434, "Ġëĭ¤ë¥¸": 16435, "Ġrequested": 16436, "Ġtorque": 16437, "noÅĽci": 16438, "oured": 16439, "ĠStaff": 16440, "Ġstain": 16441, "ĠAlan": 16442, "Ġvere": 16443, "ĠWinter": 16444, "Ġdefect": 16445, "iedy": 16446, "Ġbeats": 16447, "Ġhá": 16448, "umn": 16449, "oons": 16450, "itudes": 16451, "Ġseit": 16452, "oly": 16453, "Ġreserv": 16454, "Ġextr": 16455, "Ġphysician": 16456, "visor": 16457, "Ġhandful": 16458, "ĠNations": 16459, "Ġì¢ĭìĿĢ": 16460, "uccess": 16461, "Ġupstairs": 16462, "ĠSquare": 16463, "Ġhein": 16464, "ĠSeason": 16465, "olis": 16466, "Ġprince": 16467, "Ġdefensive": 16468, "ç½": 16469, "ĠмеÑģÑĤ": 16470, "Ñĸй": 16471, "ĠاÙĨ": 16472, "umble": 16473, "ê¹ĮìļĶ": 16474, "Ġassass": 16475, "Ġcircular": 16476, "Ġqualities": 16477, "Ġhmm": 16478, "Ġblown": 16479, "ĠLiz": 16480, "ĠKur": 16481, "ĠSA": 16482, "Ġfindings": 16483, "Ġcolours": 16484, "Ġdelle": 16485, "ĠIR": 16486, "ĠAth": 16487, "ĠDub": 16488, "ĠOx": 16489, "ĠØ®": 16490, "Ġpockets": 16491, "Ġgrill": 16492, "Ġswitching": 16493, "Ġpreferred": 16494, "ĠWales": 16495, "Ġexemplo": 16496, "Ġchopped": 16497, "Ġvaccination": 16498, "Ġneuro": 16499, "Ġspecify": 16500, "ivos": 16501, "Ġserá": 16502, "Ġzie": 16503, "Ġà®®": 16504, "Ġresulting": 16505, "ĠUgh": 16506, "Ġmessed": 16507, "CD": 16508, "Ġpaar": 16509, "Ġcomer": 16510, "Ġcouch": 16511, "ĠFestival": 16512, "Ġ49": 16513, "vous": 16514, "zens": 16515, "種": 16516, "ĠKennedy": 16517, "ĠTs": 16518, "Ġë³´ìĹ": 16519, "Ġdemonstration": 16520, "Ġunto": 16521, "Ġfrustrating": 16522, "Ġlaboratory": 16523, "Ġegy": 16524, "Ġbeautifully": 16525, "Ġìŀ¬ë": 16526, "Ġalgu": 16527, "Ġöyle": 16528, "ä½łçľĭ": 16529, "ĠPH": 16530, "Ġfortune": 16531, "Ġcleaner": 16532, "ĠRobin": 16533, "Ġsaus": 16534, "ĠGeld": 16535, "Ġkat": 16536, "obs": 16537, "Ġolur": 16538, "Ġmatt": 16539, "Ġquesta": 16540, "Ġsuggestion": 16541, "encer": 16542, "оÑģÑĤ": 16543, "Ġradar": 16544, "Ġìŀ¡": 16545, "isha": 16546, "ந": 16547, "ãĤĵãģª": 16548, "jes": 16549, "Ġveel": 16550, "ìĤ°": 16551, "Ġauthors": 16552, "ãĢİ": 16553, "plan": 16554, "Ġcollaborative": 16555, "Ġinstinct": 16556, "Ġfarming": 16557, "auge": 16558, "Edu": 16559, "Ġmembership": 16560, "Ġsimultaneously": 16561, "Ġbake": 16562, "Ġkä": 16563, "Ġlectures": 16564, "ÑĩеÑģ": 16565, "Ġprendre": 16566, "Ġcollaps": 16567, "ĠSaya": 16568, "ĠFut": 16569, "Ġyog": 16570, "ĠRather": 16571, "رÙĬ": 16572, "Ġcamps": 16573, "олод": 16574, "Ġsimulation": 16575, "ĠMak": 16576, "Laughs": 16577, "Ġgrey": 16578, "Ġsentences": 16579, "yen": 16580, "ĠUnless": 16581, "Je": 16582, "ĠSatan": 16583, "ĠÑĤакже": 16584, "ĠNA": 16585, "Ġbron": 16586, "Ġ?]": 16587, "Ġsouls": 16588, "Ġlightning": 16589, "Ġimagined": 16590, "Ġczyli": 16591, "psilon": 16592, "etta": 16593, "Ġbelieving": 16594, "Ġstrongest": 16595, "ĠCON": 16596, "Ġquelques": 16597, "Ġimmigrants": 16598, "Ġwallet": 16599, "éĢĻæĺ¯": 16600, "ĠJersey": 16601, "Ġimplications": 16602, "Ġforb": 16603, "ãĢı": 16604, "Ġunbelievable": 16605, "اء": 16606, "Ġoperational": 16607, "üs": 16608, "ĠGM": 16609, "Ġê·¸ëŁ°ëį°": 16610, "Ġgracias": 16611, "Ġentend": 16612, "ĠRegard": 16613, "rob": 16614, "ĠÑĤеÑħ": 16615, "èı": 16616, "ĠRevolution": 16617, "Ġwaar": 16618, "ĠBiz": 16619, "theless": 16620, "Ġsponsored": 16621, "quier": 16622, "ĠìĿ¼ë": 16623, "Ġtek": 16624, "ĠëIJł": 16625, "igkeit": 16626, "ĠLuck": 16627, "ĠCertainly": 16628, "Ġtoll": 16629, "ĠниÑĩего": 16630, "ĠMoney": 16631, "ĠÑģÑĤоÑĢ": 16632, "ĠDouble": 16633, "ĠWolf": 16634, "Ġchunk": 16635, "άν": 16636, "ités": 16637, "oning": 16638, "Mar": 16639, "Ġgrandes": 16640, "Ġcollections": 16641, "ĠEuropa": 16642, "ĠаÑĢ": 16643, "ĠâĢĭâĢĭâĢĭ": 16644, "Ġê·¸ëŁ¬ë©´": 16645, "ĠобÑĬ": 16646, "Ġãģª": 16647, "Ġìĭľê°Ħ": 16648, "ĠCustom": 16649, "Ġì²ĺ": 16650, "ÑĸлÑĮ": 16651, "Ġindividually": 16652, "íĹ": 16653, "Ġdozen": 16654, "Ġowe": 16655, "ĠVictoria": 16656, "åı¯èĥ½": 16657, "Ġbeet": 16658, "urb": 16659, "Ġanalog": 16660, "ição": 16661, "Ĥľ": 16662, "soever": 16663, "Ġmodo": 16664, "Ġsubscribed": 16665, "ìŀ¬": 16666, "Ġentities": 16667, "çīĩ": 16668, "Ġcloset": 16669, "Ġresponding": 16670, "Ġprinter": 16671, "ĠStephan": 16672, "ĠbyÅĤ": 16673, "ĠDom": 16674, "ĠFern": 16675, "ĠPier": 16676, "ĠwiÄĻc": 16677, "Ġhence": 16678, "Ġmodules": 16679, "ãĥ¬": 16680, "Ġëͱ": 16681, "ĠDanny": 16682, "ĠÑģебе": 16683, "Ġvad": 16684, "ĠìĹĦ": 16685, "Ġsous": 16686, "Ġsphere": 16687, "BY": 16688, "ĠPed": 16689, "igned": 16690, "Ġwheat": 16691, "Ġunders": 16692, "Ġevolve": 16693, "Ġdeclar": 16694, "Ġlightly": 16695, "Ġidentifying": 16696, "æĦıæĢĿ": 16697, "Ġlegendary": 16698, "Ġgenuine": 16699, "Ġgrind": 16700, "ĠUne": 16701, "geben": 16702, "Ġbicy": 16703, "Ġjumps": 16704, "Ġprovince": 16705, "ziÄĻ": 16706, "Ġ×IJ׳×Ļ": 16707, "Ġhoc": 16708, "Ġбл": 16709, "ĠGrad": 16710, "Ġrevenge": 16711, "ĠاÙĦت": 16712, "ooh": 16713, "æĭľ": 16714, "аÑĨии": 16715, "å¹³": 16716, "Ġelectro": 16717, "ĠëIJIJ": 16718, "ãģ§ãģ¯": 16719, "Ġfals": 16720, "riel": 16721, "oker": 16722, "ĠExcellent": 16723, "ĠMorgan": 16724, "Ġbrick": 16725, "Ġsubstantial": 16726, "Ġpollution": 16727, "ĠTür": 16728, "ĠEvet": 16729, "Ġlung": 16730, "ãģĸ": 16731, "×Ļש": 16732, "ommes": 16733, "Ġrealizing": 16734, "Ġhumble": 16735, "ĠLock": 16736, "Ġbod": 16737, "Ġìĸ¸": 16738, "Ġpeers": 16739, "uzz": 16740, "Ġembedded": 16741, "Ġclaro": 16742, "Ġaggreg": 16743, "Ġemployers": 16744, "ĠRaj": 16745, "Ġãģ¨": 16746, "ĠYi": 16747, "Ġjeu": 16748, "aters": 16749, "Ġstrikes": 16750, "nos": 16751, "autres": 16752, "dr": 16753, "opher": 16754, "ĠApparently": 16755, "íĺĦ": 16756, "Ġinfant": 16757, "اب": 16758, "ÑĤÑĭ": 16759, "íĽ": 16760, "Ú¯": 16761, "Ġredes": 16762, "acaģım": 16763, "ĠDAVID": 16764, "ĠChicken": 16765, "Ġperspectives": 16766, "Ġviewer": 16767, "Ġshar": 16768, "ĠпÑĢоиз": 16769, "ligt": 16770, "eros": 16771, "itable": 16772, "илоÑģÑĮ": 16773, "ĠdifÃŃ": 16774, "´ëį°": 16775, "Ġretired": 16776, "Ġthats": 16777, "zenie": 16778, "beiten": 16779, "Ġmycket": 16780, "ĠRab": 16781, "Ġinflamm": 16782, "ì°®": 16783, "Ġdum": 16784, "Ġdaddy": 16785, "æľŁ": 16786, "Ġimmers": 16787, "Ġplaylist": 16788, "à¯Ĩ": 16789, "Ġtraum": 16790, "Ġrefuse": 16791, "step": 16792, "à®ļ": 16793, "cup": 16794, "Ġpops": 16795, "rimin": 16796, "ayım": 16797, "Ġald": 16798, "Ġunnecess": 16799, "Ġdah": 16800, "ĠIrish": 16801, "Ġcompr": 16802, "laÅŁ": 16803, "TP": 16804, "Ġtranslated": 16805, "Sc": 16806, "ceÄŁim": 16807, "´IJ": 16808, "Ġdrei": 16809, "ĠлÑİдей": 16810, "Ġquiero": 16811, "Ġhele": 16812, "zlich": 16813, "Ġapples": 16814, "Ġdistricts": 16815, "Ġcredits": 16816, "Ġasp": 16817, "Ġëĭ¨": 16818, "oral": 16819, "å½±": 16820, "Ġstepping": 16821, "ĠVa": 16822, "Ġgains": 16823, "65": 16824, "Ġnuestra": 16825, "eday": 16826, "assador": 16827, "ĠLind": 16828, "Ġcrops": 16829, "ciendo": 16830, "igue": 16831, "Ġbana": 16832, "Am": 16833, "Ġpent": 16834, "Ġaddiction": 16835, "Ġpackaging": 16836, "äd": 16837, "ª¨": 16838, "Ġperquè": 16839, "Ġcampaigns": 16840, "Ġsteep": 16841, "Ġneue": 16842, "Ġembarrassed": 16843, "Ġdistinction": 16844, "itzer": 16845, "åijĬ": 16846, "Ġregistration": 16847, "Ġllam": 16848, "ĠAlmighty": 16849, "liest": 16850, "Ġuz": 16851, "nak": 16852, "çº": 16853, "Ġteraz": 16854, "iamente": 16855, "Ġtransactions": 16856, "Ġcôt": 16857, "Ġswitched": 16858, "Ġcombo": 16859, "Ġprayers": 16860, "Ġinternship": 16861, "Ġaddresses": 16862, "Ġcharity": 16863, "ĠWOO": 16864, "Ġbait": 16865, "è¿ĩ": 16866, "Ġ�": 16867, "Ġfica": 16868, "ĠTyler": 16869, "aru": 16870, "Ġatoms": 16871, "ĠLevel": 16872, "ĠпоÑĤом": 16873, "Ġfame": 16874, "ulk": 16875, "Ġteaches": 16876, "Ġrebuild": 16877, "едÑĮ": 16878, "ĠIndonesia": 16879, "ushi": 16880, "ĠShort": 16881, "Ġensuring": 16882, "fs": 16883, "ele": 16884, "Ġmarginal": 16885, "Ġconclude": 16886, "amt": 16887, "Ġverify": 16888, "ĠMcDonald": 16889, "Ġskal": 16890, "Ġreconst": 16891, "ĠMann": 16892, "Ġbasement": 16893, "Ġtransformed": 16894, "Ġoccasionally": 16895, "zone": 16896, "ĠDans": 16897, "Ġкакой": 16898, "Ġdiagnosed": 16899, "ĠÏĦα": 16900, "Ġcommands": 16901, "Ġpresidential": 16902, "Ġabb": 16903, "Ġbracket": 16904, "ĠLem": 16905, "Ã¥ng": 16906, "Ġfavorites": 16907, "Ġrevol": 16908, "ĠíĬ¹": 16909, "Ġharass": 16910, "éħ": 16911, "Ġcleans": 16912, "ständ": 16913, "Ġknocked": 16914, "Ġpeoples": 16915, "Ġmusicians": 16916, "Ġmutual": 16917, "ĠCold": 16918, "88": 16919, "zej": 16920, "atie": 16921, "ĠHonor": 16922, "Ġobsessed": 16923, "ĠMUSIC": 16924, "ĠBreak": 16925, "úng": 16926, "Ġmodify": 16927, "Ġsöyle": 16928, "Ġ×ŀ×Ķ": 16929, "ĠOnline": 16930, "fo": 16931, "ĠMiller": 16932, "Ġliking": 16933, "Ġinhab": 16934, "Ġgratitude": 16935, "ĠJournal": 16936, "arness": 16937, "John": 16938, "ĠGit": 16939, "åīĽ": 16940, "Ġsincere": 16941, "ĠSci": 16942, "ĠEli": 16943, "Ġsymbols": 16944, "Ġmanually": 16945, "εÏĤ": 16946, "ĠвÑĸд": 16947, "ĠFat": 16948, "Ġlabels": 16949, "Ġsophisticated": 16950, "umps": 16951, "Ġreleases": 16952, "Ġ47": 16953, "ĠOM": 16954, "ê°Ģë": 16955, "ĠBien": 16956, "ĠRef": 16957, "è¨ĺ": 16958, "ĠSta": 16959, "ĠEgg": 16960, "Ġindicator": 16961, "pson": 16962, "Ġnasıl": 16963, "Right": 16964, "Ġconvey": 16965, "Ġknot": 16966, "Ġconnects": 16967, "ulas": 16968, "Ġpreced": 16969, "Ġinequality": 16970, "amiento": 16971, "Ġreply": 16972, "OY": 16973, "Ġdismiss": 16974, "ĠëIJľ": 16975, "çĦ¡": 16976, "ĠÑħоÑĢоÑĪо": 16977, "Ġméd": 16978, "Ġrandomly": 16979, "ĠOnt": 16980, "uard": 16981, "Ġpulls": 16982, "ĠÑĤепеÑĢÑĮ": 16983, "ĠNeed": 16984, "ĠSoft": 16985, "Ġstrengths": 16986, "Ġgoed": 16987, "umen": 16988, "æŃ»": 16989, "Ġíݸ": 16990, "Ġдоб": 16991, "Ġclarity": 16992, "ĠAi": 16993, "Ġballoon": 16994, "ĠPand": 16995, "ĠìķĦëĭ": 16996, "Ġshiny": 16997, "Ġsmallest": 16998, "onia": 16999, "hill": 17000, "oting": 17001, "Ġeing": 17002, "Ġmerely": 17003, "Ġseus": 17004, "Ġнеп": 17005, "ĠíĨµ": 17006, "Ġguides": 17007, "Ġspecialist": 17008, "Ġsteak": 17009, "ãĤĪãģĨ": 17010, "Ġmigration": 17011, "quele": 17012, "Ġruined": 17013, "Ġpupp": 17014, "女": 17015, "Ġkend": 17016, "angan": 17017, "Ġpalm": 17018, "Ġunfair": 17019, "Ġzm": 17020, "ĠDV": 17021, "chester": 17022, "иÑİ": 17023, "Ġooh": 17024, "erg": 17025, "ATH": 17026, "°©": 17027, "åĵª": 17028, "rison": 17029, "Ġinvolving": 17030, "Ġpartly": 17031, "ançais": 17032, "Ġvow": 17033, "Ġprominent": 17034, "Ġcryst": 17035, "iba": 17036, "Ġdeserves": 17037, "Ġovert": 17038, "Ġsensit": 17039, "ĠWhe": 17040, "Ġtighten": 17041, "Ġintimid": 17042, "Ġaliment": 17043, "will": 17044, "Ġstrengthen": 17045, "ĠTan": 17046, "åıĪ": 17047, "ãģĹãģ¾ãģĻ": 17048, "oni": 17049, "ĠMun": 17050, "Ġproph": 17051, "Ġrehears": 17052, "ĠKle": 17053, "Ġveces": 17054, "Ġwondered": 17055, "oki": 17056, "Ġsenses": 17057, "´ìĭ": 17058, "Æ°á»Ľ": 17059, "ĠÈĻi": 17060, "Ġmuchos": 17061, "Ġwatches": 17062, "ortunate": 17063, "ĠJuan": 17064, "ìŀĸìķĦ": 17065, "ÑĢе": 17066, "ei": 17067, "ionen": 17068, "Ġexperimental": 17069, "Ġdaughters": 17070, "à¸Ľ": 17071, "Ġmentally": 17072, "becca": 17073, "aware": 17074, "ìĦĿ": 17075, "Ġwhatsoever": 17076, "Ġenables": 17077, "ĠLow": 17078, "oid": 17079, "à¸Ĭ": 17080, "ód": 17081, "غ": 17082, "Ġconstructed": 17083, "ĠLadies": 17084, "Ġaccused": 17085, "Ġан": 17086, "Dan": 17087, "Ġspawn": 17088, "Ġcontainers": 17089, "Ġartistic": 17090, "ıp": 17091, "Ġdiscl": 17092, "Ġautres": 17093, "inas": 17094, "ĠNation": 17095, "Ġnag": 17096, "bean": 17097, "whe": 17098, "ľëıĦ": 17099, "ĠSeoul": 17100, "Ġíı¬": 17101, "ĠNich": 17102, "Ġcomplement": 17103, "Ġinterven": 17104, "ĠModel": 17105, "ĠOrange": 17106, "namon": 17107, "Ġcalculation": 17108, "see": 17109, "Ġustedes": 17110, "Ġleb": 17111, "Ġdoct": 17112, "Ñĸн": 17113, "Ġfoster": 17114, "Ġelastic": 17115, "ĠAhh": 17116, "Ġace": 17117, "ĠPink": 17118, "ĠJeg": 17119, "Ġdeer": 17120, "ãģĹãģĦ": 17121, "sis": 17122, "Ġjako": 17123, "ĠEmma": 17124, "ÑģÑĤвенно": 17125, "Ġportrait": 17126, "Ġmaker": 17127, "Ġaument": 17128, "ÑĢоб": 17129, "Ġairplane": 17130, "Ġtransparency": 17131, "Ġadjustment": 17132, "ĠCDC": 17133, "çon": 17134, "Ġuploaded": 17135, "ĠдейÑģÑĤв": 17136, "ĠгоÑĤов": 17137, "Ġiter": 17138, "Ġcurse": 17139, "ôn": 17140, "merce": 17141, "aran": 17142, "Ġleak": 17143, "çµIJ": 17144, "Ġabsence": 17145, "Ñģкий": 17146, "Ġreaders": 17147, "aler": 17148, "Ġbeneath": 17149, "ango": 17150, "hetic": 17151, "Ġfinns": 17152, "Ġpoop": 17153, "Ġduplic": 17154, "Hi": 17155, "igs": 17156, "ologically": 17157, "opp": 17158, "Ġdizer": 17159, "ĠAllen": 17160, "Ġgli": 17161, "Ġacceleration": 17162, "Ġvitamin": 17163, "ãĥŃ": 17164, "vä": 17165, "ĠAccess": 17166, "à®Ļ": 17167, "rás": 17168, "Ġappreciated": 17169, "Ġnah": 17170, "Ġposter": 17171, "Ġtale": 17172, "Ġhighlighted": 17173, "æĸĩ": 17174, "żeli": 17175, "Ġblockchain": 17176, "Ġmicrow": 17177, "Ġcinema": 17178, "ĠChang": 17179, "ĠSearch": 17180, "usters": 17181, "ĠZero": 17182, "ĠDivision": 17183, "ÑĢаÑģ": 17184, "Ġscare": 17185, "Ġjelly": 17186, "ĠAdministration": 17187, "SO": 17188, "Ġlined": 17189, "Ġê°Ħ": 17190, "Ġgeben": 17191, "Ġsoda": 17192, "Ġwinners": 17193, "³¼": 17194, "ÙĴ": 17195, "ĠAmb": 17196, "åķıé¡Į": 17197, "åĶ": 17198, "Ġpeg": 17199, "å·±": 17200, "43": 17201, "Ġraus": 17202, "Ġrewards": 17203, "Ġinclus": 17204, "Ġhighway": 17205, "Ġhah": 17206, "Ġmultiplied": 17207, "Ġsẽ": 17208, "Ġdisciples": 17209, "Ġning": 17210, "Ġdressing": 17211, "Ġattributes": 17212, "ĠMosc": 17213, "ĠGreece": 17214, "Ġsek": 17215, "ĠLearn": 17216, "Ġjus": 17217, "rendre": 17218, "Ġpersonne": 17219, "plete": 17220, "Ġplacing": 17221, "Ġluego": 17222, "illance": 17223, "ĠобÑī": 17224, "Ġprovision": 17225, "Ġlion": 17226, "tra": 17227, "boards": 17228, "Ġbehaviour": 17229, "hey": 17230, "Ġsubscription": 17231, "Ġprotagon": 17232, "ãĥ£": 17233, "Ġvara": 17234, "ĠÅŁu": 17235, "Ġhaha": 17236, "Ġteaspoon": 17237, "æŁ": 17238, "avoir": 17239, "Ġcrypto": 17240, "ĠÑģÑĤаÑĢ": 17241, "ĠStore": 17242, "abs": 17243, "ĠStudents": 17244, "Ġlaund": 17245, "into": 17246, "Ġapproached": 17247, "°ľ": 17248, "ÑĥÑİÑī": 17249, "ĠLabor": 17250, "otes": 17251, "iatric": 17252, "ĠgroÃŁ": 17253, "utive": 17254, "Ġид": 17255, "ĠGib": 17256, "Ġplacement": 17257, "ĠdifÃŃcil": 17258, "Ġfrog": 17259, "ĠвÑģеÑħ": 17260, "ĠJr": 17261, "azed": 17262, "ÑĥÑī": 17263, "Ġê¼": 17264, "frame": 17265, "аеÑĪÑĮ": 17266, "Ġlockdown": 17267, "åij³": 17268, "Ġmedi": 17269, "Ġ×Ķ×ŀ×": 17270, "ений": 17271, "emale": 17272, "ì¢ħ": 17273, "ateral": 17274, "Ġdistant": 17275, "Ġbears": 17276, "Ġjournalist": 17277, "è§£": 17278, "ĠMarshall": 17279, "ĠIhnen": 17280, "uetooth": 17281, "bag": 17282, "ĠÄijã": 17283, "ĠHighness": 17284, "Ġì°į": 17285, "ика": 17286, "ĠWu": 17287, "ĠFran": 17288, "Ġpeng": 17289, "Ġfon": 17290, "Ġhypothesis": 17291, "ĠÑĢÑĥ": 17292, "Ġly": 17293, "×ļ": 17294, "ìĽĶ": 17295, "ĠRadio": 17296, "à¸ŀ": 17297, "Dav": 17298, "Ġembarrassing": 17299, "ĠìŀĪìĸ´": 17300, "Ġcasting": 17301, "Ġcage": 17302, "ĠPsych": 17303, "ĠìĿ¼ëĭ¨": 17304, "Ġž": 17305, "imb": 17306, "Ġdirectors": 17307, "SH": 17308, "ĠÏĦην": 17309, "á»ģu": 17310, "ĠkonuÅŁ": 17311, "Ġoptional": 17312, "quarters": 17313, "iker": 17314, "ĠSant": 17315, "Ġverses": 17316, "ë¶Ģ": 17317, "Ġolar": 17318, "ĠÏĩ": 17319, "ãĥķ": 17320, "Ġγια": 17321, "ĠImm": 17322, "Ġcontroversial": 17323, "Ġersten": 17324, "Ġrecip": 17325, "ĠChristianity": 17326, "Ġê´ľ": 17327, "ordon": 17328, "×ķש": 17329, "Ġslash": 17330, "ĠPf": 17331, "ÑĥдÑĮ": 17332, "×ķ×Ŀ": 17333, "ĠPerry": 17334, "Ġmamy": 17335, "Ġbackgrounds": 17336, "Ġà®İன": 17337, "Ġpendant": 17338, "ĠColumbia": 17339, "Ġinverse": 17340, "ĠÑĩеÑĢез": 17341, "Ġsv": 17342, "Ġdigging": 17343, "41": 17344, "chem": 17345, "Ġnavigation": 17346, "ĠShin": 17347, "ĠFront": 17348, "PD": 17349, "Ġbearing": 17350, "ĠWasser": 17351, "Ġwax": 17352, "ĠCHRIS": 17353, "ching": 17354, "Ġpressed": 17355, "El": 17356, "ĠDal": 17357, "onsin": 17358, "Ġbinding": 17359, "Ñģкой": 17360, "poons": 17361, "Ġmock": 17362, "arest": 17363, "кÑĢа": 17364, "MM": 17365, "Ġcorrupt": 17366, "storm": 17367, "Ġrefres": 17368, "ĠCoach": 17369, "llä": 17370, "ĠTHIS": 17371, "Ġparag": 17372, "Ġìĵ°": 17373, "pool": 17374, "Ġbillions": 17375, "Ġê¹Ģ": 17376, "group": 17377, "Ġwelcoming": 17378, "cellence": 17379, "ĠDuke": 17380, "긴": 17381, "Ġprimera": 17382, "ìł¸": 17383, "Ġpond": 17384, "Ġstatue": 17385, "Ġ구ë": 17386, "Ġhatch": 17387, "Ġinstrumental": 17388, "Ġresidential": 17389, "커": 17390, "Ġaccepting": 17391, "oshi": 17392, "date": 17393, "Ġì͍": 17394, "Ġplanted": 17395, "Ġjoking": 17396, "ĠìĦľ": 17397, "Ġhated": 17398, "ĠÑĢаÑģÑģк": 17399, "Ġslept": 17400, "Ġpackages": 17401, "Ġislands": 17402, "esen": 17403, "ģı": 17404, "Ġdiagon": 17405, "ĠOsc": 17406, "Ġmesh": 17407, "Ġscales": 17408, "arity": 17409, "ĠDefense": 17410, "ãģ¡ãĤĩ": 17411, "ĠLewis": 17412, "ĠÑģегоднÑı": 17413, "Ġflies": 17414, "uinely": 17415, "ĠConsider": 17416, "Ġstark": 17417, "hew": 17418, "ĠAsÃŃ": 17419, "³´ë": 17420, "Ġpropose": 17421, "Ġíķĺë©´": 17422, "odo": 17423, "ĠNormally": 17424, "Ġheeft": 17425, "ĠHarris": 17426, "gro": 17427, "ĠBlood": 17428, "base": 17429, "ĠiOS": 17430, "Ġtouches": 17431, "Ġinspir": 17432, "Ġ×ĵ": 17433, "Ġbinary": 17434, "Ġì¶Ķ": 17435, "Ġserial": 17436, "Ġion": 17437, "Ġunemployment": 17438, "Ġodds": 17439, "ĠFab": 17440, "ĠFBI": 17441, "BRUN": 17442, "Ġweights": 17443, "νο": 17444, "atile": 17445, "Ġnurses": 17446, "Ġinvolvement": 17447, "Ġíͼ": 17448, "Ġgovernance": 17449, "ĠâĤ¬": 17450, "ÑĢÑĥп": 17451, "ierra": 17452, "íĺķ": 17453, "ĠJerry": 17454, "Ġbeard": 17455, "Ġsalvation": 17456, "ĠAlong": 17457, "gentle": 17458, "ĠKi": 17459, "bol": 17460, "ĠPlat": 17461, "Ġhasht": 17462, "è¿ij": 17463, "Ġware": 17464, "Ġpartie": 17465, "ycz": 17466, "Ġintr": 17467, "Fih": 17468, "nent": 17469, "Ġcheat": 17470, "ilen": 17471, "Ġë¯": 17472, "orie": 17473, "Ġfácil": 17474, "etric": 17475, "Ġaffecting": 17476, "unciation": 17477, "Ġaffairs": 17478, "Ġbee": 17479, "Ġviewing": 17480, "Ġorang": 17481, "ĠLan": 17482, "ĠСÑĤ": 17483, "ä¸ĸ": 17484, "ĠMes": 17485, "ĥģ": 17486, "erie": 17487, "Ġespa": 17488, "Ġinterpre": 17489, "Ġpossess": 17490, "Ġpurely": 17491, "rito": 17492, "found": 17493, "asma": 17494, "ìłģìĿ¸": 17495, "Ġexamine": 17496, "ĠÑĥм": 17497, "Ġbesch": 17498, "ĠTomorrow": 17499, "ĠBlock": 17500, "Ġvariant": 17501, "Ġpreference": 17502, "Ġcoaches": 17503, "Ġmedications": 17504, "ĠíĺĦ": 17505, "Ġempire": 17506, "ëĦ¤": 17507, "ĠIllinois": 17508, "Ġcrispy": 17509, "Ġthì": 17510, "Ġbees": 17511, "77": 17512, "Ġglow": 17513, "èº": 17514, "ĠStudies": 17515, "åIJĦ": 17516, "ĠChallenge": 17517, "Ġunlikely": 17518, "Ч": 17519, "ıyorsun": 17520, "DIE": 17521, "Ġminimize": 17522, "izard": 17523, "Ġún": 17524, "Ġencontrar": 17525, "ĠKill": 17526, "å»": 17527, "Ġvanilla": 17528, "ĠGrant": 17529, "ĠGT": 17530, "sea": 17531, "Ġsought": 17532, "вод": 17533, "Ġnäm": 17534, "ĠAunt": 17535, "OWN": 17536, "Ġpumpkin": 17537, "stellen": 17538, "Ġrag": 17539, "егда": 17540, "Ġstoryt": 17541, "Ġforum": 17542, "æ©Ł": 17543, "Ġestaba": 17544, "uche": 17545, "Ġcongress": 17546, "ĠRey": 17547, "Ġdramatically": 17548, "ĠSport": 17549, "ĠYellow": 17550, "Ġê³ĦìĨį": 17551, "Ġdisgusting": 17552, "ĠRecent": 17553, "Ġacquired": 17554, "Ġcables": 17555, "çĶļ": 17556, "din": 17557, "Ġvisto": 17558, "Ġcommunicating": 17559, "ÑģÑĤавлÑı": 17560, "еÑģÑĤо": 17561, "ãĥ»ãĥ»ãĥ»": 17562, "Ġrég": 17563, "Ġsocks": 17564, "Ġproces": 17565, "because": 17566, "Ġutter": 17567, "Ġcolocar": 17568, "Ġnewest": 17569, "Ġgramm": 17570, "表": 17571, "ä¸įçŁ¥éģĵ": 17572, "Ġshifting": 17573, "Ġcarrier": 17574, "ĠÑģкоÑĢ": 17575, "ĠSchw": 17576, "Ġexecuted": 17577, "Ġmaintained": 17578, "ĠÏĨ": 17579, "ĠMoses": 17580, "Ġdisse": 17581, "Ġhorr": 17582, "ãĢľ": 17583, "Ġrally": 17584, "Ġallem": 17585, "ĠEventually": 17586, "Ġdiyor": 17587, "lvania": 17588, "Ġschnell": 17589, "Ġê³¼": 17590, "Ġ매": 17591, "Ġstruggles": 17592, "late": 17593, "Ġclarify": 17594, "ément": 17595, "Ġmultiplic": 17596, "ибо": 17597, "Ġjourn": 17598, "Ġfragr": 17599, "Ġsurprisingly": 17600, "Ġdesperate": 17601, "52": 17602, "Ġsul": 17603, "ĠRead": 17604, "ĠFried": 17605, "Ġmond": 17606, "woo": 17607, "Ġorganizing": 17608, "ãģĹãĤĩãģĨ": 17609, "ĠSoon": 17610, "ĠвопÑĢоÑģ": 17611, "ĠNur": 17612, "ĠÐĹд": 17613, "Ġspider": 17614, "еÑģÑı": 17615, "Ġtutorials": 17616, "Ġnutrients": 17617, "orer": 17618, "Ġcoefficient": 17619, "Ġarrangement": 17620, "Ġpricing": 17621, "nan": 17622, "yu": 17623, "BL": 17624, "Ġtribe": 17625, "ĠHoward": 17626, "unks": 17627, "Ġnewer": 17628, "Ġprovin": 17629, "Ġprediction": 17630, "hos": 17631, "Ġolsun": 17632, "ĠAround": 17633, "Ġvier": 17634, "ĠÑģÑĤоÑĢон": 17635, "Ġvalley": 17636, "ĠEla": 17637, "ifi": 17638, "Ġgalaxy": 17639, "Ġtranqu": 17640, "Ġadvers": 17641, "ĠTemple": 17642, "iffs": 17643, "igence": 17644, "èĩªå·±": 17645, "Ġkönnte": 17646, "ĠÄijó": 17647, "Did": 17648, "Ġphotographs": 17649, "ĠAWS": 17650, "ÑĨиÑı": 17651, "Ġguards": 17652, "Ġappointed": 17653, "ĠGil": 17654, "Ġмом": 17655, "Ġcod": 17656, "ĠUnlike": 17657, "Ġevenly": 17658, "isconsin": 17659, "Ġestou": 17660, "Ġmnie": 17661, "ĠExec": 17662, "ĠMV": 17663, "ĠEine": 17664, "ä¿¡": 17665, "ĠRoger": 17666, "ĠFac": 17667, "ĠList": 17668, "Ġfuer": 17669, "аеÑĤе": 17670, "omed": 17671, "Ġattraction": 17672, "èī²": 17673, "Ġterrain": 17674, "ĠDrop": 17675, "Ġcorporations": 17676, "Ġsciences": 17677, "Ġthrone": 17678, "ãģĦãģŁ": 17679, "Ġaj": 17680, "ĠRot": 17681, "çī¹": 17682, "Ġsupporters": 17683, "ĠBere": 17684, "Here": 17685, "Ġdiferentes": 17686, "Ġsignificance": 17687, "Ïĥη": 17688, "æĪij覺å¾Ĺ": 17689, "Ġclamp": 17690, "ĠëĮĢë": 17691, "Ġfabulous": 17692, "rez": 17693, "æĮģ": 17694, "Ġassumptions": 17695, "uther": 17696, "wid": 17697, "pot": 17698, "è¿İ": 17699, "Ġyan": 17700, "ulin": 17701, "ÑĢÑĭв": 17702, "ĠSlow": 17703, "ĠPennsy": 17704, "Ġíķ´ìĦľ": 17705, "Ġmeio": 17706, "Ġwealthy": 17707, "ĠEight": 17708, "Ġpulse": 17709, "Ġfriction": 17710, "idity": 17711, "ĠHoll": 17712, "iyorum": 17713, "Ġsounded": 17714, "ĠCarr": 17715, "Ġfork": 17716, "âĺ": 17717, "ĠPA": 17718, "Ġconspir": 17719, "Ġcoding": 17720, "rt": 17721, "ĠTyp": 17722, "Ġìĸij": 17723, "Ġпог": 17724, "Ġmiser": 17725, "ĠÑģмоÑĤÑĢ": 17726, "ĠSweden": 17727, "Ġolarak": 17728, "ĠZhang": 17729, "ĠChi": 17730, "ĠTitan": 17731, "Ġscreening": 17732, "ĠSpider": 17733, "ĠÅŀimdi": 17734, "Ġobstacles": 17735, "lara": 17736, "Ġchallenged": 17737, "pse": 17738, "TON": 17739, "ụ": 17740, "ĠPi": 17741, "Ġlagi": 17742, "ieurs": 17743, "Ġhurting": 17744, "Ġneglect": 17745, "Ġgenerating": 17746, "Ġyoungest": 17747, "Ġaudit": 17748, "ĠÑĢез": 17749, "Ïģά": 17750, "Ġdonate": 17751, "ĠPDF": 17752, "Ġvisits": 17753, "Ġcruise": 17754, "PP": 17755, "aser": 17756, "Ġwsp": 17757, "backs": 17758, "ivals": 17759, "ãģĨãĤĵ": 17760, "Ġdeve": 17761, "Ġproport": 17762, "Ġcath": 17763, "ĠEffect": 17764, "Ġwinds": 17765, "ĠìĻĶ": 17766, "Ġcharts": 17767, "Ġsama": 17768, "Ġautomation": 17769, "Ġпока": 17770, "Ġolan": 17771, "Ġboats": 17772, "Ġcafe": 17773, "Ġdenied": 17774, "ĠMama": 17775, "Ġblocking": 17776, "ĠThor": 17777, "Ġphenomenal": 17778, "Ġstakeholders": 17779, "Ġunos": 17780, "ÑĥеÑĤ": 17781, "ĠAbraham": 17782, "ãģ§ãĤĤ": 17783, "Ġdetection": 17784, "Ġjuris": 17785, "Ġpowered": 17786, "zial": 17787, "Ġwelfare": 17788, "Ġupgrad": 17789, "Ġmożna": 17790, "ĠCase": 17791, "cular": 17792, "ĶìĿ´": 17793, "ãĥģ": 17794, "ĠGuess": 17795, "Ġcycles": 17796, "ä¾ĭ": 17797, "給": 17798, "rock": 17799, "umi": 17800, "Ġelite": 17801, "Ġquè": 17802, "åł±": 17803, "ÑĤом": 17804, "Ġshore": 17805, "gunta": 17806, "Ġku": 17807, "Ġfaithful": 17808, "ĠJeremy": 17809, "aid": 17810, "à·": 17811, "ugal": 17812, "å°įåķĬ": 17813, "ĠVel": 17814, "Ġvrai": 17815, "stell": 17816, "¨¸": 17817, "Ġkol": 17818, "è½": 17819, "Ġquanto": 17820, "ĠзаÑĢ": 17821, "Ġ2002": 17822, "esy": 17823, "Ġreserve": 17824, "ĠмоменÑĤ": 17825, "Ġdeployed": 17826, "Ġdefining": 17827, "Ġsau": 17828, "Ġgaat": 17829, "\")": 17830, "Ġtransmit": 17831, "Ġpublishing": 17832, "Ġranking": 17833, "Ġoffense": 17834, "Ġ46": 17835, "pin": 17836, "ĠTaking": 17837, "Ġentitled": 17838, "Ġgenuinely": 17839, "Ġvariations": 17840, "Ġfinde": 17841, "Ġtau": 17842, "Ġunfortunate": 17843, "ĠRah": 17844, "ports": 17845, "ĠcÅ": 17846, "Ġmonkey": 17847, "Ġbrac": 17848, "wei": 17849, "lung": 17850, "Ġartif": 17851, "Ġsyrup": 17852, "ĠÐĶав": 17853, "Ġlifted": 17854, "Ġchez": 17855, "ĠAdvent": 17856, "ĠStock": 17857, "Ġdol": 17858, "мен": 17859, "иÑĪÑĮ": 17860, "Ġyn": 17861, "gio": 17862, "det": 17863, "Ġdesse": 17864, "Ġgri": 17865, "ĠChairman": 17866, "çħ": 17867, "Ġcuenta": 17868, "anim": 17869, "Ġcrab": 17870, "Ġescal": 17871, "Ġpremière": 17872, "ĠGef": 17873, "Ġdining": 17874, "Ġseventh": 17875, "Ġchasing": 17876, "ĠTower": 17877, "Ġbrutal": 17878, "Ġfundamentally": 17879, "ãģ¨ãģĨ": 17880, "лениÑı": 17881, "stage": 17882, "Ġacquis": 17883, "Ġcylinder": 17884, "Ġcommander": 17885, "mem": 17886, "ĠUV": 17887, "happy": 17888, "Ġepsilon": 17889, "Ġinvitation": 17890, "Ġfarmer": 17891, "chair": 17892, "Ġdestiny": 17893, "Ġsovere": 17894, "ĠHebrew": 17895, "Ġservant": 17896, "Ġbew": 17897, "Ġgast": 17898, "uties": 17899, "Ġadministrative": 17900, "ĠCommand": 17901, "éta": 17902, "Ġnitrogen": 17903, "ê·¼": 17904, "Ġabi": 17905, "Ġvillain": 17906, "Ġblanket": 17907, "ĠSend": 17908, "Ġbeaten": 17909, "²Ħ": 17910, "Ġvolunt": 17911, "Ġscholar": 17912, "ĠEmperor": 17913, "Ġ43": 17914, "vable": 17915, "ĠDus": 17916, "ĠGU": 17917, "Ġtargeting": 17918, "www": 17919, "Ġamendment": 17920, "ìĨĮë": 17921, "Ġting": 17922, "Ġnasty": 17923, "Ġgauge": 17924, "ĠÑĢод": 17925, "ĠHans": 17926, "Your": 17927, "αν": 17928, "Ġprojet": 17929, "ĠHawaii": 17930, "Ġsuspicious": 17931, "Ġschw": 17932, "Ġremoval": 17933, "Ġintrig": 17934, "ĠMU": 17935, "Ġponto": 17936, "ा": 17937, "ĠобÑĢаз": 17938, "Ġguessing": 17939, "pace": 17940, "Ġmothers": 17941, "Ġmillimeter": 17942, "ление": 17943, "没æľī": 17944, "Ġavailability": 17945, "icz": 17946, "æŃ¤": 17947, "Ġfract": 17948, "Ġbases": 17949, "km": 17950, "ĠBTS": 17951, "ĠField": 17952, "Ġdzie": 17953, "Ġsegundo": 17954, "ĠëĤĺëĬĶ": 17955, "Ġlegitimate": 17956, "imas": 17957, "Ġвн": 17958, "Ġcorruption": 17959, "Ġsmash": 17960, "ĠValent": 17961, "Ġaligned": 17962, "ĠPennsylvania": 17963, "Ġgab": 17964, "ĠEun": 17965, "enth": 17966, "ĠMorning": 17967, "Ġcandle": 17968, "Ġbackpack": 17969, "ĠIslamic": 17970, "ações": 17971, "Ġencry": 17972, "Ġmushrooms": 17973, "íĮĮ": 17974, "dit": 17975, "Ġtransit": 17976, "ĠWisconsin": 17977, "Ġparticipated": 17978, "ĠIls": 17979, "Ġunfold": 17980, "¶Ģë": 17981, "Ġprofits": 17982, "Ġwarming": 17983, "ĠGang": 17984, "Ġnetworking": 17985, "Ġmega": 17986, "Ġthoroughly": 17987, "lements": 17988, "ĠHm": 17989, "Ġdeciding": 17990, "Ġemotionally": 17991, "Ġexhausted": 17992, "ĠÐŁÐ¾ÑĤ": 17993, "cido": 17994, "ĠHTML": 17995, "Ġcopyright": 17996, "Ġmelody": 17997, "yim": 17998, "Ġanders": 17999, "oshop": 18000, "Ġë³¼": 18001, "Ġathlete": 18002, "ĠGE": 18003, "Ġfrequent": 18004, "Ġdesires": 18005, "Ġneeding": 18006, "ĠYun": 18007, "Ġrifle": 18008, "Ġlover": 18009, "'T": 18010, "Ġdense": 18011, "Ġtão": 18012, "Ġnotified": 18013, "Ġidi": 18014, "ìĹŃ": 18015, "íĨ": 18016, "Ġinteracting": 18017, "Ġrapport": 18018, "еÑĢи": 18019, "ski": 18020, "Ġbesser": 18021, "Ġmanufacturer": 18022, "ĠKyle": 18023, "Ġaccountable": 18024, "ĠSak": 18025, "ĠPil": 18026, "ĠDomin": 18027, "Ġpresum": 18028, "ĠÐĴÑģе": 18029, "Ġvinegar": 18030, "Ġguaranteed": 18031, "çľĭåΰ": 18032, "Ġhandled": 18033, "éŁ³": 18034, "cat": 18035, "Ġcivilization": 18036, "Ġaccomp": 18037, "ĠVM": 18038, "émon": 18039, "Ġdeze": 18040, "Ġgrades": 18041, "Ġsollte": 18042, "Ġstaring": 18043, "×IJת": 18044, "arnt": 18045, "Ġhorizon": 18046, "Ġtravail": 18047, "hour": 18048, "第ä¸Ģ": 18049, "ĠED": 18050, "ĠDak": 18051, "Ġny": 18052, "Ġconve": 18053, "ĠCham": 18054, "Ġfirms": 18055, "ĠLiu": 18056, "ĠÑģÑĤÑĢан": 18057, "Ġlibert": 18058, "Ġlenses": 18059, "Ġintake": 18060, "ĠвÑĭб": 18061, "Ġmensen": 18062, "hel": 18063, "Ġpractition": 18064, "Ġ350": 18065, "ãĤ³": 18066, "FO": 18067, "Ġbeds": 18068, "Ġancestors": 18069, "ĠìĹĦì²Ń": 18070, "Ġdisturb": 18071, "ĠLastly": 18072, "ĠSupport": 18073, "ีà¹ī": 18074, "ĠCorona": 18075, "Ġenthusi": 18076, "Ġвозм": 18077, "ĠìĤ¬ëŀĮë": 18078, "Ġ52": 18079, "bird": 18080, "Ġreduces": 18081, "ĠìŀĪìĿĦ": 18082, "ĠGene": 18083, "êµIJ": 18084, "ÄĻp": 18085, "ĠÃľber": 18086, "Ġconcerning": 18087, "user": 18088, "Ġconcentrate": 18089, "ĠWHAT": 18090, "ishop": 18091, "onymous": 18092, "nold": 18093, "Ġsuggesting": 18094, "©°": 18095, "ĠFish": 18096, "........": 18097, "Ġvessel": 18098, "Ġtrabajo": 18099, "ãģµ": 18100, "ĠOcean": 18101, "å§IJ": 18102, "yg": 18103, "Ġtowns": 18104, "del": 18105, "Ġterrifying": 18106, "ĠçalÄ±ÅŁ": 18107, "Ġsino": 18108, "Ġeats": 18109, "Ġgez": 18110, "Ġgeme": 18111, "ĠìĻĦ": 18112, "Ġcompart": 18113, "Ġimplementing": 18114, "ĠPotter": 18115, "ĠGermans": 18116, "ĠgÅĤ": 18117, "Ġtennis": 18118, "Ġcarpet": 18119, "auer": 18120, "ĠSaudi": 18121, "yeong": 18122, "Ġcurry": 18123, "ĠForest": 18124, "Ñĭл": 18125, "Ġfifteen": 18126, "Ġbolts": 18127, "Ġ{\\": 18128, "¬´": 18129, "Ġsettlement": 18130, "Ġlange": 18131, "Ġbam": 18132, "Get": 18133, "íķĻ": 18134, "Ġswap": 18135, "ĠKhan": 18136, "Ġcommence": 18137, "Ġquarantine": 18138, "Ġscored": 18139, "çĸ": 18140, "Ġ1950": 18141, "Ġthicker": 18142, "Ġsûr": 18143, "åı£": 18144, "ĠLarry": 18145, "Ġallez": 18146, "ìĭľëĬĶ": 18147, "Ġgü": 18148, "Ġspectacular": 18149, "//": 18150, "both": 18151, "Ġstats": 18152, "妳": 18153, "ĠNancy": 18154, "Ġbunu": 18155, "Ġcrust": 18156, "Ġactivated": 18157, "Ġê·¸ëŀ": 18158, "outhe": 18159, "Ġports": 18160, "Ġneural": 18161, "Ġjaw": 18162, "Ġobservations": 18163, "Ġvoit": 18164, "aban": 18165, "ải": 18166, "¦¬ë¥¼": 18167, "omes": 18168, "à¯ĭ": 18169, "qui": 18170, "Ġkindness": 18171, "Ðij": 18172, "Ġ41": 18173, "Ġmoderate": 18174, "Ġangels": 18175, "ĠTamb": 18176, "èt": 18177, "Ġchlor": 18178, "ĠBilly": 18179, "ì²ĺë": 18180, "acon": 18181, "Ġselecting": 18182, "ĠDelta": 18183, "Ġnull": 18184, "denly": 18185, "Ġciud": 18186, "Ġtendency": 18187, "Ġbreakdown": 18188, "Ġmint": 18189, "ÑĦоÑĢм": 18190, "orph": 18191, "Ġdawn": 18192, "spr": 18193, "ĠWILL": 18194, "ächlich": 18195, "Ġpuppy": 18196, "700": 18197, "Ġத": 18198, "Ġfails": 18199, "ĠConc": 18200, "Ġrelatives": 18201, "Ġinviting": 18202, "Ġautonom": 18203, "Ġcomposed": 18204, "Ġunity": 18205, "Ġdecis": 18206, "Ġaccessories": 18207, "ĠCass": 18208, "Ġbist": 18209, "ĠTip": 18210, "째": 18211, "Ġpunt": 18212, "Ġráp": 18213, "é̲": 18214, "ANK": 18215, "ãģļ": 18216, "exist": 18217, "Ġcompatible": 18218, "Ġner": 18219, "ĠемÑĥ": 18220, "Ġaplic": 18221, "Ġbapt": 18222, "Ġfailing": 18223, "ĠTamam": 18224, "Ġoscill": 18225, "Ġletzten": 18226, "Ġrepeatedly": 18227, "Ġjungle": 18228, "ĠPush": 18229, "hai": 18230, "Ġη": 18231, "Ġdeadly": 18232, "Ñıж": 18233, "wiÄħ": 18234, "ĠCommon": 18235, "ĠÎķ": 18236, "Ġskate": 18237, "TC": 18238, "ĠMini": 18239, "Ġhobby": 18240, "ần": 18241, "Ġroutes": 18242, "Ġamigos": 18243, "Ġconjun": 18244, "Ġpartnerships": 18245, "Ġnovo": 18246, "Ġaver": 18247, "Ġpouvez": 18248, "bridge": 18249, "Ġpreoc": 18250, "him": 18251, "Ġturb": 18252, "Ġsob": 18253, "ĠSnap": 18254, "Ġì°¸": 18255, "minute": 18256, "Ġtraject": 18257, "ujÄĻ": 18258, "Ġeager": 18259, "Ġregulatory": 18260, "Ġbanking": 18261, "bling": 18262, "ÑĪÑĮ": 18263, "aż": 18264, "Ġbizarre": 18265, "itated": 18266, "dire": 18267, "Ġthreatened": 18268, "Ġshining": 18269, "Ġnesse": 18270, "Ġcorps": 18271, "ĠÑģÑĥ": 18272, "Ġteles": 18273, "Ġtemp": 18274, "tem": 18275, "Ġкан": 18276, "Ġfever": 18277, "New": 18278, "Ġheavier": 18279, "ĠSah": 18280, "bud": 18281, "Ġoutros": 18282, "Ġì°¾": 18283, "Ġëªħ": 18284, "arring": 18285, "Ġê´ľì°®": 18286, "ĠNap": 18287, "Ġsemin": 18288, "ĠThan": 18289, "ifs": 18290, "Ġdesen": 18291, "ĠÑĤакое": 18292, "Ġloses": 18293, "ĠBalt": 18294, "kon": 18295, "ĠнапÑĢ": 18296, "Ġvois": 18297, "ĠMoscow": 18298, "Ġchairs": 18299, "his": 18300, "Ġrefugees": 18301, "kg": 18302, "Ġkole": 18303, "į¨": 18304, "аÑģибо": 18305, "¦½": 18306, "ĠUniverse": 18307, "ĠDirect": 18308, "Ġcheating": 18309, "ĠCin": 18310, "Ġpatri": 18311, "Ġadvise": 18312, "ĠNether": 18313, "Ġprimeiro": 18314, "Ġmentioning": 18315, "nut": 18316, "56": 18317, "arı": 18318, "Ġpetite": 18319, "bled": 18320, "Ġpensar": 18321, "icio": 18322, "IND": 18323, "Ġveteran": 18324, "Ġladder": 18325, "Ġconsequence": 18326, "ожал": 18327, "ĠBurn": 18328, "Ġrug": 18329, "ĠMade": 18330, "Ġgit": 18331, "\"...": 18332, "Ġcompetitors": 18333, "Ġprzed": 18334, "Ġapparent": 18335, "ĠArgentina": 18336, "ĠWorking": 18337, "Ġcollaborate": 18338, "woman": 18339, "Ġretain": 18340, "Ġleurs": 18341, "Ġdashboard": 18342, "×Ļ×ĵ": 18343, "ĠEarly": 18344, "BM": 18345, "ĠеÑij": 18346, "олог": 18347, "Ġsatisfying": 18348, "Ġoftentimes": 18349, "Ġmapping": 18350, "ünkü": 18351, "arth": 18352, "fold": 18353, "Ġlaunching": 18354, "Ġaura": 18355, "Ġprecision": 18356, "works": 18357, "God": 18358, "Ġstrap": 18359, "ĠImper": 18360, "Ġrivers": 18361, "Ġ|": 18362, "Ġcuer": 18363, "regon": 18364, "Ġarrival": 18365, "каÑħ": 18366, "ĠMiami": 18367, "анÑĭ": 18368, "Ġsurvivors": 18369, "ĠSenior": 18370, "David": 18371, "Ġestado": 18372, "Ġsectors": 18373, "Ġpopping": 18374, "Ġchim": 18375, "ayı": 18376, "Ġkunnen": 18377, "Ġgallery": 18378, "Ġsunlight": 18379, "esehen": 18380, "Ġyelling": 18381, "ĠMein": 18382, "ĠPhoenix": 18383, "Ġmano": 18384, "Ġhistoria": 18385, "Ġoccurring": 18386, "欸": 18387, "ì¸": 18388, "ади": 18389, "å¾ħ": 18390, "Ġinstitutional": 18391, "ĠTut": 18392, "ç²": 18393, "Ġslaves": 18394, "ãģ©ãģĨ": 18395, "Ġforgiveness": 18396, "Ġtwin": 18397, "ĠHyun": 18398, "нÑĮ": 18399, "ĠKomm": 18400, "andra": 18401, "shot": 18402, "ssä": 18403, "ĠÑĨе": 18404, "atta": 18405, "Ġexpense": 18406, "ĠGPU": 18407, "ĠPast": 18408, "ribly": 18409, "ĠëŃIJìķ¼": 18410, "Ġгода": 18411, "Ġrespir": 18412, "æĿ±": 18413, "ĠQueens": 18414, "hops": 18415, "Ġsérie": 18416, "Ġpref": 18417, "Ġcomed": 18418, "Ġplut": 18419, "ĠOverall": 18420, "ĠãģĿ": 18421, "Ġcush": 18422, "Ġringing": 18423, "Ġincorrect": 18424, "ĠÑģÑĤÑĢ": 18425, "Ġgeometry": 18426, "Ġadvertis": 18427, "ĠШ": 18428, "Ġreviewed": 18429, "ãģĤãģĤ": 18430, "Ġdozens": 18431, "Ġdetermination": 18432, "ĠPhill": 18433, "Ġcontributed": 18434, "ĠCit": 18435, "Ġpassengers": 18436, "Ġcôté": 18437, "Ġrever": 18438, "Ġtechnological": 18439, "Ġallen": 18440, "Ġraining": 18441, "avi": 18442, "Ġsalty": 18443, "Ġtyping": 18444, "ĠÑĤе": 18445, "Ġtilt": 18446, "Ġì¹ĺ": 18447, "ĠоÑĢ": 18448, "ĠпÑĢÑıм": 18449, "Ġrou": 18450, "Ġarena": 18451, "arat": 18452, "åĪ«": 18453, "HHHH": 18454, "Ġmanufacturers": 18455, "ĠEdward": 18456, "Ġtuck": 18457, "Ġblows": 18458, "ingo": 18459, "ĠMarc": 18460, "ìķĦìĦľ": 18461, "Mich": 18462, "ĠClean": 18463, "è´": 18464, "esto": 18465, "ĠPack": 18466, "Ġshaft": 18467, "BRUNO": 18468, "Ġaven": 18469, "uur": 18470, "ÑģколÑĮко": 18471, "ê´Ģ": 18472, "Ġautomated": 18473, "Ġventure": 18474, "Ġsurveillance": 18475, "ĠGrow": 18476, "ĠEmer": 18477, "ĠдоÑĢ": 18478, "Ġinvestor": 18479, "ĠYok": 18480, "Ġlatter": 18481, "ĠNI": 18482, "Ġfunctioning": 18483, "ĠHamilton": 18484, "Ġ51": 18485, "Ġmurdered": 18486, "Ġanchor": 18487, "Ġcuc": 18488, "ĠSCP": 18489, "ĠMadam": 18490, "Ġconstraints": 18491, "Ġbarn": 18492, "anken": 18493, "Ġë§İìĿĢ": 18494, "ĠMotor": 18495, "ĠDoing": 18496, "Ġamen": 18497, "etts": 18498, "Ġinstructor": 18499, "egt": 18500, "ako": 18501, "Ġposture": 18502, "ivia": 18503, "ĠPolish": 18504, "Ġдва": 18505, "Ġcolorful": 18506, "Ġelbow": 18507, "Ġparle": 18508, "Ġpasser": 18509, "Ġcondem": 18510, "ortal": 18511, "Ġfertil": 18512, "اد": 18513, "ĠColomb": 18514, "Ġalignment": 18515, "Ġastronaut": 18516, "ĠMut": 18517, "Ġsalmon": 18518, "Ġstructured": 18519, "ŀר": 18520, "Ġclicks": 18521, "Ġmiej": 18522, "æĶ¿": 18523, "ãģĦãĤĦ": 18524, "ĠRound": 18525, "Ġrainbow": 18526, "ĠVA": 18527, "ãģĶãģĸ": 18528, "ì§Ī": 18529, "otz": 18530, ",</": 18531, "ĠNicole": 18532, "lishing": 18533, "Ġwhilst": 18534, "Ġrepublic": 18535, "Ġtamam": 18536, "verted": 18537, "Ġrecognizing": 18538, "Ġглав": 18539, "Ġdub": 18540, "ĠJos": 18541, "falls": 18542, "ichi": 18543, "ĠczÄĻ": 18544, "ĠЦ": 18545, "ĠMitch": 18546, "CR": 18547, "click": 18548, "ãģĦãģ¦": 18549, "Ġstunning": 18550, "ĠJulia": 18551, "mers": 18552, "ĠPoly": 18553, "Ġdessa": 18554, "Ġinté": 18555, "Ġê³łë": 18556, "ĠdoÄŁ": 18557, "Ġdiver": 18558, "Ġstriking": 18559, "aphor": 18560, "Ġapenas": 18561, "ouses": 18562, "Ġtragedy": 18563, "ĠFan": 18564, "ĠTurkish": 18565, "Ġprophet": 18566, "Ġdistancing": 18567, "ĠHem": 18568, "Ġcartoon": 18569, "Ke": 18570, "anting": 18571, "ĠClark": 18572, "ç¿": 18573, "Ġdavon": 18574, "Ġíħ": 18575, "Ġyummy": 18576, "Ġcompromise": 18577, "Ġstartup": 18578, "ritt": 18579, "Ġcertified": 18580, "Ġpillow": 18581, "bere": 18582, "ì¤Ģ": 18583, "Ġseguir": 18584, "Ġstadium": 18585, "ativo": 18586, "Ġsimpler": 18587, "³¸": 18588, "Ġvisa": 18589, "Ġpathway": 18590, "Ġnuevo": 18591, "Ġray": 18592, "ãĥIJ": 18593, "éľ": 18594, "Ã¶ÃŁ": 18595, "Ġзан": 18596, "Ġcelebrity": 18597, "за": 18598, "Ġeines": 18599, "ĠGiven": 18600, "ĠAra": 18601, "ĠJob": 18602, "Ġyak": 18603, "ĠArbeit": 18604, "ressing": 18605, "ánd": 18606, "Ġgrabbed": 18607, "pend": 18608, "Ġsine": 18609, "irk": 18610, "ĠÐŀÑĤ": 18611, "ĠFle": 18612, "ichen": 18613, "ç¦": 18614, "ĠNeil": 18615, "èĻŁ": 18616, "Ġrepeating": 18617, "Ġdrawings": 18618, "rise": 18619, "Ġglitter": 18620, "five": 18621, "Ġsurt": 18622, "Ġsicher": 18623, "Ġadjustments": 18624, "ĠéĤ£": 18625, "ippi": 18626, "cke": 18627, "Ġrepresentatives": 18628, "Ġmidst": 18629, "Ġspoil": 18630, "meye": 18631, "Ġtags": 18632, "Ġyep": 18633, "ĠStephanie": 18634, "Ġgere": 18635, "ĠRud": 18636, "çĭ": 18637, "Ġgros": 18638, "Ġqueue": 18639, "Ġaccord": 18640, "Ġorganisation": 18641, "endy": 18642, "ĠText": 18643, "üyor": 18644, "ĠÃŃ": 18645, "Ġconclus": 18646, "Ġì¤Ģë": 18647, "Ġamp": 18648, "ĠLess": 18649, "ĠëIJĺëĬĶ": 18650, "cano": 18651, "ĠPix": 18652, "aped": 18653, "Ġdarauf": 18654, "uo": 18655, "ynth": 18656, "abel": 18657, "ĠDone": 18658, "Ġdick": 18659, "athon": 18660, "Ġhilar": 18661, "acco": 18662, "ĠìĨį": 18663, "ĠOregon": 18664, "ĠWeil": 18665, "Ġmathematics": 18666, "Ġalm": 18667, "Ġpixels": 18668, "ĠfrÃ¥n": 18669, "бо": 18670, "FC": 18671, "нÑİ": 18672, "heim": 18673, "gos": 18674, "ĠForget": 18675, "fend": 18676, "ĠVoilÃł": 18677, "ĠGreet": 18678, "ĠαÏħÏĦ": 18679, "Ġrecur": 18680, "æĶ¶": 18681, "51": 18682, "ĠìŀĪê³ł": 18683, "At": 18684, "Ġyards": 18685, "иÑĤи": 18686, "Ġoffset": 18687, "rolling": 18688, "ĠÐŁÐ¾Ñģ": 18689, "Ġenlight": 18690, "ĠPad": 18691, "limited": 18692, "илÑĮно": 18693, "ĠSara": 18694, "ĠÑģделаÑĤÑĮ": 18695, "mart": 18696, "ĠJump": 18697, "Ġadorable": 18698, "orse": 18699, "cheering": 18700, "Ġempathy": 18701, "ĠTonight": 18702, "orp": 18703, "ĠHunter": 18704, "Point": 18705, "га": 18706, "Ġpassenger": 18707, "ĠKnight": 18708, "Ġseemingly": 18709, "huh": 18710, "Ġtheatre": 18711, "Ġtomb": 18712, "Ġdepressed": 18713, "Ġsummon": 18714, "Ġsatisfaction": 18715, "doors": 18716, "ĠHouston": 18717, "аÑİÑī": 18718, "ĠRio": 18719, "глÑı": 18720, "Ġarranged": 18721, "Ġhandles": 18722, "Ġtrillion": 18723, "Ġnightmare": 18724, "ĠQuando": 18725, "Ġole": 18726, "ĠGuide": 18727, "ooo": 18728, "Ġbile": 18729, "Ġempez": 18730, "Ġ72": 18731, "cribed": 18732, "Ġprogression": 18733, "ĠLinux": 18734, "리": 18735, "Ġì²ĺìĿĮ": 18736, "Ġfossil": 18737, "Ġquero": 18738, "ìĨ¡": 18739, "ativa": 18740, "Ġpuzz": 18741, "ĠZus": 18742, "ãĤª": 18743, "Ġthrilled": 18744, "ĠCB": 18745, "Ġminer": 18746, "ÑĢаÑī": 18747, "ĠSAR": 18748, "ĠNos": 18749, "ĠгоÑĢод": 18750, "Ġcamb": 18751, "ĠÑĤа": 18752, "Ġresulted": 18753, "ĠDick": 18754, "oung": 18755, "Ġcomics": 18756, "Ġabsolut": 18757, "stan": 18758, "dimensional": 18759, "Ġtense": 18760, "mus": 18761, "ĠIntell": 18762, "ĠÑįÑĤÑĥ": 18763, "Ġphases": 18764, "Ġvolta": 18765, "Ġvão": 18766, "bound": 18767, "ĠAnderson": 18768, "Ġcuriosity": 18769, "Ġpont": 18770, "éĢĻ裡": 18771, "Ġdemonstrated": 18772, "oline": 18773, "ĠSpeed": 18774, "Ġmama": 18775, "Ġshocking": 18776, "Ġkiedy": 18777, "Ġearthquake": 18778, "Ġimplies": 18779, "Ġenters": 18780, "ŀĢ": 18781, "Ġelevator": 18782, "Ġdelighted": 18783, "ĠMitt": 18784, "ĠBased": 18785, "ĠDol": 18786, "Ġken": 18787, "Ġworrying": 18788, "Ġfiled": 18789, "ailand": 18790, "ĠмеÑĤ": 18791, "Ġmasc": 18792, "ĠÎij": 18793, "ĠJulie": 18794, "Ġdimensional": 18795, "human": 18796, "Tok": 18797, "ÿ": 18798, "Ġunst": 18799, "Ġseule": 18800, "Ġembar": 18801, "Ġíķ©ëĭĪëĭ¤": 18802, "acion": 18803, "Ġìī": 18804, "Ġë¶Ģë¶Ħ": 18805, "Ġheated": 18806, "â̦â̦": 18807, "\"!": 18808, "Ġrealise": 18809, "еÑĤÑĭ": 18810, "ienia": 18811, "iez": 18812, "Ġfüh": 18813, "ĠEsse": 18814, "Ġps": 18815, "Ġdó": 18816, "asters": 18817, "Ġons": 18818, "PM": 18819, "Ġretro": 18820, "maker": 18821, "when": 18822, "Ġella": 18823, "ĠLiving": 18824, "ĠLam": 18825, "Ġtrong": 18826, "Ġapprove": 18827, "Ġθα": 18828, "Ġsung": 18829, "ениÑİ": 18830, "ĠRemove": 18831, "ène": 18832, "iren": 18833, "Ġstranger": 18834, "инÑĭ": 18835, "Ġvæ": 18836, "after": 18837, "otto": 18838, "Ķë¡ľ": 18839, "ĠAhora": 18840, "mill": 18841, "ISH": 18842, "Ġgraduating": 18843, "kte": 18844, "Ġrenov": 18845, "Ġprocessed": 18846, "keys": 18847, "еко": 18848, "Ġenrich": 18849, "ĠÅŁek": 18850, "Ġinsec": 18851, "ĠNan": 18852, "cakes": 18853, "Ġillusion": 18854, "ĺ를": 18855, "Ġairl": 18856, "ims": 18857, "Ġanten": 18858, "ững": 18859, "sn": 18860, "Ġprecisa": 18861, "기ìŀIJ": 18862, "ĠاÙĦع": 18863, "Ġforemost": 18864, "Ġparagraph": 18865, "avais": 18866, "ĠвоÑģ": 18867, "Ġmans": 18868, "ÃŃfic": 18869, "bot": 18870, "ĠعÙĨ": 18871, "Ġbroth": 18872, "Ġalternate": 18873, "ĠChapter": 18874, "Ġvectors": 18875, "esar": 18876, "Ġindication": 18877, "ĠNein": 18878, "¶ģ": 18879, "Ġjeans": 18880, "YE": 18881, "cond": 18882, "Ġunited": 18883, "abi": 18884, "ĠSerge": 18885, "Ġpartially": 18886, "Ġmacro": 18887, "æīį": 18888, "å¼µ": 18889, "Ġethical": 18890, "ruit": 18891, "Ġshifted": 18892, "Ġcabe": 18893, "Ġmathematical": 18894, "Ġrude": 18895, "×Ļ×ķת": 18896, "ĠMerc": 18897, "Ġganze": 18898, "icion": 18899, "Ġunconscious": 18900, "Ġburnt": 18901, "ĠÑĢеб": 18902, "íĬ¸ë": 18903, "Ġcharm": 18904, "andal": 18905, "ì²ľ": 18906, "othy": 18907, "ĠHadi": 18908, "Ġappreciation": 18909, "END": 18910, "Ġréal": 18911, "¶Ħëĵ¤": 18912, "ĠNag": 18913, "ł¤ê³ł": 18914, "ĠLauren": 18915, "ĠvỼi": 18916, "ĠBridge": 18917, "ĠUmm": 18918, "ĠWeg": 18919, "Ġchaque": 18920, "ĠSoph": 18921, "Ġgdzie": 18922, "íijľ": 18923, "Ġster": 18924, "ĠBla": 18925, "Ġreflects": 18926, "Ġbenchmark": 18927, "ваÑĤ": 18928, "amine": 18929, "ãģ¡ãĤĥ": 18930, "Ġanh": 18931, "Ġcontinent": 18932, "ĠFDA": 18933, "ì¡°": 18934, "Ġêtes": 18935, "×Ļ×IJ": 18936, "å¼Ģ": 18937, "Ġbloody": 18938, "ĠNine": 18939, "ielt": 18940, "emand": 18941, "Ġë³´ê³ł": 18942, "Ġtidak": 18943, "ĠScient": 18944, "plex": 18945, "osten": 18946, "Ġanimated": 18947, "assa": 18948, "Ġderived": 18949, "ĠиÑģÑĤоÑĢ": 18950, "ĠMig": 18951, "ìħĺ": 18952, "Ġros": 18953, "plus": 18954, "osaur": 18955, "Ġ^": 18956, "Ġintensive": 18957, "Ġglobally": 18958, "Ġdiferen": 18959, "ìĿ´ê³ł": 18960, "ä½łçļĦ": 18961, "Äħd": 18962, "Ġdés": 18963, "Ġpresentations": 18964, "ĠCro": 18965, "Ġesses": 18966, "ĠBetween": 18967, "Pa": 18968, "Ġnaw": 18969, "à¸Ńà¸ĩ": 18970, "Ġbreed": 18971, "ichte": 18972, "ĠÐŀни": 18973, "ĠBuilding": 18974, "Ġconform": 18975, "MO": 18976, "ĠÐĸ": 18977, "ĠKid": 18978, "nas": 18979, "ĠDue": 18980, "rés": 18981, "Ġdiox": 18982, "ĠBin": 18983, "Ġtaxi": 18984, "Ġsap": 18985, "ĠHub": 18986, "çĤºä»Ģ麼": 18987, "Ġcentered": 18988, "Ġsurge": 18989, "Ġavons": 18990, "Ġlearnt": 18991, "ĠYam": 18992, "ĠDiese": 18993, "ники": 18994, "ĠBeij": 18995, "Will": 18996, "Ġattempted": 18997, "Ġgrief": 18998, "ój": 18999, "Ġkidney": 19000, "Ġopponents": 19001, "æĽ´": 19002, "Ġnome": 19003, "57": 19004, "ÑıÑĤно": 19005, "Ġmidnight": 19006, "Announcer": 19007, "acity": 19008, "oned": 19009, "Ġpuedes": 19010, "Ġproblematic": 19011, "Ġcops": 19012, "ĠPete": 19013, "rint": 19014, "unted": 19015, "Ġbip": 19016, "æ¢": 19017, "ĠÃĢ": 19018, "Ġcens": 19019, "atively": 19020, "Ġä¸į": 19021, "Ġurgent": 19022, "Ġstruggled": 19023, "achus": 19024, "Ġmicrowave": 19025, "ĠSide": 19026, "ĠDenn": 19027, "ĠÑıв": 19028, "Ġurge": 19029, "Ġforcing": 19030, "wang": 19031, "ĠкоÑĤоÑĢаÑı": 19032, "Ġmamm": 19033, "ĠðŁİ": 19034, "Ġtribes": 19035, "ĠShadow": 19036, "ĠSang": 19037, "ĠHitler": 19038, "Ġlun": 19039, "Ġscent": 19040, "ì§ij": 19041, "Ġoverwhelmed": 19042, "Ġbombs": 19043, "Ġcrimin": 19044, "Ġconsolid": 19045, "Ġmolecular": 19046, "×ķ×§": 19047, "nor": 19048, "Ġperceived": 19049, "Ġvé": 19050, "Ġaltogether": 19051, "Ġorth": 19052, "Ġvem": 19053, "Ġzwar": 19054, "izo": 19055, "Å«": 19056, "Ġmelted": 19057, "orden": 19058, "ĠCharlotte": 19059, "ĠExcel": 19060, "arta": 19061, "ìľł": 19062, "ĠGew": 19063, "Ġromance": 19064, "eremos": 19065, "Ġcolonial": 19066, "Ġtraditionally": 19067, "Ġquan": 19068, "hoo": 19069, "Ġchampionship": 19070, "Ġarbitr": 19071, "ìħĶ": 19072, "Ġмин": 19073, "Ġselfish": 19074, "Ġblew": 19075, "rying": 19076, "Ġoperators": 19077, "Ġjurisd": 19078, "ıħ": 19079, "uition": 19080, "ĠEC": 19081, "ĠAnybody": 19082, "vate": 19083, "ieties": 19084, "Ġanalyst": 19085, "´ìĹIJ": 19086, "ĠвÑģегда": 19087, "çek": 19088, "ĠKun": 19089, "Ġaging": 19090, "Õ¡": 19091, "ÑĢаÑĦ": 19092, "ĠMoment": 19093, "ĠHua": 19094, "èĥ": 19095, "then": 19096, "ела": 19097, "estone": 19098, "Ġende": 19099, "Ġawarded": 19100, "Ġnächsten": 19101, "ĠSpot": 19102, "ĠNeg": 19103, "Ġfairy": 19104, "代": 19105, "ĠCover": 19106, "Ġdeposit": 19107, "Ġstressful": 19108, "Ġjunk": 19109, "Ġmetabol": 19110, "Ja": 19111, "Ġê·Ģ": 19112, "Ġundergraduate": 19113, "Ġcancell": 19114, "Ġconsensus": 19115, "Ġoso": 19116, "éĩij": 19117, "ặ": 19118, "ÄŁer": 19119, "rada": 19120, "ĠPalace": 19121, "Ġpedal": 19122, "Ġexagger": 19123, "Ġbehavioral": 19124, "player": 19125, "lles": 19126, "Ġconnector": 19127, "Ġskept": 19128, "įĶëĿ¼ê³ł": 19129, "Ġmitt": 19130, "ĠHaha": 19131, "Ġpeque": 19132, "ĠGott": 19133, "fang": 19134, "à°": 19135, "jos": 19136, "Ġkicking": 19137, "Ġmounted": 19138, "Ġreplacing": 19139, "vos": 19140, "Ġquietly": 19141, "Ġmilit": 19142, "Ġowns": 19143, "Ġniveau": 19144, "Ġaur": 19145, "ĠBuy": 19146, "Ġpredicted": 19147, "Ġcows": 19148, "Ġponer": 19149, "ĠDri": 19150, "Ġremarks": 19151, "Ġreporter": 19152, "ĠarkadaÅŁ": 19153, "еÑģÑĤи": 19154, "Ġsaves": 19155, "Ġçoc": 19156, "Ġmetaphor": 19157, "ĠKel": 19158, "station": 19159, "sembly": 19160, "Ġadvisor": 19161, "Ġworkshops": 19162, "Ġaccounting": 19163, "Ġtok": 19164, "nier": 19165, "inner": 19166, "Ġburada": 19167, "ĠBB": 19168, "ĠOlympic": 19169, "ĠPract": 19170, "Christ": 19171, "ĠÑģÑİ": 19172, "Ġkas": 19173, "Ġviewed": 19174, "Ġmarkers": 19175, "Ġfoto": 19176, "getic": 19177, "ĠLucas": 19178, "Ġpads": 19179, "ĠJoh": 19180, "ĠCDU": 19181, "affen": 19182, "arem": 19183, "ĠBeck": 19184, "ĠGosh": 19185, "shit": 19186, "ãģĮãģ¨ãģĨ": 19187, "ĠMater": 19188, "abulary": 19189, "ĠRoom": 19190, "llen": 19191, "ĠFollowing": 19192, "Ġdoit": 19193, "balls": 19194, "ixa": 19195, "Ġgrounds": 19196, "ĠìŀĪëĬĶëį°": 19197, "LS": 19198, "Ġwildlife": 19199, "ĠSQL": 19200, "Ġshifts": 19201, "ä¸Ģé»ŀ": 19202, "Book": 19203, "Ġhosted": 19204, "llor": 19205, "Ġsnaps": 19206, "Ġbesoin": 19207, "Ġש×Ķ": 19208, "Ġpeanut": 19209, "äft": 19210, "¹ł": 19211, "ÅĽl": 19212, "Audience": 19213, "ĠBarbara": 19214, "Ġadoption": 19215, "Ġwolf": 19216, "ĠоÑģнов": 19217, "arda": 19218, "Ġexpose": 19219, "Ġì¦": 19220, "jas": 19221, "Äĵ": 19222, "Ġcountless": 19223, "Ġì§ģ": 19224, "health": 19225, "uent": 19226, "iso": 19227, "otion": 19228, "Ġhunger": 19229, "Ġmois": 19230, "offs": 19231, "Ġclaiming": 19232, "ĠÎļ": 19233, "ĠBelg": 19234, "Ġнай": 19235, "기ëıĦ": 19236, "Ġunpre": 19237, "Ġged": 19238, "ĠIo": 19239, "ĠпоÑģмоÑĤÑĢ": 19240, "ĠcoÅĽ": 19241, "ĠNarrator": 19242, "ĠÃĩok": 19243, "íĻ©": 19244, "à¸Ńย": 19245, "cipl": 19246, "Ġtimer": 19247, "Ġdefic": 19248, "avin": 19249, "Ġcategor": 19250, "Ġthrows": 19251, "ĠëĤľ": 19252, "ĠпоÑģлед": 19253, "ĠThai": 19254, "Ġmascul": 19255, "Ġbekommen": 19256, "Ġinternation": 19257, "ulse": 19258, "Ġaye": 19259, "Ġpoi": 19260, "Ġpixel": 19261, "Chris": 19262, "Ġstove": 19263, "οι": 19264, "Ġgenerator": 19265, "Ġ컬ë": 19266, "Ġacadem": 19267, "Ġpracticed": 19268, "Ġaquest": 19269, "Ġcontributing": 19270, "ĠIg": 19271, "Ġợ": 19272, "Ġcontaining": 19273, "Ġwrestling": 19274, "ĠÑĩего": 19275, "haupt": 19276, "Ġessas": 19277, "velope": 19278, "Ġexceptional": 19279, "YU": 19280, "ĠApplause": 19281, "ricane": 19282, "Ġconvenience": 19283, "ĠделаÑĤÑĮ": 19284, "илиÑģÑĮ": 19285, "ĠEnviron": 19286, "85": 19287, "Ġcâ": 19288, "ĠìķĪëħķíķĺìĦ¸ìļĶ": 19289, "ĠMO": 19290, "ĠPope": 19291, "Ġsah": 19292, "obi": 19293, "Ġmasters": 19294, "aines": 19295, "Ġblessings": 19296, "Ġobey": 19297, "Ġflux": 19298, "Ġbrow": 19299, "Ġìĭ¤": 19300, "Ġpopularity": 19301, "ĠLamb": 19302, "zeug": 19303, "ìĻĶ": 19304, "ıĦë¡Ŀ": 19305, "ituation": 19306, "Ġaccompan": 19307, "Ġdialog": 19308, "ĠJamie": 19309, "åĬłæ²¹": 19310, "Ġsewing": 19311, "Ġbleeding": 19312, "Ġbail": 19313, "Ġthreads": 19314, "odge": 19315, "ĠShang": 19316, "Ġdeployment": 19317, "ched": 19318, "Ġsatisfy": 19319, "Ġlaz": 19320, "Ġmissile": 19321, "ĠLinked": 19322, "Ġmakers": 19323, "cium": 19324, "fre": 19325, "Ġ먼": 19326, "Ġ무ë": 19327, "ĠEdge": 19328, "Ġsocieties": 19329, "Ġagua": 19330, "Ġsynchron": 19331, "¡ł": 19332, "unft": 19333, "Ġunm": 19334, "Ġtriang": 19335, "Ġinjust": 19336, "top": 19337, "Ġoral": 19338, "kor": 19339, "Ġíķ¨": 19340, "ldigt": 19341, "ceÄŁ": 19342, "quet": 19343, "ĠLeo": 19344, "Ġsavoir": 19345, "Ġeastern": 19346, "ieu": 19347, "Ġexped": 19348, "ĠСп": 19349, "Ġunnecessary": 19350, "ĠPerform": 19351, "ĠMing": 19352, "ĠÑĢав": 19353, "Ġintentions": 19354, "Ġcompression": 19355, "ĠSac": 19356, "ολ": 19357, "arson": 19358, "Ġtrouve": 19359, "ĠMuhammad": 19360, "ĠвÑĭÑģ": 19361, "Ġfinite": 19362, "ĠнаÑħод": 19363, "uga": 19364, "ÑĢазÑĥ": 19365, "Ġcelebrated": 19366, "Ġconfess": 19367, "Ġsquares": 19368, "ĠGordon": 19369, "ĠëĤĺìĺ": 19370, "Ġsyndrome": 19371, "Ġcompletion": 19372, "Ġbacking": 19373, "Ġdarf": 19374, "ĠQuran": 19375, "Ġintermediate": 19376, "Ġker": 19377, "Ġdü": 19378, "hesive": 19379, "Ġaccountability": 19380, "ĠRebecca": 19381, "èijĹ": 19382, "ĠSleep": 19383, "Ġdifférent": 19384, "ols": 19385, "ĠRice": 19386, "Ġ본": 19387, "Ġantibiot": 19388, "ÏĦά": 19389, "rz": 19390, "ambling": 19391, "Ġsensitivity": 19392, "Ġchron": 19393, "allas": 19394, "64": 19395, "Ġfleet": 19396, "Ġoptimistic": 19397, "Ñģкого": 19398, "Ġjadi": 19399, "ailleurs": 19400, "ĠEnough": 19401, "Ġsenin": 19402, "Ġpacks": 19403, "bn": 19404, "ĠArea": 19405, "ĠTro": 19406, "¨ë¦¬": 19407, "аÑĶ": 19408, "ĠThom": 19409, "Ġharmony": 19410, "ника": 19411, "Ġsomeday": 19412, "ISE": 19413, "ĠBroadway": 19414, "lares": 19415, "erness": 19416, "à¹Ħม": 19417, "ĠTenn": 19418, "ĠNATO": 19419, "ãĤĬãģ¾ãģĻ": 19420, "Ġminutos": 19421, "ĠKansas": 19422, "ĠMong": 19423, "Ġcompte": 19424, "åĽĽ": 19425, "Ĭ¤": 19426, "ĠìĹŃ": 19427, "Ġsuperhero": 19428, "ĠGarden": 19429, "ĠMos": 19430, "Ġattachment": 19431, "Ġbust": 19432, "à¯Ĭ": 19433, "ĠThailand": 19434, "stat": 19435, "Ġspice": 19436, "ĠLeb": 19437, "Ġleap": 19438, "zech": 19439, "GL": 19440, "Ġverl": 19441, "Ġfixing": 19442, "Ġë³´ë©´": 19443, "Ġporn": 19444, "Ġbüy": 19445, "ĠÙħا": 19446, "ĠVirt": 19447, "ĠTommy": 19448, "Ġcargo": 19449, "ĠOlha": 19450, "Ġroku": 19451, "ÙĥÙĨ": 19452, "Ġbaked": 19453, "Ġtactics": 19454, "Ġmarketplace": 19455, "Ġktóra": 19456, "arlo": 19457, "Ġswitches": 19458, "Ġcache": 19459, "ĠHR": 19460, "ĠGan": 19461, "ĠGPS": 19462, "Ġduas": 19463, "heres": 19464, "еÑĢÑĪ": 19465, "track": 19466, "Ġlungs": 19467, "Station": 19468, "iggles": 19469, "Ġcamping": 19470, "åĵĩ": 19471, "Ġcompleting": 19472, "amas": 19473, "Ġcycl": 19474, "Ġprototype": 19475, "ĠJudge": 19476, "otypes": 19477, "Ġinfections": 19478, "ł¤ë": 19479, "еÑĢг": 19480, "oba": 19481, "ĠBod": 19482, "ĠSecondly": 19483, "Ġapost": 19484, "Ġsogar": 19485, "Ġreass": 19486, "iek": 19487, "æĸ¼": 19488, "Ġashamed": 19489, "Ġcurves": 19490, "Ġваж": 19491, "Ġensemble": 19492, "atur": 19493, "Ġphotographer": 19494, "Ġeighth": 19495, "Ġwasted": 19496, "ç®Ĺ": 19497, "Ġdamp": 19498, "Ġмал": 19499, "arena": 19500, "Ġinternally": 19501, "Ġheels": 19502, "ĠSalt": 19503, "Ġblir": 19504, "ĪëĤĺ": 19505, "Ġcontrary": 19506, "Ġprima": 19507, "Ġoss": 19508, "Ġrabbit": 19509, "Ġautor": 19510, "Ġbroadly": 19511, "ÃŃst": 19512, "Ġbacks": 19513, "íĶĦ": 19514, "eto": 19515, "Ġjury": 19516, "è±": 19517, "Ġprostu": 19518, "Ġbara": 19519, "Ġparliament": 19520, "orient": 19521, "илаÑģÑĮ": 19522, "Ġindirect": 19523, "ám": 19524, "ĠÃ¥r": 19525, "Ġtraits": 19526, "ĠdÃŃas": 19527, "ÙĦÙħ": 19528, "ĠCT": 19529, "alyst": 19530, "Ġlivest": 19531, "Ġkos": 19532, "May": 19533, "ĠJing": 19534, "Ġjournalists": 19535, "Ñĩик": 19536, "arms": 19537, "Ġê°IJìĤ¬": 19538, "Ġиме": 19539, "Ġégal": 19540, "ĠNewton": 19541, "Ġrecovered": 19542, "Ġbrauchen": 19543, "ĠBron": 19544, "ано": 19545, "Ġpale": 19546, "prises": 19547, "Ġhoras": 19548, "chts": 19549, "éĢļ": 19550, "ÿÿ": 19551, "akers": 19552, "ĠAlaska": 19553, "ziej": 19554, "Ġscoop": 19555, "ìĿ´ê°Ģ": 19556, "ãģķãģĦ": 19557, "cor": 19558, "élé": 19559, "Ġsurg": 19560, "Ġviene": 19561, "ĠKrist": 19562, "54": 19563, "Ġbanned": 19564, "Ġsmoothly": 19565, "Ġtreats": 19566, "Ġpronounce": 19567, "Ġflush": 19568, "Ġcambi": 19569, "Ġmusician": 19570, "ĠAshley": 19571, "ĠSPD": 19572, "ĠBobby": 19573, "Ġgloss": 19574, "respect": 19575, "Ġreviewing": 19576, "Ġgeneric": 19577, "Æ°á»Ľc": 19578, "atsächlich": 19579, "Ġhealthier": 19580, "ubers": 19581, "Ġдан": 19582, "ĠMedicare": 19583, "53": 19584, "Ġcomplaints": 19585, "jac": 19586, "Ġagricultural": 19587, "Spe": 19588, "ĠJong": 19589, "Ġdioxide": 19590, "겨": 19591, "elijk": 19592, "ĠShit": 19593, "aints": 19594, "ĠIan": 19595, "ĠSimply": 19596, "ĠStre": 19597, "æľĭ": 19598, "ĠGDP": 19599, "59": 19600, "asz": 19601, "ĠKatie": 19602, "ĠбÑĢ": 19603, "Ġpeek": 19604, "owych": 19605, "Ġresort": 19606, "Ġresidence": 19607, "Ġspices": 19608, "ció": 19609, "Ġjeder": 19610, "Ġemo": 19611, "arium": 19612, "Ġpuff": 19613, "ë§ī": 19614, "ÑĥлÑĮÑĤ": 19615, "Ġmeta": 19616, "ĠìłĦë": 19617, "Ġoptimization": 19618, "gang": 19619, "ĠíķĦ": 19620, "Ġefficiently": 19621, "Ġvisually": 19622, "Ġfrost": 19623, "ĠArthur": 19624, "Ġż": 19625, "Ġachieving": 19626, "Ġrotating": 19627, "Ġlining": 19628, "Ġoccupied": 19629, "å¼Ł": 19630, "mentation": 19631, "Ġstretching": 19632, "Ġstall": 19633, "ostic": 19634, "ĠSever": 19635, "Ġgluc": 19636, "Ġróż": 19637, "Ġoutreach": 19638, "stra": 19639, "iken": 19640, "Ġìĸĺ기": 19641, "ĠJoin": 19642, "Ġimpe": 19643, "Ġcompensation": 19644, "ĠTat": 19645, "ĠCarlos": 19646, "ührt": 19647, "ĠFrancis": 19648, "cji": 19649, "yeah": 19650, "Ġmembrane": 19651, "Ġexhale": 19652, "Ġreli": 19653, "ĠOR": 19654, "Ġrefrigerator": 19655, "ĠVenez": 19656, "Like": 19657, "Ġraises": 19658, "ottle": 19659, "atura": 19660, "Ġruler": 19661, "Ġweer": 19662, "Ġguided": 19663, "ĠMagn": 19664, "ĠCorpor": 19665, "įĶ": 19666, "Ġattribute": 19667, "ĠWoah": 19668, "Ġarrows": 19669, "Ġawait": 19670, "ĠPrim": 19671, "Ġdignity": 19672, "ĠOntario": 19673, "ischer": 19674, "ĠìĭĿ": 19675, "imen": 19676, "ouver": 19677, "ASS": 19678, "á»ĩn": 19679, "opy": 19680, "achusetts": 19681, "Ġelderly": 19682, "åİŁ": 19683, "FA": 19684, "ĠDaily": 19685, "shine": 19686, "Ġ56": 19687, "è¢": 19688, "ierno": 19689, "Ġskilled": 19690, "ĠgroÃŁe": 19691, "ĠOak": 19692, "第äºĮ": 19693, "iggle": 19694, "елей": 19695, "Ġbiraz": 19696, "Ġarguing": 19697, "ĠпоÑįÑĤомÑĥ": 19698, "Ġdrift": 19699, "Ġharness": 19700, "Ġdeixar": 19701, "autre": 19702, "ĠSeeing": 19703, "Ġcapitalism": 19704, "ĠEld": 19705, "zione": 19706, "ĠBeyond": 19707, "Ġperfection": 19708, "Ġhoe": 19709, "Ġdeclare": 19710, "алаÑģÑĮ": 19711, "Ġpoke": 19712, "Ġס": 19713, "Ġfighters": 19714, "ê²łëĭ¤": 19715, "оÑĢов": 19716, "Ġaccordingly": 19717, "ĠIsa": 19718, "Ġoptimize": 19719, "ĠMinistry": 19720, "Ġsage": 19721, "ìĭľë©´": 19722, "Ġbeni": 19723, "Ġdonation": 19724, "Ġcleared": 19725, "ĠLuckily": 19726, "Ġharmful": 19727, "µì»¤": 19728, "Ġcement": 19729, "пиÑģ": 19730, "Ġdedi": 19731, "ĠCraig": 19732, "Ġdemons": 19733, "Ġcustomize": 19734, "Ġignored": 19735, "ĠTian": 19736, "Ġhoped": 19737, "ĠBureau": 19738, "Ġri": 19739, "ĠYah": 19740, "Ġsocket": 19741, "Ġfeaturing": 19742, "Ġparf": 19743, "ĠTE": 19744, "ĠTeacher": 19745, "Ġcatalog": 19746, "ê°Ģì§Ģê³ł": 19747, "ĠSeite": 19748, "Ġcone": 19749, "ĠPalestin": 19750, "Ġgewoon": 19751, "Ġgaining": 19752, "ĠØ¢": 19753, "Ġcatast": 19754, "Ġneighbour": 19755, "IST": 19756, "Ġstealing": 19757, "Ġtrois": 19758, "Ġintend": 19759, "ĠShoot": 19760, "Ġpione": 19761, "ĠIntel": 19762, "ĠLIN": 19763, "Ġbrighter": 19764, "ĠYesterday": 19765, "Ġsow": 19766, "sin": 19767, "ods": 19768, "Ġethics": 19769, "Ġinterviewed": 19770, "rell": 19771, "Ġrefreshing": 19772, "sÃ¥": 19773, "Ġabsurd": 19774, "Ġphosph": 19775, "fil": 19776, "Ġstehen": 19777, "vals": 19778, "Ġcared": 19779, "æĪĸ": 19780, "Ġdell": 19781, "bone": 19782, "Ġhoch": 19783, "Ġpup": 19784, "Ġio": 19785, "Ġacontece": 19786, "elles": 19787, "ĠSpl": 19788, "igi": 19789, "Ġtän": 19790, "Ġelephant": 19791, "Ġgates": 19792, "Ġslices": 19793, "Ġprank": 19794, "okrat": 19795, "Ġhilarious": 19796, "ĠSid": 19797, "ĠëĴ¤": 19798, "Ġessere": 19799, "Ġtelephone": 19800, "inally": 19801, "rator": 19802, "Ġhelicopter": 19803, "ĠiÅŁte": 19804, "Ġgid": 19805, "Ġtourist": 19806, "Ġconflicts": 19807, "аÑĤа": 19808, "Ġté": 19809, "Ġassert": 19810, "Ġlaundry": 19811, "ĠBom": 19812, "Ġspecialized": 19813, "ĠModern": 19814, "ograf": 19815, "Ġano": 19816, "Ġretrie": 19817, "ĠPutin": 19818, "ĠHAR": 19819, "ĠмаÑĪ": 19820, "ĠαÏĢÏĮ": 19821, "Ġtutti": 19822, "ĠвÑĤоÑĢ": 19823, "ìĸµ": 19824, "ĠBul": 19825, "ëĭ¤ë©´": 19826, "ÅĤe": 19827, "æľĭåıĭ": 19828, "arin": 19829, "Ġtherapist": 19830, "ĠgÃ¥r": 19831, "ĠCzy": 19832, "ppe": 19833, "mir": 19834, "ĠTerm": 19835, "ĠBear": 19836, "lace": 19837, "ĠMoreover": 19838, "ĠDisc": 19839, "ĠíĥĢ": 19840, "Ġtitled": 19841, "Ġstrips": 19842, "ĠFahr": 19843, "ĠRing": 19844, "rando": 19845, "afa": 19846, "身": 19847, "Ġshorts": 19848, "Ġtrunk": 19849, "Ġsentido": 19850, "Ïīν": 19851, "Ġacres": 19852, "Ġoverd": 19853, "ĠOlympics": 19854, "åı«": 19855, "ĠMerci": 19856, "ĠëĤĺìĺ¤": 19857, "Ġgerm": 19858, "ammed": 19859, "Ġpregunt": 19860, "ĠNut": 19861, "Ġ</": 19862, "Ġtravels": 19863, "Ġvocabulary": 19864, "eten": 19865, "oder": 19866, "Ġconsuming": 19867, "writing": 19868, "è¶ħ": 19869, "Ġappearing": 19870, "Ġadjusted": 19871, "sem": 19872, "Ġfrente": 19873, "Ġmaximize": 19874, "Ġzwischen": 19875, "Ġzam": 19876, "conscious": 19877, "zek": 19878, "谢谢": 19879, "hao": 19880, "ì²ĺëŁ¼": 19881, "ĠEpisode": 19882, "Ġvisibility": 19883, "Ġmijn": 19884, "Ġvielen": 19885, "ĠBrothers": 19886, "×Ļ×ij": 19887, "Ġväldigt": 19888, "Ġcrushed": 19889, "ufen": 19890, "ä½łåĢij": 19891, "actic": 19892, "ĠBed": 19893, "ĠFA": 19894, "issippi": 19895, "Ġremot": 19896, "Ġpets": 19897, "Ġthunder": 19898, "ĠMam": 19899, "ìķµì»¤": 19900, "parents": 19901, "Ġbı": 19902, "Ġsurtout": 19903, "Ġsegments": 19904, "Ġnehmen": 19905, "Ġutiliz": 19906, "ĠRuby": 19907, "Ġrá»ĵi": 19908, "Ġhappily": 19909, "Ġbush": 19910, "ultan": 19911, "çİ©": 19912, "ظ": 19913, "ĠHil": 19914, "Ġlawn": 19915, "Ġeyebrows": 19916, "mez": 19917, "ĠSyd": 19918, "rep": 19919, "inf": 19920, "éłŃ": 19921, "Ġoverhead": 19922, "cznie": 19923, "Ġoxid": 19924, "ĠWol": 19925, "Ġdestroying": 19926, "ĠAdditionally": 19927, "umbled": 19928, "dep": 19929, "Ġdepos": 19930, "Ġcommod": 19931, "Ġcakes": 19932, "Ġtalents": 19933, "Ġpourquoi": 19934, "Ġcontempl": 19935, "nels": 19936, "оÑī": 19937, "ĠArabic": 19938, "ĠMaryland": 19939, "çİĭ": 19940, "owo": 19941, "ĠPla": 19942, "ÄŁlum": 19943, "Ġprophe": 19944, "ĠRepresent": 19945, "opol": 19946, "accord": 19947, "ĠMeaning": 19948, "Ġjoints": 19949, "Ġbrakes": 19950, "ckt": 19951, "Ġ1999": 19952, "Ġpublication": 19953, "ĠReview": 19954, "ойд": 19955, "Ġniche": 19956, "Ġsignifica": 19957, "Ġdebr": 19958, "Ġoverlap": 19959, "Ġdemanding": 19960, "ĠSó": 19961, "Ġsubsequent": 19962, "Ġquotes": 19963, "ĠCurrently": 19964, "Ġpreventing": 19965, "Ġ130": 19966, "ĠCel": 19967, "onn": 19968, "wnież": 19969, "ìķ½": 19970, "Ġкакие": 19971, "ACH": 19972, "Ġgum": 19973, "ĠIsraeli": 19974, "ìľ¼ëĭĪê¹Į": 19975, "å¨": 19976, "rukt": 19977, "Ġclapping": 19978, "ĠMassachusetts": 19979, "Ġresilience": 19980, "Ġsubscribing": 19981, "Ġjewelry": 19982, "gebra": 19983, "Ġcorrection": 19984, "boo": 19985, "ئ": 19986, "lio": 19987, "sam": 19988, "Ġenvelope": 19989, "kal": 19990, "ĠFarm": 19991, "Ġcattle": 19992, "Ġbras": 19993, "Ġrepent": 19994, "Ġtones": 19995, "osion": 19996, "pection": 19997, "Ġdenen": 19998, "ÈĽi": 19999, "ĠMarg": 20000, "Ġacquire": 20001, "iblings": 20002, "Ġaspir": 20003, "Ġsized": 20004, "Ġalc": 20005, "Ġvibration": 20006, "til": 20007, "emin": 20008, "Ġcorrelation": 20009, "Ġsingular": 20010, "ĠпоÑıв": 20011, "rek": 20012, "Ġchapters": 20013, "mbre": 20014, "Ġaudition": 20015, "ças": 20016, "Ġvamp": 20017, "Ġtes": 20018, "ĠÑĢазв": 20019, "Ġrespected": 20020, "cin": 20021, "Ġfuckin": 20022, "Ġüberhaupt": 20023, "Ġпоб": 20024, "Ġalike": 20025, "¶Ī": 20026, "robi": 20027, "ît": 20028, "ĠTouch": 20029, "anza": 20030, "Ġfirmly": 20031, "ĠGreetings": 20032, "scale": 20033, "dad": 20034, "акÑĤи": 20035, "Ġbackyard": 20036, "ожд": 20037, "Gr": 20038, "ĠSTE": 20039, "оÑĢÑĤ": 20040, "Ġhätte": 20041, "ĠFirstly": 20042, "ĠOften": 20043, "asures": 20044, "Ġdraws": 20045, "redit": 20046, "ATE": 20047, "Pe": 20048, "CP": 20049, "Ġcompelling": 20050, "Ġsubsid": 20051, "Ġneighborhoods": 20052, "Ġdiplom": 20053, "Ġentender": 20054, "pering": 20055, "aug": 20056, "chat": 20057, "ÐĿÑĥ": 20058, "ĠDoll": 20059, "ĠìłIJ": 20060, "Ġhose": 20061, "nar": 20062, "Ġrewarding": 20063, "ĠSold": 20064, "Ġtaki": 20065, "Ġblades": 20066, "ĠKath": 20067, "Ġjogo": 20068, "Ġsensation": 20069, "uana": 20070, "pel": 20071, "ĠRecently": 20072, "Ġpolymer": 20073, "ĠUP": 20074, "---": 20075, "Ġhover": 20076, "Ġruled": 20077, "æµ·": 20078, "Ġ×Ķ×IJ×": 20079, "Ġaffection": 20080, "ĠÄijá»ĥ": 20081, "Ġbree": 20082, "ç§ģ": 20083, "ĠLay": 20084, "ĠYong": 20085, "Ġreceiver": 20086, "ľë¥¼": 20087, "Ġdisso": 20088, "ĠQing": 20089, "Ġév": 20090, "Ġmúsica": 20091, "Ġaesthetic": 20092, "ĠBreat": 20093, "ĠTA": 20094, "Ġaccurately": 20095, "?âĢĭ": 20096, "Ġwages": 20097, "rawdÄĻ": 20098, "Ġswallow": 20099, "Ġcomplaint": 20100, "Ġlied": 20101, "becue": 20102, "Ġrelaxing": 20103, "ĠPokémon": 20104, "Ġtecn": 20105, "bang": 20106, "³´ì": 20107, "Ġquien": 20108, "номÑĥ": 20109, "Ġhabitat": 20110, "......": 20111, "abling": 20112, "ĠÑĤакие": 20113, "Ġbesond": 20114, "Ġemployed": 20115, "Ġarrives": 20116, "Ġvessels": 20117, "ĠAx": 20118, "Ġdisplays": 20119, "150": 20120, "ologie": 20121, "ĠìĹIJ": 20122, "Ġclo": 20123, "Ġдов": 20124, "ĠÐŀд": 20125, "Ġvuel": 20126, "èĬ±": 20127, "wend": 20128, "Ġslipp": 20129, "urp": 20130, "ĠLot": 20131, "Ġbullets": 20132, "Ġrage": 20133, "Ġskirt": 20134, "ientes": 20135, "Ġnhững": 20136, "ĠNatural": 20137, "Ġhind": 20138, "Ġworkload": 20139, "mu": 20140, "íĥľ": 20141, "Ġsunset": 20142, "вол": 20143, "pit": 20144, "åįģ": 20145, "ĠASH": 20146, "Ġë¶Ħëĵ¤": 20147, "Ġdownstairs": 20148, "éŃ": 20149, "Ġcounted": 20150, "Ġnaz": 20151, "×ķפ": 20152, "ĠPhilippines": 20153, "Ġ110": 20154, "ĠParker": 20155, "Ġgitu": 20156, "Ġinteres": 20157, "Ġumbre": 20158, "ĠNature": 20159, "Ġjer": 20160, "enos": 20161, "Ġpanelists": 20162, "Ġcoating": 20163, "Ġcherry": 20164, "ĠPent": 20165, "ĠMist": 20166, "regation": 20167, "Ġvind": 20168, "ĠCorps": 20169, "ĠMission": 20170, "Ġnoble": 20171, "Ġfonction": 20172, "Ġwarrior": 20173, "Ġprotests": 20174, "ouri": 20175, "Ġconstitutional": 20176, "ÅĤam": 20177, "Ġemerged": 20178, "Ġdye": 20179, "ĠTrying": 20180, "igm": 20181, "ä¸Ģ个": 20182, "équ": 20183, "LO": 20184, "ĠVerm": 20185, "erving": 20186, "ĠTIM": 20187, "ĠCi": 20188, "Ġfreezer": 20189, "Ġgrupo": 20190, "ĠSports": 20191, "ĠпÑĢог": 20192, "ĠÙĦا": 20193, "otherap": 20194, "iffany": 20195, "bian": 20196, "Ġranked": 20197, "Ġproposals": 20198, "ĠÄijây": 20199, "Ġfreezing": 20200, "Ġinsects": 20201, "vil": 20202, "Ġcompost": 20203, "çݰ": 20204, "Ġsemana": 20205, "Ġdistinguish": 20206, "Ġfacilitate": 20207, "Ġplusieurs": 20208, "Ġverg": 20209, "Ġalguns": 20210, "ĠTikTok": 20211, "ĠExpress": 20212, "менÑĤ": 20213, "SU": 20214, "Ġintimate": 20215, "ĠAuthor": 20216, "Ġwitnesses": 20217, "Ġkalau": 20218, "Ġargued": 20219, "Ġavoiding": 20220, "ctive": 20221, "Ġpursuing": 20222, "Ġsyll": 20223, "ável": 20224, "ĠAtlanta": 20225, "ĠUtah": 20226, "ĠTill": 20227, "Ġerf": 20228, "Ġ2022": 20229, "äter": 20230, "Ġfuneral": 20231, "ĠFlash": 20232, "ĠAtlantic": 20233, "Ġgele": 20234, "ì¦Ī": 20235, "Ġmortgage": 20236, "ĠëĦĺ": 20237, "licht": 20238, "Ġambitious": 20239, "ĠBeijing": 20240, "Ġdiving": 20241, "Ġunbox": 20242, "illas": 20243, "Ġotras": 20244, "Ġevac": 20245, "Ġmarine": 20246, "ĠÑģозд": 20247, "ĠCreate": 20248, "Ġgj": 20249, "Ġfrequencies": 20250, "ington": 20251, "ĠRomans": 20252, "Ġaiming": 20253, "ĠBuff": 20254, "Ġemperor": 20255, "ĠMoi": 20256, "Ġpromising": 20257, "ãģľ": 20258, "Ġalguma": 20259, "Ġpasa": 20260, "Ġdisorders": 20261, "SI": 20262, "Ġsucceeded": 20263, "Ġcuerpo": 20264, "Ġsodium": 20265, "Ġstub": 20266, "heiro": 20267, "Ġdelayed": 20268, "etera": 20269, "tw": 20270, "Ġsync": 20271, "hd": 20272, "Ġtourists": 20273, "Ġsyst": 20274, "Ġmét": 20275, "Ġqualify": 20276, "ĠOthers": 20277, "llers": 20278, "аÑĤелÑĮно": 20279, "ĠÐŀна": 20280, "Ġperceive": 20281, "Ġê²Ģ": 20282, "Ġê°Ģìŀ¥": 20283, "ĠиÑģк": 20284, "ĠMatter": 20285, "ĠBluetooth": 20286, "Ġpearl": 20287, "Ġarise": 20288, "Ġmonument": 20289, "Ġименно": 20290, "agi": 20291, "ÙĦÙĬ": 20292, "Ġrho": 20293, "Ġsmarter": 20294, "Ġconj": 20295, "ока": 20296, "Ġkeen": 20297, "ĠTreat": 20298, "клÑİÑĩ": 20299, "Ġpacket": 20300, "elsius": 20301, "ĠAlab": 20302, "ини": 20303, "Ġpsi": 20304, "Ġenjoyable": 20305, "ĠEllen": 20306, "Ġвм": 20307, "Ġeliminated": 20308, "ĠRow": 20309, "Ġzombie": 20310, "ĠKu": 20311, "Ġphrases": 20312, "Ġgren": 20313, "uter": 20314, "Ġdirekt": 20315, "×ĸ": 20316, "enen": 20317, "usa": 20318, "ĠÑģлов": 20319, "İ": 20320, "ĠGh": 20321, "Ġcorrid": 20322, "Ġqueer": 20323, "ĠLinda": 20324, "Ġona": 20325, "Ġobligation": 20326, "dar": 20327, "Ġص": 20328, "emment": 20329, "acies": 20330, "Ġscrewed": 20331, "Ġnak": 20332, "Ġayud": 20333, "ä¸Ķ": 20334, "ár": 20335, "lez": 20336, "Ġdrown": 20337, "ĠMedicine": 20338, "Ġlabs": 20339, "Ġjusqu": 20340, "ĠGonna": 20341, "Ġterrorist": 20342, "quest": 20343, "Ġfarther": 20344, "Ġreplied": 20345, "ĠSW": 20346, "ĠMississippi": 20347, "ishna": 20348, "Ġholder": 20349, "Ġreign": 20350, "Ġacceptance": 20351, "Ġul": 20352, "¶Į": 20353, "ĠHotel": 20354, "ĠCooper": 20355, "tan": 20356, "ĠGrab": 20357, "Ġvapor": 20358, "Ġacted": 20359, "ĠKang": 20360, "fan": 20361, "ĠìĿ´ìĥģ": 20362, "çĶļ麼": 20363, "utet": 20364, "Ġwordt": 20365, "Ġfarms": 20366, "dat": 20367, "Ġcouples": 20368, "Ġbeads": 20369, "ientos": 20370, "Then": 20371, "ä¿Ĥ": 20372, "osity": 20373, "ĠStanford": 20374, ".-": 20375, "Wait": 20376, "Ġdatas": 20377, "oire": 20378, "Ġhashtag": 20379, "imme": 20380, "Ġencountered": 20381, "Ġshouting": 20382, "Ġresistant": 20383, "ĠSeung": 20384, "Ġtragic": 20385, "ĠDraw": 20386, ",,": 20387, "Ġshowcase": 20388, "ĠAF": 20389, "ĠStri": 20390, "Ġbacked": 20391, "ĠÑĥг": 20392, "ĠбÑĥдÑĥÑĤ": 20393, "ĠCole": 20394, "eurs": 20395, "(?)": 20396, "Ġescaped": 20397, "AST": 20398, "ĠAssembly": 20399, "Ġsticker": 20400, "Ġmieux": 20401, "Ġentertaining": 20402, "ĠDON": 20403, "ĠAmend": 20404, "ĠKarl": 20405, "Ġinhib": 20406, "sst": 20407, "ieg": 20408, "~~~": 20409, "Ġhooked": 20410, "Ġliteral": 20411, "Ġsunny": 20412, "steps": 20413, "Ġë°ľë": 20414, "ĠMarine": 20415, "Ġsue": 20416, "Ġprisoners": 20417, "ĠEb": 20418, "58": 20419, "Ġdrums": 20420, "Ġguilt": 20421, "alg": 20422, "Ġhappier": 20423, "ĠCM": 20424, "ĠìķĦëĭĪìķ¼": 20425, "ĠÐŁÐµÑĢ": 20426, "ÑĥлÑı": 20427, "Ġkeyword": 20428, "ĠParce": 20429, "ĠForeign": 20430, "ĠAmanda": 20431, "ç¥ŀ": 20432, "Ġ목": 20433, "pless": 20434, "ά": 20435, "ómo": 20436, "Ġqualquer": 20437, "ìĿ´ëĿ¼ê³ł": 20438, "Ġconspiracy": 20439, "Ġstrawberry": 20440, "Ġhatten": 20441, "Es": 20442, "Ġspos": 20443, "Ġvillages": 20444, "Ġlev": 20445, "ĠÑģÑĢед": 20446, "Ġwaking": 20447, "Ġcalculations": 20448, "ĠÙħع": 20449, "Ġpouring": 20450, "Ġlebih": 20451, "Ġpolish": 20452, "ĠTout": 20453, "Ġfunktion": 20454, "мо": 20455, "ĠTi": 20456, "Ġwasting": 20457, "istically": 20458, "Ġmanipulate": 20459, "Ġsimplify": 20460, "Ġteammates": 20461, "Ġбо": 20462, "Ġcontam": 20463, "ĠQuite": 20464, "Ġkurz": 20465, "ĠCand": 20466, "type": 20467, "outheast": 20468, "Ġfinancially": 20469, "олн": 20470, "elson": 20471, "Ġforehead": 20472, "uage": 20473, "naudible": 20474, "ĠBehind": 20475, "Ġnegotiations": 20476, "Ġë§ĪìĿĮ": 20477, "Ġalternatives": 20478, "rank": 20479, "holder": 20480, "æĩī": 20481, "Ġhealed": 20482, "ÑĤоÑĩ": 20483, "ĠSpec": 20484, "ä»¶": 20485, "ä»ĸåĢij": 20486, "Ġexhibit": 20487, "Ġshallow": 20488, "Ġgob": 20489, "Ġëľ": 20490, "Ġfrustration": 20491, "ÃŃo": 20492, "Ġmelting": 20493, "ĠStorm": 20494, "Ġpatent": 20495, "ĠBarcel": 20496, "Ġpedest": 20497, "ÙĪÙħ": 20498, "Ġtai": 20499, "ĠMode": 20500, "Ġwil": 20501, "Ġ모르": 20502, "Ġégalement": 20503, "éĤ£éº¼": 20504, "Ġ×IJ×Ĺ": 20505, "ayan": 20506, "Ġamazed": 20507, "ì§ĢëĬĶ": 20508, "Ġhaciendo": 20509, "ĠìĿ´ìķ¼": 20510, "λα": 20511, "à¸Ĥ": 20512, "еÑĤа": 20513, "Ġexams": 20514, "Ġtravelling": 20515, "Press": 20516, "иÑĢÑĥ": 20517, "Ġbaseline": 20518, "Ġbuses": 20519, "Ġreinfor": 20520, "venant": 20521, "ĠTruth": 20522, "Ŀ½": 20523, "obe": 20524, "Ġyell": 20525, "Ġsausage": 20526, "TF": 20527, "ĠEvil": 20528, "Ġmeiner": 20529, "×Ļ×§": 20530, "Ġhopeful": 20531, "Ġrównież": 20532, "ĠPerò": 20533, "two": 20534, "nder": 20535, "ĠмиÑĢ": 20536, "Ġconscience": 20537, "ĠWarren": 20538, "icky": 20539, "Ġaimed": 20540, "Ġgöra": 20541, "XT": 20542, "Ġpyram": 20543, "Red": 20544, "鼻": 20545, "atu": 20546, "ĠEsta": 20547, "Ġearnings": 20548, "Ġhats": 20549, "ĠStadt": 20550, "icket": 20551, "points": 20552, "inander": 20553, "Ġmotorcycle": 20554, "ĠëıĮ": 20555, "Ġíķ´ìķ¼": 20556, "kom": 20557, "ĠDing": 20558, "æĴ": 20559, "Ġrecurs": 20560, "Ġestimates": 20561, "Ġderni": 20562, "Ġversch": 20563, "ãģĿãģ®": 20564, "ĠMIC": 20565, "иваÑĤÑĮ": 20566, "ĠпÑĢоÑĪ": 20567, "Ġdost": 20568, "ĠвÑģÑĤÑĢ": 20569, "Ġwiel": 20570, "Ġsiblings": 20571, "Ġдев": 20572, "Ġearliest": 20573, "Ġfatigue": 20574, "Ġnhi": 20575, "Ġgusta": 20576, "Ġbonne": 20577, "æľĢå¾Į": 20578, "from": 20579, "ĠJenny": 20580, "Ġsupposedly": 20581, "intage": 20582, "Ġcounties": 20583, "Ġunre": 20584, "Ġplanting": 20585, "ĠGrac": 20586, "ĠGenesis": 20587, "ĠAlpha": 20588, "ysz": 20589, "Ġtile": 20590, "Ġê²½ìļ°": 20591, "Ġ×Ļש": 20592, "quel": 20593, "Ġdistribute": 20594, "def": 20595, "éral": 20596, "Ġclutch": 20597, "adelph": 20598, "ĠPlayStation": 20599, "Ħ¸": 20600, "Ġsj": 20601, "breaking": 20602, "ĠëIJĺë": 20603, "ĠCuba": 20604, "ĠRussians": 20605, "ĠMARK": 20606, "Ġperse": 20607, "Ġrestricted": 20608, "iges": 20609, "ĠTravel": 20610, "Ġelectronics": 20611, "Ġquarters": 20612, "ĠKeith": 20613, "sized": 20614, "Ġdeadline": 20615, "arenth": 20616, "ĠvÃŃdeos": 20617, "Ġprotocols": 20618, "amment": 20619, "ĠTraining": 20620, "Ġâ": 20621, "Ġsequel": 20622, "нак": 20623, "Ġkeinen": 20624, "Ġmattress": 20625, "luding": 20626, "Ġclassified": 20627, "Ġreactor": 20628, "ĠKont": 20629, "Ġpassar": 20630, "Ġhonour": 20631, "orig": 20632, "INA": 20633, "ĠNathan": 20634, "ва": 20635, "ĠÑģказаÑĤÑĮ": 20636, "tır": 20637, "Ġexclusively": 20638, "Ġshades": 20639, "ĠпÑĢоÑĨ": 20640, "Ġoccasions": 20641, "ija": 20642, "çļĦæĻĤåĢĻ": 20643, "åݲ": 20644, "æħ¢": 20645, "fig": 20646, "Ġtus": 20647, "Ġremem": 20648, "ĠChristopher": 20649, "Ġslime": 20650, "Ġalguna": 20651, "ĠFortunately": 20652, "Ġlors": 20653, "voll": 20654, "aver": 20655, "Ġoutlet": 20656, "ĠLinkedIn": 20657, "ĠExecutive": 20658, "Ġorgans": 20659, "ĠBegin": 20660, "ĠíĻĶ": 20661, "Ġtransplant": 20662, "ragen": 20663, "VO": 20664, "ĠFör": 20665, "ĠباÙĦ": 20666, "ĠAndre": 20667, "isine": 20668, "Ġlasts": 20669, "Ġhistória": 20670, "Ġluz": 20671, "Ġcollar": 20672, "Ġkidna": 20673, "Ġoptical": 20674, "iov": 20675, "Ġtob": 20676, "Ġexterior": 20677, "Ġmetric": 20678, "ieur": 20679, "Ġtroll": 20680, "ĠÑĢоз": 20681, "æĺŁ": 20682, "Ġtô": 20683, "ĠìĺĪìģ": 20684, "ĠGesetz": 20685, "Ġед": 20686, "Ġdenominator": 20687, "ì³": 20688, "Ġlett": 20689, "åħī": 20690, "ĠgrÃ¶ÃŁ": 20691, "é¡ĺ": 20692, "ĠLuther": 20693, "Ġreste": 20694, "Ġresemb": 20695, "Ġpermet": 20696, "ksi": 20697, "Ġfisher": 20698, "ãģŁãģĦ": 20699, "ĠVon": 20700, "íͼ": 20701, "ĠÏĥÏĦο": 20702, "Ġlocks": 20703, "Ġshoots": 20704, "Ġkamu": 20705, "ĠKer": 20706, "ĠObs": 20707, "çĿĢ": 20708, "Ġbili": 20709, "Ġë°±": 20710, "Ġtorture": 20711, "assy": 20712, "Ġиг": 20713, "Ġlasting": 20714, "好çļĦ": 20715, "Ġtienes": 20716, "Ġreceives": 20717, "ĠOscar": 20718, "Ġremembering": 20719, "Ġproblemas": 20720, "Ġia": 20721, "åĺĽ": 20722, "Ġmemorable": 20723, "Ġjours": 20724, "Ġfaçon": 20725, "amic": 20726, "Ġë´¤": 20727, "atique": 20728, "ĠëŃĶê°Ģ": 20729, "Ġzip": 20730, "halt": 20731, "ĠðŁĺ": 20732, "Ġfries": 20733, "Ġfinden": 20734, "gra": 20735, "ÑĢÑĥд": 20736, "import": 20737, "Ġëĭ¬ë": 20738, "Ġiki": 20739, "Ġcomplaining": 20740, "Ġfazendo": 20741, "Ġgoogle": 20742, "Ġtabs": 20743, "Ġëĵ¤ìĸ´ì": 20744, "ãĤ¦": 20745, "ugo": 20746, "ierto": 20747, "aufen": 20748, "Ġ먼ìłĢ": 20749, "Ġskulle": 20750, "Ġsuiv": 20751, "Ġspy": 20752, "ĠKai": 20753, "éĤ£åĢĭ": 20754, "Ġmartial": 20755, "Ġonder": 20756, "誰": 20757, "atility": 20758, "Ġirgendwie": 20759, "Ġclap": 20760, "intell": 20761, "Ġinstalling": 20762, "Ġuniqu": 20763, "ĠCentre": 20764, "asts": 20765, "uar": 20766, "Ġrevis": 20767, "Ġthreatening": 20768, "rais": 20769, "Ġcuid": 20770, "ska": 20771, "Ġresolved": 20772, "Ġrides": 20773, "Ġfailures": 20774, "Ġsemb": 20775, "Ġmales": 20776, "UFF": 20777, "å¾Īå¤ļ": 20778, "Ġtrês": 20779, "apped": 20780, "Ġnewspapers": 20781, "riet": 20782, "Ġapplauds": 20783, "Ðĵ": 20784, "Ġãģ¯": 20785, "ĠNC": 20786, "åįĥ": 20787, "æĻĤéĸĵ": 20788, "Ġheter": 20789, "Ġhazard": 20790, "Ġry": 20791, "Ġstrictly": 20792, "Ġ54": 20793, "Ġëĵ¤ìĸ´ê°Ģ": 20794, "Ġspont": 20795, "Ġtatsächlich": 20796, "Ġë§IJìĶ": 20797, "laub": 20798, "Ġabsorbed": 20799, "acaģız": 20800, "Ġonu": 20801, "ĠÐIJн": 20802, "Ġexplicitly": 20803, "Ġìŀ¬": 20804, "ĠFuture": 20805, "achten": 20806, "Ãło": 20807, "yon": 20808, "Ġseria": 20809, "ĠHerren": 20810, "cej": 20811, "ĠAlbert": 20812, "ìĿ´ëĬĶ": 20813, "ector": 20814, "Ġpacking": 20815, "Ġvirtue": 20816, "Ġvenir": 20817, "DD": 20818, "Ġyaz": 20819, "Ġlogs": 20820, "ĠPhotoshop": 20821, "Ġsid": 20822, "lings": 20823, "Ġremotely": 20824, "ĠDifferent": 20825, "Ġoperated": 20826, "lights": 20827, "Ġdiscrimin": 20828, "istance": 20829, "ĠGRE": 20830, "Ġplac": 20831, "Ġshirts": 20832, "Ġjustify": 20833, "Ġtrabalho": 20834, "util": 20835, "voc": 20836, "Ġquart": 20837, "ĠΤ": 20838, "SC": 20839, "ĠSR": 20840, "Ġ-\"": 20841, "Ġhesitate": 20842, "Ġpak": 20843, "èĩ³": 20844, "gua": 20845, "Jo": 20846, "Ġsouvent": 20847, "ĠAngela": 20848, "essee": 20849, "adelphia": 20850, "arks": 20851, "Ġweed": 20852, "Ġkannst": 20853, "åĤĻ": 20854, "Ġê·¸ëŁ¬ëĭĪê¹Į": 20855, "Ġplutôt": 20856, "ĠCommander": 20857, "Ġsummarize": 20858, "à¯Ģ": 20859, "Ġ98": 20860, "ãģĩ": 20861, "Ġdevelopments": 20862, "ĠCost": 20863, "Ġtheoretical": 20864, "Ġore": 20865, "Ġmetall": 20866, "οÏħν": 20867, "fahr": 20868, "ÐļÐIJ": 20869, "Ġchuck": 20870, "Ġadapted": 20871, "ĠOklah": 20872, "ĠNetherlands": 20873, "Ġpoet": 20874, "sto": 20875, "kat": 20876, "Ġwears": 20877, "ç¯": 20878, "Ġìĸ´ëĶĶ": 20879, "ĠEsto": 20880, "Ġlaughed": 20881, "Ġdonner": 20882, "Ġëį°": 20883, "ĠìĽIJë": 20884, "ocur": 20885, "ĠKick": 20886, "ĠDetroit": 20887, "Ġbicycle": 20888, "Ġlacking": 20889, "phabet": 20890, "ĠKend": 20891, "Ass": 20892, "Ġreveals": 20893, "ĠÎł": 20894, "ĠNoah": 20895, "¦¬ëĬĶ": 20896, "Ġsells": 20897, "ĠAlabama": 20898, "Ġterrific": 20899, "ĠElement": 20900, "ĠíĨ": 20901, "Ġturbo": 20902, "ĠHom": 20903, "Ġtheorem": 20904, "Ġadventures": 20905, "Ġpurchasing": 20906, "ĠTá": 20907, "ĠмаÑĤ": 20908, "Ġvemos": 20909, "Ġduties": 20910, "Ġwenig": 20911, "Ġbooth": 20912, "Ġentrar": 20913, "VA": 20914, "Ġgears": 20915, "ĠJae": 20916, "èn": 20917, "Ġcalcium": 20918, "ĠRoberts": 20919, "ĠпÑĢоблем": 20920, "Ġribbon": 20921, "ĠназÑĭв": 20922, "Ġlav": 20923, "Ġinterventions": 20924, "ĠUltra": 20925, "Ġnamely": 20926, "Ġadequate": 20927, "Ġrecap": 20928, "Ġdock": 20929, "fting": 20930, "Ġvoi": 20931, "Ġconsultation": 20932, "ĠÑģем": 20933, "Ġpodem": 20934, "Ġpossession": 20935, "Ġclues": 20936, "ĠRussell": 20937, "Ġrenewable": 20938, "åݲ害": 20939, "ĠÑĥз": 20940, "information": 20941, "iggers": 20942, "With": 20943, "wno": 20944, "Ġelaborate": 20945, "ctoral": 20946, "ĠDow": 20947, "Ġramen": 20948, "æıIJ": 20949, "á»ķ": 20950, "Ġerste": 20951, "ĠZel": 20952, "ãĥĹ": 20953, "Ġquasi": 20954, "Ġнак": 20955, "ç§Ĵ": 20956, "ĠStars": 20957, "Ġtribal": 20958, "Ġseated": 20959, "Ġwol": 20960, "Ġchol": 20961, "ämä": 20962, "Ġoutbreak": 20963, "Ġcres": 20964, "Ġunserer": 20965, "Ġíijľ": 20966, "Ġunderwater": 20967, "Ġassure": 20968, "OOD": 20969, "ĠnaprawdÄĻ": 20970, "Ġestablishment": 20971, "Ġincon": 20972, "Ġdiferente": 20973, "Ġexcus": 20974, "ĠDim": 20975, "оÑħ": 20976, "ĠLing": 20977, "rolog": 20978, "Ġãģ¾": 20979, "Ġoutdoors": 20980, "naj": 20981, "Ġepidemic": 20982, "Ġunters": 20983, "Ġ3000": 20984, "ĠGabriel": 20985, "ĠìĹĨëĬĶ": 20986, "Ġencl": 20987, "ĠOder": 20988, "ĠFoot": 20989, "pas": 20990, "ĠZuk": 20991, "åĵ¡": 20992, "Ġworkflow": 20993, "Ġunp": 20994, "Ġalliance": 20995, "enschaft": 20996, "Ġyogurt": 20997, "ине": 20998, "Ġeru": 20999, "Ġfiz": 21000, "äºĶ": 21001, "ĠaÅŁ": 21002, "Ġaprend": 21003, "Ġcualquier": 21004, "Ġcarrots": 21005, "ının": 21006, "afood": 21007, "Ġfloors": 21008, "Ġkeywords": 21009, "Ġspotted": 21010, "Ġdrank": 21011, "Ġparas": 21012, "Ġúltimo": 21013, "Ġhablar": 21014, "Ġprosecut": 21015, "ìĹIJëıĦ": 21016, "éĸĭå§ĭ": 21017, "Ġép": 21018, "Ġstickers": 21019, "Ġpushes": 21020, "kh": 21021, "Ġrestart": 21022, "ĠThunder": 21023, "á»Ŀi": 21024, "Ġmuita": 21025, "Ġfox": 21026, "ardeÅŁ": 21027, "ĠZach": 21028, "ĠMinecraft": 21029, "ç¸": 21030, "Ġ====": 21031, "Ġgöre": 21032, "Ġstance": 21033, "igung": 21034, "ÙİÙij": 21035, "kä": 21036, "Ġteachings": 21037, "éĨ": 21038, "Ġdecay": 21039, "Ġric": 21040, "omena": 21041, "ĠвÑģем": 21042, "chten": 21043, "ĠVert": 21044, "ĠíķľêµŃ": 21045, "¬´ë": 21046, "Ġcoc": 21047, ":)": 21048, "keiten": 21049, "ĠBA": 21050, "etheless": 21051, "Ġheadquarters": 21052, "Ġspike": 21053, "ĠBase": 21054, "Ġ101": 21055, "Ġcoordinates": 21056, "Ġtard": 21057, "Ġboiled": 21058, "ĠMonster": 21059, "Ġnotebook": 21060, "Ġê´Ģ": 21061, "ĠWake": 21062, "ĠSetting": 21063, "ìĿ´ìĹ": 21064, "ĠSydney": 21065, "ĠFinn": 21066, "Ġlobby": 21067, "å¾ŀ": 21068, "Ġseniors": 21069, "ниÑħ": 21070, "avan": 21071, "ĠJE": 21072, "Ġtraff": 21073, "think": 21074, "Ġslap": 21075, "ĠCastle": 21076, "©ng": 21077, "Ġalgunos": 21078, "ĠPersonally": 21079, "ĠMale": 21080, "íĭ°": 21081, "ĠGenerally": 21082, "ĠPel": 21083, "Ġdias": 21084, "Ġevolving": 21085, "itol": 21086, "воÑĢ": 21087, "Ġplein": 21088, "Ġflights": 21089, "Ġeleven": 21090, "owej": 21091, "á»ijng": 21092, "Ġaku": 21093, "Ġglance": 21094, "Ġconnectivity": 21095, "Ġbald": 21096, "ÑĭÑĩ": 21097, "Ġintest": 21098, "ág": 21099, "ĠGRÃľ": 21100, "iblical": 21101, "ĠPapa": 21102, "Ġpity": 21103, "Ġfaint": 21104, "Ġwurden": 21105, "Ġlegally": 21106, "Ġprey": 21107, "ĠSciences": 21108, "ĠпÑĢоÑģ": 21109, "Ġtrainer": 21110, "Ġproblème": 21111, "Ġkilo": 21112, "кого": 21113, "Ġbridges": 21114, "89": 21115, "Ġlasted": 21116, "Ġelegant": 21117, "bows": 21118, "Ġpalab": 21119, "Ġdirectory": 21120, "ä¸įæľĥ": 21121, "Ġbulb": 21122, "people": 21123, "IX": 21124, "Ġgeb": 21125, "Ġ66": 21126, "ĠTennessee": 21127, "ahlen": 21128, "ieval": 21129, "Ġcaut": 21130, "ĠDamen": 21131, "plo": 21132, "iane": 21133, "але": 21134, "attan": 21135, "ĠاÙĦس": 21136, "Ġrisky": 21137, "Ġsleeve": 21138, "Ġincidents": 21139, "Ġë°ķ": 21140, "Co": 21141, "Ġapplicable": 21142, "Ġimperial": 21143, "ĠPhilip": 21144, "ĠYea": 21145, "еÑĢо": 21146, "Ġпоказ": 21147, "üne": 21148, "ìĺĢ": 21149, "Hub": 21150, "tor": 21151, "Ġsigu": 21152, "cend": 21153, "Ġpolitically": 21154, "ĠìĤ´": 21155, "Ġpars": 21156, "Ġouv": 21157, "Ġprimeira": 21158, "ĠShah": 21159, "Ġsatur": 21160, "Ġcombust": 21161, "Ġpromoted": 21162, "주ë": 21163, "æĢķ": 21164, "Ġtemplates": 21165, "Ġëĭ¬": 21166, "Ġhaul": 21167, "ĠÑĤеÑĢ": 21168, "Ġsliding": 21169, "cedented": 21170, "Ġãģ®": 21171, "children": 21172, "MR": 21173, "ĠWei": 21174, "Ġbör": 21175, "æĹ©": 21176, "Ġpróximo": 21177, "arÃŃa": 21178, "Ġsampling": 21179, "елен": 21180, "esi": 21181, "ĠDanielle": 21182, "ĠOklahoma": 21183, "èħ": 21184, "çķĮ": 21185, "еÑģп": 21186, "ĠDVD": 21187, "ĠвÑĭп": 21188, "rous": 21189, "cons": 21190, "Ġenhanced": 21191, "éĽ£": 21192, "Ġpastor": 21193, "ĠSuddenly": 21194, "è®ĵ": 21195, "far": 21196, "PER": 21197, "ĠNg": 21198, "1000": 21199, "Ġchew": 21200, "Ġrumors": 21201, "ĠAna": 21202, "Ġannées": 21203, "ĠÑĥÑģÑĤ": 21204, "ĠPhiladelphia": 21205, "åĹ¯": 21206, "еждÑĥ": 21207, "Ġeffectiveness": 21208, "è¿Ļæł·": 21209, "été": 21210, "Ġding": 21211, "Ġreligions": 21212, "Ġaged": 21213, "zieÄĩ": 21214, "ĠRic": 21215, "ĠKap": 21216, "ĠPage": 21217, "Ġsü": 21218, "Ġnämlich": 21219, "Ġmankind": 21220, "Ġresting": 21221, "Ġinfluences": 21222, "ĠSchul": 21223, "Ġнев": 21224, "Ġmana": 21225, "Ġconsumed": 21226, "ĠPom": 21227, "ç¾İåľĭ": 21228, "Ġconseguir": 21229, "ĠThanksgiving": 21230, "ĠHindu": 21231, "lais": 21232, "Ġthrive": 21233, "Ġcontour": 21234, "аÑĨиÑı": 21235, "Ġfalando": 21236, "ĠJá": 21237, "zan": 21238, "иÑĤÑĥ": 21239, "ipher": 21240, "jamin": 21241, "ĠHallo": 21242, "Ġ160": 21243, "ĠоÑģоб": 21244, "Ġmete": 21245, "ĠìķĮë": 21246, "ĠBarcelona": 21247, "letter": 21248, "ĠÐĿеÑĤ": 21249, "åĻ": 21250, "Ġademás": 21251, "Ġcoordination": 21252, "unts": 21253, "Ġslop": 21254, "ĠпÑĢид": 21255, "ì§Ģë§ī": 21256, "Ġquestioning": 21257, "Ġdiesel": 21258, "Ġdej": 21259, "Ġaffirm": 21260, "įĶëĿ¼ê³łìļĶ": 21261, "ienne": 21262, "Ġcrank": 21263, "Ġpredictions": 21264, "Ġphysi": 21265, "chsel": 21266, "Ġcombinations": 21267, "Ġexcellence": 21268, "éĢĻ麼": 21269, "á»Ŀ": 21270, "width": 21271, "weed": 21272, "Ħ를": 21273, "Ħë§Ī": 21274, "Ġalto": 21275, "Ġdairy": 21276, "ĠNormal": 21277, "ppen": 21278, "Ġoben": 21279, "Ġdevastating": 21280, "Ġpoz": 21281, "ĠHus": 21282, "maz": 21283, "Ġwarned": 21284, "Ġdenk": 21285, "ĠAuss": 21286, "Ġtrades": 21287, "hell": 21288, "Ġprimero": 21289, "Ġmia": 21290, "ваÑĢ": 21291, "بÙĬ": 21292, "Ġkicks": 21293, "ĠaÄŁ": 21294, "ĠMü": 21295, "Ġluc": 21296, "ением": 21297, "ĠStandard": 21298, "rice": 21299, "ĠCub": 21300, "Ġgou": 21301, "ĠJoão": 21302, "ÑĥÑģк": 21303, "Ġenqu": 21304, "£Į": 21305, "gew": 21306, "Ġíģ°": 21307, "owania": 21308, "iani": 21309, "Ġfakt": 21310, "Ñıни": 21311, "Ġbef": 21312, "Ġthumbna": 21313, "Ġceux": 21314, "æŃ¡è¿İ": 21315, "apple": 21316, "NEN": 21317, "Ġgad": 21318, "apon": 21319, "ĠFantastic": 21320, "Ġconcentrated": 21321, "girl": 21322, "lene": 21323, "ĠÐĶлÑı": 21324, "Ġéta": 21325, "aan": 21326, "Ġoutta": 21327, "Ġnarc": 21328, "ĠBody": 21329, "brush": 21330, "Ġlegislative": 21331, "ĠMegan": 21332, "Ġmistaken": 21333, "ĠMissouri": 21334, "Ġlabeled": 21335, "лÑıеÑĤÑģÑı": 21336, "Ġrealised": 21337, "yorsun": 21338, "ãģĤãĤĬãģĮãģ¨ãģĨ": 21339, "ĠSafety": 21340, "Ġaccelerate": 21341, "Ġsanctions": 21342, "Ġpee": 21343, "Ġjuego": 21344, "Ġpeppers": 21345, "Ġwal": 21346, "ê¸ī": 21347, "ellow": 21348, "Ġжен": 21349, "Ġcinco": 21350, "ĠÑģиÑģÑĤ": 21351, "covery": 21352, "Ġgram": 21353, "Ġépo": 21354, "ĠBMW": 21355, "ivol": 21356, "ĠChem": 21357, "çļĦ話": 21358, "usement": 21359, "ĠSuppose": 21360, "Ġê°Ģì§Ģê³ł": 21361, "Ġmillenn": 21362, "ĠTun": 21363, "Ġmedal": 21364, "Ġhacia": 21365, "Ġstimulus": 21366, "Ġbrightness": 21367, "aient": 21368, "ĠHands": 21369, "inet": 21370, "Ġcoalition": 21371, "åѸ": 21372, "Ġrises": 21373, "rina": 21374, "Ġscoot": 21375, "Ġãģ§": 21376, "Ġdefending": 21377, "Ġinvers": 21378, "Ġhills": 21379, "Ġfulfilled": 21380, "åΰäºĨ": 21381, "llie": 21382, "Ġadoles": 21383, "ĠChase": 21384, "åĸľæŃ¡": 21385, "ĠJJ": 21386, "Ġneuen": 21387, "ĠTru": 21388, "Ġinherit": 21389, "Ġsixty": 21390, "ĠExp": 21391, "ĠClay": 21392, "оÑģоб": 21393, "arna": 21394, "ĠImperial": 21395, "ĠÑįÑĤа": 21396, "Ġsocially": 21397, "aty": 21398, "odynam": 21399, "Ġribs": 21400, "omic": 21401, "ĠTol": 21402, "олж": 21403, "Ġ1998": 21404, "Ġfram": 21405, "Ġranks": 21406, "ĠбÑĥдÑĥ": 21407, "ĠColon": 21408, "Hz": 21409, "Ġaccommodate": 21410, "Ġexplode": 21411, "íĦ°ë": 21412, "HAEL": 21413, "ĠHart": 21414, "Ġжизни": 21415, "æ¡": 21416, "Ġdelicate": 21417, "ł×Ĺ": 21418, "Ġtofu": 21419, "Ġachievements": 21420, "ĠSor": 21421, "Ġagreements": 21422, "Ġ57": 21423, "Ġtamp": 21424, "Ġfrançais": 21425, "Ġherbs": 21426, "corn": 21427, "Ġkonk": 21428, "ANA": 21429, "ĠQi": 21430, "Ġpróp": 21431, "Ġtiger": 21432, "Ġëijĺ": 21433, "Äĥm": 21434, "Ġapprent": 21435, "ahan": 21436, "Ġruling": 21437, "Ġtsp": 21438, "Ġtwitter": 21439, "Ġteenager": 21440, "bus": 21441, "ĠíĴ": 21442, "ĠAmendment": 21443, "Ġtapping": 21444, "ĠAPIs": 21445, "åł´": 21446, "Ġmatched": 21447, "ë©´": 21448, "WA": 21449, "ĠBeauty": 21450, "Ġinevitable": 21451, "Ġgases": 21452, "ĠÙ¾": 21453, "high": 21454, "ĠOpt": 21455, "Ġpredomin": 21456, "ÏģÏĮ": 21457, "Ġtubes": 21458, "Ġìķł": 21459, "ĠAa": 21460, "Ġæľī": 21461, "ometown": 21462, "ĠIM": 21463, "Ġdesar": 21464, "ären": 21465, "ĠмаÑģ": 21466, "ĠMöglich": 21467, "Ġrental": 21468, "Ġíķ¨ê»ĺ": 21469, "ĠDiana": 21470, "Ġautism": 21471, "ĠPuerto": 21472, "ıld": 21473, "Ġfalan": 21474, "Ġdreaming": 21475, "Ġgute": 21476, "Ġкам": 21477, "Ġwreck": 21478, "Ġstorytelling": 21479, "ĠLegend": 21480, "ĠUkrain": 21481, "ĠпÑĢоиÑģ": 21482, "ĠSK": 21483, "Ġíĸī": 21484, "ĠÅĽwi": 21485, "ĠBelieve": 21486, "Ġmostrar": 21487, "ĠTodd": 21488, "ĠNiger": 21489, "icting": 21490, "hard": 21491, "://": 21492, "irable": 21493, "igation": 21494, "ĠMembers": 21495, "ĠìłľíĴĪ": 21496, "Ġdiscour": 21497, "ٽ": 21498, "rika": 21499, "ĠDN": 21500, "ĠFif": 21501, "ĠCapital": 21502, "ÑĢом": 21503, "ĠSans": 21504, "yun": 21505, "Ġpilots": 21506, "Ġtrat": 21507, "Ġnyt": 21508, "Ġ민": 21509, "Ġexponential": 21510, "Ġemerge": 21511, "Ġtrajectory": 21512, "ĠпоÑĩемÑĥ": 21513, "Ġsealed": 21514, "atti": 21515, "Ġwides": 21516, "ĠогÑĢ": 21517, "iances": 21518, "Ġwitnessed": 21519, "Or": 21520, "osi": 21521, "ĠJoel": 21522, "onal": 21523, "èģ½": 21524, "ĠInte": 21525, "cedes": 21526, "ĠGotta": 21527, "anium": 21528, "Ġfemales": 21529, "ĠLebens": 21530, "Ġmoistur": 21531, "ĠSimple": 21532, "ĠDoch": 21533, "ará": 21534, "Ġgesehen": 21535, "UST": 21536, "Æ¡i": 21537, "Ġclassification": 21538, "Ġdiagonal": 21539, "Ġpermett": 21540, "comp": 21541, "ĠاÙĦØŃ": 21542, "ĠMalays": 21543, "Ġgehört": 21544, "Ġpopped": 21545, "Ġcontacted": 21546, "Ġ׼׾": 21547, "Ġ140": 21548, "Ġadaptation": 21549, "Ġmanus": 21550, "Ġturkey": 21551, "Ġpreach": 21552, "bright": 21553, "Ġdowns": 21554, "Ġunprecedented": 21555, "Ġmighty": 21556, "Ġcater": 21557, "itti": 21558, "gs": 21559, "ĠDeputy": 21560, "write": 21561, "ĠBless": 21562, "ác": 21563, "Ġsummit": 21564, "Ġëı¼ìļĶ": 21565, "Ġthoughtful": 21566, "Ġshred": 21567, "singing": 21568, "ĠлÑĥÑĩÑĪе": 21569, "Ġyen": 21570, "Ġvibrant": 21571, "ĠWalter": 21572, "Ġhosts": 21573, "Ġambul": 21574, "Ġinvasion": 21575, "ogan": 21576, "Ġreasoning": 21577, "Ġsucc": 21578, "лекÑĤ": 21579, "Ġfala": 21580, "Ġkings": 21581, "Ġgoin": 21582, "Ġcalib": 21583, "ĠGRÃľNEN": 21584, "oter": 21585, "Ġeinz": 21586, "Ġinsulin": 21587, "Ĭ¨": 21588, "Ġscaling": 21589, "ĠCorn": 21590, "hyd": 21591, "Ġmatte": 21592, "PL": 21593, "Ġaliens": 21594, "ĠSeg": 21595, "è¯Ŀ": 21596, "esti": 21597, "astics": 21598, "Ġwarmer": 21599, "Ġingen": 21600, "ĠML": 21601, "Ġrode": 21602, "ĠEye": 21603, "beits": 21604, "ĠBarn": 21605, "»,": 21606, "ĠChuck": 21607, "Ġprofitable": 21608, "uguese": 21609, "ĠArabia": 21610, "Ġcoco": 21611, "Ġpuedo": 21612, "Ġinflammation": 21613, "clip": 21614, "Ġtablespoons": 21615, "Ġìłij": 21616, "ĠSwed": 21617, "Ġanat": 21618, "ìĪł": 21619, "Ġarrib": 21620, "Ġdancer": 21621, "ĠCarter": 21622, "Ġmagnific": 21623, "store": 21624, "éģ¸": 21625, "Ġfade": 21626, "Ġaccompany": 21627, "Ġwahr": 21628, "Ġyeast": 21629, "Ġmineral": 21630, "Ġlegislature": 21631, "ä½ı": 21632, "iros": 21633, "Ġcrowded": 21634, "ÑĢаÑĪ": 21635, "ocado": 21636, "ìĸ´ìķ¼": 21637, "ĠíĽĦ": 21638, "ĠBarry": 21639, "master": 21640, "Ġnickname": 21641, "Ġ\"...": 21642, "ĠRs": 21643, "ĠMoore": 21644, "Ġvenue": 21645, "ĠбÑĥ": 21646, "ãĥ¡": 21647, "lihood": 21648, "ĠAgency": 21649, "лов": 21650, "Ġkah": 21651, "ĠìĨĮ리": 21652, "Ġmarsh": 21653, "Ġincorporated": 21654, "antwort": 21655, "Ġkimchi": 21656, "Ġwoo": 21657, "Ġdistracted": 21658, "eries": 21659, "Ġinformación": 21660, "ĠChoose": 21661, "ĠJadi": 21662, "Ġanalogy": 21663, "say": 21664, "uffle": 21665, "bok": 21666, "Ġacids": 21667, "Ġacquisition": 21668, "Ġvariants": 21669, "èµ·ä¾Ĩ": 21670, "Ġpassiert": 21671, "ìĿ´ëĤĺ": 