Spaces:
Running
Running
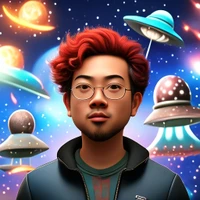
Refactor imports and update configuration for LLM response processing; add post-processing functionality and enhance README documentation.
25fca04
import unittest | |
from post_process import process_response | |
class TestProcessResponse(unittest.TestCase): | |
def test_valid_response(self): | |
resp = { | |
"english": "I scored a slam dunk yesterday at the West Park with my classmates, succeeding in a dunk.", | |
"important_words": [ | |
{ | |
"word_en": "dunk", | |
"meaning_ch": "扣篮", | |
"usage": "He made a spectacular dunk." | |
}, | |
{ | |
"word_en": "slam", | |
"meaning_ch": "猛烈撞击", | |
"usage": "The door slammed shut." | |
}, | |
{ | |
"word_en": "scored", | |
"meaning_ch": "得分", | |
"usage": "She scored the winning goal." | |
} | |
] | |
} | |
english, words = process_response(resp) | |
self.assertEqual( | |
english, "I scored a slam dunk yesterday at the West Park with my classmates, succeeding in a dunk.") | |
self.assertEqual(len(words), 3) | |
self.assertEqual(words[0]["word_en"], "dunk") | |
def test_invalid_response_missing_keys(self): | |
resp = { | |
"english": "I scored a slam dunk yesterday at the West Park with my classmates, succeeding in a dunk." | |
} | |
english, words = process_response(resp) | |
self.assertIsNone(english) | |
self.assertIsNone(words) | |
def test_invalid_response_empty(self): | |
resp = {} | |
english, words = process_response(resp) | |
self.assertIsNone(english) | |
self.assertIsNone(words) | |
def test_none_response(self): | |
english, words = process_response(None) | |
self.assertIsNone(english) | |
self.assertIsNone(words) | |
if __name__ == "__main__": | |
unittest.main() | |