21672, "ructive": 21673, "brig": 21674, "ĠãĢĮ": 21675, "epher": 21676, "ĠpH": 21677, "utlich": 21678, "å·®": 21679, "Ġrelie": 21680, "uite": 21681, "Ġreception": 21682, "Ġcoh": 21683, "ĠPrep": 21684, "Ġanticipate": 21685, "æĢ§": 21686, "kee": 21687, "Ġdesignated": 21688, "ÑıÑĤи": 21689, "ĠKor": 21690, "ĠAnim": 21691, "ühl": 21692, "ĠWhit": 21693, "Ġuncover": 21694, "ĠMaya": 21695, "ĠÑĤогда": 21696, "°ķ": 21697, "utenant": 21698, "Ġìĸ¼ë": 21699, "Ġforests": 21700, "Ġmeme": 21701, "Ġdistinguished": 21702, "ĠMarx": 21703, "ĠLion": 21704, "Ġservants": 21705, "ĠDiam": 21706, "çķ¶çĦ¶": 21707, "ĠPolicy": 21708, "į¼": 21709, "Ġtriggered": 21710, "abilir": 21711, "ĠìĿij": 21712, "Ġnegotiate": 21713, "Ġfez": 21714, "Ġerw": 21715, "Ġvaries": 21716, "Ġjemand": 21717, "Ġdischarge": 21718, "ÑģÑıÑĩ": 21719, "ĠPAR": 21720, "ĠAffairs": 21721, "Ġvoter": 21722, "Ġaten": 21723, "Ġcrois": 21724, "obil": 21725, "ĠOops": 21726, "ĠArc": 21727, "ĠHeather": 21728, "anka": 21729, "Ġsimples": 21730, "ον": 21731, "\">": 21732, "Ġchords": 21733, "ĠSanders": 21734, "Ġë¶Ħë": 21735, "Ben": 21736, "Ġdarüber": 21737, "ilians": 21738, "Ġordering": 21739, "ĠManh": 21740, "Ġkilogram": 21741, "ĠkarÅŁ": 21742, "Ġgrasp": 21743, "Ġghosts": 21744, "alen": 21745, "ĠJedi": 21746, "Ġбли": 21747, "Ġdownloaded": 21748, "Ġconducting": 21749, "ĠHak": 21750, "Ġresearcher": 21751, "ilan": 21752, "good": 21753, "ĠHannah": 21754, "ĠdÃ¼ÅŁÃ¼n": 21755, "ĠMessiah": 21756, "uity": 21757, "iona": 21758, "Ġprobable": 21759, "ĠYE": 21760, "Ġindependently": 21761, "Ġbuffer": 21762, "burn": 21763, "ourd": 21764, "ĠMcK": 21765, "Ġlingu": 21766, "ujemy": 21767, "еÑĢÑĤ": 21768, "Ġintuitive": 21769, "Ġcracks": 21770, "appropri": 21771, "nty": 21772, "Ġgeen": 21773, "Ġlend": 21774, "Ġcertification": 21775, "IDS": 21776, "unter": 21777, "pees": 21778, "Ġtrump": 21779, "Ġbankrupt": 21780, "Ġfeas": 21781, "èĹ": 21782, "Ġduż": 21783, "æ¸ħ": 21784, "Ġviruses": 21785, "Ġ58": 21786, "god": 21787, "Ġжел": 21788, "Ġstalk": 21789, "Ind": 21790, "achi": 21791, "ĠCF": 21792, "ĠCond": 21793, "Ġsanct": 21794, "Ġconten": 21795, "Ġfreed": 21796, "ĠRT": 21797, "Ġmentors": 21798, "족": 21799, "Ġportable": 21800, "ĠPaulo": 21801, "rane": 21802, "HAHA": 21803, "ĠSection": 21804, "çĨ": 21805, "hyun": 21806, "ĠÎŃÏĩ": 21807, "ĠPub": 21808, "ĠIndepend": 21809, "Ġcompounds": 21810, "ĠÑģÑĭ": 21811, "Ġmessaging": 21812, "Ġdedication": 21813, "Ġnoticing": 21814, "Ġdevoted": 21815, "ÑİÑĤÑģÑı": 21816, "Ġsnakes": 21817, "Ġbattlefield": 21818, "pers": 21819, "Ġdela": 21820, "92": 21821, "Ġhai": 21822, "illä": 21823, "érer": 21824, "every": 21825, "Ġresponsive": 21826, "×Ļ×ķ": 21827, "opf": 21828, "éī": 21829, "Ĭ¸": 21830, "Because": 21831, "Ġtourism": 21832, "Ġê·¸ê²Į": 21833, "×ķצ": 21834, "Ġcans": 21835, "stüt": 21836, "Ġdonne": 21837, "ĠDios": 21838, "ĠUber": 21839, "actory": 21840, "Ġoriented": 21841, "ĠHerm": 21842, "Ġpatron": 21843, "urf": 21844, "bei": 21845, "Ġprograma": 21846, "ĠOhh": 21847, "gener": 21848, "Ġfist": 21849, "ĠWendy": 21850, "Ġanda": 21851, "Ġguessed": 21852, "Ġfreak": 21853, "ä¸Ńåľĭ": 21854, "ĠKings": 21855, "chool": 21856, "Ġoffline": 21857, "ĠIndiana": 21858, "ĠAlliance": 21859, "Ġ53": 21860, "Ġparticul": 21861, "ĠFocus": 21862, "Ġinhabit": 21863, "Ġê°ĻìĿĢëį°": 21864, "ĠMcG": 21865, "owski": 21866, "ĠìĿ´ê±´": 21867, "ĠpaÅĦst": 21868, "они": 21869, "itta": 21870, "Ġconfirmation": 21871, "ĠBrooklyn": 21872, "Ġnoodle": 21873, "fund": 21874, "itud": 21875, "Ġgrandparents": 21876, "Ġbarbecue": 21877, "ειÏĤ": 21878, "Ġá": 21879, "Ġballot": 21880, "ĠVeter": 21881, "Ġpipes": 21882, "igious": 21883, "ĠGraph": 21884, "ested": 21885, "Ġë¸Įë": 21886, "ĠKE": 21887, "ãģ¡ãĤĩãģ£ãģ¨": 21888, "Ġeins": 21889, "Ġhatred": 21890, "ãģijãģ©": 21891, "Ġdang": 21892, "eeee": 21893, "Ġarchae": 21894, "ĠJesse": 21895, "Ġdetected": 21896, "Ġseni": 21897, "burgh": 21898, "Ġdisplacement": 21899, "Ġdop": 21900, "Ġconditioning": 21901, "ĠнеÑģколÑĮко": 21902, "Ġdisturbing": 21903, "PH": 21904, "Ġthinner": 21905, "Ġwounded": 21906, "ĠCuando": 21907, "Ġcushion": 21908, "Ġwhites": 21909, "Ġpreferences": 21910, "Ġì¤Ģë¹Ħ": 21911, "Ġkaż": 21912, "ĠGate": 21913, "ĠPath": 21914, "dles": 21915, "à¸Ħร": 21916, "imore": 21917, "Ġë³´ìŬ": 21918, "Ġdisciplines": 21919, "á»ı": 21920, "Ġmesma": 21921, "ĠìĥĪë": 21922, "Ġìĭ¬": 21923, "Ġging": 21924, "Ġumbrella": 21925, "IGHT": 21926, "Ġpension": 21927, "Ġcombining": 21928, "SS": 21929, "Ġrectangle": 21930, "á»ĩt": 21931, "Ġproxim": 21932, "ĠCow": 21933, "¸Į": 21934, "Ġintentional": 21935, "æķĻ": 21936, "Ġdecid": 21937, "ĠÑģкаж": 21938, "ĠUma": 21939, "iasm": 21940, "buz": 21941, "Ġdebris": 21942, "Ġcass": 21943, "ĠProp": 21944, "iska": 21945, "ëł¥": 21946, "esterol": 21947, "ussian": 21948, "ìĿ´ëŀij": 21949, "Ġunlimited": 21950, "Ġadmire": 21951, "Ġtightly": 21952, "Ġgenome": 21953, "ĠJunior": 21954, "venir": 21955, "gus": 21956, "ĠcÄĥ": 21957, "ĠVlad": 21958, "ĠíĤ": 21959, "Ġrelativ": 21960, "inci": 21961, "Ġaunque": 21962, "ĠBoys": 21963, "ÑĨион": 21964, "ĠSwiss": 21965, "Ġphysicians": 21966, "Ġíıī": 21967, "ĠPET": 21968, "Ġwounds": 21969, "about": 21970, "Ãłi": 21971, "onz": 21972, "urities": 21973, "ĠÑĥвид": 21974, "å·¦": 21975, "Ġmentality": 21976, "Ġvariance": 21977, "Ġsegunda": 21978, "Ġvolcano": 21979, "alie": 21980, "à¥ĩ": 21981, "Ġtiles": 21982, "ĠTerry": 21983, "ĠاÙĦÙĦÙĩ": 21984, "Ġcanon": 21985, "Ġscattered": 21986, "pton": 21987, "Ġdefinitions": 21988, "Ġalgebra": 21989, "oten": 21990, "ablo": 21991, "ijuana": 21992, "Ġwrapping": 21993, "Ġsesame": 21994, "ĠнаÑĩина": 21995, "ĠAlf": 21996, "ĠÐłÐ¾ÑģÑģ": 21997, "orno": 21998, "Ġankle": 21999, "Ġspecialty": 22000, "Ġattempting": 22001, "iliation": 22002, "Ġ1920": 22003, "Ġphenomena": 22004, "ĠProduct": 22005, "ĠBuck": 22006, "ĠAww": 22007, "seen": 22008, "Ġvoid": 22009, "ĠFranklin": 22010, "Ġadvocacy": 22011, "ĠSep": 22012, "Ġcoolest": 22013, "ĠÑģÑĢазÑĥ": 22014, "ĠQuand": 22015, "Ġ900": 22016, "ĠTrad": 22017, "dies": 22018, "Ġhash": 22019, "æĪijå°±": 22020, "ä¹Łæĺ¯": 22021, "Ġpots": 22022, "Ġsadly": 22023, "Ġviable": 22024, "ĠTiger": 22025, "ĠONE": 22026, "Ġneurons": 22027, "owanie": 22028, "ÄĹ": 22029, "ĠShar": 22030, "ĠLandes": 22031, "Ġconferences": 22032, "該": 22033, "Ġcredential": 22034, "Ġlime": 22035, "inee": 22036, "xit": 22037, "pay": 22038, "Ġincons": 22039, "Ġ>>:": 22040, "èªį": 22041, "Ġíŀĺë": 22042, "Ġlesser": 22043, "Ġspill": 22044, "Ġpremise": 22045, "Ġ365": 22046, "ĠHost": 22047, "Ġtomar": 22048, "×IJ׾": 22049, "ë²Ī": 22050, "ĠWhats": 22051, "Ġlightweight": 22052, "ĠMap": 22053, "fia": 22054, "ellschaft": 22055, "Ġvendors": 22056, "uesto": 22057, "ĠMister": 22058, "ĠÐŁÑĢи": 22059, "åı³": 22060, "hma": 22061, "Ġintentionally": 22062, "ĠTang": 22063, "éĹ®": 22064, "Ġidentification": 22065, "Ġetcetera": 22066, "ĠNee": 22067, "ĠÑĤÑĢи": 22068, "ê·¸": 22069, "Ġcryptocur": 22070, "Ġinhale": 22071, "Ġaddict": 22072, "åIJĦä½į": 22073, "Ġmau": 22074, "ĠÑĤакаÑı": 22075, "Ġë²Ħ": 22076, "Ġcomprar": 22077, "iedzieÄĩ": 22078, "ĠоÑĤно": 22079, "Ġbeginner": 22080, "ĠмÑĥж": 22081, "Ġobsc": 22082, "Ġlimiting": 22083, "ascular": 22084, "Ġinspection": 22085, "aci": 22086, "Ġrejo": 22087, "Mus": 22088, "Ġzaten": 22089, "Ġszcz": 22090, "ĠMadrid": 22091, "Ġvarieties": 22092, "ĠestÃł": 22093, "ĠShakes": 22094, "Ġkits": 22095, "Ġadminister": 22096, "Ġlava": 22097, "ĠgÃ¥": 22098, "試": 22099, "ת×Ļ": 22100, "ĠWayne": 22101, "Ġinstagram": 22102, "Ġrated": 22103, "paper": 22104, "Ġbild": 22105, "Ġpretending": 22106, "Ġobserving": 22107, "ĠÑģамом": 22108, "Ġtror": 22109, "Ġorganisms": 22110, "Ġfalta": 22111, "Ġhometown": 22112, "ç±": 22113, "Ġíĭ": 22114, "Ġcheg": 22115, "Ġì¡": 22116, "Ġcomma": 22117, "isé": 22118, "Ġlikelihood": 22119, "avored": 22120, "Ġgeldi": 22121, "ников": 22122, "Ġmedio": 22123, "Ġjakie": 22124, "ĠJup": 22125, "Ġgreenhouse": 22126, "Ġspit": 22127, "кое": 22128, "Ġкаж": 22129, "ĠGram": 22130, "ĠConference": 22131, "Ġdeficit": 22132, "sın": 22133, "inse": 22134, "uÄŁ": 22135, "Ġricht": 22136, "Ġcoincidence": 22137, "åıį": 22138, "Ġeurop": 22139, "Ġbutterfly": 22140, "pread": 22141, "Ġìĸ¼": 22142, "è̶": 22143, "Ġwavel": 22144, "ĠInfin": 22145, "ĠPlanet": 22146, "Ġselfie": 22147, "ientras": 22148, "Ġarrog": 22149, "oser": 22150, "idal": 22151, "ł×Ĺ׳×ķ": 22152, "ütün": 22153, "Ġfreshman": 22154, "ĠMachine": 22155, "ÏĥÏĦ": 22156, "ĠDia": 22157, "ìĿ´ëĭ¤": 22158, "ãģĵãģĨ": 22159, "nea": 22160, "Ġlisting": 22161, "Ġconfigure": 22162, "utor": 22163, "Up": 22164, "tschaft": 22165, "rière": 22166, "Ġupwards": 22167, "ĠÑħоÑĩÑĥ": 22168, "Ġsweep": 22169, "Br": 22170, "Ġexpressing": 22171, "Ġunhappy": 22172, "Ġmandatory": 22173, "gender": 22174, "ĠAÃŃ": 22175, "Ġindicators": 22176, "Ġoils": 22177, "note": 22178, "Ġsegur": 22179, "ожеÑĤ": 22180, "ynasty": 22181, "Ġdistances": 22182, "Ġmerge": 22183, "BERT": 22184, "Ġsurrender": 22185, "Ġbuat": 22186, "ĠAwards": 22187, "Ġseñor": 22188, "odox": 22189, "Ġflavour": 22190, "Ġabdom": 22191, "Ġconfigur": 22192, "86": 22193, "ĠDIY": 22194, "Ġrigid": 22195, "°ĺ": 22196, "Ġcorporation": 22197, "Ġgroom": 22198, "jaw": 22199, "ĠNear": 22200, "ило": 22201, "Ġopera": 22202, "ĠInnov": 22203, "иÑĢа": 22204, "ĵ±": 22205, "Ġspecified": 22206, "Ġcosm": 22207, "ĠFreedom": 22208, "Ġclown": 22209, "ĠNem": 22210, "Ġвол": 22211, "Ñijн": 22212, "Ġcharger": 22213, "à¹ģล": 22214, "Ġinfluential": 22215, "äsident": 22216, "é¤": 22217, "ĠìĦłë": 22218, "Ġvolumes": 22219, "æIJ": 22220, "Ġoutras": 22221, "ĠTwitch": 22222, "Ġfounding": 22223, "Ġawhile": 22224, "Ġcoil": 22225, "ê°Ļ": 22226, "Ġcả": 22227, "ĠThrow": 22228, "ĠHence": 22229, "ommt": 22230, "ĠBenjamin": 22231, "глÑıд": 22232, "Time": 22233, "obic": 22234, "Ġmour": 22235, "Ġdread": 22236, "ĠLÃł": 22237, "ĠChile": 22238, "Ġpreval": 22239, "Ġvain": 22240, "Ġartık": 22241, "Ġpreserved": 22242, "ĠоÑĤд": 22243, "Ġwarehouse": 22244, "Ġbeste": 22245, "ĠSeveral": 22246, "ĠSituation": 22247, "Ġcardboard": 22248, "Tod": 22249, "erna": 22250, "Ġgarant": 22251, "Ġgesture": 22252, "Ġhen": 22253, "Ġspelling": 22254, "osexual": 22255, "Ġanne": 22256, "Ġmice": 22257, "ĠMeine": 22258, "card": 22259, "Ġrebell": 22260, "Ġcerto": 22261, "Ġìľłë": 22262, "Ġverschied": 22263, "ĠBos": 22264, "Ġinvention": 22265, "Ġtrze": 22266, "Ġmanière": 22267, "ĠChad": 22268, "Ġspre": 22269, "Ġorganisations": 22270, "Ġpoorly": 22271, "Ġanterior": 22272, "Ġstair": 22273, "кÑĢ": 22274, "Ġatomic": 22275, "Ġsympath": 22276, "Ġcontinually": 22277, "Ġkleine": 22278, "ète": 22279, "иÑī": 22280, "οÏĤ": 22281, "peut": 22282, "Ġreposit": 22283, "Ġentra": 22284, "Em": 22285, "Ġfinancing": 22286, "Ġмног": 22287, "Ġthesis": 22288, "ĠComputer": 22289, "eau": 22290, "ĠTree": 22291, "Ġbride": 22292, "onsieur": 22293, "shire": 22294, "wic": 22295, "DE": 22296, "ĠìĪĺë": 22297, "Ġacom": 22298, "ĠPO": 22299, "ersch": 22300, "ĠпомоÑī": 22301, "ĠArmen": 22302, "Ġ죽": 22303, "Ġzor": 22304, "Ġprints": 22305, "ĠDass": 22306, "港": 22307, "Ġdurable": 22308, "ĠTransport": 22309, "ìŀIJê°Ģ": 22310, "Ġлег": 22311, "Ġdét": 22312, "ôle": 22313, "amous": 22314, "YN": 22315, "Ġcliff": 22316, "Ġgrammar": 22317, "ĠÐŁÐ¾ÑįÑĤомÑĥ": 22318, "ĠlÃłm": 22319, "esch": 22320, "Ġmiserable": 22321, "Ġvolts": 22322, "ĠCad": 22323, "ukan": 22324, "ÑĤив": 22325, "rust": 22326, "Ġìĺ¬ëĿ¼": 22327, "Ġverk": 22328, "Ġchickens": 22329, "ĠYoo": 22330, "Ġoutfits": 22331, "code": 22332, "Ġhierarchy": 22333, "netes": 22334, "Ġcounterpart": 22335, "Ġtôi": 22336, "Ġted": 22337, "ĠBart": 22338, "ĠëĿ¼": 22339, "ĠGenau": 22340, "Ġincoming": 22341, "ĠABC": 22342, "rique": 22343, "ĠоÑĤп": 22344, "qual": 22345, "Ġincentive": 22346, "Ġihren": 22347, "׳×Ļ": 22348, "loe": 22349, "Ġ1930": 22350, "Ġbarg": 22351, "Ġdiction": 22352, "Ġönce": 22353, "INS": 22354, "Ġreh": 22355, "isiaj": 22356, "mouth": 22357, "Ġscoring": 22358, "lık": 22359, "ĠìķĦ주": 22360, "ORIA": 22361, "ĠEstados": 22362, "Ġcompanion": 22363, "Ġassemble": 22364, "Ġpunished": 22365, "Ġital": 22366, "Ġprevents": 22367, "istes": 22368, "ĠKentucky": 22369, "Ġlocate": 22370, "Ġfasting": 22371, "ã썿ĢĿ": 22372, "ĥĢ": 22373, "ĠSeb": 22374, "ĠCrown": 22375, "opia": 22376, "Ġwhip": 22377, "usz": 22378, "ками": 22379, "Ġdatabases": 22380, "åŃĹ": 22381, "Ġprosec": 22382, "Ġ1997": 22383, "ĠìĤ´ì§Ŀ": 22384, "ĠSolar": 22385, "ĠPues": 22386, "ĠZen": 22387, "ollo": 22388, "ĠGuru": 22389, "Ġsqueez": 22390, "ĠÐĹа": 22391, "ĠÄį": 22392, "ceptions": 22393, "cca": 22394, "izable": 22395, "mand": 22396, "Ġbreakthrough": 22397, "Ġtablespoon": 22398, "ĠSEC": 22399, "ikh": 22400, "ĠSão": 22401, "Ġпло": 22402, "amen": 22403, "Ġprac": 22404, "Ġdarling": 22405, "Ġtaller": 22406, "Ġrendering": 22407, "Ġìļ°ë¦¬ê°Ģ": 22408, "ĠÏĦηÏĤ": 22409, "Ġmã": 22410, "Ġesos": 22411, "uerdo": 22412, "ĠÑģÑĩиÑĤ": 22413, "aller": 22414, "ìĹĪìĸ´ìļĶ": 22415, "Ġmillones": 22416, "lerin": 22417, "Ġpegar": 22418, "onne": 22419, "Ġenrollment": 22420, "Ġliegt": 22421, "Ġboa": 22422, "wiÄĻ": 22423, "bsp": 22424, "Ġcycling": 22425, "ĠBernie": 22426, "Ġ1989": 22427, "ĠдалÑĮ": 22428, "ĠDakota": 22429, "ĠÑģвÑıз": 22430, "ĠCP": 22431, "Ġstare": 22432, "íĤ¤": 22433, "Ġprosperity": 22434, "Ġarrangements": 22435, "Ġarriving": 22436, "mä": 22437, "Ġkayak": 22438, "ipt": 22439, "Ġpardon": 22440, "Ġrelat": 22441, "Ġverste": 22442, "ĠFig": 22443, "Ġfoil": 22444, "ĠTalking": 22445, "peare": 22446, "Ġnoi": 22447, "ĠпÑĢиÑĪ": 22448, "Ġhockey": 22449, "Ġado": 22450, "ĠOUT": 22451, "67": 22452, "Ġhormones": 22453, "ĠAvenue": 22454, "ĠSuperman": 22455, "Ġprescription": 22456, "ubernetes": 22457, "CL": 22458, "otive": 22459, "NIS": 22460, "ienen": 22461, "Ġsadness": 22462, "ĠVit": 22463, "Ty": 22464, "Ġstarter": 22465, "Ġbede": 22466, "Ġfoundations": 22467, "Ġsore": 22468, "åºĹ": 22469, "ÑīеÑģÑĤв": 22470, "ìļ°ë": 22471, "ĠÑĩÑĥв": 22472, "link": 22473, "Ġmaneu": 22474, "working": 22475, "Ãłn": 22476, "ĠAttack": 22477, "ĠCart": 22478, "veis": 22479, "ĠResp": 22480, "ensing": 22481, "Ġì¢ĭìķĦìļĶ": 22482, "Ġescuch": 22483, "ĠRNA": 22484, "Ĥ´": 22485, "Ġadop": 22486, "Ġbending": 22487, "عد": 22488, "Ġmanages": 22489, "usp": 22490, "Ġtart": 22491, "Ġrouter": 22492, "Bo": 22493, "Ġestablishing": 22494, "Ġbalancing": 22495, "Ġathletic": 22496, "ĠSlo": 22497, "Ġfills": 22498, "Ġнаб": 22499, "Ġдал": 22500, "Ġposso": 22501, "ĠVielen": 22502, "Ġcritics": 22503, "Ġlawsuit": 22504, "ĠIsaac": 22505, "ĠÑĦилÑĮм": 22506, "Ġtras": 22507, "Ġpraw": 22508, "ĠCrazy": 22509, "Ġneu": 22510, "Ġkull": 22511, "Ġtumor": 22512, "ĠAPP": 22513, "gate": 22514, "ĠARE": 22515, "98": 22516, "ĠSteam": 22517, "Ġfucked": 22518, "lage": 22519, "ĠâϬ": 22520, "ĠMD": 22521, "fy": 22522, "Ġshells": 22523, "ĠSeems": 22524, "izers": 22525, "Ġranges": 22526, "ĠAntonio": 22527, "ATION": 22528, "ĠBaba": 22529, "Ġìĥī": 22530, "kun": 22531, "Ġprayed": 22532, "ÑĢÑı": 22533, "ĠпÑĢоÑĤив": 22534, "Ġseas": 22535, "bury": 22536, "Ġ×Ķש": 22537, "Ġtrait": 22538, "ĠDepending": 22539, "Ġdre": 22540, "Ġkönnt": 22541, "ÑĨÑĥ": 22542, "Ġlipstick": 22543, "eez": 22544, "ĠпÑĢимеÑĢ": 22545, "Ġassignments": 22546, "Bob": 22547, "Ġmetals": 22548, "Ġspecially": 22549, "å°įä¸įå°į": 22550, "ĠìĺĪë": 22551, "ĠÅ¡": 22552, "Ġvista": 22553, "Ġά": 22554, "Ġtwins": 22555, "Ġnotable": 22556, "ĠSau": 22557, "Ġdévelop": 22558, "Ġçek": 22559, "Ġpolynom": 22560, "avam": 22561, "Ġtambé": 22562, "оном": 22563, "Ġplasma": 22564, "Ġefect": 22565, "Ġläng": 22566, "Ġcasi": 22567, "Ñģа": 22568, "ımı": 22569, "ãģĻãĤĭ": 22570, "ĵ¤ìĿĢ": 22571, "Ġlabour": 22572, "ossen": 22573, "ĠPun": 22574, "rif": 22575, "Ġdoses": 22576, "Ġoperates": 22577, "илли": 22578, "Ġjaar": 22579, "staw": 22580, "ĠìĤ¬ëŀij": 22581, "Ġatm": 22582, "Ġprotects": 22583, "Ġimped": 22584, "HO": 22585, "Ġcima": 22586, "Ġtoch": 22587, "abis": 22588, "Ġsendo": 22589, "laus": 22590, "Ġcurl": 22591, "ĠNum": 22592, "Ġsponsors": 22593, "Ġdébut": 22594, "ĠAlexa": 22595, "ĠBür": 22596, "ĠAmer": 22597, "Ġcope": 22598, "Ġизв": 22599, "jal": 22600, "Ġ1995": 22601, "apat": 22602, "resse": 22603, "ĠPrize": 22604, "ĠClaire": 22605, "ĠBrandon": 22606, "Ġwszystko": 22607, "Ġvalued": 22608, "à¸Ļะ": 22609, "Ġsect": 22610, "Ġsecretly": 22611, "Ġdiamonds": 22612, "ĠEvan": 22613, "ĠRPG": 22614, "ãģ«ãģª": 22615, "ĪëıĦ": 22616, "ĠUniversal": 22617, "Ġdoubts": 22618, "ĠPin": 22619, "wiÄħz": 22620, "ļ©": 22621, "Ġalbo": 22622, "Ġbraucht": 22623, "AUL": 22624, "ĠMobile": 22625, "grades": 22626, "Ġschem": 22627, "why": 22628, "ĠNicht": 22629, "pi": 22630, "gle": 22631, "Ġchorus": 22632, "Ġgly": 22633, "Ġreinforce": 22634, "Ġmuff": 22635, "ĠShen": 22636, "ĠHola": 22637, "Ñĥг": 22638, "videmment": 22639, "vial": 22640, "acious": 22641, "laimed": 22642, "ĠRico": 22643, "Ġvegg": 22644, "Ġillustration": 22645, "ĠButter": 22646, "owad": 22647, "Ġeux": 22648, "Ġenfants": 22649, "ĠLeader": 22650, "ĠVillage": 22651, "etically": 22652, "ÙĨÙĬ": 22653, "Ġstew": 22654, "Ġsurprises": 22655, "Ġcue": 22656, "ĠGrandma": 22657, "ĠCelsius": 22658, "ĠRicht": 22659, "enc": 22660, "Ġpetition": 22661, "Ġherb": 22662, "Ġwicked": 22663, "Ġschle": 22664, "ocaly": 22665, "Ġtransf": 22666, "Ġtokens": 22667, "ĠGray": 22668, "ĠBBC": 22669, "IK": 22670, "Ġ1500": 22671, "zn": 22672, "ĠNev": 22673, "Ġkoy": 22674, "Ġzar": 22675, "Ġbullshit": 22676, "ĠColombia": 22677, "ulative": 22678, "Ġwidespread": 22679, "yect": 22680, "kit": 22681, "Ġempresa": 22682, "Ġnour": 22683, "Ġburns": 22684, "atin": 22685, "aired": 22686, "Ġrevolutionary": 22687, "ĠгодÑĥ": 22688, "ĠLogan": 22689, "Ġ1996": 22690, "ĠGraham": 22691, "reb": 22692, "ĠNHS": 22693, "æľĽ": 22694, "Ġcostumes": 22695, "Ġnawet": 22696, "Ġlovers": 22697, "ĠLucy": 22698, "ĠIndigenous": 22699, "íķĺ기": 22700, "Ġimmunity": 22701, "¥´ë": 22702, "uito": 22703, "Ġexcessive": 22704, "Ġdonations": 22705, "Ġ×Ķר": 22706, "Ġ첫": 22707, "éīĦ": 22708, "Ġdrying": 22709, "melon": 22710, "Ġsurveys": 22711, "Ġ무ìĬ¨": 22712, "風": 22713, "aaa": 22714, "Ġprobe": 22715, "ancial": 22716, "Ġlouder": 22717, "Ġhotels": 22718, "Ã¼ÄŁ": 22719, "agner": 22720, "Ġorigins": 22721, "Ġë§Īì§Ģë§ī": 22722, "Ġ**": 22723, "Ġstrangers": 22724, "ĠHaus": 22725, "comed": 22726, "Ġanthrop": 22727, "Ġuso": 22728, "ĠìķĦì§ģ": 22729, "ĠYuan": 22730, "ĠíķĦìļĶ": 22731, "pler": 22732, "ressive": 22733, "Ġspraw": 22734, "ĠStew": 22735, "Ġ1994": 22736, "Ġelders": 22737, "Ġmeinen": 22738, "Ġjunt": 22739, "Ġacoust": 22740, "ĠWohn": 22741, "Ġbananas": 22742, "Ġprojection": 22743, "ĠStick": 22744, "legt": 22745, "speed": 22746, "ĠcÅ©ng": 22747, "ĠWort": 22748, "ĠBaltimore": 22749, "ĠÑĨел": 22750, "Ġdunno": 22751, "å¼·": 22752, "?,": 22753, "ãĥīãĥ³": 22754, "ĠLocal": 22755, "osto": 22756, "ÐŃ": 22757, "ода": 22758, "ĠPortuguese": 22759, "Ġtheirs": 22760, "Ġdém": 22761, "åı¦": 22762, "Ġdrauf": 22763, "ĠBuddhist": 22764, "erta": 22765, "Ge": 22766, "Ġcarrot": 22767, "ĠWonderful": 22768, "Ġsoak": 22769, "Ġchairman": 22770, "ggi": 22771, "ICA": 22772, "fried": 22773, "Ġflick": 22774, "ĠThroughout": 22775, "Ġìļ°ë": 22776, "Ġcough": 22777, "Ġfluffy": 22778, "school": 22779, "Ġripped": 22780, "--------": 22781, "ĠZukunft": 22782, "Ġнеб": 22783, "Ġsto": 22784, "ĠBO": 22785, "pent": 22786, "ĠLawrence": 22787, "ÏīÏĤ": 22788, "sticks": 22789, "ĠEins": 22790, "ĠÑĢÑĭ": 22791, "ĠStrong": 22792, "Ġcaramel": 22793, "Ġspite": 22794, "azar": 22795, "éĥ½æĺ¯": 22796, "Ġcritically": 22797, "Ġobra": 22798, "owitz": 22799, "ĠZone": 22800, "ĠÑĢек": 22801, "Ġsug": 22802, "arded": 22803, "Ġgì": 22804, "ffentlich": 22805, "anche": 22806, "ØŁ": 22807, "astically": 22808, "ìĿ¼ë": 22809, "лав": 22810, "Ġsimplest": 22811, "ĠFriend": 22812, "Ġquello": 22813, "Ġambition": 22814, "Ġabbiamo": 22815, "åºķ": 22816, "ĠÑĦоÑĢм": 22817, "ĠEssa": 22818, "Ġeducators": 22819, "Ġstatistical": 22820, "éĢĻéĤĬ": 22821, "Ġchanger": 22822, "Ġatau": 22823, "étais": 22824, "ĠShakespeare": 22825, "ëIJĺ": 22826, "Ġtriggers": 22827, "Ġrealiz": 22828, "Ġcelui": 22829, "wheel": 22830, "Ġloyalty": 22831, "Ġscreams": 22832, "kehr": 22833, "ĠMega": 22834, "east": 22835, "Ġtops": 22836, "ĠTotally": 22837, "ountain": 22838, "lord": 22839, "Ġviolation": 22840, "ĠGA": 22841, "Ġnicer": 22842, "ĠFresh": 22843, "ĠMelissa": 22844, "function": 22845, "Ġrape": 22846, "Ġexceptions": 22847, "Ġsilicon": 22848, "Ġliberty": 22849, "Ġhouseholds": 22850, "ãģįãģ¾ãģĻ": 22851, "ĠCA": 22852, "ĠÐŀб": 22853, "Ġlib": 22854, "ŀĮ": 22855, "cific": 22856, "Ġtropical": 22857, "Ġinvestigating": 22858, "HD": 22859, "Ġadapter": 22860, "ĠPitt": 22861, "ancia": 22862, "ĠShell": 22863, "friendly": 22864, "Ġconclusions": 22865, "Ġturtle": 22866, "Ġdecomp": 22867, "Ġanimations": 22868, "ĠÑģек": 22869, "insi": 22870, "Ġretention": 22871, "kie": 22872, "Ġinjection": 22873, "ĠMadison": 22874, "ì°°": 22875, "Ġvient": 22876, "Ġvaried": 22877, "Ġviolin": 22878, "ĠBil": 22879, "Ġluckily": 22880, "Ġhtt": 22881, "lä": 22882, "Ġranch": 22883, "çľĭçľĭ": 22884, "Ġsólo": 22885, "ìķħ": 22886, "ĠDerek": 22887, "ĠScripture": 22888, "оÑĢа": 22889, "Ġclassrooms": 22890, "avil": 22891, "formed": 22892, "Ġbeforehand": 22893, "ĠGem": 22894, "prech": 22895, "Ġlin": 22896, "Ġgreens": 22897, "ÑĨев": 22898, "ĠMercedes": 22899, "Ġdrought": 22900, "gasps": 22901, "Ġabortion": 22902, "Ġterribly": 22903, "Ġsposób": 22904, "Ġsecured": 22905, "Ġatrás": 22906, "Ġwavelength": 22907, "Ġgrains": 22908, "ective": 22909, "Ġspacecraft": 22910, "Ġtours": 22911, "Ġprofes": 22912, "Ġsurgeon": 22913, "ĠPie": 22914, "Ġideally": 22915, "arner": 22916, "UP": 22917, "opard": 22918, "sce": 22919, "Ġimmense": 22920, "ĠOrt": 22921, "roller": 22922, "ĠDallas": 22923, "ĠNicholas": 22924, "Ġsulf": 22925, "ĠToyota": 22926, "Ġquantities": 22927, "ceans": 22928, "Ġcui": 22929, "ança": 22930, "ĠCAN": 22931, "itzerland": 22932, "åĦ¿": 22933, "Ġzou": 22934, "ĠCyber": 22935, "legen": 22936, "ĠInit": 22937, "edu": 22938, "Ġapert": 22939, "Ġadjac": 22940, "ouv": 22941, "èĢĮä¸Ķ": 22942, "rs": 22943, "Ġcabbage": 22944, "Ġwheelchair": 22945, "inyl": 22946, "ĠDynam": 22947, "ĠìķĦëĭĪëĿ¼": 22948, "Ġling": 22949, "hl": 22950, "ĠмогÑĥ": 22951, "Ġcrisp": 22952, "Ġmij": 22953, "Ġdug": 22954, "nin": 22955, "Ġbloss": 22956, "Ġbelonging": 22957, "Ġloudly": 22958, "Ġminerals": 22959, "Ġconcluded": 22960, "Ġsearched": 22961, "96": 22962, "ĠMeet": 22963, "ĠSEO": 22964, "ĠСк": 22965, "ĠHob": 22966, "otta": 22967, "Ġpropaganda": 22968, "Ġcinnamon": 22969, "Ġhunter": 22970, "Ġgemeins": 22971, "Ġsculpture": 22972, "ulsion": 22973, "Ġväl": 22974, "Ġmagazines": 22975, "Ġcontroversy": 22976, "ä¸Ģ樣": 22977, "Ġsequences": 22978, "ãģĦãĤĭ": 22979, "ĠíļĮ": 22980, "Ġdeleted": 22981, "使": 22982, "IJëıĦ": 22983, "Ġvarying": 22984, "ãĥĨ": 22985, "Ġmounting": 22986, "Ġaffair": 22987, "Ġpathways": 22988, "æ¦": 22989, "Ġdigo": 22990, "亮": 22991, "Ġдок": 22992, "Alex": 22993, "Ġtobacco": 22994, "ĠCV": 22995, "Ġbothered": 22996, "Ġambient": 22997, "inky": 22998, "ĠSL": 22999, "Ġhates": 23000, "Ġjeżeli": 23001, "Ġcongreg": 23002, "Ġelas": 23003, "Ġdeuts": 23004, "ĠStudios": 23005, "chÄĻ": 23006, "Ġdocumented": 23007, "ĠCruz": 23008, "ĠLen": 23009, "ĠDouglas": 23010, "ĠPortugal": 23011, "enti": 23012, "Ġspouse": 23013, "Ġanalys": 23014, "avia": 23015, "Ġedited": 23016, "Ġlại": 23017, "built": 23018, "Ġville": 23019, "adora": 23020, "Ġbracelet": 23021, "Ġsushi": 23022, "Ġpm": 23023, "Ġtrails": 23024, "Ġlug": 23025, "Ġöver": 23026, "Ġsorrow": 23027, "Ġcolony": 23028, "adox": 23029, "Ġserie": 23030, "anyak": 23031, "ĠØ·": 23032, "ĠGulf": 23033, "æĺ¯ä¸įæĺ¯": 23034, "ĠPV": 23035, "ĠSamuel": 23036, "ĠKit": 23037, "ĠRal": 23038, "ontin": 23039, "expl": 23040, "Ġentries": 23041, "Ġactivists": 23042, "Ps": 23043, "Ġsant": 23044, "ĠÑĤоÑĩ": 23045, "ĠBruno": 23046, "keley": 23047, "Ġtutto": 23048, "éĶ": 23049, "Ġvintage": 23050, "Ġterrified": 23051, "ĠпоÑħ": 23052, "usive": 23053, "owers": 23054, "айÑĤ": 23055, "ëıĻ": 23056, "Ġtwisted": 23057, "ĠThought": 23058, "Ġtah": 23059, "Ġshrink": 23060, "Ġsheer": 23061, "lit": 23062, "Ġdalam": 23063, "Ġdib": 23064, "Ġvard": 23065, "owane": 23066, "Ġdobr": 23067, "ĠRena": 23068, "ĠÑģвоÑİ": 23069, "ĠpaÃŃses": 23070, "ĠEra": 23071, "ãģ®ãģ§": 23072, "ĠBUT": 23073, "sighs": 23074, "Ġ그거": 23075, "ĠgroÃŁen": 23076, "Ġ빨리": 23077, "Ġnerves": 23078, "Ġconstit": 23079, "Ġpreocup": 23080, "ĠGay": 23081, "ĠXu": 23082, "keeper": 23083, "heure": 23084, "..)": 23085, "ĠCalm": 23086, "ĠUnidos": 23087, "ĠìĿ´ê²ĥ": 23088, "ĠAqui": 23089, "ĠìłľìĿ¼": 23090, "dır": 23091, "ì¦ĺ": 23092, "your": 23093, "ĠÑįÑĤим": 23094, "2020": 23095, "Ġrund": 23096, "ĠHO": 23097, "ĠCatherine": 23098, "ieli": 23099, "Ġfusion": 23100, "Ġideology": 23101, "Ġforam": 23102, "shaped": 23103, "ĠíĽĦë": 23104, "Ġwt": 23105, "Ġretr": 23106, "Ġpréc": 23107, "Ġê°ij": 23108, "Ġopenly": 23109, "vity": 23110, "구ìļĶ": 23111, "Ġobstacle": 23112, "Ġboo": 23113, "Ġseiner": 23114, "icorn": 23115, "Ġeigenlijk": 23116, "Ġheader": 23117, "aremos": 23118, "Ġsofter": 23119, "ĠÐŁÐ¾Ð´": 23120, "Ġprejud": 23121, "Ġdefines": 23122, "ierte": 23123, "Ġblending": 23124, "Ġbelievers": 23125, "ĠWochen": 23126, "Ġникак": 23127, "ĠÐļогда": 23128, "ĠTypically": 23129, "Ġíģ¬": 23130, "管": 23131, "cios": 23132, "Ġmissiles": 23133, "Ġsponge": 23134, "ĠKitchen": 23135, "Ġtren": 23136, "ningen": 23137, "Ġscrap": 23138, "Ġserait": 23139, "´ìł": 23140, "ç¹": 23141, "Ġë°ĺë": 23142, "Ġrestored": 23143, "ĠprzykÅĤad": 23144, "ĠKubernetes": 23145, "Ġsait": 23146, "Ġuw": 23147, "Ġenabling": 23148, "Ġtravers": 23149, "amps": 23150, "åıĹ": 23151, "ĠOMG": 23152, "ensor": 23153, "Ġzosta": 23154, "Ġpronounced": 23155, "Ang": 23156, "normal": 23157, "Ġeconomies": 23158, "tin": 23159, "ĠChampion": 23160, "izen": 23161, "Ġarbeiten": 23162, "ĠGospel": 23163, "ĠZu": 23164, "nga": 23165, "Ġliteracy": 23166, "ĠMans": 23167, "Ġcirculation": 23168, "Ġadap": 23169, "ĠTotal": 23170, "Ġmereka": 23171, "Ġolacak": 23172, "ÑģÑĤаÑĤи": 23173, "Jack": 23174, "Ġmund": 23175, "Ġthief": 23176, "bies": 23177, "Ġê²ģ": 23178, "aque": 23179, "ĠÚ©ÛĮ": 23180, "ĠScar": 23181, "å²": 23182, "Ġabol": 23183, "Ġdevote": 23184, "Ġ01": 23185, "Ġsitten": 23186, "ĠVisual": 23187, "week": 23188, "some": 23189, "ingt": 23190, "Ġjournalism": 23191, "ĠHir": 23192, "ĠBachelor": 23193, "inery": 23194, "ÃľND": 23195, "ãĥŁ": 23196, "ç»Ļ": 23197, "Ġcoloring": 23198, "ĠCrist": 23199, "Ġcelebrities": 23200, "ĠÑĩиÑģ": 23201, "ĠCrit": 23202, "Ġdifferentiate": 23203, "ĠÐľÐ½Ðµ": 23204, "elim": 23205, "Ġseafood": 23206, "Ġalgumas": 23207, "otherapy": 23208, "æĪ°": 23209, "Ġglaub": 23210, "Ġarbitrary": 23211, "gens": 23212, "ĠбÑĥдем": 23213, "Ġtav": 23214, "Ġcreamy": 23215, "ĠCountry": 23216, "añ": 23217, "меÑĤ": 23218, "Ġhinter": 23219, "Ġmism": 23220, "Ġillustrate": 23221, "ÃľNDNIS": 23222, "Ġdecreasing": 23223, "Ġweniger": 23224, "AKI": 23225, "ixon": 23226, "Ġней": 23227, "Ġfatto": 23228, "Ġnerd": 23229, "çł": 23230, "Ġbitte": 23231, "Per": 23232, "Ġtane": 23233, "Ġgöz": 23234, "Ġforte": 23235, "ĠEy": 23236, "ĠнавеÑĢ": 23237, "被": 23238, "ĠWordPress": 23239, "ĠMis": 23240, "ů": 23241, "zäh": 23242, "Ġintéress": 23243, "osaurs": 23244, "ĠFalls": 23245, "Ġnessa": 23246, "97": 23247, "Ġmuseums": 23248, "Ġcorresponds": 23249, "Ġsings": 23250, "four": 23251, "Ġeder": 23252, "ĠCommunist": 23253, "oa": 23254, "nek": 23255, "ĠWHO": 23256, "Ġcorpo": 23257, "Ġmessing": 23258, "ÏĦαι": 23259, "Ġbrushes": 23260, "Ġbisc": 23261, "ĠArbeits": 23262, "ĠTax": 23263, "Ġsele": 23264, "Ġflags": 23265, "oupe": 23266, "Ġanticipated": 23267, "ãĥij": 23268, "ĠNad": 23269, "Ġpoured": 23270, "Ġml": 23271, "Ġllama": 23272, "Ġvisualize": 23273, "Ġlisteners": 23274, "ÙĦÙĥ": 23275, "alten": 23276, "Michael": 23277, "Ġcosì": 23278, "Õ¡Õ": 23279, "opus": 23280, "Ġíķ´ì£¼": 23281, "Ġhike": 23282, "ĠAttorney": 23283, "ĠHillary": 23284, "uded": 23285, "Ġíķĺì§Ģë§Į": 23286, "Ġdove": 23287, "Ġstorms": 23288, "акÑģ": 23289, "Ġdoctrine": 23290, "Ġhex": 23291, "iks": 23292, "noÅĽÄĩ": 23293, "Ġscripts": 23294, "Ġδεν": 23295, "ĠÑįÑĤиÑħ": 23296, "ĠÐĨ": 23297, "aber": 23298, "ĠVas": 23299, "Ġcentimeters": 23300, "×ŀ×Ķ": 23301, "ниб": 23302, "Ġriders": 23303, "ĠTrib": 23304, "åĮħ": 23305, "Ġtakże": 23306, "Ġnoun": 23307, "Ġicons": 23308, "Ġsolely": 23309, "minded": 23310, "Ġdispon": 23311, "ĠSwitzerland": 23312, "Ġclusters": 23313, "Ġqueda": 23314, "ailing": 23315, "Ġmanga": 23316, "Ġ68": 23317, "ĦĪ": 23318, "Ġtet": 23319, "gins": 23320, "haus": 23321, "空": 23322, "å·¥": 23323, "ĠOP": 23324, "oted": 23325, "Ġnouveau": 23326, "ALLY": 23327, "ÙĪØ¯": 23328, "òn": 23329, "Ġmortality": 23330, "ĠGitHub": 23331, "drop": 23332, "Ġdisgu": 23333, "Ġrecom": 23334, "Ġlocals": 23335, "Ġhomemade": 23336, "amba": 23337, "Ġpronunciation": 23338, "Ġalphabet": 23339, "анÑĮ": 23340, "owany": 23341, "iras": 23342, "idency": 23343, "OME": 23344, "ĠÑĢаÑģÑģ": 23345, "arak": 23346, "viamente": 23347, "Ġnonprofit": 23348, "ĠYouTuber": 23349, "Ġparenth": 23350, "ĠBoo": 23351, "vat": 23352, "ĠStir": 23353, "Ġprecip": 23354, "Ġants": 23355, "Ġally": 23356, "ĠMaori": 23357, "ĠëĮĢíķľ": 23358, "åı¯æĺ¯": 23359, "ogene": 23360, "ĠLabour": 23361, "arette": 23362, "Ġrecycling": 23363, "ensa": 23364, "Ġpursuit": 23365, "Ġsak": 23366, "ĠÐĹдеÑģÑĮ": 23367, "Ġtolerance": 23368, "Ġsaat": 23369, "Ġclicked": 23370, "âĻ¥": 23371, "Ġfacebook": 23372, "ĠInto": 23373, "Ġincentives": 23374, "기ëĬĶ": 23375, "ĠDennis": 23376, "ĠWik": 23377, "gesch": 23378, "à¹Ģà¸Ľ": 23379, "ĠÏĢα": 23380, "ĠWhoo": 23381, "Ġrounded": 23382, "Ġdope": 23383, "Ġcapturing": 23384, "ĠWarri": 23385, "Ġcivilian": 23386, "Ġcharming": 23387, "Ġesas": 23388, "Ġsustained": 23389, "Ġleaning": 23390, "Ġabundance": 23391, "ÃŃlia": 23392, "алÑĮнÑĭй": 23393, "Ġphải": 23394, "acja": 23395, "Ġê°ĻìķĦ": 23396, "activ": 23397, "าย": 23398, "Ġ97": 23399, "Ġмой": 23400, "cro": 23401, "ĠJackie": 23402, "ittees": 23403, "bracht": 23404, "ulent": 23405, "Ġìłľë": 23406, "Ġplugin": 23407, "vantage": 23408, "party": 23409, "Ġsuas": 23410, "Ġante": 23411, "Ñĥл": 23412, "ÐĿÐIJ": 23413, "æĤ¨": 23414, "ĠÏĥÏħ": 23415, "Ġmeth": 23416, "Ġenthusiasm": 23417, "ÑıÑĤÑģÑı": 23418, "íĻĶë": 23419, "Ġsynthetic": 23420, "Ġseasoning": 23421, "ĠLost": 23422, "onomy": 23423, "ĠSpark": 23424, "Ġbure": 23425, "Ġassured": 23426, "Ġimagin": 23427, "Ġcarro": 23428, "Sha": 23429, "Äħt": 23430, "нÑĥÑĤÑĮ": 23431, "ática": 23432, "TY": 23433, "Ġkern": 23434, "ĠBrazilian": 23435, "ð": 23436, "Ġsuspended": 23437, "ĠCarib": 23438, "Ġbizim": 23439, "ĠOliver": 23440, "ãģ¶": 23441, "Tom": 23442, "Ġплан": 23443, "Ġnope": 23444, "omething": 23445, "Ġbeiden": 23446, "ÑĨен": 23447, "Ġfluct": 23448, "ĠμοÏħ": 23449, "Ġfathers": 23450, "ĠBlake": 23451, "Ġupward": 23452, "ĠDash": 23453, "ĠLil": 23454, "ĠìĪĺëıĦ": 23455, "Ġrevelation": 23456, "Ġelevated": 23457, "ĠJiang": 23458, "LED": 23459, "ĠThompson": 23460, "ĠмогÑĥÑĤ": 23461, "ÑģÑĤÑĢÑĥ": 23462, "ifiers": 23463, "Ġcomeback": 23464, "Ġbuyers": 23465, "ê²°": 23466, "ĠSales": 23467, "иÑĩе": 23468, "ciones": 23469, "Ġwhistle": 23470, "Ġdull": 23471, "LEX": 23472, "Ġíķĺê²łìĬµëĭĪëĭ¤": 23473, "Ġcriminals": 23474, "Ġdescent": 23475, "ipple": 23476, "ması": 23477, "Ġfoolish": 23478, "ĠдÑĥмаÑİ": 23479, "tar": 23480, "Ġmango": 23481, "Ġchoreography": 23482, "Matt": 23483, "Ġterritor": 23484, "Ġacaba": 23485, "ĠEinstein": 23486, "ĠIBM": 23487, "ĠMetal": 23488, "ĠCrystal": 23489, "Ġrah": 23490, "Ġfoul": 23491, "ĠIslands": 23492, "Ġintact": 23493, "ĠRail": 23494, ".:": 23495, "Ġacá": 23496, "ĠпÑĢоп": 23497, "еÑĢе": 23498, "ĠWrite": 23499, "hehe": 23500, "ĠFO": 23501, "ĠÏĥÏĦη": 23502, "Ġdoin": 23503, "held": 23504, "Ġappropriately": 23505, "Ġdeliberately": 23506, "Ġarchive": 23507, "Ġgiveaway": 23508, "ãģĵãģĵ": 23509, "Ġfinale": 23510, "лаÑģ": 23511, "ено": 23512, "Æ¡n": 23513, "æ£Ĵ": 23514, "ogo": 23515, "çī©": 23516, "ĠAudience": 23517, "ãħł": 23518, "Ġsubur": 23519, "Ġheadache": 23520, "аннÑı": 23521, "ĠWitch": 23522, "ĠSwedish": 23523, "ĠBI": 23524, "Ġerase": 23525, "Ġkhi": 23526, "Ġcommentary": 23527, "ĠSultan": 23528, "íĥĿ": 23529, "ĠLeban": 23530, "Ġë³´ìĭ": 23531, "ĠPam": 23532, "pekt": 23533, "month": 23534, "Ġgrounded": 23535, "ê¾": 23536, "ĠÅŁekilde": 23537, "250": 23538, "ĠSCH": 23539, "ioso": 23540, "Ġinaug": 23541, "heimer": 23542, "Ġreflecting": 23543, "ĠRuth": 23544, "ĠOil": 23545, "Ġtrouver": 23546, "uep": 23547, "..]": 23548, "ĠìŀĪë": 23549, "Ġolha": 23550, "Ġreasonably": 23551, "Ġglitch": 23552, "UB": 23553, "ĠGran": 23554, "Ġadalah": 23555, "Ġlent": 23556, "را": 23557, "Ġtraction": 23558, "Ġadjusting": 23559, "´¤": 23560, "нибÑĥдÑĮ": 23561, "Ġдоп": 23562, "Ġstretched": 23563, "Ġort": 23564, "Ġcosine": 23565, "viol": 23566, "Ġìħ": 23567, "cir": 23568, "Ġbastard": 23569, "ä¸ĩ": 23570, "ĠÑħод": 23571, "Ġquier": 23572, "Ġpressures": 23573, "ĠAnh": 23574, "å¹¾": 23575, "Ġelles": 23576, "ĠдÑĢÑĥз": 23577, "ĠможеÑĤе": 23578, "Ġchá»": 23579, "ĠMé": 23580, "ök": 23581, "ầu": 23582, "ìłĪ": 23583, "zin": 23584, "Ġcaution": 23585, "iban": 23586, "Ġjudging": 23587, "ÑĥÑİÑĤ": 23588, "Ġbaj": 23589, "ĠСейÑĩаÑģ": 23590, "ĠPoor": 23591, "ĠNazi": 23592, "Ġupbeat": 23593, "yang": 23594, "Ġweekends": 23595, "ĠEssentially": 23596, "Ġoluyor": 23597, "Ġspatial": 23598, "acker": 23599, "Ġseller": 23600, "Ġ×IJ×ķת": 23601, "ij׾": 23602, "Ġvivid": 23603, "ĠBond": 23604, "ê¶Į": 23605, "iskt": 23606, "ãĤµ": 23607, "Ġgoat": 23608, "driver": 23609, "Ġmug": 23610, "ictional": 23611, "Ġallt": 23612, "ĠIniti": 23613, "ĠRand": 23614, "Ġfinishes": 23615, "Ġê°Ī": 23616, "Ġvitam": 23617, "Ġteenagers": 23618, "ĠMorris": 23619, "ì¤Ħ": 23620, "ĠOri": 23621, "iya": 23622, "Ġmyös": 23623, "Step": 23624, "ĠKre": 23625, "辦": 23626, "Ġdinosaur": 23627, "Ġëªĩ": 23628, "affe": 23629, "ĠëIJ©ëĭĪëĭ¤": 23630, "Ġzeg": 23631, "åĪĩ": 23632, "ĠManhattan": 23633, "Ġsujet": 23634, "uelle": 23635, "stoff": 23636, "Ġdür": 23637, "Ġsubmar": 23638, "eses": 23639, "Ġaquele": 23640, "Ġnou": 23641, "ĠFaith": 23642, "tz": 23643, "ĠÑĤомÑĥ": 23644, "aceut": 23645, "liers": 23646, "Ġbandwidth": 23647, "ưá»Ŀ": 23648, "Ġrespective": 23649, "ĠAve": 23650, "Ġspreadshe": 23651, "ĠSent": 23652, "icamente": 23653, "Ġinfra": 23654, "Ġlearners": 23655, "Ġà®ī": 23656, "aiah": 23657, "renal": 23658, "Ġmustard": 23659, "Ġhabt": 23660, "çĥ": 23661, "ĠQué": 23662, "Ġanalyzing": 23663, "æ¯ı": 23664, "Ġsolic": 23665, "Ġ×Ķ×ķ×IJ": 23666, "Ġcausa": 23667, "Ġwelcomed": 23668, "ĠSuccess": 23669, "Ġfacile": 23670, "ĠÐŁÐ¾ÑĤомÑĥ": 23671, "schein": 23672, "Ġfetch": 23673, "Ġstrat": 23674, "ĠÑģÑĤоиÑĤ": 23675, "ìĹIJìĦľëĬĶ": 23676, "ĠÑģпоÑģоб": 23677, "mam": 23678, "ĠserÃŃa": 23679, "naments": 23680, "writer": 23681, "Ġconsulting": 23682, "íĺĢ": 23683, "ĠBerkeley": 23684, "eu": 23685, "asive": 23686, "UU": 23687, "ĠAnalyt": 23688, "Ġsubmission": 23689, "Ġmagnificent": 23690, "enza": 23691, "Ġecon": 23692, "Ġprofiles": 23693, "Ġincar": 23694, "Ab": 23695, "ĠNun": 23696, "Ġhic": 23697, "screaming": 23698, "Ġresilient": 23699, "åĪ©": 23700, "grund": 23701, "Ġconcur": 23702, "Ġbereits": 23703, "LD": 23704, "Ġnurt": 23705, "ìī": 23706, "Ġfeast": 23707, "Ġencuent": 23708, "ĠMichel": 23709, "Ġsuprem": 23710, "\"]": 23711, "Ġfeeds": 23712, "ĠKollegen": 23713, "isser": 23714, "ĠFeng": 23715, "ĠWen": 23716, "mun": 23717, "ĠtenÃŃa": 23718, "ĠWrest": 23719, "Ġìĺ¤ëĬĺìĿĢ": 23720, "Ġstead": 23721, "Ġrestoration": 23722, "Ġdonated": 23723, "Ġdels": 23724, "Ġcensus": 23725, "Ġdesperately": 23726, "worthy": 23727, "HE": 23728, "ĠSpa": 23729, "ĠBryan": 23730, "Ġhj": 23731, "ĠRaw": 23732, "ìķĦë": 23733, "ĠCamera": 23734, "Ġzien": 23735, "Ġstyl": 23736, "ĠTW": 23737, "ĠCheese": 23738, "borne": 23739, "Ġobl": 23740, "ĠAlready": 23741, "Ġunstable": 23742, "Ġflames": 23743, "post": 23744, "Ha": 23745, "romagn": 23746, "ĠìĹĦë§Ī": 23747, "dest": 23748, "Ġkolej": 23749, "Ġtemporarily": 23750, "Ġdetermining": 23751, "ĠGlass": 23752, "ÑĢон": 23753, "olan": 23754, "Ġdominated": 23755, "åĮĸ": 23756, "____": 23757, "ĠÙĩذا": 23758, "ĠDana": 23759, "Ġdinheiro": 23760, "aqu": 23761, "민": 23762, "ĠÃłs": 23763, "ĠJoey": 23764, "ĠGriff": 23765, "Ġattain": 23766, "Ġtransitions": 23767, "ĠLiterally": 23768, "енд": 23769, "ĠHaven": 23770, "Ġgrabbing": 23771, "Ġcrystals": 23772, "ĠFourth": 23773, "Ġcandles": 23774, "ĠÑģлÑĥÑĩа": 23775, "rico": 23776, "Ġ5000": 23777, "etto": 23778, "Ġundo": 23779, "Ġkto": 23780, "Ġdivert": 23781, "Ġchir": 23782, "Ġpersec": 23783, "Ġhiking": 23784, "Ġannouncements": 23785, "çͱ": 23786, "зÑĭ": 23787, "Ġauc": 23788, "Ġsystemic": 23789, "ĠRM": 23790, "Ïĥα": 23791, "ĠÐĶж": 23792, "Ġyar": 23793, "ĠWard": 23794, "Ġpissed": 23795, "Ġcarn": 23796, "Ġautonomous": 23797, "ãħİãħİ": 23798, "sover": 23799, "æ²ĴéĮ¯": 23800, "å¾Ī好": 23801, "Ġreflex": 23802, "Ġgardens": 23803, "Ġdated": 23804, "ì±": 23805, "amiÄĻ": 23806, "Ġcontinuity": 23807, "Ġcitizenship": 23808, "Ġschwer": 23809, "Ġzak": 23810, "table": 23811, "ĠÑģÑĩ": 23812, "è§ģ": 23813, "ĠÏĥε": 23814, "Ġgenerates": 23815, "구ëĤĺ": 23816, "öh": 23817, "óm": 23818, "alam": 23819, "ĠJUDY": 23820, "ĠBug": 23821, "Ġãģ¦": 23822, "Ġdrones": 23823, "Ġágua": 23824, "acaks": 23825, "æļ": 23826, "ĠÐļон": 23827, "×ĸ×Ķ": 23828, "Ġstrive": 23829, "ĠAltern": 23830, "Ġnearest": 23831, "Ġproyect": 23832, "tera": 23833, "ĠASHLEY": 23834, "Ġworm": 23835, "Ġreplay": 23836, "Ġtara": 23837, "ĠIndians": 23838, "ãĤ°": 23839, "icaid": 23840, "ĠìĪľ": 23841, "Ġappealing": 23842, "ĠWes": 23843, "Ġmentions": 23844, "Ġделе": 23845, "Ġkw": 23846, "Ġfragile": 23847, "isz": 23848, "ków": 23849, "hang": 23850, "color": 23851, "Ġpresidente": 23852, "87": 23853, "еÑĦ": 23854, "çΏ": 23855, "Ġдобав": 23856, "ĠNelson": 23857, "áfic": 23858, "ĠMICHAEL": 23859, "Ġmechanic": 23860, "Ġmetres": 23861, "ĠoczywiÅĽcie": 23862, "ĠCind": 23863, "ĠogsÃ¥": 23864, "Ġlandsca": 23865, "ACE": 23866, "Ġheadlines": 23867, "Ġcatalyst": 23868, "ĠCatch": 23869, "inkles": 23870, "Ġpills": 23871, "ordo": 23872, "Ġimmigrant": 23873, "Ġexamination": 23874, "Ġaccidents": 23875, "zÄħd": 23876, "Ġquiere": 23877, "Ġnella": 23878, "Ġ67": 23879, "Ġpassa": 23880, "Ġsuperfic": 23881, "istor": 23882, "Ġnov": 23883, "ëĭµ": 23884, "Ġmandate": 23885, "isons": 23886, "ĠVirtual": 23887, "Ġselber": 23888, "Ġcounseling": 23889, "ĠNBA": 23890, "Ġsept": 23891, "Ġbeliever": 23892, "Ġmarvel": 23893, "ĠIntegr": 23894, "ĠмÑĸ": 23895, "Ġorph": 23896, "Ġbackward": 23897, "ĠGeneration": 23898, "ĠPict": 23899, "ĠÑĤоÑĤ": 23900, "Ġtapi": 23901, "prochen": 23902, "Ġhallway": 23903, "hte": 23904, "ĠÛģÛĴ": 23905, "ĠZum": 23906, "èĢģ師": 23907, "achment": 23908, "iquer": 23909, "folg": 23910, "ĠEddie": 23911, "ĠKil": 23912, "Ġwellness": 23913, "stock": 23914, "è¼ĥ": 23915, "Ġkaç": 23916, "Ġterrorism": 23917, "Ġpointer": 23918, "Of": 23919, "heric": 23920, "ĠUltimately": 23921, "Ġmeses": 23922, "ĠTrade": 23923, "Ġpint": 23924, "Ġtuition": 23925, "Ġdisagre": 23926, "Ġê²ĮìŀĦ": 23927, "Ġmanuscript": 23928, "Ġroomm": 23929, "Ġoutputs": 23930, "еÑĨи": 23931, "Ġries": 23932, "Ġsalud": 23933, "otzdem": 23934, "Ġmasses": 23935, "ĠbyÅĤa": 23936, "Ġclearing": 23937, "Ġdiscourse": 23938, "atson": 23939, "Ġfolded": 23940, "ĠJar": 23941, "ÙĦÙī": 23942, "900": 23943, "ĠÑĥÑģп": 23944, "Ġprophecy": 23945, "Ġinterfere": 23946, "иÑħод": 23947, "à¹Į": 23948, "Ġthri": 23949, "Ġ×ŀש": 23950, "Ġlazım": 23951, "Ġ1992": 23952, "Ġfuturo": 23953, "Ġlocking": 23954, "Ġembargo": 23955, "ĠNeither": 23956, "ivamente": 23957, "ĠmÃ¥ste": 23958, "Ġmik": 23959, "Ġcollector": 23960, "екоÑĤоÑĢ": 23961, "ĠGand": 23962, "Ġsentir": 23963, "ĠMight": 23964, "å¡Ķ": 23965, "Ġganzen": 23966, "UC": 23967, "Ġrelating": 23968, "SD": 23969, "Ġmosquito": 23970, "GR": 23971, "Ġhollow": 23972, "âĺħ": 23973, "ĠWalker": 23974, "Ġaffiliate": 23975, "Ġduplicate": 23976, "нем": 23977, "Ġgrape": 23978, "ĠOrganization": 23979, "Ġsynt": 23980, "Joe": 23981, "Ġgeg": 23982, "Ġrevealing": 23983, "ĠEthan": 23984, "outer": 23985, "Ġyay": 23986, "é«Ķ": 23987, "лаÑĢ": 23988, "Ġreportedly": 23989, "Ġihrer": 23990, "Ġrecognise": 23991, "Ġbumper": 23992, "ĠRandy": 23993, "ĠVenus": 23994, "tles": 23995, "Ġappetite": 23996, "Ġglucose": 23997, "Ġchodzi": 23998, "ĠFurthermore": 23999, "tir": 24000, "Ġconta": 24001, "Ġintuition": 24002, "Ġaltitude": 24003, "Ġchunks": 24004, "ĠJoshua": 24005, "ıģım": 24006, "rylic": 24007, "leans": 24008, "Ġíͼë": 24009, "LL": 24010, "Que": 24011, "Ġgor": 24012, "ĠзнаÑĩиÑĤ": 24013, "Ġpoems": 24014, "Ġexcel": 24015, "Ġexplored": 24016, "Ġpopul": 24017, "Ġincluso": 24018, "stä": 24019, "ĠGavin": 24020, "alling": 24021, "ĠÏĦον": 24022, "é©": 24023, "arbeit": 24024, "ĠGas": 24025, "Ġglorious": 24026, "rieben": 24027, "Ġspam": 24028, "Ġindoor": 24029, "Ġthrust": 24030, "ĠAld": 24031, "ĠPrior": 24032, "Ġonboard": 24033, "ãģłãģķãģĦ": 24034, "oca": 24035, "ASH": 24036, "£ł": 24037, "ĠChristine": 24038, "Ġdrawer": 24039, "Ġnoon": 24040, "Ġìŀĺë": 24041, "Ġpermanently": 24042, "æ·±": 24043, "ĠнапÑĢимеÑĢ": 24044, "Ġpodcasts": 24045, "erapeut": 24046, "prit": 24047, "Ġstainless": 24048, "ĠÚ©ÛĴ": 24049, "Ġfamilia": 24050, "ĠÑĢазÑĢ": 24051, "unto": 24052, "ĠÑģÑĤол": 24053, "Ġhä": 24054, "ĠHai": 24055, "ĠPB": 24056, "izon": 24057, "Ġkonnte": 24058, "Ġbüyük": 24059, "Ġutilizar": 24060, "ÚĨ": 24061, "Ġaquesta": 24062, "Ġmixer": 24063, "udent": 24064, "лекÑģ": 24065, "ÅĤu": 24066, "ĠÑģиÑģÑĤем": 24067, "ĠноÑĢм": 24068, "Ġfatal": 24069, "Ġconsiderations": 24070, "Ġvalidation": 24071, "Ġoli": 24072, "ĠkardeÅŁ": 24073, "ĠGLORIA": 24074, "Ġpall": 24075, "еÑģÑĤе": 24076, "Ġrectang": 24077, "Ġmedieval": 24078, "allahi": 24079, "asti": 24080, "ĠSyrian": 24081, "Ġshear": 24082, "Ġdebug": 24083, "ĠMai": 24084, "Ġknocking": 24085, "ĠLex": 24086, "ardan": 24087, "rov": 24088, "Ġmemorial": 24089, "æ°£": 24090, "ooky": 24091, "Ġstuffed": 24092, "Ġpassé": 24093, "Ġwig": 24094, "Ĥł": 24095, "Ġpróxima": 24096, "Ġ1991": 24097, "ĠмеждÑĥ": 24098, "Ġnuestros": 24099, "ĠBeast": 24100, "Ġsmo": 24101, "atched": 24102, "ologia": 24103, "Ġмод": 24104, "Ġgee": 24105, "Ġconceptual": 24106, "Ġô": 24107, "Ġdecreases": 24108, "Ġqueries": 24109, "олÑĮÑĪ": 24110, "ĠApart": 24111, "Ġexempl": 24112, "å±±": 24113, "Ġfled": 24114, "ĠOFF": 24115, "ggak": 24116, "Ġbead": 24117, "hir": 24118, "lies": 24119, "ĠClearly": 24120, "ılar": 24121, "Ġchess": 24122, "Ġwhichever": 24123, "Ġ96": 24124, "ằ": 24125, "Ġrespects": 24126, "ĠмоÑĢ": 24127, "Ġorganism": 24128, "Ġgrandpa": 24129, "ĠVie": 24130, "è·Łä½ł": 24131, "Ġflooding": 24132, "Ġupgraded": 24133, "ÑijÑĢ": 24134, "Ġcheeks": 24135, "Ġconquer": 24136, "Ġstubborn": 24137, "Ġpuzzles": 24138, "Ġauction": 24139, "Ġrelying": 24140, "ĠPROF": 24141, "ĠEsper": 24142, "ĠÐľÐ£": 24143, "Ġhype": 24144, "Ġpossibil": 24145, "Ġimprison": 24146, "ĠErn": 24147, "ìĹĪìĬµëĭĪëĭ¤": 24148, "Ġenvie": 24149, "Ġresurrection": 24150, "ä¸įè¡Į": 24151, "Ġsper": 24152, "ĠVenezuela": 24153, "som": 24154, "Ġìŀłê¹": 24155, "Ġnouvelle": 24156, "Ġcloses": 24157, "Ġ1940": 24158, "Ġqua": 24159, "ĠJared": 24160, "ĠPir": 24161, "Ġinde": 24162, "Ġscrub": 24163, "uku": 24164, "Ġrequiring": 24165, "Ġвами": 24166, "Ġconsiderable": 24167, "åIJĽ": 24168, "ilia": 24169, "Ġinne": 24170, "Ġmeinem": 24171, "Ġhardship": 24172, "Ġtraps": 24173, "roc": 24174, "ĠìĦ¤ë": 24175, "Ġresearching": 24176, "ĠMargaret": 24177, "Ġpenny": 24178, "Ġbırak": 24179, "Ñijл": 24180, "Ġwool": 24181, "Ġrhet": 24182, "Ġflatten": 24183, "çĩ": 24184, "à¹Ģร": 24185, "Ġpied": 24186, "ĠChap": 24187, "Ġunderm": 24188, "Ġfret": 24189, "Ġcrashed": 24190, "ĠFrauen": 24191, "ذÙĩ": 24192, "ivan": 24193, "Ġliterary": 24194, "latego": 24195, "Ġspäter": 24196, "Ġsimilarities": 24197, "âĨ": 24198, "ĠCoron": 24199, "ĠCreek": 24200, "Ġbosses": 24201, "Ġaccompanied": 24202, "Ġdebates": 24203, "Ġassembled": 24204, "ĠÃģ": 24205, "ĠVai": 24206, "Ġtract": 24207, "Ġsimplement": 24208, "ĠArin": 24209, "Ġvulnerability": 24210, "Ġhormone": 24211, "IEL": 24212, "OOK": 24213, "Ġrelay": 24214, "ĠAndrea": 24215, "ril": 24216, "Ġnecessity": 24217, "aceutical": 24218, "ÑİÑī": 24219, "ousing": 24220, "nahmen": 24221, "Ġfootprint": 24222, "map": 24223, "ĠTier": 24224, "annya": 24225, "intend": 24226, "åĸ®": 24227, "å¢": 24228, "Ġdecorate": 24229, "Ġzombies": 24230, "ĠHyd": 24231, "ĠSuz": 24232, "Ġcampuses": 24233, "ĠEmb": 24234, "Ġthrottle": 24235, "Ġadmin": 24236, "Ġoportun": 24237, "Ġmirrors": 24238, "Ġidentities": 24239, "ĠClin": 24240, "Ġë¹Ħë": 24241, "á¹£": 24242, "ĠOtt": 24243, "Ġblues": 24244, "Ġimpressions": 24245, "-,": 24246, "Ġvague": 24247, "afe": 24248, "Ġinferior": 24249, "erald": 24250, "Ġmedicines": 24251, "Ġpregunta": 24252, "osely": 24253, "Ġtélé": 24254, "ĠMonth": 24255, "ĠLeaders": 24256, "ĠEgyptian": 24257, "Ġration": 24258, "kers": 24259, "heits": 24260, "Ġrecht": 24261, "Play": 24262, "Ġeg": 24263, "Ġpolls": 24264, "ĠWOODR": 24265, "Ġslots": 24266, "jam": 24267, "Both": 24268, "ĠRat": 24269, "ÑĢаж": 24270, "ĠBright": 24271, "ä¸Ģå®ļ": 24272, "á»iji": 24273, "urious": 24274, "Ġsingers": 24275, "Ġlogin": 24276, "Ġtêm": 24277, "lation": 24278, "ĠMum": 24279, "ưá»Ŀng": 24280, "ĠEditor": 24281, "åIJij": 24282, "Ġinnovations": 24283, "have": 24284, "ĠSek": 24285, "Ġweaker": 24286, "ĠGob": 24287, "After": 24288, "´ì§Ģ": 24289, "Ġë¬¸ìłľ": 24290, "ãĥ¼ãĥ¼": 24291, "Ġdisadvantage": 24292, "確": 24293, "Ġgaze": 24294, "ĠMack": 24295, "Ïģί": 24296, "ĠKiss": 24297, "ĠHolo": 24298, "ĠBirth": 24299, "izi": 24300, "bab": 24301, "ä¿Ŀ": 24302, "ìĭľê³ł": 24303, "деÑĢж": 24304, "Ġsquat": 24305, "кÑĥÑģ": 24306, "uni": 24307, "ĠComme": 24308, "ĠWOODRUFF": 24309, "ĠChampionship": 24310, "Ġwelche": 24311, "ĠYouth": 24312, "zem": 24313, "Ġodpow": 24314, "Ġpersistent": 24315, "rut": 24316, "ìĶ©": 24317, "íĸ¥": 24318, "lair": 24319, "iku": 24320, "Ġvendor": 24321, "Ġchúng": 24322, "Ġfinanci": 24323, "Ġoverly": 24324, "âu": 24325, "Ġgluten": 24326, "Ġ1800": 24327, "Ġdivisions": 24328, "Ġciudad": 24329, "Ġobed": 24330, "Ġwarum": 24331, "Ġeher": 24332, "Ġelim": 24333, "ĠÐĴо": 24334, "Ġpeuvent": 24335, "ĠWanna": 24336, "Ġattendance": 24337, "Ġassessments": 24338, "ĠBog": 24339, "Ġimagery": 24340, "Ġcollectively": 24341, "Ġinformal": 24342, "ĠSchwe": 24343, "Ġdeutlich": 24344, "ĠChel": 24345, "ĠPE": 24346, "owed": 24347, "Ġbanner": 24348, "Ġshelves": 24349, "ĠReturn": 24350, "æĭ¿": 24351, "LAUGHS": 24352, "Ġcongratulate": 24353, "ĠNorway": 24354, "Ġdwell": 24355, "ĠCaribbean": 24356, "Ġnorms": 24357, "ĠAnimal": 24358, "ĠValentine": 24359, "Ġextending": 24360, "ĠVou": 24361, "orr": 24362, "ĠCheng": 24363, "¡": 24364, "ĠдоÑĢог": 24365, "Ġveg": 24366, "ĠhÃ¥": 24367, "ĠXin": 24368, "Ġì¹´ë": 24369, "emet": 24370, "Ġhypoth": 24371, "Ġinteressante": 24372, "rices": 24373, "IZ": 24374, "ĠUSD": 24375, "Ġrunner": 24376, "ĠBag": 24377, "Ġê½": 24378, "Ġcomeçar": 24379, "Ġpigs": 24380, "Ġweaknesses": 24381, "Ph": 24382, "ĠViol": 24383, "ä¸įç͍": 24384, "Ġdragging": 24385, "ĠAquÃŃ": 24386, "ĠCSS": 24387, "Ġmillimeters": 24388, "Ġestás": 24389, "Ġacute": 24390, "Ġdejar": 24391, "iÄŁ": 24392, "obra": 24393, "Love": 24394, "Ġsilk": 24395, "****": 24396, "Ġjoins": 24397, "Ġprol": 24398, "Ġê°IJìĤ¬íķ©ëĭĪëĭ¤": 24399, "æĶ¯": 24400, "ØŃد": 24401, "aghetti": 24402, "änner": 24403, "Ġstrang": 24404, "Ġdoubled": 24405, "Ġdescriptions": 24406, "Ġstellen": 24407, "Ġparti": 24408, "ç«ĭ": 24409, "²Ħë": 24410, "ĠÃ¶ÄŁ": 24411, "ighing": 24412, "Ġangular": 24413, "Ġnatuur": 24414, "ĠShel": 24415, "ươ": 24416, "Ġrays": 24417, "Ġseper": 24418, "start": 24419, "vised": 24420, "Ġrushed": 24421, "Ġinternationally": 24422, "Ġnivel": 24423, "Ġboxing": 24424, "fallen": 24425, "á»ijc": 24426, "Ġseinen": 24427, "plicity": 24428, "Ġcarboh": 24429, "ĠTravis": 24430, "uso": 24431, "ĠPhase": 24432, "Ġactivation": 24433, "Ġopio": 24434, "·¨": 24435, "Ġdecreased": 24436, "Car": 24437, "Ġbundle": 24438, "Ġexpend": 24439, "ormal": 24440, "Ġadjacent": 24441, "Ġmee": 24442, "ĠоÑĢг": 24443, "Ġtranscript": 24444, "ĠLanguage": 24445, "GS": 24446, "è§ī": 24447, "Ġseul": 24448, "Ãłnh": 24449, "Ġnya": 24450, "nings": 24451, "Ġìĭľë": 24452, "ĠëͰëĿ¼": 24453, "ĠAgr": 24454, "ÃŃd": 24455, "çķĻ": 24456, "Ġaby": 24457, "ĠNeo": 24458, "ıyoruz": 24459, "ĠThinking": 24460, "aime": 24461, "Ġvite": 24462, "Ġtravés": 24463, "Ġ×ij×¢": 24464, "Ġмед": 24465, "Our": 24466, "hoot": 24467, "Ġliner": 24468, "ĠPizza": 24469, "Ġhyg": 24470, "flies": 24471, "ĠContinue": 24472, "Ġdental": 24473, "ĠTib": 24474, "Ġregulate": 24475, "lieÃŁ": 24476, "ALK": 24477, "ĠTae": 24478, "길": 24479, "ĠBrexit": 24480, "ĠGut": 24481, "Ġoccupation": 24482, "Ġzrobi": 24483, "âm": 24484, "Ġwhisk": 24485, "ä¸ĸçķĮ": 24486, "Ġkanske": 24487, "omon": 24488, "robe": 24489, "Ġwarfare": 24490, "Ġthá»ĥ": 24491, "Ġjaki": 24492, "Ġstrokes": 24493, "Ġpeas": 24494, "ĠDamit": 24495, "HAN": 24496, "Ġinterference": 24497, "ĠминÑĥÑĤ": 24498, "NER": 24499, "outing": 24500, "Ġtextures": 24501, "Łī": 24502, "owi": 24503, "ĠíķĻ": 24504, "Ġdens": 24505, "Ġprotagonist": 24506, "änn": 24507, "Ġgoddess": 24508, "Ġwollte": 24509, "ijo": 24510, "ĠWoche": 24511, "ĠVPN": 24512, "story": 24513, "Ġkinderg": 24514, "Ġfunnel": 24515, "Ġdistress": 24516, "ноÑģÑĤÑĮÑİ": 24517, "Ġnoisy": 24518, "ĠпÑĢодолж": 24519, "Ġdaran": 24520, "Ġenzyme": 24521, "лож": 24522, "Ġmute": 24523, "Ġdwar": 24524, "Ġاس": 24525, "Ġkompl": 24526, "Ġmerit": 24527, "Ġfosse": 24528, "ĠDrink": 24529, "Ġfora": 24530, "Ġwohl": 24531, "Ġbreeze": 24532, "Ġsanit": 24533, "Ġdrin": 24534, "ĠìĿ´ê±°ëĬĶ": 24535, "Ġ62": 24536, "Ġì°¨ë": 24537, "abytes": 24538, "Ġdeeds": 24539, "Ġй": 24540, "ième": 24541, "iggling": 24542, "Ġ\"'": 24543, "ĠÑĩаÑģÑĤÑĮ": 24544, "ĠAnswer": 24545, "Ġevangel": 24546, "Ġ1080": 24547, "ĠVisit": 24548, "icient": 24549, "Ġreliability": 24550, "ÑİÑģÑĮ": 24551, "ĠEarlier": 24552, "Ġfid": 24553, "çŃīä¸Ģä¸ĭ": 24554, "Ġsleeves": 24555, "iyorsun": 24556, "Ġbib": 24557, "ĠAccount": 24558, "Ñıли": 24559, "ciplinary": 24560, "zas": 24561, "ĠбеÑĢ": 24562, "Ġnecklace": 24563, "Ġblender": 24564, "ĠPhillips": 24565, "eti": 24566, "ĠJupiter": 24567, "Ġprovoc": 24568, "ĠYears": 24569, "entre": 24570, "acio": 24571, "Ġkü": 24572, "Ġantenna": 24573, "Ġnovels": 24574, "Ġfart": 24575, "ĠSugar": 24576, "ĠJudy": 24577, "Ġcollapsed": 24578, "ç°": 24579, "ritis": 24580, "ĠìĥģíĻ©": 24581, "ÐĹЫ": 24582, "ĠVerf": 24583, "ranean": 24584, "ereum": 24585, "ĠTarget": 24586, "Ġ88": 24587, "ĠÐĺз": 24588, "ideo": 24589, "Ġregression": 24590, "ì¶ľ": 24591, "Ġmówi": 24592, "Ġstudios": 24593, "iens": 24594, "iph": 24595, "Ġfrying": 24596, "Ġfascinated": 24597, "ĠWah": 24598, "bucks": 24599, "maya": 24600, "ĠSaturn": 24601, "ĠMommy": 24602, "Ġratings": 24603, "Ġautumn": 24604, "ương": 24605, "Ġloser": 24606, "Ġcentro": 24607, "érieur": 24608, "ĠFold": 24609, "Ġsupervisor": 24610, "ĠNobel": 24611, "Ġunderest": 24612, "obia": 24613, "ĠвÑģÑı": 24614, "Ġverw": 24615, "Ġfuels": 24616, "Ġartifacts": 24617, "Ġë¶Ļ": 24618, "ĠAutom": 24619, "çļĦæĺ¯": 24620, "ÛĶ": 24621, "×ķס": 24622, "Ġihnen": 24623, "Ġ59": 24624, "ounding": 24625, "еÑĢÑĭ": 24626, "inars": 24627, "chant": 24628, "Ġaddicted": 24629, "Ġexplosive": 24630, "Ġdispers": 24631, "âĸĪ": 24632, "axis": 24633, "ARY": 24634, "Ġlum": 24635, "ĠÑĥÑģл": 24636, "ĠØĮ": 24637, "Ġrupees": 24638, "ĠPearl": 24639, "camp": 24640, "tv": 24641, "oya": 24642, "Ġconcludes": 24643, "Ġcollision": 24644, "Ġbuyer": 24645, "Ġplayground": 24646, "Ġsprings": 24647, "Ġfeminine": 24648, "ĠRas": 24649, "Ġincarcer": 24650, "íĹĺ": 24651, "Ġdialect": 24652, "Ġclosure": 24653, "Ġchatting": 24654, "Ġbabe": 24655, "Ġspotlight": 24656, "Ġnotation": 24657, "è·¯": 24658, "Star": 24659, "ião": 24660, "Ġtête": 24661, "Ġtide": 24662, "Ġjunto": 24663, "Ġsenator": 24664, "Ð¥": 24665, "Ġexcuses": 24666, "Ġblink": 24667, "Ġadmission": 24668, "ĠLily": 24669, "Ñĭми": 24670, "Ġamigo": 24671, "Ġlust": 24672, "ëĭ¬": 24673, "Ġamino": 24674, "äºĭæĥħ": 24675, "Ġconsultant": 24676, "ĠElectric": 24677, "Ġëħ¸ëŀĺ": 24678, "ujah": 24679, "Ġshooter": 24680, "ichten": 24681, "ĠUkrainian": 24682, "Ġaims": 24683, "ĠEntertain": 24684, "Ġmiracles": 24685, "èѰ": 24686, "Ġzeigen": 24687, "Ġlam": 24688, "Ġress": 24689, "ĠJill": 24690, "ylan": 24691, "Ġrook": 24692, "Ġhaya": 24693, "Ġpassport": 24694, "adata": 24695, "Ġjuicy": 24696, "conf": 24697, "лей": 24698, "ĠSz": 24699, "Ġintercept": 24700, "ãģĤãĤĬãģĮãģ¨ãģĨãģĶãģĸ": 24701, "ĠTeams": 24702, "Ġmaken": 24703, "irrel": 24704, "ĠLIKE": 24705, "áºŃy": 24706, "êµ°": 24707, "Ġshortage": 24708, "Ġparadigm": 24709, "Ġpapel": 24710, "Ġastero": 24711, "ãģ¾ãģŁ": 24712, "Ġsollen": 24713, "ĠMickey": 24714, "ĠOrleans": 24715, "Ġcholesterol": 24716, "Ġgoose": 24717, "ÑĨиÑİ": 24718, "ãģĤãĤĭ": 24719, "ĠFL": 24720, "Ġголов": 24721, "Ġtribute": 24722, "ĠGam": 24723, "Ġévidemment": 24724, "ÑıÑħ": 24725, "å®ŀ": 24726, "çͰ": 24727, "Ġinappropri": 24728, "uhan": 24729, "Ġorganizational": 24730, "ailed": 24731, "Ġendure": 24732, "Ġ76": 24733, "Ġshotgun": 24734, "Ġlivre": 24735, "Ġsuited": 24736, "Ġwarmth": 24737, "ĠSIM": 24738, "Ġenvision": 24739, "Ġdegrad": 24740, "îne": 24741, "Laughing": 24742, "ĠWhoever": 24743, "ĠBuddhism": 24744, "Ġsprinkle": 24745, "ceÄŁiz": 24746, "Ġruins": 24747, "Ġstarch": 24748, "ĠHerz": 24749, "Ġinjustice": 24750, "Ġhumidity": 24751, "ожалÑĥй": 24752, "ĠObject": 24753, "ĠIgn": 24754, "ĠExam": 24755, "igers": 24756, "Ġthou": 24757, "ĠSoy": 24758, "ivas": 24759, "Ġpoles": 24760, "math": 24761, "Ġвним": 24762, "INGING": 24763, "edral": 24764, "Ġexplor": 24765, "Ġroasted": 24766, "Ġcrawl": 24767, "Ġcoff": 24768, "Ġanom": 24769, "Ġwij": 24770, "Ġimproves": 24771, "Ġtreaty": 24772, "Ġdiscovering": 24773, "Ġstatute": 24774, "Ġmercado": 24775, "ĠÑģил": 24776, "Ġintel": 24777, "ĠChancellor": 24778, "ĠMedicaid": 24779, "ugi": 24780, "Ġverbal": 24781, "Ġdön": 24782, "Ġscripture": 24783, "Ġiteration": 24784, "eks": 24785, "ĠOxford": 24786, "Ġwäh": 24787, "ĠVad": 24788, "ĠAK": 24789, "ĠìķĦìĿ´ë": 24790, "Ġiets": 24791, "Ġneedles": 24792, "ÙĥÙħ": 24793, "Ġpasado": 24794, "Ġalbums": 24795, "Ġyea": 24796, "etzen": 24797, "ĦëıĦ": 24798, "Ġdetermines": 24799, "Ġthee": 24800, "ĠPlaying": 24801, "ärt": 24802, "Ġצ": 24803, "cled": 24804, "Ġdownward": 24805, "alone": 24806, "Ġsolu": 24807, "Ġpartition": 24808, "Ġwz": 24809, "dd": 24810, "Ġpessoal": 24811, "媽": 24812, "Ġfactories": 24813, "Ġbleibt": 24814, "มา": 24815, "alsa": 24816, "ĠNFL": 24817, "Ġfuera": 24818, "Ġreserved": 24819, "ĠEarn": 24820, "Ġhelt": 24821, "Ġshortcut": 24822, "Ġconvincing": 24823, "space": 24824, "Ġenforce": 24825, "Ġcores": 24826, "Ġefter": 24827, "Ġrecession": 24828, "xico": 24829, "Ġproposition": 24830, "arians": 24831, "ropol": 24832, "Ġ몰ë": 24833, "ĠÎľ": 24834, "ĠìļĶì¦ĺ": 24835, "Ġactivist": 24836, "Ġconviction": 24837, "Ġzab": 24838, "Ġcanceled": 24839, "ÑĤоÑĩно": 24840, "Ġή": 24841, "éĢĻæ¨£åŃIJ": 24842, "nite": 24843, "Ġfundra": 24844, "buzzer": 24845, "ело": 24846, "ications": 24847, "Ġzona": 24848, "Ġteens": 24849, "Ġmethodology": 24850, "Ġì¤ijìļĶ": 24851, "than": 24852, "ĠUl": 24853, "ĠGrey": 24854, "Ġhog": 24855, "INK": 24856, "ĠSung": 24857, "ĠClaud": 24858, "ĠCNN": 24859, "Ġdelivers": 24860, "alin": 24861, "ĠAdobe": 24862, "othe": 24863, "ĠDeswegen": 24864, "ำ": 24865, "Ġwerde": 24866, "Ġgrease": 24867, "Ġupgrades": 24868, "ĠFinland": 24869, "accept": 24870, "Ġinterrog": 24871, "bee": 24872, "Ġãģ«": 24873, "Ġprede": 24874, "ĠNep": 24875, "ĠCambridge": 24876, "Ġgraphs": 24877, "Ġhaunted": 24878, "Ñģем": 24879, "æ§": 24880, "åħĭ": 24881, "Some": 24882, "ĠMall": 24883, "Ġrehearsal": 24884, "ĠUrban": 24885, "ĠLag": 24886, "Ġnim": 24887, "ê°ķ": 24888, "Ġpositioned": 24889, "Ġavoided": 24890, "EMA": 24891, "Ġllegar": 24892, "Ġrápido": 24893, "Ġgouvern": 24894, "Ġhing": 24895, "Ġdealer": 24896, "Ġreforms": 24897, "Ġfatty": 24898, "кол": 24899, "ĠAce": 24900, "Ġnep": 24901, "Ġì²Ń": 24902, "Ġcomputation": 24903, "ĠStream": 24904, "bourne": 24905, "tur": 24906, "Por": 24907, "Ġsleepy": 24908, "Ġbanget": 24909, "ãģĤãģ®": 24910, "Ġweighs": 24911, "Ġbleiben": 24912, "ĠGren": 24913, "Ġunions": 24914, "ĠêµIJ": 24915, "Ġaprender": 24916, "uitar": 24917, "ĠJest": 24918, "uming": 24919, "ĠPlayer": 24920, "ĠExtrem": 24921, "Ġinteger": 24922, "аÑĩе": 24923, "Ġconcerts": 24924, "×ķ׼": 24925, "ĠtrochÄĻ": 24926, "ĠRepe": 24927, "éĩįè¦ģ": 24928, "à¹Ĥ": 24929, "żen": 24930, "Ġsounding": 24931, "Ġanonymous": 24932, "Ġexca": 24933, "ĠIranian": 24934, "Ġenergetic": 24935, "Ġwives": 24936, "ĠÑĨвеÑĤ": 24937, "Ġais": 24938, "ãģĭãģª": 24939, "Ġsudah": 24940, "Ġunderwear": 24941, "Ġcrunchy": 24942, "ĠPain": 24943, "Ġgerçek": 24944, "redict": 24945, "Ġmisma": 24946, "ÑĸÑĤ": 24947, "Ġsurviving": 24948, "ÎŃÏĤ": 24949, "Ġparticipant": 24950, "ĠHessen": 24951, "árias": 24952, "Ġsubway": 24953, "istä": 24954, "Ġcoral": 24955, "Ġmarijuana": 24956, "ĠMemorial": 24957, "ÑĪий": 24958, "riz": 24959, "Ġsatellites": 24960, "Ġlease": 24961, "ĠCameron": 24962, "umph": 24963, "Ġclassmates": 24964, "ähän": 24965, "ÑģÑĤве": 24966, "Ġhue": 24967, "ĵ¤ìĿĦ": 24968, "Ġproportional": 24969, "Ġnoss": 24970, "Ġlaps": 24971, "rÃ¥": 24972, "Ġbitcoin": 24973, "ÐĹЫÐļÐIJ": 24974, "Ġì¶©": 24975, "ĠÙĦÙĦ": 24976, "ĠMort": 24977, "ĠEsp": 24978, "arnos": 24979, "ĠÑģказал": 24980, "Ġänd": 24981, "åħĦ": 24982, "×Ļ×Ļ×Ŀ": 24983, "ĠGeb": 24984, "gehen": 24985, "Inaudible": 24986, "borough": 24987, "ÑĦÑĦ": 24988, "Ġfellowship": 24989, "ĠPaper": 24990, "Ġcurved": 24991, "ĠGEOR": 24992, "Ġcalculator": 24993, "ĠCatal": 24994, "ĠvÃło": 24995, "Ġbypass": 24996, "леÑĤ": 24997, "à³": 24998, "trans": 24999, "rencies": 25000, "ì¡Į": 25001, "igent": 25002, "Ġtasted": 25003, "Ġoceans": 25004, "uft": 25005, "ervice": 25006, "ĠÐľÐ£ÐĹЫÐļÐIJ": 25007, "ĠClassic": 25008, "Ġrespectively": 25009, "~)": 25010, "ître": 25011, "ĠNash": 25012, "Ġzit": 25013, "ĠìĽĥ": 25014, "ĠëĨĴ": 25015, "quote": 25016, "ĠUns": 25017, "Ġtac": 25018, "Ġproves": 25019, "ĠPortland": 25020, "bly": 25021, "Ġere": 25022, "ì¶Ķ": 25023, "Ġépoca": 25024, "ĠÑĤÑĭÑģÑıÑĩ": 25025, "76": 25026, "Ġhade": 25027, "ĠFro": 25028, "ĠpolÃŃtica": 25029, "tag": 25030, "ĠíķŃ": 25031, "Ġschö": 25032, "arett": 25033, "Ġprovisions": 25034, "Ġmotors": 25035, "Ġimaging": 25036, "Ġdok": 25037, "ulously": 25038, "Ġmeille": 25039, "çİ°åľ¨": 25040, "ëIJ": 25041, "ĠISO": 25042, "ĠSTEM": 25043, "ĠBowl": 25044, "Ġtowers": 25045, "ĠEe": 25046, "ĠPerformance": 25047, "Ġloin": 25048, "cussion": 25049, "Ġcoastal": 25050, "iale": 25051, "compass": 25052, "Ġspells": 25053, "Ġdisappointing": 25054, "Ġë²Ī째": 25055, "EER": 25056, "Ġversatile": 25057, "asury": 25058, "Ġenfin": 25059, "Ġdownside": 25060, "Ġguiding": 25061, "ĠاÙĦÙĤ": 25062, "Ġninety": 25063, "charged": 25064, "ĠFans": 25065, "Ġphilosophical": 25066, "Ġgarn": 25067, "ĠmÃ¥nga": 25068, "Ġwillingness": 25069, "Ġportions": 25070, "aben": 25071, "Ġï": 25072, "¿": 25073, "raul": 25074, "Ġsprint": 25075, "ifen": 25076, "ıyla": 25077, "ĠкÑĥп": 25078, "ãģıãģłãģķãģĦ": 25079, "Ġensuite": 25080, "ĠCapitol": 25081, "Ġ63": 25082, "ĠговоÑĢиÑĤ": 25083, "Ġappointments": 25084, "æī¾": 25085, "omiast": 25086, "Ġcareg": 25087, "Ġpublisher": 25088, "Ġheraus": 25089, "Ġεί": 25090, "ĠVS": 25091, "ãģĿãģĹãģ¦": 25092, "ä¸Ńåħ±": 25093, "Ġsacrifices": 25094, "third": 25095, "Ġhumanitarian": 25096, "ĠëĤ´ì": 25097, "imon": 25098, "Ġinequ": 25099, "Ġzob": 25100, "Ġcomfortably": 25101, "ĠDinge": 25102, "Ġcancelled": 25103, "ĠPSAKI": 25104, "ĠRobinson": 25105, "Ġfins": 25106, ")?": 25107, "ĠHistor": 25108, "ĠÑĩеловека": 25109, "Ġtbsp": 25110, "text": 25111, "kim": 25112, "Ġupdating": 25113, "Ġgeld": 25114, "feld": 25115, "ı¼": 25116, "Ġmä": 25117, "Ġcafé": 25118, "ÖĢ": 25119, "ĠSri": 25120, "ĠRegion": 25121, "ĠHahaha": 25122, "Ġfinances": 25123, "ĠاÙĦØ´": 25124, "Ġbunk": 25125, "ruk": 25126, "haft": 25127, "Ġlateral": 25128, "Ġextensions": 25129, "ĠìķĦìĿ´": 25130, "Ġdefinite": 25131, "ĠZhao": 25132, "ĠLuis": 25133, "sty": 25134, "Ġcasos": 25135, "ĠKlim": 25136, "Ġ1993": 25137, "Ġrealization": 25138, "Ġhistorian": 25139, "Ġcracked": 25140, "ëĤ´": 25141, "Ġsystème": 25142, "ĠCIA": 25143, "ĠÑĤво": 25144, "ospheric": 25145, "Ġflee": 25146, "Ġrất": 25147, "ĠRegardless": 25148, "Ġreluct": 25149, "Ġtimely": 25150, "ĠJulian": 25151, "GM": 25152, "éĴ": 25153, "adura": 25154, "é£Ł": 25155, "Ġdresses": 25156, "çģ£": 25157, "ĠëĶĶ": 25158, "Ġnominated": 25159, "Ġadvocates": 25160, "ymph": 25161, "Ġrecordings": 25162, "Ġdeviation": 25163, "Ġprioritize": 25164, "Ġspiral": 25165, "ĠYOUR": 25166, "Ġtranspose": 25167, "ampoo": 25168, "ĠìĽIJëŀĺ": 25169, "ĠVision": 25170, "Ġpolite": 25171, "Ġhamb": 25172, "ĠPatient": 25173, "æ¯Ķè¼ĥ": 25174, "íģ¬ë": 25175, "Ġsia": 25176, "Ġê³³": 25177, "Ġže": 25178, "è§Ģ": 25179, "Ġsupermarket": 25180, "ë¹": 25181, "ĠSierra": 25182, "Ġgrilled": 25183, "ĠUpon": 25184, "Ġabsent": 25185, "Ġmec": 25186, "ĠApollo": 25187, "Ġpunk": 25188, "ĠPaÅĦst": 25189, "ĠÑģвой": 25190, "Ġ거기": 25191, "Girl": 25192, "Ġskinny": 25193, "ĠPremier": 25194, "Ġterritories": 25195, "Ġliability": 25196, "Ġjerk": 25197, "ratic": 25198, "Ġdancers": 25199, "ĠÑĥÑĢов": 25200, "Ġê´Ģë": 25201, "only": 25202, "ĠStu": 25203, "Ġskeleton": 25204, "ĠëŃIJë": 25205, "Ġзакон": 25206, "ıkt": 25207, "ĠMIKE": 25208, "Ġlö": 25209, "mie": 25210, "Ġreiter": 25211, "ãģĵãĤĮãģ¯": 25212, "ĠKolleg": 25213, "ĠAdams": 25214, "licher": 25215, "Ġçocuk": 25216, "Ñıг": 25217, "Ġblush": 25218, "Ġsunshine": 25219, "Ġez": 25220, "ĠDevil": 25221, "Ġ길": 25222, "ĠãģĬ": 25223, "add": 25224, "Ġlicensed": 25225, "Ġvinyl": 25226, "ĠCzech": 25227, "imag": 25228, "Ġcracking": 25229, "Ġìº": 25230, "Ġudah": 25231, "Ġsommes": 25232, "Ġìĸ¼êµ": 25233, "waÄĩ": 25234, "Ġfres": 25235, "åij½": 25236, "ĠWalmart": 25237, "ĠТепеÑĢÑĮ": 25238, "atisf": 25239, "CI": 25240, "lang": 25241, "Ġdiffusion": 25242, "çĶ·": 25243, "Ġsomos": 25244, "ĠMakes": 25245, "æĪijæĥ³": 25246, "ĠRicky": 25247, "Ġmucha": 25248, "íķ¨": 25249, "Ġhorsepower": 25250, "asia": 25251, "Ġfibers": 25252, "Ġerm": 25253, "Ñģкие": 25254, "Ġjeste": 25255, "Ġfirefight": 25256, "Ġcuisine": 25257, "Ġbesonders": 25258, "dig": 25259, "Ġì¢ħ": 25260, "ĠÑĥж": 25261, "Ġtracing": 25262, "Ġcertains": 25263, "ĠApply": 25264, "ÑĭваÑĤÑĮ": 25265, "çĮ": 25266, "Ġbru": 25267, "ĠYES": 25268, "ĠBai": 25269, "ĠDit": 25270, "ĠBis": 25271, "Ġunle": 25272, "ÑģÑĤаÑĤоÑĩно": 25273, "ĠAwak": 25274, "..\"": 25275, "Ġ125": 25276, "Ġrooted": 25277, "Ġcautious": 25278, "const": 25279, "Ġorchestra": 25280, "çľ¼": 25281, "ĠвнÑĥÑĤ": 25282, "Ġquelqu": 25283, "ĠоÑĤвеÑĤ": 25284, "ĠMethod": 25285, "ì¹ľ": 25286, "ĠμαÏĤ": 25287, "lü": 25288, "ĠìķĦê¹Į": 25289, "Ġnaming": 25290, "Char": 25291, "ĠSicher": 25292, "Ġprivileged": 25293, "ĠFly": 25294, "Ġãģĭ": 25295, "áºŃt": 25296, "Ġadvances": 25297, "ĠZelda": 25298, "Ġandra": 25299, "Ġgrinding": 25300, "ĠEdition": 25301, "pf": 25302, "Ġwarriors": 25303, "Ġhedge": 25304, "Ġunseren": 25305, "ĠÑģÑİда": 25306, "eliness": 25307, "Ġpersonalities": 25308, "Ġfö": 25309, "'M": 25310, "ĠÑĤоÑĩно": 25311, "Ġshipped": 25312, "Ġmeteor": 25313, "Ġsurroundings": 25314, "ĠFill": 25315, "uesta": 25316, "ĠPersonal": 25317, "ĠAlle": 25318, "ORT": 25319, "ä¹ħ": 25320, "ĠSche": 25321, "VI": 25322, "Ġcomparable": 25323, "damn": 25324, "Ġditch": 25325, "YAN": 25326, "ismus": 25327, "Ġpickup": 25328, "Ġdak": 25329, "ĠEP": 25330, "best": 25331, "ĠSue": 25332, "ällt": 25333, "Ġpopcorn": 25334, "Ġfolding": 25335, "home": 25336, "иваеÑĤ": 25337, "å·²ç¶ĵ": 25338, "Ġannot": 25339, "chuck": 25340, "Ġfierce": 25341, "Ġdamaging": 25342, "Ġflop": 25343, "Ġpasar": 25344, "Ġreef": 25345, "ĠÑģвоей": 25346, "Ġzoo": 25347, "overs": 25348, "jets": 25349, "Ġprès": 25350, "ĠSilicon": 25351, "teok": 25352, "ĠSeth": 25353, "atamente": 25354, "Ġtransmitted": 25355, "Ġreplicate": 25356, "Ġslim": 25357, "ĠCream": 25358, "æĦŁãģĺ": 25359, "Ġsidewalk": 25360, "ìĪĺë": 25361, "ĠжизнÑĮ": 25362, "ĠMonica": 25363, "ä¾ĨäºĨ": 25364, "Ġcopied": 25365, "ĠTerra": 25366, "istent": 25367, "ç³»": 25368, "Ġоно": 25369, "Ġwhale": 25370, "ĠWITH": 25371, "лÑĥÑĪ": 25372, "å½±çīĩ": 25373, "ĠEen": 25374, "ĠÑģвои": 25375, "Ġordin": 25376, "Ġplural": 25377, "Ġspokes": 25378, "Ġdispute": 25379, "Ġsensible": 25380, "Ġpreaching": 25381, "Ġktórzy": 25382, "pted": 25383, "avier": 25384, "Ġpistol": 25385, "ĠTapi": 25386, "ĠÅĤ": 25387, "ffff": 25388, "Ġacrylic": 25389, "Ġignorance": 25390, "ĠZiel": 25391, "rans": 25392, "Ġwelding": 25393, "mid": 25394, "æĪijä¸į": 25395, "Ġзаним": 25396, "Ġlanes": 25397, "Ġmines": 25398, "Ġmoms": 25399, "×ķ×Ĺ": 25400, "ĠChamber": 25401, "tier": 25402, "Ġmodest": 25403, "ĠìĹ¬ê¸°ìĦľ": 25404, "Ġunas": 25405, "Ġwrench": 25406, "handed": 25407, "Ġsaturated": 25408, "ĠFang": 25409, "ĠCommissioner": 25410, "र": 25411, "Ġ×ĸ": 25412, "ĠLouisiana": 25413, "ĠMask": 25414, "Ġcubes": 25415, "ì͍": 25416, "Ġvidéos": 25417, "ĠnÃ¥gon": 25418, "Ġrider": 25419, "Ġì¶ľ": 25420, "Ġsón": 25421, "ĠLatino": 25422, "bank": 25423, "íķ´ì£¼": 25424, "ĠBrend": 25425, "Ġsexuality": 25426, "...,": 25427, "Ġforgetting": 25428, "ĠÛĮ": 25429, "ĠAvengers": 25430, "ĠBonjour": 25431, "cessor": 25432, "кÑĢаÑĹ": 25433, "cence": 25434, "Ġgeograph": 25435, "culo": 25436, "оÑģÑĤÑĮ": 25437, "Ġsweating": 25438, "íĥĢ": 25439, "Ġsymmetry": 25440, "tsÃ¥": 25441, "Ġjan": 25442, "ĠFerr": 25443, "é¦ĸ": 25444, "Ġambassador": 25445, "ziÄĻk": 25446, "Ġmusun": 25447, "ĠÑĥÑĤ": 25448, "ĠLG": 25449, "issent": 25450, "commun": 25451, "Ġcours": 25452, "Ġdevelops": 25453, "Ġbronze": 25454, "Ġsubstances": 25455, "driven": 25456, "주ìĦ¸ìļĶ": 25457, "Ġaos": 25458, "åĦĦ": 25459, "ĠPROFESS": 25460, "half": 25461, "Ġsorted": 25462, "ĠBomb": 25463, "лаг": 25464, "ĠMalaysia": 25465, "ĠChristina": 25466, "Ġteammate": 25467, "èģŀ": 25468, "FT": 25469, "Ġkı": 25470, "hearted": 25471, "++": 25472, "ogenic": 25473, "Ġbells": 25474, "ĠOuais": 25475, "Ġspecialists": 25476, "бÑĭ": 25477, "depth": 25478, "lasses": 25479, "gies": 25480, "ĠCoffee": 25481, "Ġmarking": 25482, "Ġfoll": 25483, "uli": 25484, "Ġadhesive": 25485, "ĠBot": 25486, "ĠPunkt": 25487, "eye": 25488, "ĠBub": 25489, "elong": 25490, "åζ": 25491, "ĠпÑĢик": 25492, "Ġdonor": 25493, "84": 25494, "Ġenfor": 25495, "Ġcatches": 25496, "Ġbricks": 25497, "Ġknitting": 25498, "ĠKnowing": 25499, "oks": 25500, "HY": 25501, "ride": 25502, "ĠFantasy": 25503, "iman": 25504, "Ġpse": 25505, "Ġìĺ¨": 25506, "Ġвд": 25507, "Ġrestra": 25508, "Ġevaluated": 25509, "ÑĢев": 25510, "Ġfortunately": 25511, "Ġchegar": 25512, "رب": 25513, "Ġdomains": 25514, "ibi": 25515, "arry": 25516, "Ġshutter": 25517, "Ġficou": 25518, "Mike": 25519, "Ġinclu": 25520, "Ġdonors": 25521, "Ġapl": 25522, "ĠLower": 25523, "Ġimported": 25524, "Ġacademy": 25525, "Ġfinals": 25526, "Ġdisappears": 25527, "ÙĬا": 25528, "Ġadministrator": 25529, "js": 25530, "Ġcutter": 25531, "Ġranging": 25532, "örper": 25533, "Ġconstraint": 25534, "ĠTable": 25535, "ĠShan": 25536, "vic": 25537, "ĠFix": 25538, "ĠSwift": 25539, "ounces": 25540, "ĠWarum": 25541, "Ġlettuce": 25542, "appelle": 25543, "Ġshave": 25544, "Ġbás": 25545, "Ġ77": 25546, "ĠOoo": 25547, "ao": 25548, "ĠMcM": 25549, "ĠDrew": 25550, "Ġlump": 25551, "Ġlashes": 25552, "scheinlich": 25553, "Rep": 25554, "inis": 25555, "ĠCette": 25556, "Ġcomposite": 25557, "emetery": 25558, "Ġsorte": 25559, "ĠFinancial": 25560, "оне": 25561, "rones": 25562, "ĠVoy": 25563, "Ġtéc": 25564, "ł¹": 25565, "ĠNinja": 25566, "ĠCorin": 25567, "еннÑı": 25568, "ìĿ´ìĹĪ": 25569, "Ġnich": 25570, "Ġdetective": 25571, "â̦\"": 25572, "Ïĥε": 25573, "Ŀ¼ëıĦ": 25574, "Ġë³Ģ": 25575, "Ġë¸Ķë": 25576, "Ġprope": 25577, "ĠWright": 25578, "Ġ×Ķת": 25579, "ĠShi": 25580, "ĠãģŁ": 25581, "Ġinvestigations": 25582, "éĤĦæĺ¯": 25583, "ĠPowerPoint": 25584, "ĠChu": 25585, "Ġìĺ¤í": 25586, "ĠìĻĦìłĦ": 25587, "ĠFragen": 25588, "unning": 25589, "Ġpourrait": 25590, "Ġtextbook": 25591, "мÑĭ": 25592, "Ġfahren": 25593, "ĠÑĤоÑĢ": 25594, "Ġlakes": 25595, "ünde": 25596, "Int": 25597, "ĠMetro": 25598, "Ġmansion": 25599, "Ġаб": 25600, "ĠZhou": 25601, "Ġcorridor": 25602, "Ġescol": 25603, "Ġindicating": 25604, "iaÅĤa": 25605, "Ġmommy": 25606, "Ġarchives": 25607, "Ġfounders": 25608, "engine": 25609, "ĠDieu": 25610, "Ġsickness": 25611, "Ġë³´ëĭĪê¹Į": 25612, "Ġarb": 25613, "Ġned": 25614, "ĠChop": 25615, "Ġcovid": 25616, "Ġslam": 25617, "Ġpublications": 25618, "DC": 25619, "Ġspends": 25620, "æ¾": 25621, "Ġrefugee": 25622, "Ġdile": 25623, "Ġ×IJ×ĸ": 25624, "ificar": 25625, "ĠSach": 25626, "Gu": 25627, "Ġreload": 25628, "????": 25629, "ĠjeÅĽli": 25630, "ĠÑģоÑģÑĤо": 25631, "Ġsimplicity": 25632, "Ġbullying": 25633, "Ġмол": 25634, "Ġrealidad": 25635, "Ġunclear": 25636, "appa": 25637, "levant": 25638, "ĠISIS": 25639, "ĠWatson": 25640, "Ġdein": 25641, "ĠMicro": 25642, "íķľë": 25643, "üg": 25644, "Ġdevam": 25645, "Ġtweeted": 25646, "å°İ": 25647, "Ġunderstandable": 25648, "atan": 25649, "Ġversa": 25650, "Ġpreca": 25651, "Ġvá»ģ": 25652, "ĠCopy": 25653, "ĠOracle": 25654, "Ġmindfulness": 25655, "Ġdiscret": 25656, "ernen": 25657, "ĠPle": 25658, "Have": 25659, "Ġisolate": 25660, "Ġdeu": 25661, "Ġseventy": 25662, "ĠHills": 25663, "Ġarcade": 25664, "ĠÑģпеÑĨи": 25665, "Ġsiguiente": 25666, "ĠBÃľNDNIS": 25667, "liga": 25668, "ĠвÑģÑĤÑĢеÑĩ": 25669, "ôm": 25670, "Ġtweets": 25671, "Ġschauen": 25672, "Ġcritique": 25673, "ĠðŁİµ": 25674, "Ġstatt": 25675, "ĠÑģамое": 25676, "ância": 25677, "Ġsupernatural": 25678, "Ġplugged": 25679, "Fl": 25680, "ynı": 25681, "ĠTambién": 25682, "Ġencouragement": 25683, "ĠServer": 25684, "ëĤľ": 25685, "upa": 25686, "Ġaston": 25687, "Ġhears": 25688, "ÑĢаÑħ": 25689, "Ġsche": 25690, "Ġrats": 25691, "Ġrecuper": 25692, "Ġunten": 25693, "ĠFighting": 25694, "Ġacademics": 25695, "示": 25696, "ĠSü": 25697, "ÑģкиÑħ": 25698, "Ġpaired": 25699, "ĢìĿĦ": 25700, "Ġárea": 25701, "Ġsweetness": 25702, "åıĬ": 25703, "Ġdefer": 25704, "Ġmuitas": 25705, "ĠAudio": 25706, "Ġlocker": 25707, "ÙĬد": 25708, "ĠÑģÑĤав": 25709, "Ġbuena": 25710, "ANS": 25711, "Ġdetector": 25712, "avo": 25713, "bek": 25714, "Ġαν": 25715, "íݸ": 25716, "Ġdragged": 25717, "Ġдолжен": 25718, "Ãĸ": 25719, "رة": 25720, "ìĿ´ì§Ģ": 25721, "Ġcelle": 25722, "cking": 25723, "ĠاÙĦج": 25724, "ĠCanvas": 25725, "Ġespañ": 25726, "Ġglimp": 25727, "Ġspreads": 25728, "ongo": 25729, "ĠMason": 25730, "ĠIng": 25731, "Ġê°ĢëĬ¥": 25732, "ÏĦικ": 25733, "Ġsecular": 25734, "Ġbater": 25735, "Ġinquiry": 25736, "Ġenergies": 25737, "Ġmanufactured": 25738, "Ġvegetarian": 25739, "Ġpineapple": 25740, "ÑıÑĤа": 25741, "Ġpractitioners": 25742, "2000": 25743, "Ġíķ´ìļĶ": 25744, "ĠìŬ룬ë¶Ħëĵ¤": 25745, "Ġë¶Īë": 25746, "ĠJefferson": 25747, "ĠJoan": 25748, "Ġtram": 25749, "容": 25750, "chmal": 25751, "ĠHait": 25752, "á¹ĩ": 25753, "Ġunreal": 25754, "Ġsymbolic": 25755, "Ġstealth": 25756, "Ġsplash": 25757, "ĠEntertainment": 25758, "Ġmetallic": 25759, "?\".": 25760, "è¶Ĭ": 25761, "around": 25762, "Ġdespair": 25763, "ĠNevada": 25764, "ĠFinance": 25765, "Ġkrie": 25766, "ĠLux": 25767, "ĠSmash": 25768, "keeping": 25769, "Ġзаг": 25770, "Ġnarciss": 25771, "Ġdzisiaj": 25772, "Ġtolerate": 25773, "oard": 25774, "Ġlinking": 25775, "ĠEconomic": 25776, "Ġì¼": 25777, "Ġmorph": 25778, "ĠNak": 25779, "ĠBaker": 25780, "aton": 25781, "rings": 25782, "ĠPeng": 25783, "ĠAirport": 25784, "ãģĭãģ£ãģŁ": 25785, "íķĺëĭ¤": 25786, "§ģ": 25787, "prints": 25788, "Ġhadi": 25789, "Ġempir": 25790, "ĠLives": 25791, "anners": 25792, "Ġним": 25793, "ĠPROFESSOR": 25794, "Ġpositively": 25795, "antom": 25796, "Ġbadge": 25797, "kelt": 25798, "Ġinterfer": 25799, "Ġfulfilling": 25800, "Ġvisualization": 25801, "éĹľä¿Ĥ": 25802, "ĠPrice": 25803, "��": 25804, "Ġscenery": 25805, "Ġprone": 25806, "Ġwizard": 25807, "Ġbanyak": 25808, "verb": 25809, "sky": 25810, "Ġwished": 25811, "Ġrailway": 25812, "Ġüzer": 25813, "Ġalguien": 25814, "ĠAW": 25815, "ĠколиÑĩе": 25816, "Ġreacting": 25817, "ĠBuch": 25818, "ึ": 25819, "Ġanth": 25820, "Ġsih": 25821, "Ġhust": 25822, "ĠScreen": 25823, "ilant": 25824, "aho": 25825, "Ġfragrance": 25826, "Ġelevation": 25827, "ĠMediter": 25828, "Ġë¿": 25829, "Ġéqu": 25830, "Ġwraps": 25831, "Ġinert": 25832, "Ġrecreate": 25833, "лаÑĤ": 25834, "Ġboleh": 25835, "Ġharassment": 25836, "unky": 25837, "Ġglimpse": 25838, "regierung": 25839, "Ġfutur": 25840, "Ġrepository": 25841, "Ġengra": 25842, "Ġtrafficking": 25843, "assis": 25844, "ĠTrek": 25845, "Ġë²Į": 25846, "Ġë§Īë": 25847, "ĠKab": 25848, "aniu": 25849, "give": 25850, "Ġdinosaurs": 25851, "Ġfeather": 25852, "Ġattitudes": 25853, "Ġplum": 25854, "ĠRS": 25855, "ĠAnfang": 25856, "illery": 25857, "ĠìĬ¤": 25858, "MY": 25859, "Ġtrzeba": 25860, "Ġskies": 25861, "ĠAj": 25862, "urable": 25863, "CU": 25864, "ĠShane": 25865, "Ġdeparture": 25866, "ĠTON": 25867, "ieten": 25868, "rats": 25869, "æ°Ĺ": 25870, "isu": 25871, "Ġbord": 25872, "Ġinterestingly": 25873, "çĻ»": 25874, "oughing": 25875, "Ġrushing": 25876, "Ġvolatility": 25877, "Ġpyt": 25878, "Ġformats": 25879, "ĠзаÑĤ": 25880, "Ġê¼Ń": 25881, "Ġwhatnot": 25882, "Ġcomport": 25883, "sw": 25884, "orean": 25885, "ĠRelax": 25886, "Ġclan": 25887, "ĠAH": 25888, "Ġpew": 25889, "Ġdictionary": 25890, "Take": 25891, "shirts": 25892, "ĠHugh": 25893, "ĠعÙĦÙĬ": 25894, "ĠPic": 25895, "Ġenrolled": 25896, "Ġjednak": 25897, "Ġofferings": 25898, "Ġcoraz": 25899, "Life": 25900, "Ġ!!!": 25901, "Ġcler": 25902, "ĠVideos": 25903, "ĠRodrig": 25904, "ĠIdent": 25905, "ĠPos": 25906, "ĠStage": 25907, "ĠRace": 25908, "Ġenact": 25909, "ãģĦãģ¾ãģĹãģŁ": 25910, "ĠGy": 25911, "ĠHispan": 25912, "Ġdefence": 25913, "ĠCampbell": 25914, "matic": 25915, "Ġrelev": 25916, "Ġpeach": 25917, "Ħ¸ìļĶ": 25918, "Ġparadise": 25919, "Ġceremon": 25920, "Ġannoyed": 25921, "æĮĩ": 25922, "lax": 25923, "Ġexploit": 25924, "Ġclause": 25925, "eker": 25926, "ĠBloom": 25927, "nant": 25928, "ateurs": 25929, "Ġheights": 25930, "Even": 25931, "Ñģон": 25932, "Ġoutrage": 25933, "ĠVietnamese": 25934, "ãģ¯ãģ¯": 25935, "TR": 25936, "Ġeer": 25937, "Ġcannon": 25938, "ĠComb": 25939, "IJë§Į": 25940, "è»Ĭ": 25941, "Ġê²ĥëıĦ": 25942, "Ġaccomplishments": 25943, "ĠAnalytics": 25944, "Ġshaping": 25945, "reiben": 25946, "Ġbachelor": 25947, "Ġfingert": 25948, "acked": 25949, "Ġpyramid": 25950, "ĠStewart": 25951, "ást": 25952, "Ġsurvivor": 25953, "Ġduct": 25954, "Ġdealers": 25955, "æ´»": 25956, "عÙħ": 25957, "лин": 25958, "Ġede": 25959, "×ķ×¢": 25960, "ĠÙĥاÙĨ": 25961, "ĠÏĦι": 25962, "Ġchooses": 25963, "ĠOwn": 25964, "гоÑĤов": 25965, "hire": 25966, "алÑĮнÑĭе": 25967, "ĠÐĽÑİ": 25968, "ĠоÑģÑĤав": 25969, "tech": 25970, "Ġdroit": 25971, "Ġsubjective": 25972, "enes": 25973, "Ġdivis": 25974, "avez": 25975, "Ġmaneuver": 25976, "à¹Ħà¸Ķ": 25977, "adece": 25978, "ĠEns": 25979, "acial": 25980, "ĠProtection": 25981, "ĸ´": 25982, "Ġformally": 25983, "Ġwyd": 25984, "inguém": 25985, "Ġziem": 25986, "Ġrecruiting": 25987, "×Ļ×ļ": 25988, "nem": 25989, "Ġforbidden": 25990, "ĠBapt": 25991, "×IJ׳×Ļ": 25992, "Ġsubset": 25993, "ĠMagaz": 25994, "nement": 25995, "Ġaquela": 25996, "ragon": 25997, "Ġcommittees": 25998, "Ġétaient": 25999, "udi": 26000, "ĠDawn": 26001, "Ġbore": 26002, "Ġcomposer": 26003, "ĠwiÄĻcej": 26004, "anga": 26005, "Ġdislike": 26006, "ĠDays": 26007, "åŁº": 26008, "Ġparal": 26009, "Ġmientras": 26010, "Ġheavens": 26011, "ãģĴ": 26012, "heid": 26013, "Ġtraders": 26014, "once": 26015, "Ġmascara": 26016, "ĠÏĢÏģο": 26017, "Ġwhisper": 26018, "ĠMusk": 26019, "éĽĨ": 26020, "ĠFamilie": 26021, "Allah": 26022, "ĠOlivia": 26023, "ĠPros": 26024, "Ġolika": 26025, "ilim": 26026, "Ġrépond": 26027, "ĠPeters": 26028, "Ġå¾Ī": 26029, "Ġbites": 26030, "Ġvic": 26031, "ĠNY": 26032, "emption": 26033, "Ġ450": 26034, "Ġvisuals": 26035, "Ġlieu": 26036, "ücken": 26037, "ĠSteel": 26038, "ĠGP": 26039, "wait": 26040, "Ġnoticeable": 26041, "ucha": 26042, "Ġrehabil": 26043, "Ġrejection": 26044, "ĠÑģледÑĥÑİÑī": 26045, "Ġslider": 26046, "Ġregarded": 26047, "Ġgravit": 26048, "ĠReserve": 26049, "count": 26050, "Ġbreeding": 26051, "Ġlonge": 26052, "aleb": 26053, "Ġknight": 26054, "Ġвой": 26055, "Ġprésent": 26056, "ĤĺìļĶ": 26057, "ĠSpecifically": 26058, "Ġposes": 26059, "Ġveure": 26060, "okay": 26061, "emas": 26062, "Ġãģ§ãģĻ": 26063, "ĠmajÄħ": 26064, "Ġwebinars": 26065, "Ġcannabis": 26066, "Ġdamals": 26067, "ĠNorthwest": 26068, "Ġpada": 26069, "Ġcrowds": 26070, "Ġfutures": 26071, "Ġän": 26072, "Ġcivilians": 26073, "ĠSachen": 26074, "æį": 26075, "Ġtraces": 26076, "Ġë¨¹ê³ł": 26077, "QU": 26078, "é¡ĺãģĦ": 26079, "ĠIF": 26080, "anın": 26081, "ìĤ´": 26082, "Ġbiblical": 26083, "ĠVed": 26084, "Ġstoring": 26085, "ÑĢавлÑı": 26086, "æĩī該": 26087, "Ġnast": 26088, "Ġdö": 26089, "ÑĢоп": 26090, "elia": 26091, "Ġsideways": 26092, "ĠUnderstand": 26093, "ĠQur": 26094, "Ġperpend": 26095, "ĠMillionen": 26096, "Ġwatermelon": 26097, "ĠDivine": 26098, "ultur": 26099, "abord": 26100, "Ġsuccesses": 26101, "Ġhombre": 26102, "Ġcarp": 26103, "Ġsuscept": 26104, "ungkin": 26105, "Ġkij": 26106, "ulus": 26107, "اج": 26108, "Ġnotch": 26109, "Ġpolynomial": 26110, "å¹²": 26111, "å©": 26112, "Ġúnico": 26113, "Ġtelescope": 26114, "Ġpolitique": 26115, "kiem": 26116, "ĠÎŃνα": 26117, "Ġaggregate": 26118, "ĠGeoff": 26119, "Ġtril": 26120, "ĠGRA": 26121, "Ġsubscriber": 26122, "imet": 26123, "ĠдоллаÑĢ": 26124, "oping": 26125, "Ġtherapeut": 26126, "ĠCancer": 26127, "Ġparade": 26128, "Ġirrig": 26129, "âĻªâĻª": 26130, "Ġclearer": 26131, "Ġbog": 26132, "ĠMaur": 26133, "าà¸ĩ": 26134, "ĠShanghai": 26135, "achte": 26136, "ĠKol": 26137, "elujah": 26138, "Ġhav": 26139, "ĠCrime": 26140, "sek": 26141, "Ġë¡ľ": 26142, "ienna": 26143, "ĠGor": 26144, "èĽ": 26145, "ĠпоÑĤÑĢ": 26146, "ĠкажеÑĤÑģÑı": 26147, "ĠLift": 26148, "ĠSort": 26149, "ĠPsal": 26150, "Ġping": 26151, "ĵĿ": 26152, "phis": 26153, "ĠFUCK": 26154, "ĠSyn": 26155, "Ġbamboo": 26156, "¬ìĺģ": 26157, "cuts": 26158, "Ġmmm": 26159, "Ġfunktioniert": 26160, "Ġ_": 26161, "ÃŃcio": 26162, "Stop": 26163, "Ġimaginary": 26164, "Ġnotamment": 26165, "ĠInitiative": 26166, "ãĥ¥": 26167, "ĠKurt": 26168, "Ġloosen": 26169, "Ġbuscar": 26170, "çģ«": 26171, "Ġzelf": 26172, "Ġprops": 26173, "åĽī": 26174, "Ġmoeten": 26175, "Ġmilli": 26176, "Ġhalls": 26177, "ĠMatch": 26178, "Ġbrackets": 26179, "ĠCou": 26180, "æ¦Ĥ": 26181, "ĠÐľÐ°ÑĢ": 26182, "ISA": 26183, "Ġcigarette": 26184, "Ġcompetitions": 26185, "ĠMIN": 26186, "Ġbehö": 26187, "voor": 26188, "Ġust": 26189, "ĠZi": 26190, "ĠOcc": 26191, "ulates": 26192, "Ġballoons": 26193, "Ġpronto": 26194, "ĠMiy": 26195, "ĠFile": 26196, "ĠклаÑģÑģ": 26197, "нÑĥл": 26198, "Ġcereal": 26199, "Ġincrement": 26200, "Ġrefined": 26201, "åı¦å¤ĸ": 26202, "prising": 26203, "ĠRF": 26204, "Ġrespectful": 26205, "Ġloot": 26206, "asket": 26207, "Ġdeixa": 26208, "ingle": 26209, "Ġfunciona": 26210, "ĠRevel": 26211, "Ġsober": 26212, "Ġperforms": 26213, "ĠGentle": 26214, "ãĤ¨": 26215, "Ġrecipient": 26216, "ĠHause": 26217, "Ġëĥ": 26218, "From": 26219, "Ġministers": 26220, "Ġparadox": 26221, "å°±æĺ¯èªª": 26222, "Ġtasting": 26223, "Ġ×Ķ×Ĺ": 26224, "Ġreuse": 26225, "ĠLane": 26226, "ĠÑģовеÑĢÑĪ": 26227, "Ġremembers": 26228, "Ġfeminist": 26229, "Ġcommitments": 26230, "Ġprojected": 26231, "Ġgaz": 26232, "iyoruz": 26233, "Ġobligations": 26234, "Ro": 26235, "zar": 26236, "Ġchw": 26237, "ĠJAM": 26238, "ĠbÄĻdÄħ": 26239, "aspberry": 26240, "ĠмеÑģÑĤо": 26241, "ë²ķ": 26242, "Ġregulated": 26243, "Ġwicht": 26244, "ĠTrevor": 26245, "Ġsecondly": 26246, "ĠIhre": 26247, "elsh": 26248, "Ġreporters": 26249, "ÑĤоÑĢа": 26250, "oyo": 26251, "GI": 26252, "Ġinterconnect": 26253, "éIJĺ": 26254, "OSH": 26255, "æŃ²": 26256, "Ġbrass": 26257, "Ġignoring": 26258, "ä»ĬæĹ¥": 26259, "infect": 26260, "Ġprojekt": 26261, "oret": 26262, "ÏĦαν": 26263, "ĠÑĤип": 26264, "Ġmutta": 26265, "Ġunboxing": 26266, "Ħ°": 26267, "å¡Ĭ": 26268, "Ġadvised": 26269, "ĠDenver": 26270, "Ġseverely": 26271, "ĠMhm": 26272, "Ġflipped": 26273, "Ġpien": 26274, "Ġkommun": 26275, "ĠFRE": 26276, "Ġà®ĩà®°": 26277, "ainted": 26278, "Ġknives": 26279, "Ġhabl": 26280, "Ġgeworden": 26281, "arettes": 26282, "CS": 26283, "ĠмаленÑĮ": 26284, "Ġgalax": 26285, "Ġninete": 26286, "ê±°ëĤĺ": 26287, "Ġsis": 26288, "Ġadvisory": 26289, "Ġdrilling": 26290, "ĠWouldn": 26291, "ünf": 26292, "gestellt": 26293, "ĠHelen": 26294, "Ġ×ŀ×IJ": 26295, "apolis": 26296, "Ġrzeczy": 26297, "Ġterra": 26298, "Ġhep": 26299, "Ġalgún": 26300, "ikk": 26301, "Ġastronom": 26302, "ĠStarbucks": 26303, "kÄħ": 26304, "Ġpatrol": 26305, "Ġì½Ķ": 26306, "Ġgon": 26307, "ĠãĢIJ": 26308, "Ġsonst": 26309, "Ġencounters": 26310, "Ġretrou": 26311, "Ġsharks": 26312, "Ġdor": 26313, "ĠRever": 26314, "Ġevapor": 26315, "Ġreservoir": 26316, "Ġalleged": 26317, "uler": 26318, "Ġverm": 26319, "Ġcommerce": 26320, "Ġfitted": 26321, "gem": 26322, "Ġtactical": 26323, "Ġlith": 26324, "éīĦå¡Ķ": 26325, "had": 26326, "è®Ĭ": 26327, "Ġcarbohyd": 26328, "Ġlengths": 26329, "ιο": 26330, "Ġdemographic": 26331, "Rob": 26332, "ĠSkin": 26333, "ccoli": 26334, "Ġsimplified": 26335, "Ġreadily": 26336, "ĠCum": 26337, "adesh": 26338, "ĠDÃ¥": 26339, "usst": 26340, "igne": 26341, "eton": 26342, "Ġmenor": 26343, "qi": 26344, "OOM": 26345, "à¸Ńà¸Ļ": 26346, "Ġpsychiat": 26347, "Ġeighty": 26348, "Ġмилли": 26349, "ĠTob": 26350, "edo": 26351, "ç¶²": 26352, "ĠÄijến": 26353, "Ġcircuits": 26354, "ĠLAUGH": 26355, "icism": 26356, "emor": 26357, "Ġregener": 26358, "egree": 26359, "Ġbureauc": 26360, "ĠAlber": 26361, "ä¹ĭå¾Į": 26362, "ĠWor": 26363, "夫": 26364, "Ġresin": 26365, "ĠbyÅĤy": 26366, "ĠIG": 26367, "à¯į,": 26368, "Ġ78": 26369, "Ġweeds": 26370, "ĠMyth": 26371, "93": 26372, "æ¿": 26373, "ĠëĤĺìĻĶ": 26374, "év": 26375, "á½": 26376, "ören": 26377, "çar": 26378, "ĠPAUL": 26379, "Ġdisadvant": 26380, "Ġpositioning": 26381, "Ġcocktail": 26382, "Ġagrees": 26383, "nn": 26384, "ĠSally": 26385, "Ms": 26386, "Ġinherent": 26387, "Ġmonetary": 26388, "Ġnatur": 26389, "ĠNh": 26390, "ĠImport": 26391, "Ġleben": 26392, "Ġwi": 26393, "ussy": 26394, "Ġobes": 26395, "Ġwandering": 26396, "Ġìĭłë": 26397, "Äħda": 26398, "etchup": 26399, "Ġdisposal": 26400, "ĠJA": 26401, "ĠCer": 26402, "zilla": 26403, "Ġvirgin": 26404, "ĠSlide": 26405, "andel": 26406, "Ġrighteousness": 26407, "ĠΣ": 26408, "Ġideia": 26409, "ä½łå¥½": 26410, "иÑĢоваÑĤÑĮ": 26411, "ר×IJ": 26412, "Comment": 26413, "Ġprelim": 26414, "ĠVale": 26415, "Ġì§ĢëĤľ": 26416, "ĠVanc": 26417, "OMAN": 26418, "ĠпÑĸд": 26419, "Ġyum": 26420, "stre": 26421, "cem": 26422, "Ġpocz": 26423, "Ġfragment": 26424, "ĠÑģлÑĥÑĩае": 26425, "Ġundergo": 26426, "ĠHank": 26427, "ceks": 26428, "ĠFPS": 26429, "Ġocur": 26430, "Ġdeterior": 26431, "注": 26432, "Ġempresas": 26433, "Paul": 26434, "Ġ)))": 26435, "ĠвÑĢемени": 26436, "Ġscold": 26437, "×Ļ×¢": 26438, "Ġsuspected": 26439, "Ġaccessing": 26440, "Ġsubstit": 26441, "Ġhistorians": 26442, "ä»»": 26443, "Ġдело": 26444, "Ġsocied": 26445, "rone": 26446, "Ġreden": 26447, "Ġextends": 26448, "epherd": 26449, "Ġbalcon": 26450, "ä¸įèµ·": 26451, "ĠSolo": 26452, "Ġpolitician": 26453, "олÑĮно": 26454, "Ġirgendw": 26455, "Ġtraumatic": 26456, "Ġrapper": 26457, "ĠROBERT": 26458, "Really": 26459, "æģ¯": 26460, "Ġlineup": 26461, "ASE": 26462, "Ġcontractor": 26463, "ĠCorporation": 26464, "gor": 26465, "ĠTodo": 26466, "ÑģÑĤÑĢой": 26467, "FBE": 26468, "Ġnewsletter": 26469, "ĠkoÅĦ": 26470, "alties": 26471, "ĠпÑĢиÑĩ": 26472, "ĠHeavy": 26473, "Ġswords": 26474, "Ġmanipulation": 26475, "Ġfunk": 26476, "ĠvÃ¥r": 26477, "ĠTaliban": 26478, "Ġë°¥": 26479, "Ġacne": 26480, "ürü": 26481, "Ġdeswegen": 26482, "ĠDust": 26483, "Ġsilic": 26484, "Ġhooks": 26485, "Ġblij": 26486, "Ġpetits": 26487, "Ġfilme": 26488, "ĠBereich": 26489, "ĠSaid": 26490, "Ġimposed": 26491, "Ġdiary": 26492, "ĠгоÑĢ": 26493, "ĠGates": 26494, "Ġalta": 26495, "å¸Į": 26496, "Ġchcia": 26497, "pleasant": 26498, "Ġë°Ŀ": 26499, "Ġmożemy": 26500, "ĠAustria": 26501, "Ġbroker": 26502, "Ġsucked": 26503, "èĢĥ": 26504, "Ġcompartment": 26505, "Ġclone": 26506, "Ġ×Ķ×¢": 26507, "ĠDanke": 26508, "Ġnochmal": 26509, "езд": 26510, "Ġadrenal": 26511, "Ġkleinen": 26512, "ãģ¾ãģĹãĤĩãģĨ": 26513, "Ġsubsequently": 26514, "Ġdecentral": 26515, "Ġgenetics": 26516, "Ġê´ij": 26517, "Ġmonitors": 26518, "ĠApplic": 26519, "ĠReporter": 26520, "wert": 26521, "Ġwiem": 26522, "ĠMovement": 26523, "Ġinterviewing": 26524, "Ġhairs": 26525, "Ġpuò": 26526, "ĠChelsea": 26527, "Ġcoher": 26528, "Ġcot": 26529, "Ġzas": 26530, "Ġpatches": 26531, "Ġlah": 26532, "Ñĥнк": 26533, "ĠReagan": 26534, "ĠMarco": 26535, "city": 26536, "Ġdefender": 26537, "Ġdecoration": 26538, "iji": 26539, "Ġlitter": 26540, "Ш": 26541, "Ġjego": 26542, "REW": 26543, "ĠPik": 26544, "ĠHee": 26545, "ĠIv": 26546, "Ġиде": 26547, "ĠTheater": 26548, "ĠÑĩаÑģÑĤо": 26549, "Ġsweater": 26550, "Ġhighlighting": 26551, "Ġainsi": 26552, "Ġdiplomatic": 26553, "ĠNevertheless": 26554, "å³": 26555, "ASON": 26556, "Ġpúblico": 26557, "Ġferm": 26558, "reated": 26559, "cod": 26560, "Ġ물ë": 26561, "Ġmister": 26562, "ĠVancouver": 26563, "Ġrecognizes": 26564, "ecd": 26565, "Ġcomplications": 26566, "encial": 26567, "ãģĹãģı": 26568, "Ġê°Ģì§Ģ": 26569, "ĠUltimate": 26570, "Ġvaig": 26571, "ĠMerry": 26572, "×ķ×Ĵ": 26573, "ĠMarcus": 26574, "總": 26575, "owego": 26576, "Ġmente": 26577, "Sm": 26578, "Ġaja": 26579, "ĠTao": 26580, "Ġjudicial": 26581, "Ġentrepreneurship": 26582, "Ġнемного": 26583, "Ġpis": 26584, "Ġerg": 26585, "Ġchrist": 26586, "ĠCurt": 26587, "ĠÑĢаÑģп": 26588, "λε": 26589, "ensch": 26590, "ÃŃre": 26591, "Ġfocal": 26592, "ĠDiamond": 26593, "avÃŃa": 26594, "Ġhanno": 26595, "ĠSquad": 26596, "Ġassociations": 26597, "ĠCreative": 26598, "Ġmessenger": 26599, "Ġbegging": 26600, "Ġdecimal": 26601, "ĠdÄ±ÅŁ": 26602, "Ġmetadata": 26603, "sels": 26604, "ĠÄ°ÅŁ": 26605, "ữa": 26606, "Ġdifficile": 26607, "dı": 26608, "Ġslaughter": 26609, "ĠVerg": 26610, "Ġ×Ĵ×Ŀ": 26611, "ç°¡": 26612, "æĮī": 26613, "ĠTea": 26614, "asses": 26615, "Ok": 26616, "Ġsynthes": 26617, "otiation": 26618, "Ġpainter": 26619, "Ġelbows": 26620, "Ġarchitectural": 26621, "ĠÑĢад": 26622, "Ġglor": 26623, "image": 26624, "ampa": 26625, "culiar": 26626, "ł¨": 26627, "Ġteve": 26628, "ĠStelle": 26629, "ĠBam": 26630, "Ġì´Ī": 26631, "asis": 26632, "ipedia": 26633, "ĠGI": 26634, "ĠActive": 26635, "çĦ¶åIJİ": 26636, "azi": 26637, "ãĤĮãģ¦": 26638, "ĠLucky": 26639, "íķ©": 26640, "ĠпÑĢиÑħод": 26641, "Ġrunway": 26642, "Ġauthentication": 26643, "Ġposible": 26644, "Ġsupplements": 26645, "Ġsurgical": 26646, "Gen": 26647, "Ġfeasible": 26648, "DO": 26649, "Ġoutlook": 26650, "Ġintervals": 26651, "Ġanecd": 26652, "Ãłng": 26653, "Ġstraps": 26654, "ĠShu": 26655, "udd": 26656, "issenschaft": 26657, "Ġporte": 26658, "Ġcommitting": 26659, "Ġalley": 26660, "Ġcovenant": 26661, "ĠPedro": 26662, "lessness": 26663, "ĠSolid": 26664, "ĠMolly": 26665, "ĠнекоÑĤоÑĢ": 26666, "Ġcooperate": 26667, "åĮĹ": 26668, "ollen": 26669, "Ġtuna": 26670, "Ġkindergarten": 26671, "ĠSiz": 26672, "Ġdużo": 26673, "ĠMBA": 26674, "ĠGEORGE": 26675, "ĠFisher": 26676, "å¿ĺ": 26677, "ĠCaesar": 26678, "ĠкÑĢаÑģив": 26679, "ĠDelhi": 26680, "zym": 26681, "Ġexplicar": 26682, "ê°Ģì§Ģ": 26683, "uns": 26684, "grow": 26685, "ĠпÑĢиÑģ": 26686, "Ġ86": 26687, "Ġstating": 26688, "Ġmassa": 26689, "chter": 26690, "Ġì»¬ëŁ¬": 26691, "Ġdeputy": 26692, "SM": 26693, "noc": 26694, "Ġgeography": 26695, "ĠEnterprise": 26696, "ĠCant": 26697, "öz": 26698, "Ġunpack": 26699, "ĠíĻĶë": 26700, "Ġsearches": 26701, "Ġpresidency": 26702, "Ġtrivial": 26703, "Ġpige": 26704, "oubt": 26705, "ãĤļ": 26706, "ì¼ĢìĿ´": 26707, "Ġbudgets": 26708, "Ġub": 26709, "Ġpne": 26710, "ĠYale": 26711, "ĠÅŁÃ¶yle": 26712, "regular": 26713, "Ġimperfect": 26714, "ARA": 26715, "ĠfamÃŃlia": 26716, "urm": 26717, "ĠAdventure": 26718, "ãĥĬ": 26719, "cis": 26720, "emark": 26721, "Ġnego": 26722, "Ġinappropriate": 26723, "ĠпÑĢиз": 26724, "ĠÑĢол": 26725, "Ġdreamed": 26726, "Bry": 26727, "Ġshuttle": 26728, "Ġpillars": 26729, "Ġbik": 26730, "inum": 26731, "ĠÑĥÑģ": 26732, "ĠNebr": 26733, "Ġperpendicular": 26734, "Ġbooked": 26735, "bery": 26736, "Ġvikt": 26737, "bear": 26738, "esus": 26739, "Ġвозможно": 26740, "¨¹": 26741, "Ġpresumably": 26742, "ĠMemphis": 26743, "Ġambulance": 26744, "×ķ×ŀר": 26745, "Ġthumbnail": 26746, "Ġmodification": 26747, "éĩı": 26748, "Ġinterpreted": 26749, "Ġpromo": 26750, "Ġκά": 26751, "ĠεÏĢ": 26752, "Ġacoustic": 26753, "ĠDB": 26754, "åĵİ": 26755, "Ġnonetheless": 26756, "oule": 26757, "Ġpequ": 26758, "Ġknob": 26759, "ãĤ£": 26760, "ĠëıĮìķĦ": 26761, "Ġpurchases": 26762, "ĠÃĩünkü": 26763, "Ġdividing": 26764, "perform": 26765, "raction": 26766, "healthy": 26767, "ĠTitle": 26768, "Ġuk": 26769, "Ġcerca": 26770, "Ġarguably": 26771, "Ġfale": 26772, "ë³µ": 26773, "Ġgamers": 26774, "Ġutilizing": 26775, "Ġoffended": 26776, "Ġtava": 26777, "alı": 26778, "Ġmedian": 26779, "Ġinfectious": 26780, "ĠAnnie": 26781, "Ġsmartphones": 26782, "Ġparole": 26783, "åĸĿ": 26784, "ĠEpic": 26785, "zza": 26786, "Ġunified": 26787, "Ġê·¸ëķĮ": 26788, "Ġcurtain": 26789, "ĠÄĥ": 26790, "Ġsexually": 26791, "Ġunserem": 26792, "ĠConvention": 26793, "Ġallegedly": 26794, "Ya": 26795, "ĠHoo": 26796, "enment": 26797, "æĢª": 26798, "íĽĦ": 26799, "Ġgigantic": 26800, "Ġnoting": 26801, "Ġrebo": 26802, "ĠJama": 26803, "ĠAlz": 26804, "Ġborrowed": 26805, "침": 26806, "Ġperipher": 26807, "оÑĤа": 26808, "ĠGB": 26809, "ĠGear": 26810, "Ġeconomically": 26811, "Ġtelefon": 26812, "Ġqueremos": 26813, "ĠдалÑĮÑĪе": 26814, "Ġras": 26815, "ĠTeach": 26816, "icios": 26817, "atos": 26818, "Ġpledge": 26819, "bau": 26820, "ĠHimself": 26821, "Link": 26822, "Ġespero": 26823, "Ġchromos": 26824, "ĠPER": 26825, "Ġerle": 26826, "Ġpodium": 26827, "ços": 26828, "Ġnieu": 26829, "Ġfen": 26830, "ĠGOD": 26831, "ĠChocolate": 26832, "werk": 26833, "Ġtừ": 26834, "Ġsuppress": 26835, "λη": 26836, "Ġ240": 26837, "Ġsitä": 26838, "Ġhonesty": 26839, "ĠBio": 26840, "ĠBard": 26841, "ĠобÑīем": 26842, "ĠмÑĥз": 26843, "Ġmarble": 26844, "ĠÑĨенÑĤ": 26845, "Ġprocure": 26846, "Ġrotor": 26847, "bern": 26848, "Ġtuh": 26849, "Ġheadset": 26850, "atem": 26851, "Ġwarranty": 26852, "à®´": 26853, "Ġfiling": 26854, "ιά": 26855, "Ġcomprendre": 26856, "Ġimpulse": 26857, "Ġsalv": 26858, "written": 26859, "Ġinstitute": 26860, "Kim": 26861, "ĠLGBTQ": 26862, "ficiente": 26863, "His": 26864, "ĠαÏħÏĦÏĮ": 26865, "Ġteenage": 26866, "orus": 26867, "ĠÑĢазб": 26868, "See": 26869, "ĠConserv": 26870, "á»ģn": 26871, "fulness": 26872, "Ġstrawberries": 26873, "ĠAbu": 26874, "ион": 26875, "Ġolla": 26876, "NOISE": 26877, "ĠEmploy": 26878, "Ġwiped": 26879, "urger": 26880, "Ġmodifications": 26881, "Ġíķĺì§Ģ": 26882, "Ġfootsteps": 26883, "Ġhonors": 26884, "Ġadul": 26885, "Ġflipping": 26886, "ĠHU": 26887, "ZY": 26888, "Ġintegrating": 26889, "بر": 26890, "ulla": 26891, "Ġnatuurlijk": 26892, "ĠíĹĪ": 26893, "ĠEthereum": 26894, "ÙĬÙĦ": 26895, "wed": 26896, "Ġpeaks": 26897, "ĠKes": 26898, "Ġbloom": 26899, "Ġcrashing": 26900, "Ġ911": 26901, "ĠоÑĤлиÑĩ": 26902, "Ġcontrollers": 26903, "ĠDod": 26904, "ĠвмеÑģÑĤе": 26905, "Ġsortir": 26906, "å¥ĩ": 26907, "ĠStraight": 26908, "ĠGracias": 26909, "Ġgroove": 26910, "Ġtogg": 26911, "Ġìĭ¶ìĿĢ": 26912, "éro": 26913, "Ġoutward": 26914, "ĠWA": 26915, "ĠRocky": 26916, "Ġscam": 26917, "Ġhayat": 26918, "ignty": 26919, "âĦ": 26920, "plings": 26921, "Ġantibiotics": 26922, "Ġä¸Ģ": 26923, "Ġnevertheless": 26924, "jang": 26925, "commerce": 26926, "Ġspoiler": 26927, "Ġglove": 26928, "Ġchatter": 26929, "ĠBY": 26930, "~?": 26931, "Ġíĺ¸": 26932, "Ġdemol": 26933, "wechsel": 26934, "imir": 26935, "Ġraid": 26936, "еÑĢÑħ": 26937, "ìŀIJ기": 26938, "enf": 26939, "Ġcommented": 26940, "Ġoptimized": 26941, "Ġconvicted": 26942, "Ġbats": 26943, "ĠSB": 26944, "ĠAur": 26945, "ĠTong": 26946, "Ġimplicit": 26947, "ĠJanet": 26948, "Ġreag": 26949, "ãģ²": 26950, "ĠAdvanced": 26951, "Ġimpose": 26952, "ש×Ķ": 26953, "Ġschemes": 26954, "ougher": 26955, "abolic": 26956, "Ġê±°ì£ł": 26957, "Ġslowing": 26958, "Ġwtedy": 26959, "Ġdestructive": 26960, "ĠопÑĢед": 26961, "Ġlandmark": 26962, "ĠëıĪ": 26963, "ĠWalking": 26964, "ẹ": 26965, "Ġtijd": 26966, "ĠKN": 26967, "ĠQuant": 26968, "ìĺ¤ë": 26969, "ĠкÑĢÑĥ": 26970, "Ġperder": 26971, "Ġnove": 26972, "ände": 26973, "ĠãģĹ": 26974, "bia": 26975, "Ġcustody": 26976, "Ġbiod": 26977, "æĿ±è¥¿": 26978, "Ġdirecting": 26979, "...âĢĭ": 26980, "Ġreloc": 26981, "Ġdemande": 26982, "ãĤĵãģł": 26983, "ĠoÄŁlum": 26984, "Ġодна": 26985, "ĠMilk": 26986, "åı·": 26987, "ĠKra": 26988, "ĠHonda": 26989, "Ġpue": 26990, "Ġelekt": 26991, "Ġbeginners": 26992, "Ġspear": 26993, "ÃŃnh": 26994, "ĠLuft": 26995, "Ġnig": 26996, "ĠSchools": 26997, "Ġforums": 26998, "ĠQin": 26999, "ppo": 27000, "Ġzag": 27001, "ĠЮ": 27002, "Ġtoothp": 27003, "ĠStyle": 27004, "ì´Ī": 27005, "Ġpunct": 27006, "Ġreps": 27007, "ĠAly": 27008, "Ġamendments": 27009, "Ġöz": 27010, "Ġdigits": 27011, "urai": 27012, "Ġchaotic": 27013, "ĠMasters": 27014, "eon": 27015, "ĠCash": 27016, "ĠCuz": 27017, "Ġbedeutet": 27018, "Ġscanning": 27019, "Ġжд": 27020, "неÑĤ": 27021, "Ġcertainty": 27022, "jek": 27023, "Ġdijo": 27024, "ĠClimate": 27025, "Ġrinse": 27026, "Ġkrij": 27027, "veland": 27028, "Ġsoundtrack": 27029, "ĠSafe": 27030, "ĠNova": 27031, "94": 27032, "Ġathe": 27033, "ĠVerb": 27034, "oler": 27035, "ìĿ´ì£ł": 27036, "Ġvin": 27037, "Ġrespiratory": 27038, "ĠStudy": 27039, "ĠCAM": 27040, "Ġavocado": 27041, "ĠZhen": 27042, "Ġlatency": 27043, "Ġfeathers": 27044, "Ġcontar": 27045, "ĠвеÑī": 27046, "Ġfark": 27047, "Ġblended": 27048, "Ġexploded": 27049, "ĠXX": 27050, "ĠBenim": 27051, "Ġalguém": 27052, "istoire": 27053, "Ġconfidential": 27054, "Ġmast": 27055, "Ġì¿": 27056, "geh": 27057, "Ġdisrespect": 27058, "ĠSystems": 27059, "ưa": 27060, "Ed": 27061, "Ġwys": 27062, "Ġexotic": 27063, "Ġglowing": 27064, "ùng": 27065, "ounge": 27066, "èĦ": 27067, "аниз": 27068, "Ġpalav": 27069, "ĠSword": 27070, "Ġgim": 27071, "ĠCrow": 27072, "Ġpotent": 27073, "bish": 27074, "Ġabused": 27075, "ĠJed": 27076, "Ġgambling": 27077, "ĠSpect": 27078, "Ġinvestigators": 27079, "æĻļ": 27080, "Ġratt": 27081, "Ġdob": 27082, "ĠDES": 27083, "hog": 27084, "ĠоÑĤкÑĢÑĭ": 27085, "íĮħ": 27086, "ĠденÑĮги": 27087, "Ġíĺ¹": 27088, "Ġ머리": 27089, "Ġsaturation": 27090, "Ġinherited": 27091, "ĠInnovation": 27092, "ìĹĪëįĺ": 27093, "Ġtangible": 27094, "Ġdepri": 27095, "hed": 27096, "Ġпомог": 27097, "Ġsliced": 27098, "à¥į": 27099, "Ġthế": 27100, "Å¥": 27101, "68": 27102, "Ġcorona": 27103, "Ġgifted": 27104, "Ġsoir": 27105, "Ġhumility": 27106, "ĠìĿ´ê±¸": 27107, "Ġflaws": 27108, "ĠпÑĢакÑĤи": 27109, "Ġkald": 27110, "waż": 27111, "yw": 27112, "ãĤĵãģ§ãģĻ": 27113, "irteen": 27114, "Ġcrochets": 27115, "¦¬ê°Ģ": 27116, "ĠìłĦìĹIJ": 27117, "Ġdese": 27118, "æ¥Ń": 27119, "Ġмаг": 27120, "ĠdziaÅĤ": 27121, "Ġlég": 27122, "changing": 27123, "Ġllev": 27124, "ÅĦsk": 27125, "çĶ»": 27126, "Ġ1984": 27127, "orns": 27128, "ĠWelsh": 27129, "Ġpharmaceutical": 27130, "Ġpumping": 27131, "ĠShaw": 27132, "punk": 27133, "Ġvault": 27134, "Ġkinetic": 27135, "Ġhurricane": 27136, "ĠIncluding": 27137, "ức": 27138, "ĠGrandpa": 27139, "anship": 27140, "é¦Ļ港": 27141, "ĠвÑĭÑħод": 27142, "нож": 27143, "ľł": 27144, "utta": 27145, "Ġê²ģëĭĪëĭ¤": 27146, "Ġbaz": 27147, "ĠпоÑĪ": 27148, "Ġpeculiar": 27149, "zyÄĩ": 27150, "ĠEllie": 27151, "Ġlearns": 27152, "ĠKrishna": 27153, "Ġconsecut": 27154, "Ġempath": 27155, "ĠDin": 27156, "Ġtraded": 27157, "ĠBoris": 27158, "uggage": 27159, "olla": 27160, "Ġназв": 27161, "Ġeternity": 27162, "Ġвп": 27163, "èmes": 27164, "Ġgrapp": 27165, "bé": 27166, "ĠпÑĢедÑģÑĤав": 27167, "ĠFC": 27168, "įëĭĪëĭ¤": 27169, "even": 27170, "ĠNebraska": 27171, "ortune": 27172, "Ġkarena": 27173, "ĠAgent": 27174, "Ġsting": 27175, "ĠPI": 27176, "Ġmunicipal": 27177, "powered": 27178, "Ġconsegue": 27179, "ĠManchester": 27180, "Ġrainy": 27181, "Ġbli": 27182, "Ġkost": 27183, "Ġhalten": 27184, "ĠAhhh": 27185, "insula": 27186, "erting": 27187, "ĠاÙĦÙģ": 27188, "Ġrelacion": 27189, "Ġkomen": 27190, "Ġdome": 27191, "Ġpriests": 27192, "ĠIntrodu": 27193, "rophe": 27194, "shore": 27195, "velt": 27196, "clipse": 27197, "ĠÑĢÑĥÑģ": 27198, "×Ļס": 27199, "Ġsabemos": 27200, "ĠHolland": 27201, "ogi": 27202, "anki": 27203, "ĠMats": 27204, "Ġsmoked": 27205, "ullie": 27206, "Ġeurope": 27207, "ĠдейÑģÑĤвиÑĤелÑĮно": 27208, "Ġbardziej": 27209, "Ġtransforming": 27210, "ĠEz": 27211, "opath": 27212, "Ġìĸ¸ëĭĪ": 27213, "ĠÑģÑĤан": 27214, "ằng": 27215, "ัà¹ī": 27216, "ĠOuch": 27217, "Ġclearance": 27218, "ustain": 27219, "Ġsolidarity": 27220, "Ġproving": 27221, "ĠÐĺн": 27222, "ĠÑģÑĬ": 27223, "Ġprolong": 27224, "адно": 27225, "Ġsos": 27226, "ĠDeal": 27227, "Ġ170": 27228, "mons": 27229, "Ġзем": 27230, "Ġlogged": 27231, "Ġlifelong": 27232, "Ġsensory": 27233, "Ġbehold": 27234, "ĠFAR": 27235, "ètement": 27236, "ĠFederation": 27237, "Ġdodge": 27238, "ĠShir": 27239, "Ġdragons": 27240, "ĠArctic": 27241, "Äħż": 27242, "Åį": 27243, "º": 27244, "Ġdenke": 27245, "ĠpodrÃŃa": 27246, "cole": 27247, "ÑĥлÑĮÑĤаÑĤ": 27248, "Ġsystematic": 27249, "ама": 27250, "chos": 27251, "Ġclinics": 27252, "ĠBS": 27253, "Ġtales": 27254, "usions": 27255, "Ġíά": 27256, "Ġpreservation": 27257, "Ġlore": 27258, "ĠProtest": 27259, "Ỽ": 27260, "å¸Ĥ": 27261, "Ġacknowledged": 27262, "ĠIsaiah": 27263, "ĠëķĮëĬĶ": 27264, "Ġ×ĺ": 27265, "Ġcompetitor": 27266, "Ġadvancing": 27267, "zip": 27268, "Ġtenth": 27269, "ĠLaure": 27270, "Ġhints": 27271, "Ġexercising": 27272, "ŀľë": 27273, "ĠIntelligence": 27274, "uated": 27275, "OUT": 27276, "oped": 27277, "Ġautonomy": 27278, "Ġbranding": 27279, "ĠMediterranean": 27280, "Ñĸк": 27281, "Ġscrewdriver": 27282, "Ġsupre": 27283, "Ġstap": 27284, "Ġjurisdiction": 27285, "ĠSettings": 27286, "Ġforefront": 27287, "ĠFemale": 27288, "comfort": 27289, "Ġmultiplication": 27290, "ĠMurray": 27291, "Ġbob": 27292, "ĠTas": 27293, "Ġtahu": 27294, "Ġonun": 27295, "etter": 27296, "Ġprophets": 27297, "lag": 27298, "Ġrevenues": 27299, "Ġprá": 27300, "Ġuploading": 27301, "Ġmachinery": 27302, "ascal": 27303, "ĠEstá": 27304, "ĠGoth": 27305, "ĠBald": 27306, "ĠSaw": 27307, "Ġstripes": 27308, "ìłij": 27309, "Ġpowin": 27310, "æĹ¥æľ¬": 27311, "Ġhostile": 27312, "Ġdarum": 27313, "Ġprevented": 27314, "ожалÑĥйÑģÑĤа": 27315, "Ġalgunas": 27316, "Ġhopeless": 27317, "Ġznaj": 27318, "Ġreadings": 27319, "Ġcraving": 27320, "tat": 27321, "ĠPig": 27322, "Ġliar": 27323, "çα": 27324, "Ġmultiplayer": 27325, "Ġdale": 27326, "ĠCourse": 27327, "íģ¼": 27328, "ĠKita": 27329, "Ġcustoms": 27330, "Ġresponds": 27331, "endra": 27332, "è¦ĸ": 27333, "Ġmetro": 27334, "Ñģол": 27335, "Ġmitigate": 27336, "Ġoppression": 27337, "ĠæĪijåĢij": 27338, "quinho": 27339, "Ġammo": 27340, "Ġenfer": 27341, "Ġpony": 27342, "Ġounces": 27343, "°Ķ": 27344, "ĠìĪĺê°Ģ": 27345, "Ġdicho": 27346, "ĠDeb": 27347, "Ġwonders": 27348, "ĠRoose": 27349, "Ġprizes": 27350, "ĠALEX": 27351, "Ġthankfully": 27352, "Ġtissues": 27353, "ĠÑĢавно": 27354, "ĠLuna": 27355, "intelligible": 27356, "ĠìϏ": 27357, "ê°ij": 27358, "ĠHeat": 27359, "ĠÑģид": 27360, "ĠQui": 27361, "Ġions": 27362, "Ġaccommodation": 27363, "便": 27364, "ĠKart": 27365, "ienst": 27366, "Ġtarde": 27367, "Ġsoaked": 27368, "ĠCasey": 27369, "Ġì´Ŀ": 27370, "ĠÑĢÑĥб": 27371, "Ġdifferenti": 27372, "Ġleftover": 27373, "Ġexchanges": 27374, "second": 27375, "Ġfirstly": 27376, "Ġbuilder": 27377, "rien": 27378, "Ġdw": 27379, "Ġbouncing": 27380, "?</": 27381, "ĠëĮĢíķ´ìĦľ": 27382, "ĠÑģе": 27383, "ĠMiles": 27384, "ienie": 27385, "ĠподпиÑģ": 27386, "Ġ무": 27387, "Ġarises": 27388, "Ġsubconscious": 27389, "ĠSandy": 27390, "Ġlottery": 27391, "âĢij": 27392, "amiliar": 27393, "Ġcoordinator": 27394, "èĮ": 27395, "Ġextraordin": 27396, "ĠRonald": 27397, "ĠMON": 27398, "green": 27399, "Ġmanufacture": 27400, "ĠRecord": 27401, "ĠMarketing": 27402, "иÑĨ": 27403, "Ġcredentials": 27404, "Ġupright": 27405, "ĠHeritage": 27406, "Ġgörd": 27407, "æľį": 27408, "expensive": 27409, "áºŃn": 27410, "Ġì±Ħ": 27411, "Ġoutlined": 27412, "ĠOooh": 27413, "oriented": 27414, "Ġwired": 27415, "Ġoutlets": 27416, "Ġhugely": 27417, "ĠíĸĪëĬĶëį°": 27418, "аÑĢÑĤ": 27419, "Ġlogistics": 27420, "Ġseasonal": 27421, "Ġdebe": 27422, "Ġtheor": 27423, "Ġpirate": 27424, "appy": 27425, "Ġknots": 27426, "Ġfemme": 27427, "ĠSoftware": 27428, "gende": 27429, "ÑĤаки": 27430, "Ġtemples": 27431, "Ġlimitation": 27432, "Ġamplitude": 27433, "Ġhacen": 27434, "Ġaudi": 27435, "Ġëĸ¨": 27436, "ĠWahl": 27437, "Ġnih": 27438, "Ġamplifier": 27439, "arius": 27440, "izado": 27441, "acha": 27442, "Ġkullan": 27443, "ĠTwin": 27444, "ĠForces": 27445, "Ġabrir": 27446, "ĠEPA": 27447, "ĠAha": 27448, "Ġê·¸ëŀĺëıĦ": 27449, "Ġbiom": 27450, "ĠТам": 27451, "Ġsailing": 27452, "ĠJoker": 27453, "First": 27454, "è¿Ļæĺ¯": 27455, "~]": 27456, "orsch": 27457, "Ġvære": 27458, "Ġbeetje": 27459, "ĠSpaÃŁ": 27460, "polit": 27461, "Ġturbul": 27462, "ĠìłĢíĿ¬ê°Ģ": 27463, "Ġcic": 27464, "ĠDrake": 27465, "ĠBRI": 27466, "ização": 27467, "ĠìŀĪëĭ¤": 27468, "ĠLynn": 27469, "Ġtransgender": 27470, "Ġresign": 27471, "Ġcharter": 27472, "ĠJH": 27473, "ĠHolmes": 27474, "ĠLip": 27475, "das": 27476, "Ġpediatric": 27477, "Ġmemorize": 27478, "Ġevaluating": 27479, "ĠðŁIJ": 27480, "cak": 27481, "Ġconjunction": 27482, "Ġreserves": 27483, "Ġshampoo": 27484, "Ġjudged": 27485, "Ġwidz": 27486, "VIN": 27487, "Ġaboard": 27488, "aris": 27489, "ĠRoh": 27490, "Ġcooled": 27491, "ÑģÑĤе": 27492, "cep": 27493, "rost": 27494, "hots": 27495, "ĠMelbourne": 27496, "оÑĩÑĮ": 27497, "Ġventil": 27498, "инов": 27499, "Ġmotions": 27500, "ìĹĪëĬĶëį°": 27501, "меÑĢик": 27502, "ĠChat": 27503, "Ġgouvernement": 27504, "ä¸Ģ次": 27505, "ĠKivol": 27506, "ĠKivolowitz": 27507, "Ġnói": 27508, "ĠкÑĥда": 27509, "Ġhydraul": 27510, "ĠBerg": 27511, "ylum": 27512, "ĠPräsident": 27513, "ropy": 27514, "Ġsemic": 27515, "ÑıеÑĤ": 27516, "ĠCape": 27517, "Ġcane": 27518, "Ġbringen": 27519, "Ġwiring": 27520, "unya": 27521, "Ġrepay": 27522, "ª©": 27523, "Ġwont": 27524, "ánt": 27525, "Ġgover": 27526, "ĠLiberty": 27527, "Ġelectromagn": 27528, "ĠSingh": 27529, "ĠгÑĢÑĥп": 27530, "гов": 27531, "Ī무ë": 27532, "ĠRule": 27533, "Ġunderway": 27534, "ĠFreder": 27535, "Ġturbine": 27536, "ishi": 27537, "ĠfÃŃs": 27538, "ĠCulture": 27539, "acre": 27540, "Ġwander": 27541, "Ġguerra": 27542, "Ġsöy": 27543, "ĠJur": 27544, "aways": 27545, "Ġschwier": 27546, "guard": 27547, "ĠAbd": 27548, "uction": 27549, "ĠarkadaÅŁlar": 27550, "ĠHamb": 27551, "?.": 27552, "size": 27553, "ĠOrth": 27554, "Ġsway": 27555, "ĠÎĶ": 27556, "Ġabsorption": 27557, "inees": 27558, "Ġpatrons": 27559, "Ġbeaches": 27560, "GG": 27561, "Ġcontamin": 27562, "intendent": 27563, "ĠнÑĢав": 27564, "ĠдеÑĢж": 27565, "Ġquilt": 27566, "Ġevolutionary": 27567, "ìĿ´ëĿ¼": 27568, "azioni": 27569, "Ġerkl": 27570, "ĠButler": 27571, "Ġdoo": 27572, "Ġnegotiation": 27573, "endum": 27574, "Ġterminology": 27575, "Ġkul": 27576, "ĠUnternehmen": 27577, "éric": 27578, "xi": 27579, "bad": 27580, "ĠдолжнÑĭ": 27581, "ĠMitchell": 27582, "three": 27583, "å¼ı": 27584, "Ġsubstrate": 27585, "ĠInhale": 27586, "ĠAgric": 27587, "unge": 27588, "ĠзÑĢ": 27589, "Ġadverse": 27590, "ĠìłĢëıĦ": 27591, "Ġpillar": 27592, "ĠMinuten": 27593, "ĠMate": 27594, "ĠPlatz": 27595, "Ġhelpless": 27596, "Ġalar": 27597, "Ġfrench": 27598, "Ġallocation": 27599, "Ġstems": 27600, "Ġmarathon": 27601, "ĠHARF": 27602, "ización": 27603, "Jess": 27604, "ĠзнаÑĩ": 27605, "Ġdeclaration": 27606, "EERING": 27607, "sterdam": 27608, "assium": 27609, "Ġseiz": 27610, "Ġpresidents": 27611, "take": 27612, "Ġwilderness": 27613, "Ġcosmic": 27614, "Ġ모ëijIJ": 27615, "stro": 27616, "Ġpowiedz": 27617, "ĠMagazine": 27618, "ĠVI": 27619, "ĠдеÑĢ": 27620, "Ġwürden": 27621, "Ġtablets": 27622, "Ġpierws": 27623, "Ġmortal": 27624, "Ġsupplied": 27625, "ĠNós": 27626, "ĠProper": 27627, "ĠкаждÑĭй": 27628, "ológ": 27629, "ë°©": 27630, "Ġmiscon": 27631, "Ġproximity": 27632, "ĠAlles": 27633, "Ġглаз": 27634, "Ġlame": 27635, "Ġvibes": 27636, "Ġdeemed": 27637, "Ġurine": 27638, "Ġreminding": 27639, "Ġcircumstance": 27640, "ëĵ¤ìĿ´": 27641, "Ġlaptops": 27642, "²": 27643, "íķ´ìķ¼": 27644, "ĠOmega": 27645, "ãģªãĤĵãģĭ": 27646, "NY": 27647, "Ġpumps": 27648, "Ġrails": 27649, "Ġsurpass": 27650, "ĠBros": 27651, "Ġnationally": 27652, "Ġgewesen": 27653, "享": 27654, "³´ëĭ¤": 27655, "oshing": 27656, "ê°Ī": 27657, "社": 27658, "Ġcrian": 27659, "ĠìĤ¬ëŀĮìĿ´": 27660, "caust": 27661, "æķ´": 27662, "ÑĨип": 27663, "ĠOber": 27664, "ĠDAY": 27665, "ĠCanon": 27666, "zung": 27667, "Ġê°ĸ": 27668, "ĠавÑĤом": 27669, "Ġdivorced": 27670, "×Ļפ": 27671, "Ïģε": 27672, "celand": 27673, "cier": 27674, "ÑĢез": 27675, "Today": 27676, "Ġorbital": 27677, "Ġstret": 27678, "Ġsatu": 27679, "Ġíģ¬ë": 27680, "zos": 27681, "ĠSco": 27682, "μÎŃ": 27683, "ĠGuardian": 27684, "interest": 27685, "ĠVER": 27686, "ünden": 27687, "ĠÑħоÑĤел": 27688, "tit": 27689, "By": 27690, "Ġanlat": 27691, "Show": 27692, "Ġoily": 27693, "ç¯Ģ": 27694, "Ġlegends": 27695, "Ġspeculation": 27696, "ĠWish": 27697, "Ġmonk": 27698, "GAN": 27699, "Ġhá»į": 27700, "Ġdangers": 27701, "ĠBene": 27702, "iquement": 27703, "ĠëĤĺìĻĢ": 27704, "Ġад": 27705, "Ġdiscrete": 27706, "Ãĩ": 27707, "Ġconditional": 27708, "ĠGill": 27709, "uates": 27710, "ĠÑģовÑģем": 27711, "Ġscreenshot": 27712, "cado": 27713, "Ġ모ëĵł": 27714, "Ġfingertips": 27715, "ĠMAC": 27716, "Ġdudes": 27717, "cost": 27718, "Ġbumps": 27719, "ondo": 27720, "Ġdatos": 27721, "Ġbeeps": 27722, "ĠPron": 27723, "ĠKhal": 27724, "zego": 27725, "ĠAbby": 27726, "Uh": 27727, "Yo": 27728, "ĠTel": 27729, "ĠμÎŃ": 27730, "KI": 27731, "Ġstresses": 27732, "Ġspreadsheet": 27733, "ĠNOW": 27734, "DB": 27735, "Ġliberation": 27736, "Ġpredictable": 27737, "ĠQuestions": 27738, "Ġspacing": 27739, "Ġinhabitants": 27740, "ĠzwiÄħz": 27741, "ç±³": 27742, "ĠSAP": 27743, "Ġluggage": 27744, "Ġhipp": 27745, "èĸ": 27746, "Ġtangent": 27747, "ĠvÃ¥": 27748, "алÑĮной": 27749, "sehen": 27750, "Ġprocessors": 27751, "Ġfindet": 27752, "Ġcartridge": 27753, "Ġadministrators": 27754, "Ġìĸ´ìļ": 27755, "Ġsupreme": 27756, "ĠAnti": 27757, "ĠíĶĦë¡ľ": 27758, "Ġinformative": 27759, "Ġkomt": 27760, "æĪijä¹Ł": 27761, "×Ļ×ĺ": 27762, "Assistant": 27763, "Ġlista": 27764, "öll": 27765, "Ġdistinctive": 27766, "ĠHud": 27767, "Ġsalon": 27768, "ä¸ĭä¾Ĩ": 27769, "même": 27770, "ĠMotion": 27771, "Ġseulement": 27772, "ĠMensch": 27773, "Ġpumped": 27774, "üher": 27775, "ibo": 27776, "Ġważ": 27777, "Ġquantitative": 27778, "Ù¾": 27779, "Ġ모ìĬµ": 27780, "Ġpouch": 27781, "ĠTheatre": 27782, "ahi": 27783, "Ġspinach": 27784, "Ġrealities": 27785, "Ġley": 27786, "ĠMartha": 27787, "Ġrecher": 27788, "eches": 27789, "Ġperiodic": 27790, "ocide": 27791, "ĠIncred": 27792, "Ġthấy": 27793, "oton": 27794, "ĠEso": 27795, "Ġgénéral": 27796, "ilight": 27797, "Ġimagining": 27798, "hea": 27799, "etical": 27800, "á»Ń": 27801, "ĠDemokrat": 27802, "Ġenjo": 27803, "Ġadjustable": 27804, "Ġrains": 27805, "ieważ": 27806, "Ġjustement": 27807, "Ġjustified": 27808, "ĠShake": 27809, "viv": 27810, "ìĤ¬ë¥¼": 27811, "Ġmett": 27812, "ĠEnvironmental": 27813, "Ġsolamente": 27814, "Ġintersect": 27815, "Ġ1988": 27816, "Ġsimulate": 27817, "JA": 27818, "ĠзаÑģ": 27819, "Ġconting": 27820, "ĠTek": 27821, "Ġtorch": 27822, "ĠдÑĢÑĥгой": 27823, "Ġinscre": 27824, "Ġmodelo": 27825, "ĠGeg": 27826, "ĠDemocrat": 27827, "кв": 27828, "ĠBuddy": 27829, "Ġredund": 27830, "Ġcrafts": 27831, "ĠHij": 27832, "Ġjue": 27833, "ĠKirk": 27834, "Ġkab": 27835, "ợ": 27836, "Ġaesthet": 27837, "ĠJON": 27838, "Ġsupercom": 27839, "ĠÑģиÑĤÑĥ": 27840, "ĠÏĮÏĦι": 27841, "ÙħÙĨ": 27842, "ĠEVER": 27843, "ìķĺìĸ´": 27844, "oit": 27845, "ĠCleveland": 27846, "Ġsixteen": 27847, "Ġwaterfall": 27848, "ï¸": 27849, "infl": 27850, "Ġcounselor": 27851, "ĠPunk": 27852, "Ġsprechen": 27853, "æµģ": 27854, "exc": 27855, "ĠSkills": 27856, "roz": 27857, "adamente": 27858, "Ġpancakes": 27859, "ê¸°ë¡ľ": 27860, "Ġplank": 27861, "Ġsovereignty": 27862, "Ġfui": 27863, "Ġнеоб": 27864, "ĠWii": 27865, "ĠSchol": 27866, "âĢİ": 27867, "ĠSpeak": 27868, "èĭ±": 27869, "ciliation": 27870, "Ġthigh": 27871, "Ġê±°ìĿĺ": 27872, "Ġjot": 27873, "Ġì´¬ìĺģ": 27874, "ĠÙħÛĮÚº": 27875, "ĠCCP": 27876, "ĠпоÑģÑĤ": 27877, "Ġobserver": 27878, "áb": 27879, "Ġstigma": 27880, "Ġpropriet": 27881, "Ġcidade": 27882, "ĠbaÅŁka": 27883, "عة": 27884, "kre": 27885, "ĠpowiedzieÄĩ": 27886, "Ġcease": 27887, "Ġskins": 27888, "Ġveggies": 27889, "Ġopposing": 27890, "opoly": 27891, "ĠJug": 27892, "ĠYoon": 27893, "ĠUnit": 27894, "Ġ1986": 27895, "Ġkons": 27896, "Ġdiagnostic": 27897, "Ġempowered": 27898, "Ġtho": 27899, "Ġcen": 27900, "ération": 27901, "ĠÑĹ": 27902, "Ġphysic": 27903, "ĠPractice": 27904, "å·Ŀ": 27905, "ĠSoutheast": 27906, "ĠEspa": 27907, "请": 27908, "ĠGeor": 27909, "roportion": 27910, "Ġspecs": 27911, "Ġadaptive": 27912, "ĠUnity": 27913, "ĠWorks": 27914, "ugen": 27915, "ĠMontana": 27916, "Thanks": 27917, "Ġwhipped": 27918, "Ġdungeon": 27919, "Ġvitamins": 27920, "SP": 27921, "Ġscandal": 27922, "Ġdinero": 27923, "ova": 27924, "Ġembro": 27925, "ĠEagle": 27926, "Ġtheology": 27927, "ĠVanessa": 27928, "ĠAIDS": 27929, "ëIJľ": 27930, "Ġfreel": 27931, "ĠAlzheimer": 27932, "ĠÅļ": 27933, "Her": 27934, "Ġtornado": 27935, "agens": 27936, "ĠìŀĪìĸ´ìĦľ": 27937, "ĠTransform": 27938, "Ġprocesso": 27939, "Ġmillise": 27940, "Ġprofessionally": 27941, "Ġmemb": 27942, "ocation": 27943, "Ġstyling": 27944, "ĠобÑıз": 27945, "ĠOperation": 27946, "Ġwygl": 27947, "ĠRan": 27948, "ĠçļĦ": 27949, "ĠKin": 27950, "á»±c": 27951, "ĠBAR": 27952, "Ġpaperwork": 27953, "Ġtule": 27954, "Ġqueria": 27955, "Ġcomply": 27956, "ĠHair": 27957, "×Ļ׼": 27958, "ĠпÑĢоÑģÑĤ": 27959, "Ġmutation": 27960, "Ġreprés": 27961, "Ġoctopus": 27962, "Ġimportantes": 27963, "Ġdeserved": 27964, "etr": 27965, "Ġdisasters": 27966, "lında": 27967, "iqué": 27968, "ĠDeshalb": 27969, "soo": 27970, "ossip": 27971, "Ġrelieved": 27972, "ĠCollins": 27973, "Ġwaterproof": 27974, "ĠYuk": 27975, "Ġcopying": 27976, "Ġbütün": 27977, "ĠHeute": 27978, "ĠEntre": 27979, "Ġresidual": 27980, "Ġcolonies": 27981, "Ġénorm": 27982, "ĠErin": 27983, "Ġstan": 27984, "Ġtremendously": 27985, "Ġcaptures": 27986, "ĠSai": 27987, "âce": 27988, "ĠmiaÅĤ": 27989, "Ġ87": 27990, "Ġlogging": 27991, "Ġinserted": 27992, "Ġinherently": 27993, "ìĿij": 27994, "lave": 27995, "ниÑĩ": 27996, "Ġfemmes": 27997, "Ġdép": 27998, "uks": 27999, "acia": 28000, "ĠWade": 28001, "Ġjij": 28002, "ĠVincent": 28003, "ĠIceland": 28004, "hem": 28005, "Ġapology": 28006, "ĠPeg": 28007, "Ġglued": 28008, "Ġcompanions": 28009, "ĠLiver": 28010, "Ġcriticized": 28011, "leading": 28012, "Ġsäga": 28013, "æ¼Ĥ": 28014, "Ġsquid": 28015, "Ġnarratives": 28016, "Ġtaka": 28017, "nez": 28018, "weit": 28019, "Ġtripod": 28020, "Ġexplic": 28021, "Ġspinal": 28022, "Ġapproximation": 28023, "Ġpagar": 28024, "ĠCalvin": 28025, "ĠведÑĮ": 28026, "Ġlac": 28027, "Ġproactive": 28028, "ĠTrain": 28029, "orf": 28030, "Ġsten": 28031, "Ġgrapes": 28032, "Ġmeus": 28033, "Ġautomat": 28034, "Ġbiased": 28035, "Ġchaîne": 28036, "coal": 28037, "Ġrencont": 28038, "ĠKum": 28039, "Ġfestivals": 28040, "Ġstartups": 28041, "Ġaka": 28042, "ãģ¹": 28043, "Ġcylind": 28044, "sna": 28045, "CRI": 28046, "Ġresultado": 28047, "Ġmilestone": 28048, "ĠÏħ": 28049, "Ġteleport": 28050, "zych": 28051, "62": 28052, "åħ³": 28053, "ĠFear": 28054, "Ġnucleus": 28055, "Ġshines": 28056, "hov": 28057, "ĠPartners": 28058, "ĠKas": 28059, "Ġnadie": 28060, "Ġalerts": 28061, "ĠBILL": 28062, "strong": 28063, "ĠNate": 28064, "ĠDenmark": 28065, "ĠCav": 28066, "OST": 28067, "hält": 28068, "ĠìķĦëĭĮ": 28069, "anyon": 28070, "Ġencourages": 28071, "ĠпоÑģÑĤав": 28072, "ĠHuang": 28073, "ãģĬé¡ĺãģĦ": 28074, "STA": 28075, "Ġpaints": 28076, "ãģĻãģĶ": 28077, "Ġschedules": 28078, "Ġcheated": 28079, "Ġapprox": 28080, "Ġï·": 28081, "Ġ».": 28082, "Ġsmiles": 28083, "isure": 28084, "Ġnered": 28085, "arden": 28086, "Ġcurt": 28087, "ĠëĮ": 28088, "ĠRoth": 28089, "Ġpuisque": 28090, "ĠGET": 28091, "ĠVeget": 28092, "Ġproduz": 28093, "ĠBelgium": 28094, "ĠCampus": 28095, "ר×Ļ×Ŀ": 28096, "icut": 28097, "ĠÑģним": 28098, "Ġréuss": 28099, "Ġslippery": 28100, "ĠEw": 28101, "ų": 28102, "ĠLegends": 28103, "ĠTiffany": 28104, "ализ": 28105, "ĠпеÑĢев": 28106, "ĠогÑĢом": 28107, "Ġcros": 28108, "ĠCE": 28109, "Bu": 28110, "Ġensures": 28111, "Ġgrandchildren": 28112, "Ġacuerdo": 28113, "Ġprisoner": 28114, "Ġthirsty": 28115, "bane": 28116, "Ġë¹ł": 28117, "Ġúltima": 28118, "ĠLaunch": 28119, "nity": 28120, "Ġcombustion": 28121, "Ġunicorn": 28122, "Ġfamille": 28123, "Ġlowering": 28124, "ĠYing": 28125, "building": 28126, "Ġduo": 28127, "ĠMéxico": 28128, "astian": 28129, "Ġ먹ìĿĦ": 28130, "ĠRalph": 28131, "Ġrewrite": 28132, "Ġglam": 28133, "ifique": 28134, "Er": 28135, "ĠRunning": 28136, "онов": 28137, "Ġmeanings": 28138, "Ġchewy": 28139, "ĠLeslie": 28140, "Ġfinest": 28141, "Ġhahaha": 28142, "ĠSTEP": 28143, "Ġloneliness": 28144, "rians": 28145, "Ġquestioned": 28146, "Ġesque": 28147, "Ġsinking": 28148, "Ġpeso": 28149, "ĠWrong": 28150, "asmine": 28151, "Ġdefinitive": 28152, "Ġbuys": 28153, "Ġcruc": 28154, "cool": 28155, "ĠëłĪ": 28156, "Ġpó": 28157, "Ġutilized": 28158, "Ġworthwhile": 28159, "ĠDylan": 28160, "ESE": 28161, "Ġvertex": 28162, "tı": 28163, "ĠFir": 28164, "Ġzaw": 28165, "ĠGed": 28166, "ĠÐĿап": 28167, "dz": 28168, "Ġcursor": 28169, "Ġswipe": 28170, "Ġinevitably": 28171, "Ġposters": 28172, "Ġinclined": 28173, "Ġgreeting": 28174, "Ġdisappointment": 28175, "ãģ¾ãģ§": 28176, "Ġrelação": 28177, "TT": 28178, "Ġrabb": 28179, "ĠMaine": 28180, "Ġanalyzed": 28181, "FE": 28182, "ĠÐŁÐ¾Ð»": 28183, "ĠSandra": 28184, "Ġplague": 28185, "ARE": 28186, "Ġvär": 28187, "ĠViv": 28188, "umed": 28189, "hando": 28190, "houette": 28191, "ĠBailey": 28192, "ä¸įéģİ": 28193, "yson": 28194, "Ġsemua": 28195, "Ġhardcore": 28196, "âĤ¬": 28197, "Ñĸм": 28198, "éra": 28199, "OTH": 28200, "Ġforeigners": 28201, "ĠPalestinian": 28202, "Ġproprio": 28203, "аний": 28204, "Ġmyths": 28205, "WH": 28206, "Ġninth": 28207, "ĠCreator": 28208, "лом": 28209, "ĠFlip": 28210, "Ġeman": 28211, "ĠkiÅŁ": 28212, "zieh": 28213, "ĠEarnest": 28214, "system": 28215, "ĸìĹIJ": 28216, "Ġarmies": 28217, "ĠOutside": 28218, "Ġharus": 28219, "æºĸ": 28220, "одаÑĢ": 28221, "Ġvisitor": 28222, "çŃĶ": 28223, "Ġstrengthening": 28224, "Ġ92": 28225, "vio": 28226, "Ġ리": 28227, "Ġgreedy": 28228, "Ġpoquito": 28229, "uder": 28230, "ĠKopf": 28231, "Ġëĭ¤ìĿĮìĹIJ": 28232, "Ġseis": 28233, "ático": 28234, "Ġtrusting": 28235, "ÃŃp": 28236, "ĠEmm": 28237, "leen": 28238, "ĠاÙĦÙĨ": 28239, "Ġrecruitment": 28240, "ĠFilip": 28241, "ĠÙĥÙĦ": 28242, "Clint": 28243, "ĠвеÑģ": 28244, "auft": 28245, "Ġdominate": 28246, "Ġresto": 28247, "Ġkra": 28248, "ái": 28249, "ĠCait": 28250, "rows": 28251, "Ġcountryside": 28252, "Ġ1945": 28253, "аÑĨиÑİ": 28254, "Ġди": 28255, "Ġkernel": 28256, "lov": 28257, "Ġcalculating": 28258, "دا": 28259, "ĠWalt": 28260, "Ġempowering": 28261, "Ġchassis": 28262, "linear": 28263, "гÑĥ": 28264, "Ġnova": 28265, "Ġuy": 28266, "Ġ69": 28267, "Ġencompass": 28268, "trl": 28269, "Ġcomputational": 28270, "Ġworms": 28271, "Ġnhiá»ģu": 28272, "Ġastronauts": 28273, "Ġves": 28274, "Ġsytu": 28275, "Ġdemanded": 28276, "Ġcs": 28277, "ĠMol": 28278, "Ġ`": 28279, "Ġchant": 28280, "Ġthereby": 28281, "Ġpenis": 28282, "Ġemoc": 28283, "wyn": 28284, "Ñĥже": 28285, "Ġtread": 28286, "óle": 28287, "Ġdeepest": 28288, "Ġmache": 28289, "ĠVent": 28290, "ĠAmsterdam": 28291, "ãĥĽ": 28292, "Ġrebel": 28293, "Ġ61": 28294, "ĠвкÑĥÑģ": 28295, "uffs": 28296, "ĠdoÄŁru": 28297, "ĠNapole": 28298, "ήÏĥ": 28299, "Ġworkouts": 28300, "ĠGlad": 28301, "неÑģ": 28302, "Ġtensions": 28303, "ĠShift": 28304, "ĠGuer": 28305, "íĮIJ": 28306, "Ġì¹ľêµ¬": 28307, "Ðĸ": 28308, "Ġimplant": 28309, "êu": 28310, "ê¸Ģ": 28311, "Ġauthorized": 28312, "CER": 28313, "ĠRV": 28314, "Ġhil": 28315, "lev": 28316, "cimento": 28317, "ĠUFO": 28318, "ìĥĪ": 28319, "è¨Ĥ": 28320, "wor": 28321, "Ġdances": 28322, "ĠPixel": 28323, "çľĭä¸Ģä¸ĭ": 28324, "Ġtrotzdem": 28325, "Ġobten": 28326, "ĠAlfred": 28327, "Ġcostly": 28328, "ĠStanley": 28329, "Ġterrorists": 28330, "ĠWid": 28331, "ħëĭĪëĭ¤": 28332, "Ġleicht": 28333, "ìĿ´ìĬ¤": 28334, "Ġdobrze": 28335, "Ġhesit": 28336, "Ġerzäh": 28337, "Ġeinige": 28338, "Ġhebt": 28339, "Ñģе": 28340, "Ġunpredict": 28341, "Cómo": 28342, "remos": 28343, "ĠThankfully": 28344, "Ġpurse": 28345, "chs": 28346, "ancer": 28347, "ulos": 28348, "stud": 28349, "æľīæ²Ĵæľī": 28350, "Ġneurolog": 28351, "ĠAncient": 28352, "Out": 28353, "awsze": 28354, "Ġoppose": 28355, "Ġantibodies": 28356, "ĠSomehow": 28357, "ropolitan": 28358, "ktor": 28359, "ĠÑģÑĤоÑĢонÑĭ": 28360, "Ġrockets": 28361, "Ġdisable": 28362, "Ġcatastroph": 28363, "´ìŀ": 28364, "Ġcyn": 28365, "ĠдÑĢÑĥзÑĮÑı": 28366, "Ġinstructors": 28367, "emaal": 28368, "Ġetwa": 28369, "Ġyuan": 28370, "ĠGround": 28371, "Ġpremiere": 28372, "Ñĩив": 28373, "Ġsaint": 28374, "yba": 28375, "Ġkok": 28376, "Ġcontractors": 28377, "Ġê°ģ": 28378, "Ġ×IJ׾": 28379, "Ġheadline": 28380, "Ġcompletamente": 28381, "Ġinexpensive": 28382, "Ġviu": 28383, "ĠGrande": 28384, "Ġbleed": 28385, "물": 28386, "Ġ73": 28387, "ĠtodavÃŃa": 28388, "ĠRush": 28389, "ĠElder": 28390, "ê°ĢëĬĶ": 28391, "ĠRou": 28392, "ĠженÑī": 28393, "ĠMira": 28394, "Ġdeine": 28395, "Ġkarma": 28396, "Ġumm": 28397, "Ġentsche": 28398, "ĠHolocaust": 28399, "Ġdiscoveries": 28400, "aments": 28401, "Ġraison": 28402, "Ġburgers": 28403, "Back": 28404, "Ġgdy": 28405, "ĠAG": 28406, "ĠDaw": 28407, "ìķł": 28408, "headed": 28409, "ĠClar": 28410, "Inst": 28411, "ĠLieutenant": 28412, "ĠAfD": 28413, "ĠCes": 28414, "Ġpersonalized": 28415, "Ġinterfaces": 28416, "à¸Īะ": 28417, "ĠÑĢеж": 28418, "Ġsuic": 28419, "Ġstarving": 28420, "Ġoxide": 28421, "Ġdecorated": 28422, "ĠDU": 28423, "ĠìĺĪìģĺ": 28424, "Ġquo": 28425, "Ġdistortion": 28426, "段": 28427, "Ġ먹ìĸ´ë": 28428, "Ġstakes": 28429, "æĺİçϽ": 28430, "Ġsyntax": 28431, "Ġbiết": 28432, "thy": 28433, "icie": 28434, "Ġbrasile": 28435, "isis": 28436, "RC": 28437, "Ġshook": 28438, "Ġdepths": 28439, "ĠCosta": 28440, "Ġvocals": 28441, "Ġcoaster": 28442, "Ġfalou": 28443, "ettle": 28444, "Ġkennen": 28445, "Ġderive": 28446, "Ġaids": 28447, "ĠÐĿик": 28448, "Ġentwic": 28449, "Ġvertically": 28450, "ĠÍ": 28451, "ĠSUV": 28452, "Ġfireworks": 28453, "Ġspecifics": 28454, "交": 28455, "Ġinsisted": 28456, "Ġdeshalb": 28457, "ĠGonz": 28458, "love": 28459, "ĠMilitary": 28460, "ĠPierre": 28461, "ĠâĪ": 28462, "ĠWhose": 28463, "Ġperfume": 28464, "ĠÏĢε": 28465, "Ġlowered": 28466, "Ġcrosses": 28467, "Ġtranslates": 28468, "Ġarriba": 28469, "ÃŃdo": 28470, "ĠLev": 28471, "åħ§": 28472, "ĠCiao": 28473, "Ġscholarships": 28474, "Ġgestures": 28475, "ĠÑĢезÑĥлÑĮÑĤаÑĤ": 28476, "Ġquestão": 28477, "ĠColonel": 28478, "ĠBott": 28479, "رÙģ": 28480, "NING": 28481, "ĠWatching": 28482, "ĠPurple": 28483, "ÑģÑĤÑĢан": 28484, "Ġexecutives": 28485, "ĠKris": 28486, "orneys": 28487, "еннÑĭй": 28488, "Ġcoated": 28489, "Ä©": 28490, "Ġparked": 28491, "ĠÑģвеÑĤ": 28492, "!!!!!": 28493, "ĠFloyd": 28494, "ısı": 28495, "ziÄĩ": 28496, "Ġmotivate": 28497, "ĠElon": 28498, "lean": 28499, "Ĩĵ": 28500, "Ġip": 28501, "Ġniż": 28502, "ĠExperience": 28503, "ĠTina": 28504, "ĠKollege": 28505, "ĠAmbassador": 28506, "inya": 28507, "Ġtheft": 28508, "Ġheures": 28509, "ĠMyst": 28510, "Ġmaison": 28511, "leb": 28512, "Ġbowls": 28513, "ĠBürger": 28514, "ĠRoosevelt": 28515, "RP": 28516, "ê°ĢìļĶ": 28517, "ĠDelicious": 28518, "erdings": 28519, "ĠAssociate": 28520, "ousse": 28521, "ĠCort": 28522, "ĠRepeat": 28523, "ĠGlory": 28524, "Ġcontag": 28525, "à¹Ģล": 28526, "ĠParad": 28527, "ĠKerry": 28528, "Ġê¿": 28529, "ĠWave": 28530, "å¿ħ": 28531, "Ġgateway": 28532, "çIJĥ": 28533, "!ãĢį": 28534, "Ġtranscend": 28535, "Ġdamages": 28536, "Ġtails": 28537, "Ġgravitational": 28538, "ĠShield": 28539, "Ġprimitive": 28540, "Ġcarriers": 28541, "ĠHuawei": 28542, "ÙĤد": 28543, "Ġfeliz": 28544, "ĠMia": 28545, "åĥķ": 28546, "ĠпÑĢÑıмо": 28547, "ĠпÑĢоиÑģÑħодиÑĤ": 28548, "ĠMurphy": 28549, "ĠActiv": 28550, "ãĥĥãĤ¯": 28551, "Ġdiscomfort": 28552, "×ij×Ķ": 28553, "ĠKell": 28554, "ĠCentury": 28555, "Ġspaghetti": 28556, "ĠDurch": 28557, "Ġcierto": 28558, "ĠEmpress": 28559, "Ġguts": 28560, "neg": 28561, "ĠдоÑģÑĤаÑĤоÑĩно": 28562, "Ġvoluntary": 28563, "失": 28564, "Ġsquirrel": 28565, "欢": 28566, "ãģ¡ãĤī": 28567, "ĠMaz": 28568, "´ìĭ¬": 28569, "Ġви": 28570, "ãĤ§": 28571, "ĠÑĤакиÑħ": 28572, "ĠSharon": 28573, "Ġenthusiastic": 28574, "irement": 28575, "Ġíŀĺëĵ¤": 28576, "Ġpotrze": 28577, "Ġinitiated": 28578, "ãĥ§": 28579, "ĠÅĽrod": 28580, "ĠìĿ´ë¦Ħ": 28581, "Ġremake": 28582, "Ġculmin": 28583, "Ġconfuse": 28584, "miyor": 28585, "urar": 28586, "CTOR": 28587, "Ġbunny": 28588, "Ġ大": 28589, "ä¸įèĥ½": 28590, "elp": 28591, "Ġvampire": 28592, "Ġillumin": 28593, "ĠHend": 28594, "ĠкаÑĩе": 28595, "ĠSalv": 28596, "Ġканал": 28597, "Ġporta": 28598, "Ġasshole": 28599, "Ġsupporter": 28600, "Ġskeptical": 28601, "Ġknead": 28602, "Ġìĺ¬": 28603, "eza": 28604, "Ġquê": 28605, "ĠDH": 28606, "Ġrodz": 28607, "owners": 28608, "Ġplots": 28609, "Ġdelays": 28610, "Ġbelonged": 28611, "Ġahh": 28612, "Ġcarved": 28613, "Ġrisen": 28614, "Ġorden": 28615, "phony": 28616, "issy": 28617, "!!!!!!!!": 28618, "ĠolduÄŁunu": 28619, "Ġroses": 28620, "Ġintrins": 28621, "ĠAngst": 28622, "Ġfinalement": 28623, "ì§Ŀ": 28624, "SOUND": 28625, "Ġindul": 28626, "°Į": 28627, "Ġ×ķ×Ķ": 28628, "chy": 28629, "акÑģим": 28630, "Ġnggak": 28631, "Ġliz": 28632, "Ġelectoral": 28633, "ĠShawn": 28634, "ricia": 28635, "Ġarsen": 28636, "ĠPep": 28637, "Ġ2030": 28638, "Ġtrophy": 28639, "Ġsmoother": 28640, "Ġerre": 28641, "Ġcrashes": 28642, "Ġschne": 28643, "Ġasi": 28644, "ĠMaÃŁ": 28645, "Ñĥли": 28646, "ÑĩеÑģки": 28647, "ieves": 28648, "REAM": 28649, "Ġstirring": 28650, "ãĥĢ": 28651, "usta": 28652, "Ġinver": 28653, "sight": 28654, "ordu": 28655, "oor": 28656, "ĠÄĥn": 28657, "Ġpermitted": 28658, "ÑĢÑĮ": 28659, "Ġchalk": 28660, "ãĤĪãģĹ": 28661, "Ġtattoos": 28662, "ĠRelations": 28663, "ĠHoy": 28664, "ksam": 28665, "Ġdentist": 28666, "Ġ미êµŃ": 28667, "Ġsofa": 28668, "ĠÑĶ": 28669, "Ġforme": 28670, "ÙĤØ©": 28671, "Ġë²ł": 28672, "Ġembraced": 28673, "mil": 28674, "Ġsunglasses": 28675, "Ġê°Ķ": 28676, "Ġseamless": 28677, "Ġbeep": 28678, "ächst": 28679, "Ġsweets": 28680, "Ġsemaine": 28681, "Ġirrelevant": 28682, "Ġdesenvol": 28683, "ÏģÏī": 28684, "ĠпÑĢоизвод": 28685, "angs": 28686, "Ġaroma": 28687, "Ġpools": 28688, "Ġgiá»Ŀ": 28689, "ĠUg": 28690, "Ġclimbed": 28691, "Ġtrending": 28692, "Ġseperti": 28693, "ĠBarr": 28694, "ĠpÅĤ": 28695, "ĠOriginally": 28696, "ĠÚ¯": 28697, "utto": 28698, "Ĭ¸ë": 28699, "ĠкоÑĤоÑĢÑĭÑħ": 28700, "ĠзаÑħ": 28701, "Ġeigenen": 28702, "Ġmurderer": 28703, "ername": 28704, "Åŀ": 28705, "Ġannouncing": 28706, "ĠPlatform": 28707, "Ġexplanations": 28708, "Ġpresente": 28709, "ĠNasıl": 28710, "Ġorphan": 28711, "ĠFortnite": 28712, "rospect": 28713, "eredith": 28714, "ĠìĹĨìĸ´": 28715, "ĠNIH": 28716, "wagen": 28717, "Ġremed": 28718, "§Ģë": 28719, "mont": 28720, "ĠJeffrey": 28721, "prom": 28722, "Ġfünf": 28723, "Ġназад": 28724, "Ġcucumber": 28725, "ĠSummit": 28726, "åĪĿ": 28727, "§¤": 28728, "ÐĿÐIJЯ": 28729, "ĠJet": 28730, "Ġcambio": 28731, "ÑĥйÑĤе": 28732, "Ġcubic": 28733, "Ġdisproportion": 28734, "erez": 28735, "Ġmadness": 28736, "çĹĽ": 28737, "Ġtint": 28738, "Ġfueron": 28739, "Ġky": 28740, "Ġbipart": 28741, "ãģ¾ãģĽ": 28742, "Sam": 28743, "Ġë½": 28744, "Ġriv": 28745, "ĠTank": 28746, "ĠëĨĵ": 28747, "Ġrendered": 28748, "ÅĽlÄĻ": 28749, "conds": 28750, "Ġdisruption": 28751, "Ġinconven": 28752, "Ġquiser": 28753, "Ġdenial": 28754, "Ġgalaxies": 28755, "Ġsovereign": 28756, "Ġpolsk": 28757, "ÏģÏİ": 28758, "Ġmex": 28759, "Ġcaracter": 28760, "ĠLego": 28761, "anden": 28762, ".'\"": 28763, "ĠíĶĮë": 28764, "Ġcompressor": 28765, "ĠMovie": 28766, "Ġapplicants": 28767, "ziehen": 28768, "Ġvegetation": 28769, "Ġbelle": 28770, "ĠGOOD": 28771, "ĠBau": 28772, "Ġresent": 28773, "sex": 28774, "amentos": 28775, "Ġ×Ķ×ĸ×Ķ": 28776, "Ġoverload": 28777, "Ġsilicone": 28778, "еÑģÑĤно": 28779, "Ġdenken": 28780, "Ġdefinit": 28781, "ĠWasn": 28782, "Ġaltered": 28783, "ĠSoo": 28784, "ĠWing": 28785, "indre": 28786, "ĠNPC": 28787, "ÏģÎŃ": 28788, "ĠTwenty": 28789, "ĠLiebe": 28790, "Ġhomelessness": 28791, "oulder": 28792, "ĠÐĺÑĤак": 28793, "ÑģкаÑı": 28794, "Ġcuatro": 28795, "ĠHarvey": 28796, "Ġphilan": 28797, "ĠBeet": 28798, "Ġpolicing": 28799, "ĠAlexand": 28800, "Ġмолод": 28801, "Ġmüs": 28802, "Ġhizo": 28803, "ë³´ëĭ¤": 28804, "Ġпозвол": 28805, "ĠпÑĭÑĤ": 28806, "оÑĩемÑĥ": 28807, "Ġíĥľ": 28808, "Ġcryptocurrency": 28809, "Ġloro": 28810, "Ġsummation": 28811, "Ġbakalım": 28812, "Ġneuros": 28813, "Ø¥": 28814, "Ġможем": 28815, "Ġüst": 28816, "Ġpreliminary": 28817, "Ġhorns": 28818, "ĠTI": 28819, "ÙĥÙĦ": 28820, "YO": 28821, "Ġhinge": 28822, "Ġrepairs": 28823, "Ġbonding": 28824, "Ġbize": 28825, "ĠÑĪÑĤ": 28826, "Ġmotive": 28827, "ĠNigeria": 28828, "120": 28829, "block": 28830, "Ġaviation": 28831, "ĠKommun": 28832, "Ġоказ": 28833, "Ġtenha": 28834, "Ġeducating": 28835, "Ġstaat": 28836, "æ¶Ī": 28837, "ĠÑģколÑĮко": 28838, "Ġfrightened": 28839, "Ġseeks": 28840, "ÑĢÑĥÑĪ": 28841, "quent": 28842, "ĠNou": 28843, "Ġprat": 28844, "ĠShot": 28845, "Work": 28846, "karang": 28847, "ĠLightning": 28848, "nolds": 28849, "rolled": 28850, "glass": 28851, "Ġcredibility": 28852, "ITY": 28853, "Ġatmospheric": 28854, "Ġhavia": 28855, "ändern": 28856, "cheers": 28857, "These": 28858, "ĠCell": 28859, "Ġmagnes": 28860, "ĠBravo": 28861, "season": 28862, "ĠÅŁeyler": 28863, "ðŁİ": 28864, "white": 28865, "ĠMB": 28866, "Ġstacked": 28867, "Ġ74": 28868, "Ġдавай": 28869, "Ġpave": 28870, "ĠоÑħ": 28871, "Ġdataset": 28872, "Ġretour": 28873, "Ġmaturity": 28874, "Ġquase": 28875, "Ġ93": 28876, "ĠSym": 28877, "Ġbriefing": 28878, "Ġculturally": 28879, "Ġì·¨": 28880, "inhas": 28881, "Ġmadam": 28882, "Ġajudar": 28883, "ĠTibet": 28884, "Ġleaks": 28885, "cile": 28886, "Ġtheaters": 28887, "ìĺ¨": 28888, "ãĥĸ": 28889, "72": 28890, "ĠWash": 28891, "ĠQuality": 28892, "ĠIvan": 28893, "ĠBent": 28894, "igator": 28895, "ĠGeschichte": 28896, "Ġreactive": 28897, "Ġ1900": 28898, "æ¡Ī": 28899, "Ġcontradict": 28900, "Ġziemlich": 28901, "Ġcohort": 28902, "á»§": 28903, "Ġpestic": 28904, "Ġoraz": 28905, "Ġtellement": 28906, "é¾": 28907, "ĠNowadays": 28908, "crew": 28909, "Steve": 28910, "Ġfictional": 28911, "Ġilk": 28912, "ãģĤãģ£": 28913, "Ġgasoline": 28914, "zam": 28915, "Ġpancake": 28916, "ència": 28917, "Ġmuitos": 28918, "Ġbury": 28919, "Ġkop": 28920, "ĠIQ": 28921, "Ġreservation": 28922, "ĠUpdate": 28923, "Ġjej": 28924, "ĠEyes": 28925, "åıij": 28926, "Ġvive": 28927, "Ġchce": 28928, "ĠIni": 28929, "respons": 28930, "Ġreflective": 28931, "ĠWan": 28932, "Ñĸз": 28933, "Ġenca": 28934, "Ġembod": 28935, "ĠBurger": 28936, "Ġacademia": 28937, "ĠCirc": 28938, "ĠпÑĢек": 28939, "Ġanlam": 28940, "Ġphilanthrop": 28941, "ĠBaÅŁ": 28942, "ĠAudi": 28943, "Ġvost": 28944, "ä½łçŁ¥éģĵ": 28945, "Ġreper": 28946, "Peter": 28947, "Ġconsoles": 28948, "Ġscrut": 28949, "ĠTurner": 28950, "ĠбÑĭв": 28951, "III": 28952, "訴": 28953, "ĠFlight": 28954, "à¸ĸ": 28955, "ĠRaven": 28956, "Ġcorros": 28957, "fern": 28958, "Ġprova": 28959, "ĠSev": 28960, "Ġrecipro": 28961, "Ġ1985": 28962, "Ġnueva": 28963, "Ġdab": 28964, "ãĢģãĢĮ": 28965, "Ġmez": 28966, "ĠStark": 28967, "ppings": 28968, "оÑģÑĤи": 28969, "ì¦Ŀ": 28970, "Ġframing": 28971, "ĠÐłÐ°Ð·": 28972, "Ġpostp": 28973, "ĠShannon": 28974, "ĠкÑĥÑĢ": 28975, "Ġjakby": 28976, "iennent": 28977, "ĠMaps": 28978, "ĠRevelation": 28979, "ĠÑģÑĤал": 28980, "ìļ´ëį°": 28981, "Ġdevant": 28982, "ĠGiving": 28983, "ĠWAS": 28984, "Ġкого": 28985, "Ġrema": 28986, "ĠRC": 28987, "nÃŃ": 28988, "Ġslipped": 28989, "ĠRams": 28990, "Ġweet": 28991, "Ġmasculine": 28992, "ĠEc": 28993, "Ġreop": 28994, "ĠPlant": 28995, "ĠMAY": 28996, "Ġspikes": 28997, "Ġnozzle": 28998, "ĠWikipedia": 28999, "ĠCoh": 29000, "ISSA": 29001, "chlossen": 29002, "ì§Ģ를": 29003, "Ġ미ë": 29004, "ĠNeder": 29005, "Josh": 29006, "ĠÐłÐ¾ÑģÑģии": 29007, "Ġ1987": 29008, "ĠTheory": 29009, "ekk": 29010, "Ġutan": 29011, "Ġдома": 29012, "chu": 29013, "ĠÑģб": 29014, "Ġaprove": 29015, "VEN": 29016, "ueprint": 29017, "Ġ84": 29018, "æ¼Ĥ亮": 29019, "Cor": 29020, "Ġricher": 29021, "Ġsandwiches": 29022, "atsu": 29023, "ÑĪиÑħ": 29024, "Ġlatt": 29025, "~~~~": 29026, "friends": 29027, "Ġdernière": 29028, "Ġstereo": 29029, "ĠÑįкÑģп": 29030, "Ġprotections": 29031, "Ġhaut": 29032, "Everyone": 29033, "Ġenterprises": 29034, "ĠMostly": 29035, "ĠSpotify": 29036, "ĠSex": 29037, "Ġung": 29038, "Į를": 29039, "Ġactivism": 29040, "ctica": 29041, "original": 29042, "ĠпÑĢогÑĢам": 29043, "Ġbroccoli": 29044, "à¦": 29045, "огÑĢаÑĦ": 29046, "Ġsekarang": 29047, "Ġcrafting": 29048, "Ġбан": 29049, "ãģ»ãģ©": 29050, "ĠRaz": 29051, "Ġnaive": 29052, "Ġscrolling": 29053, "Ġnumerical": 29054, "Ġscheduling": 29055, "Ġapartments": 29056, "çį": 29057, "Ġstretches": 29058, "acey": 29059, "ĠHER": 29060, "ãĤº": 29061, "Ġzinc": 29062, "Ġdarn": 29063, "Ġcél": 29064, "Ġwardrobe": 29065, "Ġredirect": 29066, "Ġjum": 29067, "ĠStrange": 29068, "ĠnÃło": 29069, "Ġexperimenting": 29070, "éré": 29071, "Ġvoulez": 29072, "Ġgebe": 29073, "ĠKann": 29074, "ĠÄijá»Ļ": 29075, "ĠMaxim": 29076, "ĠKön": 29077, "ĠGlas": 29078, "Ġpolished": 29079, "Ġnuma": 29080, "Ich": 29081, "Ġrituals": 29082, "ĠSI": 29083, "иÑĤели": 29084, "Ġinfilt": 29085, "Ġscarf": 29086, "ophy": 29087, "Ġyine": 29088, "Ġcivic": 29089, "ĠMeng": 29090, "änge": 29091, "Õ¥": 29092, "histoire": 29093, "ĠOke": 29094, "ĠìĺĨ": 29095, "Ġsollten": 29096, "Ġ82": 29097, "馬": 29098, "Ġprescribed": 29099, "ĠDubai": 29100, "ĠEltern": 29101, "Ġnationwide": 29102, "Ġskating": 29103, "iary": 29104, "Ġrewarded": 29105, "Ġmorality": 29106, "ĠMaggie": 29107, "ĠOhhh": 29108, "ĠFahren": 29109, "olved": 29110, "æĹ¶åĢĻ": 29111, "Ġdeuxième": 29112, "techn": 29113, "role": 29114, "Ġleider": 29115, "ĠJAY": 29116, "ĠинÑĦоÑĢм": 29117, "Ġcaffe": 29118, "reichen": 29119, "Ġkart": 29120, "ĠCute": 29121, "ffective": 29122, "Ġbully": 29123, "agar": 29124, "Ġcommodity": 29125, "Ġobrig": 29126, "OUR": 29127, "Ġunpleasant": 29128, "nox": 29129, "Jul": 29130, "olith": 29131, "ÑĤоÑıÑī": 29132, "ĠBella": 29133, "Ġdolls": 29134, "ĠHoff": 29135, "Ġadvisors": 29136, "Ġtransfers": 29137, "ĠGoku": 29138, "Ġ1200": 29139, "inhos": 29140, "Pal": 29141, "Ġëĺij": 29142, "Ġrept": 29143, "Ġaccomplishment": 29144, "Ġweave": 29145, "Ġoversight": 29146, "Ġunhealthy": 29147, "Ġfilt": 29148, "Ġpudding": 29149, "ĠMiguel": 29150, "Ġchuckles": 29151, "åı°çģ£": 29152, "version": 29153, "Ġconfession": 29154, "value": 29155, "Ġtriumph": 29156, "Ġsair": 29157, "Ġëħ¸": 29158, "Ġarte": 29159, "ĠMaterial": 29160, "uti": 29161, "Ġliquor": 29162, "ĠBayern": 29163, "ĠMail": 29164, "Ġíĸ¥": 29165, "Ñģком": 29166, "Ġcheapest": 29167, "ĠÑĩаÑģÑĤи": 29168, "ĠJobs": 29169, "ĠCanyon": 29170, "harma": 29171, "aley": 29172, "andro": 29173, "Ġappearances": 29174, "prof": 29175, "Ġоз": 29176, "lagen": 29177, "Ġ//": 29178, "ĠлиÑĪÑĮ": 29179, "Ġrecovering": 29180, "дж": 29181, "psy": 29182, "ãĥ¢": 29183, "Ġswift": 29184, "ĠSpin": 29185, "å¸Ī": 29186, "Ġseinem": 29187, "Ġdolph": 29188, "führ": 29189, "ât": 29190, "Ġaltijd": 29191, "ĠMarty": 29192, "ĠHoch": 29193, "Ġpredators": 29194, "Ġvorher": 29195, "ĠÐĶавай": 29196, "Ġfragments": 29197, "Ġpastry": 29198, "Ġcommen": 29199, "ĠSana": 29200, "Ġê±´ëį°": 29201, "ussen": 29202, "Ġtela": 29203, "ĠNina": 29204, "lek": 29205, "Ġcries": 29206, "Ġthighs": 29207, "ĠFlex": 29208, "ĠBuzz": 29209, "ãĦ": 29210, "Us": 29211, "Ġpaso": 29212, "Ġdeclined": 29213, "ĠNy": 29214, "balance": 29215, "Ġmasa": 29216, "Ġjos": 29217, "ãģªãĤĭ": 29218, "ĠСпаÑģибо": 29219, "achu": 29220, "loud": 29221, "Ġpena": 29222, "ĠWald": 29223, "Ġelimination": 29224, "ĠвеÑģÑĮ": 29225, "orage": 29226, "Ġmisunderstanding": 29227, "Ġendorse": 29228, "Ġogóle": 29229, "Ġgreed": 29230, "Ġklein": 29231, "׾×Ķ": 29232, "REY": 29233, "ĠEating": 29234, "Ġseminar": 29235, "ĠBirthday": 29236, "Ġquelle": 29237, "ĠMulti": 29238, "Ġtirar": 29239, "Ġperch": 29240, "Ġlavor": 29241, "ĠJia": 29242, "Ġmutations": 29243, "Ġcigarettes": 29244, "ÙĪØ¬": 29245, "Ġcousins": 29246, "Ġcapsule": 29247, "Ġhorrific": 29248, "Ġstur": 29249, "Ġzeigt": 29250, "nuts": 29251, "Ġmeanwhile": 29252, "ĠColin": 29253, "Ġgobierno": 29254, "Ġgw": 29255, "Ġuhh": 29256, "ĠJER": 29257, "specific": 29258, "Ġallegations": 29259, "Ġë©ĭ": 29260, "ĠElla": 29261, "ooked": 29262, "ĠFit": 29263, "affle": 29264, "ĠAprès": 29265, "ĠDuck": 29266, "Ġcellular": 29267, "ców": 29268, "ĠÑĩÑĥвÑģÑĤв": 29269, "genommen": 29270, "ìĬ¤íĬ¸": 29271, "Ġlain": 29272, "isol": 29273, "Ġholders": 29274, "Ġbooster": 29275, "ĠSasha": 29276, "ÑĭваеÑĤ": 29277, "ģ¼": 29278, "Ġseparating": 29279, "Ġreinforcement": 29280, "Ġодной": 29281, "ìĹĨ": 29282, "IDE": 29283, "ĠOption": 29284, "phon": 29285, "Ġplais": 29286, "ĠCamb": 29287, "ĠíĻĺ": 29288, "Ġuncommon": 29289, "\":": 29290, "miyorum": 29291, "moi": 29292, "acje": 29293, "ажÑĥ": 29294, "Õ¶": 29295, "Ġgems": 29296, "üler": 29297, "ools": 29298, "Ġenzymes": 29299, "Ġkidnapped": 29300, "Ġketchup": 29301, "talk": 29302, "Ġzach": 29303, "Ġwasher": 29304, "ãĢĤãĢĤ": 29305, "ĠArchitect": 29306, "venue": 29307, "ĠPlanning": 29308, "éĢģ": 29309, "ĠSavior": 29310, "ĠгÑĢÑĥпп": 29311, "íĬ¼": 29312, "arya": 29313, "Ġproceso": 29314, "Ġlimbs": 29315, "Ġrealizes": 29316, "iander": 29317, "FS": 29318, "aji": 29319, "Ġunite": 29320, "ĠìĿĺë": 29321, "ĠpossÃŃvel": 29322, "raits": 29323, "ĠAgre": 29324, "ÛĮÚ©": 29325, "ìĦľëıĦ": 29326, "æİī": 29327, "Ġвел": 29328, "ĠмеÑģÑı": 29329, "anor": 29330, "Pat": 29331, "Ġdernier": 29332, "ÏĥÏĦε": 29333, "ĠкакаÑı": 29334, "Ġlässt": 29335, "æİ°": 29336, "ĠMeh": 29337, "Ġngh": 29338, "Ġamateur": 29339, "è«ĸ": 29340, "Fe": 29341, "Ġê¶ģ": 29342, "Ġsituación": 29343, "Ġsedan": 29344, "Ġcleansing": 29345, "lasting": 29346, "Ġcommunist": 29347, "ANE": 29348, "Ġirregular": 29349, "Ġsout": 29350, "ĠCarney": 29351, "Ġallemaal": 29352, "ĠmuchÃŃs": 29353, "Ġlibro": 29354, "ÐŃÑĤо": 29355, "Ġап": 29356, "Ġcontinuation": 29357, "ĠLor": 29358, "?\",": 29359, "quin": 29360, "Ġcharacterized": 29361, "ajes": 29362, "Ġsights": 29363, "ĠÑıзÑĭ": 29364, "ĠUhh": 29365, "è·³": 29366, "birth": 29367, "dong": 29368, "Ġhablando": 29369, "Ġsymptom": 29370, "çµĤ": 29371, "Ġcapacitor": 29372, "Ġtransported": 29373, "Ġignorant": 29374, "Ġникогда": 29375, "Ġdrip": 29376, "ĠEva": 29377, "Ġadject": 29378, "Ġmassively": 29379, "ĠEthi": 29380, "ĠCircle": 29381, "Ġrainfall": 29382, "ĠMouse": 29383, "Ġrefund": 29384, "ĠZw": 29385, "assemb": 29386, "Ġ220": 29387, "ĠOrd": 29388, "è§Ĵ": 29389, "Ġveins": 29390, "ĠGiant": 29391, "Ġmãe": 29392, "Ġvap": 29393, "Ġmisses": 29394, "οÏħÏĤ": 29395, "Mo": 29396, "ĠEntwick": 29397, "INT": 29398, "ÙĨت": 29399, "Ġtheoretically": 29400, "Ġtearing": 29401, "Ġtroubled": 29402, "prem": 29403, "Ġrepetitive": 29404, "Ġâĸ": 29405, "Ġheavenly": 29406, "ĠAmber": 29407, "Ġполож": 29408, "Ġíķ´ì¤": 29409, "Ġvowel": 29410, "anking": 29411, "ĠWirtschaft": 29412, "Ġirr": 29413, "Ġcozy": 29414, "Ġunfamiliar": 29415, "ĠPors": 29416, "Ġë§ŀìķĦ": 29417, "ĠTimothy": 29418, "ÑģолÑİÑĤ": 29419, "pex": 29420, "ĠVIS": 29421, ")(": 29422, "Ġsuperst": 29423, "Ġimprov": 29424, "ĠBeng": 29425, "Ġdisconnected": 29426, "Ġapt": 29427, "ÑĢен": 29428, "ĠExtra": 29429, "Ġбел": 29430, "shop": 29431, "dings": 29432, "ĠConnecticut": 29433, "ì°¬": 29434, "ĠGC": 29435, "åıĸ": 29436, "beh": 29437, "Jeremy": 29438, "ĠBatt": 29439, "ãģ¸": 29440, "atha": 29441, "ĠZusammen": 29442, "screams": 29443, "Ġgras": 29444, "afft": 29445, "ĠInitially": 29446, "ĠBrett": 29447, "Ġspecifications": 29448, "Ġseaweed": 29449, "Ġoath": 29450, "Ġfountain": 29451, "ĠкоÑĤоÑĢой": 29452, "ĠStein": 29453, "èģ²": 29454, "ĠCorinth": 29455, "Ġconjug": 29456, "å·¦åı³": 29457, "Ġcompensate": 29458, "ĠëĬIJëĤĮìĿ´": 29459, "Ġonze": 29460, "Ġskincare": 29461, "Brian": 29462, "Ġservir": 29463, "}}": 29464, "ĠVik": 29465, "Ġunint": 29466, "Ġsuppliers": 29467, "Ġbalcony": 29468, "Ġenergia": 29469, "ometric": 29470, "зÑı": 29471, "Ġsigh": 29472, "ĠTOM": 29473, "ĠPure": 29474, "ytt": 29475, "ÑĭÑģ": 29476, "ĠRainbow": 29477, "ĠPitts": 29478, "×Ļ×ŀ": 29479, "Ġstatues": 29480, "heads": 29481, "Ġcoupled": 29482, "éĮ¢": 29483, "Ġherd": 29484, "ä½ĵ": 29485, "Ġexcluded": 29486, "Ġgilt": 29487, "ĠÑİ": 29488, "Ġswoje": 29489, "ĠSver": 29490, "63": 29491, "issant": 29492, "Ġdürfen": 29493, "łĪë": 29494, "Ġkissing": 29495, "oof": 29496, "以ä¸Ĭ": 29497, "Ġcursed": 29498, "Ġshowers": 29499, "Ġswinging": 29500, "Ġreproduce": 29501, "ãģ¨ãģĦãģĨãģĵãģ¨": 29502, "Ġsätt": 29503, "elcome": 29504, "Ġfundamentals": 29505, "Ġalmond": 29506, "Ġpé": 29507, "Ġwellbeing": 29508, "Ġhunters": 29509, "å¾Ģ": 29510, "Sec": 29511, "ĵľë¦´": 29512, "Ġemission": 29513, "Ġpsychologist": 29514, "Ġbetrayed": 29515, "ĠReynolds": 29516, "LES": 29517, "Ġpolling": 29518, "Ġnegatively": 29519, "Ġcombines": 29520, "׾×IJ": 29521, "аÑĢа": 29522, "λλά": 29523, "ĠTurns": 29524, "OTT": 29525, "Ġ×Ķ×Ļ": 29526, "aison": 29527, "Ġairline": 29528, "Ġrestriction": 29529, "wal": 29530, "Ġaurait": 29531, "ĠLebanon": 29532, "ĠMOR": 29533, "Ġmonkeys": 29534, "éner": 29535, "ÑĸÑĹ": 29536, "Ġmotherf": 29537, "ĠÙĩذÙĩ": 29538, "Ġfeu": 29539, "ühren": 29540, "Ġhygiene": 29541, "enteen": 29542, "Des": 29543, "Ġdissip": 29544, "Est": 29545, "Ġsaints": 29546, "Ġpotassium": 29547, "Ġreckon": 29548, "Clintus": 29549, "Ġmanifestation": 29550, "ĠAppro": 29551, "ĠInspect": 29552, "Ġventilation": 29553, "Ġhelm": 29554, "Ġkara": 29555, "าà¸Ļ": 29556, "Ġfavorable": 29557, "ĠìķĬìķĺ": 29558, "ĠHispanic": 29559, "à¸ľ": 29560, "Ġ×Ķ׼": 29561, "Ġvalidate": 29562, "ĠResident": 29563, "Ġcomenz": 29564, "beiter": 29565, "erer": 29566, "ä¸Ģèµ·": 29567, "Ġdado": 29568, "atching": 29569, "metros": 29570, "ĠHin": 29571, "ĠDum": 29572, "Ġhazır": 29573, "ĠNatalie": 29574, "Ġencryption": 29575, "оÑĩка": 29576, "mma": 29577, "houses": 29578, "Ġanalytical": 29579, "ĠDang": 29580, "first": 29581, "æŃĮ": 29582, "çºĮ": 29583, "ĠEnc": 29584, "cando": 29585, "Ġludzi": 29586, "wart": 29587, "Ġstatistic": 29588, "ĠìĤ°": 29589, "Ġcommenting": 29590, "Ġcoordinated": 29591, "ĠHyper": 29592, "åļ": 29593, "ĠBert": 29594, "çľ¾": 29595, "ĠHip": 29596, "kem": 29597, "ünü": 29598, "Ġzal": 29599, "ĠíķĺëĬĶëį°": 29600, "ĠRobot": 29601, "éĸ±": 29602, "rawn": 29603, "Ġrhetoric": 29604, "ullah": 29605, "ĠDiet": 29606, "Ġtakich": 29607, "Ġpossessed": 29608, "ĵľëĬĶ": 29609, "Ġwakes": 29610, "ĠRaf": 29611, "Mart": 29612, "Ġecc": 29613, "ĠFM": 29614, "Ġdific": 29615, "ĠAllez": 29616, "Ġcured": 29617, "åѦ": 29618, "ĠQuad": 29619, "Ġbele": 29620, "Ġjournals": 29621, "Ġtad": 29622, "Ġsociales": 29623, "æĩĤ": 29624, "Ġwhats": 29625, "ĠBass": 29626, "Ġjestem": 29627, "ĠSadly": 29628, "ĠSource": 29629, "Ġüç": 29630, "altung": 29631, "ierten": 29632, "Ġjullie": 29633, "ifa": 29634, "ĠÐļоÑĢ": 29635, "ĠDoor": 29636, "ĠÐĿад": 29637, "ĠздоÑĢов": 29638, "Ġrumor": 29639, "Ġpies": 29640, "ĠпеÑĢе": 29641, "ĠоÑĤв": 29642, "еннÑĭе": 29643, "Host": 29644, "ĠSophie": 29645, "anten": 29646, "Any": 29647, "ĠAufg": 29648, "ç¨ĭ": 29649, "ĠHDR": 29650, "ĠRocket": 29651, "resso": 29652, "Ġverde": 29653, "Ġprésident": 29654, "Ġindoors": 29655, "Ġstagger": 29656, "Ġstato": 29657, "ĠDial": 29658, "Ġbuzzing": 29659, "emer": 29660, "ĠÐĴÑģÑij": 29661, "ĠдеÑĢев": 29662, "Ġpouv": 29663, "Ġstrands": 29664, "Ġê²ĥìĿ´": 29665, "ĠParl": 29666, "окой": 29667, "Ġsip": 29668, "Ġ(*": 29669, "ängt": 29670, "Ġdeber": 29671, "ĠAin": 29672, "Ġdrastically": 29673, "ĠSlowly": 29674, "ĠBrig": 29675, "ĠTorah": 29676, "Ġache": 29677, "Ġ???": 29678, "ĠDob": 29679, "kannt": 29680, "Mary": 29681, "Ġstam": 29682, "ĠDemon": 29683, "pla": 29684, "ĠFreund": 29685, "ĠBenn": 29686, "Ġhighs": 29687, "Ġکر": 29688, "ĠPrepare": 29689, "Ġproxy": 29690, "Ġcampo": 29691, "ĠAugen": 29692, "£¨ë": 29693, "ĠChloe": 29694, "icularly": 29695, "young": 29696, "ĠãģĮ": 29697, "©Ķë": 29698, "Ġscratching": 29699, "Ġglac": 29700, "Ġgemeinsam": 29701, "anal": 29702, "acaksın": 29703, "ĠForum": 29704, "ennial": 29705, "ĠResources": 29706, "ã썿ĢĿãģĦãģ¾ãģĻ": 29707, "Ġmeisten": 29708, "ĠFell": 29709, "Ġunanim": 29710, "ĠTB": 29711, "ĠSelbst": 29712, "æĨ": 29713, "Ġintimidating": 29714, "ĠGefühl": 29715, "Ġì½Ķë¡ľ": 29716, "æĭī": 29717, "idor": 29718, "iciones": 29719, "arsa": 29720, "]..": 29721, "azo": 29722, "Ġkendi": 29723, "ĠTage": 29724, "termin": 29725, "ĠProzent": 29726, "Maybe": 29727, "lé": 29728, "Ġquesti": 29729, "Ġmemes": 29730, "Ġcorre": 29731, "ĠVIP": 29732, "ĠGallery": 29733, "Ġurgency": 29734, "Ġnoche": 29735, "Ġkindly": 29736, "ĠMeredith": 29737, "ĠváºŃy": 29738, "ĠاÙĦب": 29739, "ĠEstado": 29740, "åĩºä¾Ĩ": 29741, "zug": 29742, "oque": 29743, "Ġobesity": 29744, "Off": 29745, "ĠEuropeans": 29746, "öd": 29747, "ì¹´ë": 29748, "Ġhoop": 29749, "Ġenjoys": 29750, "ĠChip": 29751, "patient": 29752, "Ġmicroscope": 29753, "Ġlegitim": 29754, "ĠÑıвлÑıеÑĤÑģÑı": 29755, "Ïĥι": 29756, "argent": 29757, "Ġsham": 29758, "Ġlicensing": 29759, "olia": 29760, "Sorry": 29761, "rama": 29762, "Ġaccelerated": 29763, "Ġwym": 29764, "Ġfairness": 29765, "ĠReading": 29766, "Ġslack": 29767, "ĠDok": 29768, "ziÄĻkujÄĻ": 29769, "Ġrubbing": 29770, "аÑĤÑĥ": 29771, "Ġallocated": 29772, "jung": 29773, "Ġpains": 29774, "Ġwinding": 29775, "Ġgeliyor": 29776, "ĠCU": 29777, "mot": 29778, "cock": 29779, "ĠPosition": 29780, "bros": 29781, "Ġlivestream": 29782, "ĠBrain": 29783, "ì°©": 29784, "Ġprzek": 29785, "ĠEi": 29786, "ĠCoco": 29787, "ба": 29788, "Ġshovel": 29789, "ãĥıãĥı": 29790, "ea": 29791, "Ġchocol": 29792, "Ġrebellion": 29793, "Ġshowc": 29794, "ĠHalo": 29795, "Ġdividend": 29796, "mission": 29797, "Ġusando": 29798, "Ġ[\"": 29799, "Ġfalei": 29800, "æĽ¸": 29801, "Black": 29802, "ĠSurely": 29803, "ĠÅ»": 29804, "Ġphilosopher": 29805, "ä½łä»¬": 29806, "Ġoverhe": 29807, "ĠBorn": 29808, "Ġobjetivo": 29809, "Ġ128": 29810, "scheid": 29811, "ĠNazis": 29812, "Ġsolche": 29813, "lift": 29814, "cede": 29815, "adors": 29816, "Ġmarshm": 29817, "ĠLORD": 29818, "ĶìĿ´íģ¬": 29819, "Ġowning": 29820, "Cont": 29821, "Ġlandscapes": 29822, "Ġlending": 29823, "ĠAuthority": 29824, "овой": 29825, "oqu": 29826, "ĠSes": 29827, "ĠFerrari": 29828, "Ġresponsabil": 29829, "Ġvários": 29830, "Ġdelic": 29831, "Ġembark": 29832, "Ġembroider": 29833, "Ġframeworks": 29834, "Ġsimmer": 29835, "Ġnacional": 29836, "Ġremainder": 29837, "ĠVielleicht": 29838, "Ġquieres": 29839, "ìĹĶ": 29840, "Ġtestoster": 29841, "ihen": 29842, "ĠOz": 29843, "èle": 29844, "Ġportrayed": 29845, "κε": 29846, "ĠPolitik": 29847, "Ġaperture": 29848, "Ġbland": 29849, "indust": 29850, "ĠобÑĢаÑĤ": 29851, "ĠThous": 29852, "Bay": 29853, "Ġdando": 29854, "Ġsher": 29855, "Ġadmissions": 29856, "ĠCrew": 29857, "ĠÑĸн": 29858, "SINGING": 29859, "Ġounce": 29860, "Ġiy": 29861, "Ġbasil": 29862, "Ġovertime": 29863, "Ġthreaten": 29864, "Ġpartnered": 29865, "ĠCann": 29866, "avana": 29867, "ĠзнаеÑĤе": 29868, "éĢĻäºĽ": 29869, "ĠоÑĤÑģ": 29870, "ĠTudo": 29871, "ì½Ķ": 29872, "ĠëĨĢë": 29873, "fel": 29874, "Ġrearr": 29875, "Ġinward": 29876, "ĠRogers": 29877, "à¹ĥห": 29878, "Ġtweak": 29879, "Ġdryer": 29880, "cession": 29881, "Ġrigorous": 29882, "ĠDaar": 29883, "omics": 29884, "Ġfats": 29885, "vad": 29886, "Ġzipper": 29887, "acceptable": 29888, "Ġdemonstrating": 29889, "ĠYum": 29890, "Ġbeau": 29891, "Ġroster": 29892, "Ġpredominantly": 29893, "еÑĢÑĥ": 29894, "ningar": 29895, "Ġtriangles": 29896, "Ġtexting": 29897, "Ġberries": 29898, "ĠìĤ¬ì§Ħ": 29899, "éĶĻ": 29900, "adder": 29901, "Ġfaites": 29902, "ĠImage": 29903, "lere": 29904, "Ġbounds": 29905, "ĠLaur": 29906, "ĠìķĦ무ë": 29907, "Ġmio": 29908, "Ġusa": 29909, "Ġذ": 29910, "Ġtoen": 29911, "ĠJang": 29912, "že": 29913, "chod": 29914, "anan": 29915, "ĠобÑĢазом": 29916, "Ġpersever": 29917, "ĠSwe": 29918, "Ġaugment": 29919, "ä¸ĥ": 29920, "uggling": 29921, "ièrement": 29922, "istles": 29923, "acjÄĻ": 29924, "91": 29925, "Ġmah": 29926, "ĠKIR": 29927, "Die": 29928, "Ġdownhill": 29929, "Ġ1968": 29930, "оÑĢоÑĪо": 29931, "å¹¹": 29932, "ographics": 29933, "Ġtässä": 29934, "ê²łì£ł": 29935, "ĠлиÑĩ": 29936, "AUDIO": 29937, "ĠплоÑħ": 29938, "Ġproposing": 29939, "éł»": 29940, "Ġtempted": 29941, "Ġconverting": 29942, "ĠLehr": 29943, "Ġpersone": 29944, "ĠFeeling": 29945, "ìĸ´ì£¼": 29946, "ombres": 29947, "Ġ׾×Ļ": 29948, "Ġguru": 29949, "Ġdement": 29950, "низ": 29951, "иÑĤелей": 29952, "Ġcompañ": 29953, "æľª": 29954, "å¸ĮæľĽ": 29955, "Ġredo": 29956, "Ġconductor": 29957, "mia": 29958, "Ġidols": 29959, "ĠMul": 29960, "Ġinex": 29961, "Ġtämä": 29962, "Ġimpacting": 29963, "Ġdaylight": 29964, "gil": 29965, "Ġhelfen": 29966, "Ġentsprech": 29967, "ĠwiÄĻks": 29968, "Ġscriptures": 29969, "Ġdismissed": 29970, "ãĥ³ãĥĪ": 29971, "ĠPodcast": 29972, "Ùħر": 29973, "Ġannually": 29974, "Ġusable": 29975, "Ġlibre": 29976, "озм": 29977, "Ġrubbish": 29978, "çļĦ人": 29979, "Ġcontinuar": 29980, "Ġhumili": 29981, "Ġspeeches": 29982, "ÑĢаÑĩ": 29983, "bard": 29984, "71": 29985, "><": 29986, "ologÃŃa": 29987, "wealth": 29988, "Ġmeditate": 29989, "ĵ¤ìĿĺ": 29990, "ĠCraft": 29991, "è§īå¾Ĺ": 29992, "æĻ®": 29993, "riv": 29994, "ĠAgainst": 29995, "Ġceramic": 29996, "espère": 29997, "Ġcompetent": 29998, "ĠHopkins": 29999, "Ġkilos": 30000, "Ġgravel": 30001, "Ġpiston": 30002, "Ġfriendships": 30003, "Ġescre": 30004, "Ġvoz": 30005, "ĠGesellschaft": 30006, "Ġunterstüt": 30007, "Ġmuj": 30008, "Ġwarnings": 30009, "pos": 30010, "ĠProfessional": 30011, "wszy": 30012, "odle": 30013, "bands": 30014, "Ġteamwork": 30015, "stellung": 30016, "Ġdx": 30017, "åįĬ": 30018, "Ġattorneys": 30019, "Ġweitere": 30020, "ãħĭãħĭãħĭ": 30021, "ĠOriginal": 30022, "×Ļ×Ĺ": 30023, "Ġbroadcasting": 30024, "ĠпеÑĢвÑĭй": 30025, "uchi": 30026, "Ġheure": 30027, "Ġgrabs": 30028, "ĠWOR": 30029, "ĠPlaid": 30030, "Min": 30031, "Ġpaz": 30032, "ĠPuis": 30033, "umu": 30034, "itates": 30035, "Ġcoats": 30036, "Ġbuen": 30037, "Ġheir": 30038, "Ġpneum": 30039, "שר": 30040, "enser": 30041, "ĠJUDGE": 30042, "Ġblonde": 30043, "á¹Ľ": 30044, "Ġgak": 30045, "Ġsık": 30046, "Ġquoted": 30047, "Ġequipo": 30048, "Ġwishing": 30049, "ÃŃcia": 30050, "Ġverbs": 30051, "çµĦ": 30052, "ĠCanadians": 30053, "Ġgoverning": 30054, "ĠEvans": 30055, "Euro": 30056, "Ġgenres": 30057, "Ġunterschied": 30058, "ĠBecky": 30059, "³¼ê²ĮìļĶ": 30060, "Ġeinge": 30061, "ĠRaise": 30062, "oland": 30063, "ĠStrateg": 30064, "Ġeres": 30065, "ĠVeterans": 30066, "Ġbreakout": 30067, "Ġsanté": 30068, "Ġadel": 30069, "Ġinvestigated": 30070, "Ġpeur": 30071, "Ġagile": 30072, "Ġrailroad": 30073, "anska": 30074, "Ġей": 30075, "Ġexpos": 30076, "atories": 30077, "ĠContent": 30078, "Ġtruths": 30079, "ĠTrail": 30080, "Ġgua": 30081, "Ġpores": 30082, "Ġwritings": 30083, "ĠUhr": 30084, "ĠThats": 30085, "Ġicing": 30086, "OC": 30087, "ĠProduction": 30088, "Ġcarne": 30089, "ISS": 30090, "Ġninguém": 30091, "non": 30092, "Ġvicious": 30093, "×ķ×Ķ": 30094, "Ġreconnect": 30095, "Ġcentres": 30096, "ĠKem": 30097, "Ġcrease": 30098, "ĠìĿ´ë¯¸": 30099, "айÑĤеÑģÑĮ": 30100, "ĠбоÑĢ": 30101, "ĠHayır": 30102, "ĠÑģÑĥд": 30103, "Ġúnica": 30104, "owaÅĤ": 30105, "Ġadher": 30106, "hua": 30107, "ZZ": 30108, "Ġpreciso": 30109, "Ġcurrents": 30110, "Ġseasoned": 30111, "ĠIoT": 30112, "ĠBishop": 30113, "è¨Ī": 30114, "sted": 30115, "ĠBernard": 30116, "ì¤ĺ": 30117, "æ²»": 30118, "ĠGlenn": 30119, "Ġktórym": 30120, "ืà¹Ī": 30121, "Ġastrolog": 30122, "ĠKot": 30123, "å¤ľ": 30124, "Ġparfois": 30125, "Ġforwards": 30126, "ĠWiÄĻ": 30127, "ĠÎĺ": 30128, "Ġnano": 30129, "è»į": 30130, "sub": 30131, "ĠBrill": 30132, "Ġgrit": 30133, "Ġcited": 30134, "gado": 30135, "Ġmelts": 30136, "Ġforcé": 30137, "âĸĪâĸĪ": 30138, "Ġbajo": 30139, "Ġdiscretion": 30140, "°°": 30141, "ativity": 30142, "Ġsituated": 30143, "ãĥ«ãĤ¯": 30144, "Ñīее": 30145, "åľ°æĸ¹": 30146, "ĠпÑĢинÑĨип": 30147, "amaz": 30148, "Ġaquarium": 30149, "Ġdissolve": 30150, "ĠGods": 30151, "Super": 30152, "Ġamid": 30153, "zk": 30154, "ĠãģĦ": 30155, "éłIJ": 30156, "ampf": 30157, "Ġhela": 30158, "'!": 30159, "Ġdevelopmental": 30160, "ĠDise": 30161, "ĠÑĢабоÑĤаеÑĤ": 30162, "Ġsnapshot": 30163, "好好": 30164, "Õ¸": 30165, "ĠYue": 30166, "ĠHulk": 30167, "ĠDoom": 30168, "ĠFelix": 30169, "Ġréf": 30170, "Male": 30171, "ç·Ĭ": 30172, "phants": 30173, "ENS": 30174, "ĠMechan": 30175, "ĠGolf": 30176, "åĨįè¦ĭ": 30177, "Ġgenerosity": 30178, "ätze": 30179, "Ġunlocked": 30180, "ĠãĤĴ": 30181, "íĥģ": 30182, "ocalypse": 30183, "Alright": 30184, "Ġê°ľë": 30185, "Ġ×IJ×ij׾": 30186, "ĠKeeping": 30187, "Ġcollaborating": 30188, "chief": 30189, "ĠFernando": 30190, "Ġchefs": 30191, "Ġíͼë¶Ģ": 30192, "Ġskipped": 30193, "Ġpersonn": 30194, "Ġaxe": 30195, "chez": 30196, "Ġextraction": 30197, "ĠAV": 30198, "ĠGibbs": 30199, "Ġíľ": 30200, "Ġsı": 30201, "IAM": 30202, "View": 30203, "ĠGRANT": 30204, "Ġ몸": 30205, "Ġverification": 30206, "Ġdepicted": 30207, "ĠMoz": 30208, "oux": 30209, "Ġtul": 30210, "Ġscanner": 30211, "Ġcomedian": 30212, "ĠVolks": 30213, "ĠJEFF": 30214, "è¨Ĥéĸ±": 30215, "§Ħ": 30216, "Ġdistraction": 30217, "rá": 30218, "ĠINTER": 30219, "Ġsincer": 30220, "Ġ×ŀת": 30221, "Ġש׳": 30222, "Ġconstructive": 30223, "arf": 30224, "ĠëĪĦë": 30225, "Ġeco": 30226, "ramos": 30227, "Ġrenewed": 30228, "inement": 30229, "ĠUb": 30230, "ĠPepper": 30231, "ì§Ģê°Ģ": 30232, "ĠDarwin": 30233, "Ġmerchand": 30234, "Ġvárias": 30235, "èce": 30236, "NG": 30237, "ĠìľĦíķ´ìĦľ": 30238, "ĠакÑĤив": 30239, "ĠUnters": 30240, "عÙĦ": 30241, "Ġintric": 30242, "omma": 30243, "ieving": 30244, "ĠCaroline": 30245, "åĵģ": 30246, "ĠPRES": 30247, "Ġperformer": 30248, "Ġautour": 30249, "ãģ¾ãģĽãĤĵ": 30250, "Ġutterly": 30251, "Ġsynthesis": 30252, "Ġlesbian": 30253, "Ġretrieve": 30254, "Ġmaneira": 30255, "Ġimpair": 30256, "Ġmentoring": 30257, "ĠSouls": 30258, "ĠGoPro": 30259, "ÑĢаÑĤÑĮ": 30260, "Ġcose": 30261, "ĠSSD": 30262, "IRE": 30263, "Ġupfront": 30264, "ĠAun": 30265, "Ġgamer": 30266, "Ġlitt": 30267, "Ġaggression": 30268, "ĠLikewise": 30269, "ĠBetty": 30270, "ĠDart": 30271, "ĠDLC": 30272, "ishment": 30273, "ìŀ¥ìĿĦ": 30274, "Ġ对": 30275, "ç»ı": 30276, "cream": 30277, "ĠBabylon": 30278, "Ġnug": 30279, "brar": 30280, "Ġaynı": 30281, "amily": 30282, "bike": 30283, "ahahaha": 30284, "loyd": 30285, "Ġmira": 30286, "Ġperme": 30287, "ĠGaming": 30288, "Ġfirmware": 30289, "Ma": 30290, "Ġassisted": 30291, "atics": 30292, "Ġìķŀìľ¼ë¡ľ": 30293, "ĠMental": 30294, "niejs": 30295, "ĠIz": 30296, "owÄħ": 30297, "Ġtougher": 30298, "Ġdeed": 30299, "èĭ¦": 30300, "Ġstylish": 30301, "ĠTools": 30302, "ĠHamp": 30303, "Ġsunscreen": 30304, "Ġarticulate": 30305, "iye": 30306, "иÑĦ": 30307, "ĠSpread": 30308, "ĠHAVE": 30309, "Ġswirl": 30310, "Ġsponsoring": 30311, "ä»ĭ": 30312, "iovascular": 30313, "mesi": 30314, "Ġrelaxation": 30315, "ĠÑģвоиÑħ": 30316, "Ġmargins": 30317, "ĠsaÄŁ": 30318, "ĠPride": 30319, "ĠÏĦοÏħÏĤ": 30320, "иÑĨи": 30321, "enci": 30322, "Does": 30323, "Ġcorpse": 30324, "Ġendurance": 30325, "Ġíŀĺ": 30326, "ì¹´": 30327, "Ġhaircut": 30328, "Ġinterrupted": 30329, "Ġwindy": 30330, "ĠCaleb": 30331, "ÏģÏĩ": 30332, "ĠPourquoi": 30333, "Ġholistic": 30334, "uclear": 30335, "ĠWhole": 30336, "士": 30337, "Act": 30338, "Ġgallon": 30339, "cade": 30340, "ĠRegional": 30341, "roads": 30342, "ĠSchne": 30343, "áng": 30344, "Ġизмен": 30345, "ãĤĪãģŃ": 30346, "Ġmenus": 30347, "Ġsplitting": 30348, "Ġpriced": 30349, "ĠÎĵ": 30350, "Ġusername": 30351, "ĠÐŀÑĩ": 30352, "Ġcompressed": 30353, "yin": 30354, "Ġguardian": 30355, "Ġgoof": 30356, "Ġchecklist": 30357, "Ġinterchange": 30358, "Ġexpedition": 30359, "Ġextern": 30360, "Ġinfrared": 30361, "engo": 30362, "Ġdenying": 30363, "Ġpackets": 30364, "onent": 30365, "BB": 30366, "ĠIncre": 30367, "Ġsini": 30368, "ÃŁer": 30369, "èg": 30370, "maal": 30371, "generation": 30372, "Ġminorities": 30373, "Ġllevar": 30374, "Ġnomination": 30375, "Ġconsid": 30376, "Ġ×ľ×¢": 30377, "muÅŁ": 30378, "ĠEsc": 30379, "Ġnumerator": 30380, "Ġkaik": 30381, "Ġktórych": 30382, "iesen": 30383, "Ġvê": 30384, "ĠUSS": 30385, "ĠPrivate": 30386, "Ġодно": 30387, "Ġalém": 30388, "ÃŃtulo": 30389, "Ġlimb": 30390, "Ġforgiven": 30391, "Ġdisclosure": 30392, "ÏĦί": 30393, "Ġningún": 30394, "Ġtherapeutic": 30395, "Ġnegotiating": 30396, "ĠNike": 30397, "enseful": 30398, "Ġincap": 30399, "Ġflagship": 30400, "town": 30401, "âĪ": 30402, "ĠÏĢολ": 30403, "Ġwolves": 30404, "Ġviolations": 30405, "ĠArnold": 30406, "Ġintervene": 30407, "Ġheater": 30408, "Ġrecursos": 30409, "Ġmaid": 30410, "ê²¼": 30411, "ĠдавайÑĤе": 30412, "ĠCelebr": 30413, "Ġcape": 30414, "ĠSty": 30415, "ainen": 30416, "site": 30417, "bij": 30418, "ĠполÑĮз": 30419, "Ġframed": 30420, "Ġpublishers": 30421, "ĠÑĩÑĥÑĤÑĮ": 30422, "Ġtemptation": 30423, "Ġcerteza": 30424, "Ġexempt": 30425, "ìĬ¹": 30426, "selling": 30427, "ĠTask": 30428, "hoon": 30429, "ĠCoc": 30430, "ĠParks": 30431, "Ġrepetition": 30432, "ĠÑĤÑĥда": 30433, "Ġensl": 30434, "ĠdeÄŁiÅŁ": 30435, "ĠOrlando": 30436, "ĠMainten": 30437, "æŃ¢": 30438, "ocument": 30439, "ĠHC": 30440, "Ġscooter": 30441, "ĠнапиÑģ": 30442, "Ġtighter": 30443, "Ġtease": 30444, "Ġremoves": 30445, "Ġkijken": 30446, "ĠÑģÑĥÑīеÑģÑĤв": 30447, "Ġthé": 30448, "ĠвÑĭглÑıд": 30449, "Ġrelieve": 30450, "Ġmitä": 30451, "Ġstationary": 30452, "öff": 30453, "pable": 30454, "Ġarter": 30455, "Ġdéf": 30456, "rative": 30457, "Ġconect": 30458, "Ġsaddle": 30459, "ĠDiane": 30460, "Ġcommemor": 30461, "fendim": 30462, "SÃŃ": 30463, "Ġíģ´ë": 30464, "Ġmange": 30465, "atte": 30466, "Ġarrogant": 30467, "Ġrobotic": 30468, "ĠgiÃł": 30469, "æĺ¯çļĦ": 30470, "Ġneighbourhood": 30471, "isson": 30472, "Ġдвиж": 30473, "ĠRI": 30474, "ĠNorman": 30475, "brand": 30476, "amation": 30477, "Ġrazor": 30478, "Ġmurders": 30479, "ĠÑĤÑĥ": 30480, "Ġwszystkim": 30481, "Ġutilities": 30482, "Ġmicroscop": 30483, "ê¿": 30484, "Ġdaqui": 30485, "ollar": 30486, "ĠÐĶавайÑĤе": 30487, "Ġannée": 30488, "Ġkilometres": 30489, "Ġhomosexual": 30490, "Ġarchitects": 30491, "ãģ¡ãģ¯": 30492, "Ġniye": 30493, "LER": 30494, "Ġmicrophones": 30495, "ĠStunden": 30496, "Ġconsecutive": 30497, "ienda": 30498, "vänd": 30499, "DER": 30500, "Ġlifts": 30501, "ĠMeat": 30502, "Ġsavez": 30503, "íĸĪëįĺ": 30504, "Men": 30505, "Ġdismant": 30506, "거를": 30507, "Ġinsulation": 30508, "Ġscall": 30509, "Ġspooky": 30510, "Ġparc": 30511, "Ġballet": 30512, "ĠWhatsApp": 30513, "Ġfranc": 30514, "Ġdeliberate": 30515, "ĠíħĮ": 30516, "Ġmars": 30517, "ĠZur": 30518, "Pr": 30519, "disciplinary": 30520, "Ġobsession": 30521, "ме": 30522, "Ġmarching": 30523, "ĠEmergency": 30524, "iguous": 30525, "Ġszy": 30526, "ĠLands": 30527, "Ġboarding": 30528, "ĠпоÑĩÑĤи": 30529, "Ġenvy": 30530, "Ġcompassionate": 30531, "Ġmerci": 30532, "Ġdesirable": 30533, "dale": 30534, "Ġcanım": 30535, "ĠAntar": 30536, "temps": 30537, "Ġconfigured": 30538, "ĠCompared": 30539, "neh": 30540, "icating": 30541, "Ġnickel": 30542, "ÙĪÙĤ": 30543, "ÙĥÙĪÙĨ": 30544, "opes": 30545, "Ġformulas": 30546, "ĠÐķÑģÑĤÑĮ": 30547, "Ġpobl": 30548, "ĠPJ": 30549, "ĠLud": 30550, "ä»ĬåĽŀ": 30551, "ĠBrid": 30552, "ĠHog": 30553, "ĠBris": 30554, "Jen": 30555, "Ġshading": 30556, "ĠYas": 30557, "Ġdisturbed": 30558, "Ġrecommending": 30559, "Ġcé": 30560, "ĠHOW": 30561, "ìĹĪìĸ´": 30562, "Ġreversed": 30563, "ĠInterestingly": 30564, "ioxid": 30565, "åħŃ": 30566, "Ġìĺ¤ì¼ĢìĿ´": 30567, "ếu": 30568, "xx": 30569, "Ġouais": 30570, "ĠYouTubers": 30571, "ĠRosa": 30572, "ĠHaupt": 30573, "jadi": 30574, "Ġvlogs": 30575, "Ġcultura": 30576, "ĠLeadership": 30577, "ĠHep": 30578, "Ġillum": 30579, "´ëıĻ": 30580, "Ġcustomized": 30581, "Ġmarca": 30582, "Ġquatro": 30583, "Ġнаг": 30584, "ĠSpaceX": 30585, "ĠEigen": 30586, "asting": 30587, "ĠolduÄŁu": 30588, "Ġforts": 30589, "ãģī": 30590, "riment": 30591, "iencia": 30592, "Ġtenir": 30593, "roffen": 30594, "Ġ1979": 30595, "Ġcie": 30596, "ĠëIJĺê³ł": 30597, "Ġescri": 30598, "ÏĮÏĤ": 30599, "íı¬": 30600, "uzzy": 30601, "Cong": 30602, "ìĿ¸ìĿ´": 30603, "Great": 30604, "sil": 30605, "éch": 30606, "ãģ¨ãģĭ": 30607, "Ġmultic": 30608, "ĠDisk": 30609, "²ķ": 30610, "Ġfazla": 30611, "Ġlevant": 30612, "Ġabajo": 30613, "urry": 30614, "stru": 30615, "Ġ먹ëĬĶ": 30616, "Ġaccessory": 30617, "Ġдвиг": 30618, "ĠRid": 30619, "2019": 30620, "Ġdownstream": 30621, "æķ¸": 30622, "Ġkaz": 30623, "utan": 30624, "Ġcharcoal": 30625, "Ġafect": 30626, "wu": 30627, "Ġcontexts": 30628, "Ġfeared": 30629, "ĠìĦ¤": 30630, "Ġhistories": 30631, "Ġfas": 30632, "ensible": 30633, "Ġcocoa": 30634, "illar": 30635, "geons": 30636, "Ġspirituality": 30637, "ĠPew": 30638, "Ġpharmacy": 30639, "Ġpassions": 30640, "Ġbos": 30641, "Ġallá": 30642, "Ġthriving": 30643, "ĠReact": 30644, "Ġoccupy": 30645, "Ġwithdrawal": 30646, "Ġallowance": 30647, "ĠFraktion": 30648, "Ġbuddies": 30649, "Ġidle": 30650, "Ġdissolved": 30651, "Ġprevalent": 30652, "Ġmilitar": 30653, "Ġsensing": 30654, "Ġpojaw": 30655, "Ġancora": 30656, "Ġabundant": 30657, "Ġhairst": 30658, "ãģĤãĤĮ": 30659, "Ġtwee": 30660, "Ġnächste": 30661, "ĠMöglichkeit": 30662, "Ġhoo": 30663, "ufficient": 30664, "Ġfantast": 30665, "Ġedible": 30666, "Ġëĸ¨ìĸ´ì": 30667, "ìĽĥ": 30668, "Ġvein": 30669, "ucci": 30670, "Ġdevotion": 30671, "Ġconcealer": 30672, "income": 30673, "Ġrecycled": 30674, "ĠìĬ¤íĥĢ": 30675, "Ġpontos": 30676, "Ġdessus": 30677, "Ġvérit": 30678, "Ġreflections": 30679, "ĠAA": 30680, "Ġtakeaway": 30681, "bare": 30682, "ĠContact": 30683, "eil": 30684, "ĠHear": 30685, "Ġmirac": 30686, "ĠGerilim": 30687, "ĠÑģамÑĭй": 30688, "Ġvivo": 30689, "Ġkilograms": 30690, "ĠCrim": 30691, "ût": 30692, "78": 30693, "Ġsincerely": 30694, "raz": 30695, "Ġë³µ": 30696, "Ġarriv": 30697, "Ġconception": 30698, "ĠPersian": 30699, "Ġsjäl": 30700, "Ġstarring": 30701, "ĠìķĦ무": 30702, "ĠForever": 30703, "еÑģÑĤÑĮ": 30704, "Ġveil": 30705, "Ġsubtit": 30706, "odka": 30707, "ĠоÑĤноÑĪ": 30708, "Ġcooks": 30709, "енÑı": 30710, "Kay": 30711, "Ġniños": 30712, "ĠPhone": 30713, "Ġstitching": 30714, "Ġfingerprint": 30715, "é¢ĺ": 30716, "λά": 30717, "Ġdedicate": 30718, "ĠLob": 30719, "Ġblacks": 30720, "ĠBle": 30721, "bout": 30722, "ĠÄijang": 30723, "Ġeks": 30724, "Ġsquash": 30725, "ĠKü": 30726, "odi": 30727, "ĠnÆ°á»Ľc": 30728, "Ġvoyage": 30729, "Ġplayful": 30730, "ĠØ¥ÙĦÙī": 30731, "anic": 30732, "Ġcondemn": 30733, "ĠBöyle": 30734, "ĠPolize": 30735, "ãĤ¿ãĥ¼": 30736, "Ġayuda": 30737, "Ġpam": 30738, "à¹Ħà¸Ľ": 30739, "ĠKathy": 30740, "един": 30741, "нова": 30742, "Ġbrig": 30743, "eger": 30744, "Ġeagle": 30745, "Ġvisions": 30746, "ĠíķŃìĥģ": 30747, "Ġshitty": 30748, "Ġhott": 30749, "ĠBritt": 30750, "utors": 30751, "ENTE": 30752, "æĽ²": 30753, "Ġphon": 30754, "ĠBing": 30755, "ĠподдеÑĢж": 30756, "spring": 30757, "æĸ¯": 30758, "etten": 30759, "Ġpilgr": 30760, "Ġediyor": 30761, "енÑĤÑĭ": 30762, "aggio": 30763, "Ġjul": 30764, "Ġcomprend": 30765, "teil": 30766, "Ġز": 30767, "Ġperformers": 30768, "Ġinfamous": 30769, "ĠMK": 30770, "çª": 30771, "æ³ģ": 30772, "otle": 30773, "eff": 30774, "ĠHash": 30775, "Ġcoward": 30776, "ĠBRA": 30777, "ĠDD": 30778, "Ġcomida": 30779, "Ġplata": 30780, "Ġflap": 30781, "ĠMehr": 30782, "ribution": 30783, "ĠYemen": 30784, "Ġmysteries": 30785, "Ġİyi": 30786, "Ġstell": 30787, "Ġeyeliner": 30788, "Ġdeles": 30789, "Ġnailed": 30790, "Ġillnesses": 30791, "Ġstacks": 30792, "Ġtrabajar": 30793, "flower": 30794, "ciu": 30795, "Ġcrude": 30796, "Ġsubstantially": 30797, "Ġhomem": 30798, "Ġnephew": 30799, "Ġstamps": 30800, "Ġcarbs": 30801, "ÑĮÑĤе": 30802, "mooth": 30803, "Ġtunnels": 30804, "acie": 30805, "æ³¢": 30806, "ĠSeñ": 30807, "ĠHera": 30808, "ĠìķĦëĭĪìĹIJìļĶ": 30809, "ĠWyoming": 30810, "ĠHDMI": 30811, "ĠLis": 30812, "ución": 30813, "Ġsteer": 30814, "оÑİ": 30815, "иÑĤа": 30816, "NT": 30817, "Ġìĸ¼êµ´": 30818, "Ġpalms": 30819, "Ġneon": 30820, "ованиÑı": 30821, "Ġfiltering": 30822, "Ġjouer": 30823, "ĠHö": 30824, "ĠнеÑģ": 30825, "ê²łìĸ´ìļĶ": 30826, "Ġ81": 30827, "Ġstoryline": 30828, "Ġprzep": 30829, "Ġthanking": 30830, "ĠBoeing": 30831, "Ġsoftly": 30832, "jem": 30833, "алÑĮнÑĭÑħ": 30834, "Ġflashlight": 30835, "ĠпÑĥ": 30836, "ĠWOMAN": 30837, "ắc": 30838, "ÃŃch": 30839, "Ġluxurious": 30840, "Ġwün": 30841, "Ġimpactful": 30842, "Ġconson": 30843, "reu": 30844, "irring": 30845, "ifter": 30846, "Ġconstituents": 30847, "èIJ½": 30848, "Ġ94": 30849, "ĠTou": 30850, "gom": 30851, "ĠìĥĿê°ģìĿĦ": 30852, "Ġstereotypes": 30853, "Ġmożli": 30854, "åĪĨ享": 30855, "Ĥ¨": 30856, "Ġpencils": 30857, "ĠÑģлож": 30858, "Ġihrem": 30859, "ĠBesch": 30860, "ĠKoh": 30861, "ĠEntscheid": 30862, "Ġlek": 30863, "Ġförs": 30864, "Ġtotalmente": 30865, "Ġlively": 30866, "Ġentropy": 30867, "Ġdiscern": 30868, "ĠÐĹна": 30869, "Ġdov": 30870, "Ġmythology": 30871, "è¨ĺå¾Ĺ": 30872, "apanese": 30873, "Ġapproximate": 30874, "аÑĤив": 30875, "ifiable": 30876, "ĠSeo": 30877, "åĢĴ": 30878, "´ìĭ¬íŀĪ": 30879, "Ġìĺ·": 30880, "Ġtemporal": 30881, "ĠiT": 30882, "Ġestat": 30883, "ким": 30884, "Ġsprink": 30885, "Ġgrund": 30886, "Ġinfantry": 30887, "Ġschaffen": 30888, "ç´Ħ": 30889, "Ġank": 30890, "riages": 30891, "ĠYeon": 30892, "ĠMoroc": 30893, "Ġinvasive": 30894, "ģĶ": 30895, "Ġparenting": 30896, "ĠRis": 30897, "ibile": 30898, "Ġmods": 30899, "å½¢": 30900, "ĠпÑĢовеÑĢ": 30901, "ĠThing": 30902, "ĠWherever": 30903, "Ġacknowledging": 30904, "Ġpawn": 30905, "ummer": 30906, "orb": 30907, "69": 30908, "Ġretrouve": 30909, "Ġrelies": 30910, "ĠHighway": 30911, "Ġawe": 30912, "ãģ§ãģĻãģĭ": 30913, "itaire": 30914, "Ġapplicant": 30915, "Ġaisle": 30916, "worm": 30917, "Ġpayload": 30918, "Ġcarre": 30919, "ĠBach": 30920, "æł¼": 30921, "Ġì¹ľêµ¬ë": 30922, "ние": 30923, "ĠitÃŃs": 30924, "onnaise": 30925, "sol": 30926, "èı¯": 30927, "algia": 30928, "Ġrocking": 30929, "Ġbesten": 30930, "rites": 30931, "^^": 30932, "иной": 30933, "Ġbaixo": 30934, "Ġ기ìĸµ": 30935, "оÑĤÑĢи": 30936, "sim": 30937, "Ġincarn": 30938, "ëĭ¤ìĿĮ": 30939, "Ġlick": 30940, "sided": 30941, "Ġ71": 30942, "forder": 30943, "Ġresonance": 30944, "Ġtegen": 30945, "Ġmetaph": 30946, "owser": 30947, "Ġ×IJ׳×Ĺ׳×ķ": 30948, "?ãĢį": 30949, "Ġspielen": 30950, "Ġvolley": 30951, "ĶìĿ´íģ¬ìĹħ": 30952, "looked": 30953, "Ġsentenced": 30954, "Ġmultiplying": 30955, "Ġideals": 30956, "Ġwahrscheinlich": 30957, "Ġdeposits": 30958, "bilir": 30959, "Ġeffet": 30960, "illon": 30961, "Īë§Į": 30962, "Ġtestimon": 30963, "Ġzawsze": 30964, "ĠпÑĢоÑĨеÑģÑģ": 30965, "ĠLav": 30966, "ä¸įéĮ¯": 30967, "Ġtravailler": 30968, "Ġlaisse": 30969, "ĠMountains": 30970, "ĠÑĢоб": 30971, "Ġexamined": 30972, "itus": 30973, "Was": 30974, "лÑĭ": 30975, "Ġattributed": 30976, "ĠìĬ¹": 30977, "ĠBaron": 30978, "Ġgep": 30979, "Ġattent": 30980, "ĠCollection": 30981, "Ġtheat": 30982, "ĠCai": 30983, "Ġwells": 30984, "Ġhumano": 30985, "çĹħ": 30986, "ĠHast": 30987, "ĠÑħоÑĤÑı": 30988, "czas": 30989, "Ġpermits": 30990, "Ġlegg": 30991, "Ġepo": 30992, "ĠFen": 30993, "Ġthi": 30994, "ĠFoi": 30995, "Ġélect": 30996, "Ġ83": 30997, "Ġoverth": 30998, "Ġè¬Ŀè¬Ŀ": 30999, "Ġtenant": 31000, "è²·": 31001, "Next": 31002, "Ġpraised": 31003, "security": 31004, "ĠImpact": 31005, "为ä»Ģä¹Ī": 31006, "Ġvouch": 31007, "Ġnegó": 31008, "Ġunve": 31009, "Ġcriticize": 31010, "ĠKenya": 31011, "Ġtactic": 31012, "Ġlogr": 31013, "Ġpois": 31014, "Ġpapa": 31015, "speaks": 31016, "ðŁij": 31017, "ispers": 31018, "Ġsurplus": 31019, "Ġcolder": 31020, "åįĹ": 31021, "åIJ¬": 31022, "plets": 31023, "ĠVienna": 31024, "ĠLead": 31025, "Ġaerial": 31026, "ĠTah": 31027, "енÑĤов": 31028, "ĠGreeks": 31029, "Cam": 31030, "Ġmáxim": 31031, "Ġkuin": 31032, "chio": 31033, "Ġdemonstrates": 31034, "anos": 31035, "ĠCert": 31036, "ĠÑįн": 31037, "Ġblogs": 31038, "ĠìĦľìļ¸": 31039, "Ġbeams": 31040, "иков": 31041, "Ġprompted": 31042, "Ġfrightening": 31043, "ĠPorsche": 31044, "ãģĪãģ¦": 31045, "larını": 31046, "Ġchilling": 31047, "isphere": 31048, "Ġflashing": 31049, "ĠKard": 31050, "bread": 31051, "Ġexh": 31052, "Ġtycker": 31053, "Ġecological": 31054, "ĠMae": 31055, "Ġ×ŀ×IJ×ķ×ĵ": 31056, "ĠëĤĺëıĦ": 31057, "лон": 31058, "yss": 31059, "Ġpergunt": 31060, "Ġprix": 31061, "izzard": 31062, "Ġcancers": 31063, "Ġ91": 31064, "susp": 31065, "ĠItem": 31066, "ÅŁa": 31067, "Ġpest": 31068, "ĠtakÄħ": 31069, "Ġlymph": 31070, "ĠPatri": 31071, "fill": 31072, "Ġreconna": 31073, "Ġoptimism": 31074, "Ġmimic": 31075, "Ġì²ľ": 31076, "ĠMadame": 31077, "ocy": 31078, "lining": 31079, "åijĬ訴": 31080, "erme": 31081, "Ġfolders": 31082, "ĠczÅĤ": 31083, "uchar": 31084, "Ġcurso": 31085, "Ġbreach": 31086, "ниÑĤÑĮ": 31087, "ĠpamiÄĻ": 31088, "Ġelig": 31089, "Ġautop": 31090, "Flow": 31091, "Ġprogrammed": 31092, "ĠProcess": 31093, "Ġfigur": 31094, "ĠSF": 31095, "ĠEles": 31096, "Ġprogrammes": 31097, "Ġdizzy": 31098, "ìĭľê°Ħ": 31099, "Ġлибо": 31100, "Ġsniff": 31101, "ĠSebastian": 31102, "ĠHye": 31103, "Ġ4000": 31104, "Ġpermite": 31105, "æ¢Ŀ": 31106, "ĠзаÑī": 31107, "Ġguit": 31108, "ĠDais": 31109, "Ġaccordance": 31110, "Ġmodular": 31111, "ogeneous": 31112, "æĭį": 31113, "Ġpouquinho": 31114, "Ġartillery": 31115, "Ġlubric": 31116, "Ġvolcan": 31117, "ĠNH": 31118, "ð٤": 31119, "Ġdean": 31120, "Rh": 31121, "Ġministre": 31122, "åĿIJ": 31123, "ĠInv": 31124, "ĠBulgar": 31125, "ĠDaten": 31126, "èİ": 31127, "Im": 31128, "Ġoriginated": 31129, "ĠNixon": 31130, "integr": 31131, "Ġlacks": 31132, "ĠNacht": 31133, "ìĸ´ëĤĺ": 31134, "camera": 31135, "Ġradish": 31136, "kiye": 31137, "Ġanges": 31138, "Ġpréf": 31139, "juk": 31140, "ĠBee": 31141, "ĠBU": 31142, "ĠвоÑģп": 31143, "ĠBT": 31144, "êmes": 31145, "ĠStück": 31146, "ĠInk": 31147, "æĪĸèĢħ": 31148, "ĠSergeant": 31149, "ĠMultip": 31150, "Ġhiçbir": 31151, "ĠСам": 31152, "ĠDé": 31153, "olph": 31154, "ìĸ¸": 31155, "Ġimpat": 31156, "ĠìķĬê³ł": 31157, "ĠÑĤакого": 31158, "ĠнавеÑĢное": 31159, "Ġunpredictable": 31160, "Ġmend": 31161, "ĠìĹĨìĸ´ìļĶ": 31162, "ĠjakieÅĽ": 31163, "Ġanni": 31164, "Ġdonné": 31165, "ĠKirsty": 31166, "Ġrectangular": 31167, "Ġempezar": 31168, "ĠExchange": 31169, "ê°Ķ": 31170, "Ġéconom": 31171, "ãģĵãĤĵ": 31172, "elin": 31173, "reibt": 31174, "Ġ×Ķפ": 31175, "Ġcemetery": 31176, "Ġespañol": 31177, "olin": 31178, "лÑİд": 31179, "Ġgrâce": 31180, "allen": 31181, "ĠPhilos": 31182, "ĠErst": 31183, "ĠìĥĪ": 31184, "ĠVid": 31185, "Give": 31186, "OH": 31187, "μο": 31188, "ĠPare": 31189, "Ġmetabolism": 31190, "Ġmaple": 31191, "Ġaxle": 31192, "ĠDy": 31193, "Ġkomme": 31194, "Ïİν": 31195, "Ġgreatness": 31196, "Ġverified": 31197, "Ġspé": 31198, "ĠFahrenheit": 31199, "ĠBren": 31200, "ĠConfeder": 31201, "Ġhistoire": 31202, "Ġeliminating": 31203, "ĠAdding": 31204, "ĠAbi": 31205, "æĿİ": 31206, "Ġhospitality": 31207, "tim": 31208, "Ġbonito": 31209, "Ġpartes": 31210, "ĠдÑĢÑĥгиÑħ": 31211, "ĠShay": 31212, "ĠSed": 31213, "Ġregrets": 31214, "Ñıми": 31215, "Ġtenants": 31216, "éĢŁ": 31217, "ĠPTS": 31218, "Ġdevi": 31219, "ĠLate": 31220, "uez": 31221, "Ġsöyl": 31222, "ãĤ»": 31223, "Ġìŀ¬ë°Į": 31224, "Ġtoggle": 31225, "Ġmasking": 31226, "алÑĮного": 31227, "Ġpersön": 31228, "Ġamerican": 31229, "fik": 31230, "ĠRGB": 31231, "enson": 31232, "ĠKA": 31233, "wwww": 31234, "ĠÑĢег": 31235, "metics": 31236, "Ġeducator": 31237, "ãĤ·ãĥ«ãĤ¯": 31238, "park": 31239, "елÑĮзÑı": 31240, "arus": 31241, "ÑĢеÑĤ": 31242, "Ġfeito": 31243, "Ġchoir": 31244, "Ġlargo": 31245, "Ġeens": 31246, "Ġwatts": 31247, "ĠSingle": 31248, "Ġsusceptible": 31249, "icer": 31250, "ĠвклÑİÑĩ": 31251, "Ġpus": 31252, "íĻĺ": 31253, "Eng": 31254, "Ġfantas": 31255, "Ġspecification": 31256, "Ġconfronted": 31257, "ĠColumbus": 31258, "ивеÑĤ": 31259, "arım": 31260, "Ġcaffeine": 31261, "munition": 31262, "Ġmigrants": 31263, "lide": 31264, "itations": 31265, "ĠGeme": 31266, "ẫ": 31267, "Ġplanner": 31268, "Ġstimulate": 31269, "Ġaproxim": 31270, "ceu": 31271, "ĠNom": 31272, "Ġvog": 31273, "ĠÑĢаÑģÑĤ": 31274, "Ġenseñ": 31275, "Ġsellers": 31276, "Ġguten": 31277, "zd": 31278, "Cal": 31279, "Ġdescript": 31280, "Ġreconciliation": 31281, "zinho": 31282, "á¹ĩa": 31283, "ãģĺãĤĥãģĤ": 31284, "acyj": 31285, "ĠCOL": 31286, "saw": 31287, "ĠíĻķìĿ¸": 31288, "Ġvarit": 31289, "Ġpartnering": 31290, "Ġdetention": 31291, "Ġbombing": 31292, "clapping": 31293, "iencies": 31294, "ondu": 31295, "AME": 31296, "Ġê°ĻìĬµëĭĪëĭ¤": 31297, "cÃŃa": 31298, "ĠпоÑģÑĤо": 31299, "ĠASMR": 31300, "Ġhomepage": 31301, "Ġsiè": 31302, "antha": 31303, "ĠPoll": 31304, "Ġigen": 31305, "cych": 31306, "Ġê°ijìŀIJ기": 31307, "Ġconsiderably": 31308, "ä»ĸçļĦ": 31309, "ĠArist": 31310, "Ġwithstand": 31311, "Ġqualitative": 31312, "ĠKraft": 31313, "ĠÑįлекÑĤ": 31314, "ĠBead": 31315, "екÑĤив": 31316, "Ġcrushing": 31317, "ì³IJ": 31318, "Ġnavy": 31319, "ÙĪÚº": 31320, "sho": 31321, "Ġoak": 31322, "ippers": 31323, "Ġsoils": 31324, "Ġpigment": 31325, "Ġevitar": 31326, "ãĥĩ": 31327, "Ġfuse": 31328, "ĠDale": 31329, ":\"": 31330, "Ġcomplètement": 31331, "Ġkel": 31332, "à¹Ĩ": 31333, "Ġquatre": 31334, "ĠUM": 31335, "Ġë§IJë": 31336, "æł¹": 31337, "ÃŃr": 31338, "Ġleisure": 31339, "ĠHousing": 31340, "Ġfolds": 31341, "estion": 31342, "ARS": 31343, "Ġmash": 31344, "urpose": 31345, "Ġaccumulated": 31346, "ĠStuff": 31347, "èªŀ": 31348, "Ġtapes": 31349, "ĠÑģилÑĮно": 31350, "ĠLOVE": 31351, "Ġ1982": 31352, "Ġscars": 31353, "Ġcapitalist": 31354, "ĠNed": 31355, "Ġsoften": 31356, "Ġnotably": 31357, "Ġforcément": 31358, "ĠRaum": 31359, "ĠнеобÑħод": 31360, "Ġtrademark": 31361, "Ġfertig": 31362, "Ġ?!": 31363, "æĹł": 31364, "Ġreinforced": 31365, "Ġrecharge": 31366, "ĠPutting": 31367, "Ġvillains": 31368, "Ġhandic": 31369, "Ġadvertisement": 31370, "تÙĬ": 31371, "ĠÑģÑĥм": 31372, "ĠRiley": 31373, "×ķ×ij×": 31374, "京": 31375, "Os": 31376, "از": 31377, "Boy": 31378, "Ġsquish": 31379, "ocket": 31380, "Ġtestify": 31381, "æ¼Ķ": 31382, "Ġ׾×ŀ×": 31383, "ĠмаÑģÑģ": 31384, "manuel": 31385, "ĠArkansas": 31386, "iffe": 31387, "Ġanalysts": 31388, "ĠDeaf": 31389, "Ġjó": 31390, "Ġgroceries": 31391, "ĠWheel": 31392, "ĠÑĢиÑģ": 31393, "Ġcòn": 31394, "ĠCob": 31395, "Ġprisons": 31396, "ève": 31397, "ĠCabinet": 31398, "Ġposed": 31399, "Ġguerre": 31400, "ĠLloyd": 31401, "Ġclerk": 31402, "Ġcrises": 31403, "ĠSho": 31404, "ĠOre": 31405, "ĠFootball": 31406, "ĠAdvis": 31407, "ĠZheng": 31408, "èį": 31409, "ĠAMY": 31410, "Ġunfor": 31411, "Ġmonaster": 31412, "Ġcompile": 31413, "Ġimmortal": 31414, "atable": 31415, "Ġparano": 31416, "Ġtiver": 31417, "ĠSteph": 31418, "ĠFuÃŁ": 31419, "Ġdiscontin": 31420, "Ġripe": 31421, "Ġhacking": 31422, "Ġsiendo": 31423, "Ġseguro": 31424, "altres": 31425, "Ġanderes": 31426, "Ġ리ë": 31427, "Ġexports": 31428, "æŃ¥": 31429, "Ġtabii": 31430, "Ġ기ëĭ¤ë": 31431, "Ġbothering": 31432, "Ġpickle": 31433, "ĠBRIAN": 31434, "Ġaltar": 31435, "ĠпÑĢиб": 31436, "Ġtransferring": 31437, "ĠVors": 31438, "ĠÙĩÙĪ": 31439, "ĠZa": 31440, "ĠFrances": 31441, "Ġbrowse": 31442, "emit": 31443, "Ġchewing": 31444, "ĠFreddy": 31445, "Ġeditors": 31446, "älle": 31447, "ĠíĮĢ": 31448, "ĠSque": 31449, "ĠCultural": 31450, "awk": 31451, "ĠSache": 31452, "ĠCarbon": 31453, "ắt": 31454, "FL": 31455, "ĠNGO": 31456, "peÅĤ": 31457, "ĠSou": 31458, "Ġhvor": 31459, "unintelligible": 31460, "Ġë²ķ": 31461, "Ġ°": 31462, "iin": 31463, "Ġ×¢×Ŀ": 31464, "Ġderrière": 31465, "Ġczym": 31466, "ĠApost": 31467, "Ġregarder": 31468, "Ġagrade": 31469, "ĠCandy": 31470, "Ġmare": 31471, "Ġintroduces": 31472, "birds": 31473, "Ġuniquely": 31474, "Ġmuk": 31475, "Ġcooker": 31476, "Ġcrews": 31477, "Ġjeito": 31478, "ERT": 31479, "¶Ħë": 31480, "nisse": 31481, "Ġef": 31482, "Ġcarte": 31483, "ĠYak": 31484, "ĠPAT": 31485, "ино": 31486, "bokki": 31487, "Ġmates": 31488, "Ġdistint": 31489, "Ġì½Ķë¡ľëĤĺ": 31490, "Ġyıl": 31491, "Ġκάν": 31492, "Ġconfigurations": 31493, "enga": 31494, "recht": 31495, "Happy": 31496, "ãĤĦãģ£ãģ¦": 31497, "invest": 31498, "Ġreconstruct": 31499, "ĠÑįÑĤомÑĥ": 31500, "Ġmosque": 31501, "raum": 31502, "Ġvoyez": 31503, "ĠNBC": 31504, "ĠìŀIJìĭł": 31505, "Ġsturdy": 31506, "Ġкап": 31507, "Ġansch": 31508, "alid": 31509, "Ġmasih": 31510, "ĠREP": 31511, "Ġì½Ķë": 31512, "Ġdeduct": 31513, "Ġsalir": 31514, "wurf": 31515, "ilot": 31516, "ĠMutter": 31517, "olds": 31518, "ĠFEMA": 31519, "ĠBib": 31520, "Ġneighboring": 31521, "Ġbliss": 31522, "Ġíĺ¼": 31523, "лиÑģÑĮ": 31524, "ĠÑĤÑĢеб": 31525, "Ġå°±æĺ¯": 31526, "Ġgrenade": 31527, "Ġegal": 31528, "Ġfinely": 31529, "Ġpetals": 31530, "Ġkeer": 31531, "Ġchyba": 31532, "Ġskipping": 31533, "Ġthirteen": 31534, "Ġgravy": 31535, "ĠSAT": 31536, "61": 31537, "Ġног": 31538, "Ġmins": 31539, "ITE": 31540, "Ġsozial": 31541, "íķĺë©´ìĦľ": 31542, "ruktur": 31543, "Ġвозмож": 31544, "ĠопÑıÑĤÑĮ": 31545, "Ġarth": 31546, "ĠCuban": 31547, "Ġtreasures": 31548, "Ġfertilizer": 31549, "Ġawakening": 31550, "Ġë°±ìĭł": 31551, "Ġrall": 31552, "Ġdepict": 31553, "ĠPablo": 31554, "Ġnineteen": 31555, "Ġwatt": 31556, "Ġentirety": 31557, "KS": 31558, "ĠWoods": 31559, "Sch": 31560, "ĠÚ©ÙĪ": 31561, "ĠDry": 31562, "ãģŀ": 31563, "uve": 31564, "Ġreconstruction": 31565, "Ġanatomy": 31566, "Ī를": 31567, "Ġbaba": 31568, "Ġlistener": 31569, "Ġsharpen": 31570, "ĠPeru": 31571, "ĠвÑĭз": 31572, "Ġrecreation": 31573, "Ġinitiate": 31574, "Ġcalor": 31575, "ĠNaj": 31576, "gee": 31577, "ĠFeels": 31578, "ĠSnapchat": 31579, "ĠTet": 31580, "ĠNest": 31581, "ĠDaf": 31582, "ĠFinish": 31583, "ĠÑĤаким": 31584, "úc": 31585, "izens": 31586, "Ġspins": 31587, "Ġembry": 31588, "Ġpassages": 31589, "Ġcient": 31590, "Ġjustification": 31591, "ä»ĸ說": 31592, "Ġolmaz": 31593, "Ġflooded": 31594, "Ġemoji": 31595, "Ġembracing": 31596, "Ġdiscard": 31597, "ĠBasic": 31598, "agog": 31599, "ĠìľĦíķ´": 31600, "Ġasylum": 31601, "erin": 31602, "Ġfim": 31603, "Ġninja": 31604, "Ġautomate": 31605, "Ġallergic": 31606, "ÿÿÿÿ": 31607, "amam": 31608, "ĠмаÑĢ": 31609, "ĠOi": 31610, "äus": 31611, "Ġinduct": 31612, "ĠBEN": 31613, "ĠzÅĤ": 31614, "Ġkażdy": 31615, "ĠAMP": 31616, "nÄĽ": 31617, "Sure": 31618, "Ġquil": 31619, "Ġespec": 31620, "rok": 31621, "BSCRI": 31622, "Ġliebe": 31623, "pus": 31624, "achsen": 31625, "Ġcricket": 31626, "ëĬIJ": 31627, "ĠFrame": 31628, "ekkür": 31629, "arb": 31630, "ĠpÅĻ": 31631, "иÑģÑģ": 31632, "Ġzeggen": 31633, "Ġdoubles": 31634, "ĠDre": 31635, "test": 31636, "insp": 31637, "boys": 31638, "Ġmão": 31639, "ĠVerse": 31640, "Ġmuscular": 31641, "ĠMALE": 31642, "Ġdulu": 31643, "Ġoccasional": 31644, "Lo": 31645, "conomic": 31646, "Ġvak": 31647, "Ġremedy": 31648, "å¤ł": 31649, "ĠâĻªâĻªâĻª": 31650, "vem": 31651, "Ġönem": 31652, "ĠkarÅŁÄ±": 31653, "ĠSharp": 31654, "hur": 31655, "Ġë°©ë²ķ": 31656, "Ġgrandson": 31657, "Ġaktiv": 31658, "ĠThrones": 31659, "ĠìķĪìĹIJ": 31660, "Ġtots": 31661, "Ġsubd": 31662, "ĠPaula": 31663, "Ġgraves": 31664, "ĠBrent": 31665, "ĠникÑĤо": 31666, "Ġsöz": 31667, "Ġcrec": 31668, "ĠVladimir": 31669, "çĸ«": 31670, "Ġпой": 31671, "Ġ\"-": 31672, "Ġpsy": 31673, "atri": 31674, "idan": 31675, "Ġaún": 31676, "Ġstandardized": 31677, "ì¹ĺë": 31678, "ĠкÑĢов": 31679, "ĠZhu": 31680, "something": 31681, "Ġ750": 31682, "Ġmujeres": 31683, "Ġait": 31684, "éĹ´": 31685, "agu": 31686, "Ġcorrected": 31687, "ikka": 31688, "eled": 31689, "ĠCareer": 31690, "owym": 31691, "Ġroommate": 31692, "Ġdescendants": 31693, "ĠNapoleon": 31694, "ĠÐĶо": 31695, "íĸĪìĸ´ìļĶ": 31696, "Ġbunun": 31697, "ĠMicha": 31698, "ç·ļ": 31699, "Ġdescob": 31700, "PI": 31701, "Ġpalabra": 31702, "Ġtracked": 31703, "Ġdependence": 31704, "ĠBarack": 31705, "åģĩ": 31706, "Ġfertility": 31707, "ĠSouthwest": 31708, "Ġincomplete": 31709, "Ġcomunic": 31710, "Ġcompris": 31711, "ĠRestaur": 31712, "Ġacron": 31713, "κα": 31714, "Ġapprentices": 31715, "Ġmusst": 31716, "ĠAbr": 31717, "Ġpentru": 31718, "ĠConsort": 31719, "ĠAvec": 31720, "Ġdumplings": 31721, "LR": 31722, "Ġwszystkie": 31723, "Ġswamp": 31724, "нев": 31725, "uggle": 31726, "Ġwatercolor": 31727, "Ġproton": 31728, "ĠEspaña": 31729, "ocking": 31730, "овал": 31731, "Ġtakim": 31732, "Very": 31733, "Ġdementia": 31734, "ĠÅŁeyi": 31735, "Jac": 31736, "ĠMacBook": 31737, "ĠLiv": 31738, "fficients": 31739, "ĠHunt": 31740, "Ġoverlay": 31741, "æĦŁè¦º": 31742, "ĠSkype": 31743, "punkt": 31744, "Ġconfined": 31745, "ĠAdrian": 31746, "رÙĥ": 31747, "ĠJeep": 31748, "Ġenquanto": 31749, "Ġanest": 31750, "оÑĤвеÑĤ": 31751, "ĠменÑĮ": 31752, "Ġirrigation": 31753, "á»ijn": 31754, "Ġeighteen": 31755, "ĠPon": 31756, "Ġrescued": 31757, "Ġ1983": 31758, "rü": 31759, "jae": 31760, "ĠJeong": 31761, "Ġamazingly": 31762, "ĠFDP": 31763, "Ġbackstage": 31764, "cue": 31765, "ĠÏĥÏĦην": 31766, "ĠاÙĦص": 31767, "Ġlivestock": 31768, "ĠWarner": 31769, "Ġmajors": 31770, "ãĥģãĥ£": 31771, "Ġcooperative": 31772, "ĠBrady": 31773, "rained": 31774, "rieb": 31775, "Ġ×ij×ŀ×": 31776, "ĠдоволÑĮно": 31777, "ĠFE": 31778, "Ġleaked": 31779, "ĠMercury": 31780, "Ġpersuade": 31781, "Ġtransformer": 31782, "ĠNorweg": 31783, "ĠìŬ룬": 31784, "ĠzrobiÄĩ": 31785, "Ġcardiovascular": 31786, "ĠCrash": 31787, "Ġgossip": 31788, "аÑģÑĤÑĮ": 31789, "Ġ쪽": 31790, "Ġswept": 31791, "ĠHorn": 31792, "ĠAté": 31793, "Ġbukan": 31794, "ĠKaw": 31795, "KY": 31796, "ĠStories": 31797, "Gary": 31798, "Ġgardening": 31799, "ĠQuickly": 31800, "ĠFalcon": 31801, "Ġovat": 31802, "cı": 31803, "ĠComplet": 31804, "ĠDate": 31805, "ĠпÑĢим": 31806, "Ġläuft": 31807, "ĠAudrey": 31808, "ĠWent": 31809, "ĠpelÃŃcul": 31810, "Ġcarriage": 31811, "Ġunacceptable": 31812, "nymi": 31813, "ĠÑģлÑĭÑĪ": 31814, "Ġterre": 31815, "uellement": 31816, "EEEE": 31817, "Ġpharmac": 31818, "hões": 31819, "Ġzich": 31820, "Ġmigrate": 31821, "ĠFry": 31822, "ñana": 31823, "ĠMuito": 31824, "EOVER": 31825, "Ġfortress": 31826, "ĠCompan": 31827, "ĠJSON": 31828, "ordnung": 31829, "Ġwarto": 31830, "Ġungef": 31831, "ìħĶìĦľ": 31832, "ĠÑĢок": 31833, "Ġpaddle": 31834, "Jared": 31835, "Ġsubmitting": 31836, "Ġlatch": 31837, "Ġfug": 31838, "ĠкоÑģ": 31839, "ĠEf": 31840, "Ġlaunches": 31841, "Ġft": 31842, "otechn": 31843, "Ġtravelled": 31844, "اÙģ": 31845, "éģķ": 31846, "Ġproch": 31847, "Ġdedim": 31848, "83": 31849, "Ġrebound": 31850, "ĠLU": 31851, "path": 31852, "ĠÑģпÑĢав": 31853, "Ġöl": 31854, "ĠíĤ¤": 31855, "Ġprivat": 31856, "Ġtractor": 31857, "ĠAttention": 31858, "Ser": 31859, "Ġcoses": 31860, "ária": 31861, "pal": 31862, "ĠìĿĢ": 31863, "Ġsuccessor": 31864, "Ġconnectors": 31865, "ĠÑĥÑģÑĤанов": 31866, "Ġgenocide": 31867, "Ġsufficiently": 31868, "ĠAixò": 31869, "Ġstabilize": 31870, "Ġcongest": 31871, "Ġcarving": 31872, "Ġzost": 31873, "ĠбÑĭÑģÑĤÑĢо": 31874, "Ġshortest": 31875, "Ġlivel": 31876, "Ġ89": 31877, "éģĬ": 31878, "Ġerk": 31879, "Ġportraits": 31880, "à¥Ģ": 31881, "èĺ": 31882, "boat": 31883, "llah": 31884, "ANC": 31885, "Ġempirical": 31886, "ĠEcho": 31887, "ĠNederland": 31888, "è¿Ļä¹Ī": 31889, "Net": 31890, "Ġcuidado": 31891, "ĠRoma": 31892, "Ġcalf": 31893, "Ġgiants": 31894, "ĠExplorer": 31895, "ĠCollect": 31896, "alition": 31897, "ĠDestiny": 31898, "Ġausge": 31899, "ĠEdu": 31900, "ĠClo": 31901, "Ġearrings": 31902, "ĠTrack": 31903, "ĠROS": 31904, "ĠBelle": 31905, "çϾ": 31906, "Ġpueda": 31907, "Ġdaytime": 31908, "Ġsupplier": 31909, "ĠSV": 31910, "ĠExhale": 31911, "Ġgalera": 31912, "course": 31913, "Ġcentimeter": 31914, "ĠBast": 31915, "mud": 31916, "Ġsangat": 31917, "ĠPhysical": 31918, "Ġprivately": 31919, "Ġtrata": 31920, "lynn": 31921, "illi": 31922, "Ġë©ĶìĿ´íģ¬ìĹħ": 31923, "Ġcrystall": 31924, "Ġpods": 31925, "ản": 31926, "inator": 31927, "ĠRecords": 31928, "å®ĺ": 31929, "ÄŁimiz": 31930, "issement": 31931, "hare": 31932, "hadow": 31933, "ĠDK": 31934, "ĠìķĮê³ł": 31935, "Ġwyn": 31936, "Ġrequesting": 31937, "ĠDonna": 31938, "ĠìĹ´ìĭ¬íŀĪ": 31939, "inea": 31940, "Ġexert": 31941, "ĠDuncan": 31942, "ĠвеÑĩ": 31943, "ĠHah": 31944, "à¤Ĥ": 31945, "ĠLif": 31946, "ĠFinding": 31947, "ĠNov": 31948, "Ġзнак": 31949, "ĠоÑĦ": 31950, "ĠQuè": 31951, "Ġquarterback": 31952, "ĠÑĦак": 31953, "Ġbipartisan": 31954, "ÄŁin": 31955, "Ġnécess": 31956, "Ġreferendum": 31957, "Ġcompiler": 31958, "Ġprobabil": 31959, "еди": 31960, "Ġtrader": 31961, "æĺĵ": 31962, "ĠRum": 31963, "geme": 31964, "Ġdio": 31965, "ĠbÄĻdziemy": 31966, "ĠÏĢά": 31967, "꾸": 31968, "×ķ×ĺ": 31969, "Ġà¤ķ": 31970, "Ġблаг": 31971, "Ġscalp": 31972, "ĠPause": 31973, "Ġcaption": 31974, "Ġendanger": 31975, "Ġenlar": 31976, "Ġrotten": 31977, "ãĥĥãĥĪ": 31978, "Ġwah": 31979, "èĤī": 31980, "Ġdzi": 31981, "ĠInstall": 31982, "Ay": 31983, "Ġcrear": 31984, "енÑĤа": 31985, "Ġweighing": 31986, "Ġbutterflies": 31987, "ĠGast": 31988, "äºķ": 31989, "horn": 31990, "warz": 31991, "ICEOVER": 31992, "ĠнайÑĤи": 31993, "Ġcoefficients": 31994, "ç°¡åĸ®": 31995, "ĠSpencer": 31996, "ĠHigher": 31997, "Ġcowork": 31998, "å¨ĺ": 31999, "ĠкоÑĤоÑĢое": 32000, "Ġmonit": 32001, "Ġdysfunction": 32002, "ĠÑģÑĤанов": 32003, "Ġtournaments": 32004, "Ġoyster": 32005, "BN": 32006, "Ġtrud": 32007, "slow": 32008, "ĠPenny": 32009, "ĠOdys": 32010, "ær": 32011, "Ġfou": 32012, "Ġenjoyment": 32013, "аÑĤÑĭ": 32014, "ĠwyglÄħda": 32015, "алÑĮнаÑı": 32016, "ĠProtect": 32017, "Ġmoy": 32018, "Ġclaw": 32019, "Ġsuspicion": 32020, "Ġsacrificed": 32021, "Ġgosto": 32022, "Big": 32023, "Ġaggressively": 32024, "Ġvorne": 32025, "ãĥł": 32026, "Ġblamed": 32027, "ĠSehr": 32028, "פר": 32029, "cito": 32030, "Ġseals": 32031, "Ġmujer": 32032, "ĠWeird": 32033, "Ġforens": 32034, "Ġcontributes": 32035, "estra": 32036, "Ġpog": 32037, "LOL": 32038, "Ġhacerlo": 32039, "оÑĤÑĮ": 32040, "fiction": 32041, "79": 32042, "λο": 32043, "大æ¦Ĥ": 32044, "声": 32045, "ĠÑĤоб": 32046, "ĠGS": 32047, "ĠClara": 32048, "itez": 32049, "Ġadvocating": 32050, "ĠíĶĦë": 32051, "sung": 32052, "Ġvertices": 32053, "Ġnavigating": 32054, "Ġeuropé": 32055, "çļĨ": 32056, "Ġslowed": 32057, "Ġforeground": 32058, "ĠIndustrial": 32059, "Ġadore": 32060, "ìĭŃ": 32061, "Ġcréer": 32062, "æŀĹ": 32063, "chnitt": 32064, "Ġunaware": 32065, "Ġcurly": 32066, "entar": 32067, "Ġler": 32068, "Ġprohibited": 32069, "ĠHeroes": 32070, "ĠReed": 32071, "uca": 32072, "Ġsmok": 32073, "Ġkunna": 32074, "zeitig": 32075, "immen": 32076, "ĠLun": 32077, "ĠабÑģолÑİÑĤ": 32078, "Ġdegli": 32079, "Ġvillagers": 32080, "Ġpreset": 32081, "zept": 32082, "uds": 32083, "Ġemit": 32084, "ä½łè¦ģ": 32085, "Ġëī": 32086, "ëĬĶì§Ģ": 32087, "нако": 32088, "Ġosób": 32089, "Ġ1969": 32090, "ĠÐIJÑĢ": 32091, "Ġmanchmal": 32092, "ĠBrock": 32093, "Ġmantra": 32094, "ĠWIL": 32095, "bach": 32096, "inä": 32097, "elas": 32098, "keln": 32099, "Ġdisciple": 32100, "Ġqualc": 32101, "Ġdehyd": 32102, "ìĿ´ëĿ¼ëĬĶ": 32103, "Af": 32104, "ìĦ±ìĿ´": 32105, "Ryan": 32106, "Ġpuppet": 32107, "ĠдÑĢÑĥгие": 32108, "Ġrud": 32109, "Ġpending": 32110, "Plus": 32111, "ĠìķĬìĿĦ": 32112, "Ġbá»ĭ": 32113, "ĠSega": 32114, "çe": 32115, "Ġprogrammer": 32116, "bli": 32117, "Ġunl": 32118, "Ġenslaved": 32119, "Ġsociété": 32120, "Äģh": 32121, "Ġinheritance": 32122, "ĠBangl": 32123, "ermaid": 32124, "Ġpractitioner": 32125, "ĠStalin": 32126, "ĠUser": 32127, "cible": 32128, "Ġcardiac": 32129, "ĠKoreans": 32130, "Ġdumped": 32131, "Ġ×Ķ×Ļ×Ķ": 32132, "áis": 32133, "Ġhydraulic": 32134, "oubtedly": 32135, "ĠPit": 32136, "Ġpicnic": 32137, "Ġbehöver": 32138, "ĠÑģмог": 32139, "Ġbraking": 32140, "é»ij": 32141, "utar": 32142, "ĠìĦ¸ë": 32143, "ubl": 32144, "Ġüz": 32145, "Ġmajesty": 32146, "Ġbers": 32147, "utable": 32148, "Ġhotter": 32149, "çħ§": 32150, "ÛĮÙĨ": 32151, "Ġbiases": 32152, "Ġsubjected": 32153, "Ġnaughty": 32154, "Ġcircus": 32155, "ãģĹãģĭ": 32156, "ĠImmedi": 32157, "ĠStefan": 32158, "ĠTriple": 32159, "enk": 32160, "Ġwit": 32161, "Ġrecycle": 32162, "emie": 32163, "dated": 32164, "Ġunload": 32165, "Ġpopula": 32166, "chin": 32167, "Ġyields": 32168, "Ġenglish": 32169, "ĠBonnie": 32170, "Ġspiders": 32171, "Ãģ": 32172, "Ġerosion": 32173, "éĥ¨åĪĨ": 32174, "ĠNICK": 32175, "иÑıÑħ": 32176, "Ġimpart": 32177, "Ġкни": 32178, "Ġresolutions": 32179, "Ġlithium": 32180, "Ġconvergence": 32181, "ĠTara": 32182, "Ġдве": 32183, "ths": 32184, "ĠCindy": 32185, "æĪijè¦ģ": 32186, "幫": 32187, "ĠDIE": 32188, "Ġassurance": 32189, "ĠопиÑģ": 32190, "Ġbuckets": 32191, "Ġcues": 32192, "ĠQuiet": 32193, "Ġsimilarity": 32194, "Ġfoundational": 32195, "ĠMinist": 32196, "滿": 32197, "Ġpian": 32198, "Ġcentr": 32199, "Ġnumb": 32200, "Ġmonks": 32201, "ujourd": 32202, "enzie": 32203, "Ġskateboard": 32204, "Ġdlatego": 32205, "ĠÑģоÑĤ": 32206, "ĠAE": 32207, "Ġmasterpiece": 32208, "ĠSolomon": 32209, "ĠReddit": 32210, "Ġriot": 32211, "abl": 32212, "ĠJazz": 32213, "Ġelectromagnetic": 32214, "Ġinsecure": 32215, "ĠCompet": 32216, "geries": 32217, "обод": 32218, "ł×ķ": 32219, "ðŁĴ": 32220, "Ġsenators": 32221, "ĠBrisbane": 32222, "ĠAlb": 32223, "uttering": 32224, "ĠAllow": 32225, "zero": 32226, "Ġpai": 32227, "ĠÐIJлекÑģ": 32228, "ĠDisplay": 32229, "ĠBlade": 32230, "ĠApps": 32231, "Ġpä": 32232, "ĠдеÑģÑı": 32233, "Ġquella": 32234, "ĠGao": 32235, "еннÑĭÑħ": 32236, "Ġspoilers": 32237, "Ġgallons": 32238, "ĠÙĦÙĬ": 32239, "ĠZion": 32240, "æľīä¸Ģ": 32241, "onie": 32242, "ragt": 32243, "ĠChand": 32244, "Ġë³ij": 32245, "Ġblunt": 32246, "Ġusu": 32247, "ĠKad": 32248, "rakt": 32249, "Ġcinematic": 32250, "Ġammunition": 32251, "rene": 32252, "Ġfourteen": 32253, "ĠCarn": 32254, "crit": 32255, "Ġtenure": 32256, "vu": 32257, "Ġprincipalmente": 32258, "Ġalleen": 32259, "éĢĻä¸Ģ": 32260, "Ġkomplett": 32261, "Ġdüny": 32262, "James": 32263, "Ġreceptor": 32264, "Ġoneself": 32265, "guru": 32266, "Ġmerchant": 32267, "liness": 32268, "Ġoverlooked": 32269, "Ġharmonic": 32270, "éķ¿": 32271, "ieso": 32272, "×ķ×ŀ": 32273, "colm": 32274, "ĠпÑĢоекÑĤ": 32275, "ĠAda": 32276, "اس": 32277, "Tim": 32278, "Ġrecurring": 32279, "Ġproceeds": 32280, "ĠParticularly": 32281, "ĠDownload": 32282, "etrical": 32283, "Ġmatrices": 32284, "Ġproyecto": 32285, "ancies": 32286, "ĠUhm": 32287, "Ġcaves": 32288, "Ġìĸ´ëł¤": 32289, "ĠLeaf": 32290, "ĠобÑĭÑĩ": 32291, "ĠìĿ´ìľł": 32292, "Europe": 32293, "ĠtÄħ": 32294, "Ġpuls": 32295, "Ġtakiego": 32296, "ÐĿе": 32297, "GU": 32298, "Ġfors": 32299, "Ïģγ": 32300, "Ġfotos": 32301, "Ġ))": 32302, "Ġ멤ë": 32303, "Ġaquilo": 32304, "ĠKurd": 32305, "ï¸ı": 32306, "ptic": 32307, "ĠDort": 32308, "Ġmisery": 32309, "auso": 32310, "åĬŁ": 32311, "chuckling": 32312, "ĠRidge": 32313, "ĠíĸĪìĬµëĭĪëĭ¤": 32314, "Ġ***": 32315, "客": 32316, "ĠHmmm": 32317, "Ġgeographic": 32318, "Ġanys": 32319, "Ġtalvez": 32320, "Ġskelet": 32321, "Ġsignatures": 32322, "Ġliters": 32323, "IJë©´": 32324, "ĠÑģвоего": 32325, "Ġskiing": 32326, "ĠÐľÐ¾Ñģ": 32327, "Ġadopting": 32328, "Ġhaft": 32329, "Ġsymmetric": 32330, "ĠLiqu": 32331, "Ġthyroid": 32332, "Ġmisin": 32333, "lude": 32334, "Ġhull": 32335, "ĠXD": 32336, "ĠGust": 32337, "zeich": 32338, "Ġvibrations": 32339, "Ġesemp": 32340, "ĠвÑģÑİ": 32341, "ĠQuem": 32342, "Ġübrig": 32343, "ĠSke": 32344, "ĠLynch": 32345, "rooms": 32346, "artet": 32347, "fest": 32348, "Ġfrüher": 32349, "Ġlure": 32350, "ä¸į好æĦıæĢĿ": 32351, "ĠìķĮìķĦ": 32352, "ĠWIN": 32353, "ĠRYAN": 32354, "ĠкоÑĤоÑĢÑĥÑİ": 32355, "ĠKash": 32356, "Ġ×Ķ×ŀ": 32357, "Ġsafeg": 32358, "ĠHallelujah": 32359, "ĠдвÑĥÑħ": 32360, "Ġstaple": 32361, "Ġsediment": 32362, "ĠActs": 32363, "Ġblaming": 32364, "Ġmainland": 32365, "Ġsporting": 32366, "Ġdecorations": 32367, "Ġexecuting": 32368, "Ġparan": 32369, "ĠDollar": 32370, "Ġprojections": 32371, "Ġcommissioned": 32372, "Ġbour": 32373, "öm": 32374, "Ġsteamed": 32375, "ĠëŃĺ": 32376, "Ġpetrol": 32377, "Ġcelular": 32378, "帶": 32379, "ĠHungary": 32380, "Ġrented": 32381, "ĠваÑĢи": 32382, "bbie": 32383, "Ġsécur": 32384, "üll": 32385, "Ġswings": 32386, "between": 32387, "ĠиÑĤ": 32388, "estro": 32389, "Ġniemand": 32390, "ĠìĤ¼": 32391, "ĠPardon": 32392, "esses": 32393, "ĠMID": 32394, "Ġcentralized": 32395, "ĠAlien": 32396, "culos": 32397, "Ġcrise": 32398, "裡éĿ¢": 32399, "Ġclasse": 32400, "beitet": 32401, "iÄŁi": 32402, "Ġwhales": 32403, "Ġperimeter": 32404, "Ġtying": 32405, "Ġstrony": 32406, "Ġlikewise": 32407, "ĠPunch": 32408, "Da": 32409, "ĠBaptist": 32410, "Ġsorting": 32411, "Ġiv": 32412, "Ġíķ©": 32413, "Ġrehab": 32414, "Ġeta": 32415, "river": 32416, "Ġsai": 32417, "ãģĦãģŁãģł": 32418, "odus": 32419, "ãģĬé¡ĺãģĦãģĹãģ¾ãģĻ": 32420, "Ġessayer": 32421, "Ġturtles": 32422, "ĠHazrat": 32423, "Ġfabrics": 32424, "Ġcavity": 32425, "Ġponieważ": 32426, "Ġschlecht": 32427, "Ġsalsa": 32428, "ÅŁekkür": 32429, "Ġseating": 32430, "Ġeconomists": 32431, "Ġmang": 32432, "Ġseguinte": 32433, "Ġrang": 32434, "Ġratios": 32435, "Ġconstell": 32436, "Ġlongtemps": 32437, "uating": 32438, "Ġspoiled": 32439, "Ġrecipients": 32440, "Ġsniper": 32441, "ä¹ĭåīį": 32442, "ìĬµëĭĪê¹Į": 32443, "Ġwp": 32444, "ĠLINKE": 32445, "Ġflare": 32446, "ĠAdri": 32447, "ñas": 32448, "Ġbackl": 32449, "mÃ¤ÃŁ": 32450, "ĠBend": 32451, "Ġworkloads": 32452, "ĠÑģÑĥп": 32453, "Ġ1975": 32454, "имÑģÑı": 32455, "ане": 32456, "Ġмон": 32457, "Ġaspirations": 32458, "ĠAer": 32459, "ĠговоÑĢиÑĤÑĮ": 32460, "ĠQian": 32461, "å¦Ī": 32462, "Ġcompromised": 32463, "Ġyolk": 32464, "лаÑģÑĤ": 32465, "Ġhemen": 32466, "rove": 32467, "dens": 32468, "ĠкомменÑĤ": 32469, "Ġ---": 32470, "Ġfluores": 32471, "ноÑģ": 32472, "ĠLiverpool": 32473, "ĠÑģобой": 32474, "ĠZwe": 32475, "Ġlumin": 32476, "ĠOG": 32477, "á¸": 32478, "holm": 32479, "profits": 32480, "SN": 32481, "Ġproportions": 32482, "Ġmica": 32483, "ĠBoh": 32484, "ĠAtlas": 32485, "Ġunsure": 32486, "Ġtouring": 32487, "Ġnied": 32488, "ĠtÄĻ": 32489, "Ġimperative": 32490, "Ġdemek": 32491, "ĠSheriff": 32492, "rance": 32493, "Ġhomeland": 32494, "ĠHail": 32495, "ĠGanz": 32496, "ymm": 32497, "Mon": 32498, "åĨ·": 32499, "vida": 32500, "Ġdesarroll": 32501, "æĬĢ": 32502, "Ġintriguing": 32503, "ĠHugo": 32504, "ĠãĤĤ": 32505, "é¬": 32506, "аÑĨ": 32507, "ĠWiÄĻc": 32508, "atted": 32509, "ĠìķĦëĭĪê³ł": 32510, "ĠVari": 32511, "ád": 32512, "Ġsurreal": 32513, "Ġdisparities": 32514, "Ġmó": 32515, "ullen": 32516, "ĠìŀĪëĭ¤ê³ł": 32517, "ĠпожалÑĥйÑģÑĤа": 32518, "Ġmains": 32519, "Ġeject": 32520, "Ġmethane": 32521, "Ġmarginalized": 32522, "Ġchilli": 32523, "rès": 32524, "Ġyem": 32525, "ä½łæĺ¯": 32526, "ĠChun": 32527, "Ġdebts": 32528, "Ġdownloading": 32529, "ĠAthens": 32530, "isierung": 32531, "ryn": 32532, "Ġtekn": 32533, "ĠQuindi": 32534, "éľĢ": 32535, "Ġtaraf": 32536, "Ġhé": 32537, "Ġconsciously": 32538, "Ġfixes": 32539, "uckle": 32540, "mayın": 32541, "Ġfrei": 32542, "Ġspa": 32543, "Ġì§Ħíĸī": 32544, "ĠاÙĦذ": 32545, "ĠÑĥк": 32546, "lett": 32547, "ĠolmuÅŁ": 32548, "Ġcheesy": 32549, "าà¸ģ": 32550, "naire": 32551, "Ġwiden": 32552, "Ġlien": 32553, "Ġescaping": 32554, "iggs": 32555, "ĠBlick": 32556, "cÄħ": 32557, "ĠìĦľë": 32558, "Ġ×Ķס": 32559, "ĠвпеÑĢ": 32560, "ophone": 32561, "iell": 32562, "ĠSUBSCRI": 32563, "Ġlions": 32564, "Ġê·¸ê²ĥ": 32565, "Ġinspires": 32566, "Ġguarantees": 32567, "Ġcomeça": 32568, "ĠGrowing": 32569, "Ġneglig": 32570, "ĠFrankf": 32571, "Ġgegeben": 32572, "ĠÄijầu": 32573, "Ġendlich": 32574, "Ġìį¨": 32575, "ĠTT": 32576, "ĠLith": 32577, "ÏĢα": 32578, "astern": 32579, "ĠAzer": 32580, "Ġlunar": 32581, "hic": 32582, "ĠнаÑĢод": 32583, "Ġnenhum": 32584, "è·ij": 32585, "ĠSalvador": 32586, "ĠProgress": 32587, "Ġprivileges": 32588, "ĠëıĻìķĪ": 32589, "Ġantagon": 32590, "ĠImpf": 32591, "Ġdescub": 32592, "ĠLei": 32593, "ĠìĥĪë¡ľ": 32594, "Ñĩе": 32595, "Ġdólares": 32596, "ĠMeghan": 32597, "ĠWire": 32598, "too": 32599, "aying": 32600, "usc": 32601, "Ġtud": 32602, "Ġappeals": 32603, "educ": 32604, "Ġpane": 32605, "Ġji": 32606, "Ġdecks": 32607, "ĠAlter": 32608, "Ġå°±": 32609, "ìĦ¤": 32610, "åĪĨéIJĺ": 32611, "Ġproductions": 32612, "ĠWILLIAM": 32613, "Ġimplied": 32614, "Ġfulfillment": 32615, "ĠAah": 32616, "Ġsaja": 32617, "xus": 32618, "ĠÎļαι": 32619, "Ãłs": 32620, "ucch": 32621, "око": 32622, "ĠDiscord": 32623, "ĠSY": 32624, "jsk": 32625, "ĠWallace": 32626, "unction": 32627, "Daniel": 32628, "Ġköt": 32629, "ijah": 32630, "Ġmarche": 32631, "Ġdisgr": 32632, "Ġmungkin": 32633, "Ġalma": 32634, "³µ": 32635, "Ġextensively": 32636, "ĠFloren": 32637, "ĠAllison": 32638, "ãĤ±": 32639, "ÙĬÙħ": 32640, "Ġjuven": 32641, "ĠRenaissance": 32642, "Ġfundraising": 32643, "ĠChaos": 32644, "Ġparaly": 32645, "Ġnarrator": 32646, "Ġecosystems": 32647, "Ash": 32648, "Ġmitigation": 32649, "ĠAujourd": 32650, "ĠIdee": 32651, "!,": 32652, "Ġ½": 32653, "Ġlandlord": 32654, "Ġdefects": 32655, "Ġacre": 32656, "ulsive": 32657, "Ġalgae": 32658, "pek": 32659, "Ġemba": 32660, "ĠRoc": 32661, "éĽ¢": 32662, "ksom": 32663, "äche": 32664, "Ġleuk": 32665, "Ġleveraging": 32666, "Ġê·¸ëłĩì§Ģ": 32667, "ĠPalm": 32668, "Ġäven": 32669, "Ġlis": 32670, "ĠInsp": 32671, "ĠRita": 32672, "ĠAbb": 32673, "ithm": 32674, "Ġsupervision": 32675, "Ġrevisit": 32676, "ĠpiÄĻ": 32677, "Ġeuh": 32678, "Ġfades": 32679, "Ġmotto": 32680, "åį¡": 32681, "езж": 32682, "ĠShim": 32683, "Ġrelevance": 32684, "Ġoo": 32685, "Ġostat": 32686, "nica": 32687, "Ġchoix": 32688, "ĠFaculty": 32689, "Ġì¤ijìĹIJ": 32690, "ĠAbove": 32691, "ĠнеболÑĮÑĪ": 32692, "Ġsequencing": 32693, "Ġnutrient": 32694, "Ġconquered": 32695, "Ġdigestive": 32696, "Ġbackdrop": 32697, "ĠLori": 32698, "ailable": 32699, "Game": 32700, "Ġneglected": 32701, "omorph": 32702, "illah": 32703, "Ġkne": 32704, "Ġsiitä": 32705, "Ġworkspace": 32706, "ĠVenice": 32707, "ĠKne": 32708, "Ñīо": 32709, "ħĢ": 32710, "ĠHass": 32711, "Ġvita": 32712, "Ŀ¼ë©´": 32713, "Ġlays": 32714, "ências": 32715, "érica": 32716, "ĠLl": 32717, "æ±Ĥ": 32718, "ĠCoca": 32719, "ĠWHY": 32720, "èĪŀ": 32721, "Ġrouting": 32722, "Ġpermissions": 32723, "Ġdings": 32724, "prend": 32725, "program": 32726, "Ġcrocod": 32727, "bral": 32728, "AAAAAAAA": 32729, "agit": 32730, "ĠNä": 32731, "Ġgekommen": 32732, "atten": 32733, "Ġreferenced": 32734, "Ġpairing": 32735, "ĠPartner": 32736, "ĠCoronavirus": 32737, "ÑĸÑģ": 32738, "è½ī": 32739, "Ġ×Ķ×ĵ": 32740, "ĠespecÃŃfic": 32741, "arsi": 32742, "quelle": 32743, "Ġspontaneous": 32744, "çĨ±": 32745, "Ġê²ĥìĿĦ": 32746, "ĠÐŁÐ¾Ñģле": 32747, "ĠاÙĦد": 32748, "ĠShout": 32749, "Ġнал": 32750, "Ġdisguise": 32751, "ĠJord": 32752, "Ġwee": 32753, "Ġmiejsc": 32754, "Ġserum": 32755, "Ġplaisir": 32756, "Ġcredible": 32757, "ĠbÃ¥": 32758, "ĠAJ": 32759, "mares": 32760, "Ġrods": 32761, "Ġeran": 32762, "ãģ¾ãģĤ": 32763, "Ġpää": 32764, "ĠUA": 32765, "ĠUnknown": 32766, "ĠÙĦÙħ": 32767, "ĠRabbi": 32768, "Ġlaat": 32769, "Ġhairstyle": 32770, "Ġغ": 32771, "éģĭ": 32772, "Ġcach": 32773, "ĠWriting": 32774, "оÑĩки": 32775, "abad": 32776, "Ġstraighten": 32777, "--\"": 32778, "wife": 32779, "Ġhottest": 32780, "Ġpunya": 32781, "ĠFashion": 32782, "griff": 32783, "ĠQR": 32784, "otch": 32785, "ĠÐľÐ¾Ð¶ÐµÑĤ": 32786, "Cloud": 32787, "ĠStrike": 32788, "ĠHein": 32789, "Ġ羣çļĦ": 32790, "Ġlei": 32791, "ĠFlow": 32792, "wegs": 32793, "Ġhabr": 32794, "åīĽåīĽ": 32795, "nahme": 32796, "Ìģ": 32797, "Ġpleasing": 32798, "opping": 32799, "Ġ구ëıħ": 32800, "Ġdran": 32801, "Ġbangs": 32802, "Ġ79": 32803, "Ġsket": 32804, "Ġcaval": 32805, "ĠMacron": 32806, "Ġweighted": 32807, "Ġmuted": 32808, "Ġnuestras": 32809, "EEP": 32810, "Ġmathematic": 32811, "ĠMRI": 32812, "agus": 32813, "Ġtherapies": 32814, "θε": 32815, "Ġunpl": 32816, "Ġcommencer": 32817, "full": 32818, "Ġtowels": 32819, "Ġprue": 32820, "Ġlicenses": 32821, "׼×ķ׾": 32822, "ĠÐŁÐ¾ÑĩемÑĥ": 32823, "Ġpointless": 32824, "Bye": 32825, "Ġeligibility": 32826, "Ġscrape": 32827, "Ġabusive": 32828, "ĠMant": 32829, "Ġjeunes": 32830, "tal": 32831, "ĠPrincip": 32832, "ĠOrthodox": 32833, "Ġmelod": 32834, "ĠмаÑĤеÑĢи": 32835, "Ġprosecutor": 32836, "Ġopioid": 32837, "ĠÑĥвеÑĢ": 32838, "ĠBeen": 32839, "Ġìłijì¢ħ": 32840, "Ġdynasty": 32841, "Ġajuda": 32842, "Ġentreg": 32843, "Ġweighed": 32844, "Ġeure": 32845, "ĠBem": 32846, "Ġabnormal": 32847, "82": 32848, "ĠJR": 32849, "ĠAkt": 32850, "ĠBri": 32851, "út": 32852, "Ġstagn": 32853, "!*": 32854, "Ġwegen": 32855, "Ġleaking": 32856, "ĠWords": 32857, "ĠMau": 32858, "Ġvue": 32859, "ĠLiam": 32860, "анием": 32861, "Ġclinicians": 32862, "ĠPump": 32863, "Ġförst": 32864, "?...": 32865, "Ġautomotive": 32866, "ĠOwen": 32867, "zusagen": 32868, "ĠHundred": 32869, "Ġdecentralized": 32870, "Ġbulbs": 32871, "Ġ׾׼": 32872, "Ġprovinces": 32873, "ĠMilan": 32874, "81": 32875, "kas": 32876, "Ġëĵ£": 32877, "Ġforça": 32878, "Ġrightly": 32879, "å³¶": 32880, "rÄħ": 32881, "Ġvenues": 32882, "Ġwai": 32883, "Ġpredicting": 32884, "ĠWiFi": 32885, "Ġê¶ģê¸Ī": 32886, "رÙĪ": 32887, "Ġ×Ķ×ĸ": 32888, "century": 32889, "Ġgradual": 32890, "ĠProbleme": 32891, "ĠìĹħ": 32892, "Ġcoping": 32893, "ĠBrus": 32894, "Ġpeanuts": 32895, "irtschaft": 32896, "Ġзал": 32897, "ĠTroy": 32898, "Ġsperm": 32899, "ĠMitar": 32900, "ĠTürkiye": 32901, "grand": 32902, "¦Ń": 32903, "Ġ×ŀס": 32904, "Ġpans": 32905, "ĠKnowledge": 32906, "berly": 32907, "ĠÐķго": 32908, "Ġdanced": 32909, "ĠFrost": 32910, "ĠBurg": 32911, "Ġbiting": 32912, "ìłķìĿĦ": 32913, "meal": 32914, "Ġheroic": 32915, "Ġmotherboard": 32916, "ĠLicht": 32917, "ãģ£ãģ": 32918, "llan": 32919, "айн": 32920, "ĠÑĢÑıд": 32921, "Ġà¹Ģà¸": 32922, "onen": 32923, "irie": 32924, "Art": 32925, "rang": 32926, "νη": 32927, "Ġnewborn": 32928, "Ġamis": 32929, "ĠاÙĪØ±": 32930, "Ġsophom": 32931, "ĠCareful": 32932, "Ġprospects": 32933, "ensen": 32934, "Ġthrill": 32935, "ĠViá»ĩt": 32936, "Adam": 32937, "rition": 32938, "entric": 32939, "uden": 32940, "Ġcertificates": 32941, "Ġashes": 32942, "調": 32943, "playing": 32944, "Ġsadece": 32945, "Ġost": 32946, "Ġairplanes": 32947, "ÑĢок": 32948, "oner": 32949, "Ġmagnesium": 32950, "Ġgoddamn": 32951, "Ġ1972": 32952, "ĠSchule": 32953, "Ġtemat": 32954, "Ġpartout": 32955, "à¯Ĥ": 32956, "Ġinve": 32957, "ĠScientists": 32958, "ĠHudson": 32959, "winning": 32960, "ceksin": 32961, "Ġcongressional": 32962, "oru": 32963, "Ġropes": 32964, "вед": 32965, "Ġmadre": 32966, "Ġferry": 32967, "ĠCohen": 32968, "ĠPred": 32969, "Ġvagy": 32970, "ĠбеÑģп": 32971, "Ġmultim": 32972, "Ġdrainage": 32973, "Ġsimulator": 32974, "giggles": 32975, "ĠStadium": 32976, "обÑī": 32977, "Ġnotices": 32978, "Ġcrawling": 32979, "Ġgroupe": 32980, "åı¸": 32981, "ĠktoÅĽ": 32982, "ĠYoga": 32983, "Ġmedida": 32984, "ĠÑħваÑĤ": 32985, "ĠLite": 32986, "Ġrav": 32987, "orama": 32988, "Ġdiscord": 32989, "ĠDIRE": 32990, "Ġteh": 32991, "ĠNurs": 32992, "ç²ī": 32993, "Ġpitched": 32994, "Ġbarking": 32995, "ĠCoke": 32996, "wiad": 32997, "Ġpopulated": 32998, "éϤ": 32999, "pelled": 33000, "Ġбог": 33001, "Ġpewno": 33002, "ĠCube": 33003, "Ġrecruited": 33004, "éĢĻ種": 33005, "ĠCara": 33006, "ıģını": 33007, "imated": 33008, "ĠÑĪкол": 33009, "icional": 33010, "ĠпÑĢоÑĦ": 33011, "Ġcontamination": 33012, "Ġúltimos": 33013, "Ġfearful": 33014, "Ġelephants": 33015, "usi": 33016, "ĠiTunes": 33017, "ĠSwami": 33018, "ê¼": 33019, "ĠìĦ¤ëªħ": 33020, "ĠRichards": 33021, "Ġmagnets": 33022, "ĠRichtung": 33023, "ĠLegion": 33024, "èıľ": 33025, "Ġkitty": 33026, "Ġkissed": 33027, "Ġwatering": 33028, "Ġcono": 33029, "ĠPalestine": 33030, "idir": 33031, "Ġmaze": 33032, "Ġfluids": 33033, "ĠProducer": 33034, "ĠKrsna": 33035, "好åķ¦": 33036, "laf": 33037, "Ġ×IJ×ķ": 33038, "Ġmiesz": 33039, "ĠXing": 33040, "ointed": 33041, "sein": 33042, "ĠFuk": 33043, "ĠDepression": 33044, "ĠDuty": 33045, "ĠPanther": 33046, "Ġsund": 33047, "Ġrefere": 33048, "Ġexclusion": 33049, "Ġnaval": 33050, "ĠWinston": 33051, "Ġslogan": 33052, "Ġhypothetical": 33053, "Ġelevate": 33054, "ëł¹": 33055, "Ġcabeça": 33056, "ĠGesund": 33057, "meter": 33058, "ĠìķĦëĭĪë©´": 33059, "Ġcloudy": 33060, "â̦?": 33061, "ĠSchritt": 33062, "ĠJS": 33063, "ìį": 33064, "ĠSprings": 33065, "ĠBatter": 33066, "·°": 33067, "Ġtailor": 33068, "ĠPTSD": 33069, "ĠGent": 33070, "ĠbaÄŁ": 33071, "Ġspatula": 33072, "Ġcray": 33073, "ĠLegisl": 33074, "Ġsú": 33075, "Ġleve": 33076, "าม": 33077, "Ġerad": 33078, "Ġdong": 33079, "Ġderm": 33080, "ĠBanks": 33081, "icho": 33082, "åħĪçĶŁ": 33083, "ĠFranz": 33084, "ravel": 33085, "éģĶ": 33086, "оло": 33087, "Ġflute": 33088, "ĠEk": 33089, "Ġjoyful": 33090, "Ġchased": 33091, "ĠLarge": 33092, "Over": 33093, "Ġentrepreneurial": 33094, "Ġconsiders": 33095, "Ñĥем": 33096, "opa": 33097, "Ġdormir": 33098, "ĠElementary": 33099, "Ġprzypad": 33100, "ÑĥÑģка": 33101, "ĠоÑĩеÑĢ": 33102, "ugene": 33103, "Ġtenido": 33104, "Ġlugares": 33105, "ë¥": 33106, "ĠÑĩаÑģÑĤ": 33107, "Ġsao": 33108, "Ġbraid": 33109, "ĠVere": 33110, "ĠReich": 33111, "ĠPoss": 33112, "Ġinan": 33113, "wand": 33114, "ref": 33115, "Ġmontrer": 33116, "Ġ1981": 33117, "çķª": 33118, "asında": 33119, "Ġchrome": 33120, "ĠTrinity": 33121, "Ġexploitation": 33122, "ĠSense": 33123, "ĠCMS": 33124, "ĠNoble": 33125, "ĠìĦłíĥĿ": 33126, "Ġswelling": 33127, "electronic": 33128, "]?": 33129, "Ġbrushing": 33130, "Ġliquidity": 33131, "ĠHook": 33132, "ĠConnor": 33133, "ĠAlum": 33134, "Ġgucken": 33135, "suite": 33136, "Ġwiele": 33137, "Ġbarrels": 33138, "ĠRegel": 33139, "ĠMent": 33140, "ĠTrip": 33141, "ĠBrush": 33142, "ĠErik": 33143, "urate": 33144, "ÉĻr": 33145, "ĠCyr": 33146, "ouble": 33147, "ĠBecca": 33148, "Ġpasswords": 33149, "ű": 33150, "borg": 33151, "Ġvendo": 33152, "ĠClaus": 33153, "ĠFaz": 33154, "indest": 33155, "Ġdeceased": 33156, "Ġcomparisons": 33157, "ĠLCD": 33158, "ĠPork": 33159, "Ġeventual": 33160, "Ġpatreon": 33161, "Ġinability": 33162, "Ġextinction": 33163, "Ġì¢ĭìķĦíķĺëĬĶ": 33164, "ĠÑģоÑģ": 33165, "aju": 33166, "Ġ×ij×IJ×": 33167, "Ġsofort": 33168, "Ġdestined": 33169, "ĠRin": 33170, "Ġmouths": 33171, "ĠNatürlich": 33172, "Ġpreserving": 33173, "Ġlimp": 33174, "黨": 33175, "ocused": 33176, "инг": 33177, "Ġexposing": 33178, "Ġξ": 33179, "ëį": 33180, "laugh": 33181, "Ġhiss": 33182, "ãģłãģĭãĤī": 33183, "Ġindie": 33184, "Ġdetal": 33185, "ÑĢавÑģÑĤв": 33186, "Ġtrên": 33187, "æķ°": 33188, "Ġogni": 33189, "Ġsimplemente": 33190, "Ġ1978": 33191, "Ġgoo": 33192, "Ġ1967": 33193, "Ġgenug": 33194, "hö": 33195, "Ġhistó": 33196, "å®Ł": 33197, "Ġlobster": 33198, "cendo": 33199, "Ġteil": 33200, "Ġallevi": 33201, "0000": 33202, "OLD": 33203, "Ġpesos": 33204, "Ġbonuses": 33205, "Ġami": 33206, "Ġrevival": 33207, "ĠHorse": 33208, "Ġsack": 33209, "Talk": 33210, "Ġmulher": 33211, "ĠпоÑģÑĤоÑıн": 33212, "ĠHood": 33213, "Huh": 33214, "Ġë¶ģ": 33215, "Ġhyung": 33216, "ĠMeeting": 33217, "Ġimporta": 33218, "Ġì°¾ìķĦ": 33219, "ĠVern": 33220, "Ġstripped": 33221, "Ġrefuses": 33222, "Ġqualifications": 33223, "opl": 33224, "ĢëıĦ": 33225, "ixÃŃ": 33226, "Ġdiab": 33227, "itime": 33228, "flows": 33229, "Ġinac": 33230, "ĠGong": 33231, "Ġmeaningless": 33232, "Ġcourageous": 33233, "Ġmicrobi": 33234, "azy": 33235, "hist": 33236, "Ġvolunteering": 33237, "VIE": 33238, "Ġviolated": 33239, "Ġsympathy": 33240, "ĠEdit": 33241, "好åĥı": 33242, "electric": 33243, "product": 33244, "Ġpandemia": 33245, "Ġgeometric": 33246, "ĠConvers": 33247, "gre": 33248, "Ġglut": 33249, "isted": 33250, "ĠاÙĦÙĥ": 33251, "ĠChain": 33252, "ĠPresent": 33253, "ĠYin": 33254, "ĠÑģог": 33255, "ĠVlog": 33256, "Ġìĸ´ë¨¸": 33257, "Ġdonn": 33258, "Ġhitch": 33259, "ucking": 33260, "ãģĬãģĦ": 33261, "wald": 33262, "risk": 33263, "Ġhari": 33264, "ĠKens": 33265, "ĠIdol": 33266, "Ġвнимание": 33267, "Ġtodd": 33268, "Ġsmashed": 33269, "Ġinvari": 33270, "ĠконÑĤÑĢ": 33271, "Ġautistic": 33272, "ìŀ¥ëĭĺ": 33273, "Res": 33274, "дÑĭ": 33275, "chau": 33276, "Ġselv": 33277, "Ġhätten": 33278, "ि": 33279, "Ġexpects": 33280, "Ïģη": 33281, "Ġaçık": 33282, "ĠHTTP": 33283, "leÅŁ": 33284, "Ġsweeping": 33285, "ĠBeta": 33286, "Ġcounterparts": 33287, "abile": 33288, "ĠSims": 33289, "Cs": 33290, "Ġrepar": 33291, "squ": 33292, "Ġprovincial": 33293, "Ġshareholders": 33294, "Ġrunter": 33295, "Ġgedacht": 33296, "ĠTeen": 33297, "Ġgrands": 33298, "çĶ¢": 33299, "agles": 33300, "Ġrocky": 33301, "vens": 33302, "Ġrivals": 33303, "unal": 33304, "Ġreacts": 33305, "ë©": 33306, "Ġmercury": 33307, "ĠLuigi": 33308, "Ġог": 33309, "ĠJUST": 33310, "Ġlod": 33311, "Ġcortex": 33312, "wig": 33313, "Ġlakh": 33314, "ì¤ijìĹIJ": 33315, "ĠVic": 33316, "ĠMund": 33317, "Ġmapped": 33318, "ĠDell": 33319, "ĠDruck": 33320, "Ġlifes": 33321, "алÑĮное": 33322, "ividual": 33323, "adım": 33324, "Ġatrav": 33325, "ĠFlug": 33326, "ĠKlein": 33327, "ê±°ìķ¼": 33328, "หà¸Ļ": 33329, "Ġappli": 33330, "ா?": 33331, "üyorum": 33332, "ĠинÑĤеÑĢеÑģно": 33333, "Ġdisinfect": 33334, ">-": 33335, "Ġchampagne": 33336, "Ġkla": 33337, "opers": 33338, "Trans": 33339, "ĠDesert": 33340, "Ġcultivate": 33341, "ĠFucking": 33342, "idelity": 33343, "ĠÑĤан": 33344, "Ġincub": 33345, "Ġtemu": 33346, "Ġlearner": 33347, "founder": 33348, "ĠSyl": 33349, "ãĤĢ": 33350, "Ġfato": 33351, "zier": 33352, "ĠìĹĨìĿ´": 33353, "Ġì΍": 33354, "Ġpsycho": 33355, "ĠÑĤелеÑĦ": 33356, "Ġregarde": 33357, "Ġrepresentations": 33358, "Ġlitigation": 33359, "Ġspann": 33360, "ults": 33361, "bior": 33362, "è¦ĭãģ¦": 33363, "ä¸įå¤ļ": 33364, "ĠSurvey": 33365, "ĠLEDs": 33366, "Ġträ": 33367, "Ġlên": 33368, "Ġantioxid": 33369, "еÑĢом": 33370, "Ġinduction": 33371, "Ġfooled": 33372, "ätzlich": 33373, "ĠговоÑĢÑıÑĤ": 33374, "ĠFact": 33375, "umbai": 33376, "Ġwiggle": 33377, "NOUN": 33378, "Ġdévelopp": 33379, "ĠClaro": 33380, "Ġì¸": 33381, "ë¬": 33382, "ãģªãĤĵãģł": 33383, "Ġaccumulate": 33384, "Ġmaintains": 33385, "ëĦ": 33386, "ĠFighter": 33387, "íĨł": 33388, "Ġmatin": 33389, "Ġcoupon": 33390, "Ġstunt": 33391, "Ġdebuted": 33392, "å¾ħãģ£ãģ¦": 33393, "Ġprag": 33394, "иваем": 33395, "73": 33396, "Ġexpres": 33397, "Ġìĺ¤ë¹ł": 33398, "ĠпеÑĢÑģон": 33399, "Ġcalculus": 33400, "Ġabrupt": 33401, "ĠInspector": 33402, "ourt": 33403, "æĸĻ": 33404, "źniej": 33405, "intense": 33406, "Ba": 33407, "Ġlounge": 33408, "Ġasthma": 33409, "ĠHiç": 33410, "ª»": 33411, "Ġeditorial": 33412, "Ġseize": 33413, "Ġkır": 33414, "Ġmouve": 33415, "Ġtierra": 33416, "Ġtestosterone": 33417, "Ġrh": 33418, "ĠKingston": 33419, "ELLE": 33420, "ĠRepresentative": 33421, "Ġ1974": 33422, "Ġiba": 33423, "Ts": 33424, "Ġsorta": 33425, "Ġ(?)": 33426, "ĠتÙĪ": 33427, "ĠëĤ´ëł¤": 33428, "Ġbekommt": 33429, "Ġspiritually": 33430, "Ġdistorted": 33431, "Mad": 33432, "Ġreim": 33433, "ánh": 33434, "ĠOttoman": 33435, "ĠRelig": 33436, "ĠEls": 33437, "Ġretained": 33438, "ĠLaughs": 33439, "æĢ»": 33440, "ĠSAS": 33441, "ĠколиÑĩеÑģÑĤво": 33442, "×ķתר": 33443, "Ġinnovate": 33444, "Ġkork": 33445, "ĠÑĢаÑģÑģказÑĭв": 33446, "ondere": 33447, "ivi": 33448, "aye": 33449, "ounty": 33450, "ĠполÑĥÑĩаеÑĤÑģÑı": 33451, "Ġbuns": 33452, "åħ«": 33453, "Ġyüzden": 33454, "Ġsurgeries": 33455, "Ø£ÙĨ": 33456, "Ġbankruptcy": 33457, "welt": 33458, "Ġsiamo": 33459, "Ġdarkest": 33460, "ĠHann": 33461, "gga": 33462, "Ġformas": 33463, "ĠDj": 33464, "named": 33465, "Ġshields": 33466, "ueller": 33467, "ĠFew": 33468, "Ġlace": 33469, "Ġfurious": 33470, "ĠYU": 33471, "Ġsocietal": 33472, "Ġjudgement": 33473, "ĠDos": 33474, "Ġjab": 33475, "laws": 33476, "Ġreinvent": 33477, "ĠKatherine": 33478, "ĠChoi": 33479, "adows": 33480, "Ġrans": 33481, "oden": 33482, "ĠMidwest": 33483, "nın": 33484, "Ġdeport": 33485, "ĠDip": 33486, "ç´ħ": 33487, "Ġatención": 33488, "ĠCourtney": 33489, "ividad": 33490, "ĠÚ©Ûģ": 33491, "Ġefficacy": 33492, "ĠBrooks": 33493, "Ġreferral": 33494, "ĠконÑĨ": 33495, "Ġmalicious": 33496, "Ġkir": 33497, "ĠGoddess": 33498, "Ġfunky": 33499, "Ġinterim": 33500, "ĠKörper": 33501, "Ġìĸ¼ë§": 33502, "kur": 33503, "Ġкли": 33504, "Ġtrucs": 33505, "gesetz": 33506, "Ġzug": 33507, "ĠGlück": 33508, "ĠMinute": 33509, "Ġprestigious": 33510, "Ġniez": 33511, "Ġconcentrations": 33512, "лаÑģÑĤи": 33513, "ĠSis": 33514, "ĠVitamin": 33515, "kov": 33516, "ĠPBS": 33517, "Ġнее": 33518, "Ġretailers": 33519, "Ġconventions": 33520, "ĠSamantha": 33521, "Ġproudly": 33522, "Jordan": 33523, "ĠJASON": 33524, "atk": 33525, "Ġtriste": 33526, "Ġstär": 33527, "Ġreiterate": 33528, "Ġposterior": 33529, "Ġ1973": 33530, "ĠPine": 33531, "ĠJuliet": 33532, "Ġpedir": 33533, "kil": 33534, "Ġoverlapping": 33535, "Ġexclude": 33536, "Ġeconóm": 33537, "Ġaccepts": 33538, "ĠSter": 33539, "決": 33540, "Ġìļ´ëıĻ": 33541, "estab": 33542, "Ġtug": 33543, "arg": 33544, "Ġlivro": 33545, "اص": 33546, "Ġseams": 33547, "Ġburaya": 33548, "Ġello": 33549, "ĠTM": 33550, "ĠPaw": 33551, "ĠIndex": 33552, "Exc": 33553, "Ġinspirational": 33554, "Ġdunk": 33555, "è°ģ": 33556, "akter": 33557, "Ġconditioner": 33558, "ĠSalut": 33559, "ÅĤec": 33560, "Ġìī½": 33561, "ĠÑĥзна": 33562, "ĠRomeo": 33563, "fruit": 33564, "ĠYO": 33565, "Ġchá»ī": 33566, "бÑĥ": 33567, "bons": 33568, "Ġreproductive": 33569, "Ġorada": 33570, "Ġíļ¨": 33571, "Ġtentar": 33572, "Ġmañana": 33573, "ãĤ¬": 33574, "Ġsolvent": 33575, "Jessica": 33576, "ĠLegal": 33577, "Ġtua": 33578, "Ġsic": 33579, "ĠEQ": 33580, "aukee": 33581, "ìĭľëĭ¤": 33582, "ĠÅŀu": 33583, "Ġadhere": 33584, "ĠTul": 33585, "Ġà®Ĩ": 33586, "Ġtextbooks": 33587, "ĠFifth": 33588, "Ġexperi": 33589, "Ġchic": 33590, "Ġheap": 33591, "inely": 33592, "atra": 33593, "Two": 33594, "Ġhelemaal": 33595, "Ġfren": 33596, "æİ¨": 33597, "Ġbisher": 33598, "اش": 33599, "ĠìĦłìĥĿ": 33600, "ĠTages": 33601, "Ġsá»±": 33602, "Ġbullied": 33603, "ؤ": 33604, "Ġbenefited": 33605, "ĠPreviously": 33606, "ĠÑįÑĦÑĦ": 33607, "Ùį": 33608, "Ġsenate": 33609, "ĠMorm": 33610, "ijke": 33611, "ĠFlu": 33612, "Ġincorporating": 33613, "jack": 33614, "ĠпиÑĤ": 33615, "Ġimply": 33616, "Ġhacks": 33617, "ĠRICH": 33618, "ĠкваÑĢ": 33619, "ĠпÑĢекÑĢаÑģ": 33620, "Ġdependency": 33621, "Ġìļ©": 33622, "Ġì±ħ": 33623, "Ġwährend": 33624, "Ġsulla": 33625, "ĠPittsburgh": 33626, "Ġesempio": 33627, "¼ë¡ľ": 33628, "prot": 33629, "ĠRosen": 33630, "ĠIndependence": 33631, "Ġparsley": 33632, "iegen": 33633, "Ġhaw": 33634, "Ġaquell": 33635, "ĠCAP": 33636, "ĠÑĢабоÑĤаÑĤÑĮ": 33637, "ĠCliff": 33638, "ionar": 33639, "Ġsecuring": 33640, "æĪijåĢijçļĦ": 33641, "νε": 33642, "Ġutilis": 33643, "Ġcoule": 33644, "ĠPing": 33645, "Ġtrek": 33646, "Ġfak": 33647, "Ġenorme": 33648, "Ġìĭ«": 33649, "让": 33650, "Ġdoubling": 33651, "ĠнÑĢавиÑĤÑģÑı": 33652, "Ġhed": 33653, "hoven": 33654, "ĠStanding": 33655, "ĠmÃŃn": 33656, "ĠJimin": 33657, "Ġmonarch": 33658, "Ġcoke": 33659, "Ġmr": 33660, "Ġclic": 33661, "Ãį": 33662, "Ġimpeachment": 33663, "Ġdurability": 33664, "Ġvarios": 33665, "Ġcommercials": 33666, "Ġgreetings": 33667, "ĠRi": 33668, "ĠAppreci": 33669, "ìŀĪëĬĶ": 33670, "Ġrésult": 33671, "ért": 33672, "Ġsalute": 33673, "Ġpoderia": 33674, "Ġsunrise": 33675, "veck": 33676, "Ġreluctant": 33677, "Ġcommissioner": 33678, "念": 33679, "âte": 33680, "ĠKenny": 33681, "ĠSiri": 33682, "ãĥĥãĥĹ": 33683, "ĠëĬĺ": 33684, "ĠEE": 33685, "Ġunch": 33686, "кон": 33687, "ĠاÙĦØ¥": 33688, "Ġbelts": 33689, "Ġhass": 33690, "ĠмоÑı": 33691, "Ġdisplaced": 33692, "Ġabra": 33693, "ÎŃλ": 33694, "Ġscratches": 33695, "Ġcomet": 33696, "Ġauthorization": 33697, "ĠLLC": 33698, "Ġproduk": 33699, "Ġrehabilitation": 33700, "åŀ": 33701, "ÑĸÑĩ": 33702, "uding": 33703, "olit": 33704, "Ġ105": 33705, "Ġexpands": 33706, "Ġaltri": 33707, "ĠKomment": 33708, "Ġanf": 33709, "Pl": 33710, "ĠMana": 33711, "fed": 33712, "Ġbri": 33713, "Ġora": 33714, "Gs": 33715, "ĠGur": 33716, "uckland": 33717, "Ġjunction": 33718, "Ġironic": 33719, "ĠFeed": 33720, "Ġprakt": 33721, "ĠHammer": 33722, "ĮëıĦ": 33723, "ĠTracy": 33724, "çµ±": 33725, "ĠAside": 33726, "него": 33727, "ĠиÑģполÑĮзоваÑĤÑĮ": 33728, "Ġzaj": 33729, "Ġequitable": 33730, "Ġcurb": 33731, "ĠãģĵãĤĮ": 33732, "Ġderivatives": 33733, "Ġpuppies": 33734, "ĠKenneth": 33735, "ĠCompl": 33736, "igram": 33737, "ĠGarcia": 33738, ")\"": 33739, "ĠHarbor": 33740, "estial": 33741, "Ġä¾Ĩ": 33742, "Ġers": 33743, "æ¹": 33744, "Ġunwanted": 33745, "Ġbelang": 33746, "аго": 33747, "emb": 33748, "dos": 33749, "ĠìĻľë": 33750, "ĠBudget": 33751, "Ġbattling": 33752, "ØŃت": 33753, "kok": 33754, "наÑĩала": 33755, "Ġplag": 33756, "Ġcantidad": 33757, "Ġgrupos": 33758, "Ġplugins": 33759, "lerini": 33760, "ĠимееÑĤ": 33761, "Ġsozusagen": 33762, "olics": 33763, "Ġpueblo": 33764, "Ġreminis": 33765, "rän": 33766, "ĠMorrison": 33767, "Ġlinha": 33768, "Ġbreaths": 33769, "ĠTaste": 33770, "Ġenfrent": 33771, "ĠDocker": 33772, "Ġден": 33773, "Ġethnicity": 33774, "Ġwob": 33775, "Ġsuffers": 33776, "Ġtransitioning": 33777, "ĠRange": 33778, "ÄĻdzy": 33779, "ĠкаÑĤ": 33780, "Ġsyner": 33781, "Ġdonut": 33782, "Ġprobabilities": 33783, "ĠOmar": 33784, "Which": 33785, "uish": 33786, "isin": 33787, "Ġdemos": 33788, "ĠìłĢ기": 33789, "Ġëĺijê°Ļ": 33790, "Ġедин": 33791, "Ġcerve": 33792, "Ġjoka": 33793, "IAN": 33794, "Ġkilometer": 33795, "Ġhorizontally": 33796, "ĠBhag": 33797, "Ġ->": 33798, "ĠMonitor": 33799, "Ġknowledgeable": 33800, "Ġfav": 33801, "Ġpinned": 33802, "ĠeBay": 33803, "icker": 33804, "Ġìŀłê¹IJë§Į": 33805, "ĠXiaomi": 33806, "Ġcapit": 33807, "Ġnp": 33808, "Ġ1965": 33809, "hoe": 33810, "Ġnok": 33811, "ĠSage": 33812, "ĠнелÑĮзÑı": 33813, "ĠTow": 33814, "gam": 33815, "Ġdicen": 33816, "ĠSUBSCRIBE": 33817, "Ġreboot": 33818, "Ġpaj": 33819, "Ġë³´ìŬë": 33820, "Ġthicken": 33821, "ĠReality": 33822, "idän": 33823, "Na": 33824, "Ġê²ĥìĿĢ": 33825, "!!)": 33826, "Ġroutines": 33827, "Ġодного": 33828, "Ġexting": 33829, "Ġì¦Ŀ": 33830, "Ġsulfur": 33831, "Ġcarve": 33832, "Ġasteroid": 33833, "ĠWarrior": 33834, "Ġphotographers": 33835, "Ġpell": 33836, "Ġcrossover": 33837, "æĪijçŁ¥éģĵ": 33838, "Ġhacemos": 33839, "ĠNej": 33840, "Ġsettling": 33841, "Ġirm": 33842, "ĠBooks": 33843, "ientôt": 33844, "Ġespacio": 33845, "ĠScholars": 33846, "Ġdoomed": 33847, "ĠIRS": 33848, "wohl": 33849, "Ġsegue": 33850, "ĠëĪĦê°Ģ": 33851, "Ġpratic": 33852, "BT": 33853, "ĠConsidering": 33854, "ĠBuffalo": 33855, "Ġtrainings": 33856, "Ġgebru": 33857, "ĠGleich": 33858, "Ġpirates": 33859, "Ġenvelop": 33860, "Ġreopen": 33861, "imat": 33862, "Ġtee": 33863, "Ġsued": 33864, "feh": 33865, "Ġ×Ķ×§": 33866, "Ġdiets": 33867, "Ġjuntos": 33868, "asto": 33869, "Ġmisunderstood": 33870, "Ġruim": 33871, "Ġclassify": 33872, "ĠпÑĢодÑĥк": 33873, "Ġinse": 33874, "Ġillustrated": 33875, "Ġcorrosion": 33876, "Ġaccred": 33877, "ĠAuntie": 33878, "ĠпÑĢивеÑĤ": 33879, "ĠLIVE": 33880, "Ġrek": 33881, "Ġreceipt": 33882, "åΰåºķ": 33883, "ĠBarbie": 33884, "ĠSnake": 33885, "turn": 33886, "Jeff": 33887, "ãģĬãģĬ": 33888, "ķĦ": 33889, "VOICEOVER": 33890, "coll": 33891, "Ġrunners": 33892, "ìłľë": 33893, "osos": 33894, "moon": 33895, "Ġkeynote": 33896, "ĠInstit": 33897, "SPEAK": 33898, "Ġplugs": 33899, "Ġcurv": 33900, "ĠYuri": 33901, "ĠTheres": 33902, "ĠPs": 33903, "ĠμÏĢο": 33904, "Ġconverter": 33905, "Ġrefine": 33906, "Ġbadass": 33907, "Ġοι": 33908, "Ġregen": 33909, "azzi": 33910, "ÙĬÙģ": 33911, "Ġseized": 33912, "Ġiçer": 33913, "ilee": 33914, "Ġupstream": 33915, "Ġbuds": 33916, "Ġpim": 33917, "Ġíķĺ루": 33918, "Ġalluded": 33919, "Ġthemed": 33920, "Ġconsisting": 33921, "Ġbons": 33922, "unuz": 33923, "ĠпÑĢовод": 33924, "ĠLovely": 33925, "à¥ĭ": 33926, "Ġparach": 33927, "ĠStaats": 33928, "éļĬ": 33929, "Ġselective": 33930, "Ġfase": 33931, "ĠGeorget": 33932, "Ġcocaine": 33933, "Ġreproduction": 33934, "ĠLara": 33935, "ĠLD": 33936, "Ġgh": 33937, "Jon": 33938, "ĠlÃ¥": 33939, "ĠëijIJë": 33940, "Ġtyped": 33941, "ĠBana": 33942, "ëĵľë": 33943, "Ġsavory": 33944, "ĠZomb": 33945, "standen": 33946, "Ġpedestrian": 33947, "Ġdifférents": 33948, "Ġìĭ¸": 33949, "èī¯": 33950, "Ġcomplained": 33951, "ç¦ı": 33952, "ĠÐļÑĤо": 33953, "Ġ׾פ": 33954, "aliÅĽmy": 33955, "Ġmortar": 33956, "Ġverdict": 33957, "Ġsuficiente": 33958, "ĠMillion": 33959, "mittel": 33960, "inals": 33961, "ĠاÙĦØ®": 33962, "аÑİÑģÑĮ": 33963, "ĠmiÄĻdzy": 33964, "ĠOle": 33965, "Ġinvert": 33966, "czyÄĩ": 33967, "озможно": 33968, "starter": 33969, "Ġauditor": 33970, "ĠScout": 33971, "chien": 33972, "ĠSverige": 33973, "uffled": 33974, "Ġzehn": 33975, "ĠAuckland": 33976, "Ġargent": 33977, "Ġ1976": 33978, "ĠHoe": 33979, "Ġbothers": 33980, "Ġsocialist": 33981, "Ġpliers": 33982, "Ġemergen": 33983, "ĠXP": 33984, "еÑĢов": 33985, "More": 33986, "ĠLevi": 33987, "ĠAnders": 33988, "ibilidad": 33989, "ĠParents": 33990, "Ġinduced": 33991, "ìĸ´ì¤": 33992, "Ġbalances": 33993, "ĠвÑĭÑĪ": 33994, "Ġsubmarine": 33995, "Start": 33996, "Ġdries": 33997, "Ġvolver": 33998, "Ġticking": 33999, "cott": 34000, "Ġfaj": 34001, "prés": 34002, "ĠSabb": 34003, "ĠзаÑĩ": 34004, "ĠпокÑĥп": 34005, "Ġbaptized": 34006, "ĠBrilliant": 34007, "ĠÐijог": 34008, "Ġmots": 34009, "bits": 34010, "Ġlattice": 34011, "æĪijè·Łä½ł": 34012, "Ġcoriander": 34013, "Ġresidency": 34014, "ync": 34015, "Ġpierwszy": 34016, "ĠKnock": 34017, "ĠZap": 34018, "ĠÐķв": 34019, "견": 34020, "å°ıå¿ĥ": 34021, "Ġuneven": 34022, "ĠJas": 34023, "odor": 34024, "ç¿Ĵ": 34025, "74": 34026, "ĠSite": 34027, "Ġaconteceu": 34028, "ympt": 34029, "Ġtrilogy": 34030, "Ġlantern": 34031, "ĠZucker": 34032, "vari": 34033, "welling": 34034, "ĠPotato": 34035, "gomery": 34036, "Ġreacted": 34037, "ĠChron": 34038, "Ġjede": 34039, "beeld": 34040, "Ġtwent": 34041, "Ġlact": 34042, "æ¨Ĥ": 34043, "Ġrése": 34044, "Ġrelent": 34045, "Ġfurnace": 34046, "Ġwidget": 34047, "Ġearthquakes": 34048, "ĠAdjust": 34049, "ilit": 34050, "ĠØ£ÙĪ": 34051, "Ġhearings": 34052, "Ġdefendant": 34053, "irsiniz": 34054, "Ġbask": 34055, "cja": 34056, "ľ¨": 34057, "Ġrifles": 34058, "Ġinstal": 34059, "ĠForgive": 34060, "pical": 34061, "ĠÐŀÑĩенÑĮ": 34062, "Ġpetites": 34063, "Ġhp": 34064, "Ġrenowned": 34065, "ĠInn": 34066, "Ġ주ìĦ¸ìļĶ": 34067, "Ġemphasized": 34068, "éĹ®é¢ĺ": 34069, "ĠìŀĪì£ł": 34070, "Ġê²ĥìľ¼ë¡ľ": 34071, "ãĤĨ": 34072, "Åĵ": 34073, "gili": 34074, "Dave": 34075, "Ġexhausting": 34076, "ÅĤug": 34077, "Ġschema": 34078, "μά": 34079, "cycl": 34080, "Ġautant": 34081, "Ġparcel": 34082, "Ġmateria": 34083, "ĠBerry": 34084, "ĠÑģами": 34085, "Ġextracted": 34086, "ĠSaying": 34087, "ismatic": 34088, "ĠпопÑĢоб": 34089, "Ġneuron": 34090, "graph": 34091, "ľë©´": 34092, "Ġenclosure": 34093, "ĠJohann": 34094, "Ġaftermath": 34095, "ÑĤоб": 34096, "Ġuży": 34097, "Ġsamp": 34098, "360": 34099, "ĠMei": 34100, "Ġtaco": 34101, "Ġreceptors": 34102, "Ġpunches": 34103, "ĠHoje": 34104, "ĠÙĩÙĨا": 34105, "=\"#": 34106, "ĠAngular": 34107, "Ġmusique": 34108, "Ġrol": 34109, "Ġñ": 34110, "sterreich": 34111, "Ġclam": 34112, "ĠTreasury": 34113, "chemical": 34114, "Ġapar": 34115, "Ġappend": 34116, "Ġforbid": 34117, "ĠHamburg": 34118, "аков": 34119, "Ġê¸Ī": 34120, "ilda": 34121, "Ġpreparations": 34122, "ĠmogÄħ": 34123, "Ġcamino": 34124, "Eric": 34125, "ĠBlind": 34126, "èĪĩ": 34127, "å¹´çļĦ": 34128, "ĠDiscovery": 34129, "ì¸ł": 34130, "çζ": 34131, "Ġinterpreter": 34132, "Ġbred": 34133, "ĠPsalm": 34134, "Ġdefended": 34135, "ìī¬": 34136, "ĠErfahr": 34137, "ĠPeach": 34138, "Ġmoons": 34139, "ĠOst": 34140, "Ġspécial": 34141, "Ġarriver": 34142, "ĠWis": 34143, "uci": 34144, "Ġrobotics": 34145, "IVE": 34146, "Ġsiege": 34147, "arla": 34148, "Ġseparates": 34149, "ĠTC": 34150, "íı°": 34151, "quisite": 34152, "Ġparentheses": 34153, "ике": 34154, "ç«Ļ": 34155, "Ġtrous": 34156, "建": 34157, "ĠÑģилÑĮ": 34158, "Ġbeers": 34159, "ĠплаÑĤ": 34160, "ãģĻãģĶãģĦ": 34161, "Ġsola": 34162, "Ġdès": 34163, "mingham": 34164, "ikte": 34165, "Ġoops": 34166, "Ġtwitch": 34167, "å°ĩ": 34168, "ÏĪ": 34169, "ĠShouldn": 34170, "uvre": 34171, "Ġleer": 34172, "criptions": 34173, "Ġeyeshadow": 34174, "ĠGuo": 34175, "ĠPowell": 34176, "Ġsupuesto": 34177, "Ġana": 34178, "rals": 34179, "ĠMontreal": 34180, "Ġsurfing": 34181, "ĠÐŁÐµÑĢв": 34182, "×ŀ×ķ": 34183, "Ġmilliseconds": 34184, "Ġsuburbs": 34185, "Ġplaneta": 34186, "ÑĥÑĪка": 34187, "hrlich": 34188, "ĠHY": 34189, "ĠسÛĴ": 34190, "ĠMM": 34191, "ĠEff": 34192, "åı¯æĦĽ": 34193, "ĠHS": 34194, "anson": 34195, "Ġì§ģìłij": 34196, "Ġsuo": 34197, "Ġdeploying": 34198, "Ġkunt": 34199, "tering": 34200, "Ġerect": 34201, "ìŀ¥ìĿ´": 34202, "ĠìĿĮìĭĿ": 34203, "Ġspecimen": 34204, "!...": 34205, "æĪij說": 34206, "Ġligne": 34207, "Ġkonst": 34208, "adequ": 34209, "Ġìĥģíĥľ": 34210, "Ġaccessed": 34211, "ĠPole": 34212, "kill": 34213, "Ġë²Ħë": 34214, "Ġauthenticity": 34215, "Ġappelle": 34216, "ulle": 34217, "Ġrevision": 34218, "Ġgoats": 34219, "гли": 34220, "Ġpau": 34221, "ĠRanger": 34222, "ĠImag": 34223, "author": 34224, "Ġeve": 34225, "ĠMessenger": 34226, "Ġnay": 34227, "Ġwholes": 34228, "ätte": 34229, "Ġonwards": 34230, "ĠDepois": 34231, "ĠíijľíĺĦ": 34232, "ĠSARS": 34233, "Ġwszystkich": 34234, "Ġdestru": 34235, "umbing": 34236, "Ġcompatibility": 34237, "Ġmisinformation": 34238, "odore": 34239, "ĠFavor": 34240, "eko": 34241, "ıĮ": 34242, "waukee": 34243, "ĠTeaching": 34244, "ĠKO": 34245, "Ġbetting": 34246, "Ġquests": 34247, "Ġvivre": 34248, "ĠмÑĥзÑĭ": 34249, "Ġsaga": 34250, "Ġswell": 34251, "Ġgehe": 34252, "æĢİ麼樣": 34253, "ĠоÑĢганиз": 34254, "Ġgide": 34255, "ĠGross": 34256, "Ġdalej": 34257, "Ġclaws": 34258, "á»Ļc": 34259, "Ġprejudice": 34260, "Ġinsign": 34261, "ihood": 34262, "Ġpled": 34263, "Ġdónde": 34264, "ĠPolitical": 34265, "Ġpremises": 34266, "undert": 34267, "عت": 34268, "onnen": 34269, "Ġespaço": 34270, "Ġfé": 34271, "ĠHarrison": 34272, "ĠCensus": 34273, "Ġcardio": 34274, "Ġdiy": 34275, "Ġmilieu": 34276, "Ġjournée": 34277, "ĠRelease": 34278, "NIE": 34279, "ĠMuk": 34280, "idée": 34281, "á»įi": 34282, "Ġiçinde": 34283, "ŀĻ": 34284, "Ġresonate": 34285, "Ġmoles": 34286, "ĠFlying": 34287, "ĠGloria": 34288, "ĠPastor": 34289, "ĠArena": 34290, "好ä¸į好": 34291, "NON": 34292, "олов": 34293, "ĠallÃŃ": 34294, "omat": 34295, "ìĸ´ëıĦ": 34296, "ĠcaracterÃŃst": 34297, "Ġdeclining": 34298, "ÑĸÑı": 34299, "anco": 34300, "ĠInform": 34301, "Ġbargain": 34302, "Ġbushes": 34303, "ĠNaturally": 34304, "Ġrechts": 34305, "ĠTensor": 34306, "ĠPatricia": 34307, "Ġprincipio": 34308, "ĠMumbai": 34309, "Ġwomb": 34310, "Ġnostra": 34311, "Ġdilemma": 34312, "Ġirgendwann": 34313, "Ġ1964": 34314, "ĠenergÃŃa": 34315, "ĠнаÑĢ": 34316, "Ġsegregation": 34317, "ĠAthlet": 34318, "Ġ»,": 34319, "Ġyeni": 34320, "ĠSeit": 34321, "Ġvenom": 34322, "Ġdakika": 34323, "ĠëıĮë": 34324, "ĠÃīl": 34325, "Ġfus": 34326, "ĠMog": 34327, "¦½ëĭĪëĭ¤": 34328, "Ġremar": 34329, "ĠTeddy": 34330, "Ġbreasts": 34331, "icans": 34332, "æĶ¶çľĭ": 34333, "kap": 34334, "ĠhÆ¡n": 34335, "ĠJP": 34336, "ãĥ³ãĤ¿": 34337, "Ġresurrect": 34338, "ĠìĿ¸ë": 34339, "herical": 34340, "Ġfotograf": 34341, "ĠJosé": 34342, "Ġlivelihood": 34343, "Ġbibli": 34344, "teri": 34345, "Ġvorstellen": 34346, "ĠAAA": 34347, "Ġassessing": 34348, "YA": 34349, "Ġsplend": 34350, "Ġexcav": 34351, "Ġbaptism": 34352, "yll": 34353, "wow": 34354, "Mac": 34355, "Ġplastics": 34356, "teokbokki": 34357, "Ġintéressant": 34358, "Ġcommanded": 34359, "Ġfamously": 34360, "ĠÐĺли": 34361, "ĠManuel": 34362, "Ġsouthwest": 34363, "Ġdeformation": 34364, "ÃŃculo": 34365, "ĠнаÑħодиÑĤÑģÑı": 34366, "ĠPatter": 34367, "degree": 34368, "ĠczÄĻsto": 34369, "\"-": 34370, "Ġìħĭ": 34371, "Ġmanger": 34372, "ĠTrustee": 34373, "Ģ리": 34374, "Ġpuntos": 34375, "ivable": 34376, "Ġvolatile": 34377, "ĠëĬIJ": 34378, "Ġinstability": 34379, "Ġciel": 34380, "ciÄħ": 34381, "Ġpurity": 34382, "ноÑģÑĤ": 34383, "Sil": 34384, "edar": 34385, "åύ": 34386, "NOUNCER": 34387, "Ġspelled": 34388, "GER": 34389, "Ġsanctuary": 34390, "Ġaccelerating": 34391, "Ġscout": 34392, "ĠпÑĢев": 34393, "fahren": 34394, "ãģĵãģ¡ãĤī": 34395, "ĠëĤĺìĺ¨": 34396, "ĠpoczÄħt": 34397, "ĠMeu": 34398, "kaar": 34399, "³´ê³ł": 34400, "akra": 34401, "Down": 34402, "ĠÃĦr": 34403, "ĠElite": 34404, "Ġallons": 34405, "Ġmayonnaise": 34406, "ĠSustain": 34407, "prisingly": 34408, "Ġsupervis": 34409, "Ġê·¸ëłĩì£ł": 34410, "Ġunemployed": 34411, "Ġfreshly": 34412, "Ġ×ŀ×¢": 34413, "ĠDh": 34414, "Ġtackling": 34415, "Ġogr": 34416, "Ġì´Īë": 34417, "ãĤĪãĤį": 34418, "Ġloft": 34419, "arah": 34420, "ĠAirl": 34421, "ĠDir": 34422, "ĠÐľÐ¾Ð¶Ð½Ð¾": 34423, "Ġbooking": 34424, "ĠCRA": 34425, "Ġhttps": 34426, "Ġchoke": 34427, "Ġgown": 34428, "Ġnoite": 34429, "Ġzac": 34430, "istol": 34431, "Ġsecre": 34432, "Ġresembles": 34433, "Ġcuad": 34434, "ìĤ¬ê°Ģ": 34435, "show": 34436, "Ġblanc": 34437, "Ġagu": 34438, "ĠPrint": 34439, "asted": 34440, "ĠWeather": 34441, "ipl": 34442, "Ġobscure": 34443, "Ġconte": 34444, "oughs": 34445, ");": 34446, "ĠDame": 34447, "ä¸Ģ缴": 34448, "Ġclarification": 34449, "Ġintimacy": 34450, "Ġuphold": 34451, "ĠMirror": 34452, "Ġwagon": 34453, "xide": 34454, "Ġclog": 34455, "apper": 34456, "ĠImmediately": 34457, "úde": 34458, "Ġtouchdown": 34459, "Ġrooft": 34460, "аÑĪа": 34461, "Ġçıkt": 34462, "Ġlaisser": 34463, "ĠUnreal": 34464, "ensitive": 34465, "Ġ123": 34466, "Ġplaster": 34467, "Ġducks": 34468, "Ġetme": 34469, "Ġbishop": 34470, "brevi": 34471, "Ġbic": 34472, "ä¸ĭåİ»": 34473, "Ġruntime": 34474, "Ġambitions": 34475, "маÑĤ": 34476, "ĠWein": 34477, "ĠMari": 34478, "ĠíĬ¸ë": 34479, "Ġresolver": 34480, "ĠngÃły": 34481, "ĠRise": 34482, "ãĤĪãģĨãģ«": 34483, "ĠCrus": 34484, "Ġmerchandise": 34485, "Ġeli": 34486, "Ġstatewide": 34487, "Ġowl": 34488, "éģł": 34489, "æĶ¹": 34490, "Ġtwisting": 34491, "Ġcontaminated": 34492, "ĠCommerce": 34493, "hythm": 34494, "ĠÃĪ": 34495, "Ġìĭ¤ë": 34496, "Ġmusste": 34497, "uir": 34498, "Ġsums": 34499, "ĠSomewhere": 34500, "ãĥİ": 34501, "Ġkami": 34502, "Ġaired": 34503, "ĠANDREW": 34504, "Ġêº": 34505, "Ġviendo": 34506, "Ġantibody": 34507, "Ġabsolument": 34508, "Ġprotesters": 34509, "ĠQuébec": 34510, "stadt": 34511, "Shaun": 34512, "Ġchambers": 34513, "ĠWear": 34514, "ĠEffects": 34515, "Ġhazards": 34516, "Ġnei": 34517, "Ġcorazón": 34518, "Ġá¼": 34519, "ĠSG": 34520, "Ķ©": 34521, "ĠìĹŃìĭľ": 34522, "Ġcomfy": 34523, "ĠCody": 34524, "Ġpensando": 34525, "Ġganska": 34526, "ĠAcross": 34527, "öllig": 34528, "abyte": 34529, "Ġwedge": 34530, "Ġkalian": 34531, "Ġsigue": 34532, "endes": 34533, "ĠGroÃŁ": 34534, "Ġutiliser": 34535, "Ġflown": 34536, "аниÑİ": 34537, "Ġlevar": 34538, "restrial": 34539, "Ġillustrations": 34540, "Ġaslında": 34541, "BLEEP": 34542, "ĠдоÑģÑĤ": 34543, "Ġturret": 34544, "Ġsuitcase": 34545, "ziÄĻki": 34546, "Ġsketches": 34547, "Ġacred": 34548, "ĠRei": 34549, "Ġtsun": 34550, "ĠSag": 34551, "Ġthirds": 34552, "ĠKIRBY": 34553, "rai": 34554, "Ġhumanos": 34555, "Ġrecommends": 34556, "Ġextraordinarily": 34557, "Ġcommencement": 34558, "KN": 34559, "opez": 34560, "Ġ×ijש": 34561, "Ġlethal": 34562, "ĠEstamos": 34563, "Ġinspector": 34564, "ĠSeok": 34565, "eun": 34566, "Ġoffshore": 34567, "Ġgettin": 34568, "years": 34569, "ĠSilence": 34570, "ĠNatur": 34571, "upun": 34572, "Ġtrzy": 34573, "Ġnoget": 34574, "Ġhamburger": 34575, "ĠPraise": 34576, "énd": 34577, "Ġ1971": 34578, "ylie": 34579, "krit": 34580, "ĠìĥĿê°ģìĿ´": 34581, "çļ®": 34582, "Ġmomentos": 34583, "Ġesté": 34584, "Ġdissemin": 34585, "Ġgigs": 34586, "Ġdesaf": 34587, "Ġavis": 34588, "ĠZoo": 34589, "ĠìķĬìĿĢ": 34590, "häng": 34591, "åı¥": 34592, "hake": 34593, "ĠBism": 34594, "Ġrethink": 34595, "ĠMalcolm": 34596, "Ġidentifies": 34597, "lower": 34598, "ixel": 34599, "ĠtvÃ¥": 34600, "ked": 34601, "ierz": 34602, "Ġöffentlich": 34603, "Ġproclaim": 34604, "soon": 34605, "lol": 34606, "Ġloi": 34607, "Ġbitten": 34608, "rollo": 34609, "Ġsermon": 34610, "Ġesqu": 34611, "Ġjackets": 34612, "Ġgráfic": 34613, "ĠпоказÑĭв": 34614, "Ġcabeza": 34615, "chodzi": 34616, "Ġpelvis": 34617, "Ġnostalgia": 34618, "Ġbrew": 34619, "Ġshortcuts": 34620, "ĠAdemás": 34621, "Ġsuperficial": 34622, "åħ©åĢĭ": 34623, "Ġboca": 34624, "ĠæĪijæĺ¯": 34625, "imentos": 34626, "åĽłä¸º": 34627, "Ġsprouts": 34628, "é£Ľ": 34629, "ĠJonas": 34630, "ĠFlorence": 34631, "static": 34632, "daughter": 34633, "*)": 34634, "ÅĤby": 34635, "fashion": 34636, "ĠGinger": 34637, "Ġ매ë": 34638, "Ġhustle": 34639, "utos": 34640, "ĠÑĤÑıж": 34641, "ĠLös": 34642, "ש×Ļ×Ŀ": 34643, "anych": 34644, "tuber": 34645, "Ġtidy": 34646, "Ġfrontal": 34647, "Ġwhiskey": 34648, "Ġhumid": 34649, "ĠÎŁ": 34650, "Ġridge": 34651, "Ġmarin": 34652, "Ġbientôt": 34653, "ĠCarrie": 34654, "chw": 34655, "Ġtahun": 34656, "ĠErgeb": 34657, "FR": 34658, "Ġìłķë¶Ģ": 34659, "ĠSoldier": 34660, "Ġenlightenment": 34661, "Ġexamining": 34662, "ĠNotre": 34663, "Ġeram": 34664, "ĠSunny": 34665, "Ġlayered": 34666, "ĠDazu": 34667, "rades": 34668, "好åIJĥ": 34669, "ĠнаÑĪей": 34670, "Ġtimber": 34671, "Ġmanners": 34672, "ĠBirmingham": 34673, "Ġminiature": 34674, "ometers": 34675, "Ġfiller": 34676, "ĠRip": 34677, "ĠKomb": 34678, "owner": 34679, "ì¿": 34680, "idian": 34681, "Ġdemás": 34682, "ĠÙĪØª": 34683, "Ġprecautions": 34684, "Ġgoverno": 34685, "zelf": 34686, "ĠComplete": 34687, "å¸ĥ": 34688, "ĠPhantom": 34689, "ãģ¾ãģļ": 34690, "Ġнез": 34691, "ĠкаÑĢÑĤ": 34692, "ĠAntwort": 34693, "ĠPfizer": 34694, "ĠFranco": 34695, "ĠwÅĤ": 34696, "Ġfrig": 34697, "esper": 34698, "Ġkale": 34699, "Ġfilmmaker": 34700, "Ġkurt": 34701, "Ġinvalid": 34702, "å±Ģ": 34703, "arella": 34704, "Äĥng": 34705, "ramento": 34706, "Ġnutritional": 34707, "Ġdictators": 34708, "Ġafin": 34709, "Ġfuzzy": 34710, "ĠGina": 34711, "ót": 34712, "ĠExtremadura": 34713, "Ġdemonstrations": 34714, "ĠMontgomery": 34715, "íķ´ìĦ¤": 34716, "ĠGandhi": 34717, "ãĥĿ": 34718, "ç½®": 34719, "Ġreunion": 34720, "ĠjakiÅĽ": 34721, "ĠZug": 34722, "OUGH": 34723, "lifting": 34724, "Ġà²": 34725, "á¹Ľá¹£": 34726, "eb": 34727, "ĠWOW": 34728, "ĠShiva": 34729, "ometry": 34730, "Ġwildly": 34731, "Ġtended": 34732, "Ġmegap": 34733, "ì²ĺ": 34734, "Ġnause": 34735, "Ġgerek": 34736, "ãĥĭ": 34737, "ĠMarcel": 34738, "Ġneste": 34739, "خر": 34740, "Ġfeh": 34741, "åĨħ": 34742, "suspenseful": 34743, "ĠWrestle": 34744, "ĠPalestinians": 34745, "ĠGORD": 34746, "iyet": 34747, "ĠÑĢади": 34748, "Ġversuchen": 34749, "Ġtransistor": 34750, "ĠÐŁÑĢоÑģÑĤо": 34751, "ĠпонÑĢав": 34752, "Ġrhyme": 34753, "ĠVermont": 34754, "platz": 34755, "è®°": 34756, "ĠÄ°ÅŁte": 34757, "ĠHag": 34758, "ĠÐĺм": 34759, "ĠÑĢаÑģÑģказ": 34760, "Ġmetros": 34761, "ĠInfinity": 34762, "wolf": 34763, "ibal": 34764, "ftig": 34765, "ĠÚĨ": 34766, "Ġíĺ¹ìĭľ": 34767, "Ġoggi": 34768, "Ġdisposit": 34769, "ĠпÑĢил": 34770, "ĠвÑĭпол": 34771, "Ġthôi": 34772, "ĠKENN": 34773, "Ġhanding": 34774, "actus": 34775, "Ġtacos": 34776, "Ġformerly": 34777, "ĠCorinthians": 34778, "ãģ«ãģ¯": 34779, "ÑĨÑĸÑĹ": 34780, "Ġpadre": 34781, "Ġcongregation": 34782, "æij": 34783, "fert": 34784, "Ġsubir": 34785, "aiser": 34786, "qua": 34787, "araoh": 34788, "ĠCurry": 34789, "ĠìķĬëĬĶ": 34790, "елÑİ": 34791, "Ġfuss": 34792, "Ġbooty": 34793, "Ġlows": 34794, "Ġhommes": 34795, "ĠMH": 34796, "ĠDisneyland": 34797, "went": 34798, "Ġresidue": 34799, "Ġbeeping": 34800, "è¼ķ": 34801, "ätta": 34802, "Ġmould": 34803, "ĠProjekt": 34804, "stalk": 34805, "Ġartifact": 34806, "ĠAntrag": 34807, "ĠAMD": 34808, "ĠCrypt": 34809, "Ġë©Ķ": 34810, "ĠFelipe": 34811, "ĠCOB": 34812, "elu": 34813, "Ġselfies": 34814, "ĠSanti": 34815, "chutz": 34816, "ĠУкÑĢаÑĹ": 34817, "gesamt": 34818, "Ġflock": 34819, "jaz": 34820, "plain": 34821, "Ġwrinkles": 34822, "Ġreais": 34823, "Ġpaljon": 34824, "Ġempowerment": 34825, "Ġattendees": 34826, "ppa": 34827, "Ġneden": 34828, "онÑĭ": 34829, "Ġtimeframe": 34830, "ĠCherry": 34831, "Ġidée": 34832, "Ġgag": 34833, "Ġdonkey": 34834, "Ġông": 34835, "ĠHare": 34836, "éļĽ": 34837, "ĠKara": 34838, "Ġacompan": 34839, "places": 34840, "imientos": 34841, "ĠHamm": 34842, "би": 34843, "uben": 34844, "iliyor": 34845, "Ġthirst": 34846, "Ġkry": 34847, "ĠGeorgetown": 34848, "׳×Ķ": 34849, "Ġorch": 34850, "Ġheartbeat": 34851, "Ġtransformations": 34852, "estones": 34853, "ĠKH": 34854, "Ġcartoons": 34855, "Ġanci": 34856, "Ġworthless": 34857, "Ġtailored": 34858, "pu": 34859, "Americans": 34860, "Ġpiles": 34861, "ĠMonkey": 34862, "Ġbasin": 34863, "ĠTemper": 34864, "ĠPaint": 34865, "Ġpunching": 34866, "Ġbaik": 34867, "ĠOakland": 34868, "vre": 34869, "ÅŁallah": 34870, "ydd": 34871, "Ġcasually": 34872, "odu": 34873, "Ġcoded": 34874, "ĠNorwegian": 34875, "ĠVince": 34876, "Ġpremature": 34877, "ĠPromise": 34878, "екÑģÑĤ": 34879, "Ġdevastated": 34880, "ĠPremium": 34881, "ĠParam": 34882, "ĠÃĸyle": 34883, "umuz": 34884, "PO": 34885, "rators": 34886, "Ġlamps": 34887, "Ġterritorial": 34888, "Ġbackbone": 34889, "listed": 34890, "DY": 34891, "ĠاÙĦر": 34892, "Ġpursued": 34893, "ĠCommons": 34894, "Ġ곡": 34895, "locks": 34896, "edor": 34897, "Ġconceived": 34898, "gere": 34899, "Ġdisappearing": 34900, "ĠSull": 34901, "ĠìŰë": 34902, "Ġhoffe": 34903, "Ġdetox": 34904, "íĶĮ": 34905, "Ġretir": 34906, "ĠëģĿëĤ": 34907, "Ġpergunta": 34908, "ĠBOY": 34909, "ç²¾": 34910, "Ġpenn": 34911, "æĿ¥äºĨ": 34912, "hés": 34913, "hon": 34914, "Ġcatastrophic": 34915, "Ġaust": 34916, "Ġtorso": 34917, "Ġìĸ´ëĬIJ": 34918, "ĠìĤ¬ëŀĮëĵ¤ìĿ´": 34919, "Ġmarvelous": 34920, "ĠHarley": 34921, "achine": 34922, "Ġtiế": 34923, "itto": 34924, "ĠIÃŃm": 34925, "ylon": 34926, "Ġshutdown": 34927, ".''": 34928, "Ġapologies": 34929, "ĠCommunication": 34930, "ĠговоÑĢÑİ": 34931, "ãģĤãĥ¼": 34932, "âĦ¢": 34933, "ÃŃveis": 34934, "acun": 34935, "Ġretaining": 34936, "Ġcontradiction": 34937, "ĠADAM": 34938, "COM": 34939, "Bryan": 34940, "ĠMonsieur": 34941, "Ġadapting": 34942, "ШÐIJ": 34943, "ĠScr": 34944, "ändert": 34945, "Ġplaus": 34946, "ä»Ĭ天çļĦ": 34947, "Ġonset": 34948, "Ġassistants": 34949, "Ġvalves": 34950, "Ġscatter": 34951, "ĠRust": 34952, "awia": 34953, "Ġreadiness": 34954, "Ġpais": 34955, "Ġbible": 34956, "Ġambiente": 34957, "ĠамеÑĢик": 34958, "Ġuncond": 34959, "Ġkalk": 34960, "åĬ¨": 34961, "Ġmoc": 34962, "unn": 34963, "Ġactu": 34964, "Ġhumming": 34965, "issimo": 34966, "ĠPatrol": 34967, "gow": 34968, "ãĥ¤": 34969, "ĠTHEY": 34970, "ĠBoden": 34971, "ĠBie": 34972, "Ġreel": 34973, "ĠÑĥÑģлов": 34974, "Ġendeavor": 34975, "ĠPeriod": 34976, "ustomed": 34977, "mals": 34978, "alon": 34979, "Box": 34980, "ĠÏĥαÏĤ": 34981, "Ġomdat": 34982, "Ġaltre": 34983, "ĠHeh": 34984, "kad": 34985, "Ġprotector": 34986, "Ġdominance": 34987, "odynamic": 34988, "Ġcommunicated": 34989, "kö": 34990, "Ġpredecessor": 34991, "ĠLuk": 34992, "ĠFlower": 34993, "Ġãģ©": 34994, "poque": 34995, "ÑĤиÑĢов": 34996, "Ġretrospect": 34997, "Ġdecisive": 34998, "Ġexempel": 34999, "{\\": 35000, "ĠRück": 35001, "rite": 35002, "ĠZeus": 35003, "Ġcalorie": 35004, "Ġattractions": 35005, "ĠHinter": 35006, "Ġuhm": 35007, "ĠíĮIJ": 35008, "Ġrulers": 35009, "Ġdiscouraged": 35010, "Ġacontecer": 35011, "Ġaccents": 35012, "ĠOptim": 35013, "ĠAlg": 35014, "kids": 35015, "2021": 35016, "ĠLindsay": 35017, "Ġfilmmakers": 35018, "prowad": 35019, "Ġterug": 35020, "ëĭ´": 35021, "ĠSommer": 35022, "2018": 35023, "Ġborrowing": 35024, "ĠTransfer": 35025, "ноп": 35026, "arias": 35027, "Ġheadphone": 35028, "ì¼ľ": 35029, "Ġtranslating": 35030, "Ġaufge": 35031, "à®ªà®Ł": 35032, "weis": 35033, "avant": 35034, "paid": 35035, "baby": 35036, "Ġtoughest": 35037, "Ġrepeats": 35038, "ĠTeresa": 35039, "Lord": 35040, "Ġacabar": 35041, "ĠRide": 35042, "dir": 35043, "Ġleng": 35044, "Ġdwa": 35045, "Ġheadaches": 35046, "Ġnữa": 35047, "ĠнаÑģÑĤоÑıÑī": 35048, "Ġboils": 35049, "Ġlonging": 35050, "rias": 35051, "ório": 35052, "ĠParadise": 35053, "ĠSeñor": 35054, "erdem": 35055, "Ġreinst": 35056, "Ġsalaries": 35057, "Ġinsecurity": 35058, "ÅĤoÅĽci": 35059, "ĠабÑģолÑİÑĤно": 35060, "inken": 35061, "ĠEddy": 35062, "udos": 35063, "Ġdummy": 35064, "Ðļак": 35065, "six": 35066, "Ġinbox": 35067, "ẩ": 35068, "People": 35069, "á»ĵng": 35070, "Ġorganizers": 35071, "find": 35072, "Ġül": 35073, "ĠCOM": 35074, "ża": 35075, "weile": 35076, "Commentary": 35077, "íĬ¸ë¥¼": 35078, "ĠMittel": 35079, "kus": 35080, "èĽĭ": 35081, "न": 35082, "iral": 35083, "Ġgarment": 35084, "ικά": 35085, "Ġstool": 35086, "payers": 35087, "Ġshimmer": 35088, "ĠOllie": 35089, "ĠJeżeli": 35090, "è¿ĺæľī": 35091, "Ġ1977": 35092, "Ġjeux": 35093, "Ġextinct": 35094, "ĠTransportation": 35095, "ĠMaker": 35096, "Ġjohn": 35097, "Ġrichest": 35098, "Ġtraumat": 35099, "Ġliegen": 35100, "´ë¥¼": 35101, "è¿ĻéĩĮ": 35102, "Ġunrest": 35103, "ĠStraw": 35104, "æĭľæĭľ": 35105, "Ġcoma": 35106, "ĠKristen": 35107, "ĠÐļонеÑĩно": 35108, "ĠBryce": 35109, "ĠÑıкÑĸ": 35110, "Ġpearls": 35111, "ĠпонимаÑİ": 35112, "Ġadditions": 35113, "Ġasympt": 35114, "ĠменÑĮÑĪе": 35115, "Ġscans": 35116, "Child": 35117, "ĠHide": 35118, "кÑĥÑİ": 35119, "etas": 35120, "Ġdank": 35121, "Ġpleas": 35122, "Ġessays": 35123, "Ġjets": 35124, "åħĴ": 35125, "Ġвед": 35126, "Ġpositives": 35127, "hof": 35128, "-)": 35129, "zzo": 35130, "Ġstarters": 35131, "Ġsmiled": 35132, "Ġ1944": 35133, "quiera": 35134, "Ġrok": 35135, "Ġpuesto": 35136, "Nico": 35137, "Ġsimulations": 35138, "Ġà¶": 35139, "Ġintrigued": 35140, "ĠOverwatch": 35141, "åĸĤ": 35142, "sigh": 35143, "bai": 35144, "Ġë§IJê³ł": 35145, "idé": 35146, "Ġcrabs": 35147, "áºŃp": 35148, "ĠIraqi": 35149, "ìĿ´ë¥¼": 35150, "ÑĤÑı": 35151, "ĠSophia": 35152, "ĠDNS": 35153, "Ġönemli": 35154, "ĠLuo": 35155, "Ŀ¤": 35156, "ĠCounsel": 35157, "ligen": 35158, "анÑĮÑĪе": 35159, "Ġtrumpet": 35160, "Ġdapat": 35161, "ĠJM": 35162, "ĠEVERY": 35163, "Ġå°įä¸įå°į": 35164, "夢": 35165, "ĠLayer": 35166, "Ġcô": 35167, "нал": 35168, "ĠJoo": 35169, "ĠHack": 35170, "Ġsunt": 35171, "ĠLeonard": 35172, "ĠFirebase": 35173, "änger": 35174, "Ġexploding": 35175, "voy": 35176, "Ġì¦IJ": 35177, "ĠÑģеÑĢÑĮ": 35178, "Ġseverity": 35179, "Ġbestimm": 35180, "çµIJæŀľ": 35181, "Ġtiring": 35182, "Ġprocurement": 35183, "Ġdiplomacy": 35184, "Ġdecorative": 35185, "ĠÙĬا": 35186, "Ġpenetration": 35187, "Õ«": 35188, "Ġoutright": 35189, "ENE": 35190, "ĠUni": 35191, "odles": 35192, "Ġzeros": 35193, "Ġdelightful": 35194, "jm": 35195, "Ġdopo": 35196, "没äºĭ": 35197, "Ġpositivity": 35198, "ĠVISTA": 35199, "ĠResource": 35200, "íĥĢë": 35201, "ÑĪие": 35202, "Carl": 35203, "Ġpiping": 35204, "Ġchopping": 35205, "ĠGanze": 35206, "üss": 35207, "ĠAo": 35208, "Ġshattered": 35209, "ĠDetective": 35210, "Ġundoubtedly": 35211, "Ġhalluc": 35212, "Ġench": 35213, "ÑĭÑĩно": 35214, "ÑĥлÑıÑĢ": 35215, "isesti": 35216, "Ġpedals": 35217, "Ġdurum": 35218, "¤íĶ": 35219, "laimer": 35220, "Ġpropre": 35221, "Cu": 35222, "Ġtranslator": 35223, "ĠcaÅĤ": 35224, "Ġ그걸": 35225, "ĠcaÅĤy": 35226, "UA": 35227, "Ġrevised": 35228, "Ġподоб": 35229, "ĠArticle": 35230, "ĠHaiti": 35231, "ĠÃĵ": 35232, "ĠCtrl": 35233, "Ġrozm": 35234, "lait": 35235, "Ġletzte": 35236, "ispering": 35237, "display": 35238, "Ġaluminium": 35239, "Ġpalabras": 35240, "Ġconocer": 35241, "Ġzitten": 35242, "Ġdirig": 35243, "åıªæľī": 35244, "Ġbrainstorm": 35245, "Ġwifi": 35246, "ĠParticip": 35247, "Ġviewpoint": 35248, "ĠQuan": 35249, "Ġhierarch": 35250, "Welcome": 35251, "対": 35252, "Ġoffen": 35253, "ĠRecovery": 35254, "gano": 35255, "Would": 35256, "Ġrepro": 35257, "Ġperceptions": 35258, "Ġdemasi": 35259, "ĠBangladesh": 35260, "ĠIncredible": 35261, "Ġletzt": 35262, "Ġbehaving": 35263, "Ġastonishing": 35264, "ĠâĨ": 35265, "ĠëĤ¨ìŀIJ": 35266, "èµ°äºĨ": 35267, "ãĥĶ": 35268, "ĠGORDON": 35269, "CAR": 35270, "?!\"": 35271, "ĠPrest": 35272, "Ġë§ŀìķĦìļĶ": 35273, "Ġtand": 35274, "Ġlash": 35275, "çĬ": 35276, "ificant": 35277, "Ġintoler": 35278, "ĠгеÑĢо": 35279, "Ġteu": 35280, "aso": 35281, "ĠÑģовеÑĤ": 35282, "Ġtravelers": 35283, "ĠSynd": 35284, "ĠвеÑĢÑģ": 35285, "Fonda": 35286, "adı": 35287, "Ġtranscription": 35288, "Ġtitanium": 35289, "Ġtwists": 35290, "Ġgearbox": 35291, "ensation": 35292, "fat": 35293, "Coll": 35294, "ĠCommonwealth": 35295, "zon": 35296, "ĠPolizei": 35297, "ĠAPPLAUSE": 35298, "fry": 35299, "ĠJuda": 35300, "esteem": 35301, "Ġsock": 35302, "ĠJugend": 35303, "ĠкÑģÑĤаÑĤи": 35304, "ĠDro": 35305, "Ġprochaine": 35306, "ãĥ¼ãĥ«": 35307, "Ġliksom": 35308, "ĠEnergie": 35309, "ĠMarina": 35310, "Ġ230": 35311, "Ġê°ĢìĦľ": 35312, "umping": 35313, "Ġlone": 35314, "ç´ļ": 35315, "Ġfonts": 35316, "Ġbusinessman": 35317, "Ġply": 35318, "Ġdoe": 35319, "grid": 35320, "ĠMilwaukee": 35321, "ĠEden": 35322, "!\".": 35323, "ĠÛĮÛģ": 35324, "ogens": 35325, "Ġteaser": 35326, "Ġquién": 35327, "Ġincentiv": 35328, "govern": 35329, "Ġchildcare": 35330, "Ġsneakers": 35331, "Ġimprisoned": 35332, "®": 35333, "иÑĤеÑģÑĮ": 35334, "anbul": 35335, "Ġregain": 35336, "Ġtranquil": 35337, "Redner": 35338, "鼨": 35339, "IFA": 35340, "Ġideological": 35341, "ĠmayorÃŃa": 35342, "Ġbureau": 35343, "eterm": 35344, "ĠDID": 35345, "ìĬ·": 35346, "Ġwaving": 35347, "Ġbeb": 35348, "Ġár": 35349, "Ġкв": 35350, "Ġenvoy": 35351, "anut": 35352, "икÑĥ": 35353, "ĠEnvironment": 35354, "ĠAssass": 35355, "ãĤĵãģ§": 35356, "ĠBread": 35357, "ĠТÑĥÑĤ": 35358, "Ġstaircase": 35359, "ĠDisease": 35360, "Ġaucun": 35361, "ĠëĭĪ": 35362, "Ġconfrontation": 35363, "Ġ1941": 35364, "Ġirony": 35365, "Ġworsh": 35366, "ãĤĮãĤĭ": 35367, "Ġfick": 35368, "ĠNaomi": 35369, "Ġbackside": 35370, "ieux": 35371, "Kap": 35372, "Ġvedere": 35373, "Ġlengthy": 35374, "Ġbreaker": 35375, "ĠRolle": 35376, "Ġpredator": 35377, "Ġnossos": 35378, "Ġadvertise": 35379, "è³ĩ": 35380, "ÑĢоде": 35381, "Rednerwechsel": 35382, "reten": 35383, "Ġcollectors": 35384, "ıģımız": 35385, "Ġtrig": 35386, "Ġaxes": 35387, "inters": 35388, "Ġpenalties": 35389, "ĠOsman": 35390, "ĠJenna": 35391, "Ġflakes": 35392, "Ġtrainers": 35393, "Ġstunned": 35394, "ĠScroll": 35395, "ĠPip": 35396, "ĠнаÑģÑĤ": 35397, "ĠnhÃł": 35398, "ĠSmack": 35399, "ẫn": 35400, "ratos": 35401, "ĠÑĢабоÑĤÑĭ": 35402, "Ġucz": 35403, "ĠLemon": 35404, "ĠSind": 35405, "Ġpsychic": 35406, "ĠAbg": 35407, "Ġmammals": 35408, "Ġimmersive": 35409, "Ġbots": 35410, "Ġverschiedene": 35411, "Ġgeral": 35412, "Ġfollower": 35413, "Ġä»ĸ": 35414, "Ġseguridad": 35415, "Ġimmersed": 35416, "feito": 35417, "cross": 35418, "Ġöld": 35419, "íĥĦ": 35420, "Ġãģĵãģ®": 35421, "Ġ×Ķ×Ļ×IJ": 35422, "ĠJian": 35423, "Ġbiliyor": 35424, "area": 35425, "Ġkaf": 35426, "Ġgodt": 35427, "çĽ¸ä¿¡": 35428, "Ġë°©ìĨ¡": 35429, "Ġdetriment": 35430, "æ¥ļ": 35431, "Ñĸл": 35432, "ĠÄijâu": 35433, "Ġchloride": 35434, "øre": 35435, "lei": 35436, "Ġmonte": 35437, "Ġdifférentes": 35438, "à¯ģ.": 35439, "Ġcaregivers": 35440, "Ġinadequ": 35441, "Ġfarewell": 35442, "ĠÑĤипа": 35443, "ontec": 35444, "ĠEph": 35445, "HHH": 35446, "ĠTodos": 35447, "ĠСШÐIJ": 35448, "Ġtrov": 35449, "Ġlige": 35450, "Ġcông": 35451, "ĠCiv": 35452, "Ġcapaz": 35453, "ĠVallahi": 35454, "Ġqueste": 35455, "Ġreplica": 35456, "سب": 35457, "zna": 35458, "ĠÑģлÑĥж": 35459, "ĠPT": 35460, "wave": 35461, "ieni": 35462, "Ġrelied": 35463, "develop": 35464, "Ġdeme": 35465, "ĠAman": 35466, "Ġ[...]": 35467, "Ġcompliments": 35468, "uais": 35469, "ĠíĮ¨": 35470, "Ġsmelling": 35471, "Ġdadurch": 35472, "ÙĪØª": 35473, "Ġoranges": 35474, "Ġлай": 35475, "Ġstabilization": 35476, "åĢį": 35477, "ãĤĮãģŁ": 35478, "楽": 35479, "Ġappliances": 35480, "Ġhm": 35481, "ĥIJë©´": 35482, "odynamics": 35483, "ĠciÄĻ": 35484, "ĠCott": 35485, "MON": 35486, "ĠMang": 35487, "æĶ¯æĮģ": 35488, "Ġallerdings": 35489, "ική": 35490, "shots": 35491, "Ġts": 35492, "ĠGör": 35493, "ĠCHAR": 35494, "Ġ:(": 35495, "Ġwrath": 35496, "Ġfique": 35497, "Ġführen": 35498, "Ġtestament": 35499, "Ġ^^": 35500, "á¹Ľá¹£á¹ĩa": 35501, "ALD": 35502, "Ġtexto": 35503, "ĠDogs": 35504, "Ġsib": 35505, "Ġpathetic": 35506, "ocks": 35507, "Ġradically": 35508, "ĠMORE": 35509, "ĠJAMES": 35510, "Ġingl": 35511, "ĠTechnical": 35512, "Ġporch": 35513, "ĠUT": 35514, "ĠобÑıзаÑĤелÑĮно": 35515, "Ġrenewal": 35516, "Ġaesthetics": 35517, "ikum": 35518, "Ġbeverage": 35519, "dern": 35520, "Ġpredictive": 35521, "Ġchuy": 35522, "ĠRegarding": 35523, "ĠForward": 35524, "ĠÙĪÙĦ": 35525, "Ġcontextual": 35526, "Ġdwarf": 35527, "Ġprehe": 35528, "Ġgoverned": 35529, "ħĦ": 35530, "Ġtrabalhar": 35531, "Ġnegócio": 35532, "ĠболÑĮÑĪой": 35533, "еÑĩаÑĤ": 35534, "ĠдÑĥÑħ": 35535, "Ġfloods": 35536, "Ġbowling": 35537, "ĠOB": 35538, "ĠHär": 35539, "Ġgrading": 35540, "주ëĬĶ": 35541, "Ġgars": 35542, "dling": 35543, "Ġrak": 35544, "ëĪ": 35545, "creat": 35546, "ĠÑīе": 35547, "Ġneighbours": 35548, "food": 35549, "Query": 35550, "Ġheroin": 35551, "iceps": 35552, "ĠKinda": 35553, "NET": 35554, "Ġmari": 35555, "Ġimitate": 35556, "Ġachter": 35557, "Ġsettlements": 35558, "rare": 35559, "cciones": 35560, "Ġëĵľ": 35561, "Ġfik": 35562, "itung": 35563, "ĠмакÑģим": 35564, "Ġelf": 35565, "Ġdalla": 35566, "ĠPolsce": 35567, "ĠPul": 35568, "ЧÑĤо": 35569, "ĠMorgen": 35570, "ØŃÙħ": 35571, "Ġsupremacy": 35572, "Ġkys": 35573, "ĠHurricane": 35574, "ĠGTA": 35575, "ĠFeh": 35576, "Ġfinalmente": 35577, "mund": 35578, "ĠKrie": 35579, "époque": 35580, "ĠTucker": 35581, "ITT": 35582, "Ġlur": 35583, "Ġdipping": 35584, "äv": 35585, "Ġeerste": 35586, "ĠFlint": 35587, "bildung": 35588, "ูà¹ī": 35589, "Ġtoim": 35590, "Ġpracy": 35591, "Ġtransforms": 35592, "Ġspeeding": 35593, "Ġpresenter": 35594, "Ġfellows": 35595, "filled": 35596, "ieza": 35597, "Ġadvising": 35598, "ĠInterview": 35599, "игÑĢ": 35600, "wehr": 35601, "ĠDante": 35602, "pture": 35603, "Ī문": 35604, "¯¸ë": 35605, "IJIJ": 35606, "ĠCounter": 35607, "Ġcrist": 35608, "Ġì§ľ": 35609, "Ġjeune": 35610, "ĠÑģÑĤÑĢаÑĪ": 35611, "ĠmieÄĩ": 35612, "Ġtutor": 35613, "Ġmasala": 35614, "Ġpowdered": 35615, "Ġnau": 35616, "ĠFrederick": 35617, "Ġbilling": 35618, "ĠEisen": 35619, "ĠдобÑĢ": 35620, "Ġmest": 35621, "æ½": 35622, "Ġsnipp": 35623, "Ġmono": 35624, "ĠAlo": 35625, "ĠMercy": 35626, "érience": 35627, "Ġcasualties": 35628, "ĠANNOUNCER": 35629, "ä»İ": 35630, "Ġtocar": 35631, "Ġbacterial": 35632, "Ho": 35633, "Ġstreak": 35634, "ĠJENN": 35635, "Ġplast": 35636, "Ñģлед": 35637, "Ġreapp": 35638, "Ġpaycheck": 35639, "Ġminers": 35640, "habt": 35641, "ĠJap": 35642, "нÑĥÑĤ": 35643, "Ġredemption": 35644, "Ġquir": 35645, "hnlich": 35646, "Ġaccumulation": 35647, "Ġshove": 35648, "Ġadrenaline": 35649, "Make": 35650, "ĠHern": 35651, "ossing": 35652, "ĠVil": 35653, "ubby": 35654, "hertz": 35655, "breaks": 35656, "Ġspur": 35657, "ĠDaha": 35658, "USTIN": 35659, "Ġcontinuer": 35660, "ĠSaul": 35661, "ãģ®ãģ¯": 35662, "ĠíıŃ": 35663, "ĠëIJĺë©´": 35664, "Ġë§IJìĶĢ": 35665, "Ġож": 35666, "Ġsuspects": 35667, "Ġlaquelle": 35668, "ĠMuchas": 35669, "Ġvöllig": 35670, "ulen": 35671, "Ġimpres": 35672, "Ġlobb": 35673, "enee": 35674, "Ġнаж": 35675, "Ta": 35676, "Ġréalité": 35677, "ĠRex": 35678, "Ġharvesting": 35679, "Ġestr": 35680, "æ¶": 35681, "ospace": 35682, "OSS": 35683, "Ġdisturbance": 35684, "assic": 35685, "ĠIsab": 35686, "Ġdécouv": 35687, "ĠHampshire": 35688, "Ġornament": 35689, "Ġluôn": 35690, "ĠUW": 35691, "ĠjÄħ": 35692, "éĤ£ä¹Ī": 35693, "Ġrespecto": 35694, "Ġcomunidad": 35695, "Ġcomigo": 35696, "agna": 35697, "Ġintrinsic": 35698, "ĠAlumni": 35699, "Ġsesleri": 35700, "Ġestimation": 35701, "âĢĶâĢĶ": 35702, "Ġproduit": 35703, "ãĢĤãĢį": 35704, "ĠвÑĢ": 35705, "Ġwhirl": 35706, "Ġacces": 35707, "çu": 35708, "Ġvariability": 35709, "Ġvodka": 35710, "itsu": 35711, "Ġinternships": 35712, "Ġallocate": 35713, "RR": 35714, "íĽĪ": 35715, "Ġinstructional": 35716, "tant": 35717, "Ġà®ħத": 35718, "Ġinvites": 35719, "Ġhak": 35720, "Ġscares": 35721, "Ġeclipse": 35722, "пов": 35723, "колÑĮ": 35724, "ativas": 35725, "Ġstabbed": 35726, "ĠDOM": 35727, "ä¸įåΰ": 35728, "roots": 35729, "ĠPicture": 35730, "íĺ¼": 35731, "ĠCHA": 35732, "iec": 35733, "ıı": 35734, "hanol": 35735, "Ġmisunderstand": 35736, "Ray": 35737, "Ġroadmap": 35738, "ocumented": 35739, "izione": 35740, "ĠOlive": 35741, "rift": 35742, "Ġ×Ķ׳": 35743, "æ¯į": 35744, "lest": 35745, ";;": 35746, "ĠEA": 35747, "éľĢè¦ģ": 35748, "одÑĥ": 35749, "Ġhobbies": 35750, "Ġburial": 35751, "ãģ«ãģ¡ãģ¯": 35752, "Ф": 35753, "lege": 35754, "ĠHJ": 35755, "Ġobjection": 35756, "ĠãģŃ": 35757, "ctory": 35758, "Ġincremental": 35759, "Ġgymn": 35760, "Ġepidemi": 35761, "ÑģÑĭл": 35762, "Ãij": 35763, "Ġadvancement": 35764, "Ġparch": 35765, "News": 35766, "Ġayr": 35767, "лам": 35768, "Ġ׾ש": 35769, "Ġdiploma": 35770, "ãģ¡ãĤĥãĤĵ": 35771, "Ġrobbed": 35772, "Only": 35773, "Ġincur": 35774, "Ġchanting": 35775, "Ġíķ´ëıĦ": 35776, "Ġriches": 35777, "ĠCarmen": 35778, "Ġnostro": 35779, "λÎŃ": 35780, "ĠPowder": 35781, "à¹Ģห": 35782, "ĠìŀĪìľ¼ë©´": 35783, "Ġgerçekten": 35784, "ĠPikachu": 35785, "емон": 35786, "OLL": 35787, "Ġplanetary": 35788, "Ġslows": 35789, "Ġclockwise": 35790, "alion": 35791, "ĠìĮ": 35792, "Ġvern": 35793, "Ġhomme": 35794, "Ġendpoint": 35795, "Ġinnocence": 35796, "Ġelementos": 35797, "Ġsophomore": 35798, "Ġnotions": 35799, "ĠCouldn": 35800, "pur": 35801, "Ġzat": 35802, "Ġobsess": 35803, "Ġmotivo": 35804, "ĠKub": 35805, "ĠDrug": 35806, "Ant": 35807, "ĠPlayers": 35808, "ĠHumans": 35809, "Ġmelee": 35810, "ĠWildlife": 35811, "ĠVP": 35812, "Ġvolcanic": 35813, "Ġcomin": 35814, "ĠGuang": 35815, "ĠÏĦιÏĤ": 35816, "ĠоÑģобенно": 35817, "ĠSize": 35818, "Listen": 35819, "ĠAaa": 35820, "appro": 35821, "Ġbarbar": 35822, "ĠParkinson": 35823, "нÑıÑĤÑĮ": 35824, "åį°": 35825, "Ġunderestimate": 35826, "Ġsubstitution": 35827, "Ġcosmetic": 35828, "ä¸ĭ次": 35829, "Ġwillen": 35830, "Ġbeide": 35831, "anni": 35832, "Ġconditioned": 35833, "ĠDebbie": 35834, "Ġisto": 35835, "ĠEdwards": 35836, "ìĽĮìļĶ": 35837, "ĠÑĤов": 35838, "Ġabbrevi": 35839, "ĠMün": 35840, "ĠPrinc": 35841, "ĠLiang": 35842, "Ġstink": 35843, "Ġradioactive": 35844, "ãģĨãĤı": 35845, "Ġacontec": 35846, "Ġuncon": 35847, "ĠTurbo": 35848, "ãģIJ": 35849, "Ġkisses": 35850, "æĺ¯ä»Ģ麼": 35851, "еÑĤÑĢов": 35852, "Ġfrontier": 35853, "ĠSpy": 35854, "ĠBelarus": 35855, "ĠCBS": 35856, "á»Ĺ": 35857, "amoto": 35858, "íķľëį°": 35859, "ĠÑģÑĤÑĢо": 35860, "ĠEnfin": 35861, "Ġbreadth": 35862, "éĺ²": 35863, "ĠCafe": 35864, "ĠDafür": 35865, "ĠBour": 35866, "aras": 35867, "Ġblueprint": 35868, "anı": 35869, "Ġconstants": 35870, "Ġattacker": 35871, "ĠFormula": 35872, "zaÄĩ": 35873, "Ġsowie": 35874, "Ġeyebrow": 35875, "obook": 35876, "Ġsetzen": 35877, "第ä¸ī": 35878, "onsider": 35879, "awning": 35880, "Ġsöyleye": 35881, "Ġinvaded": 35882, "Ġpronouns": 35883, "Ġdobry": 35884, "Si": 35885, "ĠХоÑĤ": 35886, "Ġvolleyball": 35887, "Ġlament": 35888, "isches": 35889, "arme": 35890, "api": 35891, "ĠWiki": 35892, "лиÑĪ": 35893, "Ġkasih": 35894, "Ġpess": 35895, "ĠÑĦоÑĤ": 35896, "ĠSul": 35897, "å¾·": 35898, "Ġpseudo": 35899, "Ġmemo": 35900, "ĠìŰìĬµ": 35901, "ĠдоллаÑĢов": 35902, "ĠпеÑĢем": 35903, "ĠReach": 35904, "miral": 35905, "alted": 35906, "Ġstatut": 35907, "reading": 35908, "Ġsöyled": 35909, "ĠLindsey": 35910, "ĠAhmad": 35911, "ë¶Ģë": 35912, "ĠСегоднÑı": 35913, "Ġprzygot": 35914, "Ġhyster": 35915, "URE": 35916, "ĠNeigh": 35917, "Reporter": 35918, "ĠBunu": 35919, "ĠTreaty": 35920, "ĠRank": 35921, "ĠFame": 35922, "inished": 35923, "Ġgeared": 35924, "Ġcompose": 35925, "odia": 35926, "ĠLon": 35927, "ĠjesteÅĽmy": 35928, "ĠDIRECTOR": 35929, "Ġelkaar": 35930, "ĠViel": 35931, "×IJש": 35932, "ynthia": 35933, "並": 35934, "Ġmère": 35935, "ĠTomato": 35936, "Ġexatamente": 35937, "niÄĻ": 35938, "ĠFrei": 35939, "ĠDif": 35940, "Ġopenings": 35941, "Ġgraphical": 35942, "ĠÑĥдоб": 35943, "ĠвÑģп": 35944, "ĠWeekly": 35945, "ева": 35946, "Ġhangs": 35947, "Ġunsafe": 35948, "Ġemblem": 35949, "ĠKolleginnen": 35950, "alay": 35951, "Ġksi": 35952, "Ġhides": 35953, "Ġolmay": 35954, "Ġentste": 35955, "Ġarthritis": 35956, "ÃŁerdem": 35957, "Ġbinnen": 35958, "Ġlistens": 35959, "ĠHess": 35960, "åĨįä¾Ĩ": 35961, "ĠLouise": 35962, "lden": 35963, "енÑģ": 35964, "ĠVersion": 35965, "ĠAgriculture": 35966, "ìĬ¤ë¥¼": 35967, "ман": 35968, "ëĦ¤ìļĶ": 35969, "Ġwines": 35970, "ĠINF": 35971, "rul": 35972, "ĠJK": 35973, "ıyorlar": 35974, "shield": 35975, "reath": 35976, "Ġterus": 35977, "ĠLum": 35978, "Ġanticipation": 35979, "Ġaccustomed": 35980, "ĠMina": 35981, "Ġwield": 35982, "ioè": 35983, "mera": 35984, "Ġcountdown": 35985, "Ġcling": 35986, "Ġcommend": 35987, "Ġfaktiskt": 35988, "Ġdefenses": 35989, "Ġcockpit": 35990, "Ġкоманд": 35991, "Ġdishwas": 35992, "ĠThanos": 35993, "Ġkidneys": 35994, "Ġsehe": 35995, "Ġmicrobes": 35996, "Ġcuff": 35997, "ĠвÑĭÑģок": 35998, "ĠSpicy": 35999, "çŃīçŃī": 36000, "வர": 36001, "culus": 36002, "orc": 36003, "ç¾ħ": 36004, "ixes": 36005, "ĠCredit": 36006, "Ġraj": 36007, "Ġbringt": 36008, "ĠNiss": 36009, "Ġgrim": 36010, "ĠSOL": 36011, "Ġtenim": 36012, "ĠSudan": 36013, "ĠSpart": 36014, "Ġpromotes": 36015, "ĠNossa": 36016, "ĠÑģоÑģÑĤоÑıни": 36017, "Ġì°©": 36018, "Ġuncont": 36019, "ĠLiberal": 36020, "ĠТолÑĮко": 36021, "ĠViele": 36022, "Ġktórej": 36023, "Ġ****": 36024, "Max": 36025, "ĠЧÑĤобÑĭ": 36026, "350": 36027, "Ġíĺ¼ìŀIJ": 36028, "Ġë¶Ħëĵ¤ìĿ´": 36029, "Ġwarp": 36030, "Ġtenga": 36031, "Ġsympathetic": 36032, "Ġbizi": 36033, "ĠZack": 36034, "iedo": 36035, "Ġëī´ì": 36036, "piel": 36037, "ĠÑĤол": 36038, "Ġscaled": 36039, "ĠPETER": 36040, "ĠCOMM": 36041, "ĠCame": 36042, "Ġcatastrophe": 36043, "Ġsweaty": 36044, "igration": 36045, "Ġstuffing": 36046, "ĠÏĢολÏį": 36047, "ĠDriver": 36048, "zyst": 36049, "Tech": 36050, "Ġassessed": 36051, "ĠSurface": 36052, "ırım": 36053, "sur": 36054, "lerweile": 36055, "Ġдог": 36056, "Ġshutting": 36057, "Ġfractions": 36058, "ĠÑģол": 36059, "everyone": 36060, "Ġern": 36061, "ĠÐĿов": 36062, "Ġdefenders": 36063, "Ġversucht": 36064, "ãĥ³ãĥĢ": 36065, "Ġpolity": 36066, "ĠÐŁÐ¾Ð½": 36067, "verständ": 36068, "Ġbrowsers": 36069, "Ġtransformative": 36070, "Ġdictate": 36071, "ĠLEGO": 36072, "Ġninguna": 36073, "ê´ij": 36074, "Ġpizz": 36075, "ĠHarold": 36076, "ĠLopez": 36077, "Ú¾ÛĮ": 36078, "anız": 36079, "atchet": 36080, "ÙĬت": 36081, "Ġlernen": 36082, "Ġê·ĢìŬ": 36083, "Ġhoused": 36084, "Ġcleanse": 36085, "ĠWAT": 36086, "laration": 36087, "Ġbytes": 36088, "Ġtucked": 36089, "Ġfaults": 36090, "до": 36091, "FX": 36092, "Ġìĸ¼ë§ĪëĤĺ": 36093, "Ġdeform": 36094, "Ġcontracting": 36095, "ĠTIME": 36096, "irse": 36097, "Ġneben": 36098, "Ġcerc": 36099, "ĠArmstrong": 36100, "Ġtester": 36101, "Ġparfait": 36102, "Ġjealousy": 36103, "Ġtoxins": 36104, "Ġdisbel": 36105, "ÑĥÑĢÑĭ": 36106, "impression": 36107, "Ġprostate": 36108, "Ġfirewall": 36109, "Ġclassics": 36110, "еÑĩÑĮ": 36111, "Ġsocialism": 36112, "Ġgracious": 36113, "ĠÑģнова": 36114, "ĠднÑı": 36115, "Ġburner": 36116, "ĠMinor": 36117, "Ġìļ°ë¦¬ë": 36118, "Ġjedes": 36119, "Ġcontinuum": 36120, "Ġhots": 36121, "Ġoccurrence": 36122, "Ġadministered": 36123, "ĠзамеÑĤ": 36124, "Ġhesitation": 36125, "Ġdrills": 36126, "erca": 36127, "ĠвÑĤоÑĢой": 36128, "Ġsteadily": 36129, "Ġinsanlar": 36130, "Ġihan": 36131, "íij": 36132, "Ġhelper": 36133, "ĠSenin": 36134, "åģľ": 36135, "ование": 36136, "ĠERIC": 36137, "bla": 36138, "ĠAcademic": 36139, "Ġhumanities": 36140, "black": 36141, "umpy": 36142, "ortex": 36143, "ĠìłĪë": 36144, "ĠØ¥ÙĨ": 36145, "Ġdisclose": 36146, "ĠElijah": 36147, "ĠλÎŃ": 36148, "ĠQuer": 36149, "بÙĦ": 36150, "ãĤ¡": 36151, "Tell": 36152, "arle": 36153, "ÑĸÑĢ": 36154, "Ġaugmented": 36155, "Ġë¹ĦìĬ·": 36156, "Ġandroid": 36157, "त": 36158, "arma": 36159, "Ġszer": 36160, "geord": 36161, "Ġgeek": 36162, "Ġyeux": 36163, "Ġpong": 36164, "ĠãģĿãģĨ": 36165, "Ġtortured": 36166, "ĠBath": 36167, "zig": 36168, "asonable": 36169, "Ġnets": 36170, "Ġbaru": 36171, "ĠFlat": 36172, "ĠVater": 36173, "ĠTerror": 36174, "ĠAvo": 36175, "Ġceremonies": 36176, "roe": 36177, "Ù쨳": 36178, "Ops": 36179, "Ġhyvin": 36180, "Ġapresent": 36181, "olor": 36182, "ĠигÑĢÑĭ": 36183, "orton": 36184, "Ġê·¸ëŀ¬": 36185, "Ġlookin": 36186, "ĠTY": 36187, "ĠMint": 36188, "Add": 36189, "Ġmite": 36190, "ĠSmoke": 36191, "Ġnota": 36192, "Ġmoss": 36193, "ĠAbend": 36194, "Ġ컨": 36195, "Ġexaggerated": 36196, "fires": 36197, "Ġredist": 36198, "ffiti": 36199, "Ġopenness": 36200, "ê°IJìĿ´": 36201, "endeu": 36202, "енной": 36203, "Watch": 36204, "Ġavatar": 36205, "ĠPey": 36206, "urun": 36207, "Ġsenza": 36208, "Ġì§ĢìĹŃ": 36209, "ĠNatomiast": 36210, "Ġemergence": 36211, "rays": 36212, "Ġcrafted": 36213, "gary": 36214, "ãģłãģij": 36215, "üng": 36216, "-\"": 36217, "Ġhacked": 36218, "Ġstray": 36219, "encie": 36220, "emo": 36221, "Ġcomen": 36222, "ĠKız": 36223, "ĠJasmine": 36224, "ĠHindi": 36225, "manas": 36226, "Ġinfinitely": 36227, "emon": 36228, "ìĿ¸ëį°ìļĶ": 36229, "jak": 36230, "Ġroaring": 36231, "érique": 36232, "sweise": 36233, "ĠRolex": 36234, "åł±å°İ": 36235, "ĠStuart": 36236, "bnb": 36237, "Ġdiagnose": 36238, "Ġcoherent": 36239, "ĠMJ": 36240, "æºĸåĤĻ": 36241, "Ġpike": 36242, "lav": 36243, "Ġorchestral": 36244, "аÑģÑĤи": 36245, "Ġterminar": 36246, "Ġgatherings": 36247, "Ġcompliant": 36248, "Ġupgrading": 36249, "Ġregulator": 36250, "Ġlanç": 36251, "éĢ£": 36252, "Ġmerchants": 36253, "tawa": 36254, "Ġmonitored": 36255, "Ġrendre": 36256, "两": 36257, "Ġunterwegs": 36258, "anguard": 36259, "gard": 36260, "ĠBelow": 36261, "duino": 36262, "ĠЦе": 36263, "Ġimpedance": 36264, "ìľ¡": 36265, "份": 36266, "Ġaktuell": 36267, "ĠVatic": 36268, "åŃ©": 36269, "Ġstewards": 36270, "Ġbrightest": 36271, "Ġkenn": 36272, "Ġkau": 36273, "ĠMatrix": 36274, "ĠBark": 36275, "ĠðŁij": 36276, "Ġtaper": 36277, "Ġcasino": 36278, "ר×Ķ": 36279, "ysical": 36280, "Ġbuilders": 36281, "ĠczÅĤowie": 36282, "ĠNepal": 36283, "Ġ!\"": 36284, "Ġterme": 36285, "Ġinnych": 36286, "Ġmaths": 36287, "Ġdrafted": 36288, "ĠBalk": 36289, "Ġhesitant": 36290, "Ġvoltar": 36291, "Ġrevive": 36292, "ĠÑĦилÑĮма": 36293, "Ġassassin": 36294, "ĠSolutions": 36295, "Ġduel": 36296, "Ġbearings": 36297, "à¸Ħะ": 36298, "Ġrookie": 36299, "ikat": 36300, "Ġbiscuits": 36301, "Ġcords": 36302, "ÑĥваÑĤи": 36303, "ARIN": 36304, "Ġprogressing": 36305, "ĠGir": 36306, "Ġpenetrate": 36307, "ĠStorage": 36308, "eight": 36309, "ĠÑĤÑĢÑĥ": 36310, "ĠdonÃŃt": 36311, "Ġsizin": 36312, "Ġoutdated": 36313, "ĠнаÑĪи": 36314, "Ġaffir": 36315, "Ġspoons": 36316, "Ġoni": 36317, "Ġflank": 36318, "ĠGol": 36319, "hã": 36320, "Ġpéri": 36321, "Ġhonorable": 36322, "ĠBreathe": 36323, "scenes": 36324, "Ġobviamente": 36325, "икÑģ": 36326, "Ġש×ŀ×": 36327, "Ġsmoothie": 36328, "ŀĪë": 36329, "Ġdime": 36330, "ĠíĸĪìĸ´ìļĶ": 36331, "Ġappel": 36332, "ĠCatholics": 36333, "Ġsingles": 36334, "Ġlaten": 36335, "Ġçünkü": 36336, "ĠVader": 36337, "æıĽ": 36338, "Ġvardı": 36339, "ĠIstanbul": 36340, "gré": 36341, "ĠElsa": 36342, "ël": 36343, "Ġinvece": 36344, "Ġcrane": 36345, "Ġobe": 36346, "ĠShark": 36347, "Ġsmack": 36348, "Ġrestoring": 36349, ".\\": 36350, "Ġë¹łë": 36351, "Ġfaded": 36352, "umbers": 36353, "Singing": 36354, "Ġdepressing": 36355, "thest": 36356, "ĠWahr": 36357, "Ġmultitude": 36358, "ÑĢавÑģÑĤвÑĥйÑĤе": 36359, "rijk": 36360, "eka": 36361, "Ġcompletes": 36362, "ĠWells": 36363, "Ġroy": 36364, "ĠPray": 36365, "ĠKalau": 36366, "izin": 36367, "iaÅĤem": 36368, "Ġlocom": 36369, "ĠNashville": 36370, "ĠPentagon": 36371, "미": 36372, "ĠNEW": 36373, "ÄħÄĩ": 36374, "ÃŃss": 36375, "Ġmarrying": 36376, "Ġfeud": 36377, "íĻķ": 36378, "æĢ¥": 36379, ")!": 36380, "ĠOperations": 36381, "ÑĥÑĶ": 36382, "Ġmoje": 36383, "Ġinstructed": 36384, "ĠëĪĦ구": 36385, "Ġ×Ķ×Ĵ": 36386, "ĠпомоÑīÑĮÑİ": 36387, "Ġsabia": 36388, "ìķĺìĸ´ìļĶ": 36389, "plane": 36390, "pri": 36391, "ĠполноÑģÑĤÑĮÑİ": 36392, "ĠKitty": 36393, "Ġpróprio": 36394, "edere": 36395, "Ġinteresante": 36396, "Ġде": 36397, "Ġcondensed": 36398, "Ġavent": 36399, "TOR": 36400, "Ġgreasy": 36401, "ARK": 36402, "orta": 36403, "AJ": 36404, "Ġdisreg": 36405, "Ġcorrections": 36406, "Ġstero": 36407, "Ġinfluenza": 36408, "Ġdesses": 36409, "Ġballots": 36410, "Ġmeget": 36411, "Ġmafia": 36412, "Ġböl": 36413, "nost": 36414, "ĠÑģÑĤаÑĤÑĮ": 36415, "Ġresponder": 36416, "Ġhinten": 36417, "grav": 36418, "à¸Ńะ": 36419, "ynchron": 36420, "Ġviens": 36421, "Ġsamo": 36422, "Ġdt": 36423, "pannt": 36424, "ĠÅĽwiat": 36425, "ĠзапиÑģ": 36426, "Ġmerged": 36427, "Ġkep": 36428, "Ġmisleading": 36429, "Ġdigamos": 36430, "Ġammon": 36431, "è¾Ľ": 36432, "chet": 36433, "Ġê°Ģìł¸": 36434, "Ġuni": 36435, "ĠëIJĺëĬĶëį°": 36436, "ĠнапÑĢав": 36437, "ĠкоÑĤоÑĢого": 36438, "Ġanimate": 36439, "×ķ×IJ×": 36440, "еÑĢв": 36441, "Ġminced": 36442, "Ġkaum": 36443, "ãģĤãģģ": 36444, "ÏĢε": 36445, "лег": 36446, "existing": 36447, "Ġplataform": 36448, "ĠKRIS": 36449, "ìĽł": 36450, "ĠFamilien": 36451, "ĠLibya": 36452, "Ġbiodiversity": 36453, "Ġidiots": 36454, "irdi": 36455, "Ġszyb": 36456, "ĠRolling": 36457, "ücht": 36458, "ĠÑĥдив": 36459, "ÑģÑĥд": 36460, "Ġrealizar": 36461, "Ġcanned": 36462, "ĠÑĢан": 36463, "Ġmetabolic": 36464, "ĠBeef": 36465, "Ġkilka": 36466, "лÑİÑģ": 36467, "Ġregistry": 36468, "моÑĤÑĢиÑĤе": 36469, "Ġvielä": 36470, "Ġodc": 36471, "Ġcondemned": 36472, "æ©ĭ": 36473, "fal": 36474, "ĠDil": 36475, "woÅĽci": 36476, "Aw": 36477, "Ġstatistically": 36478, "Ġsogen": 36479, "ĠBETH": 36480, "Ġshaving": 36481, "幸": 36482, "ocal": 36483, "ĠFunny": 36484, "Ġpeacefully": 36485, "Ġaddictive": 36486, "ĠInsert": 36487, "lauf": 36488, "Ġexperiencia": 36489, "é¦ĸåħĪ": 36490, "иÑĤелÑı": 36491, "ÃŃgen": 36492, "ágina": 36493, "Ġabdomen": 36494, "íķľëĭ¤": 36495, "icus": 36496, "imana": 36497, "ìį¨": 36498, "arching": 36499, "Ġkonkret": 36500, "ìķĺë": 36501, "ека": 36502, "oufl": 36503, "ivel": 36504, "Ġnude": 36505, "ètres": 36506, "Ġmonsieur": 36507, "Ġclash": 36508, "Ġtherapists": 36509, "Ġcubed": 36510, "Ġretrouver": 36511, "Ġwaveform": 36512, "Ġpotem": 36513, "ĠFormer": 36514, "isión": 36515, "åºľ": 36516, "Ġ×IJ×Ŀ": 36517, "undos": 36518, "ĠMeinung": 36519, "صÙĦ": 36520, "ĠJude": 36521, "ĠnÃ¥r": 36522, "ĠLeonardo": 36523, "ĠCristo": 36524, "ĠGOT": 36525, "ÑģÑĤÑĢÑĥк": 36526, "LAN": 36527, "ĠgÃ¥ng": 36528, "Ġdéb": 36529, "ĠFrankfurt": 36530, "Ġcrappy": 36531, "Ġlil": 36532, "année": 36533, "ĠмеÑģÑĤе": 36534, "RET": 36535, "ĠNer": 36536, "ĠCOSTA": 36537, "Ġjedem": 36538, "Ġcurtains": 36539, "Ġiterations": 36540, "Ġunav": 36541, "Ġplaque": 36542, "orum": 36543, "Ġζ": 36544, "Ġnúmeros": 36545, "Ġdesap": 36546, "²½": 36547, "Ġcompiled": 36548, "Ġrefle": 36549, "Ġrankings": 36550, "Ġrepaired": 36551, "ĠÐĿапÑĢ": 36552, "Ġdownloads": 36553, "Ġarmour": 36554, "Ġ×Ļ×ķתר": 36555, "Ġlongevity": 36556, "ĠTONER": 36557, "ĠкомменÑĤаÑĢ": 36558, "Ġczego": 36559, "Ġnotify": 36560, "Ġairports": 36561, "Ġenduring": 36562, "lette": 36563, "Ġapparat": 36564, "Ġhabil": 36565, "á»ĩc": 36566, "nad": 36567, "ICO": 36568, "ĠBrah": 36569, "Ġsegún": 36570, "Ġgovernors": 36571, "kaha": 36572, "ĠSchluss": 36573, "Ġodpowied": 36574, "irting": 36575, "Ġrempl": 36576, "ĠAboriginal": 36577, "identally": 36578, "Ġenhancing": 36579, "licting": 36580, "ĠHawaiian": 36581, "Ġstriving": 36582, "ĠNiet": 36583, "Ġznaczy": 36584, "Ġobedience": 36585, "ĠnÃ¥got": 36586, "Ġexpired": 36587, "Ġ1918": 36588, "presented": 36589, "Ġprowad": 36590, "ĠTerr": 36591, "ĠPrinceton": 36592, "Ġmorgen": 36593, "Ġattracting": 36594, "ĠSigma": 36595, "igner": 36596, "ĠRechts": 36597, "ĠPeki": 36598, "Ġmethy": 36599, "Ġhamm": 36600, "Ġdireito": 36601, "Ġdelegation": 36602, "иваÑİÑĤ": 36603, "Ġgin": 36604, "Young": 36605, "Ġdependencies": 36606, "ĠBradley": 36607, "buds": 36608, "Ġfis": 36609, "Ġpytanie": 36610, "Ġinterconnected": 36611, "Ġembaixo": 36612, "ĠSas": 36613, "Ġruh": 36614, "ĠSicht": 36615, "Sur": 36616, "Ġsuperb": 36617, "ĠSabbath": 36618, "ĠDanger": 36619, "kol": 36620, "Ġhou": 36621, "supp": 36622, "ĠNacional": 36623, "Ġsuccession": 36624, "Ġvá": 36625, "ĠMaÃŁnahmen": 36626, "ĠJessie": 36627, "ĠIdaho": 36628, "forest": 36629, "ħĺ": 36630, "Ġ×ŀ×ĵ": 36631, "ĠØ£ÙĬ": 36632, "Ġsweetheart": 36633, "Ġneatly": 36634, "ĠEvangel": 36635, "곡": 36636, "ĠSuite": 36637, "ública": 36638, "ĠÑĥли": 36639, "ĠAnnouncer": 36640, "ligh": 36641, "Ġsensations": 36642, "Ġshelters": 36643, "Ġhart": 36644, "Ġsqueezing": 36645, "ĠRivers": 36646, "ĠCooking": 36647, "ì±ħ": 36648, "personal": 36649, "Ġmanos": 36650, "ÑijÑĤÑģÑı": 36651, "wij": 36652, "Ġgogg": 36653, "ĠMilli": 36654, "ĠFP": 36655, "ünst": 36656, "ĠLS": 36657, "Ġspraying": 36658, "Ġfaux": 36659, "Ġautograph": 36660, "ologic": 36661, "Ġtorment": 36662, "Ġencrypted": 36663, "á»ħ": 36664, "Ġestre": 36665, "ç¹¼": 36666, "à±": 36667, "Ġstumbled": 36668, "Ġaider": 36669, "Ġsaben": 36670, "xter": 36671, "ĠCities": 36672, "ĠTürk": 36673, "ëĭ¥": 36674, "chine": 36675, "Ġtopping": 36676, "Ġpoisoned": 36677, "ĠRomania": 36678, "×ĵ×Ļ": 36679, "Ģë¡ľ": 36680, "ĠпоÑĢÑıд": 36681, "Ġchirping": 36682, "ĠìĻĦë": 36683, "×ij×¢": 36684, "Ġcuanto": 36685, "Ġdonating": 36686, "ĠRegent": 36687, "ĠBeruf": 36688, "Ġdistracting": 36689, "Ġstamina": 36690, "ĠDarren": 36691, "Ġì¶ķ": 36692, "lists": 36693, "dal": 36694, "chuss": 36695, "Ġeconomist": 36696, "ãģĪãĥ¼": 36697, "orgt": 36698, "Ġistiyorum": 36699, "è¿Ľ": 36700, "ĠSurprise": 36701, "ĠHao": 36702, "Ġìµľê³ł": 36703, "ĠGW": 36704, "ĠInner": 36705, "Ġquieren": 36706, "Ġminded": 36707, "Ġsupercomputer": 36708, "Ġdiagrams": 36709, "íĬľë": 36710, "ê²łìĸ´": 36711, "ĠобÑĬÑıÑģ": 36712, "Ġestaban": 36713, "Ġdestroys": 36714, "ĠBreaking": 36715, "ĠkarÄ±ÅŁ": 36716, "Ġrebuilding": 36717, "ľëĮĢ": 36718, "ливо": 36719, "ĠSauce": 36720, "ĠFusion": 36721, "×ķ×ŀ×": 36722, "ĠQuinn": 36723, "Ġgauche": 36724, "ĠÙĪØ£": 36725, "ĠÈ": 36726, "çĵľ": 36727, "Ġtechno": 36728, "Ġdispatch": 36729, "ĠaÅŁk": 36730, "Ġeinzel": 36731, "ĠGmail": 36732, "çŀ": 36733, "Ġê°ľìĿ¸": 36734, "ĠÑģемÑĮ": 36735, "Ġjourneys": 36736, "Ġiht": 36737, "Ġfibre": 36738, "Ġdramas": 36739, "ouched": 36740, "Ġrename": 36741, "ĠопеÑĢ": 36742, "Ġpoo": 36743, "ĠDru": 36744, "ĠиÑĤог": 36745, "Ġzast": 36746, "Ġcoz": 36747, "Ġzucch": 36748, "Ġobtaining": 36749, "Ġcommute": 36750, "Ġsubmer": 36751, "ĠVish": 36752, "ĠRabb": 36753, "ogg": 36754, "Ġhut": 36755, "íĸĪìĸ´": 36756, "æ¯Ķå¦Ĥ": 36757, "eremi": 36758, "Ġμα": 36759, "Ġdiskut": 36760, "ĠбÑĥк": 36761, "Ġimpaired": 36762, "depend": 36763, "ĠÙĪØ§": 36764, "ĠÑĢÑĥк": 36765, "ĠбаÑĢ": 36766, "Ġoxidation": 36767, "Ġsituação": 36768, "ÉĻn": 36769, "ução": 36770, "Ġsagte": 36771, "ĠSER": 36772, "ĠCake": 36773, "Ġturmeric": 36774, "ĠKak": 36775, "bung": 36776, "ĠKá¹Ľá¹£á¹ĩa": 36777, "Ġpoisoning": 36778, "Ġslipping": 36779, "ĠSays": 36780, "å°±åı¯ä»¥": 36781, "òng": 36782, "çŁ³": 36783, "«": 36784, "ĠClaudia": 36785, "ĠCharacter": 36786, "ниÑĨ": 36787, "coat": 36788, "Ġprogressed": 36789, "ĠFergus": 36790, "Ġìĺ¤ëĬ": 36791, "Ġoat": 36792, "ordable": 36793, "ĠLey": 36794, "ĠHeraus": 36795, "Ġresultados": 36796, "ĠKayla": 36797, "Ġriff": 36798, "Ġchegou": 36799, "Ġxi": 36800, "Ġspacious": 36801, "Ġrecognised": 36802, "Ġech": 36803, "ĠTie": 36804, "Ġlauncher": 36805, "Jim": 36806, "Ġsuppression": 36807, "ĠImpossible": 36808, "Ġguitars": 36809, "ĠFourier": 36810, "иÑĩеÑģкий": 36811, "ĠTherap": 36812, "ĠKaf": 36813, "centered": 36814, "ĠÑģооÑĤвеÑĤ": 36815, "Ġklim": 36816, "Ġcarbohydrates": 36817, "ignant": 36818, "ĠAstron": 36819, "Ġemple": 36820, "Ġdrastic": 36821, "ĠмиÑĢе": 36822, "вин": 36823, "uw": 36824, "Ġprettier": 36825, "Ġdonuts": 36826, "ĠAthena": 36827, "Ġdissert": 36828, "Ġplante": 36829, "Ġuranium": 36830, "ìĿĮë": 36831, "aré": 36832, "Ġrzecz": 36833, "Ġdisplaying": 36834, "æĪ²": 36835, "Ġsarc": 36836, "rão": 36837, "Ġtampoco": 36838, "Ġphilosophers": 36839, "ĠRecht": 36840, "æĵļ": 36841, "Ġcomentarios": 36842, "yse": 36843, "Ġìľ¤": 36844, "Ġmise": 36845, "ĠGin": 36846, "Ġном": 36847, "ĠFROM": 36848, "liner": 36849, "atif": 36850, "ĠspoÅĤec": 36851, "xa": 36852, "ĠÑĤÑĢÑĥд": 36853, "Ġwag": 36854, "기ìĹIJ": 36855, "ĠMG": 36856, "Ġoffspring": 36857, "ĠUnderstanding": 36858, "åıªæĺ¯": 36859, "ORA": 36860, "Ġwhirring": 36861, "Ġsurrend": 36862, "Ġpoker": 36863, "Ġmonuments": 36864, "ĠâĻ©": 36865, "Ġorganised": 36866, "ĠSozial": 36867, "ĠFactory": 36868, "Ñħа": 36869, "Ġresemble": 36870, "зд": 36871, "Ġexplosions": 36872, "Ġpayroll": 36873, "Ġomn": 36874, "ĠJorge": 36875, "ιÏĥ": 36876, "Ġfracture": 36877, "Ġpersecution": 36878, "Ġdemais": 36879, "ECH": 36880, ",)": 36881, "Ġcriar": 36882, "ĠJOSH": 36883, "Ġdemographics": 36884, "Ġ1600": 36885, "Ġcurrencies": 36886, "ĠTips": 36887, "ĠéĢĻåĢĭ": 36888, "ĠRefer": 36889, "ĠDancing": 36890, "Ġinconsistent": 36891, "Ġdeh": 36892, "Ġimmens": 36893, "Ġmeist": 36894, "Ġimpatient": 36895, "Ġbehaves": 36896, "æĿ¾": 36897, "ĠëĤ´ìļ©": 36898, "Ġbackstory": 36899, "Ġagreeing": 36900, "ĠÅģ": 36901, "ihin": 36902, "Ġtemperatura": 36903, "ĠBackground": 36904, "Ġnutzen": 36905, "Ġëħ¹": 36906, "ĠMänner": 36907, "Ġcollaborations": 36908, "ĠKos": 36909, "éģİåİ»": 36910, "Ġnightmares": 36911, "ëĵ±": 36912, "ĠQueensland": 36913, "Ġassociates": 36914, "ĠKok": 36915, "Ġfactorial": 36916, "ĠHyung": 36917, "Ġê·¸ëĭ¤ìĿĮ": 36918, "Ġfilho": 36919, "Ġelét": 36920, "Ġíĸīë³µ": 36921, "°±": 36922, "Ġgefunden": 36923, "Ġsemicondu": 36924, "Ġcounselors": 36925, "ĠUpper": 36926, "ĠAub": 36927, "ickers": 36928, "Ver": 36929, "Ġnorthwest": 36930, "ĠMaintenant": 36931, "ĠLakes": 36932, "аÑıв": 36933, "inté": 36934, "ì°½": 36935, "Ġгаз": 36936, "Ġgiorn": 36937, "Ġdigitally": 36938, "ĠCircuit": 36939, "ì¼Ģ": 36940, "ãĤĬãģ¾ãģĹãģŁ": 36941, "Ġcheerful": 36942, "ĠPeterson": 36943, "ĠDanish": 36944, "ativos": 36945, "Ġliken": 36946, "Ġharbor": 36947, "алиÑģÑĤ": 36948, "xe": 36949, "Ġcurls": 36950, "ĠRhod": 36951, "End": 36952, "ĠET": 36953, "Ġacquaint": 36954, "ĠKelvin": 36955, "Ġtrif": 36956, "ĠAway": 36957, "ìŀIJëĬĶ": 36958, "vs": 36959, "Ġpágina": 36960, "Ġinlet": 36961, "ĠSantos": 36962, "Ġìļ°ìĻĢ": 36963, "Ġyapıyorsun": 36964, "theme": 36965, "Ġsouff": 36966, "Ġinjected": 36967, "Ġpóźniej": 36968, "iverso": 36969, "amped": 36970, "Ġdaher": 36971, "Ġdagger": 36972, "ĠлÑİбим": 36973, "Ġtummy": 36974, "Ġenlightened": 36975, "cents": 36976, "ĠDah": 36977, "Ġcuest": 36978, "ä¾Ĩ說": 36979, "ILY": 36980, "Ġ×ijר": 36981, "Ġbanging": 36982, "ĠEmil": 36983, "ĠCler": 36984, "ĠBorder": 36985, "ижÑĥ": 36986, "Ġpresenters": 36987, "ĠSTUD": 36988, "coins": 36989, "ĠíĻį": 36990, "Ġperks": 36991, "Ġparap": 36992, "Ġcertaines": 36993, "ĠLore": 36994, "öst": 36995, "ĠMARTIN": 36996, "Ġbios": 36997, "Ġwhereby": 36998, "verts": 36999, "ĠMiranda": 37000, "Ġstip": 37001, "澤": 37002, "andez": 37003, "׼׾": 37004, "ujin": 37005, "Ġê¾": 37006, "Ġallergies": 37007, "plate": 37008, "Ġyapıl": 37009, "Ġundertake": 37010, "ĠëĤĺê°Ģ": 37011, "Part": 37012, "Ġkızım": 37013, "hguru": 37014, "ãģĤãģ¨": 37015, "ĠJohns": 37016, "Ġeyelashes": 37017, "Ġdrained": 37018, "ĠstÃ¥r": 37019, "ãģĤãĤĬãģ¾ãģĻ": 37020, "ĠJade": 37021, "Ġcalend": 37022, "film": 37023, "Ġmesa": 37024, "Ġludzie": 37025, "Ġattracts": 37026, "Ġjuices": 37027, "Ġкил": 37028, "Ġnieuwe": 37029, "Ġmencion": 37030, "Ġignition": 37031, "Ġbladder": 37032, "andaag": 37033, "ĠExtension": 37034, "íĤ¨": 37035, "feed": 37036, "ĠÙĪÙĩ": 37037, "Ġspun": 37038, "Ġtät": 37039, "оÑĢоÑĤ": 37040, "tyard": 37041, "ronics": 37042, "ĠHuge": 37043, "Ñĥжд": 37044, "string": 37045, "Ġunjust": 37046, "Ġprawn": 37047, "Ġfrosting": 37048, "Ġdisappearance": 37049, "iosa": 37050, "Ġcardi": 37051, "ĠPriest": 37052, "ĠcientÃŃfic": 37053, "åĵªè£¡": 37054, "ĠÐĴаÑģ": 37055, "Ġë¶Ģíĥģ": 37056, "Ġthieves": 37057, "Ġphysique": 37058, "ĠEugene": 37059, "Ġблиз": 37060, "Ġmonopoly": 37061, "Ġbiography": 37062, "ĠhoÅŁ": 37063, "Ġtö": 37064, "mac": 37065, "Ġshocks": 37066, "ìĦ¸ë": 37067, "hit": 37068, "Ġsnug": 37069, "Ġincl": 37070, "Ġdedic": 37071, "Ġultras": 37072, "ĠизвеÑģÑĤ": 37073, "Ġutilization": 37074, "ĠÑģовеÑĢÑĪенно": 37075, "Ġservi": 37076, "stag": 37077, "180": 37078, "Ġsewer": 37079, "ĠChoice": 37080, "Ġdischarged": 37081, "ĠJD": 37082, "олеÑĤ": 37083, "ĠкваÑĢÑĤи": 37084, "Ġtelescop": 37085, "ĠJeÅĽli": 37086, "ĠNana": 37087, "cale": 37088, "ĠÑĤон": 37089, "mmm": 37090, "äºĨåIJ§": 37091, "Ġgehabt": 37092, "ëĤł": 37093, "æĬķ": 37094, "à¸Ļà¸Ļ": 37095, "Ġether": 37096, "Ġzen": 37097, "Ġresearched": 37098, "ĠCzyli": 37099, "å®Įåħ¨": 37100, "workers": 37101, "Ġ경찰": 37102, "Ġsheriff": 37103, "allo": 37104, "Ġtipos": 37105, "Ġprosecution": 37106, "Ġfrogs": 37107, "Ġfalt": 37108, "jd": 37109, "ĠíĮĶ": 37110, "Ġfiltered": 37111, "ĠOft": 37112, "Ġìį": 37113, "Ġdisfr": 37114, "ĠMustang": 37115, "Ġwoah": 37116, "ĠREALLY": 37117, "Ġмогли": 37118, "Ġentrada": 37119, "ĠигÑĢа": 37120, "Ġmixes": 37121, "ĠавÑĤомоб": 37122, "ÐĻ": 37123, "Ġshin": 37124, "Ġparanormal": 37125, "Ġsomeplace": 37126, "Ġdishon": 37127, "etaan": 37128, "Ġfuerte": 37129, "Ù¹": 37130, "Ġdoom": 37131, "ìĪľ": 37132, "Ġexistential": 37133, "Ġbuld": 37134, "ĠSDK": 37135, "ĠпÑĢавда": 37136, "Ġturnover": 37137, "ĠìĹ¬ê¸°ìĹIJ": 37138, "Ġह": 37139, "Ġmodeled": 37140, "Ġbugün": 37141, "Ġexperimentation": 37142, "Ġmornings": 37143, "Ġmedo": 37144, "Stevie": 37145, "Ġplayable": 37146, "Ġairlines": 37147, "gments": 37148, "Ġ기ë¶Ħ": 37149, "ĠTomb": 37150, "ĠMVP": 37151, "AUDIENCE": 37152, "Ġcheckout": 37153, "Ġpasst": 37154, "Ġbeispiel": 37155, "ĠLinks": 37156, "heavy": 37157, "Ġquestionable": 37158, "Ġìĵ°ë": 37159, "Ġsill": 37160, "Ġmanipulated": 37161, "ĠLoren": 37162, "Ġìľ¼": 37163, "Ġverge": 37164, "ák": 37165, "IES": 37166, "Ġsabot": 37167, "ĠCustomer": 37168, "ależy": 37169, "Ġnominee": 37170, "ĠGad": 37171, "Ġnouvelles": 37172, "ĠSPE": 37173, "istling": 37174, "Ġoval": 37175, "обÑĢаж": 37176, "ifty": 37177, "éĩİ": 37178, "Ġbezel": 37179, "yet": 37180, "Ġfreight": 37181, "ĠHanım": 37182, "rÃŃa": 37183, "Ġzoning": 37184, "Ġindem": 37185, "ĠBü": 37186, "Ġfeminism": 37187, "Ġvoix": 37188, "Ġoficial": 37189, "Ġdiyorum": 37190, "»IJ": 37191, "Ġarose": 37192, "Ġparar": 37193, "ìĿ¸ì§Ģ": 37194, "ĠMartine": 37195, "ĠLect": 37196, "Ġrester": 37197, "Ġdrowning": 37198, "uya": 37199, "cida": 37200, "ĠAriel": 37201, "Ġ02": 37202, "Ġ×Ķ×Ķ": 37203, "ç´ł": 37204, "ĠWert": 37205, "ТÑĭ": 37206, "Ġwidow": 37207, "Ġparchment": 37208, "Ġcottage": 37209, "ĠXL": 37210, "ĠSlack": 37211, "ĠNES": 37212, "Ġrobe": 37213, "Ġgimm": 37214, "Ġcaminho": 37215, "ĠHarper": 37216, "Ġcitrus": 37217, "Ġfirefighters": 37218, "Ġdopamine": 37219, "elets": 37220, "Ġdemocrat": 37221, "ìłľë¡ľ": 37222, "Ġplayback": 37223, "oj": 37224, "ĠпÑĢок": 37225, "ĠSullivan": 37226, "semble": 37227, "ĠWorth": 37228, "ĠMustafa": 37229, "าร": 37230, "Ġmets": 37231, "éĸĢ": 37232, "лоÑģÑĮ": 37233, "Ġinertia": 37234, "Ġuniforms": 37235, "è¶³": 37236, "ério": 37237, "×ķר×Ķ": 37238, "ént": 37239, "Ġà®Ĵ": 37240, "ĠÑģамÑĭÑħ": 37241, "Ġvoulais": 37242, "ĠZimmer": 37243, "ê²łë": 37244, "ĠноÑģ": 37245, "encias": 37246, "Ġrelación": 37247, "Ġ걸ë": 37248, "Ġfaction": 37249, "Ġgosp": 37250, "полож": 37251, "nap": 37252, "hak": 37253, "Ġproceedings": 37254, "ĠìĨĶ": 37255, "ìķĦëĭĪ": 37256, "ĠìŀIJ기": 37257, "Ġwerd": 37258, "Ġsof": 37259, "Ġschlim": 37260, "Ġflavored": 37261, "Ġquadratic": 37262, "ĠBoot": 37263, "Ġpublicity": 37264, "ĠCaro": 37265, "Ġ?\"": 37266, "ниÑĨа": 37267, "mania": 37268, "ĠSUR": 37269, "ĠBUR": 37270, "lance": 37271, "ética": 37272, "Ġzobaczy": 37273, "Ġtrio": 37274, "sama": 37275, "ĠtaÅŁ": 37276, "Ġasymm": 37277, "resser": 37278, "Ġتع": 37279, "ĠпеÑģ": 37280, "Ġbeginnings": 37281, "ladım": 37282, "ĠбÑĭÑģÑĤÑĢ": 37283, "Ġmoo": 37284, "ĠGeneva": 37285, "Ġåľ¨": 37286, "erus": 37287, "borah": 37288, "Ġrefusing": 37289, "bull": 37290, "ĠWaiting": 37291, "ĠIndividual": 37292, "Ġanonym": 37293, "imens": 37294, "Ġmedidas": 37295, "Ġfragrant": 37296, "Ġdirectement": 37297, "ĠìķĦë§Ī": 37298, "uria": 37299, "Ġspherical": 37300, "Ġabge": 37301, "ĠVictorian": 37302, "Ġspectacle": 37303, "ĠRodriguez": 37304, "Ġocup": 37305, "ĠNär": 37306, "marks": 37307, "ngulo": 37308, "ĠLuci": 37309, "Ġshouted": 37310, "Ġregulators": 37311, "ÄŁini": 37312, "Ġdisent": 37313, "ĠÑĢÑĭн": 37314, "ëĤ¨": 37315, "ĠìĤ´ë": 37316, "Ġproblèmes": 37317, "ĠFinger": 37318, "assemble": 37319, "Ġpear": 37320, "Ġdroite": 37321, "ĠEverywhere": 37322, "tam": 37323, "оÑĤив": 37324, "вой": 37325, "ordinate": 37326, "ĠLak": 37327, "ĠmỼi": 37328, "ĠTelevision": 37329, "Ġexponentially": 37330, "avas": 37331, "Ġblev": 37332, "ĠMT": 37333, "俺": 37334, "Connell": 37335, "ĠêµŃ민": 37336, "ĠÑģвоим": 37337, "Ġacha": 37338, "ĠDynasty": 37339, "Jin": 37340, "Ġtore": 37341, "Ġflor": 37342, "Ġмногие": 37343, "æ²Ĵäºĭ": 37344, "owan": 37345, "bah": 37346, "Ġì£Ħ": 37347, "ĠCela": 37348, "Ġìµľê·¼": 37349, "Ġpermettre": 37350, "Ġabras": 37351, "Ġverstehen": 37352, "Ġescort": 37353, "ĠThem": 37354, "ärke": 37355, "porter": 37356, "Ġkahkaha": 37357, "Ġhect": 37358, "Ġdau": 37359, "wah": 37360, "olve": 37361, "ĠAges": 37362, "schaft": 37363, "ĠStell": 37364, "nelle": 37365, "ĠEnsuite": 37366, "ĠÐĴÑģем": 37367, "Ġcréd": 37368, "ĠPP": 37369, "lords": 37370, "grunting": 37371, "Ġcontraction": 37372, "Got": 37373, "Ġacquiring": 37374, "Ġsopr": 37375, "Ġpoisonous": 37376, "RNA": 37377, "Ġanar": 37378, "ĠHof": 37379, "')": 37380, "Ġremarkably": 37381, "Ġinternacional": 37382, "ücke": 37383, "inqu": 37384, "Ġduy": 37385, "Ġbeasts": 37386, "ĠLAN": 37387, "Ġprecedent": 37388, "ĠRPM": 37389, "åij¨": 37390, "Ġselon": 37391, "Ġmorte": 37392, "Ġcomeçou": 37393, "Ñıла": 37394, "Ġinterpreting": 37395, "ĠBurke": 37396, "ÑĤÑĢа": 37397, "ĠìĿ´ë٬": 37398, "Ġpessim": 37399, "ĠNok": 37400, "íĮĿ": 37401, "Female": 37402, "Ġìĭ¤í": 37403, "ĻĢ": 37404, "Ġstimulation": 37405, "Ġslick": 37406, "Ġê°ĢëĬĶ": 37407, "Ġказ": 37408, "ĠHBO": 37409, "Ġpapier": 37410, "Ġkönnten": 37411, "Ñĥбли": 37412, "ĠConstant": 37413, "SPEAKING": 37414, "ĠktórÄħ": 37415, "Ġcosmetics": 37416, "ĠTrend": 37417, "Ġrobbery": 37418, "Ġtitt": 37419, "Ġgjort": 37420, "Ġdietary": 37421, "łĮ": 37422, "ĠKirby": 37423, "ĠпÑĢимеÑĢно": 37424, "Ġqualification": 37425, "Ġìķī": 37426, "Ġcabinets": 37427, "Ġhttp": 37428, "ĠErica": 37429, "義": 37430, "Ġdisadvantages": 37431, "Ġchattering": 37432, "yz": 37433, "feit": 37434, "Ġguild": 37435, "ĠETF": 37436, "ĠDragons": 37437, "ĠHERE": 37438, "venth": 37439, "ÙĦاÙħ": 37440, "Ġmarché": 37441, "Dam": 37442, "Ġphoton": 37443, "Ġestable": 37444, "Mag": 37445, "Ġolhar": 37446, "Ġcoupling": 37447, "ĠHilfe": 37448, "ĠWizard": 37449, "Ġмало": 37450, "help": 37451, "ĠlÃŃnea": 37452, "Ġì«": 37453, "Ġstandalone": 37454, "Ġmorale": 37455, "Ġzweite": 37456, "ãĤĪãĤįãģĹãģı": 37457, "ährt": 37458, "Ġdotted": 37459, "Ġdripping": 37460, "ĠFlag": 37461, "éĿĴ": 37462, "rocket": 37463, "rategy": 37464, "irim": 37465, "Ġíķĺë©´ìĦľ": 37466, "Ġsogenan": 37467, "ĠUno": 37468, "ĠSchutz": 37469, "Ġestilo": 37470, "ĠSubs": 37471, "ĠDaisy": 37472, "ÐĿеÑĤ": 37473, "'...": 37474, "Ġplatinum": 37475, "Ġbirl": 37476, "ĠSovi": 37477, "Ġviolate": 37478, "ÑĥеÑĤÑģÑı": 37479, "rill": 37480, "Ġtraz": 37481, "Ġsnip": 37482, "Ġcumpl": 37483, "à¸Ńà¸ģ": 37484, "Ġcuk": 37485, "éħĴ": 37486, "ĠParlament": 37487, "Ġhypert": 37488, "Ġpulp": 37489, "Ġtongues": 37490, "atto": 37491, "Ġbusca": 37492, "ihn": 37493, "ERO": 37494, "ĠÙĬع": 37495, "Ġvarias": 37496, "ĠMarian": 37497, "Ġbounded": 37498, "Ġpitching": 37499, "Ġdeficiency": 37500, "ĠBlessed": 37501, "ĠExerc": 37502, "uchs": 37503, "Ġnhưng": 37504, "æľ¬å½ĵ": 37505, "Ġraped": 37506, "hales": 37507, "Ġmala": 37508, "pic": 37509, "Ġ401": 37510, "ÅĽniej": 37511, "arina": 37512, "ëĵ¤ìĿĦ": 37513, "otti": 37514, "Ġдолго": 37515, "Ġtracker": 37516, "ĠShelby": 37517, "Ġvanished": 37518, "Ġbakery": 37519, "Kapı": 37520, "Jesus": 37521, "ĠKR": 37522, "JO": 37523, "ħ¸": 37524, "Ġdiscs": 37525, "ìĦ¯": 37526, "ì§Ģë": 37527, "×Ļצ": 37528, "emary": 37529, "Kendra": 37530, "Ġyük": 37531, "ückt": 37532, "Ġvaz": 37533, "Ġkup": 37534, "aktu": 37535, "ĠÑģпаÑģибо": 37536, "Ġaik": 37537, "Ġnursery": 37538, "Ġendangered": 37539, "êmement": 37540, "ematics": 37541, "Ġresponders": 37542, "ĠRepresentatives": 37543, "Ġsculptures": 37544, "igkeiten": 37545, "Ġdepl": 37546, "Ġinterpretations": 37547, "Ġdeadlines": 37548, "Ġ1942": 37549, "ÃĹ": 37550, "Ġsugars": 37551, "emu": 37552, "lively": 37553, "Ġrecreational": 37554, "Ġdistort": 37555, "Ġunderscore": 37556, "Ġunquote": 37557, "Ġsafest": 37558, "Ġswollen": 37559, "Ġanalyses": 37560, "Ġcommencé": 37561, "妹": 37562, "andin": 37563, "ĠХоÑĢоÑĪо": 37564, "Ġdiarr": 37565, "ãģ¾ãģģ": 37566, "ziest": 37567, "Ġtoothbrush": 37568, "éł»éģĵ": 37569, "uations": 37570, "Ġcade": 37571, "Ġbacklash": 37572, "hind": 37573, "Ġrisque": 37574, "zess": 37575, "ĠìĿ´ìķ¼ê¸°": 37576, "Ġesperar": 37577, "Ġtranslations": 37578, "ioned": 37579, "groans": 37580, "ĠпÑĥÑĤ": 37581, "Ġgenetically": 37582, "éĢł": 37583, "Ġhappiest": 37584, "Ġwerk": 37585, "atoon": 37586, "Ġmusi": 37587, "Ġfunção": 37588, "ĠìŀħëĭĪëĭ¤": 37589, "ĠÑĢай": 37590, "Ġbevor": 37591, "BLANK": 37592, "Ġrepentance": 37593, "Put": 37594, "Ġpotrzeb": 37595, "Ġsala": 37596, "Ġcampa": 37597, "WER": 37598, "ĠdecÃŃa": 37599, "Ġsécurité": 37600, "ĠAppreciate": 37601, "Ñĩи": 37602, "ĠRandom": 37603, "ë³Ħ": 37604, "kah": 37605, "Ġmöj": 37606, "Ġsäger": 37607, "Ġ×Ļ׼×ķ׾": 37608, "Ġ190": 37609, "xtures": 37610, "Eu": 37611, "Ġgä": 37612, "Ġ×ijת": 37613, "ĠCroat": 37614, "apo": 37615, "PLE": 37616, "Ġpersistence": 37617, "åĬ©": 37618, "Ġblends": 37619, "Ġtreffen": 37620, "ĠSantiago": 37621, "ydia": 37622, "aldo": 37623, "ĠTensorFlow": 37624, "ĠDual": 37625, "ãĥľ": 37626, "Ġchiff": 37627, "ìĹ´": 37628, "Ġcontracted": 37629, "Ġsegreg": 37630, "ĠFairy": 37631, "Ġwisely": 37632, "Ġvulnerabilities": 37633, "Ġhandheld": 37634, "Ġgadgets": 37635, "ĠboÅŁ": 37636, "ĠPopular": 37637, "Ġcurvature": 37638, "문": 37639, "ĠMARY": 37640, "ìĿ´ìĬ": 37641, "Ġformulation": 37642, "Ġcelery": 37643, "Ġblurry": 37644, "ĠTS": 37645, "alez": 37646, "Ġws": 37647, "Ġprogramm": 37648, "ĠStack": 37649, "ĠJIM": 37650, "овали": 37651, "ıll": 37652, "Ġpère": 37653, "ĠKanye": 37654, "ĠDelaware": 37655, "Ġãģł": 37656, "Ġdaunting": 37657, "ĠбеÑģ": 37658, "ĠStupid": 37659, "big": 37660, "fficial": 37661, "Ġprecipitation": 37662, "Ġplung": 37663, "ục": 37664, "burse": 37665, "Ġdarle": 37666, "Ġcripp": 37667, "Ġpioneer": 37668, "Ġdisput": 37669, "Ġsean": 37670, "ãģĵãĤĵãģª": 37671, "Ġresistor": 37672, "Ġallein": 37673, "ipples": 37674, "arel": 37675, "Ġendors": 37676, "zust": 37677, "ĠÑĢебÑıÑĤа": 37678, "eded": 37679, "Ġì¹´ë©Ķë": 37680, "Ġlleva": 37681, "Ġkennt": 37682, "Ġбал": 37683, "ĠDocument": 37684, "ĠKnights": 37685, "Ġbuckle": 37686, "Ġìī¬": 37687, "Ġalk": 37688, "ĠEveryday": 37689, "atters": 37690, "Ġtoilets": 37691, "Ġjugar": 37692, "ĠìŀĪì§Ģ": 37693, "Ġgenauso": 37694, "ĠLandesregierung": 37695, "ãģ£ãģ±": 37696, "ije": 37697, "Ġtrailers": 37698, "ĠTigers": 37699, "Ġgitti": 37700, "Ġforgiving": 37701, "Ġconcurrent": 37702, "ĠVu": 37703, "ĠíĬ¹íŀĪ": 37704, "ĠBROWN": 37705, "ounded": 37706, "\";": 37707, "Ġtremb": 37708, "Ġtiet": 37709, "ĠÑĢежим": 37710, "Ġnutshell": 37711, "елиÑĩ": 37712, "Ġlosers": 37713, "ricting": 37714, "Ġredeem": 37715, "defined": 37716, "Nice": 37717, "Ġbroadband": 37718, "KO": 37719, "Ġteasing": 37720, "Ġpartisan": 37721, "ıma": 37722, "Ġìŀ¬ë¯¸": 37723, "ĠJourney": 37724, "Ġslopes": 37725, "uning": 37726, "grunts": 37727, "Ġtäll": 37728, "Ġuncovered": 37729, "ĠmyÅĽlÄĻ": 37730, "ĠEsther": 37731, "äºİ": 37732, "ĠHealthy": 37733, "Ġë°ij": 37734, "rée": 37735, "Ġpolarization": 37736, "Ġflav": 37737, "Ġcambiar": 37738, "Ġyr": 37739, "ĠRanch": 37740, "Ġsplits": 37741, "Ġtrouvé": 37742, "åľĭå®¶": 37743, "Ġrecorder": 37744, "Ġdépart": 37745, "ÙĪØ¨": 37746, "ĠKry": 37747, "Ġinteressant": 37748, "Ġederim": 37749, "ÅĽwiad": 37750, "ilateral": 37751, "wright": 37752, "Ġpourra": 37753, "êter": 37754, "Ġcamel": 37755, "áŀ": 37756, "Ġrapidement": 37757, "Ġmej": 37758, "Ġstiffness": 37759, "ADAS": 37760, "Ġdiffers": 37761, "Ġalot": 37762, "ĠSig": 37763, "ÑıÑĤелÑĮ": 37764, "Ġabstraction": 37765, "åľĺ": 37766, "Ġkeiner": 37767, "grupp": 37768, "ĠSherlock": 37769, "íĺĶ": 37770, "Ġcite": 37771, "Ġoverflow": 37772, "Ġtại": 37773, "úcar": 37774, "bula": 37775, "Ġconjunto": 37776, "ĠCI": 37777, "Ġmoderator": 37778, "Ġindirectly": 37779, "Ġalleine": 37780, "âĤ": 37781, "ÑĪиб": 37782, "Ġбаб": 37783, "Ġdanach": 37784, "Ġ1939": 37785, "Ġpromet": 37786, "Ġdestinations": 37787, "ĠIllust": 37788, "ικÏĮ": 37789, "Ġsabes": 37790, "Ġheh": 37791, "ĠGesetzent": 37792, "ĠMiz": 37793, "енко": 37794, "ĠMys": 37795, "Ь": 37796, "ĠJudaism": 37797, "Ġmustache": 37798, "Ġstimmt": 37799, "ĠGaza": 37800, "Ġvolte": 37801, "Ġnuo": 37802, "Ġmón": 37803, "ĠComput": 37804, "ูà¹Ī": 37805, "ĠRadi": 37806, "Ġexceptionally": 37807, "Ġassumes": 37808, "éĸĭå¿ĥ": 37809, "ãģĪãģ°": 37810, "inform": 37811, "Ġshrine": 37812, "æĵĬ": 37813, "Ġimplication": 37814, "ĠFitz": 37815, "æ²ĴéĹľä¿Ĥ": 37816, "!.": 37817, "Ġlt": 37818, "Ġalloy": 37819, "Ġethic": 37820, "Ġmonastery": 37821, "ìĭľì£ł": 37822, "icação": 37823, "Ġcoordinating": 37824, "ĠMoto": 37825, "Ġoverlook": 37826, "Ġchois": 37827, "Ġantibiotic": 37828, "ĠMinne": 37829, "ĠBJ": 37830, "ĠApa": 37831, "orian": 37832, "Ġspilled": 37833, "Jam": 37834, "Ġhusbands": 37835, "Ġcreations": 37836, "Ġañ": 37837, "üssel": 37838, "ĠìĿ´ìļ©": 37839, "Ġanalyse": 37840, "rose": 37841, "Ġpunched": 37842, "Ġpresque": 37843, "Ġastronomy": 37844, "Ġschwierig": 37845, "ĠEbola": 37846, "Ġcis": 37847, "Ġacet": 37848, "ĠFX": 37849, "endre": 37850, "ĠìĿĮìķħ": 37851, "Ġwebpage": 37852, "Ġfreaked": 37853, "Ġlatte": 37854, "Ġì¿ł": 37855, "Ġ머ë": 37856, "Never": 37857, "Gra": 37858, "íĻĶ를": 37859, "eyed": 37860, "Ġë°ľëĿ¼": 37861, "Ġespera": 37862, "Ġaparece": 37863, "ração": 37864, "Ġdisruptive": 37865, "ĠJoint": 37866, "urous": 37867, "reas": 37868, "ĠquerÃŃa": 37869, "Ġdistributions": 37870, "Ġexponent": 37871, "ì¹ĺ를": 37872, "Ġdl": 37873, "zhou": 37874, "ĠHearing": 37875, "å·®ä¸įå¤ļ": 37876, "ĠCraw": 37877, "Ġfloats": 37878, "ounced": 37879, "Lab": 37880, "World": 37881, "Ġburdens": 37882, "Ġauthoritarian": 37883, "ĠBolt": 37884, "ĠоднÑĥ": 37885, "Ġpigeon": 37886, "Ġdistractions": 37887, "ĠHerausforder": 37888, "Ġzest": 37889, "esc": 37890, "Ġshakes": 37891, "atas": 37892, "ĠÙħØ´": 37893, "holes": 37894, "Ġthinkers": 37895, "alta": 37896, "Ġarche": 37897, "ĠSuk": 37898, "anha": 37899, "Ġtempting": 37900, "Ġyoutuber": 37901, "Ġvì": 37902, "ĠdziaÅĤa": 37903, "ĠVatican": 37904, "Park": 37905, "Ġsupers": 37906, "ĠNikki": 37907, "ëĬIJë": 37908, "orang": 37909, "ramient": 37910, "鬼": 37911, "Ġê°ĸê³ł": 37912, "Ġdesserts": 37913, "Ġavere": 37914, "ĠGregory": 37915, "Ġëĵ¤ìĸ´ìĺ": 37916, "Ġcosting": 37917, "ĠClinic": 37918, "Ġrebels": 37919, "ĠMob": 37920, "Ġbunlar": 37921, "ĠYours": 37922, "ertime": 37923, "Ġretali": 37924, "mara": 37925, "atus": 37926, "alles": 37927, "ĠдÑĢ": 37928, "ĠдиÑģ": 37929, "Ġdiscounts": 37930, "ĠGUY": 37931, "Ġкакое": 37932, "ĠExperiment": 37933, "rement": 37934, "ĠXiang": 37935, "Ġbate": 37936, "WE": 37937, "Ġspecialize": 37938, "Ġdeity": 37939, "ĠLoki": 37940, "mag": 37941, "ĠNit": 37942, "West": 37943, "Ġmaternal": 37944, "Ġquis": 37945, "åŁºæľ¬": 37946, "broken": 37947, "Ġlasers": 37948, "Ġhakk": 37949, "ĠAngels": 37950, "Ġmastery": 37951, "antis": 37952, "Tiffany": 37953, "eee": 37954, "çij": 37955, "orem": 37956, "Ġinacc": 37957, "Ġjurisdictions": 37958, "ĠKardash": 37959, "æľº": 37960, "Il": 37961, "ĠSinn": 37962, "åĭķçĶ»": 37963, "Ġathletics": 37964, "cÄĻ": 37965, "Ġloosely": 37966, "Ġdieta": 37967, "Ag": 37968, "Ġ??": 37969, "ĠëĮĢíijľ": 37970, "Ġsuperv": 37971, "Ġnutrit": 37972, "Ġdrifting": 37973, "ĠìĦłìĥĿëĭĺ": 37974, "ĠпонÑıл": 37975, "ĠVictory": 37976, "ÙĦØ©": 37977, "×ķ׳×Ķ": 37978, "ĠпиÑĪ": 37979, "Ġshaved": 37980, "Ġmesure": 37981, "onden": 37982, "Ùĥر": 37983, "Ġexile": 37984, "ĠDesde": 37985, "ĠPinterest": 37986, "Ġattachments": 37987, "Ġhombres": 37988, "Ġfines": 37989, "ĠìĦ¸ìĥģ": 37990, "Ġsleeps": 37991, "ĠTaco": 37992, "ĠIRA": 37993, "rios": 37994, "Ġoll": 37995, "etes": 37996, "Ġunut": 37997, "fashioned": 37998, "Ġtreball": 37999, "ĠNearly": 38000, "ĠÑĢеалÑĮно": 38001, "Ġchil": 38002, "é̱": 38003, "ÄŁa": 38004, "ĠMEL": 38005, "roscop": 38006, "ĠCG": 38007, "Ġvenge": 38008, "Ġdishwasher": 38009, "algic": 38010, "Ġmodifier": 38011, "Ġembassy": 38012, "timer": 38013, "emics": 38014, "Ġintricate": 38015, "Ġevet": 38016, "ĠëĮĢë°ķ": 38017, "Ġisot": 38018, "ĠнаÑĥÑĩ": 38019, "ĠQuiz": 38020, "reso": 38021, "δÏİ": 38022, "Ġyelled": 38023, "Ġfeder": 38024, "ELLER": 38025, "Ġexceeded": 38026, "onas": 38027, "icano": 38028, "ĠживоÑĤ": 38029, "ĠMao": 38030, "ĠKazuto": 38031, "Ġãħĭãħĭãħĭãħĭ": 38032, "Ġfrontline": 38033, "ĠHungarian": 38034, "Ġüberall": 38035, "awat": 38036, "Ġgrips": 38037, "ições": 38038, "arnya": 38039, "ĠÍ¡": 38040, "Ġseid": 38041, "Ġanak": 38042, "Ġacabou": 38043, "íķij": 38044, "Ġnotorious": 38045, "ĠGodzilla": 38046, "Ġovercoming": 38047, "ĠPend": 38048, "Ġolabilir": 38049, "ülme": 38050, "Ġerhalten": 38051, "ãĤīãģĦ": 38052, "ê·¹": 38053, "ĠMeter": 38054, "Ġstaan": 38055, "Ol": 38056, "Ġchats": 38057, "ĠBuenos": 38058, "ÃŃve": 38059, "aluable": 38060, "Ġstrategically": 38061, "Ġcomprised": 38062, "ĠпеÑĢÑģонаж": 38063, "Ġwann": 38064, "ĠCen": 38065, "ниÑĤе": 38066, "Łģ": 38067, "ĠÑĤобой": 38068, "iad": 38069, "ĠkardeÅŁim": 38070, "ĠCongressman": 38071, "reaming": 38072, "homme": 38073, "Ġcommunaut": 38074, "Ġalcoholic": 38075, "Ġpickled": 38076, "Ġacord": 38077, "position": 38078, "egól": 38079, "Ġtroubling": 38080, "ĠMarcheg": 38081, "Ġzumindest": 38082, "Ġseamlessly": 38083, "Ġolun": 38084, "ĠTVs": 38085, "ĠпÑĢакÑĤиÑĩеÑģки": 38086, "Ġbackend": 38087, "ãģĵãĤĵãģ«ãģ¡ãģ¯": 38088, "idable": 38089, "Ġgadget": 38090, "Ġfaço": 38091, "ĠMarchegiani": 38092, "Ġë°¤": 38093, "Ġaccidental": 38094, "ĠLP": 38095, "Ġeldest": 38096, "ĠAdmiral": 38097, "ĠnÄĥm": 38098, "lever": 38099, "Ġpastel": 38100, "Ġfondo": 38101, "Connie": 38102, "Ġtercer": 38103, "Ġpact": 38104, "ĠMonte": 38105, "Ġmeats": 38106, "ĠSMS": 38107, "ĠAustralians": 38108, "ç¼": 38109, "Rhett": 38110, "Ġexactement": 38111, "Ġë¹¼": 38112, "ĠMOD": 38113, "ç¡": 38114, "ĠRapt": 38115, "ĠNoch": 38116, "Ġabort": 38117, "ĠNaval": 38118, "ĠFuji": 38119, "INTER": 38120, "ĠновÑĭй": 38121, "Ġmiejsce": 38122, "ĠICU": 38123, "ĠGraduate": 38124, "ĠGlen": 38125, "ardi": 38126, "ĠÈĺ": 38127, "Ġsolder": 38128, "Ġprofessions": 38129, "Ġorthog": 38130, "omn": 38131, "introdu": 38132, "ĠDenise": 38133, "ìŀIJ를": 38134, "Ġcorrespondence": 38135, "AMA": 38136, "Ġinflict": 38137, "Ġfand": 38138, "ĠGü": 38139, "ĠÑĩеÑĤ": 38140, "Ġtraced": 38141, "Ġpatents": 38142, "Ġambush": 38143, "Ġlotta": 38144, "ffer": 38145, "ĠWagner": 38146, "Ġimperson": 38147, "Ġextrêmement": 38148, "ÙĤت": 38149, "conduct": 38150, "Att": 38151, "ĠMueller": 38152, "ĠAlicia": 38153, "Ġcyc": 38154, "Ġhacker": 38155, "Ġtys": 38156, "Ġhail": 38157, "ĠзаÑıв": 38158, "Ġpasso": 38159, "Ġì¶Ķê°Ģ": 38160, "ĠÎĪ": 38161, "Ġpackaged": 38162, "ĠCynthia": 38163, "heet": 38164, "ä¸ŃåĽ½": 38165, "ĠNissan": 38166, "ĠQuesto": 38167, "é¨": 38168, "did": 38169, "Ġμια": 38170, "ĠEllis": 38171, "ĠAnalysis": 38172, "cemos": 38173, "Ġaseg": 38174, "ĠMyster": 38175, "ĠCao": 38176, "Ġtuv": 38177, "ĠIndustry": 38178, "ì£¼ê³ł": 38179, "otal": 38180, "Ġpequeño": 38181, "bras": 38182, "Ġcomprehend": 38183, "ĠSimpson": 38184, "ÑģÑĤвие": 38185, "ocracy": 38186, "иÑĩеÑģки": 38187, "ĠMush": 38188, "ĠLaurie": 38189, "Ġtriangular": 38190, "ĠPresents": 38191, "ĠKunden": 38192, "ç´¹": 38193, "æŃ¦": 38194, "ĠIss": 38195, "ĠDeck": 38196, "á»ĥn": 38197, "ĠDarkness": 38198, "Ġinflammatory": 38199, "eremiah": 38200, "Ġwarmed": 38201, "veyard": 38202, "ĠMemory": 38203, "etty": 38204, "Ġtaxpayers": 38205, "à¸ĵ": 38206, "Ø¡": 38207, "Ġpractise": 38208, "ëĭ¬ë": 38209, "Ġdrilled": 38210, "mÃ¼ÅŁ": 38211, "logo": 38212, "ĠFach": 38213, "¤ë¡ľ": 38214, "Ġübrigens": 38215, "Ġkonnten": 38216, "Ġnormalmente": 38217, "Ġargues": 38218, "ilingual": 38219, "°ë¥¼": 38220, "egal": 38221, "Ġtravaill": 38222, "ovy": 38223, "аÑĤо": 38224, "Ġruth": 38225, "ĠLights": 38226, "Ġconsisted": 38227, "×ijר×Ļ×Ŀ": 38228, "Ġstereotype": 38229, "Ġpayer": 38230, "ĠRee": 38231, "ĠAirbnb": 38232, "Ġdrowned": 38233, "ĠZoe": 38234, "Ġcanopy": 38235, "Ġbarr": 38236, "ĠноÑĩ": 38237, "Ġpagan": 38238, "Ġjars": 38239, "Ġrê": 38240, "erver": 38241, "æĪ¿": 38242, "ieben": 38243, "Ġespect": 38244, "ĠFi": 38245, "Ġunwilling": 38246, "Ġtechnician": 38247, "ặt": 38248, "member": 38249, "ĠCanal": 38250, "سÙħ": 38251, "Ġlieber": 38252, "Ġinference": 38253, "Ġhonoring": 38254, "åijµ": 38255, "ĠCampaign": 38256, "Ġlineage": 38257, "ĠStress": 38258, "Ġvictories": 38259, "Ġdeja": 38260, "×£": 38261, "êtes": 38262, "blick": 38263, "Ġменее": 38264, "oths": 38265, "ĠCouple": 38266, "Jason": 38267, "ĠNicolas": 38268, "екÑģ": 38269, "lib": 38270, "Ġherramient": 38271, "Ġ×IJ×ķ×ŀר": 38272, "Ġвидим": 38273, "millimeter": 38274, "Ġsilhouette": 38275, "Ġdriveway": 38276, "Ġcherish": 38277, "ãħłãħł": 38278, "Ġransom": 38279, "Ġinterdisciplinary": 38280, "ĠPortal": 38281, "Ġtrag": 38282, "thood": 38283, "Ġtedious": 38284, "Ġglossy": 38285, "Ġprépar": 38286, "ĠCay": 38287, "ĠTook": 38288, "ĠBottom": 38289, "Ġzig": 38290, "å«": 38291, "åį±": 38292, "represented": 38293, "à¹Ģลย": 38294, "Ġdesarrollo": 38295, "ìĦľë": 38296, "Ġviscos": 38297, "Ġmilligram": 38298, "ĠGund": 38299, "Ġferment": 38300, "drum": 38301, "Ġdrawers": 38302, "Laugh": 38303, "Ġpelos": 38304, "Ġpavement": 38305, "Ġmemoir": 38306, "avait": 38307, "Ġ2050": 38308, "¤ë¥¼": 38309, "Ġrazón": 38310, "Ġflourish": 38311, "Ġstern": 38312, "ä¸Ī": 38313, "ĠChung": 38314, "Ġserpent": 38315, "ĠGentlemen": 38316, "羣çļĦå¾Ī": 38317, "kook": 38318, "Ġlut": 38319, "importe": 38320, "parent": 38321, "Ġwsz": 38322, "Ġscree": 38323, "ĠMitarbeiter": 38324, "å·´": 38325, "mut": 38326, "Ġìĸĺ기를": 38327, "Ġsemble": 38328, "ĠOW": 38329, "Ġinvestigator": 38330, "ĠCheryl": 38331, "ĠGerald": 38332, "Ġprere": 38333, "Ġcompares": 38334, "nyt": 38335, "Ġdiferença": 38336, "?-": 38337, "Ġquá": 38338, "ר×Ļ": 38339, "Sen": 38340, "Ġheps": 38341, "Ġgratuit": 38342, "Ġconsort": 38343, "ĠSTOP": 38344, "ĠProtestant": 38345, "Ġelectrode": 38346, "âĹ": 38347, "Ġsecurely": 38348, "иÑĩеÑģкой": 38349, "Ġtää": 38350, "Ġregisters": 38351, "ĠHeavenly": 38352, "ogly": 38353, "issä": 38354, "ĠPhysics": 38355, "ĠMerkel": 38356, "Ġrév": 38357, "éĻ¢": 38358, "Ġerased": 38359, "ĠSacramento": 38360, "Ġcoffin": 38361, "Ġexacer": 38362, "Ġlanz": 38363, "Ġpoets": 38364, "ulif": 38365, "Ġì¹ĺë": 38366, "ĠNerd": 38367, "ĠNCT": 38368, "ĠHour": 38369, "nehmer": 38370, "ŀĺëıĦ": 38371, "ĠPrinci": 38372, "Sw": 38373, "mies": 38374, "armed": 38375, "ĠBeatles": 38376, "Ġpropagation": 38377, "Ġexchanged": 38378, "Ġcumulative": 38379, "Ġì§ijìĹIJ": 38380, "Ġdefeating": 38381, "æĬ±": 38382, "bels": 38383, "Ġwes": 38384, "ĠOdyssey": 38385, "ä½łæĥ³": 38386, "avior": 38387, "ĠìľĦìĹIJ": 38388, "Ġbrit": 38389, "Ġhijo": 38390, "DAY": 38391, "ĠاÙĦتÙĬ": 38392, "ĠСеÑĢг": 38393, "Ñĥка": 38394, "edsiÄĻ": 38395, "Ġimpos": 38396, "Ġellas": 38397, "Ġfirearms": 38398, "ĠNR": 38399, "Ġ×ij×IJ": 38400, "ĠÐŁÐ¾ÐºÐ°": 38401, "awi": 38402, "ĠìĦ±ê³µ": 38403, "Ġpupils": 38404, "ĠTack": 38405, "Ġfrase": 38406, "ĠShip": 38407, "Ġstad": 38408, "举": 38409, "ĠGreater": 38410, "unun": 38411, "immung": 38412, "grown": 38413, "ĠNXT": 38414, "ĠAmericas": 38415, "fox": 38416, "Ġmanten": 38417, "éłIJåĤĻ": 38418, "ĠÑģок": 38419, "Ġrikt": 38420, "lectric": 38421, "deep": 38422, "ĠзнаеÑĪÑĮ": 38423, "Ġbenut": 38424, "ĠInfrast": 38425, "ĠEmir": 38426, "ĠоÑĤпÑĢав": 38427, "ĠKimchi": 38428, "ĠFinnish": 38429, "´ìłģ": 38430, "inaire": 38431, "Ġoike": 38432, "æ¸ħæ¥ļ": 38433, "Ġhostage": 38434, "ĠButton": 38435, "ÙĤÙĬ": 38436, "eking": 38437, "ĠKazakh": 38438, "Ġcomforting": 38439, "Ġsog": 38440, "Ġgreeted": 38441, "guitar": 38442, "payer": 38443, "Ġrelational": 38444, "Ġconstruir": 38445, "çī¹åĪ¥": 38446, "opian": 38447, "ĠVolume": 38448, "ieth": 38449, "ÑģÑĤвом": 38450, "urrection": 38451, "liÅĽmy": 38452, "Ġhemisphere": 38453, "ĠBean": 38454, "IGN": 38455, "Ġkötü": 38456, "ĠFallout": 38457, "Ġbrace": 38458, "ç¹¼çºĮ": 38459, "ÏĢά": 38460, "ĠHAS": 38461, "Ġgé": 38462, "Ġcharacterize": 38463, "ặc": 38464, "ĠMilky": 38465, "Ġtumors": 38466, "Ġnuit": 38467, "ĠGaz": 38468, "ĠìŀĪëĭ¤ëĬĶ": 38469, "ĠгаÑĢ": 38470, "essment": 38471, "ĠAbe": 38472, "Ġë½ij": 38473, "ĠEinsatz": 38474, "JIN": 38475, "jä": 38476, "Cry": 38477, "ĠPromised": 38478, "ĠÑģеÑĢд": 38479, "okus": 38480, "Ġscalable": 38481, "ĠпоÑģмоÑĤÑĢеÑĤÑĮ": 38482, "ücklich": 38483, "Ġrealism": 38484, "Ġmayo": 38485, "Ġjuvenile": 38486, "Ġheadlights": 38487, "ĠgörÃ¼ÅŁ": 38488, "ĠReform": 38489, "Ġhalves": 38490, "czne": 38491, "Ġbreakup": 38492, "żej": 38493, "Ġrätt": 38494, "Day": 38495, "ĠìĿ¼ë³¸": 38496, "Ġmuerte": 38497, "Ġtunes": 38498, "ĠSmile": 38499, "record": 38500, "Ġrecherche": 38501, "atisfied": 38502, "Ġpozi": 38503, "Ġcelebrations": 38504, "isexual": 38505, "ĠROB": 38506, "thirds": 38507, "ĠFortune": 38508, "ĠÑĤой": 38509, "Ġbranded": 38510, "loo": 38511, "Ġdud": 38512, "Ġrandomized": 38513, "Ġcombin": 38514, "ä¸ĢäºĽ": 38515, "ieran": 38516, "czenia": 38517, "įãĥ«": 38518, "Ġcurator": 38519, "Ġartery": 38520, "ĠÑĥÑĪ": 38521, "ĠÑĩиÑĤ": 38522, "Ġsubsidies": 38523, "Ġblossom": 38524, "ĠTwilight": 38525, "Ġhyvä": 38526, "ĠPompe": 38527, "ĠCisco": 38528, "ĠÐŁÑĢо": 38529, "Ġbiri": 38530, "Ġgern": 38531, "Ġrebuilt": 38532, "Ġwcze": 38533, "Ġbenefici": 38534, "Ġdrummer": 38535, "Ġsolids": 38536, "Ġdiyorsun": 38537, "ãģĤãĤĬãģĮãģ¨ãģĨãģĶãģĸãģĦãģ¾ãģĹãģŁ": 38538, "lated": 38539, "Ġmuddy": 38540, "Ġholog": 38541, "Ġclaps": 38542, "ĠRings": 38543, "ĠOkey": 38544, "ĠBrave": 38545, "Ġvaluation": 38546, "Ġmigrant": 38547, "Ġintermitt": 38548, "Ġeigene": 38549, "iliary": 38550, "ãĥ¼ãĥĪ": 38551, "markt": 38552, "kr": 38553, "ĠRib": 38554, "á»Ļi": 38555, "Ġaccusations": 38556, "Ġarab": 38557, "wash": 38558, "ĠBardzo": 38559, "Ġugh": 38560, "esters": 38561, "ophren": 38562, "Ġalimentos": 38563, "ĠUz": 38564, "ÖĤ": 38565, "Ġ650": 38566, "ĠпÑĢиеÑħ": 38567, "FI": 38568, "Ġsampai": 38569, "Ġparlé": 38570, "hesion": 38571, "Ġsır": 38572, "Ġapparatus": 38573, "Ġcorrelated": 38574, "ĠPrincipal": 38575, "Ġcorr": 38576, "ĠOfficial": 38577, "иÑĩеÑģкие": 38578, "Ġterminals": 38579, "Should": 38580, "Ġvacun": 38581, "Ġstellt": 38582, "Ġmooi": 38583, "etzung": 38584, "ĠкÑĢа": 38585, "Ġdai": 38586, "Ġпож": 38587, "Team": 38588, "ĠPPE": 38589, "ĠÐŀÑģ": 38590, "ĠLeah": 38591, "ĠIvy": 38592, "yst": 38593, "Ġuhhh": 38594, "Ġnighttime": 38595, "Ġtrendy": 38596, "Ġsecurities": 38597, "Ġcontinents": 38598, "Ġfirsthand": 38599, "ĠVeron": 38600, "ĠëĤ®": 38601, "Ġbrowsing": 38602, "ĠCada": 38603, "tro": 38604, "Ġtramp": 38605, "reib": 38606, "Ġerstmal": 38607, "irler": 38608, "Ġpsic": 38609, "Ġgetir": 38610, "ĠNP": 38611, "Ġdzieci": 38612, "обÑĢаз": 38613, "Ġmagician": 38614, "Ġscrutiny": 38615, "Ġslab": 38616, "ĠOT": 38617, "isty": 38618, "iries": 38619, "orest": 38620, "Ġtasked": 38621, "Ġmorally": 38622, "ìķ¼ì§Ģ": 38623, "ustered": 38624, "Ġfools": 38625, "Ġirrespons": 38626, "Ġeinf": 38627, "Ġviá»ĩc": 38628, "Ġscor": 38629, "Ġpillows": 38630, "ĠGegen": 38631, "Ġtutte": 38632, "Ġquarterly": 38633, "Ġdidnt": 38634, "ĠGym": 38635, "ĠEther": 38636, "ĠØ«": 38637, "лиÑĪком": 38638, "Ġsignaling": 38639, "ĠNode": 38640, "ĠDoncs": 38641, "Ġyah": 38642, "ĠKanal": 38643, "Ġfading": 38644, "etin": 38645, "Ġinfluencers": 38646, "Ġmedals": 38647, "Ġengineered": 38648, "Ġfermented": 38649, "ê²łì§Ģë§Į": 38650, "ĠBeethoven": 38651, "×ŀש": 38652, "inental": 38653, "ĠìķĮ볤": 38654, "ütfen": 38655, "alnya": 38656, "Ġovere": 38657, "Ġdenkt": 38658, "акÑĤеÑĢ": 38659, "Ġâĺ": 38660, "Ġnecesit": 38661, "Ġgenerators": 38662, "grass": 38663, "ĠподÑĥм": 38664, "lieÃŁen": 38665, "Bar": 38666, "ľëıĻ": 38667, "ĠдеÑĤей": 38668, "Ġsucking": 38669, "Ġstencil": 38670, "Ġprimo": 38671, "ĠBreath": 38672, "strom": 38673, "Ġimmensely": 38674, "Ġappreh": 38675, "ìłķìĿ´": 38676, "Pop": 38677, "Ġjong": 38678, "ĠGiul": 38679, "ĠADHD": 38680, "Ġhören": 38681, "Ġelo": 38682, "ivent": 38683, "Ġrus": 38684, "Ġoutrageous": 38685, "Ġmastered": 38686, "Ġ커": 38687, "ÙĪÙģ": 38688, "ipes": 38689, "ĠRudy": 38690, "Jacob": 38691, "Ġbullish": 38692, "Ġtapped": 38693, "Ġfaud": 38694, "izophren": 38695, "ĠÑģоÑħ": 38696, "ĠDarling": 38697, "Ġ1963": 38698, "ĠPrevention": 38699, "²Ķ": 38700, "Ġabdominal": 38701, "stones": 38702, "Ġavaient": 38703, "á»ķi": 38704, "make": 38705, "Ġsare": 38706, "ĠInstant": 38707, "кам": 38708, "Ġkeeper": 38709, "Ġblankets": 38710, "ãģ§ãģĹãĤĩãģĨ": 38711, "Ġsweats": 38712, "ĠMinneapolis": 38713, "åħ¨éĥ¨": 38714, "Ġgenommen": 38715, "Ġfasten": 38716, "ĠBrussels": 38717, "åij¼": 38718, "Ġcafeter": 38719, "Ġabsorbing": 38720, "Ġhago": 38721, "ĠElmo": 38722, "Ġgusto": 38723, "ĠYap": 38724, "Música": 38725, "Ġtert": 38726, "Ġbanda": 38727, "Ġmily": 38728, "Ġthereafter": 38729, "ĠStockholm": 38730, "ĠCarson": 38731, "Ġcalibration": 38732, "avaÅŁ": 38733, "ansa": 38734, "ikke": 38735, "Ġforesee": 38736, "Ġqualche": 38737, "Ġdeste": 38738, "æ¤": 38739, "ünüz": 38740, "Ġforge": 38741, "Dis": 38742, "esten": 38743, "Ġδια": 38744, "Ġencaps": 38745, "ĠGespr": 38746, "Ġchercher": 38747, "ickets": 38748, "ÑĤоÑĢÑĭ": 38749, "Cr": 38750, "ĠТакже": 38751, "Ġrabbits": 38752, "ĠDot": 38753, "heiten": 38754, "Ġcausal": 38755, "ĠFoster": 38756, "ajÄħc": 38757, "Ġbereit": 38758, "Ġayudar": 38759, "é«Ļ": 38760, "ãģ³": 38761, "song": 38762, "comb": 38763, "Ġfringe": 38764, "Ġcybersecurity": 38765, "Ġ뾨": 38766, "Ġkier": 38767, "Ġbeschäft": 38768, "ĠконÑĨе": 38769, "Ġfacilit": 38770, "ĠNamen": 38771, "Ġbilateral": 38772, "tx": 38773, "ĠWissenschaft": 38774, "Ġnuances": 38775, "Ġripping": 38776, "Ġfy": 38777, "ĠSicherheit": 38778, "ĠGhana": 38779, "olon": 38780, "Ġtopped": 38781, "ĠMorocco": 38782, "Ġradial": 38783, "ĠLEE": 38784, "ĠAndreas": 38785, "edd": 38786, "ĠìĹ´ë": 38787, "ĠAirlines": 38788, "ãģĵãĤį": 38789, "Ġvalores": 38790, "ê·ľ": 38791, "Hy": 38792, "ĠзадаÑĩ": 38793, "ĠKendall": 38794, "ĠÑħаÑĢ": 38795, "ĠVamp": 38796, "Ġpython": 38797, "Ġmanageable": 38798, "ĠGente": 38799, "oise": 38800, "iciary": 38801, "Ġimposs": 38802, "ĠBunny": 38803, "iesta": 38804, "Andrew": 38805, "Ġsert": 38806, "ĠCec": 38807, "zzarella": 38808, "Ġautomobile": 38809, "ĠTiere": 38810, "allows": 38811, "åĨĨ": 38812, "Ġë°Ģ": 38813, "ĠScorp": 38814, "ĠJelly": 38815, "agara": 38816, "ĠStretch": 38817, "Ġredef": 38818, "Ġexacerb": 38819, "ĠSHA": 38820, "éf": 38821, "orsa": 38822, "Ġflawed": 38823, "ĠNoel": 38824, "?!?": 38825, "Ġprocent": 38826, "Ġmenstru": 38827, "ĠпÑĢоÑĩ": 38828, "Ġinfants": 38829, "ðŁİµ": 38830, "pause": 38831, "ĠRacing": 38832, "Ġ1948": 38833, "Ġsuperintendent": 38834, "idores": 38835, "idy": 38836, "brahim": 38837, "Ġunlucky": 38838, "Ġperk": 38839, "anci": 38840, "Ġë§ĮëĤĺ": 38841, "ĠÐľÐ¾Ñģкв": 38842, "Ġfinans": 38843, "Ġdiferencia": 38844, "łĪìĿ´": 38845, "éħį": 38846, "ORY": 38847, "ĠTac": 38848, "ÛĮا": 38849, "Ġdesem": 38850, "Ġважно": 38851, "ĠJU": 38852, "ĠìŀĪìŀĸìķĦìļĶ": 38853, "ĠÎĿ": 38854, "Ġinformations": 38855, "ĠHEL": 38856, "hst": 38857, "ĠпоговоÑĢ": 38858, "Ġvoiture": 38859, "Ġreus": 38860, "ändig": 38861, "ĠпоÑħож": 38862, "jing": 38863, "Ġdru": 38864, "altra": 38865, "Ġproduits": 38866, "Ġkite": 38867, "Ġeyeball": 38868, "ĠBelt": 38869, "ĠRestaurant": 38870, "Ġgamb": 38871, "Ġporridge": 38872, "itters": 38873, "Ġconverts": 38874, "Ġyardım": 38875, "Ġmáximo": 38876, "wirtschaft": 38877, "ĠíķĺëĤĺë": 38878, "Ġì¤Ģ": 38879, "Ġiceberg": 38880, "Ġvorbei": 38881, "Ġ256": 38882, "ocratic": 38883, "Ġreckless": 38884, "onner": 38885, "Ġmús": 38886, "Ġlogically": 38887, "ĠPrison": 38888, "ĠNetz": 38889, "Ġvacant": 38890, "Ġnimmt": 38891, "ĠHARR": 38892, "Ġзов": 38893, "ĠDee": 38894, "ringe": 38895, "niest": 38896, "ĠRules": 38897, "ìĬ¤ëٽ": 38898, "cussions": 38899, "Ġfloral": 38900, "Ġconstrained": 38901, "Ġdifferentiation": 38902, "ĠQuebec": 38903, "ĠÛģÛĮÚº": 38904, "Ġpública": 38905, "itel": 38906, "Ġaccommodations": 38907, "ĠGrü": 38908, "íľ": 38909, "Ġpickles": 38910, "иÑĩеÑģкиÑħ": 38911, "Ġcommissions": 38912, "ĠBaek": 38913, "ĠçocuÄŁ": 38914, "ĠMedium": 38915, "Ġperiodically": 38916, "Ġwonderfully": 38917, "Ġstaffing": 38918, "ìĽIJë": 38919, "rire": 38920, "fle": 38921, "ĠMcL": 38922, "ĠÑĤеп": 38923, "ĠпеÑĢек": 38924, "нолог": 38925, "Ġíģ¬ê²Į": 38926, "çϼçı¾": 38927, "Ġprosperous": 38928, "ĠSpiritual": 38929, "ĠChick": 38930, "DIA": 38931, "ĠÐŁÑĢивеÑĤ": 38932, "ĠperÃŃ": 38933, "ÑĮÑİÑĤ": 38934, "Ġconsultants": 38935, "ĠEarl": 38936, "ä»Ĭå¹´": 38937, "Ġruining": 38938, "оÑĢе": 38939, "Ġpenser": 38940, "Ġtakiej": 38941, "Ġstrengthened": 38942, "ĠLiquid": 38943, "онеÑĨ": 38944, "аваÑĤÑĮ": 38945, "Ġcamer": 38946, "Ġdisagreement": 38947, "Ġbathing": 38948, "ĠYosh": 38949, "aal": 38950, "prechen": 38951, "RISADAS": 38952, "Ġsuperstar": 38953, "æģŃ": 38954, "лÑıÑĤÑĮ": 38955, "Ġnib": 38956, "ĠTherm": 38957, "ĠDANIEL": 38958, "Ġpaw": 38959, "Ġliquids": 38960, "Ġcapacit": 38961, "arken": 38962, "Ġvagina": 38963, "Ġmashed": 38964, "Ġemerges": 38965, "yscy": 38966, "Ġunrelated": 38967, "ĠGuild": 38968, "Ġinverted": 38969, "itives": 38970, "Tra": 38971, "Ġbegr": 38972, "Ġalte": 38973, "ì§ķ": 38974, "ãĤģãģ¦": 38975, "ĠÑĢазÑĢабоÑĤ": 38976, "finder": 38977, "Ġдалее": 38978, "ĠблагодаÑĢ": 38979, "walker": 38980, "Ġcrater": 38981, "assadors": 38982, "rences": 38983, "inski": 38984, "ĠKIM": 38985, "ĠElliot": 38986, "2017": 38987, "ĠSr": 38988, "inka": 38989, "anov": 38990, "Ġìŀĺ못": 38991, "Ġproprietary": 38992, "displaystyle": 38993, "ĠÑģим": 38994, "Ġизб": 38995, "ĠPanel": 38996, "Ġinstincts": 38997, "ĠCommunications": 38998, "麻": 38999, "midt": 39000, "Ġë§Įëĵ¤ìĸ´": 39001, "ĠÑģлова": 39002, "ĠGilbert": 39003, "缮åīį": 39004, "Так": 39005, "voorbeeld": 39006, "еÑİÑģÑĮ": 39007, "aryn": 39008, "quez": 39009, "Ġdart": 39010, "ÑĸÑĪ": 39011, "ĠHut": 39012, "Sal": 39013, "Ġsoutheast": 39014, "Ġpesticides": 39015, "Ġhelicopters": 39016, "Ġendured": 39017, "iada": 39018, "Ġbrewing": 39019, "ìŬë": 39020, "ĠÑģвобод": 39021, "ĠSaints": 39022, "ĠFrançais": 39023, "ĠEconomics": 39024, "Ġdisloc": 39025, "ophobia": 39026, "Camer": 39027, "Ġnegotiated": 39028, "ĠÑģÑĤали": 39029, "ìĬ¤íģ": 39030, "ogie": 39031, "Ġtsunami": 39032, "Ġpeeled": 39033, "Ġmotivations": 39034, "è¨Ń": 39035, "ostat": 39036, "flan": 39037, "ĠDAC": 39038, "Ġkav": 39039, "'RE": 39040, "ĠPearson": 39041, "bbe": 39042, "czenie": 39043, "Ġatenção": 39044, "íĨµëł¹": 39045, "ãģ£ãģ¡": 39046, "ĠÑĥдаÑĢ": 39047, "Ġintroductory": 39048, "ĠIci": 39049, "ëĮĢë": 39050, "akat": 39051, "Ġtrench": 39052, "Ġproceeded": 39053, "ĠCoin": 39054, "Ġderecho": 39055, "ĠRede": 39056, "æ¯Ľ": 39057, "аннÑĭй": 39058, "Ġincarcerated": 39059, "ĠRichmond": 39060, "Rock": 39061, "ĠPav": 39062, "ĠKarma": 39063, "uges": 39064, "Ġconteú": 39065, "ë¹Ħ": 39066, "Ġê·¸ë§Į": 39067, "ĠGone": 39068, "ĠwspóÅĤ": 39069, "ĠRahmen": 39070, "unken": 39071, "Ġì¤ijìļĶíķľ": 39072, "Ġib": 39073, "Ġattaching": 39074, "Hay": 39075, "Ġsuka": 39076, "ìį¹": 39077, "Ġpivotal": 39078, "ĠRespect": 39079, "ÃŃda": 39080, "IB": 39081, "ĠVerantwort": 39082, "wiet": 39083, "Ġforensic": 39084, "ÑĢиÑģÑĤ": 39085, "ĠпÑĢинÑĨипе": 39086, "Ġmarkings": 39087, "Ġkettle": 39088, "ĠOpera": 39089, "ĠDoctors": 39090, "Ġshredded": 39091, "Ġrecuer": 39092, "Ġvigil": 39093, "ĠFail": 39094, "Ġentrev": 39095, "ĠдÑĥÑĪ": 39096, "Ġoutbreaks": 39097, "èµ°åIJ§": 39098, "ĠÏĢο": 39099, "Ġrogue": 39100, "angled": 39101, "Ġyearly": 39102, "ĠCreed": 39103, "Ġwam": 39104, "Ġlotus": 39105, "ê³¼ë": 39106, "ãĢģãĢģ": 39107, "ĠSpit": 39108, "ĠItu": 39109, "Ġstrains": 39110, "Ġstamped": 39111, "Ġplaint": 39112, "Ġpotion": 39113, "Ġconsolidation": 39114, "è©ķ": 39115, "оÑĩкÑĥ": 39116, "Ġvlogging": 39117, "Ġslate": 39118, "ĠAuft": 39119, "ĠIncor": 39120, "ừng": 39121, "§IJ": 39122, "enh": 39123, "ĠheiÃŁ": 39124, "Ġdomest": 39125, "ĠStrom": 39126, "åį³": 39127, "akis": 39128, "Ġfragen": 39129, "Ġfiner": 39130, "ĠSug": 39131, "Ġuphill": 39132, "Ġéén": 39133, "â̦)": 39134, "ĠÑģоп": 39135, "ĠCorey": 39136, "Ġsiebie": 39137, "Ġmuse": 39138, "Ġcloves": 39139, "Ġpous": 39140, "ĠFinanz": 39141, "ĠRoute": 39142, "amat": 39143, "Ġmutually": 39144, "ĠвнÑĥÑĤÑĢи": 39145, "ĠSelena": 39146, "ëĶ": 39147, "ĠGaussian": 39148, "ë¶ĢíĦ°": 39149, "Ġ×ij׼": 39150, "Ġejerc": 39151, "å¾®": 39152, "kea": 39153, "ĠGerry": 39154, "ĠSic": 39155, "大çļĦ": 39156, "Ġ1966": 39157, "iese": 39158, "Ġfossils": 39159, "Ġestad": 39160, "ĠKane": 39161, "ciÄĩ": 39162, "ĠìľłíĬľë": 39163, "Ġпам": 39164, "ĠCruise": 39165, "intérieur": 39166, "Ġbekannt": 39167, "ĠPode": 39168, "Ġdemander": 39169, "Rem": 39170, "Ġinvade": 39171, "Ġdecorating": 39172, "ropic": 39173, "Ġcowboy": 39174, "ĠPhoto": 39175, "opolit": 39176, "Ġì»¬ëŁ¬ë": 39177, "Ġreap": 39178, "Ġhandwriting": 39179, "à¹Ħร": 39180, "Ġëļ": 39181, "Ġبعد": 39182, "ĠMt": 39183, "ÙĢ": 39184, "Ġspaceship": 39185, "Ġnationalism": 39186, "Ġcouncils": 39187, "ĠGriffin": 39188, "ĠAhmed": 39189, "Ġclich": 39190, "ĠOL": 39191, "wl": 39192, "ĠPilot": 39193, "å®®": 39194, "Ġacronym": 39195, "Ġgels": 39196, "Ġelectroly": 39197, "èĵ": 39198, "Ġмной": 39199, "Ġepisod": 39200, "ĠDieses": 39201, "ĠATP": 39202, "Ġediyorum": 39203, "Ġexpresses": 39204, "Ġexhibits": 39205, "Comm": 39206, "ĠкÑĢÑĥп": 39207, "Ġmatar": 39208, "Ġ2025": 39209, "ĠArtem": 39210, "vasive": 39211, "rÃł": 39212, "ĠbeÅŁ": 39213, "é»ĥ": 39214, "Ġlizard": 39215, "Ġfille": 39216, "Ġì§Ī문": 39217, "ĠмоÑī": 39218, "Ġtür": 39219, "Ġculprit": 39220, "Ġwoven": 39221, "ĠANY": 39222, "nim": 39223, "Ġtay": 39224, "Ġpromin": 39225, "Ġacompa": 39226, "Ġidé": 39227, "Ġboiler": 39228, "ĠThemen": 39229, "Ġavenue": 39230, "ĠMud": 39231, "ĠновÑĭе": 39232, "Ġwitnessing": 39233, "Ġlance": 39234, "ĠCHAN": 39235, "ĠBever": 39236, "تÙħ": 39237, "Ġchemotherapy": 39238, "King": 39239, "ĠbÄĻdÄĻ": 39240, "Ġatual": 39241, "Ġtive": 39242, "Ġtalkin": 39243, "Ġquedar": 39244, "ieÃŁ": 39245, "edel": 39246, "Ġìĸ´ìłľ": 39247, "Ġjogar": 39248, "Ġör": 39249, "Ġundertaking": 39250, "ĠStrength": 39251, "Ġmilhões": 39252, "ĠWine": 39253, "ĠMolt": 39254, "讲": 39255, "ãģijãĤĮ": 39256, "Ġundermine": 39257, "ĠArchives": 39258, "vana": 39259, "mercial": 39260, "MC": 39261, "Ġcaste": 39262, "пÑĢ": 39263, "Ġlegislators": 39264, "ulators": 39265, "ênio": 39266, "Ġëį°ë": 39267, "ĠÑħоÑĤиÑĤе": 39268, "Ġнек": 39269, "Ġsurn": 39270, "Ġconsci": 39271, "ĠPOW": 39272, "Ġculinary": 39273, "ĠKAT": 39274, "ĠFolks": 39275, "Ñĭваем": 39276, "Ġвок": 39277, "ãģijãĤĭ": 39278, "service": 39279, "pts": 39280, "Ġпобед": 39281, "æĺ¯åķĬ": 39282, "Ġtents": 39283, "Ġnord": 39284, "STE": 39285, "Ġrepublican": 39286, "Ġwyk": 39287, "Ġminions": 39288, "èĻķ": 39289, "Ġmemang": 39290, "jest": 39291, "Ġcomparative": 39292, "Ġtyle": 39293, "carbon": 39294, "bedingt": 39295, "ksen": 39296, "Ġnegativity": 39297, "Ġsjälv": 39298, "Ġdú": 39299, "æīĢæľī": 39300, "Ġrecalled": 39301, "cra": 39302, "ĠTada": 39303, "ĠÑĢÑĥки": 39304, "ĠопÑĢедел": 39305, "Ġprocrast": 39306, "Ġjogos": 39307, "ĠOo": 39308, "ĠHearts": 39309, "Ġéch": 39310, "ĠksiÄħż": 39311, "Ġcoarse": 39312, "ĠTube": 39313, "ĠGreens": 39314, "Ġén": 39315, "Ġdumbbell": 39316, "ĠÑĤи": 39317, "Ġquerer": 39318, "اØŃ": 39319, "Ïĥει": 39320, "ĠпÑĢавилÑĮно": 39321, "Ġпап": 39322, "Ġcompra": 39323, "Ġtér": 39324, "ĠAntes": 39325, "Ġoptimum": 39326, "Ġbiscuit": 39327, "κι": 39328, "aczego": 39329, "Ġìĭľê°ĦìĿ´": 39330, "ĠMarines": 39331, "vero": 39332, "Ġvaccinations": 39333, "Ġpetty": 39334, "riters": 39335, "Ġал": 39336, "country": 39337, "Ġcounters": 39338, "Ġattendant": 39339, "ĠHui": 39340, "ãģ¨ãģĦãģĨãģĵãģ¨ãģ§": 39341, "cka": 39342, "ÑģÑĤвеннÑĭй": 39343, "guy": 39344, "Ġtricked": 39345, "ĠRED": 39346, "Ġthrilling": 39347, "ÏĢοι": 39348, "Ġpiggy": 39349, "Ġanunci": 39350, "ORTER": 39351, "ĠValue": 39352, "Ġrond": 39353, "ĠADA": 39354, "Ġposer": 39355, "hores": 39356, "ĠRoland": 39357, "ĵ¯": 39358, "Ġnoir": 39359, "Ġש×IJ×": 39360, "ë°ľ": 39361, "iemand": 39362, "ĠпоÑĤеÑĢ": 39363, "ê³³": 39364, "Ġê±±": 39365, "Ġformatting": 39366, "ĠLed": 39367, "è§Ģçľ¾": 39368, "Ġkillers": 39369, "ĠÄijấy": 39370, "Ġhaar": 39371, "again": 39372, "!</": 39373, "Ġsomethin": 39374, "Ġcoughing": 39375, "Ġnave": 39376, "Ġprospective": 39377, "ĠHK": 39378, "ĠRescue": 39379, "maybe": 39380, "gger": 39381, "ĠÑĢабоÑĤÑĥ": 39382, "×ķ׾×Ŀ": 39383, "tails": 39384, "íķĺíķĺ": 39385, "Ġeyelid": 39386, "Ġcustomization": 39387, "avilion": 39388, "Ġprochain": 39389, "Ġglaze": 39390, "æĥħæ³ģ": 39391, "Sim": 39392, "ĠопаÑģ": 39393, "Ġmosquitoes": 39394, "Ġfent": 39395, "Ġcapacities": 39396, "Ġapostles": 39397, "Ġaltura": 39398, "Ġ묻": 39399, "Ġseront": 39400, "ĠAnytime": 39401, "¥´ëĬĶ": 39402, "Ġcosplay": 39403, "Ġspac": 39404, "Ġsamen": 39405, "ãĥĦ": 39406, "ucc": 39407, "ières": 39408, "Ġsibling": 39409, "ĠCock": 39410, "Ġëıħ": 39411, "ĠпÑĢедÑģÑĤавлÑı": 39412, "Ġinstallment": 39413, "Ġdije": 39414, "ĠMCU": 39415, "ĠEH": 39416, "ĠNing": 39417, "Ġprepares": 39418, "Ġhypocr": 39419, "pty": 39420, "Ġkadın": 39421, "ĠFrozen": 39422, "haul": 39423, "ĠKylie": 39424, "éĢĻæ¨£çļĦ": 39425, "Ġshuffle": 39426, "Ġelemental": 39427, "ĠauÃŁer": 39428, "ĠKNOW": 39429, "ĠALISSA": 39430, "ZA": 39431, "ì²ł": 39432, "ç¾İåħĥ": 39433, "Ġrecite": 39434, "Ġscrib": 39435, "Ġ115": 39436, "ä¼ij": 39437, "Ġstarred": 39438, "Ġlequel": 39439, "Ġbrewer": 39440, "ĠOpportun": 39441, "Ġrä": 39442, "Ġchopsticks": 39443, "ĠKah": 39444, "ĠEthiopia": 39445, "Ġhandmade": 39446, "Ġerfolg": 39447, "ĠDz": 39448, "ittens": 39449, "èªįçĤº": 39450, "вал": 39451, "ην": 39452, "åĬŀ": 39453, "ãĥĵ": 39454, "bringen": 39455, "Ġunplug": 39456, "Ġoffs": 39457, "Ġherman": 39458, "lied": 39459, "asonic": 39460, "ĠSerbia": 39461, "ĠGuatem": 39462, "Ġ...\"": 39463, "Ġerreichen": 39464, "Ġambiguous": 39465, "ĠWhitney": 39466, "zuf": 39467, "MAND": 39468, "łµ": 39469, "Ġsqueezed": 39470, "ãģĿãģĨãģł": 39471, "yas": 39472, "é¾į": 39473, "ĠShock": 39474, "Ġutilise": 39475, "uko": 39476, "bolt": 39477, "Ġmotif": 39478, "Ġinmates": 39479, "Ġcorrupted": 39480, "Ġconcret": 39481, "ĠCritical": 39482, "ĠSinging": 39483, "ĠÑĦÑĥнк": 39484, "éŃĶ": 39485, "nova": 39486, "rebbe": 39487, "dt": 39488, "Unis": 39489, "Ġwebcam": 39490, "Ġcamoufl": 39491, "Ken": 39492, "Ġlawsuits": 39493, "ĠConsumer": 39494, "Ġrecoll": 39495, "Ġkleiner": 39496, "ĠFIFA": 39497, "Ġ1962": 39498, "èѦ": 39499, "Ġmalad": 39500, "Ġì°½": 39501, "ĠÃ¥t": 39502, "Ġinfluencer": 39503, "ĠArtist": 39504, "sti": 39505, "ãģªãĤĭãģ»ãģ©": 39506, "วย": 39507, "ysÅĤ": 39508, "ĠBian": 39509, "ĪëĦ¤": 39510, "Ġfireplace": 39511, "ĠApplication": 39512, "Ġmniej": 39513, "Ġacidic": 39514, "ĠMormon": 39515, "ssa": 39516, "åĭĻ": 39517, "Ġsneaky": 39518, "Ġojos": 39519, "Ġvoud": 39520, "ĠDai": 39521, "Ġgrassroots": 39522, "ĠUnbelievable": 39523, "ĠGabe": 39524, "ĠExtreme": 39525, "Ġhassle": 39526, "Ġcob": 39527, "mumbling": 39528, "Pass": 39529, "Į룬": 39530, "Ġsystematically": 39531, "Ġseventeen": 39532, "ÏĢει": 39533, "âĻ¡": 39534, "ĠкоÑĤ": 39535, "Ġsendiri": 39536, "Ġbathrooms": 39537, "ĠStern": 39538, "ĠArduino": 39539, "è¹": 39540, "cribing": 39541, "Ġreopening": 39542, "Ġcerv": 39543, "pee": 39544, "ARI": 39545, "Ġcadre": 39546, "ĠAnch": 39547, "Lee": 39548, "ĠMAX": 39549, "Ġmänn": 39550, "Ġchores": 39551, "Ġadesso": 39552, "æĿij": 39553, "ĠNig": 39554, "Ġdissertation": 39555, "ĠVay": 39556, "STALK": 39557, "ака": 39558, "avat": 39559, "çł´": 39560, "Ġpunkt": 39561, "Ġpadding": 39562, "ĠTempl": 39563, "Ġeje": 39564, "ĠíĦ°": 39565, "Ġazt": 39566, "ĠëĮĢíĨµëł¹": 39567, "Ġrearrange": 39568, "ách": 39569, "ĠìĤ¬ëŀĮëĵ¤": 39570, "Ġfreakin": 39571, "crire": 39572, "Ġ커ë": 39573, "ĠExplain": 39574, "ĠÏĦÏīν": 39575, "Ġbodily": 39576, "ĠLeist": 39577, "Ġsigui": 39578, "Ġbunker": 39579, "Ġazul": 39580, "ĠHaush": 39581, "Sub": 39582, "ĠÐIJнд": 39583, "ĠкÑĢай": 39584, "Ġillegally": 39585, "ĠMuy": 39586, "ĠFei": 39587, "ĠBanana": 39588, "Ġscholarly": 39589, "ĠPrzy": 39590, "ĠMoss": 39591, "ĠFilter": 39592, "Ġìĸ´ëĸ¡": 39593, "ĠMaxwell": 39594, "tense": 39595, "Ġlongitud": 39596, "Ġlangsam": 39597, "Ġ×ŀ×§": 39598, "smith": 39599, "izada": 39600, "ĠноÑĢмалÑĮно": 39601, "ĠVoll": 39602, "ĠElena": 39603, "æĸ¹éĿ¢": 39604, "ĠÑħоÑĤÑĮ": 39605, "ĠDabei": 39606, "Ġconservatives": 39607, "Ġprópria": 39608, "ĠDieser": 39609, "ĠBrenda": 39610, "ookie": 39611, "Ġbanc": 39612, "ãĥ¯": 39613, "ìĿ´ì¦": 39614, "ìĽĥìĿĮ": 39615, "Ġkeh": 39616, "Ġweddings": 39617, "Ġthunderstorm": 39618, "æĶ¾å¿ĥ": 39619, "ĠCoordin": 39620, "ìĪĺê°Ģ": 39621, "Ġprzeci": 39622, "éĴ±": 39623, "OSSTALK": 39624, "maan": 39625, "Ġê±´ë": 39626, "ĠبÙĩ": 39627, "Ġżad": 39628, "Ġyacht": 39629, "Ġgöt": 39630, "Ġbleach": 39631, "Ġshorten": 39632, "ĠÑģÑĤало": 39633, "usan": 39634, "ĠìŀIJìŰ": 39635, "Ġders": 39636, "xis": 39637, "įĶëĭĪ": 39638, "Ġquantidade": 39639, "Ġoppressed": 39640, "ĠзаконÑĩ": 39641, "ä¸Ī夫": 39642, "ãģĪãģĪ": 39643, "ĠÑĩеÑĤÑĭ": 39644, "ĠÐĿапÑĢимеÑĢ": 39645, "ulp": 39646, "æĢĸ": 39647, "ÙĤÙĪÙĦ": 39648, "оÑĩе": 39649, "άλ": 39650, "zeniu": 39651, "Ġformations": 39652, "Ġsparked": 39653, "ĠEntwicklung": 39654, "alls": 39655, "Ġvivir": 39656, "Ġexpiration": 39657, "otine": 39658, "ĠЧеÑĢ": 39659, "ĠTurning": 39660, "Ġtariffs": 39661, "ĠnastÄĻp": 39662, "Ġabide": 39663, "iksi": 39664, "Ġflashes": 39665, "Ġdisputes": 39666, "Ġì²´": 39667, "Ġmerak": 39668, "Ġenormously": 39669, "zahl": 39670, "Ġführt": 39671, "вон": 39672, "ĠзавиÑģ": 39673, "Ġperseverance": 39674, "Ġdividends": 39675, "Ġcontestants": 39676, "ĠproszÄĻ": 39677, "ĠFranken": 39678, "ãĤįãģĨ": 39679, "Ġexplorer": 39680, "Ġbuffalo": 39681, "âĢķ": 39682, "Ġecology": 39683, "Ġscalar": 39684, "Ġcran": 39685, "εÏĦαι": 39686, "żyÄĩ": 39687, "ĠìļĶë": 39688, "Ġgia": 39689, "ĠGog": 39690, "ĠPriv": 39691, "Ġë§IJìĿĦ": 39692, "ĠReason": 39693, "raktion": 39694, "ĠDeborah": 39695, "Ġkitten": 39696, "ĠEdin": 39697, "ä¹¾": 39698, "piej": 39699, "Ġëĭ´": 39700, "Ġmáqu": 39701, "Ġbidding": 39702, "Ġaffinity": 39703, "Ġaika": 39704, "folk": 39705, "ĠConse": 39706, "Ġdeutschen": 39707, "èĨ": 39708, "Ġdebit": 39709, "ıģın": 39710, "isel": 39711, "Ġì¤ijêµŃ": 39712, "ĠëŃIJê°Ģ": 39713, "Ġtrustworthy": 39714, "ĠStarted": 39715, "æķij": 39716, "ürd": 39717, "ĠпонÑıÑĤно": 39718, "Ġscientifically": 39719, "Pods": 39720, "CROSSTALK": 39721, "Ġpreguntas": 39722, "Ġcalming": 39723, "ĠPremiere": 39724, "׼ש": 39725, "ĠÑħолод": 39726, "Ġcapita": 39727, "Ġtoma": 39728, "Ġmurm": 39729, "Ġfuerza": 39730, "ĠHani": 39731, "æĪijæľī": 39732, "üf": 39733, "arlos": 39734, "Ġhäuf": 39735, "ãģijãģ¦": 39736, "Ġosoby": 39737, "jego": 39738, "ĠпиÑģ": 39739, "Ġcalmly": 39740, "idet": 39741, "buch": 39742, "gone": 39743, "Ġviscosity": 39744, "Ġmodal": 39745, "Ġgesam": 39746, "ĠHz": 39747, "Ġmunicipalities": 39748, "Ġcirculating": 39749, "olina": 39750, "Sho": 39751, "é¢ij": 39752, "ĠBened": 39753, "olu": 39754, "Ġrests": 39755, "ĠlÃ¥ng": 39756, "ĠÐŀднако": 39757, "Ġprzew": 39758, "Ġpepp": 39759, "Ġmarriages": 39760, "ĠBIG": 39761, "andan": 39762, "Ġmagically": 39763, "Ġbabys": 39764, "ĠëĮĵ": 39765, "Ġhackers": 39766, "Baby": 39767, "ĠMonst": 39768, "Ġcier": 39769, "ĠArabs": 39770, "Ġмагаз": 39771, "ĠIndonesian": 39772, "ãģĦãģĨãģĵãģ¨": 39773, "ĠMarkt": 39774, "Ġdachte": 39775, "ĠSchüler": 39776, "ĠVND": 39777, "Ġspielt": 39778, "Ġperlu": 39779, "ãĤ´": 39780, "åŃĺ": 39781, "ĠпÑĢоÑħод": 39782, "Ġsalted": 39783, "Ġimprovis": 39784, "ĠInstr": 39785, "velmente": 39786, "Ġness": 39787, "Ġfungus": 39788, "Ġcollaborators": 39789, "ĠVirus": 39790, "estar": 39791, "Ġprojector": 39792, "ĠÐŁÑĢав": 39793, "Ġagility": 39794, "×Ļ׳×ķ": 39795, "erel": 39796, "Ġвозв": 39797, "Ġбаз": 39798, "ĠCathy": 39799, "ÄŁu": 39800, "ĠговоÑĢил": 39801, "bility": 39802, "ĠLanc": 39803, "ĠKimberly": 39804, "ĠBrief": 39805, "åħ·": 39806, "Ġutveck": 39807, "Ġgoggles": 39808, "Ġpreschool": 39809, "ç§į": 39810, "ATHER": 39811, "Ġmotives": 39812, "ĠBong": 39813, "EX": 39814, "Ġchilly": 39815, "ĠAdvisory": 39816, "âĢĭâĢĭ": 39817, "ĠкоÑĤоÑĢом": 39818, "Ġtraitor": 39819, "Ġdemasiado": 39820, "ĠÑĨен": 39821, "Ġмои": 39822, "åŀĭ": 39823, "Ġmultif": 39824, "ìͬ": 39825, "ĠAlexis": 39826, "Ġziet": 39827, "ĠRama": 39828, "brance": 39829, "Ġsanction": 39830, "itous": 39831, "×ķ×ļ": 39832, "Ġë³´ëĤ": 39833, "ÑģÑĤанов": 39834, "è¶£": 39835, "ĠÑĢеÑģ": 39836, "ĠChurchill": 39837, "ĠпÑĢез": 39838, "ĠIO": 39839, "ĠGee": 39840, "ĠGather": 39841, "atori": 39842, "Tyler": 39843, "Ġнемнож": 39844, "ĠbÃ¥de": 39845, "ĠKiller": 39846, "Ġtuber": 39847, "ĠRamadan": 39848, "á¿": 39849, "ieht": 39850, "Ġstrangely": 39851, "лÑĥ": 39852, "Ġredesign": 39853, "Ġincumb": 39854, "Ġberaber": 39855, "ĠVolkswagen": 39856, "metal": 39857, "dzy": 39858, "pción": 39859, "ĠìķĬìķĦ": 39860, "åͱ": 39861, "头": 39862, "ĠGoodness": 39863, "иваеÑĤÑģÑı": 39864, "bahn": 39865, "ĠAntarctica": 39866, "екÑĤоÑĢ": 39867, "Ġhomeowners": 39868, "zeigt": 39869, "ĠíĺĦìŀ¬": 39870, "ì§ĢëıĦ": 39871, "Ġgeographical": 39872, "thinking": 39873, "Ġgosta": 39874, "ĠImam": 39875, "uliflower": 39876, "dag": 39877, "annt": 39878, "akov": 39879, "Ġdownwards": 39880, "ì²´ê°Ģ": 39881, "CUBE": 39882, "ĠÐļÑģÑĤаÑĤи": 39883, "Ġполов": 39884, "Ġplateau": 39885, "ãģĦãģį": 39886, "ḥ": 39887, "Ġchlorine": 39888, "Ġaccelerator": 39889, "Ġsolves": 39890, "ĠGrass": 39891, "piano": 39892, "Ġکا": 39893, "Ġبت": 39894, "ĠRochester": 39895, "ĠÙĩÙĬ": 39896, "Ġcollects": 39897, "įĶëĿ¼": 39898, "ĠCheer": 39899, "lingen": 39900, "ĠÑĢазг": 39901, "Ġaméric": 39902, "hta": 39903, "ECT": 39904, "Ġartific": 39905, "ĠPayPal": 39906, "hana": 39907, "Stephen": 39908, "ĠGest": 39909, "phalt": 39910, "Ġreplication": 39911, "ĠWillie": 39912, "Ġneutr": 39913, "Ġirrational": 39914, "Ġdados": 39915, "ĠAid": 39916, "kam": 39917, "anter": 39918, "ĠдÑĥже": 39919, "Ġdeton": 39920, "Ġhare": 39921, "Ġbets": 39922, "bagai": 39923, "Ġstained": 39924, "Ġplausible": 39925, "Ġpeeling": 39926, "ĠcrÃŃt": 39927, "Ġgrote": 39928, "ì¶°": 39929, "¥´ê²Į": 39930, "altet": 39931, "Phone": 39932, "Fil": 39933, "SQL": 39934, "Ġgefallen": 39935, "åıĶ": 39936, "Ġsaúde": 39937, "ĠTamil": 39938, "cous": 39939, "Ġглавное": 39940, "Ġatravés": 39941, "ussia": 39942, "Ġzweiten": 39943, "ĠElvis": 39944, "Ġmover": 39945, "Ġlimite": 39946, "追": 39947, "arez": 39948, "¥´ê³ł": 39949, "ĠKranken": 39950, "üre": 39951, "ĠìķĬìķĦìļĶ": 39952, "ĠthÃłnh": 39953, "Ġprofoundly": 39954, "Ġbedrooms": 39955, "Ġtoothpaste": 39956, "ĠAccept": 39957, "ético": 39958, "Ġküç": 39959, "ĠAry": 39960, "adin": 39961, "Ġgranular": 39962, "ected": 39963, "Ġmenjadi": 39964, "Ġcompetence": 39965, "doc": 39966, "Ġsparkling": 39967, "Ġì¢ĭìĿĦ": 39968, "Ġconstructing": 39969, "Ġamusement": 39970, "ĠInsurance": 39971, "ĠFeuer": 39972, "Ġrenovation": 39973, "such": 39974, "plat": 39975, "Ġprosth": 39976, "Ġbey": 39977, "ĠCompletely": 39978, "Ġzod": 39979, "aln": 39980, "Vict": 39981, "Ġconfirms": 39982, "ätz": 39983, "âĸ": 39984, "hammer": 39985, "ĠзнаеÑĤ": 39986, "Ġadmired": 39987, "łë¥¼": 39988, "ĠFruit": 39989, "erten": 39990, "Ġniece": 39991, "ĠTiny": 39992, "Ġplumbing": 39993, "erma": 39994, "Ġлегко": 39995, "Ġwindshield": 39996, "ĠÑģмеÑĢ": 39997, "Ġbzw": 39998, "Ġabolition": 39999, "ĠSadhguru": 40000, "Ġpreached": 40001, "ĠCreating": 40002, "çīĽ": 40003, "pered": 40004, "Ġvolont": 40005, "Ġquint": 40006, "Ġprinters": 40007, "Ġnegro": 40008, "Ġgrosse": 40009, "ĠThy": 40010, "ĠFellows": 40011, "æİ¥ä¸ĭä¾Ĩ": 40012, "Ġstanie": 40013, "Ġnewcom": 40014, "ĠHue": 40015, "ĠFreunde": 40016, "ĠConstruction": 40017, "Ġadversity": 40018, "Ġnegatives": 40019, "Ġhazardous": 40020, "Ġcompelled": 40021, "Ġwok": 40022, "ĠOy": 40023, "па": 40024, "ª¨ë": 40025, "Ġrendez": 40026, "Ġoverc": 40027, "Ġweaving": 40028, "ĠидеÑĤ": 40029, "Ġprosecutors": 40030, "Ġaudiobook": 40031, "Ġancestor": 40032, "Ġundergoing": 40033, "Ġpounding": 40034, "ãģĤãĤĬãģĮãģ¨ãģĨãģĶãģĸãģĦãģ¾ãģĻ": 40035, "ĠíĴĢ": 40036, "Ġ춤": 40037, "Ġtulee": 40038, "ĠìĹ´ì": 40039, "Ġzoals": 40040, "Ġnein": 40041, "éŃļ": 40042, "Ġoke": 40043, "ĠJoyce": 40044, "Ġnud": 40045, "Ġdiligence": 40046, "ĠLabs": 40047, "Ġvents": 40048, "Ġancestral": 40049, "หม": 40050, "ĠмÑĥжÑĩ": 40051, "Ġnomés": 40052, "表示": 40053, "wali": 40054, "qing": 40055, "ĠMultiple": 40056, "ĠConsult": 40057, "Ġistedi": 40058, "ĠDoy": 40059, "akah": 40060, "Ġdisciplined": 40061, "Ġalternating": 40062, "çĴ": 40063, "Ġverme": 40064, "ĠоÑī": 40065, "Ġtota": 40066, "ĠPrag": 40067, "Ġsworn": 40068, "Ġbeber": 40069, "ĠAufgabe": 40070, "ìļ´ë": 40071, "辦æ³ķ": 40072, "Ġyup": 40073, "Ġreclaim": 40074, "onut": 40075, "Ġaucune": 40076, "Ġamph": 40077, "ĠÅĽwie": 40078, "Ġaa": 40079, "iscover": 40080, "ĠArg": 40081, "cież": 40082, "Ġdessas": 40083, "ĠWäh": 40084, "ỹ": 40085, "Ġдавно": 40086, "Ġsilently": 40087, "arc": 40088, "ĠíĽĦë³´": 40089, "Ġtweeting": 40090, "ĠOnd": 40091, "é¡ŀ": 40092, "¦¬ë©´": 40093, "Ġbowel": 40094, "ìħ¨ìĸ´ìļĶ": 40095, "èģĬ": 40096, "OSE": 40097, "Ġpropio": 40098, "ĠKunst": 40099, "kung": 40100, "Ġdonnées": 40101, "ĠHorizon": 40102, "ĠFrog": 40103, "åĢĭ人": 40104, "Ġarist": 40105, "âl": 40106, "Ġкож": 40107, "Ġsegundos": 40108, "ĠShortly": 40109, "ĠCrowd": 40110, "iran": 40111, "ĠwÅĤaÅĽci": 40112, "ĠLac": 40113, "idente": 40114, "Ġê°ĢìŀIJ": 40115, "Ġlen": 40116, "ĠSUS": 40117, "ĠMotors": 40118, "ĠTrent": 40119, "omie": 40120, "Ġtransmitter": 40121, "ĠAssad": 40122, "Ġpsychiatric": 40123, "ĠжиÑĤÑĮ": 40124, "Ġoutlines": 40125, "Ġeffectivement": 40126, "ĠReligion": 40127, "preh": 40128, "Ġдолжна": 40129, "Ġ͡°": 40130, "ĠConservation": 40131, "Ġá»": 40132, "Ġзай": 40133, "Ġreside": 40134, "Ġcompleto": 40135, "KEN": 40136, "ĠëĤĺìĺ¤ëĬĶ": 40137, "Ġsuburban": 40138, "Ġrépondre": 40139, "ĠÑĢазлиÑĩ": 40140, "Ġgalleries": 40141, "Ġrapt": 40142, "æĦŁè¬Ŀ": 40143, ")...": 40144, "Ġcruelty": 40145, "ĠVMware": 40146, "íά": 40147, "Ġhayır": 40148, "Ġgrouping": 40149, "ĠRider": 40150, "Ġsyllable": 40151, "Ġbeispielsweise": 40152, "Ġsafeguard": 40153, "ĠpelÃŃcula": 40154, "arti": 40155, "ĠСо": 40156, "Ġchega": 40157, "ĠкомÑĥ": 40158, "Ġseism": 40159, "Ġharmless": 40160, "ĠWarriors": 40161, "ãģĦãģ¤": 40162, "ĠпÑģ": 40163, "Ġshameless": 40164, "ĠBaum": 40165, "install": 40166, "Ġtoolkit": 40167, "Ġpipelines": 40168, "Ġpussy": 40169, "Ġconceal": 40170, "Ġprotesting": 40171, "ochond": 40172, "Ġdua": 40173, "ĠPose": 40174, "Ġhelium": 40175, "ĠUX": 40176, "ikle": 40177, "ĠSuff": 40178, "ĠìĦ¸ê³Ħ": 40179, "ingers": 40180, "ĠÑģлÑĥÑĩай": 40181, "Ġdescending": 40182, "Ġæ²Ĵæľī": 40183, "Ġmontage": 40184, "High": 40185, "ĠìĿ´ìĸ": 40186, "ĠIdi": 40187, "Ġ×ijס": 40188, "Ġexpressive": 40189, "ç§ĭ": 40190, "Ġполез": 40191, "Ġpone": 40192, "Ġadolescent": 40193, "аннÑĭе": 40194, "Ġassassination": 40195, "weisen": 40196, "ematically": 40197, "auth": 40198, "Ġurg": 40199, "Ġganhar": 40200, "Ġfundo": 40201, "ĠRhode": 40202, "ĠиÑģÑĤоÑĢии": 40203, "Ġcompartil": 40204, "æķ¢": 40205, "Ġdiminished": 40206, "Ġapprentice": 40207, "ĠÐijÑĥд": 40208, "Ġphotons": 40209, "Ġcód": 40210, "å¹ķ": 40211, "æ¬Ĭ": 40212, "onak": 40213, "Ġadelante": 40214, "Ġchu": 40215, "opic": 40216, "ĠaixÃŃ": 40217, "eddar": 40218, "ĠCongrats": 40219, "mor": 40220, "好åIJ§": 40221, "Ġreservations": 40222, "ĠToby": 40223, "ĠKern": 40224, "Ġrazem": 40225, "Ġforged": 40226, "Ġhorrifying": 40227, "ÙĬع": 40228, "ĠJoining": 40229, "ãĥ©ãĤ¤": 40230, "ĠAuth": 40231, "dah": 40232, "Ġconsig": 40233, "Ġintimidated": 40234, "Ġperipheral": 40235, "Ġmeno": 40236, "Ġdetecting": 40237, "Ġteor": 40238, "Ġtagged": 40239, "Ġnostalgic": 40240, "Ġ미ìķĪ": 40241, "å̼": 40242, "Ġverdi": 40243, "Ġlabeling": 40244, "под": 40245, "astes": 40246, "Ġvist": 40247, "Ġcyt": 40248, "Ġflips": 40249, "ÑĢиз": 40250, "balanced": 40251, "ãģªãģı": 40252, "ĠоÑĪиб": 40253, "Ġdestin": 40254, "lasse": 40255, "erei": 40256, "Ġkalo": 40257, "Ġarqu": 40258, "Ġplano": 40259, "Ġordinance": 40260, "Ġcompilation": 40261, "ĠVocês": 40262, "ĠEco": 40263, "Ġì¶Ķì²ľ": 40264, "Ġencima": 40265, "ĠGarrett": 40266, "ĠCord": 40267, "ölker": 40268, "ĠArrow": 40269, "Ġprotons": 40270, ",âĢĭ": 40271, "Ġì²ĺë": 40272, "Ġscand": 40273, "Ġbeige": 40274, "cong": 40275, "Ġbiking": 40276, "ĠTL": 40277, "Ñĥнд": 40278, "ĠìĨĶì§ģ": 40279, "ĠVilla": 40280, "ĠJACK": 40281, "以åıĬ": 40282, "ĠÃ¶ÄŁren": 40283, "Ġtemas": 40284, "ĠKyung": 40285, "Jenn": 40286, "Ġcud": 40287, "Ġimposing": 40288, "Ġcommandments": 40289, "ĠMeans": 40290, "ĠDär": 40291, "Ġrecomend": 40292, "Ġdisposition": 40293, "اÙĩ": 40294, "Ġthu": 40295, "Ġreductions": 40296, "Ġdiu": 40297, "Ġ×ķ×IJ×": 40298, "ĠиÑģÑģлед": 40299, "thren": 40300, "Ġlados": 40301, "ĠRB": 40302, "ixed": 40303, "Ġìı": 40304, "Fr": 40305, "still": 40306, "Ġolmas": 40307, "CHUCK": 40308, "ĠíĨł": 40309, "ĠIndependent": 40310, "ÐĴÐŀ": 40311, "Ġpits": 40312, "Ġundertaken": 40313, "Ġfør": 40314, "ĠNaw": 40315, "ĠìŀijìĹħ": 40316, "Ġshepherd": 40317, "Ġlangue": 40318, "ĠJab": 40319, "ĠDrum": 40320, "ĠElekt": 40321, "æĭ¬": 40322, "ãģĺãĤĥãģªãģĦ": 40323, "á»ijt": 40324, "ĠìĿ´ìª½": 40325, "Ġbeginnen": 40326, "ĠFury": 40327, "á»ĥu": 40328, "sections": 40329, "Ġsprayed": 40330, "Ġmár": 40331, "ĠVolt": 40332, "ĠSeong": 40333, "иÑĤел": 40334, "duction": 40335, "asan": 40336, "Ġjudgments": 40337, "imaan": 40338, "ŀת": 40339, "Ġsiento": 40340, "ĠACT": 40341, "ĠBH": 40342, "dev": 40343, "Ġì¢ĭìķĦíķĺ": 40344, "Ġjorn": 40345, "ISTIN": 40346, "Ġroar": 40347, "Ġimmersion": 40348, "affles": 40349, "Ġtrainee": 40350, "ĠBillboard": 40351, "resses": 40352, "ĠWarm": 40353, "ĠRoberto": 40354, "Ġutilizz": 40355, "ĠIgor": 40356, "Ġrash": 40357, "Ġanalytic": 40358, "iram": 40359, "Ġsymmetrical": 40360, "Ġlifespan": 40361, "Ġeater": 40362, "ĠBloomberg": 40363, "aterial": 40364, "Ġ믿": 40365, "Ġister": 40366, "Ġinvaluable": 40367, "Ġassisting": 40368, "Ġshack": 40369, "μαÏĦα": 40370, "jis": 40371, "eniz": 40372, "ĠпÑĢедлож": 40373, "Ġdeclaring": 40374, "ĠViking": 40375, "ĠAssim": 40376, "Ġexpenditure": 40377, "Ġposing": 40378, "ĠOnun": 40379, "Ġinic": 40380, "аÑİÑĤÑĮ": 40381, "rev": 40382, "Ġmiedo": 40383, "Ġfilthy": 40384, "ĠIB": 40385, "ĠDiscover": 40386, "ichtet": 40387, "million": 40388, "¶Ħëĵ¤ìĿ´": 40389, "Ġambigu": 40390, "ĠFlynn": 40391, "bardziej": 40392, "Ġincomp": 40393, "авно": 40394, "zia": 40395, "Ġinfluencing": 40396, "Ġworldly": 40397, "ĠSalesforce": 40398, "zet": 40399, "Ġparticulier": 40400, "ĠKoch": 40401, "Ġ1943": 40402, "Ġtoner": 40403, "ĠÑįкÑģпеÑĢ": 40404, "Ġsuscri": 40405, "Ġtriggering": 40406, "ICES": 40407, "ìĬ¤ê°Ģ": 40408, "δα": 40409, "ÑĢабоÑĤ": 40410, "Ġafterward": 40411, "pine": 40412, "ĠIL": 40413, "areth": 40414, "Ġпал": 40415, "Ġsaker": 40416, "Ġ1947": 40417, "AF": 40418, "uyorsun": 40419, "ĠìĬ¤ë": 40420, "Ġquantify": 40421, "Ġmentorship": 40422, "Ġllega": 40423, "ĠTamara": 40424, "Ġoptimizing": 40425, "Ġfronts": 40426, "osters": 40427, "Ġesquer": 40428, "Ġsubmissions": 40429, "Ġannih": 40430, "Ġsuction": 40431, "luence": 40432, "chieden": 40433, "INGS": 40434, "Ġ×ij×Ķ": 40435, "ĠÑģÑĨен": 40436, "Ġwielu": 40437, "Ġobjeto": 40438, "Ġboobs": 40439, "ĠGeschäft": 40440, "Ġearbuds": 40441, "ĠÑĢанÑĮÑĪе": 40442, "Ġroutinely": 40443, "Ġcollagen": 40444, "одÑĭ": 40445, "ĠCinnamon": 40446, "Ġbaix": 40447, "دÙħ": 40448, "frage": 40449, "Ġкноп": 40450, "Ġdeception": 40451, "Ġunexpectedly": 40452, "Ġsmelled": 40453, "Ġloos": 40454, "Ġhighlighter": 40455, "Ġ기본": 40456, "ĠGlasgow": 40457, "owana": 40458, "mn": 40459, "ĠJeremiah": 40460, "ĠDatab": 40461, "iete": 40462, "Ġbaw": 40463, "Ġpropia": 40464, "Ġpropri": 40465, "OOOOOOOO": 40466, "inker": 40467, "Ġperturb": 40468, "ĠFake": 40469, "ìĿ´ìĸ": 40470, "imming": 40471, "Ġundocumented": 40472, "Ġtrabajando": 40473, "Ġroam": 40474, "Ġдолжно": 40475, "Ġarbe": 40476, "Ġani": 40477, "atal": 40478, "Ġarada": 40479, "ĠAnda": 40480, "ĠìĽĢ": 40481, "ĠBranch": 40482, "oires": 40483, "Ġoutsider": 40484, "dollar": 40485, "å½ĵçĦ¶": 40486, "isses": 40487, "beans": 40488, "ĠGig": 40489, "çĿ¡": 40490, "rados": 40491, "ĠSut": 40492, "ĠLance": 40493, "edsiÄĻbior": 40494, "Ġcola": 40495, "onents": 40496, "Ġreconsider": 40497, "ãĤ¹ãĥĪ": 40498, "Ġmondo": 40499, "ãĥ³ãĥįãĥ«": 40500, "Ġunsuccess": 40501, "ĠKä": 40502, "è¾¹": 40503, "Ġregel": 40504, "Ġbisog": 40505, "etus": 40506, "Ġunravel": 40507, "Ġsweetie": 40508, "Ġreprésent": 40509, "ouring": 40510, "Ġgroundwater": 40511, "ĠBew": 40512, "Ġscratched": 40513, "Ġcassette": 40514, "Ġcider": 40515, "pis": 40516, "ĠÑģама": 40517, "Ġglobalization": 40518, "Ġdegradation": 40519, "Ġdegener": 40520, "ĠRosie": 40521, "ickt": 40522, "Ġoverweight": 40523, "ĠMEM": 40524, "Ġguardians": 40525, "Ġconsec": 40526, "Hmm": 40527, "æĪijåľ¨": 40528, "ĠпоÑĤÑĢеб": 40529, "Ġmeva": 40530, "Ġgraffiti": 40531, "Ġflirt": 40532, "ĠBP": 40533, "Ġjusto": 40534, "ĠThousands": 40535, "çĶľ": 40536, "٬ìļ´": 40537, ".*": 40538, "ĠRAW": 40539, "Ġfluor": 40540, "iyi": 40541, "antal": 40542, "jed": 40543, "ĠSheng": 40544, "ĠElise": 40545, "ĠCharge": 40546, "ìĿ´íĬ¸": 40547, "Ġcones": 40548, "nies": 40549, "gia": 40550, "ĠнаÑĩала": 40551, "ĠDharma": 40552, "Ġëĭ¤ìĸij": 40553, "Ġfavors": 40554, "ĠTrung": 40555, "hetto": 40556, "Ġpozw": 40557, "Ġlongo": 40558, "Ġkelu": 40559, "Ġdigestion": 40560, "ĠEig": 40561, "ĠTHERE": 40562, "Ġtiers": 40563, "Ġsunk": 40564, "Ġmystical": 40565, "zub": 40566, "ĠÃīt": 40567, "Ġanticipating": 40568, "ĠVine": 40569, "YY": 40570, "Ġconcentrating": 40571, "ĠAgreement": 40572, "Ġоколо": 40573, "Ġlidt": 40574, "ĠYao": 40575, "ĠÑģлиÑĪком": 40576, "rÃŃ": 40577, "ISTINCT": 40578, "ĠOFFIC": 40579, "Ġsoaking": 40580, "Ġsiihen": 40581, "Ġreferencing": 40582, "ĠTampa": 40583, "aney": 40584, "Ġrespuesta": 40585, "ĠCoalition": 40586, "ĠÑģоглаÑģ": 40587, "ankind": 40588, "ĠëĽ": 40589, "ĠYummy": 40590, "ë°°": 40591, "Ġonc": 40592, "uição": 40593, "Ġtheo": 40594, "Ġmural": 40595, "ĠTeachers": 40596, "Ġwaits": 40597, "Ġrenting": 40598, "ĠHarmon": 40599, "ĠeÅŁ": 40600, "ĠMunich": 40601, "íĻľ": 40602, "ìĸ¼": 40603, "cards": 40604, "Ġrouge": 40605, "Ġnên": 40606, "club": 40607, "Ġunseen": 40608, "Ġdepreci": 40609, "Ġcomputed": 40610, "Ġwiping": 40611, "ĠElli": 40612, "identified": 40613, "Ġclutter": 40614, "roleum": 40615, "Ġtelef": 40616, "Ġleveling": 40617, "ĠWoody": 40618, "ĠGus": 40619, "ĠBennett": 40620, "Ġsitio": 40621, "iÅĤ": 40622, "Ġpossessions": 40623, "ĠNatasha": 40624, "oldown": 40625, "ĠÑģообÑī": 40626, "ĠLic": 40627, "Ġë§Įëĵł": 40628, "Ġlorsque": 40629, "weh": 40630, "Ġмам": 40631, "liter": 40632, "adomo": 40633, "Ġfini": 40634, "ÏİÏĤ": 40635, "ĠÑĥбий": 40636, "Ġindisp": 40637, "Ġtelevis": 40638, "Ġpá": 40639, "ĠCreo": 40640, "ÃŃll": 40641, "Ġgur": 40642, "ĠMAL": 40643, "ĠÑĢазнÑĭÑħ": 40644, "Ġziehen": 40645, "Ġfashioned": 40646, "Ġdebating": 40647, "ĠSoup": 40648, "ĠProvince": 40649, "ê·¸ëłĩ": 40650, "Ġimproper": 40651, "Ġimagen": 40652, "ĠÑģделал": 40653, "Ġlogos": 40654, "Ġevento": 40655, "è§Ĩ": 40656, "ảo": 40657, "larda": 40658, "ĠназÑĭваеÑĤÑģÑı": 40659, "Ġverf": 40660, "Ġscreenshots": 40661, "×ķ×ĵ×¢": 40662, "ĠAurora": 40663, "ĠBali": 40664, "tered": 40665, "Ġcontagious": 40666, "Ġcompartir": 40667, "venidos": 40668, "rike": 40669, "ĠвÑĭглÑıдиÑĤ": 40670, "Ġfreedoms": 40671, "nicas": 40672, "ł¤ìĦľ": 40673, "Ġreduz": 40674, "ĠEcu": 40675, "Ġabonn": 40676, "ĠSEÃij": 40677, "ĠBitch": 40678, "Ġprojeto": 40679, "иÑĩно": 40680, "ettre": 40681, "ANNA": 40682, "thank": 40683, "ĠAO": 40684, "æīĢ以åij¢": 40685, "arnish": 40686, "ieÃŁen": 40687, "Ġripple": 40688, "Ġpantry": 40689, "ĠGH": 40690, "γα": 40691, "ĠìĿ´ë²ĪìĹIJ": 40692, "Ġvalidated": 40693, "Ġbrushed": 40694, "ĠEmin": 40695, "ĠDarth": 40696, "esin": 40697, ",.": 40698, "Ġvalle": 40699, "Ġjersey": 40700, "ulan": 40701, "Read": 40702, "ĠRangers": 40703, "Ġsoothing": 40704, "Ġcomplementary": 40705, "ĠVerkehr": 40706, "acakt": 40707, "Ġbatht": 40708, "ĠND": 40709, "Son": 40710, "ĠíĻĶìŀ¥": 40711, "ĠAvi": 40712, "ĠSAL": 40713, "aisse": 40714, "Ġsemaines": 40715, "ĠSurv": 40716, "wier": 40717, "Ġвидел": 40718, "Ġsiete": 40719, "ĶëıĦ": 40720, "ĠRamsay": 40721, "ĠQueensborough": 40722, "ĠMenge": 40723, "ĠFoods": 40724, "Ġtheological": 40725, "Ġ[#": 40726, "Ġвони": 40727, "Ġimmin": 40728, "iosity": 40729, "ĠAbgeord": 40730, "ĠAcho": 40731, "ĠÃĶ": 40732, "Ġstains": 40733, "Ġrealistically": 40734, "Ġfashionable": 40735, "ĠCEOs": 40736, "ĠSkill": 40737, "Ġвже": 40738, "Ġdever": 40739, "ĠPlug": 40740, "æª": 40741, "Pod": 40742, "Ġloaf": 40743, "Ġgebracht": 40744, "Ġabsorbs": 40745, "ĠGranny": 40746, "Ġmalware": 40747, "agÄĻ": 40748, "Ġcivilizations": 40749, "ĠÏģ": 40750, "Ġhält": 40751, "СТ": 40752, "great": 40753, "Ġlayering": 40754, "sings": 40755, "ĠвÑĸн": 40756, "Ġrecognizable": 40757, "Ġwoj": 40758, "Ġweten": 40759, "第ä¸ĢåĢĭ": 40760, "γο": 40761, "Student": 40762, "Ġdéfin": 40763, "please": 40764, "ench": 40765, "Ġattic": 40766, "ĠOttawa": 40767, "Ġopted": 40768, "Ġcaptiv": 40769, "ĠmÅĤ": 40770, "ĠYA": 40771, "ĠWand": 40772, "Ġbounty": 40773, "Ġ270": 40774, "Ġspeculate": 40775, "Ġenhancement": 40776, "Ġcommodities": 40777, "ĠMilton": 40778, "ej": 40779, "alom": 40780, "Das": 40781, "Ġcooldown": 40782, "ר×IJ׾": 40783, "Ġ×IJפ": 40784, "ĠwczeÅĽniej": 40785, "Ġelong": 40786, "Ġdiode": 40787, "inação": 40788, "ĠIris": 40789, "ĠIb": 40790, "Ġsummoned": 40791, "Ġrespe": 40792, "ĠRach": 40793, "注æĦı": 40794, "Ġ»:": 40795, "éĨĴ": 40796, "Ġvur": 40797, "Ġmovimento": 40798, "Ġfluent": 40799, "ĠEvolution": 40800, "ĠButt": 40801, "ificación": 40802, "ĶĶìĸ´": 40803, "ĠÑįнеÑĢг": 40804, "Ġmanipulating": 40805, "Ġpositiv": 40806, "моÑģ": 40807, "Ġwiz": 40808, "Ġintox": 40809, "ÎŃÏģ": 40810, "емÑģÑı": 40811, "ivesse": 40812, "imizi": 40813, "Ġìļ¸": 40814, "Ġknocks": 40815, "Ġcongestion": 40816, "ĠIdeally": 40817, "ĠHolding": 40818, "Ġpobre": 40819, "ĠJUL": 40820, "Ġë¶Ħëĵ¤ìĿĢ": 40821, "Ġακ": 40822, "ĠFerguson": 40823, "ĠLaboratory": 40824, "richten": 40825, "rophy": 40826, "production": 40827, "assung": 40828, "ITA": 40829, "Ġsiècle": 40830, "רת": 40831, "cision": 40832, "Ġפ×Ķ": 40833, "ĠIrene": 40834, "anca": 40835, "ĠìĤ¬ê³ł": 40836, "Ġpinpoint": 40837, "Ġdesignation": 40838, "ÅŁam": 40839, "lÄ±ÅŁ": 40840, "aat": 40841, "ĠnÃ¥gra": 40842, "Ġmythical": 40843, "ĠDeclaration": 40844, "Ġìŀ¡ìķĦ": 40845, "Ġbyte": 40846, ".âĻª": 40847, "Del": 40848, "Ġíį¼": 40849, "Ġnutritious": 40850, "ĠÑĢÑĥблей": 40851, "åĤ³": 40852, "SAY": 40853, "Master": 40854, "ĠÑĦоÑĤогÑĢаÑĦ": 40855, "ĠëĴ¤ìĹIJ": 40856, "Ġneh": 40857, "Ġdokument": 40858, "çªģ": 40859, "Ġczasu": 40860, "Ġcontinua": 40861, "ĠSilent": 40862, "Ġtensor": 40863, "Ġtanta": 40864, "Ġirgendwo": 40865, "ĠLET": 40866, "ĠShakt": 40867, "lama": 40868, "chlag": 40869, "Ġdingen": 40870, "ÑģÑĤÑĢа": 40871, "Ġehrlich": 40872, "ĠMacht": 40873, "rels": 40874, "Ãłcies": 40875, "video": 40876, "Ġnaturale": 40877, "ĠSTEVE": 40878, "umm": 40879, "BACK": 40880, "Ġ720": 40881, "ãģ§ãģĹãģŁ": 40882, "Ġmomencie": 40883, "ĠSwan": 40884, "Ġtechnicians": 40885, "Ġgeehr": 40886, "ĠMend": 40887, "Reg": 40888, "Ġscaff": 40889, "Ġaide": 40890, "Ġë³´ëĬĶ": 40891, "Ġpresses": 40892, "lerde": 40893, "\\'": 40894, "Ġultrasound": 40895, "Ġdisclaimer": 40896, "ĠMits": 40897, "ĠHoliday": 40898, "Ġexternally": 40899, "ĠFate": 40900, "INO": 40901, "ĠCats": 40902, "ë°ķ": 40903, "umo": 40904, "control": 40905, "ĠtheCUBE": 40906, "tic": 40907, "ierungs": 40908, "Ġзнаком": 40909, "Ġfreestyle": 40910, "MANDARIN": 40911, "Ġise": 40912, "aurus": 40913, "許": 40914, "ĠStrategy": 40915, "ĠBeam": 40916, "räge": 40917, "Ġexploited": 40918, "ãģĪãģ£": 40919, "idis": 40920, "Ġchime": 40921, "ĠPeninsula": 40922, "Ġmerits": 40923, "Ġaltro": 40924, "ĠTOP": 40925, "ĠSens": 40926, "ĠKant": 40927, "oras": 40928, "Ġroyalty": 40929, "ĠIDE": 40930, "å¤ī": 40931, "racy": 40932, "ĠTHOM": 40933, "omos": 40934, "Ġlänger": 40935, "Ġnumbered": 40936, "Um": 40937, "ĠNiye": 40938, "θη": 40939, "zyka": 40940, "lime": 40941, "ĠPersonen": 40942, "Ġvalidity": 40943, "Ġcontrat": 40944, "ĠComic": 40945, "çons": 40946, "ĠHeidi": 40947, "Ġzg": 40948, "Ġrenamed": 40949, "Ġcumin": 40950, "ĠJF": 40951, "inel": 40952, "Ġenforced": 40953, "Ġchama": 40954, "лиÑĩно": 40955, "ẻ": 40956, "Ġденег": 40957, "Ġprofund": 40958, "Ġpelvic": 40959, "Ġpalavra": 40960, "Ġextras": 40961, "Ġankles": 40962, "ìĹIJìĦľëıĦ": 40963, "ĠTF": 40964, "Ġinsanely": 40965, "ĠмÑıÑģ": 40966, "Ġréponse": 40967, "Ġgöster": 40968, "ĠBBQ": 40969, "ĠÑĥÑĩаÑģÑĤ": 40970, "Ġshaken": 40971, "ãĤ«ãĥ³ãĤ¿": 40972, "Ġalmonds": 40973, "dish": 40974, "ĠPG": 40975, "ĠBlizzard": 40976, "ÑĮого": 40977, "Ġãħ": 40978, "Ġknapp": 40979, "Too": 40980, "Ġunde": 40981, "Ġmounts": 40982, "омина": 40983, "Ġnortheast": 40984, "Ġcensorship": 40985, "ÑıÑĤÑĮÑģÑı": 40986, "lr": 40987, "Ġlawmakers": 40988, "ĠsÃ¥dan": 40989, "Ġinsider": 40990, "Ġcleanup": 40991, "ĠNada": 40992, "óc": 40993, "Ġharvested": 40994, "ĠDespués": 40995, "íļį": 40996, "Ġredundant": 40997, "ENA": 40998, "Ġdelegate": 40999, "Ġburg": 41000, "ĠAlison": 41001, "æĸ°èģŀ": 41002, "Ġcelestial": 41003, "Ġsinners": 41004, "Ġmartyr": 41005, "ĠPerm": 41006, "Ġspecimens": 41007, "Ġmitochond": 41008, "Ġmaravil": 41009, "Ġcavalry": 41010, "Ġarrays": 41011, "Ġannex": 41012, "Ġlaboratories": 41013, "ĠByz": 41014, "Ġatac": 41015, "ĠÑģложно": 41016, "Ġtopl": 41017, "Ġgeri": 41018, "ĠCombat": 41019, "ÑģÑıÑĤ": 41020, "eken": 41021, "ĠÐĴлад": 41022, "Ġajust": 41023, "Ġmarque": 41024, "Ġlookout": 41025, "ĠLol": 41026, "Ġrooftop": 41027, "ĠOrion": 41028, "Ġбой": 41029, "Ġheartbreaking": 41030, "Ġdetto": 41031, "zh": 41032, "ätter": 41033, "cera": 41034, "Ġheats": 41035, "Ġantiqu": 41036, "Ġunfinished": 41037, "ĠKazu": 41038, "ılı": 41039, "Ġslightest": 41040, "leo": 41041, "ĠvÃ¥ra": 41042, "Ġverschiedenen": 41043, "Ġlotion": 41044, "ä½łå°±": 41045, "æĮº": 41046, "ÑĪего": 41047, "ctional": 41048, "ĠìĿ´ìł": 41049, "dragon": 41050, "Ġresonates": 41051, "Ġinm": 41052, "avic": 41053, "Ġfulfil": 41054, "Ġ기ëĮĢ": 41055, "Ġjustamente": 41056, "ĠдоÑģÑĤÑĥп": 41057, "Ġ그건": 41058, "Ġreconcile": 41059, "ĠSchön": 41060, "ĠAvoid": 41061, "ê¹Ģ": 41062, "'D": 41063, "Ġconfinement": 41064, "Ġíij": 41065, "Ġmotivating": 41066, "ĠBrittany": 41067, "ĠãģĻ": 41068, "Ġscreamed": 41069, "object": 41070, "Ġdecree": 41071, "Ġtravaille": 41072, "issible": 41073, "Ġbusted": 41074, "process": 41075, "Ġmassacre": 41076, "ĠnghÄ©": 41077, "ilyn": 41078, "ĠвÑĢоде": 41079, "Ġpoetic": 41080, "Ġnhất": 41081, "Ġironically": 41082, "usu": 41083, "nio": 41084, "Ġstaging": 41085, "omedical": 41086, "leased": 41087, "ĠìĥĪë¡ľìļ´": 41088, "ĠNZ": 41089, "acting": 41090, "ĠBattlefield": 41091, "playful": 41092, "Vi": 41093, "Ġseñora": 41094, "Ġprompts": 41095, "lichkeit": 41096, "Ġçıkar": 41097, "jiang": 41098, "Ġpicky": 41099, "ĠCave": 41100, "Ġmiraculous": 41101, "ĠHughes": 41102, "2016": 41103, "Ġxu": 41104, "ĠDorothy": 41105, "Ġvirtues": 41106, "Ġretract": 41107, "Ġtyr": 41108, "Ġcharismatic": 41109, "Ġbola": 41110, "é¼": 41111, "Ġë§IJìĶĢë": 41112, "Ġparental": 41113, "Ġmillionaire": 41114, "ariat": 41115, "æĶ¿åºľ": 41116, "Ġinvoke": 41117, "żenie": 41118, "Ġextremes": 41119, "ĠAku": 41120, "ividade": 41121, "Ġï·º": 41122, "Ġìĭľì²Ń": 41123, "ĠGarlic": 41124, "RIA": 41125, "ĠдоÑģ": 41126, "ĠPont": 41127, "Ġmilj": 41128, "elli": 41129, "Ġracket": 41130, "Ġcompetit": 41131, "ĠWhis": 41132, "Ġrealt": 41133, "ignment": 41134, "estre": 41135, "Ġpernah": 41136, "ĠOpening": 41137, "ĠFS": 41138, "ĠDemokraten": 41139, "acements": 41140, "Ġworldview": 41141, "Ġplayoffs": 41142, "ĠCAD": 41143, "Ġétant": 41144, "Ġyemek": 41145, "Ġsentiments": 41146, "odel": 41147, "buster": 41148, "aÅŁ": 41149, "ĠKY": 41150, "czÄĻ": 41151, "Ġschöne": 41152, "ape": 41153, "ĠRaspberry": 41154, "Ġcredited": 41155, "ĠHidden": 41156, "Ġsausages": 41157, "ruce": 41158, "ĠBev": 41159, "ilantro": 41160, "Ġpokemon": 41161, "Ġê°Ģ격": 41162, "Ġproceeding": 41163, "Ġveio": 41164, "Ġ175": 41165, "è¸": 41166, "max": 41167, "Ġfrater": 41168, "ìłĦìĹIJ": 41169, "Ġegent": 41170, "Ġ2500": 41171, "usch": 41172, "Tube": 41173, "Ġamplify": 41174, "Ġprawd": 41175, "Ġodor": 41176, "ĠScan": 41177, "Ġplotting": 41178, "ithmetic": 41179, "Ġresigned": 41180, "ĠSCOTT": 41181, "Ġstereoty": 41182, "Ġdoable": 41183, "ĠComplex": 41184, "ÙģÙĬ": 41185, "tım": 41186, "ÑĢиг": 41187, "lardan": 41188, "eso": 41189, "DEN": 41190, "Ġhoodie": 41191, "ĠCAT": 41192, "اط": 41193, "Ġbonded": 41194, "ĠBurns": 41195, "опаÑģ": 41196, "ĠrÄĻ": 41197, "εια": 41198, "ĠоÑĤделÑĮ": 41199, "Ġtimeless": 41200, "ĠVij": 41201, "ĠPanama": 41202, "Ġreorgan": 41203, "ĠTä": 41204, "ĠPluto": 41205, "Orange": 41206, "Ġпойд": 41207, "ĠBristol": 41208, "uced": 41209, "ĠëIJĺìĸ´": 41210, "Ġunbedingt": 41211, "adle": 41212, "Ġvolunteered": 41213, "Ġmieli": 41214, "ĠEdinburgh": 41215, "ikal": 41216, "Ġalten": 41217, "ĠArsen": 41218, "Ġmouvement": 41219, "Ġantique": 41220, "Ġbh": 41221, "ĠHers": 41222, "Ġsaute": 41223, "Ġaspire": 41224, "Ġspheres": 41225, "ĠWam": 41226, "ắm": 41227, "Ġwipes": 41228, "Ġ280": 41229, "ĠVeh": 41230, "Ġcoloca": 41231, "аÑĦ": 41232, "ĠвозможноÑģÑĤÑĮ": 41233, "Ġphysiological": 41234, "hwa": 41235, "etu": 41236, "Ġprolonged": 41237, "Ġexperiência": 41238, "Ġвидно": 41239, "Ġquarant": 41240, "Ġpuedan": 41241, "èĶ": 41242, "vine": 41243, "ĠUSDA": 41244, "phem": 41245, "Ġformidable": 41246, "Ġflatter": 41247, "ìĸ´ì§Ģ": 41248, "Ġbén": 41249, "à¹ģà¸ķ": 41250, "Ġë¬¼ë¡ł": 41251, "Ġfactions": 41252, "ĠLeaving": 41253, "Ġ×IJת×Ķ": 41254, "ĠExpert": 41255, "dio": 41256, "ĠVerd": 41257, "ãģ¿ãģŁãģĦ": 41258, "Ġsint": 41259, "ÙĨد": 41260, "number": 41261, "Ġowed": 41262, "Ġinduce": 41263, "ĠFreddie": 41264, "abo": 41265, "ĠFilipino": 41266, "¯¼ë": 41267, "believably": 41268, "athlon": 41269, "amaan": 41270, "Ġdevenir": 41271, "ĠGos": 41272, "ĠJenkins": 41273, "bait": 41274, "Ġbins": 41275, "ĠMICH": 41276, "uyorum": 41277, "igrade": 41278, "isso": 41279, "ĠìĹ´": 41280, "ĠìķĦë¹ł": 41281, "Ġdiarrhea": 41282, "Ġtornar": 41283, "addin": 41284, "Ġungefähr": 41285, "Ġrestroom": 41286, "Ġpsychiatrist": 41287, "ĠKickstarter": 41288, "Ġgera": 41289, "Ġalred": 41290, "ĠWrap": 41291, "ÏĮÏĥ": 41292, "Ġsinner": 41293, "CHEERING": 41294, "Ġkilow": 41295, "Ġdeterminant": 41296, "Ġdemonic": 41297, "idences": 41298, "chas": 41299, "ĠDed": 41300, "å¼ķ": 41301, "Ġstumble": 41302, "ĠUrs": 41303, "Ġdeceived": 41304, "ĠTER": 41305, "ĠCó": 41306, "elled": 41307, "Ġnotwend": 41308, "Ġì§Ģê¸Īê¹Įì§Ģ": 41309, "Ġpartido": 41310, "Ġdescended": 41311, "Ġvardır": 41312, "Ġenacted": 41313, "ĠczÄĻÅĽci": 41314, "å·¥ä½ľ": 41315, "Ġtrainees": 41316, "Ġaudible": 41317, "Ġmalf": 41318, "Ġveo": 41319, "ìn": 41320, "ĠGPA": 41321, "ĠAppe": 41322, "åĤ·": 41323, "Ġrut": 41324, "ĠCarla": 41325, "kach": 41326, "Ġsavior": 41327, "itched": 41328, "Ġclimax": 41329, "аÑĤелÑı": 41330, "ĠMcConnell": 41331, "олÑı": 41332, "ereye": 41333, "ĠÑģозн": 41334, "Ġcabo": 41335, "ĠSne": 41336, "ĠAffordable": 41337, "ĠsarÃł": 41338, "Ġlegitimacy": 41339, "Ġscarce": 41340, "...</": 41341, "Ġ108": 41342, "Ġacum": 41343, "ĠFrankly": 41344, "Ġradiator": 41345, "Ġgenerals": 41346, "Ġdivides": 41347, "Ġcheesecake": 41348, "Ġsorcer": 41349, "Ġmisconception": 41350, "Ġhardships": 41351, "ĠOnePlus": 41352, "üyorsun": 41353, "ĠSoviets": 41354, "ĠItalia": 41355, "icki": 41356, "ĠAfterwards": 41357, "Ġridiculously": 41358, "ĠgdzieÅĽ": 41359, "ĠNotes": 41360, "ÙĥاÙĨ": 41361, "Ġroman": 41362, "Ġorganizer": 41363, "Ġcourtyard": 41364, "ĠÑĩеловеÑĩ": 41365, "ĠWitness": 41366, "ĠпÑıÑĤ": 41367, "ĠChill": 41368, "ĠValve": 41369, "Ġάλλ": 41370, "ĠKP": 41371, "chluss": 41372, "Ġdeflect": 41373, "ĠToni": 41374, "Ġclair": 41375, "Ġstacking": 41376, "ä½İ": 41377, "raszam": 41378, "ĠSonra": 41379, "ãģ£ãģ¡ãĤĥ": 41380, "ĠAtari": 41381, "Ġpasó": 41382, "Ġcharms": 41383, "anst": 41384, "Ġterce": 41385, "ĠLilly": 41386, "Ġpsychologically": 41387, "ĠcÅĵ": 41388, "uste": 41389, "¥´ì": 41390, "CTV": 41391, "Ġmiel": 41392, "çļĩ": 41393, "Care": 41394, "ĠâĢij": 41395, "Ġsnapped": 41396, "ãģ©ãĤĤ": 41397, "Ġê°IJë": 41398, "оÑĤÑĭ": 41399, "Ġmês": 41400, ".?": 41401, "Ġtonnes": 41402, "×ķ×ĵ×Ķ": 41403, "à¸Ħà¸Ļ": 41404, "Tu": 41405, "Ġdistributing": 41406, "Ġcrackers": 41407, "Ġcoração": 41408, "ämän": 41409, "ä½łåľ¨": 41410, "clamation": 41411, "оÑĢд": 41412, "ĵľë¦´ê²ĮìļĶ": 41413, "ĠUnterschied": 41414, "Fine": 41415, "cko": 41416, "ĠÑĢебен": 41417, "Ġspic": 41418, "Ġdoctoral": 41419, "ĠÑģкоÑĢее": 41420, "univers": 41421, "acula": 41422, "ĠÃĸsterreich": 41423, "Ġgrinder": 41424, "Ġambos": 41425, "Ġvastly": 41426, "éĢĻåĢĭæĺ¯": 41427, "Ġconfessed": 41428, "ĠShh": 41429, "anders": 41430, "ĠGuan": 41431, "ĠнеобÑħодимо": 41432, "Ġchampionships": 41433, "ĠVul": 41434, "ĠPhi": 41435, "ĠMeasure": 41436, "æľ¨": 41437, "Ġinsgesamt": 41438, "æħ¢æħ¢": 41439, "vette": 41440, "Ġgenom": 41441, "indung": 41442, "gli": 41443, "Det": 41444, "Ġunmute": 41445, "ãģ¾ãĤĬ": 41446, "Ġsauces": 41447, "ĠDw": 41448, "×ijת": 41449, "ĠBRE": 41450, "Ġnurture": 41451, "Ġdetained": 41452, "ĠBeer": 41453, "ĠмиÑĢа": 41454, "ве": 41455, "ĠBirds": 41456, "Ġmeilleur": 41457, "Ġrewind": 41458, "Ġpore": 41459, "×Ļ×ĸ": 41460, "éger": 41461, "quela": 41462, "Ġtrousers": 41463, "Ġsiinä": 41464, "ĠGaga": 41465, "ĠBRAND": 41466, "leben": 41467, "Ġraspberry": 41468, "ä»ĺ": 41469, "ilik": 41470, "Ġversão": 41471, "lak": 41472, "Ġlogar": 41473, "ĠMIDI": 41474, "ĠìľĦíķľ": 41475, "ĠпÑĢоизоÑĪ": 41476, "Ġsteril": 41477, "Ġharmed": 41478, "авлив": 41479, "ĠÑģÑģÑĭл": 41480, "Ġlacked": 41481, "Ġcontacting": 41482, "Ġ기ìŀIJ": 41483, "Ġgefähr": 41484, "Ġcoy": 41485, "ikel": 41486, "Ġbinge": 41487, "Ġorthogonal": 41488, "Ġentendu": 41489, "ĠThirty": 41490, "Ġsmartest": 41491, "å¤ļå°ij": 41492, "Ġrasa": 41493, "ĠQuá»ijc": 41494, "ÑĭваÑİÑĤ": 41495, "Ġslut": 41496, "лÑĥÑĩ": 41497, "igten": 41498, "ĠÑĢаб": 41499, "Ġtaman": 41500, "Ġqualidade": 41501, "Ġdomination": 41502, "Ġsinus": 41503, "Ġprogrammers": 41504, "Ġallergy": 41505, "ĠTorres": 41506, "ĠAustrian": 41507, "nants": 41508, "å®ĮæĪIJ": 41509, "Mel": 41510, "ĠÑĥвелиÑĩ": 41511, "ĠAgg": 41512, "Ġsok": 41513, "Ġpluck": 41514, "Ġbinds": 41515, "Ġpropor": 41516, "ĠMaf": 41517, "Ġosob": 41518, "ĠVIC": 41519, "é¥": 41520, "ĠзаÑĩем": 41521, "Ġexhibitions": 41522, "Ġetti": 41523, "cza": 41524, "ĠнаÑĪиÑħ": 41525, "ĠMitte": 41526, "обÑĭÑĤи": 41527, "Ġclocks": 41528, "Ġrico": 41529, "æĶ»": 41530, "ĠиÑģÑĤоÑĢиÑı": 41531, "Ġschizophren": 41532, "Ġfluff": 41533, "ĠÑģобиÑĢ": 41534, "Ġapoy": 41535, "Ġprinces": 41536, "Ġbraces": 41537, "ĠFIR": 41538, "ĠSna": 41539, "Ġ;)": 41540, "venes": 41541, "Ġvuelta": 41542, "Ġmies": 41543, "Ġbroom": 41544, "Ġmerry": 41545, "Ġespecialmente": 41546, "ĠAlban": 41547, "ĠпоÑģÑĤоÑıнно": 41548, "ĠLena": 41549, "ĠCult": 41550, "also": 41551, "Ġquoting": 41552, "Ġgenere": 41553, "ĠYar": 41554, "ĠLage": 41555, "Ġdemost": 41556, "Ġdage": 41557, "ĠEcuador": 41558, "Ġanvänd": 41559, "uÃŁen": 41560, "Ġë°ĽìķĦ": 41561, "Ġpsychologists": 41562, "ĠLars": 41563, "Ġpossa": 41564, "Ġoutgoing": 41565, "Ġmetic": 41566, "Ġbaggage": 41567, "eria": 41568, "Ġrichtige": 41569, "ìĭľìĹIJ": 41570, "ĠÑģоÑħÑĢан": 41571, "Ġrooting": 41572, "Ġdroplets": 41573, "çļĨãģķãĤĵ": 41574, "Ġnasal": 41575, "ĠCox": 41576, "Xi": 41577, "Ġdisposable": 41578, "Ġbutcher": 41579, "ĠZar": 41580, "ĠArmenian": 41581, "Ġë¿Įë": 41582, "ĠFool": 41583, "ĠCBD": 41584, "Ġsost": 41585, "Ġperish": 41586, "ĠRép": 41587, "ç´°": 41588, "ãģĿãĤĮãģ§ãģ¯": 41589, "ĠFreud": 41590, "Ġfandom": 41591, "Ġbloque": 41592, "Ġinventor": 41593, "Ġabre": 41594, "Ġénormément": 41595, "Ġimports": 41596, "éĪ": 41597, "Ġotur": 41598, "ĠRyu": 41599, "ĠâĨĴ": 41600, "Ġsecondo": 41601, "Ġincompet": 41602, "Ġincarceration": 41603, "Ġascend": 41604, "bene": 41605, "åĸľæ¬¢": 41606, "Ġolurs": 41607, "noch": 41608, "Ġbreeds": 41609, "лиз": 41610, "ĠVerfüg": 41611, "Ġmailing": 41612, "really": 41613, "Ġesf": 41614, "Ġpele": 41615, "Ġleash": 41616, "Ġdisks": 41617, "ĠзамеÑĩ": 41618, "ìķĦìķĦ": 41619, "abouts": 41620, "ĠMull": 41621, "ĠDent": 41622, "edereen": 41623, "Drive": 41624, "Ġtipping": 41625, "Ġnigga": 41626, "ordum": 41627, "Ġporter": 41628, "Ġkaraoke": 41629, "Ġdocumentaries": 41630, "ĠRIGHT": 41631, "ĠPurd": 41632, "ĠоÑģÑĤан": 41633, "клад": 41634, "érence": 41635, "Ġê±¸ë¡ľ": 41636, "ĠÑĤоп": 41637, "ĠWong": 41638, "ä¸į对": 41639, "ĠпÑĢиÑĢ": 41640, "Ġnominal": 41641, "Ġaula": 41642, "ĠÑįкÑĢан": 41643, "Ġcherche": 41644, "ĠThr": 41645, "åħ¶å®ŀ": 41646, "Ġlaufen": 41647, "ĠKathleen": 41648, "Ġreactors": 41649, "ihat": 41650, "Ġsided": 41651, "ĠSimone": 41652, "Ġguideline": 41653, "important": 41654, "bumps": 41655, "tone": 41656, "Ġentreprises": 41657, "Ġconstitute": 41658, "oscope": 41659, "ĠMystery": 41660, "cycles": 41661, "ĠWarsaw": 41662, "Ġbursts": 41663, "ĠZhong": 41664, "å®ĮäºĨ": 41665, "ĠSARAH": 41666, "ĠëĬIJê»": 41667, "éį": 41668, "Ġbeacon": 41669, "åįĩ": 41670, "ADE": 41671, "Ġì§ĢëĤĺ": 41672, "Ġersch": 41673, "Ġintegers": 41674, "ĠCrossing": 41675, "source": 41676, "Ġschooling": 41677, "ĠROM": 41678, "atorium": 41679, "ĠìŀĪê²Į": 41680, "Ġrôle": 41681, "ÐķÐĿ": 41682, "Chat": 41683, "Ġshrinking": 41684, "Ġreimburse": 41685, "Ġlumber": 41686, "ücks": 41687, "Ġsalah": 41688, "Mother": 41689, "Ġkali": 41690, "ĠQatar": 41691, "otional": 41692, "Ġopacity": 41693, "Ġnee": 41694, "ĠCory": 41695, "Ġ측": 41696, "Ġturbulent": 41697, "zers": 41698, "ĠÑĤеÑģÑĤ": 41699, "Ġécrit": 41700, "Ġë³´íĨµ": 41701, "Ġdisgrace": 41702, "Ġì¹´": 41703, "Ġcourtesy": 41704, "inga": 41705, "Ġhugging": 41706, "ĠABS": 41707, "mith": 41708, "Ġinsufficient": 41709, "Ġcrooked": 41710, "Ġê·¸ëĮĢë¡ľ": 41711, "ìĭ¤í": 41712, "Ġsimulated": 41713, "ĠëĦ¤ê°Ģ": 41714, "Ġbö": 41715, "ĠOtto": 41716, "LING": 41717, "Ġillustrates": 41718, "ĠDestroy": 41719, "Ġ1961": 41720, "ĠTagen": 41721, "Ġmelon": 41722, "ĠPascal": 41723, "QUE": 41724, "ĠполÑĥÑĩиÑĤÑĮ": 41725, "Ġincidence": 41726, "ĠStevens": 41727, "ĠGins": 41728, "rue": 41729, "Ġunreasonable": 41730, "ĠJie": 41731, "ysics": 41732, "Ġ몰ëĿ¼": 41733, "Ġfishes": 41734, "©´ì": 41735, "Ġprecurs": 41736, "ĠmogÄĻ": 41737, "tight": 41738, "eté": 41739, "Ġmundial": 41740, "ìĹĪëĭ¤": 41741, "â̦!": 41742, "BU": 41743, "Ġsociology": 41744, "Ġbrutality": 41745, "Ġpersonaje": 41746, "ĠnÃŃvel": 41747, "Ġfazem": 41748, "Ġessen": 41749, "Ġdwelling": 41750, "Ġcommercially": 41751, "Ġedits": 41752, "Ġdues": 41753, "ĠGSA": 41754, "ìĿ¸ê°Ģ": 41755, "ĠíĹĪíĮĿ": 41756, "ĠYahoo": 41757, "енеÑĢ": 41758, "ìľ¨": 41759, "ÑĥÑĪки": 41760, "left": 41761, "Ġcaptive": 41762, "cipher": 41763, "Ġ×ŀ×ŀ×": 41764, "ĠгÑĢом": 41765, "Ġinnate": 41766, "Ġimpul": 41767, "ĠìŬìŀIJ": 41768, "Ġswallowed": 41769, "ĠTabii": 41770, "ìĿ´ìĭ": 41771, "ĠÑģоÑģÑĤав": 41772, "Ġoyun": 41773, "Ġobrigado": 41774, "ĠAph": 41775, "Katie": 41776, "Ġcena": 41777, "ĠAllÄģh": 41778, "ÙĪØ³": 41779, "Ġprzyp": 41780, "Ġpept": 41781, "Ġvoluntarily": 41782, "ĠOÄŁlum": 41783, "ĠElo": 41784, "oue": 41785, "Bir": 41786, "burger": 41787, "ĠSBS": 41788, "Ġ6000": 41789, "Ġpromotional": 41790, "ĠHerrn": 41791, "Ġstamping": 41792, "Ġqualifying": 41793, "Ġcosmos": 41794, "Ġafar": 41795, "æ±Ł": 41796, "abus": 41797, "Ġdads": 41798, "ãģŃãģĩ": 41799, "ĠÑįконом": 41800, "incarn": 41801, "Ġìĸ´ëĶ": 41802, "Ġлеж": 41803, "ĠBET": 41804, "Ġнайд": 41805, "onter": 41806, "Ġreusable": 41807, "Ġkomma": 41808, "ĠBij": 41809, "ĠTeraz": 41810, "ĠOlá": 41811, "ĠìķĦ침": 41812, "ĠÑĢазмеÑĢ": 41813, "awan": 41814, "Ġcarta": 41815, "æIJŀ": 41816, "iceless": 41817, "Ġsme": 41818, "ĠTutaj": 41819, "ĠÈĺi": 41820, "Ġprobation": 41821, "Ġadequately": 41822, "ĠPresidential": 41823, "indruck": 41824, "blade": 41825, "Ġveulent": 41826, "Ġcioè": 41827, "åĮħæĭ¬": 41828, "Ġreverb": 41829, "Ġgegenüber": 41830, "ĠEspero": 41831, "Ġbege": 41832, "ĠSTUDENT": 41833, "sound": 41834, "ĠDü": 41835, "Ġoffend": 41836, "Ġ\"..": 41837, "kennt": 41838, "ĠÑģлÑĥÑĪ": 41839, "Ġpurposely": 41840, "ĠLit": 41841, "ĠíĽ¨": 41842, "ucher": 41843, "Ġhina": 41844, "ých": 41845, "ignon": 41846, "THE": 41847, "Ġglide": 41848, "ourcing": 41849, "ĠØ£ÙĨا": 41850, "Ġollut": 41851, "Ġarchety": 41852, "Ġshady": 41853, "Ġsomm": 41854, "Ġepile": 41855, "Keep": 41856, "Ġnajbardziej": 41857, "à¤ķ": 41858, "itutional": 41859, "Ġмай": 41860, "Ġsinful": 41861, "ĠBronx": 41862, "ĠглÑĥб": 41863, "Ġvam": 41864, "Ġpresets": 41865, "ĠDag": 41866, "ĠìĻĦìĦ±": 41867, "Ġcreek": 41868, "itures": 41869, "ĠLords": 41870, "ött": 41871, "UNT": 41872, "Ra": 41873, "Ġinequalities": 41874, "Ġcollateral": 41875, "Ġwrists": 41876, "Ġgrouped": 41877, "ĠобÑĭÑĩно": 41878, "Ġarmored": 41879, "Ġtung": 41880, "Ġconverge": 41881, "Ġbok": 41882, "ĠDodge": 41883, "нÑıÑı": 41884, "Ġfleeing": 41885, "ĠMartinez": 41886, "ĠDreams": 41887, "kek": 41888, "Ġsociale": 41889, "ĠPlaza": 41890, "دة": 41891, "Ġkell": 41892, "ĠStellen": 41893, "felt": 41894, "ĠÑģпаÑģ": 41895, "ĠPv": 41896, "Ġcanción": 41897, "ĠHert": 41898, "ĠBalance": 41899, "Ġselves": 41900, "Ġvandaag": 41901, "Ġpry": 41902, "Ġnajle": 41903, "ĠвидиÑĤе": 41904, "Ġvelvet": 41905, "Ġgroot": 41906, "Ġfout": 41907, "模": 41908, "ĠSchulen": 41909, "ĠMohammed": 41910, "ĠCenters": 41911, "Ġhaver": 41912, "Ġfreuen": 41913, "¤íĬ¸": 41914, "лан": 41915, "POS": 41916, "inki": 41917, "Ġëĭµ": 41918, "Ġparalyzed": 41919, "GLISH": 41920, "Ġcasts": 41921, "ĠVC": 41922, "ìĿ´ìħĺ": 41923, "Ġتھ": 41924, "票": 41925, "Ġì¤ĺ": 41926, "Ġר×ķצ": 41927, "Ġsuced": 41928, "Ġprogresses": 41929, "ĠEÄŁer": 41930, "°ëıĦ": 41931, "Ġinstallations": 41932, "pedo": 41933, "еÑĢб": 41934, "interpret": 41935, "Ġê³łë¯¼": 41936, "ĠAzerbai": 41937, "ividades": 41938, "Ġì£ĦìĨ¡": 41939, "Ġentfer": 41940, "Ġchwil": 41941, "ĠHerbert": 41942, "ĠAlexandria": 41943, "yty": 41944, "Ġsechs": 41945, "Ġcaliber": 41946, "ĠWeise": 41947, "ĠHeck": 41948, "ĠYug": 41949, "ĠاÙĦØ·": 41950, "Ġpesar": 41951, "Ġcigar": 41952, "Ġmél": 41953, "Ġhaird": 41954, "Ġprzypadku": 41955, "Ġconfidently": 41956, "Ġanarch": 41957, "ĠGian": 41958, "Ġdobre": 41959, "cjÄĻ": 41960, "awy": 41961, "ĠRece": 41962, "ĠGobierno": 41963, "Ġcarga": 41964, "umsy": 41965, "Ġnorte": 41966, "Ġhandler": 41967, "Ġrespecting": 41968, "Ġallied": 41969, "ĠPiet": 41970, "ichtlich": 41971, "Ġolds": 41972, "Ġdusty": 41973, "Ġgry": 41974, "Ġ-...": 41975, "GHT": 41976, "Ġneo": 41977, "Ñĩики": 41978, "ежд": 41979, "aide": 41980, "ĠбÑĥло": 41981, "íį¼": 41982, "Ġtemporada": 41983, "Ġdoute": 41984, "âĺĨ": 41985, "ĠìĪł": 41986, "ĠJUSTIN": 41987, "auto": 41988, "Ġrationale": 41989, "prob": 41990, "Ġfishy": 41991, "Ġdoorway": 41992, "Ġemptiness": 41993, "еннаÑı": 41994, "Ġbrag": 41995, "ĠÐĵде": 41996, "çξ": 41997, "Ġtransient": 41998, "Ġmittlerweile": 41999, "ĠBret": 42000, "Ġfij": 42001, "Ġdeposited": 42002, "NS": 42003, "ĠìķŀìĹIJ": 42004, "Ġkimse": 42005, "Ġcharities": 42006, "ĠMillenn": 42007, "dogs": 42008, "Ġmoyen": 42009, "Ġnuevos": 42010, "ĠCookie": 42011, "parable": 42012, "doing": 42013, "ĠSail": 42014, "Ġicy": 42015, "haba": 42016, "Ġqueens": 42017, "Ġchocolates": 42018, "ĠNay": 42019, "ĠÑĦин": 42020, "Ġvec": 42021, "Ġhelmets": 42022, "TM": 42023, "ĠArmed": 42024, "Ġimpairment": 42025, "ĠTus": 42026, "ĠMême": 42027, "omez": 42028, "ĠRequ": 42029, "ĠInvestig": 42030, "íİĺ": 42031, "Ġgolpe": 42032, "ĠRac": 42033, "igraph": 42034, "Ġkwest": 42035, "Ġsailors": 42036, "Ġstatutory": 42037, "Ġmilestones": 42038, "ĠMash": 42039, "ĠGesetzentwurf": 42040, "éĬ": 42041, "Ġcoloured": 42042, "huma": 42043, "Ġyere": 42044, "Ġsubtitles": 42045, "Ġembodied": 42046, "Ġmisschien": 42047, "ĠiPh": 42048, "ützen": 42049, "Ġdetached": 42050, "Ġdescrição": 42051, "ciamo": 42052, "Ġrecoil": 42053, "ĠÐŃÑĤоÑĤ": 42054, "Ġexported": 42055, "ĠAlone": 42056, "antry": 42057, "Ġestan": 42058, "ĠSod": 42059, "Ġlavoro": 42060, "æĬĬå®ĥ": 42061, "ר×ij": 42062, "ĠÄijá»ĭ": 42063, "Ġswag": 42064, "ĠPCB": 42065, "ĠKaiser": 42066, "ĠModer": 42067, "jug": 42068, "Ġtextile": 42069, "Tw": 42070, "Ġnac": 42071, "frei": 42072, "Ġretard": 42073, "iscern": 42074, "Ġtallest": 42075, "ĠLuca": 42076, "Rah": 42077, "Ġpreacher": 42078, "Ġjut": 42079, "ĠRica": 42080, "iciency": 42081, "ĠÄijiá»ģu": 42082, "Ġkaufen": 42083, "Ġnett": 42084, "Ġdiscut": 42085, "Ġdeprived": 42086, "¡Ń": 42087, "Ġspricht": 42088, "Ġenclosed": 42089, "ĠSubst": 42090, "ç§ij": 42091, "ĠRabbit": 42092, "prised": 42093, "Ġbitches": 42094, "ìŁģ": 42095, "çīĪ": 42096, "Ġtapa": 42097, "ĠEssen": 42098, "ĠBao": 42099, "Ġdevient": 42100, "ĠWuhan": 42101, "ĠTipp": 42102, "Ġdisast": 42103, "ÑģÑĤвÑĥ": 42104, "ublique": 42105, "Ġqualité": 42106, "Ġinadequate": 42107, "Ġbargaining": 42108, "ĠGotcha": 42109, "евиÑĩ": 42110, "ievous": 42111, "erton": 42112, "blue": 42113, "ĠìĽĢì§ģ": 42114, "Ġsandbox": 42115, "ĠRein": 42116, "親": 42117, "ĠìĿ´ê²ĥëıĦ": 42118, "Ġsax": 42119, "zogen": 42120, "unächst": 42121, "Ġherkes": 42122, "Ġ-,": 42123, "zeni": 42124, "rising": 42125, "Ġresposta": 42126, "Ġpromotions": 42127, "ĠUnterstüt": 42128, "ĠMAS": 42129, "Nothing": 42130, "otics": 42131, "ĠвÑĭй": 42132, "Ġrotates": 42133, "kien": 42134, "Ġhabla": 42135, "ĠDani": 42136, "union": 42137, "Ġwack": 42138, "Ġarchaeological": 42139, "ĠCurtis": 42140, "ĠHoriz": 42141, "Ġ골ë": 42142, "Ġwaiver": 42143, "åĺ¿": 42144, "Bon": 42145, "Ġrotated": 42146, "Ġpitcher": 42147, "Ġinad": 42148, "Ġhugs": 42149, "ĠNortheast": 42150, "×Ļת×Ļ": 42151, "Ġplea": 42152, "Ġcupcake": 42153, "ĠLY": 42154, "Ġfamili": 42155, "Ġgroo": 42156, "ĠBlair": 42157, "Ġlij": 42158, "Ġhabitats": 42159, "Ġcommunism": 42160, "osium": 42161, "bars": 42162, "ĠFreeman": 42163, "neo": 42164, "Ġdiffuse": 42165, "Ġcylinders": 42166, "ĠDebat": 42167, "íĸĪëĬĶëį°": 42168, "еÑĪе": 42169, "Ġfingerprints": 42170, "Ġamar": 42171, "вид": 42172, "ĠìłķëıĦë¡ľ": 42173, "Ġaffiliated": 42174, "ĠÑħоÑĩеÑĤ": 42175, "ãģ°ãģĦ": 42176, "Ġetiqu": 42177, "ĠchÃŃnh": 42178, "æģŃåĸľ": 42179, "Ġcruising": 42180, "ĠWeihn": 42181, "ç͵": 42182, "ĠTitanic": 42183, "ç´Ģ": 42184, "ĠNast": 42185, "Ġëĵ¤ë": 42186, "Ġвал": 42187, "Ġdemi": 42188, "ĠKristin": 42189, "MIN": 42190, "Ġrigor": 42191, "Ġmoto": 42192, "ĠLAKE": 42193, "ĠíĻľ": 42194, "Ġë§Įìķ½": 42195, "ĠStro": 42196, "Ġprototypes": 42197, "ĠLC": 42198, "ìĿ¸ìĿĦ": 42199, "ÑĢим": 42200, "Ġviolating": 42201, "Ġgiorno": 42202, "Ġchildish": 42203, "æ°Ķ": 42204, "Ġ×IJ×Ĺ×ĵ": 42205, "Ġoverdose": 42206, "agogue": 42207, "адÑĨ": 42208, "heus": 42209, "ĠговоÑĢÑı": 42210, "Ġincr": 42211, "Ġdebated": 42212, "ÙħÙĦ": 42213, "Ġchicks": 42214, "Ġquin": 42215, "LAUGHING": 42216, "Ġtightening": 42217, "Ġsupervisors": 42218, "ĠHawk": 42219, "ĠBaz": 42220, "ĠповÑĤоÑĢ": 42221, "Ġблок": 42222, "Äģn": 42223, "Ġdumping": 42224, "Ġfacto": 42225, "berger": 42226, "Ġarsenal": 42227, "ĠAfricans": 42228, "¡Ģ": 42229, "Ġcafeteria": 42230, "feeding": 42231, "quila": 42232, "ĠpaÅĦstwo": 42233, "ınt": 42234, "Ħ±": 42235, "Ġenvironmentally": 42236, "Ġdesprés": 42237, "ĠWilly": 42238, "ĠPaÅĦstwo": 42239, "ĠGG": 42240, "Ġchacun": 42241, "Ġdirectional": 42242, "Ġhört": 42243, "ĠðĿ": 42244, "enary": 42245, "Ġvoiced": 42246, "aģı": 42247, "Ġpope": 42248, "Ġcomrades": 42249, "ĠGibson": 42250, "ĠACC": 42251, "vik": 42252, "Ġmodelling": 42253, "Ġaggi": 42254, "ãģªãĤĵãģ§ãģĻ": 42255, "Ġconversions": 42256, "Ġaverages": 42257, "Ellie": 42258, "Ġgestellt": 42259, "ĠUE": 42260, "osaic": 42261, "ÐĴоÑĤ": 42262, "Say": 42263, "ĠÑģамого": 42264, "Ġmesures": 42265, "isiert": 42266, "gasp": 42267, "voice": 42268, "Ġcheckpoint": 42269, "Ġpercentages": 42270, "Ġdisrupted": 42271, "ĠTuc": 42272, "ĠHomer": 42273, "ĠWAY": 42274, "ĠTurks": 42275, "heen": 42276, "imoto": 42277, "ĠOC": 42278, "ÃŃna": 42279, "ziel": 42280, "Ġmudar": 42281, "ãĥIJãĤ¤": 42282, "gesetzt": 42283, "Ġmejores": 42284, "ĠCJ": 42285, "наÑĢÑĥж": 42286, "Ġmodulus": 42287, "Ġmodulation": 42288, "Ġreplies": 42289, "Ġlarva": 42290, "Ġgider": 42291, "ĠMandarin": 42292, "ĠпоÑģмоÑĤÑĢим": 42293, "Ġsacrificing": 42294, "Ġpreço": 42295, "Ġoysters": 42296, "ĠMyan": 42297, "ologue": 42298, "ĠWit": 42299, "Ġdû": 42300, "ĠLeuten": 42301, "Ġpater": 42302, "ĠKENNETH": 42303, "абаÑĤ": 42304, "arthy": 42305, "Ġsociedad": 42306, "Ġniño": 42307, "евой": 42308, "ĠjÄĻ": 42309, "Ġadvertised": 42310, "ĠPepsi": 42311, "uteur": 42312, "Ġmasse": 42313, "Ġscattering": 42314, "Ġyön": 42315, "Ġdesapare": 42316, "ĠHubble": 42317, "ĠHé": 42318, "krä": 42319, "ĠDare": 42320, "Ġoverride": 42321, "ĠElaine": 42322, "ĠDublin": 42323, "dullah": 42324, "Mat": 42325, "ĠGarr": 42326, "...'": 42327, "Ġadulthood": 42328, "EZ": 42329, "Ġbelangrijk": 42330, "ienza": 42331, "Ġuniverso": 42332, "Ġstellar": 42333, "íĶĦë": 42334, "Ġê²°êµŃ": 42335, "Ġconstellation": 42336, "ĠShelley": 42337, "Ġmultit": 42338, "Ġmascot": 42339, "Ġhospitalized": 42340, "ĠðĿĺ": 42341, "оÑĢÑĭ": 42342, "adia": 42343, "ĠMikey": 42344, "ĠAmerika": 42345, "Ġhairy": 42346, "Hold": 42347, "ắn": 42348, "kiego": 42349, "è§Ĥ": 42350, "à¹Ģà¸Ķ": 42351, "Ġrivalry": 42352, "ĠJonah": 42353, "Ġsurgeons": 42354, "Ġrelatable": 42355, "èĴ": 42356, "Ġswims": 42357, "Ġbillionaire": 42358, "modern": 42359, "Ġdocumenting": 42360, "ĠDae": 42361, "Ġswatch": 42362, "Ġpuisse": 42363, "Ġmasuk": 42364, "Ġmarc": 42365, "Ġkró": 42366, "ĠPetersburg": 42367, "ĠAristotle": 42368, "ixe": 42369, "Produ": 42370, "Ġними": 42371, "Ġkana": 42372, "ĠЩ": 42373, "Ġvomit": 42374, "ĠWorkers": 42375, "popular": 42376, "ĠBieber": 42377, "еÑĤи": 42378, "étique": 42379, "Ġencant": 42380, "gran": 42381, "fir": 42382, "Ġanthem": 42383, "ÑģÑĥдаÑĢ": 42384, "Last": 42385, "Ġhag": 42386, "Ġvicinity": 42387, "renched": 42388, "anding": 42389, "ĠголоÑģ": 42390, "ĠCorner": 42391, "ÐĴÑĭ": 42392, "osas": 42393, "ievers": 42394, "cional": 42395, "Ġvigor": 42396, "Ġrejoice": 42397, "ĠciÄħ": 42398, "Ġкоп": 42399, "Ġqualcosa": 42400, "dessus": 42401, "Ġев": 42402, "ĠScandin": 42403, "ĠSmooth": 42404, "ä½łè¯´": 42405, "hape": 42406, "Ġëĭ¬ëĿ¼": 42407, "ĠTU": 42408, "Ġlyric": 42409, "Ġbess": 42410, "éIJ": 42411, "ÑģÑĤÑĢÑĥменÑĤ": 42412, "ĠActing": 42413, "ĠOrchest": 42414, "école": 42415, "Ġdolor": 42416, "Ġíĭ°": 42417, "Ġvergessen": 42418, "Ġeyelids": 42419, "ĠTanz": 42420, "веÑĢж": 42421, "Ġìķłë": 42422, "ué": 42423, "Ġscène": 42424, "Ġìļ°ë¦¬ëĬĶ": 42425, "Ġcrate": 42426, "kick": 42427, "ĠTheme": 42428, "Ġ320": 42429, "Ġgarnish": 42430, "Ġmetre": 42431, "Ġconvex": 42432, "plants": 42433, "esian": 42434, "Ġê±°ì§Ģ": 42435, "Ġmédi": 42436, "ĠMedal": 42437, "130": 42438, "ĠAlma": 42439, "æľīé»ŀ": 42440, "Cola": 42441, "ĠваÑĢианÑĤ": 42442, "Ġgord": 42443, "Ġavanz": 42444, "Ġwhispering": 42445, "Ġintestine": 42446, "ÐłÐķ": 42447, "ĠLISA": 42448, "amız": 42449, "SPD": 42450, "Ġpec": 42451, "Ġpastors": 42452, "Ġmuá»ijn": 42453, "ocre": 42454, "Sun": 42455, "ĠÑĤакÑĥÑİ": 42456, "Ġrevital": 42457, "Ġincomes": 42458, "Ġdetailing": 42459, "ĠBacon": 42460, "Ġëħ¸ëŀĺë": 42461, "Ġparrot": 42462, "Ġcollaborated": 42463, "hesia": 42464, "Ġseva": 42465, "Ġphysicist": 42466, "ĠBACK": 42467, "׾×Ļ": 42468, "Ġbipolar": 42469, "Ïģεί": 42470, "cros": 42471, "Ġked": 42472, "Ġeconomical": 42473, "Ġendings": 42474, "Ġticks": 42475, "Ġê·¼": 42476, "ĠOliv": 42477, "ongs": 42478, "Ġcontinental": 42479, "Ġweiterhin": 42480, "Ġactivating": 42481, "Ġpollen": 42482, "ĠAnk": 42483, "bay": 42484, "Ġ׾×Ĺ": 42485, "ĠEggs": 42486, "ĠRAMSAY": 42487, "ĠBER": 42488, "ĠíĽ¨ìͬ": 42489, "Ġpassado": 42490, "Ġgroundbreaking": 42491, "presa": 42492, "Ġhilft": 42493, "ĠTechnically": 42494, "ÑĨий": 42495, "NI": 42496, "Ġturnout": 42497, "ĠLap": 42498, "ĠGwen": 42499, "ĠVikt": 42500, "Ġescola": 42501, "ĠCinema": 42502, "æ°¸": 42503, "ĠãģĨ": 42504, "Ġconsumo": 42505, "ĠPurdue": 42506, "Ġsemanas": 42507, "ĠPRESID": 42508, "ưng": 42509, "Ġsach": 42510, "æĢİ麼辦": 42511, "Ġsavage": 42512, "ĠRW": 42513, "Ġ550": 42514, "bold": 42515, "ĠSimmons": 42516, "Ġslang": 42517, "ĠNaru": 42518, "ĠTheo": 42519, "íĸĪëĭ¤": 42520, ".�": 42521, "Ġseizure": 42522, "Ġhive": 42523, "Ġcellphone": 42524, "奶": 42525, "iiii": 42526, "ĠMusical": 42527, "ĠNuclear": 42528, "è¡Ĺ": 42529, "áveis": 42530, "Ġprestige": 42531, "Ġbalm": 42532, "Ġrefill": 42533, "yah": 42534, "hart": 42535, "Ġtaps": 42536, "Ġdispose": 42537, "ĠMick": 42538, "Ġthermometer": 42539, "ãģªãĤī": 42540, "Ġobedient": 42541, "Ġinformações": 42542, "ĠWide": 42543, "mom": 42544, "Sud": 42545, "Ġsuspend": 42546, "ĠObserv": 42547, "ĠлеÑģ": 42548, "Ġtratar": 42549, "ĠKatrina": 42550, "Ġtheres": 42551, "äºŀ": 42552, "Ġtexted": 42553, "Ġstör": 42554, "Ġsnail": 42555, "ĠFiona": 42556, "Ġvictorious": 42557, "Ġlibrarian": 42558, "pract": 42559, "Ġfino": 42560, "ĠArms": 42561, "ppt": 42562, "luk": 42563, "Ġtyres": 42564, "Ġtoc": 42565, "ĠKommunen": 42566, "ç¯Ģ缮": 42567, "Ġrevolt": 42568, "Ġmotivates": 42569, "Ġbisexual": 42570, "Ġwus": 42571, "Ġhandlar": 42572, "ĠMUELLER": 42573, "Ġexpectancy": 42574, "Ġembody": 42575, "ĠPrimary": 42576, "åİŁåĽł": 42577, "ÑĢей": 42578, "Ġunscrew": 42579, "iantly": 42580, ",â̦": 42581, "Ġsnel": 42582, "Ġprevalence": 42583, "Ġeruption": 42584, "Ġdescriptive": 42585, "vag": 42586, "ĠбÑĥкв": 42587, "Ġmêmes": 42588, "Ġethn": 42589, "Ġhijos": 42590, "ĠAbdul": 42591, "ĠZahl": 42592, "belt": 42593, "Ġgöst": 42594, "ĠTheresa": 42595, "ĠSUN": 42596, "ĠBake": 42597, "Ġå¿«": 42598, "Ġoptics": 42599, "Ġapocalypse": 42600, "purpose": 42601, "Ġróżnych": 42602, "Ġcrus": 42603, "ĠÐĹем": 42604, "Ġhardened": 42605, "ĠTD": 42606, "Ġgraveyard": 42607, "ĠSiber": 42608, "ĠPorter": 42609, "Ġexplodes": 42610, "ĠSofia": 42611, "ĠÐĴедÑĮ": 42612, "Ġweakened": 42613, "æĺ¯æĪij": 42614, "ULL": 42615, "Ġpinky": 42616, "Ġchapel": 42617, "ĠFres": 42618, "ĠпÑĢиг": 42619, "MER": 42620, "ĠSchmidt": 42621, "ĠDud": 42622, "æŁ¥": 42623, "estens": 42624, "Ġnuance": 42625, "Ġmodifying": 42626, "ĠMöglichkeiten": 42627, "ĠAnat": 42628, "Ġeccentric": 42629, "ĠScrew": 42630, "ĠLeh": 42631, "Ġhomogeneous": 42632, "ĠTall": 42633, "ĠRicardo": 42634, "Ãļ": 42635, "igns": 42636, "ĠлиÑĪ": 42637, "Ġgefragt": 42638, "Run": 42639, "caster": 42640, "noise": 42641, "Ġasynchron": 42642, "ÄĻdzie": 42643, "Ġ×ŀ×Ĺ": 42644, "Ġsuppressed": 42645, "Arthur": 42646, "ήÏĤ": 42647, "âr": 42648, "dist": 42649, "Ġкад": 42650, "Ġhör": 42651, "Ġ135": 42652, "ĠMozart": 42653, "ĠÑģобÑĭÑĤи": 42654, "ĠNursing": 42655, "ĠHahah": 42656, "ĠDop": 42657, "Ġpoliceman": 42658, "´ìĹIJìĦľ": 42659, "Ġê´Ģ볨": 42660, "hyuk": 42661, "Ġrugged": 42662, "Ġnuggets": 42663, "ĠComms": 42664, "Stud": 42665, "ĠÑģвое": 42666, "Ġczasie": 42667, "ãĤ½": 42668, "Ġrégion": 42669, "Ġfishermen": 42670, "ĠLT": 42671, "Ãĵ": 42672, "ciaż": 42673, "hei": 42674, "Ġcrumbs": 42675, "ĠImmer": 42676, "ĠFeld": 42677, "these": 42678, "Ġadvertisers": 42679, "Ġroaming": 42680, "Ġfunniest": 42681, "ĠNYU": 42682, "Ġhehe": 42683, "Ġpoking": 42684, "ĠìķĪëı¼": 42685, "istical": 42686, "Ġopaque": 42687, "uç": 42688, "wire": 42689, "ĠWeber": 42690, "ĠJacques": 42691, "Ġ210": 42692, "üp": 42693, "uyu": 42694, "Ġenfermed": 42695, "Ġbumped": 42696, "ĠSew": 42697, "ĠChanel": 42698, "Ġpersönlich": 42699, "Ġbetrayal": 42700, "Ġalleviate": 42701, "Ġvähän": 42702, "Ġguesses": 42703, "ĠCeline": 42704, "assing": 42705, "stroke": 42706, "Ġì¡°ë": 42707, "å¤ı": 42708, "ĠÑĤеÑħнолог": 42709, "ĠоÑģÑĤÑĢ": 42710, "Ġsoient": 42711, "Dear": 42712, "Ġjs": 42713, "Ġgesprochen": 42714, "athi": 42715, "ç¿»": 42716, "Å¡e": 42717, "Set": 42718, "oger": 42719, "ĠRig": 42720, "ĠмеÑĩ": 42721, "Ġservicios": 42722, "ĠRut": 42723, "ĠÐŀй": 42724, "ĠMyanmar": 42725, "ifie": 42726, "Ġsnapping": 42727, "ĠKamera": 42728, "Ġfestive": 42729, "ĠFY": 42730, "ĠCarolyn": 42731, "Ñĸб": 42732, "Ġleggings": 42733, "Ġyat": 42734, "Ġergon": 42735, "Ġepisód": 42736, "Ġanomaly": 42737, "uestos": 42738, "Id": 42739, "Ġevacuation": 42740, "Ġgigabytes": 42741, "Ġandare": 42742, "ĠRent": 42743, "mt": 42744, "istine": 42745, "Ġestrat": 42746, "ettu": 42747, "Ġreceber": 42748, "Ġdramat": 42749, "ricular": 42750, "alnız": 42751, "ĠSeni": 42752, "Ġoyn": 42753, "ĠChemical": 42754, "ĠÑģÑħ": 42755, "Ġturf": 42756, "Ġ1917": 42757, "iscernible": 42758, "Ġmantener": 42759, "Ġexcer": 42760, "Ġspectral": 42761, "Ġneuroscience": 42762, "Ġmicrof": 42763, "Ġforeigner": 42764, "ĠLanka": 42765, "ä½łåı¯ä»¥": 42766, "ĠÑĤвоÑĢ": 42767, "Ġtossed": 42768, "Ġpoblación": 42769, "Ġmateix": 42770, "Ġsiellä": 42771, "Ġott": 42772, "Ġcompuls": 42773, "akukan": 42774, "Ġmanifested": 42775, "Ġìĵ¸": 42776, "Ġutmost": 42777, "Ġreversal": 42778, "Ġplacebo": 42779, "Ġblat": 42780, "ĠStunde": 42781, "manship": 42782, "Ġatte": 42783, "ĠìĨĮê°ľ": 42784, "Ġistem": 42785, "Ġannat": 42786, "ĠPlaystation": 42787, "Ġzad": 42788, "Ġquitting": 42789, "Ġfamine": 42790, "ĠRough": 42791, "ĠFlame": 42792, "Ġheut": 42793, "Ġoportunidad": 42794, "Ġfaisait": 42795, "ĠDP": 42796, "Ġdiciendo": 42797, "ĠMelanie": 42798, "ĠCarne": 42799, "meg": 42800, "petto": 42801, "JUN": 42802, "ĠлÑİбой": 42803, "Ġoste": 42804, "ĠJJonak": 42805, "Ġtheatrical": 42806, "Ġinvinci": 42807, "Ġcommunion": 42808, "vocal": 42809, "Eh": 42810, "ĠDetails": 42811, "Ġstroll": 42812, "ĠRaymond": 42813, "ĠAmelia": 42814, "ij¥": 42815, "Ġprodukt": 42816, "Ġnuevas": 42817, "Ġmustn": 42818, "mayı": 42819, "colored": 42820, "dec": 42821, "Ġhjäl": 42822, "Ġsentimental": 42823, "Ġrealms": 42824, "Ġkrit": 42825, "Ġsext": 42826, "ĠPsychology": 42827, "èĪī": 42828, "hil": 42829, "ĠкоÑĢаб": 42830, "ĠëĤ´ìĿ¼": 42831, "ĠUnderstood": 42832, "ĠGuten": 42833, "Ġgangs": 42834, "Ġevenings": 42835, "æĢİæ¨£": 42836, "Ent": 42837, "ĠLegacy": 42838, "ĠCongo": 42839, "Ġdurchaus": 42840, "Ġbuoy": 42841, "erella": 42842, "WAN": 42843, "Pre": 42844, "ĠÑĢед": 42845, "ĠCrisis": 42846, "ãģªãģŁ": 42847, "ĠìĿ¼ìĿ´": 42848, "Ġmanuscripts": 42849, "еÑĤÑĢ": 42850, "Ġnonprofits": 42851, "Ġdictator": 42852, "Ġbaskets": 42853, "ĠIsh": 42854, "Ġperto": 42855, "Ġdatasets": 42856, "Ġample": 42857, "gebaut": 42858, "Ġcontributor": 42859, "Ġciao": 42860, "Ġconfirming": 42861, "ĠUCLA": 42862, "âϬ": 42863, "ĠÑģн": 42864, "Ġoverturn": 42865, "åIJī": 42866, "Ġunrealistic": 42867, "ĠPiece": 42868, "ocate": 42869, "Ġfällt": 42870, "pox": 42871, "Ġë³´ìĭľë©´": 42872, "Ġë©Ķë": 42873, "ĠCreation": 42874, "Ñİда": 42875, "Ġ×Ķ×IJ": 42876, "Ġwhack": 42877, "olithic": 42878, "cely": 42879, "ĠÑģовÑĢем": 42880, "Ġsequential": 42881, "Ġprofesional": 42882, "Ġcools": 42883, "Ġrepente": 42884, "Ġaire": 42885, "ennes": 42886, "ritos": 42887, "ĠÐĴид": 42888, "Ġkör": 42889, "ĠBitte": 42890, "ulars": 42891, "Ġincorrectly": 42892, "Ġsharply": 42893, "Ġbombard": 42894, "ëĭĺìĿ´": 42895, "Ġchromosome": 42896, "Ġadvertisements": 42897, "hun": 42898, "ĠÑīоб": 42899, "ĠÐĶаже": 42900, "Ġbathtub": 42901, "ĠSno": 42902, "ÙIJÙij": 42903, "Ġbuffet": 42904, "ĠGrid": 42905, "ĠBrew": 42906, "iset": 42907, "ĠImportant": 42908, "ümüz": 42909, "Ġveto": 42910, "ĠWerk": 42911, "ĠSham": 42912, "kra": 42913, "ileen": 42914, "heard": 42915, "Ġdraining": 42916, "Ġklass": 42917, "Ġbakayım": 42918, "cture": 42919, "ä½łèªª": 42920, "amour": 42921, "Ġsponsorship": 42922, "Ġdistill": 42923, "Ġpatio": 42924, "Ġkomb": 42925, "Ġoverwhelmingly": 42926, "ĠJamaica": 42927, "uiten": 42928, "Little": 42929, "ĠLOT": 42930, "taÄĩ": 42931, "Ġcommanders": 42932, "ĠWatts": 42933, "ĠOptions": 42934, "ìĿ´ë©´": 42935, "ACT": 42936, "Ġindispens": 42937, "ĠForsch": 42938, "otom": 42939, "ĠÎŃÏĩει": 42940, "Ġpraising": 42941, "ĠìĺģìĥģìĿĦ": 42942, "Ġaman": 42943, "Ġhypnot": 42944, "thms": 42945, "Ġnaszej": 42946, "Ġmourning": 42947, "ĠSAY": 42948, "cyj": 42949, "ĠгоÑģÑĥдаÑĢ": 42950, "Ġcau": 42951, "mee": 42952, "Ġtadi": 42953, "Med": 42954, "Ġcalidad": 42955, "ãĥŁãĥ¼": 42956, "Ġstripe": 42957, "Ġεν": 42958, "ĠKaty": 42959, "ĠEscape": 42960, "ĠãĤĵ": 42961, "Ġmüsste": 42962, "ĠاÙĦا": 42963, "кÑĤ": 42964, "Ġjobbar": 42965, "ĠJeju": 42966, "orar": 42967, "ĠSerá": 42968, "ĠMessi": 42969, "áz": 42970, "ĠTran": 42971, "Ġpiercing": 42972, "Ġarithmetic": 42973, "Ġstaggering": 42974, "Ġplugging": 42975, "ĠKAR": 42976, "vl": 42977, "´ìĺ": 42978, "ĠRegierung": 42979, "ĠOczywiÅĽcie": 42980, "ĠEdgar": 42981, "Ġconductivity": 42982, "yelling": 42983, "vais": 42984, "adian": 42985, "Ġbulky": 42986, "ĠÑģÑĢав": 42987, "ĠпÑĢом": 42988, "Ġpaved": 42989, "Ġbends": 42990, "ĠSkillshare": 42991, "ĠMmmm": 42992, "ĠHorror": 42993, "Ġtumb": 42994, "Ġgoofy": 42995, "ĠMeow": 42996, "×Ļ׾×ķ": 42997, "ĠWass": 42998, "ĠScale": 42999, "ĠRak": 43000, "Ġprojecting": 43001, "Ġlinguistic": 43002, "ĠWorlds": 43003, "ensemble": 43004, "Ġpega": 43005, "stoppable": 43006, "Ġimbalance": 43007, "Ġø": 43008, "Ġthriller": 43009, "колÑĮкÑĥ": 43010, "Ġleftovers": 43011, "Ġcaveat": 43012, "ĠSTR": 43013, "undai": 43014, "Ġwatery": 43015, "ĠMarin": 43016, "ãĥ³ãĤ°": 43017, "Ġeggplant": 43018, "ĠJB": 43019, "ÙħÙĥÙĨ": 43020, "vidia": 43021, "ĠFIN": 43022, "icable": 43023, "Ġpodob": 43024, "Ġcohesive": 43025, "ĠVerfügung": 43026, "ĠPlato": 43027, "аÑĢиÑī": 43028, "Ġkot": 43029, "ĠÐŁÐ¾Ð¼": 43030, "ĠдокÑĥм": 43031, "Ġimplants": 43032, "issez": 43033, "Bre": 43034, "Ġgasps": 43035, "ĠTED": 43036, "rato": 43037, "JI": 43038, "Ġavenues": 43039, "ĠChong": 43040, "ladı": 43041, "رض": 43042, "Ġinici": 43043, "ĠSubaru": 43044, "æķħ": 43045, "éģĬæĪ²": 43046, "à¸ĭ": 43047, "Ġacht": 43048, "ĠArchitecture": 43049, "ĠвеÑīи": 43050, "ĠDevOps": 43051, "Ġtoppings": 43052, "Ġobsol": 43053, "aina": 43054, "ĠBangkok": 43055, "estruct": 43056, "Ġkob": 43057, "Ġëĵ¯": 43058, "ĠÑĢазнÑĭе": 43059, "Ġree": 43060, "Ġbijvoorbeeld": 43061, "ĠDemocracy": 43062, "à¹Ģรา": 43063, "ĠконÑĤ": 43064, "Ġseç": 43065, "Ġrahat": 43066, "Ġparliamentary": 43067, "ĠBash": 43068, "æĬĵ": 43069, "ziaÅĤ": 43070, "ITCH": 43071, "ĠBubble": 43072, "któ": 43073, "Whoa": 43074, "Ġflats": 43075, "æķĪ": 43076, "zne": 43077, "Ġservicio": 43078, "ĠDew": 43079, "Õ¸ÖĤ": 43080, "Ġunterstützen": 43081, "ĠWinds": 43082, "éĤ£ä¸ª": 43083, "ĠìĸĺëĬĶ": 43084, "Ġevaluations": 43085, "Ġreca": 43086, "Ġelves": 43087, "cheer": 43088, "Ġjal": 43089, "Ġrested": 43090, "Ġquienes": 43091, "ĠBrooke": 43092, "Ġë§ĪìĿĮìĹIJ": 43093, "Ġinten": 43094, "Ġoats": 43095, "Ġreferee": 43096, "Ġpneumonia": 43097, "Ġdelve": 43098, "peace": 43099, "eny": 43100, "Ġmostra": 43101, "ĠCannon": 43102, "ÏģοÏį": 43103, "ĠÐIJл": 43104, "Ġmonumental": 43105, "οÏįμε": 43106, "immers": 43107, "avian": 43108, "ĠделаеÑĤ": 43109, "Ġpitches": 43110, "ĠGrove": 43111, "Ġseminars": 43112, "Ġrécup": 43113, "ĠVoor": 43114, "Ġdeven": 43115, "ĠdB": 43116, "Ġboosting": 43117, "egan": 43118, "Ġwelt": 43119, "ĠGuatemala": 43120, "Ġmileage": 43121, "Ġbehand": 43122, "ĠWaar": 43123, "ĠSurf": 43124, "Ġcauliflower": 43125, "ĠTyr": 43126, "Ġmiteinander": 43127, "Ġdaring": 43128, "ĠSitting": 43129, "dled": 43130, "Ġresentment": 43131, "mÃ¤ÃŁig": 43132, "Ġfilmmaking": 43133, "warts": 43134, "thought": 43135, "ologique": 43136, "ĠCOR": 43137, "Ġaccounted": 43138, "Ġaper": 43139, "ĠINT": 43140, "olare": 43141, "Ġacompañ": 43142, "èŃĺ": 43143, "ĠÆ¡i": 43144, "ä¹Ŀ": 43145, "Ġmermaid": 43146, "ĠBentley": 43147, "atore": 43148, "Ġpren": 43149, "Ġethanol": 43150, "Ġastronomers": 43151, "seat": 43152, "keepers": 43153, "Ġexemption": 43154, "Ġamo": 43155, "ĠëĤĺìĦľ": 43156, "Ġinhal": 43157, "Ġbows": 43158, "ÑģкÑĥÑİ": 43159, "3000": 43160, "Ġfermentation": 43161, "Ġsinks": 43162, "Ġcomercial": 43163, "Ġstump": 43164, "Ġcele": 43165, "ĠSisters": 43166, "ĠRegister": 43167, "Ġsoort": 43168, "Ġnatomiast": 43169, "Ġ그림": 43170, "ĠÅŀey": 43171, "Ġhyped": 43172, "ĠRafael": 43173, "ĠEis": 43174, "ĠBasil": 43175, "ĠAssassin": 43176, "ĠAde": 43177, "rÃ¥n": 43178, "Ġonlar": 43179, "Ġmovimiento": 43180, "Ġadditionally": 43181, "Ġslit": 43182, "ĠChry": 43183, "ĠInterviewer": 43184, "׾ק": 43185, "Ġdisl": 43186, "Ġligger": 43187, "Ñĥки": 43188, "berish": 43189, "ĠÑĢÑıдом": 43190, "ARON": 43191, "],,": 43192, "Ġlumière": 43193, "Ġolvid": 43194, "Ġfreue": 43195, "ĠTing": 43196, "ĠKö": 43197, "Ġgeo": 43198, "Ġdyed": 43199, "ãģ§ãģį": 43200, "ÑĪей": 43201, "Ġżycie": 43202, "Ġie": 43203, "Ġtaxpayer": 43204, "ĠpeÅĤ": 43205, "Ġdécidé": 43206, "ĠcÅĵur": 43207, "Ġentwickelt": 43208, "ĠHQ": 43209, "KK": 43210, "odar": 43211, "Ġhone": 43212, "Ġconfiance": 43213, "Ġissuing": 43214, "Ġdiagnost": 43215, "ĠìŀĦ": 43216, "ĠкÑĢÑĥÑĤ": 43217, "ĠкаÑģ": 43218, "Ġþ": 43219, "Ġrestrictive": 43220, "ĠCastro": 43221, "ĠuÄŁ": 43222, "Ġempre": 43223, "ĠMoo": 43224, "ĠFigure": 43225, "phonetic": 43226, "Prof": 43227, "ĠпÑĢе": 43228, "Ġtilted": 43229, "ĠNegative": 43230, "ĠLimited": 43231, "meno": 43232, "lamation": 43233, "Ġtrustees": 43234, "Ġintensely": 43235, "Ġaçıl": 43236, "ĠUsed": 43237, "Ġzul": 43238, "Ġappreciative": 43239, "Ġtinc": 43240, "Ġconquest": 43241, "ĠعÙĨد": 43242, "Ġsuicidal": 43243, "Ġmulheres": 43244, "Ġdetach": 43245, "Ġkamera": 43246, "ĠAirPods": 43247, "INDISTINCT": 43248, "глий": 43249, "ĠëĥĦ": 43250, "Ġwrestle": 43251, "æ´Ĺ": 43252, "Ġfirearm": 43253, "Ġlire": 43254, "pra": 43255, "Ġjewels": 43256, "ĠCornell": 43257, "Ġíķłê²ĮìļĶ": 43258, "Ġsucker": 43259, "Ġnombreux": 43260, "ĠFerm": 43261, "ìĽIJìĿ´": 43262, "ĠPis": 43263, "ĠизÑĥÑĩ": 43264, "Ġmiten": 43265, "Ġcev": 43266, "ĠURLs": 43267, "ĠCAS": 43268, "Ġåı¯ä»¥": 43269, "finden": 43270, "Ġbravery": 43271, "ĠÑģлово": 43272, "Ġnenhuma": 43273, "Ġencuentra": 43274, "ĠShirley": 43275, "Ġpercept": 43276, "frames": 43277, "ĠRover": 43278, "ĠAlberta": 43279, "occ": 43280, "ĠëĿ¼ê³ł": 43281, "Ġsúper": 43282, "Ġpresume": 43283, "Ġgland": 43284, "Ġpacing": 43285, "Ġneurot": 43286, "Ġsno": 43287, "Ġplotted": 43288, "ĠpaÅĦstwa": 43289, "ĠOwner": 43290, "ĠDefence": 43291, "ridges": 43292, "Ġwallpaper": 43293, "onian": 43294, "Bro": 43295, "ĠAriana": 43296, "缴æİ¥": 43297, "kry": 43298, "Ġnarration": 43299, "Ġcriança": 43300, "ĠAlrighty": 43301, "ĠìĿ½": 43302, "Ġìĵ°ê³ł": 43303, "Ġliberated": 43304, "Ġexceeds": 43305, "Ġdominating": 43306, "Ġbakın": 43307, "lk": 43308, "Ġslapped": 43309, "ÐĹд": 43310, "umental": 43311, "gettable": 43312, "ĠRoz": 43313, "ĠGul": 43314, "ouvert": 43315, "Ġsmashing": 43316, "azuje": 43317, "Sir": 43318, "Ġgrated": 43319, "ä½łæľī": 43320, "ATT": 43321, "Ġarticulated": 43322, "Ġstora": 43323, "Ġextrater": 43324, "á»ī": 43325, "ÏĥÏī": 43326, "wir": 43327, "ĠMete": 43328, "Imp": 43329, "Ġhoor": 43330, "phase": 43331, "ĠÑĩÑĥд": 43332, "ĠбÑĢаÑĤ": 43333, "Ġidag": 43334, "Ġcinq": 43335, "Ġaparecer": 43336, "ĠICE": 43337, "åĪĹ": 43338, "Ġquieter": 43339, "Ġfalsch": 43340, "adic": 43341, "ĠплÑİÑģ": 43342, "ĠMenu": 43343, "uxe": 43344, "ĠTôi": 43345, "ĠMIL": 43346, "ĠHaj": 43347, "verbs": 43348, "Ġtubing": 43349, "Ġmachst": 43350, "Ġdall": 43351, "Ter": 43352, "Ġgelen": 43353, "Ġcucumbers": 43354, "Ġwidgets": 43355, "Ġdevrait": 43356, "Ġmike": 43357, "Ġintra": 43358, "íķŃ": 43359, "ĠÃħ": 43360, "ĠHund": 43361, "æ§ĭ": 43362, "quarter": 43363, "Ġew": 43364, "Ġkeluar": 43365, "Ġmats": 43366, "ĠTrick": 43367, "ĠInfinite": 43368, "ŀ¨": 43369, "Ġpeac": 43370, "ĠProte": 43371, "à¥Ī": 43372, "Ġ1700": 43373, "ĠRais": 43374, "à¹Ĭ": 43375, "ählt": 43376, "ifica": 43377, "aimer": 43378, "aÄĩ": 43379, "Ġakl": 43380, "ĠVolvo": 43381, "ĠTyson": 43382, "ĠRong": 43383, "irsin": 43384, "ĠâĻ¥": 43385, "Ġparody": 43386, "national": 43387, "pod": 43388, "ayd": 43389, "ambled": 43390, "Ġgovernmental": 43391, "Ġconfort": 43392, "icides": 43393, "Ġnasze": 43394, "ĠShepherd": 43395, "ĠKontakt": 43396, "Ġdisproportionately": 43397, "ĠклÑİÑĩ": 43398, "ĠtÃŃtulo": 43399, "Ġsina": 43400, "Ġcompositions": 43401, "ĠPF": 43402, "Ġverkl": 43403, "Ġsuivre": 43404, "Ġasta": 43405, "Ġstakeholder": 43406, "Ġsamma": 43407, "ĠBLACK": 43408, "Ġnodig": 43409, "Ġleva": 43410, "Ġjuegos": 43411, "Ġernst": 43412, "Ġbottoms": 43413, "ĠSignal": 43414, "Ġpollut": 43415, "Ġdura": 43416, "Musik": 43417, "Ġкомна": 43418, "ĠвÑģей": 43419, "alter": 43420, "ĠStef": 43421, "ĠBigQuery": 43422, "ĠVerantwortung": 43423, "Ġëĭ¹ìŰ": 43424, "Ġquizz": 43425, "ĠLetter": 43426, "ĠInvestment": 43427, "ÑĪÑĤ": 43428, "IJëį°": 43429, "Ġencoding": 43430, "Ġtänker": 43431, "ĠKw": 43432, "annie": 43433, "åĭĿ": 43434, "110": 43435, "Ġzwy": 43436, "Ġì§§": 43437, "Ġdaw": 43438, "estä": 43439, "Ġdeceive": 43440, "ĠLänder": 43441, "isko": 43442, "Ġpodstaw": 43443, "ĠPharaoh": 43444, "쳤": 43445, "éĻIJ": 43446, "últ": 43447, "Ġtyö": 43448, "Ġmusimy": 43449, "質": 43450, "Ġpc": 43451, "ĠNT": 43452, "ĠCostco": 43453, "Ġå°ı": 43454, "ĠÏĥοÏħ": 43455, "Ġunin": 43456, "rounds": 43457, "Ġreminders": 43458, "Ġpuisqu": 43459, "Ġkrijgen": 43460, "Ġworkflows": 43461, "neten": 43462, "ĠëIJĺì§Ģ": 43463, "Ġsleek": 43464, "Ġcoworkers": 43465, "amientos": 43466, "Ġwitches": 43467, "baar": 43468, "eties": 43469, "Ġunnatural": 43470, "ĠSick": 43471, "ĠEfendi": 43472, "ãĥ³ãĥĢãĥĽ": 43473, "jcie": 43474, "Ġchamado": 43475, "ìĺĢìĬµëĭĪëĭ¤": 43476, "ĠprzedsiÄĻbior": 43477, "Ġbookstore": 43478, "Ġìŀłê¹IJ": 43479, "ĠSepar": 43480, "angi": 43481, "Evet": 43482, "Ġemergencies": 43483, "ĠXML": 43484, "нд": 43485, "¥´ë©´": 43486, "Ġê¿Ī": 43487, "Ġëĵ¤ê³ł": 43488, "Ġsut": 43489, "ĠWiz": 43490, "å±ķ": 43491, "Ġdynamically": 43492, "operation": 43493, "dot": 43494, "Ġinefficient": 43495, "clears": 43496, "Ġmundane": 43497, "ĠVeronica": 43498, "èĮ¶": 43499, "رت": 43500, "pose": 43501, "pai": 43502, "Ġnylon": 43503, "Ġaumentar": 43504, "ĠalltsÃ¥": 43505, "vak": 43506, "Ġcapacidad": 43507, "ĠWrestling": 43508, "Ġfertile": 43509, "Ġmég": 43510, "ĠNano": 43511, "аÑĤели": 43512, "Ġìĸ´ì©": 43513, "Ġtoca": 43514, "ĠEg": 43515, "âģ": 43516, "Ġì³": 43517, "luent": 43518, "Ġsolem": 43519, "Ġcinemat": 43520, "ĠQuel": 43521, "Ġorbits": 43522, "ĠHarm": 43523, "ricanes": 43524, "Ġblurred": 43525, "å¦Ĥä½ķ": 43526, "ĠاÙĦذÙĬ": 43527, "Ġjin": 43528, "Ġgrenades": 43529, "Ġatroc": 43530, "Ġwherein": 43531, "Ġreplen": 43532, "ĠComics": 43533, "edaan": 43534, "Ġdenim": 43535, "Ġembarrassment": 43536, "ĠGomez": 43537, "ĠBusan": 43538, "ivities": 43539, "Ġsaliva": 43540, "Ġmerk": 43541, "Ġilgili": 43542, "ĠкÑĢÑĥг": 43543, "Ġoccupational": 43544, "ĠSahib": 43545, "Sta": 43546, "Ġadviser": 43547, "ĠTruly": 43548, "ĠYEAH": 43549, "ĠìŀĪëĬĶëį°ìļĶ": 43550, "zew": 43551, "baren": 43552, "Ġstol": 43553, "Ġbelongings": 43554, "ĠResearchers": 43555, "Ġefendim": 43556, "ÏħÏĩ": 43557, "ÅĤÄħcz": 43558, "ĠUng": 43559, "ĠJub": 43560, "Ġcerebral": 43561, "á»ĩu": 43562, "Ġצר": 43563, "ĠподаÑĢ": 43564, "Ġmarched": 43565, "Ġawaken": 43566, "Ġako": 43567, "Ġacept": 43568, "Ġinitiation": 43569, "è¯ī": 43570, "lot": 43571, "ĠwÅĤas": 43572, "ĠMongol": 43573, "utral": 43574, "Ġtentang": 43575, "Ġinversion": 43576, "ĠìĿ´íĽĦ": 43577, "Ġlok": 43578, "ÅĤbym": 43579, "RS": 43580, "Ġstos": 43581, "Ġinteracts": 43582, "ĠCalendar": 43583, "Ġvanish": 43584, "Ġphysiology": 43585, "Ġlinearly": 43586, "ĠJY": 43587, "ÄŁan": 43588, "funded": 43589, "iziert": 43590, "Ġzmian": 43591, "ĠGrill": 43592, "Ġunbelievably": 43593, "otechnology": 43594, "ĠCars": 43595, "ĠÙĨÛģ": 43596, "ĠFolge": 43597, "ĠBeverly": 43598, "äischen": 43599, "Ġaumento": 43600, "ìĽĮìĦľ": 43601, "Ġmailbox": 43602, "Ġsteeds": 43603, "ĠPeak": 43604, "å·§": 43605, "Ġwykor": 43606, "Ġprawda": 43607, "иÑĤÑĭ": 43608, "Ġdiscours": 43609, "Ġaccuse": 43610, "cesso": 43611, "uire": 43612, "Ġпопад": 43613, "Ġtha": 43614, "Ġmeasurable": 43615, "beeping": 43616, "ĠInnen": 43617, "ĠпÑıÑĤÑĮ": 43618, "Ġcompeted": 43619, "ĠItalians": 43620, "Ġencontra": 43621, "Ġniew": 43622, "Ġfiltration": 43623, "ĠпÑĢоÑĦеÑģÑģ": 43624, "Ġpajamas": 43625, "Ġcilantro": 43626, "ĠSoc": 43627, "Luc": 43628, "Ġê¹Ģë": 43629, "ĠOdd": 43630, "Ġhydration": 43631, "мов": 43632, "Ġplywood": 43633, "ĠCompetition": 43634, "изнеÑģ": 43635, "flight": 43636, "ĠBeit": 43637, "bourg": 43638, "Ġcoils": 43639, "Ġcâmera": 43640, "Ġamended": 43641, "Äģm": 43642, "Angel": 43643, "ĠStacy": 43644, "flo": 43645, "Ġnormale": 43646, "Ġconsonant": 43647, "Ġaccompanying": 43648, "кÑĸ": 43649, "Ġirritated": 43650, "ĠfÃ¥tt": 43651, "Ġcrocodile": 43652, "IJĺëĬĶ": 43653, "Ġalbeit": 43654, "ĠPhilosophy": 43655, "ç´¯": 43656, "ÅĨ": 43657, "ytic": 43658, "Ġrèg": 43659, "Ġfrança": 43660, "Ġattentive": 43661, "Ham": 43662, "Ġalrededor": 43663, "æĿ¿": 43664, "sei": 43665, "ĠÑģвид": 43666, "Ġgimbal": 43667, "Ġchina": 43668, "ĠðŁİ¶": 43669, "ĠÐĴам": 43670, "Ġstimulating": 43671, "ĠOra": 43672, "ytes": 43673, "Ġheft": 43674, "Ġhaters": 43675, "Ġcomplexes": 43676, "Ġ03": 43677, "ród": 43678, "clear": 43679, "Ġbesteht": 43680, "çķĻè¨Ģ": 43681, "wny": 43682, "moil": 43683, "Ġsloppy": 43684, "Ġinsignificant": 43685, "Ġdubbed": 43686, "Ġëĸł": 43687, "Ġconsigo": 43688, "лÑĥÑĪай": 43689, "Sn": 43690, "Ġ×Ķצ": 43691, "ĠÎĮ": 43692, "Ġnadzie": 43693, "Ġfreshmen": 43694, "taa": 43695, "ĠuwagÄĻ": 43696, "ĠFavorite": 43697, "ĠCriminal": 43698, "Ġeviden": 43699, "Ġsymb": 43700, "Les": 43701, "ĠBeau": 43702, "uned": 43703, "plement": 43704, "Ac": 43705, "Ġdermat": 43706, "ĠNolan": 43707, "Ñĭп": 43708, "Ġsitt": 43709, "Ġeverlasting": 43710, "Ġestavam": 43711, "Ġмик": 43712, "Ġkhác": 43713, "Ġinvit": 43714, "Ġtreble": 43715, "Ġjig": 43716, "mani": 43717, "Ġtuvo": 43718, "ĠRUS": 43719, "ĠErde": 43720, "ĠDziÄĻkujÄĻ": 43721, "Ġblueberries": 43722, "kell": 43723, "acions": 43724, "çĪ·": 43725, "ви": 43726, "LET": 43727, "Ġsprout": 43728, "Ġspor": 43729, "Ġbên": 43730, "ĠMona": 43731, "ĠContain": 43732, "ĠKeys": 43733, "озÑı": 43734, "Ġfunción": 43735, "Ġrappelle": 43736, "Ġevolves": 43737, "Ġscraping": 43738, "Ġcomentários": 43739, "Ġpratique": 43740, "Ġauxiliary": 43741, "ĠSponge": 43742, "Ñģким": 43743, "uvo": 43744, "ĠÑģамо": 43745, "Ġsank": 43746, "Ġhighways": 43747, "Ġinventions": 43748, "Ġиногда": 43749, "Ġcreatively": 43750, "Ġbenchmarks": 43751, "oncé": 43752, "alal": 43753, "Ġsotto": 43754, "Ġcalves": 43755, "ĠMov": 43756, "Ġlavender": 43757, "Ġeyeballs": 43758, "Ġawaiting": 43759, "ĠPaty": 43760, "ÙĦÙĩ": 43761, "Ġembroidery": 43762, "Ġduh": 43763, "Ġcamar": 43764, "ĠBOB": 43765, "Ġspaced": 43766, "ĠgÅĤos": 43767, "аемÑģÑı": 43768, "Ġescapes": 43769, "ĠRogue": 43770, "zcz": 43771, "èŀ": 43772, "¬ë¥¼": 43773, "ĠMoże": 43774, "ĠеÑģÑĤе": 43775, "ĠBurada": 43776, "éĮ²": 43777, "wd": 43778, "uuuu": 43779, "Ġsash": 43780, "ĠLub": 43781, "Ġnotebooks": 43782, "Ġmae": 43783, "Ġconflicting": 43784, "Ġsummertime": 43785, "acas": 43786, "Ġbauen": 43787, "blowing": 43788, "ạo": 43789, "Ġìĸ¸ìłľ": 43790, "ä»ĬæĹ¥ãģ¯": 43791, "ĠSenhor": 43792, "ĠiPhones": 43793, "ĠQuarter": 43794, "ĠìłľëĮĢë¡ľ": 43795, "uÃŁ": 43796, "Ġë§Ī무ë": 43797, "Ġsettlers": 43798, "Ġcrest": 43799, "Ġtransc": 43800, "æĽ¾": 43801, "Ġriots": 43802, "Ġclones": 43803, "ĠOprah": 43804, "ίζ": 43805, "Ġpals": 43806, ".......": 43807, "ãģĶãģĸãģĦãģ¾ãģĻ": 43808, "ĠÑĢоÑģÑģ": 43809, "ĠLaser": 43810, "Ġzaczy": 43811, "Ġsevi": 43812, "Ġregeneration": 43813, "ìĹ¼": 43814, "would": 43815, "Ġüzerine": 43816, "ĠStraÃŁe": 43817, "Ġvengeance": 43818, "Ġrer": 43819, "ĠSafari": 43820, "ĠHEY": 43821, "çķ«": 43822, "Ġsacar": 43823, "Ġimagem": 43824, "ĠBundest": 43825, "mesan": 43826, "ĠPaste": 43827, "Ġsizz": 43828, "ĠпоÑģÑĤÑĥп": 43829, "×Ķ×ķ": 43830, "trad": 43831, "Ġfrançaise": 43832, "ĠBou": 43833, "Ġbarre": 43834, "ĠZhi": 43835, "ĠGeez": 43836, "ihad": 43837, "Ġreconoc": 43838, "Ġpelig": 43839, "Ġindices": 43840, "Ġë°ĶëĢ": 43841, "Ġconduction": 43842, "Ġìķħ": 43843, "Ġzeker": 43844, "Ġfum": 43845, "ĠWür": 43846, "breaker": 43847, "Ġsprite": 43848, "Crowd": 43849, "Ġopener": 43850, "Ġolv": 43851, "Ġbuenas": 43852, "ĠSilk": 43853, "ĠHIM": 43854, "kop": 43855, "compl": 43856, "Ġpossono": 43857, "³Ģ": 43858, "Ġoscillator": 43859, "ĠSith": 43860, "èĥ¡": 43861, "ажи": 43862, "Ġraft": 43863, "hall": 43864, "Ġschneller": 43865, "Ġimporting": 43866, "Ġassembling": 43867, "Ġubiqu": 43868, "Ġactivates": 43869, "acci": 43870, "ĵľë¥¼": 43871, "Ġcomposers": 43872, "ĠACL": 43873, "Conf": 43874, "Ġì½ĺ": 43875, "ĠнекоÑĤоÑĢÑĭе": 43876, "Ġcandies": 43877, "åĬłåħ¥": 43878, "ĠMuss": 43879, "à¹ĥà¸Ĭ": 43880, "Ġduda": 43881, "ником": 43882, "meden": 43883, "Ġìĸ´ëķĮ": 43884, "ĠYeshua": 43885, "zag": 43886, "hodou": 43887, "Ġaloud": 43888, "ĠPalmer": 43889, "imize": 43890, "ãĤ·ãĥ§": 43891, "Ġmaritime": 43892, "Ġcommunal": 43893, "Ġbadges": 43894, "Ġrugby": 43895, "Ġmarshmallow": 43896, "Ġfiery": 43897, "Ġaccountant": 43898, "Ġabla": 43899, "ĠMonroe": 43900, "ĠFont": 43901, "ĠBoost": 43902, "ĠBarnes": 43903, "answer": 43904, "ĠBurning": 43905, "Ġä¸įæĺ¯": 43906, "Ġangef": 43907, "ĠWesley": 43908, "lls": 43909, "ìµ": 43910, "ש׾": 43911, "iliÅĽmy": 43912, "×IJף": 43913, "amura": 43914, "ĠFuj": 43915, "Ġpani": 43916, "ĠTrop": 43917, "arbeiten": 43918, "Ġrue": 43919, "ĠRare": 43920, "ängen": 43921, "ĠÑģмоÑĤÑĢеÑĤÑĮ": 43922, "ĠÐļаÑĢ": 43923, "ĠMTV": 43924, "boarding": 43925, "][": 43926, "ĠëłĪë": 43927, "stanbul": 43928, "pielt": 43929, "ĠHardy": 43930, "ĠEngagement": 43931, "ĠDienst": 43932, "Ġwären": 43933, "Ġfuego": 43934, "Ġestruct": 43935, "Ġcalam": 43936, "ĠResponse": 43937, "ĠãĤĦ": 43938, "ĠMohammad": 43939, "Ġresisting": 43940, "Ġdurant": 43941, "èģ¯": 43942, "åĨµ": 43943, "ĠOLED": 43944, "Ġverz": 43945, "män": 43946, "ĠÙĨÛĴ": 43947, "Ġparanoid": 43948, "ĠAware": 43949, "ĠEngineers": 43950, "Ġprocedural": 43951, "Ġpersonnage": 43952, "Ġfarklı": 43953, "é¡Ĩ": 43954, "flowing": 43955, "ĠмеÑģÑĤа": 43956, "ĠBare": 43957, "istem": 43958, "ĠpoczÄħtku": 43959, "Ġpersonajes": 43960, "Ġìĸ´ëłµ": 43961, "Ńī": 43962, "ĠХоÑĤÑı": 43963, "Ġunsett": 43964, "ĠAbsol": 43965, "Ġấy": 43966, "ĠMAYOR": 43967, "полне": 43968, "Ġinforming": 43969, "Ġamps": 43970, "ÐŁÑĢ": 43971, "ĠëŃĶ": 43972, "aeda": 43973, "Ġ×Ķ×ij×": 43974, "ấn": 43975, "kelijk": 43976, "Ġatheist": 43977, "Ġtrout": 43978, "Ġneues": 43979, "ĠNokia": 43980, "machen": 43981, "Ġwholesale": 43982, "ırd": 43983, "Ins": 43984, "ĠÑįп": 43985, "Ġprick": 43986, "ĠKindern": 43987, "à¸Ĺำ": 43988, "Ġclassy": 43989, "Ġînt": 43990, "ĠShopify": 43991, "ĠÑģоÑĢ": 43992, "ĠзакÑĢÑĭ": 43993, "zuk": 43994, "Ġuniversally": 43995, "Ġteaspoons": 43996, "Ġrecount": 43997, "ĠnÃ¥gonting": 43998, "ĠXue": 43999, "isième": 44000, "Ġweakest": 44001, "ĠteÅŁekkür": 44002, "Ġmathematically": 44003, "ĠHos": 44004, "Ġíķľëĭ¤": 44005, "Ġpartager": 44006, "ĠDarr": 44007, "êº": 44008, "Ġεκ": 44009, "Ġgerms": 44010, "Ġgelir": 44011, "Ġdul": 44012, ",-": 44013, "Ġìĸ¸ë": 44014, "Ġ×ŀצ": 44015, "ĠÑıÑĢ": 44016, "Ġquotid": 44017, "Ġprzysz": 44018, "Ġhardness": 44019, "Ġaquatic": 44020, "ĠJungle": 44021, "ĠPCR": 44022, "ĠEliot": 44023, "Ġostr": 44024, "Ġmapa": 44025, "essä": 44026, "ĠGIR": 44027, "ĠDriving": 44028, "ĠSami": 44029, "ĠMedien": 44030, "ĠCompanies": 44031, "ĠPharm": 44032, "seits": 44033, "ĠRim": 44034, "ĠοÏĢο": 44035, "Ġweiteren": 44036, "Ġpizzas": 44037, "ĠLydia": 44038, "ĠHeights": 44039, "Ġsincerity": 44040, "Ġnossas": 44041, "ĠdÅĤ": 44042, "Ġalarming": 44043, "ĠCauc": 44044, "ĠÑģмÑĭÑģ": 44045, "facing": 44046, "bags": 44047, "WW": 44048, "ĠØ´ÙĬ": 44049, "Ġcourtroom": 44050, "ĠPhillip": 44051, "Ġê²ĥì²ĺëŁ¼": 44052, "ĠSpieler": 44053, "ãĤıãģĭ": 44054, "Ġkant": 44055, "Ġadmitting": 44056, "ãĥģãĥ£ãĥ³ãĥįãĥ«": 44057, "Ġcontainment": 44058, "å¼ł": 44059, "Ġremovable": 44060, "Ġjumper": 44061, "focused": 44062, "ĠиÑĤоге": 44063, "ĠТем": 44064, "Ġvase": 44065, "ĠUSC": 44066, "ĠMonate": 44067, "ĠJacobs": 44068, "ĠHOL": 44069, "iked": 44070, "erweise": 44071, "Ġgoodies": 44072, "Ġhomage": 44073, "׼ש×Ļ×ķ": 44074, "Ġquais": 44075, "Ġinicial": 44076, "Ġguarding": 44077, "Ġdazz": 44078, "Ġcombos": 44079, "ĠÑĥпÑĢав": 44080, "ĠTalent": 44081, "å¥ĩæĢª": 44082, "Ġór": 44083, "Ġintermittent": 44084, "ĠMcCarthy": 44085, "Ġspans": 44086, "Ġtyre": 44087, "Ġquy": 44088, "èĪĪ": 44089, "jut": 44090, "ĠZent": 44091, "Ġgat": 44092, "大åĵ¥": 44093, "Ġscaffold": 44094, "Ġnecesario": 44095, "ĠZahlen": 44096, "ĠSAND": 44097, "ĠPU": 44098, "Everything": 44099, "----------------": 44100, "ĠвзÑıÑĤÑĮ": 44101, "Ġsparks": 44102, "Ġpendulum": 44103, "×ŀף": 44104, "Ġìĥīê¹": 44105, "Ġmultiplier": 44106, "Ġладно": 44107, "urat": 44108, "Ġupsetting": 44109, "è¡Ģ": 44110, "bak": 44111, "ĠìµľëĮĢ": 44112, "Ġanál": 44113, "ĠJOE": 44114, "Ġkosten": 44115, "ĠPatty": 44116, "ĠGuin": 44117, "cked": 44118, "ĠEgyptians": 44119, "ĠCitizens": 44120, "ר׼": 44121, "ĠÐķÑīе": 44122, "Ġйого": 44123, "Ġsnowfl": 44124, "Ġlekker": 44125, "Ġacost": 44126, "ĠBabe": 44127, "Ġgamble": 44128, "Ġadjective": 44129, "кими": 44130, "oys": 44131, "Ġmontre": 44132, "ĠHyundai": 44133, "Ġmoisturizing": 44134, "Ġmozzarella": 44135, "OOO": 44136, "Ġfacult": 44137, "Ġdoet": 44138, "Ġfearless": 44139, "Ġespresso": 44140, "Ġallora": 44141, "ĠCinc": 44142, "ãĥ¼ãĤ¸": 44143, "Ġconteúdo": 44144, "ĠPelosi": 44145, "Ġminder": 44146, "root": 44147, "Ġíķłë": 44148, "Ġпад": 44149, "ĠCalling": 44150, "ĠConfig": 44151, "ĠConsole": 44152, "insky": 44153, "énergie": 44154, "Ġsolitary": 44155, "оде": 44156, "Ġguarded": 44157, "160": 44158, "ĠпÑģиÑħ": 44159, "ĠShap": 44160, "Ġtitre": 44161, "ologne": 44162, "ĠпаÑĢÑĥ": 44163, "ĠPRE": 44164, "ãĥ¼ãĥī": 44165, "Ġln": 44166, "ĠMitgl": 44167, "ĠCarry": 44168, "Ġspind": 44169, "ĠCanton": 44170, "Ġkingdoms": 44171, "remo": 44172, "Ġraging": 44173, "Ġincapable": 44174, "ĠWR": 44175, "åĨįè§ģ": 44176, "ĠÑģобÑģÑĤвен": 44177, "ĠкакиÑħ": 44178, "ĠSHE": 44179, "ëĭ¹íŀĪ": 44180, "Ġscarcity": 44181, "Ġperde": 44182, "Ġexits": 44183, "ĠSinger": 44184, "Ġsupper": 44185, "Ġmunicipality": 44186, "ĠDiversity": 44187, "Ġtiro": 44188, "iels": 44189, "ĠlÃŃder": 44190, "Ġbluff": 44191, "Ġatra": 44192, "lys": 44193, "Ġmahd": 44194, "Ġcódigo": 44195, "ĠHarlem": 44196, "rule": 44197, "icity": 44198, "Ġsimplistic": 44199, "ĠKonst": 44200, "åģ¥": 44201, "ELLI": 44202, "Ġförsta": 44203, "Ġconstitutes": 44204, "ĠÑģÑĤоÑĢонÑĥ": 44205, "Ġurged": 44206, "ĠPanda": 44207, "ì°¨ë": 44208, "rece": 44209, "Ġpatriot": 44210, "ĠCrush": 44211, "Ġwink": 44212, "ойÑĤи": 44213, "urança": 44214, "Ġseizures": 44215, "Ġelectrod": 44216, "ĠDonkey": 44217, "ĠIU": 44218, "ĠMOS": 44219, "Ġalkal": 44220, "ì´ī": 44221, "besondere": 44222, "Ġparallels": 44223, "Ġbitterness": 44224, "ättre": 44225, "essional": 44226, "Ġsoybean": 44227, "Ġcollab": 44228, "ĠReporting": 44229, "å§Ķ": 44230, "Ġкомпании": 44231, "Ġwszyscy": 44232, "ĠCrunch": 44233, "iseen": 44234, "Ġambassadors": 44235, "ĠChev": 44236, "åįĪ": 44237, "овÑĭе": 44238, "sca": 44239, "ĠÑĢеÑĪил": 44240, "оÑĤо": 44241, "Ġgleichzeitig": 44242, "mern": 44243, "üst": 44244, "ĠHae": 44245, "³´ê²łìĬµëĭĪëĭ¤": 44246, "Ġshores": 44247, "Ġdepress": 44248, "Ġahor": 44249, "ĠSteuer": 44250, "ahh": 44251, "Ġrevise": 44252, "ĠÑģамÑĭе": 44253, "jat": 44254, "Ġherbal": 44255, "Ġcuánt": 44256, "Ġbuna": 44257, "niejsze": 44258, "Finally": 44259, "×ķ×ĸ": 44260, "cje": 44261, "ĠìŀĪê±°ëĵłìļĶ": 44262, "ĠëĤĺëĪ": 44263, "Ġprzest": 44264, "ãĥ¼ãĥł": 44265, "lica": 44266, "ĠDuch": 44267, "å°įå°į": 44268, "ÑĸйÑģÑĮ": 44269, "passen": 44270, "Ġsatisfies": 44271, "ĠAdditional": 44272, "Ġcámara": 44273, "еÑĩение": 44274, "Ġpomp": 44275, "Ġë§IJìĿ´": 44276, "ĠMills": 44277, "евид": 44278, "Ġrespectable": 44279, "Ġfilament": 44280, "Ġvender": 44281, "Ġmattered": 44282, "oure": 44283, "층": 44284, "Korean": 44285, "Ġestudio": 44286, "Ġcactus": 44287, "ĠVive": 44288, "ĠRag": 44289, "Ġcompliqué": 44290, "ĠÙĪÛģ": 44291, "Ġtao": 44292, "¦¿": 44293, "Since": 44294, "Ġjeopard": 44295, "ĠSell": 44296, "åºĶ": 44297, "ĠìĺĽ": 44298, "Ġketo": 44299, "Ġintelig": 44300, "ĠAngeb": 44301, "Ġtiden": 44302, "Ġsocio": 44303, "Ġreminiscent": 44304, "Ġcaregiver": 44305, "Space": 44306, "ĠExercise": 44307, "ĠBecome": 44308, "êts": 44309, "akk": 44310, "!..": 44311, "ĠÑģпÑĢоÑģ": 44312, "ĠαÏĢο": 44313, "Ġshootings": 44314, "Ġape": 44315, "ĠSammy": 44316, "ĠKung": 44317, "Ġcuál": 44318, "ĠLup": 44319, "æĿŁ": 44320, "ä¾Ĩåΰ": 44321, "ĠÑģÑĤÑĥд": 44322, "Ġsweeter": 44323, "Ġcomum": 44324, "ĠAds": 44325, "hyung": 44326, "ĠбÑĥдÑĥÑī": 44327, "Ġwaffle": 44328, "ĠOrb": 44329, "Ġlaut": 44330, "Ġforecasting": 44331, "åª": 44332, "Ġrapping": 44333, "Ġprefers": 44334, "Ġbenz": 44335, "Ġnik": 44336, "ĠBahn": 44337, "Ġsanding": 44338, "Ġimminent": 44339, "ĠпÑĢоблемÑĭ": 44340, "Ġdoivent": 44341, "ола": 44342, "Ġżycia": 44343, "ihu": 44344, "Ġexistem": 44345, "ĠInterior": 44346, "ĠTakes": 44347, "Ġtoddler": 44348, "Ġdictatorship": 44349, "ĠSmithson": 44350, "ĠAllahu": 44351, "ÏİÏģα": 44352, "ìķĺìĬµëĭĪëĭ¤": 44353, "ĠVote": 44354, "ĠSmells": 44355, "одно": 44356, "Ġhindsight": 44357, "VR": 44358, "ĠPatch": 44359, "ĠJahres": 44360, "Ġsouvenir": 44361, "Ġneutron": 44362, "Ġlongtime": 44363, "Ġsayin": 44364, "ä¹IJ": 44365, "asaki": 44366, "ĠоÑģÑĤанов": 44367, "Ġexpelled": 44368, "Ġcryptocurrencies": 44369, "ĠMurder": 44370, "ĠCitizen": 44371, "WAY": 44372, "Ġplu": 44373, "Ġlemonade": 44374, "Ġconveniently": 44375, "ĠHI": 44376, "Ġ2023": 44377, "ש×ķת": 44378, "аÑĨион": 44379, "Ġ뼰": 44380, "ĠÙĦÙĥÙĨ": 44381, "Ġнемножко": 44382, "Ġunused": 44383, "Ġmaioria": 44384, "Ġastrology": 44385, "ĠDownt": 44386, "Nick": 44387, "Ġpreoccup": 44388, "Ġdemain": 44389, "×ŀ×¢": 44390, "ĠводÑĭ": 44391, "ĠSanskrit": 44392, "Ġprêt": 44393, "Ġstranded": 44394, "Ġrefin": 44395, "ĠпÑĢиним": 44396, "ĠповеÑĢÑħ": 44397, "à¯į?": 44398, "Ġzrob": 44399, "Ġintertw": 44400, "ĠDavidson": 44401, "лена": 44402, "ĠпонÑıÑĤÑĮ": 44403, "ĠReno": 44404, "ĠполÑĥÑĩилоÑģÑĮ": 44405, "Ġcorrespondent": 44406, "ĠUran": 44407, "else": 44408, "··": 44409, "Ġtutoring": 44410, "Ġgranddaughter": 44411, "luded": 44412, "Ġstesso": 44413, "Ġhết": 44414, "Ġgegangen": 44415, "ĠÐĿÐIJ": 44416, "Ġantig": 44417, "background": 44418, "Ġgedaan": 44419, "Ġfavored": 44420, "ĠEmmanuel": 44421, "Ġiod": 44422, "Ġclamps": 44423, "Ġcomple": 44424, "ĠAdvance": 44425, "ĠìŀĪê³łìļĶ": 44426, "ĠRox": 44427, "ĠìĹIJë": 44428, "Ġintestines": 44429, "Ġpercussion": 44430, "Ġlegitimately": 44431, "ĠEternal": 44432, "family": 44433, "alog": 44434, "Brad": 44435, "ениÑĤÑĮ": 44436, "ĠÑģнаÑĩала": 44437, "Ġcerta": 44438, "Ġakkor": 44439, "ĠεδÏİ": 44440, "Ġoctave": 44441, "ĠVac": 44442, "моÑĤÑĢи": 44443, "ĠÃītats": 44444, "Ġlongue": 44445, "Ġdissoci": 44446, "ÑĢÑıд": 44447, "hein": 44448, "Ġpantalla": 44449, "Ġindications": 44450, "ĠLt": 44451, "ĠGrade": 44452, "è£Ŀ": 44453, "oine": 44454, "bug": 44455, "ĠVerizon": 44456, "ĠAlém": 44457, "Ġviennent": 44458, "ĠÑĩиÑģÑĤ": 44459, "ĠBeni": 44460, "ĠTsch": 44461, "ĠTP": 44462, "Ġinsulting": 44463, "ĠWeight": 44464, "Ġadaptations": 44465, "ĠhabÃŃan": 44466, "Ġclique": 44467, "oÅĽci": 44468, "juna": 44469, "Ġsuchen": 44470, "ĠGoes": 44471, "ĠExodus": 44472, "Cho": 44473, "Ġantis": 44474, "ĠíĮĮë": 44475, "seven": 44476, "ĠÑĩаÑģов": 44477, "Ġballistic": 44478, "zony": 44479, "ICIA": 44480, "ĠпÑĢеÑģÑĤ": 44481, "Ġsimplesmente": 44482, "ĠCollabor": 44483, "Fred": 44484, "ĠÑĤелеÑĦон": 44485, "ĠRavi": 44486, "íķ´ì¤": 44487, "пеÑĢв": 44488, "ĠìŀĪìľ¼ëĭĪê¹Į": 44489, "Ġót": 44490, "Ġaleg": 44491, "úp": 44492, "Ġdisregard": 44493, "Ġindent": 44494, "cloud": 44495, "chlagen": 44496, "Ġiterate": 44497, "Ġgeneralized": 44498, "ãģĹãģ¾ãģĹãģŁ": 44499, "ह": 44500, "eleri": 44501, "Ġdisastrous": 44502, "ĠÑģÑĤала": 44503, "³ij": 44504, "KNOWN": 44505, "Ġrichness": 44506, "Ġconscient": 44507, "ichts": 44508, "ĠÑįлем": 44509, "بد": 44510, "irens": 44511, "Ġhaunting": 44512, "ructures": 44513, "attack": 44514, "Ġcupcakes": 44515, "sque": 44516, "Ġnaszego": 44517, "Ġanthropology": 44518, "ãģŁãģł": 44519, "ãģµãģµ": 44520, "chae": 44521, "Ġdiscovers": 44522, "ĠPersonality": 44523, "ĠΤο": 44524, "ĠdiÄŁer": 44525, "åįĢ": 44526, "ĠнеÑij": 44527, "ĠAnita": 44528, "Ġ[âĻª": 44529, "ĠCarm": 44530, "ĠBenny": 44531, "ìĬ¬": 44532, "Ġpupil": 44533, "Ġocas": 44534, "ället": 44535, "jÅĽÄĩ": 44536, "大ä¸Ī夫": 44537, "amental": 44538, "ĠоÑĤноÑģ": 44539, "Ġpid": 44540, "Ġarmp": 44541, "REE": 44542, "ĠоÑĤкÑĢÑĭв": 44543, "Ġuda": 44544, "ĠSyndrome": 44545, "ĠStandards": 44546, "ãģĪãĤĭ": 44547, "Ġpointers": 44548, "Ġenam": 44549, "ĠTig": 44550, "ÃŃz": 44551, "Ġнами": 44552, "Ġunchanged": 44553, "Ġturmoil": 44554, "ứng": 44555, "!!\"": 44556, "5000": 44557, "Ġ물ìĸ´ë": 44558, "Ġmerging": 44559, "Ġentscheiden": 44560, "åĩºæĿ¥": 44561, "forme": 44562, "Ġtrimmed": 44563, "Ġdared": 44564, "Ġaspiration": 44565, "ĠMythical": 44566, "ĠHej": 44567, "ĠAlej": 44568, "ÑĨо": 44569, "оÑĤÑĥ": 44570, "Ze": 44571, "ĠинÑģÑĤÑĢÑĥменÑĤ": 44572, "ĠRTX": 44573, "Ġlocalized": 44574, "çļĦè¯Ŀ": 44575, "Ġsurrounds": 44576, "Ġempieza": 44577, "Ġclase": 44578, "Ġà¸ģ": 44579, "ĠRapid": 44580, "ominous": 44581, "igail": 44582, "ĠÑĪиÑĢ": 44583, "Ġlæ": 44584, "Ġzasad": 44585, "Ġunfolding": 44586, "?!?!": 44587, "ĠìĪľê°Ħ": 44588, "ĠPolski": 44589, "ĠKauf": 44590, "ĠCelt": 44591, "itic": 44592, "Ġtoolbox": 44593, "ĠPocket": 44594, "ĠìĦľë¡ľ": 44595, "Ġbelki": 44596, "Ġadmiration": 44597, "phr": 44598, "ĠProdukt": 44599, "ĠTruck": 44600, "ãģİ": 44601, "ĠdrauÃŁen": 44602, "waÅĤ": 44603, "ĠHebrews": 44604, "Ġíķĺê²Į": 44605, "ĠACE": 44606, "urgence": 44607, "aurais": 44608, "Ġcharitable": 44609, "ıt": 44610, "Ġarmas": 44611, "ĠGedanken": 44612, "reating": 44613, "porte": 44614, "Ġimprint": 44615, "fäh": 44616, "ĠподÑħод": 44617, "Ġoutset": 44618, "วà¸ģ": 44619, "енного": 44620, "Class": 44621, "Ġvanity": 44622, "ĠVOICES": 44623, "Ġ260": 44624, "resident": 44625, "USE": 44626, "Ġê°Ģìļ´ëį°": 44627, "é½": 44628, "Ġthroughput": 44629, "Ġcuma": 44630, "ìļ±": 44631, "ãĥ¼ãĥ³": 44632, "ĠплоÑī": 44633, "Ġpartis": 44634, "ĠAnimation": 44635, "§Īë": 44636, "Cre": 44637, "ötzlich": 44638, "Ġmagg": 44639, "Ġclumsy": 44640, "Ġbottlene": 44641, "Ġbirlikte": 44642, "ĠGamb": 44643, "Ġ׼ף": 44644, "Ġmetropolitan": 44645, "该": 44646, "æİĴ": 44647, "Ooh": 44648, "Ġobjections": 44649, "ĠÙħت": 44650, "Ġмел": 44651, "Ġremnants": 44652, "ĠXavier": 44653, "Rich": 44654, "Ġolsa": 44655, "ĠPill": 44656, "Ġgroans": 44657, "ĠNaruhodou": 44658, "ĠContract": 44659, "ада": 44660, "nai": 44661, "ĠÑĦиз": 44662, "Ġops": 44663, "ạt": 44664, "Ġparachute": 44665, "Ġnell": 44666, "ĠEntscheidung": 44667, "׾×Ļ×Ŀ": 44668, "Ġtruthful": 44669, "Ġsharper": 44670, "Ġbureaucracy": 44671, "cart": 44672, "ĠинÑĤ": 44673, "wiek": 44674, "Ġwillingly": 44675, "ĠHerman": 44676, "Ġmehrere": 44677, "Ġelites": 44678, "ĠArmor": 44679, "ãĥĪãĥŁãĥ¼": 44680, "Ġembora": 44681, "ĠRecogn": 44682, "ĠлÑİблÑİ": 44683, "ĠExcellence": 44684, "ibel": 44685, "Ġexporting": 44686, "ì²´ìłģ": 44687, "Kelly": 44688, "Cameraman": 44689, "Ġslips": 44690, "Ġfigura": 44691, "Ġãģ¡": 44692, "Ġkoll": 44693, "ĠPandemie": 44694, "çıŃ": 44695, "Ġtimed": 44696, "lieÃŁlich": 44697, "Ġ×ŀ׼": 44698, "ĠperÃŃodo": 44699, "å¿Ĺ": 44700, "ivat": 44701, "Ġquestionnaire": 44702, "Ġpériode": 44703, "ç©¶": 44704, "Ġsighs": 44705, "Ġallegiance": 44706, "ĠXV": 44707, "ĠKensuke": 44708, "ĠGesundheits": 44709, "Ġpositivo": 44710, "ĠJaneiro": 44711, "ĠSEE": 44712, "Ġاست": 44713, "ĠKelsey": 44714, "tober": 44715, "Ġαλλά": 44716, "ĠParent": 44717, "ĠDayton": 44718, "ĠBilder": 44719, "ourage": 44720, "Ġseres": 44721, "ĠmuchÃŃsimo": 44722, "ĠRealm": 44723, "ĠOFFICER": 44724, "ersonic": 44725, "ãĤĤãģ®": 44726, "onya": 44727, "Ġê¸ī": 44728, "Ġancestry": 44729, "ĠJurassic": 44730, "Ġcentigrade": 44731, "ấu": 44732, "ujÄħc": 44733, "mans": 44734, "Ġtio": 44735, "ĠMoż": 44736, "Ġtragen": 44737, "Ġstared": 44738, "Ġschematic": 44739, "Ġpassou": 44740, "Ġmeatballs": 44741, "ÅĤoÅĽÄĩ": 44742, "Ġsynchronous": 44743, "Ġpermis": 44744, "arial": 44745, "Ġzer": 44746, "Ġparity": 44747, "ĠAvatar": 44748, "indeer": 44749, "eston": 44750, "Ġmeidän": 44751, "ĠCly": 44752, "´ī": 44753, "Ġestrogen": 44754, "Ġcentimet": 44755, "çĻº": 44756, "Ġconvictions": 44757, "Ġpossiamo": 44758, "Ġperdu": 44759, "Ġpathogens": 44760, "ĠQuin": 44761, "ĠPrograms": 44762, "ĠPoints": 44763, "rament": 44764, "rail": 44765, "Ġvy": 44766, "Ġgraft": 44767, "Ġbart": 44768, "ĠLotus": 44769, "à¨": 44770, "Ġë³´ìĭľ": 44771, "ramer": 44772, "Father": 44773, "Ġëľ»": 44774, "Ġ×Ķ×Ŀ": 44775, "Ġtrazer": 44776, "Ġtark": 44777, "èces": 44778, "forth": 44779, "ĠÑģделали": 44780, "Ġzucchini": 44781, "Ġwaktu": 44782, "Ġentertained": 44783, "ĠMilliarden": 44784, "Ġshaky": 44785, "Ġprzede": 44786, "¸Įë": 44787, "Ġreversible": 44788, "ĠNAU": 44789, "uins": 44790, "érêt": 44791, "annen": 44792, "ĠHunting": 44793, "ĠFellow": 44794, "élior": 44795, "Ġrotations": 44796, "Ġgranny": 44797, "xton": 44798, "ĠÑģÑĤановиÑĤÑģÑı": 44799, "ĠнаÑĩал": 44800, "Ġarteries": 44801, "rió": 44802, "ĠполÑĮзов": 44803, "ĠÐijÑĭ": 44804, "Ġnovelty": 44805, "pound": 44806, "Ġweirdest": 44807, "Ġbois": 44808, "émie": 44809, "upl": 44810, "ATA": 44811, "Ġtehd": 44812, "ĠNir": 44813, "sınız": 44814, "!\",": 44815, "åijĬè¯ī": 44816, "Ġimmort": 44817, "Ġelk": 44818, "аниÑĩ": 44819, "Ġfabrication": 44820, "ĠNoise": 44821, "ĠAvant": 44822, "رÛĮ": 44823, "wat": 44824, "Ġwhooshing": 44825, "Ġ׼×Ļ": 44826, "ĠÐĹнаÑĩиÑĤ": 44827, "Ġcentrif": 44828, "ansing": 44829, "Sound": 44830, "ĠëĿ¼ë": 44831, "Ġcaptions": 44832, "à³į": 44833, "Ġorgas": 44834, "Ġdolphins": 44835, "ĠBlend": 44836, "ĠTaj": 44837, "ĠCCTV": 44838, "Ġinom": 44839, "Ġeditions": 44840, "Ġburnout": 44841, "Ġbättre": 44842, "ĠCasa": 44843, "ovich": 44844, "Ġmolten": 44845, "Ġblindfold": 44846, "ĠGue": 44847, "æĹ¶éĹ´": 44848, "Ġspinner": 44849, "Ġmöglichst": 44850, "ĠVÃł": 44851, "eneca": 44852, "Ġmédico": 44853, "å¹¹åĺĽ": 44854, "ástico": 44855, "Ġard": 44856, "ĠSundays": 44857, "ĠRemote": 44858, "Ġìĸ¼ë§Ī": 44859, "ĠtrÆ°á»Ľc": 44860, "ìħ¨ë": 44861, "Ġdopp": 44862, "ĠbeÄŁ": 44863, "icana": 44864, "ĠëĤĺì¤ijìĹIJ": 44865, "çİĩ": 44866, "Ġholiness": 44867, "direct": 44868, "ĠìĺģíĻĶ": 44869, "Ġculpa": 44870, "ĠStitch": 44871, "lightly": 44872, "амен": 44873, "ĠмеÑĪ": 44874, "ĠпеÑĩ": 44875, "Ġyhte": 44876, "osphere": 44877, "Ġìĵ°ëĬĶ": 44878, "ék": 44879, "Ġseriousness": 44880, "Ġgarments": 44881, "Ġconcise": 44882, "ĠSJ": 44883, "Ġverloren": 44884, "Ġparecer": 44885, "ĠUNC": 44886, "ìĬ¤íĥĢ": 44887, "Ġenfant": 44888, "Ġbomber": 44889, "ĠGift": 44890, "Ġì¢ĭëĭ¤": 44891, "Ġrhythms": 44892, "ĠKlar": 44893, "人æ°ij": 44894, "ownik": 44895, "ĠReverend": 44896, "Ġemitted": 44897, "lassen": 44898, "Ġrevenir": 44899, "Ġarising": 44900, "Ġprecisamente": 44901, "Ġinterpol": 44902, "ĠTenemos": 44903, "obed": 44904, "Ġtecnologia": 44905, "Ġnerede": 44906, "ĠVisa": 44907, "Ġsava": 44908, "Ġescrever": 44909, "Ġassaulted": 44910, "ĠFleisch": 44911, "ĠCouncillors": 44912, "Ġê°Ģê¹Į": 44913, "Ġbegg": 44914, "ĠDeveloper": 44915, "ĠBronze": 44916, "ĠBonus": 44917, "Ġרק": 44918, "fact": 44919, "Ġendlessly": 44920, "Ġmacam": 44921, "ĠrzeczywiÅĽcie": 44922, "Ġhovering": 44923, "ège": 44924, "Ġpoorest": 44925, "ĠSched": 44926, "mile": 44927, "issements": 44928, "acÄĥ": 44929, "Ġ립": 44930, "Ġvaccin": 44931, "Ġfuturistic": 44932, "ĠWindow": 44933, "паÑĢ": 44934, "ĠÑĢоÑģ": 44935, "Ġlowers": 44936, "acs": 44937, "ĠÐIJлекÑģанд": 44938, "ĠAlert": 44939, "ieme": 44940, "ĠCaucas": 44941, "Ġjaws": 44942, "Ġhunted": 44943, "ìĹ½": 44944, "ĠبÙĨ": 44945, "Ġ׾׳×ķ": 44946, "Ġturbines": 44947, "Ġlumps": 44948, "ĠAllies": 44949, "ahlt": 44950, "Ġsubscriptions": 44951, "Ġnouveaux": 44952, "uger": 44953, "bones": 44954, "Ġberry": 44955, "ĠìĦłë¬¼": 44956, "ĠManufact": 44957, "ĠLunch": 44958, "ê·¸ëŀĺ": 44959, "Ġhydrated": 44960, "Ġachei": 44961, "ĠYaz": 44962, "ĠTibetan": 44963, "ĠQuantum": 44964, "ĠJerome": 44965, "ĠоÑīÑĥÑī": 44966, "ован": 44967, "motion": 44968, "ĠController": 44969, "energetic": 44970, "ĠÑģкоÑĢо": 44971, "Ġvowels": 44972, "ĠÑĥжаÑģ": 44973, "Ġhoof": 44974, "ĠBullet": 44975, "imagin": 44976, "׳×Ļ×Ŀ": 44977, "Ġengagements": 44978, "ĠBlues": 44979, "Ġañad": 44980, "Ġfps": 44981, "Ġcaterp": 44982, "Ġsá»ij": 44983, "ĠTribe": 44984, "ç¶ļ": 44985, "пон": 44986, "iferation": 44987, "Ġrumah": 44988, "ĠPunj": 44989, "lab": 44990, "Ġcomprehension": 44991, "bringing": 44992, "Wo": 44993, "Ġtik": 44994, "Ġanyhow": 44995, "以åīį": 44996, "áticas": 44997, "Ġsitzen": 44998, "Ġkolay": 44999, "ĠConfederate": 45000, "ĠCalled": 45001, "Ġnaszych": 45002, "ĠdziÄĻki": 45003, "Ġcloak": 45004, "ĠGoog": 45005, "ĠAshe": 45006, "象": 45007, "enan": 45008, "ĠмÑĭÑĪ": 45009, "ĠвеÑĤ": 45010, "ĠSpo": 45011, "ĠSket": 45012, "ĠHenderson": 45013, "ilah": 45014, "ĠбезопаÑģ": 45015, "Ġsekali": 45016, "ìĸ´ê°Ģ": 45017, "Ġsnare": 45018, "Ġrằng": 45019, "Ġförsö": 45020, "szych": 45021, "Ġübers": 45022, "Ġstratég": 45023, "ĠìºIJë": 45024, "Ġrappers": 45025, "Ġcep": 45026, "ĠHasta": 45027, "Ġhorribly": 45028, "Ġfrüh": 45029, "Ġبع": 45030, "Ġmantle": 45031, "ãĢħ": 45032, "funding": 45033, "Ġzust": 45034, "ĠPens": 45035, "sed": 45036, "ĠíŤ": 45037, "Ġgereki": 45038, "Ġalarms": 45039, "ĠWha": 45040, "ĠMarkus": 45041, "aksi": 45042, "ĠÐIJле": 45043, "klore": 45044, "Ġéner": 45045, "Ġtilde": 45046, "boxing": 45047, "ĠìĦŀ": 45048, "Ġencontramos": 45049, "ĠPhar": 45050, "наком": 45051, "óst": 45052, "Ġİs": 45053, "Ġëĭĺ": 45054, "Ġsquats": 45055, "Ġpretended": 45056, "Ġdez": 45057, "Ġê´ľì°®ìķĦ": 45058, "jach": 45059, "ëĿ¼ê³ł": 45060, "ĠíĻķì§Ħ": 45061, "ĠAnsch": 45062, "imerk": 45063, "Ġconjugate": 45064, "Ġpeninsula": 45065, "Ġgorilla": 45066, "Ġphotographed": 45067, "ĠAunque": 45068, "Ġentren": 45069, "ĠDeutschen": 45070, "ĠAladdin": 45071, "Ġ무ìĦľ": 45072, "ĠStella": 45073, "ĠElection": 45074, "outine": 45075, "Grand": 45076, "ĠWak": 45077, "ĠSergio": 45078, "horse": 45079, "ahon": 45080, "ĠFamilies": 45081, "Ġhating": 45082, "ĠBett": 45083, "à¸Ļะà¸Ħะ": 45084, "Ġcurling": 45085, "ĠIsraelis": 45086, "Ġ׾×IJ×": 45087, "ĠMyers": 45088, "Ġscanned": 45089, "ĠBEC": 45090, "ileri": 45091, "Ġcalle": 45092, "ĠMinh": 45093, "Ġmicron": 45094, "Ġconduc": 45095, "ÃŃv": 45096, "ĠвозÑĮ": 45097, "Ġactionable": 45098, "ĠTrustees": 45099, "Ġtief": 45100, "Ġheaders": 45101, "Ġanimales": 45102, "ìĽĢ": 45103, "лоÑħ": 45104, "unity": 45105, "lya": 45106, "Ġjangan": 45107, "Ġhani": 45108, "Ġcasing": 45109, "Ġjóvenes": 45110, "ĠSplit": 45111, "ĠCarlo": 45112, "ĠBeim": 45113, "å°įä¸įèµ·": 45114, "Ġnuanced": 45115, "Ġteddy": 45116, "ĠClan": 45117, "ächen": 45118, "pier": 45119, "Ġдополн": 45120, "Ġdiaper": 45121, "effective": 45122, "ĠNiagara": 45123, "Ġwart": 45124, "Ġcorro": 45125, "ĠKampf": 45126, "zte": 45127, "Ġdéveloppement": 45128, "Ġattackers": 45129, "ĠSherman": 45130, "Ġ1914": 45131, "Ġmeow": 45132, "ĠPÃ¥": 45133, "ìº": 45134, "cit": 45135, "Ġcoupe": 45136, "Ġê·¸ëĭ¤ìĿĮìĹIJ": 45137, "Ġhumour": 45138, "Ġcole": 45139, "ĠWarning": 45140, "ĠTil": 45141, "calm": 45142, "buat": 45143, "Ġcine": 45144, "kiej": 45145, "Kevin": 45146, "Ġmilligrams": 45147, "×ĵר": 45148, "ariamente": 45149, "Ġoro": 45150, "ĠHod": 45151, "ertos": 45152, "Ġlihat": 45153, "Ġfullest": 45154, "Ġgrandi": 45155, "Ġбок": 45156, "Ġwholly": 45157, "Ġmahdoll": 45158, "Ġcontroll": 45159, "ĠBunun": 45160, "èĬĤ": 45161, "Ġdipped": 45162, "Ġregión": 45163, "ĠÙĦÙĪ": 45164, "Ġбаг": 45165, "Ġpremiers": 45166, "Ġchá»ĭ": 45167, "ĠæīĢ以": 45168, "è±Ĩ": 45169, "idez": 45170, "Ġquota": 45171, "Ġghee": 45172, "arkan": 45173, "Ġgelatin": 45174, "ĠClerk": 45175, "bbles": 45176, "ĠPaige": 45177, "Ġstaged": 45178, "Ġsociais": 45179, "ĠBizim": 45180, "Ġvelocidade": 45181, "Ġmalaria": 45182, "Ġshortened": 45183, "Ġsalut": 45184, "ĠHehe": 45185, "Ġvá»ĭ": 45186, "ĠTaiwanese": 45187, "ĠArri": 45188, "gres": 45189, "åİ»äºĨ": 45190, "()": 45191, "riad": 45192, "ijIJë": 45193, "Ġãģ¾ãģĻ": 45194, "Ġmasculinity": 45195, "LP": 45196, "Ġëĸ¡": 45197, "Ġtérmin": 45198, "ĠVä": 45199, "ĠSeiten": 45200, "Ġrespectfully": 45201, "áo": 45202, "Ġtotalement": 45203, "Ġscraps": 45204, "Ġinfring": 45205, "ĠBose": 45206, "amar": 45207, "ĠLuiza": 45208, "ĠARM": 45209, "ĠплоÑħо": 45210, "Ġmeillä": 45211, "ĠDion": 45212, "å¼Ģå§ĭ": 45213, "Ġsouha": 45214, "Ġgeschafft": 45215, "Ġconvolution": 45216, "ĠâĢijâĢij": 45217, "Ġ144": 45218, "lingt": 45219, "Ġmännisk": 45220, "Ġgustado": 45221, "Ġcoined": 45222, "ĠLulu": 45223, "å®ĥçļĦ": 45224, "opot": 45225, "ĠPrayer": 45226, "Ġroasting": 45227, "Ġchromosomes": 45228, "飯": 45229, "еле": 45230, "Blue": 45231, "ĠErfolg": 45232, "èĩªçͱ": 45233, "ĠпÑĢидÑĥм": 45234, "Ġrisking": 45235, "ĠGuardians": 45236, "Ġ2024": 45237, "èse": 45238, "ĠбÑĥдÑĤо": 45239, "Ġconserve": 45240, "ĠBringing": 45241, "ĠAstra": 45242, "à¹Ģà¸Ĥ": 45243, "ĠкакÑĥÑİ": 45244, "respace": 45245, "ĠÐŀп": 45246, "ĠвокÑĢÑĥг": 45247, "æħĭ": 45248, "Ġmasked": 45249, "ĠShy": 45250, "ĠNim": 45251, "endas": 45252, "Ġíı¬ìĿ¸": 45253, "Ġ모ìĸij": 45254, "Ġvaleur": 45255, "ĠNegro": 45256, "ĠCDs": 45257, "inkling": 45258, "Ġmontón": 45259, "ĠHond": 45260, "Real": 45261, "Ġfullness": 45262, "ĠWhoops": 45263, "ĠShank": 45264, "ĠBran": 45265, "Ġtransluc": 45266, "Ġerr": 45267, "ĠGardens": 45268, "oyu": 45269, "Ġaffirmative": 45270, "ä¸ĭä¸Ģ": 45271, "Ġpottery": 45272, "live": 45273, "iau": 45274, "mount": 45275, "Ġfluctuations": 45276, "åŁİ": 45277, "ÃŃem": 45278, "Ġpulses": 45279, "Ġcrianças": 45280, "ίαÏĤ": 45281, "Ġbasta": 45282, "ENNIS": 45283, "ĠкоÑĢп": 45284, "ĠFunk": 45285, "ĠéĢĻ": 45286, "Ã¥rt": 45287, "ĠзаÑĤем": 45288, "Ġparasites": 45289, "ãĥĻ": 45290, "Ġairflow": 45291, "ĠXuan": 45292, "Gülme": 45293, "Ġblooming": 45294, "Ġmummy": 45295, "Ġbao": 45296, "ĠClap": 45297, "antics": 45298, "skin": 45299, "centric": 45300, "before": 45301, "ĠRICHARD": 45302, "ĠHahn": 45303, "TAKE": 45304, "ĠÑĤÑĢеÑĤÑĮ": 45305, "Ġpressured": 45306, "ĠKurz": 45307, "isti": 45308, "ĠнаÑĪего": 45309, "Ġsemiconductor": 45310, "ĠClint": 45311, "Ġplup": 45312, "ĠOrigin": 45313, "ĠEvents": 45314, "Ġê±±ìłķ": 45315, "mpfen": 45316, "NEY": 45317, "ĠDW": 45318, "Ġë¶ģíķľ": 45319, "Ġinforms": 45320, "Ġforsk": 45321, "Ġamiga": 45322, "ĠCincinn": 45323, "Str": 45324, "Ġparish": 45325, "Ġ커íĶ": 45326, "Ġsizi": 45327, "Ġplantation": 45328, "Ġbliver": 45329, "ĠполиÑĤ": 45330, "Ġsubdiv": 45331, "Ġrant": 45332, "Ġprincipals": 45333, "åIJ¦": 45334, "Ġkunne": 45335, "ügen": 45336, "arespace": 45337, "Ġvallahi": 45338, "Ġcollapsing": 45339, "اÙĦÙħ": 45340, "Ġlider": 45341, "Ġtama": 45342, "Ġgagner": 45343, "rolle": 45344, "Ġë§IJìĶĢëĵľë": 45345, "Ġcathedral": 45346, "ĠWebs": 45347, "ĠPolitics": 45348, "ãģĹãģ¾": 45349, "ãģ£ãģ¦ãĤĭ": 45350, "ĠDenis": 45351, "Ġtuo": 45352, "Ġrefract": 45353, "Ġdisintegr": 45354, "stes": 45355, "ĠлÑİбов": 45356, "Ġwilt": 45357, "Ġtrusts": 45358, "Ġkomun": 45359, "ĠBasket": 45360, "~!!": 45361, "nae": 45362, "ĠÐļол": 45363, "Ġsyllables": 45364, "ĠHenri": 45365, "ĠNab": 45366, "ÙĪØ¹": 45367, "Ġwn": 45368, "Ġkamp": 45369, "ĠPrague": 45370, "ĠBreakfast": 45371, "Ġê·¸ëŁ´": 45372, "Ġchut": 45373, "Ġ330": 45374, "ĠIndustries": 45375, "ä¸į管": 45376, "ĠiÅŁi": 45377, "ĠGoldman": 45378, "Ġİns": 45379, "ussa": 45380, "ithe": 45381, "ĦIJ": 45382, "ĠSOUND": 45383, "алÑĮнÑĭм": 45384, ".(": 45385, "ĠгоÑĢаз": 45386, "Ġdagegen": 45387, "Ġë®": 45388, "Ġwaiter": 45389, "length": 45390, "ĠÏĥÏĦα": 45391, "Ġchunky": 45392, "Sa": 45393, "Ġrusty": 45394, "ĠJudith": 45395, "750": 45396, "Ġepoxy": 45397, "ì¹ł": 45398, "åı²": 45399, "metro": 45400, "Ġrejecting": 45401, "Ġsquishy": 45402, "Ġplupart": 45403, "Ġméth": 45404, "Ġaspiring": 45405, "ĠDrama": 45406, "Ġuplift": 45407, "§Īëĭ¤": 45408, "................": 45409, "ł¤ìļĶ": 45410, "Ġtécnica": 45411, "Ġpasando": 45412, "Those": 45413, "ĠÑĢаздел": 45414, "Ġmediocre": 45415, "ĠNickel": 45416, "Ġsuperheroes": 45417, "Ġmissionary": 45418, "ĠParece": 45419, "Ġrotational": 45420, "Ġprett": 45421, "ãģĿãģĨãģĿãģĨ": 45422, "Ġlama": 45423, "Ġcanyon": 45424, "Ġbeter": 45425, "ĠProvost": 45426, "Ġhvis": 45427, "Ġdeactiv": 45428, "ĠHels": 45429, "pflicht": 45430, "Something": 45431, "ĠPierce": 45432, "Ġê²Ģì°°": 45433, "lungen": 45434, "Ġsizing": 45435, "Ġlatitude": 45436, "ĠNonetheless": 45437, "omnia": 45438, "ĠSabrina": 45439, "ĠDynamic": 45440, "åĥ¹": 45441, "onta": 45442, "ìĨIJ": 45443, "Ġdirective": 45444, "ĠDepot": 45445, "Ġfueled": 45446, "Ġexpire": 45447, "Ġcomún": 45448, "ĠSexual": 45449, "ĠGore": 45450, "Ġrestless": 45451, "ĠJAKE": 45452, "ÑĤеÑĢеÑģ": 45453, "ĠÑĤÑĢан": 45454, "ĠHolz": 45455, "å°Ĩ": 45456, "ĠActor": 45457, "æĿ¯": 45458, "call": 45459, "Ġemailed": 45460, "ĠPear": 45461, "Ñĥди": 45462, "ÑĢал": 45463, "ĠmÃły": 45464, "ĠCHEERING": 45465, "å®īåħ¨": 45466, "Ġretailer": 45467, "Ġprotr": 45468, "Ġdiscarded": 45469, "ĠHIS": 45470, "Ġevangelical": 45471, "ĠElse": 45472, "Ġexplores": 45473, "Ġcriticizing": 45474, "ifik": 45475, "Ġwhipping": 45476, "Ġopis": 45477, "oused": 45478, "Free": 45479, "ĠíĮ¬": 45480, "Ġmics": 45481, "running": 45482, "Ob": 45483, "itié": 45484, "Ġnecesita": 45485, "ĠDominican": 45486, "ĠBagh": 45487, "Ġtendencies": 45488, "ĠMetropolitan": 45489, "Åijl": 45490, "Ġзнаем": 45491, "ĠZam": 45492, "ĠDeadpool": 45493, "ależ": 45494, "Ġinvestigative": 45495, "ĠPronunciation": 45496, "Ġemulate": 45497, "Ġbanco": 45498, "Ġ-âĻª": 45499, "åĪ»": 45500, "Ġoverarching": 45501, "liches": 45502, "ĠвозвÑĢаÑī": 45503, "ĠScary": 45504, "ĠKia": 45505, "åľŁ": 45506, "ronting": 45507, "inned": 45508, "ĠÛģÙĪ": 45509, "ìĪĺ를": 45510, "ç¾İåij³": 45511, "wel": 45512, "Ġë³Ħë¡ľ": 45513, "Ġunintention": 45514, "aaS": 45515, "Ġnicest": 45516, "ĠTesting": 45517, "ĠISIL": 45518, "ogenous": 45519, "ĠØŁ": 45520, "Ġlieutenant": 45521, "Ġbrauch": 45522, "ĠTir": 45523, "drive": 45524, "Ġtolerant": 45525, "Ġshooters": 45526, "ĠìĺĪë»IJ": 45527, "殺": 45528, "onton": 45529, "Ġteria": 45530, "ietet": 45531, "Ron": 45532, "leigh": 45533, "gae": 45534, "Ġolmak": 45535, "ĠClone": 45536, "sold": 45537, "Ġskeletons": 45538, "Ġincumbent": 45539, "оме": 45540, "CON": 45541, "Ġleven": 45542, "Ġmillennials": 45543, "Ġequator": 45544, "ĠFeder": 45545, "ĠAlexandra": 45546, "Ġvrij": 45547, "ĠHealthcare": 45548, "Ġíķij": 45549, "Ġemphasizing": 45550, "Ġdialogues": 45551, "Ġchilled": 45552, "Ġprow": 45553, "ĠPassion": 45554, "ĠLaden": 45555, "ariest": 45556, "aphrag": 45557, "Ġadditive": 45558, "ĠStaat": 45559, "ĠNept": 45560, "ĠHAM": 45561, "à¹Ģà¸Ń": 45562, "days": 45563, "ĠíĸĪëįĺ": 45564, "Ġvoila": 45565, "ĠÑħл": 45566, "ĠDeutsche": 45567, "quir": 45568, "Open": 45569, "Ġranged": 45570, "Ġlevers": 45571, "ĠMansion": 45572, "pared": 45573, "ĠTitans": 45574, "atoire": 45575, "Ġengages": 45576, "yez": 45577, "naden": 45578, "Ġobstruct": 45579, "ĠEmmy": 45580, "åķĨ": 45581, "°¥": 45582, "Ġtroph": 45583, "Ġtakeaways": 45584, "+.": 45585, "tycznie": 45586, "hésitez": 45587, "ĠpodÃŃa": 45588, "Ġ주ëĬĶ": 45589, "Ġcitation": 45590, "ĠAqua": 45591, "Ġdebugging": 45592, "ван": 45593, "Ġëĭ¹ìĭł": 45594, "ĠاÙĦÙĬ": 45595, "Ġinstantaneous": 45596, "ĠAutumn": 45597, "Ġkepada": 45598, "Ġgetan": 45599, "hini": 45600, "ynthesis": 45601, "ĠпеÑĢи": 45602, "ĠMaced": 45603, "Pac": 45604, "untu": 45605, "Bra": 45606, "ĠгоÑĢаздо": 45607, "Ġ1959": 45608, "ĠÑĤемпеÑĢ": 45609, "Ġsane": 45610, "ĠOUR": 45611, "asu": 45612, "Ġ무ìĹ": 45613, "Ġvalleys": 45614, "Ġlistings": 45615, "Ġprzedstaw": 45616, "Ġgummy": 45617, "Ġcortisol": 45618, "ĠObrig": 45619, "ĠAllied": 45620, "ожÑĥ": 45621, "Ġgénér": 45622, "Ġdocs": 45623, "ĠChili": 45624, "ĠAbdullah": 45625, "Kit": 45626, "Ġcontributors": 45627, "гоÑĢ": 45628, "леÑĢ": 45629, "Ġbinder": 45630, "Ġmodèle": 45631, "íħIJ": 45632, "Ġinteiro": 45633, "mis": 45634, "fera": 45635, "اذ": 45636, "Mania": 45637, "ĠíĻľëıĻ": 45638, "Ġë´IJìļĶ": 45639, "ĠJaz": 45640, "ç»ĵ": 45641, "ÑĸлÑĮки": 45642, "rishna": 45643, "Ġêµ°": 45644, "Ġtamanho": 45645, "Ġappliance": 45646, "ĠResistance": 45647, "ĠLOOK": 45648, "ĠHyp": 45649, "ĠHeil": 45650, "Fire": 45651, "uju": 45652, "Ġheals": 45653, "Ġmalt": 45654, "ĠVERY": 45655, "ĠÑħоÑĩеÑĪÑĮ": 45656, "Ġlinger": 45657, "ĠNarr": 45658, "ĠRegular": 45659, "ĠLoop": 45660, "ĠLeno": 45661, "Ġsortie": 45662, "ĠServe": 45663, "ĠìĿµ": 45664, "ĠLuego": 45665, "ittä": 45666, "Ġundes": 45667, "è³½": 45668, "å¦Ĥæŀľä½ł": 45669, "Ġslippers": 45670, "Ġonda": 45671, "ĠÄIJây": 45672, "Ġtaped": 45673, "Ġtraverse": 45674, "Ġrelativity": 45675, "ĠYoshi": 45676, "cjon": 45677, "ilated": 45678, "actively": 45679, "ĠСов": 45680, "æĪijè§īå¾Ĺ": 45681, "ĠPOL": 45682, "ÐłÐĺ": 45683, "inflamm": 45684, "cheerful": 45685, "Ġ×ŀ×IJ×": 45686, "Ġ>>[": 45687, "minster": 45688, "Ġвли": 45689, "Ġidentifier": 45690, "ĠLambda": 45691, "Ġtros": 45692, "Ġflawless": 45693, "Ġdetrimental": 45694, "Ġbunları": 45695, "War": 45696, "Ġregião": 45697, "羣çļĦæĺ¯": 45698, "ĠBike": 45699, "cessors": 45700, "Ġcùng": 45701, "ĠRN": 45702, "Ġê½ĥ": 45703, "Ġküçük": 45704, "ĠBeginning": 45705, "íĺ¸ë": 45706, "Ġgewe": 45707, "Ġdenote": 45708, "ĠAlberto": 45709, "Ġprobiot": 45710, "Ġode": 45711, "Ġmolar": 45712, "Ġbursting": 45713, "assumed": 45714, "Ġfootprints": 45715, "veda": 45716, "Ġsteroids": 45717, "Ġflaming": 45718, "ĠEller": 45719, "Ġerkennen": 45720, "ätzen": 45721, "Ġlifecycle": 45722, "ĠDOU": 45723, "ĠKarena": 45724, "ĠGuerra": 45725, "è¿ĺæĺ¯": 45726, "Ġsinister": 45727, "Ġpodéis": 45728, "Ġparab": 45729, "Ġoko": 45730, "Ġmatéri": 45731, "Ġcaric": 45732, "sonaro": 45733, "Ġpraticamente": 45734, "ÑĥÑģа": 45735, "Ġcomunque": 45736, "Ġvigilant": 45737, "Ġregimes": 45738, "ĠShooting": 45739, "Ġraids": 45740, "ĠNora": 45741, "ĠWieder": 45742, "mens": 45743, "ĠÑģод": 45744, "Ġê²½ìļ°ìĹIJëĬĶ": 45745, "ĠвÑħод": 45746, "Ġautobi": 45747, "ĠSchn": 45748, "ĠRobbie": 45749, "ĠFitness": 45750, "ĠконÑĦ": 45751, "Ġpenguin": 45752, "моÑĤÑĢÑı": 45753, "Ġминим": 45754, "plays": 45755, "Ġdelegates": 45756, "Mer": 45757, "Ġsistem": 45758, "ĠMichaels": 45759, "male": 45760, "اع": 45761, "Ġcách": 45762, "ĠHä": 45763, "Ġ×Ļ×ķ×ĵ×¢": 45764, "Ġsuperpower": 45765, "Ġstron": 45766, "Ġrover": 45767, "Ġdépend": 45768, "éϳ": 45769, "Ġretiring": 45770, "Ġvampires": 45771, "Ġmerde": 45772, "ĠChanging": 45773, "Ġtame": 45774, "Ġspokesperson": 45775, "Ġcay": 45776, "Ġflirting": 45777, "ĠGrö": 45778, "Ġwär": 45779, "Ġwyb": 45780, "Ġcoeur": 45781, "ạnh": 45782, "ĠìĻĢìĦľ": 45783, "Ġconnais": 45784, "ĠHundreds": 45785, "ĠBea": 45786, "ĠαÏĢ": 45787, "pruch": 45788, "Ġsociedade": 45789, "ĠWhilst": 45790, "ĠKait": 45791, "espace": 45792, "Ġchia": 45793, "ĠErm": 45794, "Ġë°Ķê¿": 45795, "Ġfences": 45796, "ĠMortal": 45797, "ê²ģ": 45798, "ĠгÑĢаÑĦ": 45799, "ĠHomeland": 45800, "ĠJUN": 45801, "isst": 45802, "Ġparlar": 45803, "Ġsporty": 45804, "éo": 45805, "Ġdeepen": 45806, "ĠBehavior": 45807, "éĢı": 45808, "åĵĪåĵĪåĵĪ": 45809, "Ġerrand": 45810, "Ġrotary": 45811, "ĠWellington": 45812, "Wind": 45813, "Ġmesela": 45814, "ảng": 45815, "iende": 45816, "Ġexcell": 45817, "ĠGenius": 45818, "ĠEduardo": 45819, "æľī人": 45820, "ĠÅŁunu": 45821, "Ġİstanbul": 45822, "Ġproduto": 45823, "Ġãħİãħİ": 45824, "OFF": 45825, "Ġwollt": 45826, "çĪĨ": 45827, "Ġëī´ìĬ¤": 45828, "Ġlass": 45829, "Ġhertz": 45830, "Ġaromatic": 45831, "Ġзвон": 45832, "Ġautoc": 45833, "ĠLust": 45834, "Ġ112": 45835, "ĠÎĹ": 45836, "Ġreviewers": 45837, "Ġreceptive": 45838, "å°įäºĨ": 45839, "ând": 45840, "oglo": 45841, "ĠìķĦëĭĻ": 45842, "Ġngo": 45843, "ÑĸÑĤи": 45844, "Ã¥t": 45845, "cono": 45846, "Ġtekrar": 45847, "Ġì£¼ê³ł": 45848, "ĠgelmiÅŁ": 45849, "Ġbedtime": 45850, "ĠArgh": 45851, "ADA": 45852, "ĠгоÑĢода": 45853, "ĠÄĩ": 45854, "Ġalliances": 45855, "giggling": 45856, "Ġyerde": 45857, "Ġspies": 45858, "Ġgutes": 45859, "çi": 45860, "Ġalltid": 45861, "ĠLah": 45862, "ŀIJë": 45863, "ĠdokÅĤad": 45864, "ÙĪÙĬ": 45865, "Ġtoxicity": 45866, "Ġcancellation": 45867, "Ġ1958": 45868, "dro": 45869, "ĠìŀijìĿĢ": 45870, "ĠMotorola": 45871, "Ġmultin": 45872, "Ġenthusiasts": 45873, "ĠMighty": 45874, "ĠCoconut": 45875, ":ãĢĮ": 45876, "ĠPictures": 45877, "Ġsangre": 45878, "Ġblinking": 45879, "olesome": 45880, "ĠìĬ¤íĥĢìĿ¼": 45881, "FP": 45882, "Ġbooming": 45883, "ĠдеÑģÑıÑĤ": 45884, "Ġratchet": 45885, "Ġtimelines": 45886, "leness": 45887, "Ġcages": 45888, "ĠGoodnight": 45889, "ometimes": 45890, "Ġcunning": 45891, "ĠRisk": 45892, "uled": 45893, "dade": 45894, "Ġprata": 45895, "ĠgustarÃŃa": 45896, "amus": 45897, "ĠJinping": 45898, "Ġestrut": 45899, "Ġdescobrir": 45900, "ĠMÄģ": 45901, "ĠAllan": 45902, "ĠåĪĨ": 45903, "Ġ׾ק": 45904, "Ġpreserv": 45905, "ĠStrawberry": 45906, "Äı": 45907, "Lu": 45908, "Ġkro": 45909, "ĠReports": 45910, "ìħĶìķ¼": 45911, "Ġvalt": 45912, "Ġpouvait": 45913, "Ġappar": 45914, "ĠBone": 45915, "Ġpreferably": 45916, "ĠRepública": 45917, "å°±åΰ": 45918, "Ġherzlich": 45919, "Ġchimney": 45920, "Ġçev": 45921, "Ġvisas": 45922, "Ġverr": 45923, "Ġcultivation": 45924, "ĠArmenia": 45925, "ĠвдÑĢÑĥг": 45926, "Ġcockro": 45927, "retched": 45928, "artz": 45929, "ĠлÑİдÑıм": 45930, "ĠpolÃŃticas": 45931, "ĠPanz": 45932, "ĠAKA": 45933, "ĠëĪĮ룬": 45934, "Ġerro": 45935, "Ġcamper": 45936, "Ġ102": 45937, "स": 45938, "done": 45939, "Ġhoard": 45940, "ĠÐŁÐ¾ÑĤом": 45941, "jeong": 45942, "Ġdesta": 45943, "pak": 45944, "Ġinim": 45945, "Ġgrowers": 45946, "ĠMessage": 45947, "Ġelector": 45948, "engage": 45949, "ĠForbes": 45950, "ĠCincinnati": 45951, "Ġdifférence": 45952, "df": 45953, "Ġspar": 45954, "Ġawaits": 45955, "ĠUSSR": 45956, "ĠRising": 45957, "ĠHoÅŁ": 45958, "Ġfooting": 45959, "Ġcondiciones": 45960, "ÑĤоÑĢов": 45961, "Ġclinician": 45962, "ĠDiskuss": 45963, "å£ĵ": 45964, "ר×Ĵ": 45965, "×¥": 45966, "iteit": 45967, "gren": 45968, "Ġcharisma": 45969, "Ġleuke": 45970, "Ġirritating": 45971, "Ġcirca": 45972, "ĠRhodes": 45973, "Ġpior": 45974, "Ġhandicap": 45975, "royable": 45976, "Ġvull": 45977, "OG": 45978, "ĠinÃŃcio": 45979, "ieri": 45980, "Ġsplashing": 45981, "Ġdemise": 45982, "Ġassistir": 45983, "ÑĩÑĤо": 45984, "Ġcovert": 45985, "ĠGud": 45986, "à¸ī": 45987, "klär": 45988, "ĠìŀIJ꾸": 45989, "Ġverändert": 45990, "ĠREM": 45991, "ĠConven": 45992, "atge": 45993, "Ġpierwsze": 45994, "Ġclergy": 45995, "lington": 45996, "liv": 45997, "VPN": 45998, "ĠÑģожал": 45999, "ĠHate": 46000, "ãģ¨ãģĵãĤį": 46001, "ÏĨο": 46002, "ĠRespons": 46003, "озд": 46004, "Ġetmek": 46005, "Ġchemin": 46006, "ÙħØ©": 46007, "Ġê°Ģ족": 46008, "Tre": 46009, "Ġumas": 46010, "ĠBurton": 46011, "Ġpatriarch": 46012, "ĠSmithsonian": 46013, "¥ĺ": 46014, "Moon": 46015, "Air": 46016, "Ġmedios": 46017, "Ġeraser": 46018, "Ġwollten": 46019, "Ġpareil": 46020, "ĠBillie": 46021, "æĬ½": 46022, "еÑĢÑĤв": 46023, "Ġparlament": 46024, "Ġagony": 46025, "ĠQUE": 46026, "sequently": 46027, "Another": 46028, "ĠWhew": 46029, "ĠAnnual": 46030, "Ġseben": 46031, "ìĥģìĿĦ": 46032, "values": 46033, "ŀľë§Į": 46034, "Ġsinon": 46035, "ereal": 46036, "ĠEnlight": 46037, "ĠChemistry": 46038, "ĠCatalunya": 46039, "Ġdoctr": 46040, "anton": 46041, "Ġstuk": 46042, "ĠPlate": 46043, "ĠKardashian": 46044, "Ġfilos": 46045, "ĠWet": 46046, "ĠпопÑĭÑĤ": 46047, "Ġunknowns": 46048, "ĠSchon": 46049, "ĠBaldwin": 46050, "Ġtelescopes": 46051, "ĠGucci": 46052, "oxide": 46053, "ĠConservative": 46054, "ìĦ±ìĿĦ": 46055, "Ġhinaus": 46056, "Power": 46057, "Ġê±´ê°ķ": 46058, "Ġprevail": 46059, "orman": 46060, "machine": 46061, "Ġ1946": 46062, "Ġunbel": 46063, "Ġschaut": 46064, "Ġpiel": 46065, "eenth": 46066, "Ġobjectively": 46067, "Ġchakra": 46068, "audio": 46069, "Ġchicos": 46070, "ĠVault": 46071, "å°Ī": 46072, "Ġmedicinal": 46073, "ĠTail": 46074, "While": 46075, "Ġasphalt": 46076, "Ġfroze": 46077, "ĠEK": 46078, "unching": 46079, "nosis": 46080, "2015": 46081, "ĠGri": 46082, "Ġoddly": 46083, "ĠMär": 46084, "ĠAeg": 46085, "colo": 46086, "Par": 46087, "Ġëĵ¤ìĸ´ë": 46088, "Ġvinden": 46089, "ĠOVER": 46090, "Ġiced": 46091, "Ġscorp": 46092, "Ġhac": 46093, "qualified": 46094, "ĠÑĥвидеÑĤÑĮ": 46095, "ermo": 46096, "HEN": 46097, "Ġsoi": 46098, "Ġmultiples": 46099, "Ġlayouts": 46100, "Ġblindness": 46101, "ĠBowser": 46102, "ĠподÑĤ": 46103, "ĠÃİ": 46104, "ventional": 46105, "Ġmata": 46106, "madı": 46107, "Ġgeez": 46108, "Ġcadence": 46109, "Ġważne": 46110, "ĠChristie": 46111, "venge": 46112, "Call": 46113, "Ġturnaround": 46114, "Ġblob": 46115, "ĠЯк": 46116, "ĠVoiceover": 46117, "Ġperil": 46118, "ĠJaime": 46119, "ĠHOY": 46120, "lane": 46121, "Ġsebel": 46122, "ĠDuo": 46123, "ĠHistorical": 46124, "Ġdni": 46125, "Ġgema": 46126, "yk": 46127, "Ġsabem": 46128, "ắng": 46129, "Ġvars": 46130, "ĠRonnie": 46131, "ĠRonaldo": 46132, "ĠPerquè": 46133, "nsinn": 46134, "hair": 46135, "Ġrelentless": 46136, "Ġlyn": 46137, "Ġtraveler": 46138, "æĢİ麼äºĨ": 46139, "nine": 46140, "Ġantim": 46141, "Ġì¼Ģ": 46142, "Ġsnowball": 46143, "ĠÑħаÑĢакÑĤеÑĢ": 46144, "Ġinterns": 46145, "Ġconstituency": 46146, "ĠÐĿам": 46147, "׾׾": 46148, "VEL": 46149, "Ġviktigt": 46150, "Ġapoyo": 46151, "ÙĦب": 46152, "Ġjard": 46153, "Ġheightened": 46154, "ÑĢоÑģÑĤ": 46155, "ĠSMITH": 46156, "Ġдела": 46157, "Ġrepairing": 46158, "Ġrigt": 46159, "ĠSheikh": 46160, "ĠBritney": 46161, "Ġeverytime": 46162, "Ġadventurous": 46163, "ockey": 46164, "ernt": 46165, "Ġataque": 46166, "ĠAlternatively": 46167, "effect": 46168, "Ġpalavras": 46169, "ĠElliott": 46170, "Ġréussi": 46171, "Ġhypertension": 46172, "ĠManual": 46173, "Ġprophetic": 46174, "Ġhandc": 46175, "ÑĮе": 46176, "Ġrefrain": 46177, "ĠSquid": 46178, "ìŀ¡": 46179, "Ġкоман": 46180, "ällen": 46181, "Ġllegó": 46182, "Ġbash": 46183, "iony": 46184, "ĠÑģклад": 46185, "Ġкаб": 46186, "Ġcareless": 46187, "ĠPool": 46188, "Ġtrás": 46189, "Ġfils": 46190, "ĠSchr": 46191, "Ġsprawd": 46192, "ĠMonaten": 46193, "Ġunforgettable": 46194, "ĠCotton": 46195, "Ġinconvenient": 46196, "ĠRX": 46197, "oris": 46198, "Ġhumbled": 46199, "ת×Ĺ": 46200, "Ġآپ": 46201, "ĠincreÃŃ": 46202, "ĠKommentare": 46203, "èĪĴ": 46204, "ración": 46205, "Ġvantage": 46206, "ĠSeal": 46207, "ĠìĿ´ê±°ë¥¼": 46208, "Ġjoue": 46209, "ãģĿãģĨãģ§ãģĻãģŃ": 46210, "Ġìĺ¤ëŀĺ": 46211, "ĠиÑģпÑĭÑĤ": 46212, "oben": 46213, "Ġgrate": 46214, "Ġcontrole": 46215, "ĠPercy": 46216, "ÅĤada": 46217, "Ġsimultaneous": 46218, "Ġprototy": 46219, "ĠgroÃŁer": 46220, "Ġbewusst": 46221, "inizi": 46222, "Ġpassieren": 46223, "ĠHappiness": 46224, "åīĩ": 46225, "shi": 46226, "geht": 46227, "Ġstationed": 46228, "ĠErgebnis": 46229, "Ġdirectamente": 46230, "Ġsurvives": 46231, "Ġpersones": 46232, "BERG": 46233, "Ġvomiting": 46234, "Ġconhecer": 46235, "Ġadjour": 46236, "ĠCivic": 46237, "pei": 46238, "burst": 46239, "Ġëĭ¤ëĭĪ": 46240, "éı": 46241, "Ġsled": 46242, "Ġplataforma": 46243, "ĠSect": 46244, "ĠDefin": 46245, "çĻ»éĮ²": 46246, "énom": 46247, "chnet": 46248, "Ġprofitability": 46249, "Ġerreicht": 46250, "á»ıi": 46251, "cation": 46252, "Ġì§Ģê¸": 46253, "Ġperdre": 46254, "Ġfelony": 46255, "Ġ1957": 46256, "æĪijå¾Ī": 46257, "Ġunsuccessful": 46258, "Ġnagyon": 46259, "Ġelasticity": 46260, "Ġfacade": 46261, "Ġearthly": 46262, "ĠамеÑĢикан": 46263, "Ġconn": 46264, "cla": 46265, "Du": 46266, "Ġpolitiques": 46267, "Ġhalo": 46268, "iantes": 46269, "Ġмоей": 46270, "ãĥ³ãĥī": 46271, "tones": 46272, "elier": 46273, "è®ļ": 46274, "htaking": 46275, "Ġwichtige": 46276, "Ġanno": 46277, "ĠLok": 46278, "illions": 46279, "Ġviver": 46280, "Ġsolchen": 46281, "Ġsuf": 46282, "ĠSalz": 46283, "ĠNvidia": 46284, "zuge": 46285, "ĠSpike": 46286, "Video": 46287, "Ġtwor": 46288, "ĠAla": 46289, "èijī": 46290, "Ġhanya": 46291, "ĠAdm": 46292, "ìĿµ": 46293, "ĠPatienten": 46294, "ĠOnion": 46295, "ĠKobe": 46296, "ĠScene": 46297, "ĠRash": 46298, "æ¨Ļ": 46299, "ÑĢаÑģÑĤ": 46300, "istani": 46301, "General": 46302, "leye": 46303, "imbap": 46304, "Ġconcealed": 46305, "ĠFridays": 46306, "ĠWool": 46307, "ĠновÑĭÑħ": 46308, "شر": 46309, "Ġê²°ê³¼": 46310, "Ġjedoch": 46311, "´ìĭľ": 46312, "ĵ¤ëıĦ": 46313, "Ġìŀ¥ëĤľ": 46314, "ukt": 46315, "Lou": 46316, "Ġ먹ìĸ´": 46317, "ĠExpect": 46318, "Ġдомой": 46319, "Ġirresponsible": 46320, "Ġacerca": 46321, "ĠZust": 46322, "ר×ĺ": 46323, "UI": 46324, "Ġyoutubers": 46325, "ĠPositive": 46326, "Ġsocioe": 46327, "Ġsnatch": 46328, "èĥĮ": 46329, "Ġrefreshed": 46330, "Ġnominations": 46331, "ĠPatt": 46332, "Ġobsolete": 46333, "ĠdemiÅŁ": 46334, "åı¤": 46335, "ormuÅŁ": 46336, "ĠìĨĶì§ģíŀĪ": 46337, "Ġfla": 46338, "Ġcraziest": 46339, "ĠZie": 46340, "ĠTú": 46341, "zep": 46342, "icem": 46343, "Ġë©ĭìŀĪ": 46344, "Ġcynical": 46345, "ãģĿãĤĵãģª": 46346, "Ġtresp": 46347, "Ġcraz": 46348, "Õ¥Õ": 46349, "Ġnelle": 46350, "Ġmph": 46351, "ĠNered": 46352, "ĠKob": 46353, "ĠEck": 46354, "¨¸ëĭĪ": 46355, "Jan": 46356, "ĠТогда": 46357, "Ġdeci": 46358, "ĠVog": 46359, "Ġbubbling": 46360, "éĢĢ": 46361, "úa": 46362, "Ġproductos": 46363, "iberal": 46364, "Ġreplicated": 46365, "ĠImprove": 46366, "illary": 46367, "Cha": 46368, "Ġrédu": 46369, "ĥIJíķĺë©´": 46370, "Ġconnot": 46371, "ĠKrit": 46372, "ĠдÑĥÑħов": 46373, "Ġtreadmill": 46374, "ĠPW": 46375, "ĠзовÑĥÑĤ": 46376, "Ġclams": 46377, "Ġdrafting": 46378, "Ġ1956": 46379, "unta": 46380, "Ġexpenditures": 46381, "ĠHoover": 46382, "WOO": 46383, "ÑĪее": 46384, "Ġdeduction": 46385, "monary": 46386, "Ġrecib": 46387, "Ġpovo": 46388, "ĠëįĶë": 46389, "ĠPAL": 46390, "ĠBlow": 46391, "Ġwyp": 46392, "Ġdestac": 46393, "deal": 46394, "Graeme": 46395, "Ġnécessaire": 46396, "Ġdamned": 46397, "Ġ1938": 46398, "Ġìĭ¤ìłľë¡ľ": 46399, "Ġtroop": 46400, "Ġinsightful": 46401, "ĠTJ": 46402, "ĠоÑģв": 46403, "Ġfidelity": 46404, "ĠSkip": 46405, "ĠMayo": 46406, "ë§Ŀ": 46407, "appe": 46408, "Ġblas": 46409, "ĠWY": 46410, "ĠGN": 46411, "ctar": 46412, "Su": 46413, "Ġcuent": 46414, "hews": 46415, "Ġcorpses": 46416, "Abs": 46417, "Ġwastewater": 46418, "Ġciek": 46419, "ĠOnu": 46420, "Ġexplosives": 46421, "Ġarma": 46422, "ĠSTEPHAN": 46423, "politik": 46424, "ĠOsaka": 46425, "taÅĤ": 46426, "Ġyapıyor": 46427, "Ġizquier": 46428, "Ġbeleza": 46429, "ĠWyatt": 46430, "åIJ¸": 46431, "Ġsuk": 46432, "Ġspecjal": 46433, "Ġdanke": 46434, "whistle": 46435, "ĠfÃŃsica": 46436, "ĠHarriet": 46437, "ĠìķĦíĮĮ": 46438, "Ġwillkommen": 46439, "iping": 46440, "ĠÑģмоÑĤÑĢиÑĤе": 46441, "ĠможеÑĪÑĮ": 46442, "Ġinaccurate": 46443, "Ġarrogance": 46444, "ĠRemo": 46445, "γά": 46446, "assed": 46447, "Ġdeliveries": 46448, "Ġstinky": 46449, "ĠпеÑĢеж": 46450, "jay": 46451, "Ġtransitional": 46452, "Ġrere": 46453, "ĠNGOs": 46454, "ĠATM": 46455, "خت": 46456, "iology": 46457, "Ġвлад": 46458, "Ġschme": 46459, "ĠShine": 46460, "ìķ¡": 46461, "pants": 46462, "Ġserge": 46463, "Ġsenhor": 46464, "Ġabduct": 46465, "ĠBryant": 46466, "VES": 46467, "Ġawakened": 46468, "ĠLaz": 46469, "ropolis": 46470, "ĠLao": 46471, "è¾Ľèĭ¦": 46472, "Ġvilla": 46473, "Ġsummers": 46474, "Ġenthal": 46475, "Ġ1949": 46476, "Via": 46477, "Ġìĸ´ì¨": 46478, "Ġtendon": 46479, "Ġviolet": 46480, "Ġintellectually": 46481, "Ġbounced": 46482, "araus": 46483, "Ġ1919": 46484, "Ġvraag": 46485, "Ġspel": 46486, "ĠSchwar": 46487, "Scott": 46488, "ĠIndo": 46489, "Ġë§Ŀ": 46490, "Ġcanonical": 46491, "ĠIKE": 46492, "ĠthatÃŃs": 46493, "Ġmellan": 46494, "æ¯Ĵ": 46495, "igmat": 46496, "Could": 46497, "...?)": 46498, "Ġfoarte": 46499, "ĠKumar": 46500, "rendo": 46501, "Ġélé": 46502, "à´": 46503, "valuation": 46504, "cases": 46505, "Ġintuitively": 46506, "hong": 46507, "etted": 46508, "Ġsouven": 46509, "Ġmorb": 46510, "Ġcors": 46511, "ĠNV": 46512, "ĠHasan": 46513, "æĥħåĨµ": 46514, "ieved": 46515, "Ġì§Ģê¸ĪìĿĢ": 46516, "Ġdumpling": 46517, "Ġcontrôle": 46518, "Ġambiguity": 46519, "æ©Łæľĥ": 46520, "Ġcog": 46521, "ĠScriptures": 46522, "Ġcai": 46523, "Ġbever": 46524, "大家éĥ½": 46525, "Ġhuis": 46526, "Ġaime": 46527, "Ġerklären": 46528, "ĠLM": 46529, "ĠFey": 46530, "éļ¾": 46531, "றத": 46532, "Ġsupervised": 46533, "Ġjewe": 46534, "spl": 46535, "ĠÑĨенÑĤÑĢ": 46536, "Ġcollisions": 46537, "ÙĦÙģ": 46538, "ĠHogwarts": 46539, "ĠDurham": 46540, "×ķ×£": 46541, "Ġphosphate": 46542, "Ġoversee": 46543, "Ġinspections": 46544, "Ġbrinc": 46545, "ĠZak": 46546, "Ġpayoff": 46547, "Ġchaud": 46548, "ĠHunger": 46549, "ãos": 46550, "vir": 46551, "Ġfiance": 46552, "Ġboug": 46553, "lived": 46554, "cry": 46555, "åĽŀä¾Ĩ": 46556, "Ġjointly": 46557, "Ġgirlfriends": 46558, "ĠNexus": 46559, "¦¬ê²łìĬµëĭĪëĭ¤": 46560, "ĠKwang": 46561, "åĵĪåĽī": 46562, "å§ij": 46563, "ÅĤÄĻ": 46564, "ĠNeden": 46565, "iece": 46566, "Ġinserting": 46567, "æŁĵ": 46568, "ĠMummy": 46569, "ĠGlobe": 46570, "Ġlee": 46571, "Ġgerman": 46572, "Ġcreams": 46573, "acho": 46574, "Ġchưa": 46575, "ĠGalile": 46576, "Ġfürs": 46577, "Ġestiver": 46578, "cidos": 46579, "Christian": 46580, "Ġlorsqu": 46581, "Ġcutest": 46582, "vale": 46583, "ĠкÑĢеп": 46584, "Ġwary": 46585, "Ġslicing": 46586, "Ġesperando": 46587, "ĠVander": 46588, "ĠDeixa": 46589, "Ġ1954": 46590, "ĠmówiÄħ": 46591, "ÑĸÑĶ": 46592, "Ġtooling": 46593, "Ġrestor": 46594, "Ġposición": 46595, "Ġintentar": 46596, "ĠApache": 46597, "OUL": 46598, "ĠÙĪØ¨": 46599, "Ġmatière": 46600, "ãĥ¼ãĤĵ": 46601, "Ġlinen": 46602, "Ġestratég": 46603, "ĠMutta": 46604, "顯": 46605, "è¡ĮäºĨ": 46606, "Ġparting": 46607, "Ġminimizing": 46608, "Ġapprendre": 46609, "æľĿ": 46610, "Ġанглий": 46611, "ĠDoo": 46612, "ĠFirefox": 46613, "cómo": 46614, "Ġgeopolit": 46615, "Ġmakan": 46616, "Ġmogelijk": 46617, "ĠÏĢεÏģι": 46618, "Ġcứ": 46619, "Ġinstaller": 46620, "Ġdibuj": 46621, "ĠHeath": 46622, "loop": 46623, "ĠBroken": 46624, "HYUN": 46625, "shelf": 46626, "Ġfizer": 46627, "Ġenhances": 46628, "ä¾ĭãģĪãģ°": 46629, "ĠдоÑģÑĤи": 46630, "ĠPUB": 46631, "ĠKollegin": 46632, "Ġattained": 46633, "ľ": 46634, "Ġmistress": 46635, "ĠOftentimes": 46636, "×ŀ×Ļ×Ŀ": 46637, "Ġbewe": 46638, "ĠSora": 46639, "rauen": 46640, "baum": 46641, "Ġrollers": 46642, "Ġmering": 46643, "ĠPAC": 46644, "ĠнÑĸ": 46645, "ĠRépublique": 46646, "ĠÑĤÑĢав": 46647, "ĠVanguard": 46648, "uciones": 46649, "Ġ무ëĮĢ": 46650, "Ġgour": 46651, "¯¤": 46652, "ĠÏī": 46653, "Ġsauna": 46654, "Ġpeine": 46655, "ĠValerie": 46656, "ĠSikh": 46657, "fendimiz": 46658, "bero": 46659, "ĠÑĩи": 46660, "ĠdoÅĽwiad": 46661, "ĠEuros": 46662, "Ġcommentaires": 46663, "Ġtweaks": 46664, "ĠFaster": 46665, "ĠÑĢаÑģк": 46666, "Ġprogressively": 46667, "ĠEuch": 46668, "boro": 46669, "ĠIngred": 46670, "Cap": 46671, "Ġuncheck": 46672, "Ġìĺ¤ë¥¸": 46673, "Ġwre": 46674, "ĠFT": 46675, "örung": 46676, "Ġmemorized": 46677, "ĠDinner": 46678, "ĠPhew": 46679, "oubl": 46680, "Ġputa": 46681, "Ġadmits": 46682, "езде": 46683, "opod": 46684, "Ġpanda": 46685, "Ġhinges": 46686, "cipe": 46687, "Ġtransact": 46688, "Ġpodia": 46689, "Ġpics": 46690, "Ġcriterion": 46691, "ĠOrchestra": 46692, "ĠBlog": 46693, "Ġsolemn": 46694, "ĠPixar": 46695, "Three": 46696, "Ġвниз": 46697, "ĠVolunte": 46698, "ĠSavage": 46699, "ĠPVC": 46700, "ĠCaf": 46701, "Ġwykon": 46702, "Ġgraders": 46703, "Ġcrouch": 46704, "Ġcliche": 46705, "Ġsoybeans": 46706, "ĠMUR": 46707, "ĠGonzalez": 46708, "ĠMimi": 46709, "ĠBolsonaro": 46710, "Ġdiaphrag": 46711, "Ġbilang": 46712, "ëIJĺëĬĶ": 46713, "éĤ£æĪijåĢij": 46714, "Ġregulating": 46715, "Mc": 46716, "Judge": 46717, "Ġнож": 46718, "ĠjakÄħ": 46719, "itesse": 46720, "ĠWij": 46721, "Ġlata": 46722, "groaning": 46723, "POSING": 46724, "Ġ×IJ×ķת×ķ": 46725, "Ġhaga": 46726, "Ġgrounding": 46727, "Ġviolently": 46728, "Ġtills": 46729, "Ġengag": 46730, "ĠHollow": 46731, "ĠпопÑĥлÑıÑĢ": 46732, "Ġwprowad": 46733, "Ġreplaces": 46734, "Ġfluorescent": 46735, "urgical": 46736, "iggly": 46737, "ĠTraditional": 46738, "tte": 46739, "ĠÙĦÙĩ": 46740, "Ġphosphorus": 46741, "Ġapron": 46742, "ĠWaters": 46743, "ĠKultur": 46744, "авай": 46745, "Ġolives": 46746, "Ġ×Ķ×IJ׾": 46747, "Ġteilweise": 46748, "Ġsencill": 46749, "Ġprends": 46750, "Ġnarrower": 46751, "Ġjätte": 46752, "ĠInformationen": 46753, "ìĥģìĿ´": 46754, "Ġstarve": 46755, "Ġfrick": 46756, "ĠBeweg": 46757, "ल": 46758, "Ġdolphin": 46759, "ĠLAUGHTER": 46760, "ĠINTERVIE": 46761, "åĶī": 46762, "ĠyanlÄ±ÅŁ": 46763, "Ġtorpedo": 46764, "Ġshortages": 46765, "ìĿ´ëĵľ": 46766, "ıldı": 46767, "Ġpaws": 46768, "Ġozone": 46769, "Ġcultivated": 46770, "ĠFot": 46771, "Ġnotor": 46772, "ноз": 46773, "ĠкоÑĪ": 46774, "Ġtouchscreen": 46775, "ĠAlly": 46776, "æľĢè¿ij": 46777, "Ġë§ĽìŀĪìĸ´ìļĶ": 46778, "ĠСеÑĢ": 46779, "Ġвполне": 46780, "Ġpaprika": 46781, "ĠDustin": 46782, "Ġefecto": 46783, "Ġopini": 46784, "Ġmuut": 46785, "Ġhá»įc": 46786, "Ġinterject": 46787, "ÄĻt": 46788, "Ġbutts": 46789, "urez": 46790, "ĠPike": 46791, "ĠHok": 46792, "ĠGuinea": 46793, "ĠCathedral": 46794, "Ġ1400": 46795, "Cra": 46796, "+,": 46797, "ë§Ľ": 46798, "³´ëıĦë¡Ŀ": 46799, "abyrin": 46800, "Ġvideog": 46801, "ĠоÑĢÑĥж": 46802, "Ġuž": 46803, "Ġbuscando": 46804, "ĠAssistance": 46805, "éϽ": 46806, "Ġmelhores": 46807, "ì¡´": 46808, "Ġëģ¼": 46809, "ĠRJ": 46810, "ĠتÙħ": 46811, "Ġomin": 46812, "Ġmotorcycles": 46813, "ĠSapp": 46814, "Ġsupplying": 46815, "ĠAlgun": 46816, "Ġaerospace": 46817, "×¢×ľ": 46818, "occup": 46819, "leist": 46820, "Ġê±°ëĬĶ": 46821, "Ġcompleta": 46822, "bres": 46823, "!(": 46824, "ĠÐŁÑĢед": 46825, "Ġdisadvantaged": 46826, "ĠAttend": 46827, "ĠJudah": 46828, "á»ĭch": 46829, "ylene": 46830, "actly": 46831, "Ġsetups": 46832, "Ġammonia": 46833, "ĠSchweiz": 46834, "ĠShame": 46835, "Ġbande": 46836, "ĠFuel": 46837, "Ġtroublesome": 46838, "Ġnumero": 46839, "ĠMOM": 46840, "ĠпÑĢедлаг": 46841, "mentioned": 46842, "ĠболÑĮÑĪое": 46843, "ĠViktor": 46844, "ĠStyles": 46845, "Ġcrucified": 46846, "ructured": 46847, "environ": 46848, "Ġmorals": 46849, "Ġmeditating": 46850, "Ġaxial": 46851, "isance": 46852, "ĠAbst": 46853, "Green": 46854, "Ġê±´ì": 46855, "Ġquadrant": 46856, "Ġpergi": 46857, "Ġcameraman": 46858, "ĠSequ": 46859, "Ġpaused": 46860, "ĠLaughing": 46861, "ê·Ģ": 46862, "?..": 46863, "ĠÅ»e": 46864, "Ġpermitir": 46865, "Ġdetectors": 46866, "ĠHUD": 46867, "aval": 46868, "ĠìĹ¬ê¸°ê¹Įì§Ģ": 46869, "Ġhubs": 46870, "Ġbestimmt": 46871, "ĠбÑĥдеÑĤе": 46872, "INTERPOSING": 46873, "Ġtengan": 46874, "Ġcrave": 46875, "ĠBundesregierung": 46876, "ĠBloody": 46877, "Ġusability": 46878, "ĠEas": 46879, "ĠÄijá»Ļng": 46880, "Ġ1955": 46881, "Ġkriegen": 46882, "Ġhabitual": 46883, "Ġessentials": 46884, "riminal": 46885, "Ġroommates": 46886, "éĤ£å°±": 46887, "ĠпеÑĢеÑħод": 46888, "Ġnghi": 46889, "Ġmening": 46890, "ĠSymphony": 46891, "ĠHug": 46892, "aggi": 46893, "Ġwied": 46894, "Ġmitad": 46895, "ãģ£ãģ¦ãģĦãģĨ": 46896, "teenth": 46897, "idaÄĩ": 46898, "Save": 46899, "ĠrobiÄĩ": 46900, "Ġbounces": 46901, "°ĸìĹIJ": 46902, "stars": 46903, "Ġpragmatic": 46904, "Ġcognition": 46905, "Ġwrapper": 46906, "Ġwarten": 46907, "adh": 46908, "Ġpensa": 46909, "ĠHertz": 46910, "ĠnÄĽ": 46911, "ĠReid": 46912, "ĠPCs": 46913, "ĠMole": 46914, "Ġ.....": 46915, "Ġprecio": 46916, "ĠChampionships": 46917, "ê°ĢëĿ½": 46918, "Ġvér": 46919, "Ġcorridors": 46920, "ĠElectronic": 46921, "Sl": 46922, "Ġале": 46923, "Ġoverthrow": 46924, "Ġkabul": 46925, "ĠRES": 46926, "ĠCyberpunk": 46927, "огод": 46928, "ĠÐĿав": 46929, "Ġwan": 46930, "Ġmanifestations": 46931, "Ġcuales": 46932, "ĠWise": 46933, "ĠLösung": 46934, "Ġexfol": 46935, "Ġearns": 46936, "ÑĥÑģÑĤиÑĤÑĮ": 46937, "Ġsapp": 46938, "ĠBraun": 46939, "ĠBRANDON": 46940, "ì¹Ļ": 46941, "Ġsano": 46942, "ĠFEL": 46943, "ÑĭвайÑĤеÑģÑĮ": 46944, "ождениÑı": 46945, "Ġsewn": 46946, "Fun": 46947, "Ġreciprocal": 46948, "Ġexpansive": 46949, "ĠTraffic": 46950, "Ġktórego": 46951, "ĠÙĪØ³": 46952, "æĺ¥": 46953, "Ġ빨": 46954, "prove": 46955, "igare": 46956, "Ġloh": 46957, "اض": 46958, "Hope": 46959, "Ġdevotees": 46960, "ĠGom": 46961, "Ġsteals": 46962, "ĠUms": 46963, "ĠTwice": 46964, "ãĤ²": 46965, "iyim": 46966, "Ġrhythmic": 46967, "ĠVorte": 46968, "Ġprefix": 46969, "omination": 46970, "Ġdato": 46971, "Ġcustard": 46972, "ĠVOICE": 46973, "å·ŀ": 46974, "Ġmeny": 46975, "istors": 46976, "Ġíĺij": 46977, "ĠìĤ´ìķĦ": 46978, "ĠíĥĦ": 46979, "Ġkort": 46980, "Ġaba": 46981, "ĠVera": 46982, "epy": 46983, "Ġì¹´ë©ĶëĿ¼": 46984, "Ġsubmerged": 46985, "ĠClock": 46986, "Ġthumbnails": 46987, "Ġboast": 46988, "ĠFare": 46989, "!!]": 46990, "ĠÅĽm": 46991, "Ġkaikki": 46992, "ĠTechnologies": 46993, "ìϏ": 46994, "ãĥĴ": 46995, "иÑĤай": 46996, "å°ıæĻĤ": 46997, "ĠаÑĤ": 46998, "Ġknobs": 46999, "Ġreicht": 47000, "ượng": 47001, "glio": 47002, "Ġë§ĽìĿ´": 47003, "ê°IJìĿĦ": 47004, "Ġjotka": 47005, "ĠHandy": 47006, "ĠHaben": 47007, "nous": 47008, "Ġinland": 47009, "Ġamazon": 47010, "hooting": 47011, "SL": 47012, "Ġleisten": 47013, "~\"": 47014, "Ġprovoke": 47015, "ĠTwist": 47016, "Ġ×ij×Ĺ": 47017, "Ġdeparted": 47018, "ê°ľë¥¼": 47019, "Ġkonse": 47020, "ĠCarwyn": 47021, "íķĺìĭł": 47022, "idental": 47023, "ESCO": 47024, "Ġtteokbokki": 47025, "Ġdizendo": 47026, "ç·´": 47027, "ındaki": 47028, "imasu": 47029, "afar": 47030, "Ġlandfill": 47031, "Ġcorrecting": 47032, "Ġclears": 47033, "ĠNummer": 47034, "HAM": 47035, "Ġcartridges": 47036, "ĠDiesel": 47037, "paced": 47038, "Ġobliv": 47039, "Ġmoyens": 47040, "ĠSinne": 47041, "ĠPreis": 47042, "iliz": 47043, "ĠÑģмож": 47044, "Ġbroaden": 47045, "ä»ĸæĺ¯": 47046, "xes": 47047, "Ġcarbohydrate": 47048, "íĺ¹": 47049, "seok": 47050, "Ġechoes": 47051, "Ġcess": 47052, "ë°Ķ": 47053, "ĠбизнеÑģ": 47054, "Ġllamado": 47055, "Ġessent": 47056, "ĠìĿ¼ë°ĺ": 47057, "ĠAires": 47058, "phen": 47059, "Ġzebra": 47060, "Ġsymbolism": 47061, "Once": 47062, "Ġracks": 47063, "ĠKafka": 47064, "ĠÑģеÑĢÑĮез": 47065, "Ġsinn": 47066, "picious": 47067, "kaa": 47068, "Ġmotherfucker": 47069, "Ġapprenticeship": 47070, "Ġrpm": 47071, "Ġtaxation": 47072, "Ġfurry": 47073, "ĠSacred": 47074, "ĠÑĢазм": 47075, "pora": 47076, "enges": 47077, "ĠíĹĪë": 47078, "ĠÑģин": 47079, "Ġsanitizer": 47080, "Ġcringe": 47081, "ĠSca": 47082, "оÑĩно": 47083, "Ġofere": 47084, "Ġmelodies": 47085, "ĠVelvet": 47086, "ĠIhrer": 47087, "ĠHybrid": 47088, "ĠGiov": 47089, "Ġirgendwas": 47090, "Ġdepende": 47091, "ĠUsers": 47092, "Ġhump": 47093, "driving": 47094, "Ġsf": 47095, "Ġruthless": 47096, "à¹Ģà¸Ħ": 47097, "Ġlemons": 47098, "Ġföret": 47099, "ĠOj": 47100, "Ġмама": 47101, "Ġinterpersonal": 47102, "Ġgev": 47103, "Ġabnorm": 47104, "иÑģл": 47105, "Ġинд": 47106, "Ġkontroll": 47107, "Ġregres": 47108, "Ġledge": 47109, "Ġerzählt": 47110, "ĠTact": 47111, "Ġarrivé": 47112, "Ġsubstantive": 47113, "Ġspoonful": 47114, "zwischen": 47115, "ooooo": 47116, "Ġcontenido": 47117, "Ġbesl": 47118, "á»ĥm": 47119, "kten": 47120, "Jamie": 47121, "Ġsandy": 47122, "ä¸įåIJĮ": 47123, "âĭ": 47124, "Ġpase": 47125, "Ġdette": 47126, "ĠBelgian": 47127, "ê°ľë": 47128, "ulares": 47129, "rud": 47130, "igor": 47131, "ĠíĮ¬ë": 47132, "Ġremedies": 47133, "Ġblasting": 47134, "ĠSich": 47135, "Ġожид": 47136, "Ġmonstr": 47137, "Ġmanifold": 47138, "Ġglauben": 47139, "ĠEST": 47140, "Ġstreamline": 47141, "Ġlobbying": 47142, "ĠGothic": 47143, "toire": 47144, "..'": 47145, "Ġdémocr": 47146, "ĠнаблÑİд": 47147, "Ġwspól": 47148, "ĠczÄĻÅĽÄĩ": 47149, "ä¸ĭéĿ¢": 47150, "isés": 47151, "gangen": 47152, "Ġbezpie": 47153, "remlin": 47154, "ê°Ŀ": 47155, "Still": 47156, "Ġresides": 47157, "Ġgelecek": 47158, "Ġtéléphone": 47159, "Ġpewn": 47160, "Ġleopard": 47161, "Ġcomplimentary": 47162, "Ġcrib": 47163, "ĠAnimals": 47164, "Ġgeil": 47165, "essel": 47166, "Ġgarder": 47167, "Ġcatchy": 47168, "樹": 47169, "ĠEts": 47170, "ĠCommercial": 47171, "ĠDENNIS": 47172, "ĠCoordinator": 47173, "ĠAbigail": 47174, "ffffff": 47175, "ấp": 47176, "Ġpequeña": 47177, "Ġinjections": 47178, "cekt": 47179, "Ġphilanthropy": 47180, "Ġpuck": 47181, "Ġcelebrates": 47182, "ĠDunk": 47183, "ĠDlatego": 47184, "ãģ¾ãģł": 47185, "δή": 47186, "graduate": 47187, "ĠMobil": 47188, "till": 47189, "acam": 47190, "Ġyolks": 47191, "Ġtangled": 47192, "Ġmaniac": 47193, "Ġobliged": 47194, "ĠLaink": 47195, "Ġverder": 47196, "ĠDamon": 47197, "Ġmutant": 47198, "Ġhopping": 47199, "Ġreins": 47200, "Ġinverter": 47201, "Ġcontempt": 47202, "×ł×¡": 47203, "learning": 47204, "Miss": 47205, "ĠÐĵоÑģ": 47206, "ĠMeyer": 47207, "ê»ĺìĦľ": 47208, "é£İ": 47209, "×ķ׳×Ļ×Ŀ": 47210, "asking": 47211, "Ġtrimming": 47212, "Ġtreasury": 47213, "Ġsente": 47214, "Aust": 47215, "ĠUnterstützung": 47216, "ĠComedy": 47217, "ĠAnakin": 47218, "é¹": 47219, "ÑĢÑĥÑĤ": 47220, "ĠHari": 47221, "ographers": 47222, "Ġoatmeal": 47223, "ĠBots": 47224, "ä¸įäºĨ": 47225, "ĠпалÑĮ": 47226, "Ġacknowledgement": 47227, "xic": 47228, "Ġê´Ģìĭ¬": 47229, "gasping": 47230, "Ġãģķ": 47231, "Ġterrace": 47232, "Ġornaments": 47233, "ĠMER": 47234, "committee": 47235, "ĠìĹĨìĬµëĭĪëĭ¤": 47236, "Ġrij": 47237, "é³": 47238, "צ×Ŀ": 47239, "leme": 47240, "Ġliberties": 47241, "Ġfellas": 47242, "ĠCopper": 47243, "bench": 47244, "ĠIdea": 47245, "á»įn": 47246, "ÑĪа": 47247, "Ġversión": 47248, "ÏĦοÏį": 47249, "ĠÐľÐ¸": 47250, "ĠпÑĢилож": 47251, "Ġboxer": 47252, "ĠTanner": 47253, "ĠMoy": 47254, "ì¹ĺëĬĶ": 47255, "Thr": 47256, "Ġtinham": 47257, "Ġpolishing": 47258, "Ġconsequently": 47259, "Ġamenities": 47260, "ĠKI": 47261, "ĠGREEN": 47262, "ĠFrankie": 47263, "ниÑĤ": 47264, "ittel": 47265, "Ñģкое": 47266, "ursed": 47267, "Ġupbringing": 47268, "Ġthứ": 47269, "ĠìĭĿìľ¼ë¡ľ": 47270, "Ġwhim": 47271, "Ġchinese": 47272, "confidence": 47273, "ĠJeder": 47274, "ãģªãģ®ãģ§": 47275, "ajcie": 47276, "ĠTous": 47277, "ĠPowers": 47278, "ừa": 47279, "othermal": 47280, "ĠвÑĭÑĪе": 47281, "rale": 47282, "اخ": 47283, "Ġì§ĢìĽIJ": 47284, "Ġépisode": 47285, "Ġsulph": 47286, "Ġencara": 47287, "kraft": 47288, "aları": 47289, "ĠComes": 47290, "Ġdivul": 47291, "ĠRudolph": 47292, "ĠMuse": 47293, "Ġutens": 47294, "ĠìŀIJ주": 47295, "Ġpana": 47296, "ĠVegeta": 47297, "ĠPHP": 47298, "ĠNSA": 47299, "entin": 47300, "ĠCarnegie": 47301, "اÙĬ": 47302, "iÄĻcy": 47303, "Harry": 47304, "Ġfır": 47305, "Сп": 47306, "Ġgladly": 47307, "Ġaveraging": 47308, "íķĺê²łìĬµëĭĪëĭ¤": 47309, "лÑıÑİÑĤÑģÑı": 47310, "ĠÐľÐµÐ½Ñı": 47311, "Ġquotation": 47312, "rires": 47313, "itchens": 47314, "ayed": 47315, "Ġunatt": 47316, "ĠPerez": 47317, "ĠоÑĤмеÑĤ": 47318, "Ġtactile": 47319, "ĠEuh": 47320, "isini": 47321, "buh": 47322, "Ġhatır": 47323, "ĠìŀĪìľ¼": 47324, "Ġpolicymakers": 47325, "³´ìĦ¸ìļĶ": 47326, "acı": 47327, "Ġκι": 47328, "Ġregistering": 47329, "reto": 47330, "ĠSprinkle": 47331, "ĠGrammy": 47332, "axter": 47333, "Ġби": 47334, "Ġsitter": 47335, "Ġpredic": 47336, "Ġthinly": 47337, "Ġstrum": 47338, "Ġaggrav": 47339, "Ġaha": 47340, "رج": 47341, "mellow": 47342, "Ġconstante": 47343, "ĠLaut": 47344, "iston": 47345, "Ġtransitioned": 47346, "ĠCambodia": 47347, "ãģĦãģįãģ¾ãģĻ": 47348, "è·Łå¤§å®¶": 47349, "arted": 47350, "Ġmisf": 47351, "ĠPunkte": 47352, "Įëĵł": 47353, "Ġtrembling": 47354, "Ġgespannt": 47355, "ĠعÙĦÙĬÙĩ": 47356, "ĠникакиÑħ": 47357, "Ġë¶Ģëĵľë": 47358, "ĠÑĢазвиÑĤ": 47359, "Ġitchy": 47360, "Ġciento": 47361, "Ġplains": 47362, "Ġkittens": 47363, "Ġbacklog": 47364, "ĠPresiding": 47365, "pta": 47366, "Ġhavoc": 47367, "ĠDarrin": 47368, "ĠÐĽÑİб": 47369, "Ġsegregated": 47370, "Ġghetto": 47371, "Ġerlebt": 47372, "Ġdrugiej": 47373, "ĠSixt": 47374, "åıĥ": 47375, "ระ": 47376, "uencia": 47377, "Ġíķĺ기": 47378, "ĠëĨį": 47379, "Ġrobi": 47380, "Ġpioneers": 47381, "Ġmilliards": 47382, "ĠWitcher": 47383, "Ġ무ìĹĩ": 47384, "orro": 47385, "mass": 47386, "Ġdivergence": 47387, "ĠRivera": 47388, "ĠNoodles": 47389, "Ġendroit": 47390, "ĠKosten": 47391, "ĠдÑĢÑĥга": 47392, "ĠmÃŃnimo": 47393, "ĠKazakhstan": 47394, "تÙĩ": 47395, "ĠвоздÑĥ": 47396, "Ġgeschrieben": 47397, "ĠNil": 47398, "Ñģки": 47399, "ĠFrüh": 47400, "Ġbeverages": 47401, "æºIJ": 47402, "ĠGon": 47403, "æĺ¨": 47404, "Arin": 47405, "ĠIntro": 47406, "ocalyptic": 47407, "Ġexhaustion": 47408, "ĠStatus": 47409, "ĠBattery": 47410, "ész": 47411, "£¼ë": 47412, "airy": 47413, "Ġë³´ìŬëĵľë": 47414, "Ġdisparity": 47415, "ÙĮ": 47416, "ĠTucson": 47417, "Ġbrightly": 47418, "problem": 47419, "Ġbiomass": 47420, "éĻį": 47421, "§ī": 47422, "Ġhurdle": 47423, "Ġwavelengths": 47424, "Ġ<<": 47425, "Ġteamed": 47426, "FFFF": 47427, "ĠSlim": 47428, "omial": 47429, "Ġunveiled": 47430, "ĠVerein": 47431, "ÙĤØ·": 47432, "estry": 47433, "Ġclás": 47434, "Ġcheddar": 47435, "Ġaccusing": 47436, "ĠScientific": 47437, "ĠбÑĥде": 47438, "ĠCyrus": 47439, "εÏĦε": 47440, "Ĩĵê³ł": 47441, "Ġë³Ħ": 47442, "Ġcurd": 47443, "Ġreferrals": 47444, "shift": 47445, "åįķ": 47446, "ników": 47447, "Ġmier": 47448, "Ġconfronting": 47449, "ê²ĥëıĦ": 47450, "awl": 47451, "Ġtryin": 47452, "Ġê·¸ëŀĺìļĶ": 47453, "Ġchiar": 47454, "Ġìĺ¤ëĬĺëıĦ": 47455, "æĶ¿æ²»": 47456, "esque": 47457, "Ġmismos": 47458, "ĠShak": 47459, "Ġsociaux": 47460, "ĠpiÅŁ": 47461, "ĠkiÅŁi": 47462, "Ġcyan": 47463, "hay": 47464, "bew": 47465, "bod": 47466, "Ġι": 47467, "ĠMainly": 47468, "ÑİÑĤÑĮ": 47469, "habitude": 47470, "ĠÑģпокой": 47471, "è·ŁæĪij": 47472, "Ġprecon": 47473, "ĠMandy": 47474, "ðŁ¤£": 47475, "illos": 47476, "Ġgrupp": 47477, "Ġcrumble": 47478, "Ġconstructor": 47479, "ervices": 47480, "Ġlighthouse": 47481, "ĠConcept": 47482, "анÑĤи": 47483, "altro": 47484, "hope": 47485, "ĠAlleg": 47486, "ìĸ´ë¥¼": 47487, "pieces": 47488, "ounter": 47489, "ĠíķĺëĭĪê¹Į": 47490, "ĠìĿ¸íĦ°ë": 47491, "Ġvéritable": 47492, "Ġthreaded": 47493, "blind": 47494, "ĤĺëĿ¼": 47495, "Ġtrays": 47496, "ĠEdison": 47497, "ĠÃĸz": 47498, "ĠStevie": 47499, "Ġlender": 47500, "Ġbrigade": 47501, "Ġdeutsche": 47502, "muffled": 47503, "bart": 47504, "Ġinsanity": 47505, "Ġsavvy": 47506, "Ġsensational": 47507, "Ġderechos": 47508, "ĠMX": 47509, "ĠпÑĢеп": 47510, "Ġthreatens": 47511, "ĠrealtÃł": 47512, "Ġindicative": 47513, "Ġchops": 47514, "Ġbenefiting": 47515, "ĠVernon": 47516, "ĠStrand": 47517, "nun": 47518, "quently": 47519, "101": 47520, "Ġeel": 47521, "ìĪĻ": 47522, "rints": 47523, "ĠÙħس": 47524, "Ġبد": 47525, "ĠпоÑģÑĤÑĢо": 47526, "ĠyapmÄ±ÅŁ": 47527, "Ġolması": 47528, "Ġiedereen": 47529, "olé": 47530, "kef": 47531, "Ġë°ľìĥĿ": 47532, "Ġrained": 47533, "Ġalmighty": 47534, "ĠвÑĭд": 47535, "ĠCPR": 47536, "Fre": 47537, "Ġinhabited": 47538, "Ġarbets": 47539, "Ġakin": 47540, "аÑģÑĤв": 47541, "vania": 47542, "Ġhäufig": 47543, "ĠMatte": 47544, "sorry": 47545, "Jenny": 47546, "ĠгÑĢад": 47547, "Ġwhit": 47548, "Ġbrokers": 47549, "å¯Ł": 47550, "Ġhine": 47551, "asten": 47552, "ĠгÑĢÑĥ": 47553, "MB": 47554, "ĠPRI": 47555, "Sab": 47556, "Ġwrestler": 47557, "Ġfacilitating": 47558, "Ġehkä": 47559, "ĠCred": 47560, "Ġ127": 47561, "Ġnothin": 47562, "Ġmandated": 47563, "å¯Į": 47564, "ÑĥÑĤÑģÑĤв": 47565, "Frank": 47566, "Ġwors": 47567, "ĠdzieÅĦ": 47568, "ĠUnderground": 47569, "Ġznajdu": 47570, "ĠBä": 47571, "ĠPrinzip": 47572, "аÑĤелей": 47573, "Ġveterinar": 47574, "Ġsplendid": 47575, "Ġrozp": 47576, "Ġpsychopath": 47577, "igon": 47578, "Ġhops": 47579, "Ġcần": 47580, "ĠXian": 47581, "Ġtroisième": 47582, "Ġproducto": 47583, "ĠdeÄŁer": 47584, "ĠContinuing": 47585, "ивал": 47586, "cık": 47587, "Ġmoisturizer": 47588, "White": 47589, "Ġsiis": 47590, "ĠEverest": 47591, "ienced": 47592, "Ġcảm": 47593, "ĠJapon": 47594, "´ìłĦ": 47595, "ĠtenÃŃan": 47596, "Ġencanta": 47597, "Mm": 47598, "Ġdropdown": 47599, "ĠIya": 47600, "³´ë©´": 47601, "Ġwording": 47602, "ĠSqueeze": 47603, "ĠMaple": 47604, "Ġclarified": 47605, "ĠMunicip": 47606, "ĠRouge": 47607, "ĠNicki": 47608, "ĠGoo": 47609, "volt": 47610, "tek": 47611, "fecture": 47612, "fred": 47613, "arrive": 47614, "ãĥ¼ãģĦ": 47615, "tez": 47616, "Ep": 47617, "Ġobras": 47618, "ĠVID": 47619, "ĠRiv": 47620, "ĠModi": 47621, "ibe": 47622, "Ġacontecendo": 47623, "Ġimitation": 47624, "Ġcamouflage": 47625, "Ġspanning": 47626, "ĠSECRET": 47627, "ĠOreo": 47628, "ìĨĮ리": 47629, "Ġhunch": 47630, "ĠcaÅĤe": 47631, "Ġspontaneously": 47632, "ĠPerd": 47633, "Ġetap": 47634, "ĠHole": 47635, "ĠDisability": 47636, "Ġafterlife": 47637, "æģ©": 47638, "Ġtestified": 47639, "Ġpresup": 47640, "Ġpetroleum": 47641, "Ġcontrario": 47642, "ĠAssessment": 47643, "ÄŁlu": 47644, "Ġpests": 47645, "Ġdilig": 47646, "ĠвÑģÑĤÑĢеÑĤ": 47647, "Ġconséqu": 47648, "Ġcannons": 47649, "Ġcanoe": 47650, "ĠMile": 47651, "Ġcitoy": 47652, "Ġbegged": 47653, "ĠMinnie": 47654, "ÅĤych": 47655, "Ġprincipe": 47656, "ÏĢÏĮν": 47657, "mniej": 47658, "Ġwert": 47659, "Ġëĭ¤ëĵ¤": 47660, "anse": 47661, "Ġuncles": 47662, "Ġprovocative": 47663, "Ġintersections": 47664, "Ġdemocrats": 47665, "ĠJulius": 47666, "инки": 47667, "ygusal": 47668, "Ġ׾×ķ": 47669, "Ġgjorde": 47670, "Ġgasket": 47671, "ĠBock": 47672, "Ġİn": 47673, "breat": 47674, "ĠEquity": 47675, "ardı": 47676, "Ġканале": 47677, "Ġдней": 47678, "ĠtỼi": 47679, "Ġfixture": 47680, "Ġabuses": 47681, "Ġvaya": 47682, "Ġouvert": 47683, "Ġmulticultural": 47684, "Ġcontexto": 47685, "ĠSesame": 47686, "Ġdépl": 47687, "Ġconsomm": 47688, "ĠParte": 47689, "Ġpem": 47690, "ĠConan": 47691, "ĠбÑĸлÑĮ": 47692, "Ġpersuaded": 47693, "Ġdrains": 47694, "Moo": 47695, "FORE": 47696, "ĠбаÑĤ": 47697, "Ġfod": 47698, "ĠProducts": 47699, "ì§Ħì§ľ": 47700, "Ġ\"[": 47701, "ĠWick": 47702, "ĠNaruto": 47703, "нали": 47704, "ryw": 47705, "Ġlodge": 47706, "Ġinh": 47707, "Ġvontade": 47708, "Ġdij": 47709, "ĠJesús": 47710, "Looking": 47711, "Ġforearm": 47712, "ĠIntegration": 47713, "ĠHARRIS": 47714, "Ġtoolbar": 47715, "leader": 47716, "Ġseldom": 47717, "ĠбÑĢоÑģ": 47718, "ĠKook": 47719, "онд": 47720, "Ġmonopol": 47721, "Ġmillet": 47722, "Ġlira": 47723, "ĠAsians": 47724, "Ġ1890": 47725, "ciÄŁim": 47726, "Ġeden": 47727, "ĠIKEA": 47728, "ĠNeighbor": 47729, "ĠKazuya": 47730, "üd": 47731, "Ġpsychedel": 47732, "Ġenvisioned": 47733, "åĿĹ": 47734, "Ġï·»": 47735, "Ġwunder": 47736, "ĠBulgaria": 47737, "Brid": 47738, "Ġmarrow": 47739, "Ġdepiction": 47740, "ĠTin": 47741, "ĠPharise": 47742, "Ġeinzige": 47743, "Ġblindly": 47744, "ãģĽãģ¦": 47745, "Ġdefens": 47746, "Dire": 47747, "Ġvibrating": 47748, "Ġtrolls": 47749, "Ġdisrespectful": 47750, "Ġwod": 47751, "Ġstimuli": 47752, "Ġcreeping": 47753, "Ġclairement": 47754, "Ġscariest": 47755, "Ġdécouvrir": 47756, "Ġ104": 47757, "ĠвеÑĢÑħ": 47758, "ĠÅĤat": 47759, "Ġróżne": 47760, "Ġbarley": 47761, "ĠRepl": 47762, "ĠTwe": 47763, "kke": 47764, "ĠãģĿãĤĮ": 47765, "ĠRedmi": 47766, "ĠMetroid": 47767, "ĠήÏĦαν": 47768, "Check": 47769, "ĠSEN": 47770, "Ġido": 47771, "ÑĤоÑĢии": 47772, "óp": 47773, "UNKNOWN": 47774, "Ġändern": 47775, "ĠJuice": 47776, "ĠGesicht": 47777, "å°±æľĥ": 47778, "ĠнаÑģÑĤолÑĮко": 47779, "íĥķ": 47780, "ÂŃ": 47781, "exhales": 47782, "Ġì´ī": 47783, "Ġjsem": 47784, "ÏĢÏīÏĤ": 47785, "Ġitt": 47786, "ëªħìĿ´": 47787, "Ġremix": 47788, "Ġblossoms": 47789, "ĠRenee": 47790, "isations": 47791, "ìĬ¤íĦ°": 47792, "Ġë³´ìĿ´ëĬĶ": 47793, "uestas": 47794, "opedia": 47795, "ĠAim": 47796, "ìĿ´ì¦Ī": 47797, "scene": 47798, "Ġleakage": 47799, "uckt": 47800, "Sad": 47801, "Ask": 47802, "Ġsuspense": 47803, "Ġimpost": 47804, "ĠStrategic": 47805, "ĠItÃŃs": 47806, "âĢĮ": 47807, "Ġkeyboards": 47808, "Ġamusing": 47809, "ogr": 47810, "iderman": 47811, "ŀĸ": 47812, "ĠвижÑĥ": 47813, "Ġdips": 47814, "Ġapologized": 47815, "ĠSTAR": 47816, "Ġescuela": 47817, "ĠChing": 47818, "нениÑı": 47819, "Ġë¶Ģë¶ĦìĿ´": 47820, "ĠFleet": 47821, "Ġsamb": 47822, "Ġentsprechend": 47823, "Ġelectrodes": 47824, "ĠFreiheit": 47825, "æĪijä¸įçŁ¥éģĵ": 47826, "ĠShrim": 47827, "iÃŁe": 47828, "Ġselections": 47829, "Ġfordi": 47830, "Ġdoss": 47831, "ÑıÑĩ": 47832, "Ġdiscriminate": 47833, "ĠAuÃŁerdem": 47834, "Ġdesenvolv": 47835, "ĠInternal": 47836, "ĠBenedict": 47837, "å¯Ĩ": 47838, "ĠShiv": 47839, "Missy": 47840, "ĠобнаÑĢÑĥж": 47841, "ĠнаÑģÑĤÑĢо": 47842, "Ġcontrolar": 47843, "ĠLia": 47844, "Ġopioids": 47845, "antu": 47846, "Ġcupboard": 47847, "æģIJ": 47848, "ге": 47849, "achts": 47850, "Ġcurated": 47851, "Ġxem": 47852, "Ġweary": 47853, "Ġbrethren": 47854, "Ġbudgeting": 47855, "Ġpourtant": 47856, "éļ»": 47857, "aisia": 47858, "ĠоÑĤвеÑĩ": 47859, "ĠGIS": 47860, "μαι": 47861, "Ġש×Ķ×ķ×IJ": 47862, "Ġsaud": 47863, "ĠlỼ": 47864, "ÐķТ": 47865, "ubine": 47866, "ĠнÑĥжен": 47867, "Ġkidnapping": 47868, "Ġbrat": 47869, "ĠTerre": 47870, "ĠMonet": 47871, "Ġë§ĪìĬ¤íģ": 47872, "Ġflashy": 47873, "ĠISBN": 47874, "Ġfreelance": 47875, "iage": 47876, "Ġjunge": 47877, "ì¶©": 47878, "ceral": 47879, "ĠÑĤоÑĩки": 47880, "Ġformulate": 47881, "ĠFER": 47882, "ĠDartmouth": 47883, "ìľ¼ë©´ìĦľ": 47884, "å¢ĥ": 47885, "owiÄħ": 47886, "ĠëĶĶìŀIJ": 47887, "Ġregiment": 47888, "Ġmetabolismo": 47889, "ĠParr": 47890, "Ġì¶©ë¶Ħ": 47891, "Ġsanity": 47892, "ĠLal": 47893, "ĠGö": 47894, "ĠGla": 47895, "Ġproto": 47896, "Ġmicroscopic": 47897, "Ġkang": 47898, "ĠScalia": 47899, "Ġpug": 47900, "ĠScore": 47901, "ĠSavannah": 47902, "Ġgarde": 47903, "ĠNOR": 47904, "å°įåIJ§": 47905, "Ġscheint": 47906, "ĠpóÅĤ": 47907, "Ġcorri": 47908, "Ġbrute": 47909, "ĠÅĤad": 47910, "ä»ĸ们": 47911, "Ġsucceeding": 47912, "Ġbicycles": 47913, "Non": 47914, "Ġseekers": 47915, "Ġunconditional": 47916, "Ġrhymes": 47917, "ĠGarage": 47918, "Ġinvoice": 47919, "Ġcanvi": 47920, "neck": 47921, "Ġcustomizable": 47922, "iritual": 47923, "Queen": 47924, "íķĺìĭľëĬĶ": 47925, "Ġpowerless": 47926, "Ġcsak": 47927, "ä¸įä¼ļ": 47928, "isoft": 47929, "ĠìłķíĻķ": 47930, "Ġnhân": 47931, "ĠMAND": 47932, "ĠHaf": 47933, "Ġrevolves": 47934, "ä¹Łåı¯ä»¥": 47935, "ovan": 47936, "aroo": 47937, "ĠGrind": 47938, "éĽª": 47939, "Ġindispensable": 47940, "Ġconsulted": 47941, "ĠClinical": 47942, "Acc": 47943, "Ġolhos": 47944, "Ġmonter": 47945, "ĠHana": 47946, "etah": 47947, "Ġvaan": 47948, "Ġtigers": 47949, "Ġcaucus": 47950, "ðŁĺĤ": 47951, "³´ìŀIJ": 47952, "powers": 47953, "iums": 47954, "ĠíĨłë": 47955, "Ġtradicional": 47956, "Ġresonated": 47957, "Ġìĭłê¸°": 47958, "them": 47959, "Robert": 47960, "Ġelemento": 47961, "Ġantid": 47962, "ĠобÑģ": 47963, "Ġnatives": 47964, "Ġloca": 47965, "owment": 47966, "ĠTight": 47967, "ĠæĢĿ": 47968, "Ġmelan": 47969, "ĠNue": 47970, "amis": 47971, "Ġsorgen": 47972, "asına": 47973, "Home": 47974, "ĠPUBG": 47975, "Ġawfully": 47976, "ĠShore": 47977, "ĠPerché": 47978, "ĠLau": 47979, "ĠCinderella": 47980, "ĠChest": 47981, "Ġsemantic": 47982, "Ġdeserted": 47983, "ĠMomo": 47984, "ĠHernandez": 47985, "genes": 47986, "ĠAdult": 47987, "иÑĩеÑģкого": 47988, "oshima": 47989, "ĠcaracterÃŃsticas": 47990, "ĠKL": 47991, "´ìŀ¥": 47992, "ocar": 47993, "Ġfehlt": 47994, "Ġdruk": 47995, "ĠPoppy": 47996, "ENGLISH": 47997, "ĠVergleich": 47998, "Brien": 47999, "Ġrecomp": 48000, "ĠÑģд": 48001, "Ġmerger": 48002, "Ġmarketers": 48003, "Ġhoneymoon": 48004, "Ġpenso": 48005, "Ġbelli": 48006, "еÑĤÑĥ": 48007, "Ġbanker": 48008, "Camera": 48009, "ĠStall": 48010, "ĠStamp": 48011, "ĠBite": 48012, "ежде": 48013, "Ġsür": 48014, "Ġgüç": 48015, "ĠPassover": 48016, "ĠBugün": 48017, "ĠÑģожалениÑİ": 48018, "Ġниз": 48019, "Ġmanure": 48020, "Ġglacier": 48021, "è«ĩ": 48022, "RAY": 48023, "terror": 48024, "Ġsalads": 48025, "Ġhurricanes": 48026, "ĠDesigner": 48027, "atorio": 48028, "Ġfactual": 48029, "ĠTammy": 48030, "ĠзвÑĥÑĩ": 48031, "Ġintroductions": 48032, "Ġhousekeeping": 48033, "Ġhanger": 48034, "ëĭĺë": 48035, "akte": 48036, "ĠCola": 48037, "']": 48038, "ĠGender": 48039, "оÑĢон": 48040, "ipse": 48041, "icias": 48042, "Ġsuccessive": 48043, "Ġpolitic": 48044, "Ġhöher": 48045, "ĠQiao": 48046, "ĠGimme": 48047, "Ġлож": 48048, "Ġseb": 48049, "ĠWeiter": 48050, "ĠSakura": 48051, "ĠBoulder": 48052, "ĠAmérica": 48053, "peÅĤnie": 48054, "ĠtecnologÃŃa": 48055, "ishops": 48056, "fur": 48057, "Ġmoonlight": 48058, "Ġdispersed": 48059, "Ġrez": 48060, "енное": 48061, "алÑĮнÑĥÑİ": 48062, "ĠTwelve": 48063, "ĠHOR": 48064, "ìĭ¤íŀĪ": 48065, "ilage": 48066, "Ġshaded": 48067, "Ġresumes": 48068, "ĠPeanut": 48069, "ĠMILL": 48070, "apons": 48071, "ĠUFC": 48072, "ĠSole": 48073, "Ġjoystick": 48074, "ĠOlivier": 48075, "warming": 48076, "Ġsyllabus": 48077, "ĠобÑīе": 48078, "Ġhiá»ĩn": 48079, "Ġfesta": 48080, "Ġcradle": 48081, "ĠZac": 48082, "Ġremembrance": 48083, "Ġê°ĻìķĦìĦľ": 48084, "ĠpiÄĻk": 48085, "Ġcoexist": 48086, "ĠVII": 48087, "Ġáreas": 48088, "Ġuważ": 48089, "Ġobservers": 48090, "Ġmänniskor": 48091, "coon": 48092, "ĠDAM": 48093, "Ġnaszym": 48094, "Ġalligator": 48095, "ĠFreeze": 48096, "ĠEstate": 48097, "ĠÑĤÑĢади": 48098, "Ġundercover": 48099, "Ġnies": 48100, "ĠFehler": 48101, "plin": 48102, "ĠKabul": 48103, "ilate": 48104, "Ġê³łìĸij": 48105, "Ġmop": 48106, "ìĦ¼": 48107, "Ġanderer": 48108, "ĠKELL": 48109, "оки": 48110, "ĠжеÑģÑĤ": 48111, "Ġgrazing": 48112, "ĠdaÃŃ": 48113, "Ġcapitalize": 48114, "Ġapex": 48115, "Ġnurturing": 48116, "Ġcortar": 48117, "Ġcontrac": 48118, "ımızı": 48119, "Ġtandem": 48120, "éĥ½æľī": 48121, "gement": 48122, "ĠÑģиÑģÑĤема": 48123, "Ġmanque": 48124, "iajÄħ": 48125, "WOR": 48126, "Ġاب": 48127, "Ġcarts": 48128, "ANO": 48129, "Ġë°Ľê³ł": 48130, "ĠCena": 48131, "ĠBiology": 48132, "idar": 48133, "Ġaż": 48134, "erne": 48135, "anu": 48136, "Ġthanked": 48137, "Ġsubmarines": 48138, "Ġmanic": 48139, "Ġмоз": 48140, "ä¼Ĭ": 48141, "instant": 48142, "essential": 48143, "Ġsamurai": 48144, "Ġpasti": 48145, "Ġalan": 48146, "Ġbroch": 48147, "Ġbaker": 48148, "ĠGuill": 48149, "¨¼": 48150, "Ġwithdrawn": 48151, "ëĭĿ": 48152, "Perfect": 48153, "quency": 48154, "Ġstreamlined": 48155, "Ġ1300": 48156, "´ëıĦ": 48157, "Ġëĸłë": 48158, "Ġãģ¯ãģĦ": 48159, "Ġhvad": 48160, "ä¸Ģå®ļè¦ģ": 48161, "Ġverbally": 48162, "ĠKons": 48163, "Ġì¡°ìĭ¬": 48164, "Ġdiez": 48165, "æİ°æİ°": 48166, "Ġchuckling": 48167, "ĠMih": 48168, "Ġrallies": 48169, "Ġmanter": 48170, "Ġearnest": 48171, "super": 48172, "Ġgece": 48173, "ĠRend": 48174, "ĠGerade": 48175, "jenigen": 48176, "ĠVall": 48177, "ĠìŀĪëĤĺ": 48178, "ĠÑģказала": 48179, "Ġtrabalh": 48180, "ĠнаÑĪем": 48181, "ĠмеÑħ": 48182, "ikit": 48183, "Ġnouns": 48184, "Ġneurological": 48185, "Ġmotivational": 48186, "ĠMcMahon": 48187, "ĠFinished": 48188, "Ġë³´ìĿ´": 48189, "ĠFields": 48190, "Ġadolescents": 48191, "ĠTisch": 48192, "ĠNeben": 48193, "ĠFlowers": 48194, "ĠEnerg": 48195, "Ġdiret": 48196, "ĠThi": 48197, "ĠPicas": 48198, "æĥľ": 48199, "æĢİä¹Īæł·": 48200, "Ġavete": 48201, "ĠFors": 48202, "ĠChapel": 48203, "Não": 48204, "Et": 48205, "ĠÑģодеÑĢж": 48206, "reno": 48207, "Ġsven": 48208, "ĠdostÄĻp": 48209, "nee": 48210, "ĠSnapdragon": 48211, "ĠIDs": 48212, "ìķĺëĬĶëį°": 48213, "ר×ļ": 48214, "Ġsunflower": 48215, "Ġperpetual": 48216, "ç³ĸ": 48217, "Ġknights": 48218, "Ġgird": 48219, "ĠTold": 48220, "Ġvolcanoes": 48221, "Ġadversary": 48222, "ĠEconomy": 48223, "Ġextrapol": 48224, "Ġbluetooth": 48225, "Ġzooming": 48226, "Ġskys": 48227, "Ġgenial": 48228, "ÃŃculos": 48229, "ambre": 48230, "ĠмеÑĢ": 48231, "Ġteeny": 48232, "Ġstressing": 48233, "ìķĮ": 48234, "ONY": 48235, "Ġtranslucent": 48236, "Ġrounding": 48237, "Ġgrues": 48238, "×Ļ׳×Ķ": 48239, "après": 48240, "Ġprueba": 48241, "Ġpolygon": 48242, "Ġblueberry": 48243, "ĠProgramm": 48244, "Ġtrenches": 48245, "Ġsebagai": 48246, "Ġpalate": 48247, "Ġlaude": 48248, "Ġbehaved": 48249, "Ġlongitudinal": 48250, "ĠModule": 48251, "Ġadmir": 48252, "λι": 48253, "Greg": 48254, "Ġwyst": 48255, "Ġpropagate": 48256, "Ġmolds": 48257, "ĠTub": 48258, "ĠLoud": 48259, "usto": 48260, "Ġunstoppable": 48261, "Ġreinforcing": 48262, "éĿŀ常çļĦ": 48263, "ĠпÑĢоблема": 48264, "Ġpotencial": 48265, "Ġhemp": 48266, "ìŀĶ": 48267, "य": 48268, "Ġoptic": 48269, "Ġerfolgreich": 48270, "ÑģÑĭ": 48271, "олÑĮÑĪе": 48272, "urst": 48273, "ĠPois": 48274, "Ġrespondents": 48275, "Ġnehme": 48276, "ĠExternal": 48277, "olate": 48278, "Hyun": 48279, "Ġquartz": 48280, "Ġmathematician": 48281, "Ġbásicamente": 48282, "Ġail": 48283, "ìłľë¥¼": 48284, "attutto": 48285, "Ġnooit": 48286, "Ġafflict": 48287, "ĠOlga": 48288, "èŃ·": 48289, "ĠнаÑĤ": 48290, "Ġdites": 48291, "Ġrealidade": 48292, "Ġkän": 48293, "Ġuniqueness": 48294, "Ġpadres": 48295, "Ġsubsidi": 48296, "Ġpigeons": 48297, "βα": 48298, "stad": 48299, "Ġderen": 48300, "ĠСлед": 48301, "doo": 48302, "ĠопиÑģании": 48303, "Ġamber": 48304, "Ġgoosebumps": 48305, "ĠfrÃ¥gor": 48306, "ĠVital": 48307, "ĠIsraelites": 48308, "wasser": 48309, "Isn": 48310, "Ġcommits": 48311, "ĠSTEVEN": 48312, "ĠBevölker": 48313, "uitive": 48314, "Ġlegen": 48315, "Ġbruk": 48316, "иÑĢован": 48317, "ynen": 48318, "helm": 48319, "Ġgenerational": 48320, "ĠLändern": 48321, "οιÏĢÏĮν": 48322, "uzu": 48323, "Ġcaller": 48324, "онÑĮ": 48325, "ümü": 48326, "Ġbesar": 48327, "Ġplats": 48328, "Ġmigrated": 48329, "Ġjap": 48330, "ĠWAR": 48331, "Ġdissect": 48332, "ĠZusch": 48333, "ĠZeiten": 48334, "ĠLions": 48335, "ĠDF": 48336, "âĶ": 48337, "кив": 48338, "Ġpedestrians": 48339, "ĠMarilyn": 48340, "dock": 48341, "Ġyht": 48342, "Ġreincarn": 48343, "ĠSono": 48344, "ĠGrowth": 48345, "ÑĥÑģов": 48346, "Ġdungeons": 48347, "Ġbagus": 48348, "kich": 48349, "ĠÑĥкÑĢаÑĹ": 48350, "éĨ«": 48351, "ĠKeller": 48352, "chemistry": 48353, "Japanese": 48354, "Ġwillst": 48355, "Ġdecomposition": 48356, "ĠÑģÑĤен": 48357, "Ġrevived": 48358, "íķĻêµIJ": 48359, "ĠÅĵ": 48360, "ä½IJ": 48361, "ìĭ¸": 48362, "ippy": 48363, "Ġhourly": 48364, "jän": 48365, "ĠWorkshop": 48366, "Ŀ¼ìĦľ": 48367, "Ġcuarto": 48368, "Ġpatrim": 48369, "ĠBurch": 48370, "ĠìŀĪ기": 48371, "Ġhepat": 48372, "ĠhÃłng": 48373, "ĠëĮĢíķ´": 48374, "ĠваÑĪи": 48375, "Ġrework": 48376, "Ġparse": 48377, "Ġçıktı": 48378, "ĠSax": 48379, "ĠMongo": 48380, "ĠAaah": 48381, "ramble": 48382, "DJ": 48383, "Ġstabilized": 48384, "ĠSpeech": 48385, "Books": 48386, "Ġhurdles": 48387, "ĠWO": 48388, "ĠLamborg": 48389, "Ġ1933": 48390, "Ġvorbere": 48391, "Ġclinically": 48392, "Ġbreathtaking": 48393, "ĠGateway": 48394, "пеÑĢвÑĭÑħ": 48395, "uters": 48396, "Ġë¹µ": 48397, "Ġyeter": 48398, "Ġpulley": 48399, "Ġmuffin": 48400, "ĠPrefer": 48401, "ĠPence": 48402, "Ġinformação": 48403, "ìĬ¤íĬ¸ë": 48404, "ãĤ¸ãĥ£": 48405, "ĠTurtle": 48406, "ĠRegina": 48407, "ĠLoad": 48408, "does": 48409, "panze": 48410, "¸Ķ": 48411, "Ġmina": 48412, "ĠLatinos": 48413, "ammers": 48414, "ĠTort": 48415, "ĠBeyonce": 48416, "имоÑģÑĤи": 48417, "ĠвопÑĢоÑģÑĭ": 48418, "Ġbulun": 48419, "èĢĮå·²": 48420, "inek": 48421, "bereich": 48422, "Ġpasture": 48423, "ĠOA": 48424, "ĠMelt": 48425, "ĠEtt": 48426, "ĠDY": 48427, "Ġobwohl": 48428, "Ġleagues": 48429, "ÑĤеÑģÑĮ": 48430, "ĠкÑĥÑģ": 48431, "Ġvors": 48432, "Ġtopp": 48433, "ographical": 48434, "asst": 48435, "Ġlindo": 48436, "Ġë°ĿíĺĶ": 48437, "Ġréfl": 48438, "Ġclimbs": 48439, "Ġvarsa": 48440, "Ġmethyl": 48441, "ĠKarere": 48442, "Æ°á»Ł": 48443, "Rad": 48444, "Ġpreparedness": 48445, "онÑĩ": 48446, "ĠOD": 48447, "ĠCGI": 48448, "Ġम": 48449, "Ġspeechless": 48450, "Ġlasci": 48451, "Ġbolag": 48452, "ĠÑħоÑĩеÑĤÑģÑı": 48453, "Ġgrieving": 48454, "ĠJohannes": 48455, "ĠCarroll": 48456, "adaki": 48457, "άë": 48458, "ĠsÅĤu": 48459, "Ġinnerhalb": 48460, "Ġgymnastics": 48461, "пÑĢи": 48462, "ifiques": 48463, "Ġkarate": 48464, "Ġdomu": 48465, "ãģĿãĤĮãģ§": 48466, "OTHER": 48467, "Ġdemandé": 48468, "Ġbooklet": 48469, "ĠKyoto": 48470, "Ġwoh": 48471, "ĠMarÃŃa": 48472, "violent": 48473, "JE": 48474, "Ġlóg": 48475, "Ġbrutally": 48476, "cot": 48477, "ĠÙħÛĮ": 48478, "ĠWarsz": 48479, "å®Ī": 48480, "wol": 48481, "Ġmikä": 48482, "ĠPronounce": 48483, "ĠBrendan": 48484, "Ġroup": 48485, "Ġitaliano": 48486, "å¦ĤæŃ¤": 48487, "ĠкомпÑĮÑİÑĤ": 48488, "Ġurging": 48489, "edes": 48490, "Ġcarbono": 48491, "ĠRichardson": 48492, "ĠÐĿаÑĩ": 48493, "ĠTrainer": 48494, "ĠCrimea": 48495, "Ġdiapers": 48496, "Ġcovet": 48497, "ĠMahar": 48498, "ĠHutch": 48499, "ĠAusw": 48500, "berty": 48501, "Ġindifferent": 48502, "кÑĢеÑĤ": 48503, "uldade": 48504, "Ġharms": 48505, "¢ÙĨ": 48506, "lesia": 48507, "Ġgio": 48508, "ĠMistress": 48509, "ĠKnox": 48510, "ĠFREE": 48511, "Ġ루ë": 48512, "ĠнаÑĪа": 48513, "Ġinvincible": 48514, "Ġmaiden": 48515, "ĠJeez": 48516, "Ġbreve": 48517, "pole": 48518, "Ġcriticisms": 48519, "ĠRusia": 48520, "म": 48521, "phin": 48522, "ĠCompare": 48523, "ĠBON": 48524, "Ġsneaking": 48525, "ĠRails": 48526, "ĠGeral": 48527, "Ġ1953": 48528, "Hola": 48529, "ĠопÑĭÑĤ": 48530, "Ġrainforest": 48531, "Ġbelum": 48532, "ĠObi": 48533, "ĠISS": 48534, "ãĤĮãģªãģĦ": 48535, "ĠСв": 48536, "Ġblond": 48537, "Ġwzgl": 48538, "ĠpowiedziaÅĤ": 48539, "Ġchoking": 48540, "ĠSongs": 48541, "ĠBiraz": 48542, "Ġyells": 48543, "Ġstylist": 48544, "ÏĮÏĦε": 48545, "Ġschreiben": 48546, "ĠJaw": 48547, "ĠEleven": 48548, "ĠRif": 48549, "/.": 48550, "Ġìĺ¤ëŀľë§Į": 48551, "Ġtreaties": 48552, "uffed": 48553, "ĠâĪĴ": 48554, "Ġroofs": 48555, "à¹Ģส": 48556, "Ġë»": 48557, "Ġsparkle": 48558, "ĠKiev": 48559, "ĠArgu": 48560, "erecht": 48561, "ĠÐĿадо": 48562, "ĠFIL": 48563, "Ġmolta": 48564, "ĠDevi": 48565, "Ġcampe": 48566, "Ġbenevol": 48567, "ĠTough": 48568, "Ġmoim": 48569, "Ġevacuate": 48570, "Ġerrado": 48571, "å©Ĩ": 48572, "ÑĢÑĥго": 48573, "Ġíİĺ": 48574, "ĠÎĵια": 48575, "Ġweaken": 48576, "Ġilluminated": 48577, "Ġsiglo": 48578, "ĠVacc": 48579, "ией": 48580, "alis": 48581, "ĠÑĥÑģÑĤÑĢой": 48582, "Ġdona": 48583, "ÅĤos": 48584, "üman": 48585, "Ġproducción": 48586, "Ġclot": 48587, "ĠMango": 48588, "Ġuneasy": 48589, "Ġshuts": 48590, "ĠExamples": 48591, "vell": 48592, "ebe": 48593, "Ġpromptly": 48594, "ĠTeles": 48595, "ĠпÑĢоÑĪл": 48596, "Ġpuerta": 48597, "Ġüberzeug": 48598, "Ġcoch": 48599, "social": 48600, "ĠBenson": 48601, "ĠMeth": 48602, "ĠExped": 48603, "Ġsupplemental": 48604, "Ġconceive": 48605, "Ġ×ĺ×ķ×ij": 48606, "Ġcaptivity": 48607, "ıĻìķĪ": 48608, "ĠÑħÑĥд": 48609, "forming": 48610, "Ġuploads": 48611, "Ġturbulence": 48612, "joint": 48613, "Ġsatisfactory": 48614, "ĠAnime": 48615, "Ġwashes": 48616, "Ġliberals": 48617, "ĠSunshine": 48618, "ĠREAL": 48619, "ublik": 48620, "binary": 48621, "Tony": 48622, "Ġpolarized": 48623, "Ġenriched": 48624, "taking": 48625, "ĠëģĿëĤĺ": 48626, "Ġpleasures": 48627, "Ġextermin": 48628, "inese": 48629, "atl": 48630, "vär": 48631, "аÑĢÑĭ": 48632, "ĠmyÅĽ": 48633, "narrator": 48634, "Ġодном": 48635, "ĠnajwiÄĻ": 48636, "Ġmobilize": 48637, "Ġmillor": 48638, "Ġata": 48639, "æ··": 48640, "ĠpolÃŃtico": 48641, "Ġplead": 48642, "Ġpainters": 48643, "ĠSow": 48644, "оÑĦ": 48645, "ĠìĺĽëĤł": 48646, "ĠÑĩÑĤоб": 48647, "Ġsabor": 48648, "ĠUndert": 48649, "ĠJERRY": 48650, "Å¡ÃŃ": 48651, "Ġë°ĸìĹIJ": 48652, "Ġprécéd": 48653, "Ġannotation": 48654, "ĠInaudible": 48655, "Ġtextured": 48656, "Ġfisherman": 48657, "vordan": 48658, "icherung": 48659, "ĠìłģìĿ´": 48660, "Ġgezeigt": 48661, "Ġmandates": 48662, "Ġbeak": 48663, "ĠTWO": 48664, "ĠAkbar": 48665, "ilian": 48666, "Ġtiếp": 48667, "Ġsuperiority": 48668, "inku": 48669, "Ġlys": 48670, "ĠFCC": 48671, "ĠCPA": 48672, "ustering": 48673, "nicos": 48674, "anja": 48675, "Ġchills": 48676, "ĠCage": 48677, "Ġsealing": 48678, "Ġsaç": 48679, "Ġdedans": 48680, "ĠAlger": 48681, "Ġspezie": 48682, "Ġcoloss": 48683, "ıyı": 48684, "clockwise": 48685, "Ġexactamente": 48686, "Ġiemand": 48687, "amı": 48688, "Ġmandar": 48689, "raj": 48690, "faced": 48691, "agua": 48692, "Ġê¹Ķë": 48693, "Ġinsbesondere": 48694, "Ġdrizzle": 48695, "Ġdiminish": 48696, "ĠYoda": 48697, "AI": 48698, "Ġbilmiyorum": 48699, "ĠMMA": 48700, "ategory": 48701, "ĠпеÑĢеп": 48702, "Ġparticipar": 48703, "Ġnormalized": 48704, "Ġcomplexities": 48705, "æ´²": 48706, "æİ§": 48707, "аÑĢов": 48708, "mist": 48709, "icha": 48710, "Group": 48711, "Ġresiliency": 48712, "Ġnogle": 48713, "ĠCNC": 48714, "prü": 48715, "Ġphysicists": 48716, "нок": 48717, "LI": 48718, "Ġstuffs": 48719, "Ġsistemas": 48720, "Ġinterfering": 48721, "ĠMarvin": 48722, "ército": 48723, "ĠìĹĨê³ł": 48724, "Ġsonic": 48725, "Ġequiv": 48726, "Ġabord": 48727, "ĠRamen": 48728, "Ġ09": 48729, "medim": 48730, "atiques": 48731, "ĠделаÑİÑĤ": 48732, "Ġunanimously": 48733, "Ġskirts": 48734, "ĠíĬ¹ë³Ħ": 48735, "ĠPrix": 48736, "kami": 48737, "Ġfruition": 48738, "Ġbirthdays": 48739, "иком": 48740, "Ġinaugural": 48741, "Ġcorrelate": 48742, "ĠTory": 48743, "ĠëĤĺìģ": 48744, "Ġdew": 48745, "ĠPrecis": 48746, "ihi": 48747, "Ġë¬¸ìłľê°Ģ": 48748, "Ġciting": 48749, "ĠLana": 48750, "ĠKag": 48751, "Ġplaythrough": 48752, "ĠProtocol": 48753, "frist": 48754, "hovah": 48755, "Ġmerciful": 48756, "Ġbilingual": 48757, "ĠGuitar": 48758, "rh": 48759, "Ġglamorous": 48760, "ĠVikings": 48761, "ĠOoooh": 48762, "íķĺëĬĶëį°": 48763, "ĠUganda": 48764, "Ġcollapses": 48765, "entry": 48766, "Ġantioxidants": 48767, "ëĤĺë": 48768, "ÑĪаÑı": 48769, "Ġtrivia": 48770, "Ġgäller": 48771, "Ġfungi": 48772, "Ġmilks": 48773, "Ġdicht": 48774, "μη": 48775, "poke": 48776, "ĠвÑĭпÑĥÑģк": 48777, "Ġfeeder": 48778, "ĠAlcohol": 48779, "hower": 48780, "Ġdeserving": 48781, "ĠRebel": 48782, "iosis": 48783, "Ġ103": 48784, "Ġhandout": 48785, "Ġenm": 48786, "Ġlandlords": 48787, "Ġgeology": 48788, "rils": 48789, "Ġcobra": 48790, "ĠVold": 48791, "ĠPanch": 48792, "ĠGREG": 48793, "Ġpross": 48794, "Ġbracelets": 48795, "ĠVega": 48796, "Ġrozum": 48797, "款": 48798, "азд": 48799, "ĠLynd": 48800, "ĠHonors": 48801, "Ġsurrendered": 48802, "Ġlibrarians": 48803, "125": 48804, "ĠÑģиг": 48805, "Ġuniformly": 48806, "ĠEagles": 48807, "ìķĻ": 48808, "иÑĤан": 48809, "andid": 48810, "ĠìłĪëĮĢ": 48811, "Ġض": 48812, "Ġarrests": 48813, "ĠCSV": 48814, "ĠAzerbaijan": 48815, "ortic": 48816, "ĠDX": 48817, "ĠAdventures": 48818, "Ġabus": 48819, "ĠFau": 48820, "Ġschlimm": 48821, "Ġrattling": 48822, "Ġconsumes": 48823, "ĠTolkien": 48824, "Ġresurrected": 48825, "ĠXY": 48826, "íĬ¸ê°Ģ": 48827, "ĠвÑĭÑģÑĤÑĥп": 48828, "ĠAngie": 48829, "żenia": 48830, "Mic": 48831, "ĠSheila": 48832, "achtet": 48833, "Ġoverst": 48834, "Ġlâ": 48835, "Ġineffective": 48836, "æĿ¡": 48837, "æĢİä¹ĪäºĨ": 48838, "å¿Ļ": 48839, "Ġwichtiger": 48840, "Ġvino": 48841, "Ġpum": 48842, "Ġangled": 48843, "ĠPione": 48844, "ĠMỹ": 48845, "ãģĿãĤĮãģ¯": 48846, "woÅĽÄĩ": 48847, "draw": 48848, "ัà¹Ī": 48849, "markets": 48850, "Ġcafes": 48851, "ĠCem": 48852, "âĿ¤": 48853, "ĠSuit": 48854, "MK": 48855, "Ġemphasizes": 48856, "Ġtortilla": 48857, "Ġmejorar": 48858, "ĠSurviv": 48859, "casting": 48860, "Ġeducación": 48861, "ĠGum": 48862, "uely": 48863, "ĠìĹ¬ê¸°ëĬĶ": 48864, "Ġstretchy": 48865, "ença": 48866, "Ġwithhold": 48867, "Ġexiting": 48868, "Ġenthalpy": 48869, "ĠTransit": 48870, "ılmÄ±ÅŁ": 48871, "alies": 48872, "Ġsalvar": 48873, "Ġleaned": 48874, "ĠgroÃŁes": 48875, "Ġfitt": 48876, "аки": 48877, "Sarah": 48878, "Ġhostel": 48879, "Ġfingerna": 48880, "ĠnadziejÄĻ": 48881, "wives": 48882, "Rec": 48883, "Ġspool": 48884, "аÑĤов": 48885, "ĠEnemy": 48886, "Ġfury": 48887, "Ġdetta": 48888, "ĠFay": 48889, "éļ¨": 48890, "ÑıÑİÑĤ": 48891, "Ġaproximadamente": 48892, "Ġsilos": 48893, "Ġmagist": 48894, "Ġcree": 48895, "ĠKrank": 48896, "ĠDOWN": 48897, "Ġstartled": 48898, "Ġreborn": 48899, "ĠUmwelt": 48900, "ĠSuzanne": 48901, "ниÑĨÑĭ": 48902, "outez": 48903, "ĠJAC": 48904, "yards": 48905, "radas": 48906, "rau": 48907, "ipts": 48908, "hail": 48909, "Ġparagraphs": 48910, "Ġmeglio": 48911, "Ġisolating": 48912, "Ġaceite": 48913, "ĠHarsh": 48914, "Ġcyst": 48915, "ĠBlockchain": 48916, "ĠÑħоÑĢоÑĪий": 48917, "Ġvirtuous": 48918, "Ġinvestigación": 48919, "Ġdevoir": 48920, "Ġmasturb": 48921, "ĠSale": 48922, "ÙĬرة": 48923, "ĠΧ": 48924, "ĠStraÃŁen": 48925, "Ġdikk": 48926, "Ġafore": 48927, "ĠJungkook": 48928, "Ġchociaż": 48929, "ĠDebatte": 48930, "Ġweirdly": 48931, "Ġviaje": 48932, "regist": 48933, "Help": 48934, "Ġkinderen": 48935, "Ġformulated": 48936, "Ġenfim": 48937, "ĠTowards": 48938, "коÑĹ": 48939, "ivering": 48940, "ĠдеÑĤи": 48941, "charger": 48942, "Ġpurl": 48943, "Ġacademically": 48944, "ĠNurse": 48945, "Ġdeleting": 48946, "ayo": 48947, "Ġrefusal": 48948, "Ġdepicts": 48949, "ĠDracula": 48950, "Ġtoasted": 48951, "ĠZombie": 48952, "ĠSuperior": 48953, "ĠBold": 48954, "Ġquizzes": 48955, "Ġgle": 48956, "450": 48957, "Ġcomeço": 48958, "ynn": 48959, "Ġverst": 48960, "ĠOlaf": 48961, "Ġpomoc": 48962, "ĠSask": 48963, "ëĺ": 48964, "ĠTCP": 48965, "ĠProperty": 48966, "íķĺì£ł": 48967, "à¸ľà¸¡": 48968, "boom": 48969, "aros": 48970, "ĠÑĢоÑģÑģий": 48971, "ĠбÑĭваеÑĤ": 48972, "åĩºåİ»": 48973, "ĠìĿ´ìķ¼ê¸°ë¥¼": 48974, "Ġcombien": 48975, "vacc": 48976, "Ġebenfalls": 48977, "para": 48978, "Ġзм": 48979, "Ġdesperation": 48980, "ordre": 48981, "Ġש׾×Ļ": 48982, "Ġgenerously": 48983, "ĠÐŀк": 48984, "Ġorbiting": 48985, "></": 48986, "ĠespÃŃ": 48987, "ĠCOP": 48988, "åŃ©åŃIJ": 48989, "visible": 48990, "ĠпÑĢеÑģÑĤÑĥп": 48991, "Ġstitched": 48992, "à¯Ī.": 48993, "Ġlatent": 48994, "ĠPrab": 48995, "ĠMcN": 48996, "ĠHealing": 48997, "ĠCuriosity": 48998, "cert": 48999, "Ġ민주": 49000, "Ġpatiently": 49001, "ĠYT": 49002, "foreign": 49003, "Ġvẫn": 49004, "Ġindustri": 49005, "Ġcocktails": 49006, "Ġbrighten": 49007, "Ġconsolidated": 49008, "аÑĢд": 49009, "ltry": 49010, "Ġgrille": 49011, "Ġbona": 49012, "Ġdiligently": 49013, "ĠWrestleMania": 49014, "erkt": 49015, "energy": 49016, "999": 49017, "à®ķவ": 49018, "Ġtote": 49019, "iono": 49020, "DIO": 49021, "Ġschizophrenia": 49022, "Ġpostponed": 49023, "ĠQiu": 49024, "ĠÏĥÏħν": 49025, "ĠzdjÄĻ": 49026, "Ġspannend": 49027, "ĠDIS": 49028, "Rel": 49029, "Ġrhin": 49030, "immune": 49031, "Old": 49032, "Ġplötzlich": 49033, "Ġmound": 49034, "Ġastronomical": 49035, "ĠGuid": 49036, "ĠCul": 49037, "HI": 49038, "ĠÅł": 49039, "Ġrepo": 49040, "ĠMaurice": 49041, "ä¸ĢçĤ¹": 49042, "Ġbandits": 49043, "ĠDesktop": 49044, "äss": 49045, "fta": 49046, "Ġlicence": 49047, "Ġimaginar": 49048, "ĠEntreprene": 49049, "xo": 49050, "Ġë§ĽìŀĪëĬĶ": 49051, "Ġ×Ķ×ij": 49052, "Ġpumpkins": 49053, "Ġkanssa": 49054, "ĠjÄĻzy": 49055, "Ġcommunauté": 49056, "bür": 49057, "Ġerhö": 49058, "ĠWolver": 49059, "ĠSharing": 49060, "令": 49061, "Ġpakai": 49062, "Ġinsulted": 49063, "ÐľÑĭ": 49064, "оÑĹ": 49065, "Ġconsiste": 49066, "æĮij": 49067, "Ġyoungsters": 49068, "Ġgleichen": 49069, "weder": 49070, "Ġmote": 49071, "Ġclauses": 49072, "état": 49073, "prus": 49074, "Ġwast": 49075, "ç»ĻæĪij": 49076, "ĠCrisp": 49077, "ĠçĦ¶å¾Į": 49078, "Ġoffenders": 49079, "Ġconvection": 49080, "Ġconfian": 49081, "ollow": 49082, "amet": 49083, "ĠÑĹÑħ": 49084, "第äºĮåĢĭ": 49085, "fficiency": 49086, "Ġunglaub": 49087, "igans": 49088, "Ġmarketed": 49089, "ĠVAN": 49090, "Ġproclaimed": 49091, "Ġcélulas": 49092, "Ġcollide": 49093, "ĠOculus": 49094, "adore": 49095, "Ji": 49096, "Ġsustaining": 49097, "ĠFasc": 49098, "Ġsetzt": 49099, "Ġnosaltres": 49100, "Most": 49101, "ĠвÑĩ": 49102, "Ġnauc": 49103, "ĠBhar": 49104, "çΏçΏ": 49105, "æĪijè·Łä½łè¬Ľ": 49106, "Ġyêu": 49107, "Ġtimest": 49108, "Ġpertama": 49109, "irmi": 49110, "Ġzwr": 49111, "Ġverbess": 49112, "Ġvortex": 49113, "ĠSTACK": 49114, "ثر": 49115, "¹Ħë": 49116, "ĶĶìĺ¤": 49117, "Ġlinkage": 49118, "ĠFraser": 49119, "enario": 49120, "ĠëĿ¼ëĬĶ": 49121, "ĠìĦłë°°": 49122, "hthal": 49123, "Ġê¹Į": 49124, "ĠKhông": 49125, "Ãĥ": 49126, "Ġscrambled": 49127, "ĠEink": 49128, "Ġmicroorgan": 49129, "Ġnarcissist": 49130, "ĠKombat": 49131, "Ġë§¡": 49132, "ĠAGA": 49133, "Ġperfekt": 49134, "ĠSerie": 49135, "determ": 49136, "-'": 49137, "Ġponytail": 49138, "Ġkoska": 49139, "ìĵ": 49140, "Ġobec": 49141, "Ġchests": 49142, "veer": 49143, "Ġuprising": 49144, "Ġstoked": 49145, "associ": 49146, "Ġprodução": 49147, "ĠShape": 49148, "ìłľê°Ģ": 49149, "ĠëͰ": 49150, "Ġjon": 49151, "Ġinadvert": 49152, "antas": 49153, "ĠнаконеÑĨ": 49154, "Ġå°įåķĬ": 49155, "ĠArsenal": 49156, "Ġproteg": 49157, "Ġliberté": 49158, "Ġglare": 49159, "åĪļ": 49160, "å·²ç»ı": 49161, "Ġverein": 49162, "Ġinserts": 49163, "ĠJana": 49164, "Ġwydaje": 49165, "ÅĤum": 49166, "Ġ%.": 49167, "origine": 49168, "Ġsynagogue": 49169, "Ġfallait": 49170, "Ġdisobed": 49171, "Ġantic": 49172, "ĠCycl": 49173, "Ġasynchronous": 49174, "Ġë²Įìį¨": 49175, "Ġgesund": 49176, "Ġgagn": 49177, "Ġpea": 49178, "Ġgrin": 49179, "ést": 49180, "Ġsauc": 49181, "ĠMäd": 49182, "íķ´ëıĦ": 49183, "pps": 49184, "ĠεÏĢι": 49185, "Ġpeuple": 49186, "Ġdeben": 49187, "ĠBree": 49188, "ĠÑĢолÑĮ": 49189, "Ġкаким": 49190, "Ġútil": 49191, "Ġdistributor": 49192, "алÑĭ": 49193, "ĠswojÄħ": 49194, "Ġfolklore": 49195, "Ġreceivers": 49196, "ĠMOO": 49197, "bins": 49198, "astre": 49199, "ìķĪë": 49200, "ĠëĦ£ê³ł": 49201, "Ġmultimedia": 49202, "Ġgebaut": 49203, "овÑĭÑħ": 49204, "ãy": 49205, "Ġdane": 49206, "okol": 49207, "emitism": 49208, "ONEY": 49209, "ĠyaÄŁ": 49210, "Ġchauff": 49211, "容æĺĵ": 49212, "Ġesfuer": 49213, "Äĥn": 49214, "ertas": 49215, "Ġfonctionne": 49216, "omina": 49217, "Ġivory": 49218, "ĠYoutuber": 49219, "ĠSkywalker": 49220, "иÑĩеÑģкаÑı": 49221, "toi": 49222, "Ġveya": 49223, "Ġgelernt": 49224, "Ġchancellor": 49225, "ĠStatistics": 49226, "Ġwelded": 49227, "Ġondan": 49228, "ĠSei": 49229, "Ġmedically": 49230, "Ġenergized": 49231, "ĠVia": 49232, "Ġвик": 49233, "Ġuninter": 49234, "Ġhighness": 49235, "ĠíĮĶë": 49236, "Ġamplified": 49237, "ĠSergey": 49238, "ĠMins": 49239, "warm": 49240, "pell": 49241, "ophile": 49242, "Ġhè": 49243, "ĠBelo": 49244, "ĠSketch": 49245, "Ġcharacterization": 49246, "ansen": 49247, "ĠÑĤÑĥÑĢ": 49248, "Ġãħĭãħĭãħĭ": 49249, "Note": 49250, "ĠkoÅŁ": 49251, "Ġciert": 49252, "flu": 49253, "Ġbaht": 49254, "ĠDowntown": 49255, "ĠCRIS": 49256, "odie": 49257, "140": 49258, "Ġlitres": 49259, "Ġgriev": 49260, "æ§ĺ": 49261, "Ġì͍ê°Ģ": 49262, "Ġsucceeds": 49263, "Ġ__": 49264, "enting": 49265, "Ġvimos": 49266, "Ġsì": 49267, "defense": 49268, "ĠMcD": 49269, "ĠMarion": 49270, "ĠDont": 49271, "ĠDDR": 49272, "ĠLazar": 49273, "ĠDAR": 49274, "Ġkuv": 49275, "Kn": 49276, "Ġsembla": 49277, "Ġairborne": 49278, "ĠViolence": 49279, "ëIJIJ": 49280, "Ġrestraint": 49281, "Ġwhistles": 49282, "Ġscolded": 49283, "Ġacceso": 49284, "Ġabsolutamente": 49285, "ĠTyl": 49286, "ĠSap": 49287, "¶Ģë¶Ħ": 49288, "itäten": 49289, "adem": 49290, "Ġý": 49291, "Ġprescribe": 49292, "ĠMage": 49293, "ĠHelena": 49294, "å¾Īæľī": 49295, "亲": 49296, "vt": 49297, "Ġvienen": 49298, "Ġsneez": 49299, "Ġmolé": 49300, "Æ°á»Łng": 49301, "Ġtransporting": 49302, "ĠLean": 49303, "Ġkung": 49304, "ÑĥÑĢа": 49305, "ÏĦÎŃ": 49306, "utches": 49307, "onders": 49308, "liyor": 49309, "Nat": 49310, "Ġzij": 49311, "Ġmammal": 49312, "Ġkäyt": 49313, "ĠJoanna": 49314, "sent": 49315, "Ġस": 49316, "Ġvested": 49317, "ĠErfahrung": 49318, "okee": 49319, "Ġclipping": 49320, "ĠListening": 49321, "Ġ(#": 49322, "fö": 49323, "Ġvidare": 49324, "Ġbrittle": 49325, "ĠSTART": 49326, "ĠDamas": 49327, "ĠYog": 49328, "ãĤĵãģ¨": 49329, "gart": 49330, "Ġverlier": 49331, "Ġheartfelt": 49332, "ĠdoÅĽÄĩ": 49333, "ì¹ĺê°Ģ": 49334, ".»": 49335, "Ġmaximal": 49336, "Ġdistintos": 49337, "ĠìĻľëĥIJíķĺë©´": 49338, "Ġsailed": 49339, "Ġconveyed": 49340, "ĠTinder": 49341, "ĠSUPER": 49342, "ниÑĨÑĥ": 49343, "controlled": 49344, "Ġfunz": 49345, "Ġbastards": 49346, "ĠGinsburg": 49347, "Ġnuovo": 49348, "ĠPere": 49349, "ĠJES": 49350, "ĠDingen": 49351, "ĠBets": 49352, "umba": 49353, "acción": 49354, "ĠìŀĪì§Ģë§Į": 49355, "Ġretra": 49356, "ĠLaurent": 49357, "Ġpozy": 49358, "Ġgrooves": 49359, "Ġmáquina": 49360, "Ġminion": 49361, "Ġdeinen": 49362, "ĠShaun": 49363, "×Ļ×Ļ": 49364, "Ġhonorary": 49365, "osaurus": 49366, "Ġzeit": 49367, "Ġespecie": 49368, "ĠBCE": 49369, "аÑĤе": 49370, "Justin": 49371, "ĠWheels": 49372, "ĠìĿ´íķ´": 49373, "ĠبÙĬÙĨ": 49374, "Ġpropulsion": 49375, "Ġperceber": 49376, "ĠNewman": 49377, "å´": 49378, "culosis": 49379, "Mi": 49380, "ĠаккÑĥ": 49381, "Ġmastering": 49382, "Ġläh": 49383, "Ġfists": 49384, "ä»Ķ": 49385, "Ġmarinade": 49386, "Lilly": 49387, "Ġëħ¸ëł¥": 49388, "ĠYH": 49389, "Ġurgently": 49390, "Ġinformational": 49391, "Ġacordo": 49392, "izzy": 49393, "ãģĦãģı": 49394, "ìĿ´ìĸ´": 49395, "imar": 49396, "ĠëĤĺìĺ¤ë": 49397, "Ġtwenties": 49398, "Ġrasp": 49399, "Ġbumpy": 49400, "بة": 49401, "worker": 49402, "Ġquickest": 49403, "Ġattaches": 49404, "виг": 49405, "ĠëĤĺíĥĢë": 49406, "Ġpuree": 49407, "Ġoversized": 49408, "Ġstirred": 49409, "Ġjakim": 49410, "Ġhomicide": 49411, "ãĤĤãģĹ": 49412, "iscilla": 49413, "Ġì±Ļ": 49414, "Ġspeculative": 49415, "Ġassists": 49416, "main": 49417, "jähr": 49418, "indet": 49419, "ĠÅŁur": 49420, "Ġforecasts": 49421, "Ġdiversion": 49422, "Ġtare": 49423, "Ġogl": 49424, "ĠOrganisation": 49425, "ĠChevy": 49426, "Ġbaja": 49427, "andır": 49428, "ĠÙĪÙĦا": 49429, "Ġradiant": 49430, "Ġliaison": 49431, "Ġdemokrat": 49432, "ĠMARC": 49433, "ÏĢοÏħ": 49434, "Ġrunt": 49435, "Ġprécis": 49436, "Ġgeven": 49437, "Ġvéhic": 49438, "ĠJESS": 49439, "STR": 49440, "Ġìĸĺë": 49441, "Ġvisionary": 49442, "Ġburadan": 49443, "ĠãģĤãĤĬ": 49444, "Ġrebirth": 49445, "Ġexhibited": 49446, "ĠMetall": 49447, "olie": 49448, "elyn": 49449, "Ġflavours": 49450, "Ġescrito": 49451, "ĠDelete": 49452, "ĠìķĮìķĺìĸ´": 49453, "ĠÑĥкÑĢаÑĹн": 49454, "Ġinterrupting": 49455, "Ġidentific": 49456, "ĠSuzuki": 49457, "ĠLanding": 49458, "ä»¶äºĭæĥħ": 49459, "andi": 49460, "Ġestran": 49461, "Ġcouleur": 49462, "Ġagrad": 49463, "ĠSny": 49464, "Ġà®ĩல": 49465, "Ġander": 49466, "Ġrua": 49467, "Ġprise": 49468, "Ġlaure": 49469, "ĠíĬĢ": 49470, "Ġmoderation": 49471, "Ġerfahren": 49472, "Ġdeconst": 49473, "ĠReese": 49474, "ĠPK": 49475, "etos": 49476, "ãģĵãĤĮãģ§": 49477, "ĠGravity": 49478, "ĠEren": 49479, "Ġoverboard": 49480, "Ġmüsst": 49481, "ĠEmail": 49482, "еÑĢм": 49483, "ydi": 49484, "iÄĻdzy": 49485, "ĠLOU": 49486, "ĠFuÃŁball": 49487, "ĠRD": 49488, "alts": 49489, "ĠìĬ¤íĬ¸ë": 49490, "ĠÐļÑĢаÑģ": 49491, "Ġtelev": 49492, "ĠÑĢо": 49493, "Ġresignation": 49494, "Ġjingle": 49495, "ĠStudien": 49496, "ĠIX": 49497, "ĠSentinel": 49498, "ĠPang": 49499, "éĦ": 49500, "Jake": 49501, "Ġpersonagem": 49502, "Ġmédia": 49503, "ĠChern": 49504, "antically": 49505, "Ġthá»Ŀi": 49506, "Ġparalysis": 49507, "Ġjapanese": 49508, "Ġconex": 49509, "Ġefic": 49510, "Ġunderside": 49511, "Ġneol": 49512, "Ġfian": 49513, "имоÑģÑĤÑĮ": 49514, "Ġquirky": 49515, "Ġpista": 49516, "ĠClement": 49517, "nothing": 49518, "ĠпоеÑħ": 49519, "Ġhorrend": 49520, "Ġconsolidate": 49521, "ploys": 49522, "emaker": 49523, "Jennifer": 49524, "Ġnuméro": 49525, "Ġfamoso": 49526, "ĠNeptune": 49527, "ĠíĸĪìĸ´": 49528, "ĠпÑĢезид": 49529, "Ġsitcom": 49530, "Ġserio": 49531, "Ġmue": 49532, "Ġglands": 49533, "Ġbörjar": 49534, "ĠYJ": 49535, "ĠRiot": 49536, "paragus": 49537, "Ġsegurança": 49538, "Ġimmature": 49539, "ĠMadonna": 49540, "à¸į": 49541, "Ġlingering": 49542, "Ġacesso": 49543, "ĠOrient": 49544, "ĠRecomm": 49545, "Ġcomplac": 49546, "founded": 49547, "attend": 49548, "Ġcielo": 49549, "ĠZhan": 49550, "naires": 49551, "cco": 49552, "Ġ×IJ׳": 49553, "Ġstata": 49554, "Ġcontradictory": 49555, "ĠSé": 49556, "ĠSAN": 49557, "ĠConnie": 49558, "Ġëĭ¹ìĭľ": 49559, "ĠÑģамой": 49560, "Ġmajestic": 49561, "ĠPenguin": 49562, "ĠCOME": 49563, "ÃŃcios": 49564, "pero": 49565, "Ġmg": 49566, "Ġfauc": 49567, "Ġcorrer": 49568, "ĠGottes": 49569, "ĠAnglo": 49570, "Har": 49571, "á»Ĺi": 49572, "Ġvitesse": 49573, "Ġannouncer": 49574, "ĠOmaha": 49575, "kum": 49576, "Ġspared": 49577, "ĠÑĢаза": 49578, "ĠполÑĥÑĩиÑĤÑģÑı": 49579, "Ġtähän": 49580, "Ġпонад": 49581, "Ġpertaining": 49582, "ĠRate": 49583, "iern": 49584, "Gold": 49585, "Ġteste": 49586, "ĠdeÄŁild": 49587, "Ġdamping": 49588, "ĠPartnership": 49589, "zysta": 49590, "geld": 49591, "Ġsmokes": 49592, "ĠMarriage": 49593, "쪽ìĹIJ": 49594, "èħ³": 49595, "isce": 49596, "Ġtryna": 49597, "ĠDirectory": 49598, "ĠëĤĺìĺ¬": 49599, "Ġshameful": 49600, "Ġmentre": 49601, "Ġassigning": 49602, "æĺ¯éĢĻæ¨£": 49603, "Ġrepertoire": 49604, "Ġobjetos": 49605, "稱": 49606, "Ġunderworld": 49607, "Ġendeavors": 49608, "Ġignite": 49609, "ĠÙĪØ¬": 49610, "Ġexperient": 49611, "ĠÐĹап": 49612, "ĠзаклÑİÑĩ": 49613, "Ġvoltages": 49614, "Ġniego": 49615, "Ġdeficits": 49616, "Ġbuenos": 49617, "ĠSleeping": 49618, "ĠSalem": 49619, "Ġunlocking": 49620, "Ġinteracted": 49621, "Ġentendeu": 49622, "ĠSuperintendent": 49623, "Ġszczegól": 49624, "Ġquas": 49625, "Ġpaling": 49626, "Ġkho": 49627, "بØŃ": 49628, "Ġcolabor": 49629, "ĠпÑĢигоÑĤов": 49630, "Ġmauv": 49631, "ĠJudas": 49632, "ĠAssist": 49633, "ĠÑĤеÑĢÑĢи": 49634, "ĠнаÑģколÑĮко": 49635, "Ġsubsidy": 49636, "ĠEmbassy": 49637, "Ġdagen": 49638, "ĠSanto": 49639, "èά": 49640, "ש×ķ×ij": 49641, "Ġabruptly": 49642, "ĠAdapt": 49643, "Ġvaak": 49644, "Ġpostal": 49645, "Ġinvestir": 49646, "Ġfiquei": 49647, "Ġdowntime": 49648, "ĠWebb": 49649, "ĠNCAA": 49650, "ĠEstoy": 49651, "олоÑĤ": 49652, "ĠìĤ¬ê±´": 49653, "Ġnationalist": 49654, "ĠKathryn": 49655, "ĠKop": 49656, "éª": 49657, "Sean": 49658, "ONA": 49659, "ĠBj": 49660, "×¢×Ŀ": 49661, "ÃŃb": 49662, "idamente": 49663, "Ġглаза": 49664, "Ġunnie": 49665, "Ġgemaakt": 49666, "ĠINTERVIEWER": 49667, "ĠHaut": 49668, "ίο": 49669, "geois": 49670, "wydd": 49671, "Ġколи": 49672, "Ġtightened": 49673, "Ġplanners": 49674, "Ġherum": 49675, "Ġgörün": 49676, "Ġelectronically": 49677, "Ġceram": 49678, "Ġëĭ¤ìĸijíķľ": 49679, "Ġepilepsy": 49680, "ĠeÄŁ": 49681, "lins": 49682, "ĠShiny": 49683, "æł¡": 49684, "ĠÑģолн": 49685, "Ġmacaron": 49686, "Ġimpacto": 49687, "ĠVegan": 49688, "zeÅĦ": 49689, "ĠRapha": 49690, "ĠPars": 49691, "ĠLEO": 49692, "ãģĬãģ£": 49693, "cü": 49694, "Ġ׾×Ķ×Ļ×ķת": 49695, "Ġähnlich": 49696, "Ġfloss": 49697, "ĠAZ": 49698, "Ġmöchten": 49699, "Ġgrooming": 49700, "Ġgrasses": 49701, "ranch": 49702, "Ġrecibir": 49703, "Ġbouncy": 49704, "ĠHobby": 49705, "Ġviktig": 49706, "Ġbegitu": 49707, "ĠPicasso": 49708, "ĠKush": 49709, "모": 49710, "Ġobstruction": 49711, "Ġë¶ĦìľĦ": 49712, "Ġmicrob": 49713, "ĠWestminster": 49714, "rops": 49715, "dul": 49716, "Ġdevo": 49717, "ĠLehrer": 49718, "ĠAdvisor": 49719, "ucken": 49720, "ĠбÑĥм": 49721, "Ġflattering": 49722, "ĠTruman": 49723, "ĠSempre": 49724, "ĠMcCain": 49725, "ĠHindus": 49726, "Julia": 49727, "Ġwatershed": 49728, "Ġlush": 49729, "ìłĦë": 49730, "Before": 49731, "ĠÐĴÑĤоÑĢ": 49732, "ĠSaaS": 49733, "Ġsitzt": 49734, "Ġbeetle": 49735, "ĠEssential": 49736, "enko": 49737, "ĠëķĮëıĦ": 49738, "Ġrevving": 49739, "Ġpoorer": 49740, "Ġcoerc": 49741, "Ġidee": 49742, "Ġcoû": 49743, "alet": 49744, "Ġzdrow": 49745, "Ġfender": 49746, "growth": 49747, "DING": 49748, "Ġzde": 49749, "ä¸ĬéĿ¢": 49750, "ENTS": 49751, "Ġfacets": 49752, "éļª": 49753, "ushima": 49754, "ĠÅŁeh": 49755, "Ġparasite": 49756, "Ġlapse": 49757, "ĠMeer": 49758, "ĠKund": 49759, "Ġslog": 49760, "Ġbrunch": 49761, "ĠChart": 49762, "arz": 49763, "ĠMUS": 49764, "Ġoffenses": 49765, "Ġinglés": 49766, "Ġfoliage": 49767, "oplan": 49768, "Aut": 49769, "ĠJacqu": 49770, "tak": 49771, "iembre": 49772, "Ġxen": 49773, "Ġnominees": 49774, "Ġbiomedical": 49775, "ésus": 49776, "Ġestuv": 49777, "ÏĦÏĮ": 49778, "ATHAN": 49779, "Ġíķľëį°": 49780, "Ġheed": 49781, "crosstalk": 49782, "Bill": 49783, "Ġspouses": 49784, "ĠÑģÑİж": 49785, "Ġverso": 49786, "ĠSven": 49787, "ĠCau": 49788, "cuz": 49789, "Ġë³´ìĦ¸ìļĶ": 49790, "ĠÑħозÑı": 49791, "Ġmocking": 49792, "ĠOna": 49793, "ĠDá": 49794, "Ġfruitful": 49795, "Ġbanquet": 49796, "udding": 49797, "inctions": 49798, "dert": 49799, "sud": 49800, "Ġdescon": 49801, "ĠJC": 49802, "Ġ§": 49803, "Ġpubli": 49804, "ëĪĪ": 49805, "éģķãģĨ": 49806, "Ġentschieden": 49807, "ĠROI": 49808, "ãģįãģŁ": 49809, "ĠìĥĿê²¼": 49810, "Ġkäytt": 49811, "yani": 49812, "shaw": 49813, "Ġunleash": 49814, "Ġmanne": 49815, "Ġhistogram": 49816, "æĬ¥": 49817, "à¸Ńะà¹Ħร": 49818, "Ġgn": 49819, "Ġfella": 49820, "Ġeinges": 49821, "ĠBuilt": 49822, "Ġrepresenta": 49823, "Ġpunishing": 49824, "Ġoutsiders": 49825, "нÑĥÑĤÑĮÑģÑı": 49826, "current": 49827, "Ġfamiliarity": 49828, "Ġдив": 49829, "Ġprojets": 49830, "Ġaqueles": 49831, "ĠGlue": 49832, "those": 49833, "Ġinception": 49834, "Ġaquellos": 49835, "Ġillusions": 49836, "Ġattends": 49837, "rese": 49838, "Ġswarm": 49839, "Ġswab": 49840, "Ġregardez": 49841, "Ġposição": 49842, "Ġakhir": 49843, "Ġextracting": 49844, "Ġanecdote": 49845, "ĠTale": 49846, "Ġвин": 49847, "Ġabges": 49848, "ĠoluÅŁ": 49849, "Ġcomplicado": 49850, "Ġcovari": 49851, "ÑĸÑĤÑĮ": 49852, "Der": 49853, "Ġ×Ļ×Ķ": 49854, "Form": 49855, "Ġìĸ´ì¨Įëĵł": 49856, "Ġreadable": 49857, "Ġinhibit": 49858, "Ġdecipher": 49859, "ĠAngry": 49860, "pg": 49861, "வத": 49862, "ĠÑģобÑģÑĤвенно": 49863, "Ġsamh": 49864, "Ġescr": 49865, "Ġencompasses": 49866, "Ġauster": 49867, "Ġconfisc": 49868, "ĠMandal": 49869, "Ġ}": 49870, "atcher": 49871, "=#": 49872, "çļĦæĹ¶åĢĻ": 49873, "Ġкино": 49874, "Ġstal": 49875, "lungs": 49876, "Ġvole": 49877, "Ġrequis": 49878, "ĠãĤĪ": 49879, "Ġpén": 49880, "Ġlecturer": 49881, "Ġinscription": 49882, "Ġcervical": 49883, "ĠTreasure": 49884, "ĠJW": 49885, "comings": 49886, "Ġeyesight": 49887, "ĠTails": 49888, "ÃŃsimo": 49889, "Ġworksheet": 49890, "Ġswiftly": 49891, "Ġconos": 49892, "Ġeliminates": 49893, "ĠBlaze": 49894, "алог": 49895, "Ġpictured": 49896, "Ġgiraffe": 49897, "ĠLogic": 49898, "åĺī": 49899, "Ġenrichment": 49900, "Fit": 49901, "Ġunintended": 49902, "Ġpersecuted": 49903, "akap": 49904, "ë°ĺ": 49905, "Ġbarber": 49906, "Ġarbeitet": 49907, "ĠSurprisingly": 49908, "ĠAutob": 49909, "unku": 49910, "prov": 49911, "ĠLoch": 49912, "obyl": 49913, "ĠподгоÑĤов": 49914, "Ġéconomique": 49915, "Ġpatt": 49916, "Ġceased": 49917, "ĠÑģпиÑģ": 49918, "Ġnuclei": 49919, "Ġiste": 49920, "ĠWag": 49921, "ĠzupeÅĤnie": 49922, "Ġproverb": 49923, "ĠAhÃŃ": 49924, "åĽŀåİ»": 49925, "liamo": 49926, "Ġreliably": 49927, "Ġpik": 49928, "ĠTrading": 49929, "ĠColeman": 49930, "Ġανα": 49931, "Ġmagari": 49932, "ĠPHIL": 49933, "Ġshedding": 49934, "ohner": 49935, "Ġpornography": 49936, "Ġbeneficiaries": 49937, "âĢ¢": 49938, "enin": 49939, "Ġresolving": 49940, "ĠÑģпоÑĢÑĤ": 49941, "Ġбег": 49942, "Ġnectar": 49943, "ultura": 49944, "imsical": 49945, "ĮĢ를": 49946, "å¹´åīį": 49947, "ãģĹãĤĥ": 49948, "Ġvisão": 49949, "éģİä¾Ĩ": 49950, "ÿÿÿÿÿÿÿÿ": 49951, "attform": 49952, "Ġë§ŀëĬĶ": 49953, "Ġpilgrimage": 49954, "Ġmating": 49955, "ĠReaper": 49956, "ĠBref": 49957, "çĶŁæ´»": 49958, "Ġ×ij×ĵ": 49959, "Ġnovamente": 49960, "Ġgrilling": 49961, "ĠWireless": 49962, "ĠRomanian": 49963, "ÒĽ": 49964, "ìľłë": 49965, "hait": 49966, "ĠBora": 49967, "ARRY": 49968, "Ġhypotheses": 49969, "马": 49970, "ikut": 49971, "ĠìķĦë²Ħ": 49972, "ĠÑĸз": 49973, "Ġnationale": 49974, "تÙī": 49975, "üllt": 49976, "Ġéléments": 49977, "ĠWare": 49978, "Ġ(-": 49979, "алÑĮном": 49980, "Ġindict": 49981, "ĠStones": 49982, "ãģŁãĤģ": 49983, "explosion": 49984, "ĠëĥĦìĥĪ": 49985, "Ġfelic": 49986, "Ġjudiciary": 49987, "Ġincarnation": 49988, "Ġinning": 49989, "Ġformul": 49990, "Ġshipment": 49991, "Ġreindeer": 49992, "æĴŃ": 49993, "ĠознаÑĩ": 49994, "Ġenvol": 49995, "undy": 49996, "ĠзнаÑĤÑĮ": 49997, "Ġвидели": 49998, "Ġexcluding": 49999, "death": 50000, "Ġberm": 50001, "Ġsoprattutto": 50002, "Ġdebido": 50003, "ĠZig": 50004, "ĠOv": 50005, "ĠKEVIN": 50006, "ĠPale": 50007, "ĠMire": 50008, "Ġandar": 50009, "including": 50010, "Ġswapped": 50011, "Ġmisconceptions": 50012, "Ġspong": 50013, "réal": 50014, "Ġorbitals": 50015, "Ġhashtags": 50016, "orit": 50017, "Ġmauvais": 50018, "иÑģа": 50019, "Ġlivres": 50020, "ĠIPS": 50021, "Ġ04": 50022, "ög": 50023, "instr": 50024, "ĠвнеÑĪ": 50025, "Ġhice": 50026, "isée": 50027, "Ġowes": 50028, "Ġesimerk": 50029, "ĠUH": 50030, "Ġirritation": 50031, "Ġgiggles": 50032, "Ġcolonialism": 50033, "ĠBliss": 50034, "strings": 50035, "Ġreunited": 50036, "ĠPsaki": 50037, "wach": 50038, "Ġcliffs": 50039, "ĠFalse": 50040, "äg": 50041, "pipe": 50042, "Ġwhopping": 50043, "Ġmeringue": 50044, "Ġbung": 50045, "industrie": 50046, "Ġleche": 50047, "ĠLoy": 50048, "Ġdrie": 50049, "Ġpassat": 50050, "Ġoleh": 50051, "Ġcéu": 50052, "ĠGabrie": 50053, "Ġreefs": 50054, "Ġbombers": 50055, "Ġepisódio": 50056, "ĠRug": 50057, "ĠProse": 50058, "onos": 50059, "Ġobese": 50060, "Ġgoog": 50061, "Ġpiace": 50062, "flanzen": 50063, "éĴŁ": 50064, "Ġflaps": 50065, "ĠAlto": 50066, "é£Łãģ¹": 50067, "Fin": 50068, "Ġresize": 50069, "ê·¸ëŀ¨": 50070, "è²»": 50071, "Nathan": 50072, "ŀĪ볤": 50073, "ĠÑĤай": 50074, "ĠNFT": 50075, "Ġsneeze": 50076, "Ġshroud": 50077, "ié": 50078, "Ġveramente": 50079, "Ġcascade": 50080, "ĠOok": 50081, "ìĹĨìĿ´": 50082, "Ġinfused": 50083, "fps": 50084, "center": 50085, "Ġgrappling": 50086, "ĠWohnung": 50087, "ĠTumb": 50088, "ĠImma": 50089, "ĠDuygusal": 50090, "енÑĤи": 50091, "Ġstewardship": 50092, "Ġharp": 50093, "Ġendorsed": 50094, "ılan": 50095, "Ġодним": 50096, "Ġcompetency": 50097, "Ġbert": 50098, "ĠTales": 50099, "Ġrhe": 50100, "Ġohh": 50101, "Ġê°Ħëĭ¨": 50102, "ĠmRNA": 50103, "Ġgangster": 50104, "ĠRunner": 50105, "еннÑĭм": 50106, "phoria": 50107, "ĠwÅĤaÅĽciwie": 50108, "Ġquarto": 50109, "Ġorganise": 50110, "ĠVet": 50111, "Pad": 50112, "ĠÙħØ«": 50113, "Ġstinks": 50114, "ĠDul": 50115, "uem": 50116, "isiej": 50117, "Top": 50118, "Ġtussen": 50119, "ĠEfendimiz": 50120, "ĠBoule": 50121, "ĠSloven": 50122, "ĠLö": 50123, "Ñijз": 50124, "ÑĢип": 50125, "cave": 50126, "Ġboî": 50127, "Ġapologise": 50128, "ĠMarly": 50129, "ĠExport": 50130, "ĠCaitlin": 50131, "Ġtavalla": 50132, "Ġentails": 50133, "Ġbrom": 50134, "ĠCopenh": 50135, "Ġwalnut": 50136, "Ġinsists": 50137, "Ġcuá»Ļc": 50138, "ĠQuit": 50139, "ĠDevice": 50140, "×Ĵ×Ŀ": 50141, "ĠDOT": 50142, "Ġvelocidad": 50143, "LIE": 50144, "Cool": 50145, "Ġsanitation": 50146, "Ġolho": 50147, "ĠEB": 50148, "ĠíĻķìĭ¤íŀĪ": 50149, "ĠÐľÐ¸Ñħ": 50150, "Ġzuk": 50151, "Ġsurname": 50152, "ĠSchuld": 50153, "ruff": 50154, "cultural": 50155, "ĠÑģÑĤолÑĮко": 50156, "æĻļä¸Ĭ": 50157, "Įëį°": 50158, "Ġtorto": 50159, "Ġbackups": 50160, "ÑĢий": 50161, "relax": 50162, "Ġsynergy": 50163, "Ġbuffs": 50164, "Ġapo": 50165, "ĠWellness": 50166, "rounded": 50167, "Ġuniverses": 50168, "Ġfera": 50169, "Ġstandby": 50170, "ĠSilva": 50171, "ĠJI": 50172, "ensored": 50173, "ĠìĹĨëĭ¤": 50174, "ĠÐIJв": 50175, "ĠоÑĤдел": 50176, "Ġfø": 50177, "ĠRockef": 50178, "ĠCompass": 50179, "ĠBears": 50180, "Ġä¸įè¦ģ": 50181, "Turn": 50182, "Ġthá»±c": 50183, "Ġpossibile": 50184, "Ġestem": 50185, "ĠCroatia": 50186, "Ġtätä": 50187, "ĠCAL": 50188, "à¹Ģà¸ŀ": 50189, "ĠÑģÑĤÑĢаÑħ": 50190, "Ġsalts": 50191, "Ġminimalist": 50192, "Ġincorporates": 50193, "ĠÙĨÛģÛĮÚº": 50194, "acao": 50195, "Ġslammed": 50196, "Ġcama": 50197, "Text": 50198, "!!!!!!": 50199, "Ġalcanz": 50200, "éma": 50201, "Ġincense": 50202, "Ġharden": 50203, "Ġgranting": 50204, "ĠNai": 50205, "ĠFirma": 50206, "Ġhypoc": 50207, "job": 50208, "ĠRH": 50209, "zur": 50210, "илÑı": 50211, "Ġź": 50212, "Ġdares": 50213, "anh": 50214, "Ġë§Įíģ¼": 50215, "Ġcuestión": 50216, "ĠLima": 50217, "æĻ¯": 50218, "Ġassunto": 50219, "ĠIPO": 50220, "ĠBengal": 50221, "ĠBier": 50222, "Ġpsyche": 50223, "Ġacquainted": 50224, "ĠGün": 50225, "ози": 50226, "ÅĽciÄħ": 50227, "AG": 50228, "Ġmalfunction": 50229, "Ġasteroids": 50230, "irez": 50231, "amorph": 50232, "ĠÑģоÑĤÑĢÑĥд": 50233, "Ġfreshwater": 50234, "Ġarran": 50235, "ĠпÑĢÑĭ": 50236, "ног": 50237, "Ġdiabetic": 50238, "ĠÙĤاÙĦ": 50239, "Ġoppress": 50240, "Ġcapacitance": 50241, "performance": 50242, "crates": 50243, "Ġapostle": 50244, "ĠJEN": 50245, "OULD": 50246, "Intro": 50247, "Ġstalls": 50248, "ĠABOUT": 50249, "cticamente": 50250, "Ġdiligent": 50251, "Ġmanifests": 50252, "ĠPakistani": 50253, "Ġ('": 50254, "åľº": 50255, "": 50256} \ No newline at end of file diff --git a/whisper/audio.py b/whisper/audio.py new file mode 100644 index 0000000000000000000000000000000000000000..de8a1951408b8bf08639f17f01b3fa764a82d83b --- /dev/null +++ b/whisper/audio.py @@ -0,0 +1,124 @@ +import os +from functools import lru_cache +from typing import Union + +import ffmpeg +import numpy as np +import torch +import torch.nn.functional as F + +from .utils import exact_div + +# hard-coded audio hyperparameters +SAMPLE_RATE = 16000 +N_FFT = 400 +N_MELS = 80 +HOP_LENGTH = 160 +CHUNK_LENGTH = 30 +N_SAMPLES = CHUNK_LENGTH * SAMPLE_RATE # 480000: number of samples in a chunk +N_FRAMES = exact_div(N_SAMPLES, HOP_LENGTH) # 3000: number of frames in a mel spectrogram input + + +def load_audio(file: str, sr: int = SAMPLE_RATE): + """ + Open an audio file and read as mono waveform, resampling as necessary + + Parameters + ---------- + file: str + The audio file to open + + sr: int + The sample rate to resample the audio if necessary + + Returns + ------- + A NumPy array containing the audio waveform, in float32 dtype. + """ + try: + # This launches a subprocess to decode audio while down-mixing and resampling as necessary. + # Requires the ffmpeg CLI and `ffmpeg-python` package to be installed. + out, _ = ( + ffmpeg.input(file, threads=0) + .output("-", format="s16le", acodec="pcm_s16le", ac=1, ar=sr) + .run(cmd=["ffmpeg", "-nostdin"], capture_stdout=True, capture_stderr=True) + ) + except ffmpeg.Error as e: + raise RuntimeError(f"Failed to load audio: {e.stderr.decode()}") from e + + return np.frombuffer(out, np.int16).flatten().astype(np.float32) / 32768.0 + + +def pad_or_trim(array, length: int = N_SAMPLES, *, axis: int = -1): + """ + Pad or trim the audio array to N_SAMPLES, as expected by the encoder. + """ + if torch.is_tensor(array): + if array.shape[axis] > length: + array = array.index_select(dim=axis, index=torch.arange(length, device=array.device)) + + if array.shape[axis] < length: + pad_widths = [(0, 0)] * array.ndim + pad_widths[axis] = (0, length - array.shape[axis]) + array = F.pad(array, [pad for sizes in pad_widths[::-1] for pad in sizes]) + else: + if array.shape[axis] > length: + array = array.take(indices=range(length), axis=axis) + + if array.shape[axis] < length: + pad_widths = [(0, 0)] * array.ndim + pad_widths[axis] = (0, length - array.shape[axis]) + array = np.pad(array, pad_widths) + + return array + + +@lru_cache(maxsize=None) +def mel_filters(device, n_mels: int = N_MELS) -> torch.Tensor: + """ + load the mel filterbank matrix for projecting STFT into a Mel spectrogram. + Allows decoupling librosa dependency; saved using: + + np.savez_compressed( + "mel_filters.npz", + mel_80=librosa.filters.mel(sr=16000, n_fft=400, n_mels=80), + ) + """ + assert n_mels == 80, f"Unsupported n_mels: {n_mels}" + with np.load(os.path.join(os.path.dirname(__file__), "assets", "mel_filters.npz")) as f: + return torch.from_numpy(f[f"mel_{n_mels}"]).to(device) + + +def log_mel_spectrogram(audio: Union[str, np.ndarray, torch.Tensor], n_mels: int = N_MELS): + """ + Compute the log-Mel spectrogram of + + Parameters + ---------- + audio: Union[str, np.ndarray, torch.Tensor], shape = (*) + The path to audio or either a NumPy array or Tensor containing the audio waveform in 16 kHz + + n_mels: int + The number of Mel-frequency filters, only 80 is supported + + Returns + ------- + torch.Tensor, shape = (80, n_frames) + A Tensor that contains the Mel spectrogram + """ + if not torch.is_tensor(audio): + if isinstance(audio, str): + audio = load_audio(audio) + audio = torch.from_numpy(audio) + + window = torch.hann_window(N_FFT).to(audio.device) + stft = torch.stft(audio, N_FFT, HOP_LENGTH, window=window, return_complex=True) + magnitudes = stft[..., :-1].abs() ** 2 + + filters = mel_filters(audio.device, n_mels) + mel_spec = filters @ magnitudes + + log_spec = torch.clamp(mel_spec, min=1e-10).log10() + log_spec = torch.maximum(log_spec, log_spec.max() - 8.0) + log_spec = (log_spec + 4.0) / 4.0 + return log_spec diff --git a/whisper/decoding.py b/whisper/decoding.py new file mode 100644 index 0000000000000000000000000000000000000000..603546d4c9ff67514d2567576935b974fe373bef --- /dev/null +++ b/whisper/decoding.py @@ -0,0 +1,712 @@ +from dataclasses import dataclass, field +from typing import Dict, List, Tuple, Iterable, Optional, Sequence, Union, TYPE_CHECKING + +import numpy as np +import torch +import torch.nn.functional as F +from torch import Tensor +from torch.distributions import Categorical + +from .audio import CHUNK_LENGTH +from .tokenizer import Tokenizer, get_tokenizer +from .utils import compression_ratio + +if TYPE_CHECKING: + from .model import Whisper + + +@torch.no_grad() +def detect_language(model: "Whisper", mel: Tensor, tokenizer: Tokenizer = None) -> Tuple[Tensor, List[dict]]: + """ + Detect the spoken language in the audio, and return them as list of strings, along with the ids + of the most probable language tokens and the probability distribution over all language tokens. + This is performed outside the main decode loop in order to not interfere with kv-caching. + + Returns + ------- + language_tokens : Tensor, shape = (n_audio,) + ids of the most probable language tokens, which appears after the startoftranscript token. + language_probs : List[Dict[str, float]], length = n_audio + list of dictionaries containing the probability distribution over all languages. + """ + if tokenizer is None: + tokenizer = get_tokenizer(model.is_multilingual) + if tokenizer.language is None or tokenizer.language_token not in tokenizer.sot_sequence: + raise ValueError(f"This model doesn't have language tokens so it can't perform lang id") + + single = mel.ndim == 2 + if single: + mel = mel.unsqueeze(0) + + # skip encoder forward pass if already-encoded audio features were given + if mel.shape[-2:] != (model.dims.n_audio_ctx, model.dims.n_audio_state): + mel = model.encoder(mel) + + # forward pass using a single token, startoftranscript + n_audio = mel.shape[0] + x = torch.tensor([[tokenizer.sot]] * n_audio).to(mel.device) # [n_audio, 1] + logits = model.logits(x, mel)[:, 0] + + # collect detected languages; suppress all non-language tokens + mask = torch.ones(logits.shape[-1], dtype=torch.bool) + mask[list(tokenizer.all_language_tokens)] = False + logits[:, mask] = -np.inf + language_tokens = logits.argmax(dim=-1) + language_token_probs = logits.softmax(dim=-1).cpu() + language_probs = [ + { + c: language_token_probs[i, j].item() + for j, c in zip(tokenizer.all_language_tokens, tokenizer.all_language_codes) + } + for i in range(n_audio) + ] + + if single: + language_tokens = language_tokens[0] + language_probs = language_probs[0] + + return language_tokens, language_probs + + +@dataclass(frozen=True) +class DecodingOptions: + task: str = "transcribe" # whether to perform X->X "transcribe" or X->English "translate" + language: Optional[str] = None # language that the audio is in; uses detected language if None + + # sampling-related options + temperature: float = 0.0 + sample_len: Optional[int] = None # maximum number of tokens to sample + best_of: Optional[int] = None # number of independent samples to collect, when t > 0 + beam_size: Optional[int] = None # number of beams in beam search, when t == 0 + patience: Optional[float] = None # patience in beam search (https://arxiv.org/abs/2204.05424) + + # options for ranking generations (either beams or best-of-N samples) + length_penalty: Optional[float] = None # "alpha" in Google NMT, None defaults to length norm + + # prompt, prefix, and token suppression + prompt: Optional[Union[str, List[int]]] = None # text or tokens for the previous context + prefix: Optional[Union[str, List[int]]] = None # text or tokens to prefix the current context + suppress_blank: bool = True # this will suppress blank outputs + + # list of tokens ids (or comma-separated token ids) to suppress + # "-1" will suppress a set of symbols as defined in `tokenizer.non_speech_tokens()` + suppress_tokens: Optional[Union[str, Iterable[int]]] = "-1" + + # timestamp sampling options + without_timestamps: bool = False # use <|notimestamps|> to sample text tokens only + max_initial_timestamp: Optional[float] = 1.0 # the initial timestamp cannot be later than this + + # implementation details + fp16: bool = True # use fp16 for most of the calculation + + +@dataclass(frozen=True) +class DecodingResult: + audio_features: Tensor + language: str + language_probs: Optional[Dict[str, float]] = None + tokens: List[int] = field(default_factory=list) + text: str = "" + avg_logprob: float = np.nan + no_speech_prob: float = np.nan + temperature: float = np.nan + compression_ratio: float = np.nan + + +class Inference: + def logits(self, tokens: Tensor, audio_features: Tensor) -> Tensor: + """Perform a forward pass on the decoder and return per-token logits""" + raise NotImplementedError + + def rearrange_kv_cache(self, source_indices) -> None: + """Update the key-value cache according to the updated beams""" + raise NotImplementedError + + def cleanup_caching(self) -> None: + """Clean up any resources or hooks after decoding is finished""" + pass + + +class PyTorchInference(Inference): + def __init__(self, model: "Whisper", initial_token_length: int): + self.model: "Whisper" = model + self.initial_token_length = initial_token_length + self.kv_cache = {} + self.hooks = [] + + def logits(self, tokens: Tensor, audio_features: Tensor) -> Tensor: + if not self.kv_cache: + self.kv_cache, self.hooks = self.model.install_kv_cache_hooks() + + if tokens.shape[-1] > self.initial_token_length: + # only need to use the last token except in the first forward pass + tokens = tokens[:, -1:] + + return self.model.decoder(tokens, audio_features, kv_cache=self.kv_cache) + + def cleanup_caching(self): + for hook in self.hooks: + hook.remove() + + self.kv_cache = {} + self.hooks = [] + + def rearrange_kv_cache(self, source_indices): + for module, tensor in self.kv_cache.items(): + # update the key/value cache to contain the selected sequences + self.kv_cache[module] = tensor[source_indices].detach() + + +class SequenceRanker: + def rank(self, tokens: List[List[Tensor]], sum_logprobs: List[List[float]]) -> List[int]: + """ + Given a list of groups of samples and their cumulative log probabilities, + return the indices of the samples in each group to select as the final result + """ + raise NotImplementedError + + +class MaximumLikelihoodRanker(SequenceRanker): + """ + Select the sample with the highest log probabilities, penalized using either + a simple length normalization or Google NMT paper's length penalty + """ + + def __init__(self, length_penalty: Optional[float]): + self.length_penalty = length_penalty + + def rank(self, tokens: List[List[Tensor]], sum_logprobs: List[List[float]]): + def scores(logprobs, lengths): + result = [] + for logprob, length in zip(logprobs, lengths): + if self.length_penalty is None: + penalty = length + else: + # from the Google NMT paper + penalty = ((5 + length) / 6) ** self.length_penalty + result.append(logprob / penalty) + return result + + # get the sequence with the highest score + lengths = [[len(t) for t in s] for s in tokens] + return [np.argmax(scores(p, l)) for p, l in zip(sum_logprobs, lengths)] + + +class TokenDecoder: + def reset(self): + """Initialize any stateful variables for decoding a new sequence""" + + def update(self, tokens: Tensor, logits: Tensor, sum_logprobs: Tensor) -> Tuple[Tensor, bool]: + """Specify how to select the next token, based on the current trace and logits + + Parameters + ---------- + tokens : Tensor, shape = (n_batch, current_sequence_length) + all tokens in the context so far, including the prefix and sot_sequence tokens + + logits : Tensor, shape = (n_batch, vocab_size) + per-token logits of the probability distribution at the current step + + sum_logprobs : Tensor, shape = (n_batch) + cumulative log probabilities for each sequence + + Returns + ------- + tokens : Tensor, shape = (n_batch, current_sequence_length + 1) + the tokens, appended with the selected next token + + completed : bool + True if all sequences has reached the end of text + + """ + raise NotImplementedError + + def finalize( + self, tokens: Tensor, sum_logprobs: Tensor + ) -> Tuple[Sequence[Sequence[Tensor]], List[List[float]]]: + """Finalize search and return the final candidate sequences + + Parameters + ---------- + tokens : Tensor, shape = (n_audio, n_group, current_sequence_length) + all tokens in the context so far, including the prefix and sot_sequence + + sum_logprobs : Tensor, shape = (n_audio, n_group) + cumulative log probabilities for each sequence + + Returns + ------- + tokens : Sequence[Sequence[Tensor]], length = n_audio + sequence of Tensors containing candidate token sequences, for each audio input + + sum_logprobs : List[List[float]], length = n_audio + sequence of cumulative log probabilities corresponding to the above + + """ + raise NotImplementedError + + +class GreedyDecoder(TokenDecoder): + def __init__(self, temperature: float, eot: int): + self.temperature = temperature + self.eot = eot + + def update(self, tokens: Tensor, logits: Tensor, sum_logprobs: Tensor) -> Tuple[Tensor, bool]: + temperature = self.temperature + if temperature == 0: + next_tokens = logits.argmax(dim=-1) + else: + next_tokens = Categorical(logits=logits / temperature).sample() + + logprobs = F.log_softmax(logits.float(), dim=-1) + current_logprobs = logprobs[torch.arange(logprobs.shape[0]), next_tokens] + sum_logprobs += current_logprobs * (tokens[:, -1] != self.eot) + + next_tokens[tokens[:, -1] == self.eot] = self.eot + tokens = torch.cat([tokens, next_tokens[:, None]], dim=-1) + + completed = (tokens[:, -1] == self.eot).all() + return tokens, completed + + def finalize(self, tokens: Tensor, sum_logprobs: Tensor): + # make sure each sequence has at least one EOT token at the end + tokens = F.pad(tokens, (0, 1), value=self.eot) + return tokens, sum_logprobs.tolist() + + +class BeamSearchDecoder(TokenDecoder): + def __init__(self, beam_size: int, eot: int, inference: Inference, patience: Optional[float] = None): + self.beam_size = beam_size + self.eot = eot + self.inference = inference + self.patience = patience or 1.0 + self.max_candidates: int = round(beam_size * self.patience) + self.finished_sequences = None + + assert self.max_candidates > 0, f"Invalid beam size ({beam_size}) or patience ({patience})" + + def reset(self): + self.finished_sequences = None + + def update(self, tokens: Tensor, logits: Tensor, sum_logprobs: Tensor) -> Tuple[Tensor, bool]: + if tokens.shape[0] % self.beam_size != 0: + raise ValueError(f"{tokens.shape}[0] % {self.beam_size} != 0") + + n_audio = tokens.shape[0] // self.beam_size + if self.finished_sequences is None: # for the first update + self.finished_sequences = [{} for _ in range(n_audio)] + + logprobs = F.log_softmax(logits.float(), dim=-1) + next_tokens, source_indices, finished_sequences = [], [], [] + for i in range(n_audio): + scores, sources, finished = {}, {}, {} + + # STEP 1: calculate the cumulative log probabilities for possible candidates + for j in range(self.beam_size): + idx = i * self.beam_size + j + prefix = tokens[idx].tolist() + for logprob, token in zip(*logprobs[idx].topk(self.beam_size + 1)): + new_logprob = (sum_logprobs[idx] + logprob).item() + sequence = tuple(prefix + [token.item()]) + scores[sequence] = new_logprob + sources[sequence] = idx + + # STEP 2: rank the candidates and keep the top beam_size sequences for each audio + saved = 0 + for sequence in sorted(scores, key=scores.get, reverse=True): + if sequence[-1] == self.eot: + finished[sequence] = scores[sequence] + else: + sum_logprobs[len(next_tokens)] = scores[sequence] + next_tokens.append(sequence) + source_indices.append(sources[sequence]) + + saved += 1 + if saved == self.beam_size: + break + + finished_sequences.append(finished) + + tokens = torch.tensor(next_tokens, device=tokens.device) + self.inference.rearrange_kv_cache(source_indices) + + # add newly finished sequences to self.finished_sequences + assert len(self.finished_sequences) == len(finished_sequences) + for previously_finished, newly_finished in zip(self.finished_sequences, finished_sequences): + for seq in sorted(newly_finished, key=newly_finished.get, reverse=True): + if len(previously_finished) >= self.max_candidates: + break # the candidate list is full + previously_finished[seq] = newly_finished[seq] + + # mark as completed if all audio has enough number of samples + completed = all( + len(sequences) >= self.max_candidates for sequences in self.finished_sequences + ) + return tokens, completed + + def finalize(self, preceding_tokens: Tensor, sum_logprobs: Tensor): + # collect all finished sequences, including patience, and add unfinished ones if not enough + sum_logprobs = sum_logprobs.cpu() + for i, sequences in enumerate(self.finished_sequences): + if len(sequences) < self.beam_size: # when not enough sequences are finished + for j in list(np.argsort(sum_logprobs[i]))[::-1]: + sequence = preceding_tokens[i, j].tolist() + [self.eot] + sequences[tuple(sequence)] = sum_logprobs[i][j].item() + if len(sequences) >= self.beam_size: + break + + tokens: List[List[Tensor]] = [ + [torch.tensor(seq) for seq in sequences.keys()] for sequences in self.finished_sequences + ] + sum_logprobs: List[List[float]] = [ + list(sequences.values()) for sequences in self.finished_sequences + ] + return tokens, sum_logprobs + + +class LogitFilter: + def apply(self, logits: Tensor, tokens: Tensor) -> None: + """Apply any filtering or masking to logits in-place + + Parameters + ---------- + logits : Tensor, shape = (n_batch, vocab_size) + per-token logits of the probability distribution at the current step + + tokens : Tensor, shape = (n_batch, current_sequence_length) + all tokens in the context so far, including the prefix and sot_sequence tokens + + """ + raise NotImplementedError + + +class SuppressBlank(LogitFilter): + def __init__(self, tokenizer: Tokenizer, sample_begin: int): + self.tokenizer = tokenizer + self.sample_begin = sample_begin + + def apply(self, logits: Tensor, tokens: Tensor): + if tokens.shape[1] == self.sample_begin: + logits[:, self.tokenizer.encode(" ") + [self.tokenizer.eot]] = -np.inf + + +class SuppressTokens(LogitFilter): + def __init__(self, suppress_tokens: Sequence[int]): + self.suppress_tokens = list(suppress_tokens) + + def apply(self, logits: Tensor, tokens: Tensor): + logits[:, self.suppress_tokens] = -np.inf + + +class ApplyTimestampRules(LogitFilter): + def __init__( + self, tokenizer: Tokenizer, sample_begin: int, max_initial_timestamp_index: Optional[int] + ): + self.tokenizer = tokenizer + self.sample_begin = sample_begin + self.max_initial_timestamp_index = max_initial_timestamp_index + + def apply(self, logits: Tensor, tokens: Tensor): + # suppress <|notimestamps|> which is handled by without_timestamps + if self.tokenizer.no_timestamps is not None: + logits[:, self.tokenizer.no_timestamps] = -np.inf + + # timestamps have to appear in pairs, except directly before EOT; mask logits accordingly + for k in range(tokens.shape[0]): + seq = [t for t in tokens[k, self.sample_begin :].tolist()] + last_was_timestamp = len(seq) >= 1 and seq[-1] >= self.tokenizer.timestamp_begin + penultimate_was_timestamp = len(seq) < 2 or seq[-2] >= self.tokenizer.timestamp_begin + + if last_was_timestamp: + if penultimate_was_timestamp: # has to be non-timestamp + logits[k, self.tokenizer.timestamp_begin :] = -np.inf + else: # cannot be normal text tokens + logits[k, : self.tokenizer.eot] = -np.inf + + if tokens.shape[1] == self.sample_begin: + # suppress generating non-timestamp tokens at the beginning + logits[:, : self.tokenizer.timestamp_begin] = -np.inf + + # apply the `max_initial_timestamp` option + if self.max_initial_timestamp_index is not None: + last_allowed = self.tokenizer.timestamp_begin + self.max_initial_timestamp_index + logits[:, last_allowed + 1 :] = -np.inf + + # if sum of probability over timestamps is above any other token, sample timestamp + logprobs = F.log_softmax(logits.float(), dim=-1) + for k in range(tokens.shape[0]): + timestamp_logprob = logprobs[k, self.tokenizer.timestamp_begin :].logsumexp(dim=-1) + max_text_token_logprob = logprobs[k, : self.tokenizer.timestamp_begin].max() + if timestamp_logprob > max_text_token_logprob: + logits[k, : self.tokenizer.timestamp_begin] = -np.inf + + +class DecodingTask: + inference: Inference + sequence_ranker: SequenceRanker + decoder: TokenDecoder + logit_filters: List[LogitFilter] + + def __init__(self, model: "Whisper", options: DecodingOptions): + self.model = model + + language = options.language or "en" + tokenizer = get_tokenizer(model.is_multilingual, language=language, task=options.task) + self.tokenizer: Tokenizer = tokenizer + self.options: DecodingOptions = self._verify_options(options) + + self.n_group: int = options.beam_size or options.best_of or 1 + self.n_ctx: int = model.dims.n_text_ctx + self.sample_len: int = options.sample_len or model.dims.n_text_ctx // 2 + + self.sot_sequence: Tuple[int] = tokenizer.sot_sequence + if self.options.without_timestamps: + self.sot_sequence = tokenizer.sot_sequence_including_notimestamps + + self.initial_tokens: Tuple[int] = self._get_initial_tokens() + self.sample_begin: int = len(self.initial_tokens) + self.sot_index: int = self.initial_tokens.index(tokenizer.sot) + + # inference: implements the forward pass through the decoder, including kv caching + self.inference = PyTorchInference(model, len(self.initial_tokens)) + + # sequence ranker: implements how to rank a group of sampled sequences + self.sequence_ranker = MaximumLikelihoodRanker(options.length_penalty) + + # decoder: implements how to select the next tokens, given the autoregressive distribution + if options.beam_size is not None: + self.decoder = BeamSearchDecoder( + options.beam_size, tokenizer.eot, self.inference, options.patience + ) + else: + self.decoder = GreedyDecoder(options.temperature, tokenizer.eot) + + # logit filters: applies various rules to suppress or penalize certain tokens + self.logit_filters = [] + if self.options.suppress_blank: + self.logit_filters.append(SuppressBlank(self.tokenizer, self.sample_begin)) + if self.options.suppress_tokens: + self.logit_filters.append(SuppressTokens(self._get_suppress_tokens())) + if not options.without_timestamps: + precision = CHUNK_LENGTH / model.dims.n_audio_ctx # usually 0.02 seconds + max_initial_timestamp_index = None + if options.max_initial_timestamp: + max_initial_timestamp_index = round(self.options.max_initial_timestamp / precision) + self.logit_filters.append( + ApplyTimestampRules(tokenizer, self.sample_begin, max_initial_timestamp_index) + ) + + def _verify_options(self, options: DecodingOptions) -> DecodingOptions: + if options.beam_size is not None and options.best_of is not None: + raise ValueError("beam_size and best_of can't be given together") + if options.temperature == 0: + if options.best_of is not None: + raise ValueError("best_of with greedy sampling (T=0) is not compatible") + if options.patience is not None and options.beam_size is None: + raise ValueError("patience requires beam_size to be given") + if options.length_penalty is not None and not (0 <= options.length_penalty <= 1): + raise ValueError("length_penalty (alpha) should be a value between 0 and 1") + + return options + + def _get_initial_tokens(self) -> Tuple[int]: + tokens = list(self.sot_sequence) + prefix = self.options.prefix + prompt = self.options.prompt + + if prefix: + prefix_tokens = ( + self.tokenizer.encode(" " + prefix.strip()) if isinstance(prefix, str) else prefix + ) + if self.sample_len is not None: + max_prefix_len = self.n_ctx // 2 - self.sample_len + prefix_tokens = prefix_tokens[-max_prefix_len:] + tokens = tokens + prefix_tokens + + if prompt: + prompt_tokens = ( + self.tokenizer.encode(" " + prompt.strip()) if isinstance(prompt, str) else prompt + ) + tokens = [self.tokenizer.sot_prev] + prompt_tokens[-(self.n_ctx // 2 - 1) :] + tokens + + return tuple(tokens) + + def _get_suppress_tokens(self) -> Tuple[int]: + suppress_tokens = self.options.suppress_tokens + + if isinstance(suppress_tokens, str): + suppress_tokens = [int(t) for t in suppress_tokens.split(",")] + + if -1 in suppress_tokens: + suppress_tokens = [t for t in suppress_tokens if t >= 0] + suppress_tokens.extend(self.tokenizer.non_speech_tokens) + elif suppress_tokens is None or len(suppress_tokens) == 0: + suppress_tokens = [] # interpret empty string as an empty list + else: + assert isinstance(suppress_tokens, list), "suppress_tokens must be a list" + + suppress_tokens.extend( + [self.tokenizer.sot, self.tokenizer.sot_prev, self.tokenizer.sot_lm] + ) + if self.tokenizer.no_speech is not None: + # no-speech probability is collected separately + suppress_tokens.append(self.tokenizer.no_speech) + + return tuple(sorted(set(suppress_tokens))) + + def _get_audio_features(self, mel: Tensor): + if self.options.fp16: + mel = mel.half() + + if mel.shape[-2:] == (self.model.dims.n_audio_ctx, self.model.dims.n_audio_state): + # encoded audio features are given; skip audio encoding + print("encoded audio features are given; skip audio encoding") + audio_features = mel + else: + print(mel.shape) + print("===============================") + audio_features = self.model.encoder(mel) + + if audio_features.dtype != (torch.float16 if self.options.fp16 else torch.float32): + return TypeError(f"audio_features has an incorrect dtype: {audio_features.dtype}") + + return audio_features + + def _detect_language(self, audio_features: Tensor, tokens: Tensor): + languages = [self.options.language] * audio_features.shape[0] + lang_probs = None + + if self.options.language is None or self.options.task == "lang_id": + lang_tokens, lang_probs = self.model.detect_language(audio_features, self.tokenizer) + languages = [max(probs, key=probs.get) for probs in lang_probs] + if self.options.language is None: + tokens[:, self.sot_index + 1] = lang_tokens # write language tokens + + return languages, lang_probs + + def _main_loop(self, audio_features: Tensor, tokens: Tensor): + assert audio_features.shape[0] == tokens.shape[0] + n_batch = tokens.shape[0] + sum_logprobs: Tensor = torch.zeros(n_batch, device=audio_features.device) + no_speech_probs = [np.nan] * n_batch + + try: + for i in range(self.sample_len): + logits = self.inference.logits(tokens, audio_features) + + if i == 0 and self.tokenizer.no_speech is not None: # save no_speech_probs + probs_at_sot = logits[:, self.sot_index].float().softmax(dim=-1) + no_speech_probs = probs_at_sot[:, self.tokenizer.no_speech].tolist() + + # now we need to consider the logits at the last token only + logits = logits[:, -1] + + # apply the logit filters, e.g. for suppressing or applying penalty to + for logit_filter in self.logit_filters: + logit_filter.apply(logits, tokens) + + # expand the tokens tensor with the selected next tokens + tokens, completed = self.decoder.update(tokens, logits, sum_logprobs) + + if completed or tokens.shape[-1] > self.n_ctx: + break + finally: + self.inference.cleanup_caching() + + return tokens, sum_logprobs, no_speech_probs + + @torch.no_grad() + def run(self, mel: Tensor) -> List[DecodingResult]: + self.decoder.reset() + tokenizer: Tokenizer = self.tokenizer + n_audio: int = mel.shape[0] + + audio_features: Tensor = self._get_audio_features(mel) # encoder forward pass + tokens: Tensor = torch.tensor([self.initial_tokens]).repeat(n_audio, 1) + + # detect language if requested, overwriting the language token + languages, language_probs = self._detect_language(audio_features, tokens) + if self.options.task == "lang_id": + return [ + DecodingResult(audio_features=features, language=language, language_probs=probs) + for features, language, probs in zip(audio_features, languages, language_probs) + ] + + # repeat the audio & text tensors by the group size, for beam search or best-of-n sampling + audio_features = audio_features.repeat_interleave(self.n_group, dim=0) + tokens = tokens.repeat_interleave(self.n_group, dim=0).to(audio_features.device) + + # call the main sampling loop + tokens, sum_logprobs, no_speech_probs = self._main_loop(audio_features, tokens) + + # reshape the tensors to have (n_audio, n_group) as the first two dimensions + audio_features = audio_features[:: self.n_group] + no_speech_probs = no_speech_probs[:: self.n_group] + assert audio_features.shape[0] == len(no_speech_probs) == n_audio + + tokens = tokens.reshape(n_audio, self.n_group, -1) + sum_logprobs = sum_logprobs.reshape(n_audio, self.n_group) + + # get the final candidates for each group, and slice between the first sampled token and EOT + tokens, sum_logprobs = self.decoder.finalize(tokens, sum_logprobs) + tokens: List[List[Tensor]] = [ + [t[self.sample_begin : (t == tokenizer.eot).nonzero()[0, 0]] for t in s] for s in tokens + ] + + # select the top-ranked sample in each group + selected = self.sequence_ranker.rank(tokens, sum_logprobs) + tokens: List[List[int]] = [t[i].tolist() for i, t in zip(selected, tokens)] + texts: List[str] = [tokenizer.decode(t).strip() for t in tokens] + + sum_logprobs: List[float] = [lp[i] for i, lp in zip(selected, sum_logprobs)] + avg_logprobs: List[float] = [lp / (len(t) + 1) for t, lp in zip(tokens, sum_logprobs)] + + fields = (texts, languages, tokens, audio_features, avg_logprobs, no_speech_probs) + if len(set(map(len, fields))) != 1: + raise RuntimeError(f"inconsistent result lengths: {list(map(len, fields))}") + + return [ + DecodingResult( + audio_features=features, + language=language, + tokens=tokens, + text=text, + avg_logprob=avg_logprob, + no_speech_prob=no_speech_prob, + temperature=self.options.temperature, + compression_ratio=compression_ratio(text), + ) + for text, language, tokens, features, avg_logprob, no_speech_prob in zip(*fields) + ] + + +@torch.no_grad() +def decode(model: "Whisper", mel: Tensor, options: DecodingOptions = DecodingOptions()) -> Union[DecodingResult, List[DecodingResult]]: + """ + Performs decoding of 30-second audio segment(s), provided as Mel spectrogram(s). + + Parameters + ---------- + model: Whisper + the Whisper model instance + + mel: torch.Tensor, shape = (80, 3000) or (*, 80, 3000) + A tensor containing the Mel spectrogram(s) + + options: DecodingOptions + A dataclass that contains all necessary options for decoding 30-second segments + + Returns + ------- + result: Union[DecodingResult, List[DecodingResult]] + The result(s) of decoding contained in `DecodingResult` dataclass instance(s) + """ + single = mel.ndim == 2 + if single: + mel = mel.unsqueeze(0) + result = DecodingTask(model, options).run(mel) + + if single: + result = result[0] + + return result diff --git a/whisper/inference.py b/whisper/inference.py new file mode 100644 index 0000000000000000000000000000000000000000..9de5f82a28a9278ec0c4ef31dda32eb65852cc4b --- /dev/null +++ b/whisper/inference.py @@ -0,0 +1,59 @@ +import os +import numpy as np +import argparse +import torch + +from whisper.model import Whisper, ModelDimensions +from whisper.audio import load_audio, pad_or_trim, log_mel_spectrogram + + +def load_model(path) -> Whisper: + device = "cuda" if torch.cuda.is_available() else "cpu" + checkpoint = torch.load(path, map_location=device) + dims = ModelDimensions(**checkpoint["dims"]) + model = Whisper(dims) + model.load_state_dict(checkpoint["model_state_dict"]) + return model.to(device) + + +def pred_ppg(whisper: Whisper, wavPath, ppgPath): + audio = load_audio(wavPath) + audln = audio.shape[0] + ppg_a = [] + idx_s = 0 + while (idx_s + 25 * 16000 < audln): + short = audio[idx_s:idx_s + 25 * 16000] + idx_s = idx_s + 25 * 16000 + ppgln = 25 * 16000 // 320 + # short = pad_or_trim(short) + mel = log_mel_spectrogram(short).to(whisper.device) + with torch.no_grad(): + ppg = whisper.encoder(mel.unsqueeze(0)).squeeze().data.cpu().float().numpy() + ppg = ppg[:ppgln,] # [length, dim=1024] + ppg_a.extend(ppg) + if (idx_s < audln): + short = audio[idx_s:audln] + ppgln = (audln - idx_s) // 320 + # short = pad_or_trim(short) + mel = log_mel_spectrogram(short).to(whisper.device) + with torch.no_grad(): + ppg = whisper.encoder(mel.unsqueeze(0)).squeeze().data.cpu().float().numpy() + ppg = ppg[:ppgln,] # [length, dim=1024] + ppg_a.extend(ppg) + np.save(ppgPath, ppg_a, allow_pickle=False) + + +if __name__ == "__main__": + parser = argparse.ArgumentParser() + parser.description = 'please enter embed parameter ...' + parser.add_argument("-w", "--wav", help="wav", dest="wav") + parser.add_argument("-p", "--ppg", help="ppg", dest="ppg") + args = parser.parse_args() + print(args.wav) + print(args.ppg) + + wavPath = args.wav + ppgPath = args.ppg + + whisper = load_model(os.path.join("whisper_pretrain", "medium.pt")) + pred_ppg(whisper, wavPath, ppgPath) diff --git a/whisper/model.py b/whisper/model.py new file mode 100644 index 0000000000000000000000000000000000000000..cb3781c17a1e78a33bf62246e5134e8512206d0d --- /dev/null +++ b/whisper/model.py @@ -0,0 +1,269 @@ +from dataclasses import dataclass +from typing import Dict +from typing import Iterable, Optional + +import numpy as np +import torch +import torch.nn.functional as F +from torch import Tensor +from torch import nn + +from .decoding import detect_language as detect_language_function, decode as decode_function + + +@dataclass +class ModelDimensions: + n_mels: int + n_audio_ctx: int + n_audio_state: int + n_audio_head: int + n_audio_layer: int + n_vocab: int + n_text_ctx: int + n_text_state: int + n_text_head: int + n_text_layer: int + + +class LayerNorm(nn.LayerNorm): + def forward(self, x: Tensor) -> Tensor: + return super().forward(x.float()).type(x.dtype) + + +class Linear(nn.Linear): + def forward(self, x: Tensor) -> Tensor: + return F.linear( + x, self.weight.to(x.dtype), None if self.bias is None else self.bias.to(x.dtype) + ) + + +class Conv1d(nn.Conv1d): + def _conv_forward(self, x: Tensor, weight: Tensor, bias: Optional[Tensor]) -> Tensor: + return super()._conv_forward( + x, weight.to(x.dtype), None if bias is None else bias.to(x.dtype) + ) + + +def sinusoids(length, channels, max_timescale=10000): + """Returns sinusoids for positional embedding""" + assert channels % 2 == 0 + log_timescale_increment = np.log(max_timescale) / (channels // 2 - 1) + inv_timescales = torch.exp(-log_timescale_increment * torch.arange(channels // 2)) + scaled_time = torch.arange(length)[:, np.newaxis] * inv_timescales[np.newaxis, :] + return torch.cat([torch.sin(scaled_time), torch.cos(scaled_time)], dim=1) + + +class MultiHeadAttention(nn.Module): + def __init__(self, n_state: int, n_head: int): + super().__init__() + self.n_head = n_head + self.query = Linear(n_state, n_state) + self.key = Linear(n_state, n_state, bias=False) + self.value = Linear(n_state, n_state) + self.out = Linear(n_state, n_state) + + def forward( + self, + x: Tensor, + xa: Optional[Tensor] = None, + mask: Optional[Tensor] = None, + kv_cache: Optional[dict] = None, + ): + q = self.query(x) + + if kv_cache is None or xa is None or self.key not in kv_cache: + # hooks, if installed (i.e. kv_cache is not None), will prepend the cached kv tensors; + # otherwise, perform key/value projections for self- or cross-attention as usual. + k = self.key(x if xa is None else xa) + v = self.value(x if xa is None else xa) + else: + # for cross-attention, calculate keys and values once and reuse in subsequent calls. + k = kv_cache[self.key] + v = kv_cache[self.value] + + wv, qk = self.qkv_attention(q, k, v, mask) + return self.out(wv), qk + + def qkv_attention(self, q: Tensor, k: Tensor, v: Tensor, mask: Optional[Tensor] = None): + n_batch, n_ctx, n_state = q.shape + scale = (n_state // self.n_head) ** -0.25 + q = q.view(*q.shape[:2], self.n_head, -1).permute(0, 2, 1, 3) * scale + k = k.view(*k.shape[:2], self.n_head, -1).permute(0, 2, 3, 1) * scale + v = v.view(*v.shape[:2], self.n_head, -1).permute(0, 2, 1, 3) + + qk = q @ k + if mask is not None: + qk = qk + mask[:n_ctx, :n_ctx] + qk = qk.float() + + w = F.softmax(qk, dim=-1).to(q.dtype) + return (w @ v).permute(0, 2, 1, 3).flatten(start_dim=2), qk.detach() + + +class ResidualAttentionBlock(nn.Module): + def __init__(self, n_state: int, n_head: int, cross_attention: bool = False): + super().__init__() + + self.attn = MultiHeadAttention(n_state, n_head) + self.attn_ln = LayerNorm(n_state) + + self.cross_attn = MultiHeadAttention(n_state, n_head) if cross_attention else None + self.cross_attn_ln = LayerNorm(n_state) if cross_attention else None + + n_mlp = n_state * 4 + self.mlp = nn.Sequential(Linear(n_state, n_mlp), nn.GELU(), Linear(n_mlp, n_state)) + self.mlp_ln = LayerNorm(n_state) + + def forward( + self, + x: Tensor, + xa: Optional[Tensor] = None, + mask: Optional[Tensor] = None, + kv_cache: Optional[dict] = None, + ): + x = x + self.attn(self.attn_ln(x), mask=mask, kv_cache=kv_cache)[0] + if self.cross_attn: + x = x + self.cross_attn(self.cross_attn_ln(x), xa, kv_cache=kv_cache)[0] + x = x + self.mlp(self.mlp_ln(x)) + return x + + +class AudioEncoder(nn.Module): + def __init__(self, n_mels: int, n_ctx: int, n_state: int, n_head: int, n_layer: int): + super().__init__() + self.conv1 = Conv1d(n_mels, n_state, kernel_size=3, padding=1) + self.conv2 = Conv1d(n_state, n_state, kernel_size=3, stride=2, padding=1) + self.register_buffer("positional_embedding", sinusoids(n_ctx, n_state)) + + self.blocks: Iterable[ResidualAttentionBlock] = nn.ModuleList( + [ResidualAttentionBlock(n_state, n_head) for _ in range(n_layer)] + ) + self.ln_post = LayerNorm(n_state) + + def forward(self, x: Tensor): + """ + x : torch.Tensor, shape = (batch_size, n_mels, n_ctx) + the mel spectrogram of the audio + """ + x = F.gelu(self.conv1(x)) + x = F.gelu(self.conv2(x)) + x = x.permute(0, 2, 1) + + len_x = x.shape[1] + len_e = self.positional_embedding.shape[0] + assert len_x <= len_e, "incorrect audio shape" + pos_e = self.positional_embedding[:len_x, :] + x = (x + pos_e).to(x.dtype) + + for block in self.blocks: + x = block(x) + + x = self.ln_post(x) + return x + + +class TextDecoder(nn.Module): + def __init__(self, n_vocab: int, n_ctx: int, n_state: int, n_head: int, n_layer: int): + super().__init__() + + self.token_embedding = nn.Embedding(n_vocab, n_state) + self.positional_embedding = nn.Parameter(torch.empty(n_ctx, n_state)) + + self.blocks: Iterable[ResidualAttentionBlock] = nn.ModuleList( + [ResidualAttentionBlock(n_state, n_head, cross_attention=True) for _ in range(n_layer)] + ) + self.ln = LayerNorm(n_state) + + mask = torch.empty(n_ctx, n_ctx).fill_(-np.inf).triu_(1) + self.register_buffer("mask", mask, persistent=False) + + def forward(self, x: Tensor, xa: Tensor, kv_cache: Optional[dict] = None): + """ + x : torch.LongTensor, shape = (batch_size, <= n_ctx) + the text tokens + xa : torch.Tensor, shape = (batch_size, n_mels, n_audio_ctx) + the encoded audio features to be attended on + """ + offset = next(iter(kv_cache.values())).shape[1] if kv_cache else 0 + x = self.token_embedding(x) + self.positional_embedding[offset : offset + x.shape[-1]] + x = x.to(xa.dtype) + + for block in self.blocks: + x = block(x, xa, mask=self.mask, kv_cache=kv_cache) + + x = self.ln(x) + logits = (x @ torch.transpose(self.token_embedding.weight.to(x.dtype), 0, 1)).float() + + return logits + + +class Whisper(nn.Module): + def __init__(self, dims: ModelDimensions): + super().__init__() + self.dims = dims + self.encoder = AudioEncoder( + self.dims.n_mels, + self.dims.n_audio_ctx, + self.dims.n_audio_state, + self.dims.n_audio_head, + self.dims.n_audio_layer, + ) + self.decoder = TextDecoder( + self.dims.n_vocab, + self.dims.n_text_ctx, + self.dims.n_text_state, + self.dims.n_text_head, + self.dims.n_text_layer, + ) + + def embed_audio(self, mel: torch.Tensor): + return self.encoder(mel) + + def logits(self, tokens: torch.Tensor, audio_features: torch.Tensor): + return self.decoder(tokens, audio_features) + + def forward(self, mel: torch.Tensor, tokens: torch.Tensor) -> Dict[str, torch.Tensor]: + return self.decoder(tokens, self.encoder(mel)) + + @property + def device(self): + return next(self.parameters()).device + + @property + def is_multilingual(self): + return self.dims.n_vocab == 51865 + + def install_kv_cache_hooks(self, cache: Optional[dict] = None): + """ + The `MultiHeadAttention` module optionally accepts `kv_cache` which stores the key and value + tensors calculated for the previous positions. This method returns a dictionary that stores + all caches, and the necessary hooks for the key and value projection modules that save the + intermediate tensors to be reused during later calculations. + + Returns + ------- + cache : Dict[nn.Module, torch.Tensor] + A dictionary object mapping the key/value projection modules to its cache + hooks : List[RemovableHandle] + List of PyTorch RemovableHandle objects to stop the hooks to be called + """ + cache = {**cache} if cache is not None else {} + hooks = [] + + def save_to_cache(module, _, output): + if module not in cache or output.shape[1] > self.decoder.positional_embedding.shape[0]: + cache[module] = output # save as-is, for the first token or cross attention + else: + cache[module] = torch.cat([cache[module], output], dim=1).detach() + return cache[module] + + def install_hooks(layer: nn.Module): + if isinstance(layer, MultiHeadAttention): + hooks.append(layer.key.register_forward_hook(save_to_cache)) + hooks.append(layer.value.register_forward_hook(save_to_cache)) + + self.decoder.apply(install_hooks) + return cache, hooks + + detect_language = detect_language_function + decode = decode_function diff --git a/whisper/normalizers/__init__.py b/whisper/normalizers/__init__.py new file mode 100644 index 0000000000000000000000000000000000000000..0b10d5a9d6389368fe7f3bac38e3b3cd9cc1470f --- /dev/null +++ b/whisper/normalizers/__init__.py @@ -0,0 +1,2 @@ +from .basic import BasicTextNormalizer +from .english import EnglishTextNormalizer diff --git a/whisper/normalizers/basic.py b/whisper/normalizers/basic.py new file mode 100644 index 0000000000000000000000000000000000000000..ef8d249bfca2cd10bccdd56950504581e2598560 --- /dev/null +++ b/whisper/normalizers/basic.py @@ -0,0 +1,71 @@ +import re +import unicodedata + +import regex + +# non-ASCII letters that are not separated by "NFKD" normalization +ADDITIONAL_DIACRITICS = { + "œ": "oe", + "Œ": "OE", + "ø": "o", + "Ø": "O", + "æ": "ae", + "Æ": "AE", + "ß": "ss", + "ẞ": "SS", + "đ": "d", + "Đ": "D", + "ð": "d", + "Ð": "D", + "þ": "th", + "Þ": "th", + "ł": "l", + "Ł": "L", +} + + +def remove_symbols_and_diacritics(s: str, keep=""): + """ + Replace any other markers, symbols, and punctuations with a space, + and drop any diacritics (category 'Mn' and some manual mappings) + """ + return "".join( + c + if c in keep + else ADDITIONAL_DIACRITICS[c] + if c in ADDITIONAL_DIACRITICS + else "" + if unicodedata.category(c) == "Mn" + else " " + if unicodedata.category(c)[0] in "MSP" + else c + for c in unicodedata.normalize("NFKD", s) + ) + + +def remove_symbols(s: str): + """ + Replace any other markers, symbols, punctuations with a space, keeping diacritics + """ + return "".join( + " " if unicodedata.category(c)[0] in "MSP" else c for c in unicodedata.normalize("NFKC", s) + ) + + +class BasicTextNormalizer: + def __init__(self, remove_diacritics: bool = False, split_letters: bool = False): + self.clean = remove_symbols_and_diacritics if remove_diacritics else remove_symbols + self.split_letters = split_letters + + def __call__(self, s: str): + s = s.lower() + s = re.sub(r"[<\[][^>\]]*[>\]]", "", s) # remove words between brackets + s = re.sub(r"\(([^)]+?)\)", "", s) # remove words between parenthesis + s = self.clean(s).lower() + + if self.split_letters: + s = " ".join(regex.findall(r"\X", s, regex.U)) + + s = re.sub(r"\s+", " ", s) # replace any successive whitespace characters with a space + + return s diff --git a/whisper/normalizers/english.json b/whisper/normalizers/english.json new file mode 100644 index 0000000000000000000000000000000000000000..74a1c3521d9af4a84a0aa65d95b0859138640799 --- /dev/null +++ b/whisper/normalizers/english.json @@ -0,0 +1,1741 @@ +{ + "accessorise": "accessorize", + "accessorised": "accessorized", + "accessorises": "accessorizes", + "accessorising": "accessorizing", + "acclimatisation": "acclimatization", + "acclimatise": "acclimatize", + "acclimatised": "acclimatized", + "acclimatises": "acclimatizes", + "acclimatising": "acclimatizing", + "accoutrements": "accouterments", + "aeon": "eon", + "aeons": "eons", + "aerogramme": "aerogram", + "aerogrammes": "aerograms", + "aeroplane": "airplane", + "aeroplanes": "airplanes", + "aesthete": "esthete", + "aesthetes": "esthetes", + "aesthetic": "esthetic", + "aesthetically": "esthetically", + "aesthetics": "esthetics", + "aetiology": "etiology", + "ageing": "aging", + "aggrandisement": "aggrandizement", + "agonise": "agonize", + "agonised": "agonized", + "agonises": "agonizes", + "agonising": "agonizing", + "agonisingly": "agonizingly", + "almanack": "almanac", + "almanacks": "almanacs", + "aluminium": "aluminum", + "amortisable": "amortizable", + "amortisation": "amortization", + "amortisations": "amortizations", + "amortise": "amortize", + "amortised": "amortized", + "amortises": "amortizes", + "amortising": "amortizing", + "amphitheatre": "amphitheater", + "amphitheatres": "amphitheaters", + "anaemia": "anemia", + "anaemic": "anemic", + "anaesthesia": "anesthesia", + "anaesthetic": "anesthetic", + "anaesthetics": "anesthetics", + "anaesthetise": "anesthetize", + "anaesthetised": "anesthetized", + "anaesthetises": "anesthetizes", + "anaesthetising": "anesthetizing", + "anaesthetist": "anesthetist", + "anaesthetists": "anesthetists", + "anaesthetize": "anesthetize", + "anaesthetized": "anesthetized", + "anaesthetizes": "anesthetizes", + "anaesthetizing": "anesthetizing", + "analogue": "analog", + "analogues": "analogs", + "analyse": "analyze", + "analysed": "analyzed", + "analyses": "analyzes", + "analysing": "analyzing", + "anglicise": "anglicize", + "anglicised": "anglicized", + "anglicises": "anglicizes", + "anglicising": "anglicizing", + "annualised": "annualized", + "antagonise": "antagonize", + "antagonised": "antagonized", + "antagonises": "antagonizes", + "antagonising": "antagonizing", + "apologise": "apologize", + "apologised": "apologized", + "apologises": "apologizes", + "apologising": "apologizing", + "appal": "appall", + "appals": "appalls", + "appetiser": "appetizer", + "appetisers": "appetizers", + "appetising": "appetizing", + "appetisingly": "appetizingly", + "arbour": "arbor", + "arbours": "arbors", + "archeological": "archaeological", + "archaeologically": "archeologically", + "archaeologist": "archeologist", + "archaeologists": "archeologists", + "archaeology": "archeology</span>", + "ardour": "ardor", + "armour": "armor", + "armoured": "armored", + "armourer": "armorer", + "armourers": "armorers", + "armouries": "armories", + "armoury": "armory", + "artefact": "artifact", + "artefacts": "artifacts", + "authorise": "authorize", + "authorised": "authorized", + "authorises": "authorizes", + "authorising": "authorizing", + "axe": "ax", + "backpedalled": "backpedaled", + "backpedalling": "backpedaling", + "bannister": "banister", + "bannisters": "banisters", + "baptise": "baptize", + "baptised": "baptized", + "baptises": "baptizes", + "baptising": "baptizing", + "bastardise": "bastardize", + "bastardised": "bastardized", + "bastardises": "bastardizes", + "bastardising": "bastardizing", + "battleax": "battleaxe", + "baulk": "balk", + "baulked": "balked", + "baulking": "balking", + "baulks": "balks", + "bedevilled": "bedeviled", + "bedevilling": "bedeviling", + "behaviour": "behavior", + "behavioural": "behavioral", + "behaviourism": "behaviorism", + "behaviourist": "behaviorist", + "behaviourists": "behaviorists", + "behaviours": "behaviors", + "behove": "behoove", + "behoved": "behooved", + "behoves": "behooves", + "bejewelled": "bejeweled", + "belabour": "belabor", + "belaboured": "belabored", + "belabouring": "belaboring", + "belabours": "belabors", + "bevelled": "beveled", + "bevvies": "bevies", + "bevvy": "bevy", + "biassed": "biased", + "biassing": "biasing", + "bingeing": "binging", + "bougainvillaea": "bougainvillea", + "bougainvillaeas": "bougainvilleas", + "bowdlerise": "bowdlerize", + "bowdlerised": "bowdlerized", + "bowdlerises": "bowdlerizes", + "bowdlerising": "bowdlerizing", + "breathalyse": "breathalyze", + "breathalysed": "breathalyzed", + "breathalyser": "breathalyzer", + "breathalysers": "breathalyzers", + "breathalyses": "breathalyzes", + "breathalysing": "breathalyzing", + "brutalise": "brutalize", + "brutalised": "brutalized", + "brutalises": "brutalizes", + "brutalising": "brutalizing", + "busses": "buses", + "bussing": "busing", + "caesarean": "cesarean", + "caesareans": "cesareans", + "calibre": "caliber", + "calibres": "calibers", + "calliper": "caliper", + "callipers": "calipers", + "callisthenics": "calisthenics", + "canalise": "canalize", + "canalised": "canalized", + "canalises": "canalizes", + "canalising": "canalizing", + "cancelation": "cancellation", + "cancelations": "cancellations", + "cancelled": "canceled", + "cancelling": "canceling", + "candour": "candor", + "cannibalise": "cannibalize", + "cannibalised": "cannibalized", + "cannibalises": "cannibalizes", + "cannibalising": "cannibalizing", + "canonise": "canonize", + "canonised": "canonized", + "canonises": "canonizes", + "canonising": "canonizing", + "capitalise": "capitalize", + "capitalised": "capitalized", + "capitalises": "capitalizes", + "capitalising": "capitalizing", + "caramelise": "caramelize", + "caramelised": "caramelized", + "caramelises": "caramelizes", + "caramelising": "caramelizing", + "carbonise": "carbonize", + "carbonised": "carbonized", + "carbonises": "carbonizes", + "carbonising": "carbonizing", + "carolled": "caroled", + "carolling": "caroling", + "catalogue": "catalog", + "catalogued": "cataloged", + "catalogues": "catalogs", + "cataloguing": "cataloging", + "catalyse": "catalyze", + "catalysed": "catalyzed", + "catalyses": "catalyzes", + "catalysing": "catalyzing", + "categorise": "categorize", + "categorised": "categorized", + "categorises": "categorizes", + "categorising": "categorizing", + "cauterise": "cauterize", + "cauterised": "cauterized", + "cauterises": "cauterizes", + "cauterising": "cauterizing", + "cavilled": "caviled", + "cavilling": "caviling", + "centigramme": "centigram", + "centigrammes": "centigrams", + "centilitre": "centiliter", + "centilitres": "centiliters", + "centimetre": "centimeter", + "centimetres": "centimeters", + "centralise": "centralize", + "centralised": "centralized", + "centralises": "centralizes", + "centralising": "centralizing", + "centre": "center", + "centred": "centered", + "centrefold": "centerfold", + "centrefolds": "centerfolds", + "centrepiece": "centerpiece", + "centrepieces": "centerpieces", + "centres": "centers", + "channelled": "channeled", + "channelling": "channeling", + "characterise": "characterize", + "characterised": "characterized", + "characterises": "characterizes", + "characterising": "characterizing", + "cheque": "check", + "chequebook": "checkbook", + "chequebooks": "checkbooks", + "chequered": "checkered", + "cheques": "checks", + "chilli": "chili", + "chimaera": "chimera", + "chimaeras": "chimeras", + "chiselled": "chiseled", + "chiselling": "chiseling", + "circularise": "circularize", + "circularised": "circularized", + "circularises": "circularizes", + "circularising": "circularizing", + "civilise": "civilize", + "civilised": "civilized", + "civilises": "civilizes", + "civilising": "civilizing", + "clamour": "clamor", + "clamoured": "clamored", + "clamouring": "clamoring", + "clamours": "clamors", + "clangour": "clangor", + "clarinettist": "clarinetist", + "clarinettists": "clarinetists", + "collectivise": "collectivize", + "collectivised": "collectivized", + "collectivises": "collectivizes", + "collectivising": "collectivizing", + "colonisation": "colonization", + "colonise": "colonize", + "colonised": "colonized", + "coloniser": "colonizer", + "colonisers": "colonizers", + "colonises": "colonizes", + "colonising": "colonizing", + "colour": "color", + "colourant": "colorant", + "colourants": "colorants", + "coloured": "colored", + "coloureds": "coloreds", + "colourful": "colorful", + "colourfully": "colorfully", + "colouring": "coloring", + "colourize": "colorize", + "colourized": "colorized", + "colourizes": "colorizes", + "colourizing": "colorizing", + "colourless": "colorless", + "colours": "colors", + "commercialise": "commercialize", + "commercialised": "commercialized", + "commercialises": "commercializes", + "commercialising": "commercializing", + "compartmentalise": "compartmentalize", + "compartmentalised": "compartmentalized", + "compartmentalises": "compartmentalizes", + "compartmentalising": "compartmentalizing", + "computerise": "computerize", + "computerised": "computerized", + "computerises": "computerizes", + "computerising": "computerizing", + "conceptualise": "conceptualize", + "conceptualised": "conceptualized", + "conceptualises": "conceptualizes", + "conceptualising": "conceptualizing", + "connexion": "connection", + "connexions": "connections", + "contextualise": "contextualize", + "contextualised": "contextualized", + "contextualises": "contextualizes", + "contextualising": "contextualizing", + "cosier": "cozier", + "cosies": "cozies", + "cosiest": "coziest", + "cosily": "cozily", + "cosiness": "coziness", + "cosy": "cozy", + "councillor": "councilor", + "councillors": "councilors", + "counselled": "counseled", + "counselling": "counseling", + "counsellor": "counselor", + "counsellors": "counselors", + "crenelated": "crenellated", + "criminalise": "criminalize", + "criminalised": "criminalized", + "criminalises": "criminalizes", + "criminalising": "criminalizing", + "criticise": "criticize", + "criticised": "criticized", + "criticises": "criticizes", + "criticising": "criticizing", + "crueller": "crueler", + "cruellest": "cruelest", + "crystallisation": "crystallization", + "crystallise": "crystallize", + "crystallised": "crystallized", + "crystallises": "crystallizes", + "crystallising": "crystallizing", + "cudgelled": "cudgeled", + "cudgelling": "cudgeling", + "customise": "customize", + "customised": "customized", + "customises": "customizes", + "customising": "customizing", + "cypher": "cipher", + "cyphers": "ciphers", + "decentralisation": "decentralization", + "decentralise": "decentralize", + "decentralised": "decentralized", + "decentralises": "decentralizes", + "decentralising": "decentralizing", + "decriminalisation": "decriminalization", + "decriminalise": "decriminalize", + "decriminalised": "decriminalized", + "decriminalises": "decriminalizes", + "decriminalising": "decriminalizing", + "defence": "defense", + "defenceless": "defenseless", + "defences": "defenses", + "dehumanisation": "dehumanization", + "dehumanise": "dehumanize", + "dehumanised": "dehumanized", + "dehumanises": "dehumanizes", + "dehumanising": "dehumanizing", + "demeanour": "demeanor", + "demilitarisation": "demilitarization", + "demilitarise": "demilitarize", + "demilitarised": "demilitarized", + "demilitarises": "demilitarizes", + "demilitarising": "demilitarizing", + "demobilisation": "demobilization", + "demobilise": "demobilize", + "demobilised": "demobilized", + "demobilises": "demobilizes", + "demobilising": "demobilizing", + "democratisation": "democratization", + "democratise": "democratize", + "democratised": "democratized", + "democratises": "democratizes", + "democratising": "democratizing", + "demonise": "demonize", + "demonised": "demonized", + "demonises": "demonizes", + "demonising": "demonizing", + "demoralisation": "demoralization", + "demoralise": "demoralize", + "demoralised": "demoralized", + "demoralises": "demoralizes", + "demoralising": "demoralizing", + "denationalisation": "denationalization", + "denationalise": "denationalize", + "denationalised": "denationalized", + "denationalises": "denationalizes", + "denationalising": "denationalizing", + "deodorise": "deodorize", + "deodorised": "deodorized", + "deodorises": "deodorizes", + "deodorising": "deodorizing", + "depersonalise": "depersonalize", + "depersonalised": "depersonalized", + "depersonalises": "depersonalizes", + "depersonalising": "depersonalizing", + "deputise": "deputize", + "deputised": "deputized", + "deputises": "deputizes", + "deputising": "deputizing", + "desensitisation": "desensitization", + "desensitise": "desensitize", + "desensitised": "desensitized", + "desensitises": "desensitizes", + "desensitising": "desensitizing", + "destabilisation": "destabilization", + "destabilise": "destabilize", + "destabilised": "destabilized", + "destabilises": "destabilizes", + "destabilising": "destabilizing", + "dialled": "dialed", + "dialling": "dialing", + "dialogue": "dialog", + "dialogues": "dialogs", + "diarrhoea": "diarrhea", + "digitise": "digitize", + "digitised": "digitized", + "digitises": "digitizes", + "digitising": "digitizing", + "disc": "disk", + "discolour": "discolor", + "discoloured": "discolored", + "discolouring": "discoloring", + "discolours": "discolors", + "discs": "disks", + "disembowelled": "disemboweled", + "disembowelling": "disemboweling", + "disfavour": "disfavor", + "dishevelled": "disheveled", + "dishonour": "dishonor", + "dishonourable": "dishonorable", + "dishonourably": "dishonorably", + "dishonoured": "dishonored", + "dishonouring": "dishonoring", + "dishonours": "dishonors", + "disorganisation": "disorganization", + "disorganised": "disorganized", + "distil": "distill", + "distils": "distills", + "dramatisation": "dramatization", + "dramatisations": "dramatizations", + "dramatise": "dramatize", + "dramatised": "dramatized", + "dramatises": "dramatizes", + "dramatising": "dramatizing", + "draught": "draft", + "draughtboard": "draftboard", + "draughtboards": "draftboards", + "draughtier": "draftier", + "draughtiest": "draftiest", + "draughts": "drafts", + "draughtsman": "draftsman", + "draughtsmanship": "draftsmanship", + "draughtsmen": "draftsmen", + "draughtswoman": "draftswoman", + "draughtswomen": "draftswomen", + "draughty": "drafty", + "drivelled": "driveled", + "drivelling": "driveling", + "duelled": "dueled", + "duelling": "dueling", + "economise": "economize", + "economised": "economized", + "economises": "economizes", + "economising": "economizing", + "edoema": "edema", + "editorialise": "editorialize", + "editorialised": "editorialized", + "editorialises": "editorializes", + "editorialising": "editorializing", + "empathise": "empathize", + "empathised": "empathized", + "empathises": "empathizes", + "empathising": "empathizing", + "emphasise": "emphasize", + "emphasised": "emphasized", + "emphasises": "emphasizes", + "emphasising": "emphasizing", + "enamelled": "enameled", + "enamelling": "enameling", + "enamoured": "enamored", + "encyclopaedia": "encyclopedia", + "encyclopaedias": "encyclopedias", + "encyclopaedic": "encyclopedic", + "endeavour": "endeavor", + "endeavoured": "endeavored", + "endeavouring": "endeavoring", + "endeavours": "endeavors", + "energise": "energize", + "energised": "energized", + "energises": "energizes", + "energising": "energizing", + "enrol": "enroll", + "enrols": "enrolls", + "enthral": "enthrall", + "enthrals": "enthralls", + "epaulette": "epaulet", + "epaulettes": "epaulets", + "epicentre": "epicenter", + "epicentres": "epicenters", + "epilogue": "epilog", + "epilogues": "epilogs", + "epitomise": "epitomize", + "epitomised": "epitomized", + "epitomises": "epitomizes", + "epitomising": "epitomizing", + "equalisation": "equalization", + "equalise": "equalize", + "equalised": "equalized", + "equaliser": "equalizer", + "equalisers": "equalizers", + "equalises": "equalizes", + "equalising": "equalizing", + "eulogise": "eulogize", + "eulogised": "eulogized", + "eulogises": "eulogizes", + "eulogising": "eulogizing", + "evangelise": "evangelize", + "evangelised": "evangelized", + "evangelises": "evangelizes", + "evangelising": "evangelizing", + "exorcise": "exorcize", + "exorcised": "exorcized", + "exorcises": "exorcizes", + "exorcising": "exorcizing", + "extemporisation": "extemporization", + "extemporise": "extemporize", + "extemporised": "extemporized", + "extemporises": "extemporizes", + "extemporising": "extemporizing", + "externalisation": "externalization", + "externalisations": "externalizations", + "externalise": "externalize", + "externalised": "externalized", + "externalises": "externalizes", + "externalising": "externalizing", + "factorise": "factorize", + "factorised": "factorized", + "factorises": "factorizes", + "factorising": "factorizing", + "faecal": "fecal", + "faeces": "feces", + "familiarisation": "familiarization", + "familiarise": "familiarize", + "familiarised": "familiarized", + "familiarises": "familiarizes", + "familiarising": "familiarizing", + "fantasise": "fantasize", + "fantasised": "fantasized", + "fantasises": "fantasizes", + "fantasising": "fantasizing", + "favour": "favor", + "favourable": "favorable", + "favourably": "favorably", + "favoured": "favored", + "favouring": "favoring", + "favourite": "favorite", + "favourites": "favorites", + "favouritism": "favoritism", + "favours": "favors", + "feminise": "feminize", + "feminised": "feminized", + "feminises": "feminizes", + "feminising": "feminizing", + "fertilisation": "fertilization", + "fertilise": "fertilize", + "fertilised": "fertilized", + "fertiliser": "fertilizer", + "fertilisers": "fertilizers", + "fertilises": "fertilizes", + "fertilising": "fertilizing", + "fervour": "fervor", + "fibre": "fiber", + "fibreglass": "fiberglass", + "fibres": "fibers", + "fictionalisation": "fictionalization", + "fictionalisations": "fictionalizations", + "fictionalise": "fictionalize", + "fictionalised": "fictionalized", + "fictionalises": "fictionalizes", + "fictionalising": "fictionalizing", + "fillet": "filet", + "filleted": "fileted", + "filleting": "fileting", + "fillets": "filets", + "finalisation": "finalization", + "finalise": "finalize", + "finalised": "finalized", + "finalises": "finalizes", + "finalising": "finalizing", + "flautist": "flutist", + "flautists": "flutists", + "flavour": "flavor", + "flavoured": "flavored", + "flavouring": "flavoring", + "flavourings": "flavorings", + "flavourless": "flavorless", + "flavours": "flavors", + "flavoursome": "flavorsome", + "flyer / flier": "flier / flyer", + "foetal": "fetal", + "foetid": "fetid", + "foetus": "fetus", + "foetuses": "fetuses", + "formalisation": "formalization", + "formalise": "formalize", + "formalised": "formalized", + "formalises": "formalizes", + "formalising": "formalizing", + "fossilisation": "fossilization", + "fossilise": "fossilize", + "fossilised": "fossilized", + "fossilises": "fossilizes", + "fossilising": "fossilizing", + "fraternisation": "fraternization", + "fraternise": "fraternize", + "fraternised": "fraternized", + "fraternises": "fraternizes", + "fraternising": "fraternizing", + "fulfil": "fulfill", + "fulfilment": "fulfillment", + "fulfils": "fulfills", + "funnelled": "funneled", + "funnelling": "funneling", + "galvanise": "galvanize", + "galvanised": "galvanized", + "galvanises": "galvanizes", + "galvanising": "galvanizing", + "gambolled": "gamboled", + "gambolling": "gamboling", + "gaol": "jail", + "gaolbird": "jailbird", + "gaolbirds": "jailbirds", + "gaolbreak": "jailbreak", + "gaolbreaks": "jailbreaks", + "gaoled": "jailed", + "gaoler": "jailer", + "gaolers": "jailers", + "gaoling": "jailing", + "gaols": "jails", + "gasses": "gases", + "gage": "gauge", + "gaged": "gauged", + "gages": "gauges", + "gaging": "gauging", + "generalisation": "generalization", + "generalisations": "generalizations", + "generalise": "generalize", + "generalised": "generalized", + "generalises": "generalizes", + "generalising": "generalizing", + "ghettoise": "ghettoize", + "ghettoised": "ghettoized", + "ghettoises": "ghettoizes", + "ghettoising": "ghettoizing", + "gipsies": "gypsies", + "glamorise": "glamorize", + "glamorised": "glamorized", + "glamorises": "glamorizes", + "glamorising": "glamorizing", + "glamor": "glamour", + "globalisation": "globalization", + "globalise": "globalize", + "globalised": "globalized", + "globalises": "globalizes", + "globalising": "globalizing", + "glueing": "gluing", + "goitre": "goiter", + "goitres": "goiters", + "gonorrhoea": "gonorrhea", + "gramme": "gram", + "grammes": "grams", + "gravelled": "graveled", + "grey": "gray", + "greyed": "grayed", + "greying": "graying", + "greyish": "grayish", + "greyness": "grayness", + "greys": "grays", + "grovelled": "groveled", + "grovelling": "groveling", + "groyne": "groin", + "groynes": "groins", + "gruelling": "grueling", + "gruellingly": "gruelingly", + "gryphon": "griffin", + "gryphons": "griffins", + "gynaecological": "gynecological", + "gynaecologist": "gynecologist", + "gynaecologists": "gynecologists", + "gynaecology": "gynecology", + "haematological": "hematological", + "haematologist": "hematologist", + "haematologists": "hematologists", + "haematology": "hematology", + "haemoglobin": "hemoglobin", + "haemophilia": "hemophilia", + "haemophiliac": "hemophiliac", + "haemophiliacs": "hemophiliacs", + "haemorrhage": "hemorrhage", + "haemorrhaged": "hemorrhaged", + "haemorrhages": "hemorrhages", + "haemorrhaging": "hemorrhaging", + "haemorrhoids": "hemorrhoids", + "harbour": "harbor", + "harboured": "harbored", + "harbouring": "harboring", + "harbours": "harbors", + "harmonisation": "harmonization", + "harmonise": "harmonize", + "harmonised": "harmonized", + "harmonises": "harmonizes", + "harmonising": "harmonizing", + "homoeopath": "homeopath", + "homoeopathic": "homeopathic", + "homoeopaths": "homeopaths", + "homoeopathy": "homeopathy", + "homogenise": "homogenize", + "homogenised": "homogenized", + "homogenises": "homogenizes", + "homogenising": "homogenizing", + "honour": "honor", + "honourable": "honorable", + "honourably": "honorably", + "honoured": "honored", + "honouring": "honoring", + "honours": "honors", + "hospitalisation": "hospitalization", + "hospitalise": "hospitalize", + "hospitalised": "hospitalized", + "hospitalises": "hospitalizes", + "hospitalising": "hospitalizing", + "humanise": "humanize", + "humanised": "humanized", + "humanises": "humanizes", + "humanising": "humanizing", + "humour": "humor", + "humoured": "humored", + "humouring": "humoring", + "humourless": "humorless", + "humours": "humors", + "hybridise": "hybridize", + "hybridised": "hybridized", + "hybridises": "hybridizes", + "hybridising": "hybridizing", + "hypnotise": "hypnotize", + "hypnotised": "hypnotized", + "hypnotises": "hypnotizes", + "hypnotising": "hypnotizing", + "hypothesise": "hypothesize", + "hypothesised": "hypothesized", + "hypothesises": "hypothesizes", + "hypothesising": "hypothesizing", + "idealisation": "idealization", + "idealise": "idealize", + "idealised": "idealized", + "idealises": "idealizes", + "idealising": "idealizing", + "idolise": "idolize", + "idolised": "idolized", + "idolises": "idolizes", + "idolising": "idolizing", + "immobilisation": "immobilization", + "immobilise": "immobilize", + "immobilised": "immobilized", + "immobiliser": "immobilizer", + "immobilisers": "immobilizers", + "immobilises": "immobilizes", + "immobilising": "immobilizing", + "immortalise": "immortalize", + "immortalised": "immortalized", + "immortalises": "immortalizes", + "immortalising": "immortalizing", + "immunisation": "immunization", + "immunise": "immunize", + "immunised": "immunized", + "immunises": "immunizes", + "immunising": "immunizing", + "impanelled": "impaneled", + "impanelling": "impaneling", + "imperilled": "imperiled", + "imperilling": "imperiling", + "individualise": "individualize", + "individualised": "individualized", + "individualises": "individualizes", + "individualising": "individualizing", + "industrialise": "industrialize", + "industrialised": "industrialized", + "industrialises": "industrializes", + "industrialising": "industrializing", + "inflexion": "inflection", + "inflexions": "inflections", + "initialise": "initialize", + "initialised": "initialized", + "initialises": "initializes", + "initialising": "initializing", + "initialled": "initialed", + "initialling": "initialing", + "instal": "install", + "instalment": "installment", + "instalments": "installments", + "instals": "installs", + "instil": "instill", + "instils": "instills", + "institutionalisation": "institutionalization", + "institutionalise": "institutionalize", + "institutionalised": "institutionalized", + "institutionalises": "institutionalizes", + "institutionalising": "institutionalizing", + "intellectualise": "intellectualize", + "intellectualised": "intellectualized", + "intellectualises": "intellectualizes", + "intellectualising": "intellectualizing", + "internalisation": "internalization", + "internalise": "internalize", + "internalised": "internalized", + "internalises": "internalizes", + "internalising": "internalizing", + "internationalisation": "internationalization", + "internationalise": "internationalize", + "internationalised": "internationalized", + "internationalises": "internationalizes", + "internationalising": "internationalizing", + "ionisation": "ionization", + "ionise": "ionize", + "ionised": "ionized", + "ioniser": "ionizer", + "ionisers": "ionizers", + "ionises": "ionizes", + "ionising": "ionizing", + "italicise": "italicize", + "italicised": "italicized", + "italicises": "italicizes", + "italicising": "italicizing", + "itemise": "itemize", + "itemised": "itemized", + "itemises": "itemizes", + "itemising": "itemizing", + "jeopardise": "jeopardize", + "jeopardised": "jeopardized", + "jeopardises": "jeopardizes", + "jeopardising": "jeopardizing", + "jewelled": "jeweled", + "jeweller": "jeweler", + "jewellers": "jewelers", + "jewellery": "jewelry", + "judgement": "judgment", + "kilogramme": "kilogram", + "kilogrammes": "kilograms", + "kilometre": "kilometer", + "kilometres": "kilometers", + "labelled": "labeled", + "labelling": "labeling", + "labour": "labor", + "laboured": "labored", + "labourer": "laborer", + "labourers": "laborers", + "labouring": "laboring", + "labours": "labors", + "lacklustre": "lackluster", + "legalisation": "legalization", + "legalise": "legalize", + "legalised": "legalized", + "legalises": "legalizes", + "legalising": "legalizing", + "legitimise": "legitimize", + "legitimised": "legitimized", + "legitimises": "legitimizes", + "legitimising": "legitimizing", + "leukaemia": "leukemia", + "levelled": "leveled", + "leveller": "leveler", + "levellers": "levelers", + "levelling": "leveling", + "libelled": "libeled", + "libelling": "libeling", + "libellous": "libelous", + "liberalisation": "liberalization", + "liberalise": "liberalize", + "liberalised": "liberalized", + "liberalises": "liberalizes", + "liberalising": "liberalizing", + "licence": "license", + "licenced": "licensed", + "licences": "licenses", + "licencing": "licensing", + "likeable": "likable", + "lionisation": "lionization", + "lionise": "lionize", + "lionised": "lionized", + "lionises": "lionizes", + "lionising": "lionizing", + "liquidise": "liquidize", + "liquidised": "liquidized", + "liquidiser": "liquidizer", + "liquidisers": "liquidizers", + "liquidises": "liquidizes", + "liquidising": "liquidizing", + "litre": "liter", + "litres": "liters", + "localise": "localize", + "localised": "localized", + "localises": "localizes", + "localising": "localizing", + "louvre": "louver", + "louvred": "louvered", + "louvres": "louvers", + "lustre": "luster", + "magnetise": "magnetize", + "magnetised": "magnetized", + "magnetises": "magnetizes", + "magnetising": "magnetizing", + "manoeuvrability": "maneuverability", + "manoeuvrable": "maneuverable", + "manoeuvre": "maneuver", + "manoeuvred": "maneuvered", + "manoeuvres": "maneuvers", + "manoeuvring": "maneuvering", + "manoeuvrings": "maneuverings", + "marginalisation": "marginalization", + "marginalise": "marginalize", + "marginalised": "marginalized", + "marginalises": "marginalizes", + "marginalising": "marginalizing", + "marshalled": "marshaled", + "marshalling": "marshaling", + "marvelled": "marveled", + "marvelling": "marveling", + "marvellous": "marvelous", + "marvellously": "marvelously", + "materialisation": "materialization", + "materialise": "materialize", + "materialised": "materialized", + "materialises": "materializes", + "materialising": "materializing", + "maximisation": "maximization", + "maximise": "maximize", + "maximised": "maximized", + "maximises": "maximizes", + "maximising": "maximizing", + "meagre": "meager", + "mechanisation": "mechanization", + "mechanise": "mechanize", + "mechanised": "mechanized", + "mechanises": "mechanizes", + "mechanising": "mechanizing", + "mediaeval": "medieval", + "memorialise": "memorialize", + "memorialised": "memorialized", + "memorialises": "memorializes", + "memorialising": "memorializing", + "memorise": "memorize", + "memorised": "memorized", + "memorises": "memorizes", + "memorising": "memorizing", + "mesmerise": "mesmerize", + "mesmerised": "mesmerized", + "mesmerises": "mesmerizes", + "mesmerising": "mesmerizing", + "metabolise": "metabolize", + "metabolised": "metabolized", + "metabolises": "metabolizes", + "metabolising": "metabolizing", + "metre": "meter", + "metres": "meters", + "micrometre": "micrometer", + "micrometres": "micrometers", + "militarise": "militarize", + "militarised": "militarized", + "militarises": "militarizes", + "militarising": "militarizing", + "milligramme": "milligram", + "milligrammes": "milligrams", + "millilitre": "milliliter", + "millilitres": "milliliters", + "millimetre": "millimeter", + "millimetres": "millimeters", + "miniaturisation": "miniaturization", + "miniaturise": "miniaturize", + "miniaturised": "miniaturized", + "miniaturises": "miniaturizes", + "miniaturising": "miniaturizing", + "minibusses": "minibuses", + "minimise": "minimize", + "minimised": "minimized", + "minimises": "minimizes", + "minimising": "minimizing", + "misbehaviour": "misbehavior", + "misdemeanour": "misdemeanor", + "misdemeanours": "misdemeanors", + "misspelt": "misspelled", + "mitre": "miter", + "mitres": "miters", + "mobilisation": "mobilization", + "mobilise": "mobilize", + "mobilised": "mobilized", + "mobilises": "mobilizes", + "mobilising": "mobilizing", + "modelled": "modeled", + "modeller": "modeler", + "modellers": "modelers", + "modelling": "modeling", + "modernise": "modernize", + "modernised": "modernized", + "modernises": "modernizes", + "modernising": "modernizing", + "moisturise": "moisturize", + "moisturised": "moisturized", + "moisturiser": "moisturizer", + "moisturisers": "moisturizers", + "moisturises": "moisturizes", + "moisturising": "moisturizing", + "monologue": "monolog", + "monologues": "monologs", + "monopolisation": "monopolization", + "monopolise": "monopolize", + "monopolised": "monopolized", + "monopolises": "monopolizes", + "monopolising": "monopolizing", + "moralise": "moralize", + "moralised": "moralized", + "moralises": "moralizes", + "moralising": "moralizing", + "motorised": "motorized", + "mould": "mold", + "moulded": "molded", + "moulder": "molder", + "mouldered": "moldered", + "mouldering": "moldering", + "moulders": "molders", + "mouldier": "moldier", + "mouldiest": "moldiest", + "moulding": "molding", + "mouldings": "moldings", + "moulds": "molds", + "mouldy": "moldy", + "moult": "molt", + "moulted": "molted", + "moulting": "molting", + "moults": "molts", + "moustache": "mustache", + "moustached": "mustached", + "moustaches": "mustaches", + "moustachioed": "mustachioed", + "multicoloured": "multicolored", + "nationalisation": "nationalization", + "nationalisations": "nationalizations", + "nationalise": "nationalize", + "nationalised": "nationalized", + "nationalises": "nationalizes", + "nationalising": "nationalizing", + "naturalisation": "naturalization", + "naturalise": "naturalize", + "naturalised": "naturalized", + "naturalises": "naturalizes", + "naturalising": "naturalizing", + "neighbour": "neighbor", + "neighbourhood": "neighborhood", + "neighbourhoods": "neighborhoods", + "neighbouring": "neighboring", + "neighbourliness": "neighborliness", + "neighbourly": "neighborly", + "neighbours": "neighbors", + "neutralisation": "neutralization", + "neutralise": "neutralize", + "neutralised": "neutralized", + "neutralises": "neutralizes", + "neutralising": "neutralizing", + "normalisation": "normalization", + "normalise": "normalize", + "normalised": "normalized", + "normalises": "normalizes", + "normalising": "normalizing", + "odour": "odor", + "odourless": "odorless", + "odours": "odors", + "oesophagus": "esophagus", + "oesophaguses": "esophaguses", + "oestrogen": "estrogen", + "offence": "offense", + "offences": "offenses", + "omelette": "omelet", + "omelettes": "omelets", + "optimise": "optimize", + "optimised": "optimized", + "optimises": "optimizes", + "optimising": "optimizing", + "organisation": "organization", + "organisational": "organizational", + "organisations": "organizations", + "organise": "organize", + "organised": "organized", + "organiser": "organizer", + "organisers": "organizers", + "organises": "organizes", + "organising": "organizing", + "orthopaedic": "orthopedic", + "orthopaedics": "orthopedics", + "ostracise": "ostracize", + "ostracised": "ostracized", + "ostracises": "ostracizes", + "ostracising": "ostracizing", + "outmanoeuvre": "outmaneuver", + "outmanoeuvred": "outmaneuvered", + "outmanoeuvres": "outmaneuvers", + "outmanoeuvring": "outmaneuvering", + "overemphasise": "overemphasize", + "overemphasised": "overemphasized", + "overemphasises": "overemphasizes", + "overemphasising": "overemphasizing", + "oxidisation": "oxidization", + "oxidise": "oxidize", + "oxidised": "oxidized", + "oxidises": "oxidizes", + "oxidising": "oxidizing", + "paederast": "pederast", + "paederasts": "pederasts", + "paediatric": "pediatric", + "paediatrician": "pediatrician", + "paediatricians": "pediatricians", + "paediatrics": "pediatrics", + "paedophile": "pedophile", + "paedophiles": "pedophiles", + "paedophilia": "pedophilia", + "palaeolithic": "paleolithic", + "palaeontologist": "paleontologist", + "palaeontologists": "paleontologists", + "palaeontology": "paleontology", + "panelled": "paneled", + "panelling": "paneling", + "panellist": "panelist", + "panellists": "panelists", + "paralyse": "paralyze", + "paralysed": "paralyzed", + "paralyses": "paralyzes", + "paralysing": "paralyzing", + "parcelled": "parceled", + "parcelling": "parceling", + "parlour": "parlor", + "parlours": "parlors", + "particularise": "particularize", + "particularised": "particularized", + "particularises": "particularizes", + "particularising": "particularizing", + "passivisation": "passivization", + "passivise": "passivize", + "passivised": "passivized", + "passivises": "passivizes", + "passivising": "passivizing", + "pasteurisation": "pasteurization", + "pasteurise": "pasteurize", + "pasteurised": "pasteurized", + "pasteurises": "pasteurizes", + "pasteurising": "pasteurizing", + "patronise": "patronize", + "patronised": "patronized", + "patronises": "patronizes", + "patronising": "patronizing", + "patronisingly": "patronizingly", + "pedalled": "pedaled", + "pedalling": "pedaling", + "pedestrianisation": "pedestrianization", + "pedestrianise": "pedestrianize", + "pedestrianised": "pedestrianized", + "pedestrianises": "pedestrianizes", + "pedestrianising": "pedestrianizing", + "penalise": "penalize", + "penalised": "penalized", + "penalises": "penalizes", + "penalising": "penalizing", + "pencilled": "penciled", + "pencilling": "penciling", + "personalise": "personalize", + "personalised": "personalized", + "personalises": "personalizes", + "personalising": "personalizing", + "pharmacopoeia": "pharmacopeia", + "pharmacopoeias": "pharmacopeias", + "philosophise": "philosophize", + "philosophised": "philosophized", + "philosophises": "philosophizes", + "philosophising": "philosophizing", + "philtre": "filter", + "philtres": "filters", + "phoney": "phony", + "plagiarise": "plagiarize", + "plagiarised": "plagiarized", + "plagiarises": "plagiarizes", + "plagiarising": "plagiarizing", + "plough": "plow", + "ploughed": "plowed", + "ploughing": "plowing", + "ploughman": "plowman", + "ploughmen": "plowmen", + "ploughs": "plows", + "ploughshare": "plowshare", + "ploughshares": "plowshares", + "polarisation": "polarization", + "polarise": "polarize", + "polarised": "polarized", + "polarises": "polarizes", + "polarising": "polarizing", + "politicisation": "politicization", + "politicise": "politicize", + "politicised": "politicized", + "politicises": "politicizes", + "politicising": "politicizing", + "popularisation": "popularization", + "popularise": "popularize", + "popularised": "popularized", + "popularises": "popularizes", + "popularising": "popularizing", + "pouffe": "pouf", + "pouffes": "poufs", + "practise": "practice", + "practised": "practiced", + "practises": "practices", + "practising": "practicing", + "praesidium": "presidium", + "praesidiums": "presidiums", + "pressurisation": "pressurization", + "pressurise": "pressurize", + "pressurised": "pressurized", + "pressurises": "pressurizes", + "pressurising": "pressurizing", + "pretence": "pretense", + "pretences": "pretenses", + "primaeval": "primeval", + "prioritisation": "prioritization", + "prioritise": "prioritize", + "prioritised": "prioritized", + "prioritises": "prioritizes", + "prioritising": "prioritizing", + "privatisation": "privatization", + "privatisations": "privatizations", + "privatise": "privatize", + "privatised": "privatized", + "privatises": "privatizes", + "privatising": "privatizing", + "professionalisation": "professionalization", + "professionalise": "professionalize", + "professionalised": "professionalized", + "professionalises": "professionalizes", + "professionalising": "professionalizing", + "programme": "program", + "programmes": "programs", + "prologue": "prolog", + "prologues": "prologs", + "propagandise": "propagandize", + "propagandised": "propagandized", + "propagandises": "propagandizes", + "propagandising": "propagandizing", + "proselytise": "proselytize", + "proselytised": "proselytized", + "proselytiser": "proselytizer", + "proselytisers": "proselytizers", + "proselytises": "proselytizes", + "proselytising": "proselytizing", + "psychoanalyse": "psychoanalyze", + "psychoanalysed": "psychoanalyzed", + "psychoanalyses": "psychoanalyzes", + "psychoanalysing": "psychoanalyzing", + "publicise": "publicize", + "publicised": "publicized", + "publicises": "publicizes", + "publicising": "publicizing", + "pulverisation": "pulverization", + "pulverise": "pulverize", + "pulverised": "pulverized", + "pulverises": "pulverizes", + "pulverising": "pulverizing", + "pummelled": "pummel", + "pummelling": "pummeled", + "pyjama": "pajama", + "pyjamas": "pajamas", + "pzazz": "pizzazz", + "quarrelled": "quarreled", + "quarrelling": "quarreling", + "radicalise": "radicalize", + "radicalised": "radicalized", + "radicalises": "radicalizes", + "radicalising": "radicalizing", + "rancour": "rancor", + "randomise": "randomize", + "randomised": "randomized", + "randomises": "randomizes", + "randomising": "randomizing", + "rationalisation": "rationalization", + "rationalisations": "rationalizations", + "rationalise": "rationalize", + "rationalised": "rationalized", + "rationalises": "rationalizes", + "rationalising": "rationalizing", + "ravelled": "raveled", + "ravelling": "raveling", + "realisable": "realizable", + "realisation": "realization", + "realisations": "realizations", + "realise": "realize", + "realised": "realized", + "realises": "realizes", + "realising": "realizing", + "recognisable": "recognizable", + "recognisably": "recognizably", + "recognisance": "recognizance", + "recognise": "recognize", + "recognised": "recognized", + "recognises": "recognizes", + "recognising": "recognizing", + "reconnoitre": "reconnoiter", + "reconnoitred": "reconnoitered", + "reconnoitres": "reconnoiters", + "reconnoitring": "reconnoitering", + "refuelled": "refueled", + "refuelling": "refueling", + "regularisation": "regularization", + "regularise": "regularize", + "regularised": "regularized", + "regularises": "regularizes", + "regularising": "regularizing", + "remodelled": "remodeled", + "remodelling": "remodeling", + "remould": "remold", + "remoulded": "remolded", + "remoulding": "remolding", + "remoulds": "remolds", + "reorganisation": "reorganization", + "reorganisations": "reorganizations", + "reorganise": "reorganize", + "reorganised": "reorganized", + "reorganises": "reorganizes", + "reorganising": "reorganizing", + "revelled": "reveled", + "reveller": "reveler", + "revellers": "revelers", + "revelling": "reveling", + "revitalise": "revitalize", + "revitalised": "revitalized", + "revitalises": "revitalizes", + "revitalising": "revitalizing", + "revolutionise": "revolutionize", + "revolutionised": "revolutionized", + "revolutionises": "revolutionizes", + "revolutionising": "revolutionizing", + "rhapsodise": "rhapsodize", + "rhapsodised": "rhapsodized", + "rhapsodises": "rhapsodizes", + "rhapsodising": "rhapsodizing", + "rigour": "rigor", + "rigours": "rigors", + "ritualised": "ritualized", + "rivalled": "rivaled", + "rivalling": "rivaling", + "romanticise": "romanticize", + "romanticised": "romanticized", + "romanticises": "romanticizes", + "romanticising": "romanticizing", + "rumour": "rumor", + "rumoured": "rumored", + "rumours": "rumors", + "sabre": "saber", + "sabres": "sabers", + "saltpetre": "saltpeter", + "sanitise": "sanitize", + "sanitised": "sanitized", + "sanitises": "sanitizes", + "sanitising": "sanitizing", + "satirise": "satirize", + "satirised": "satirized", + "satirises": "satirizes", + "satirising": "satirizing", + "saviour": "savior", + "saviours": "saviors", + "savour": "savor", + "savoured": "savored", + "savouries": "savories", + "savouring": "savoring", + "savours": "savors", + "savoury": "savory", + "scandalise": "scandalize", + "scandalised": "scandalized", + "scandalises": "scandalizes", + "scandalising": "scandalizing", + "sceptic": "skeptic", + "sceptical": "skeptical", + "sceptically": "skeptically", + "scepticism": "skepticism", + "sceptics": "skeptics", + "sceptre": "scepter", + "sceptres": "scepters", + "scrutinise": "scrutinize", + "scrutinised": "scrutinized", + "scrutinises": "scrutinizes", + "scrutinising": "scrutinizing", + "secularisation": "secularization", + "secularise": "secularize", + "secularised": "secularized", + "secularises": "secularizes", + "secularising": "secularizing", + "sensationalise": "sensationalize", + "sensationalised": "sensationalized", + "sensationalises": "sensationalizes", + "sensationalising": "sensationalizing", + "sensitise": "sensitize", + "sensitised": "sensitized", + "sensitises": "sensitizes", + "sensitising": "sensitizing", + "sentimentalise": "sentimentalize", + "sentimentalised": "sentimentalized", + "sentimentalises": "sentimentalizes", + "sentimentalising": "sentimentalizing", + "sepulchre": "sepulcher", + "sepulchres": "sepulchers", + "serialisation": "serialization", + "serialisations": "serializations", + "serialise": "serialize", + "serialised": "serialized", + "serialises": "serializes", + "serialising": "serializing", + "sermonise": "sermonize", + "sermonised": "sermonized", + "sermonises": "sermonizes", + "sermonising": "sermonizing", + "sheikh": "sheik", + "shovelled": "shoveled", + "shovelling": "shoveling", + "shrivelled": "shriveled", + "shrivelling": "shriveling", + "signalise": "signalize", + "signalised": "signalized", + "signalises": "signalizes", + "signalising": "signalizing", + "signalled": "signaled", + "signalling": "signaling", + "smoulder": "smolder", + "smouldered": "smoldered", + "smouldering": "smoldering", + "smoulders": "smolders", + "snivelled": "sniveled", + "snivelling": "sniveling", + "snorkelled": "snorkeled", + "snorkelling": "snorkeling", + "snowplough": "snowplow", + "snowploughs": "snowplow", + "socialisation": "socialization", + "socialise": "socialize", + "socialised": "socialized", + "socialises": "socializes", + "socialising": "socializing", + "sodomise": "sodomize", + "sodomised": "sodomized", + "sodomises": "sodomizes", + "sodomising": "sodomizing", + "solemnise": "solemnize", + "solemnised": "solemnized", + "solemnises": "solemnizes", + "solemnising": "solemnizing", + "sombre": "somber", + "specialisation": "specialization", + "specialisations": "specializations", + "specialise": "specialize", + "specialised": "specialized", + "specialises": "specializes", + "specialising": "specializing", + "spectre": "specter", + "spectres": "specters", + "spiralled": "spiraled", + "spiralling": "spiraling", + "splendour": "splendor", + "splendours": "splendors", + "squirrelled": "squirreled", + "squirrelling": "squirreling", + "stabilisation": "stabilization", + "stabilise": "stabilize", + "stabilised": "stabilized", + "stabiliser": "stabilizer", + "stabilisers": "stabilizers", + "stabilises": "stabilizes", + "stabilising": "stabilizing", + "standardisation": "standardization", + "standardise": "standardize", + "standardised": "standardized", + "standardises": "standardizes", + "standardising": "standardizing", + "stencilled": "stenciled", + "stencilling": "stenciling", + "sterilisation": "sterilization", + "sterilisations": "sterilizations", + "sterilise": "sterilize", + "sterilised": "sterilized", + "steriliser": "sterilizer", + "sterilisers": "sterilizers", + "sterilises": "sterilizes", + "sterilising": "sterilizing", + "stigmatisation": "stigmatization", + "stigmatise": "stigmatize", + "stigmatised": "stigmatized", + "stigmatises": "stigmatizes", + "stigmatising": "stigmatizing", + "storey": "story", + "storeys": "stories", + "subsidisation": "subsidization", + "subsidise": "subsidize", + "subsidised": "subsidized", + "subsidiser": "subsidizer", + "subsidisers": "subsidizers", + "subsidises": "subsidizes", + "subsidising": "subsidizing", + "succour": "succor", + "succoured": "succored", + "succouring": "succoring", + "succours": "succors", + "sulphate": "sulfate", + "sulphates": "sulfates", + "sulphide": "sulfide", + "sulphides": "sulfides", + "sulphur": "sulfur", + "sulphurous": "sulfurous", + "summarise": "summarize", + "summarised": "summarized", + "summarises": "summarizes", + "summarising": "summarizing", + "swivelled": "swiveled", + "swivelling": "swiveling", + "symbolise": "symbolize", + "symbolised": "symbolized", + "symbolises": "symbolizes", + "symbolising": "symbolizing", + "sympathise": "sympathize", + "sympathised": "sympathized", + "sympathiser": "sympathizer", + "sympathisers": "sympathizers", + "sympathises": "sympathizes", + "sympathising": "sympathizing", + "synchronisation": "synchronization", + "synchronise": "synchronize", + "synchronised": "synchronized", + "synchronises": "synchronizes", + "synchronising": "synchronizing", + "synthesise": "synthesize", + "synthesised": "synthesized", + "synthesiser": "synthesizer", + "synthesisers": "synthesizers", + "synthesises": "synthesizes", + "synthesising": "synthesizing", + "syphon": "siphon", + "syphoned": "siphoned", + "syphoning": "siphoning", + "syphons": "siphons", + "systematisation": "systematization", + "systematise": "systematize", + "systematised": "systematized", + "systematises": "systematizes", + "systematising": "systematizing", + "tantalise": "tantalize", + "tantalised": "tantalized", + "tantalises": "tantalizes", + "tantalising": "tantalizing", + "tantalisingly": "tantalizingly", + "tasselled": "tasseled", + "technicolour": "technicolor", + "temporise": "temporize", + "temporised": "temporized", + "temporises": "temporizes", + "temporising": "temporizing", + "tenderise": "tenderize", + "tenderised": "tenderized", + "tenderises": "tenderizes", + "tenderising": "tenderizing", + "terrorise": "terrorize", + "terrorised": "terrorized", + "terrorises": "terrorizes", + "terrorising": "terrorizing", + "theatre": "theater", + "theatregoer": "theatergoer", + "theatregoers": "theatergoers", + "theatres": "theaters", + "theorise": "theorize", + "theorised": "theorized", + "theorises": "theorizes", + "theorising": "theorizing", + "tonne": "ton", + "tonnes": "tons", + "towelled": "toweled", + "towelling": "toweling", + "toxaemia": "toxemia", + "tranquillise": "tranquilize", + "tranquillised": "tranquilized", + "tranquilliser": "tranquilizer", + "tranquillisers": "tranquilizers", + "tranquillises": "tranquilizes", + "tranquillising": "tranquilizing", + "tranquillity": "tranquility", + "tranquillize": "tranquilize", + "tranquillized": "tranquilized", + "tranquillizer": "tranquilizer", + "tranquillizers": "tranquilizers", + "tranquillizes": "tranquilizes", + "tranquillizing": "tranquilizing", + "tranquilly": "tranquility", + "transistorised": "transistorized", + "traumatise": "traumatize", + "traumatised": "traumatized", + "traumatises": "traumatizes", + "traumatising": "traumatizing", + "travelled": "traveled", + "traveller": "traveler", + "travellers": "travelers", + "travelling": "traveling", + "travelog": "travelogue", + "travelogs": "travelogues", + "trialled": "trialed", + "trialling": "trialing", + "tricolour": "tricolor", + "tricolours": "tricolors", + "trivialise": "trivialize", + "trivialised": "trivialized", + "trivialises": "trivializes", + "trivialising": "trivializing", + "tumour": "tumor", + "tumours": "tumors", + "tunnelled": "tunneled", + "tunnelling": "tunneling", + "tyrannise": "tyrannize", + "tyrannised": "tyrannized", + "tyrannises": "tyrannizes", + "tyrannising": "tyrannizing", + "tyre": "tire", + "tyres": "tires", + "unauthorised": "unauthorized", + "uncivilised": "uncivilized", + "underutilised": "underutilized", + "unequalled": "unequaled", + "unfavourable": "unfavorable", + "unfavourably": "unfavorably", + "unionisation": "unionization", + "unionise": "unionize", + "unionised": "unionized", + "unionises": "unionizes", + "unionising": "unionizing", + "unorganised": "unorganized", + "unravelled": "unraveled", + "unravelling": "unraveling", + "unrecognisable": "unrecognizable", + "unrecognised": "unrecognized", + "unrivalled": "unrivaled", + "unsavoury": "unsavory", + "untrammelled": "untrammeled", + "urbanisation": "urbanization", + "urbanise": "urbanize", + "urbanised": "urbanized", + "urbanises": "urbanizes", + "urbanising": "urbanizing", + "utilisable": "utilizable", + "utilisation": "utilization", + "utilise": "utilize", + "utilised": "utilized", + "utilises": "utilizes", + "utilising": "utilizing", + "valour": "valor", + "vandalise": "vandalize", + "vandalised": "vandalized", + "vandalises": "vandalizes", + "vandalising": "vandalizing", + "vaporisation": "vaporization", + "vaporise": "vaporize", + "vaporised": "vaporized", + "vaporises": "vaporizes", + "vaporising": "vaporizing", + "vapour": "vapor", + "vapours": "vapors", + "verbalise": "verbalize", + "verbalised": "verbalized", + "verbalises": "verbalizes", + "verbalising": "verbalizing", + "victimisation": "victimization", + "victimise": "victimize", + "victimised": "victimized", + "victimises": "victimizes", + "victimising": "victimizing", + "videodisc": "videodisk", + "videodiscs": "videodisks", + "vigour": "vigor", + "visualisation": "visualization", + "visualisations": "visualizations", + "visualise": "visualize", + "visualised": "visualized", + "visualises": "visualizes", + "visualising": "visualizing", + "vocalisation": "vocalization", + "vocalisations": "vocalizations", + "vocalise": "vocalize", + "vocalised": "vocalized", + "vocalises": "vocalizes", + "vocalising": "vocalizing", + "vulcanised": "vulcanized", + "vulgarisation": "vulgarization", + "vulgarise": "vulgarize", + "vulgarised": "vulgarized", + "vulgarises": "vulgarizes", + "vulgarising": "vulgarizing", + "waggon": "wagon", + "waggons": "wagons", + "watercolour": "watercolor", + "watercolours": "watercolors", + "weaselled": "weaseled", + "weaselling": "weaseling", + "westernisation": "westernization", + "westernise": "westernize", + "westernised": "westernized", + "westernises": "westernizes", + "westernising": "westernizing", + "womanise": "womanize", + "womanised": "womanized", + "womaniser": "womanizer", + "womanisers": "womanizers", + "womanises": "womanizes", + "womanising": "womanizing", + "woollen": "woolen", + "woollens": "woolens", + "woollies": "woolies", + "woolly": "wooly", + "worshipped": "worshiped", + "worshipping": "worshiping", + "worshipper": "worshiper", + "yodelled": "yodeled", + "yodelling": "yodeling", + "yoghourt": "yogurt", + "yoghourts": "yogurts", + "yoghurt": "yogurt", + "yoghurts": "yogurts", + "mhm": "hmm", + "mmm": "hmm" +} \ No newline at end of file diff --git a/whisper/normalizers/english.py b/whisper/normalizers/english.py new file mode 100644 index 0000000000000000000000000000000000000000..d5c2bb4ebef1a04b9ab14970c10c3fb0d4e948d4 --- /dev/null +++ b/whisper/normalizers/english.py @@ -0,0 +1,543 @@ +import json +import os +import re +from fractions import Fraction +from typing import Iterator, List, Match, Optional, Union + +from more_itertools import windowed + +from .basic import remove_symbols_and_diacritics + + +class EnglishNumberNormalizer: + """ + Convert any spelled-out numbers into arabic numbers, while handling: + + - remove any commas + - keep the suffixes such as: `1960s`, `274th`, `32nd`, etc. + - spell out currency symbols after the number. e.g. `$20 million` -> `20000000 dollars` + - spell out `one` and `ones` + - interpret successive single-digit numbers as nominal: `one oh one` -> `101` + """ + + def __init__(self): + super().__init__() + + self.zeros = {"o", "oh", "zero"} + self.ones = { + name: i + for i, name in enumerate( + [ + "one", + "two", + "three", + "four", + "five", + "six", + "seven", + "eight", + "nine", + "ten", + "eleven", + "twelve", + "thirteen", + "fourteen", + "fifteen", + "sixteen", + "seventeen", + "eighteen", + "nineteen", + ], + start=1, + ) + } + self.ones_plural = { + "sixes" if name == "six" else name + "s": (value, "s") + for name, value in self.ones.items() + } + self.ones_ordinal = { + "zeroth": (0, "th"), + "first": (1, "st"), + "second": (2, "nd"), + "third": (3, "rd"), + "fifth": (5, "th"), + "twelfth": (12, "th"), + **{ + name + ("h" if name.endswith("t") else "th"): (value, "th") + for name, value in self.ones.items() + if value > 3 and value != 5 and value != 12 + }, + } + self.ones_suffixed = {**self.ones_plural, **self.ones_ordinal} + + self.tens = { + "twenty": 20, + "thirty": 30, + "forty": 40, + "fifty": 50, + "sixty": 60, + "seventy": 70, + "eighty": 80, + "ninety": 90, + } + self.tens_plural = { + name.replace("y", "ies"): (value, "s") for name, value in self.tens.items() + } + self.tens_ordinal = { + name.replace("y", "ieth"): (value, "th") for name, value in self.tens.items() + } + self.tens_suffixed = {**self.tens_plural, **self.tens_ordinal} + + self.multipliers = { + "hundred": 100, + "thousand": 1_000, + "million": 1_000_000, + "billion": 1_000_000_000, + "trillion": 1_000_000_000_000, + "quadrillion": 1_000_000_000_000_000, + "quintillion": 1_000_000_000_000_000_000, + "sextillion": 1_000_000_000_000_000_000_000, + "septillion": 1_000_000_000_000_000_000_000_000, + "octillion": 1_000_000_000_000_000_000_000_000_000, + "nonillion": 1_000_000_000_000_000_000_000_000_000_000, + "decillion": 1_000_000_000_000_000_000_000_000_000_000_000, + } + self.multipliers_plural = { + name + "s": (value, "s") for name, value in self.multipliers.items() + } + self.multipliers_ordinal = { + name + "th": (value, "th") for name, value in self.multipliers.items() + } + self.multipliers_suffixed = {**self.multipliers_plural, **self.multipliers_ordinal} + self.decimals = {*self.ones, *self.tens, *self.zeros} + + self.preceding_prefixers = { + "minus": "-", + "negative": "-", + "plus": "+", + "positive": "+", + } + self.following_prefixers = { + "pound": "£", + "pounds": "£", + "euro": "€", + "euros": "€", + "dollar": "$", + "dollars": "$", + "cent": "¢", + "cents": "¢", + } + self.prefixes = set( + list(self.preceding_prefixers.values()) + list(self.following_prefixers.values()) + ) + self.suffixers = { + "per": {"cent": "%"}, + "percent": "%", + } + self.specials = {"and", "double", "triple", "point"} + + self.words = set( + [ + key + for mapping in [ + self.zeros, + self.ones, + self.ones_suffixed, + self.tens, + self.tens_suffixed, + self.multipliers, + self.multipliers_suffixed, + self.preceding_prefixers, + self.following_prefixers, + self.suffixers, + self.specials, + ] + for key in mapping + ] + ) + self.literal_words = {"one", "ones"} + + def process_words(self, words: List[str]) -> Iterator[str]: + prefix: Optional[str] = None + value: Optional[Union[str, int]] = None + skip = False + + def to_fraction(s: str): + try: + return Fraction(s) + except ValueError: + return None + + def output(result: Union[str, int]): + nonlocal prefix, value + result = str(result) + if prefix is not None: + result = prefix + result + value = None + prefix = None + return result + + if len(words) == 0: + return + + for prev, current, next in windowed([None] + words + [None], 3): + if skip: + skip = False + continue + + next_is_numeric = next is not None and re.match(r"^\d+(\.\d+)?$", next) + has_prefix = current[0] in self.prefixes + current_without_prefix = current[1:] if has_prefix else current + if re.match(r"^\d+(\.\d+)?$", current_without_prefix): + # arabic numbers (potentially with signs and fractions) + f = to_fraction(current_without_prefix) + assert f is not None + if value is not None: + if isinstance(value, str) and value.endswith("."): + # concatenate decimals / ip address components + value = str(value) + str(current) + continue + else: + yield output(value) + + prefix = current[0] if has_prefix else prefix + if f.denominator == 1: + value = f.numerator # store integers as int + else: + value = current_without_prefix + elif current not in self.words: + # non-numeric words + if value is not None: + yield output(value) + yield output(current) + elif current in self.zeros: + value = str(value or "") + "0" + elif current in self.ones: + ones = self.ones[current] + + if value is None: + value = ones + elif isinstance(value, str) or prev in self.ones: + if prev in self.tens and ones < 10: # replace the last zero with the digit + assert value[-1] == "0" + value = value[:-1] + str(ones) + else: + value = str(value) + str(ones) + elif ones < 10: + if value % 10 == 0: + value += ones + else: + value = str(value) + str(ones) + else: # eleven to nineteen + if value % 100 == 0: + value += ones + else: + value = str(value) + str(ones) + elif current in self.ones_suffixed: + # ordinal or cardinal; yield the number right away + ones, suffix = self.ones_suffixed[current] + if value is None: + yield output(str(ones) + suffix) + elif isinstance(value, str) or prev in self.ones: + if prev in self.tens and ones < 10: + assert value[-1] == "0" + yield output(value[:-1] + str(ones) + suffix) + else: + yield output(str(value) + str(ones) + suffix) + elif ones < 10: + if value % 10 == 0: + yield output(str(value + ones) + suffix) + else: + yield output(str(value) + str(ones) + suffix) + else: # eleven to nineteen + if value % 100 == 0: + yield output(str(value + ones) + suffix) + else: + yield output(str(value) + str(ones) + suffix) + value = None + elif current in self.tens: + tens = self.tens[current] + if value is None: + value = tens + elif isinstance(value, str): + value = str(value) + str(tens) + else: + if value % 100 == 0: + value += tens + else: + value = str(value) + str(tens) + elif current in self.tens_suffixed: + # ordinal or cardinal; yield the number right away + tens, suffix = self.tens_suffixed[current] + if value is None: + yield output(str(tens) + suffix) + elif isinstance(value, str): + yield output(str(value) + str(tens) + suffix) + else: + if value % 100 == 0: + yield output(str(value + tens) + suffix) + else: + yield output(str(value) + str(tens) + suffix) + elif current in self.multipliers: + multiplier = self.multipliers[current] + if value is None: + value = multiplier + elif isinstance(value, str) or value == 0: + f = to_fraction(value) + p = f * multiplier if f is not None else None + if f is not None and p.denominator == 1: + value = p.numerator + else: + yield output(value) + value = multiplier + else: + before = value // 1000 * 1000 + residual = value % 1000 + value = before + residual * multiplier + elif current in self.multipliers_suffixed: + multiplier, suffix = self.multipliers_suffixed[current] + if value is None: + yield output(str(multiplier) + suffix) + elif isinstance(value, str): + f = to_fraction(value) + p = f * multiplier if f is not None else None + if f is not None and p.denominator == 1: + yield output(str(p.numerator) + suffix) + else: + yield output(value) + yield output(str(multiplier) + suffix) + else: # int + before = value // 1000 * 1000 + residual = value % 1000 + value = before + residual * multiplier + yield output(str(value) + suffix) + value = None + elif current in self.preceding_prefixers: + # apply prefix (positive, minus, etc.) if it precedes a number + if value is not None: + yield output(value) + + if next in self.words or next_is_numeric: + prefix = self.preceding_prefixers[current] + else: + yield output(current) + elif current in self.following_prefixers: + # apply prefix (dollars, cents, etc.) only after a number + if value is not None: + prefix = self.following_prefixers[current] + yield output(value) + else: + yield output(current) + elif current in self.suffixers: + # apply suffix symbols (percent -> '%') + if value is not None: + suffix = self.suffixers[current] + if isinstance(suffix, dict): + if next in suffix: + yield output(str(value) + suffix[next]) + skip = True + else: + yield output(value) + yield output(current) + else: + yield output(str(value) + suffix) + else: + yield output(current) + elif current in self.specials: + if next not in self.words and not next_is_numeric: + # apply special handling only if the next word can be numeric + if value is not None: + yield output(value) + yield output(current) + elif current == "and": + # ignore "and" after hundreds, thousands, etc. + if prev not in self.multipliers: + if value is not None: + yield output(value) + yield output(current) + elif current == "double" or current == "triple": + if next in self.ones or next in self.zeros: + repeats = 2 if current == "double" else 3 + ones = self.ones.get(next, 0) + value = str(value or "") + str(ones) * repeats + skip = True + else: + if value is not None: + yield output(value) + yield output(current) + elif current == "point": + if next in self.decimals or next_is_numeric: + value = str(value or "") + "." + else: + # should all have been covered at this point + raise ValueError(f"Unexpected token: {current}") + else: + # all should have been covered at this point + raise ValueError(f"Unexpected token: {current}") + + if value is not None: + yield output(value) + + def preprocess(self, s: str): + # replace "<number> and a half" with "<number> point five" + results = [] + + segments = re.split(r"\band\s+a\s+half\b", s) + for i, segment in enumerate(segments): + if len(segment.strip()) == 0: + continue + if i == len(segments) - 1: + results.append(segment) + else: + results.append(segment) + last_word = segment.rsplit(maxsplit=2)[-1] + if last_word in self.decimals or last_word in self.multipliers: + results.append("point five") + else: + results.append("and a half") + + s = " ".join(results) + + # put a space at number/letter boundary + s = re.sub(r"([a-z])([0-9])", r"\1 \2", s) + s = re.sub(r"([0-9])([a-z])", r"\1 \2", s) + + # but remove spaces which could be a suffix + s = re.sub(r"([0-9])\s+(st|nd|rd|th|s)\b", r"\1\2", s) + + return s + + def postprocess(self, s: str): + def combine_cents(m: Match): + try: + currency = m.group(1) + integer = m.group(2) + cents = int(m.group(3)) + return f"{currency}{integer}.{cents:02d}" + except ValueError: + return m.string + + def extract_cents(m: Match): + try: + return f"¢{int(m.group(1))}" + except ValueError: + return m.string + + # apply currency postprocessing; "$2 and ¢7" -> "$2.07" + s = re.sub(r"([€£$])([0-9]+) (?:and )?¢([0-9]{1,2})\b", combine_cents, s) + s = re.sub(r"[€£$]0.([0-9]{1,2})\b", extract_cents, s) + + # write "one(s)" instead of "1(s)", just for the readability + s = re.sub(r"\b1(s?)\b", r"one\1", s) + + return s + + def __call__(self, s: str): + s = self.preprocess(s) + s = " ".join(word for word in self.process_words(s.split()) if word is not None) + s = self.postprocess(s) + + return s + + +class EnglishSpellingNormalizer: + """ + Applies British-American spelling mappings as listed in [1]. + + [1] https://www.tysto.com/uk-us-spelling-list.html + """ + + def __init__(self): + mapping_path = os.path.join(os.path.dirname(__file__), "english.json") + self.mapping = json.load(open(mapping_path)) + + def __call__(self, s: str): + return " ".join(self.mapping.get(word, word) for word in s.split()) + + +class EnglishTextNormalizer: + def __init__(self): + self.ignore_patterns = r"\b(hmm|mm|mhm|mmm|uh|um)\b" + self.replacers = { + # common contractions + r"\bwon't\b": "will not", + r"\bcan't\b": "can not", + r"\blet's\b": "let us", + r"\bain't\b": "aint", + r"\by'all\b": "you all", + r"\bwanna\b": "want to", + r"\bgotta\b": "got to", + r"\bgonna\b": "going to", + r"\bi'ma\b": "i am going to", + r"\bimma\b": "i am going to", + r"\bwoulda\b": "would have", + r"\bcoulda\b": "could have", + r"\bshoulda\b": "should have", + r"\bma'am\b": "madam", + # contractions in titles/prefixes + r"\bmr\b": "mister ", + r"\bmrs\b": "missus ", + r"\bst\b": "saint ", + r"\bdr\b": "doctor ", + r"\bprof\b": "professor ", + r"\bcapt\b": "captain ", + r"\bgov\b": "governor ", + r"\bald\b": "alderman ", + r"\bgen\b": "general ", + r"\bsen\b": "senator ", + r"\brep\b": "representative ", + r"\bpres\b": "president ", + r"\brev\b": "reverend ", + r"\bhon\b": "honorable ", + r"\basst\b": "assistant ", + r"\bassoc\b": "associate ", + r"\blt\b": "lieutenant ", + r"\bcol\b": "colonel ", + r"\bjr\b": "junior ", + r"\bsr\b": "senior ", + r"\besq\b": "esquire ", + # prefect tenses, ideally it should be any past participles, but it's harder.. + r"'d been\b": " had been", + r"'s been\b": " has been", + r"'d gone\b": " had gone", + r"'s gone\b": " has gone", + r"'d done\b": " had done", # "'s done" is ambiguous + r"'s got\b": " has got", + # general contractions + r"n't\b": " not", + r"'re\b": " are", + r"'s\b": " is", + r"'d\b": " would", + r"'ll\b": " will", + r"'t\b": " not", + r"'ve\b": " have", + r"'m\b": " am", + } + self.standardize_numbers = EnglishNumberNormalizer() + self.standardize_spellings = EnglishSpellingNormalizer() + + def __call__(self, s: str): + s = s.lower() + + s = re.sub(r"[<\[][^>\]]*[>\]]", "", s) # remove words between brackets + s = re.sub(r"\(([^)]+?)\)", "", s) # remove words between parenthesis + s = re.sub(self.ignore_patterns, "", s) + s = re.sub(r"\s+'", "'", s) # standardize when there's a space before an apostrophe + + for pattern, replacement in self.replacers.items(): + s = re.sub(pattern, replacement, s) + + s = re.sub(r"(\d),(\d)", r"\1\2", s) # remove commas between digits + s = re.sub(r"\.([^0-9]|$)", r" \1", s) # remove periods not followed by numbers + s = remove_symbols_and_diacritics(s, keep=".%$¢€£") # keep some symbols for numerics + + s = self.standardize_numbers(s) + s = self.standardize_spellings(s) + + # now remove prefix/suffix symbols that are not preceded/followed by numbers + s = re.sub(r"[.$¢€£]([^0-9])", r" \1", s) + s = re.sub(r"([^0-9])%", r"\1 ", s) + + s = re.sub(r"\s+", " ", s) # replace any successive whitespace characters with a space + + return s diff --git a/whisper/tokenizer.py b/whisper/tokenizer.py new file mode 100644 index 0000000000000000000000000000000000000000..a27cb359ee891590d3f793624f9f8ec768a26cc3 --- /dev/null +++ b/whisper/tokenizer.py @@ -0,0 +1,331 @@ +import os +from dataclasses import dataclass +from functools import lru_cache +from typing import List, Optional, Tuple, Union + +import numpy as np +import torch +from transformers import GPT2TokenizerFast + +LANGUAGES = { + "en": "english", + "zh": "chinese", + "de": "german", + "es": "spanish", + "ru": "russian", + "ko": "korean", + "fr": "french", + "ja": "japanese", + "pt": "portuguese", + "tr": "turkish", + "pl": "polish", + "ca": "catalan", + "nl": "dutch", + "ar": "arabic", + "sv": "swedish", + "it": "italian", + "id": "indonesian", + "hi": "hindi", + "fi": "finnish", + "vi": "vietnamese", + "he": "hebrew", + "uk": "ukrainian", + "el": "greek", + "ms": "malay", + "cs": "czech", + "ro": "romanian", + "da": "danish", + "hu": "hungarian", + "ta": "tamil", + "no": "norwegian", + "th": "thai", + "ur": "urdu", + "hr": "croatian", + "bg": "bulgarian", + "lt": "lithuanian", + "la": "latin", + "mi": "maori", + "ml": "malayalam", + "cy": "welsh", + "sk": "slovak", + "te": "telugu", + "fa": "persian", + "lv": "latvian", + "bn": "bengali", + "sr": "serbian", + "az": "azerbaijani", + "sl": "slovenian", + "kn": "kannada", + "et": "estonian", + "mk": "macedonian", + "br": "breton", + "eu": "basque", + "is": "icelandic", + "hy": "armenian", + "ne": "nepali", + "mn": "mongolian", + "bs": "bosnian", + "kk": "kazakh", + "sq": "albanian", + "sw": "swahili", + "gl": "galician", + "mr": "marathi", + "pa": "punjabi", + "si": "sinhala", + "km": "khmer", + "sn": "shona", + "yo": "yoruba", + "so": "somali", + "af": "afrikaans", + "oc": "occitan", + "ka": "georgian", + "be": "belarusian", + "tg": "tajik", + "sd": "sindhi", + "gu": "gujarati", + "am": "amharic", + "yi": "yiddish", + "lo": "lao", + "uz": "uzbek", + "fo": "faroese", + "ht": "haitian creole", + "ps": "pashto", + "tk": "turkmen", + "nn": "nynorsk", + "mt": "maltese", + "sa": "sanskrit", + "lb": "luxembourgish", + "my": "myanmar", + "bo": "tibetan", + "tl": "tagalog", + "mg": "malagasy", + "as": "assamese", + "tt": "tatar", + "haw": "hawaiian", + "ln": "lingala", + "ha": "hausa", + "ba": "bashkir", + "jw": "javanese", + "su": "sundanese", +} + +# language code lookup by name, with a few language aliases +TO_LANGUAGE_CODE = { + **{language: code for code, language in LANGUAGES.items()}, + "burmese": "my", + "valencian": "ca", + "flemish": "nl", + "haitian": "ht", + "letzeburgesch": "lb", + "pushto": "ps", + "panjabi": "pa", + "moldavian": "ro", + "moldovan": "ro", + "sinhalese": "si", + "castilian": "es", +} + + +@dataclass(frozen=True) +class Tokenizer: + """A thin wrapper around `GPT2TokenizerFast` providing quick access to special tokens""" + + tokenizer: "GPT2TokenizerFast" + language: Optional[str] + sot_sequence: Tuple[int] + + def encode(self, text, **kwargs): + return self.tokenizer.encode(text, **kwargs) + + def decode(self, token_ids: Union[int, List[int], np.ndarray, torch.Tensor], **kwargs): + return self.tokenizer.decode(token_ids, **kwargs) + + def decode_with_timestamps(self, tokens) -> str: + """ + Timestamp tokens are above the special tokens' id range and are ignored by `decode()`. + This method decodes given tokens with timestamps tokens annotated, e.g. "<|1.08|>". + """ + outputs = [[]] + for token in tokens: + if token >= self.timestamp_begin: + timestamp = f"<|{(token - self.timestamp_begin) * 0.02:.2f}|>" + outputs.append(timestamp) + outputs.append([]) + else: + outputs[-1].append(token) + outputs = [s if isinstance(s, str) else self.tokenizer.decode(s) for s in outputs] + return "".join(outputs) + + @property + @lru_cache() + def eot(self) -> int: + return self.tokenizer.eos_token_id + + @property + @lru_cache() + def sot(self) -> int: + return self._get_single_token_id("<|startoftranscript|>") + + @property + @lru_cache() + def sot_lm(self) -> int: + return self._get_single_token_id("<|startoflm|>") + + @property + @lru_cache() + def sot_prev(self) -> int: + return self._get_single_token_id("<|startofprev|>") + + @property + @lru_cache() + def no_speech(self) -> int: + return self._get_single_token_id("<|nospeech|>") + + @property + @lru_cache() + def no_timestamps(self) -> int: + return self._get_single_token_id("<|notimestamps|>") + + @property + @lru_cache() + def timestamp_begin(self) -> int: + return self.tokenizer.all_special_ids[-1] + 1 + + @property + @lru_cache() + def language_token(self) -> int: + """Returns the token id corresponding to the value of the `language` field""" + if self.language is None: + raise ValueError(f"This tokenizer does not have language token configured") + + additional_tokens = dict( + zip( + self.tokenizer.additional_special_tokens, + self.tokenizer.additional_special_tokens_ids, + ) + ) + candidate = f"<|{self.language}|>" + if candidate in additional_tokens: + return additional_tokens[candidate] + + raise KeyError(f"Language {self.language} not found in tokenizer.") + + @property + @lru_cache() + def all_language_tokens(self) -> Tuple[int]: + result = [] + for token, token_id in zip( + self.tokenizer.additional_special_tokens, + self.tokenizer.additional_special_tokens_ids, + ): + if token.strip("<|>") in LANGUAGES: + result.append(token_id) + return tuple(result) + + @property + @lru_cache() + def all_language_codes(self) -> Tuple[str]: + return tuple(self.decode([l]).strip("<|>") for l in self.all_language_tokens) + + @property + @lru_cache() + def sot_sequence_including_notimestamps(self) -> Tuple[int]: + return tuple(list(self.sot_sequence) + [self.no_timestamps]) + + @property + @lru_cache() + def non_speech_tokens(self) -> Tuple[int]: + """ + Returns the list of tokens to suppress in order to avoid any speaker tags or non-speech + annotations, to prevent sampling texts that are not actually spoken in the audio, e.g. + + - ♪♪♪ + - ( SPEAKING FOREIGN LANGUAGE ) + - [DAVID] Hey there, + + keeping basic punctuations like commas, periods, question marks, exclamation points, etc. + """ + symbols = list("\"#()*+/:;<=>@[\\]^_`{|}~「」『』") + symbols += "<< >> <<< >>> -- --- -( -[ (' (\" (( )) ((( ))) [[ ]] {{ }} ♪♪ ♪♪♪".split() + + # symbols that may be a single token or multiple tokens depending on the tokenizer. + # In case they're multiple tokens, suppress the first token, which is safe because: + # These are between U+2640 and U+267F miscellaneous symbols that are okay to suppress + # in generations, and in the 3-byte UTF-8 representation they share the first two bytes. + miscellaneous = set("♩♪♫♬♭♮♯") + assert all(0x2640 <= ord(c) <= 0x267F for c in miscellaneous) + + # allow hyphens "-" and single quotes "'" between words, but not at the beginning of a word + result = {self.tokenizer.encode(" -")[0], self.tokenizer.encode(" '")[0]} + for symbol in symbols + list(miscellaneous): + for tokens in [self.tokenizer.encode(symbol), self.tokenizer.encode(" " + symbol)]: + if len(tokens) == 1 or symbol in miscellaneous: + result.add(tokens[0]) + + return tuple(sorted(result)) + + def _get_single_token_id(self, text) -> int: + tokens = self.tokenizer.encode(text) + assert len(tokens) == 1, f"{text} is not encoded as a single token" + return tokens[0] + + +@lru_cache(maxsize=None) +def build_tokenizer(name: str = "gpt2"): + os.environ["TOKENIZERS_PARALLELISM"] = "false" + path = os.path.join(os.path.dirname(__file__), "assets", name) + tokenizer = GPT2TokenizerFast.from_pretrained(path) + + specials = [ + "<|startoftranscript|>", + *[f"<|{lang}|>" for lang in LANGUAGES.keys()], + "<|translate|>", + "<|transcribe|>", + "<|startoflm|>", + "<|startofprev|>", + "<|nospeech|>", + "<|notimestamps|>", + ] + + tokenizer.add_special_tokens(dict(additional_special_tokens=specials)) + return tokenizer + + +@lru_cache(maxsize=None) +def get_tokenizer( + multilingual: bool, + *, + task: Optional[str] = None, # Literal["transcribe", "translate", None] + language: Optional[str] = None, +) -> Tokenizer: + if language is not None: + language = language.lower() + if language not in LANGUAGES: + if language in TO_LANGUAGE_CODE: + language = TO_LANGUAGE_CODE[language] + else: + raise ValueError(f"Unsupported language: {language}") + + if multilingual: + tokenizer_name = "multilingual" + task = task or "transcribe" + language = language or "en" + else: + tokenizer_name = "gpt2" + task = None + language = None + + tokenizer = build_tokenizer(name=tokenizer_name) + all_special_ids: List[int] = tokenizer.all_special_ids + sot: int = all_special_ids[1] + translate: int = all_special_ids[-6] + transcribe: int = all_special_ids[-5] + + langs = tuple(LANGUAGES.keys()) + sot_sequence = [sot] + if language is not None: + sot_sequence.append(sot + 1 + langs.index(language)) + if task is not None: + sot_sequence.append(transcribe if task == "transcribe" else translate) + + return Tokenizer(tokenizer=tokenizer, language=language, sot_sequence=tuple(sot_sequence)) diff --git a/whisper/utils.py b/whisper/utils.py new file mode 100644 index 0000000000000000000000000000000000000000..5dacc173c40bcd6e999d728862e29a968000b12e --- /dev/null +++ b/whisper/utils.py @@ -0,0 +1,163 @@ +import json +import os +import sys +import zlib +from typing import Callable, TextIO + +system_encoding = sys.getdefaultencoding() + +if system_encoding != "utf-8": + def make_safe(string): + # replaces any character not representable using the system default encoding with an '?', + # avoiding UnicodeEncodeError (https://github.com/openai/whisper/discussions/729). + return string.encode(system_encoding, errors="replace").decode(system_encoding) +else: + def make_safe(string): + # utf-8 can encode any Unicode code point, so no need to do the round-trip encoding + return string + + +def exact_div(x, y): + assert x % y == 0 + return x // y + + +def str2bool(string): + str2val = {"True": True, "False": False} + if string in str2val: + return str2val[string] + else: + raise ValueError(f"Expected one of {set(str2val.keys())}, got {string}") + + +def optional_int(string): + return None if string == "None" else int(string) + + +def optional_float(string): + return None if string == "None" else float(string) + + +def compression_ratio(text) -> float: + text_bytes = text.encode("utf-8") + return len(text_bytes) / len(zlib.compress(text_bytes)) + + +def format_timestamp(seconds: float, always_include_hours: bool = False, decimal_marker: str = '.'): + assert seconds >= 0, "non-negative timestamp expected" + milliseconds = round(seconds * 1000.0) + + hours = milliseconds // 3_600_000 + milliseconds -= hours * 3_600_000 + + minutes = milliseconds // 60_000 + milliseconds -= minutes * 60_000 + + seconds = milliseconds // 1_000 + milliseconds -= seconds * 1_000 + + hours_marker = f"{hours:02d}:" if always_include_hours or hours > 0 else "" + return f"{hours_marker}{minutes:02d}:{seconds:02d}{decimal_marker}{milliseconds:03d}" + + +class ResultWriter: + extension: str + + def __init__(self, output_dir: str): + self.output_dir = output_dir + + def __call__(self, result: dict, audio_path: str): + audio_basename = os.path.basename(audio_path) + output_path = os.path.join(self.output_dir, audio_basename + "." + self.extension) + + with open(output_path, "w", encoding="utf-8") as f: + self.write_result(result, file=f) + + def write_result(self, result: dict, file: TextIO): + raise NotImplementedError + + +class WriteTXT(ResultWriter): + extension: str = "txt" + + def write_result(self, result: dict, file: TextIO): + for segment in result["segments"]: + print(segment['text'].strip(), file=file, flush=True) + + +class WriteVTT(ResultWriter): + extension: str = "vtt" + + def write_result(self, result: dict, file: TextIO): + print("WEBVTT\n", file=file) + for segment in result["segments"]: + print( + f"{format_timestamp(segment['start'])} --> {format_timestamp(segment['end'])}\n" + f"{segment['text'].strip().replace('-->', '->')}\n", + file=file, + flush=True, + ) + + +class WriteSRT(ResultWriter): + extension: str = "srt" + + def write_result(self, result: dict, file: TextIO): + for i, segment in enumerate(result["segments"], start=1): + # write srt lines + print( + f"{i}\n" + f"{format_timestamp(segment['start'], always_include_hours=True, decimal_marker=',')} --> " + f"{format_timestamp(segment['end'], always_include_hours=True, decimal_marker=',')}\n" + f"{segment['text'].strip().replace('-->', '->')}\n", + file=file, + flush=True, + ) + + +class WriteTSV(ResultWriter): + """ + Write a transcript to a file in TSV (tab-separated values) format containing lines like: + <start time in integer milliseconds>\t<end time in integer milliseconds>\t<transcript text> + + Using integer milliseconds as start and end times means there's no chance of interference from + an environment setting a language encoding that causes the decimal in a floating point number + to appear as a comma; also is faster and more efficient to parse & store, e.g., in C++. + """ + extension: str = "tsv" + + def write_result(self, result: dict, file: TextIO): + print("start", "end", "text", sep="\t", file=file) + for segment in result["segments"]: + print(round(1000 * segment['start']), file=file, end="\t") + print(round(1000 * segment['end']), file=file, end="\t") + print(segment['text'].strip().replace("\t", " "), file=file, flush=True) + + +class WriteJSON(ResultWriter): + extension: str = "json" + + def write_result(self, result: dict, file: TextIO): + json.dump(result, file) + + +def get_writer(output_format: str, output_dir: str) -> Callable[[dict, TextIO], None]: + writers = { + "txt": WriteTXT, + "vtt": WriteVTT, + "srt": WriteSRT, + "tsv": WriteTSV, + "json": WriteJSON, + } + + if output_format == "all": + all_writers = [writer(output_dir) for writer in writers.values()] + + def write_all(result: dict, file: TextIO): + for writer in all_writers: + writer(result, file) + + return write_all + + return writers[output_format](output_dir) + diff --git a/whisper_pretrain/README.md b/whisper_pretrain/README.md new file mode 100644 index 0000000000000000000000000000000000000000..1093e1dcbb792f7a86a0d3a3a1bf14691ab998b5 --- /dev/null +++ b/whisper_pretrain/README.md @@ -0,0 +1,3 @@ +Path for: + + medium.pt \ No newline at end of file diff --git a/whisper_pretrain/medium.pt b/whisper_pretrain/medium.pt new file mode 100644 index 0000000000000000000000000000000000000000..8aca41c710014a3d39774cd7592fa086177c672f --- /dev/null +++ b/whisper_pretrain/medium.pt @@ -0,0 +1,3 @@ +version https://git-lfs.github.com/spec/v1 +oid sha256:345ae4da62f9b3d59415adc60127b97c714f32e89e936602e85993674d08dcb1 +size 1528008539